Typeerror: ‘Type’ Object Is Not Subscriptable
Python is a versatile programming language that allows for the creation and manipulation of objects. Objects are the fundamental building blocks of Python programs, representing real-world entities or abstract concepts. Each object belongs to a specific type, which defines the set of operations that can be performed on it. However, sometimes developers may encounter an error message that says “TypeError: ‘type’ object is not subscriptable.” In this article, we will explore the concept of objects and types in Python, understand subscripting, identify common causes of this error, and learn how to resolve it through correct syntax usage.
Understanding the concept of objects and types in Python
In Python, every value is an object, and every object has a type. The type of an object determines its behavior and the set of operations that can be performed on it. The built-in types in Python include integers, floating-point numbers, strings, lists, dictionaries, and more. Additionally, developers can define their own custom types by creating classes.
The concept of subscripting in Python
Subscripting is the act of accessing a specific element or a range of elements in an iterable object such as a list, tuple, or string. The square brackets notation is used for subscripting, where the index or indices of the desired elements are provided inside the brackets. For example, to access the first element of a list, we use myList[0].
Common causes of the ‘type’ object is not subscriptable error
The “TypeError: ‘type’ object is not subscriptable” error occurs when you try to access an element of an object that does not support subscripting. The most common cause of this error is mistakenly applying the square brackets notation to a type object instead of an instance of that type. For example, consider the following code snippet:
myList = list
element = myList[0]
In this case, the variable myList refers to the built-in list type, not an actual list object. Hence, trying to access an element using the subscript operator raises the TypeError.
How to identify and debug the ‘type’ object is not subscriptable error
To identify the “TypeError: ‘type’ object is not subscriptable” error, carefully examine the code that triggers the error message. Look for instances where subscripting is performed on a type object rather than an instance of that type. Once identified, you can begin debugging the error by modifying the code to ensure that subscripting operations are applied to appropriate objects.
Resolving the ‘type’ object is not subscriptable error through correct syntax usage
To resolve the “TypeError: ‘type’ object is not subscriptable” error, it is important to use the correct syntax when performing subscripting operations. Ensure that you are applying the square brackets notation to an instance of a type that supports subscripting, such as a list or a string. For example, modifying the previous code snippet to create an instance of the list type:
myList = list()
element = myList[0]
By creating an instance of the list using parentheses, we can now subscript the list object without raising the TypeError.
Handling the ‘type’ object is not subscriptable error in specific scenarios
In some cases, the “TypeError: ‘type’ object is not subscriptable” error may arise due to specific scenarios. For instance, this error can occur when attempting to access elements of a type hint or an instance method. In such situations, it is crucial to understand the structure and behavior of the objects involved. Consult the documentation or seek guidance from the relevant Python resources to learn the correct method of accessing the desired elements.
Best practices to prevent the ‘type’ object is not subscriptable error
To prevent the “TypeError: ‘type’ object is not subscriptable” error, follow these best practices:
1. Double-check the usage of square brackets: Ensure that the square brackets notation is being applied to objects that support subscripting, such as lists, strings, or tuples.
2. Verify the object type: Make sure that the object you are subscripting is an instance of a type that supports subscripting. Avoid mistakenly applying subscripting operations to type objects.
Alternative solutions to the ‘type’ object is not subscriptable error
If you encounter the “TypeError: ‘type’ object is not subscriptable” error and modifying the code to use the correct syntax is not feasible or desirable, alternative solutions can be explored. These alternatives may involve different approaches to achieve the desired outcome or utilizing different data structures or methods that support subscripting or a similar form of element access.
FAQs
1. What does the error message ‘TypeError: ‘type’ object is not subscriptable’ mean?
The error message indicates that you are trying to apply the subscript operator to a type object, which does not support subscripting. Only instances of types that support subscripting, such as lists or strings, can be subscripted.
2. How can I fix the ‘type’ object is not subscriptable error?
To fix the error, ensure that you are applying the subscript operator to an instance of a type that supports subscripting, rather than a type object itself. Create an instance of the desired type using parentheses if necessary.
3. Why does the ‘type’ object is not subscriptable error occur?
The error occurs when you mistakenly apply the subscript operator to a type object instead of an instance of that type. This can happen if you forget to create an instance of the type or inadvertently use the type object itself.
4. Can this error occur in specific scenarios other than types?
Yes, the error can occur when trying to access elements of a type hint or an instance method. In such cases, consult the relevant documentation or resources to understand the correct method of accessing the desired elements.
In conclusion, the “TypeError: ‘type’ object is not subscriptable” error can be resolved by ensuring the correct usage of subscripting syntax and applying it to instances of types that support subscripting. By understanding the underlying concepts of objects, types, and subscripting in Python, developers can successfully navigate and debug such errors.
How To Fix Object Is Not Subscriptable In Python
What Does Type Object Is Not Subscriptable Mean?
Python is a versatile programming language that is known for its simplicity and readability. However, like any programming language, Python can sometimes throw cryptic error messages that may leave beginners scratching their heads. One such error message is “TypeError: ‘type’ object is not subscriptable”. In this article, we will dive into this error and explore its meaning, common causes, and possible solutions.
Understanding the Error Message
Before we delve into the specifics, it is essential to understand the terms used in the error message. In Python, objects are instances of classes. Every object belongs to a specific type, which is defined by its class. The concept of subscriptability refers to the ability to access elements within objects using square brackets, like a list or a dictionary.
In programming, accessing elements within an object is achieved using indexing or slicing. Indexing involves specifying the position of the desired element, while slicing allows the extraction of a subset of elements. However, the error message “TypeError: ‘type’ object is not subscriptable” suggests that we are trying to apply subscript operator ([]) on an object whose type does not support it.
Common Causes
Now that we understand the error message, let’s discuss some common causes that can lead to this error:
1. Confusing Class and Object: The most common cause of this error is mistakenly using the class instead of the object itself. For example, if you have defined a class named “Car” and try to apply indexing directly on the class, you will receive the “type object is not subscriptable” error.
2. Forgetting to Instantiate the Class: In Python, classes act as blueprints for creating objects. If you forget to create an object from a class and use indexing on the class directly, you will encounter this error.
3. Overriding Special Methods: Special methods, such as “__getitem__”, allow objects to be subscriptable. If you override these methods incorrectly or forget to include them in your custom class, the error will arise.
