Writing To A Text File C#
Handling text file operations is a common task in programming, and C# provides several methods to read from and write to text files. In this article, we will explore the different ways to handle text files in C#, including opening and closing a text file, writing text to a file, reading text from a file, appending text to an existing file, handling exceptions, and best practices.
Overview of Text File Handling in C#
Text file handling in C# involves interacting with the file system, which includes tasks such as creating, opening, writing, reading, and closing text files. To perform these operations, C# provides the FileStream, StreamReader, and StreamWriter classes, which allow you to handle different aspects of text file manipulation.
Opening and Closing a Text File
Before performing any operations on a text file, you need to open it. To open a text file in C#, you can use the FileStream class. Alternatively, you can use the StreamReader or StreamWriter classes, which provide higher-level functionality and handle the file opening operation as well.
To open a file using the FileStream class, you need to provide the file path and specify the file mode, which can be FileMode.Create, FileMode.Open, FileMode.Append, or FileMode.CreateNew. Once you are done working with the text file, you should close it to release system resources.
Writing Text to a Text File
To write text to a text file in C#, you can use the StreamWriter class, which provides methods to write text characters, strings, and lines. First, you need to create an instance of the StreamWriter class by passing the file path and specifying whether you want to append or overwrite the file if it already exists.
Once the StreamWriter instance is created, you can use its various methods, such as Write, WriteAsync, WriteLine, and WriteLineAsync, to write text to the file. After writing data, make sure to flush the stream to ensure that all the data is written to the file. Finally, close the StreamWriter to release system resources.
Reading Text from a Text File
To read text from a text file in C#, you can use the StreamReader class. Similar to the StreamWriter class, you need to create an instance of the StreamReader by specifying the file path. After that, you can use the Read, ReadAsync, ReadLine, and ReadLineAsync methods to read text from the file.
When reading text from a file, you should check for the end of the file using the EndOfStream property or check if the returned value is null. After reading the data, close the StreamReader to release system resources.
Appending Text to an Existing Text File
To append text to an existing text file in C#, you can use the StreamWriter class along with the FileMode.Append file mode. This mode ensures that any data you write will be appended to the end of the file without overwriting its existing content.
To append text, create an instance of the StreamWriter class with FileMode.Append, and then use the Write, WriteAsync, WriteLine, or WriteLineAsync methods to write the text to the file. Finally, flush the stream and close the StreamWriter.
Handling Exceptions while Writing or Reading Text Files
When working with text files, it is essential to handle exceptions that may occur during reading or writing operations. Exceptions can be caused by various factors, such as incorrect file paths, insufficient permissions, or issues with disk IO.
To handle exceptions, you can use try-catch blocks. Place the file operations within the try block and catch any exceptions that may occur. Common exceptions you may encounter include FileNotFoundException, UnauthorizedAccessException, and IOException. Handle each exception appropriately, displaying an error message or taking corrective actions.
Best Practices for Writing and Reading Text Files
When working with text files in C#, it is essential to follow best practices to ensure efficient and error-free code. Here are some recommended practices:
1. Use using Statements: To ensure that resources are properly disposed of, use the using statement when working with StreamReader, StreamWriter, or FileStream. The using statement automatically calls the Dispose method, releasing system resources.
2. Error Handling: As mentioned earlier, always handle exceptions that may occur during file operations. This ensures that your program can handle unexpected situations gracefully and provides relevant error messages to users.
3. Close the File: After using a StreamReader or StreamWriter, remember to close the file by calling the Close method. This ensures that any pending data is written to the file and system resources are released.
4. Read/Write in Chunks: When dealing with large text files, it is recommended to read or write the data in smaller chunks instead of reading or writing the entire file at once. This approach reduces memory usage and improves performance.
5. Use Buffering: To improve performance, enable buffering when reading or writing large amounts of data from/to text files. Buffering reduces the number of disk IO operations by storing data in memory before writing it to the file.
Frequently Asked Questions (FAQs)
Q: How do I read a file in C#?
A: To read a file in C#, you can use the StreamReader class. Create an instance of the StreamReader by specifying the file path, and then use the Read, ReadLine, or ReadToEnd methods to read the file’s content.
Q: How do I write a text file in C#?
A: To write a text file in C#, you can use the StreamWriter class. Create an instance of the StreamWriter by specifying the file path and whether you want to append or overwrite the file. Then, use the Write, WriteLine, or other related methods to write text to the file.
