Unity Display Text For A Few Seconds
Unity is a popular game development platform known for its versatility and user-friendly interface. One of the essential features of any game or application is displaying text for a few seconds to provide important information or instructions to the users. In this article, we will explore various techniques and methods to achieve this functionality using Unity’s robust text display capabilities.
Introduction to Unity Display Text
Unity provides several ways to display text, including the Text and TextMesh components. These components allow developers to add text elements to the scene and modify their properties, such as font, size, and color. However, simply adding static text to the scene is often not enough, and we need to control its display time or even add animation effects to enhance the user experience. Let’s dive deeper into implementing these functionalities.
Implementing a Timer Function
To display text for a few seconds, we need to implement a timer function that tracks the elapsed time and hides the text after the desired duration. One effective approach is to use Unity’s built-in IEnumerator mechanism combined with the WaitForSeconds function. Here’s an example:
“`
IEnumerator DisplayTextForSeconds(float seconds)
{
textObject.SetActive(true); // Show the text
yield return new WaitForSeconds(seconds);
textObject.SetActive(false); // Hide the text
}
“`
In this code snippet, we first set the text object to active, which makes it visible to the user. Then, we use the WaitForSeconds function to wait for the specified number of seconds before executing the next line of code, which hides the text by setting it to inactive.
Controlling the Display Time
Unity provides various ways to control the display time of the text. You can adjust the duration by passing different values to the WaitForSeconds function in the previous code snippet. Additionally, you can also pause the timer or change its behavior using techniques like Unity’s WaitForSecondsRealtime or WaitForSecondsScaled. These functions allow you to create more dynamic text display scenarios by manipulating or extending the timer duration based on certain conditions, such as player input or game events.
Adding Animation Effects
Adding animation effects to the display text can greatly enhance the user experience and make the information more engaging. Unity offers several animation techniques that can be combined with text display functionalities. You can utilize the Animation interface to create animations for the text, such as fading in or out, scaling, rotating, or even applying complex motion behaviors.
You can also leverage Unity’s powerful animation asset, Animator, to create more advanced animations. By specifying animation states and transitions, you can easily control the display time, animation duration, and even trigger animation events based on specific conditions or user interactions.
Advanced Techniques for Text Display
While the aforementioned techniques cover the basics of displaying text for a few seconds in Unity, there are several advanced techniques that can take your text display capabilities to the next level. Let’s briefly discuss some of these techniques:
1. Unity Actions: UnityAction and UnityEvent are powerful features that allow you to invoke methods or trigger events based on certain conditions. You can leverage UnityActions to execute specific actions after the text is displayed for a few seconds. For example, you can call a method to trigger the next level or perform a specific game logic.
2. Coroutines: Unity’s Coroutine system provides more flexibility in managing complex behaviors and sequences. By using StartCoroutine and yield return, you can create custom logic for displaying text for a few seconds along with more intricate conditions and transitions.
Conclusion
In this article, we have explored various techniques and methods for displaying text for a few seconds using Unity’s versatile text display capabilities. We started by implementing a timer function using Unity’s IEnumerator mechanism and WaitForSeconds function. Then, we discussed controlling the display time, adding animation effects, and advanced techniques like Unity Actions and Coroutines. By leveraging these techniques, you can create engaging and interactive text displays that enhance the overall user experience in your Unity projects.
Frequently Asked Questions (FAQs)
Q: How can I make text appear and disappear in Unity?
A: You can make text appear and disappear in Unity by enabling or disabling the text object using the SetActive() method or modifying the text component’s alpha value.
Q: How do I hide text in Unity?
A: To hide text in Unity, you can set the text object’s visibility to false by calling SetActive(false) or modifying the text component’s alpha value to 0.
Q: How can I pause the execution for a few seconds in Unity?
A: You can pause the execution for a few seconds in Unity by using the WaitForSeconds function combined with a Coroutine or by utilizing other techniques like WaitForSecondsRealtime or WaitForSecondsScaled.
Q: Why is Unity’s WaitForSeconds not working?
A: If WaitForSeconds is not working as expected, make sure that you are using it within a Coroutine function and have assigned a proper duration to it. Additionally, check if there are any other conditions or code conflicts interfering with the expected functionality.
Q: How can I use UnityAction to invoke a method after displaying text for a few seconds?
A: You can use UnityAction.Invoke to invoke a specific method or trigger an event after displaying text for a few seconds. Assign the desired method to the UnityAction and call Invoke within your timer function.
Q: How can I display text for a specific number of frames in Unity?
A: To display text for a specific number of frames in Unity, you can use Unity’s Time.frameCount property along with a counter variable. Increment the counter in each frame and hide the text once it reaches the desired frame count.
Q: Can I use animations to make my displayed text more engaging in Unity?
A: Yes, you can use animations to make your displayed text more engaging in Unity. Utilize the Animation or Animator interface to create various animation effects like fading, scaling, rotation, or even complex motion behaviors for your text.
Unity Waitforseconds
How To Display Text From Script Unity?
Unity is a popular game development platform that offers a wide range of tools and features to create interactive and immersive experiences. One important aspect of game development is displaying text on the screen, whether it’s for dialogue, instructions, or other in-game text. In this article, we will explore various methods to display text from a script in Unity, ensuring that your game communicates effectively with players.
Table of Contents:
1. Introduction
2. Using GUI Text
3. Using UI Text
4. Using TextMesh Pro
5. FAQs
1. Introduction:
In Unity, displaying text is achieved by attaching the text components to a game object and manipulating their properties through scripts. There are multiple ways to do this, with each approach offering its own set of advantages and limitations. Let’s explore these methods step by step.
2. Using GUI Text:
GUI Text is a basic built-in feature in Unity that allows you to display text directly on the screen. Here’s how you can use it:
a. Create a new game object or select an existing one.
b. Add the GUI Text component to the game object by selecting “Add Component” and searching for “GUI Text.”
c. Customize the font, size, color, position, and other properties of the GUI Text component from the Inspector panel.
d. To display text dynamically from a script, access the GUI Text component in your script using the GetComponent method.
e. Use the text property to modify the content of the GUI Text dynamically.
While GUI Text is relatively simple to use, it has limited versatility and lacks many advanced features offered by other methods.
3. Using UI Text:
Unity’s UI system provides a more robust and flexible way to display text compared to GUI Text. Here’s how you can use UI Text:
a. Create a new UI Text object by right-clicking in the Hierarchy panel and selecting “UI” > “Text.”
b. Customize the text’s font, size, color, alignment, and other properties from the Inspector panel.
c. To access UI Text from a script, use the GetComponent method to retrieve the UI Text component.
d. Manipulate the text property to update the displayed text dynamically.
UI Text offers a wide range of options for styling, formatting, and animation, making it suitable for most text display requirements. It also works seamlessly with Unity’s Canvas system, enabling easy positioning and scaling.
4. Using TextMesh Pro:
TextMesh Pro is a powerful third-party asset that provides even more advanced text features compared to the built-in Unity methods. Although it requires importing and setting up, it offers unparalleled customization and performance benefits. Here’s how you can use TextMesh Pro:
a. Install and import the TextMesh Pro package into your project via the Unity Package Manager.
b. Create a new TextMesh Pro GameObject by right-clicking in the Hierarchy panel and selecting “3D Object” > “TextMeshPro – Text.”
c. Configure the text’s font, size, color, spacing, and other advanced settings using the TextMeshPro component in the Inspector panel.
d. Access the TextMeshPro component from your script using the GetComponent method.
e. Update the text property to display dynamically changing text.
TextMesh Pro excels in rendering high-quality, dynamically scalable, and fully customizable text. It supports complex text formatting, rich text, multi-line displays, and even text effects like gradient colors, outlines, and shadows.
5. FAQs:
Q1. Can I display different languages using these methods?
A1. Yes, all the methods mentioned—GUI Text, UI Text, and TextMesh Pro—support displaying text in various languages and character sets.
Q2. Can I animate text using these methods?
A2. While GUI Text does not offer built-in animation, both UI Text and TextMesh Pro provide animation options. You can modify properties like position, scale, rotation, color, and opacity over time using Unity’s animation system or via script.
Q3. Which method should I choose?
