Update Document Requires Atomic Operators
Introduction to Atomic Operators: Defining the Concept and Significance
In the world of document updates, atomic operators play a crucial role in ensuring data integrity and consistency. With the increasing complexity of data operations, it has become essential to have atomic operators that can update documents in a reliable and efficient manner. Atomic operators are specialized database operations that perform an update on a single document in a single operation, guaranteeing that no other operations can interfere or create inconsistencies.
Benefits of Using Atomic Operators in Document Updates
1. Data Consistency: Atomic operators ensure that updates are performed in a consistent manner, without any partial or incomplete modifications. This prevents data corruption and maintains the integrity of the overall collection.
2. Improved Performance: By performing updates in a single operation, instead of multiple separate operations, atomic operators significantly enhance the performance of document updates. This reduces the overhead and resource consumption associated with multiple operations.
3. Concurrent Multi-User Support: Atomic operators allow for concurrent updates by multiple users without the risk of conflicting modifications. This ensures that the data remains accurate and consistent even in a highly concurrent environment.
Understanding the Purpose and Functionality of Atomic Operators
Atomic operators provide a wide array of functionalities for performing document updates. Some of the key functionalities include:
1. Field Modification: Atomic operators allow for precise modifications to specific fields within a document, making it easier to update only the required attributes.
2. Conditional Updates: Atomic operators enable conditional updates, where the modification is applied only if certain conditions are met. This provides greater control over the update process and allows for dynamic updates based on specific criteria.
3. Array Manipulation: Atomic operators support various operations on arrays, such as adding elements, removing elements, modifying specific elements, and working with arrays as sets.
4. Atomic Increment and Decrement: Atomic operators offer built-in functionalities to increment or decrement numeric values atomically. This is particularly useful for scenarios where counters or statistics need to be updated reliably.
Commonly Used Atomic Operators in Document Updates
1. $set: This operator sets the value of a field in a document. It can be used to modify existing fields or add new fields if they don’t already exist.
2. $unset: The $unset operator removes a specific field from a document.
3. $inc: This operator increments the value of a numeric field by a specified amount.
4. $push: The $push operator appends a value to an array field.
5. $pull: This operator removes all instances of a specified value from an array field.
Syntax and Usage Examples for Each Atomic Operator
Here are some syntax and usage examples for the commonly used atomic operators in document updates:
1. $set:
db.collection.update({ _id: ObjectId(“…”) }, { $set: { field: value } })
2. $unset:
db.collection.update({ _id: ObjectId(“…”) }, { $unset: { field: 1 } })
3. $inc:
db.collection.update({ _id: ObjectId(“…”) }, { $inc: { field: increment } })
4. $push:
db.collection.update({ _id: ObjectId(“…”) }, { $push: { arrayField: value } })
5. $pull:
db.collection.update({ _id: ObjectId(“…”) }, { $pull: { arrayField: value } })
Best Practices for Implementing Atomic Operators in Document Updates
To ensure the effective implementation of atomic operators in document updates, it is essential to follow these best practices:
1. Carefully Plan Updates: Clearly define the desired update operation, including the fields to be modified, conditions to be met, and the expected outcome.
2. Minimize Queries: Perform updates in a single operation whenever possible, rather than making multiple separate queries. This helps reduce network latency and improves performance.
3. Use Indexes: Ensure that the fields used in update operations are appropriately indexed for better performance. This can significantly speed up the update process, especially for large collections.
Potential Challenges and Considerations While Using Atomic Operators
While atomic operators offer many benefits, there are certain challenges and considerations to keep in mind while working with them:
1. Atomicity Limitation: Atomic operators are designed for performing updates on a single document. Performing operations on multiple documents concurrently may require additional measures to ensure atomicity.
2. Limited Data Validation: Atomic operators do not provide extensive data validation capabilities. It is crucial to validate inputs before performing updates to maintain the integrity of the dataset.
Real-World Use Cases Illustrating the Use of Atomic Operators
1. E-commerce Inventory Management: Atomic operators can be used to update product quantities atomically when a purchase is made, ensuring accurate stock levels without risk of inconsistencies.
