Thread Safe Cache C#
Overview of Thread Safe Caching
In the world of software development, caching plays a significant role in improving performance and scalability of applications. Caching refers to the process of storing frequently accessed data in a high-speed storage location, allowing subsequent requests for that data to be served quickly. Thread safe caching, in particular, ensures that multiple threads can access the cache concurrently without causing data corruption or inconsistencies.
Benefits of Thread Safe Caching
1. Improved Performance and Scalability: Thread safe caching optimizes the utilization of system resources by minimizing the need for repeated expensive computations or expensive I/O operations. As multiple threads can access the cache simultaneously, it reduces the waiting time and improves overall application performance and scalability.
2. Consistency and Predictability of Cached Data: Thread safe caching ensures that the data retrieved from the cache is consistent and up-to-date, guaranteeing the accuracy and predictability of the cached data. This eliminates the risk of serving stale or outdated data to the users, ultimately enhancing the user experience.
Thread Safety in C#
1. Understanding Thread Safety: Thread safety refers to the ability of an application or code to function correctly and consistently when accessed by multiple threads simultaneously. In the context of caching, it means that the cache can handle concurrent read and write operations without causing data corruption or producing inconsistent results.
2. Challenges of Thread Safety in C#: Implementing thread safety in C# can be challenging due to the potential for race conditions, deadlocks, and performance bottlenecks. Efficient synchronization techniques must be employed to ensure mutual exclusion and ordered access to shared resources.
Synchronization Techniques in C#
1. Locking Mechanisms: C# provides various locking mechanisms such as lock keyword, Monitor class, and Mutex class to achieve thread synchronization. These mechanisms ensure that only one thread can access a critical section of code at a time, preventing concurrent access and potential data corruption.
2. Concurrent Collections: .NET framework also provides concurrent collections like ConcurrentDictionary, ConcurrentBag, and ConcurrentQueue, which are designed specifically for concurrent access scenarios. These collections internally handle synchronization and provide thread-safe operations to store and retrieve data.
Implementation of Thread Safe Cache in C#
1. Design Considerations: When implementing a thread-safe cache in C#, it is crucial to carefully design the cache structure and choose appropriate data structures for efficient access and mutation operations. The cache should be optimized for both read and write operations, considering the expected load and data size.
2. Handling Concurrent Requests: The cache should be able to handle multiple concurrent requests efficiently. Synchronization techniques like locking mechanisms or concurrent collections can be used to ensure thread safety during read and write operations.
3. Optimizing Cache Size and Expiration: To prevent the cache from becoming a memory hog, it is essential to define a size limit and implement an expiration policy for cache entries. This ensures that the cache remains efficient and only contains relevant and recent data.
4. Cleaning Up Expired Cache Entries: A mechanism should be implemented to periodically clean up expired cache entries. This ensures that stale data is removed from the cache, freeing up memory for new entries.
5. Handling Cache Invalidation: In scenarios where data in the cache becomes invalid due to external changes, a mechanism should be in place to handle cache invalidation. This could involve using callbacks or event mechanisms to update or remove stale data from the cache.
Best Practices for Thread Safe Caching in C#
1. Granularity of Locking: Locking should be performed at the appropriate level of granularity to ensure that only the necessary critical sections are protected. Overly coarse-grained locking can result in unnecessary contention, while fine-grained locking can lead to increased lock acquisition times.
2. Avoiding Deadlocks: Care should be taken to avoid potential deadlocks when implementing thread-safe caching. Circular dependency on locks and inconsistent ordering of lock acquisitions can result in deadlocks, where multiple threads are indefinitely waiting for locks.
3. Monitoring and Managing Cache Usage: It is important to monitor the usage of the cache to ensure optimal performance. Metrics such as cache hit rate, cache miss rate, and cache size should be tracked and analyzed to identify potential bottlenecks or areas of optimization.
