Test Button Click React Testing Library
React Testing Library is a popular tool used for testing React components. It provides a set of utilities that enable developers to write tests that closely resemble how a user would interact with an application. This library focuses on testing the behavior rather than implementation details, making tests more resilient to changes in the codebase.
Understanding the Purpose of Testing Buttons in React
Buttons are one of the most common user interface elements in web applications. They are often used to trigger actions or perform specific tasks. Testing buttons in React ensures that the expected behavior is exhibited when a user interacts with them. This includes checking for events, state changes, and proper rendering of UI components.
Exploring the Fundamentals of Button Click Testing
Button click testing involves simulating user interaction with a button to verify that the intended actions are executed correctly. This includes testing event handlers, checking for state changes, and validating UI updates triggered by button clicks.
Writing Test Cases for Button Click Functionality using React Testing Library
When writing test cases for button click functionality, it is important to consider the different scenarios and possible outcomes. Test cases should cover various aspects such as testing event handlers, verifying state changes, and ensuring proper rendering of UI components based on button actions.
Using FireEvent to Simulate Button Clicks in React Tests
React Testing Library provides the fireEvent utility to simulate user events. To simulate a button click, fireEvent.click can be used, passing the button element as the argument. This triggers the event handlers associated with the button click, allowing developers to test the resulting behavior.
Testing Button Click Effects and State Changes in React Components
When a button is clicked, it may trigger effects or lead to changes in the component’s state. To test these, developers can use assertions to verify the expected effects and state changes. For example, if clicking a button should hide a specific element, the test can assert that the element is not visible after the click event.
Testing User Interactions with Buttons in React Forms
In a React form, buttons are often used to submit or reset the form. Testing user interactions with buttons in forms involves simulating button clicks and validating the corresponding form actions. This includes testing form submission, form state changes, and proper validation of input fields.
Managing Asynchronous Button Click Events in React Tests
In some cases, button click events may trigger asynchronous actions such as API calls or state updates. React Testing Library provides utilities like async/await and waitFor to handle asynchronous events in tests. These can be used to wait for the expected changes or results before making assertions.
Best Practices for Testing Button Click Behavior with React Testing Library
When testing button click behavior with React Testing Library, it is important to follow best practices to ensure effective and reliable tests. Some best practices include:
1. Use the appropriate queries and assertions provided by React Testing Library to verify the expected behavior.
2. Avoid wrapping testing-library utility calls in the act() function, as it should only be used for updating state and triggering re-renders.
3. Test not only the presence of UI elements but also their content using queries like toHaveTextContent or getByText.
4. Use the getByTestId or getByText queries to target specific elements when testing button click effects.
5. If encountering issues with firing a click event, double-check that the correct DOM element is being targeted and that it exists in the rendered component.
6. For testing checkbox behavior, use the appropriate queries such as getByRole or getByLabelText to target and interact with checkboxes.
7. Test the behavior of buttons within nested elements, such as divs, by first obtaining the text within the div and using it to target the desired button.
8. If experiencing difficulties firing a click event, ensure that the element being clicked is interactable and that there are no overlapping elements obstructing the click event.
In conclusion, testing button click functionality in React components is crucial for ensuring a smooth and reliable user experience. React Testing Library provides a comprehensive set of tools and utilities to simplify the process of testing button click behavior. By following best practices and considering various scenarios, developers can effectively validate the behavior of buttons in their React applications.
How To Test Onclick Handler? | Test Reactjs Components.
Keywords searched by users: test button click react testing library Test button react testing library, Avoid wrapping testing-library util calls in act, Test checkbox react testing-library, Getbyname react testing library, toHaveTextContent react-testing-library, Get div text react testing library, Unable to fire a click event – please provide a DOM element, fireEvent change input
Categories: Top 71 Test Button Click React Testing Library
See more here: nhanvietluanvan.com
Test Button React Testing Library
Introduction
The React Testing Library has gained immense popularity among developers for its simplicity and effectiveness in testing React components. One essential aspect of React components is user interaction, and testing how they respond to user actions is crucial. The Test Button in React Testing Library plays a vital role in simulating user clicks and interactions, enabling developers to thoroughly test component behavior. This article will delve into the intricacies of the Test Button in React Testing Library, guiding you through its implementation and usage.
What is the Test Button?
The Test Button is a utility provided by the React Testing Library that allows developers to simulate user interactions with buttons in their components. This utility is built on top of React’s synthetic event system, ensuring that the behavior of the simulated click is as close to a real user interaction as possible. By utilizing the Test Button, developers can seamlessly test how their components respond to button clicks, thereby ensuring the correct functionality of their applications.
How to Use the Test Button?
Using the Test Button is straightforward, thanks to the intuitive API provided by the React Testing Library. Let’s walk through an example to understand its usage:
1. First, we need to install the necessary dependencies. If you haven’t already installed React Testing Library, you can do so by running:
“`
npm install @testing-library/react
“`
2. Once installed, we can import the Test Button utility into our test file:
“`
import { render, screen, fireEvent } from ‘@testing-library/react’;
“`
3. Next, let’s consider a simple example where we have a component that renders a button:
“`jsx
import React from ‘react’;
const MyComponent = () => {
const handleClick = () => {
// Handle button click event
};
return (
);
};
“`
4. Now, in our test file, we can simulate a button click using the Test Button utility:
“`jsx
import React from ‘react’;
import { render, screen, fireEvent } from ‘@testing-library/react’;
import MyComponent from ‘./MyComponent’;
describe(‘MyComponent’, () => {
it(‘should handle button click’, () => {
render(
const button = screen.getByText(‘Click Me’);
fireEvent.click(button);
// Assert the desired behavior
});
});
“`
In the example above, we use the `fireEvent.click()` function to simulate a button click event on the `button` element obtained using the `screen.getByText()` method. After simulating the click, we can then assert the desired behavior, validating the component’s response.
Frequently Asked Questions (FAQs):
Q1: Are there any alternatives to the Test Button utility in React Testing Library?
A1: While the Test Button utility is commonly used to simulate button clicks, you can also utilize other event simulation utilities provided by React Testing Library, such as `fireEvent.submit()` for form submission.
Q2: Can I simulate other user interactions using React Testing Library?
A2: Absolutely! React Testing Library offers various utilities to simulate user interactions. You can use `fireEvent.change()` to simulate input changes, `fireEvent.focus()` to simulate focus events, `fireEvent.keyUp()`, and many more.
Q3: How can I test the behavior of components that make API calls on button click?
A3: To test asynchronous behavior, you can leverage features like `waitFor()` and `mockResponseOnce()` provided by React Testing Library, which allow you to mock API responses and ensure that your component behaves correctly.
Q4: Can I test the styling changes that occur on button click using the Test Button utility?
A4: While the primary purpose of the Test Button utility is to simulate user interactions, you can also assert styling changes by utilizing React Testing Library’s `getComputedStyle()` function and checking for expected CSS properties.
Conclusion
The Test Button in React Testing Library provides developers with a simple and effective way to simulate user interactions with buttons in React components. By incorporating the Test Button utility into your testing workflow, you can thoroughly test the behavior of your components, ensuring the correct functionality of your applications. Remember to take advantage of other utilities provided by React Testing Library to test various user interactions. Happy testing!
Avoid Wrapping Testing-Library Util Calls In Act
When it comes to testing React components, the Testing Library family, such as React Testing Library or Angular Testing Library, has become the go-to solution for many developers. These testing libraries provide a simple and intuitive API to write tests that resemble how a user would interact with the application. However, there’s one common pitfall that developers often stumble upon while using these libraries – wrapping Testing Library utility functions in the act() function.
In this article, we’ll explore what wrapping Testing Library util calls in act() means, why it might not be the best approach, and how to avoid this anti-pattern. Furthermore, we’ll address some frequently asked questions related to this topic.
What does wrapping Testing Library util calls in act() mean?
Before diving into why wrapping Testing Library utility calls in act() might not be the best approach, let’s understand what this practice entails. The act() function is a utility provided by the React Testing Library to ensure that all updates to a component and its children are properly flushed and resolved before any assertions are made.
When using act(), you’re essentially telling React to finish all pending updates and, therefore, making sure the test’s environment accurately mirrors the application’s operational behavior. This is particularly important when dealing with asynchronous actions or state updates.
However, some developers mistakenly believe that wrapping all Testing Library utility calls, such as render() or fireEvent(), in act() is necessary for the test to work correctly. This misconception often leads to unnecessarily complicated test code and can make the tests harder to read and maintain.
Why is wrapping Testing Library util calls in act() not recommended?
While wrapping all Testing Library utility calls in act() might seem like a safe bet, it’s actually an anti-pattern that should be avoided for several reasons.
1. Excessive use of act(): Wrapping every utility call in act() can lead to excessive usage throughout the test code, making the tests verbose and less readable. act() should only be used when specifically dealing with asynchronous actions or state updates, not as a general wrapping for any Testing Library utility call.
2. Overcomplication of the tests: By wrapping util calls in act() when it’s not necessary, the code becomes unnecessarily complex. It can make it difficult to understand the intent of the test and can hinder future maintainability and debugging efforts.
3. False sense of security: Wrapping all Testing Library utility calls in act() can create a false sense of security, as it might mask the fact that certain side effects are not properly handled. Rather than relying on act() as a catch-all solution, it is crucial to identify and correctly handle asynchronous actions or updates on a case-by-case basis.
How to avoid wrapping Testing Library util calls in act()?
While avoiding the unnecessary wrapping of Testing Library utility calls in act(), it’s important to maintain the integrity and accuracy of your tests. Here are a few steps to follow to achieve this goal:
1. Identify asynchronous actions: Before writing any test, identify the specific asynchronous actions or state updates that need to be handled. This will help you determine if using act() is necessary or if the standard execution flow of the test is sufficient.
