Variable Declaration Not Allowed At This Location Javascript
Variable declaration is a fundamental concept in programming that allows developers to create named storage spaces for holding values. In JavaScript, variable declaration is typically done using the “var,” “let,” or “const” keywords. However, there are certain limitations and restrictions on where variables can be declared within the code.
Nested Blocks and Scope
In JavaScript, nested blocks refer to code blocks that are enclosed within other code blocks, such as if statements, for loops, and function declarations. Each nested block creates a new scope, which determines the accessibility and visibility of variables declared within it.
Understanding scoping rules in JavaScript is crucial for proper variable declaration. Scoping refers to the accessibility of variables within a specific section of code. In JavaScript, variables declared with the “var” keyword have function-level scope, which means they are accessible within the entire function.
Introduction to Block-Level Scope
Block-level scope was introduced in JavaScript with the “let” and “const” keywords. Unlike “var,” variables declared using “let” and “const” have block-level scope, which means they are only accessible within the block in which they are declared.
Rules for Variable Declaration within Nested Blocks
In JavaScript, variables declared with the “var” keyword can be accessed anywhere within the function scope, including nested blocks. However, variables declared with “let” or “const” keywords are limited to the block in which they are defined.
For example:
“`javascript
function example() {
var x = 10; // Accessible within the entire function
if (true) {
let y = 20; // Accessible only within the if block
const z = 30; // Accessible only within the if block
console.log(x); // Output: 10
console.log(y); // Output: 20
console.log(z); // Output: 30
}
console.log(x); // Output: 10
console.log(y); // Throws an error
console.log(z); // Throws an error
}
example();
“`
Function Scope and Hoisting
In JavaScript, variables declared with the “var” keyword are “hoisted” to the top of their scope. This means that regardless of where a variable is declared, it is moved to the top of its containing function or global scope during the compilation phase.
Variable hoisting can lead to unexpected behavior if not properly understood. For example:
“`javascript
function example() {
console.log(x); // Output: undefined
var x = 10;
console.log(x); // Output: 10
}
example();
“`
In the above example, even though the variable “x” is accessed before it is declared, it does not throw an error. This is because the variable declaration gets hoisted to the top of the function scope.
Impact of Hoisting on Variable Declaration
Hoisting can cause confusion when it comes to variable declaration. To avoid this confusion, it is recommended to always declare variables at the beginning of the scope to which they belong. This ensures that variables are defined before they are used.
For example:
“`javascript
function example() {
var x;
console.log(x); // Output: undefined
x = 10;
console.log(x); // Output: 10
}
example();
“`
Restrictions on Variable Declaration in Function Scope
In JavaScript, “var” variables are function-scoped, meaning they are accessible anywhere within the function. However, the use of “var” variables outside of function scope is not recommended.
Variables declared with “let” or “const” keywords have block-level scope, which provides better control and prevents scope leakage. Therefore, it is recommended to use “let” or “const” instead of “var” for variable declaration to improve code quality and avoid potential issues.
Global Scope and Strict Mode
Global scope refers to the accessibility of variables across the entire JavaScript program. Variables declared outside of any function or block have global scope and can be accessed from any part of the program.
However, utilizing the global scope extensively can lead to naming conflicts and make code maintenance difficult. It is considered best practice to limit the use of global scope and avoid polluting the global namespace.
Introduction to Strict Mode and Its Impact
Strict mode is a feature introduced in ECMAScript 5 that enables a stricter version of JavaScript by eliminating certain silent errors and enforcing stricter syntax rules. It helps catch common coding mistakes and makes the code more reliable and secure.
When strict mode is enabled, variable declaration without the “var,” “let,” or “const” keywords is not allowed. This helps prevent accidental global variable creation and encourages the use of proper scoping practices.
Conditional Statements and Loops
Variable declaration within conditional statements and loops also follows the scoping rules discussed earlier. Variables declared within the conditional block or loop block are only accessible within that specific block.
Specific rules apply when using variables within if statements, switch statements, and loops. It is crucial to understand the scoping rules and use the appropriate keywords to declare variables within these constructs.
Asynchronous Code and Event Listeners
Asynchronous code execution and event-driven programming bring their own set of challenges when it comes to variable declaration. Within asynchronous functions or event listeners, it is important to declare variables in the appropriate scope considering the timing and order of execution.
Handling variable declaration in asynchronous scenarios involves using techniques such as closures, promises, or async/await to manage variable scope and ensure proper access to variables at the desired time.
Precautions for Variable Declaration in Event-Driven Programming
In event-driven programming, variables declared within event listeners are only accessible within the event listener’s function scope. To access variables outside of the event listener, they need to be declared in a wider scope or stored in a higher-level object.
It is important to be cautious with variable declaration in event-driven programming to avoid scope-related bugs and ensure proper data flow between different parts of the application.
Class and Object Scope
In JavaScript, variables can also be declared within classes and objects. The scoping rules for class and object instances are similar to those for block-level scope.
Variables declared within a class or object instance can be accessed using the “this” keyword. However, variables declared outside of the class or object can be accessed directly.
Conclusion
Understanding variable declaration and scoping rules in JavaScript is crucial for writing reliable and maintainable code. By following the rules and best practices outlined in this article, developers can avoid common pitfalls and ensure their variables are declared within the appropriate scope.