A3. The method you choose depends on your specific requirements. GUI Text is simpler but lacks features, while UI Text and TextMesh Pro offer more advanced options. If your game demands high-quality text, extensive formatting, rich effects, or extensive localization, TextMesh Pro is recommended.
Q4. Can I change text from a remote source, such as a database?
A4. Yes, you can update text dynamically from remote sources using these methods. By retrieving data from a database or API, you can modify the text property accordingly to display the desired content.
In conclusion, Unity offers various methods to display text from scripts, each suited for different needs. Understanding the differences between GUI Text, UI Text, and TextMesh Pro allows you to choose the most suitable method to achieve your desired text display in Unity, enhancing the overall game experience for players.
Why Doesn T Text Show Up In Unity?
Unity is a powerful and versatile game development platform used by millions of developers worldwide. It allows you to create stunning games and interactive experiences for various platforms. Text is a crucial element in any game or application, as it helps convey information, instructions, and storylines to the players. However, sometimes developers may experience issues where text does not show up in Unity. In this article, we will explore the possible reasons behind this issue and provide solutions to help you resolve it.
1. Missing Font Assets:
One of the most common reasons why text doesn’t show up in Unity is the absence of required font assets. Unity relies on font assets to render text correctly. If you haven’t imported or assigned a font asset to your text elements, Unity will not be able to display text properly. Make sure to check if you have the necessary font assets in your project and assigned them correctly to your text components.
2. Incorrect Font Material Setup:
In some cases, text may not appear in Unity due to incorrect font material setup. Font materials are responsible for determining how text is rendered in Unity. If a font material has not been set up correctly or is missing, it can lead to the text not being displayed. To resolve this issue, ensure that your font materials are correctly assigned to your text components and configured properly.
3. Z-Fighting Issues:
Z-fighting refers to visual artifacts that occur when two or more objects share the same space in 3D rendering. If your text object is overlapping with other objects or UI elements, it might result in text not being visible. To fix this, make sure to adjust the position of the text objects or UI elements to prevent overlapping.
4. Canvas Render Mode:
The render mode of the canvas can also affect the visibility of text in Unity. The canvas is responsible for rendering UI elements, including text. If the render mode is set incorrectly, it can cause text to not show up. Check the render mode of your canvas and ensure it is appropriately set to either “Screen Space – Overlay” or “World Space” based on your requirements.
5. Text Component Settings:
Sometimes, the issue might lie within the settings of the text component itself. Check the properties of your text components such as color, size, alignment, and visibility. Ensure that the text color is not set to transparent, the font size is appropriate, and the alignment is correctly aligned for the desired location. Also, verify that the text component is enabled and not disabled, preventing it from being displayed.
FAQs:
Q1. Why is my text in Unity blurry or pixelated?
A blurry or pixelated appearance of text in Unity can be due to incorrect font size settings. Make sure that the font size is set appropriately for the resolution and display settings of your target platform. You can also consider using a font asset specifically designed for high resolutions.
Q2. I can see the text in the Unity editor, but it doesn’t show up in the game build. What could be the issue?
This issue commonly occurs when font assets are not included in the build. Ensure that the font assets are properly included in the build settings and are not marked as “Editor only.”
Q3. Why does my text disappear when I rotate the camera in Unity?
If your text disappears when you rotate the camera, it might indicate an issue with the canvas’s screen space position or UI layering. Adjust the canvas’s position and layering to ensure that the text remains visible from various camera angles.
Q4. How can I optimize text rendering in Unity?
To optimize text rendering in Unity, consider using font atlases or text meshes instead of individual text objects. This will reduce the number of draw calls and improve performance. Additionally, avoid excessively large or complex text objects to minimize any impact on performance.
In conclusion, when text doesn’t show up in Unity, it can be attributed to various factors such as missing font assets, incorrect font material setup, z-fighting issues, canvas render mode, or text component settings. By identifying and resolving these issues, you can ensure that your text is properly displayed in your Unity projects, enhancing the user experience and improving the overall quality of your games or applications.
Keywords searched by users: unity display text for a few seconds how to make text appear and disappear in unity, how to hide text unity, unity second, unity pause waitforseconds, unity yield, unity wait for seconds not working, unityaction invoke, unity wait for frames
Categories: Top 60 Unity Display Text For A Few Seconds
See more here: nhanvietluanvan.com
How To Make Text Appear And Disappear In Unity
Unity is a powerful game development platform that allows developers to create engaging and interactive experiences. Text can play a crucial role in conveying information and guiding users through a game or application. In this article, we will explore different methods to make text appear and disappear in Unity. We will cover both basic and advanced techniques, with code examples and explanations along the way.
Basic Method: Enabling and Disabling the Text Component
The most straightforward way to make text appear and disappear in Unity is by enabling and disabling the Text component. Every Text object in Unity contains a Text component, which has a property called “enabled.” By toggling the enabled property on or off, we can control the visibility of the text.
To implement this method, follow these steps:
1. Create a new GameObject in your scene and attach a Text component to it. You can do this by right-clicking in the hierarchy panel and selecting UI > Text.
2. Ensure that the GameObject with the Text component is selected. In the Inspector panel, find the Text component and uncheck the “enabled” checkbox to make it disappear.
3. Create a script (e.g., TextToggle.cs) and attach it to the GameObject with the Text component.
4. In the script, define a public method (e.g., ToggleText) that toggles the enabled property of the Text component. Use the GetComponent method to access the Text component.
Here’s an example implementation of the script:
“`csharp
using UnityEngine;
using UnityEngine.UI;
public class TextToggle : MonoBehaviour
{
private Text textComponent;
private void Start()
{
textComponent = GetComponent
}
public void ToggleText()
{
textComponent.enabled = !textComponent.enabled;
}
}
“`
Now, you can call the ToggleText method from another script or UI element to make the text appear and disappear. For example, you can create a button and call the ToggleText method when the button is clicked.
Advanced Method: Fade In and Fade Out Animations
While enabling and disabling the Text component provides a basic solution, it may not always yield the desired visual effect. To create smooth transitions, we can use animations to fade the text in and out gradually. Unity provides a powerful animation system, allowing us to animate properties of any component.
Here’s how you can achieve a fade in and fade out effect for text:
1. Create a new animation controller asset by right-clicking in the Project panel and selecting Create > Animator Controller.
2. Assign the newly created animation controller to the GameObject with the Text component by dragging it onto the GameObject in the Inspector panel.
3. Double-click the animation controller asset to open the Animator window.
4. Create two animation clips: one for fade in and another for fade out.
5. Open the fade in animation clip and select the Text component in the GameObject hierarchy.
6. In the Inspector panel, click on the “Add Property” button to add a new property to animate.
7. Choose “Canvas Group > Alpha” as the property and keyframe the values from 0 to 1 to create a fade-in effect over time.
8. Repeat the process for the fade out animation clip, but this time, keyframe the values from 1 to 0 to create a fade-out effect.
With the fade in and fade out animation clips ready, we can now trigger them in code or through UI interactions. For example, you can create a button and attach a script that plays the fade in animation when the button is clicked, and another script that plays the fade out animation when the button is clicked again.
FAQs
Q: How can I change the text content dynamically?
A: To change the text content dynamically, you can access the Text component from a script and modify its text property. For example, you can create a public variable of type string in the script attached to the GameObject with the Text component. Then, in other scripts or UI interactions, you can assign a new value to that variable, and the text content will update accordingly.
Q: Can I change the font, size, or other properties of the text?
A: Yes, you can modify various properties of the Text component to change the appearance of the text. In the Inspector panel, you can change the font, size, color, alignment, and many other properties according to your requirements.
Q: Can I apply different animations or effects to the text?
A: Yes, Unity offers a wide range of solutions for applying animations and effects to text. You can explore the Unity Asset Store for text-specific assets and plugins or utilize Unity’s built-in animation system to create custom animations.
In conclusion, making text appear and disappear in Unity can be achieved using basic techniques like enabling and disabling the Text component, or advanced methods involving animation clips. Depending on the desired visual effect and interaction, developers can choose the appropriate approach to create engaging and dynamic experiences in their Unity projects.
