Unity Wait For Seconds
Coroutine Basics
In game development, managing time intervals and controlling the execution of actions are crucial aspects to consider. Thankfully, Unity offers coroutines, a powerful tool that allows developers to handle time-based operations seamlessly. Coroutines are functions that can be paused and resumed, enabling developers to write code that runs concurrently with other code. One of the most commonly used features in coroutines is the WaitForSeconds function, which adds a delay to the execution of subsequent lines of code. Let’s explore the basics of coroutines and understand why WaitForSeconds is such a valuable asset.
Benefits of using coroutines in game development
Coroutines offer several advantages when it comes to game development. Firstly, they simplify complex time-based sequences and make the code more readable. Rather than using nested if statements and loops, developers can use coroutines to manage and execute sequences of actions with ease. Additionally, the use of coroutines allows for smoother gameplay by implementing time delays between animations, sound effects, and user input. By not blocking the main thread, coroutines also prevent the game from freezing while waiting for a specific action to occur. Overall, coroutines enhance productivity, improve gameplay, and streamline the development process.
Exploring the WaitForSeconds function
One of the key functions in coroutines is WaitForSeconds, which allows developers to introduce delays within a coroutine. The WaitForSeconds function is defined as follows:
“`csharp
IEnumerator ExampleCoroutine()
{
Debug.Log(“Start”);
yield return new WaitForSeconds(1);
Debug.Log(“After 1 second”);
yield return new WaitForSeconds(2);
Debug.Log(“After 2 seconds”);
yield return new WaitForSeconds(0.5f);
Debug.Log(“After 0.5 seconds”);
}
“`
In the above example, the WaitForSeconds function adds a delay of 1 second before executing the next line of code. Subsequently, it adds delays of 2 seconds and 0.5 seconds before executing the subsequent lines. This means that each Debug.Log statement will be executed after the specified time interval has passed. By using WaitForSeconds, developers can create time-based gameplay elements, execute animations with the desired timing, and synchronize user interactions with the game’s logic.
Understanding WaitForSeconds
Definition and purpose of the WaitForSeconds function
WaitForSeconds is a built-in function in Unity that suspends the execution of a coroutine for a specified duration of time. This duration is defined as a parameter when calling WaitForSeconds. The purpose of WaitForSeconds is mainly to introduce pauses or delays within coroutines, allowing developers to control the timing and sequencing of actions in their games. It is commonly used for purposes such as waiting for animations to complete before proceeding, implementing timed events, and managing gameplay mechanics that introduce delays.
How to use WaitForSeconds in coroutine functions
Using WaitForSeconds within a coroutine is relatively straightforward. Developers can call WaitForSeconds followed by a duration value, either as a constant or a variable. This value represents the number of seconds to wait before moving on to the next line of code. Here is an example of using WaitForSeconds in a coroutine:
“`csharp
StartCoroutine(ExampleCoroutine());
IEnumerator ExampleCoroutine()
{
Debug.Log(“Start”);
yield return new WaitForSeconds(1);
Debug.Log(“After 1 second”);
yield return new WaitForSeconds(2);
Debug.Log(“After 2 seconds”);
yield return new WaitForSeconds(0.5f);
Debug.Log(“After 0.5 seconds”);
}
“`
In this example, the coroutine function ExampleCoroutine is started using StartCoroutine. Inside the coroutine, WaitForSeconds is used to introduce delays of 1 second, 2 seconds, and 0.5 seconds. After each WaitForSeconds call, the respective Debug.Log statement is executed. This allows for precise control over the timing of actions within the coroutine.
The role of WaitForSeconds in controlling time intervals
WaitForSeconds plays a critical role in controlling time intervals within coroutines. By specifying the duration as a parameter when using WaitForSeconds, developers can precisely dictate the waiting period between actions. This functionality is crucial in scenarios where timing is critical, such as synchronized animations or timed events. With WaitForSeconds, developers can ensure that specific actions occur precisely after a given time interval, thus enhancing the overall gameplay experience and maintaining player engagement.
Working with WaitForSeconds
Implementing WaitForSeconds to delay actions in Unity
One of the primary use cases for WaitForSeconds is to introduce time delays in Unity. This functionality is especially useful when developers want to delay the execution of certain actions for a specified duration. For example, consider a scenario where upon collecting a power-up, the player’s character gains temporary invincibility. By using WaitForSeconds, developers can introduce a delay before removing the invincibility effect, giving the player a fixed period of time during which they are immune to damage.
“`csharp
IEnumerator PowerUpCoroutine()
{
player.EnableInvincibility();
yield return new WaitForSeconds(10);
player.DisableInvincibility();
}
“`
In this example, the coroutine function PowerUpCoroutine is responsible for enabling and disabling the player’s invincibility. By using WaitForSeconds, the coroutine ensures that the invincibility remains active for 10 seconds before disabling it. This creates a satisfying gameplay mechanic and allows developers to create strategic power-ups that require timing and decision-making.
Examples of using WaitForSeconds for animations, sound effects, and user input
WaitForSeconds is incredibly versatile and can be used in various scenarios within game development. Let’s explore a few examples to demonstrate its capabilities:
1. Animations: To create smooth animations with proper timing, developers can use WaitForSeconds to introduce delays between animation transitions or loops. This ensures that each animation plays out in sync with the desired timing, creating a more visually appealing experience.
“`csharp
IEnumerator AnimationCoroutine()
{
animator.Play(“Jump”);
yield return new WaitForSeconds(1);
animator.Play(“Idle”);
}
“`
In this example, the coroutine function AnimationCoroutine triggers the “Jump” animation, waits for 1 second using WaitForSeconds, and then transitions to the “Idle” animation. By controlling the timing of animations, developers can synchronize the visuals with the gameplay flow, making the game more immersive and realistic.
2. Sound effects: WaitForSeconds can also be used to delay the playback of sound effects, providing synchronized audio experiences. For instance, in a multiplayer game, the sound of a gunshot may need to align precisely with the moment a projectile hits its target. By using WaitForSeconds, developers can introduce a delay before playing the sound effect at the perfect moment.
“`csharp
IEnumerator SoundEffectCoroutine()
{
yield return new WaitForSeconds(2);
audioManager.PlaySoundEffect(“Gunshot”);
}
“`
In this example, the coroutine function SoundEffectCoroutine waits for 2 seconds before playing the “Gunshot” sound effect. By adjusting the delay value, developers can ensure that the audio matches the intended moment in the game, enhancing the overall sensory experience.
3. User input: WaitForSeconds can also be used to introduce delays when waiting for user input. For instance, in a text-based adventure game, developers can use WaitForSeconds to display a message on the screen and give players a certain amount of time to respond before proceeding with the game flow.
“`csharp
IEnumerator UserInputCoroutine()
{
UIManager.DisplayMessage(“Choose your path”);
yield return new WaitForSeconds(5);
// Process user input
}
“`
In this example, the coroutine function UserInputCoroutine waits for 5 seconds after displaying a message on the screen. This gives players sufficient time to read the text and make a decision before the game proceeds to handle their choice. This usage of WaitForSeconds adds pacing and interaction to the game, allowing players to engage with the narrative and make informed decisions.
Handling multiple WaitForSeconds calls within a coroutine
In some cases, developers may need to introduce multiple WaitForSeconds calls within a single coroutine. This can be achieved by using multiple yield return statements. Each WaitForSeconds call will function independently of others and introduce the specified delay. This allows for complex sequences of actions with precise timing. Here’s an example that demonstrates multiple WaitForSeconds calls:
“`csharp
IEnumerator MultipleWaitCoroutine()
{
Debug.Log(“Start”);
yield return new WaitForSeconds(1);
Debug.Log(“After 1 second”);
yield return new WaitForSeconds(2);
Debug.Log(“After 2 seconds”);
yield return new WaitForSeconds(0.5f);
Debug.Log(“After 0.5 seconds”);
yield return new WaitForSeconds(3);
Debug.Log(“After 3 seconds”);
}
“`
In this example, the coroutine function MultipleWaitCoroutine demonstrates the usage of multiple WaitForSeconds calls. Each WaitForSeconds introduces a specific delay, and the subsequent Debug.Log statement is executed after the respective delay. By chaining these time delays, developers can create complex sequences of actions that occur in a desired order and with precise timing.
Customizing WaitForSeconds
Modifying the duration of WaitForSeconds dynamically
While WaitForSeconds is generally used with a constant duration, it is also possible to modify the delay dynamically during runtime. This can be achieved by assigning a variable to the WaitForSeconds call. Here’s an example that demonstrates how to modify the duration of WaitForSeconds:
“`csharp
IEnumerator DynamicWaitCoroutine()
{
Debug.Log(“Start”);
float delay = 2.5f;
yield return new WaitForSeconds(delay);
Debug.Log(“After ” + delay + ” seconds”);
}
“`
In this example, the coroutine function DynamicWaitCoroutine assigns a variable named delay to the WaitForSeconds call. By modifying the value of delay, developers can introduce different durations for the time delay. This allows for dynamic control over timing when the delay duration needs to change based on certain conditions or user input.
Creating a random time delay using WaitForSeconds
WaitForSeconds can also be combined with randomization to introduce variability in time delays. By using UnityEngine.Random.Range, developers can generate a random time delay within a specified range. This can be particularly useful for creating unpredictable gameplay elements or adding a sense of randomness to certain events. Here’s an example of creating a random time delay using WaitForSeconds:
“`csharp
IEnumerator RandomDelayCoroutine()
{
Debug.Log(“Start”);
float minDelay = 1;
float maxDelay = 5;
float randomDelay = UnityEngine.Random.Range(minDelay, maxDelay);
yield return new WaitForSeconds(randomDelay);
Debug.Log(“After a random delay of ” + randomDelay + ” seconds”);
}
“`
In this example, the coroutine function RandomDelayCoroutine generates a random time delay between 1 and 5 seconds. By using UnityEngine.Random.Range, developers can create diverse gameplay experiences where certain actions occur at unpredictable intervals.
Overcoming limitations with WaitForSeconds through coding techniques
Although WaitForSeconds is a powerful tool for managing time-based operations in Unity, it has certain limitations. For instance, WaitForSeconds is not suitable for pausing a coroutine indefinitely or until a specific condition is met. In such cases, developers can overcome this limitation by using while loops, flags, or custom wait conditions. By combining WaitForSeconds with appropriate coding techniques, developers can achieve complex time control scenarios. Here’s an example that demonstrates a custom wait condition:
“`csharp
IEnumerator CustomWaitCoroutine()
{
Debug.Log(“Start”);
bool conditionMet = false;
while (!conditionMet)
{
// Check condition
if (playerHealth <= 0)
{
conditionMet = true;
}
yield return null;
}
Debug.Log("Condition met, continue with the coroutine");
}
```
In this example, the coroutine function CustomWaitCoroutine uses a while loop to continuously check a condition. Once the condition is met (e.g., playerHealth reaches 0), the loop breaks, and the coroutine proceeds with the subsequent lines of code. By leveraging programming techniques, developers can adapt WaitForSeconds to suit more complex requirements and optimize its functionality within their game development projects.
Optimizing WaitForSeconds
Best practices for efficient use of WaitForSeconds
While WaitForSeconds is an effective tool, improper use can negatively impact performance. To ensure optimal usage, developers should follow these best practices:
1. Minimize the number of unnecessary WaitForSeconds calls: Each WaitForSeconds call imposes a performance cost, so it is essential to only introduce delays when necessary. Analyze the code and ensure that each WaitForSeconds call serves a specific purpose within the gameplay or visual experience.
2. Use smaller delay values when possible: While WaitForSeconds can handle delay durations of any length, smaller values can ensure more responsive gameplay. Instead of using lengthy delays, consider using smaller increments that still achieve the desired effect. This can prevent the game from feeling sluggish or unresponsive.
3. Group multiple WaitForSeconds calls into one: If there are multiple time delays to introduce within a coroutine, consider combining them into a single WaitForSeconds call. By summing up the individual durations, developers can minimize the overhead of multiple WaitForSeconds calls and improve performance.
Alternatives to WaitForSeconds for specific scenarios
While WaitForSeconds is a versatile tool, there are situations where alternative approaches may be more suitable:
1. FixedUpdate for physics-based interactions: In scenarios that involve physics-based interactions, FixedUpdate can be a better choice than WaitForSeconds. FixedUpdate is called at a fixed time interval (typically tied to the physics engine's timestep) and is ideal for calculations involving rigid bodies, collisions, and other physics-related gameplay mechanics.
2. EventHandler and event-driven programming for complex interactions: If the time delays depend on complex interactions or require coordination between multiple elements, an event-driven programming approach may be more appropriate. By incorporating event handlers and triggers, developers can create intricate timing mechanisms without relying solely on WaitForSeconds.
3. Animation events for precise animation control: When precise control over animations is required, animation events can be used. Animation events allow developers to invoke specific functions at key points within an animation timeline, eliminating the need for explicit time delays. This approach can provide more granular control over animations and ensure timing accuracy.
