Url Encoding In C#
URL encoding is a crucial concept in web development and programming that involves transforming certain characters into a specific format to ensure their safe transmission across the internet. This article will delve into the details of URL encoding in C#, explaining what it is, why it is necessary, and the various methods available for URL encoding in C#.
What is URL Encoding?
URL encoding, also known as percent-encoding, is a process that replaces reserved or unsafe characters in a URL (Uniform Resource Locator) with a sequence of characters that can be safely transmitted over the internet. Unsafe characters typically include spaces, special symbols, and non-ASCII characters.
Character Encoding in URLs:
URLs are composed of a set of characters, which include alphabets (a-z, A-Z), digits (0-9), and a range of special characters. However, not all characters can be used directly in URLs. Some characters are reserved for specific purposes, such as delimiters or control characters, while others may have special meanings in certain contexts.
For example, the space character, which is represented as a blank space, cannot be directly included in a URL. In its encoded form, the space character is represented by the `%20` sequence. Similarly, other characters have specific encodings that need to be used when they are included in a URL.
Why URL Encoding is Necessary in C#:
URL encoding is necessary to ensure that all URL components, such as query parameters, form data, and path segments, are transmitted and interpreted correctly by web servers, browsers, and other devices. Without proper URL encoding, certain characters may be misinterpreted or lead to unexpected behavior.
For example, if a URL contains spaces instead of their encoded form `%20`, web servers may interpret them as separate path segments or query parameters rather than a single value. This can cause errors, broken links, and incorrect rendering of web pages.
URL Encoding Methods in C#:
C# provides several methods and libraries to handle URL encoding effortlessly. One of the most commonly used methods is the `System.Net.WebUtility.UrlEncode` method. This method takes a string as input and returns its URL-encoded representation.
Here’s an example of using the `UrlEncode` method in C#:
“`csharp
string originalString = “Hello, World!”;
string encodedString = System.Net.WebUtility.UrlEncode(originalString);
“`
In this example, the `UrlEncode` method is used to encode the `originalString`, producing the encoded string `%48%65%6C%6C%6F%2C%20%57%6F%72%6C%64%21`. This encoded string can now be safely included in a URL.
URL Encoding in Form Data:
When transmitting form data via HTTP requests, it is essential to URL encode the data to ensure its proper interpretation by web servers. In C#, the `UrlEncode` method can be used to encode form data before sending it in the body of an HTTP request.
“`csharp
using System;
using System.Net;
class Program
{
static void Main()
{
string formData = “name=John Doe&age=25”;
string encodedFormData = System.Net.WebUtility.UrlEncode(formData);
// Further processing or sending the encoded form data in an HTTP request
}
}
“`
URL Encoding in Query Parameters:
URL query parameters allow information to be passed to web servers via the URL itself. Just like form data, query parameters must also be properly encoded to prevent errors and misinterpretation.
The `UrlEncode` method can be used to encode query parameters as shown below:
“`csharp
using System;
using System.Net;
class Program
{
static void Main()
{
string query = “search=URL Encoding in C#”;
string encodedQuery = System.Net.WebUtility.UrlEncode(query);
// Further processing or appending the encoded query to a URL
}
}
“`
URL Decoding in C#:
Apart from encoding, C# also provides methods for decoding URL-encoded strings. The `System.Net.WebUtility.UrlDecode` method can be used to decode URL-encoded strings back to their original form.
“`csharp
string encodedString = “%48%65%6C%6C%6F%2C%20%57%6F%72%6C%64%21”;
string decodedString = System.Net.WebUtility.UrlDecode(encodedString);
“`
URL Encoding FAQs:
Q: What is the difference between URL encoding and URL decoding?
A: URL encoding involves transforming characters into a specific format for safe transmission, while URL decoding involves converting URL-encoded strings back to their original form.
Q: Can URL encoding be performed online?
A: Yes, several online tools and websites offer URL encoding and decoding functionalities.
Q: Can URL encoding be done in languages other than C#?
A: Yes, URL encoding is a concept applicable to web development in general, so it can be implemented in various programming languages such as JavaScript, Java, and more.
