You Cannot Call A Method On A Null Valued Expression
Null values are an integral concept in programming languages, representing the absence or lack of a value. In simple terms, a null value indicates that a variable or expression does not currently have a value assigned to it. This can occur for various reasons, such as uninitialized variables, empty containers, or when a value was deliberately set to null.
The Concept of Null Values
Definition of Null Values: Null values are special data types in programming languages that represent the absence of a value. They differ from other data types like integers or strings, as they do not hold any specific value. Instead, they act as placeholders or markers to indicate the lack of a value.
Purpose and Usage of Null Values: Null values serve several purposes in programming. They allow developers to distinguish between an intentional lack of value and an actual value. Additionally, null values can be used to indicate unknown or undefined values. This flexibility is especially useful in scenarios where certain data may not be available or applicable at a given time.
Handling Null Values in Programming Languages: Different programming languages handle null values in various ways. Some languages, like Java and C#, have explicit null value assignment. Other languages, like Python, use the “None” keyword to represent null values. Regardless of the language being used, handling null values is crucial to avoid null value exceptions and unexpected program behavior.
The Null Value Exception
Explanation of the Null Value Exception: A null value exception occurs when a method or operation is called on a null-valued expression. As mentioned earlier, null values do not hold any specific value, so attempting to perform actions on them can result in errors. The null value exception serves as a notification to the programmer that a null value was encountered where it should not have been.
Types of Null Value Exceptions: Null value exceptions can manifest in various forms, depending on the programming language and the specific operation being performed. Common null value exceptions include “NullPointerException” in Java, “ArgumentNullException” in C#, and “TypeError” in Python.
Causes of Null Value Exceptions: Null value exceptions can be caused by a variety of factors. Some common causes include:
1. Forgetting to initialize a variable before using it.
2. Receiving input that is expected to have a value but is null.
3. Cascading null values, where one null value results in subsequent null value exceptions.
4. Incorrectly handling or checking for null values in conditional statements.
Handling the Null Value Exception
Using Conditional Statements for Null Value Handling: One way to handle null value exceptions is by using conditional statements to check if a value is null before performing any operations on it. For example, in Java, you can use an “if” statement to check if a variable is null, and then decide whether to proceed with the operation or handle the null value gracefully.
Implementing Error Handling Mechanisms: Another approach to handling null value exceptions is by implementing error handling mechanisms in your code. This can include try-catch blocks, which allow you to catch and handle null value exceptions gracefully. By doing so, you can provide appropriate error messages or fallback solutions when encountering null values.
Utilizing Null Coalescing Operator: Some programming languages offer a null coalescing operator to simplify null value handling. This operator allows you to provide a default value or an alternative expression to be used when encountering null values. It eliminates the need for lengthy conditional statements and reduces the chances of null value exceptions.
Preventing Null Value Exceptions
Best Practices in Coding to Avoid Null Value Exceptions: Prevention is always better than cure. To avoid null value exceptions, follow these best practices:
1. Always initialize variables with appropriate default values.
2. Validate user input to ensure it does not contain null values.
3. Use conditional statements to check for null values before performing operations.
4. Implement proper error handling mechanisms to catch and handle null value exceptions.
Validating User Input to Avoid Null Values: When developing applications that rely on user input, it is essential to validate the input to avoid null values. This can be achieved by implementing form validation, restricting input types, and providing clear instructions to users.
Initializing Variables to Non-Null Values: To prevent null value exceptions, it is crucial to initialize variables with non-null values. By doing so, you can ensure that variables always have a valid value, reducing the chances of encountering null value exceptions.
Debugging Null Value Exceptions
Identifying Null Value Exceptions in Software Debugging: When encountering null value exceptions, debugging becomes essential. During the debugging process, you should track where and why null values are being assigned or passed into methods. This helps identify the root cause of the exception and formulate appropriate solutions.
Analyzing Stack Traces for Null Value Exceptions: Stack traces provide valuable information about the execution flow of a program and can help pinpoint where null value exceptions occur. By examining the stack traces, you can trace the sequence of method calls leading up to the exception and identify the source of the null value.
Evaluating the Code Flow for Potential Null Value Issues: Analyzing the code flow can help identify potential null value issues before they cause exceptions. By reviewing the logic and flow of your code, you can ensure that proper checks and handling mechanisms are in place to handle null values appropriately.
Real-Life Examples of Null Value Exceptions
Null Value Exceptions in Database Management Systems: Null value exceptions frequently occur in database management systems. For instance, if a query retrieves a null value from a database column and a method is then called on that null value, a null value exception will be raised. Proper exception handling and data validation are essential to prevent such issues.
