Write A File In C#
File handling refers to the process of reading from or writing to files on a computer. In C#, file handling is an essential task for many programs, as it allows them to store and retrieve data permanently. By understanding the concepts and techniques of file handling in C#, you can efficiently manage data storage and retrieval operations within your applications.
Opening and Creating a File in C#
Before you can read from or write to a file in C#, you need to open it. To open a file, you can use the `System.IO.File` class, which provides various methods for file manipulation. The `File.Open` method allows you to open an existing file or create a new one if it does not already exist.
Here is an example of opening a file in C#:
“`
using System.IO;
string filePath = “example.txt”;
FileStream fileStream = File.Open(filePath, FileMode.OpenOrCreate);
“`
In this example, the `example.txt` file is opened with the `FileMode.OpenOrCreate` option. This option specifies that the file should be opened if it exists, or created if it does not exist. The `fileStream` variable holds the reference to the opened file, which can be used for further file operations.
Writing Data to a File in C#
Once you have opened a file, you can start writing data to it. To write data to a file in C#, you can use the `StreamWriter` class, which simplifies the process of writing text to a file.
Here is an example of writing data to a file in C#:
“`
using System.IO;
string filePath = “example.txt”;
StreamWriter writer = new StreamWriter(filePath);
writer.WriteLine(“Hello, world!”);
writer.WriteLine(“This is an example of writing data to a file in C#.”);
writer.Close();
“`
In this example, the `StreamWriter` class is used to write data to the `example.txt` file. The `WriteLine` method is used to write a line of text to the file. Finally, the `Close` method is called to close the file and release the resources associated with it.
Appending Data to a File in C#
In addition to writing data to a file, you may also need to append data to an existing file. To append data to a file in C#, you can use the `StreamWriter` class with the `FileMode.Append` option.
Here is an example of appending data to a file in C#:
“`
using System.IO;
string filePath = “example.txt”;
StreamWriter writer = new StreamWriter(filePath, append: true);
writer.WriteLine(“This text will be appended to the end of the file.”);
writer.Close();
“`
In this example, the `StreamWriter` class is instantiated with the `FileMode.Append` option, which specifies that the data should be appended to the end of the file. The `writer.WriteLine` method is used to append a line of text to the file. Finally, the `Close` method is called to close the file.
Closing a File and Releasing Resources in C#
After you have finished reading from or writing to a file, it is important to close the file and release the associated resources. Failing to close a file can lead to resource leaks and may cause issues in your application.
To close a file in C#, you can call the `Close` method of the file stream or writer object that you used to access the file.
Here is an example of closing a file in C#:
“`
using System.IO;
string filePath = “example.txt”;
FileStream fileStream = File.Open(filePath, FileMode.Open);
// Perform file operations
fileStream.Close();
“`
In this example, the `Close` method is called on the `fileStream` object to close the file. This ensures that all resources used by the file are released.
Error Handling in File Writing Operations in C#
When performing file writing operations in C#, it is important to handle errors that may occur during the process. For example, if the file is read-only or the disk is full, an exception may be thrown.
To handle errors in file writing operations, you can use exception handling techniques such as try-catch blocks. By catching and handling exceptions, you can gracefully handle errors and prevent your application from crashing.
Here is an example of error handling in file writing operations in C#:
“`
using System;
using System.IO;
try
{
string filePath = “example.txt”;
StreamWriter writer = new StreamWriter(filePath);
writer.WriteLine(“Hello, world!”);
writer.Close();
}
catch (IOException ex)
{
Console.WriteLine(“An error occurred while writing to the file: ” + ex.Message);
}
“`
In this example, a try-catch block is used to catch any `IOException` that may occur during the file writing operation. The error message is then displayed to the console for the user to see.
FAQs
Q: How to read a file in C?
A: In C, you can use the `fopen` and `fread` functions to read a file. `fopen` opens a file, and `fread` reads data from it.
Q: How to read a text file in C?