Q: Is there a difference between URL encoding in C# and other languages?
A: The core concept of URL encoding remains the same across languages. However, the methods and libraries used may differ.
In conclusion, URL encoding is a crucial aspect of web development in C#. It ensures safe transmission and interpretation of URL components by replacing reserved or unsafe characters with their encoded counterparts. By utilizing the provided methods and libraries, developers can easily handle URL encoding and decoding in their C# applications, avoiding potential issues and errors.
Url Encoding – Web Development
What Is %2C In Url Encoding?
URL encoding, also known as percent-encoding, is a mechanism used to convert special or reserved characters in URLs (Uniform Resource Locators) into a format that can be safely transmitted and understood by computer systems. One such special character is the comma (,). In URL encoding, the comma is represented by %2C.
URLs contain a wide range of characters, including alphanumeric characters, as well as special characters such as punctuation marks and symbols. However, not all characters can be used in their original form within a URL. These reserved characters have specific meanings in URLs and are used for various purposes.
Reserved characters including the comma (,), question mark (?), slash (/), colon (:), and many others, have specific significance within a URL. Therefore, when any of these reserved characters need to be included in a URL as a part of the actual content rather than having its special meaning, the corresponding URL-encoded form must be used. This prevents any ambiguity in interpreting the URL and ensures proper communication between systems.
The purpose of URL encoding is to ensure that URLs do not contain any characters that may be misinterpreted by web servers, browsers, or other software. This is particularly important when dealing with URLs that include parameters or query strings, as these special characters can easily disrupt the intended functionality of a web application or cause technical issues.
For example, consider a URL that carries a query string parameter called “name”, which takes as its value the string “John, Doe”. If the comma in the name is not encoded, the web server may interpret it as a separator for multiple query parameters. This would result in a misinterpretation of the URL and an incorrect execution of the intended functionality. By encoding the comma as %2C, the web server will correctly understand that it is part of the value for the “name” parameter, and the URL will function as expected.
URL encoding follows a specific pattern. Every special character that needs to be encoded is represented by a percent (%) sign followed by a two-digit hexadecimal number. The hexadecimal value represents the ASCII code of the character being encoded. In the case of the comma, its ASCII code is 44, which translates to 2C in hexadecimal. Hence, the encoded form of the comma is %2C.
In addition to the comma, there are many other reserved characters that have their own corresponding encoding. For instance, the space character is encoded as %20, the question mark as %3F, and the slash as %2F.
FAQs:
Q: Why is it necessary to URL encode special characters?
A: It is necessary to URL encode special characters to ensure that they are not misinterpreted by web servers, browsers, or other software. Failure to properly encode special characters may result in issues such as incorrect functionality, broken links, or even security vulnerabilities.
Q: Are there any other special characters that need encoding?
A: Yes, in addition to the comma, there are several other reserved characters that need to be URL encoded. Some examples include the colon, slash, question mark, equal sign, ampersand, plus sign, and hash symbol, among others. Each of these characters has its own unique encoded representation.
Q: Can a URL contain multiple instances of %2C?
A: Yes, a URL can contain multiple instances of %2C if there are multiple commas that need to be URL encoded. Each occurrence of the comma will be encoded separately as %2C.
Q: Is it necessary to URL encode all characters in a URL?
A: No, it is not necessary to URL encode all characters in a URL. Only reserved characters or characters with special meaning within the URL need to be encoded. Alphanumeric characters, as well as some special characters like hyphen, underscore, and dot, do not need to be encoded.
Q: Is there a difference between URL encoding and percent-encoding?
A: No, URL encoding and percent-encoding are synonyms and refer to the same mechanism of converting special characters into their encoded representation using percent (%) sign followed by a two-digit hexadecimal number.
In conclusion, URL encoding is an essential process for ensuring the proper functioning and interpretation of URLs. The %2C represents the encoding of the comma character, which is a reserved character with a specific meaning in URLs. By encoding the comma as %2C, it can be safely transmitted and understood by computer systems without any misinterpretation. URL encoding is crucial for avoiding technical issues, maintaining consistency, and enhancing the overall reliability of web applications.
What Is %25 In Url?