Q: How do I append text to an existing text file in C#?
A: To append text to an existing text file in C#, you can use the StreamWriter class with FileMode.Append. This mode allows you to write data that will be appended to the end of the file without overwriting its existing content.
Q: How do I handle exceptions when working with text files in C#?
A: Use try-catch blocks to handle exceptions that may occur during file operations. Common exceptions include FileNotFoundException, UnauthorizedAccessException, and IOException. Handle each exception appropriately, displaying error messages or taking corrective actions.
Q: What are the best practices for reading and writing text files in C#?
A: Some best practices for reading and writing text files in C# include using using statements, proper error handling, closing files after use, reading or writing in chunks for large files, and enabling buffering for improved performance.
In conclusion, handling text files in C# involves opening, writing, reading, and closing operations. C# provides classes like FileStream, StreamReader, and StreamWriter to facilitate these tasks. Following best practices and handling exceptions will help ensure efficient and error-free text file operations in C#.
C Tutorial #31 – Fopen Create Text File
How To Write In A Text File In C?
When it comes to working with files in the C programming language, one of the most common tasks is writing data to a text file. Whether you are handling user input, storing results, or simply logging information, being able to write to a text file is essential. In this article, we will dive into the details of how to write in a text file using C, covering everything from file handling basics to advanced techniques.
Understanding File Handling in C
Before we can begin writing in a text file, it is crucial to grasp the basics of file handling in C. File handling involves a series of operations such as opening, closing, reading, and writing files. To write in a text file, you should follow these general steps:
1. Open the file: First, you need to open the file in write mode using the `fopen()` function. This function takes two arguments: the file path and the mode in which the file should be opened. For writing purposes, “w” is the appropriate mode. If the file does not exist, it will be created. However, if it exists, its contents will be truncated.
Example:
“`c
FILE *file = fopen(“example.txt”, “w”);
“`
2. Write data to the file: After successfully opening the file, you can use the `fprintf()` function to write data. It is similar to the `printf()` function but takes an additional argument specifying the file to write to. When using `fprintf()`, you should provide the file pointer obtained from the `fopen()` function.
Example:
“`c
fprintf(file, “This is the data that will be written to the file.”);
“`
3. Close the file: Once you are done writing the data, it is essential to close the file using the `fclose()` function. This step ensures that any updates are properly saved and releases the associated resources.
Example:
“`c
fclose(file);
“`
Handling Errors
When working with files, it is crucial to handle potential errors gracefully. In C, you can check for errors during file handling by examining the return value of `fopen()` and `fprintf()`. If either function fails, they will return NULL. You can then use the `perror()` function to print an error message describing the encountered issue. Additionally, you should always check if the file pointer is NULL before performing any operations on it.
Example:
“`c
FILE *file = fopen(“example.txt”, “w”);
if (file == NULL) {
perror(“Error opening the file”);
return 1;
}
fprintf(file, “This is the data that will be written to the file.”);
if (fprintf(file, “This is some additional data”) < 0) {
perror("Error writing to the file");
return 1;
}
fclose(file);
```
Appending Data to an Existing File
In some cases, you might want to append data to an existing file without overwriting its contents. Instead of using the "w" mode in `fopen()`, you should use the "a" mode. This mode allows you to write data at the end of the file, preserving the existing content.
Example:
```c
FILE *file = fopen("example.txt", "a");
```
Advanced Techniques: Writing Structured Data
While writing simple strings to a text file is straightforward, more complex data structures, such as arrays or structures, require additional considerations. One common approach is to iterate through the data and write each element individually. For instance, if you have an array of integers, you can loop through the array and write each element to the file.
Example:
```c
int numbers[] = {1, 2, 3, 4, 5};
for (int i = 0; i < sizeof(numbers) / sizeof(numbers[0]); i++) {
fprintf(file, "%d\n", numbers[i]);
}
```
Frequently Asked Questions (FAQs)
Q: Can I write to a file in C without overwriting its contents?
A: Yes, you can open a file in "a" mode using `fopen()` to append data to an existing file without overwriting its contents.
Q: How do I handle errors when writing to a file in C?
A: You can check for errors by examining the return value of `fopen()` and `fprintf()`. If either function fails, they will return NULL. You can then use `perror()` to print an error message.
