Python Else Do Nothing
Overview of the “else” Statement in Python
In Python, the “else” statement is a powerful construct that allows you to define a block of code to be executed when a specific condition is not met. It offers enhanced control flow and allows you to handle different scenarios based on the outcome of a condition.
Understanding the Syntax of the “if-else” Structure in Python
The “if-else” structure in Python follows a specific syntax:
“`python
if condition:
# code to execute if the condition is true
else:
# code to execute if the condition is false
“`
The “else” keyword is followed by a colon, and the code block to execute when the condition is false is indented under it. This structure allows you to perform different actions depending on whether a condition is true or false.
Exploring the “else” Statement in Python Conditional Statements
In Python, the “else” statement is often used in conjunction with the “if” statement to create conditional statements. When the condition specified in the “if” statement evaluates to true, the code block under it is executed. However, if the condition evaluates to false, the code block under the “else” statement is executed instead.
An example of a conditional statement using the “else” statement in Python:
“`python
age = 25
if age >= 18:
print(“You are an adult.”)
else:
print(“You are not an adult.”)
“`
In this example, if the variable “age” is greater than or equal to 18, the program will print “You are an adult.” Otherwise, it will print “You are not an adult.”
Using the “else” Statement in Python Loops for Enhanced Control Flow
The “else” statement can also be used in loops, such as “for” and “while” loops, to provide enhanced control flow. When the loop finishes executing all its iterations without encountering a “break” statement, the code block under the “else” statement is executed.
An example of using the “else” statement with a “for” loop in Python:
“`python
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 0:
print(“Zero found!”)
break
else:
print(“Zero not found!”)
“`
In this example, the loop iterates over a list of numbers. If it encounters a zero, it prints “Zero found!” and breaks out of the loop. If the loop completes all its iterations without encountering a zero, it will print “Zero not found!”.
Distinguishing the “else” Statement from Other Control Flow Keywords in Python
It is essential to distinguish the “else” statement from other control flow keywords in Python, such as “elif” and “finally”. The “elif” keyword is used to specify additional conditions in conditional statements, while the “finally” keyword is used in conjunction with exception handling. The “else” statement, on the other hand, is used to define a block of code that executes when a condition is false.
Handling Multiple “else” Statements in Nested Conditional Statements
Python allows you to nest conditional statements and include multiple “else” statements within them. This can be useful when dealing with complex scenarios that require multiple conditions to be checked.
An example of nested conditional statements with multiple “else” statements:
“`python
number = 10
if number > 10:
print(“Number is greater than 10.”)
elif number < 10:
print("Number is less than 10.")
else:
if number == 10:
print("Number is equal to 10.")
else:
print("Number is not an integer.")
```
In this example, the code checks if the number is greater than 10, less than 10, or equal to 10. If none of these conditions are met, it will print "Number is not an integer."
Common Pitfalls and Best Practices When Using the "else" Statement in Python
When using the "else" statement in Python, it's essential to be aware of common pitfalls and follow best practices to ensure clean and reliable code. Some tips to keep in mind:
1. Indentation: Ensure proper indentation when using the "else" statement. Incorrect indentation can lead to syntax errors.
2. Clear Logic: Make sure the logic in your conditional statements is clear and easy to understand. Avoid overly complex nested conditions that make it harder to follow the code flow.
3. Testing: Test your code thoroughly to cover all possible conditions and ensure the "else" statement triggers as expected.
Real-World Examples of the "else" Statement in Python
The "else" statement has various applications in real-world scenarios. Here are a few examples:
1. User Input Validation: If a user enters an incorrect value, the "else" statement can be used to prompt them to try again.
2. File Handling: When reading data from a file, the "else" statement can handle cases where the file is empty or cannot be opened.
3. Network Communication: When sending requests to a server, the "else" statement can handle cases where the connection fails or times out.
Further Learning Resources to Master the "else" Statement in Python
To further master the usage of the "else" statement in Python, consider exploring the following resources:
1. Official Python Documentation: The official Python documentation provides a comprehensive guide to the "if-else" structure and the "else" statement.
2. Online Tutorials: Numerous online tutorials, such as tutorials on Python programming websites and YouTube channels, offer step-by-step guidance on using the "else" statement effectively.
3. Practice Exercises: Solve practice exercises that involve implementing the "else" statement in various scenarios. This will reinforce your understanding and help you improve your coding skills.
FAQs
Q: Can I use the "else" statement without an "if" statement?
A: No, the "else" statement in Python always accompanies an "if" statement. It provides an alternative code path when the condition specified in the "if" statement is false.
Q: Can I use the "else" statement without the "if" statement having any code?
A: Yes, you can have an empty "if" statement followed by an "else" statement that contains code. This can be useful in certain situations.
Q: Can I use the "else" statement with a one-line "if" statement?
A: Yes, you can condense an "if-else" statement into a single line using the ternary operator. For example: `x = 5 if condition else 10`.
Q: What happens if I don't include an "else" statement?
A: If an "else" statement is not provided, the code block under it is not executed when the condition specified in the "if" statement is false.
Q: Can I use multiple "else" statements in nested conditional statements?
A: Yes, Python allows you to nest conditional statements and include multiple "else" statements within them to handle complex scenarios.
Q: What are some best practices when using the "else" statement?
A: Ensure proper indentation, keep the logic clear, and thoroughly test your code to cover all possible conditions. Following these best practices will result in clean and reliable code using the "else" statement effectively.
In conclusion, the "else" statement in Python offers enhanced control flow and allows you to handle different scenarios based on the outcome of a condition. It can be used in both conditional statements and loops to provide alternative code paths. By understanding its syntax and best practices, you can leverage the power of the "else" statement to write clear and efficient Python code.
If Else Statements In Python // Python Right Now!! // Ep 4
Keywords searched by users: python else do nothing Python if-else one line, If without else, If else Python, If in one line python without else, Empty lambda python, Pass in function python, If else Python 3, Python with statement
Categories: Top 23 Python Else Do Nothing
See more here: nhanvietluanvan.com
Python If-Else One Line
Python, being a highly flexible and expressive programming language, offers developers a variety of ways to write code that is both concise and powerful. One such feature is the ability to write if-else statements in a single line, known as Python If-Else One-Liners.
Typically, if-else statements in Python are written using multiple lines of code, with each block enclosed within the if and else keywords. However, for simple conditions, Python provides an elegant and compact alternative. Using the ternary operator, developers can express if-else logic in a single line, reducing the need for additional lines of code.
The syntax for a Python If-Else One-Liner is as follows:
Let’s take a closer look at how this syntax works:
–
–
–
For example, consider the following if-else statement:
“`python
x = 5
if x > 10:
message = “x is greater than 10”
else:
message = “x is less than or equal to 10”
“`
Using the Python If-Else One-Liner syntax, the above code can be simplified to:
“`python
x = 5
message = “x is greater than 10” if x > 10 else “x is less than or equal to 10”
“`
By condensing the if-else statement into a single line, developers can achieve greater readability and reduce unnecessary lines of code.
Benefits and Use Cases:
Python If-Else One-Liners offer several advantages, making them a popular choice among developers:
1. Improved Readability: The concise nature of If-Else One-Liners enables developers to quickly understand and process the code, especially for simple conditions.
2. Reduced Code Size: By eliminating unnecessary lines and encapsulating the logic in a single line, the codebase becomes more compact and manageable.
3. Simplified Variable Assignments: In scenarios where the output of an if-else condition is assigned to a variable, one-liners reduce the need for multiple assignment statements.
4. Expressive Code: Python’s syntax emphasizes the readability of code, making one-liners a valuable tool for writing expressive and elegant solutions.
Despite these advantages, it is important to use one-liners judiciously. In complex scenarios or situations where multiple conditions need to be evaluated, using multiple lines may enhance code clarity.
Frequently Asked Questions:
Q: Can I nest if-else statements within a one-liner?
A: Yes, if-else statements can be nested within a one-liner. However, be cautious as excessively nested one-liners may reduce code readability.
Q: Are one-liners recommended for complex conditions?
A: No, for complex conditions with multiple checks, using multiple lines is preferred to maintain code readability and organization.
Q: Can I use If-Else One-Liners without an “else” statement?
A: Yes, If-Else One-Liners can omit the “else” statement and solely focus on conditions where True evaluates to a specific expression.
Q: Can I use one-liners for non-trivial expressions?
A: Yes, one-liners can handle non-trivial expressions. However, it is important to strike a balance between concise code and maintainable code.
Q: Are If-Else One-Liners a Python-specific feature?
A: No, the concept of one-liners can be found in multiple programming languages, but Python’s syntax makes them particularly easy and expressive.
In conclusion, Python If-Else One-Liners provide developers with a concise and expressive way to handle conditions. While they offer advantages such as improved readability and reduced code size, they should be used judiciously for simple conditions. By understanding their syntax and limitations, developers can leverage one-liners to write elegant and efficient code in Python.
If Without Else
Conditional statements are a fundamental component of programming languages, allowing developers to control the flow of their code based on certain conditions. In this article, we will delve into the intricacies and applications of a conditional statement known as “if without else.”
The “if without else” statement, as the name suggests, is an if statement that lacks an accompanying else clause. It is used when a programmer wants to execute a particular set of instructions only if a specific condition is satisfied, without providing an alternative set of instructions for when the condition is not met.
Let’s take a look at the basic syntax of an if statement without an else clause:
if (condition) {
// Code to be executed if the condition is true
}
When the condition within the parentheses evaluates to true, the block of code enclosed within the curly braces will be executed. If the condition evaluates to false, the block of code will be skipped entirely, and the program flow will move on to the next statement.
Essentially, “if without else” statements provide a way to perform actions conditionally, but without defining any actions in the case where the condition is false. This can be useful in certain scenarios, allowing developers to simplify their code and improve readability.
Applications and Use Cases
1. Validation: If a programmer wants to verify whether a certain input meets specific criteria, they can employ an “if without else” statement. For example, when implementing a registration process, an if statement without an else clause can be used to validate the user’s email address. If the email address satisfies certain conditions, such as having a valid format, the registration process can proceed. In this case, no alternative actions need to be taken if the email address is invalid.