When browsing the internet, you may have come across URLs that contain strange characters or symbols. One such symbol is %25. But what does this symbol mean, and why is it used in URLs? In this article, we will delve deeper into the concept of %25 in URLs and explore its significance.
URLs, or Uniform Resource Locators, are the addresses that we use to locate and access web pages on the internet. They consist of various components, including a protocol (such as HTTP or HTTPS), a domain name, and a path or file name. Sometimes, URLs also contain additional characters to represent special characters, spaces, or reserved symbols, which otherwise cannot be directly included in a URL.
The use of %25 in a URL denotes the percent-encoded value of the character “%” itself. Percent encoding is a method to represent characters in URLs using a combination of ASCII characters. It is employed when a character is not allowed or could be misinterpreted within a URL. In this case, the percent-encoded value %25 specifically represents the “%” character.
This may lead to confusion as the “%” symbol is typically associated with percent-encoded values. For instance, %20 represents a space character, %23 represents a hash symbol (#), and %3F represents a question mark (?). However, when the percentage sign itself needs to be included as a part of the URL, it is encoded as %25.
The need for percent encoding arises due to the restrictions imposed by the URL standard, which limits the use of certain characters, such as spaces or reserved symbols, in URLs. If these characters are included without encoding, they may disrupt the structure of the URL or result in a different interpretation of its meaning.
To better understand this concept, let’s take an example. Suppose you want to include the symbol “%” in a URL. If you were to directly enter “%” into the URL, the browser may interpret it as a special character indicating a percent-encoded value. Consequently, the browser would expect to find two hexadecimal digits following the symbol “%,” resulting in an erroneous URL.
By using %25 instead, you are indicating that the “%” character should be treated as a literal character and not as part of a percent-encoded value. This helps to ensure that the symbol is correctly interpreted and does not result in any unintended consequences.
FAQs:
Q: How is %25 used in URLs?
A: %25 is used to represent the “%” character in URLs when the percent sign itself needs to be included as a part of the URL. It is a percent-encoded value that helps prevent ambiguity and ensure the correct interpretation of the symbol.
Q: Are there any other percent-encoded values in URLs?
A: Yes, there are numerous percent-encoded values used in URLs. For example, %20 represents a space character, %23 represents a hash symbol (#), and %3F represents a question mark (?). These values help in encoding characters that are not allowed or could be misinterpreted within a URL.
Q: Can %25 be used to encode other characters?
A: No, %25 is specifically used to represent the “%” character in URLs. It cannot be used to encode other characters. Each character has its own unique percent-encoded value.
Q: How can I manually percent encode a URL?
A: If you need to manually percent encode a URL, you can use online tools or libraries that provide URL encoding functions. Alternatively, you can reference the ASCII table to determine the hexadecimal values of the characters you want to encode and then replace them with the corresponding percent-encoded values.
Q: Is percent encoding the same as URL encoding?
A: Yes, percent encoding and URL encoding refer to the same concept. Percent encoding is the process of representing characters in URLs using a combination of ASCII characters, while URL encoding is a term often used to describe this process.
In conclusion, %25 in URLs represents the percent-encoded value of the character “%”. It is used to ensure that the percent sign is interpreted as a literal character and not as part of a percent-encoded value. By understanding and utilizing percent encoding, we can navigate the complexities of URLs and access the web content we desire without any issues.
Keywords searched by users: url encoding in c# c url decode, URL encode, URL encoding online, URL encode C#, url encoding and decoding in c#, URL encode JavaScript, URL encode java, url encode source code
Categories: Top 95 Url Encoding In C#
See more here: nhanvietluanvan.com
C Url Decode
Introduction:
C URL decoding is an essential technique used in programming to decode and convert URL-encoded data back into its original form. In this article, we will delve into the intricacies of C URL decoding, explore its significance in web development, discuss the decoding process, and provide some frequently asked questions (FAQs) to clarify common concerns.
Why is URL Decoding Important?