Q: Can I write complex data structures, such as arrays or structures, to a text file in C?
A: Yes, you can iterate through the data structure and write each element individually using `fprintf()`.
Q: How do I ensure the data is properly saved and the file is closed?
A: Always close the file using `fclose()` after you finish writing data. This ensures that any updates are saved, and resources are released.
In conclusion, knowing how to write in a text file is an essential skill for any C programmer. By grasping the fundamentals of file handling, error handling, and advanced techniques, you can effectively write data to text files with confidence. Remember to handle errors gracefully, close files after writing, and explore advanced techniques to accommodate various data structures. Happy coding!
Can You Write To A File In C?
When it comes to the C programming language, file handling is a crucial aspect. It allows you to read and write data to and from files, making it possible to store and retrieve information on a more permanent basis. In this article, we will explore the process of writing to a file in C, discussing various techniques and their implementation.
Writing to a file in C involves a series of steps, starting from opening a file to writing data into it and eventually closing the file. Let’s dive into each step in detail.
Step 1: Opening a File
The first step in writing to a file is to open it. The `fopen()` function is used for this purpose. It requires two arguments: the name of the file you want to open and the mode in which you want to open the file. The mode can be “w” for writing, “a” for appending, “r+” for reading and writing, and more.
Here’s an example of opening a file in write mode:
“`c
FILE *filePointer;
filePointer = fopen(“data.txt”, “w”);
“`
In this example, we declare a pointer to a `FILE` type structure named `filePointer`. We then use `fopen()` to open the file named “data.txt” in write mode.
Step 2: Writing to a File
Once the file is opened, you can use the `fprintf()` or `fputc()` functions to write data into it. The `fprintf()` function allows you to write formatted data, similar to the `printf()` function. On the other hand, `fputc()` writes a single character at a time.
Here’s an example of using both of these functions:
“`c
FILE *filePointer;
filePointer = fopen(“data.txt”, “w”);
fprintf(filePointer, “Hello, world!\n”);
fputc(‘C’, filePointer);
fclose(filePointer);
“`
In this example, we write the string “Hello, world!” to the file using `fprintf()`. Then, we use `fputc()` to write the character ‘C’. Lastly, we close the file using the `fclose()` function.
Step 3: Closing the File
After you finish writing to a file, it’s essential to close it using the `fclose()` function. This ensures that all data is saved and any resources associated with the file are released.
Here’s an example of closing a file:
“`c
fclose(filePointer);
“`
That’s it! Your file has been written and safely closed.
File Writing FAQs
Q: Can I write to an existing file in C?
A: Yes, you can write to an existing file in C. By using the appropriate file opening mode, such as “w” for write or “a” for append, you can write data to an existing file or create a new one.
Q: How can I write data to a new line in a file?
A: To write data to a new line in a file, you can use the `\n` character to insert a newline or use `fprintf()` to write a formatted string ending with `”\n”`. For example:
“`c
fprintf(filePointer, “This is a new line.\n”);
“`
Q: What happens if I attempt to write to a file that does not exist?
A: If you try to open a file that does not exist and use the “w” mode, a new file with that name will be created. If you open a file that does not exist using the “r” mode, the `fopen()` function will return `NULL`, indicating an error. It’s crucial to handle such errors in your code.
Q: Is it possible to write binary data to a file in C?
A: Yes, it is possible to write binary data to a file in C. By opening the file in binary mode using `”wb”` as the mode argument in `fopen()`, you can write binary data using functions like `fwrite()`, which is designed specifically for binary file writing.
In conclusion, writing to a file in C is a fundamental aspect of file handling. By following the necessary steps of opening, writing, and closing a file, you can effectively write data to files and store information persistently. Remember to handle errors appropriately and ensure that your data is correctly formatted for the desired purpose.
Keywords searched by users: writing to a text file c# Read file in C, Read text file in C, Write file txt in C, Output file c, Write file in C, Write line to file in c, Read file C++, Fscanf() in C
Categories: Top 39 Writing To A Text File C#
See more here: nhanvietluanvan.com
Read File In C
Introduction
Reading files is a crucial aspect of programming, especially when dealing with data input and manipulation. In the C programming language, the process of reading data from a file is essential for tasks such as data analysis, file parsing, and more. In this article, we will explore the various techniques and functions available in C for reading files, discussing their syntax, usage, and best practices.
