Unexpected Lexical Declaration In Case Block
Overview
Lexical declarations refer to statements in programming that declare variables. They play a crucial role in scoping and hoisting, ensuring the proper utilization of variables throughout the code. On the other hand, case blocks are part of switch statements, providing a way to execute different sections of code based on the value of a given expression. However, unexpected lexical declarations can occur within case blocks, leading to unintended consequences and errors in code. In this article, we will delve into the issue of unexpected lexical declarations in case blocks, discuss potential problems and consequences, and provide solutions and best practices to avoid such situations.
1. Definition and Explanation of Lexical Declarations
Lexical declarations are statements in programming that declare variables. They include the var, let, and const keywords, which are used to differentiate the scoping behavior and reassignment possibilities of variables. The var keyword declares a variable globally or locally to a function, while the let and const keywords declare block-scoped variables, restricting their scope to the respective block of code. Lexical declarations also involve the concept of hoisting, where variable declarations are moved to the top of their scope, allowing them to be accessed before their actual declaration in the code.
2. Introduction to Case Blocks
Case blocks are an integral part of switch statements in programming. Switch statements are used to compare an expression’s value with multiple possible case values and execute the corresponding code block. A case block consists of a case keyword followed by a value to match, a colon, and the code to be executed if the case matches the given value. Multiple case blocks can be specified within a switch statement, providing different execution paths based on the expression’s value.
3. The Issue of Unexpected Lexical Declarations in Case Blocks
While lexical declarations generally follow scoping rules, unexpected lexical declarations can occur within case blocks. This means that variables declared within a case block can have unexpected behavior due to the nature of how switch statements work. The switch statement evaluates the given expression and executes the matching case block. However, since case blocks are not treated as separate scopes, lexical declarations within them are subject to hoisting and can be accessed outside the expected scope.
For example, consider the following code snippet:
“`javascript
switch (expression) {
case value1:
let variable = “unexpected”;
break;
default:
// code block
}
“`
In this scenario, the variable declaration `let variable = “unexpected”;` may appear to be scoped only to the case block. However, due to hoisting, the variable’s scope is extended to the entire switch statement, leading to unexpected behavior.
4. Instances Where Unexpected Lexical Declarations Can Occur
There are several instances where unexpected lexical declarations can arise in case blocks. One common scenario is when a case block contains the same variable name as another case block within the switch statement. Consider the following example:
“`javascript
switch (expression) {
case value1:
let variable = “unexpected declaration”;
break;
case value2:
let variable = “unexpected declaration”;
break;
default:
// code block
}
“`
In this case, the redeclaration of the variable within the second case block may lead to confusion and unexpected behavior, as it is meant to be a separate variable within the case block’s scope.
The placement and order of code can also affect the occurrence of unexpected lexical declarations. If a case block is enclosed within another block, such as an if statement or a loop, lexical declarations inside the outer block can also be unexpectedly accessed from the case block.
5. Potential Problems and Consequences of Unexpected Lexical Declarations
Unexpected lexical declarations in case blocks can lead to various problems and consequences. Firstly, it can result in unintended variable scopes, where variables declared within a case block are accessible outside the expected scope. This can lead to confusion and errors, as the code may produce unexpected results.
Secondly, unexpected lexical declarations can result in bugs and difficulties in code maintenance. When encountering unexpected behavior caused by these declarations, developers may spend considerable time trying to identify the source of the issue. This can impact productivity and lead to frustration. Additionally, if code is modified or expanded in the future, unexpected lexical declarations can introduce further complexities and increase the likelihood of introducing bugs.
6. Solutions and Best Practices for Avoiding Unexpected Lexical Declarations in Case Blocks
To avoid unexpected lexical declarations in case blocks, it is crucial to follow certain best practices. Firstly, it is recommended to always define variables before the switch statement. By declaring variables in the appropriate scope and order, the chances of unintended hoisting and access to declarations within case blocks can be minimized.
Additionally, proper scoping and conditional logic should be implemented when working within case blocks. It is vital to ensure that case blocks have a clear and distinct scope, enabling the expected execution of code. By carefully organizing the placement of code and considering scoping rules, unexpected lexical declarations can be avoided.