4. Improper Initialization: If the __init__ method of a class does not define the necessary attributes, attempting to use indexing on the object will result in the aforementioned error.
Possible Solutions
Now that we have identified some common causes of the “type object is not subscriptable” error, let’s explore potential solutions:
1. Check for Proper Instantiation: Ensure that you have instantiated the class properly and are applying the subscript operator to an object, not the class itself. Double-check that you are using parentheses after the class name to create an instance.
2. Verify Class Definition: Make sure you have defined the necessary special methods, such as “__getitem__”, in your class. These methods allow instances of the class to be subscriptable.
3. Review __init__ Method: Check the __init__ method of your class and confirm that it properly initializes all the required attributes. If the necessary attributes are missing, subscripting will not work as expected.
4. Debug with Print Statements: Insert print statements in your code to debug where the error is occurring. By isolating specific lines of code and observing the output, you can identify the source of the error and take appropriate action.
FAQs
Q: Can this error occur with built-in Python data types?
A: No, this error typically occurs when attempting to apply subscripting on custom classes or objects.
Q: Can the “type object is not subscriptable” error occur with dictionaries or lists?
A: No, dictionaries and lists are by default subscriptable in Python, so this error is not applicable to these built-in data types.
Q: How can I check if an object is subscriptable before applying subscripting?
A: You can use the “isinstance()” function to determine if an object is subscriptable. For example:
“`
if isinstance(obj, list) or isinstance(obj, dict):
# The object is subscriptable
else:
# The object is not subscriptable
“`
In conclusion, the “type object is not subscriptable” error in Python occurs when trying to apply subscripting on an object that does not support it. Understanding the error message and identifying the common causes can help you troubleshoot and resolve this issue efficiently. By double-checking your code for proper instantiation, class definition, and initialization, you can avoid this error and ensure the smooth execution of your Python programs.
What Is Python Subscriptable?
Python is a versatile and dynamic programming language that allows developers to solve complex problems with ease. One of the key features of Python is its ability to handle data structures, such as lists, tuples, and dictionaries, in a flexible and efficient manner. In Python, the term “subscriptable” refers to an object’s capability to be accessed using square brackets, just like indexing.
In simpler terms, subscriptable objects in Python can be thought of as containers that hold other elements, and these individual elements can be accessed by using an index or a key. This powerful feature allows programmers to manipulate and retrieve specific data from various data structures, making Python an efficient language for working with large amounts of information.
Understanding Subscriptable Objects in Python
To better understand what Python subscriptable objects are, let’s explore a few common data structures and their subscriptable properties:
1. Lists:
Lists are ordered collections of items enclosed in square brackets and separated by commas. Each item in a list can be accessed using its index. For example, if we have a list named “fruits” containing [‘apple’, ‘banana’, ‘orange’], we can access the first item, ‘apple’, by using the index fruits[0].
2. Tuples:
Tuples are similar to lists but are immutable, meaning they cannot be modified once created. Like lists, tuples are also subscriptable and can be accessed using indexes. For example, a tuple named “coordinates” containing (4, 5) can be accessed using the index coordinates[0], returning the value 4.
3. Dictionaries:
Dictionaries are unordered collections of key-value pairs enclosed in curly braces {}. Each value in a dictionary is associated with a unique key, and these values can be accessed using the respective keys. For instance, if we have a dictionary named “student” with the key-value pairs {‘name’: ‘John’, ‘age’: 20}, we can retrieve John’s age by using the key student[‘age’].
4. Strings:
Although strings in Python are not technically containers, they are subscriptable. Each character in a string can be accessed using its index, similar to lists and tuples. For example, if we have a string named “message” containing “Hello”, we can access the first character, ‘H’, using the index message[0].
Working with Subscriptable Objects
Python offers several methods and techniques to work with subscriptable objects efficiently. Here are some commonly used ones:
1. Indexing:
Indexing is the process of accessing or retrieving a specific element from subscriptable objects. By providing the appropriate index or key, we can retrieve the desired value. However, it is important to note that indexing in Python starts from 0, meaning the first element of a list or a tuple has an index of 0.
2. Slicing:
Slicing allows us to extract a portion of a subscriptable object by specifying a range of indexes. This is particularly useful when we need to extract a subset of data from a large data structure. For example, if we have a list named “numbers” containing [1, 2, 3, 4, 5], we can extract the sub-list [2, 3, 4] by using the slicing syntax numbers[1:4].
3. Iteration:
Subscriptable objects can be easily iterated over using loops, such as for loops or list comprehensions. This allows us to perform operations on each element of the object individually. For example, if we have a list named “prices” containing [10, 20, 30], we can calculate the total sum of all prices by iterating through the list using a for loop.
4. Modifying Values:
Subscriptable objects in Python can also be modified using assignment. By providing the appropriate index or key, we can update the value of an element. For instance, if we have a list named “grades” containing [90, 85, 95], we can update the second grade to 80 by using the assignment statement grades[1] = 80.
FAQs:
Q: Can all objects in Python be subscriptable?
A: No, not all objects in Python are subscriptable. Only certain objects, such as lists, tuples, dictionaries, and strings, can be accessed using square brackets.
Q: Can subscriptable objects contain different data types?
A: Yes, subscriptable objects can contain elements of different data types. For example, a list can contain a mix of integers, strings, and even other lists.
Q: What happens if I try to access an invalid index or key?
A: If an invalid index or key is used to access a subscriptable object, Python will raise an IndexError or KeyError respectively. It is important to ensure that the index or key is within the valid range.
Q: Can I add or remove elements from subscriptable objects?
A: Yes, subscriptable objects can be modified by adding or removing elements. Lists and dictionaries offer specific methods that allow for adding and removing elements dynamically.
Q: Are there any performance considerations when working with subscriptable objects?
A: While subscriptable objects in Python provide powerful functionality, it is important to note that accessing elements using indexes or keys can have different time complexities. Lists and tuples have constant time complexity O(1), while dictionaries have average constant time complexity O(1) for retrieving elements.
In conclusion, Python subscriptable objects are key features that enable efficient data manipulation and retrieval from various data structures. By using square brackets and providing appropriate indexes or keys, we can access specific elements within lists, tuples, dictionaries, and even strings. Understanding how to work with subscriptable objects allows programmers to write more concise and effective code in Python.