Null Value Exceptions in Web Development: Web development often involves handling user input, which can contain null values. Without proper validation, these null values can cause exceptions when attempting to perform operations or store data. By validating input and using conditional statements, null value exceptions can be avoided.
Null Value Exceptions in Object-Oriented Programming: In object-oriented programming, null value exceptions can occur when accessing attributes or methods on objects that have not been properly initialized or assigned a value. By following best practices in object creation and structuring code, such exceptions can be minimized.
Resources for Handling Null Value Exceptions
Online Tutorials and Documentation: Many online tutorials and documentation resources provide guidance on handling null value exceptions in various programming languages. These resources can offer insights into best practices, specific language features, and techniques for avoiding and handling null value exceptions.
Programming Communities and Forums: Participating in programming communities and forums can provide valuable insights and assistance when dealing with null value exceptions. By engaging with experienced developers, you can learn from their expertise and gain valuable tips and tricks.
Stack Overflow Discussions and Solutions: Stack Overflow, a popular Q&A website for programmers, often contains numerous discussions and solutions related to null value exceptions. Searching for specific keywords like “Function PowerShell, PowerShell parameter, Null in PowerShell, Get-ExecutionPolicy, connect-exchangeonline you cannot call a method on a null-valued expressionyou cannot call a method on a null-valued expression” along with “null value exceptions” can lead to relevant discussions and solutions to specific issues.
You Cannot Call A Method On A Null-Valued Expression. Invalidoperation:…
How To Fix You Cannot Call A Method On A Null Valued Expression?
“You cannot call a method on a null valued expression” is a common error message that programmers encounter when working with programming languages like Java, Python, or C#. This error occurs when a method is being called on an object that has not been initialized or does not exist. In this article, we will explore the reasons behind this error and provide several solutions to fix it.
Understanding the Error:
To understand why this error occurs, it is important to grasp the concept of null values. In programming, null is a special value that represents the absence of a value or the lack of an object. When a null value is assigned to an object or variable, it means that the object or variable has not been given a valid value yet.
Methods, on the other hand, are blocks of code that perform specific tasks or operations on objects or variables. When a method is called, the code within the method is executed. However, if the object on which the method is being called is null (i.e., not initialized or does not exist), the error “You cannot call a method on a null valued expression” occurs.
Common Causes:
There are several common causes for this error:
1. Forgetting to Initialize Objects: In many cases, this error occurs when an object has not been initialized before a method is called on it. Initialization involves creating an instance of the object using the “new” keyword or assigning an existing object to a variable.
2. Null Assignments: Sometimes, developers inadvertently assign a null value to an object or variable, resulting in the error when a method is called on it.
3. Method Chaining: This error can also occur when using method chaining, where multiple methods are called sequentially. If one of the methods in the chain returns a null value, subsequent methods cannot be called on it, leading to the error.
Solutions:
Here are some solutions to fix the “You cannot call a method on a null valued expression” error:
1. Check Object Initialization: One of the first steps in resolving this error is to ensure that the object on which the method is being called has been properly initialized. Double-check the code to verify that the object has been created before the method invocation.
2. Verify Variable Assignments: If the object or variable is assigned a value, verify that it is not set to null. Assigning a valid value to the object or variable before calling the method should resolve the error.
3. Condition Checking: Use conditional statements, such as if-else, to check if the object is null before calling a method on it. This helps prevent the error by bypassing the method call if the object is null.
4. Null Checks for Method Chaining: When using method chaining, it is essential to incorporate null checks to ensure that subsequent methods are not called on null objects. This can be achieved by using the “if” statement to check if the object is null before proceeding with the method chaining.
5. Exception Handling: Implement proper exception handling techniques to gracefully handle null values and avoid the error. Catching the exception and providing an appropriate error message or fallback action can prevent program termination.
Frequently Asked Questions:
Q1. Why am I getting the “You cannot call a method on a null valued expression” error?
A1. This error occurs when a method is being invoked on an object that is null, meaning it has not been initialized or does not exist.
Q2. How can I fix this error if I forgot to initialize an object?
A2. Ensure that you initialize the object using the “new” keyword or assign an existing object to the variable before calling the method on it.
Q3. Can I use alternative solutions to handle null values?
A3. Yes, other solutions like using the Null Object Design Pattern or the Optional class (in Java) can also handle null values effectively and prevent this error.
Q4. Can I ignore this error and proceed with the program execution?
A4. It is generally not recommended to ignore this error, as it may lead to unexpected behavior or program crashes. It is best to address the error by implementing the appropriate fixes mentioned in this article.