7. FAQ
Q1: Can’t access lexical declaration: What does this error mean and how does it relate to unexpected lexical declarations in case blocks?
This error occurs when a lexical declaration, such as a variable declaration, is accessed outside its scope. In the context of unexpected lexical declarations in case blocks, this error may be encountered when attempting to access a variable declared within a case block from outside that block. It highlights the issue of variable scope and emphasizes the need to define variables in the appropriate scope to avoid such errors.
Q2: What does “Unexpected if as the only statement in an else block” mean and how is it relevant to this topic?
This warning typically occurs when an if statement is the only statement within an else block. It suggests that there may be a logical inconsistency or missing code within the else block. While not directly related to unexpected lexical declarations in case blocks, it is essential to ensure the proper structure and logic within code to prevent unforeseen issues and maintain code clarity.
Q3: What are the common problems associated with switch case statements and how can they be resolved?
Common problems with switch case statements include missing break statements, leading to unexpected fall-through behavior, and the absence of a default case when none of the case values match the expression. To solve these issues, it is recommended to include break statements after each case, ensuring the desired switch case behavior, and to always include a default case to handle unexpected values.
Q4: How does the concept of hoisting relate to unexpected lexical declarations in case blocks?
Hoisting refers to the behavior of moving variable declarations to the top of their scope. In the case of unexpected lexical declarations, hoisting can cause the variables declared within case blocks to be accessible outside the expected scope. It is important to be aware of hoisting and its implications to avoid any unintended or confusing behavior in the code.
Q5: Do all programming languages exhibit the issue of unexpected lexical declarations in case blocks?
No, the issue of unexpected lexical declarations in case blocks is specific to certain programming languages. For example, JavaScript exhibits this issue due to its scoping and hoisting rules. Other languages may have different scoping behaviors and rules governing lexical declarations, leading to distinct behaviors in similar programming constructs.
Conclusion
Unexpected lexical declarations in case blocks can result in confusion, errors, and difficulties in code maintenance. By understanding the issue at hand, identifying common scenarios, and implementing proper solutions and best practices, developers can minimize the occurrence of unexpected lexical declarations. By adhering to defined scoping rules, arranging code placement thoughtfully, and following good programming practices, the risk of encountering unexpected lexical declarations in case blocks can be significantly reduced.
Javascript Fundamentals Bangla Tutorial (Switch Case) -Part-14
What Is A Lexical Declaration?
In the world of programming, lexical declarations play a crucial role in defining variables and constants. They are an essential part of any programming language as they allow developers to assign names to values or data structures. A lexical declaration, sometimes referred to as a lexical binding, helps programmers organize and manage their code effectively.
Lexical declarations are primarily used to declare variables and constants within a particular scope. A scope refers to the part of a program where a variable or constant is accessible. Languages like JavaScript, Python, and C++ use lexical declarations extensively to define and initialize variables or constants.
Understanding Lexical Scopes:
Before diving deeper into lexical declarations, it is essential to understand lexical scopes. A lexical scope determines the accessibility and visibility of a variable or constant within a program. It is based on the physical structure of the program and the way blocks and functions are nested within each other.
Lexical scopes are nested within each other, forming a hierarchical structure. Each nested scope has access to variables and constants defined in outer scopes but not vice versa. This methodology ensures that the variables or constants used in a lower scope are independent of the ones in an outer scope, preventing conflicts and making it easier to comprehend the overall code.
Declaring Variables:
Lexical declarations are widely used to declare variables. A variable is a named storage that can hold any data value within a program. Conventionally, variables have a specific name assigned to them, making it easier to refer to a particular stored value when needed.
When declaring variables using lexical declarations, developers usually specify the variable’s name and optionally assign an initial value. For example, in JavaScript, the lexical declaration to create a variable ‘age’ with an initial value of 25 would look like this:
let age = 25;
This declaration creates a variable named ‘age’ with a value of 25 and makes it accessible within the scope it was declared in. The ‘let’ keyword is used to create block-scoped variables in JavaScript.
Defining Constants:
Similarly, lexical declarations are useful for defining constants. A constant is a variable whose value cannot be modified after it is initialized. Constants are typically used for values that shouldn’t be altered during program execution, such as mathematical or physical constants.