2. Social Media Interaction: Updating the likes or shares count on social media posts can be done atomically using atomic operators, preventing any race conditions and maintaining accurate statistics.
Future Advancements and Trends in Atomic Operations for Document Updates
As the demand for efficient data operations continues to grow, the development of new and more advanced atomic operators is expected. These future advancements may focus on increasing the flexibility and functionality of atomic operators, enabling even more precise and powerful document updates.
FAQs
Q: What is the “Update document requires atomic operators” error?
A: The “Update document requires atomic operators” error typically occurs when performing an update operation without using atomic operators in environments like MongoDB.
Q: How can I use atomic operators in Node.js for updating documents?
A: In Node.js, you can use libraries like Mongoose or the native MongoDB driver to perform atomic updates using the provided atomic operators.
Q: How can I update multiple documents using atomic operators in MongoDB?
A: Atomic operators are designed to update a single document. To update multiple documents atomically, consider using a combination of atomic operations and transactions.
Q: What is the difference between atomic increment and normal increment?
A: Atomic increment ensures that the operation is performed atomically in a single step, preventing race conditions. Normal increment is not guaranteed to be atomic and may result in inconsistencies when performed concurrently.
Q: Are atomic operators supported in other database systems?
A: Atomic operators are not exclusive to MongoDB. Many modern databases provide similar functionalities to ensure atomicity and data integrity during document updates.
Q: Can I update a subfield of a document using atomic operators?
A: Yes, atomic operators can be used to update subfields of a document. By specifying the subfield path in the update query, you can perform atomic updates on specific subfields.
How To Update Document In Mongodb ( Updateone Vs Updatemany In Mongodb )
How To Update A Document In Mongodb?
MongoDB is a popular and flexible NoSQL database used by developers worldwide. One of its key features is its ability to store and retrieve data in a document-oriented format. MongoDB documents are similar to JSON objects, consisting of key-value pairs that can be nested and indexed. In this article, we will explore how to update a document in MongoDB and delve into some commonly faced questions related to this topic.
Updating a document in MongoDB involves modifying one or more existing fields or adding new fields to an existing document. There are several methods available for updating documents within a MongoDB collection. Let’s explore a few of them:
1. UpdateOne():
The UpdateOne() method is used to update a single document that matches a specific filter. It takes two parameters: a filter object to identify the document and an update object to define the changes. For example, let’s assume we have a collection called “users”, and we want to update the age of a user with an ID of 123. The following code snippet demonstrates how to achieve this:
“`javascript
db.users.updateOne({ _id: 123 }, { $set: { age: 25 } });
“`
In this example, the filter object `{ _id: 123 }` matches the document with the specified ID, and the update object `{ $set: { age: 25 } }` sets the “age” field to 25.
2. UpdateMany():
The UpdateMany() method allows updating multiple documents that match a specific filter. This method is useful when you want to apply the same update to multiple documents simultaneously. The following example demonstrates how to increment the “likes” field of multiple posts authored by a particular user:
“`javascript
db.posts.updateMany({ author: “john_doe” }, { $inc: { likes: 1 } });
“`
In this example, the filter object `{ author: “john_doe” }` selects all documents with the specified author, and the update object `{ $inc: { likes: 1 } }` increments the “likes” field by 1.
3. findOneAndUpdate():
The findOneAndUpdate() method fetches the first document that matches the filter and atomically updates it. This method is commonly used when you need to obtain the existing document and make changes to it in a single operation. For example, let’s assume we have an inventory collection, and we want to change the price of a specific product and return the updated document:
“`javascript
const updatedProduct = db.inventory.findOneAndUpdate(
{ _id: 456 },
{ $set: { price: 9.99 } },
{ returnDocument: “after” }
);
console.log(updatedProduct);
“`
In this example, the filter object `{ _id: 456 }` selects the document based on the provided ID, and the update object `{ $set: { price: 9.99 } }` modifies the price field. The `{ returnDocument: “after” }` option ensures that the updated document is returned.
FAQs
Q: Can I update only a specific field within a document while leaving other fields untouched?
A: Yes, you can use the `$set` operator to update a specific field without altering the remaining fields. For example:
“`javascript
db.users.updateOne({ _id: 789 }, { $set: { email: “[email protected]” } });
“`
Q: How can I remove a field from a document?