How To Hide Text Unity
Hiding text unity, or blending text seamlessly into the background, can be a useful skill to possess, especially in various design and creative projects. Whether you want to create a camouflage effect, add a hidden message, or incorporate a discreet watermark, this article will provide you with comprehensive guidance on how to hide text unity effectively. Let’s delve into the various techniques and best practices to achieve this artistic endeavor.
1. Choose the Right Font and Size:
The choice of font and its size play a crucial role in hiding text unity effectively. Opt for a font that matches the background color closely, enabling it to seamlessly blend into the background. Additionally, selecting a relatively smaller font size can create a more subtle and inconspicuous effect.
2. Adjust Letter Spacing and Kerning:
Tweaking the letter spacing and kerning of the text can further enhance the hidden effect. Narrowing the space between letters or slightly overlapping them can help the text appear as a cohesive part of the background.
3. Reduce Contrast:
A lower contrast between the text and the background can aid in hiding the text unity. Choose colors with similar darkness or lightness, enabling the text to merge more naturally into the background. For instance, using a light gray text on a white background can create an inconspicuous and hidden effect.
4. Apply Texture or Patterns:
Applying textures or patterns to the text can help in concealing it effectively. By incorporating textures similar to the background, such as a wood grain texture or a subtle pattern, the text can be seamlessly integrated, making it harder to detect.
5. Gradients and Color Blending:
Another technique to achieve hidden text unity is by utilizing gradients or color blending. Gradually transitioning the text color from one that matches the background to another can create a gradual fade effect, giving the illusion of hidden text.
