Write To A Text File C#
Introduction:
Text files serve as an essential tool for storing and managing data in various programming languages. In C#, developers can efficiently write to text files using built-in file handling mechanisms. This article will delve into the advantages of writing to a text file in C#, understanding file handling mechanisms, writing text to a file using the StreamWriter class, appending text to an existing file, handling exceptions, and best practices to consider. We will also touch upon related topics such as reading files in C, C++, and other aspects of file handling in C.
Advantages of Writing to a Text File in C#:
1. Data Persistence: By writing data to a text file, you ensure its durability and longevity, as the data persists even after the execution of the program. It allows for easy data retrieval at a later stage, securing critical information.
2. Portability and Compatibility: Text files are platform-independent and can be easily shared across different devices and operating systems. This makes them ideal for data exchange between various applications and environments.
3. Human-Readable Format: Text files store data in plain text format, making them easily readable by humans. This attribute is particularly useful when dealing with configurations, logs, or any other text-based records that require manual inspection or modification.
Understanding File Handling Mechanisms in C#:
Before diving into writing to a text file in C#, it is crucial to understand the underlying file handling mechanisms. These mechanisms involve opening, reading, writing, and closing files.
1. Opening a File: In C#, you can open a file using the File.Open() method, which returns a FileStream object. This provides access to the file, allowing you to read from or write to it.
2. Reading a File: Reading from a file involves creating a StreamReader object, which allows you to retrieve content from the file line by line or character by character.
3. Writing to a File: Writing to a file in C# is facilitated by employing the StreamWriter class. It exposes methods such as Write(), WriteLine(), and AppendLine() to write character strings or specific data types into a file.
Writing Text to a File using StreamWriter class in C#:
The StreamWriter class is a useful tool for writing text to a file in C#. It offers various techniques to tailor the writing process to the specific needs of a program.
To write text to a file using the StreamWriter class, follow these steps:
1. Create an instance of the StreamWriter class, specifying the file path and name.
2. Use the StreamWriter object’s methods (e.g., Write(), WriteLine(), AppendLine()) to write desired content to the file.
3. Dispose of the StreamWriter object to release system resources and close the file.
Appending Text to an Existing File in C#:
Appending text to an existing file is a common requirement in many scenarios. C# provides the StreamWriter class with a constructor overload that allows appending text rather than overwriting the existing content.
To append text to an existing file using StreamWriter in C#, use the following steps:
1. Define the StreamWriter object with the appropriate file name and path, and set the append parameter to true.
2. Use the StreamWriter object’s methods to write or append the desired text to the file.
3. Dispose of the StreamWriter object after writing is complete.
Handling Exceptions while Writing to a Text File in C#:
When dealing with file operations, it is essential to handle exceptions effectively. Failure to do so may result in data loss, security vulnerabilities, or unexpected program behavior. Common exceptions to consider while writing to a text file include FileNotFoundException, UnauthorizedAccessException, and IOException.
To handle exceptions while writing to a text file in C#, use try-catch blocks and implement appropriate error handling logic. For example, alert users of any failures and provide options for retrying, logging errors, or gracefully terminating the program.
Best Practices and Considerations when Writing to a Text File in C#:
1. Use Using Statements: Wrap file handling objects (e.g., StreamWriter) within a “using” statement to ensure proper disposal of resources and automatic closure of the file.
2. Avoid Frequent File Access: Minimize unnecessary file operations to improve performance and reduce system overhead. Batch write operations or use buffering techniques instead.
3. Validate Inputs: Validate user inputs or data before writing them to a file to prevent data corruption, file injection attacks, or undesired behavior.
4. Handle Encoding: Ensure proper encoding when writing to a file to cater to internationalization and prevent character encoding issues.
FAQs:
Q1: How can I read a file in C?
A1: In C, you can read files using the standard library function “fopen” to open a file, “fread” or “fscanf” to read file contents, and “fclose” to close the file.
Q2: How do I read a text file in C++?
A2: In C++, file reading can be achieved using the “ifstream” class or “fstream” class. Open the file using the appropriate constructor, read line by line using “getline”, and close the file after reading.
Q3: How do I write a text file in C?
A3: To write a text file in C, open the file using “fopen” with appropriate file mode, write content using “fprintf” or “fwrite” functions, and finally close the file using “fclose”.