Q5. Are there any tools available to detect null values and potential issues in my code?
A5. Yes, various code analysis tools and IDEs provide features to detect null values or potential null reference issues in your code. These tools can help identify the source of the error and suggest possible fixes.
Conclusion:
The “You cannot call a method on a null valued expression” error occurs when attempting to invoke a method on an object that is null. By following the solutions provided in this article, such as verifying object initialization, checking variable assignments, incorporating null checks in method chaining, and implementing exception handling techniques, you can effectively fix this error. Remember to pay attention to object initialization and be cautious about assigning null values to objects or variables. These practices, coupled with proper coding techniques, will ensure smooth execution of your program and eliminate this error.
What Is The Null Method In Powershell?
PowerShell, an efficient and versatile scripting language developed by Microsoft, provides users with a wide range of functions and methods to manage and automate various tasks on Windows operating systems. Among the myriad of methods available in PowerShell, the Null method holds a unique place due to its significance in handling and dealing with null values.
In programming, a variable with a null value refers to a variable that has not been assigned any value or contains no value at all. Null values are commonly encountered in different programming languages, including PowerShell. While handling null values can be challenging, PowerShell offers the Null method to simplify the process.
Understanding the Null Method:
The Null method in PowerShell is essentially a way to check if a variable contains a null value. The method can be applied to any variable, including strings, arrays, objects, and even custom types. By using the Null method, scriptwriters can effectively check if a variable contains a null value before proceeding with further operations.
The syntax for using the Null method is straightforward. It simply involves adding “.IsNull()” after the variable name, as shown in the following example:
$myVariable.IsNull()
If the variable contains a null value, the Null method will return “True,” indicating that the variable is indeed null. Conversely, if the variable contains a value, the method will return “False,” signifying that the variable is not null.
Utilizing the Null Method in PowerShell:
The Null method proves to be quite useful in various scenarios. For instance, when writing scripts that involve user input or fetching data from external sources, it is essential to handle potential null values properly.
By using the Null method, scripts can perform conditional checks and processes, ensuring that null values are dealt with appropriately. For instance, a script can prompt the user to enter valid input if a variable is found to be null, or skip a certain step in the script altogether if a null value is detected.
Moreover, the Null method can be effectively combined with other PowerShell cmdlets or operators to enhance its capability. For example, one can use the Null method in conjunction with the If-Else statement to construct conditional checks, as shown in the following code snippet:
if ($myVariable.IsNull()) {
Write-Host “Variable is null.”
} else {
Write-Host “Variable is not null.”
}
This allows scripts to perform different actions based on whether a variable contains a null value or not.
FAQs:
Q: How can I assign a null value to a variable in PowerShell?
A: In PowerShell, a variable can be assigned a null value using the “$null” keyword. For instance, $myVariable = $null would assign a null value to the variable $myVariable.
Q: Can I use the Null method on an entire array?
A: Yes, the Null method can be applied to an entire array. By using the method on an array variable, PowerShell will check if any element within the array is null.
Q: Can the Null method be used to handle exceptions?
A: While the Null method is not directly related to exception handling, it can aid in managing null values that might cause exceptions. By checking if a variable is null before performing specific operations, the Null method can help prevent null-related exceptions.
Q: Is the Null method exclusive to PowerShell?
A: The Null method itself is not exclusive to PowerShell. However, its usage and implementation may vary across different programming languages.
Q: Are there any potential drawbacks or limitations when using the Null method?
A: It is essential to note that the Null method in PowerShell only checks for null values; it does not return any additional information on the underlying cause of null. Therefore, using appropriate error-handling techniques alongside the Null method is advisable to ensure accurate and effective scripts.
In conclusion, the Null method in PowerShell provides a simple and effective way to check for null values in variables. By incorporating the Null method into scripts, developers can confidently handle null values and create robust and error-free automation processes.
Keywords searched by users: you cannot call a method on a null valued expression Function PowerShell, PowerShell parameter, Null in PowerShell, Get-ExecutionPolicy, connect-exchangeonline you cannot call a method on a null-valued expression
Categories: Top 89 You Cannot Call A Method On A Null Valued Expression
See more here: nhanvietluanvan.com
Function Powershell
PowerShell, developed by Microsoft, is a versatile and powerful scripting language that automates the management and configuration of Windows operating systems. With its vast array of built-in commands and extensibility, PowerShell is a must-have tool for system administrators and IT professionals. In this article, we will delve into the concept of functions in PowerShell, explore their usage and benefits, and provide answers to frequently asked questions.