6. Consider Opacity:
Adjusting the opacity of the text can contribute to hiding text unity as well. Decreasing the opacity slightly can make the text translucent, allowing the background to show through and making it appear more concealed.
FAQs:
Q: Can I use any font to hide text unity?
A: While you can use any font, it is recommended to choose a font that closely matches the background color and has a smaller size, as mentioned earlier. These factors contribute to a more seamless and hidden effect.
Q: What software can I use to hide text unity?
A: You can use various software, including graphic design programs like Adobe Photoshop, Illustrator, or online image-editing tools. These programs offer extensive tools and features to help you achieve the desired hidden text effect.
Q: How can I test the visibility of the hidden text?
A: To test the visibility of the hidden text, it is recommended to view the design from different distances and on various devices. This will help determine the effectiveness of the hidden text under different circumstances.
Q: Can I hide text unity while maintaining readability?
A: Depending on the purpose and desired effect, you may need to sacrifice some readability when hiding text unity. However, it is essential to find a balance, ensuring that the hidden text is still legible to those who are consciously searching for it.
Q: Are there any copyright concerns associated with hidden text unity?
A: Hidden text unity itself does not raise copyright concerns. However, it is essential to be aware of any copyright restrictions related to the text or background image you are using. Always ensure that you have appropriate rights and permissions for the content you incorporate into your design.
In conclusion, hiding text unity is a fascinating technique that can add an artistic touch to your designs, illustrations, or any creative endeavor. By following the tips and techniques outlined in this article, you