2. Use act() sparingly: Only wrap the specific utility calls that involve asynchronous actions or state updates in act(). This keeps your code clean and maintains the readability of the tests.
3. Leverage Testing Library’s features: React Testing Library has built-in mechanisms to handle asynchronous actions, such as findBy queries or waitFor. Utilize these features instead of relying on excessive use of act().
Frequently Asked Questions:
Q1. What problems can arise when wrapping all utility calls in act()?
Wrapping all utility calls in act() can lead to verbose tests, overcomplication of the code, and a false sense of security. It can also make it harder to identify and handle specific asynchronous actions or state updates, as they may be masked by the act() wrappers.
Q2. When should I use act() with Testing Library?
act() should be used when specifically dealing with asynchronous actions or state updates. It ensures that all updates are properly flushed and resolved before assertions are made. However, it should not be used as a general wrapping mechanism for all utility calls.
Q3. Are there any exceptions where wrapping all utility calls in act() is necessary?
No, there are no exceptions where wrapping all utility calls in act() is necessary. act() should only be used when specifically dealing with asynchronous actions or state updates.
In conclusion, while Testing Library utility functions like render() or fireEvent() simplify component testing, wrapping them all in act() is not recommended. Instead, identify asynchronous actions and state updates that require act() and use it sparingly. By following this approach, you can ensure the accuracy and readability of your tests while avoiding unnecessary code complexity.
Test Checkbox React Testing-Library
Introduction
With the increasing complexity of web applications, it is crucial to ensure proper testing for reliability and functionality. In the world of React development, one powerful and popular testing library is the React Testing-Library. This article will focus specifically on testing checkboxes using React Testing-Library and provide a comprehensive guide for developers. We will cover the basics, advanced techniques, and frequently asked questions related to testing checkboxes using this library.
The Basics