A: The `$unset` operator can be used to remove a particular field from a document. For instance:
“`javascript
db.products.updateOne({ _id: 123 }, { $unset: { description: “” } });
“`
Q: Is it possible to update an array element within a document?
A: Indeed, you can use the `$set` operator with the positional `$` operator to update specific elements within an array. For example:
“`javascript
db.users.updateOne(
{ _id: 789, “preferences.color”: “blue” },
{ $set: { “preferences.$.size”: “medium” } }
);
“`
Q: Can I update multiple fields within a document at once?
A: Yes, you can combine multiple update operators within a single update object to update multiple fields simultaneously. Here’s an example:
“`javascript
db.users.updateOne(
{ _id: 123 },
{ $set: { age: 30, email: “[email protected]” } }
);
“`
In conclusion, updating documents in MongoDB is a crucial aspect of application development. With the various update methods provided by MongoDB, you have the flexibility to modify documents in a targeted and efficient manner. Understanding these methods and their usage will empower you to maintain and update your MongoDB collections effectively.
What Is Atomic Operators In Mongodb?
MongoDB is a popular NoSQL database that provides a flexible and scalable solution for handling large amounts of data. One of the key features of MongoDB is its support for atomic operators. Atomic operators allow you to perform complex operations on documents in a single atomic, or indivisible, operation. In this article, we will explore what atomic operators are, how they work in MongoDB, and their importance in ensuring data integrity.
Understanding Atomicity
Atomicity refers to the concept of indivisible operations. In the context of databases, atomicity ensures that a series of database operations are treated as a single, all-or-nothing unit. If any part of the operation fails, the entire operation is rolled back to its original state.
In MongoDB, when performing write operations, atomicity is achieved at the document level. Each operation that modifies a document or a group of documents is atomic. This is possible due to MongoDB’s powerful atomic operators.
Common Atomic Operators in MongoDB
MongoDB provides a rich set of atomic operators, each designed to perform specific actions on documents. Let’s explore some of the most commonly used atomic operators.
1. $set: This operator allows you to modify specific fields in a document without affecting other fields. For example, you can use “$set” to update the value of a field or add a new field to a document.
2. $unset: The “$unset” operator removes a specific field from a document. This can be useful when you need to delete unnecessary fields or reset the value of a particular field.
3. $inc: The “$inc” operator increments the value of a numeric field by a specific amount. You can also use negative values to decrement the field value.
4. $push: This operator appends an element to an existing array field in a document.
5. $pull: The “$pull” operator removes all instances of a specified value from an array field.
6. $addToSet: This operator adds an element to an array field only if it doesn’t already exist in the array.
Importance of Atomic Operators
Atomic operators play a crucial role in ensuring data integrity and consistency in MongoDB. Here are a few reasons why atomic operators are important:
1. Consistency: Atomic operators prevent data corruption by ensuring that operations are performed in an atomic manner. This means that if an update operation fails midway, the database rolls back to its original state, preserving data consistency.
2. Performance: By using atomic operators, you can perform complex operations within a single command, reducing the number of round trips between your application and the database server. This can significantly improve performance, especially when dealing with large volumes of data.
3. Simplicity: Atomic operators simplify the updating process, as you don’t need to fetch the entire document, modify it, and send it back to the database. Instead, you can perform targeted updates on specific fields, reducing network overhead and increasing efficiency.
Frequently Asked Questions
Q: Can I use multiple atomic operators in a single update command?
A: Yes, you can use multiple atomic operators in a single update command. This allows you to perform complex operations on documents in a single transaction-like operation.
Q: Are atomic operators limited to specific types of data?
A: No, atomic operators can be used with various data types like strings, numbers, arrays, and more. MongoDB provides specific atomic operators for each type of operation.
Q: Can I perform atomic operations on multiple documents?
A: Yes, atomic operators can be used on multiple documents as well. You can use query selectors to specify the documents you want to update and apply atomic operators to modify their fields.
Q: Do atomic operators work with nested fields in a document?