Q4: How can I write a line to a file in C?
A4: In C, you can write a line to a file using the “fprintf” function with a newline character (“\n”) or the “fputs” function to append a string.
Q5: How can I write to a file in C on Linux?
A5: Writing to a file in C on Linux is similar to writing on other platforms. Use the standard C file handling functions such as “fopen”, “fwrite”, and “fclose” to open, write, and close the file.
Conclusion:
Writing to a text file in C# offers numerous advantages, from data persistence and portability to human-readable formatting. Understanding file handling mechanisms and utilizing classes like StreamWriter empowers developers to efficiently write text to files. By considering exceptions, best practices, and related topics like reading files in C and C++, one can create robust and reliable file writing functionality in their C# applications.
#29: C File Handling | C Programming For Beginners
How To Write In A Text File In C?
C is a powerful and widely-used programming language that allows you to create a variety of applications. One common task that often arises when working with C is writing data to a text file. Being able to write to a text file is essential as it enables you to store and preserve information for future reference. In this article, we will explore the process of writing in a text file using C, covering different aspects and providing a comprehensive guide for beginners.
Understanding the Basics:
Before diving into the specifics of writing in a text file with C, it is crucial to grasp the fundamental concepts involved. A text file is an ordered sequence of characters, often used to store plain text data. Each character is represented using its corresponding ASCII value, enabling the computer to understand and process the data properly.
In C, managing file operations involves using a file pointer, which serves as a reference to the text file we want to read from or write to. A file pointer is essentially a variable of type `FILE`, which allows us to perform various operations on the file, such as opening, closing, reading, or writing data.
Writing to a Text File:
To write data to a text file in C, we typically follow a specific set of steps. Let’s explore each step in detail:
1. Open the file:
Before writing to a text file, we need to open it for writing purposes using the `fopen()` function. This function takes two parameters: the name of the file we wish to open and the mode in which we want to open it. For writing, we use the mode “w” or “wt” (text mode). For instance, to open a file named “data.txt” for writing, we can use the following line of code:
“`c
FILE *filePtr = fopen(“data.txt”, “w”);
“`
2. Check if the file was opened successfully:
It is crucial to check if the file was opened successfully before performing any operations. The `fopen()` function returns a `NULL` pointer if it fails to open the file. To handle such cases, we can use an `if` condition to check the returned value of `fopen()`:
“`c
if (filePtr == NULL) {
printf(“Error opening file!”);
// appropriate error handling
}
“`
3. Write data to the file:
Once the file is successfully opened, we can use the `fprintf()` function to write data to the file. This function takes the file pointer and the data to be written as parameters. For example, to write the string “Hello, World!” to the file, we can use the following line of code:
“`c
fprintf(filePtr, “Hello, World!”);
“`
4. Close the file:
After writing the desired data to the file, it is crucial to close the file using the `fclose()` function. This ensures that any changes made to the file are properly saved. Simply pass the file pointer as a parameter to `fclose()`:
“`c
fclose(filePtr);
“`
Frequently Asked Questions (FAQs):
Q1: Can I append data to an existing text file instead of overwriting it?
A: Yes, you can append data to an existing text file by opening it in “append” mode instead of “write” mode. Use the mode specifier “a” or “at” to open the file in append mode, as shown below:
“`c
FILE *filePtr = fopen(“data.txt”, “a”);
“`
Q2: What if I want to write multiple lines of text to the file?
A: To write multiple lines of text, you can use the newline character ‘\n’ after each line. For example:
“`c
fprintf(filePtr, “Line 1\n”);
fprintf(filePtr, “Line 2\n”);
fprintf(filePtr, “Line 3\n”);
“`
Q3: How can I write formatted data, such as integers or floating-point numbers, to a text file?
A: To write formatted data, you can use various formatting options provided by the `fprintf()` function. For example, to write an integer and a floating-point number to the file, you can use the following code:
“`c
int num = 10;
float pi = 3.14159;
fprintf(filePtr, “Integer: %d, Pi: %.2f\n”, num, pi);
“`
Q4: What precautions should I take when working with file operations?
A: It’s important to handle errors appropriately, such as checking if the file was opened successfully and handling situations where the file cannot be opened. Additionally, you should always close the file after you finish writing to ensure the changes are saved and prevent potential issues with resource allocation.
