Else Do Nothing Python
Why Use the “else” Statement in Python
The “else” statement in Python is a powerful tool that allows you to specify what should happen when a condition is not met. It provides a way to handle alternative cases or to execute a default block of code when no specific conditions are satisfied. By using the “else” statement, you can make your code more robust and handle scenarios that would otherwise produce logical errors.
Syntax of the “else” Statement
The basic syntax of the “else” statement in Python is as follows:
“`python
if condition:
# code block executed when condition is True
else:
# code block executed when condition is False
“`
The “else” block is always associated with the nearest preceding “if” statement. It is important to note that the “else” block can only be used after an “if” statement. It cannot be used on its own.
How the “else” Statement Works in Python
When the “else” statement is encountered, it is only executed if the preceding “if” statement evaluates to False. If the condition of the “if” statement is True, the “else” block will be skipped entirely.
The “else” block can contain any valid Python code, including multiple statements or nested blocks. It is important to properly indent the code within the “else” block to maintain the correct structure and avoid syntax errors.
Simple Example of Using “else” Statement in Python
Let’s see a simple example to illustrate the usage of the “else” statement in Python:
“`python
x = 5
if x > 10:
print(“x is greater than 10”)
else:
print(“x is less than or equal to 10”)
“`
In this example, the condition in the “if” statement is False because 5 is not greater than 10. Therefore, the code within the “else” block is executed, and the output will be:
“`
x is less than or equal to 10
“`
Using the “else” Statement with Conditional Statements
The “else” statement can be used in conjunction with various conditional statements in Python, such as “if-elif-else” and nested “if” statements. It allows you to handle multiple conditions and execute different code blocks based on the outcome of each condition.
For example:
“`python
x = 5
if x > 10:
print(“x is greater than 10”)
elif x < 5:
print("x is less than 5")
else:
print("x is between 5 and 10")
```
In this case, since none of the conditions in the "if" and "elif" statements evaluate to True, the code within the "else" block is executed. The output will be:
```
x is between 5 and 10
```
Using the "else" Statement with Loops
The "else" statement can also be combined with loops in Python. When used in conjunction with a "for" or "while" loop, the "else" block is executed only if the loop finishes normally, i.e., without encountering a "break" statement.
Let's take a look at an example with a "for" loop:
```python
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 6:
print("Number found!")
break
else:
print("Number not found!")
```
In this example, the loop iterates over a list of numbers. If the number 6 is found, the "break" statement is executed, and the loop is terminated. In this case, the code within the "else" block is not executed.
However, if the loop finishes without encountering a "break" statement, the code within the "else" block is executed, indicating that the number 6 was not found:
```
Number not found!
```
Nested "else" Statements
In Python, it is possible to have nested "else" statements, where one "else" block is nested within another. This allows for more complex control flow and enables you to handle multiple conditions in a hierarchical manner.
Here is an example to illustrate nested "else" statements:
```python
x = 5
y = 10
if x > 0:
if y > 5:
print(“x is positive and y is greater than 5”)
else:
print(“x is positive, but y is not greater than 5”)
else:
print(“x is not positive”)
“`
In this case, the outer “else” block is associated with the outer “if” statement, while the inner “else” block is associated with the inner “if” statement. The output will be:
“`
x is positive and y is greater than 5
“`
Using “else” Statement with Try-Except Blocks
The “else” statement can also be used in conjunction with the “try-except” blocks in Python. When an error occurs within the “try” block, the “except” block is executed. However, if no error occurs, the code within the “else” block is executed.
Let’s consider an example:
“`python
try:
x = 5 / 0
except ZeroDivisionError:
print(“Error: Division by zero!”)
else:
print(“No error occurred!”)
“`
In this example, dividing 5 by 0 would raise a ZeroDivisionError. Therefore, the code within the “except” block is executed, and the output will be:
“`
Error: Division by zero!
“`
If we modify the code to avoid the error, the code within the “else” block will be executed:
“`python
try:
x = 5 / 1
except ZeroDivisionError:
print(“Error: Division by zero!”)
else:
print(“No error occurred!”)
“`
The output will be:
“`
No error occurred!
“`
Common Mistakes and Pitfalls with the “else” Statement
While using the “else” statement in Python, it’s important to be aware of some common mistakes and pitfalls that may arise:
1. Missing indentation: Ensure that the code within the “else” block is indented properly. Failure to do so will result in a syntax error.
2. Incorrect indentation: Ensure that the indentation of the “else” statement matches the indentation of the preceding “if” statement. Mismatched indentation can lead to unexpected behavior.
3. Misinterpreting the “else” block: Remember that the “else” block is only executed if the condition of the preceding “if” statement is False. If the condition is True, the “else” block will be skipped entirely.
Python if-else one line, If in one line python without else, If else Python, Pass in function python, Empty lambda python, Exit() function Python, Class ‘NoneType Python, Return Pythonelse do nothing python.
In addition to the “else” statement, there are other related topics in Python that may be of interest. Here’s a brief overview:
Python if-else one line: Python allows you to write if-else statements on a single line, using the ternary operator. This can make the code more concise and readable. For example: `x = 5 if condition else 10`.
If in one line python without else: Similarly, if-else statements can be written on a single line without the “else” keyword. This is useful when you only want to execute code based on a condition, without providing an alternative case. For example: `print(“Hello”) if condition`.
Pass in function python: The “pass” keyword in Python is used as a placeholder when no action is required. It is often used as a stub for functions or classes that are not yet implemented. For example:
“`python
def my_function():
pass
“`
Empty lambda python: In Python, lambda functions are anonymous functions that can be created on the fly. If you need a lambda function that does nothing, you can use the “pass” keyword as the function body. For example: `my_lambda = lambda: pass`.
Exit() function Python: The “exit()” function in Python is used to terminate the execution of a program. It can be called without any arguments to exit the program immediately. For example: `exit()`.
Class ‘NoneType Python: In Python, the NoneType class represents the absence of a value. It is commonly used to indicate a null or missing value. For example, if a function does not return anything, it is implicitly returning None.
Return Python: The “return” statement in Python is used to exit a function and return a value. It can also be used to specify the default value to be returned when no conditions are met.
In conclusion, the “else” statement in Python provides a way to handle alternative cases or to specify code that should only be executed when no specific conditions are met. It is a powerful tool that can make your code more robust and flexible. By understanding its syntax and usage, you can write more efficient and reliable programs.