Performance considerations when using WaitForSeconds in large-scale projects
When working on large-scale projects, it is crucial to consider performance implications associated with WaitForSeconds. Here are some performance considerations to keep in mind:
1. Minimize the use of coroutines and WaitForSeconds in performance-critical areas: While coroutines and WaitForSeconds are powerful features, excessive usage in performance-critical areas can impact frame rate or introduce unnecessary overhead. Evaluate the impact of each WaitForSeconds call and make judicious use of coroutines to ensure optimal performance in resource-intensive sections of the game.
2. Profile and optimize coroutine execution time: Profiling is essential to identify potential bottlenecks and areas where WaitForSeconds may consume significant processing time. Optimize the coroutine execution time by reducing the number of calculations or offloading heavy computations to other parts of the game logic.
3. Group coroutines to minimize context switching: Context switching between coroutines can add overhead, especially when there are numerous WaitForSeconds calls. Whenever possible, group related coroutines or use a single coroutine with appropriate yield return statements to achieve the desired time intervals. This can help minimize context switching and improve performance.
Advanced WaitForSeconds Techniques
Chaining coroutines with WaitForSeconds
Chaining coroutines is a powerful technique that involves calling one coroutine from another coroutine. This technique becomes even more effective when combined with WaitForSeconds to create a sequence of time-based actions. By chaining coroutines with WaitForSeconds, developers can create complex and dynamic gameplay sequences. Here's an example that demonstrates chaining coroutines with WaitForSeconds:
```csharp
IEnumerator MainCoroutine()
{
Debug.Log("Start");
yield return StartCoroutine(SubCoroutine());
Debug.Log("Continue after SubCoroutine");
}
IEnumerator SubCoroutine()
{
Debug.Log("SubCoroutine start");
yield return new WaitForSeconds(2);
Debug.Log("After 2 seconds in SubCoroutine");
}
```
In this example, MainCoroutine calls SubCoroutine using StartCoroutine. Within SubCoroutine, WaitForSeconds is used to introduce a delay of 2 seconds. Once the delay is complete, the execution returns to MainCoroutine, allowing subsequent actions to be executed. By chaining coroutines with WaitForSeconds, developers can create complex and dynamic sequences of actions, adding sophistication and variety to their gameplay mechanics.
Combining WaitForSeconds with other Unity functions and events
WaitForSeconds can also be combined with other Unity functions and events to enhance the functionality and control of coroutines. By using exquisite combinations, developers can achieve even more advanced time control in their games. Here are a few examples:
1. Combining with Invoke: WaitForSeconds can be combined with Invoke to create a delayed function call. By using Invoke alongside WaitForSeconds, developers can schedule a function to be executed after a determined time delay.
```csharp
IEnumerator CombinedCoroutine()
{
Debug.Log("Start");
yield return new WaitForSeconds(2);
Invoke("DelayedFunctionCall", 2);
}
void DelayedFunctionCall()
{
Debug.Log("Delayed function call");
}
```
In this example, WaitForSeconds is leveraged to introduce a delay of 2 seconds. After the delay, Invoke is used to schedule the execution of the function DelayedFunctionCall, resulting in a function call occurring after the specified delay. This provides a comprehensive mechanism to control timing and execute actions at predetermined intervals.
2. Combining with Animation Events: Animation events can trigger specific functions at key moments within an animation timeline. By combining Animation Events with WaitForSeconds, developers can synchronize game logic with animations precisely.
```csharp
IEnumerator AnimationEventCoroutine()
{
animator.Play("AttackAnimation");
yield return new WaitForSeconds(1);
// Execute gameplay logic
Debug.Log("Attack animation finished");
}
```
In this example, WaitForSeconds is used to introduce a delay of 1 second after triggering the "AttackAnimation" animation. This provides time for the animation to play out before the coroutine continues with executing the gameplay logic. By deftly combining WaitForSeconds with Animation Events, developers can ensure seamless transitions between animations and gameplay events.
Advanced tricks and tips to enhance your use of WaitForSeconds
To further enhance the use of WaitForSeconds, here are additional tricks and tips to keep in mind:
1. Yield return null for frame-independent delays: While WaitForSeconds relies on Unity's frame rate, sometimes it is essential to introduce frame-independent delays. In such cases, developers can use yield return null within a while loop to achieve consistent delays regardless of the frame rate.
2. Use RunTimeAnimatorController to synchronize animations: To ensure precise timing between animations and gameplay elements, utilize RunTimeAnimatorController. This powerful tool allows for complex animation synchronization, synchronization with script logic, and achieving varied gameplay experiences.
3. Implement WaitForSeconds within a state machine: By incorporating a state machine architecture, developers can integrate WaitForSeconds
Unity Waitforseconds
How To Wait In Unity Without Coroutine?
In game development, timing is crucial for creating engaging and interactive experiences. Unity, one of the most popular game engines, provides several methods to implement delays or wait times in your game. While Unity’s built-in coroutines are often the go-to solution for implementing delays, there are alternative methods available that allow you to achieve similar results without using coroutines. In this article, we will explore these methods in depth and discuss their advantages and limitations.
1. Frame-Based Waiting
One way to implement delays in Unity without coroutines is by using frame-based waiting. This approach involves using a counter that increments every frame until a desired wait time is reached. Here’s an example script that demonstrates how to wait for a specific number of frames:
“`
public class FrameWaitExample : MonoBehaviour
{
private int frameCount = 0;
public int waitFrames = 60; // Waiting for 60 frames (~1 second)
private void Update()
{
if (frameCount < waitFrames)
{
frameCount++;
return;
}
// Execute the desired code after the wait time
Debug.Log("Waited for " + waitFrames + " frames!");
}
}
```
This method is simple to implement and does not rely on coroutines. However, it is not suitable for situations that require highly accurate timing, as the frame rate can vary between different devices.
2. Time-Based Waiting using Delta Time
A commonly used alternative to coroutines is time-based waiting using delta time. Instead of waiting for a fixed number of frames, we calculate the desired wait time based on real-world time using the Time.deltaTime property. Here's an example script:
```
public class TimeWaitExample : MonoBehaviour
{
public float waitTime = 1.0f; // Waiting for 1 second
private float timer = 0.0f;
private bool isWaiting = false;
private void Update()
{
if (isWaiting)
{
timer += Time.deltaTime;
if (timer >= waitTime)
{
// Execute the desired code after the wait time
Debug.Log(“Waited for ” + waitTime + ” seconds!”);
// Reset variables for subsequent waits
isWaiting = false;
timer = 0.0f;
}
}
else
{
// Start waiting
isWaiting = true;
}
}
}
“`
Using this method, you can easily introduce wait times based on real-world seconds. Additionally, this approach provides greater accuracy compared to frame-based waiting.
FAQs
Q1. Can I use multiple time-based waits simultaneously in my game?
Yes, you can use multiple time-based waits in your game. Simply create separate timer variables for each wait instance and execute the desired code for each wait as they complete.
Q2. How can I pause the game while waiting?
By using the Time.timeScale property, you can pause the game while waiting. Set Time.timeScale to zero before the wait and restore it to one after the wait is complete.
Q3. Are there any limitations or disadvantages to not using coroutines?
While using coroutines offers additional flexibility and ease of use, there are no inherent disadvantages to not using coroutines for simple wait scenarios. However, for more complex scenarios that involve simultaneous waits or nested waits, coroutines can provide a cleaner and more manageable solution.
Q4. Which method should I use for wait times in my game?
The choice of method depends on your specific requirements. If precise timing is essential, time-based waiting using delta time is recommended. If accuracy is not critical and you simply need to introduce wait times, frame-based waiting can be a viable alternative.
Q5. Can I combine coroutines with other delay methods?
Yes, you can combine coroutines with other delay methods based on your needs. Unity provides the flexibility to use multiple methods together to achieve desired effects.
In conclusion, while coroutines are a popular choice for implementing wait times in Unity, there are alternative methods available that can achieve similar results. Frame-based waiting and time-based waiting using delta time both offer ways to introduce delays without relying on coroutines. Consider the requirements of your specific scenario and choose the method that best suits your needs.
What Is Yield Unity?
Yield Unity, also known as yield farming or liquidity mining, has gained significant attention in the world of decentralized finance (DeFi) over the past year. It is a process that allows individuals to earn passive income by lending or staking their cryptocurrency assets on various DeFi platforms.
Yield Unity harnesses the concept of decentralized lending and borrowing protocols, providing individuals an opportunity to earn returns on their cryptocurrency holdings. This process works by leveraging smart contracts on the blockchain, eliminating the need for intermediaries such as banks or financial institutions.
The process typically involves depositing your cryptocurrency into a liquidity pool, which is a decentralized fund maintained by a smart contract. These pools are often created on decentralized exchanges (DEXs) or lending platforms. By adding funds to these pools, users contribute to the overall liquidity available on the platform.
In return for their contribution, participants are rewarded with interest or fees garnered from the users accessing the liquidity pool. The rewards are often distributed in the respective platform’s native tokens or tokens that represent the underlying assets.
One of the most popular platforms for yield farming is Compound Finance. Compound allows users to lend their cryptocurrency assets to borrowers and earn interest in return. The interest rates are determined by the platform’s algorithm, which factors in demand and supply dynamics.
Another prominent platform is Uniswap, which enables users to provide liquidity for token swaps in exchange for earning a portion of the fees generated by the platform. Uniswap utilizes an automated market maker (AMM) model, allowing for seamless token swaps without traditional order books.
One of the benefits of Yield Unity is the ability to earn a higher return compared to traditional financial instruments. Interest rates in DeFi protocols are often significantly higher than those offered by banks, as they are not subject to the same regulations and overhead costs. Additionally, yield farming allows participants to access the potential appreciation of the platforms’ native tokens, which can increase value over time.
However, yield farming is not without its risks. The decentralized nature of DeFi platforms comes with its own set of challenges. Smart contract vulnerabilities, hacking attempts, and market volatility are some of the risks users must consider before participating in yield farming. It is crucial to conduct thorough research and due diligence before committing funds.
Furthermore, the competition for yield farming incentives can be intense. As more participants join the market, the rewards may decrease over time. Participants must monitor the market dynamics and adjust their strategies accordingly to optimize their returns.
FAQs
Q: How much money can I make from yield farming?
A: The amount of money you can earn from yield farming depends on various factors, including the amount of funds you invest, the chosen platform, and market conditions. It is important to note that yield farming returns are subject to market volatility and can fluctuate significantly.
Q: Is yield farming risky?
A: Yes, yield farming carries certain risks. Smart contract vulnerabilities, hacking attempts, and market volatility pose potential risks that users must consider. It is advisable to only invest funds that you are willing to lose and to conduct thorough research before participating in yield farming.
Q: Can I lose money in yield farming?
A: Yes, it is possible to lose money in yield farming. Market fluctuations, smart contract vulnerabilities, and other risks can result in substantial losses. It is important to carefully assess the risks and only invest funds that you can afford to lose.
Q: How can I choose a reliable yield farming platform?
A: When selecting a yield farming platform, it is crucial to consider factors such as the platform’s security measures, reputation, user reviews, and audits conducted on the smart contracts. Conducting thorough research can minimize the risk of engaging with fraudulent or insecure platforms.
Q: Can I participate in yield farming with any cryptocurrency?
A: The availability of cryptocurrencies may vary depending on the platform you select. While popular cryptocurrencies like Ethereum are widely accepted, some platforms may have specific requirements or preferences. It is advisable to check the platform’s guidelines before participating.
In conclusion, Yield Unity or yield farming has rapidly emerged as an attractive way to earn passive income within the decentralized finance ecosystem. By lending or staking cryptocurrency assets on DeFi platforms, individuals can earn returns on their holdings through interest or fees. While it offers the potential for higher returns compared to traditional finance, participants must carefully consider the associated risks and conduct thorough research before engaging in yield farming activities.
Keywords searched by users: unity wait for seconds Unity wait for milliseconds, Unity wait for coroutine to finish, Unity delay without coroutine, C# delay 1 second, Unity coroutine wait for condition, Yield return new WaitForSeconds 0.5 f, Timer schedule unity, Invoke Unity
Categories: Top 25 Unity Wait For Seconds
See more here: nhanvietluanvan.com
Unity Wait For Milliseconds
Wait for milliseconds is a method that pauses the execution of a script for a specified duration. It is particularly useful in scenarios where you want to delay an action, create timed events, or synchronize actions with other elements in the game. Let’s dive deeper into how this functionality works and how you can use it effectively in your Unity projects.
In Unity, the WaitForSeconds() function is commonly used to introduce a delay in script execution. This function takes a single input parameter: the number of seconds the script should pause for. However, when dealing with milliseconds, you need to convert the desired duration to seconds. Unity’s Time class provides a useful property called deltaTime, which represents the time in seconds it took to complete the last frame. By multiplying deltaTime by the desired milliseconds, you can achieve the desired delay.