A: Yes, atomic operators can be used to modify nested fields in a document. You can navigate through the nested structure using dot notation and update specific fields using the appropriate atomic operator.
Conclusion
Atomic operators in MongoDB provide a powerful mechanism for performing complex operations on documents with atomicity. They help ensure data integrity, improve performance, and simplify the updating process. By understanding and utilizing the various atomic operators available in MongoDB, you can build efficient and scalable database applications.
Keywords searched by users: update document requires atomic operators Update document requires atomic operators nodejs, MongoDB update operators, Mongoinvalidargumenterror Update document requires atomic operators, Pymongo update one, Atomic operator mongodb, Mongodb update all where, MongoDB update field by another field, MongoDB update subfield
Categories: Top 76 Update Document Requires Atomic Operators
See more here: nhanvietluanvan.com
Update Document Requires Atomic Operators Nodejs
In Node.js, updating documents in a database is a common task for developers. However, when working with multiple concurrent requests, ensuring data consistency becomes crucial. Node.js provides atomic operators to update documents atomically, preventing race conditions and maintaining data integrity.
Atomic operators are unique functions in Node.js that perform atomic operations on documents in a database. These operations modify the document in a single, indivisible operation, ensuring that other concurrent requests do not interfere with the update process. By using atomic operators, developers can update documents without worrying about inconsistent or corrupted data.
Why are atomic operators important in Node.js?
In a traditional programming paradigm, updating a document involves fetching the document, modifying it, and saving it back to the database. However, in a concurrent environment where multiple requests can access the same document simultaneously, race conditions can occur. A race condition is a situation in which multiple operations interfere with each other, leading to unexpected and incorrect results.
To illustrate this, let’s consider a scenario where two users simultaneously request to update the same document with different data. Without atomic operators, both requests might read the document, perform their respective modifications, and then save it back to the database. However, the last request to save the document would overwrite the changes made by the first request, leading to data inconsistencies.
With atomic operators, the update operation is performed as a single, indivisible unit. Other concurrent requests are blocked until the update operation is completed, ensuring that no other request interferes with the update process. This prevents race conditions and maintains data consistency.
What are some common atomic operators in Node.js?
Node.js provides several atomic operators that can be used to perform atomic updates on documents. Here are some commonly used ones:
1. $set: The $set operator updates the value of a field in a document. It sets the specified field to the specified value, creating the field if it does not exist.
2. $unset: The $unset operator removes the specified field from a document.
3. $inc: The $inc operator increments the value of a field in a document by the specified amount.
4. $push: The $push operator appends an element to an array field in a document.
5. $pull: The $pull operator removes all instances of a specified value from an array field in a document.
How to use atomic operators in Node.js?
To use atomic operators in Node.js, you need to interact with a database that supports them. MongoDB, for example, is a popular NoSQL database that supports atomic operators. Here’s an example of how you can use atomic operators in Node.js with MongoDB:
“`
const MongoClient = require(‘mongodb’).MongoClient;
const url = ‘mongodb://localhost:27017’;
const dbName = ‘mydatabase’;
MongoClient.connect(url, function(err, client) {
const db = client.db(dbName);
const collection = db.collection(‘mycollection’);
// Update document atomically using $set operator
collection.updateOne(
{ _id: ObjectId(“12345”) },
{ $set: { field: newValue } }
);
client.close();
});
“`
In this example, we connect to a local MongoDB instance, specify the database name and collection, and use the `updateOne` method to atomically update a document. The first argument to `updateOne` specifies the query that matches the document to be updated, while the second argument uses the `$set` operator to update a specific field.
Frequently Asked Questions (FAQs):
Q: Can atomic operators be used with other databases besides MongoDB?
A: Yes, other databases like Couchbase and Firebase also support atomic operators in Node.js. However, the specific syntax and usage might differ depending on the database.
Q: Can atomic operators work with multiple fields in a single update operation?
A: Yes, you can use multiple atomic operators together to update multiple fields within a single update operation. For example, you can use `$set` to update one field and `$inc` to update another field in the same operation.
Q: Do atomic operators guarantee the order of update operations?
A: Atomic operators ensure atomicity and prevent race conditions, but they do not guarantee the order of update operations. If order matters, you should explicitly handle it in your code.