If Else Statements In Python // Python Right Now!! // Ep 4
Keywords searched by users: else do nothing python Python if-else one line, If in one line python without else, If else Python, Pass in function python, Empty lambda python, Exit() function Python, Class ‘NoneType Python, Return Python
Categories: Top 64 Else Do Nothing Python
See more here: nhanvietluanvan.com
Python If-Else One Line
Python, an open-source programming language known for its simplicity and versatility, offers a wide range of tools and techniques to simplify coding tasks. One such feature that stands out is the ability to write if-else statements in a single line of code. This concise syntax not only improves readability but also allows programmers to implement decision-making logic swiftly and efficiently. In this article, we will delve into the intricacies of Python if-else one-liners, explore their advantages and limitations, and provide some frequently asked questions (FAQs) to facilitate a deeper understanding.
Understanding the If-Else Statement in Python
Before diving into the realm of one-liners, it is essential to grasp the basic concept of an if-else statement in Python. Essentially, an if-else statement allows programmers to evaluate a condition and execute specific code blocks based on the outcome. It follows the syntax:
if condition:
# code block to execute if condition is True
else:
# code block to execute if condition is False
However, in situations where the code block for each condition consists of a single statement, Python provides an elegant solution – the if-else one-liner.
Python If-Else One-Liners
Python’s one-liners allow developers to write if-else statements in a compact manner, often requiring only a single line of code. This feature proves to be especially useful for simple conditionals, where their implementation is straightforward. The syntax for a typical if-else one-liner is as follows:
[on_true] if [expression] else [on_false]
Here, [expression] represents the condition to be evaluated. If the condition is True, [on_true] is executed; otherwise, [on_false] is executed. Note that both [on_true] and [on_false] can consist of simple statements or expressions.
Advantages of Python If-Else One-Liners
The use of if-else one-liners in Python offers several benefits, including:
1. Conciseness: By reducing the if-else statement to a single line, Python enhances code readability and minimizes unnecessary code clutter. This concise syntax promotes clean and elegant coding practices.
2. Improved Speed: One-liners eliminate the need for multiple lines of code, resulting in reduced compilation and execution time. This advantage is particularly crucial in scenarios where performance optimization is essential, such as data analysis or resource-intensive tasks.
3. Enhanced Readability: While concise, if-else one-liners can improve code readability when used judiciously. They allow programmers to focus on the logic rather than extraneous syntax, enabling faster comprehension and code maintenance.
4. Versatility: Python’s if-else one-liners can accommodate a wide range of expressions. From arithmetic operations to function calls and lambda expressions, these one-liners provide flexibility in decision-making logic.
Limitations and Considerations
Despite their advantages, if-else one-liners may not always be the best choice. It is crucial to bear in mind the following limitations and considerations:
1. Complex Logic: If-else one-liners are best suited for simple and concise conditionals. When dealing with more intricate decision-making or nested conditions, opting for a traditional if-else statement with multiple lines may enhance readability and maintainability.
2. Readability Overuse: Like any concise syntax, excessive use of if-else one-liners can result in code that is difficult to comprehend and maintain. It is vital to strike a balance between readability and conciseness, favoring the former in situations where the logic becomes convoluted.
3. Debugging Challenges: In cases where an if-else one-liner causes an error or unexpected behavior, debugging can be more challenging due to the compact nature of the code. Thus, it is important to write clear and tested conditionals to minimize such issues.
Python If-Else One-Liner FAQs
Q: Can multiple conditions be evaluated in a single if-else one-liner?
A: Yes, Python allows for the use of logical operators such as “and” and “or” within if-else one-liners to evaluate multiple conditions.
Q: Can I assign values based on a condition using if-else one-liners?
A: Absolutely. By utilizing if-else one-liners, programmers can assign different values to a variable based on a condition, greatly enhancing code readability.
Q: How can I handle the scenario when the if-else block involves more than one statement?
A: In such cases, if-else one-liners can still be used by enclosing the code in parentheses. For example: ([on_true]; [additional_statement]) if [expression] else [on_false].
Q: Are if-else one-liners specific to Python programming?
A: No, one-liners are not unique to Python. However, Python’s clean and concise syntax makes it especially suitable for implementing efficient if-else one-liners.
In conclusion, Python’s if-else one-liners provide a powerful tool to streamline decision-making in programming. Their concise syntax, along with enhanced readability and versatility, contributes to efficient code writing and faster execution. However, it is crucial to use one-liners judiciously, considering their limitations and potential impact on code complexity. By striking a balance between simplicity and readability, developers can effectively leverage this feature to enhance their Python programming capabilities.
If In One Line Python Without Else
When writing an if statement in Python, the general syntax follows the structure:
“`
if condition:
# block of code to execute if the condition is True
else:
# block of code to execute if the condition is False
“`
The else block is optional, but it allows you to specify the actions to be taken when the condition is false. However, in some cases, you might want to execute a single line of code based on a condition, without the need for an else statement. This is where the if in one line Python comes in handy.
To write an if statement in a single line, you can make use of the ternary operator, represented by the symbol “?”. The ternary operator allows you to assign a value or execute a code block based on a condition. The syntax is as follows:
“`
value_if_true if condition else value_if_false
“`
In this line, the value_if_true will be returned if the condition is true, otherwise, the value_if_false will be returned. This allows you to shorten your code and make it more concise, especially when dealing with simple if-else conditions.
Let’s illustrate this with a few examples. Suppose we want to determine whether a given number is even or odd using if in one line Python. We can achieve this using the following ternary operator:
“`
num = 10
result = “Even” if num % 2 == 0 else “Odd”
print(result)
“`
In this example, if the condition num % 2 == 0 is true, the value “Even” will be assigned to the variable result; otherwise, “Odd” will be assigned. Consequently, the output will be “Even”.
Similarly, you can use the if in one line Python approach to execute a single line of code based on a condition. Consider the following example where we want to print a message if a given variable is True:
“`
is_true = True
print(“It is true!”) if is_true else None
“`
In this case, the ternary operator is used to print the message “It is true!” when the condition is True. Otherwise, the code will have no output, as the value assigned is None.
If you need to execute multiple statements based on a condition, you can still utilize if in one line Python by using semicolons to separate the statements. However, it is important to note that this can quickly become unreadable and is generally not recommended for complex logic. Here’s an example:
“`
age = 18
is_adult = True if age >= 18 else False; print(“You are an adult.”) if is_adult else print(“You are not an adult.”)
“`
In this case, if the age is 18 or above, the variable is_adult will be assigned True, and the message “You are an adult” will be printed. Otherwise, if the age is below 18, the variable will be assigned False, and the message “You are not an adult” will be printed.