FAQs
1. Why am I getting the error “Variable declaration not allowed at this location” in JavaScript?
– This error occurs when a variable is declared in a location where it is not allowed according to the scoping rules in JavaScript. Check if the variable is being declared within a nested block, global scope, or any other restricted location.
2. What is the difference between function scope and block-level scope?
– Function scope refers to the accessibility of variables within a specific function, while block-level scope limits the accessibility of variables to the block in which they are declared.
3. How does hoisting impact variable declaration in JavaScript?
– Hoisting moves variable declarations to the top of their scope during the compilation phase in JavaScript. This can lead to unexpected behavior if variables are accessed before they are declared.
4. Should I use “var,” “let,” or “const” for variable declaration in JavaScript?
– It is recommended to use “let” or “const” for variable declaration instead of “var” to enforce block-level scope and prevent scope leakage.
5. How can I handle variable declaration in asynchronous JavaScript code?
– Asynchronous JavaScript code can be handled using techniques such as closures, promises, or async/await to manage variable scope and ensure proper access to variables at the desired time.
6. What are some precautions for variable declaration in event-driven programming?
– In event-driven programming, be cautious with variable declaration within event listeners to avoid scope-related bugs. Declare variables in wider scopes or store them in higher-level objects for proper data flow.
Javascript For Developers 09 – Variable Declaration
What Does Variable Declaration Not Allowed Here Mean?
In the world of programming, encountering errors is an inevitable part of the process. One common error message that developers often come across is “variable declaration not allowed here.” This message can be perplexing for beginners and even seasoned programmers, but fear not! In this article, we will delve into the intricacies of this error message and shed light on what it actually means.
Understanding Variable Declaration:
Before diving into the error message itself, it is crucial to have a clear understanding of variable declaration. In programming, a variable declaration is the act of defining a variable in a particular programming language. This process allocates memory for the variable and sets its initial data type and value (if any). Variables are essential for storing and manipulating data throughout the program’s execution.
Error Message Explained:
When you encounter the error message “variable declaration not allowed here,” it means that you are attempting to declare a variable in a scope where it is not permitted. In programming, the scope determines the portion of the code where a particular variable can be accessed or referenced. Each programming language has its own set of rules and regulations regarding variable scopes.
Common Causes:
1. Incorrect Placement:
The most common cause of this error is incorrect variable placement. Some programming languages require variables to be declared at the beginning of a block, such as within a function or loop. If you mistakenly try to declare a variable outside the allowed scope, the error message will be triggered.
2. Redeclaration:
Another cause is attempting to declare a variable with the same name in the same scope. Most programming languages do not allow redeclaration, as it can lead to ambiguity and confusion. If you try to redeclare a variable within a specific scope, the error message will be displayed.
3. Reserved Keywords:
Certain programming languages have reserved keywords, which cannot be used as variable names. If you unknowingly use a reserved keyword, you will encounter this error message. It is essential to consult the language documentation to ensure you are using appropriate variable names.
4. Incorrect Syntax:
Syntax errors can also trigger the “variable declaration not allowed here” message. If you mistakenly use incorrect syntax while declaring a variable, the compiler or interpreter may not recognize it as a valid declaration, resulting in the error message.
Resolving the Error:
To resolve the “variable declaration not allowed here” error, you should carefully review the code and identify where the variable declaration is situated. Based on the cause mentioned above, you can pinpoint the issue and make the necessary adjustments. Here are a few troubleshooting steps:
1. Check Variable Placement:
Ensure that your variable declaration is within the scope allowed by the programming language. Move the declaration to a valid location, such as within a function or loop, if required.
2. Avoid Redeclaration:
If you receive this error message due to redeclaration, review your code for duplicate variable names. Rename the duplicate variables to provide them with unique identifiers.
3. Verify Reserved Keywords:
If you suspect that a reserved keyword is being used as a variable name, double-check the language documentation to confirm. Replace any reserved keyword used as a variable name with an appropriate alternative.
4. Syntax Correction:
Check your variable declaration syntax against the language specifications or guidelines. Correct any errors and typos that might lead to the error message.
FAQs:
Q: Can this error message occur across all programming languages?
A: No, the actual error message wording may differ across programming languages, but the underlying issue remains the same. Different languages might provide error messages such as “variable scope violation” or “variable declaration not permitted here.”
Q: Why do some programming languages enforce variable scope restrictions?
A: Variable scopes are established to organize code and avoid conflicts or unintended consequences. By restricting where variables can be declared or accessed, programming languages ensure clean and predictable code behavior.
Q: I’m positive that my code is correct, but the error still persists. What could be the reason?
A: Sometimes, the error might not be related to variable declaration but could be caused by other issues, such as a misplaced statement or a missing closing bracket. Consider reviewing the surrounding code for any other potential mistakes.
Q: Can I declare a variable multiple times within a program?
A: In most cases, you can declare a variable multiple times within different scopes without any issues. However, redeclaring a variable within the same scope is generally not allowed and will result in an error.