2. Error Handling: When dealing with error conditions, an “if without else” statement can be used to execute a specific error-handling routine. If an error occurs, the code within the if block can display an error message or perform any necessary cleanup without the need for an accompanying else block. This approach simplifies error handling, especially in cases where alternative actions are not required.
3. Decision-Making: In some scenarios, developers may want to perform checks and make decisions based on a condition but without specifying any actions for the negative outcome. For instance, if an application requires a user to enter a password to access certain features, an “if without else” statement can be used to determine whether the password provided matches the expected value. If the condition is true, access can be granted, while no further actions are necessary if the condition is false.
Advantages of “If Without Else”
– Simplified Code: The absence of an else clause removes the need to define actions that should be taken when the condition is not met, resulting in cleaner, less cluttered code.
– Improved Readability: By eliminating unnecessary else clauses, developers can enhance code readability and make their intentions more apparent to others who might review or maintain the code.
– Enhanced Maintainability: With a reduced number of conditional blocks, debugging and modifying the code becomes more straightforward, as there are fewer paths to consider.
– Performance Optimization: Since the lack of an else clause means that the code inside the if block will only execute when the condition evaluates to true, it can reduce unnecessary processing and potentially improve performance in certain scenarios.
FAQs
Q1. Can’t we achieve the same result with an if-else statement?
While it is true that an if-else statement can achieve the same outcome, “if without else” provides a way to streamline the code and improve readability. In situations where the else clause is not required, simply skipping it can make the code more concise and easier to understand.
Q2. Are there any downsides to using “if without else”?
The primary downside to using “if without else” is that it provides no explicit alternative actions when the condition evaluates to false. This lack of clarity can make the code harder to understand for someone who is not familiar with the implementation.
Q3. Can an “if without else” statement be nested within another conditional statement?
Yes, an “if without else” statement can be nested within another conditional statement, just like any other type of if statement. This allows for greater flexibility and the ability to create more complex conditionals.
In conclusion, the “if without else” statement is a powerful tool in a developer’s arsenal, allowing for simpler, more readable code in certain scenarios where alternative actions are not required. By using this conditional construct judiciously, developers can enhance their code’s maintainability, readability, and potentially improve performance when executing specific conditions.