Frequently Asked Questions (FAQs):
Q: Can I achieve the same functionality without using the if in one line Python approach?
A: Yes, you can accomplish the same functionality by using a regular if statement with a block of code.
Q: Is it recommended to use if in one line Python for complex conditions?
A: No, using the ternary operator in such cases may make the code harder to read and understand. It is generally more suitable for simple conditions with single-line statements.
Q: Can I nest if in one line Python statements?
A: Technically, yes. You can nest multiple ternary operators within each other, but using multiple nested ternary operators is not recommended as it can make the code less readable and harder to debug.
Q: Do I have to use the else block in the if in one line Python approach?
A: No, the else block is optional. If you do not provide else or value_if_false, the ternary operator will return None if the condition is False.
In summary, if in one line Python without else provides a concise way to execute a single line of code or assign values based on a condition. By utilizing the ternary operator, you can simplify your code and make it more readable. However, it is important to use this approach sparingly and avoid complex conditions to ensure maintainability and clarity.
If Else Python
Python is a powerful programming language that provides programmers with various tools to control the flow of their code. Among these tools, the if else statement is a fundamental construct that allows for conditional execution. In this article, we will explore the if else statement in Python, its syntax, usage, and provide some frequently asked questions to help deepen your understanding.
Understanding the If Else Statement:
The if else statement is used to make decisions within a program based on certain conditions. It allows the execution of different code blocks depending on whether a given condition is true or false. This capability provides great flexibility and control in developing programs.
Syntax and Usage:
The syntax of an if else statement in Python is as follows:
“`
if condition:
# code block executed if the condition is true
else:
# code block executed if the condition is false
“`
The condition is typically a comparison or logical expression that evaluates to either true or false. If the condition is true, the first code block is executed; otherwise, the second code block within the else clause is executed.