Conclusion
Thread safe caching in C# is a powerful technique for improving the performance, scalability, and consistency of applications. By implementing efficient synchronization techniques and careful design considerations, developers can ensure that multiple threads can safely access and manipulate the cache without causing data corruption or inconsistencies. With the benefits of improved performance and predictability of cached data, thread safe caching proves to be an essential tool in modern software development.
FAQs
1. What is a C read-write lock in C++11?
In C++11, a C read-write lock refers to a synchronization primitive that allows multiple readers to simultaneously read a shared resource, while only allowing a single writer at a time. This allows for efficient concurrent access to the resource with minimal locking overhead.
2. What is boost::shared_mutex in C++?
boost::shared_mutex is a thread-safe synchronization primitive provided by the Boost C++ library. It allows multiple threads to acquire shared read access to a resource, while allowing exclusive write access to a single thread.
3. What is shared_lock in C++?
shared_lock is a type of lock that allows multiple threads to acquire shared read access to a resource. It is typically used in conjunction with shared_mutex to enable concurrent read operations.
4. How can shared memory mutex be used in C?
Shared memory mutex in C is typically implemented using operating system mechanisms, such as POSIX named semaphores or sysv semaphores. These mechanisms allow multiple processes to synchronize access to a shared memory region using a mutex-like mechanism.
5. Can you provide an example of multiple reader single writer lock in C++?
Certainly! Here’s a basic example of implementing a multiple reader single writer lock in C++:
“`cpp
class MultipleReaderSingleWriterLock {
private:
std::mutex mutex_;
std::condition_variable cv_;
int active_readers_, waiting_readers_;
bool has_writer_, writer_done_;
public:
MultipleReaderSingleWriterLock()
: active_readers_(0),
waiting_readers_(0),
has_writer_(false),
writer_done_(false) {}
void lockRead() {
std::unique_lock
while (has_writer_ || waiting_readers_ > 0) {
cv_.wait(lock);
}
++active_readers_;
}
void unlockRead() {
std::unique_lock
–active_readers_;
if (active_readers_ == 0 && writer_done_) {
cv_.notify_one();
}
}
void lockWrite() {
std::unique_lock
++waiting_readers_;
while (has_writer_ || active_readers_ > 0) {
cv_.wait(lock);
}
–waiting_readers_;
has_writer_ = true;
}
void unlockWrite() {
std::unique_lock
has_writer_ = false;
writer_done_ = true;
cv_.notify_all();
}
};
“`
This implementation allows multiple threads to acquire shared read access while ensuring exclusive write access for a single thread at a time, preventing data corruption and inconsistencies.
C# 101 – Thread Safe Programing
Keywords searched by users: thread safe cache c# c read-write lock c++11, boost::shared_mutex, shared lock c++, shared memory mutex c, cpp shared mutex example, multiple reader single writer lock c++
Categories: Top 72 Thread Safe Cache C#
See more here: nhanvietluanvan.com
C Read-Write Lock C++11
Introduction:
In concurrent programming, multiple threads can access shared resources simultaneously. However, when multiple threads try to modify the same data simultaneously, conflicts and errors can occur. To ensure safe and secure data access, synchronization mechanisms such as locks or mutexes are used. C++11 provides a valuable addition to concurrent programming with read-write locks, offering an efficient solution to the problem.
Understanding Read-Write Locks:
A read-write lock, also known as a multiple readers/single writer lock, allows multiple threads to concurrently read data while ensuring that only one thread can write to it at a time. This enhances the overall performance by allowing concurrent reads, while still ensuring data integrity by preventing data races during writes.
The read-write lock consists of two states: shared and exclusive. Multiple threads can hold a shared lock simultaneously, facilitating concurrent read access. However, when a thread needs exclusive access to modify the data, it must obtain an exclusive lock, blocking all other threads until the write operation has completed.
C++11’s std::shared_mutex:
C++11 introduced std::shared_timed_mutex, a type of read-write lock, in the standard library to simplify concurrent programming. Its usage is similar to other mutex types and facilitates safe and efficient shared and exclusive access to shared resources.