A: Reading a text file in C is similar to reading any other file. You can use the `fopen` and `fread` functions to read the text from the file.
Q: What is file handling in C?
A: File handling in C refers to the process of reading from or writing to files on a computer. It allows programs to store and retrieve data permanently.
Q: What is `fscanf()` in C?
A: `fscanf()` is a function in C that reads formatted input from a file. It is commonly used to read data from a file in a specific format.
Q: What is a file in C?
A: In C, a file is a collection of data stored on a computer. It can be a text file, a binary file, or any other type of file.
Q: What is the definition of a file in C?
A: In C, a file is a logical structure used to store and retrieve data. It consists of a sequence of characters or bytes organized in a specific format.
Q: How to open a file in C?
A: In C, you can use the `fopen` function to open a file. It takes the file path and the mode in which you want to open the file as parameters.
Q: How to write a file in C?
A: In C, you can use the `fopen` and `fwrite` functions to write data to a file. `fopen` is used to open the file, and `fwrite` is used to write data to it.
#29: C File Handling | C Programming For Beginners
How To Write A File In C?
Introduction:
Writing files in the C programming language is a fundamental skill for any programmer. Files are essential for storing and retrieving data, and understanding how to manipulate them efficiently is crucial. In this article, we will dive into the intricacies of writing files in C, examining the necessary techniques and offering tips to ensure success.
I. Basic File Handling:
Before exploring how to write to a file in C, we need to understand the concepts of file handling. File handling involves three primary steps: opening a file, writing data to it, and finally, closing the file.
1. Opening a File:
In C, we can open a file using the `fopen()` function, which requires two parameters: the file name and the mode. Modes include “r” for reading, “w” for writing, and “a” for appending. For writing to a file, we’ll be using the “w” mode. Here’s an example of opening a file in write mode:
“`c
FILE *filePointer;
filePointer = fopen(“data.txt”, “w”);
“`
2. Writing Data to a File:
Once a file is open, we can write data to it. The `fprintf()` function is commonly used for writing formatted data. It requires the file pointer and the data to be written as parameters. Here’s an example of writing an integer and a string to a file:
“`c
int number = 42;
char message[] = “Hello, World!”;
fprintf(filePointer, “%d %s”, number, message);
“`
3. Closing a File:
Closing a file is crucial to ensure that all data is written and resources are freed. The `fclose()` function is used to close a file and requires the file pointer as its parameter. Here’s an example of closing a file:
“`c
fclose(filePointer);
“`
II. Handling Errors:
While working with files, it’s essential to handle errors appropriately. Here are some best practices:
1. Check File Opening:
After opening a file, it is wise to check if it was opened successfully. This can be done by verifying the value returned by `fopen()`. If it is `NULL`, an error occurred. Here’s an example:
“`c
FILE *filePointer;
filePointer = fopen(“data.txt”, “w”);
if (filePointer == NULL) {
printf(“Error opening the file”);
exit(1);
}
“`
2. Verify Writing Success:
When using `fprintf()` to write data, it’s crucial to check its successful execution. The function returns the number of characters written. If this count doesn’t match the expected number, an error occurred. Here’s an example:
“`c
if (fprintf(filePointer, “%d %s”, number, message) != strlen(message) + sizeof(number)) {
printf(“Error writing to the file”);
exit(1);
}
“`
3. Handle Closing Errors:
Although `fclose()` usually works as expected, it’s important to handle potential errors. Checking the return value is advisable. If it’s different from zero, an error occurred. Here’s an example:
“`c
if (fclose(filePointer) != 0) {
printf(“Error closing the file”);
exit(1);
}
“`
III. File Positioning:
In some cases, we may need to control the file position while writing data. The `fseek()` function allows us to set the file position indicator explicitly.
1. Writing at the End of a File:
By default, when we open a file in write mode, we start writing at its beginning. However, we can alter this behavior using `fseek()`. To write at the end of the file, we can use the SEEK_END constant as the second parameter. Here’s an example:
“`c
fseek(filePointer, 0, SEEK_END);
“`
IV. FAQs:
Q1. Can I write to a file that doesn’t exist?