To illustrate this further, consider the following example:
“`
num = 10
if num > 0:
print(“The number is positive.”)
else:
print(“The number is non-positive.”)
“`
In this example, we have a variable `num` with the value of 10. The condition `num > 0` is evaluated to true, so the first code block is executed, printing “The number is positive.”
Nested If Else Statements:
In Python, it is also possible to nest if else statements within other if else statements. This allows for more complex decision-making structures. Let’s take an example to understand this concept:
“`
num = 5
if num > 0:
print(“The number is positive.”)
elif num < 0:
print("The number is negative.")
else:
print("The number is zero.")
```
In this example, we have three conditions to check. If the number is greater than zero, the first code block is executed. If the number is less than zero, the second code block is executed. Otherwise, if both conditions are false, the else block is executed.
Common Mistakes to Avoid:
1. Forgetting the colon at the end of the if and else statements: It is essential to include a colon at the end of these statements to ensure correct syntax.
2. Not indenting the code within the if and else blocks correctly: Python uses indentation to determine the code block associated with each statement. Failing to indent code properly will result in a syntax error.
Frequently Asked Questions (FAQs):
Q1. Can I have multiple conditions within a single if statement?
A1. Yes, you can use logical operators such as `and`, `or`, and `not` to combine multiple conditions within a single if statement.
Q2. Is it necessary to include the else clause in every if statement?
A2. No, the else clause is optional. If you only want to execute code when the condition is true, you can omit the else clause.
Q3. Can I have multiple elif clauses in an if else statement?
A3. Yes, you can have multiple elif clauses to check for additional conditions. However, only one code block within the if-elif-else chain will be executed.
Q4. Can the condition in an if statement be anything other than a Boolean expression?
A4. The condition in an if statement can be any expression that evaluates to either true or false. This includes comparison operators, logical operators, and even user-defined functions that return a Boolean value.