For example, if you want to pause the script for 500 milliseconds (half a second), you can write:
yield return new WaitForSeconds(0.5f);
In this code snippet, WaitForSeconds(0.5f) converts 0.5 seconds to 500 milliseconds. By using the “yield return” statement, the script pauses execution for the specified duration before continuing to the next line.
It’s important to note that the WaitForSeconds() function is part of Unity’s Coroutine system. Coroutines are special functions in Unity that allow you to pause and resume their execution, making them suitable for time-based delays. To use the WaitForSeconds() function, you need to call it from within a coroutine. Coroutines are typically triggered using the StartCoroutine() method, and you can stop them using StopCoroutine() or StopAllCoroutines().
Let’s say you want to create a game where objects spawn at regular intervals. By utilizing the wait for milliseconds functionality, you can easily achieve this. Here’s an example of a simple script that spawns objects every 2 seconds:
“`csharp
IEnumerator SpawnObjects()
{
while (true)
{
// Spawn object code
yield return new WaitForSeconds(2);
}
}
“`
In this script, the SpawnObjects() function is a coroutine. Inside the while loop, you can place the code responsible for spawning objects. After waiting for 2 seconds, the coroutine will continue from where it left off, executing the spawn object code again.
Now that we have covered the basics of using wait for milliseconds in Unity, let’s address some frequently asked questions:
FAQs:
Q: Can I use wait for milliseconds outside of coroutines?
A: No, the wait for milliseconds functionality is specifically designed to be used within coroutines. It requires the yield return statement to pause and resume execution properly.
Q: Can I use wait for milliseconds to delay animations?
A: Yes, you can utilize wait for milliseconds in combination with Unity’s animation system. By using coroutines, you can pause animations for specific durations, providing a more controlled timing for your game’s animations.
Q: Are there any alternatives to wait for milliseconds?
A: While wait for milliseconds is a convenient way to introduce delays, there are alternatives you can use depending on your specific requirements. For more complex timing scenarios, you can utilize Unity’s Timeline system, or write custom timers using the Update() function.
Q: How accurate is the wait for milliseconds functionality?
A: The accuracy of the wait for milliseconds functionality depends on various factors, including the current frame rate and the workload on the system. For most game development scenarios, the accuracy is sufficient. However, if you require precise timing, you might need to explore other approaches or consider external libraries.
In conclusion, the wait for milliseconds functionality in Unity provides an efficient way to introduce delays in script execution. By leveraging coroutines and WaitForSeconds(), developers can easily create timed events, synchronize actions, and implement various time-based functionalities in their games. Understanding how to use this feature effectively can greatly enhance the overall player experience and add depth to your Unity projects.
Unity Wait For Coroutine To Finish
## Understanding Coroutines in Unity
In Unity, coroutines are a fundamental concept that helps manage the execution of time-consuming tasks without blocking the main thread. When a coroutine is started, it runs independently of other tasks and can be paused using the `yield return` statement. This allows the engine to perform other necessary tasks while the coroutine is on hold, resulting in smoother performance and responsiveness.
## The Need to Wait for Coroutines
There are instances when you may need to wait for a coroutine to finish before proceeding. For example, you might have a gameplay sequence where certain actions must be completed in a specific order. In such cases, it is crucial to ensure that the gameplay flow follows the desired sequence by waiting for coroutines to finish.
## Waiting for Coroutines: Different Approaches
### 1. Wait Until Coroutine is Finished
The simplest approach to waiting for a coroutine to finish is to use the `yield return StartCoroutine()` statement. By using this statement, you can start a coroutine and have the main function wait until it is finished before moving on. Here is an example:
“`csharp
IEnumerator MyCoroutine()
{
// Coroutine code goes here
yield return null;
}
void Start()
{
StartCoroutine(MyCoroutine());
StartCoroutine(WaitForCoroutine());
}
IEnumerator WaitForCoroutine()
{
yield return StartCoroutine(MyCoroutine());
Debug.Log(“Coroutine finished!”);
}
“`
In the above code, the `WaitForCoroutine` waits for the `MyCoroutine` to finish before printing “Coroutine finished!” to the Unity console.
### 2. Coroutine Execution as Part of a Function
Another approach is to encapsulate the coroutine within a function and make it return an enumerator. By executing the coroutine as part of a function, you can yield the function call itself, effectively waiting until the coroutine completes. Here is an example:
“`csharp
IEnumerator MyCoroutine()
{
// Coroutine code goes here
yield return null;
}
IEnumerator WaitForCoroutine()
{
yield return MyCoroutine();
Debug.Log(“Coroutine finished!”);
}
“`
In this case, the `WaitForCoroutine` function waits for `MyCoroutine` to complete before printing the message.
### 3. Custom Yield Instructions
Unity allows developers to create custom yield instructions using the `CustomYieldInstruction` class. This enables you to define your own conditions for waiting. For example, you can create a custom yield instruction that waits until a specific condition is met within the coroutine. Here is an example:
“`csharp
public class WaitForCustomCondition : CustomYieldInstruction
{
private bool conditionMet = false;
public override bool keepWaiting => !conditionMet;
public void UpdateCondition()
{
// update the condition based on your requirements
conditionMet = true;
}
}
IEnumerator MyCoroutine()
{
// Coroutine code goes here
yield return new WaitForCustomCondition();
}
IEnumerator WaitForCoroutine()
{
var customCondition = new WaitForCustomCondition();
yield return customCondition;
Debug.Log(“Coroutine finished!”);
}
“`
In this code, the `MyCoroutine` waits for the `WaitForCustomCondition` to be met before proceeding, and the `WaitForCoroutine` sets the condition to `true` after a specific task is completed.
## FAQs
**Q: Can I use `yield return null;` to wait for a coroutine to finish?**
A: No, `yield return null;` is used within the coroutine itself to wait for the next frame. It does not wait for the coroutine to finalize its execution.
**Q: What is the difference between `yield return StartCoroutine()` and yielding a function call that contains `yield return`?**
A: When using `yield return StartCoroutine()`, you are directly yielding the coroutine itself, whereas yielding a function call that contains `yield return` encapsulates the coroutine within a function.
**Q: Can I pause a coroutine and resume it later?**
A: Yes, you can pause a coroutine by using a specific yield instruction, such as `yield return new WaitForSeconds(2f);`. This will pause the coroutine for 2 seconds before continuing from where it left off.
**Q: Are there alternative ways to achieve waiting for coroutines?**
A: While the methods discussed in this article are commonly used, there are other techniques, such as using events or checking boolean flags, to achieve similar results. The choice of approach depends on the specific requirements of your project.
In conclusion, waiting for a coroutine to finish is a common requirement in Unity game development. By utilizing the various approaches discussed in this article, you can ensure that your game flow follows the desired sequence. Understanding and implementing these methods will help you harness the power and flexibility of coroutines within Unity effectively.
Unity Delay Without Coroutine
Introduction:
In Unity, coroutines are often used to create delays in script execution. However, coroutines can become complex and resource-intensive when dealing with multiple delays simultaneously. Therefore, it’s important to explore alternative approaches that provide similar functionality without relying on coroutines. This article dives into various techniques and explains how to effectively implement delays in Unity while minimizing performance impact.
Section 1: Understanding Coroutines and Delays
To comprehend alternative approaches, we must first understand coroutines and how they are commonly used to introduce delays in Unity. A coroutine is essentially a method that can pause execution at specific points and later resume from where it left off. By utilizing the yield statement, developers can introduce delays between code execution steps, allowing for frame-independent timing.
Section 2: Issues with Coroutines
While coroutines offer great flexibility, they can present challenges when used for multiple concurrent delays. Each coroutine involves creating a separate instance, consuming memory, and adding significant overhead. As the number of simultaneous delays increases, these overheads become more pronounced and can impact performance. Therefore, it is essential to consider alternative approaches that address these issues.
Section 3: Utilizing the Update Loop
One alternative to coroutines involves a more direct utilization of Unity’s Update loop. By employing variables to store time values and checking against these values inside Update, we can effectively introduce delays without relying on coroutines. For instance, setting a delay of 3 seconds can be achieved by storing a starting time, continuously checking the difference between the current time and the starting time, and executing desired code when that difference exceeds 3 seconds.
Section 4: Implementing Delays with Time.deltaTime
Another approach involves employing Time.deltaTime, which represents the time between frames. By accumulating delta time values and checking against a target delay in the Update loop, we can create accurate delays. However, it’s important to note that Time.deltaTime may vary depending on the system’s performance, which can introduce discrepancies in timing precision.
Section 5: Utilizing Unity Timers
Unity provides a built-in timer class, which offers an alternative method for introducing delays. These timers allow developers to specify a delay duration and execute specific code once that duration has passed. Using timers can simplify the code structure and eliminate the need for complex coroutine management, enhancing performance while maintaining flexibility and precision.
Section 6: FAQs
Q1. Can delays be achieved using the Invoke method?
Yes, the Invoke method can be used to introduce delays. It provides a straightforward way to execute a method after a specified delay. However, be aware that using Invoke extensively can have performance implications due to the repeated method invocations.
Q2. Are there any performance differences between the alternative approaches mentioned?
Yes, there can be performance differences between approaches, depending on the complexity of the implementation and the number of delays involved. The direct utilization of the Update loop and Time.deltaTime may be more resource-intensive compared to Unity timers, as they require explicit code execution and continuous checks.
Q3. How can I ensure consistent timing across different systems when using alternative approaches?
Achieving precise timing across various systems can be challenging due to differences in hardware performance. It’s crucial to thoroughly test the chosen approach on different devices to ensure consistent timing. Additionally, considering the variability of Time.deltaTime can help mitigate some timing discrepancies.
Q4. Can I mix and match these alternative approaches to suit my specific needs?
Absolutely! Unity provides developers with great flexibility, so mixing and matching these approaches based on specific requirements is entirely possible. However, it’s essential to carefully plan the implementation and consider the potential impact on both performance and code readability.
Conclusion:
Delays are an integral part of game development, allowing for the creation of timed events, animations, or player input handling. While coroutines have traditionally been used for delays in Unity, they can become resource-intensive when multiple delays are required simultaneously. This article explored alternative approaches, including utilizing the Update loop, Time.deltaTime, and Unity timers. Each method offers its advantages and disadvantages, empowering developers with multiple options to achieve delays while minimizing performance impact.