A1. Yes, when opening a file in write mode, if it doesn’t exist, it will be created. However, existing files with the same name will be overwritten.
Q2. How do I write multiple lines to a file?
A2. To write multiple lines to a file, you can use a loop and repeatedly call `fprintf()` or use `fputs()` to write a string directly. Remember to add newline characters (“\n”) between lines.
Q3. How do I write binary data to a file?
A3. For writing binary data, you can use `fwrite()`. It requires the data pointer, size, number of elements, and the file pointer as parameters.
Q4. How can I append data to an existing file?
A4. To append data instead of overwriting existing content, open the file in “a” mode rather than “w” mode.
Conclusion:
Writing files in C is a fundamental skill that every programmer must master. By understanding the basics of file handling, error handling, file positioning, and practicing these concepts, you can efficiently write data to files and utilize them in your programs. Remember to check for errors and close files properly to maintain data integrity. Happy coding!
How To Write And Create A File In C?
Writing and creating files is an essential skill for any programmer, especially when working with the C programming language. Whether you are storing data, generating reports, or simply manipulating files, understanding the process of file creation and writing is crucial. In this article, we will comprehensively explore how to write and create a file in C, covering important concepts, syntax, and best practices. So let’s dive in!
1. Including necessary header files:
Before you can initiate file operations, you must include the necessary header files. The two primary headers required for file handling in C are
2. Opening a file:
To write or create a file in C, you first need to open it. The fopen() function is used for this purpose. It takes two parameters: the file name or path, and the mode. The mode specifies the type of file access you want. Common modes for writing files are “w” (write), “a” (append), or “r+” (read and write). Here is an example of opening a file for writing:
“`c
FILE* filePointer;
filePointer = fopen(“example.txt”, “w”);
“`
3. Writing to a file:
Once the file is opened successfully, you can start writing data to it. The fprintf() function is commonly used to write formatted data to a file. It takes a file pointer and the data to be written as parameters. Here’s an example showing how to write a string to a file:
“`c
fprintf(filePointer, “Hello World!\n”);
“`
You can also write variables or other data types using suitable format specifiers like %d for integers, %f for floats, etc. Remember to include the newline character (‘\n’) at the end of each line if you wish to separate the data.
4. Closing the file:
When you finish writing or performing operations on a file, it is crucial to close it properly using the fclose() function. Closing the file ensures that all written data is saved and any system resources used by the file are released. Here’s an example of closing a file:
“`c
fclose(filePointer);
“`
5. Error handling:
It is good practice to check if the file was opened successfully before proceeding with writing operations. The fopen() function returns NULL if it fails to open the specified file. Checking this helps avoid unwanted crashes or errors. Consider the following example:
“`c
FILE* filePointer;
filePointer = fopen(“example.txt”, “w”);
if (filePointer == NULL) {
printf(“Failed to open the file.\n”);
exit(1); // Terminate the program
}
“`
Frequently Asked Questions (FAQs):
Q1. Can I create a file in a specific directory?
Yes, you can create a file in a specific directory by specifying the file path along with the file name while opening it. For example:
“`c
filePointer = fopen(“/path/to/directory/example.txt”, “w”);
“`
Q2. What happens if I try to write to a file that doesn’t exist?
If the specified file doesn’t exist, using the “w” mode will create a new file with the given name. However, if you wish to append data to an existing file, you should use the “a” mode instead.
Q3. How can I check if a file exists before writing to it?
You can use the access() function provided by the
“`c
#include
if (access(“example.txt”, F_OK) != -1) {
// File exists
}
“`
Q4. How do I write binary data to a file?
You can use the fwrite() function to write binary data to a file. It takes the data, size, count, and file pointer as parameters. For example:
“`c
int data[] = {1, 2, 3, 4, 5};
fwrite(data, sizeof(int), sizeof(data) / sizeof(int), filePointer);
“`
This writes the array of integers named “data” to the file.
