Terraform If Not Null
Terraform is an infrastructure as code (IaC) tool that enables users to define and provision infrastructure resources in a declarative manner. Among its many features, Terraform includes conditional statements and expressions that allow users to control the flow of their infrastructure provisioning based on certain conditions. One such conditional statement is the “if not null” condition, which is used to check if a value is null before performing certain actions.
Handling null values in Terraform
In Terraform, null is a special value that represents the absence of a value. Null values are often encountered when working with variables, as a variable may not always have a defined value. Handling null values correctly is crucial for ensuring the reliability and stability of the infrastructure being provisioned.
To handle null values in Terraform, users can employ conditional statements and expressions to check if a variable is null before performing any actions or operations on it. This helps prevent errors and ensures that only valid and non-null values are utilized in the infrastructure provisioning process.
Conditional statements and expressions in Terraform
Terraform provides users with a range of conditional statements and expressions that can be used to control the flow of their infrastructure provisioning. These conditionals allow users to check for specific conditions and execute actions accordingly.
Some of the commonly used conditional statements and expressions in Terraform include if, else if, else, and `!= null`. The `!= null` expression, specifically, is used to check if a value is not null. This expression is often combined with other conditionals to create more complex conditional logic in Terraform.
Using “if not null” to conditionally perform actions in Terraform
The “if not null” condition in Terraform is used to conditionally perform actions or operations based on the presence or absence of a value. It can be particularly useful in scenarios where certain resources or configurations need to be created or updated only if a variable is not null.
For example, consider a situation where a variable represents the IP address of a resource. Using the “if not null” condition, you can check if the variable is null before creating or updating the resource. If the IP address is present, the resource will be created or updated; otherwise, no action will be taken.
Best practices for using “if not null” in Terraform
When using the “if not null” condition in Terraform, there are some best practices to follow:
1. Consistent variable naming: Use descriptive and consistent variable names to improve code readability and maintainability.
2. Validate inputs: Before executing your Terraform code, validate the inputs to ensure that null values are properly handled and that the desired behavior is achieved.
3. Use default values: Provide default values for variables wherever possible. This helps prevent null values and ensures that the infrastructure provisioning process can proceed even if certain variables are not explicitly set.
4. Document your code: Clearly document the purpose and behavior of your code, especially when using complex conditional logic. This helps improve understanding and facilitates maintenance.
Common pitfalls and how to avoid them when using “if not null” in Terraform
While using the “if not null” condition in Terraform can greatly enhance the flexibility and control over infrastructure provisioning, there are some common pitfalls to be aware of:
1. Not checking for null values: Failing to check for null values before performing actions can lead to unexpected errors or undesired outcomes. Always ensure that the variable you are working with is not null before proceeding with any operations.
2. Improper handling of null values: It is important to handle null values appropriately based on the specific requirements of your infrastructure. This may involve setting default values, skipping actions, or providing alternative configurations when null values are encountered.
3. Overcomplicating conditional logic: Avoid overcomplicating your conditional logic by nesting multiple “if not null” conditions or using excessive conditionals. Keep your code simple, readable, and maintainable to avoid unnecessary complexity.
4. Lack of documentation: Failing to document the use of “if not null” conditions and other conditional statements can make it difficult for other team members to understand and maintain the code. Document your code thoroughly to ensure clarity and collaboration.
FAQs
Q: Can Terraform create a resource if a variable is not null?
A: Yes, Terraform can create or update a resource based on the conditionality of a variable using the “if not null” condition.
Q: How can I check if a variable is null in Terraform?
A: To check if a variable is null in Terraform, use the expression `${variable != null}`. This will return true if the variable is not null and false if it is null.
Q: What is the best way to create a resource conditionally using “if else” in Terraform?
A: To create a resource conditionally using “if else” in Terraform, you can use the `condition ? true_value : false_value` syntax. For example, `resource “example” “example_resource” { count = var.condition ? 1 : 0 }` would create the example_resource if var.condition is true.
Q: Why can’t I use a null value as the collection in a for expression in Terraform?
A: Null values cannot be used as the collection in a for expression in Terraform because a null collection does not have any elements to iterate over.