Keywords searched by users: typeerror: ‘type’ object is not subscriptable Object is not subscriptable, ‘int’ object is not subscriptable, Error int object is not subscriptable, Type object is not subscriptable python type hint, Object is not subscriptable Python class, TypeError type object is not iterable, Object is not subscriptable FastAPI, Method’ object is not subscriptable
Categories: Top 23 Typeerror: ‘Type’ Object Is Not Subscriptable
See more here: nhanvietluanvan.com
Object Is Not Subscriptable
When it comes to programming in Python, encountering errors is an inevitable part of the learning process. One error message that often confounds beginners and even experienced programmers alike is the “object is not subscriptable” error. This error typically occurs when you try to access an item or element within an object that does not support indexing.
To delve deeper into this error and gain a comprehensive understanding, let’s break it down and explore its causes, common scenarios, and potential solutions.
Understanding the error message
The error message “object is not subscriptable” pertains to an attempt to use square brackets [] to access an element within an object that cannot be indexed. To better grasp this concept, it is important to understand the concept of subscripting and how it applies to Python.
In Python, subscripting allows you to access individual elements within iterable objects such as lists, tuples, and strings. For example, if you have a list `my_list = [1, 2, 3]`, accessing the first element can be achieved by using `my_list[0]`. Similarly, if you have a string `my_string = “Hello”`, you can access the first character by writing `my_string[0]`.
However, not all objects in Python support subscripting. Built-in objects like integers, floats, and booleans are not iterable and cannot be indexed. When attempting to access elements within these objects using square brackets, the “object is not subscriptable” error is raised.
Causes of the error
1. Using the square bracket notation on objects that do not support indexing: As mentioned earlier, attempting to subscript an object that is not iterable will trigger the error. This commonly occurs when trying to access values within numeric or boolean objects.
2. Accessing non-existent or out-of-range indices: When trying to access an out-of-range index of an iterable object, such as a list, the error will be thrown. Python uses zero-based indexing, meaning the first element is at index 0. Trying to access an element beyond the object’s length or a negative index will result in the “object is not subscriptable” error.
Solutions and best practices
To resolve the “object is not subscriptable” error, consider the following solutions and best practices:
1. Verify object type: Before attempting to subscript an object, ensure that it is actually iterable. Check the object’s documentation or use the `type()` function to confirm its type. If the object is not iterable, you will need to find an alternative approach to access its values.
2. Check indexing: Double-check your indexing syntax to ensure that the index you are trying to access falls within the valid range of the object’s elements. Remember that Python uses zero-based indexing, so the first element is at index 0.
3. Utilize the appropriate methods: If the object does not support subscripting but provides alternative methods to access its elements, make use of those methods. For example, dictionaries do not support indexing but offer the `get()` method to retrieve values based on keys.
4. Consider object conversions: If you still need to access specific elements within an object that does not support subscripting, consider converting it to a different iterable object that can be indexed. For instance, you can convert a string to a list using the `list()` function and then access its elements via indexing.
FAQs
Q1: What are some common scenarios where the “object is not subscriptable” error is encountered?
A1: This error is often encountered when attempting to subscript non-iterable objects like integers, floats, or booleans, or when accessing indices beyond the valid range of an iterable object.
Q2: How can I determine if an object is subscriptable in Python?
A2: You can check an object’s type using the `type()` function. If the type is one of the built-in iterable types like list, tuple, or string, it can be subscripted.
Q3: Can this error occur with custom objects or user-defined classes?
A3: Yes, this error can also occur with custom objects or classes. If these objects or classes do not implement the iterable protocol or do not define a meaningful way to access elements, attempting to subscript them will raise this error.
In conclusion, the “object is not subscriptable” error in Python is encountered when attempting to access elements within an object that does not support indexing. By understanding its causes and implementing the suggested solutions, you can effectively troubleshoot and overcome this error. Remember to always confirm an object’s type and check indexing syntax to ensure smooth programming experiences in Python.
‘Int’ Object Is Not Subscriptable
When working with Python, you might sometimes come across an error message that says “‘int’ object is not subscriptable”. This error can be a bit confusing, especially for novice developers. In this article, we will delve into the reasons behind this error, understand what it means, and explore ways to resolve it.
Understanding the Error Message
To grasp the problem, let’s first understand what the error message means. In Python, an object that is “subscriptable” means it supports indexing, allowing you to extract a specific element from it. The ‘int’ object, however, does not support this operation.
In Python, ‘int’ is a built-in type representing integer values. Since integers are atomic, they cannot be broken down into smaller components that can be accessed through indexing. Therefore, attempting to use square brackets [] to access a particular element of an integer will result in the mentioned error.
Common Causes of the Error
Now that we understand the error, let’s explore some common scenarios that can trigger it:
1. Attempting to index an integer: One of the most common mistakes is trying to access an index position in an integer. For example:
“`python
number = 42
print(number[0])
“`
In this case, the code will raise the “‘int’ object is not subscriptable” error, as we are trying to access the first element of the integer variable ‘number’.
2. Incorrect use of indices in other types: Sometimes, the error may not be directly related to integers but can occur due to incorrect indexing in other data types, such as lists or strings. If you mistakenly attempt to access an integer element in a list or string, it can lead to the same error message. For example:
“`python
my_list = [1, 2, 3, 4]
print(my_list[0][0])
“`
In this case, the error is raised because the first element of ‘my_list’ is an integer, and we are trying to access the first element of that integer.
3. Accidental shadowing of built-in functions: Another situation that can trigger the error is when you accidentally shadow the built-in functions, such as ‘int’, with your custom-defined variables. For instance:
“`python
int = 42
print(int[0])
“`
In this case, the ‘int’ variable refers to a user-defined variable, which is now a simple integer. As a result, calling ‘int[0]’ will raise the error.
Resolving the ‘int’ object not subscriptable Error
Now that we have identified some common causes of the error, let’s explore potential solutions:
1. Review your code for indexing mistakes: If you directly try to index an integer or incorrectly access an integer element within another data type, double-check your code for any indexing errors. Remember that only subscriptable objects, such as lists or strings, can be accessed through indexing.
2. Avoid shadowing built-in functions: To prevent naming conflicts with built-in functions or types, it is advisable to choose variable names that do not overlap with these keywords. In the example mentioned earlier, avoid using ‘int’ as a variable name.
3. Revisit your code’s logic: If you encounter the error while performing operations on mixed data types, such as lists with integers and strings, review your code’s logic to ensure you are performing the correct operations on the appropriate data types.
FAQs Section
Q1. Can I ever subscript an integer in Python?