Conclusion:
Writing in a text file using C is a fundamental programming task that allows you to store data systematically for future use. By following the steps outlined in this article, you can successfully write data to a text file in C. Remember to open the file, check for successful opening, write the desired data using `fprintf()`, and finally, close the file using `fclose()`. Writing to text files efficiently is a critical skill for any aspiring C programmer.
Can You Write To A File In C?
C programming language provides various features and functions to manipulate files. Writing data to a file is one such essential task that becomes crucial when dealing with data storage, file handling, or data processing applications. In this article, we will delve into the topic of writing to a file in C and explore the necessary concepts and functions to accomplish this task effectively.
Writing to a File in C:
To write data to a file in C, we need to perform a series of steps. Let’s break down the process into five simple steps:
1. Open the File:
Before we can write to a file, it is necessary to open it. The function used for this purpose is fopen(). This function takes two arguments: the first argument is the name of the file, and the second argument is the mode in which the file is opened. The mode could be “w” for writing, “a” for appending at the end of the file, “r+” for reading and writing, or other modes based on the specific requirements. If the file does not exist, fopen() creates one, and if the file already exists, it is truncated, i.e., its contents are cleared.
2. Write Content to the File:
Once the file is successfully opened, we can use the fprintf() or fputs() function to write content to the file. The fprintf() function allows formatted output similar to printf(), while fputs() is used for writing strings. These functions take the file pointer and the content to be written as arguments.
3. Close the File:
After writing the desired content, it is crucial to close the file using the fclose() function. This step ensures that all the changes made to the file are saved and any resources associated with the file are released.
4. Handle Errors:
While writing to a file, it is essential to handle any potential errors that may occur. For example, if the file cannot be opened or the write operation fails, appropriate error handling mechanisms should be implemented. The ferror() function can be used to identify any errors during the write operation.
5. Verify the File Contents:
To ensure the data has been successfully written to the file, it is advisable to open and read the file using file reading functions (such as fgets() or fscanf()) after the write operation is complete. This verification step adds reliability and helps identify any issues in the file writing process.
Sample Code:
To better understand the above steps, let’s consider an example where we write “Hello, World!” to a file named “output.txt”:
“`c
#include
int main() {
FILE* file = fopen(“output.txt”, “w”);
if (file == NULL) {
printf(“Unable to open the file.\n”);
return 1;
}
fprintf(file, “Hello, World!”);
fclose(file);
return 0;
}
“`
In the above code, we open a file named “output.txt” in write mode (“w”), check if the file opened successfully, write the content “Hello, World!” utilizing the fprintf() function, and finally close the file using fclose().
FAQs:
Q1: How can I check if the file was written successfully?
A1: After writing to the file, you can use the ferror() function to check if any errors occurred during the write operation. If ferror() returns non-zero, an error occurred. Additionally, you can verify the file contents using file reading functions.
Q2: Can I write multiple lines to a file using the fprintf() function?
A2: Yes, you can write multiple lines by using the “\n” escape sequence to represent a new line. For example, fprintf(file, “Line 1.\nLine 2.\nLine 3.”);
Q3: What happens if I open an existing file in write mode (“w”)?
A3: If you open an existing file in write mode, the file’s contents will be cleared (truncated) upon opening. Keep this in mind to prevent unintentional loss of data.
Q4: What is the difference between the fprintf() and fputs() functions for file writing?
A4: The fprintf() function allows formatted output similar to printf(). On the other hand, fputs() is specifically used for writing strings and does not support formatting.
Q5: Is it necessary to close the file after writing?
A5: Yes, it is crucial to close the file using fclose() after writing to ensure all changes are saved and resources are released. Leaving a file open may lead to data loss or resource leaks.
In conclusion, writing to a file in C is an important operation that requires a few essential steps. By mastering the necessary functions and understanding the process, you can successfully write data to a file in your C programs. Remember to handle errors, verify the file contents, and close the file after writing to ensure the task is accomplished accurately.