Understanding File Pointers
Before diving into the specifics of reading files in C, let’s first grasp the concept of file pointers. A file pointer is a data type that points to the specific location within a file where the next operation (such as read or write) will occur. It keeps track of the current position within the file, enabling efficient file handling.
Opening a File for Reading
To read a file in C, the first step is to open it using the `fopen()` function. The function takes two parameters: the file name (or path) and the file mode. The file mode for reading is denoted by “r”, indicating that the file will be opened in read-only mode.
“`
FILE *fp;
fp = fopen(“filename.txt”, “r”);
“`
Reading Characters
To read characters from a file, we use the `fgetc()` function. It reads the next character from the file associated with the given file pointer and returns it as an `int`. Keep in mind that `fgetc()` returns `EOF` (End-of-File) when there are no more characters to read.
“`
int c;
while ((c = fgetc(fp)) != EOF) {
// Process the character
printf(“%c”, c);
}
“`
Reading Lines
When dealing with line-based file processing, the `fgets()` function is very useful. It reads a line from the file and stores it into a string. The function takes three parameters: the string buffer, the maximum number of characters to read (including the newline character), and the file pointer.
“`
char line[100];
while (fgets(line, sizeof(line), fp)) {
// Process the line
printf(“%s”, line);
}
“`
Reading Formatted Data
If the file contains structured data, we can use formatted input functions like `fscanf()` to read specific data types. This function reads input from a file, interpreting the input according to a specified format. For instance, to read an integer from a file, we can use the following code:
“`
int num;
fscanf(fp, “%d”, &num);
printf(“The number is: %d”, num);
“`
Closing the File
After all the necessary operations, it is crucial to close the file using the `fclose()` function. This ensures that any pending data is written and releases any system resources associated with the file.
“`
fclose(fp);
“`
FAQs
Q: Can I read multiple files simultaneously?
A: Yes, you can open multiple files concurrently by utilizing multiple file pointers and employing them independently.
Q: How can I check if a file has been successfully opened?
A: The `fopen()` function returns a file pointer, which will be `NULL` if the file opening failed. It is good practice to check for the validity of the file pointer using an if condition, like so:
“`
if (fp == NULL) {
printf(“Failed to open the file!”);
exit(1);
}
“`
Q: What happens if I try to read from a non-existent file?
A: If you attempt to read from a file that does not exist, the `fopen()` function will return `NULL`, indicating a failed file opening. It is crucial to handle this scenario by checking if the file pointer is `NULL` and taking appropriate action.
Q: Can I read binary files with the techniques mentioned above?
A: Yes, the approaches discussed in this article can be used to read both text and binary files. However, when reading binary files, it is essential to ensure that you handle the data types correctly to avoid any complications.
Q: How can I rewind the file pointer to the beginning of the file?
A: If you want to process the file multiple times or if you need to read the file from the beginning again, you can use the `rewind()` function, which resets the file pointer to the beginning of the file.
Conclusion
Reading files in C is a fundamental skill that every programmer should master. Whether you are working with text or binary files, understanding file pointers and utilizing functions like `fgetc()`, `fgets()`, and `fscanf()` can greatly enhance your ability to handle data from external sources effectively. By following the guidelines and best practices outlined in this article, you are on your way to becoming a proficient file reader in C.
Read Text File In C
In C programming language, reading a text file is a common operation that allows developers to access and manipulate data stored within files. Being able to read files is essential for tasks such as data processing, configuration management, and even creating simple text-based games. In this article, we will explore various techniques and functions required to read a text file in C, along with some frequently asked questions that may arise during the process. So let’s dive in and discover the ins and outs of reading text files in C!
I. Opening a Text File
Before reading a text file, it is crucial to open it. In C, the `fopen()` function is used to open a file, and it returns a file pointer that will be used to perform file input and output operations. The basic syntax for opening a text file is as follows:
“`c
FILE *fp;
fp = fopen(“filename.txt”, “r”);
“`
In the example above, we declare a file pointer `fp` and open the file named `filename.txt` in read mode (`”r”`). The file mode can be `”r”` for read, `”w”` for write, `”a”` for append, and more.
II. Reading File Contents
Once the text file is successfully opened, we can read its content using various C standard library functions. The most commonly used functions for reading text files include `fgets()`, `fscanf()`, and `fgetc()`.