A1. No, you cannot subscript an integer directly. As mentioned earlier, integers are atomic objects and do not support indexing.
Q2. What if I mistakenly shadow the ‘int’ type with my custom variable name?
A2. If you accidentally shadow the ‘int’ type, Python will consider your custom variable as the valid reference. To resolve this issue, remember to use unique variable names that do not conflict with the built-in types or functions.
Q3. Does this error occur in other programming languages?
A3. The specific error message “‘int’ object is not subscriptable” is Python-specific and may not exist in other programming languages. However, similar issues related to indexing or unsupported operations on certain data types can arise in other languages.
Q4. Are there any workarounds for accessing integer elements?
A4. Since integers are indivisible, there is no direct workaround to access specific elements within them. However, you can convert integers to string or list representations and perform operations accordingly.
Conclusion
Understanding the “‘int’ object is not subscriptable” error is vital for Python developers aiming to write clean and efficient code. By recognizing the potential pitfalls and implementing the solutions discussed in this article, you can easily circumvent this error and enhance your coding skills. Remember to review your code carefully, avoid shadowing built-in functions, and ensure correct operations on appropriate data types to minimize such errors in the future.
Images related to the topic typeerror: ‘type’ object is not subscriptable
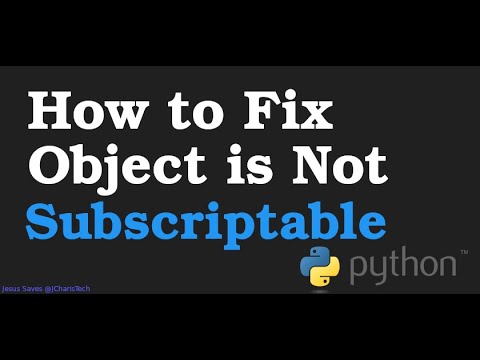
Found 19 images related to typeerror: ‘type’ object is not subscriptable theme