Q: Do atomic operators lock the entire collection during an update operation?
A: No, atomic operators do not lock the entire collection. They provide document-level atomicity, allowing other documents in the collection to be accessed and updated concurrently.
Q: Are there any performance implications when using atomic operators?
A: While atomic operators ensure data integrity, they might introduce a small performance overhead due to the additional locking and synchronization mechanisms. However, the performance impact is generally negligible unless dealing with a large number of concurrent requests.
In conclusion, atomic operators are valuable tools for ensuring data consistency when updating documents in Node.js. They provide a way to perform atomic updates, preventing race conditions and maintaining data integrity. By using atomic operators, developers can confidently update documents in a concurrent environment without worrying about inconsistent or corrupted data.
Mongodb Update Operators
MongoDB, a NoSQL database, offers a powerful and flexible way to manage your data. One of the key features that make MongoDB so popular is its update operators. These operators allow you to modify the values of existing documents, append elements to arrays, and perform other common data manipulation tasks without having to retrieve and reinsert the entire document into the database. In this article, we will explore the various update operators available in MongoDB and dive deep into their functionalities.
Understanding MongoDB Update Operators
Update operators in MongoDB are an essential part of the update process. They provide a flexible way to modify documents in a collection without needing to replace the entire document. By leveraging these operators, you can make targeted and efficient updates to specific fields or arrays within a document.
MongoDB provides a wide range of update operators, each serving a different purpose. Let’s take a closer look at some of the most commonly used update operators:
1. $set: The `$set` operator is used to update the value of a field. It can be used to modify an existing field or add a new field if it doesn’t already exist. For example, `db.collection.updateOne({ _id: 1 }, { $set: { name: “John” } })` would update the `name` field of the document with `_id` equal to 1.
2. $unset: The `$unset` operator removes a field from a document. This can be helpful when you need to delete a specific field without affecting other fields. For example, `db.collection.updateOne({ _id: 1 }, { $unset: { name: “” } })` would remove the `name` field from the document.
3. $inc: The `$inc` operator increments the value of a field by a specified amount. It is particularly useful for performing numeric operations. For example, `db.collection.updateOne({ _id: 1 }, { $inc: { count: 1 } })` would increment the `count` field of the document by 1.
4. $push: The `$push` operator appends a specified value to an array field. It helps in adding an element to an existing array. For example, `db.collection.updateOne({ _id: 1 }, { $push: { students: “Alice” } })` would append “Alice” to the `students` array.
5. $pull: The `$pull` operator removes all occurrences of a specified value from an array field. It allows you to subtract elements from an array. For example, `db.collection.updateOne({ _id: 1 }, { $pull: { students: “Alice” } })` would remove all occurrences of “Alice” from the `students` array.
6. $addToSet: The `$addToSet` operator adds a value to an array field only if it doesn’t already exist. It ensures uniqueness within the array. For example, `db.collection.updateOne({ _id: 1 }, { $addToSet: { students: “Alice” } })` would add “Alice” to the `students` array if it doesn’t already exist.
7. $pop: The `$pop` operator removes either the first or last element of an array. It can be used to easily implement a stack or queue data structure. For example, `db.collection.updateOne({ _id: 1 }, { $pop: { students: 1 } })` would remove the last element from the `students` array.
These are just a few of the update operators that MongoDB provides. Each operator offers immense flexibility and can be combined to achieve more complex updates as per your requirements.
MongoDB Update Operators FAQ
Q: Can I use multiple update operators in a single update command?
A: Yes, you can. MongoDB allows you to chain multiple update operators together to perform complex updates in a single command.
Q: What happens if I use an update operator on a field that doesn’t exist?
A: If the field specified in the update operator doesn’t exist, MongoDB will create it and perform the update.
Q: Is it possible to update multiple documents at once?
A: Yes, MongoDB provides the `updateMany()` function to update multiple documents that match a specific filter.
Q: Can I update multiple fields within a single document?
A: Absolutely. You can use multiple update operators within a single update command to modify multiple fields within a document.
Q: Do update operators work with nested fields and arrays?