In JavaScript, the ‘const’ keyword is used to create constants. For instance:
const pi = 3.14159;
This declaration creates a constant named ‘pi’ with a value of 3.14159. It guarantees that the value of ‘pi’ cannot be changed throughout the program’s execution.
Lexical Declarations and Hoisting:
Hoisting is a behavior in programming languages where declarations, either variables or functions, are moved to the top of the current scope before execution. It allows variable and function references to be made before the actual declaration is encountered in the code.
However, unlike variables declared using the ‘var’ keyword, lexical declarations using ‘let’ or ‘const’ are not hoisted. This means that they are only accessible within the section of code where they are defined. It is advised to declare lexical variables at the beginning of their respective scope to avoid any confusion or errors.
FAQs:
Q: Are lexical declarations limited to specific programming languages?
A: No, lexical declarations are a commonly used concept in many programming languages, including JavaScript, Python, C++, and many others. However, the syntax and keywords may vary depending on the language.
Q: Can I change the value of a constant declared using a lexical declaration?
A: No, once a constant is defined using a lexical declaration, its value cannot be modified. Attempting to do so will result in an error.
Q: What happens if I try to access a variable declared using a lexical declaration outside its scope?
A: Variables declared using a lexical declaration are only accessible within the scope where they were defined. Attempting to access them outside their scope will result in an error.
Q: Are lexical declarations necessary? Can’t I simply assign values without declaring them?
A: Lexical declarations provide structure and clarity to a program by assigning specific names to values. They improve code readability and maintainability, making it easier for developers to understand and debug their code. Hence, it is highly recommended to use lexical declarations for better programming practices.
Q: Can lexical declarations be used in conditional statements or loops?
A: Yes, lexical declarations can be used within conditional statements and loops. However, it is essential to ensure that the declarations are made in the appropriate scope to avoid any unintended consequences or errors.
In conclusion, lexical declarations are crucial in programming as they define variables and constants while also establishing their scope and accessibility. By assigning names to values, they enhance code organization and maintainability. Understanding lexical scopes and using lexical declarations appropriately can greatly benefit developers, enabling them to write more efficient and error-free code.
Keywords searched by users: unexpected lexical declaration in case block Can t access lexical declaration, Unexpected if as the only statement in an else block, Javascript switch case code block, Expected default case, Switch case js return, Empty block statement, Expected a break statement before case, Unexpected constant condition
Categories: Top 44 Unexpected Lexical Declaration In Case Block
See more here: nhanvietluanvan.com
Can T Access Lexical Declaration
In the world of programming and computer science, lexical scope plays a crucial role in defining the accessibility and visibility of variables within a given code block. Similarly, in the English language, lexical scope is also a fundamental concept when it comes to accessing and understanding lexical declarations. However, there are instances where access to these lexical declarations may be restricted or limited. In this article, we will explore the reasons behind this limitation and provide a comprehensive understanding of why certain lexical declarations in English may not be accessible.
What are Lexical Declarations?
Before delving into the limitations, let’s first understand what lexical declarations are. In English, lexical declarations refer to the act of defining or declaring words or phrases within a specific context or block of text. Just like in programming, where variables within a particular scope can only be accessed within that scope, lexical declarations function similarly. They help in defining the scope and providing clarity in communication. However, there are certain scenarios where these declarations cannot be accessed, which we will explore now.
1. Contextual Constraints:
One of the primary reasons for the inability to access lexical declarations is the contextual constraints imposed by the surrounding language and grammar rules. English, like any other language, has its own set of rules and guidelines that dictate the use and accessibility of lexical declarations. For instance, certain words or phrases may be restricted to specific contexts or domains. Trying to access these declarations outside their designated areas may result in confusion or misinterpretation.
2. Cultural Variation:
Language is deeply intertwined with culture, and as such, certain lexical declarations may be exclusive to a specific cultural context. For example, idiomatic expressions or colloquialisms are often deeply rooted within a particular culture. These expressions can prove puzzling or incomprehensible to individuals from other cultural backgrounds. Thus, cultural variation can limit access to lexical declarations, as they may not be understood or appreciated by everyone.