Key Operations:
1. Locking for Writing (Exclusive Access):
– std::unique_lock
2. Locking for Reading (Shared Access):
– std::shared_lock
3. Upgrade Shared Lock to Exclusive Lock:
– std::unique_lock
4. Downgrade Exclusive Lock to Shared:
– std::shared_lock
FAQs:
Q1. Why use a read-write lock instead of a mutex?
A read-write lock allows concurrent read access, which can significantly improve performance in scenarios where reads outnumber writes. Mutexes, on the other hand, provide exclusive access, leading to unnecessary serializations when multiple threads only need read access.
Q2. Does read-write lock guarantee fairness?
No, read-write locks do not provide fairness guarantees. Threads may be starved if a continuous stream of writers prevents subsequent shared access.
Q3. Can a thread with a shared lock be blocked by a writer?
No, shared locks do not block other shared locks. Hence, threads with shared locks do not prevent writers from acquiring an exclusive lock. However, if a writer is waiting to acquire an exclusive lock, subsequent threads requesting shared access will be blocked until the writer has finished.
Q4. What happens if a thread with an exclusive lock tries to acquire a shared lock?
If a thread already holds an exclusive lock and tries to acquire a shared lock, it will deadlock. The thread should either release the exclusive lock before acquiring the shared lock or upgrade the shared lock to an exclusive lock.
Q5. How does an upgrade from shared to exclusive lock work?
Upgrading from a shared lock to an exclusive lock requires acquiring a new std::unique_lock while still holding the shared lock. The upgrader lock then releases the shared lock, ensuring exclusive access.
Q6. How can an exclusive lock be downgraded to a shared lock?
An exclusive lock can be downgraded to a shared lock by releasing the exclusive lock via std::unique_lock::unlock() and then acquiring a shared lock using std::shared_lock constructor.
Q7. Can deadlock occur when using read-write locks?
Deadlocks can potentially occur if multiple threads simultaneously acquire shared locks and later attempt to upgrade them to exclusive locks. Care should be taken to avoid such situations.
Conclusion:
In concurrent programming, ensuring thread-safe access to shared data is vital for reliable and efficient applications. C++11’s std::shared_mutex, a read-write lock implementation, provides an efficient and reliable solution. By allowing concurrent read access and serializing write access, read-write locks strike a balance between performance and data integrity. Understanding the usage and nuances of read-write locks in C++11 can significantly improve concurrent programs, leading to more robust and efficient codebases.
Boost::Shared_Mutex
Understanding boost::shared_mutex:
A mutex, short for mutual exclusion, is a synchronization primitive that allows threads to take turns accessing shared data. Traditional mutexes, also known as exclusive locks, allow only one thread at a time to acquire the lock and modify the shared data. On the other hand, shared mutexes, also referred to as reader-writer locks, allow multiple threads to concurrently read the shared data as long as there are no writers holding the lock.
boost::shared_mutex is an implementation of such a reader-writer lock in the Boost Libraries. It provides two modes of locking: shared locking and exclusive locking. Multiple threads can acquire shared locks simultaneously, but only one thread can hold an exclusive lock at any given time.
Using boost::shared_mutex:
To utilize boost::shared_mutex, you need to include the necessary header file, “boost/thread/shared_mutex.hpp.” Once included, you can create an instance of boost::shared_mutex as follows:
“`cpp
boost::shared_mutex mutex;
“`
The shared_mutex variable, “mutex,” acts as a synchronization point for your shared data. To acquire a shared lock, which allows reading the shared data, you can use the boost::shared_lock class and pass the mutex as an argument:
“`cpp
boost::shared_lock
“`
Conversely, to obtain an exclusive lock, which allows both reading and modifying the shared data, you can use the boost::unique_lock class, similar to traditional mutexes:
“`cpp
boost::unique_lock
“`
It is important to note that while a thread holds an exclusive lock, no other threads, including those trying to acquire shared locks, can access the shared data. This exclusive locking ensures synchronization and prevents race conditions when modifying the shared data.