Before diving into the nitty-gritty of testing checkboxes, let’s first understand the fundamental concepts behind React Testing-Library. This library is designed to test React components in a user-centric and accessible manner. It encourages developers to test their code as users would interact with it, rather than focusing solely on implementation details.
To start testing a checkbox component, you need to import the necessary functions from React Testing-Library, such as `render` and `screen.getByRole`. These functions allow you to render the component and select elements by their defined roles, such as “checkbox” in this case.
Once the component is rendered, you can interact with the checkbox by using the `fireEvent.click` function. This simulates a user clicking on the checkbox, thereby triggering any associated behavior or state changes.
Advanced Techniques
Testing checkboxes becomes more intricate when dealing with complex scenarios, such as checkboxes with custom labels, controlled components, or handling of multiple checkboxes simultaneously. Thankfully, React Testing-Library provides various techniques to handle these situations.
1. Testing custom labels: If your checkbox component has a custom label, you can select it using `screen.getByText` function and check whether it is properly associated with the checkbox. This ensures that clicking on the label triggers the checkbox behavior.
2. Testing controlled components: Controlled checkboxes in React maintain their state through props or state variables. To test them, you need to set up the necessary props or state and verify that the checkbox reflects these changes when clicked.
3. Testing multiple checkboxes: In some cases, you may have multiple checkboxes, and you need to test their behavior collectively. React Testing-Library offers utilities like `screen.getAllByRole` to select all checkboxes and perform interactions or assertions on them simultaneously.
FAQs
Q1. How do I assert that a checkbox is checked?
To verify that a checkbox is checked, you can use the `expect` statement and the `checked` property of the checkbox element. For example:
“`javascript
expect(screen.getByRole(‘checkbox’)).toBeChecked();
“`
This assertion ensures that the checkbox is checked as expected.
Q2. How do I assert that a checkbox is not checked?