A: Yes, update operators can be applied to nested fields and arrays within a document. You can use the dot notation to access nested fields or array elements.
Q: Can I remove a specific element from an array using an update operator?
A: Yes, the `$pull` operator allows you to remove specific elements from an array by specifying their values.
Q: Is it possible to update all documents in a collection?
A: Yes, the `updateMany()` function without any filter will update all documents in a collection.
In conclusion, MongoDB Update Operators provide a powerful way to manipulate data within a collection. Understanding and effectively utilizing these operators can greatly enhance the efficiency and flexibility of your MongoDB data management. With a wide range of operators at your disposal, you have the tools to tailor your data to meet specific needs, all without replacing entire documents. So, dive into the world of MongoDB update operators and unlock the true potential of your database.
Images related to the topic update document requires atomic operators
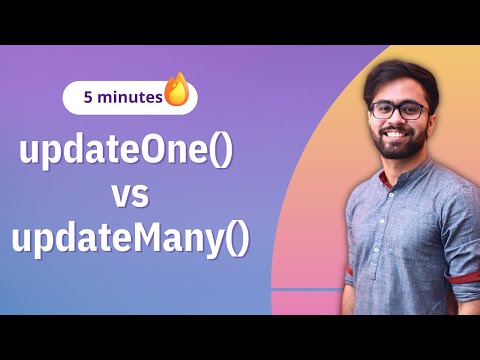