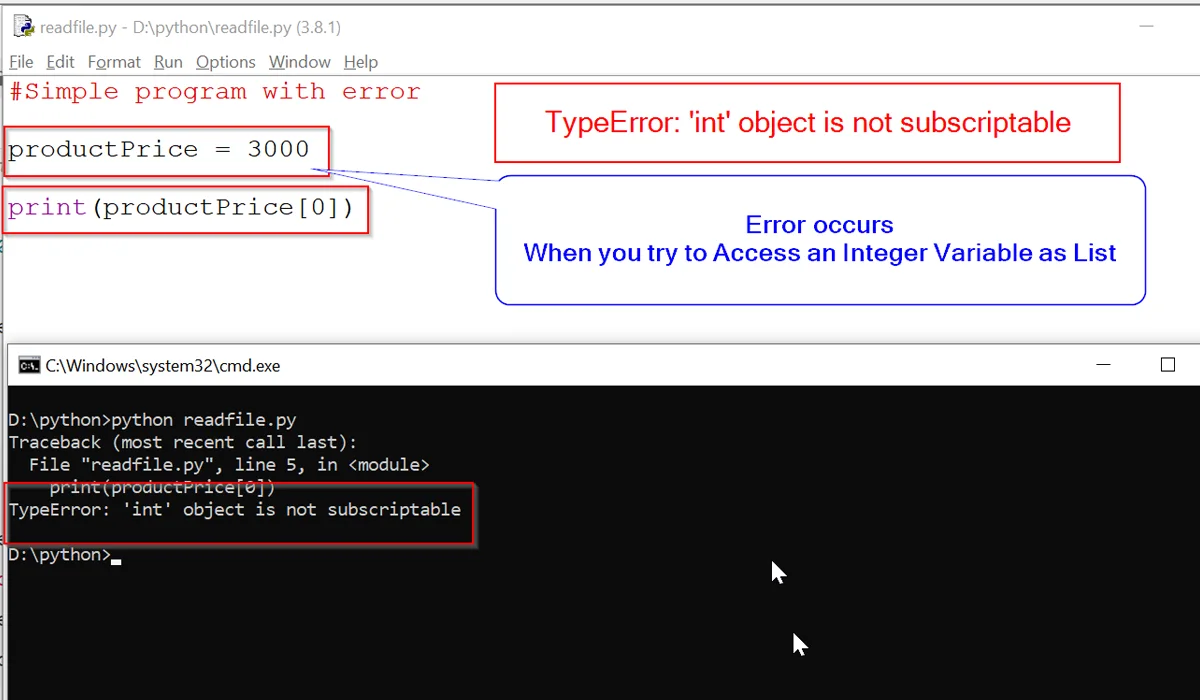
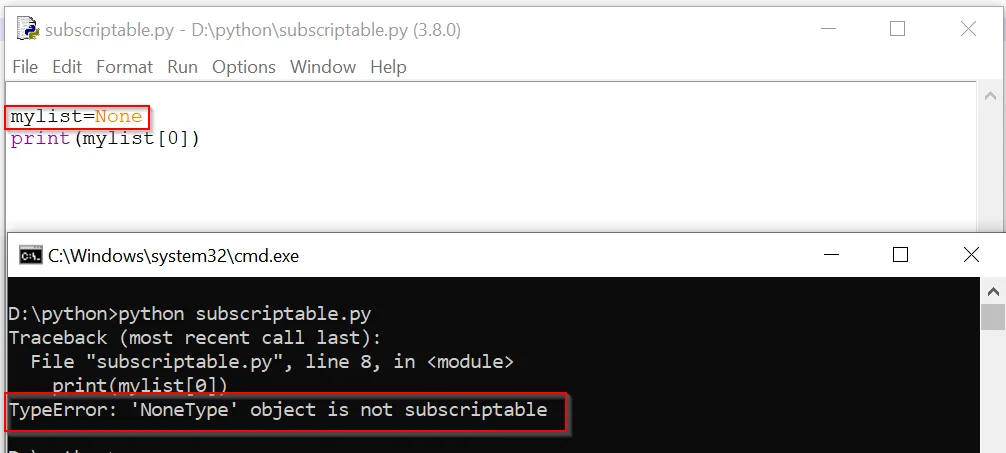
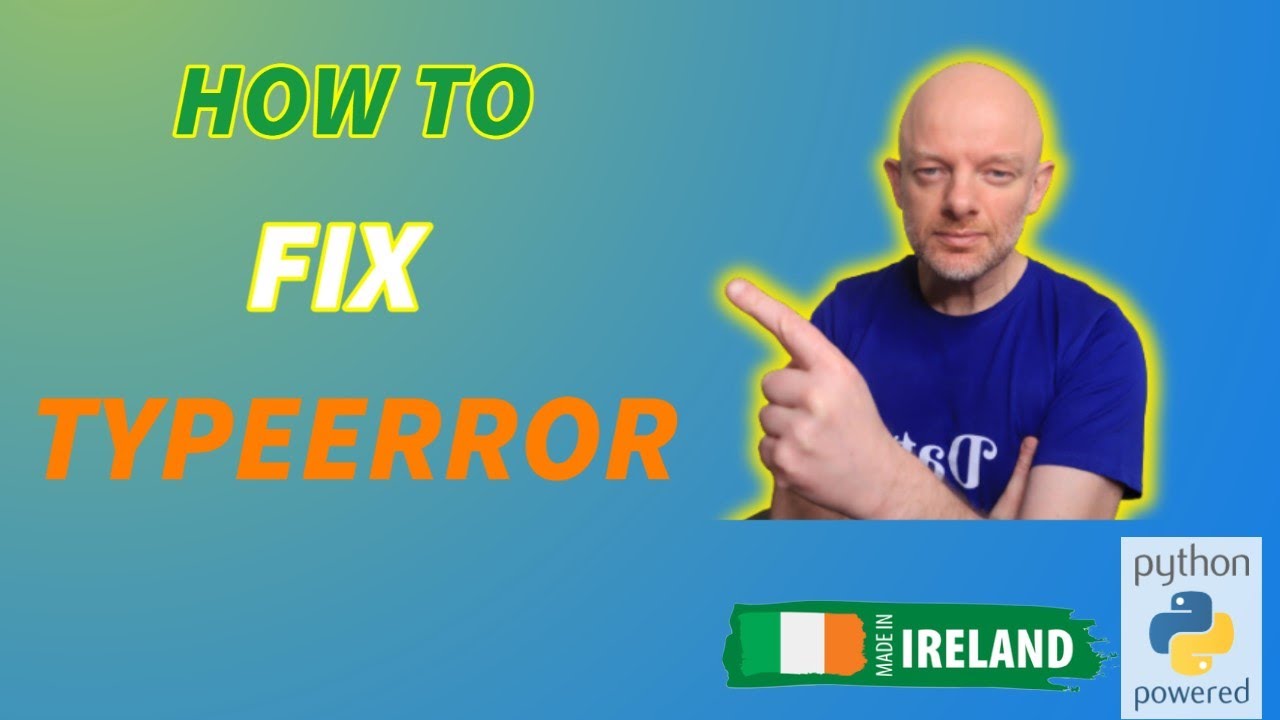


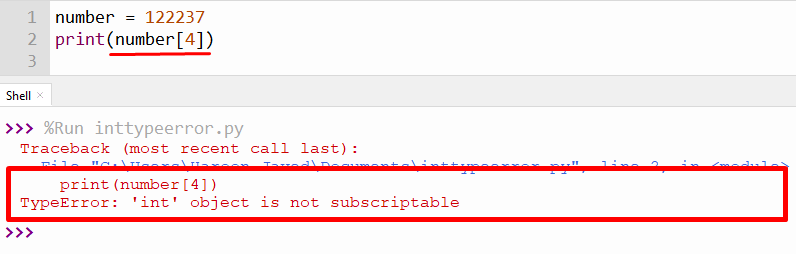


![TypeError: 'int' object is not subscriptable [Solved Python Error] Typeerror: 'Int' Object Is Not Subscriptable [Solved Python Error]](https://www.freecodecamp.org/news/content/images/2022/10/in_not_subable.png)
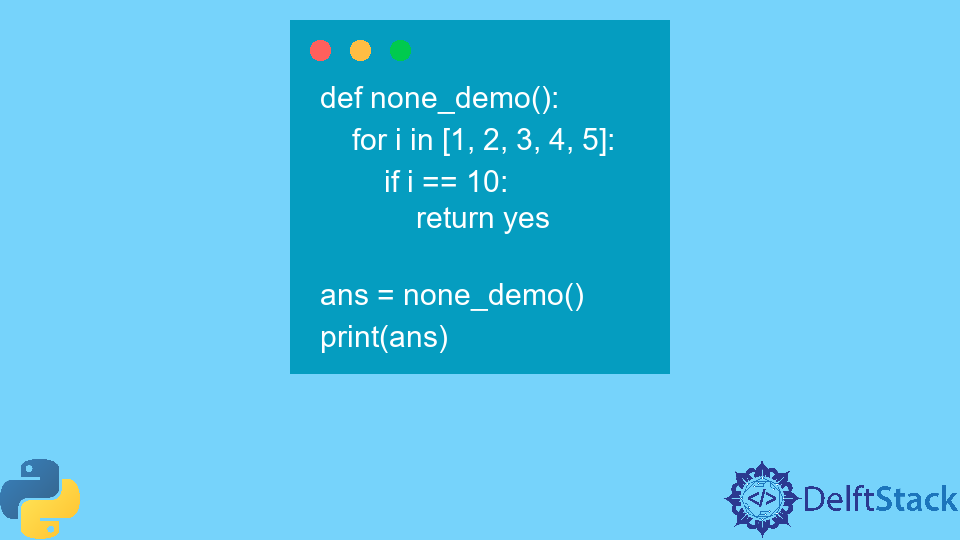


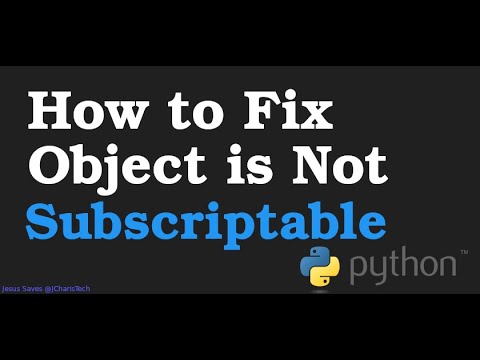


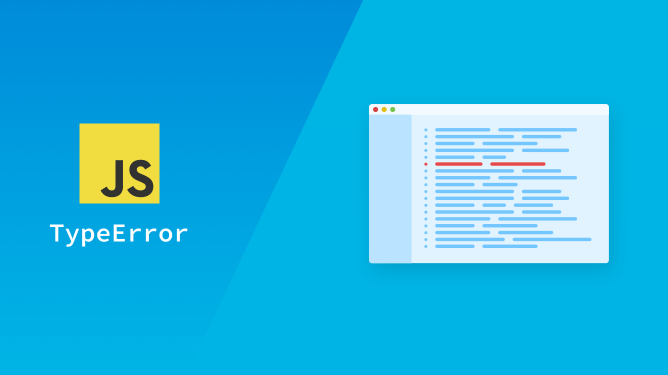
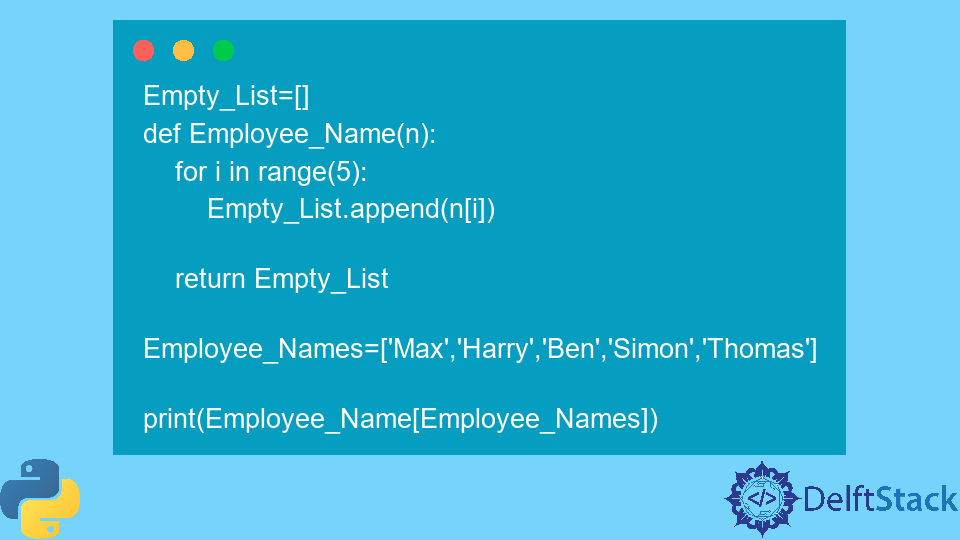
![TypeError: 'int' object is not subscriptable [Solved Python Error] Typeerror: 'Int' Object Is Not Subscriptable [Solved Python Error]](https://www.freecodecamp.org/news/content/images/2023/01/kolade-recent.jpg)
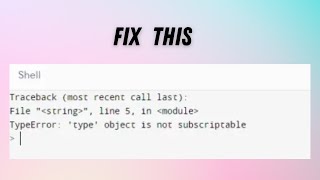