Functions in PowerShell are similar to functions in other programming languages. They are self-contained blocks of code that perform a specific task when called upon. Functions help break down complex tasks into reusable and modular components, enhancing code clarity and maintainability. In PowerShell, functions are declared using the “function” keyword followed by the function name, and enclosed within curly braces.
One of the main advantages of using functions in PowerShell is the ability to encapsulate code logic. By defining functions, you can create custom commands that can be easily reused without rewriting the same code multiple times. This not only saves time but also reduces the chances of errors. Additionally, functions in PowerShell can accept parameters, enabling you to create more flexible and dynamic scripts.
When it comes to using functions in PowerShell, the possibilities are endless. You can create functions for any task, whether it’s automating system administration tasks, managing Active Directory, manipulating files and folders, or performing network operations. PowerShell functions can be used to query and manipulate data from various sources, including databases and web services, making it a versatile choice for automation tasks.
Functions in PowerShell can be defined at different scopes, such as script level, module level, or session level. This provides flexibility in how functions are organized and accessed within your scripts. In addition, PowerShell functions can be loaded into a script from external modules or libraries, expanding the capabilities of your scripts and saving you valuable time and effort.
FAQs:
Q1: How do I create a function in PowerShell?
A: To create a function in PowerShell, use the “function” keyword followed by the function name, and enclose the function code within curly braces. For example:
“`PowerShell
function MyFunction {
# Function code goes here
}
“`
Q2: How do I pass parameters to a PowerShell function?
A: PowerShell functions can accept parameters by specifying them within parentheses after the function name. For example:
“`PowerShell
function MyFunction ($Parameter1, $Parameter2){
# Function code using the parameters goes here
}
“`
Q3: Can I return values from a PowerShell function?
A: Yes, you can use the “return” keyword to specify the value that will be returned from a PowerShell function. For example:
“`PowerShell
function MyFunction {
# Function code goes here
return $Result
}
“`
Q4: Can PowerShell functions be used for remote administration?
A: Yes, PowerShell functions can be used for remote administration by leveraging the PowerShell Remoting feature. You can execute PowerShell commands or functions on remote machines using the “Invoke-Command” cmdlet, allowing you to automate tasks across multiple systems.
Q5: Are there any limits to the complexity of PowerShell functions?
A: PowerShell functions can handle complex tasks and have no inherent limits on their complexity. However, it is good practice to keep functions concise and focused on a specific task to maintain code readability and reusability.
In conclusion, functions in PowerShell provide a powerful tool for automating various tasks in Windows environments. By encapsulating code logic within functions, you can create reusable and modular components that enhance code clarity and maintainability. With the ability to accept parameters, functions in PowerShell offer flexibility and enable the creation of dynamic scripts. PowerShell functions can be used for a wide range of automation tasks, from system administration to data manipulation, making it an invaluable tool for IT professionals. So, unleash the power of PowerShell functions and take your automation efforts to the next level!
Powershell Parameter
PowerShell, developed by Microsoft, is a powerful automation and scripting tool that enables users to manage and control their computer systems more efficiently. One of the key features of PowerShell is its ability to handle parameters, which allow users to pass input values to a script or function. In this article, we will explore PowerShell parameters in depth, their usage, types, and common use cases.
Understanding PowerShell Parameters:
In PowerShell, parameters act as placeholders that accept input values while executing a script or function. They make PowerShell scripts more flexible and allow users to customize their scripts according to specific requirements. Parameters can be used to pass values of any data type, such as strings, integers, arrays, and even objects. The usage of parameters not only offers flexibility but also improves the reusability of scripts, as they can be easily modified by changing the parameter values.
Syntax and Declaration:
To declare a parameter in PowerShell, you can use the Param keyword followed by a list of parameters enclosed in parentheses. Each parameter is defined using a name, followed by its data type, and optionally, a default value assignment. The following example demonstrates the basic syntax of parameter declaration:
“`powershell
Param (
[Parameter(Mandatory=$true)]
[string]$Name,
[int]$Age = 0,
[switch]$IsActive
)
“`
In this example, `$Name` is a mandatory string parameter, `$Age` is an optional integer parameter with a default value of 0, and `$IsActive` is a Boolean switch parameter that can be either present or absent.
Types of PowerShell Parameters:
PowerShell offers various types of parameters to cater to different scenarios. Some of the commonly used parameter types are:
1. Mandatory Parameters: These parameters require input values and cannot be omitted while executing the script or function. They are denoted by the `[Parameter(Mandatory=$true)]` attribute in the declaration.