Q5. What precautions should I take when handling file operations?
Always ensure that you have sufficient permissions to write to the desired file location. Avoid writing data without error checking, as it may lead to unexpected results. Properly close the file when you finish writing or performing operations to avoid resource leaks.
In conclusion, knowing how to write and create files in C is a fundamental skill for any programmer. By following the steps outlined in this article, you will be able to successfully open, write data to, and close files in your C programs. Additionally, the included FAQs provide answers to common queries that may arise during file handling. So go ahead and start creating and manipulating files with confidence!
Keywords searched by users: write a file in c# Read file in C, Read text file in C, File handling in C, Fscanf() in C, File in C, Definition of file in c, Open file in C, Write in C
Categories: Top 53 Write A File In C#
See more here: nhanvietluanvan.com
Read File In C
Reading files is a fundamental operation in computer programming, and the C programming language provides various mechanisms to accomplish this task efficiently. In this article, we will dive deep into the concepts and techniques related to reading files in C, exploring different approaches, best practices, and commonly encountered issues. So, let’s get started!
Understanding File Access Modes
Before we can read a file in C, it is important to understand the different access modes available. The “access mode” refers to how the file will be opened and read. C provides several file access modes, including:
1. “r” – Read mode: Opens an existing file for reading.
2. “w” – Write mode: Opens a file for writing. If the file already exists, its contents are truncated. If the file does not exist, a new file is created.
3. “a” – Append mode: Opens a file for appending. If the file does not exist, a new file is created.
4. “r+” – Read and write mode: Opens a file for both reading and writing.
5. “w+” – Read and write mode: Opens a file for both reading and writing. If the file already exists, its contents are truncated. If the file does not exist, a new file is created.
6. “a+” – Read and append mode: Opens a file for reading and appending. If the file does not exist, a new file is created.
Opening Files in C
To read a file in C, we start by opening it using the `fopen()` function. The `fopen()` function takes two arguments – the file path and the access mode. Here’s an example that demonstrates how to open a file for reading:
“`c
#include
int main() {
FILE *file = fopen(“example.txt”, “r”);
if (file == NULL) {
printf(“Error opening file!”);
return 1;
}
// Further operations on the opened file
fclose(file);
return 0;
}
“`
In the above code snippet, `fopen()` opens the file named “example.txt” in read mode (“r”). We then check if the file pointer `file` is `NULL` to ensure the file was opened successfully. If the file does not exist or if there was an error during the file opening process, we display an error message. Finally, we close the file using the `fclose()` function to free system resources.
Reading Data from Files
Once a file is opened, we can read its contents using various functions provided by the C standard library. The most commonly used functions for reading data from files are `fgetc()`, `fgets()`, and `fread()`.
The `fgetc()` function reads individual characters from the file. It returns the character read as an `int`, or `EOF` (-1) when the end of the file is reached. Here’s an example that demonstrates how to read characters from a file using `fgetc()`:
“`c
#include
int main() {
FILE *file = fopen(“example.txt”, “r”);
if (file == NULL) {
printf(“Error opening file!”);
return 1;
}
int character;
while ((character = fgetc(file)) != EOF) {
printf(“%c”, character);
}
fclose(file);
return 0;
}
“`
In the above code snippet, we use a `while` loop to keep reading characters from the file until the end is reached. Each character read is then printed using `printf()`. Finally, we close the file using `fclose()`.
In addition to `fgetc()`, the `fgets()` function can be used to read a line of text from a file. It reads characters until it reaches the newline character `\n`, the maximum specified length, or the end of the file. Here’s an example that demonstrates how to use `fgets()`:
“`c
#include
int main() {
FILE *file = fopen(“example.txt”, “r”);
if (file == NULL) {
printf(“Error opening file!”);
return 1;
}
char buffer[100];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf(“%s”, buffer);
}
fclose(file);
return 0;
}
“`
In the above code snippet, we declare a character buffer of size 100 and use `fgets()` to read lines from the file. The lines are then printed using `printf()`. Again, we close the file using `fclose()`.