will be able to seamlessly blend text into the background, creating hidden messages, camouflaged effects, or discreet watermarks. Remember to experiment and test your designs to achieve the desired hidden text effect while maintaining your intended artistic vision.
Images related to the topic unity display text for a few seconds
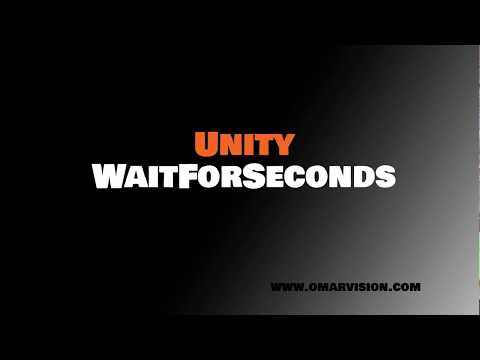
Found 42 images related to unity display text for a few seconds theme
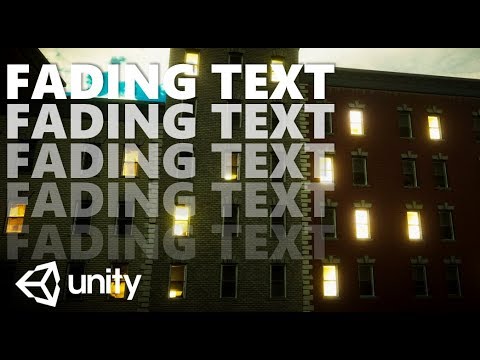
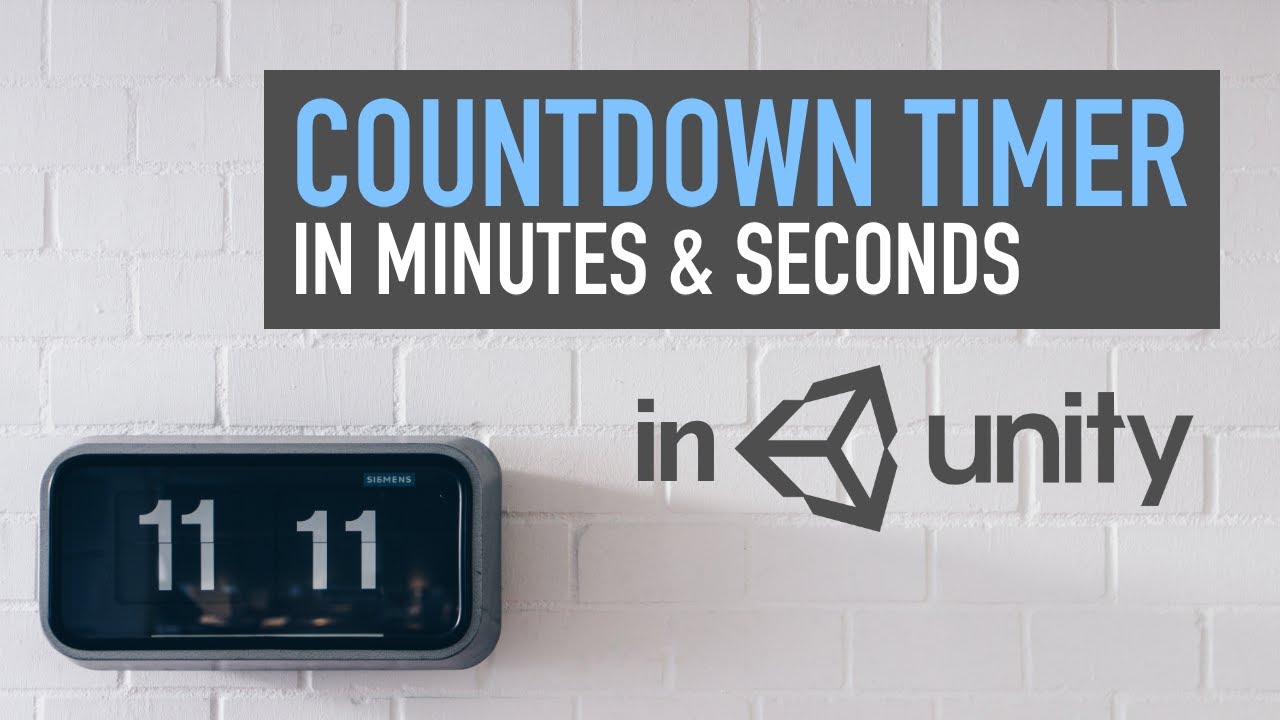
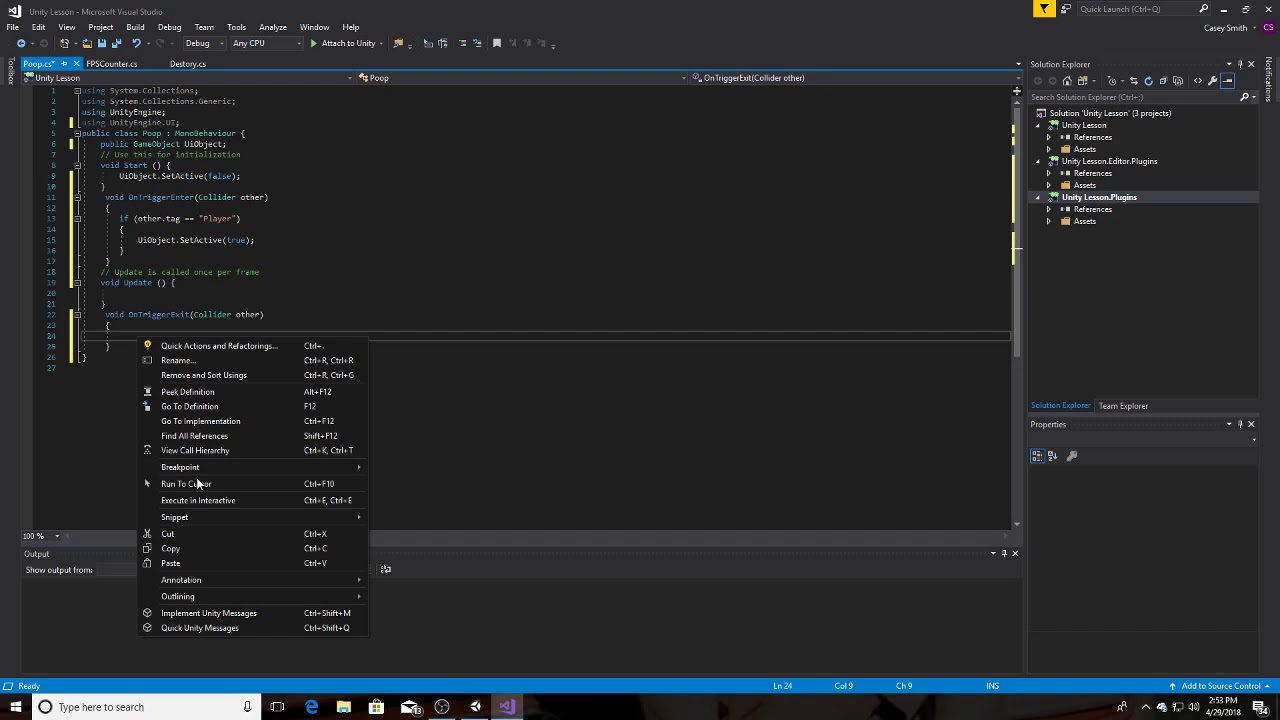
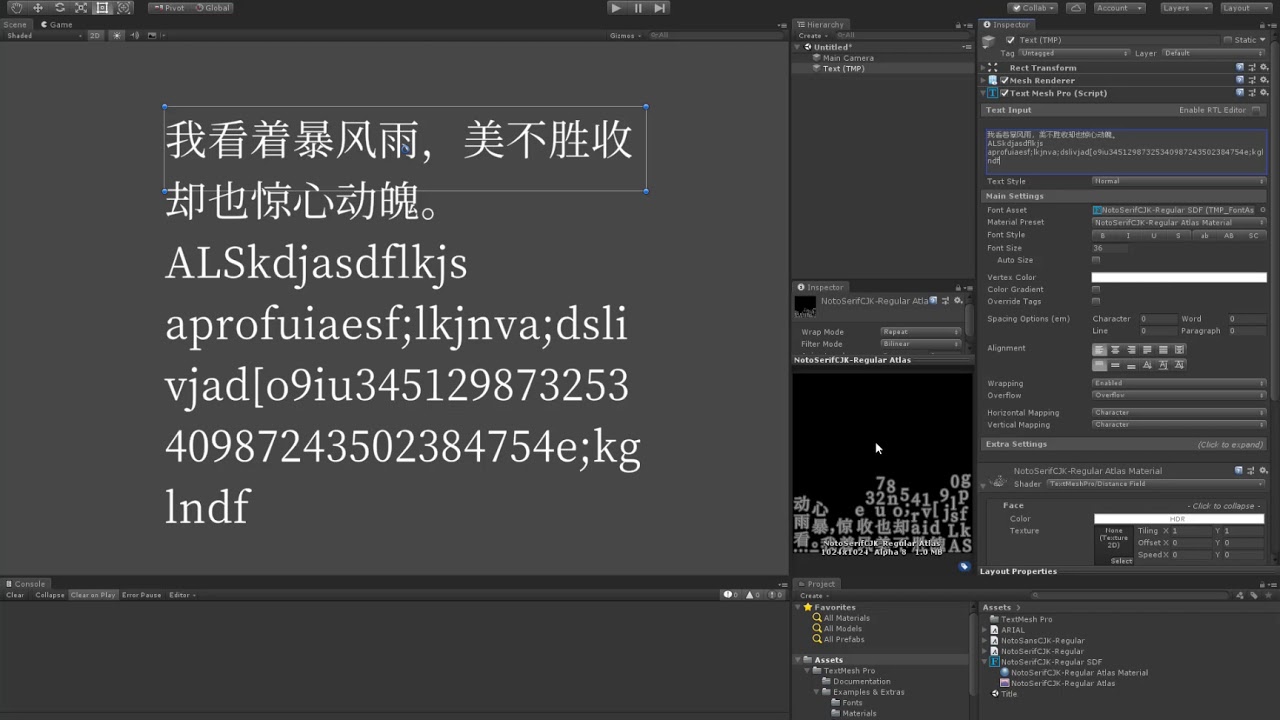
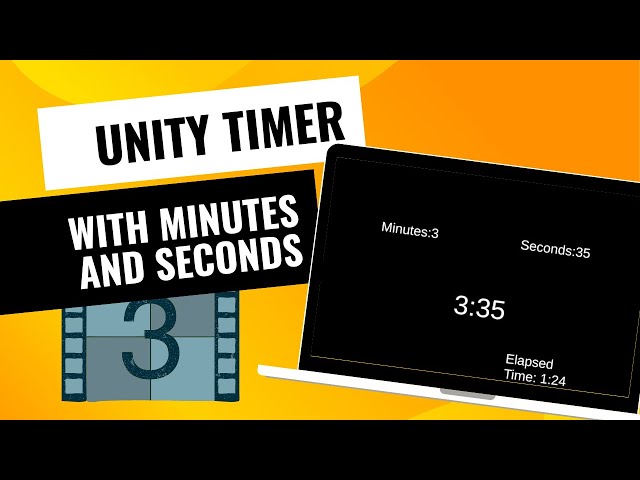
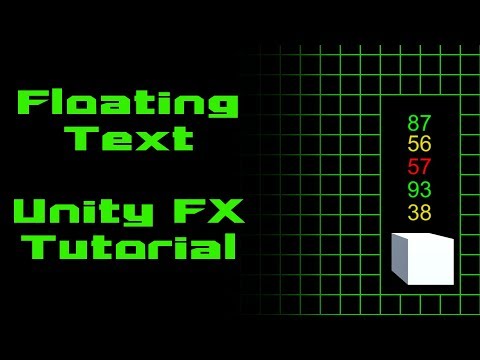
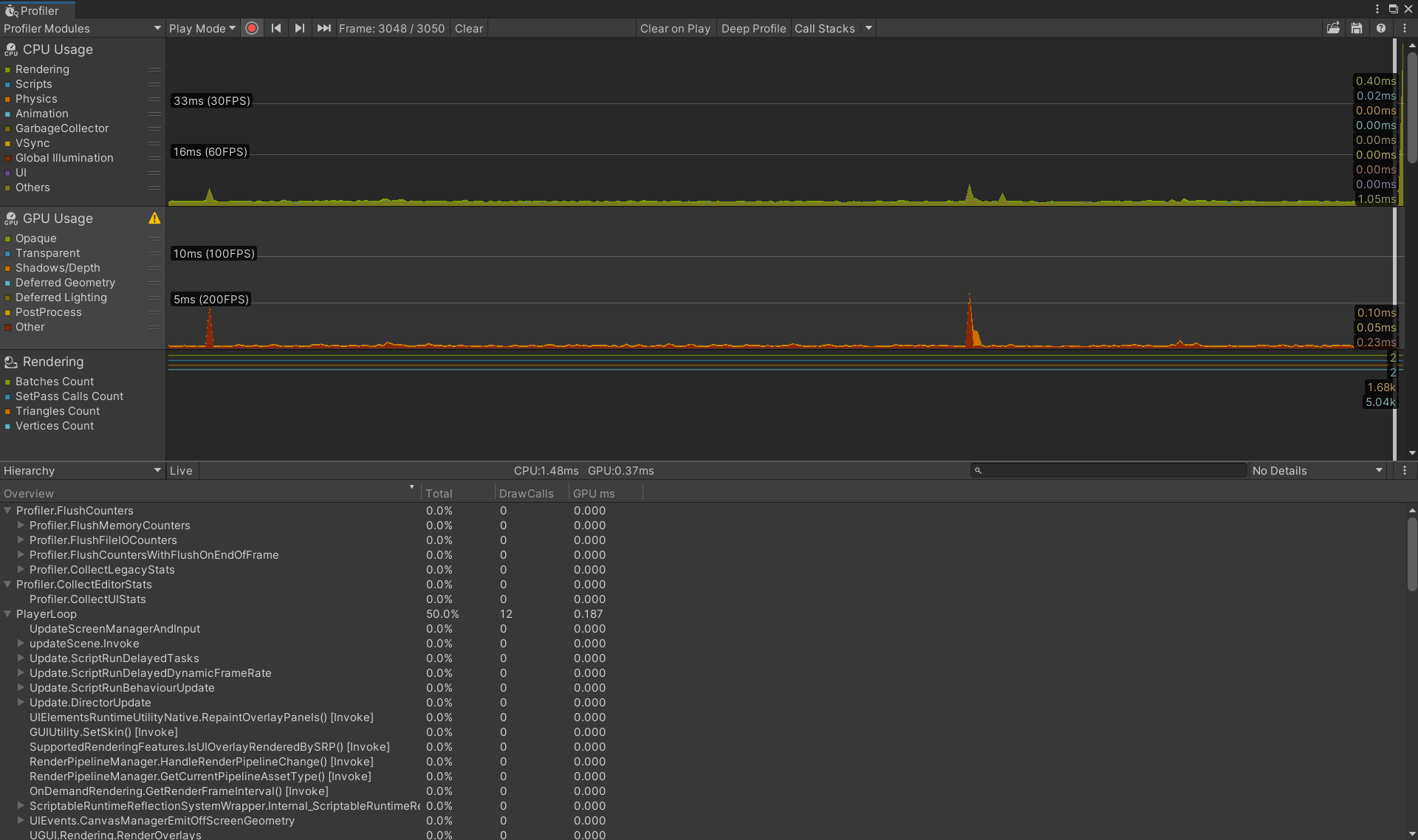
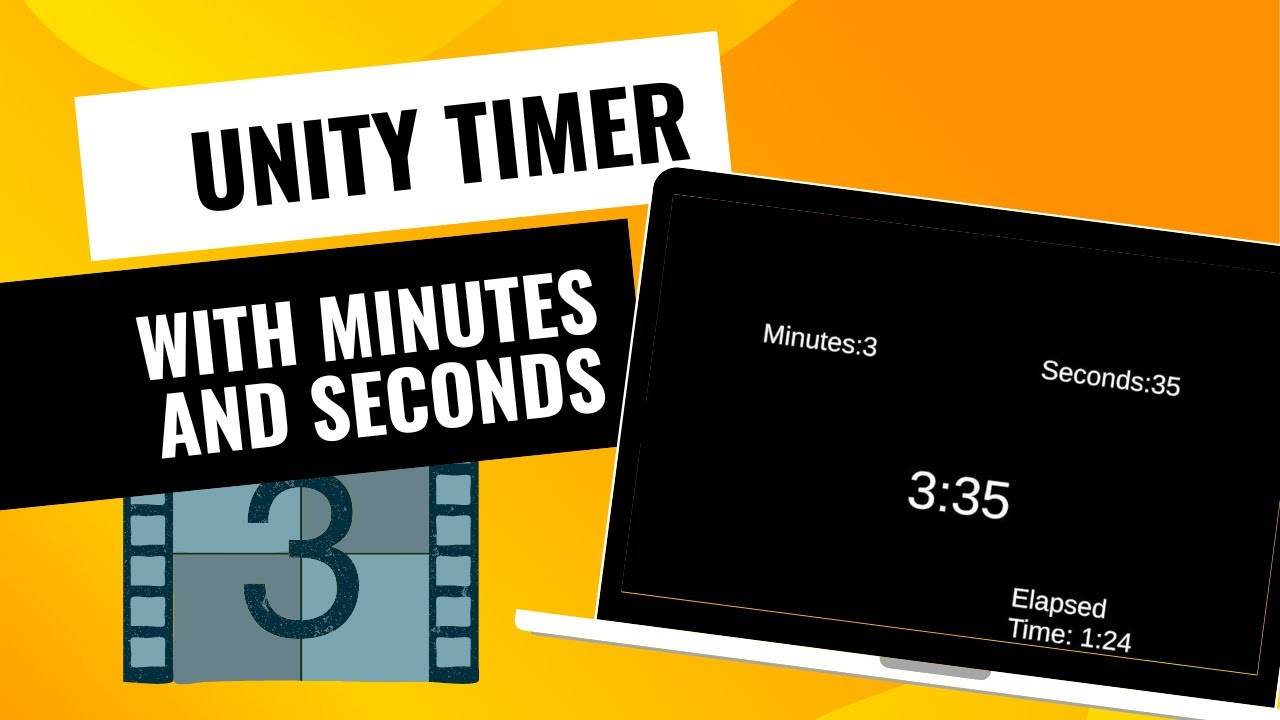
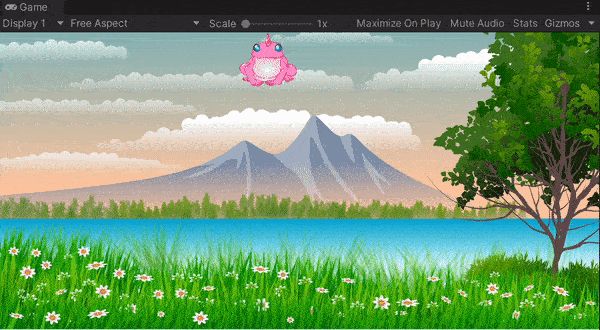
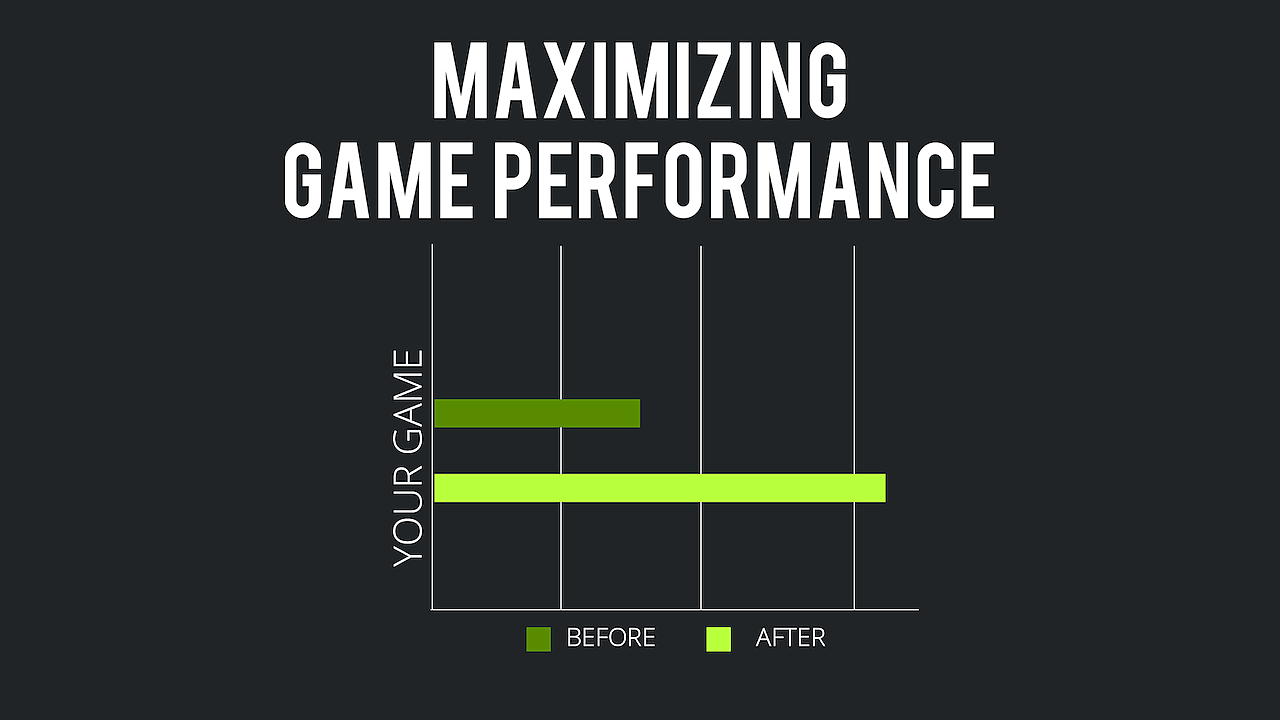
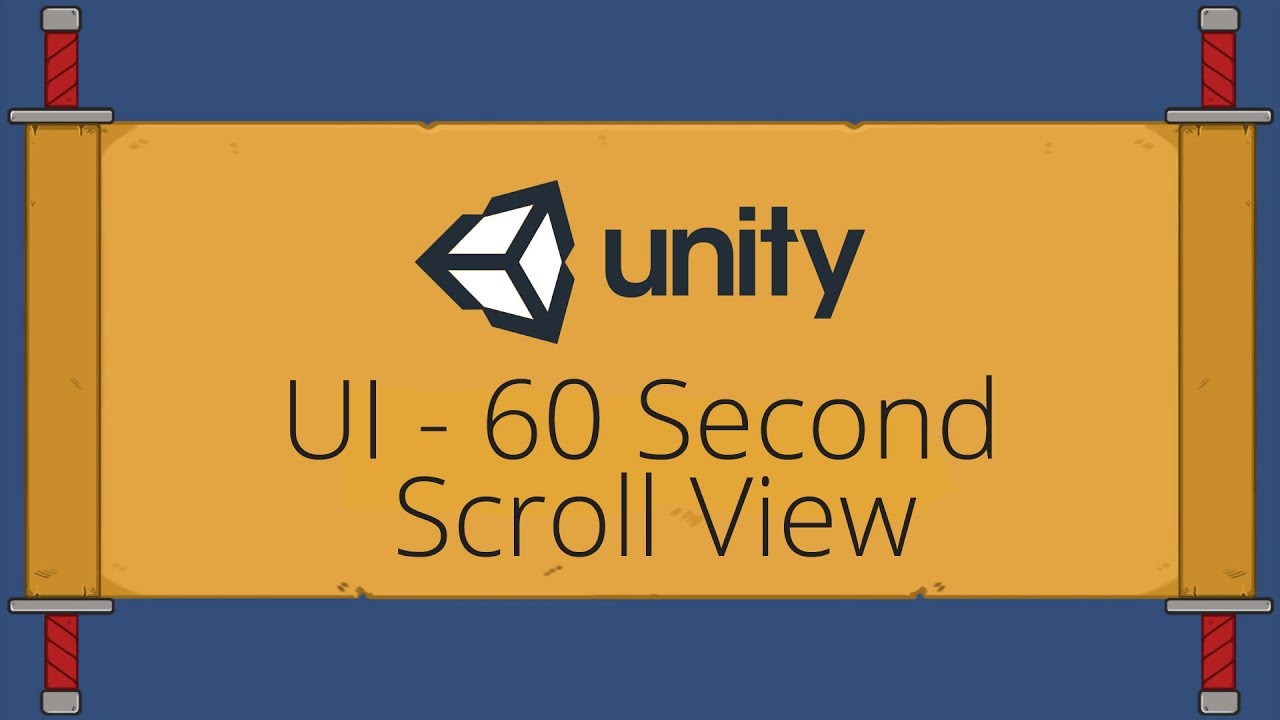

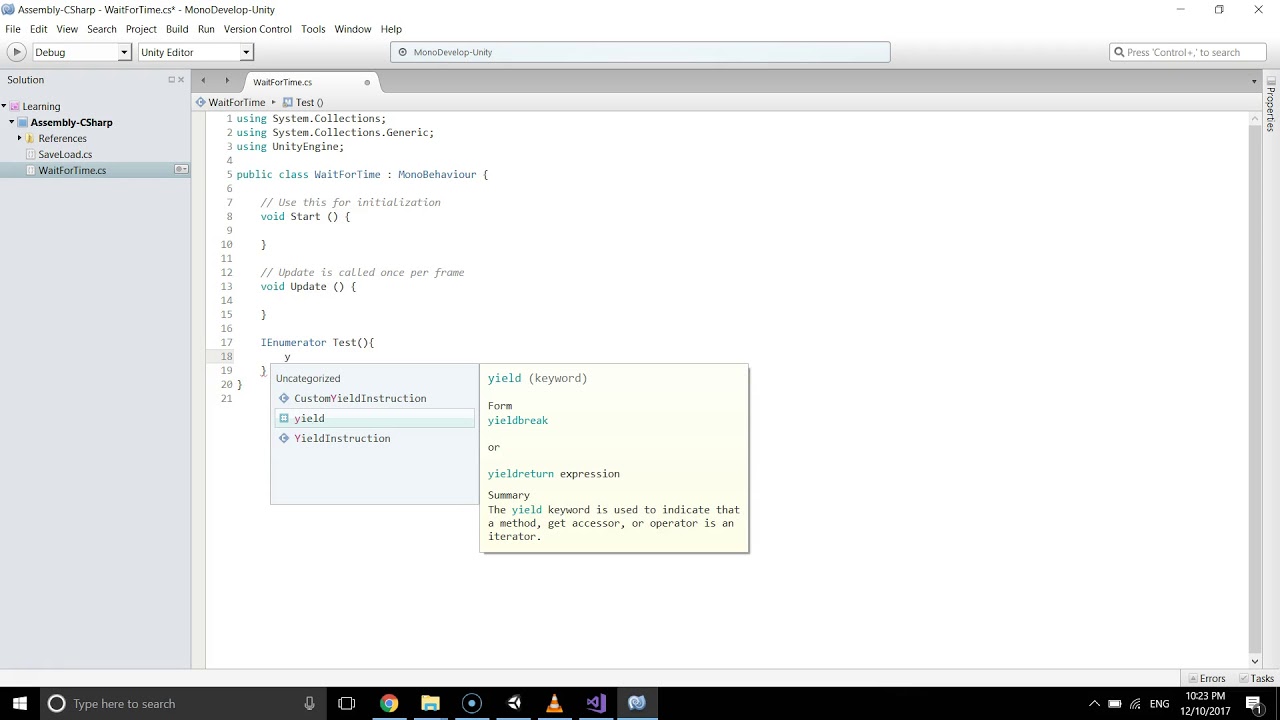
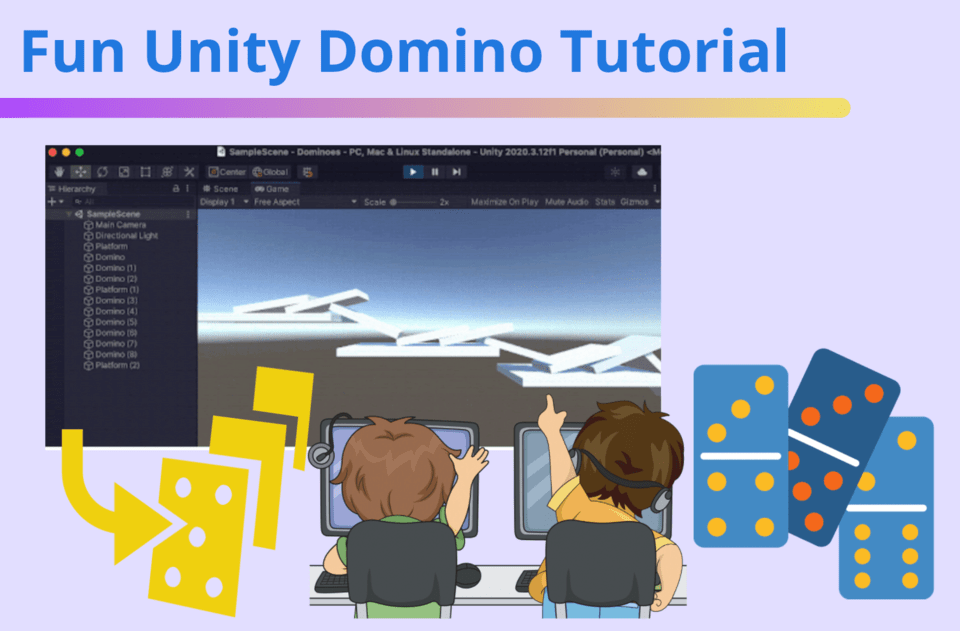