3. Expertise and Specialization:
Certain lexical declarations may be tied to specific areas of expertise or subjects of specialization. Technical jargon or scientific terminology, for instance, requires a certain level of understanding and knowledge to access and utilize effectively. If an individual lacks the necessary expertise in a particular field, they may struggle to comprehend or access the associated lexical declarations. This limitation highlights the importance of acquiring domain-specific knowledge to enhance communication and understanding.
4. Semantic Ambiguity:
Another factor that hinders access to lexical declarations is the presence of semantic ambiguity. English, like many other languages, contains words and phrases that can have multiple interpretations or meanings. In such cases, the intended lexical declaration may not be easily accessible, as different interpretations may arise based on the context or the individuals involved. This ambiguity can hinder effective communication and limit access to lexical declarations that have diverse interpretations.
FAQs:
Q: How can I overcome the limitations of accessing lexical declarations?
A: To overcome these limitations, it is essential to continuously enhance your language skills and cultural awareness. By familiarizing yourself with different cultural contexts, obtaining subject-specific knowledge, and clarifying any ambiguities, you can improve your access to lexical declarations.
Q: Are there any tools or resources available to help access restricted lexical declarations?
A: Yes, there are various resources available to enhance access to restricted lexical declarations. Language dictionaries, cultural guidebooks, and online communities that facilitate discussions on specific domains are all valuable resources that can aid in overcoming limitations.
Q: Can reliance on contextual cues compensate for the inability to access certain lexical declarations?
A: Yes, in some cases, relying on contextual cues can help compensate for the inability to access specific lexical declarations. Understanding the wider context, including the preceding and subsequent statements, can provide insights into the intended meaning and help overcome limitations.
Q: Are there any restrictions on accessing lexical declarations in programming languages?
A: Yes, programming languages also have their own set of rules and limitations when it comes to accessing lexical declarations. These limitations are often built into the language’s syntax and scope rules to ensure code integrity and efficiency.
Conclusion
Accessing lexical declarations in English may come with its fair share of limitations. Cultural constraints, contextual restrictions, semantic ambiguity, and specialization are all factors that can hinder the accessibility of these declarations. Overcoming these limitations requires a continuous effort to enhance language proficiency, acquire domain-specific knowledge, and familiarity with cultural nuances. By understanding the limitations and actively working to overcome them, individuals can enhance their linguistic skills and unlock a broader range of lexical declarations in English.
Unexpected If As The Only Statement In An Else Block
When we encounter an “else” block with an “if” statement as the sole statement inside it, we often refer to it as an “else if” statement. Historically, this construct has been widely used to handle multiple conditions that depend on each other. It allows for a chain of conditional statements, where each “else if” block is evaluated if the preceding conditions are false. Ultimately, if none of the conditions are met, the “else” block will be executed as a catch-all for any remaining cases.
However, when we encounter a situation where the “else” block contains only an “if” statement, without any accompanying alternative code, the behavior can be quite different from what we might expect. In most programming languages, this construct is valid and executes without any syntax errors. Nevertheless, the outcome may not match our initial assumptions.
To better understand this concept, let’s consider an example in a widely used programming language like Python:
“`python
if condition_1:
# Code block executed when condition_1 is true
else:
if condition_2:
# Code block executed when condition_1 is false and condition_2 is true
else:
# Code block executed when both condition_1 and condition_2 are false
“`
Here, if `condition_1` is true, the code within the first block will be executed, as expected. However, if `condition_1` is false, the program will proceed to evaluate `condition_2`. If `condition_2` is true, the corresponding code block will be executed. But here comes the unexpected part: if `condition_2` is false, the program will continue to the next line of code outside of the “if” statement altogether, skipping the “else” block entirely.
This behavior can be counterintuitive, especially if we are accustomed to the traditional use of the “else” block. It is important to recognize this peculiar aspect when using an “if” statement as the sole statement in an “else” block. Consequently, we should exercise caution to ensure that the desired behavior is achieved.
FAQs:
Q: What is the purpose of using an “if” statement as the only statement in an “else” block?
A: The main purpose is to introduce additional conditions that rely on the result of preceding conditions. It allows for a series of linked conditional statements, where each subsequent condition is evaluated only if the previous ones fail.