Keywords searched by users: write to a text file c# Read file in C, Read text file in C, Write file txt in C, Read file C++, Write line to file in c, Output file c, Write file c linux, Write file in C
Categories: Top 42 Write To A Text File C#
See more here: nhanvietluanvan.com
Read File In C
Introduction
When it comes to handling file input/output operations, the C programming language offers a wide range of functions and features. In this article, we will explore the process of reading a file in C, discussing the important concepts, techniques, and functions involved. Whether you are a beginner or an experienced programmer, this guide will provide you with a comprehensive understanding of reading files in C.
Understanding File Input/Output in C
Before diving into the intricacies of reading a file in C, it is crucial to understand the fundamentals of file input/output. In C, files are typically accessed in two forms: text files and binary files.
Text files contain human-readable data and are represented by sequences of characters. On the other hand, binary files store data in a format that is not directly readable by humans. It is vital to distinguish between these two types as the method of reading a file can differ.
The process of reading a file involves opening the file, reading its contents, and then closing the file. C provides a collection of functions that facilitate these operations.
Opening a File
To begin reading a file in C, we must first establish a connection between the program and the file using the “fopen()” function. The syntax for opening a file is as follows:
“`c
FILE *filePointer;
filePointer = fopen(“filename”, “mode”);
“`
Here, “filePointer” is a pointer to the structure “FILE” that represents the file to be opened. The “filename” parameter should contain the path and name of the file to be accessed. The “mode” parameter specifies the intention of the file access, such as ‘r’ for reading, ‘w’ for writing, or ‘a’ for appending.
Reading a File
Once the file is successfully opened, we can read its contents. C provides various functions for reading different kinds of data, such as characters, strings, or integers.
For reading characters, we use the “fgetc()” function. It reads a single character from the file and returns its ASCII value. The syntax is as follows:
“`c
int fgetc(FILE *filePointer);
“`
To read an entire line from the file, we can utilize the “fgets()” function. It reads characters from the file until it encounters a new line character (‘\n’) or reaches the specified limit. The syntax is as follows:
“`c
char* fgets(char* buffer, int limit, FILE *filePointer);
“`
For reading integers, we use the “fscanf()” function, which scans the file for the specified format and stores the value in the specified variable. The syntax is as follows:
“`c
int fscanf(FILE *filePointer, const char* format, …);
“`
Closing a File
After we have finished the reading process, it is essential to close the file to release system resources. We use the “fclose()” function to achieve this. The syntax is as follows:
“`c
int fclose(FILE *filePointer);
“`
Frequently Asked Questions (FAQs):
Q1. Can I open multiple files simultaneously in C?
Yes, you can open multiple files simultaneously in C. To do this, you need to create separate FILE pointers and open different files using the “fopen()” function.
Q2. How can I check if a file was successfully opened?
After using the “fopen()” function, you should check if the returned file pointer is “NULL.” If it is, then the file opening process failed. Otherwise, you can proceed with reading the file.
Q3. How can I handle file errors while reading?
When reading a file, it is essential to handle any potential errors to prevent program crashes or undesired behavior. You can use the “feof()” function to check if you have reached the end of the file or the “ferror()” function to check if any error occurred during file access.
Q4. Is it necessary to close a file after reading it?
Yes, it is crucial to close a file after reading it. Closing the file ensures that system resources associated with it are released, preventing any potential memory leaks or resource wastage.
Q5. How can I read a binary file in C?
Reading a binary file in C involves using the “fread()” function, which reads data from the file into a buffer. The syntax is as follows:
“`c
size_t fread(void* buffer, size_t size, size_t count, FILE* filePointer);
“`
Here, “buffer” is a pointer to the memory block that will hold the read data, “size” is the size in bytes of each element to be read, and “count” is the number of elements to be read.
Conclusion
Reading a file in C is a fundamental operation that allows us to access and process external data. Understanding the concepts and functions involved is essential for any C programmer. This article has provided a comprehensive overview of the file reading process in C, covering the various functions and techniques necessary for successful implementation. By applying this knowledge, you can confidently read files and harness the power of file input/output operations in your C programs.
Read Text File In C
Introduction
Reading text files is a common task in programming, especially when working with data from external sources or storing data for later use. In C, there are multiple methods available to read text files, each with its strengths and considerations. This article delves into the various techniques for reading text files in C, providing a comprehensive guide to assist programmers in effectively handling file input.