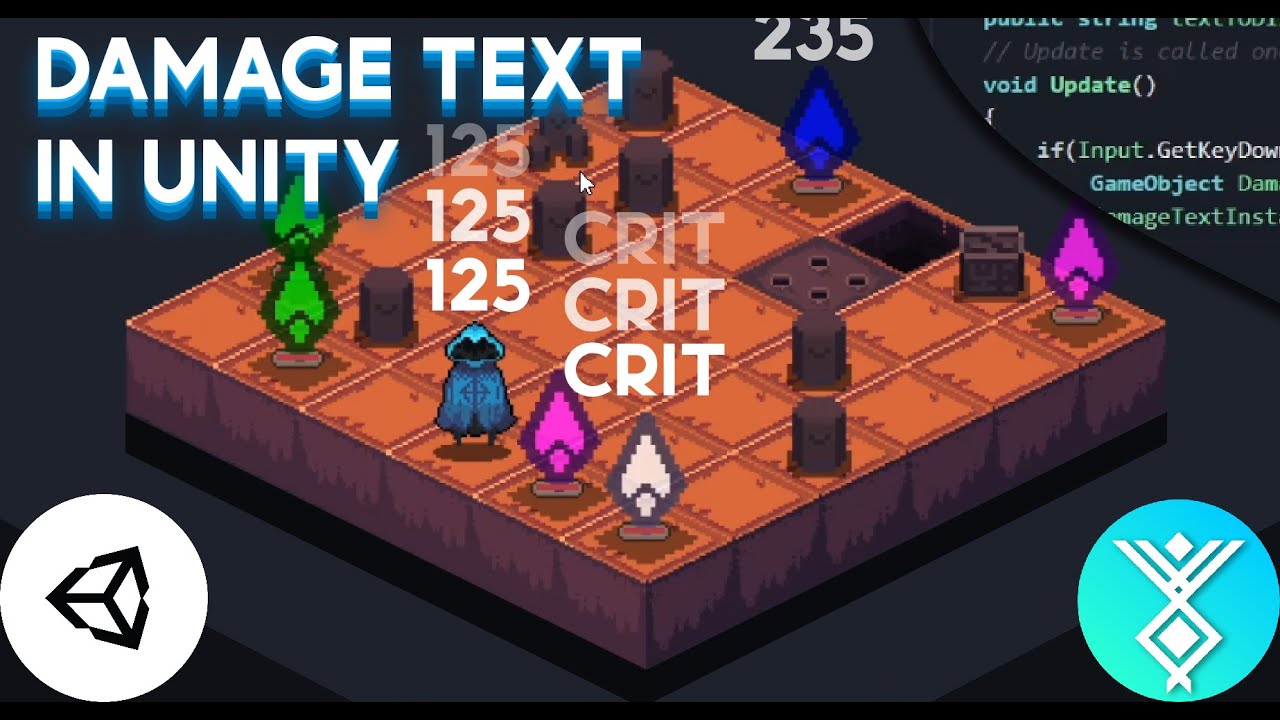
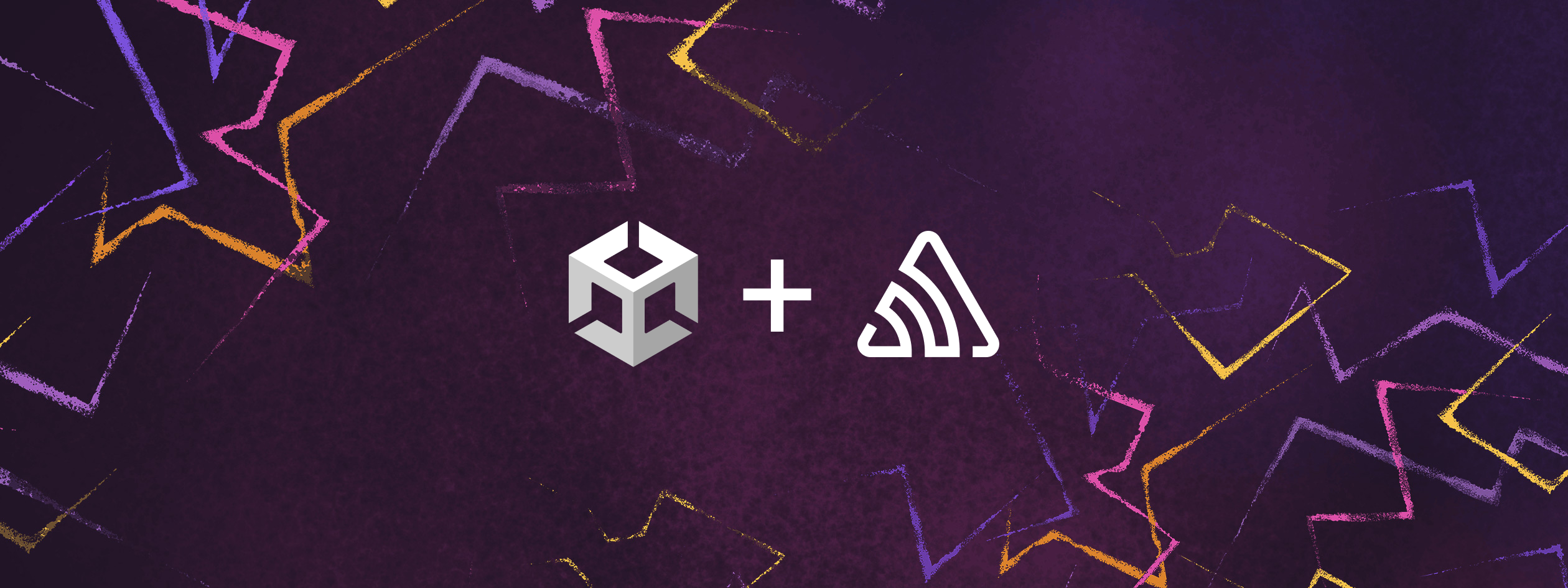

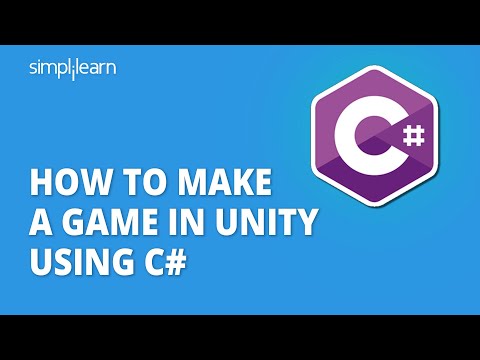
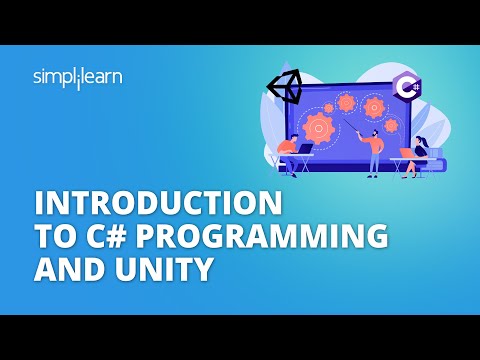

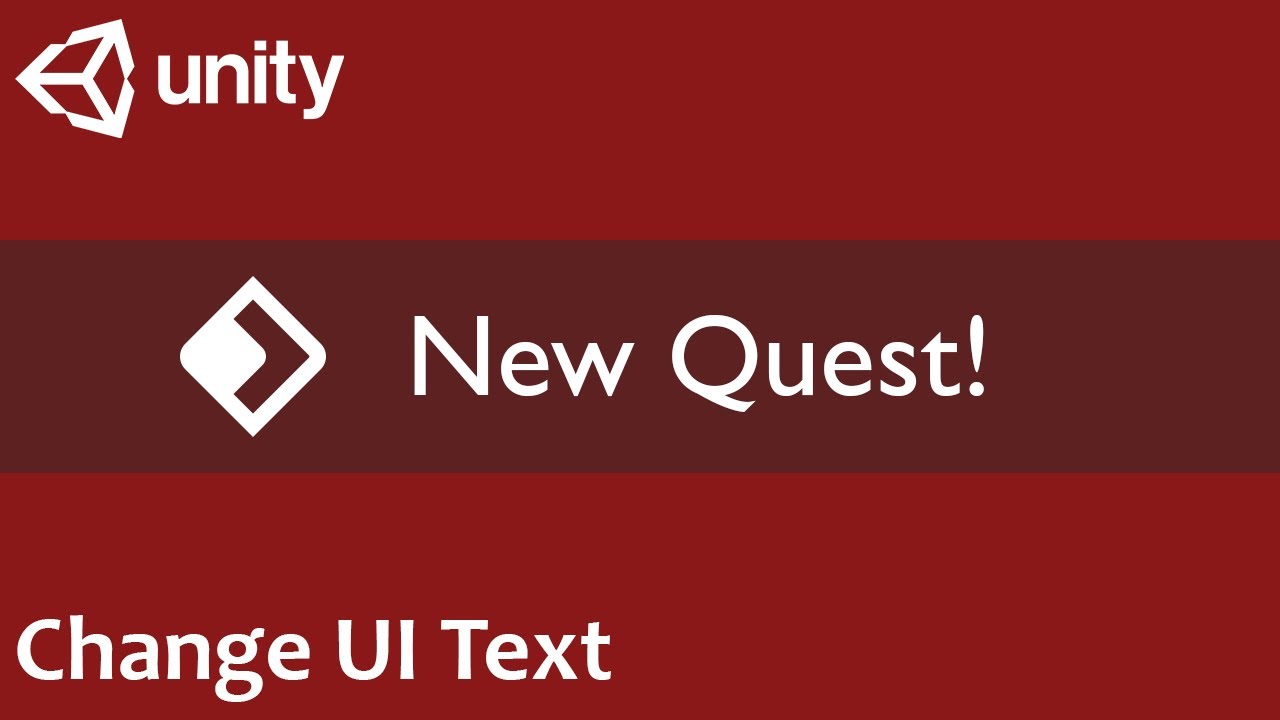
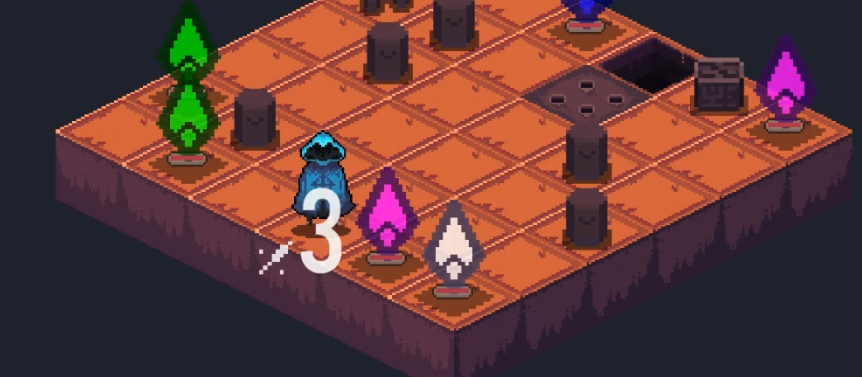

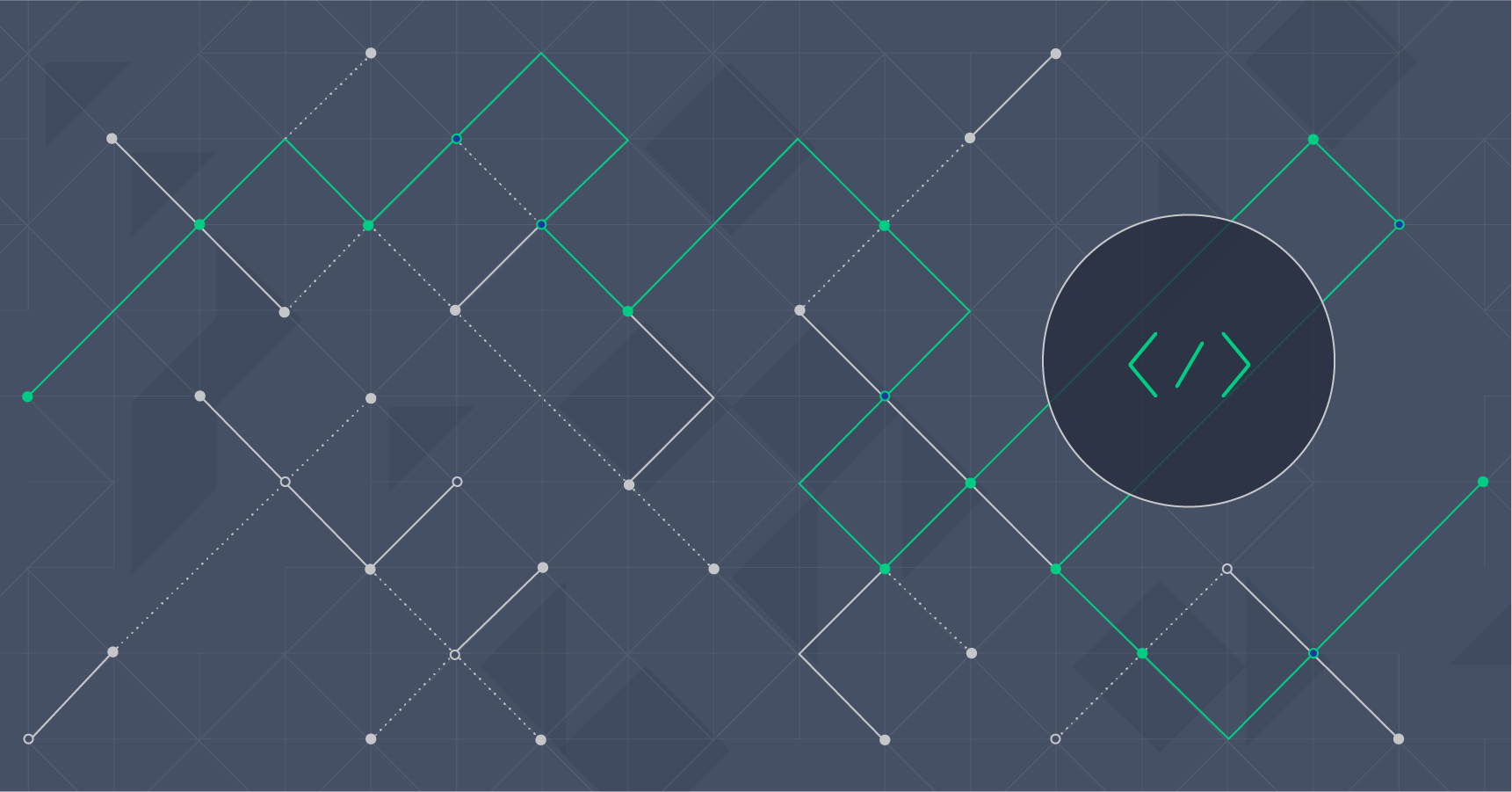
Article link: unity display text for a few seconds.
Learn more about the topic unity display text for a few seconds.
- Make text appear for 5 seconds in Unity – Stack Overflow
- { How to DISPLAY TEXT on screen in Unity } – Text Canvas Component
- Unity UI text not displayed? – Stack Overflow
- Unity – how to show text for few seconds for each good move …
- unity – How do I make Text Mesh appear five seconds later …
- Scripting API: WaitForSeconds – Unity – Manual
- Unity Tutorial: Developing Your First Unity Game – Part 2
- How to make a countdown timer in Unity (in minutes + seconds)
- Performance recommendations for Unity – Mixed Reality
See more: nhanvietluanvan.com/luat-hoc