If Else Python
### An Introduction to If-else Statements in Python
Conditional statements play a crucial role in programming as they enable decision-making based on specific conditions. The if-else statement is a fundamental construct in Python, allowing developers to choose between two alternative code blocks depending on the truthiness of a condition.
The basic syntax of an if-else statement in Python is as follows:
“`python
if condition:
# code block executed if condition is True
else:
# code block executed if condition is False
“`
The condition within the if statement is typically expressed as a logical expression or comparison operator, such as `x > y` or `a == b`. If the condition evaluates to `True`, the code block within the first indented block is executed. Otherwise, the code block within the else statement is executed.
### Multiple Conditions with elif
In addition to the if and else clauses, Python provides the elif (short for “else if”) clause, which allows for multiple conditions to be evaluated. This is particularly useful when dealing with more than two possible outcomes. The syntax for using elif is as follows:
“`python
if condition1:
# code block executed if condition1 is True
elif condition2:
# code block executed if condition2 is True
else:
# code block executed if all conditions are False
“`
The elif statement is placed after the initial if statement and before the else statement. It allows the program to check a new condition when the previous ones fail. If the condition is met, the code within the corresponding block is executed, bypassing the remaining conditions.
### Nested If-else Statements
Python also supports nested if-else statements, which are if-else blocks placed within other if-else blocks. This allows developers to create more complex decision-making structures by evaluating multiple conditions. The nested if-else statement follows the same syntax as the basic if-else construct:
“`python
if condition1:
# code block executed if condition1 is True
if condition2:
# code block executed if condition1 and condition2 are True
else:
# code block executed if condition1 is True and condition2 is False
else:
# code block executed if condition1 is False
“`
Nested if-else statements can be useful in scenarios where the outcome depends on multiple variables or conditions. However, it’s important to ensure proper indentation to maintain code readability and avoid confusion.
### Common Use Cases
if-else statements find extensive application in various real-world programming scenarios. A few common use cases of if-else statements in Python include:
1. **User input validation:** If-else statements are handy for validating user input within a program. They can check user-provided values against predefined criteria, such as checking if the input is within a specific range or meets a certain condition.