To assert that a checkbox is not checked, you can use the following expect statement:
“`javascript
expect(screen.getByRole(‘checkbox’)).not.toBeChecked();
“`
This statement confirms that the checkbox is not selected.
Q3. How can I test whether clicking on a checkbox triggers the associated behavior?
To test the behavior triggered by clicking on a checkbox, you can use the `fireEvent.click` function and assert the expected result. For example:
“`javascript
const checkbox = screen.getByRole(‘checkbox’);
fireEvent.click(checkbox);
expect(checkbox).toBeChecked();
// Add more assertions based on the expected behavior
“`
The click event simulation ensures that the checkbox behaves as expected when interacted with.
Q4. Can I use React Testing-Library with other testing frameworks?
Yes, React Testing-Library can be used with popular JavaScript testing frameworks such as Jest or Mocha. It provides a set of functions that integrate seamlessly into these frameworks, enabling developers to write tests using their preferred framework.
Conclusion
Testing checkboxes using React Testing-Library allows developers to ensure the correct behavior and functionality of checkbox components. By following the principles of user-centric testing, developers can write tests that resemble real user interactions. This comprehensive guide has covered the basics and advanced techniques of testing checkboxes and provided answers to frequently asked questions. With this knowledge, developers can confidently test their checkboxes and deliver more reliable React applications.
Images related to the topic test button click react testing library
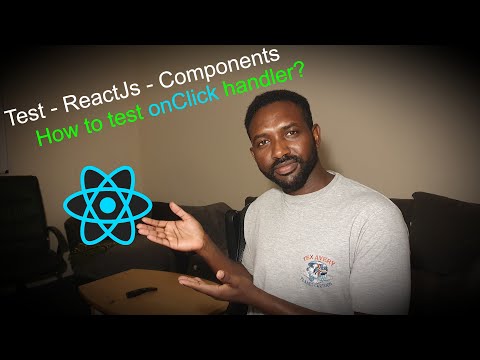
Found 14 images related to test button click react testing library theme

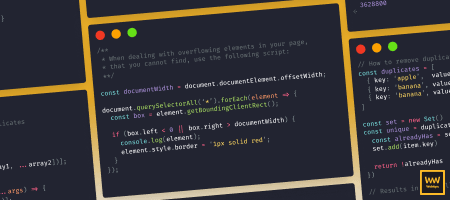

![Testing a Screen with React Native Testing Library [Live Coding] - YouTube Testing A Screen With React Native Testing Library [Live Coding] - Youtube](https://i.ytimg.com/vi/VuNPrFH9H0E/maxresdefault.jpg)
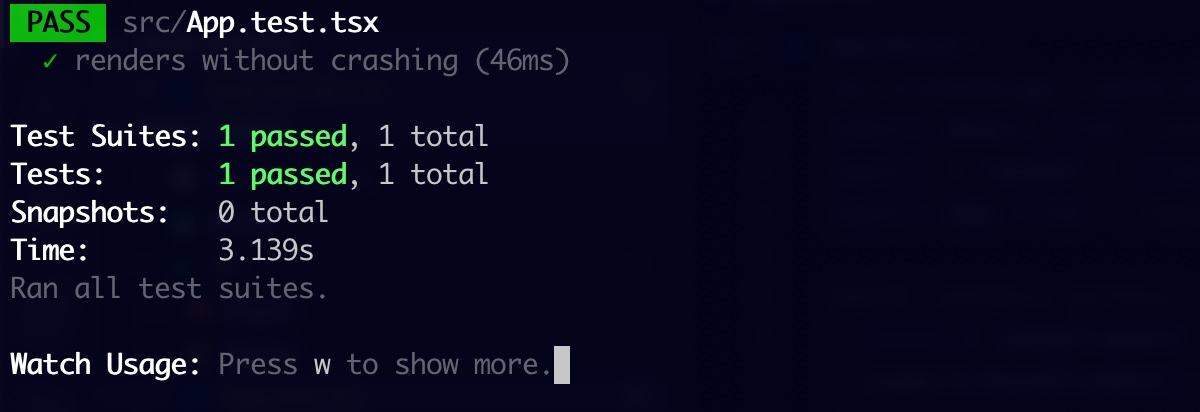


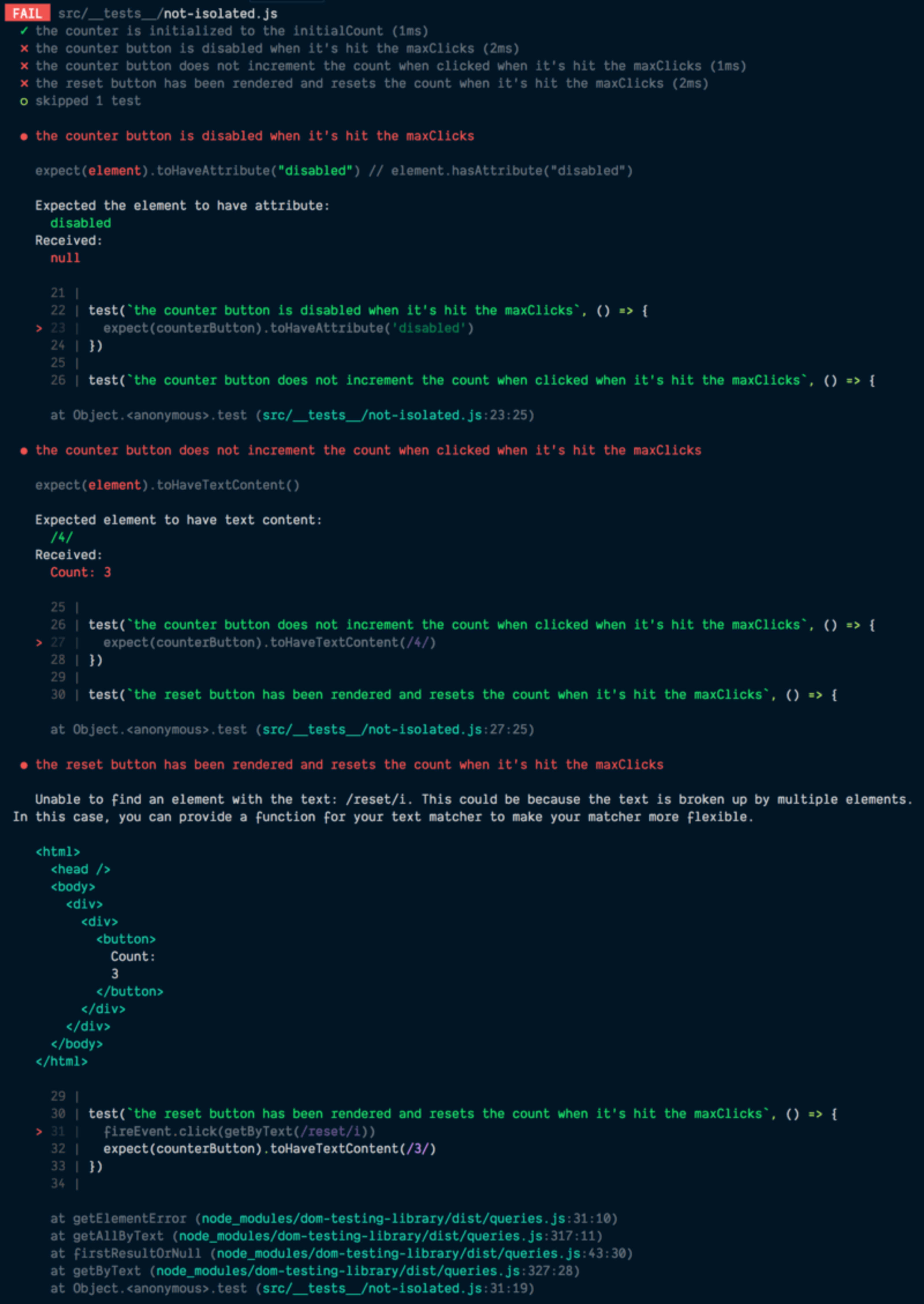
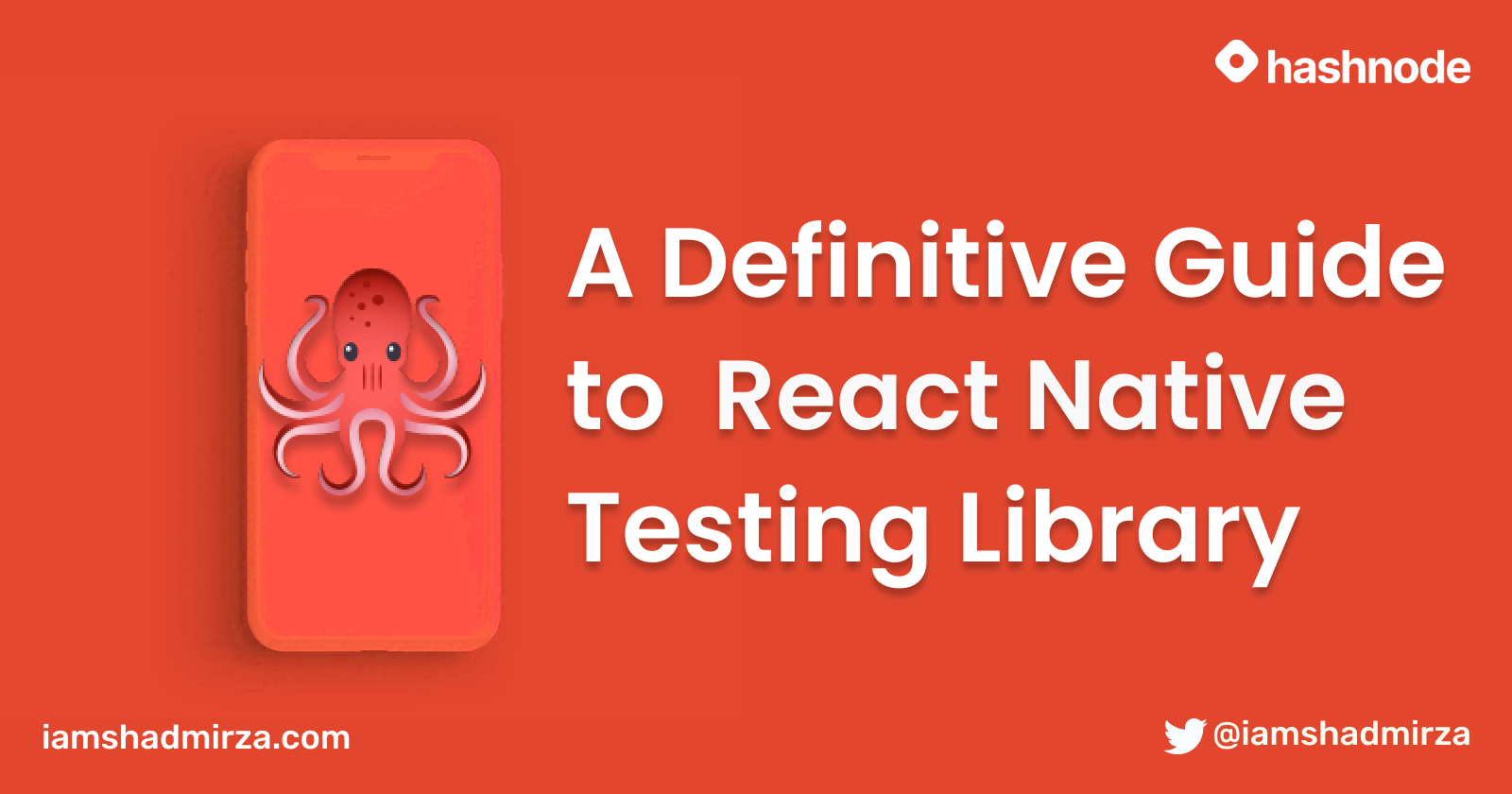
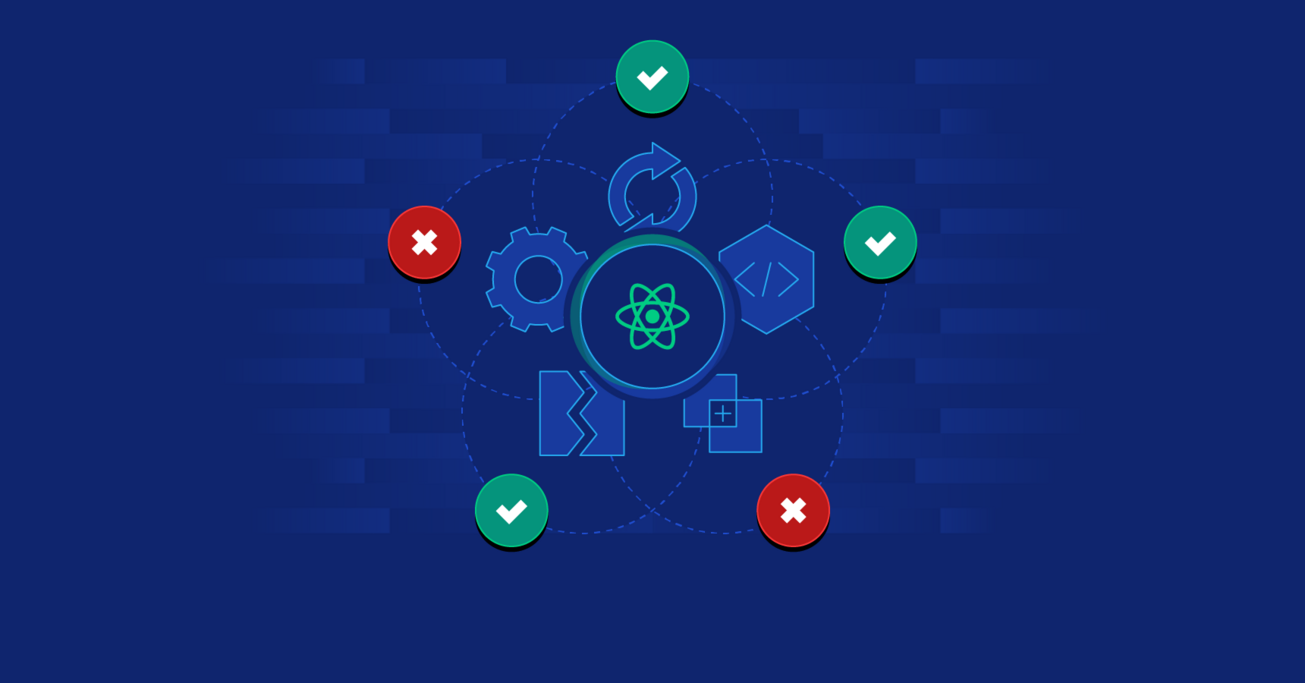
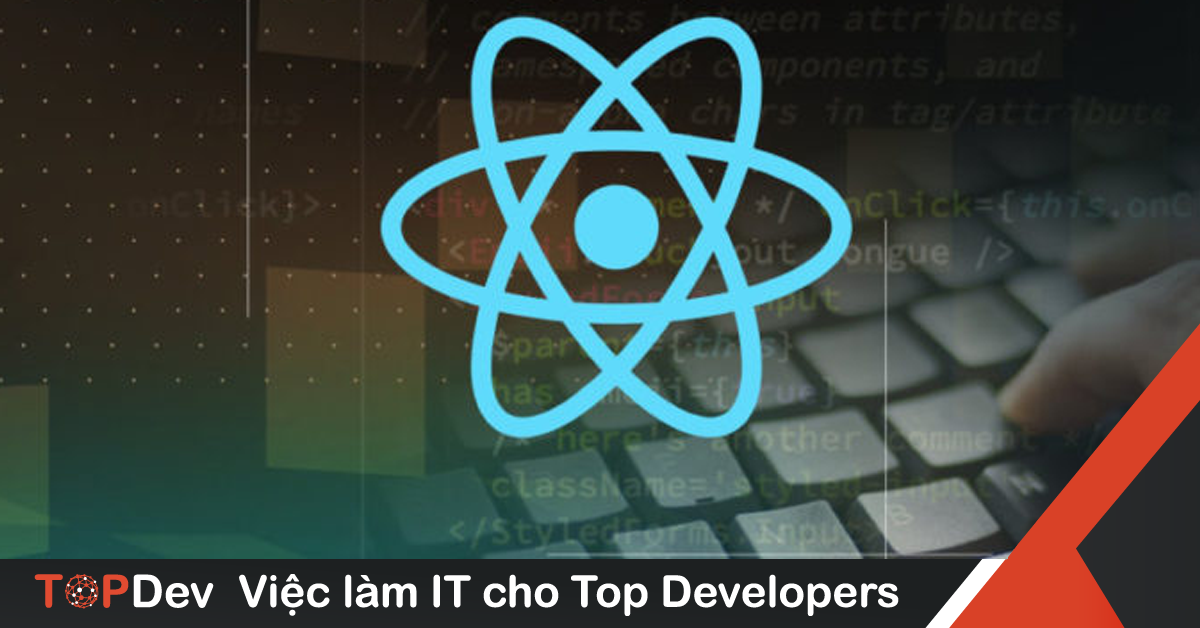
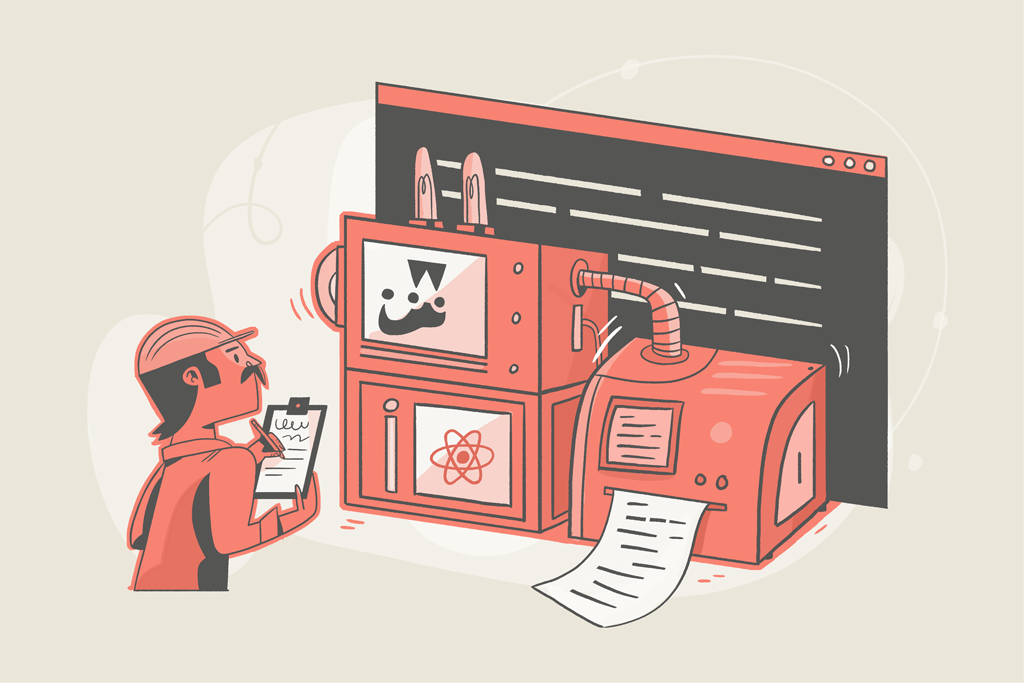
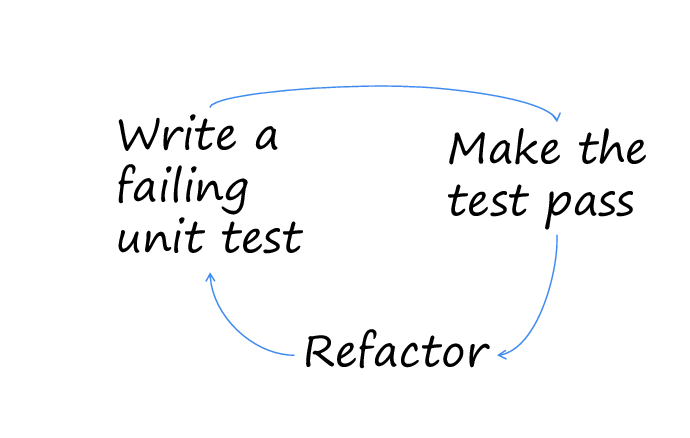
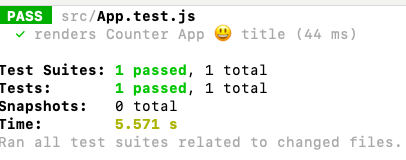