Q: Are there any programming languages that exhibit different behavior in this scenario?
A: The behavior may vary slightly between programming languages. However, the conventional behavior discussed in this article is prevalent in many popular programming languages, including Python, JavaScript, C++, and Java.
Q: Can this construct lead to programming errors?
A: Yes, it can. Using an “if” statement as the only statement in an “else” block can lead to unintended consequences. If the code within the “if” statement doesn’t alter any variables or affect the program flow, it can result in an incomplete or incorrect execution path.
Q: How can I avoid unexpected behavior when using this construct?
A: To avoid unexpected behavior, it is advisable to explicitly define the desired outcome. In cases where the “else” block should always execute, it is better to use an empty code block or an explicit comment to convey the intended purpose.
In conclusion, encountering an “if” statement as the sole statement within an “else” block can yield unexpected results if we are not aware of this particular behavior. It is crucial to comprehend the implications and ensure that the desired logic and flow of the program align with this construct. By understanding the potential pitfalls and approaching it with caution, programmers can avoid unintended consequences when utilizing this construct in their code.
Images related to the topic unexpected lexical declaration in case block
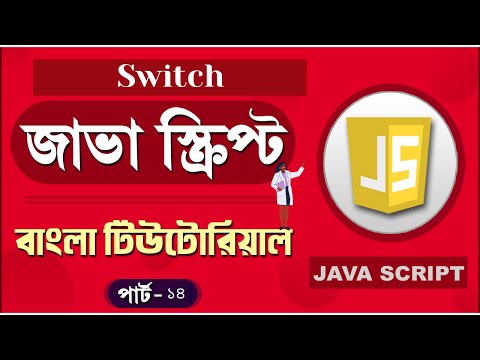
Found 5 images related to unexpected lexical declaration in case block theme
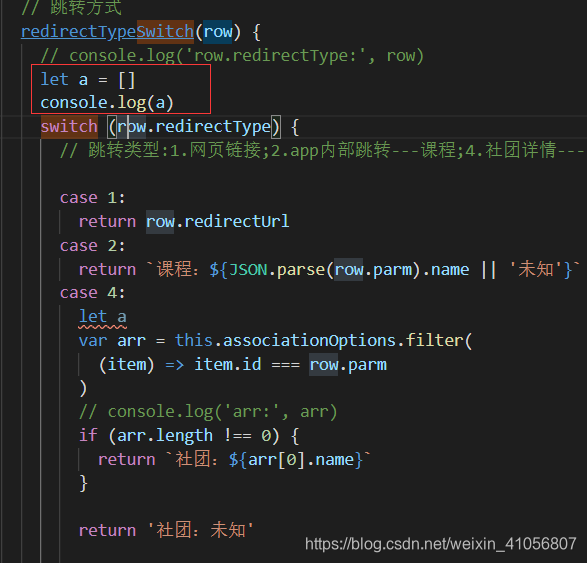
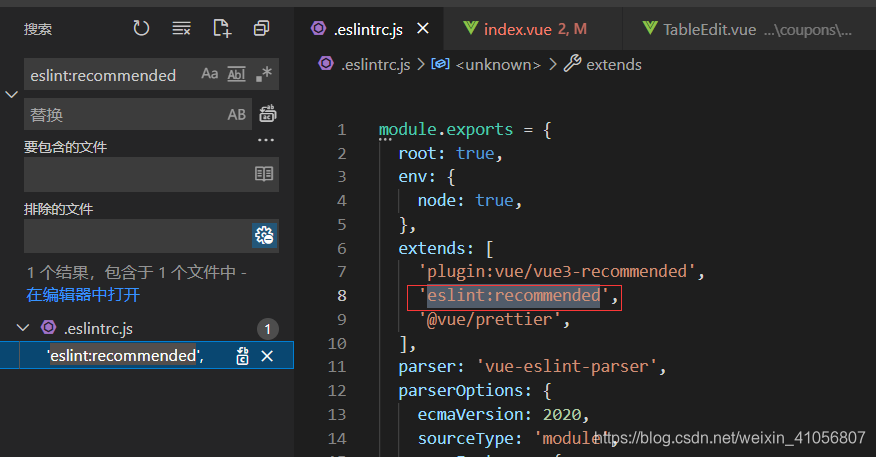
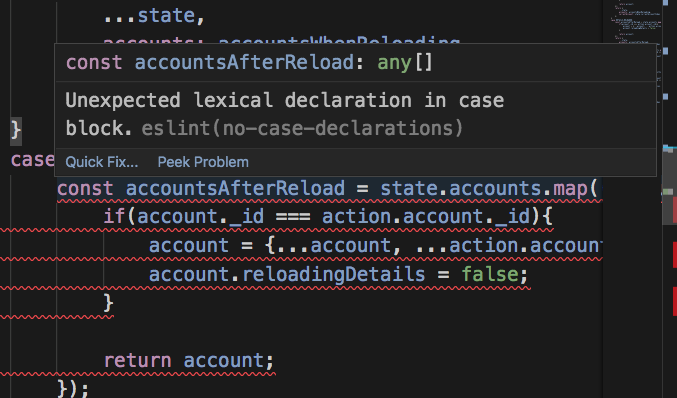

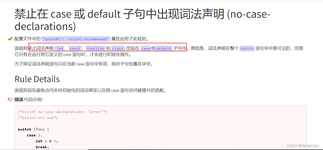