In the digital age, the internet is flooded with data transmission, and URLs play a pivotal role in facilitating communication between web servers and clients. Often, URLs contain characters that necessitate encoding to ensure compatibility with web standards and prevent issues like ambiguous parsing or data corruption. To resolve this, URL encoding converts certain characters to their corresponding ASCII hexadecimal representation, preceded by a percent sign (%). Consequently, decoding allows the original data to be accurately retrieved, making it essential in programming tasks such as handling form submissions, parsing query strings, and manipulating URLs.
URL Decoding Process:
The C language provides numerous libraries and functions to decode URL-encoded data. However, the most commonly used method is through manual decoding using the algorithmic process. Let’s outline the steps involved:
Step 1: Analysis
The first step is to analyze the URL-encoded string and identify any encoded characters. Encoded characters are represented by the percent sign followed by two hexadecimal digits. For example, “%20” represents a space character.
Step 2: Character Replacement
Once the encoded characters are identified, they must be replaced by their original values. This requires converting the hexadecimal representation to its corresponding ASCII value. For example, ‘%20′ is converted to ’32’, which is the ASCII value for a space character.
Step 3: Rebuilding the String
After replacing the encoded characters, the URL-decoded string is rebuilt by concatenating the decoded characters together in the correct order. This can be done using various string manipulation techniques, such as copying into a new array or overwriting the original encoded string.
Common Functions in C for URL Decoding:
C provides certain functions that can simplify the URL decoding process. The most common function is `strchr()` or `strrchr()`, which is used for locating and isolating individual encoded characters in the URL-encoded string. Additionally, the `sscanf()` function can be employed to convert the extracted hexadecimal value back into its decimal ASCII representation. Lastly, the `sprintf()` function allows for the concatenation and reconstruction of the URL-decoded string.
FAQs:
Q1. Is URL decoding the same as URL parsing?
A1. No, URL decoding and parsing are two distinct processes. URL decoding involves converting encoded characters back to their original form, whereas parsing refers to dissecting a URL into its components, such as protocol, domain, path, or query parameters.
Q2. What are the common situations where URL decoding is necessary?
A2. URL decoding is particularly crucial when handling web form submissions that contain special characters or when parsing query strings. It ensures proper data transmission and maintains the integrity of the information being exchanged.
Q3. Can C URL decoding handle all types of characters?
A3. C URL decoding is primarily designed to handle standard ASCII characters. However, it may encounter limitations when handling higher Unicode characters, for which more specialized libraries and functions are available.
Q4. Are there any security concerns with URL decoding?
A4. URL decoding itself poses no security risks. However, developers must be cautious when using decoded URL parameters in subsequent operations to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS).
Q5. Is C URL decoding limited to web development?
A5. While C URL decoding finds extensive usage in web development, it can also be utilized in other scenarios where URLs or encoded strings need to be manipulated, processed, or analyzed, such as network protocols, data manipulation, or custom file transfer mechanisms.
Conclusion:
C URL decoding plays a fundamental role in web development and other programming domains by ensuring the accurate retrieval of URL-encoded data. In this article, we have discussed the significance of URL decoding, the decoding process, and highlighted common functions used in C. By understanding these concepts and employing the appropriate techniques, programmers can effectively handle URL-encoded data and enhance their software applications’ functionality.
Url Encode
In today’s digital age, information sharing is at its peak. The widespread use of the internet has revolutionized how we communicate and access information. But have you ever wondered how all the data, including text, images, and videos, flow through the vast network of computers? One important aspect of this process is URL encoding. In this article, we will delve into the depths of URL encoding, its significance, and answer some frequently asked questions – equipping you with a comprehensive understanding of this vital aspect of web technology.
URL Encoding Explained
URL encoding, also known as percent encoding, is a mechanism used to convert certain characters in a URL to a specific format before transmitting them over the internet. This encoding ensures that the URL remains valid and that the data contained within it is correctly processed by web browsers, servers, and other networking components.
The Purpose of URL Encoding
URL encoding serves several key purposes:
1. Character Set Compatibility: URLs contain a specific set of characters allowed within them. When non-alphanumeric characters (such as spaces and special characters) need to be included in a URL, they are first encoded to a format compatible with the standard ASCII character set. This conversion prevents any conflicts or misinterpretation by various components involved in data transmission.