Another technique for reading data from a file is through the `fread()` function. `fread()` allows reading a specified number of elements of a certain size from a file. Here’s an example that demonstrates how to use `fread()`:
“`c
#include
typedef struct {
int id;
char name[50];
} Person;
int main() {
FILE *file = fopen(“data.bin”, “rb”);
if (file == NULL) {
printf(“Error opening file!”);
return 1;
}
Person person;
while (fread(&person, sizeof(Person), 1, file) == 1) {
printf(“ID: %d, Name: %s\n”, person.id, person.name);
}
fclose(file);
return 0;
}
“`
In the above code snippet, we define a structure `Person` that represents a simple person record. We open a binary file for reading using `”rb”` mode and use `fread()` to read `Person` records from the file. Finally, we print the values of each person record and close the file.
FAQs
Q1. Can I read multiple files simultaneously in C?
A1. Yes, you can read multiple files simultaneously by employing multiple file pointers. Each file pointer can be assigned to a different file using the `fopen()` function.
Q2. How do I check if the end of the file is reached?
A2. The `fgetc()` function returns `EOF` (-1) when the end of the file is reached. You can loop until `fgetc()` returns `EOF` to read the entire file.
Q3. What should I do if a file fails to open?
A3. If a file fails to open, `fopen()` returns `NULL`. You should check the returned value and handle the error accordingly, such as displaying an error message or terminating the program.
Q4. How can I read binary files in C?
A4. Binary files can be read using the `fread()` function. You need to specify the size of the elements to be read and the number of elements.
In conclusion, reading files in C involves opening the file in the desired access mode and using appropriate functions to read the file’s contents. By understanding file access modes and employing the appropriate techniques, you can efficiently read files in your C programs.
Read Text File In C
Firstly, let’s understand what a text file is. In simple terms, a text file is a sequence of characters stored in a file format that is readable by humans. These files generally contain plain text and do not have any specific formatting applied. To read a text file in C, we need to follow a step-by-step process.
The first step is to open the text file using the `fopen` function. This function takes two arguments: the file name and the mode. In our case, the mode should be set to `”r”`, which stands for read mode. Let’s take a look at an example:
“`c
FILE *file;
char *filename = “data.txt”; // File name
file = fopen(filename, “r”); // Opening the file in read mode
if (file == NULL) {
printf(“Unable to open the file.\n”);
return 1; // Exit if the file does not exist
}
“`
After opening the file, we need to check if the file was opened successfully. If the file pointer (`file`) is `NULL`, it means the file could not be opened, and we should handle this error appropriately. In the example above, we simply print an error message and exit the program with a return value of `1`.
Once the file is successfully opened, we can start reading its contents. The `fgetc` function is used to read characters from the file sequentially. It takes the file pointer as a parameter and returns the next character from the file. Here’s an example of reading characters from a file until the end:
“`c
int c;
while ((c = fgetc(file)) != EOF) {
printf(“%c”, c);
}
“`
In this code snippet, we use a `while` loop to read each character from the file until the end of the file (`EOF`) is encountered. We then print each character using the `printf` function. It is worth noting that characters are read from the file one by one, so this method can be relatively slow for large files.
To read an entire line from a text file, we can use the `fgets` function. This function takes three arguments: the character array or string where the line will be stored, the maximum number of characters to read (including the null terminator), and the file pointer. Here’s an example:
“`c
char line[100]; // Buffer to store the line
while (fgets(line, sizeof(line), file) != NULL) {
printf(“%s”, line);
}
“`
The `fgets` function reads characters from the file until it encounters a newline character (`’\n’`), the maximum number of characters to read has been reached, or the end of the file is reached. The line read is then stored in the `line` buffer. We can then use the `printf` function to print the line.