Q: How can I check if a string is null or empty in Terraform?
A: To check if a string is null or empty in Terraform, use the expression `${var.string_var != null && var.string_var != “”}`. This will return true if the string is not null or empty, and false otherwise.
Q: What is the purpose of the `condition` argument in Terraform resources?
A: The `condition` argument in Terraform resources allows users to conditionally create or update the resource based on the value of a variable. This helps in dynamically controlling the provisioning of infrastructure.
Q: How can I make my Terraform configurations dynamic?
A: Terraform configurations can be made dynamic by using variables, conditional statements, and expressions. By leveraging these features, you can dynamically adjust your infrastructure provisioning based on different conditions or inputs.
Q: How can I iterate over a collection of resources in Terraform using “for_each” if the collection is not null?
A: To iterate over a collection of resources in Terraform using “for_each” only if the collection is not null, you can utilize the “if not null” condition. By checking if the collection variable is not null before using it in the “for_each” argument, you can ensure that the iteration only occurs when the collection is present.
In conclusion, the “if not null” condition in Terraform provides users with a powerful tool for handling null values and performing actions conditionally in infrastructure provisioning. By following best practices and avoiding common pitfalls, users can effectively utilize this feature to create reliable and dynamic infrastructure configurations.
What Is Terraform Null_Resource And How To Use It? – Part-21
What Is The Default If Variable Is Null In Terraform?
When working with Terraform, it is essential to handle null values for variables effectively. In some scenarios, variables might not have any assigned value, and this can lead to unpredictable behavior. To overcome this challenge, Terraform provides the concept of default values for variables that are null.
Understanding Null Values in Terraform
In Terraform, null represents the absence of a value. It is used to indicate that a variable does not have an assigned value or has been intentionally set as null. Using null variables can come in handy when you want to provide conditional logic or handle empty values.
What Happens When a Variable is Null?
By default, if a variable is null, Terraform treats it as if it were not set and applies no value to the corresponding attribute. Consequently, this can lead to potential errors when the null variable is used in resource configurations. Terraform will not automatically assign default values or consider any preset values for null variables.
Default Value Feature in Terraform
To mitigate the undesired effects of null variables, Terraform introduced a default value feature. With this feature, you can specify a default value for a variable, which will be used in case the variable is null or not set. The default value can be any valid expression that evaluates to the desired value for the variable.
Applying Default Values in Terraform
To apply default values to variables in Terraform, you can use the default attribute when declaring the variables in your Terraform code. The default attribute allows you to specify the default value for the variable. Here’s an example of how to use the default attribute:
“`terraform
variable “example_variable” {
type = string
default = “default_value”
}
“`
In the above example, the variable “example_variable” is assigned the default value “default_value”. If this variable is not explicitly set or is null, Terraform will automatically assign the default value to it.
Handling Null Values Based on Default Values
It is crucial to handle null values effectively when default values are assigned to variables. To handle null values based on default values, you can make use of the coalescing operator. The coalescing operator (??) is a conditional operator that returns the value on its left if it is not null, or the value on its right if the left value is null.
Here’s an example of using the coalescing operator to handle null values:
“`terraform
variable “example_variable” {
type = string
default = “default_value”
}
resource “example_resource” “example” {
attribute = var.example_variable ?? var.example_variable_default
}
“`
In the above example, the attribute “example_variable” of the resource “example_resource” is assigned the value of “var.example_variable” if it is not null (or explicitly set), and the value of “var.example_variable_default” if it is null.
FAQs
Q1. Can I assign a default value to a variable of any type in Terraform?
Yes, Terraform allows you to assign default values to variables of any type. The default value provided should correspond to the type of the variable.
Q2. What happens if both the default attribute and a value are set for a variable in Terraform?
If both a value and a default attribute are set for a variable, the value takes precedence over the default attribute. Terraform will use the explicitly set value and ignore the default attribute in such cases.
Q3. Can I assign a null value as a default for a variable in Terraform?
Yes, you can assign a null value as a default for a variable in Terraform. This can be useful when you want to handle null values differently based on specific conditions.
Q4. Can I refer to other variables when assigning a default value in Terraform?