Q5. Can I nest if else statements indefinitely?
A5. While you can nest if else statements within each other, it is generally advisable to keep the nesting to a reasonable level for code readability and maintainability.
In conclusion, the if else statement in Python is a powerful construct that enables programmers to make decisions based on certain conditions. By understanding its syntax, usage, and common mistakes to avoid, you can leverage the full potential of this feature and develop more sophisticated and efficient programs.
Images related to the topic else do nothing python
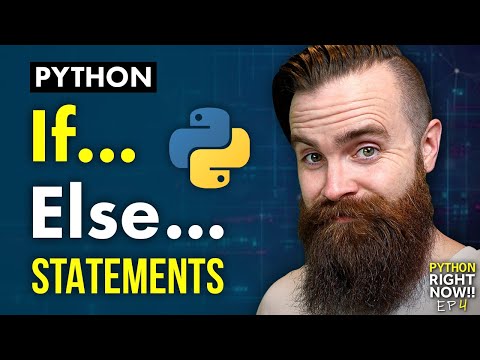
Found 27 images related to else do nothing python theme

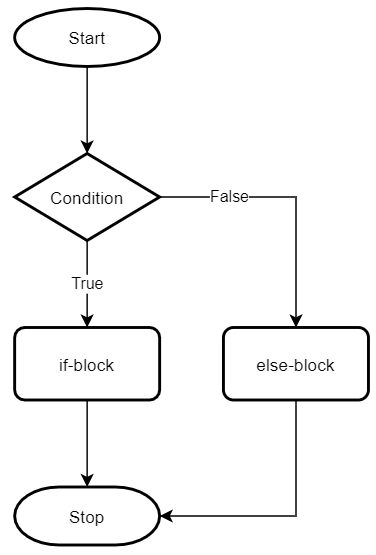

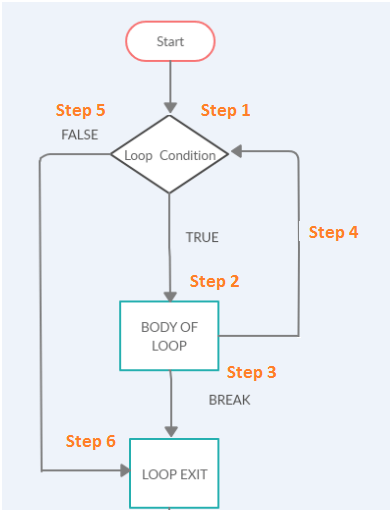
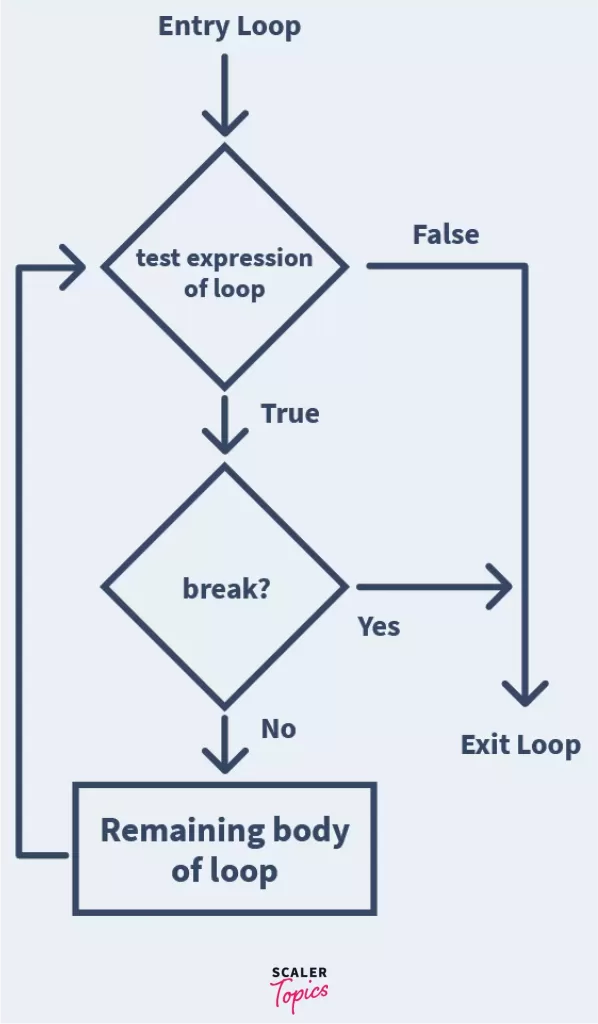
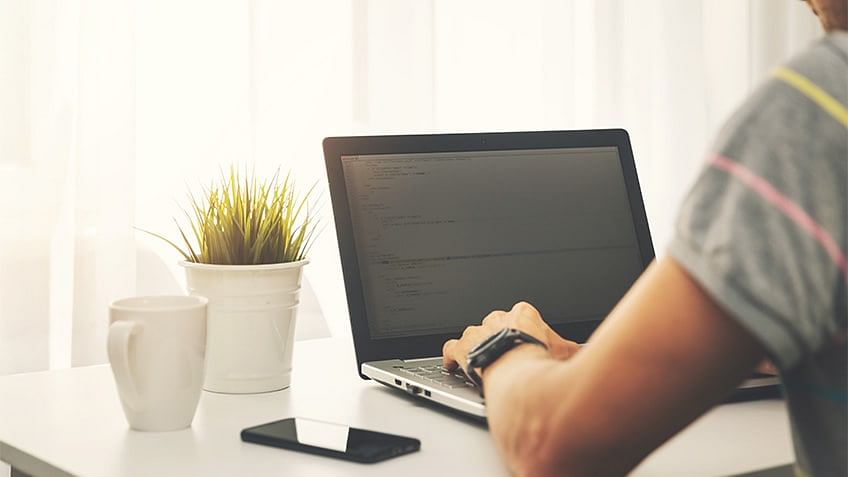
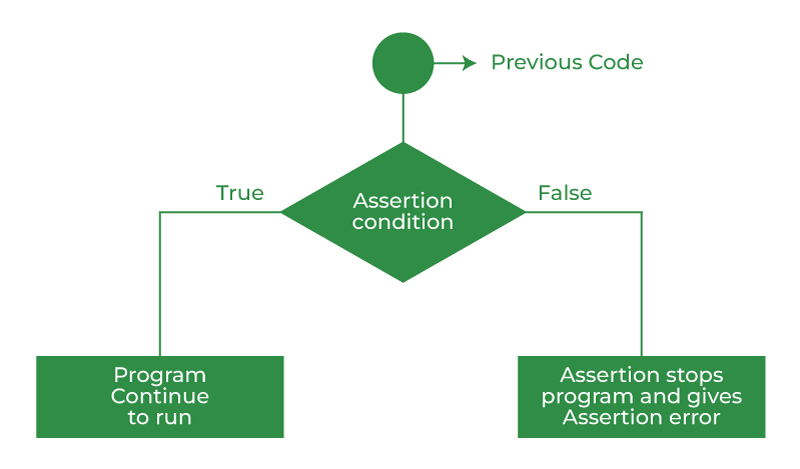
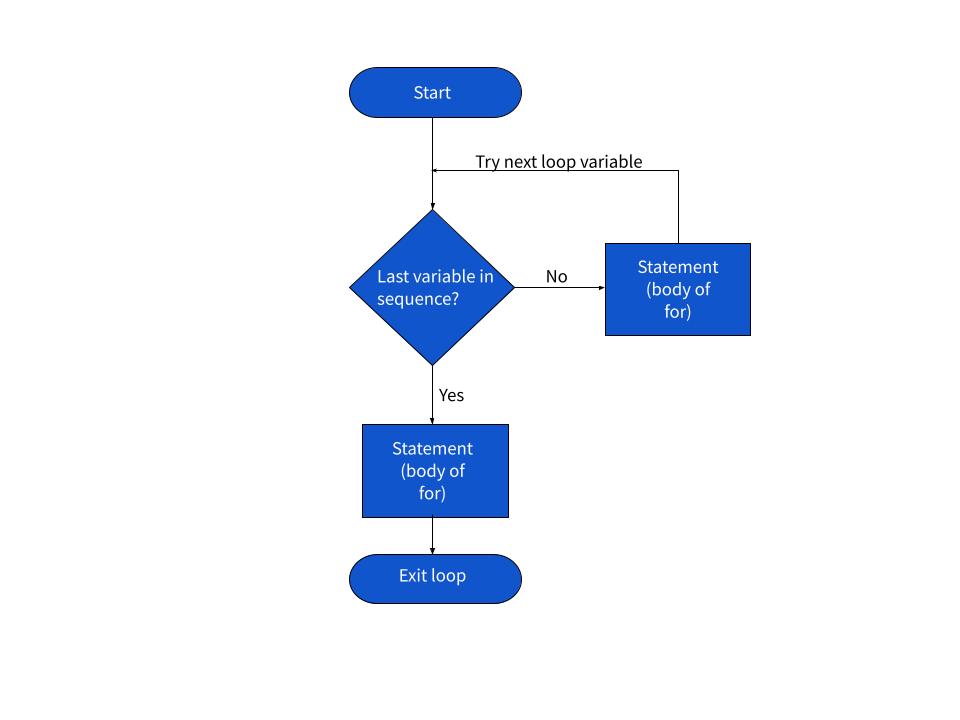
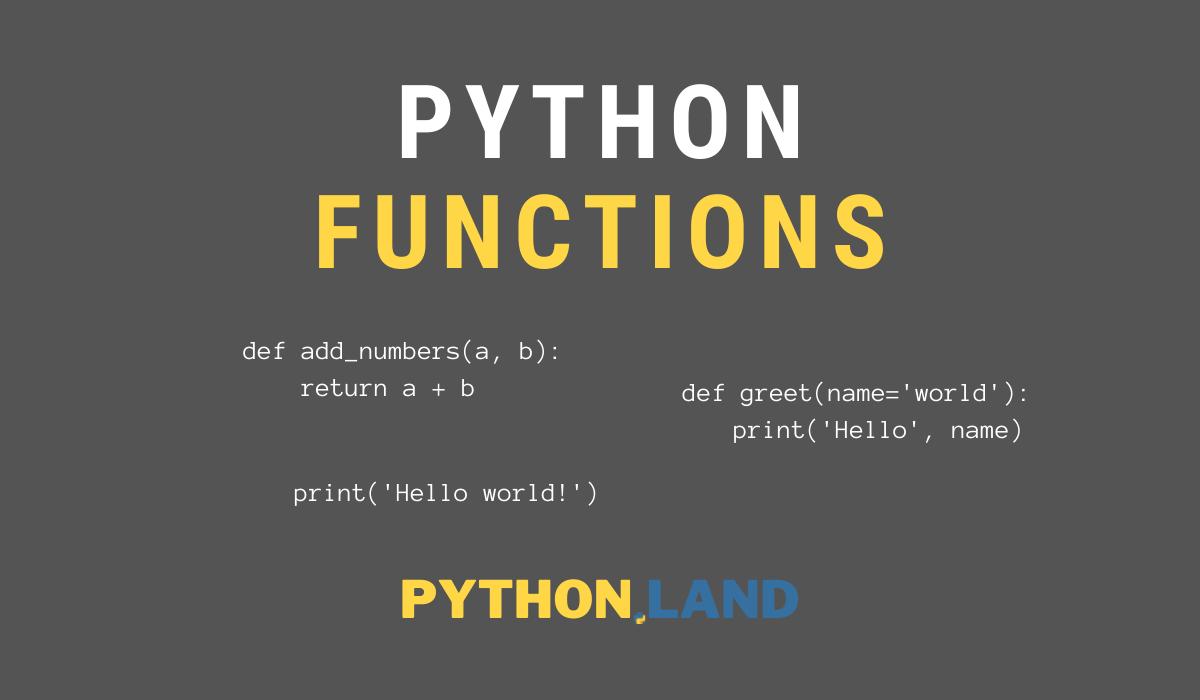

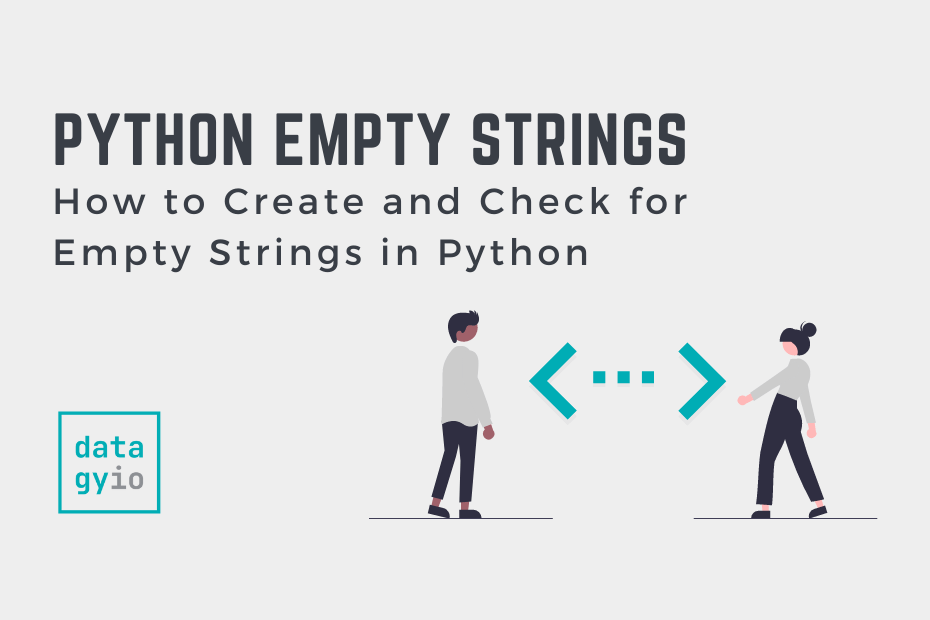
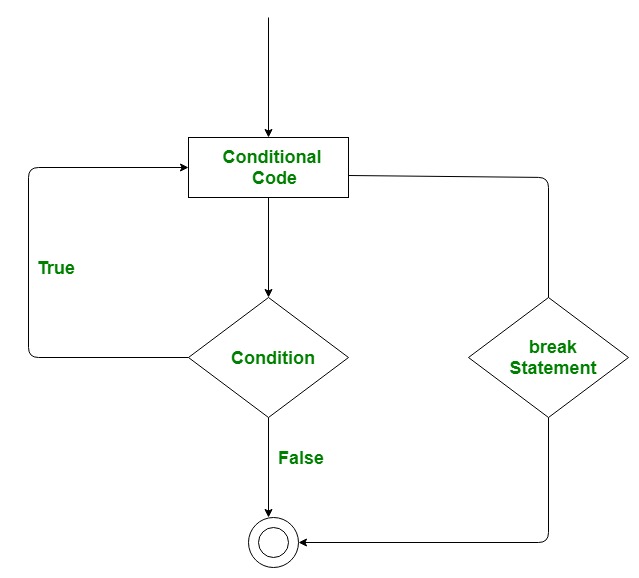

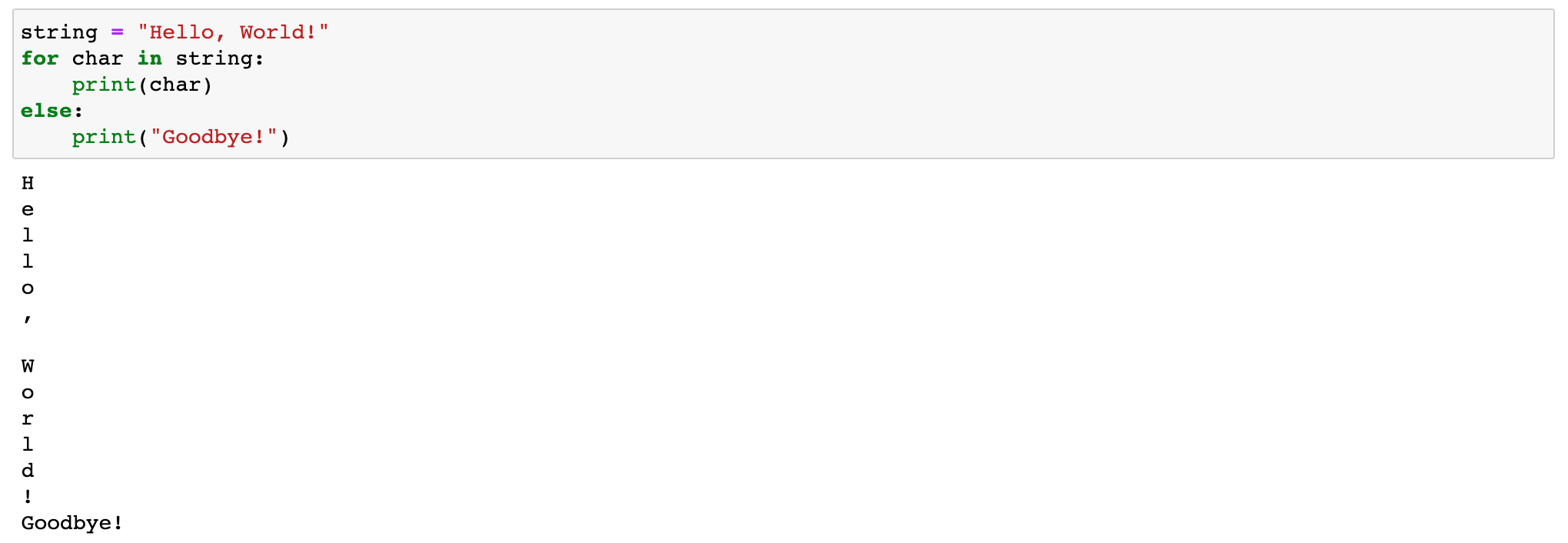
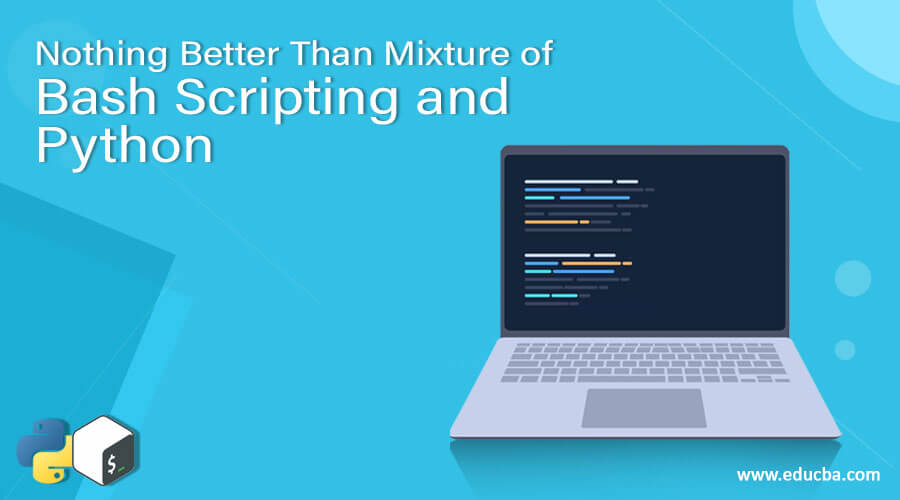