Images related to the topic unity wait for seconds
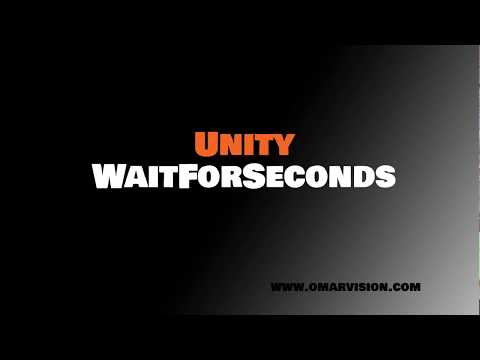
Found 6 images related to unity wait for seconds theme

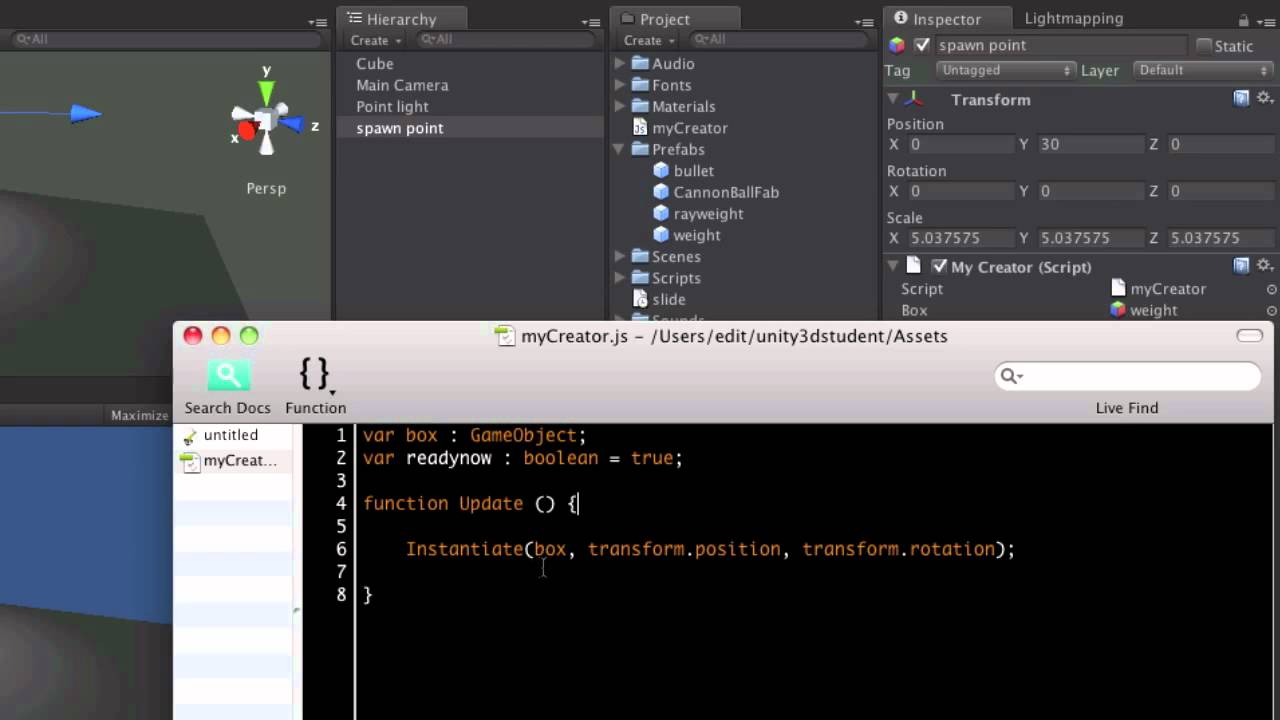


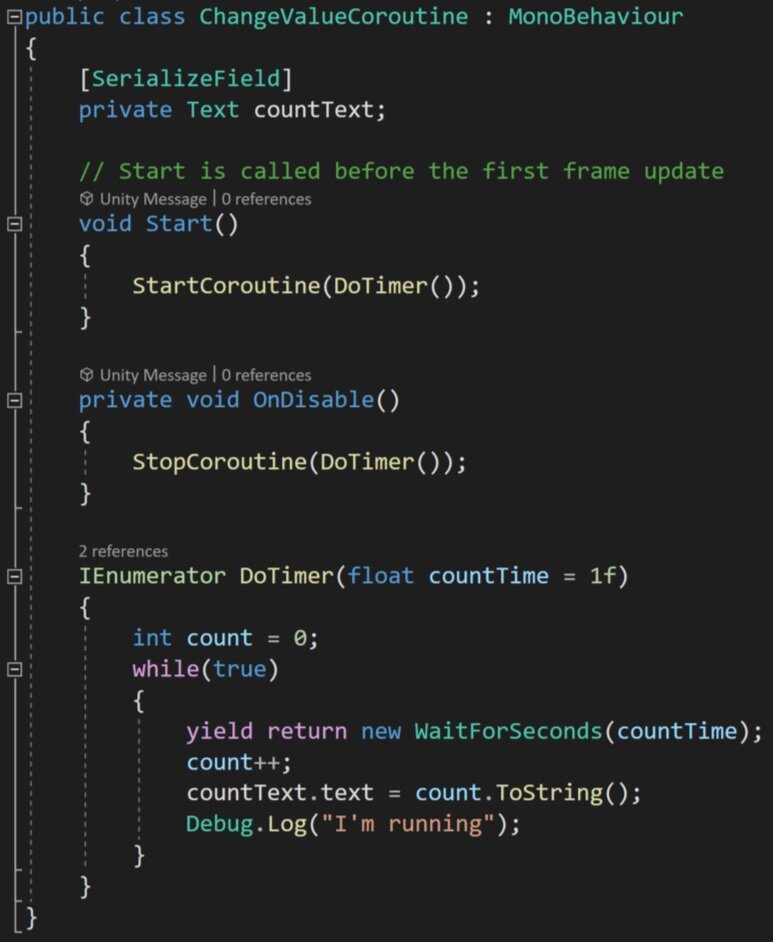
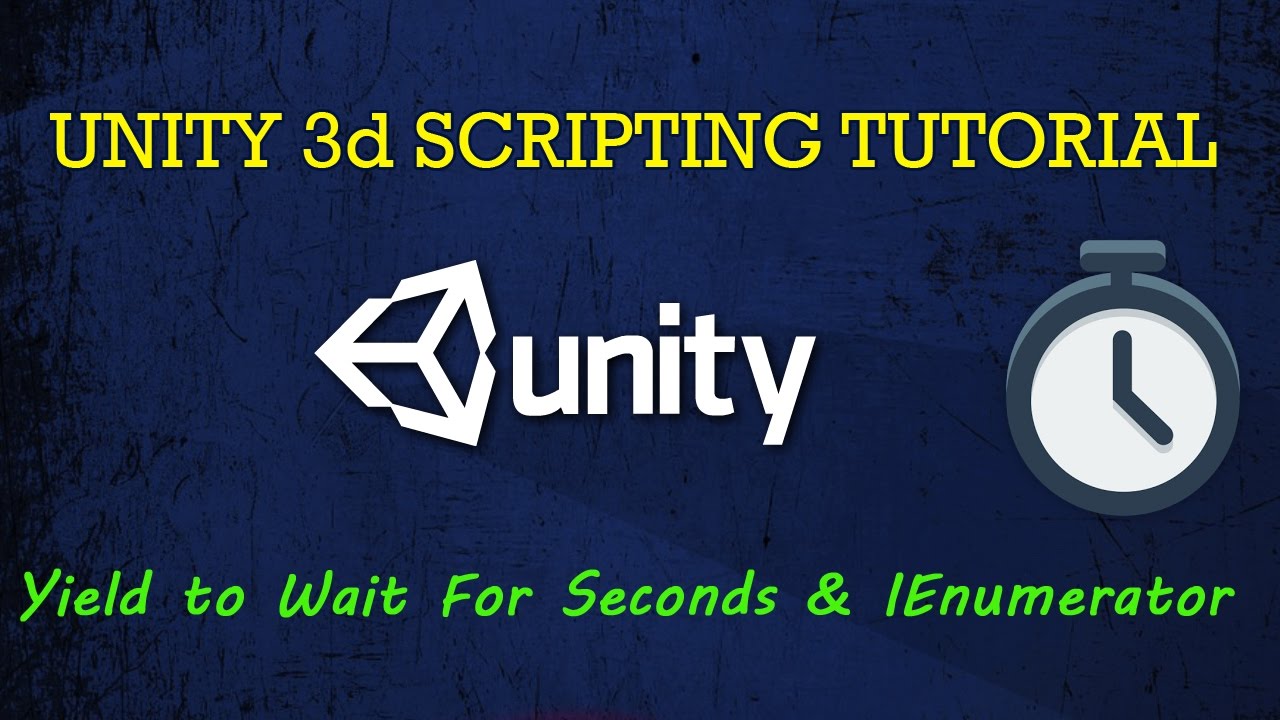
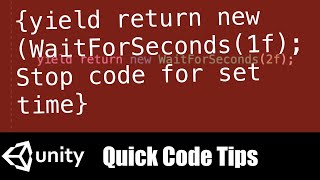
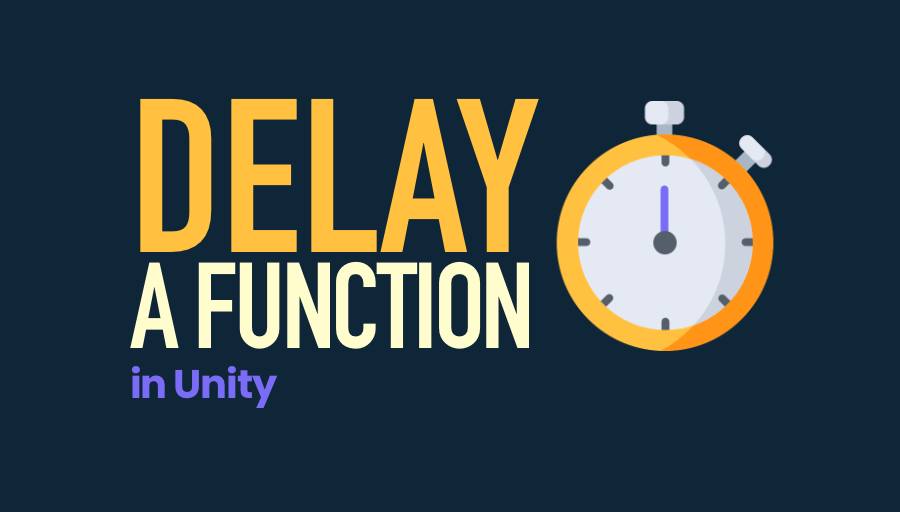
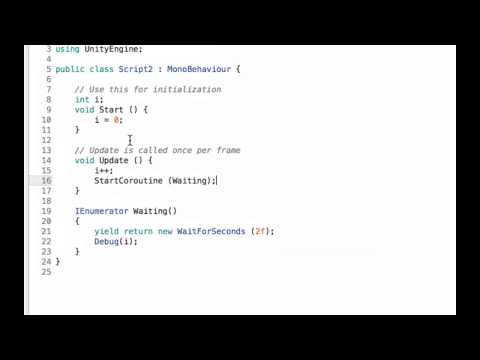
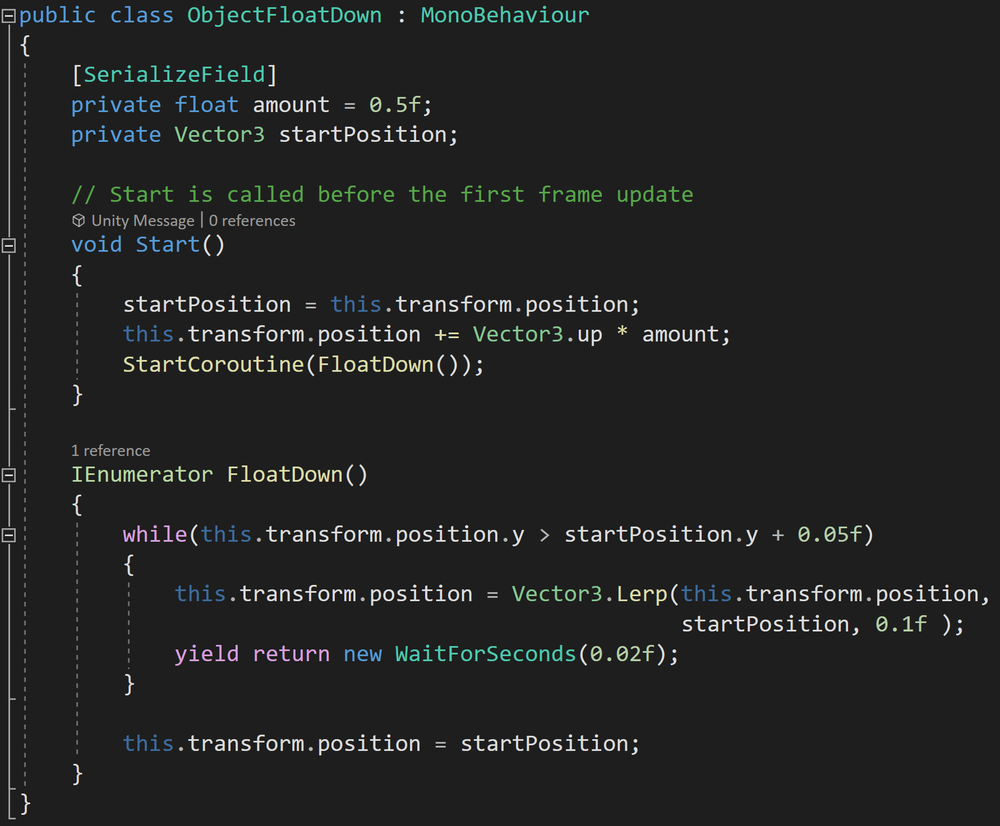
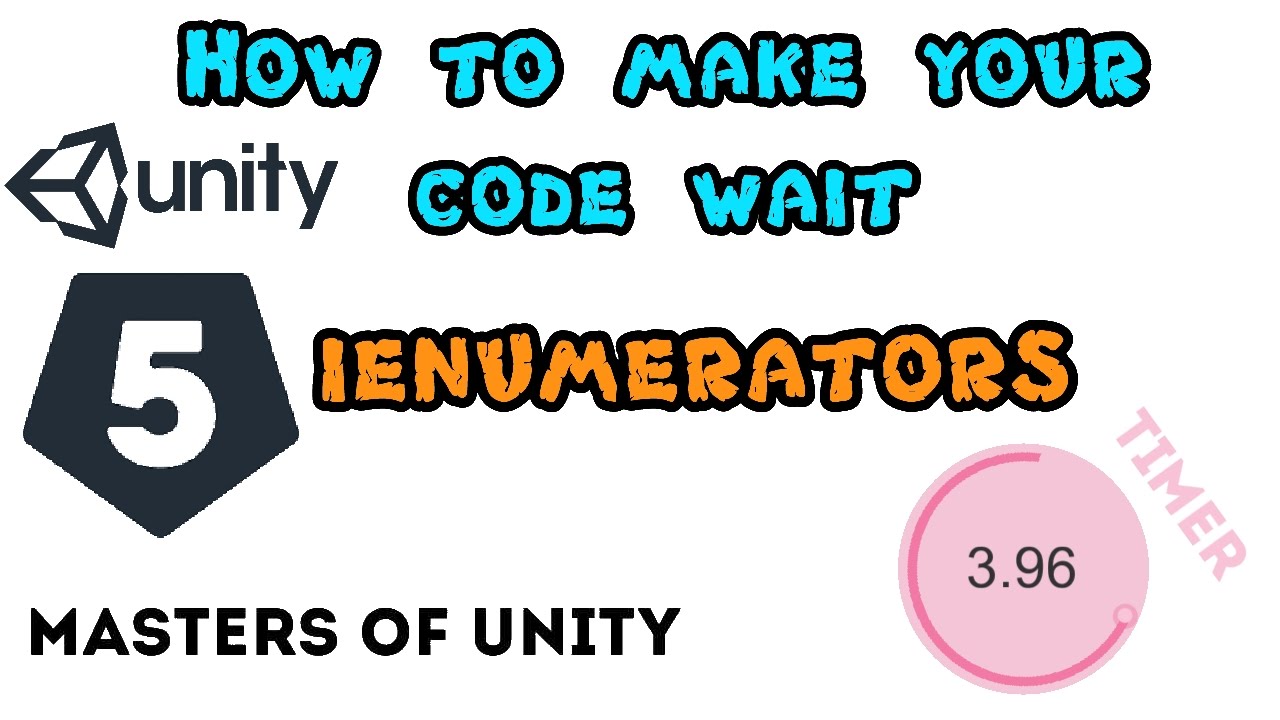
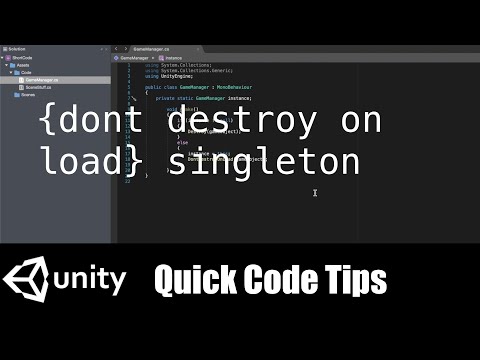
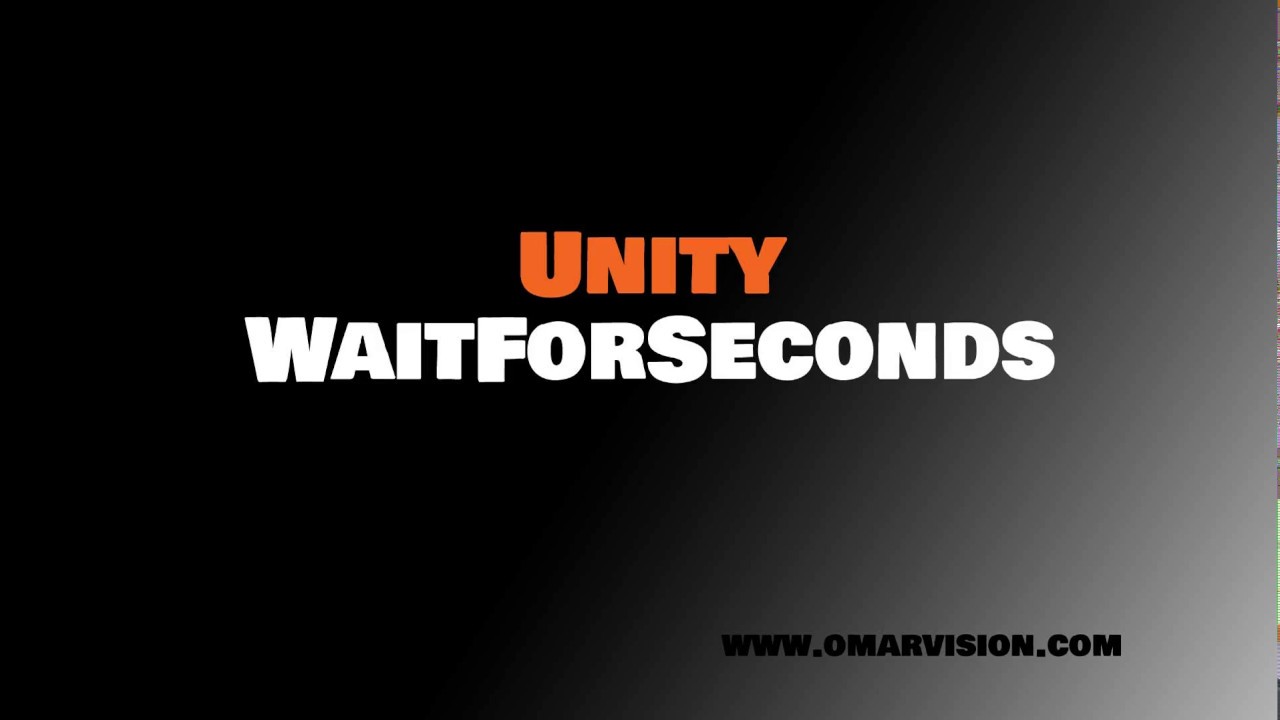

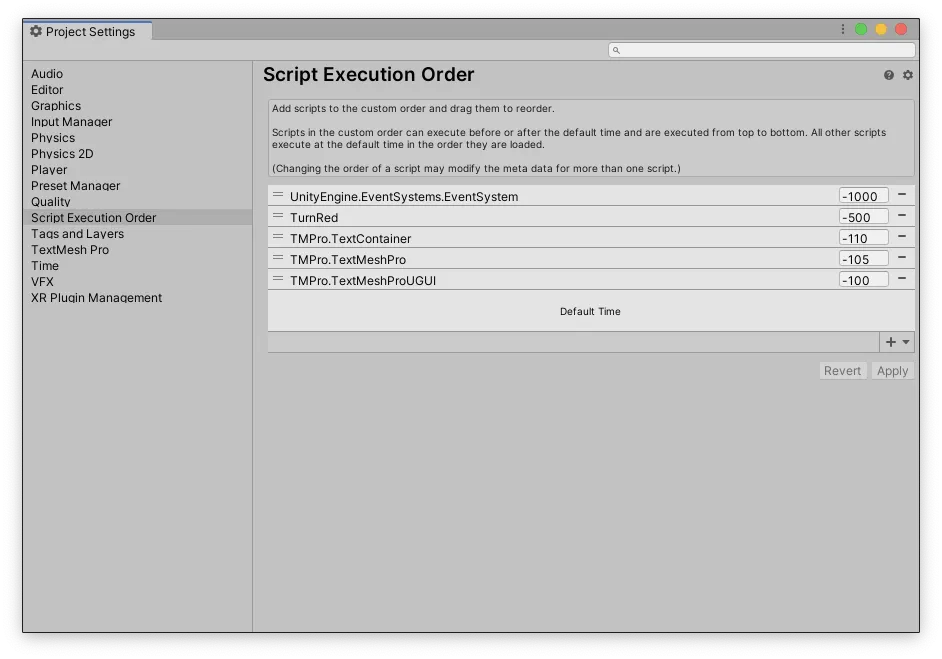