When using boost::shared_mutex, it is crucial to consider the proper locking semantics for your use case. Shared locks should be acquired when only reading the shared data, as they allow multiple threads to access it simultaneously. Exclusive locks, on the other hand, should be acquired when intending to modify the shared data exclusively, preventing any other threads from accessing it altogether.
Benefits of boost::shared_mutex:
1. Improved concurrency: With boost::shared_mutex, multiple threads can read the shared data concurrently, thereby reducing contention and improving performance. This is particularly beneficial when the number of readers outweighs the writers, as shared locks are non-blocking among readers.
2. Enhances scalability: By allowing fine-grained locking, boost::shared_mutex minimizes the amount of serialization required in multi-threaded applications. It ensures that the shared data is accessible to as many simultaneous readers as possible, significantly improving scalability.
3. Prevention of data races: The exclusive locking mechanism of boost::shared_mutex prevents multiple threads from modifying the shared data simultaneously, effectively eliminating data races and ensuring data integrity.
FAQs about boost::shared_mutex:
Q1. What happens if a thread tries to acquire an exclusive lock while others hold shared locks?
A1. The thread attempting to acquire an exclusive lock will be blocked until all shared locks are released. This ensures that no threads can read the shared data while it is being modified.
Q2. Can multiple threads acquire shared locks simultaneously?
A2. Yes, boost::shared_mutex allows multiple threads to acquire shared locks simultaneously as long as there are no exclusive locks held. This allows efficient and concurrent read-only access.
Q3. Does boost::shared_mutex provide additional features apart from shared and exclusive locking?
A3. No, boost::shared_mutex only provides shared and exclusive locking mechanisms. It does not offer any additional features. For more advanced synchronization requirements, Boost Libraries provide other synchronization primitives such as condition variables and semaphores.
Q4. Can boost::shared_mutex be used across multiple processes?
A4. No, boost::shared_mutex is designed for synchronization within a single process. If synchronization is required across multiple processes, inter-process synchronization primitives such as named semaphores or file locks can be used instead.
Q5. Are there any performance considerations when using boost::shared_mutex?
A5. While boost::shared_mutex can greatly improve concurrency and scalability, it introduces some overhead due to the synchronization mechanisms it employs. Therefore, it’s essential to consider your application’s specific requirements and profile its performance to ensure optimal results.
In conclusion, boost::shared_mutex is a valuable tool for managing concurrent access to shared data in C++ applications. By allowing multiple threads to read simultaneously and providing exclusive access for writing, it ensures proper synchronization and prevents data races. When used correctly, boost::shared_mutex can significantly enhance the performance and scalability of multi-threaded applications, enabling efficient and safe manipulation of shared data.
Shared Lock C++
Concurrency is a fundamental aspect of modern software development, allowing programs to efficiently handle multiple tasks simultaneously. However, parallel execution can introduce potential data integrity issues, as multiple threads may attempt to access shared resources concurrently. Locking mechanisms provide a solution to this problem by ensuring that only one thread can access a resource at any given time. In C++, one common type of lock is the shared lock, which allows multiple threads to read a shared resource simultaneously while ensuring exclusive access for writing.
Understanding Shared Lock
A shared lock allows concurrent read access to a shared resource while preventing write access by other threads. This type of lock is particularly useful when a resource is frequently read but infrequently written to. By allowing multiple threads to access the resource simultaneously for reading, shared locks can significantly improve the overall performance of a multi-threaded application.
In C++, shared locks are typically implemented using the `std::shared_mutex` class from the `
Using Shared Lock
Before using the shared lock, it is essential to create an instance of `std::shared_mutex` and associate it with the shared resource. Let’s consider a scenario where multiple threads need to read data from a shared container, which is guarded by a shared lock.