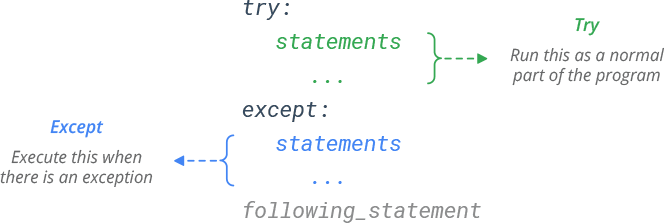

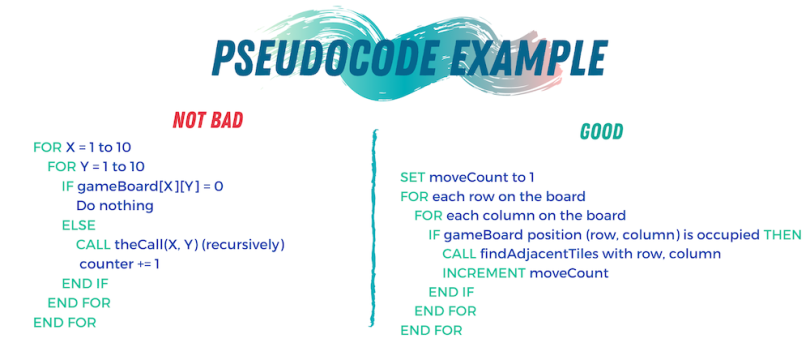
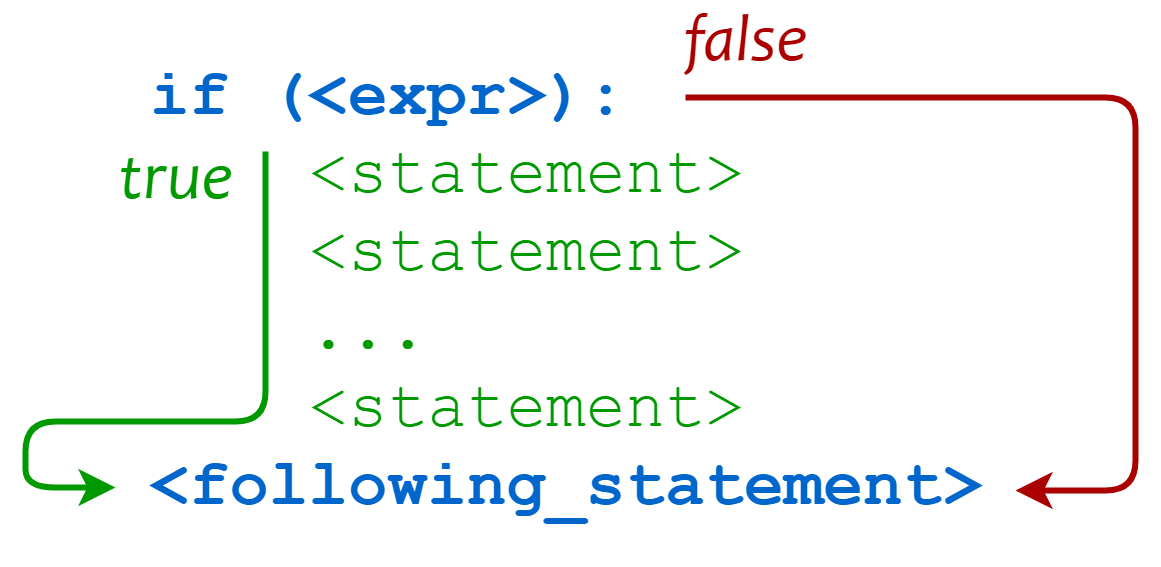
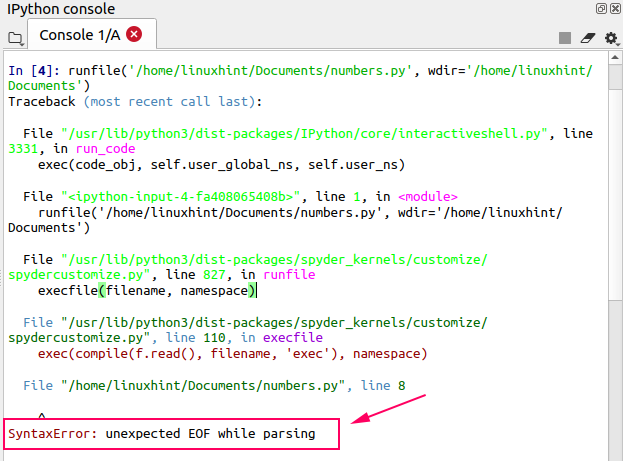
![SQLSERVER] Hướng dẫn sử dụng mệnh đề điều kiện if else trong sql server Sqlserver] Hướng Dẫn Sử Dụng Mệnh Đề Điều Kiện If Else Trong Sql Server](https://laptrinhvb.net/uploads/baiviet/images/IF-ELSE-FLOW-CHART.jpg)
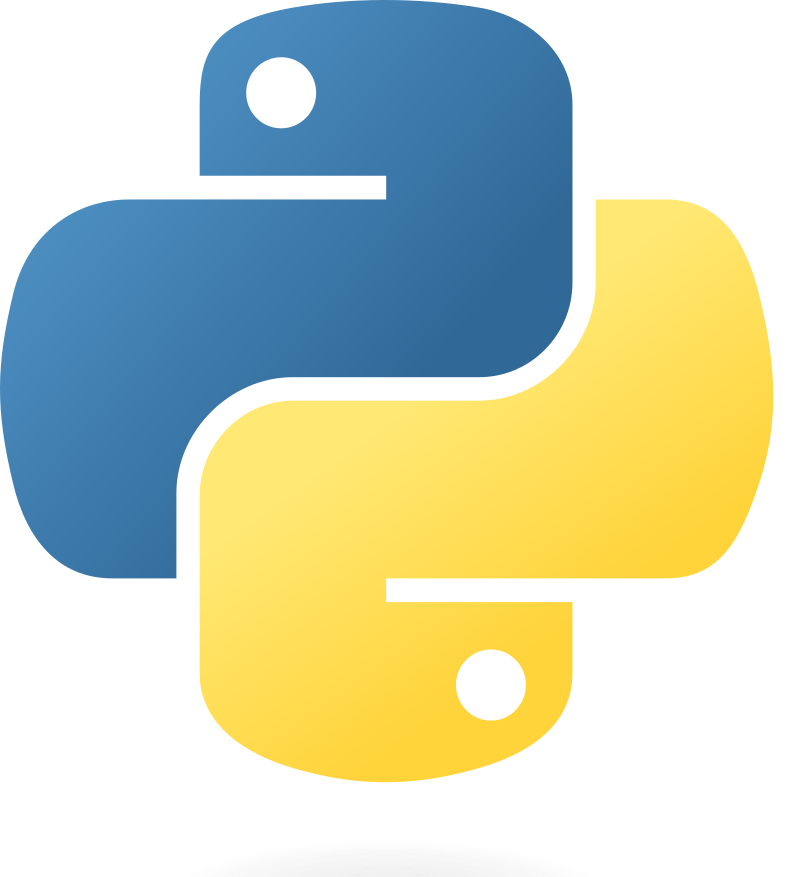
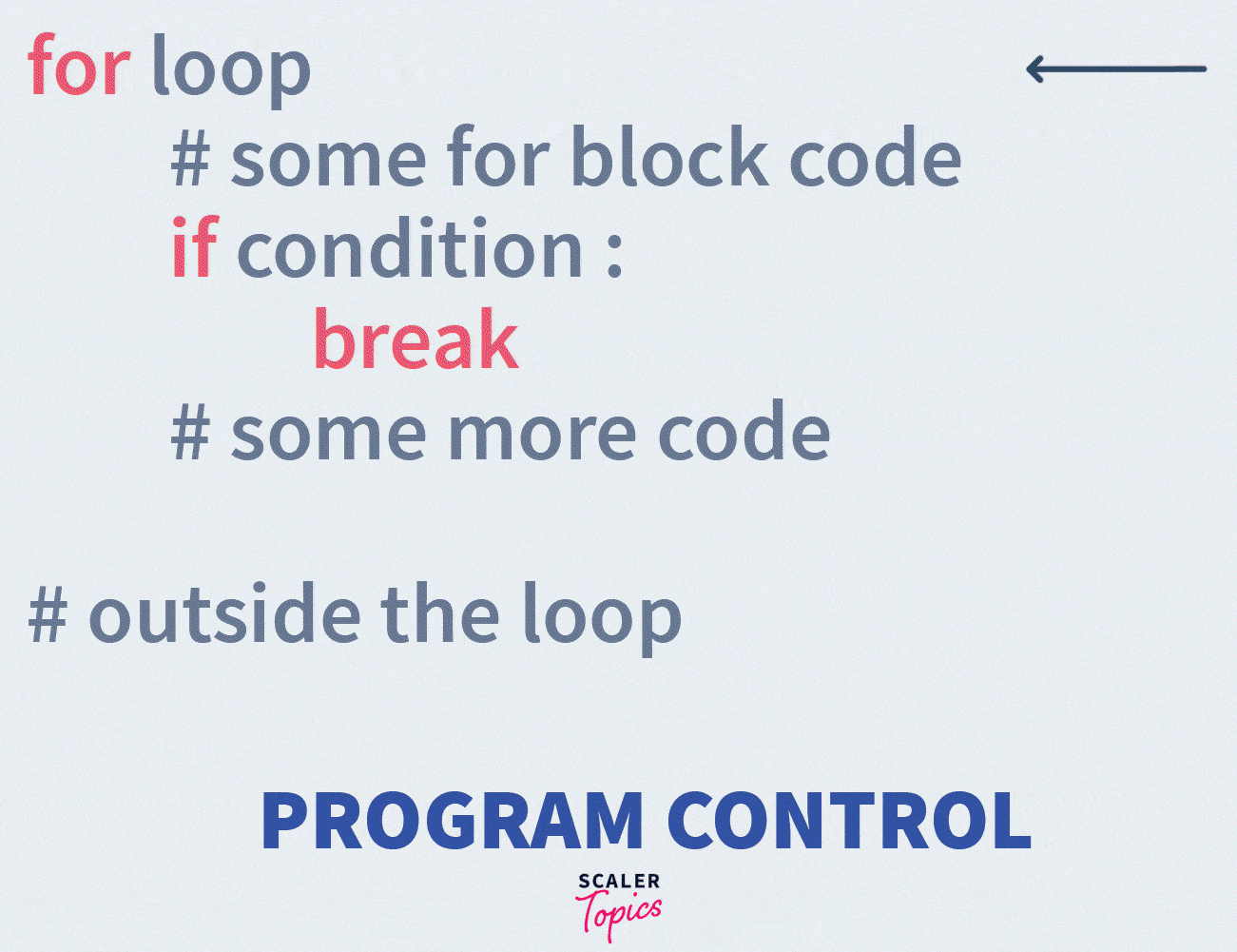
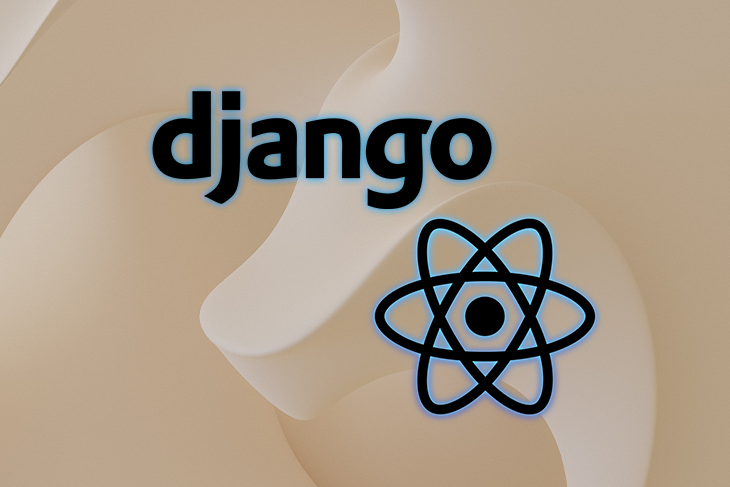

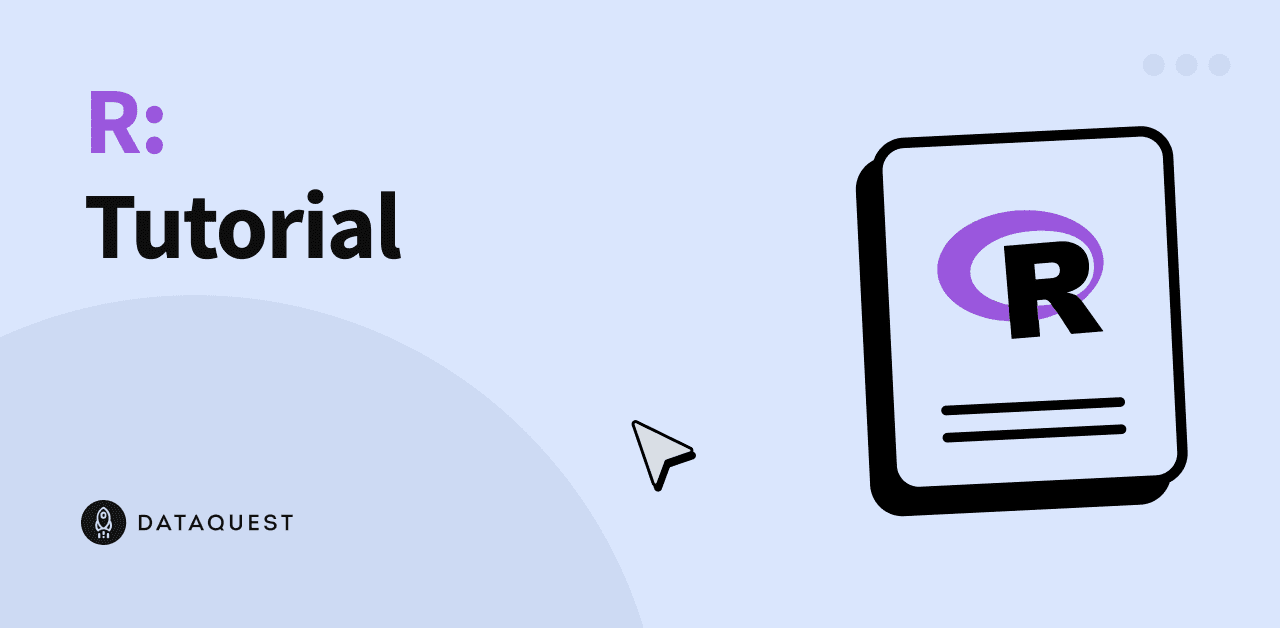
Article link: else do nothing python.
Learn more about the topic else do nothing python.
- The pass Statement: How to Do Nothing in Python
- 1.4 How to make “else:” do nothing? – Codecademy
- How do I get a python program to do nothing? – Stack Overflow
- Do Nothing Inside an if Statement in Python – Delft Stack
- 1 Easy Way To Fix The Else Do Nothing Python Error
- Python pass (Do Nothing): When And How To Use
- How to write an empty function in Python – pass statement?
- How to use pass statement in Python – Shiksha Online
- Python pass statement: When, why, and how to use it
- Python pass Keyword – W3Schools