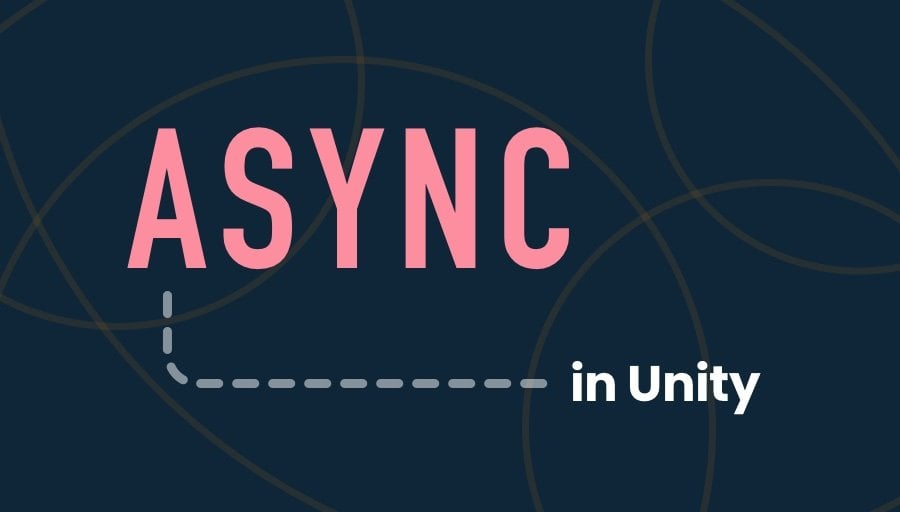

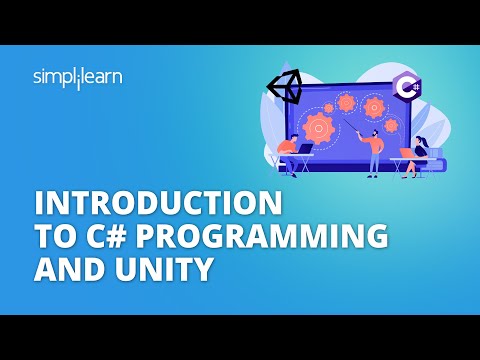
![Unity]シリアライズできるWaitForSeconds - Qiita Unity]シリアライズできるWaitforseconds - Qiita](https://qiita-user-contents.imgix.net/https%3A%2F%2Fcdn.qiita.com%2Fassets%2Fpublic%2Farticle-ogp-background-9f5428127621718a910c8b63951390ad.png?ixlib=rb-4.0.0&w=1200&mark64=aHR0cHM6Ly9xaWl0YS11c2VyLWNvbnRlbnRzLmltZ2l4Lm5ldC9-dGV4dD9peGxpYj1yYi00LjAuMCZ3PTkxNiZ0eHQ9JTVCVW5pdHklNUQlRTMlODIlQjclRTMlODMlQUElRTMlODIlQTIlRTMlODMlQTklRTMlODIlQTQlRTMlODIlQkElRTMlODElQTclRTMlODElOEQlRTMlODIlOEJXYWl0Rm9yU2Vjb25kcyZ0eHQtY29sb3I9JTIzMjEyMTIxJnR4dC1mb250PUhpcmFnaW5vJTIwU2FucyUyMFc2JnR4