Conclusion:
The “variable declaration not allowed here” error is a common stumbling block for developers. By understanding the concept of variable declaration, identifying potential causes, and following the suggested troubleshooting steps, programmers can efficiently debug and resolve this issue. Remember to consult language documentation and stay mindful of variable placement, redeclaration, reserved keywords, and syntax correctness. With practice and attention to detail, you will soon overcome this error and continue your programming journey with confidence.
Where Should You Declare Variables In Javascript?
In JavaScript, variables play a vital role in storing and manipulating data. In order to effectively use variables, it is important to understand where they should be declared within the code. The placement of variable declarations can affect the scope, availability, and lifetime of variables, ultimately impacting the functionality and efficiency of your JavaScript programs. In this article, we will explore different aspects of variable declaration in JavaScript and discuss the best practices for declaring variables.
Understanding Variable Scope
Before delving into the different approaches for declaring variables, it is important to understand the concept of scope in JavaScript. Scope refers to the accessibility and visibility of variables within different parts of a program. JavaScript has two main types of scope: global scope and local scope.
Global scope refers to variables declared outside of any function, making them accessible throughout the entire program. Any variable declared inside a function has local scope, which limits the accessibility to only within that function. It is important to note that variables declared within a block, such as an if statement or loop, have block scope and are only accessible within that specific block.
Declaration Best Practices
1. Declare Variables at the Top of Functions
A common convention in JavaScript is to declare variables at the top of their containing scope, within the function. This approach ensures that all variables used in the function are declared in one place, making it easier to understand and maintain the code. Additionally, it helps avoid unexpected variable hoisting behavior, where variables are automatically moved to the top of their scope.
For example:
“`
function exampleFunction() {
var name = ‘John’;
var age = 30;
// Function logic goes here
}
“`
2. Use let and const for Block-Scoped Variables
With the introduction of ES6, two new keywords, let and const, were introduced as alternatives to the traditional var keyword. The let keyword allows for block-scoping, meaning variables declared with let are limited to the block they are declared in. The const keyword, on the other hand, is used to declare variables that cannot be reassigned.
Using let and const for block-scoped variables is considered best practice as it helps prevent accidental variable reassignments and improves code clarity. It also ensures that variables are only accessible within their intended block, reducing the likelihood of unintended side effects.
For example:
“`
if (condition) {
let message = ‘Hello’;
console.log(message); // Output: Hello
}
console.log(message); // Throws ReferenceError
“`
3. Avoid Global Variables
While global variables may seem convenient due to their accessibility throughout the program, they can lead to various issues such as naming conflicts, unintended variable modifications, and increased risk of bugs. It is generally recommended to avoid declaring variables in the global scope unless absolutely necessary. Instead, keep variables within their respective functions or blocks to minimize potential issues.
For example:
“`
// Avoid using global variables
var globalVariable = ‘I am global’;
function printGlobalVariable() {
console.log(globalVariable);
}
// Prefer local variables
function printLocalVariable() {
var localVariable = ‘I am local’;
console.log(localVariable);
}
“`
FAQs:
Q1: Can I declare variables after using them in JavaScript?
A1: Yes, JavaScript supports a concept called variable hoisting, where variables declared with the var keyword are automatically moved to the top of their respective scope. However, it is considered best practice to declare variables at the top of their containing scope to improve code readability and avoid potential issues.
Q2: Are let and const backward-compatible with older versions of JavaScript?
A2: The let and const keywords were introduced in ES6, so they are not available in older versions of JavaScript. However, modern JavaScript environments and browsers fully support these keywords, making them safe to use in most scenarios. For older JavaScript versions, it is recommended to use the var keyword or transpile your code to ensure compatibility.
Q3: What is the difference between let and const?
A3: The main difference between let and const lies in their reassignment capabilities. Variables declared with let can be reassigned, while variables declared with const are read-only and cannot be reassigned once they are initialized. Both let and const have block scope, meaning they are only accessible within the block they are declared in.
Q4: Are there any performance differences between different variable declaration approaches?
A4: In most modern JavaScript engines, there is no significant performance difference between different variable declaration approaches. The performance impact, if any, is negligible. Therefore, it is more important to focus on code readability, maintainability, and adhering to best practices rather than worrying about performance implications.
Conclusion:
Understanding where to declare variables in JavaScript is crucial for writing clean, efficient, and bug-free code. By following best practices like declaring variables at the top of their containing scope, using block-scoped variables with let and const, and avoiding global variables as much as possible, developers can ensure their code is more readable and maintainable. It is also important to keep up with the latest JavaScript standards to make the most of the language’s capabilities and enhancements.
Keywords searched by users: variable declaration not allowed at this location javascript vhdl variable declaration not allowed in this region
Categories: Top 97 Variable Declaration Not Allowed At This Location Javascript
See more here: nhanvietluanvan.com
Vhdl Variable Declaration Not Allowed In This Region
When writing VHDL code, it is crucial to understand that variable declarations are not allowed in certain regions, particularly in sequential blocks such as processes and functions. These regions are limited to the use of signals instead of variables.
In VHDL, signals represent physical wires or lines that can store and transmit values between different processes or entities. They are intended to model the behavior of wires and connections in a digital circuit. Variables, on the other hand, are used for temporary storage within a process and are not allowed within sequential blocks.