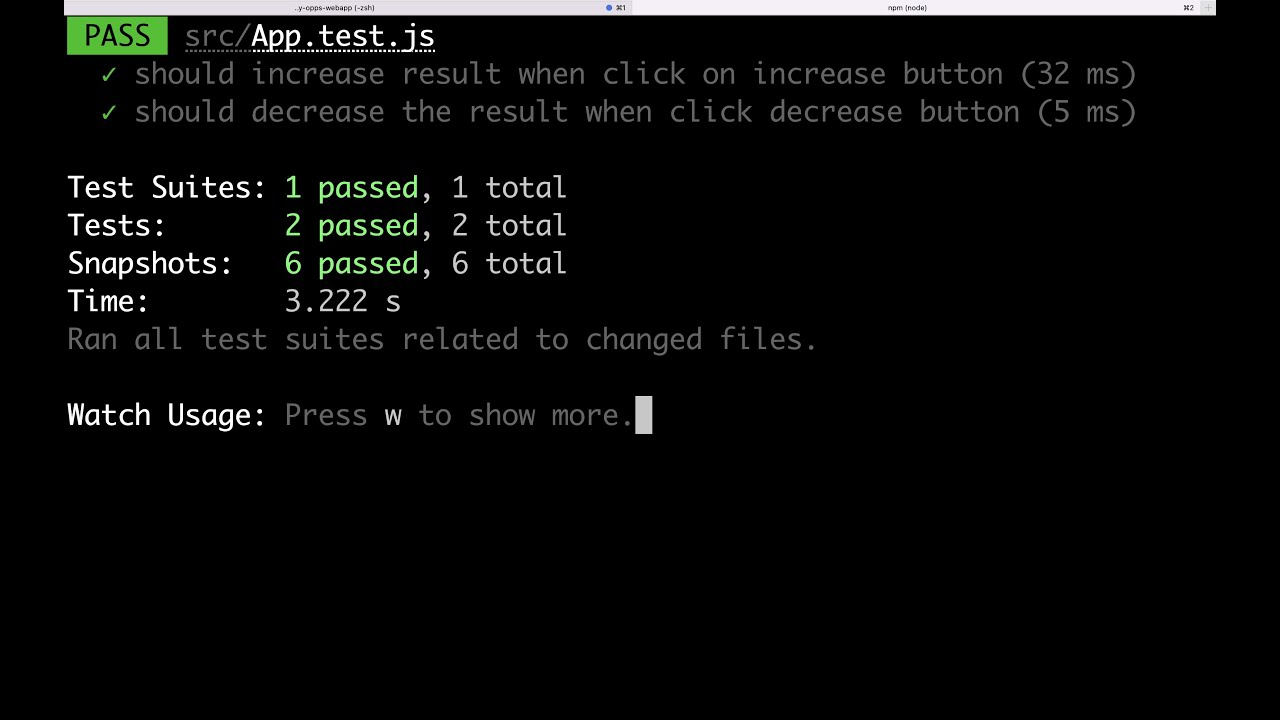
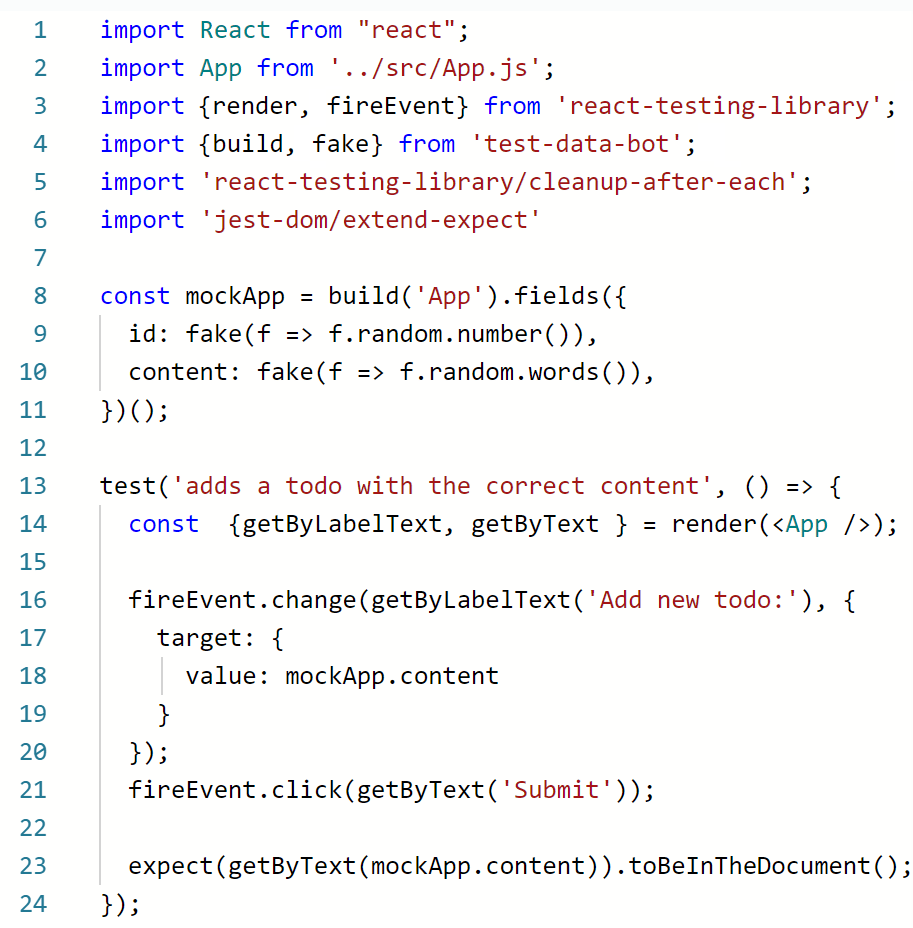

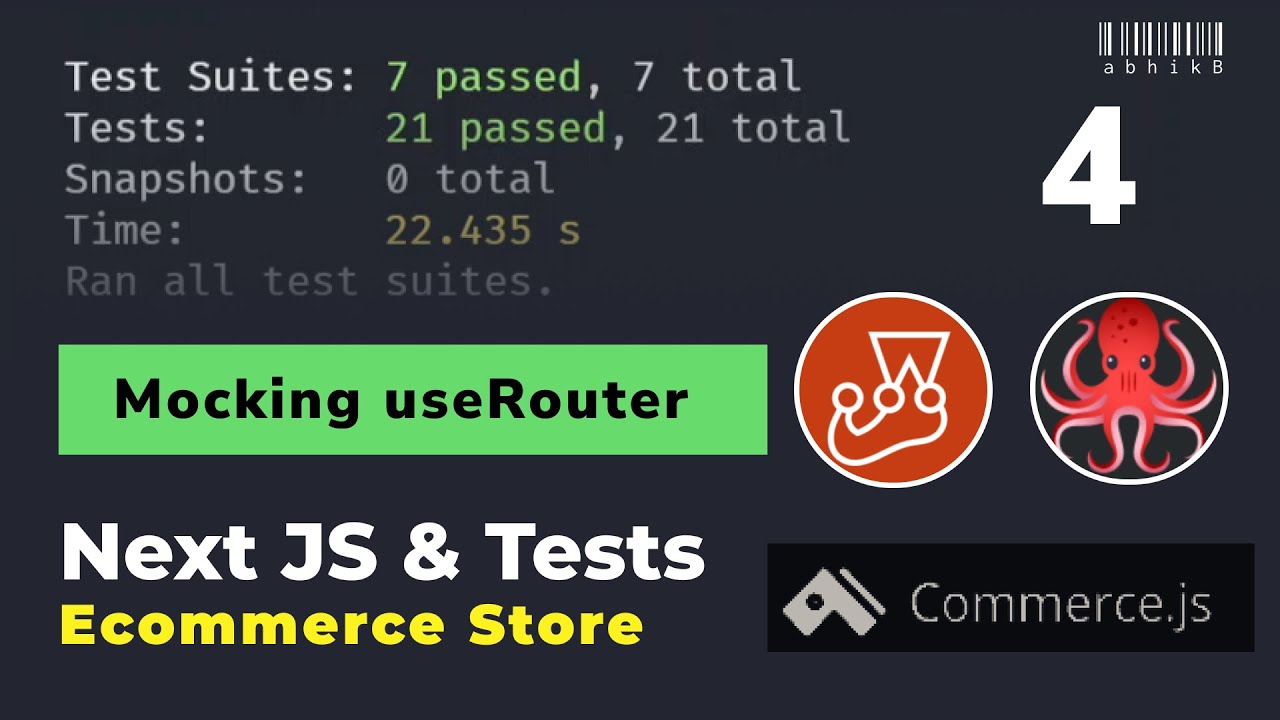
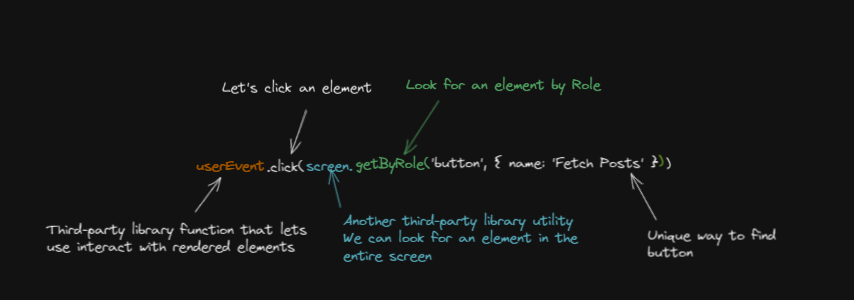
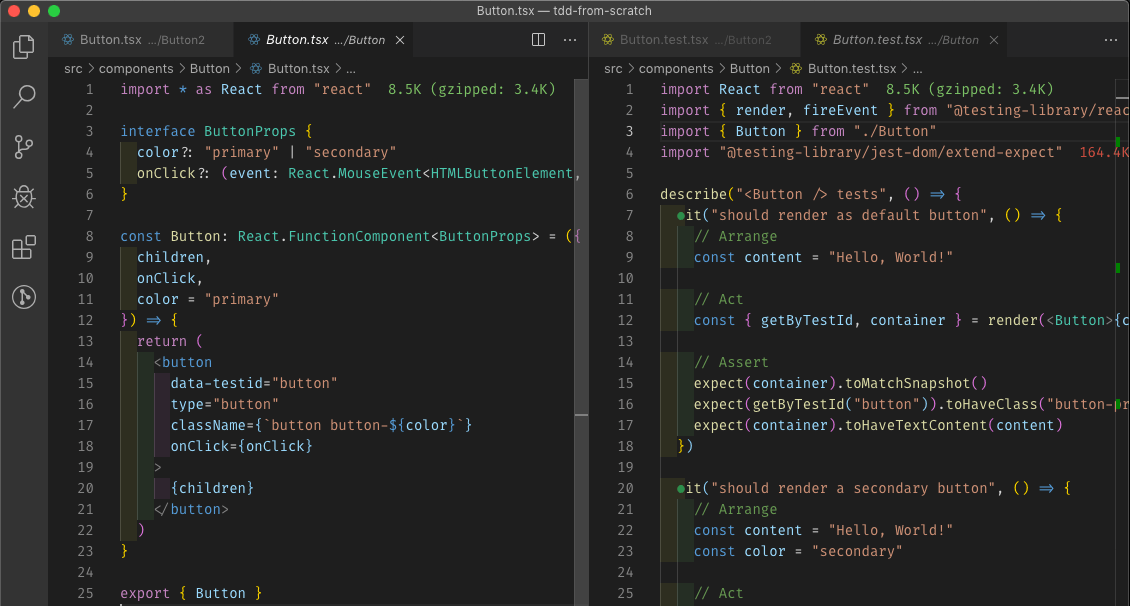
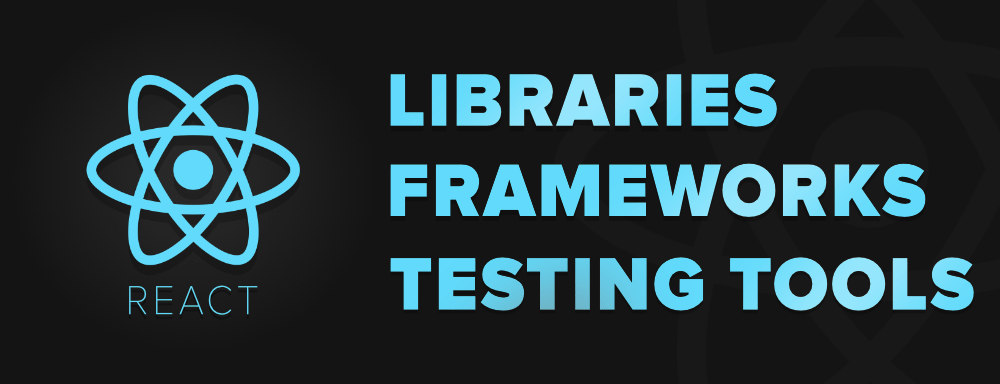
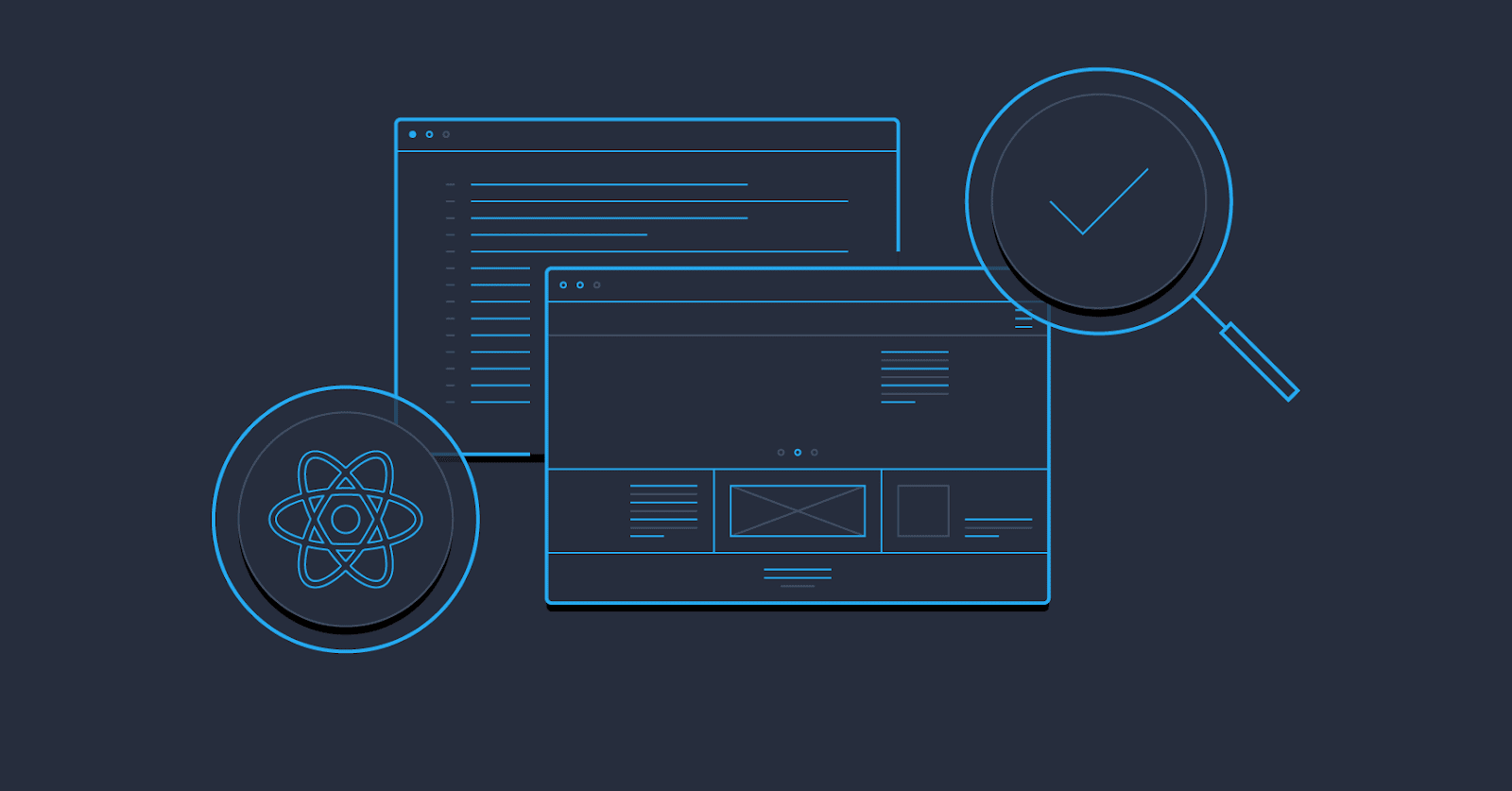


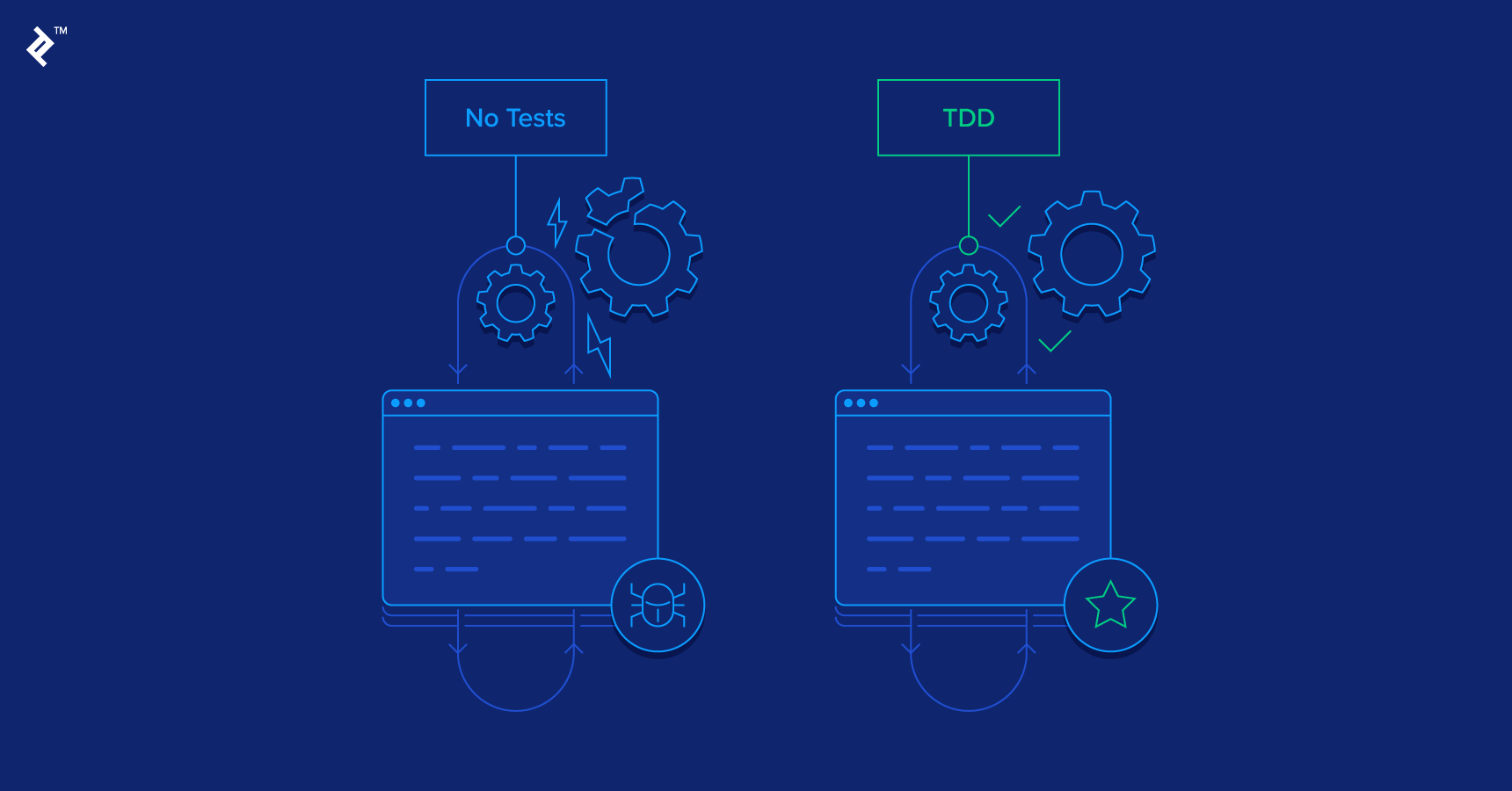
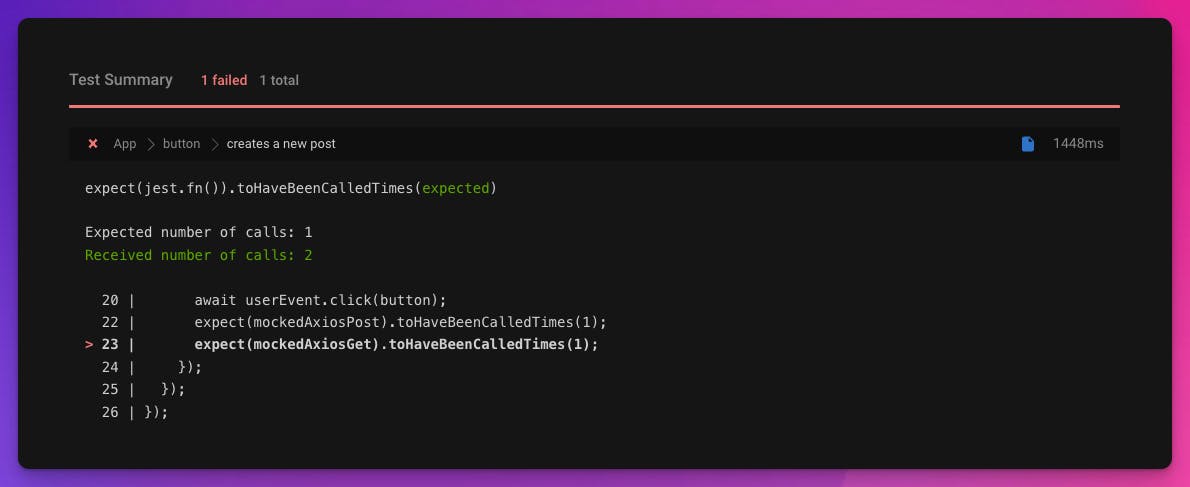
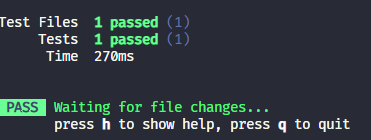
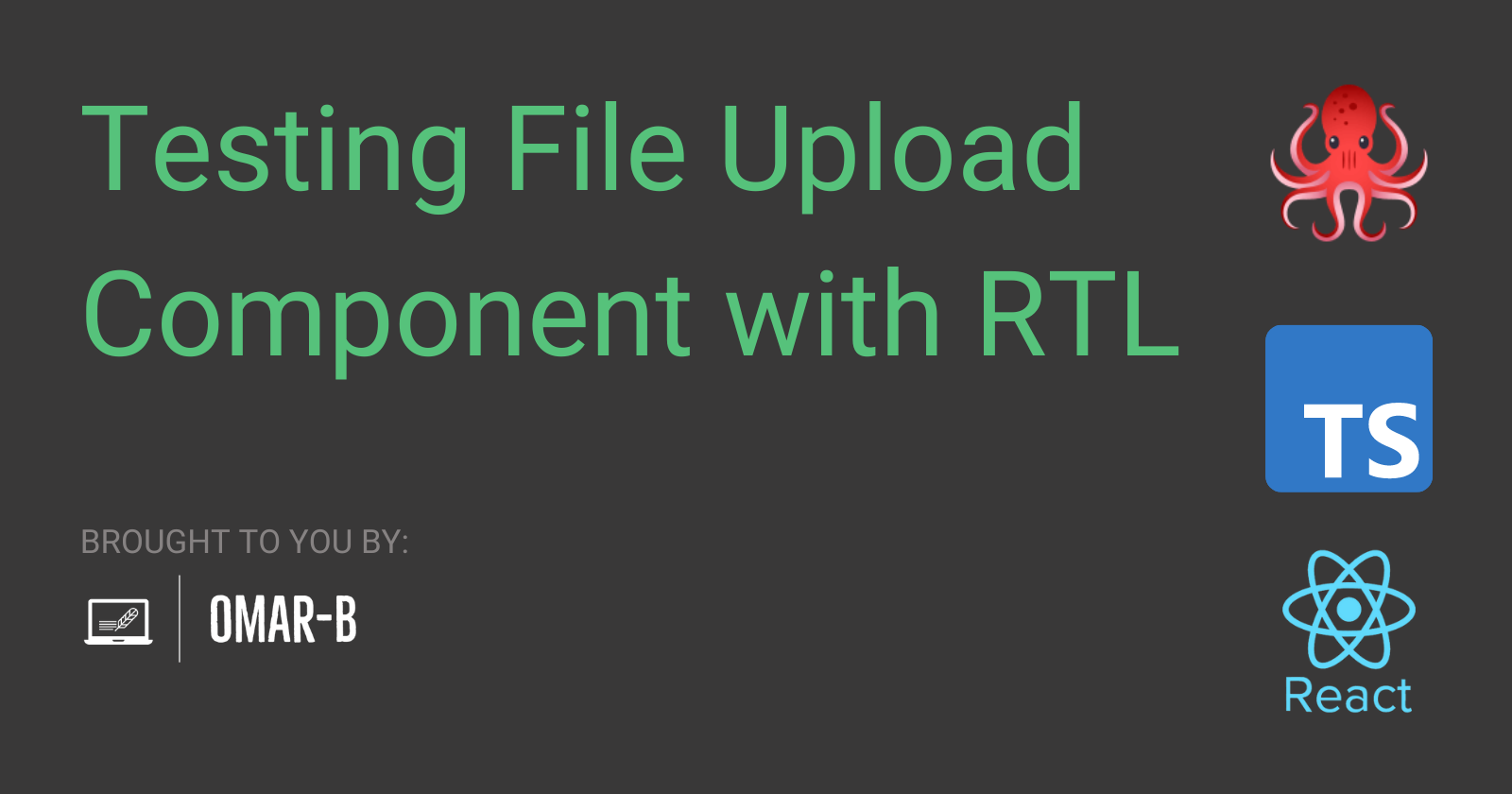
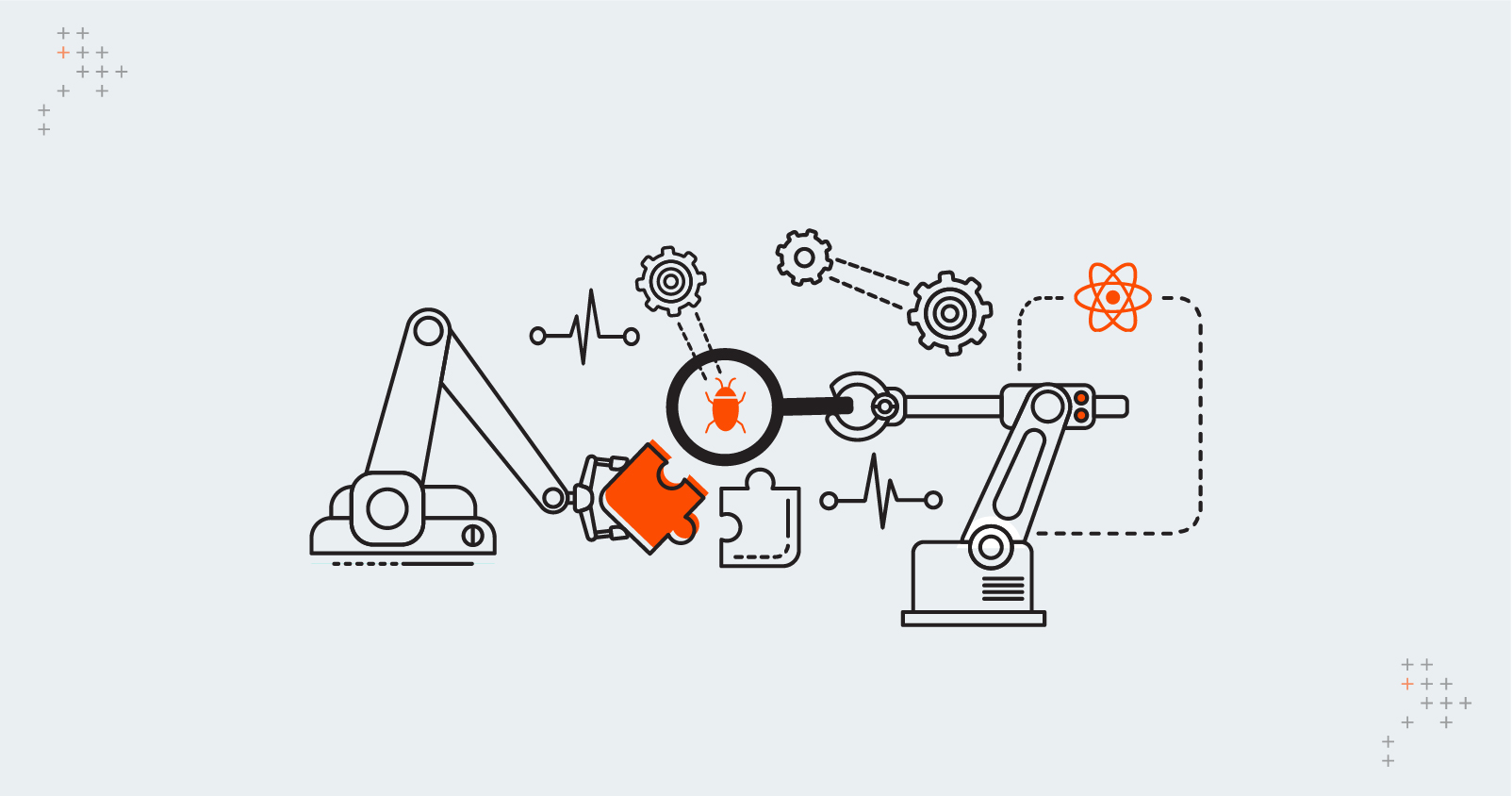
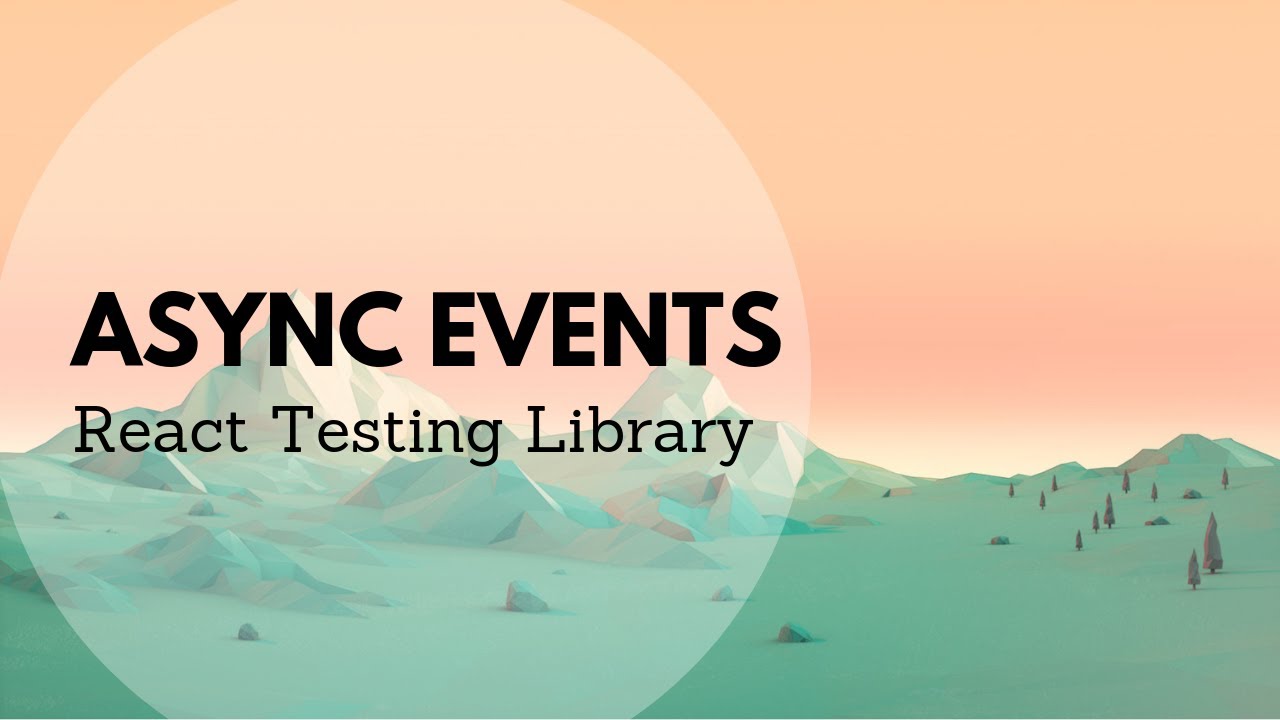
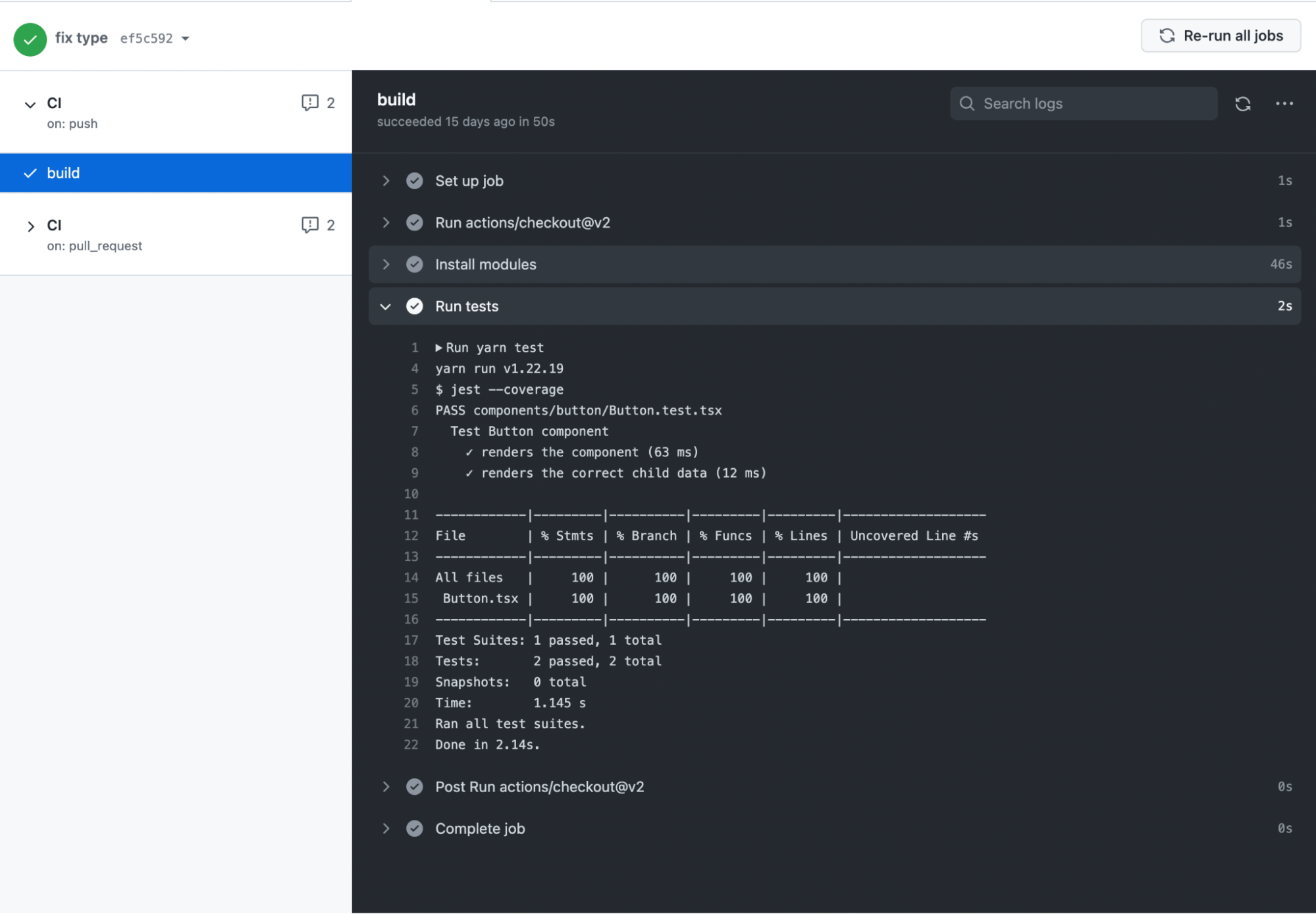
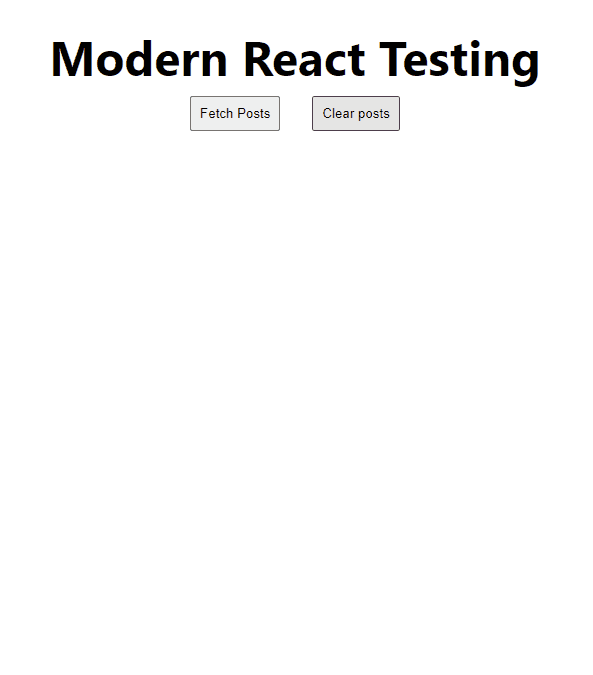
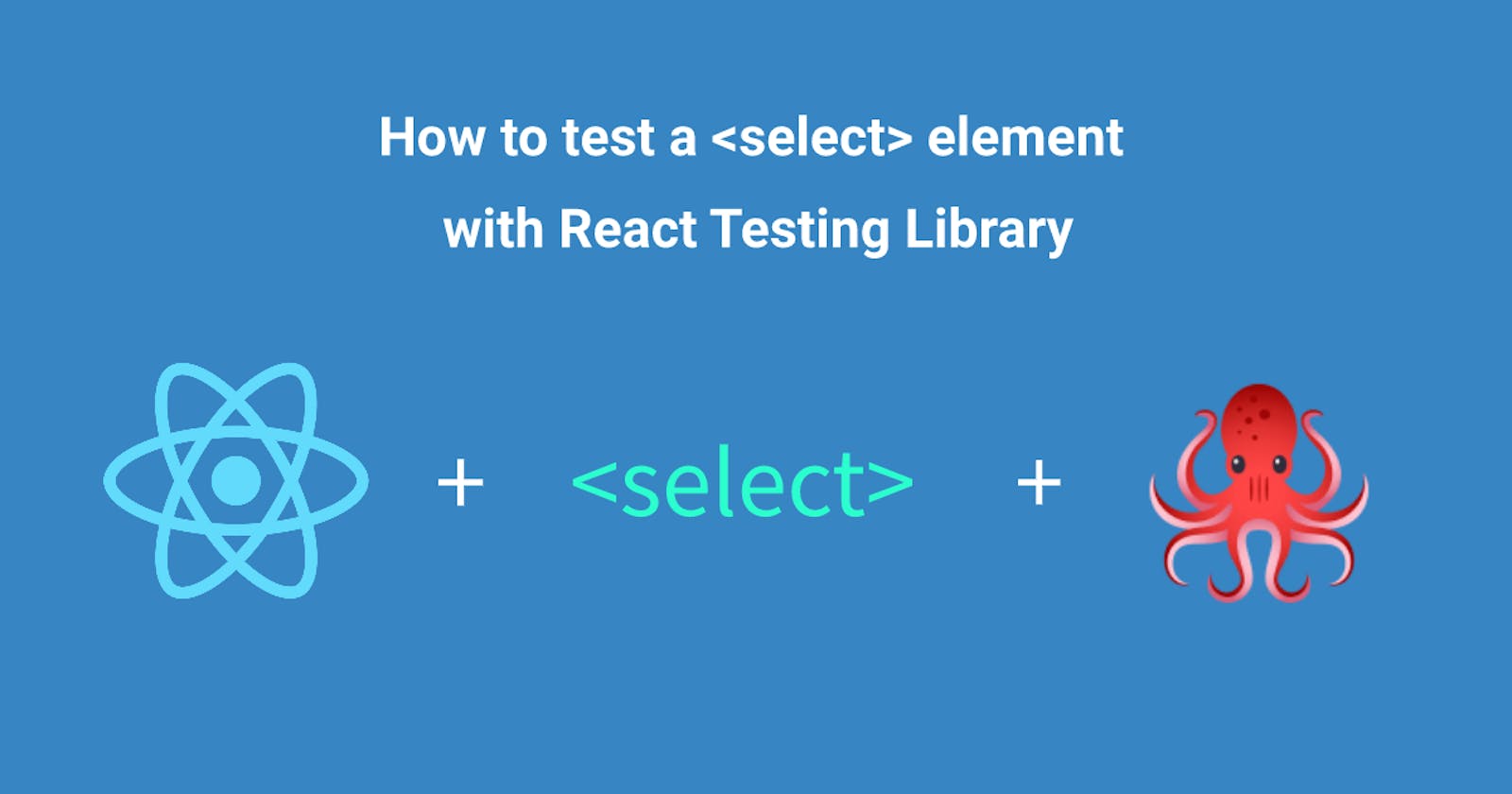
Article link: test button click react testing library.
Learn more about the topic test button click react testing library.
- Testing click event in React Testing Library – Stack Overflow
- Properly Testing Button Clicks in React Testing Library – Webtips
- How to Validate React Testing Library Click Button Events
- React Testing Library Examples
- Unit Testing with the React Testing Library – OpenReplay Blog
- Mocking an event handler – react-testing-techniques – GitHub
See more: nhanvietluanvan.com/luat-hoc