Another commonly used method to read an entire file at once is by using the `fread` function. This function reads a specified number of bytes from the file and stores them in the provided buffer. Here’s an example of reading the entire file into a buffer:
“`c
char buffer[1000]; // Buffer to store the file contents
size_t bytesRead;
bytesRead = fread(buffer, sizeof(char), sizeof(buffer) – 1, file);
buffer[bytesRead] = ‘\0’; // Null-terminate the buffer
printf(“%s”, buffer);
“`
In this example, we read a maximum of `sizeof(buffer) – 1` characters (to leave room for the null terminator) from the file using the `fread` function. The number of bytes read is stored in the `bytesRead` variable. Finally, we add the null terminator (`’\0’`) to the buffer to ensure that it is properly null-terminated, allowing us to treat it as a string.
After we finish reading the file, it is important to close it using the `fclose` function. This will free up system resources associated with the file. Here’s an example:
“`c
fclose(file); // Close the file
“`
Closing the file is essential, as leaving it open can lead to resource leaks or issues with file access in subsequent operations.
Now, let’s address some frequently asked questions about reading text files in C:
**Q: Can I read binary files in the same way as text files?**
A: No, binary files contain data in a different format, and reading them requires a different approach. Text files are typically encoded in ASCII or UTF-8, while binary files can contain any kind of data. Reading binary files often requires interpreting the data based on a predetermined structure or format.
**Q: What if I want to read only a specific section of a text file?**
A: If you want to read a specific section of a file, you can use fseek to move the file position indicator to the desired location before reading. fseek allows you to set the file position using an offset from the beginning, current position, or end of the file.
**Q: How can I handle large files efficiently?**
A: Reading large files character by character (using `fgetc`) can be slow. For better performance, consider reading the file in larger chunks using `fread`. Additionally, if your goal is to process the file line-by-line, you can read the file line by line using `fgets`.
**Q: What should I do if I encounter errors while reading a file?**
A: When reading files, it is important to check for errors during file operations such as opening, reading, and closing. You can check if a file operation succeeded by comparing the file pointer (`file`) to `NULL` or checking the return values of functions like `fgetc`, `fgets`, and `fread`. In case of an error, you should handle it appropriately, such as printing an error message and exiting the program or taking corrective actions.
In conclusion, reading text files in C is a necessary skill for any programmer dealing with file I/O operations. There are various approaches available, depending on the specific requirements of your program. Whether you need to read the file character by character, line by line, or read the entire file into a buffer, understanding these techniques will help you efficiently read and process text files in your C programs.
Images related to the topic write a file in c#
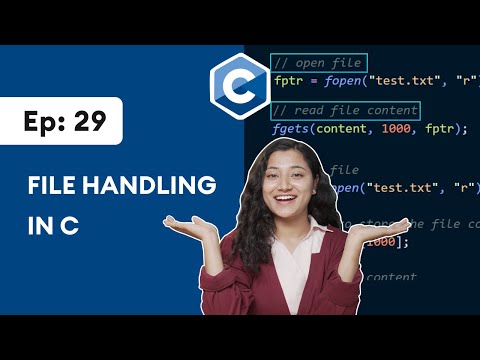
Article link: write a file in c#.
Learn more about the topic write a file in c#.
- C Files I/O: Opening, Reading, Writing and Closing a file
- C – File I/O – Tutorialspoint
- C Programming – File Input/Output
- C program to create a file and write data into file – Codeforwin
- C Files I/O: Opening, Reading, Writing and Closing a file
- C – File Handling – Read and Write Integers – C Programming
- C Programming – File Input/Output
- C Write To Files – W3Schools
- Basics of File Handling in C – GeeksforGeeks
- How to Write to File in C? – Tutorial Kart
- C Files I/O: Create, Open, Read, Write and Close a File – Guru99
- File Handling in C — How to Open, Close, and Write to Files
- C Write Text File – Learn C Programming from Scratch
See more: https://nhanvietluanvan.com/luat-hoc