Found 6 images related to update document requires atomic operators theme
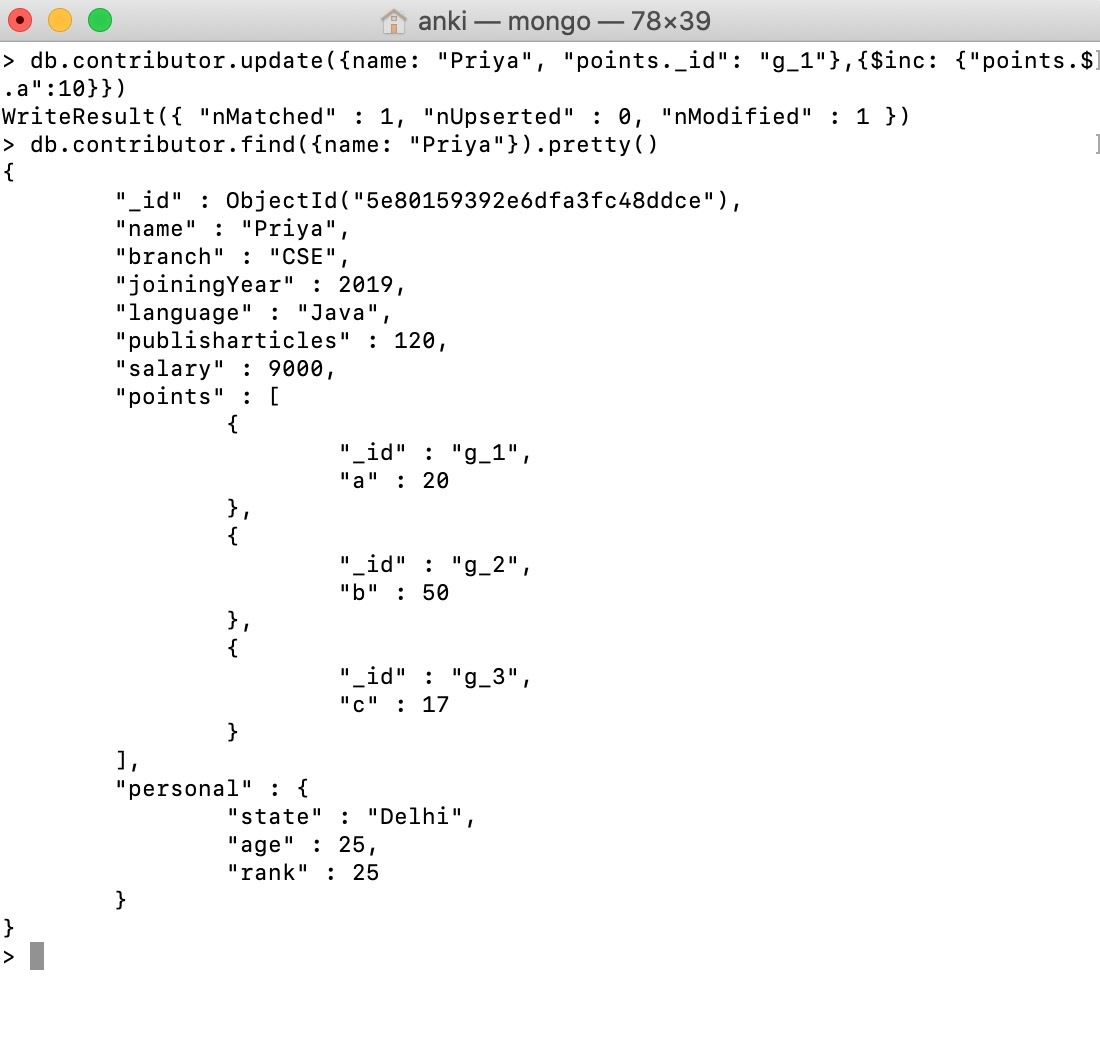

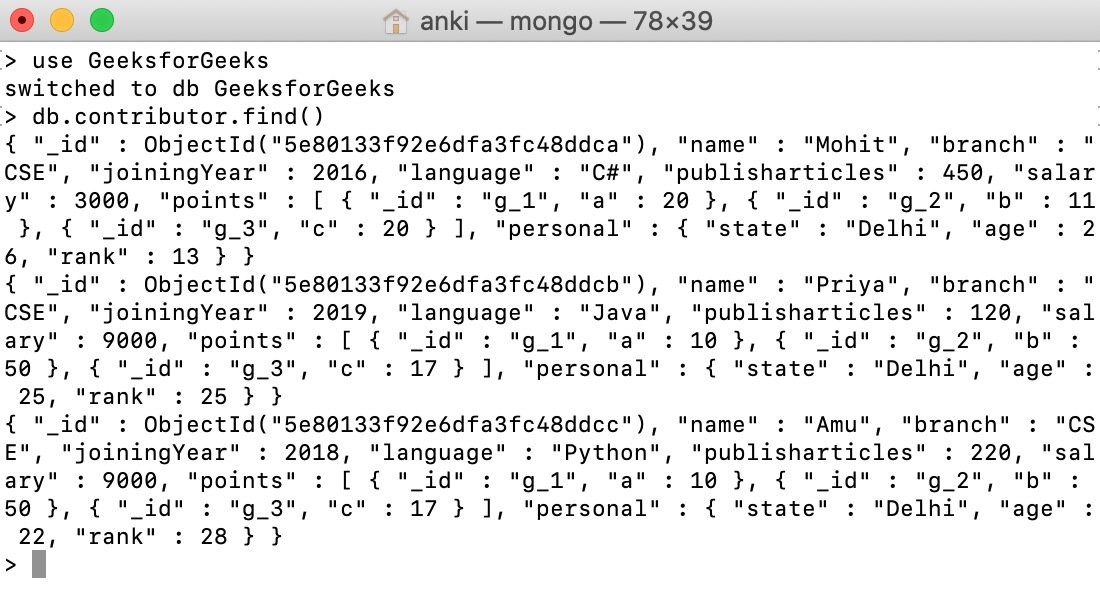


Article link: update document requires atomic operators.
Learn more about the topic update document requires atomic operators.
- Update document requires atomic operators – MongoDB
- Error: the update operation document must contain atomic …
- Update Documents — MongoDB Shell
- Model Data for Atomic Operations — MongoDB Manual
- Update Operators — MongoDB Manual
- Atomicity and Transactions — MongoDB Manual
- Error: the update operation document must … – StackChief
- Error: the update operation document must contain atomic …
- Chapter 6. Updates, atomic operations, and deletes
- When trying to generate bulkWrite update array … – GitHub
- Error: the update operation document must contain atomic …
- How To Perform MongoDB Atomic Operations – KnowledgeHut
- The replacement document must not contain atomic operators
- MongoDB – Atomic Operations – Tutorialspoint
See more: nhanvietluanvan.com/luat-hoc