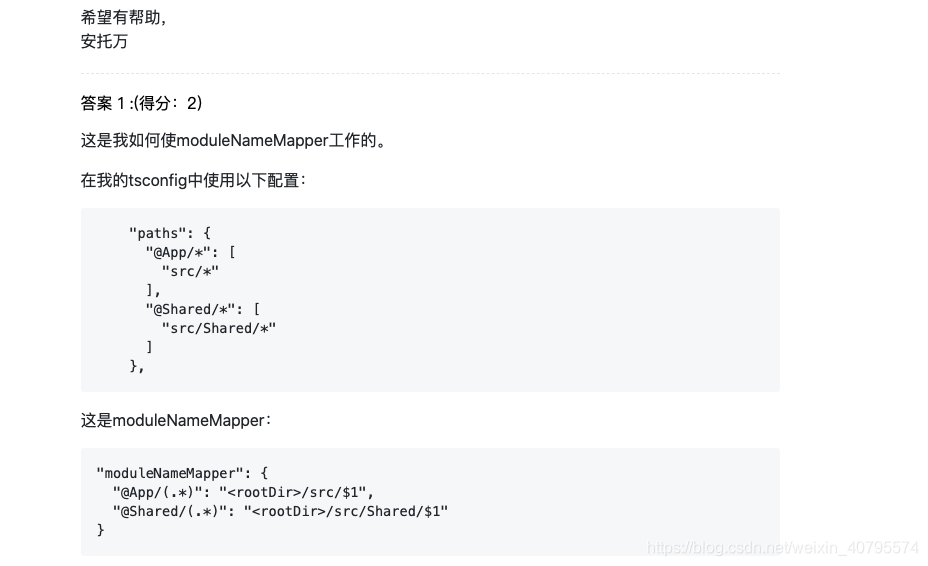
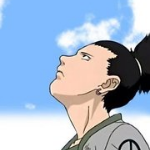
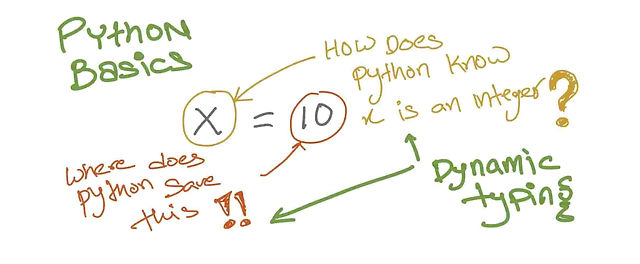
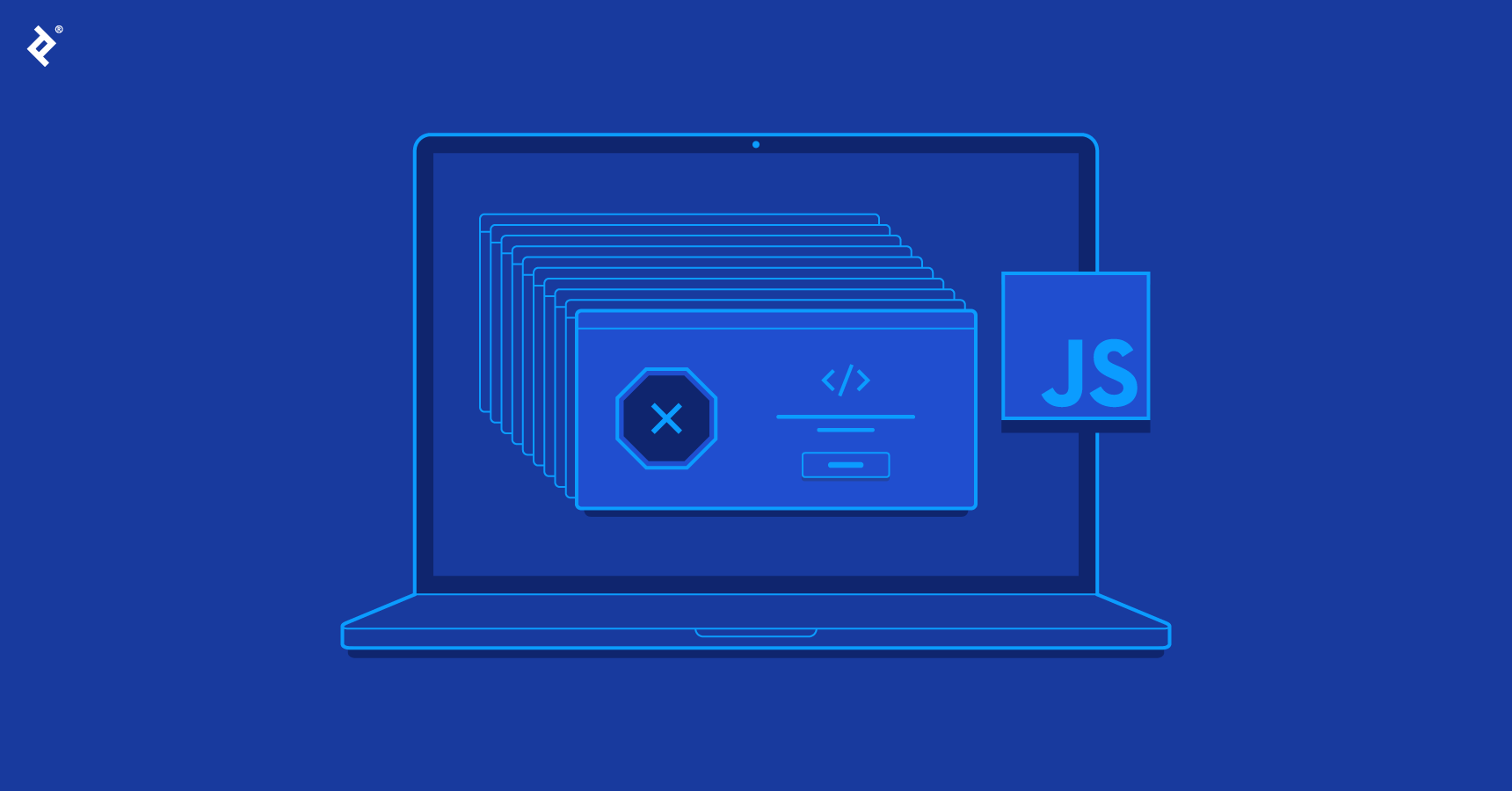
Article link: unexpected lexical declaration in case block.
Learn more about the topic unexpected lexical declaration in case block.
- eslint – unexpected lexical declaration in case block
- ESLint: Unexpected lexical declaration in case block [Fixed]
- no-case-declarations – ESLint – Pluggable JavaScript Linter
- No-case-declarations – ESLint – W3cubDocs
- unexpected lexical declaration in case block-Reactjs
- No lexical declarations inside CaseClause StatementList #4278
- Lexical Declarations (ES6) Sample
- Rule no-case-declarations – ESLint中文
- switch文 / Unexpected lexical declaration in case block. – Abillyz
- Unexpected lexical declaration in case block.eslint no-case …
See more: nhanvietluanvan.com/luat-hoc