2. Data Integrity: Since URLs are often used to transfer data, it is crucial to ensure the integrity of this data. URLs should not break or distort during transmission. URL encoding preserves the accuracy of special characters, maintaining the integrity of the data contained within the URL.
3. Security: URL encoding plays a significant role in securing the information being transmitted. Characters like spaces or special characters can have different meanings when interpreted by different software systems. By using URL encoding, potential security vulnerabilities are minimized, ensuring that the data remains secure.
URL Encoding Process
The URL encoding process is relatively straightforward. Non-alphanumeric characters are converted into a specific format that starts with a percentage sign (%), followed by two hexadecimal digits representing the ASCII code for that character. For example, the space character ‘ ‘ is encoded as %20, while the ampersand ‘&’ is encoded as %26. This standardized format ensures that both the sender and receiver understand the encoded representation, avoiding any ambiguity in interpretation.
Commonly Encoded Characters
Certain special characters frequently require URL encoding. Here are a few commonly encountered ones:
1. Space: Encoded as %20, a space is commonly replaced by ‘+’ as well. However, using ‘%20’ is a more accurate and browser-agnostic approach.
2. Ampersand (&): Represented as %26 in URL encoding, the ampersand is frequently used to separate different parameters in a URL.
3. Equals (=) and Question Mark (?): These characters are encoded as %3D and %3F, respectively. They are extensively used within URLs to assign values to parameters or separate the URL’s base part from its query string.
4. Percent Sign (%): As this character is used to initiate URL encoding, it must be encoded itself as %25 so that it does not cause any confusion.
URL Encoding in Programming Languages
URL encoding is not limited to web browsers; it is also an integral part of programming languages. Most programming languages offer URL encoding functions to handle this process conveniently. For example, in JavaScript, the encodeURIComponent() and encodeURI() functions can be used to encode URLs. Similarly, other languages like PHP, Python, and Java provide similar functionalities to encode and decode URLs.
URL Encoding FAQs:
Q: Is URL encoding the same as URL decoding?
A: No, they are not the same. URL encoding is the process of converting special characters into a standardized format, while URL decoding reverses this process to retrieve the original characters.
Q: If I don’t encode a URL, will it still work?
A: It may work in some cases, but it is strongly advised to URL encode any special characters to avoid inconsistencies and issues in data transmission.
Q: Are there any limitations on the length of a URL?
A: Yes, URLs have a maximum length determined by various factors such as web browsers, servers, and networking equipment. The maximum length varies between different implementations but is typically around 2,000-8,000 characters.
Q: Can URL encoding impact SEO?
A: URL encoding itself does not have a direct impact on SEO. However, well-constructed, human-readable URLs are generally preferred for search engine optimization.
URL encoding ensures the smooth flow of data across the internet, promoting compatibility, integrity, and security. By encoding special characters, URLs become universally understood, preventing any potential issues during transmission. Understanding and utilizing URL encoding properly is essential for web developers, programmers, and anyone involved in transferring data online. Mastering this mechanism will undoubtedly boost your confidence in harnessing the power of the internet while ensuring the seamless transfer of information – a fundamental principle in today’s interconnected world.
Url Encoding Online
In today’s digital era, URLs (Uniform Resource Locators) have become an integral part of our lives. Whether we are navigating websites, sharing links, or communicating through emails, URLs play a crucial role in enabling the seamless transmission of data on the internet. However, not all characters can be used directly in a URL. This is where URL encoding comes into the picture. In this article, we will explore URL encoding online, its importance in data transmission, and address some frequently asked questions about this vital technique.
What is URL Encoding?
URL encoding, also known as percent-encoding, is a process of converting character strings into a format that can be safely transmitted over the internet. It ensures that the data being sent is compatible with the URL structure and is not misinterpreted or lost during transmission. URLs can only contain a limited set of alphanumeric characters, along with a few special characters reserved for specific purposes, such as determining parameters or indicating file types.
URL encoding is necessary when a URL contains characters outside this permissible set, such as whitespace, non-alphanumeric symbols, or characters with special meanings in the URL syntax, like ampersands (&) or question marks (?). By encoding these characters into a specific format, they become compatible with the URL structure and can be safely transmitted.