2. **Control flow management:** If-else statements help manage the flow of program execution based on certain conditions. For instance, they can be used to determine which branch of code to execute, based on different scenarios or inputs.
3. **Error handling:** If-else statements are useful in handling errors or exceptions in programs. By checking for specific error conditions, code blocks within if or else clauses can be executed to handle these exceptional cases.
4. **Custom filters or transformations:** In data processing applications, if-else statements can be utilized to apply custom filters or transformations based on specific conditions. For instance, while processing a list of numbers, an if-else statement can be used to filter out all even numbers or transform them in a specific way.
### FAQs about If-else Statements in Python
Q1. Can an if statement contain multiple conditions?
Yes, an if statement can contain multiple logical or comparison conditions using logical operators like “and” or “or”. For example:
“`python
if condition1 and condition2:
# code block executed if both conditions are True
“`
Q2. Can “else if” be written as “elseif” in Python?
No, Python uses the keyword `elif` instead of `elseif` for the “else if” construct.
Q3. Can I have multiple elif statements in a single if-else block?
Yes, multiple elif statements can be included in a single if-else block if there are multiple conditions to be checked. This allows for more than two possible outcomes.
Q4. What happens if none of the conditions in an if-else block evaluate to True?
If none of the conditions in an if-else block evaluate to True, and there is no else clause, the block is skipped, and the program continues with the next line of code after the if-else statement.
Q5. Can I have a nested if-else block within an else clause?
Yes, it is possible to have a nested if-else block within an else clause. This allows for further condition checks based on the outcome of the initial condition check.
In conclusion, if-else statements are a powerful tool in Python programming, allowing developers to execute specific code blocks based on specific conditions. By leveraging if-else statements, programmers can enhance the control flow, handle errors, and apply custom logic to their programs. Understanding the syntax and usage of if-else statements is essential for efficient decision-making and creating more complex program structures.
Images related to the topic python else do nothing
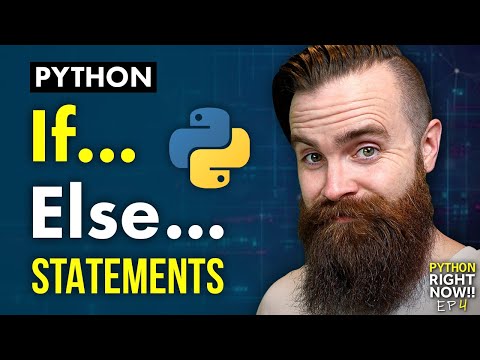
Found 41 images related to python else do nothing theme
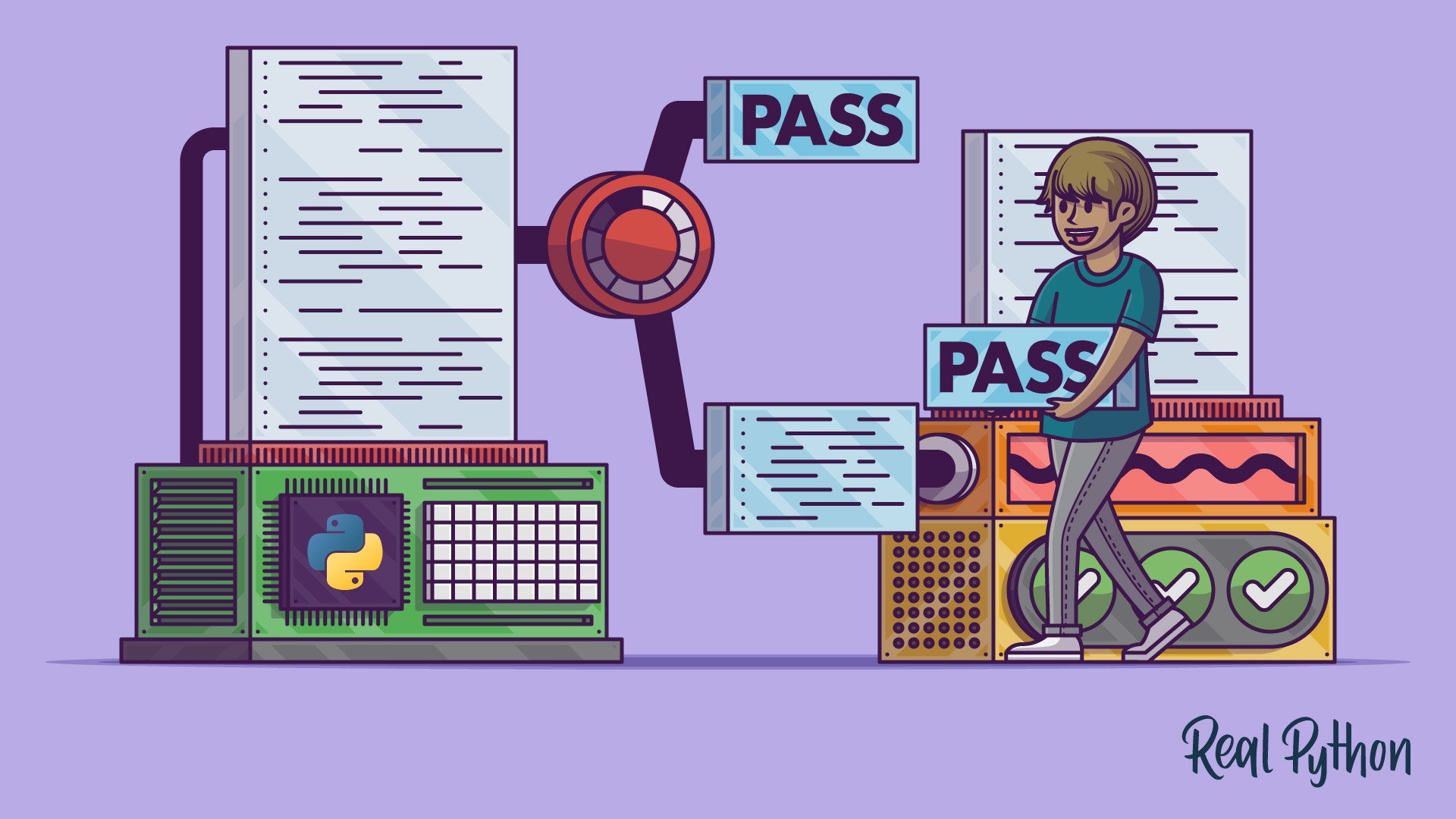
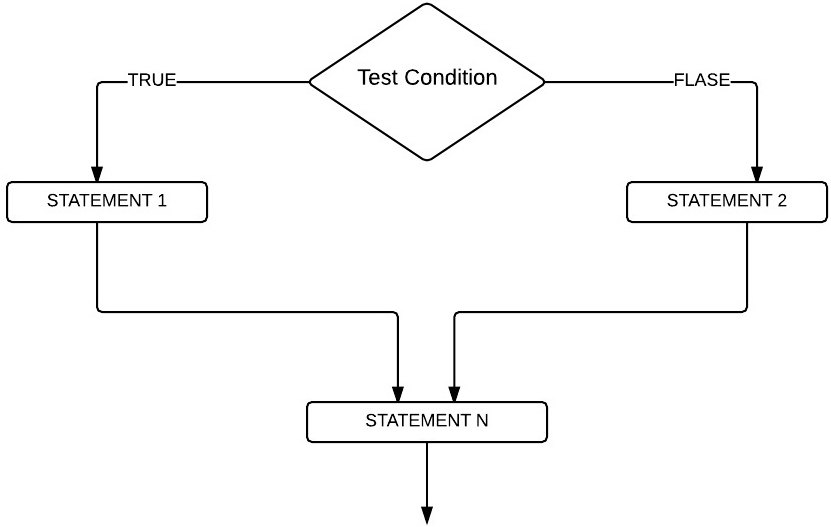
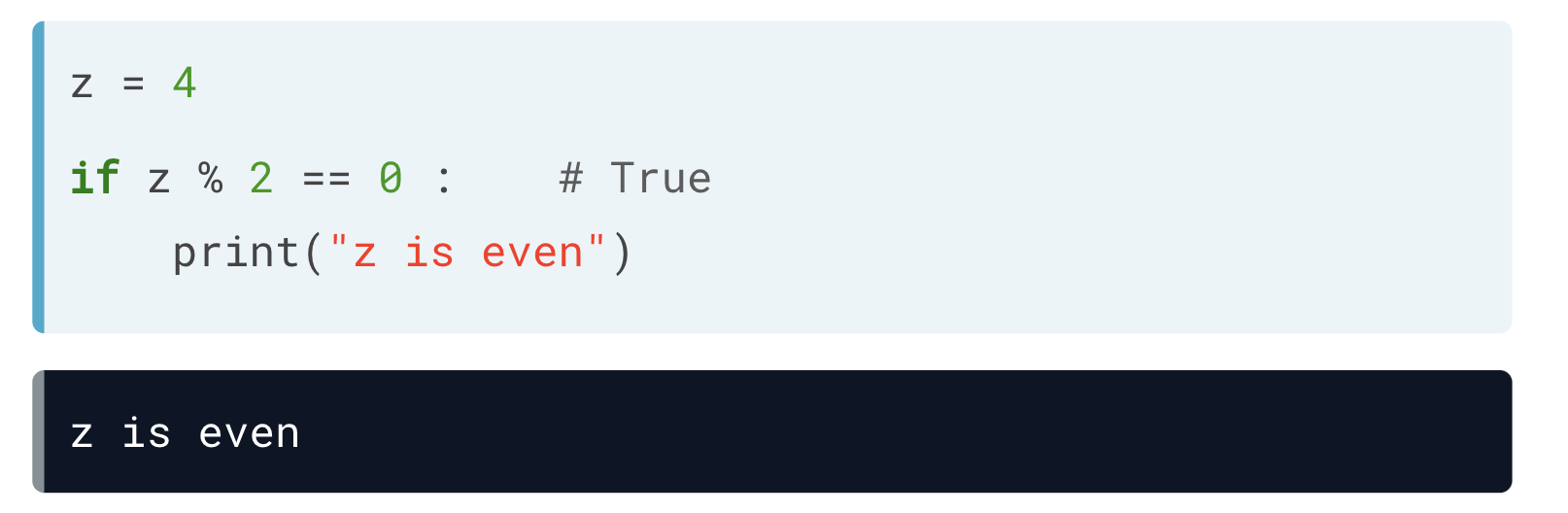


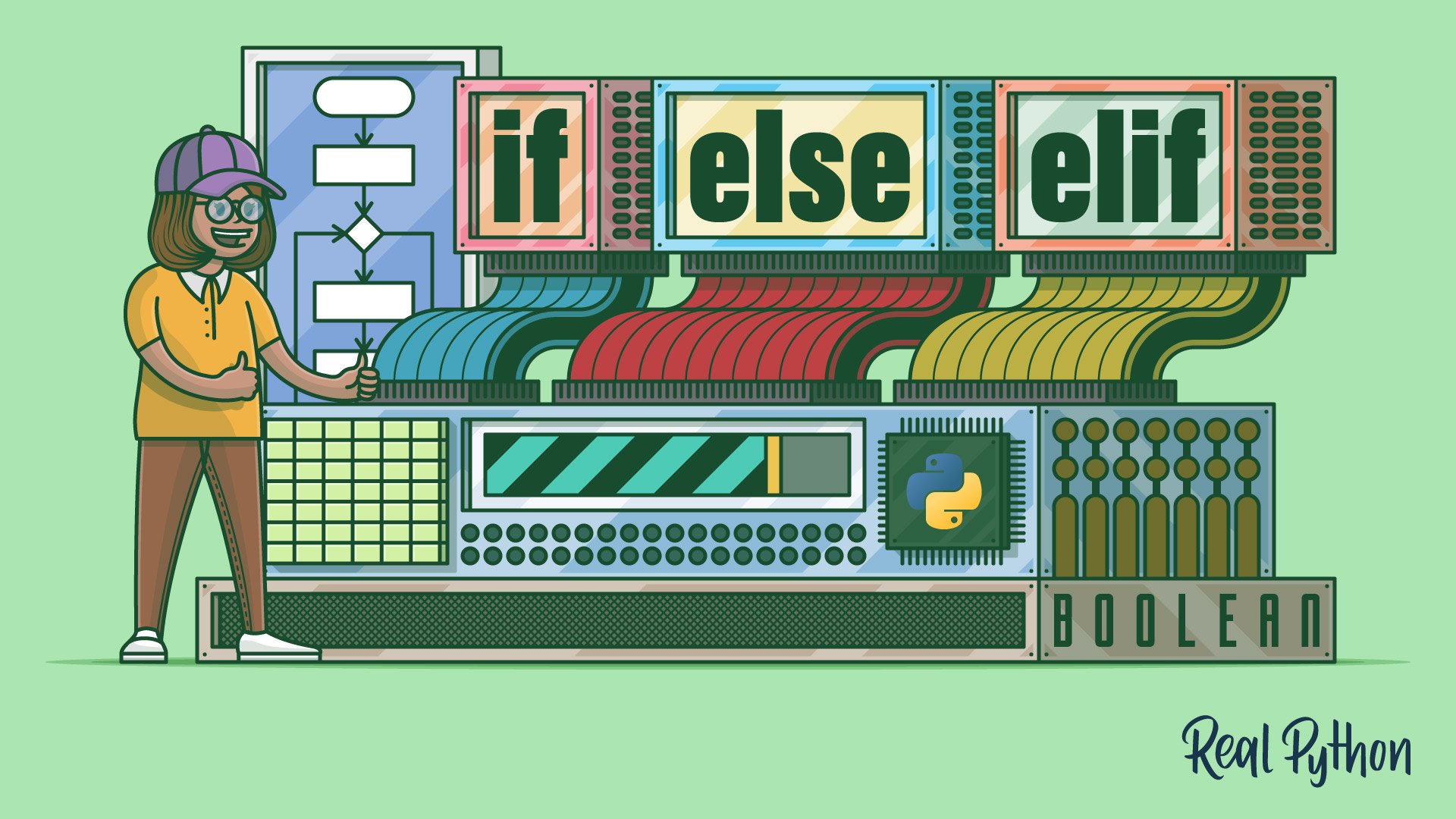
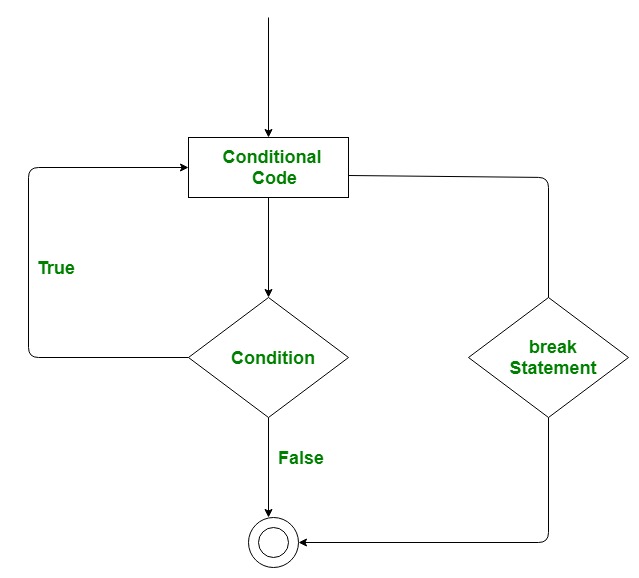
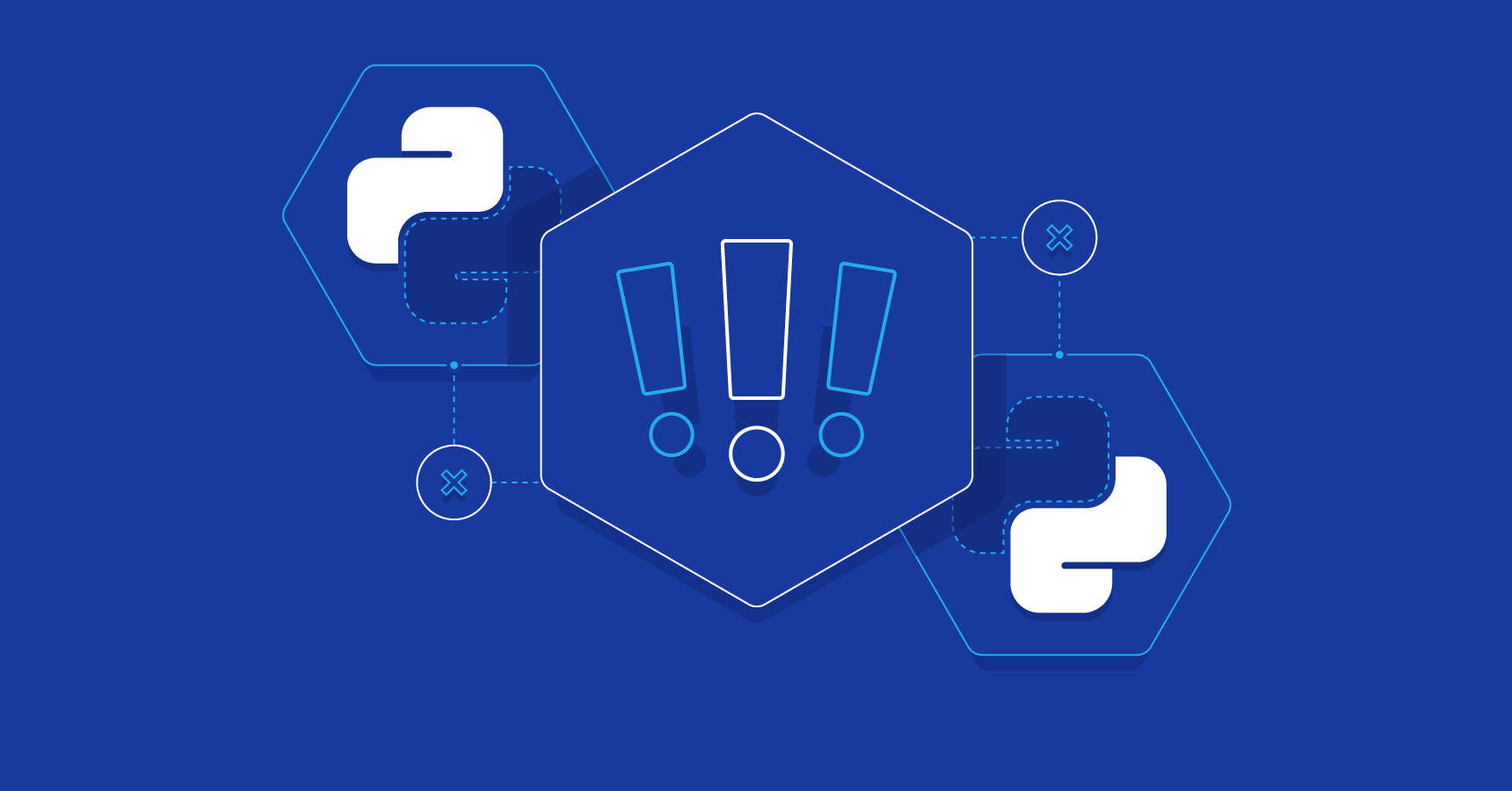


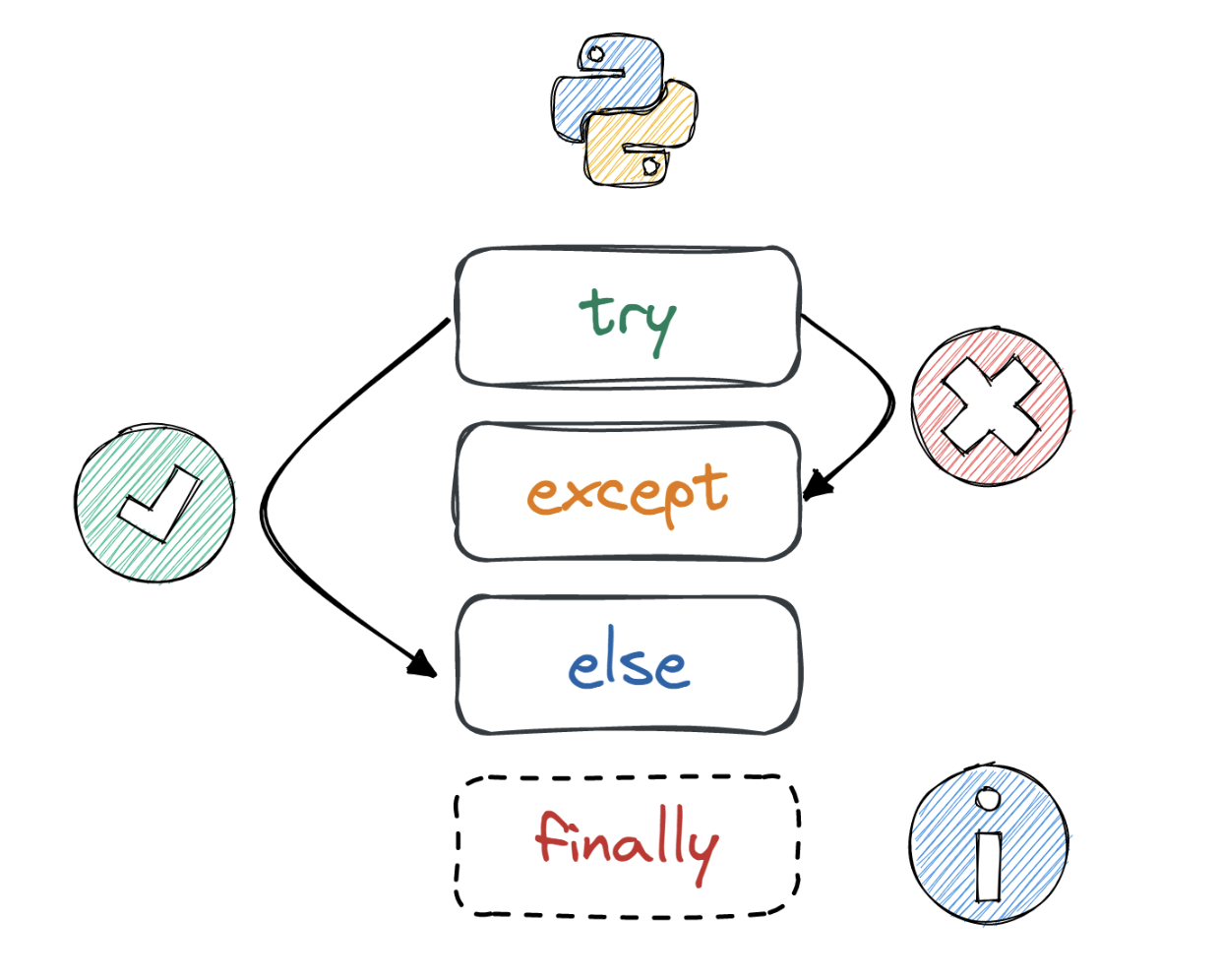
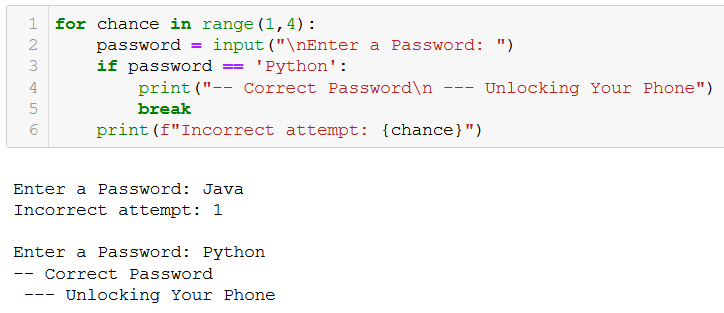
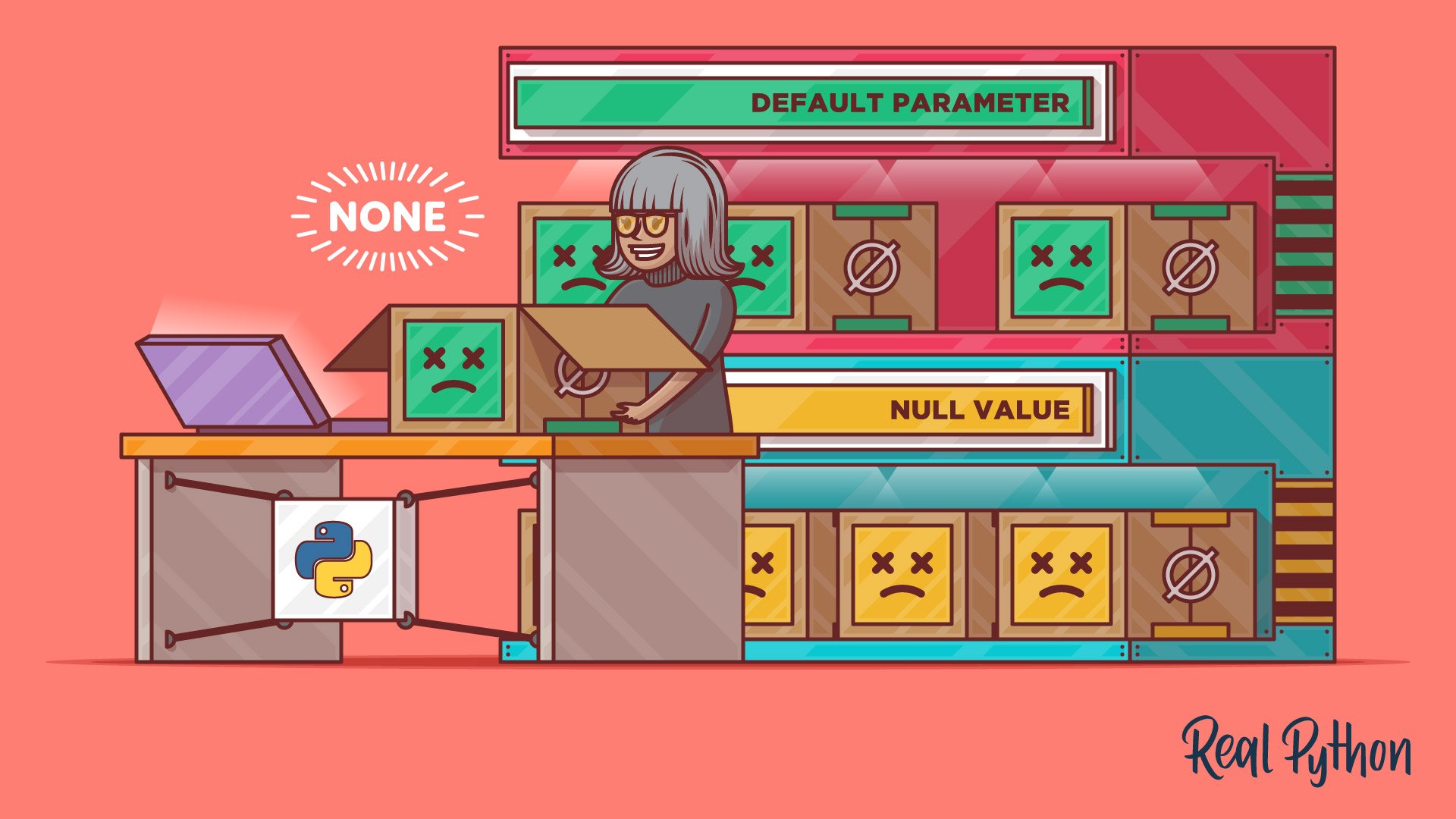

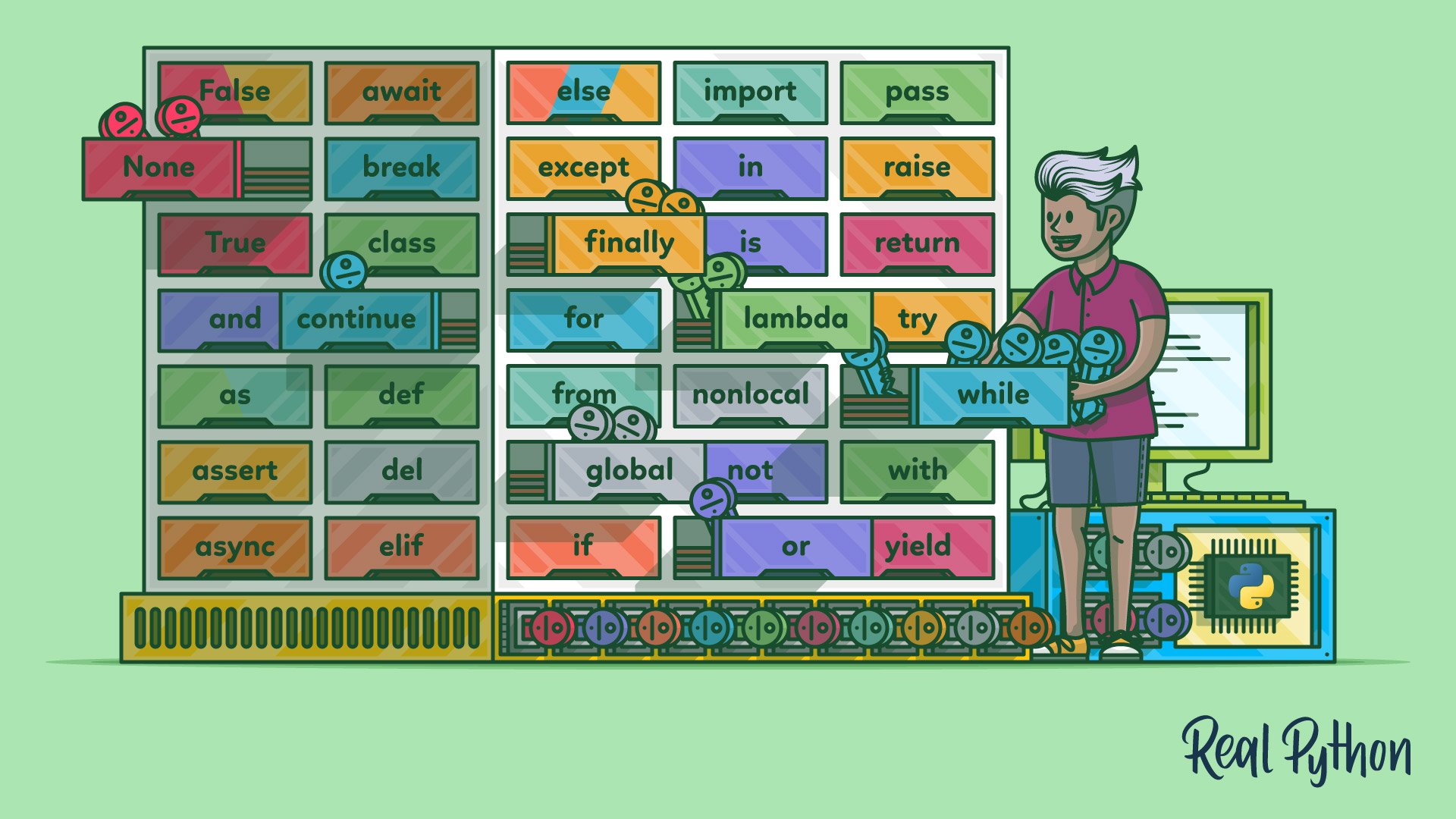
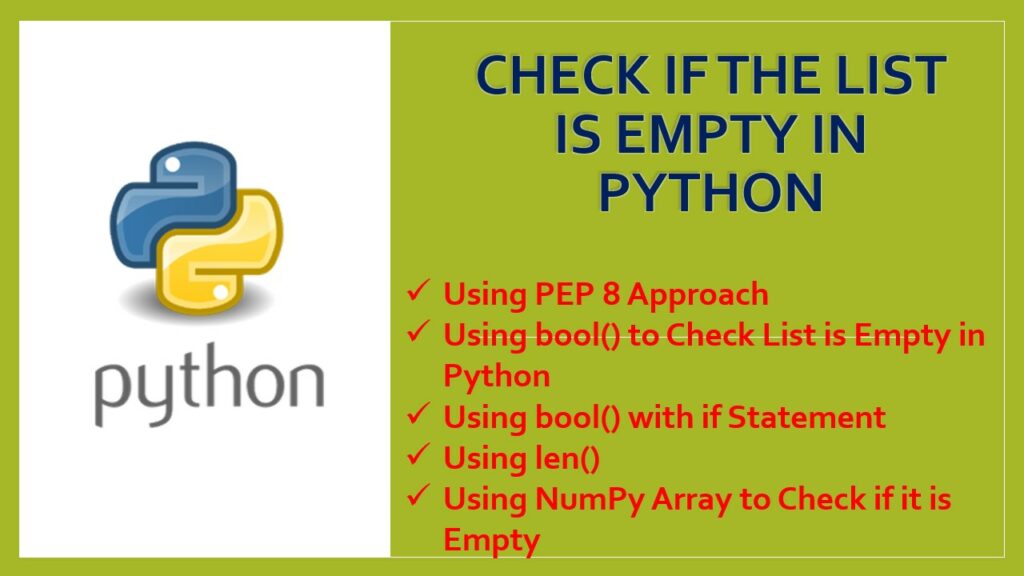