dC1zaXplPTU2JnR4dC1jbGlwPWVsbGlwc2lzJnR4dC1hbGlnbj1sZWZ0JTJDdG9wJnM9NTE1NDczMzE0NTFlYTI1Mzg5YTM3MGIwYmJiMGI1MDU&mark-x=142&mark-y=112&blend64=aHR0cHM6Ly9xaWl0YS11c2VyLWNvbnRlbnRzLmltZ2l4Lm5ldC9-dGV4dD9peGxpYj1yYi00LjAuMCZ3PTYxNiZ0eHQ9JTQwQmFzdWhlaV9BY2hlcm9uJnR4dC1jb2xvcj0lMjMyMTIxMjEmdHh0LWZvbnQ9SGlyYWdpbm8lMjBTYW5zJTIwVzYmdHh0LXNpemU9MzYmdHh0LWFsaWduPWxlZnQlMkN0b3Amcz05ZTU0MTFkNDdjYzBjZmYwZjA0ZmI4N2E3MmFkZDEyYg&blend-x=142&blend-y=491&blend-mode=normal&s=da9ef4cac412567fed5034871e92b755)
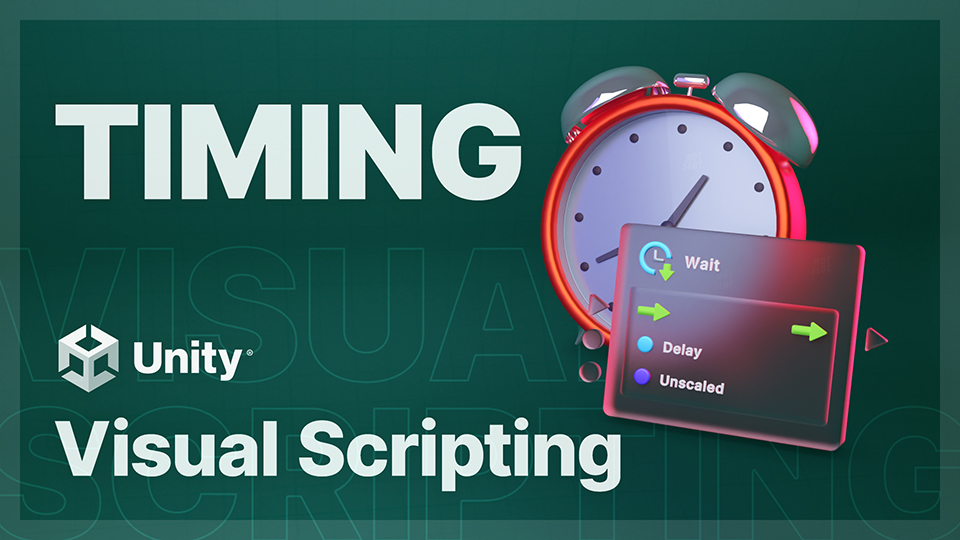
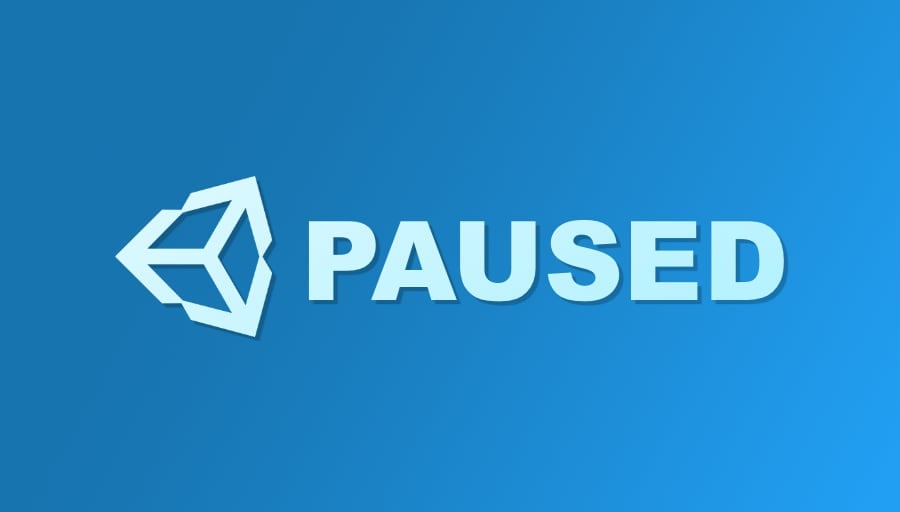
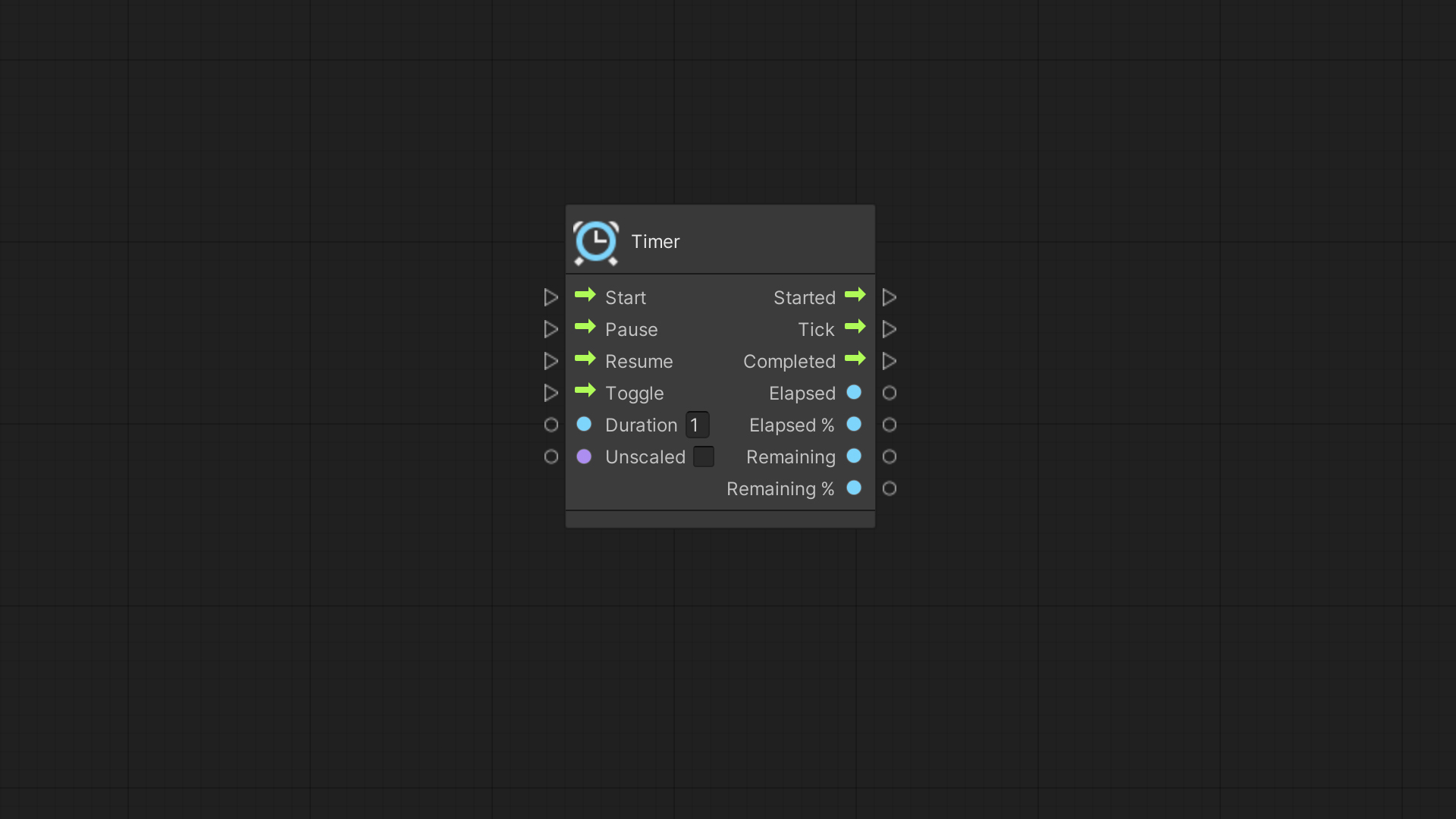