1. Using the fscanf Function
The simplest method to read text files in C is by utilizing the fscanf function. This function allows you to read formatted data from a file, processing it based on specified format specifiers. Here’s an example demonstrating how to use fscanf to read a text file:
“`c
#include
int main() {
FILE *file;
char word[100];
file = fopen(“example.txt”, “r”);
while (fscanf(file, “%s”, word) != EOF) {
printf(“%s “, word);
}
fclose(file);
return 0;
}
“`
In this example, the program opens the “example.txt” file using the `fopen` function and then reads each word from the file using `fscanf`. The `%s` format specifier is used to read a word until a whitespace character is encountered. The loop continues until `fscanf` returns `EOF` (end-of-file), indicating the file has been fully read.
2. Using fgets to Read Lines
If you need to read a text file line by line, the fgets function is a suitable choice. Unlike `fscanf`, `fgets` reads a line of text until a newline character is encountered. Here’s an example that illustrates how to use `fgets`:
“`c
#include
int main() {
FILE *file;
char line[100];
file = fopen(“example.txt”, “r”);
while (fgets(line, sizeof(line), file)) {
printf(“%s”, line);
}
fclose(file);
return 0;
}
“`
In this case, `fgets` reads a line of text from the file, storing it in the `line` array. The loop continues until the end of the file is reached, printing each line to the console using `printf`.
3. Reading Characters with fgetc
To process individual characters from a text file, the fgetc function comes in handy. It reads character by character until it reaches the end of the file. Here’s an example demonstrating the use of `fgetc`:
“`c
#include
int main() {
FILE *file;
int c;
file = fopen(“example.txt”, “r”);
while ((c = fgetc(file)) != EOF) {
putchar(c);
}
fclose(file);
return 0;
}
“`
Running this program reads the file character by character using `fgetc`. The `putchar` function allows us to print each character to the console. The loop breaks when `fgetc` returns `EOF`, indicating the end of the file.
FAQs
Q1: Can I read text files from a different directory?
A1: Yes, you can specify the file’s path when using `fopen`. For example, to read a file located in a subdirectory named “data,” you would use `fopen(“data/example.txt”, “r”)`.
Q2: How do I check if a file was successfully opened?
A2: After calling `fopen`, you can check if the returned file pointer is `NULL`. If it is, the file open operation failed.
Q3: How can I read specific data from a text file?
A3: By utilizing format specifiers with `fscanf`, you can read specific types of data, such as integers (“%d”), floating-point numbers (“%f”), or characters (“%c”).
Q4: How do I handle errors while reading a file?
A4: You can use the `feof` function to check if the end of a file has been reached, and the `ferror` function to determine if any error occurred during file operations.
Conclusion
Reading text files in C is a fundamental skill every programmer should possess. By leveraging the functions explained in this article, you can extract valuable information from files, enabling further processing and analysis in your programs. Whether you need to read files word by word, line by line, or character by character, C provides versatile solutions for all these scenarios. Happy coding!
Images related to the topic write to a text file c#
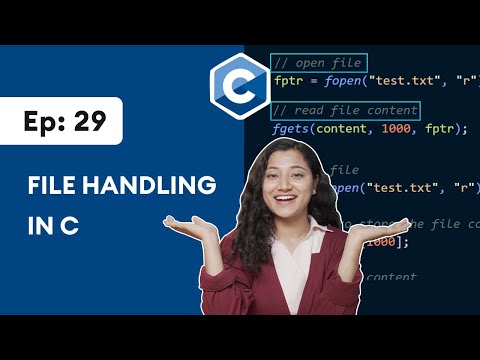
Article link: write to a text file c#.
Learn more about the topic write to a text file c#.
- C Write Text File – Learn C Programming from Scratch
- C Files I/O: Opening, Reading, Writing and Closing a file
- Write to .txt file? – Stack Overflow
- C – File I/O – Tutorialspoint
- C Programming – File Input/Output
- C Files I/O: Opening, Reading, Writing and Closing a file
- C – File Handling – Read and Write Integers – C Programming
- Reading & Writing to Text Files in C Programming – Study.com
- C Programming – File Input/Output
- Write Numerical or Text Data to File in C Programming …
- Read and write to text file in C – OpenGenus IQ
- Reading & Writing to Text Files in C Programming – Study.com
See more: https://nhanvietluanvan.com/luat-hoc