1. fgets()
The `fgets()` function allows us to read a single line of text from a file. The syntax for using `fgets()` to read a file line by line is shown below:
“`c
char buffer[255];
while (fgets(buffer, sizeof(buffer), fp) != NULL) {
// process the line stored in the buffer
}
“`
In the code snippet above, `fgets()` reads a line from the file pointed to by `fp` into the `buffer` array. The `sizeof(buffer)` specifies the maximum number of characters to read. We repeat this process until `fgets()` returns `NULL`, indicating that the end of the file has been reached.
2. fscanf()
The `fscanf()` function allows us to read formatted data from a file, which can be particularly useful for extracting specific information. For instance, let’s say we have a file named `data.txt` containing integers separated by spaces:
“`c
int num1, num2, num3;
fscanf(fp, “%d %d %d”, &num1, &num2, &num3);
“`
In the above example, `fscanf()` reads three integers from the file pointed by `fp` and stores them in `num1`, `num2`, and `num3` respectively. The format string `”%d %d %d”` indicates that we expect three integers separated by spaces.
3. fgetc()
The `fgetc()` function reads a single character from a file. This function can be used when we need granular control over file reading, like processing file content character by character. The basic usage of `fgetc()` is as follows:
“`c
char ch;
while ((ch = fgetc(fp)) != EOF) {
// process the character ‘ch’
}
“`
In this example, `fgetc()` reads a character from the file pointed by `fp` until it reaches the end-of-file (EOF) marker.
III. Closing a Text File
After we have finished reading the text file, it is crucial to close it properly using the `fclose()` function. Closing the file ensures data integrity and frees system resources. The syntax for closing a file is straightforward:
“`c
fclose(fp);
“`
Here, `fp` is the file pointer that we obtained when opening the file using `fopen()`.
FAQs:
Q1. What should I do if the file I am trying to read does not exist?
If the file you are trying to open does not exist, the `fopen()` function will return `NULL`, indicating that the file could not be opened. It is crucial to handle this scenario by checking if the file pointer is `NULL` before proceeding further.
Q2. How can I detect if the end of the file has been reached?
To detect the end-of-file marker while reading a file, you can check if the return value of the file reading function is `NULL` or `EOF`, depending on the function being used (`fgets()`, `fscanf()`, or `fgetc()`). If the return value is `NULL` or `EOF`, it indicates that the end of the file has been reached.
Q3. Can I read a text file character by character using `fscanf()`?
While `fscanf()` is primarily used for formatted reading, it is not suitable for reading files character by character. The `fgetc()` function should be used instead to read a text file character by character.
In conclusion, reading a text file in C is an essential skill for any C programmer. Understanding how to open, read, and close files, along with the different functions available, allows developers to access and manipulate file content effectively. With the insights provided in this article, you are now equipped with the knowledge required to read text files in C programming. Happy coding!
Images related to the topic writing to a text file c#
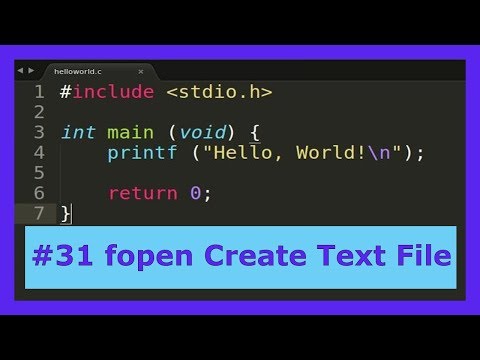
Article link: writing to a text file c#.
Learn more about the topic writing to a text file c#.
- C Write Text File – Learn C Programming from Scratch
- C Files I/O: Opening, Reading, Writing and Closing a file
- Write to .txt file? – Stack Overflow
- C – File I/O – Tutorialspoint
- C Programming – File Input/Output
- C Files I/O: Opening, Reading, Writing and Closing a file
- C – File Handling – Read and Write Integers – C Programming
- Reading & Writing to Text Files in C Programming – Study.com
- C Programming – File Input/Output
- Write Numerical or Text Data to File in C Programming …
- Reading & Writing to Text Files in C Programming – Study.com
- Read and write to text file in C – OpenGenus IQ
See more: https://nhanvietluanvan.com/luat-hoc