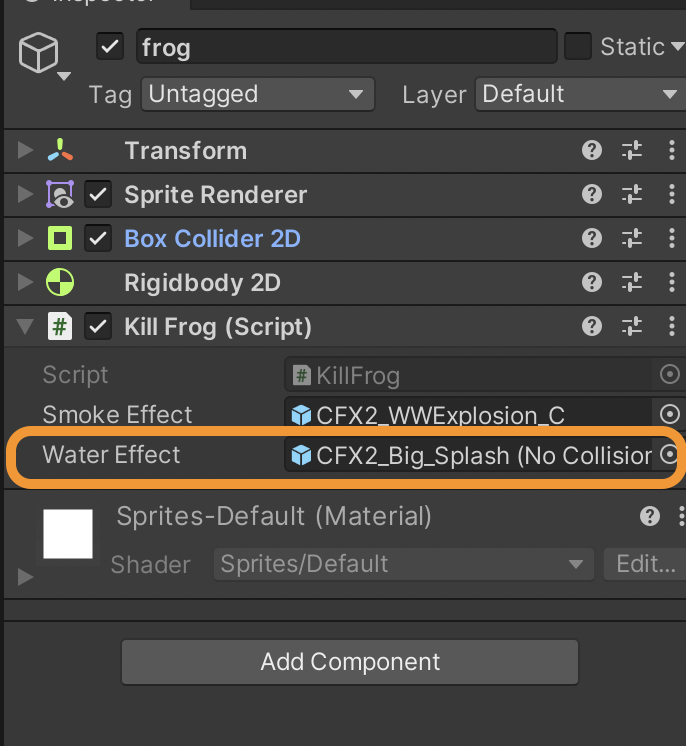

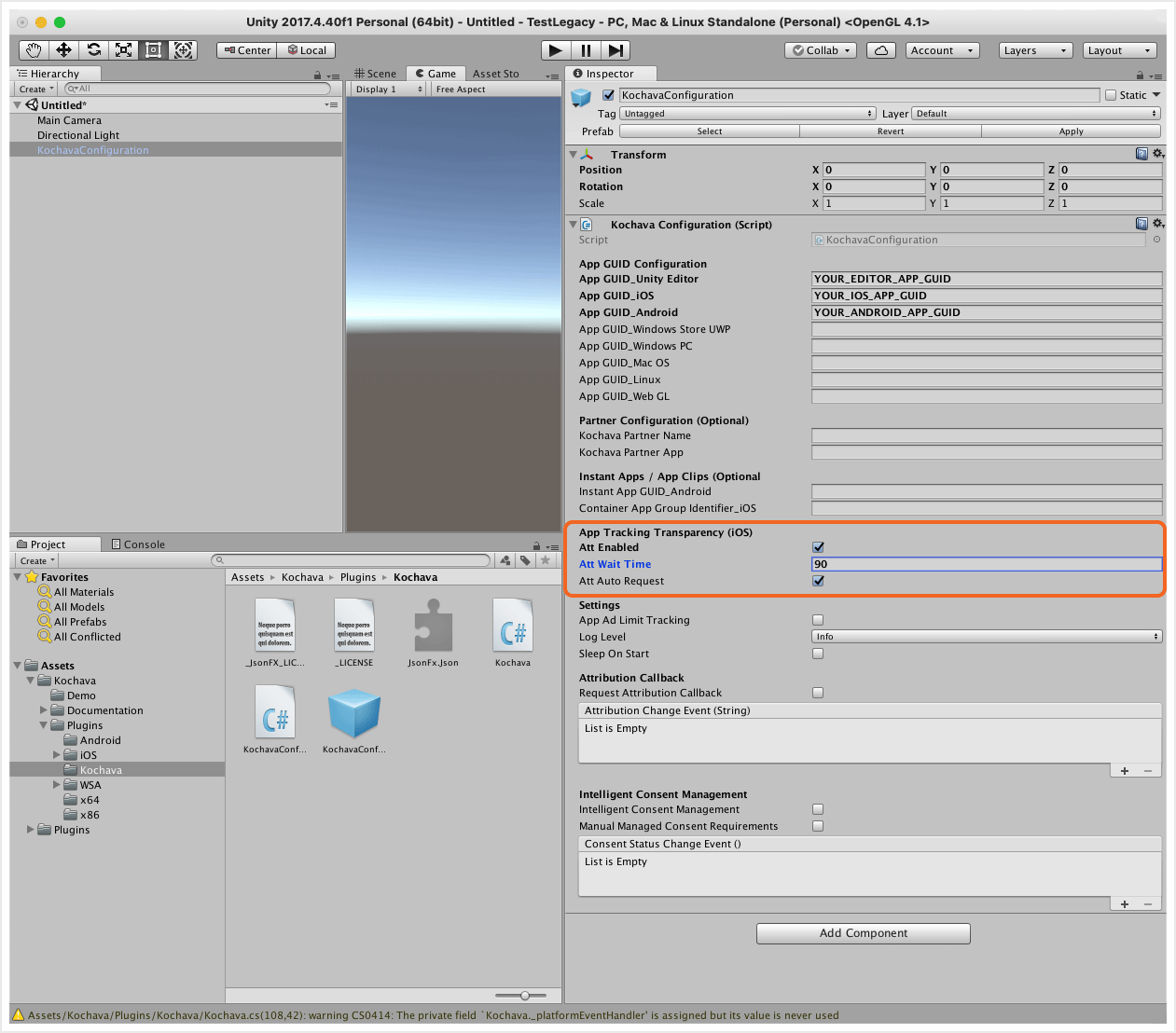

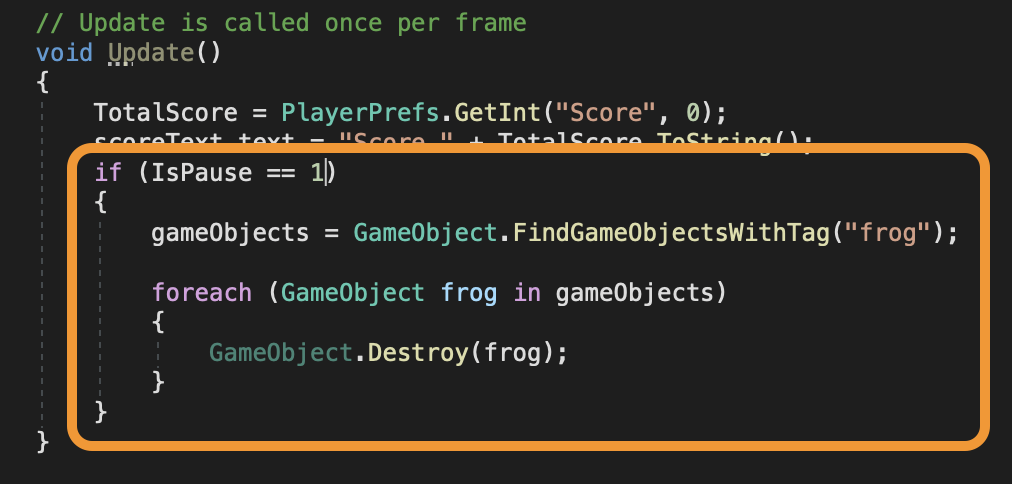
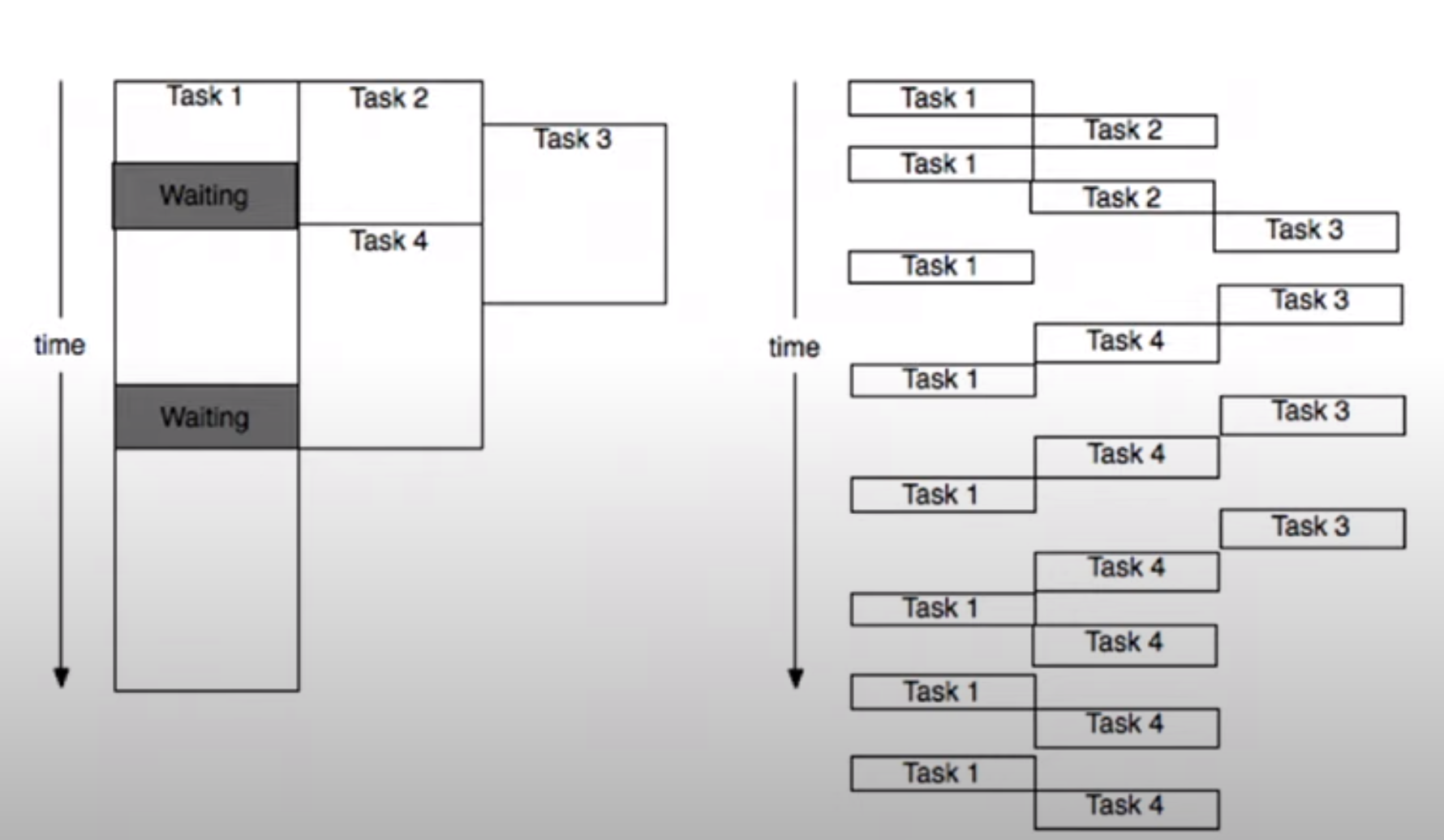
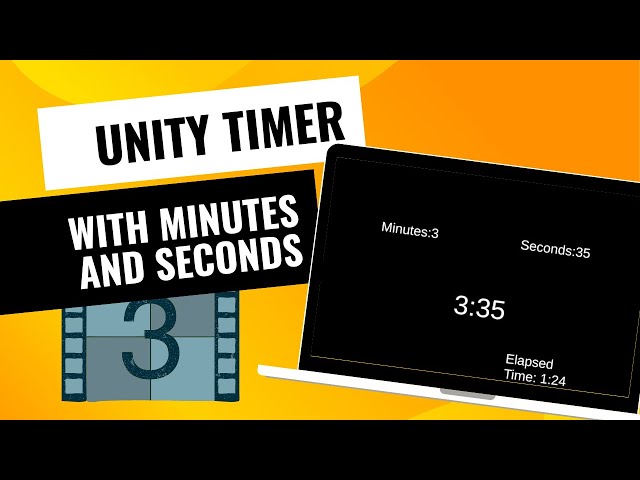
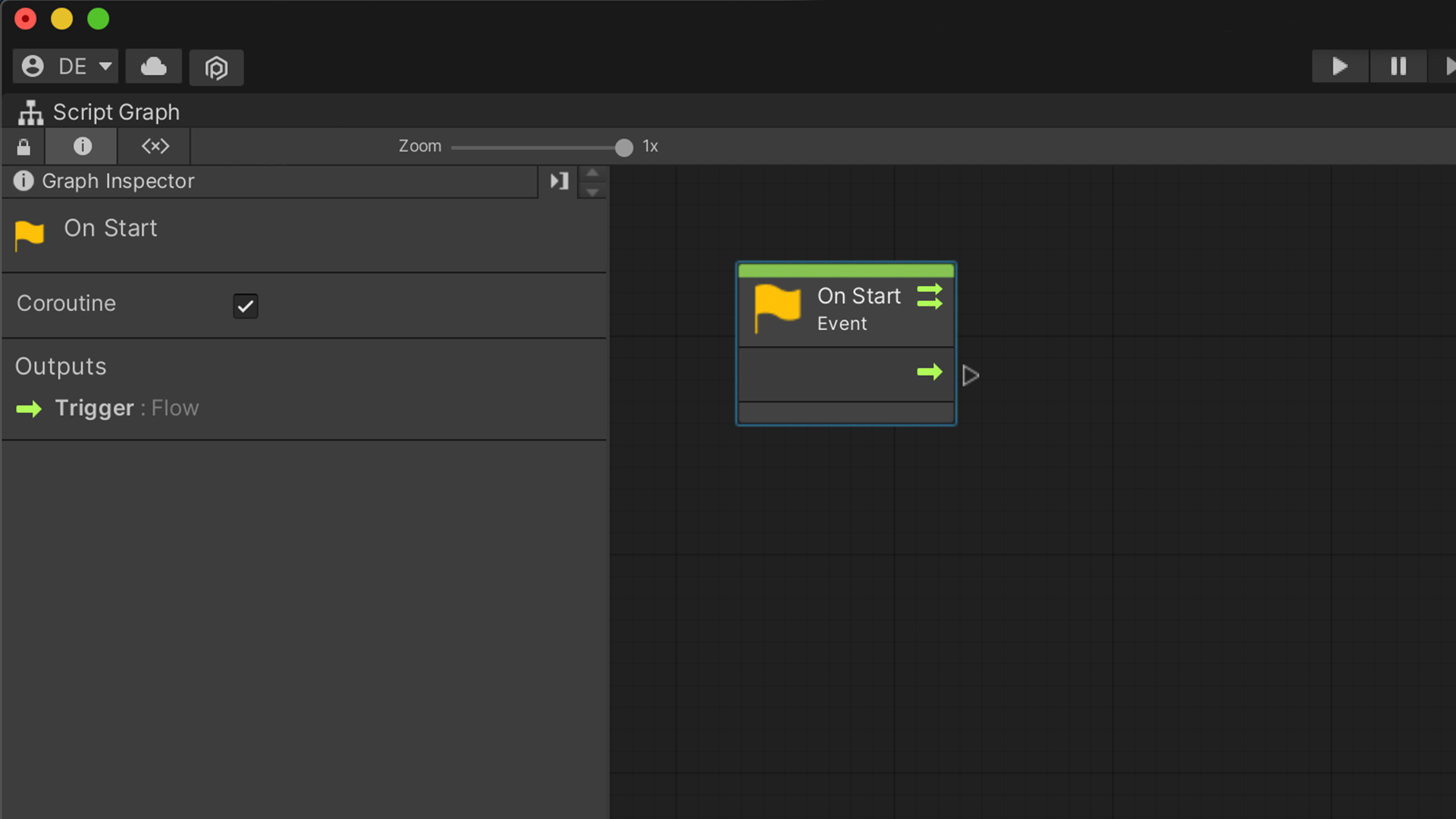
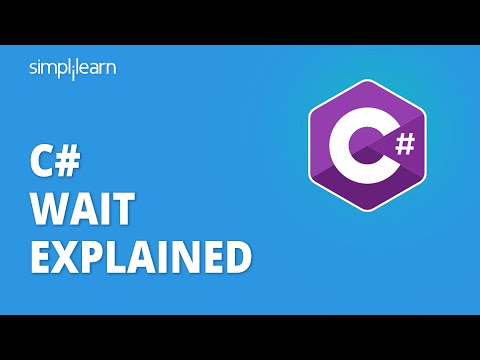

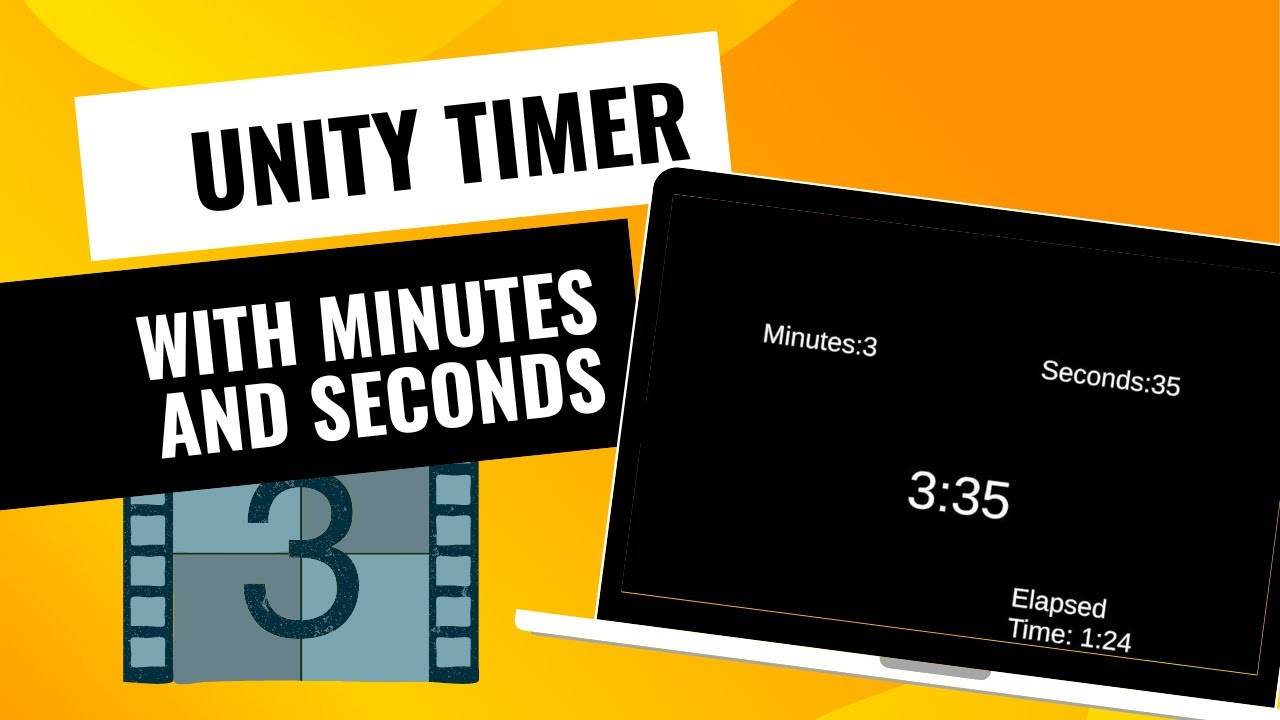

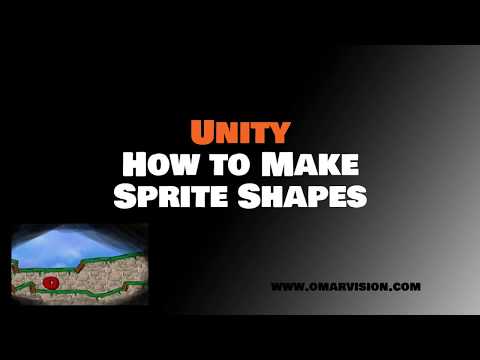
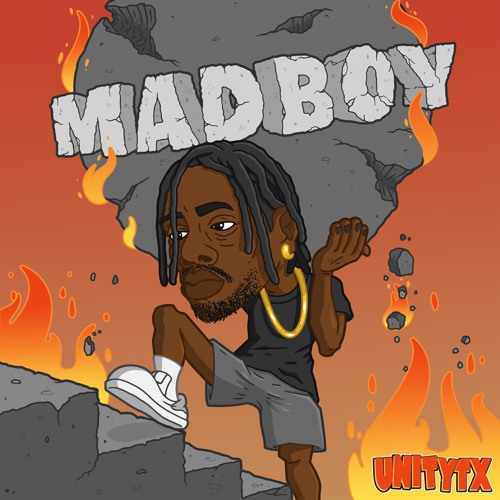

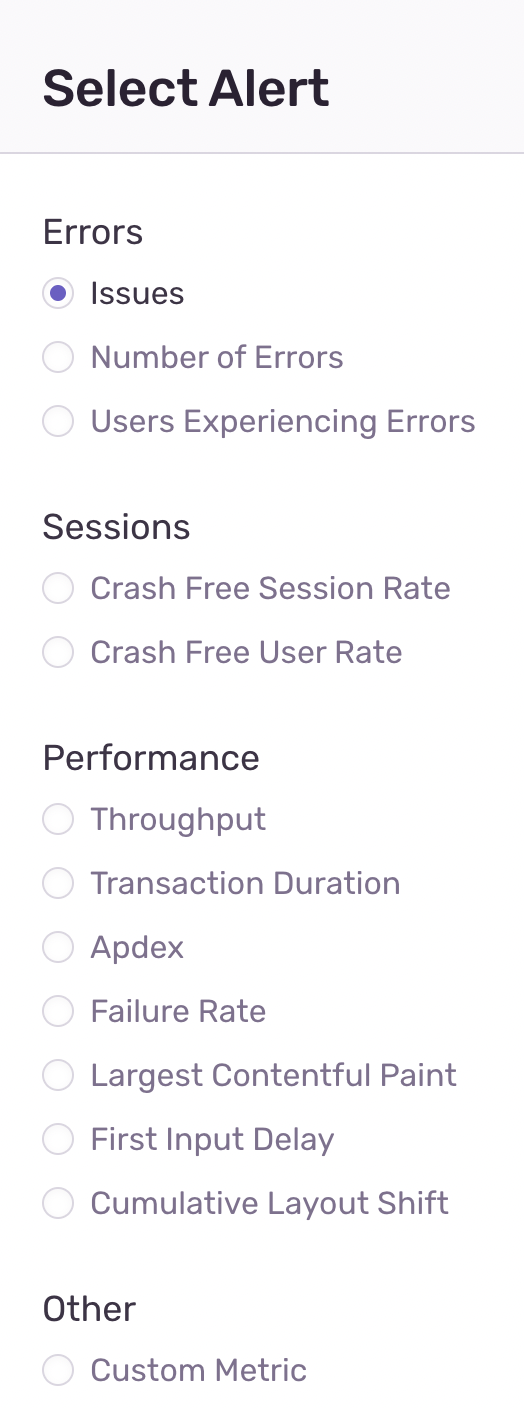
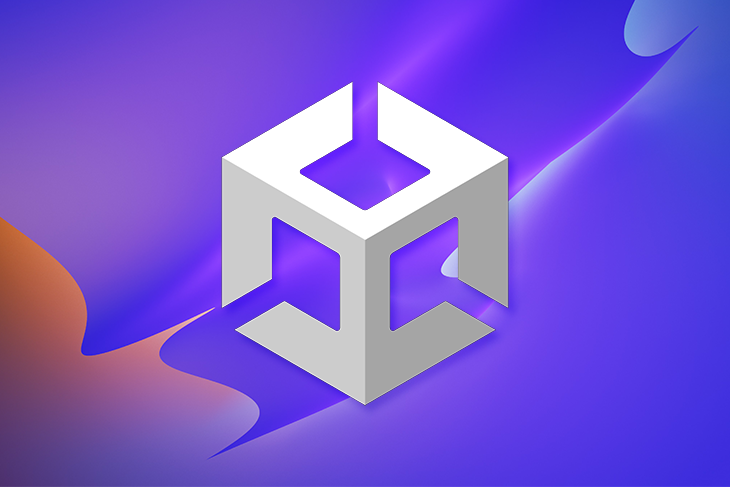
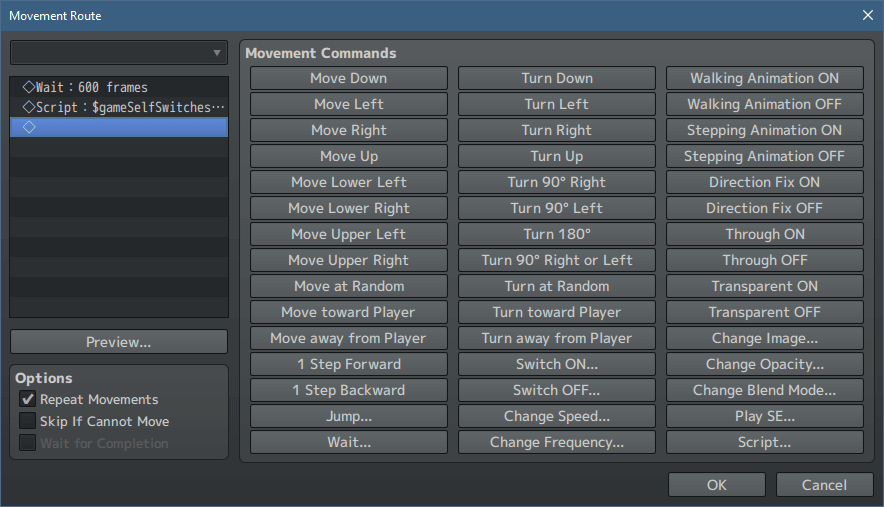


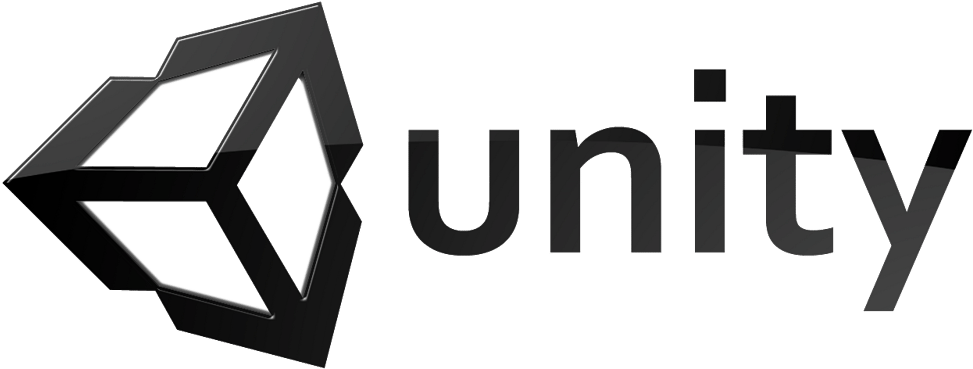
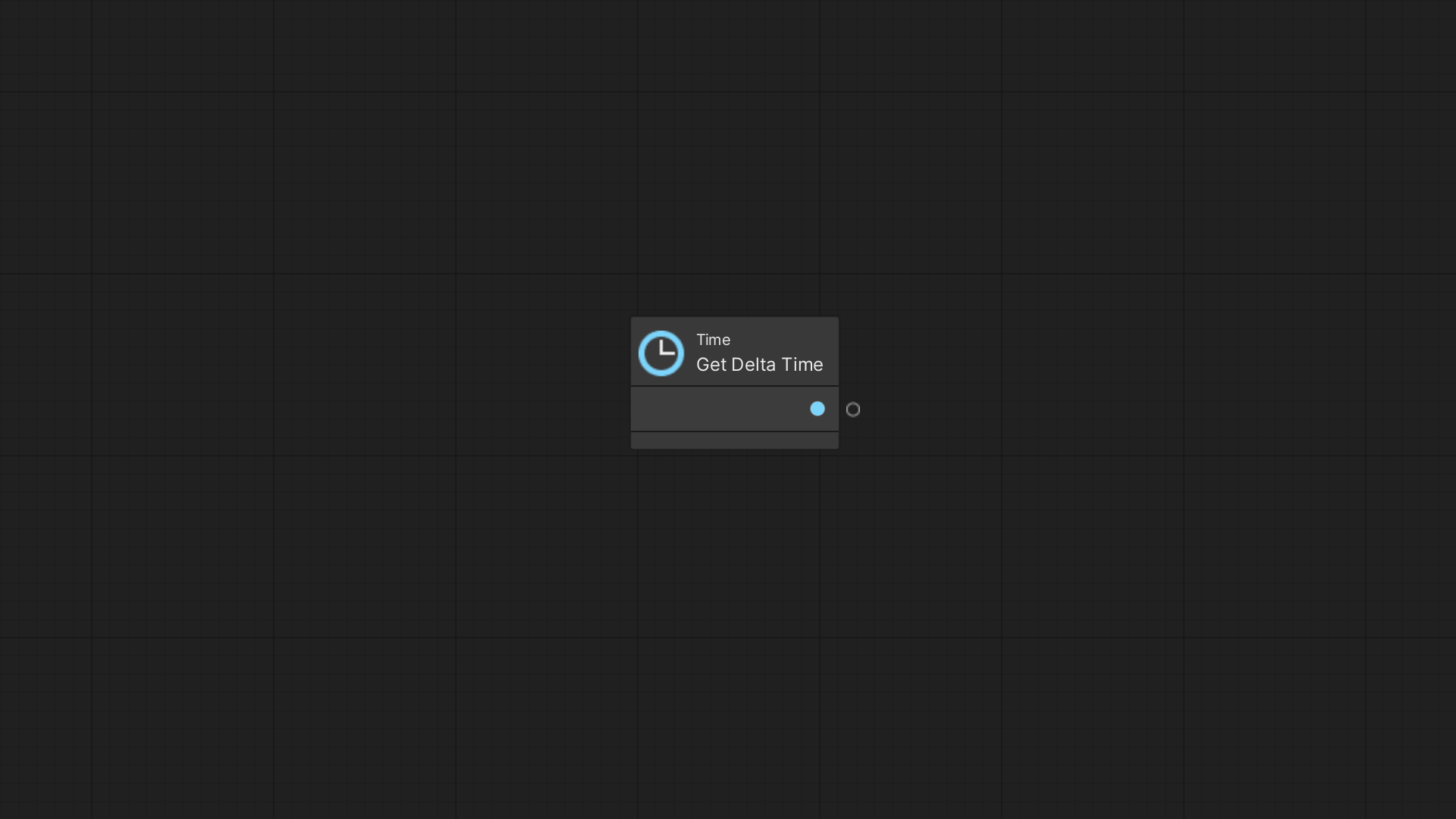