The reason for this restriction lies in the inherent nature of variables and signals in VHDL. Variables are private and exclusive to the process in which they are declared. They retain their values until changes are explicitly made within the process. Signals, on the other hand, are shared and can be accessed by multiple processes. They are used to propagate values between different processes and represent the actual interconnection of components in a system.
This limitation on variable declarations within sequential blocks ensures the preservation of the synchronous behavior of sequential designs in VHDL. Variables, being private and exclusive to a process, can lead to potential race conditions and synchronization issues if used within a sequential block.
By restricting variable usage within sequential blocks, VHDL enforces a style of coding that adheres to the synchronous design methodology. In this methodology, the behavior of the design is synchronized with a clock signal, and changes happen only at specific clock edges. The use of signals instead of variables allows for proper synchronization and avoids potential race conditions.
It is important to note that variable declarations are allowed in concurrent regions of VHDL code. Concurrent regions include architecture-level declarations and concurrent statements such as component instantiations, concurrent signal assignments, and concurrent procedure calls. In these regions, variables can be safely used as they do not affect the synchronous behavior of the design.
As with any programming language or coding practice, there are always questions that arise. Here are some frequently asked questions regarding the VHDL limitation on variable declarations within sequential blocks:
Q1. What should I do if I need to perform temporary calculations within a process?
A1. Instead of using variables, you can make use of local signals within the process. These signals can store intermediate values just like variables, but they adhere to the synchronous behavior of VHDL designs.
Q2. Can I assign a signal to a variable or vice versa within a process?