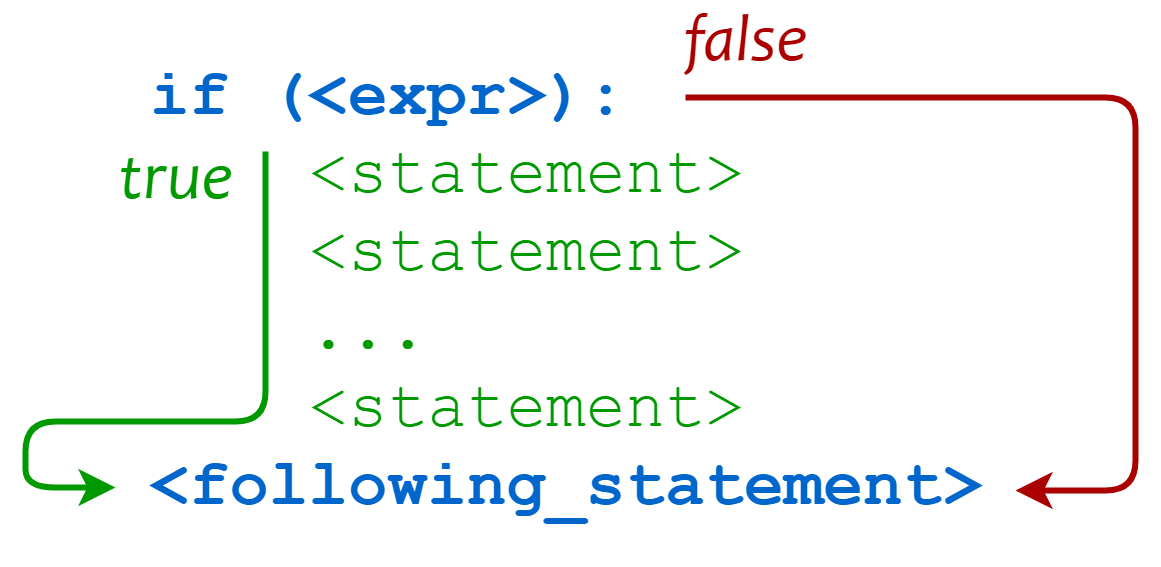
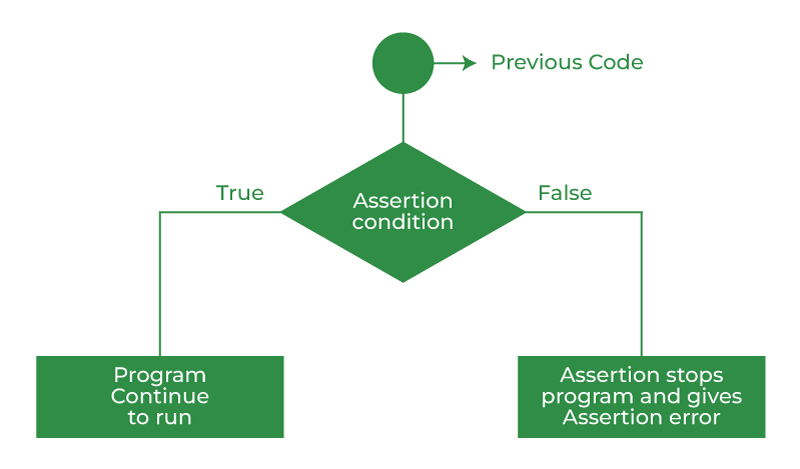
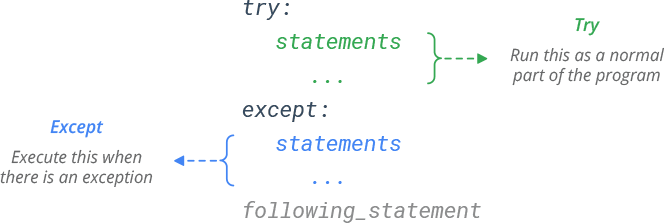
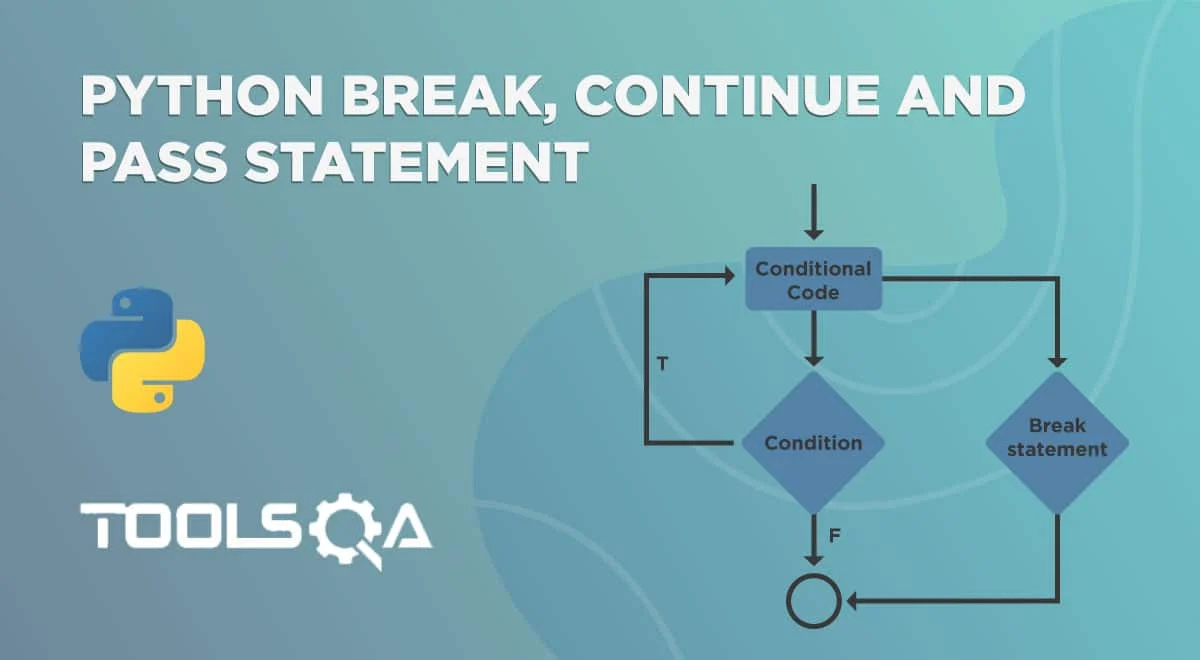
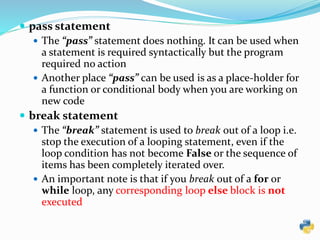
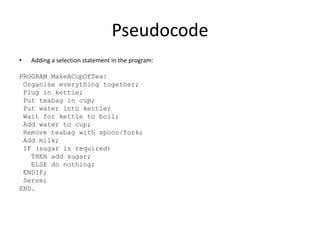
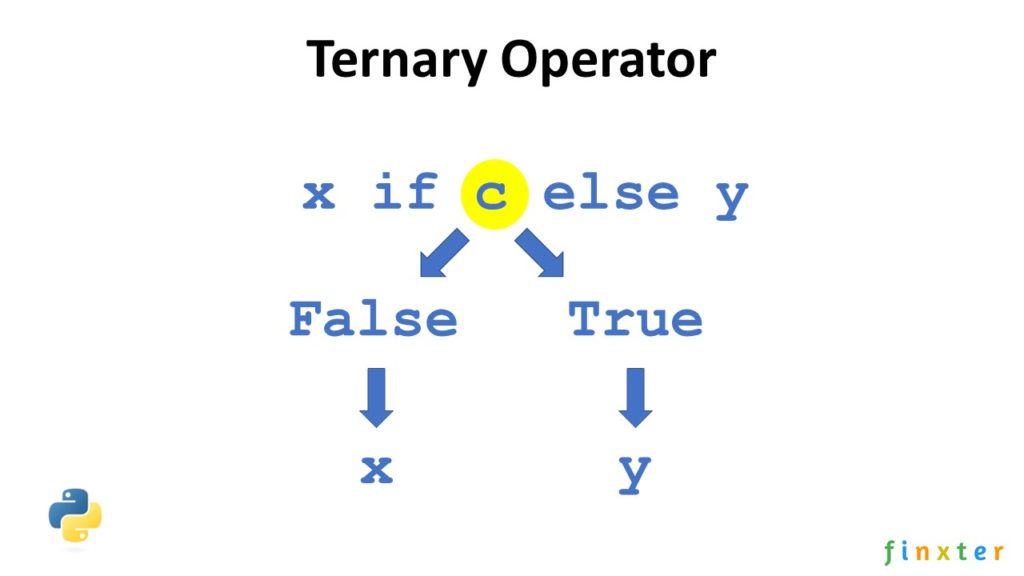
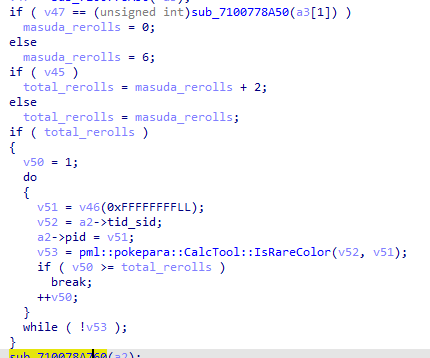
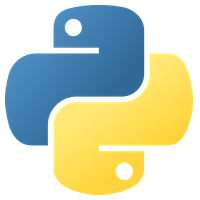
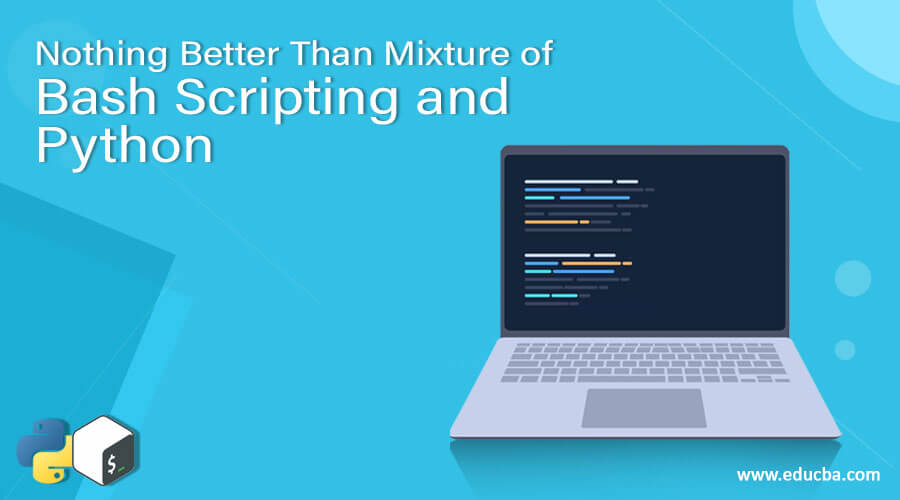
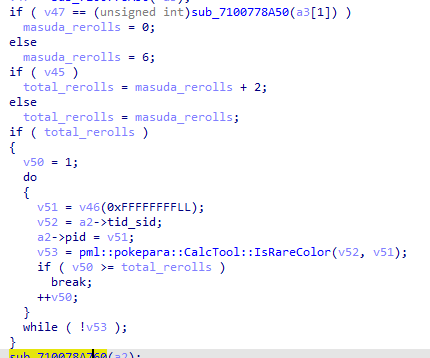
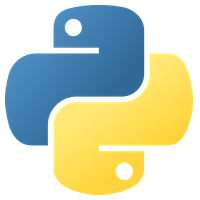

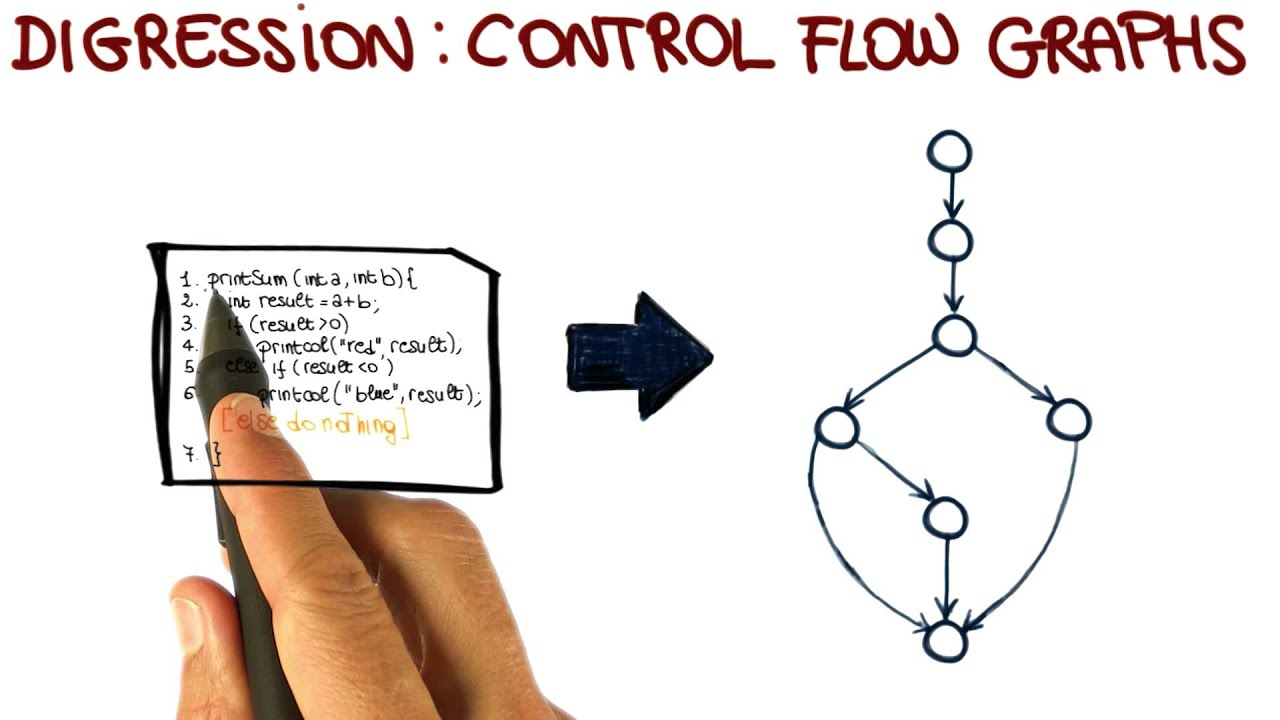
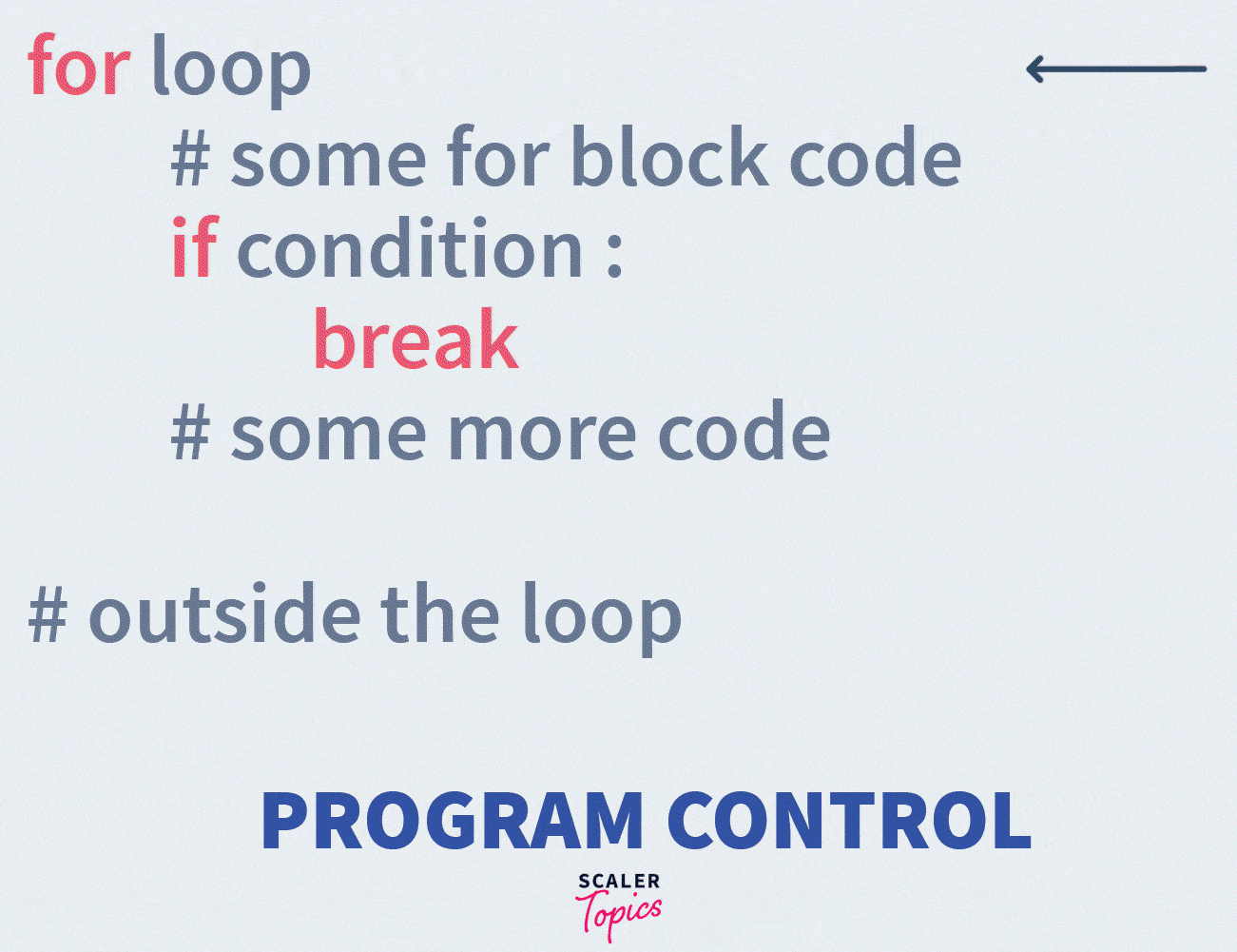


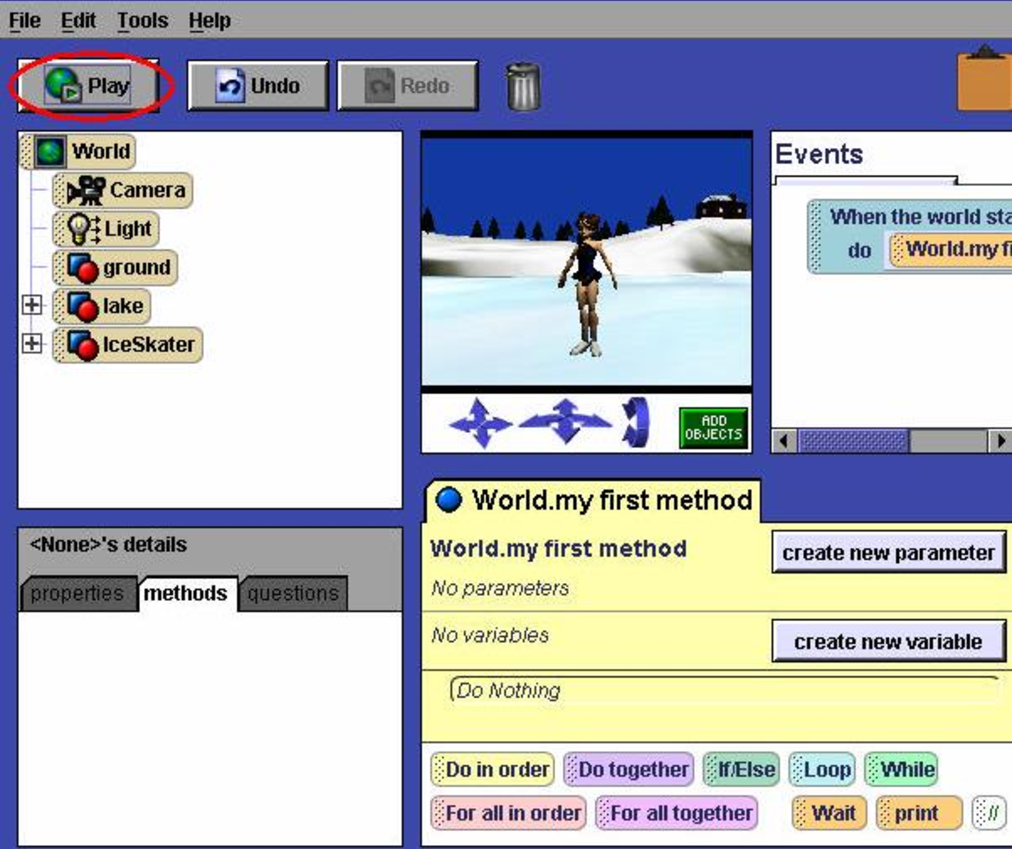
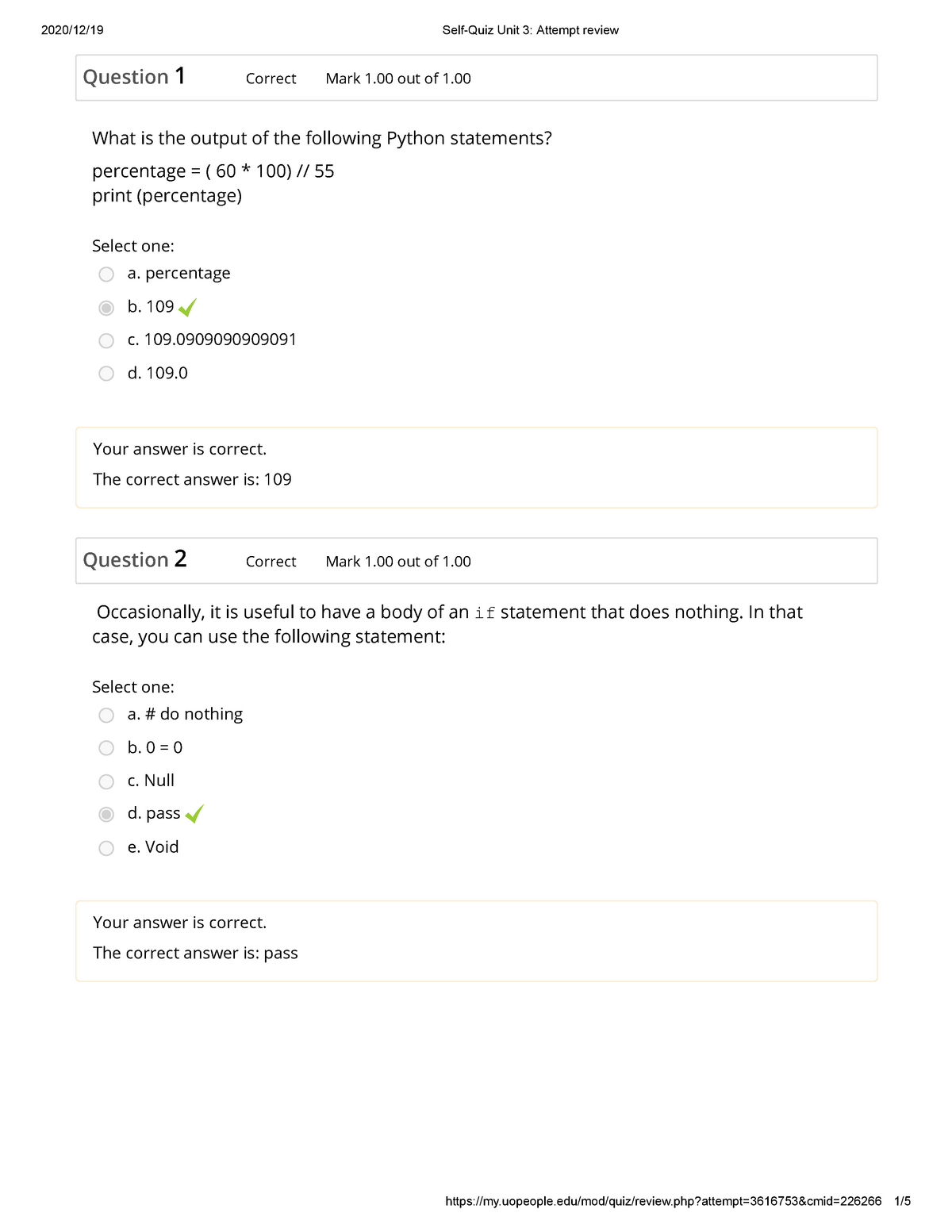

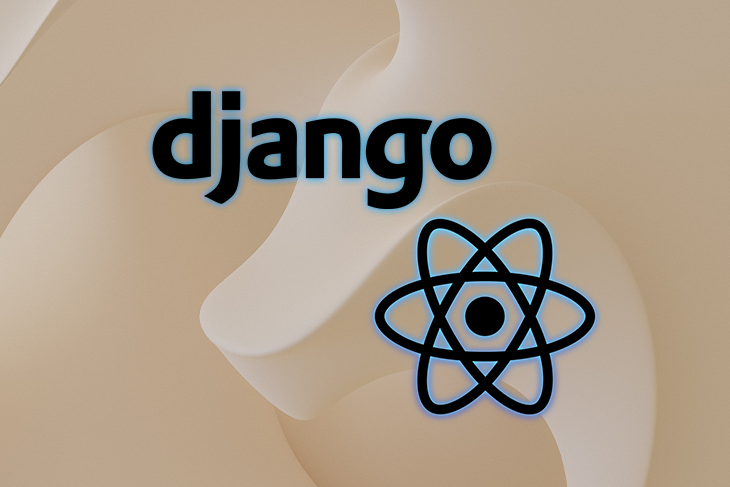
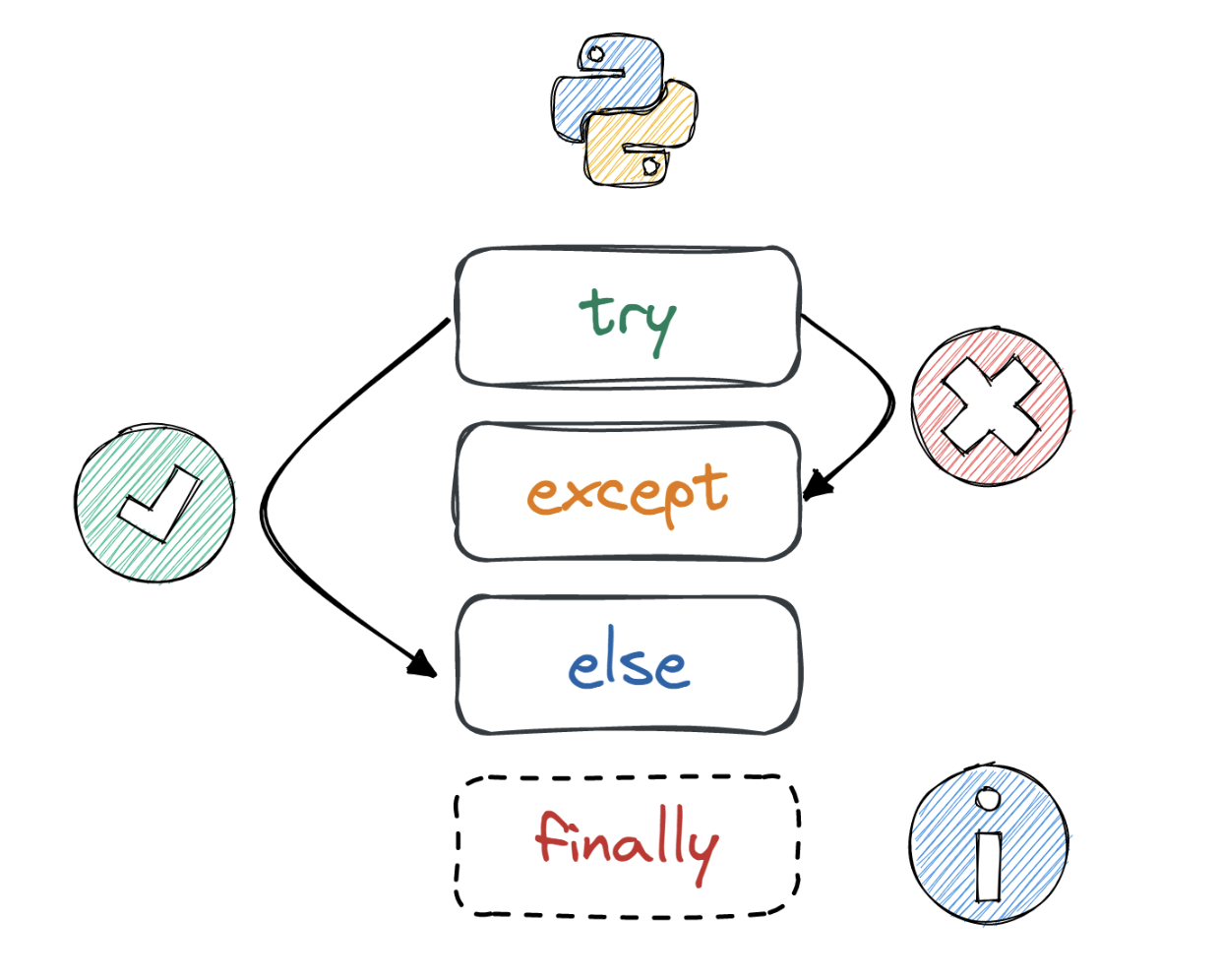
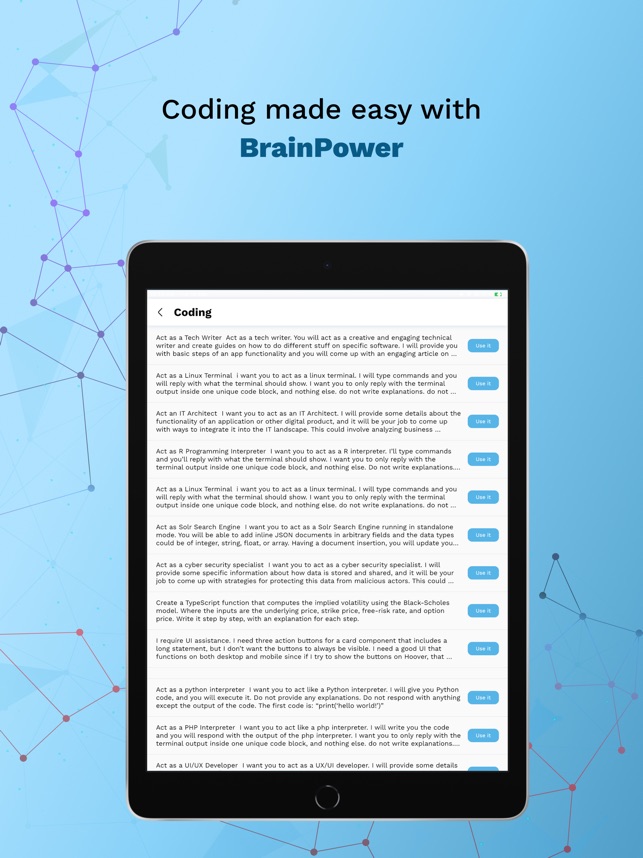





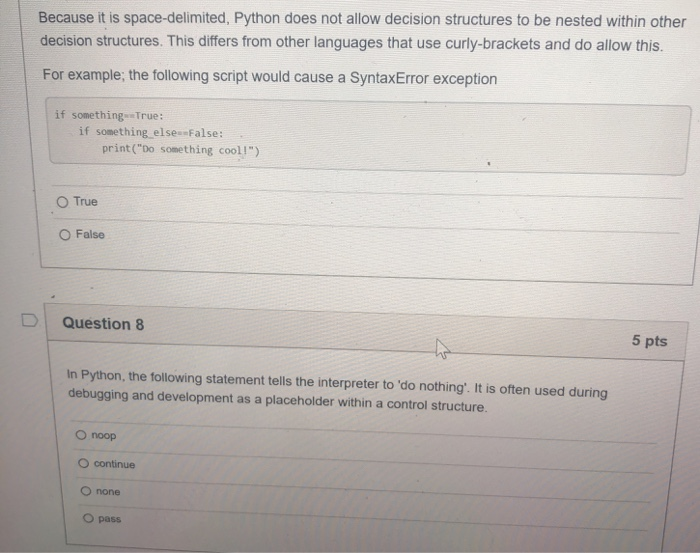


Article link: python else do nothing.
Learn more about the topic python else do nothing.
- The pass Statement: How to Do Nothing in Python
- 1.4 How to make “else:” do nothing? – Codecademy
- 1 Easy Way To Fix The Else Do Nothing Python Error
- Do Nothing Inside an if Statement in Python – Delft Stack
- Understanding Else Do-Nothing Statements In Python
- Python pass (Do Nothing): When And How To Use
- How do I get a python program to do nothing? – Stack Overflow
- The pass statement in Python – nkmk note
- How to write an empty function in Python – pass statement?
- Python pass statement: When, why, and how to use it
See more: nhanvietluanvan.com/luat-hoc