The Process of URL Encoding:
URL encoding follows a simple process to ensure that all characters in a URL are properly encoded. Each character that needs encoding is replaced by a percent sign (“%”) followed by two hexadecimal digits representing the character’s ASCII code. For example, the space character, which is represented as a space (” “), gets encoded as “%20” in a URL.
While the process may seem straightforward, implementing URL encoding can be complex, especially when dealing with dynamic data like user inputs, variables, or form submissions. However, most web development frameworks, programming languages, and online tools have built-in functions for URL encoding that make it easier to handle.
The Importance of URL Encoding:
URL encoding is essential for ensuring the correct transmission of data on the internet. By encoding characters that are not allowed in a URL, it prevents errors and misinterpretations that can result in broken links, incorrect data processing, or security vulnerabilities.
Moreover, URL encoding also plays a significant role when passing parameters or data through URLs. For instance, search queries or form submissions containing spaces or special characters need to be encoded to be safely transmitted. Without URL encoding, these characters may disrupt the URL structure, leading to unpredictable behavior or errors.
Frequently Asked Questions about URL Encoding:
Q: Can URL encoding alter the original data?
A: No, URL encoding does not change the actual content of the data being encoded. It simply modifies the representation of characters for their compatibility in a URL.
Q: Are there any online tools available for URL encoding?
A: Yes, numerous online tools provide URL encoding services. These tools allow users to input their data and instantly receive the encoded URL, simplifying the process for those who don’t have programming expertise.
Q: When should URL encoding be applied?
A: URL encoding should be applied whenever a URL contains characters outside the permitted range. This includes spaces, special characters, or non-alphanumeric symbols.
Q: How does URL encoding impact SEO?
A: URL encoding primarily focuses on ensuring the integrity of data during transmission, and its impact on SEO is minimal. However, SEO best practices recommend using clean and readable URLs, which may require encoding reserved characters like spaces using hyphens or underscores.
Q: Can URL encoding solve all URL-related issues?
A: While URL encoding is crucial for data transmission and URL integrity, it does not address all potential URL-related issues. Other considerations, such as URL length, accessibility, or server-side limitations, should also be taken into account for optimal URL handling.
In conclusion, URL encoding is an essential technique for maintaining the integrity and compatibility of data transmitted through URLs. By converting non-permissible characters into URL-safe representations, URL encoding ensures a smooth flow of information across the internet. Understanding the process of URL encoding and its significance is key to effectively working with URLs and avoiding errors or vulnerabilities.
Images related to the topic url encoding in c#
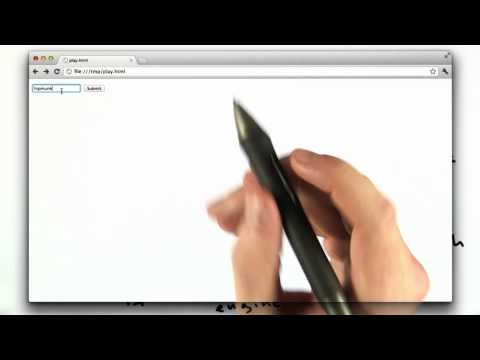
Article link: url encoding in c#.
Learn more about the topic url encoding in c#.
- urlencode – C – URL encoding – Stack Overflow
- URL Encoding in C (urlencode / encodeURIComponent)
- HTML URL Encoding Reference – W3Schools
- URL Encoding/Decoding in C – Geek Hideout
- URL encoding – Rosetta Code
- What is %2C in a URL? – Stack Overflow
- In URL `%` is replaced by `%25` when using `queryParams` while routing …
- HTML URL Encoding Reference – W3Schools
- HTML URL Encoding – W3Schools
- HttpUtility.UrlEncode Method (System.Web) – Microsoft Learn
- Working and Examples of C# URL Encode – eduCBA
- URL encoding – Wikipedia
- Objective-C and Swift URL encoding – Tutorialspoint
- HTML | URL Encoding – GeeksforGeeks
See more: https://nhanvietluanvan.com/luat-hoc