2. Optional Parameters: Optional parameters do not require input values and can be omitted if not needed. They can have default values assigned to them, which are used when no value is explicitly provided by the user.
3. Switch Parameters: Switch parameters are Boolean in nature and can be used to specify on/off states for certain actions. They are commonly used to enable or disable certain features of a script.
4. ValueFromPipeline Parameters: These parameters allow users to pass input values directly from the pipeline when executing a script or function. This provides a seamless way of using PowerShell cmdlets together, as the output of one cmdlet can be directly passed as input to another.
5. Array Parameters: Array parameters accept multiple input values of the same data type. They are useful when working with collections of data.
Using PowerShell Parameters:
Once parameters are declared, they can be accessed and utilized within the script or function. The values passed by the user, or the defaults, if not provided, are available in the corresponding parameter variables. For example, given the previous example, the parameter values can be accessed as follows:
“`powershell
Write-Host “Name: $Name”
Write-Host “Age: $Age”
Write-Host “IsActive: $IsActive”
“`
FAQs:
Q: Can I declare multiple parameters with the same data type?
A: Yes, you can declare multiple parameters with the same data type. For example, you can have multiple string parameters or integer parameters in a function or script.
Q: Are optional parameters always declared with default values?
A: No, optional parameters can be declared without default values. However, if no default value is assigned, the parameter will have a null or empty value if not provided by the user.
Q: How can I make a parameter mandatory after its initial declaration?
A: PowerShell does not support changing the mandatory attribute of a parameter dynamically. Instead, you can validate the parameter value manually within the script and prompt the user to provide a value if necessary.
Q: Can I use parameters in combination with pipelines?
A: Yes, parameters can be used together with pipelines. You can pass pipeline values to parameters using the `ValueFromPipeline` attribute, making scripts and functions more versatile and powerful.
Q: Are there any limitations on the number of parameters a script or function can have?
A: There is no fixed limit on the number of parameters a script or function can have. However, having an excessive number of parameters may make the code harder to read and maintain. It is recommended to keep the number of parameters to a reasonable level.
PowerShell parameters empower users to customize and personalize scripts and functions, adding flexibility and reusability to their PowerShell workflows. Understanding the different types of parameters and their usage can greatly enhance the efficiency and effectiveness of PowerShell scripting. Experiment with parameters and explore their vast possibilities to streamline your automation tasks and system management.
Images related to the topic you cannot call a method on a null valued expression
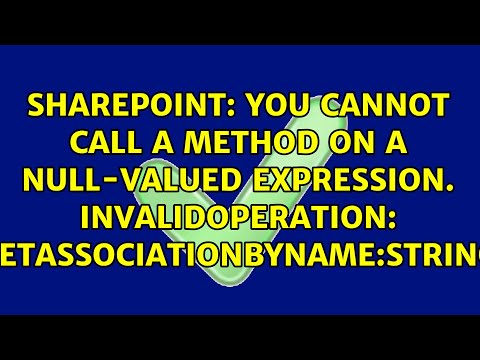
Found 11 images related to you cannot call a method on a null valued expression theme

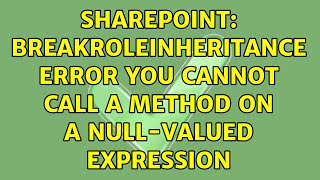
![You cannot call a method on Null valued expression at: $File = $Web.GetFile([Guid]$Result.Id) - YouTube You Cannot Call A Method On Null Valued Expression At: $File = $Web.Getfile([Guid]$Result.Id) - Youtube](https://i.ytimg.com/vi/YpnHaLN7zJU/maxresdefault.jpg)
![SOLVED] Powershell fails from Scheduled Task Solved] Powershell Fails From Scheduled Task](https://content.spiceworksstatic.com/service.community/p/post_images/0000389606/5e4c3adf/attached_image/Log.txt_-_Notepad-TCoffinger-20200218-022625.png)


Article link: you cannot call a method on a null valued expression.
Learn more about the topic you cannot call a method on a null valued expression.
- You cannot call a method on a null-valued expression
- You Cannot Call a Method on a Null Valued Expression: Guide
- You Cannot Call a Method on a Null Valued Expression: Guide
- Everything you wanted to know about $null – PowerShell | Microsoft Learn
- [SOLVED] Cannot Call a Null Method – Spiceworks Community
- Error during provisioning: “You cannot call a method on a null …
- You cannot call a method on a null-valued expression – Advice
- TFS Certificate – ou cannot call a method on a null-valued …
See more: nhanvietluanvan.com/luat-hoc