A2. No, the assignment between variables and signals is not allowed within a process. If you need to perform such assignments, you should use concurrent regions or utilize temporary signals for data transfer between processes.
Q3. Why can’t I use variables for inter-process communication?
A3. Variables are private to a process and cannot be accessed by other processes. To enable inter-process communication, signals should be used as they represent the physical interconnections of components.
Q4. What are the advantages of using signals instead of variables in sequential blocks?
A4. The use of signals ensures proper synchronization and avoids potential race conditions. It promotes a synchronous design methodology and simplifies the analysis and verification of the design.
Q5. Can I use variables for behavioral modeling in VHDL?
A5. Yes, variables can be used for behavioral modeling of combinational logic and data manipulation operations. However, they should not be used within sequential blocks.
Understanding the restriction on variable declarations within sequential blocks in VHDL is crucial for designing reliable and efficient digital circuits. By following the guidelines and utilizing signals appropriately, developers can avoid potential synchronization issues and ensure the robustness of their designs. Remember, staying within the bounds of the language’s rules and best practices is key to achieving success in VHDL-based design endeavors.
Images related to the topic variable declaration not allowed at this location javascript
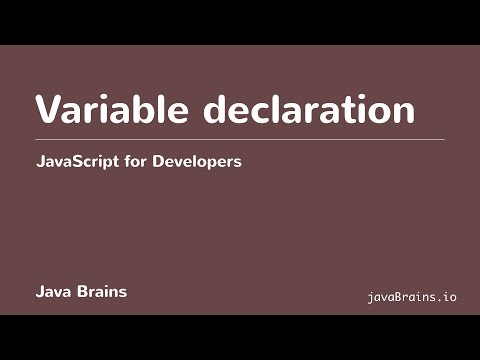
Found 37 images related to variable declaration not allowed at this location javascript theme
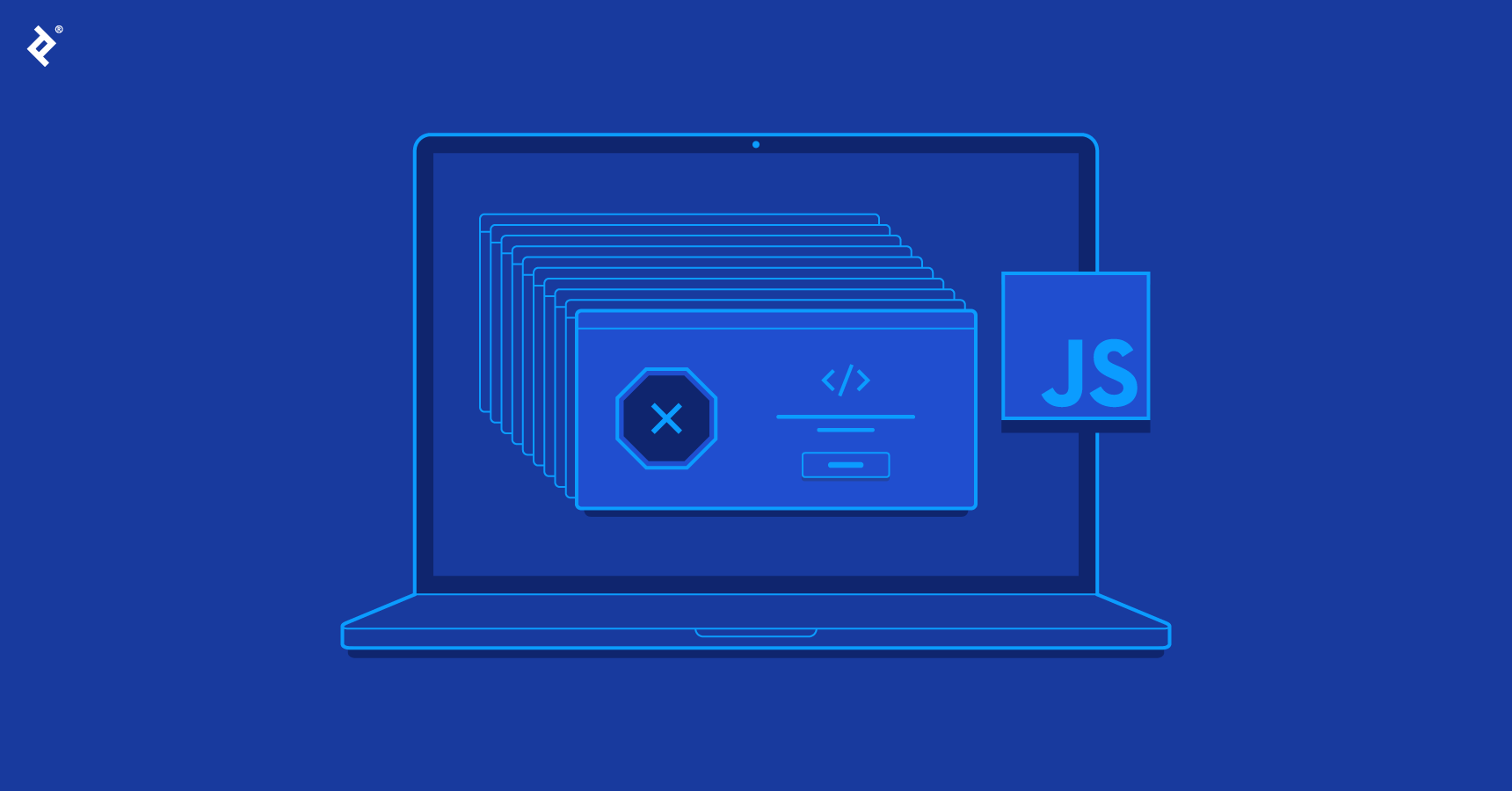
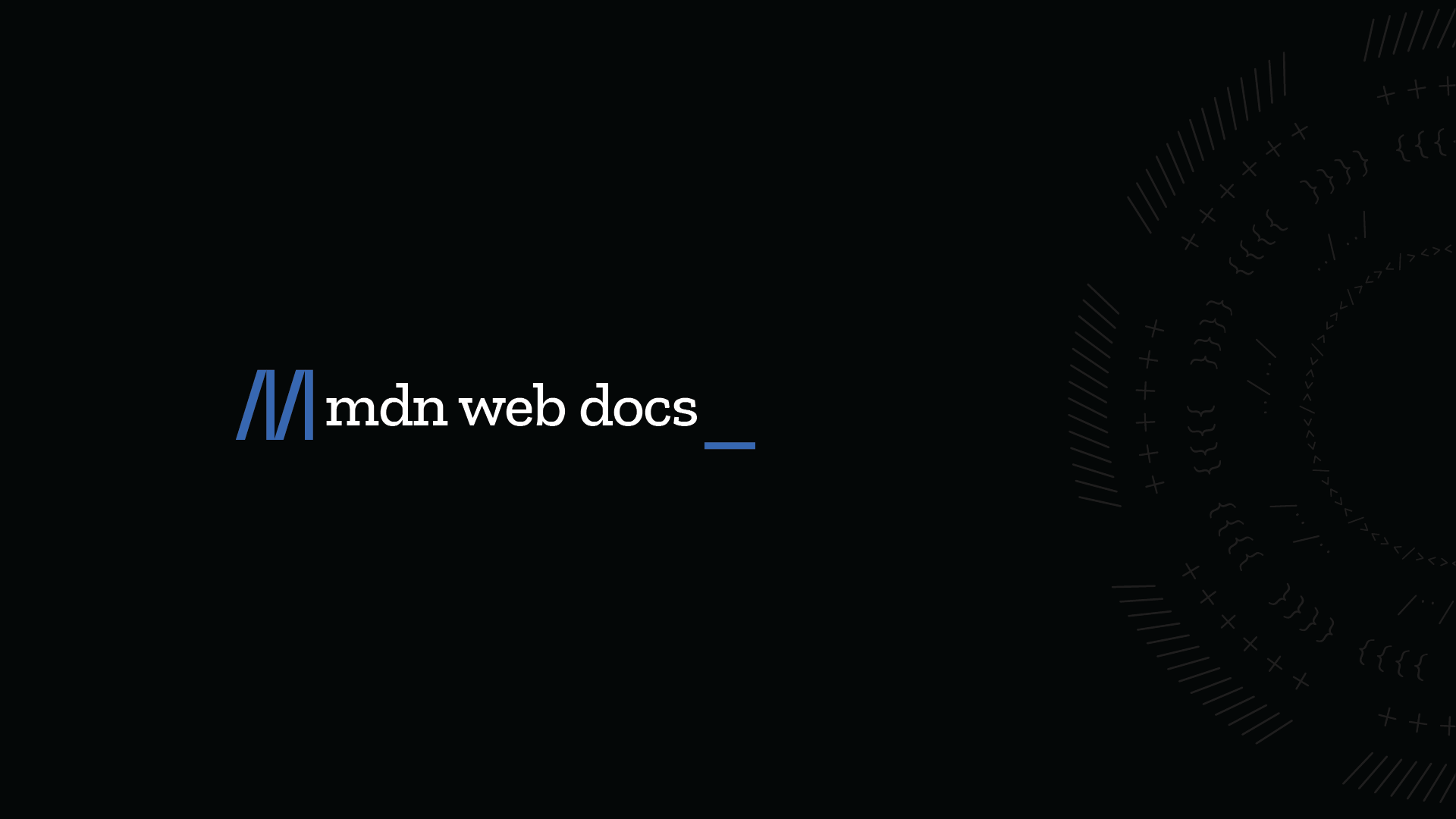
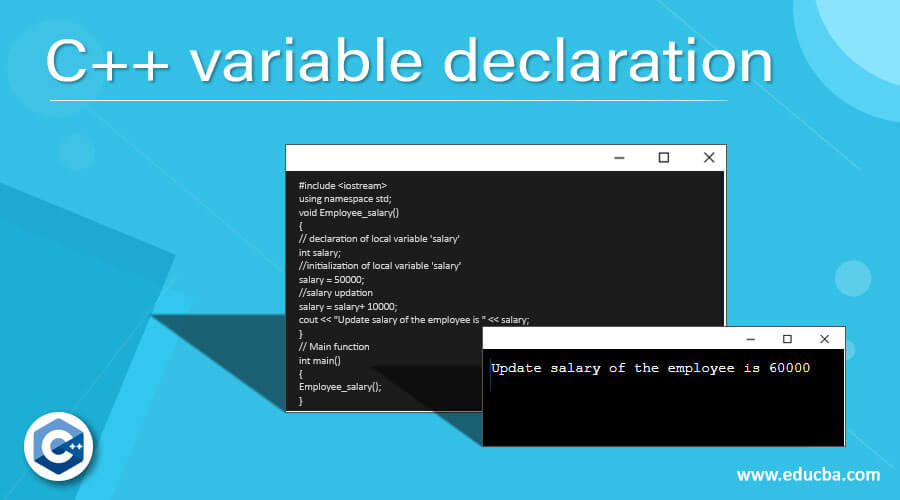

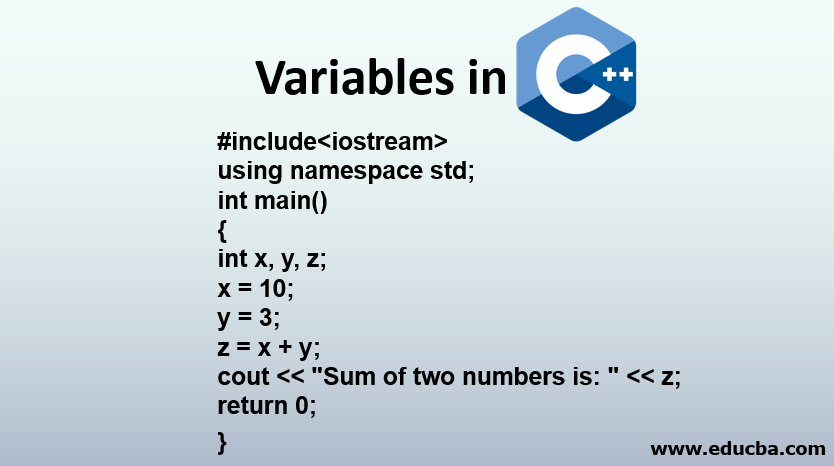


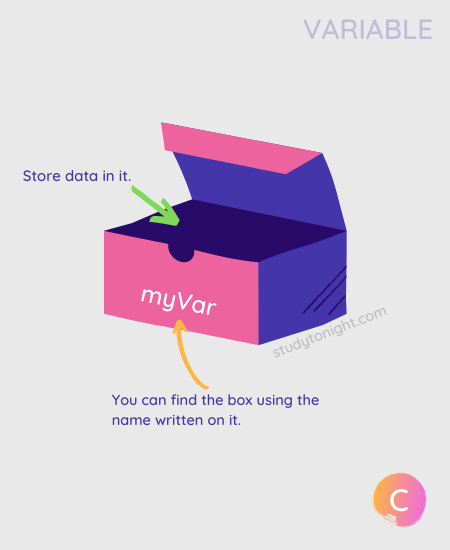
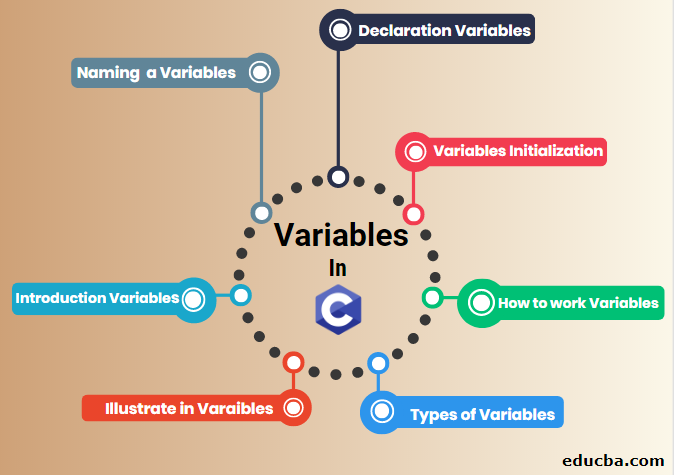

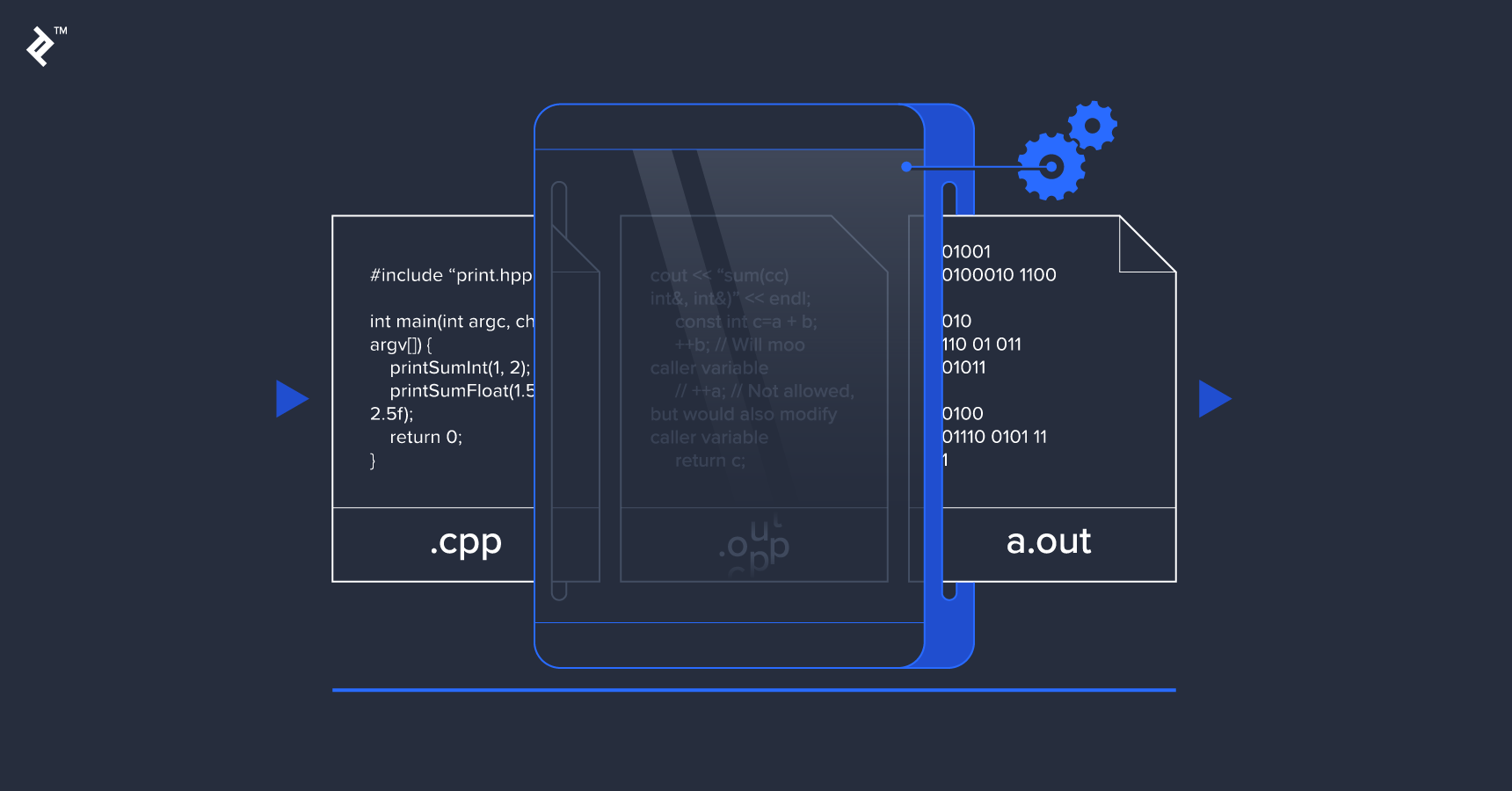
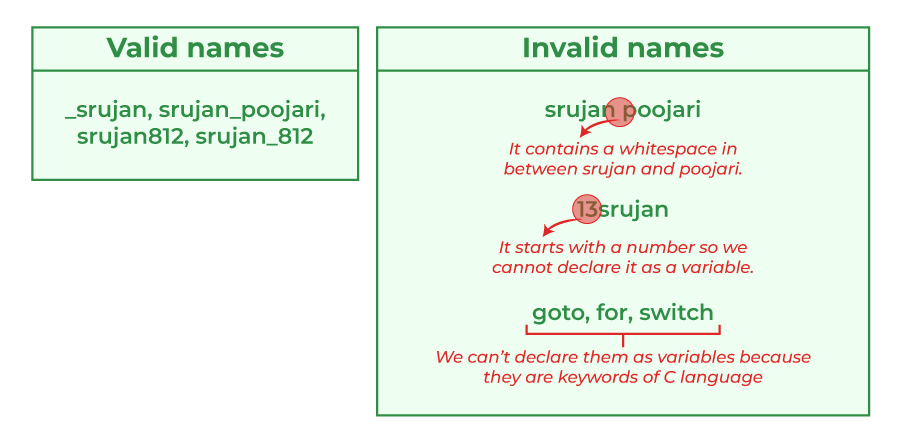
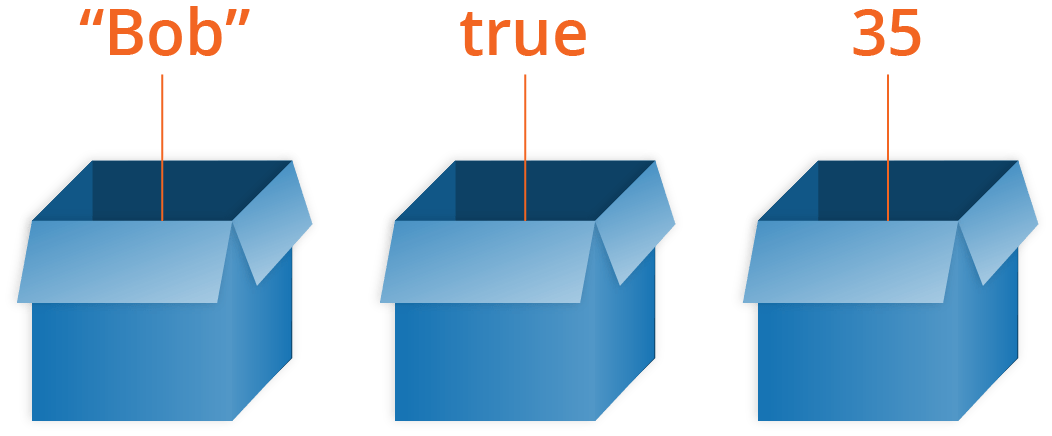
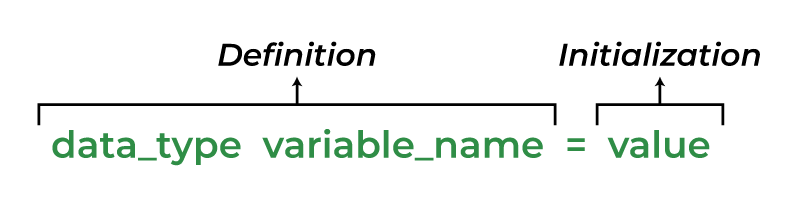
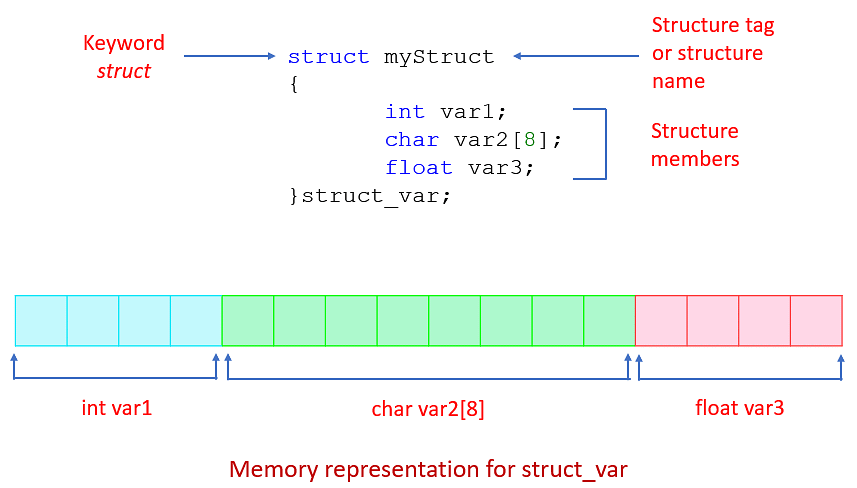
![Global Variable in Python With Examples [Updated] | Simplilearn Global Variable In Python With Examples [Updated] | Simplilearn](https://www.simplilearn.com/ice9/free_resources_article_thumb/GlobalVariableinPython_5.png)
Article link: variable declaration not allowed at this location javascript.
Learn more about the topic variable declaration not allowed at this location javascript.
- Why is variable declaration not allowed as a parameter, but …
- Variable declaration not allowed here… – java help on CodeGym
- Documentation – Variable Declaration – TypeScript
- Variable declaration not allowed here… – java help on CodeGym
- JavaScript Variables – W3Schools
- What Is the Scope of Variables in Javascript and Its Types – Simplilearn
- 4. Variables – JavaScript: The Definitive Guide, 5th Edition [Book]
- variable declaration not allowed here :HashMap – Reddit
- Why variable declaration is not allowed in ( boolean )
- How the let, const, and var Keywords Work in JavaScript
- Solved: Variable declaration in a javascript class
- JavaScript Variables – W3Schools
- Declarations | Documentation – Swift.org
- var – JavaScript – MDN Web Docs – Mozilla
See more: https://nhanvietluanvan.com/luat-hoc