“`cpp
#include
#include
#include
std::shared_mutex resourceMutex;
std::vector
void readSharedData() {
std::shared_lock
// Access the shared resource
std::cout << "Reading shared data: ";
for (const auto& data : sharedContainer) {
std::cout << data << " ";
}
std::cout << std::endl;
}
int main() {
// Populate the shared container
sharedContainer = {1, 2, 3, 4, 5};
// Create multiple threads to read shared data
std::thread t1(readSharedData);
std::thread t2(readSharedData);
// Wait for threads to finish
t1.join();
t2.join();
return 0;
}
```
In the above example, the `std::shared_lock` is used to acquire the shared lock in the `readSharedData()` function. This ensures that multiple threads can access the shared container simultaneously for reading, preventing any concurrent writes.
Benefits of Shared Lock
Shared locks offer various benefits in scenarios where multiple threads require read access to a shared resource. The key advantages include:
1. Improved Performance: By allowing multiple threads to read the resource simultaneously, shared locks can significantly improve the overall performance of a multi-threaded application, especially if reading is more common than writing.
2. Reader-Writer Synchronization: Shared locks provide synchronization between threads, ensuring that writes are deferred until all readers have completed their tasks. This approach balances concurrency while maintaining data integrity.
3. Granular Control: Shared locks allow fine-grained control over read and write access, enabling efficient resource utilization.
Frequently Asked Questions
Q1. Can multiple threads simultaneously acquire a shared lock?
Yes, multiple threads can simultaneously acquire shared locks. Shared locks allow concurrent read access to a shared resource while preventing write access by other threads. This allows multiple threads to read the resource simultaneously.
Q2. Can a shared lock be upgraded to an exclusive lock?
No, a shared lock cannot be directly upgraded to an exclusive lock without releasing the shared lock first. Releasing the shared lock allows other threads to acquire the exclusive lock for writing.
Q3. How does a shared lock ensure data integrity?
Shared locks provide synchronization between reader and writer threads. When a shared lock is acquired, it allows multiple reader threads to simultaneously read from the shared resource. However, when a writer thread wants to modify the resource, it requests an exclusive lock, causing all subsequent reader threads to wait until the writer completes its task. This ensures that writes are deferred until all readers have finished reading, maintaining data integrity.
Q4. Can a shared lock be used in a single-threaded application?
While shared locks are primarily designed to handle multi-threaded scenarios, they can also be used in single-threaded applications. However, the benefits of shared locks, such as improved performance and reader-writer synchronization, are more significant in multi-threaded environments.
Q5. Are there any performance considerations when using shared locks?
Shared locks introduce a minimal overhead when used properly. However, it is essential to use shared locks judiciously and only when necessary to avoid excessive locking and potential performance degradation. Additionally, it is crucial to ensure that the shared resource is thread-safe and any necessary synchronization is implemented correctly.
In conclusion, shared locks in C++ provide an efficient mechanism to handle concurrent read access to shared resources while maintaining data integrity. By allowing multiple threads to read simultaneously and preventing write access by other threads, shared locks can significantly enhance the performance and concurrency of multi-threaded applications. However, careful consideration should be given to the usage of shared locks and proper synchronization to ensure efficient and correct multi-threaded programming.
Images related to the topic thread safe cache c#
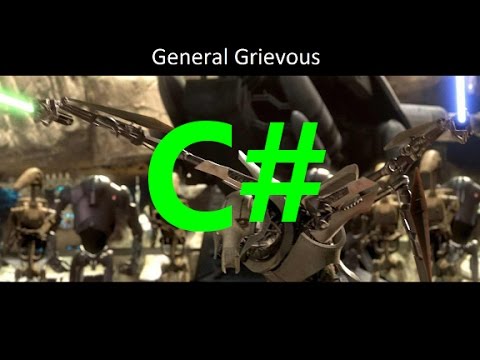
Article link: thread safe cache c#.
Learn more about the topic thread safe cache c#.
- How to make a cache thread safe – c++ – Stack Overflow
- How to make cache thread-safe in your .NET C# application
- Thread-safe LRU Cache Implementation – LeetCode
- thread-safe-cache – crates.io: Rust Package Registry
- A Lightweight Thread Safe In-Memory Keyed Generic Cache …
- What Is Thread-Safety and How to Achieve It? – Baeldung
See more: nhanvietluanvan.com/luat-hoc