Yes, you can refer to other variables when assigning a default value in Terraform. This allows you to define default values based on the values of other variables.
Q5. What happens if a null variable is used in a resource configuration without a default value in Terraform?
If a null variable is used in a resource configuration without a default value, Terraform will not automatically assign any value to the corresponding attribute. This can lead to potential errors or unexpected behavior in your infrastructure.
In conclusion, handling null values in Terraform is crucial for maintaining predictable and error-free infrastructure. By utilizing the default value feature, you can ensure that variables have meaningful values even when they are null. Taking advantage of default values and properly handling null variables will significantly enhance the robustness of your Terraform deployments.
What Is Null Value In Terraform?
Terraform is an open-source infrastructure-as-code tool that allows developers to define, manage, and provision infrastructure resources in a declarative manner. With Terraform, you can easily describe your infrastructure requirements using a domain-specific language (DSL) called HCL (HashiCorp Configuration Language) and deploy resources across various cloud providers. One crucial aspect of working with Terraform is understanding the concept of a null value.
In programming, a null value represents the absence of a value or an empty state. It is commonly used to indicate the lack of a meaningful or valid value. Similarly, in Terraform, a null value serves a similar purpose. It allows you to define a placeholder value that can be used when you need to specify an attribute without providing an actual value.
Null values are particularly useful in scenarios where you have optional or conditional attributes. By using null values, you can explicitly set an attribute to a non-value, indicating that it should not be considered or used. This allows you to define a more flexible and dynamic infrastructure configuration.
In Terraform, null is a built-in constant that you can use to represent a null value. You can assign null to any attribute or variable in your Terraform code. For example, consider a scenario where you want to define an optional tag for an AWS resource:
“`
resource “aws_instance” “example” {
ami = “ami-0c94855ba95c71c99”
instance_type = “t2.micro”
tags = var.tag_name != null ? {
Name = var.tag_name
} : null
}
“`
In this example, the tags attribute is conditionally set based on the value of the tag_name variable. If the tag_name variable is not null, the resource will be tagged with a name. Otherwise, the tags attribute will be set to null, indicating that no tags should be assigned.
Null values can also be useful when working with nested blocks or complex data structures. For instance, let’s say you are defining an Azure virtual machine resource and want to conditionally specify a data disk:
“`
resource “azurerm_virtual_machine” “example” {
name = “example-vm”
location = “eastus”
resource_group_name = “example-rg”
vm_size = “Standard_DS1_v2”
storage_data_disk {
count = var.include_data_disk ? 1 : 0
caching = var.include_data_disk ? “ReadWrite” : null
create_option = var.include_data_disk ? “Empty” : null
disk_size_gb = var.include_data_disk ? 100 : null
}
}
“`
In this example, the storage_data_disk block is conditionally set based on the value of the include_data_disk variable. If include_data_disk is true, a data disk will be included with specific attributes such as caching, create_option, and disk_size_gb. Otherwise, these attributes will be set to null, indicating that no data disk should be included.
FAQs:
1. What is the purpose of using null values in Terraform?
Null values allow you to define optional or conditional attributes in your Terraform code. They serve as placeholders for attributes that either do not have a value or should not be considered.
2. Can null values be assigned to variables in Terraform?
Yes, you can assign null values to variables in Terraform. This is particularly useful when you want to provide default values to variables but allow them to be explicitly set to null.
3. How are null values used in conditional expressions?
Null values can be used in conditional expressions to dynamically determine whether an attribute should be set to a specific value or null. This allows for more flexible and dynamic configurations.
4. Are there any limitations or considerations when using null values in Terraform?
It’s important to note that not all resources or providers handle null values in the same way. Some resources may have specific requirements or limitations when it comes to null values, so it’s essential to consult the documentation for the specific resource or provider you are working with.
5. Can null values be used in Terraform outputs?
Yes, null values can be used in Terraform outputs. For example, if you have an optional output, you can set its value to null if the condition for its generation is not met.