_typeerror-39type39-object-is-not-subscriptable-during-reading-data.jpg)
_how-to-fix-typeerror-type-object-is-not-subscriptable.jpg)
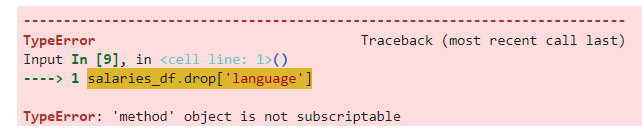
_i-have-solved-typeerror-39set39-object-is-not-subscriptable-in-python.jpg)

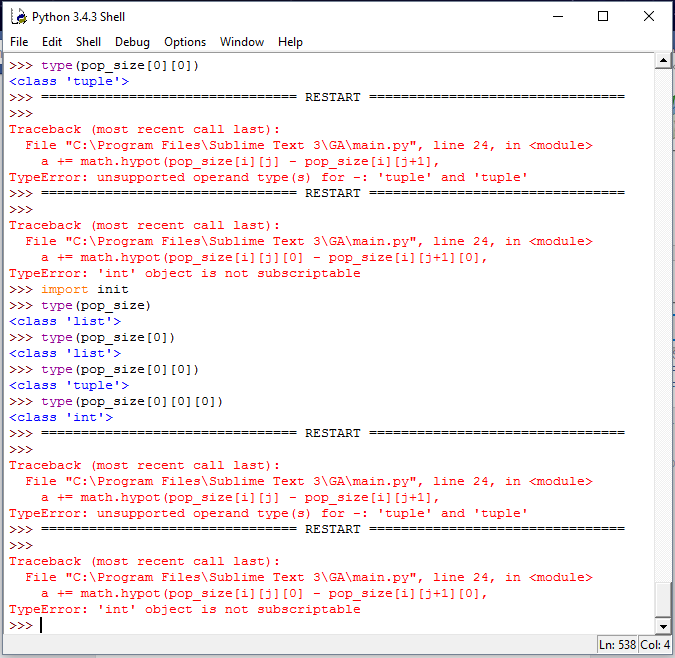
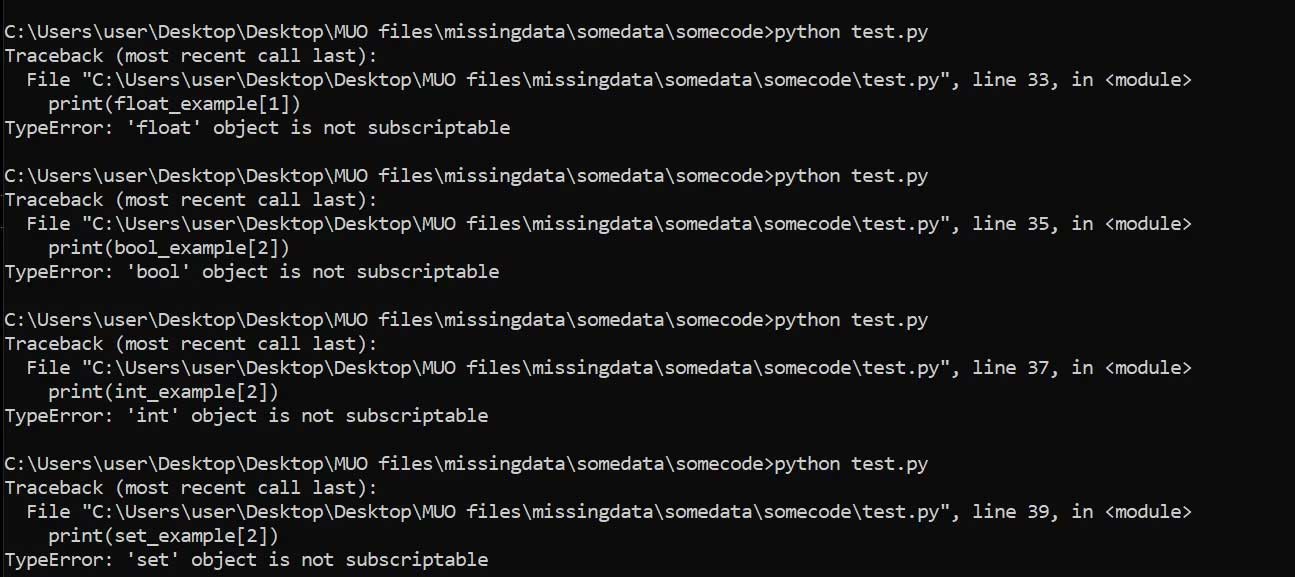
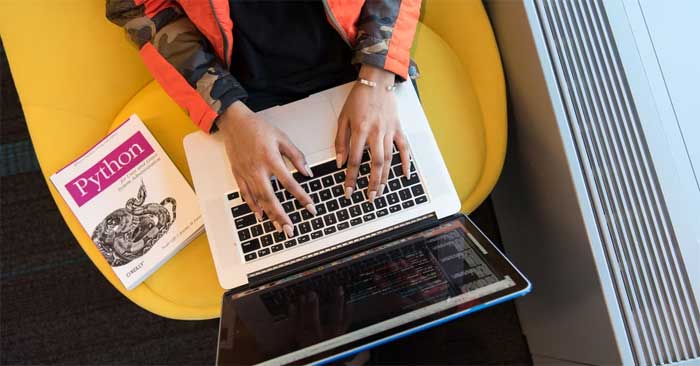
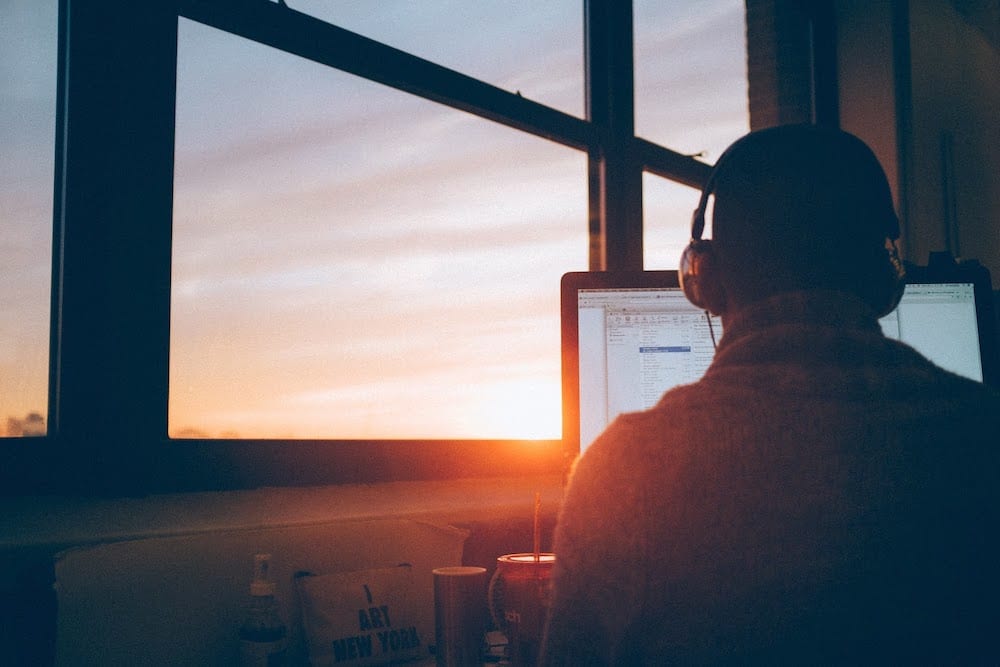

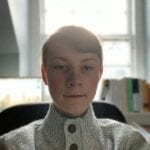
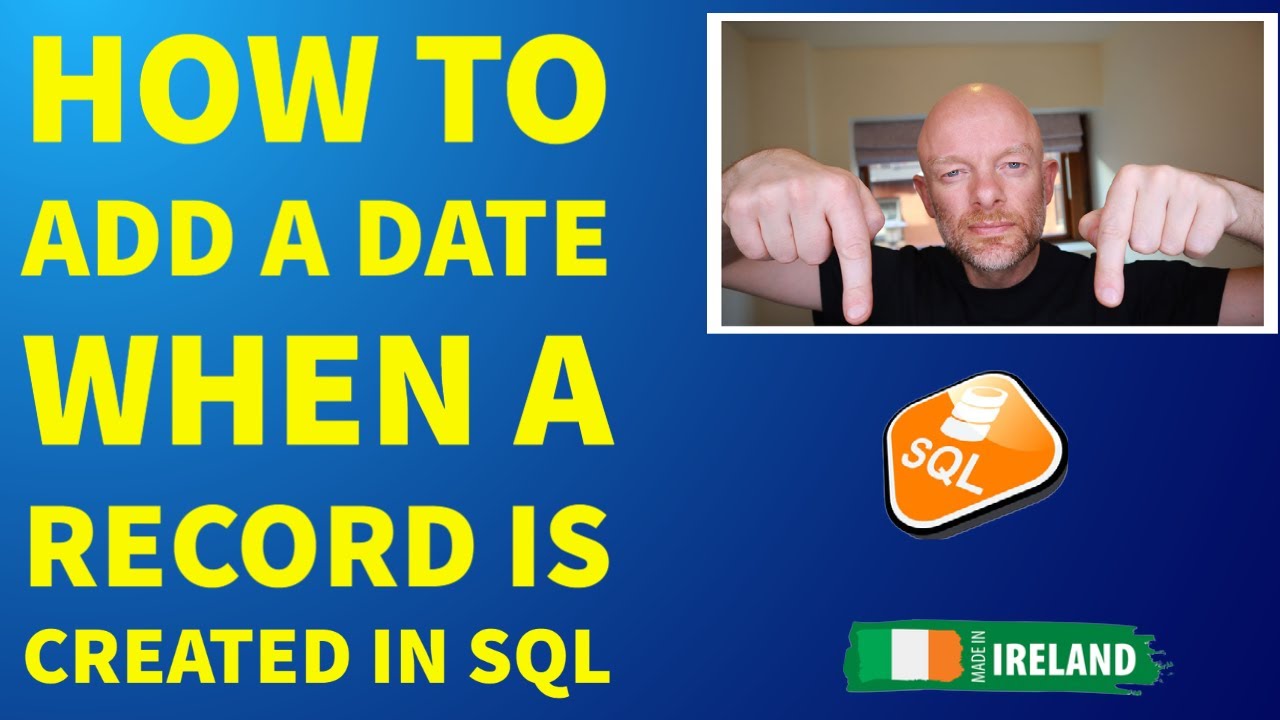


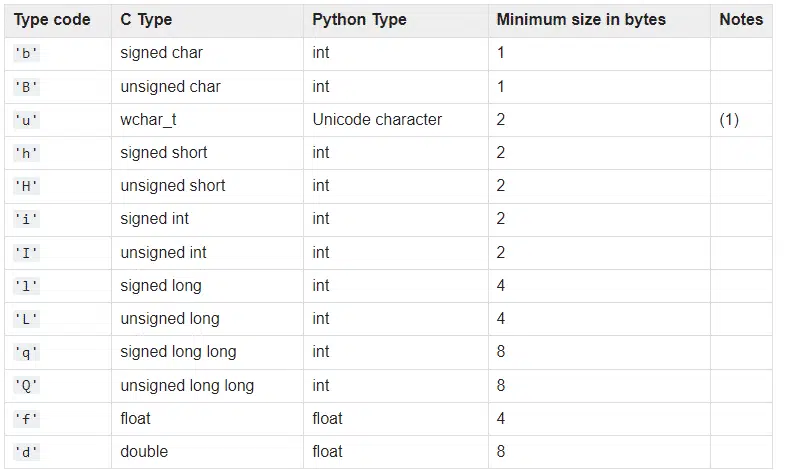
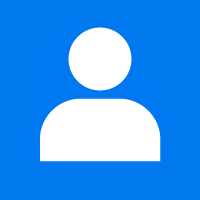

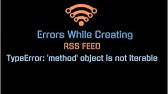

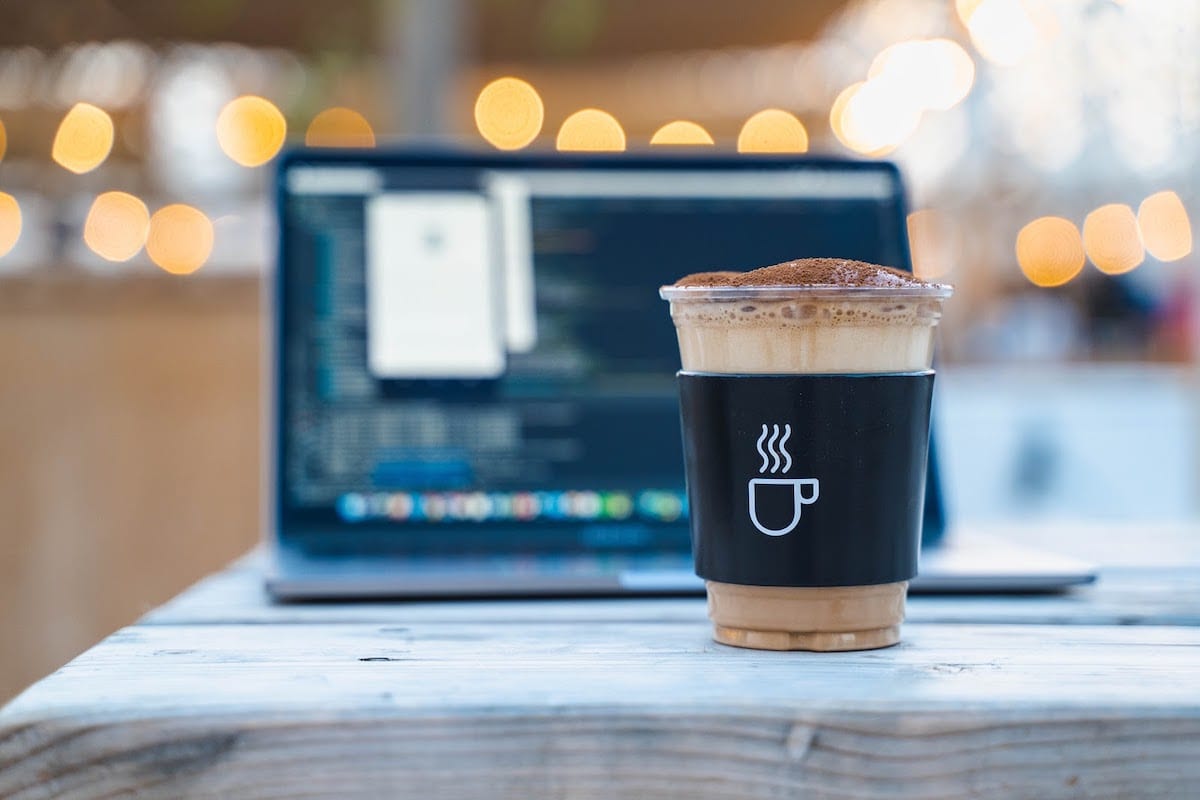
![Solved][Python] Solved][Python]](https://i0.wp.com/clay-atlas.com/wp-content/uploads/2020/08/python.jpg?fit=768%2C457&ssl=1&resize=350%2C200)
Article link: typeerror: ‘type’ object is not subscriptable.
Learn more about the topic typeerror: ‘type’ object is not subscriptable.
- TypeError: ‘int’ object is not subscriptable [Solved Python Error]
- Python TypeError: ‘type’ object is not subscriptable Solution
- Why can’t I use a dictionary named `dict` before assigning to it?
- How to fix the typeerror ‘type’ object is not subscriptable error …
- Typeerror: type object is not subscriptable ( Steps to Fix)
- Python TypeError: ‘type’ object is not subscriptable Solution
- How to Fix the “TypeError: object is not subscriptable” Error in Python
- TypeError: builtin_function_or_method object is not subscriptable Python …
- Python TypeError: Object is Not Subscriptable (How to Fix This …
- How to Fix “TypeError: ‘type’ object is not subscriptable”
- ‘NoneType’ object is not subscriptable in Python – Rollbar
See more: nhanvietluanvan.com/luat-hoc