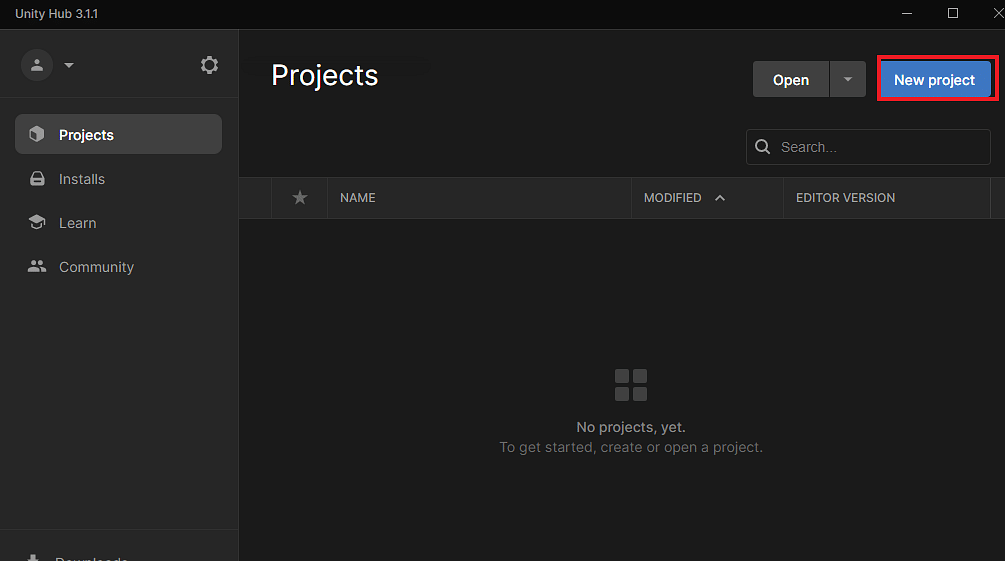
Article link: unity wait for seconds.
Learn more about the topic unity wait for seconds.
- How to make the script wait/sleep in a simple way in unity
- Using coroutines in Unity – LogRocket Blog
- Unity – Coroutines – Tutorialspoint
- Can I use Thread.Sleep(milliseconds) instead of a coroutine to pause a …
- How to delay a function in Unity – Game Dev Beginner
- How to create a C# method for waiting a number of seconds in …
- Adding a delay into the middle of a function in Unity
- How do you wait an amount of seconds in unity? : r/Unity3D
See more: nhanvietluanvan.com/luat-hoc