In conclusion, null values in Terraform provide a powerful mechanism for defining optional and conditional attributes in your infrastructure code. By using null values, you can create more flexible and dynamic configurations that adapt to different scenarios. Understanding the concept of null values and how to use them effectively will empower you to build more robust and adaptable infrastructure deployments with Terraform.
Keywords searched by users: terraform if not null Terraform create resource if variable is not null, Terraform check null, Terraform if else create resource, A null value cannot be used as the collection in a for expression terraform, Terraform check if string is null or empty, Terraform Condition, Terraform dynamic, For_each Terraform
Categories: Top 13 Terraform If Not Null
See more here: nhanvietluanvan.com
Terraform Create Resource If Variable Is Not Null
Terraform’s conditional resource creation feature allows you to specify that a resource should be created only if a certain variable or expression is not null. This is achieved by using the `count` meta-argument within the resource block and giving it a value based on a conditional statement.
Here’s an example of how this can be done:
“`hcl
resource “aws_instance” “example” {
count = var.instance_type != null ? 1 : 0
instance_type = var.instance_type
# …
}
“`
In this example, the `aws_instance` resource will only be created if the variable `instance_type` is not null. If the variable is indeed null, then the count will be set to 0, effectively preventing the creation of the resource. However, if the variable has a value, the count will be set to 1, and Terraform will proceed with creating the resource.
This conditional resource creation feature can be extremely useful in various scenarios. Suppose you have a Terraform module that provisions multiple resources, but due to custom requirements, you need to create a specific resource conditionally. For instance, in an AWS infrastructure deployment, you might want to create an additional security group only if a specific variable, such as `enable_extra_security_group`, is set to true.
By leveraging the “create resource if variable is not null” functionality, you can easily handle such cases without the need to duplicate your Terraform code or create separate modules. This not only simplifies your infrastructure setup but also contributes to maintainability and reduces code duplication, making your infrastructure deployments more scalable and efficient.
Additionally, Terraform’s conditional resource creation allows you to control costs by dynamically creating resources only when they are required. This feature is particularly beneficial when dealing with cloud providers that charge based on the number of resources provisioned. By conditionally creating resources, you can optimize your infrastructure and avoid unnecessary expenses.
Moreover, the conditional resource creation feature can be combined with other Terraform functionalities. For example, you can perform complex conditional checks using the Terraform `map` data structure and `for_each` meta-argument. This combination enables you to iterate over a set of variables and dynamically create resources based on their values.
With the basics of conditional resource creation covered, let’s address some frequently asked questions about this feature:
Q: Can I use a variable other than `count` to conditionally create resources?
A: Yes, you can use any variable or expression that evaluates to a boolean value. The `count` meta-argument is commonly used for this purpose, but you can also use `for_each`, which allows more fine-grained control.
Q: What happens if I set the count to a negative number?
A: Terraform interprets a negative count value as 0, meaning no resources will be created.
Q: Can I use this feature with other resource provisioning tools besides Terraform?
A: No, this feature is specific to Terraform and its declarative configuration language.
Q: Are there any limitations to consider?
A: While conditional resource creation is powerful, it’s important to note that it should not be overused. Overly complex or convoluted conditional expressions can make your code harder to understand and maintain. Use this feature judiciously, keeping the codebase clean and manageable.
Q: Can I use this feature with other resource types, not just AWS instances?
A: Absolutely! The conditional resource creation feature is available for all resource types supported by Terraform, regardless of the cloud provider.
In conclusion, Terraform’s “create resource if variable is not null” feature empowers you to conditionally create and manage resources based on the values of variables or expressions. By selectively creating resources, you can optimize infrastructure provisioning and reduce costs. This flexibility, coupled with Terraform’s wide range of capabilities, makes it an invaluable tool for managing cloud resources in a scalable and efficient manner.
Terraform Check Null
Introduction:
Terraform, an open-source infrastructure automation tool, allows developers to define and provision infrastructure resources through declarative code. It provides a simplified and efficient approach to managing cloud infrastructure by representing configuration files detailing the desired state of resources. One common challenge faced by Terraform users is handling null values within their configurations. This article aims to delve deeper into the topic of checking null in Terraform and provide a comprehensive guide on how to effectively address this issue.
Understanding Null in Terraform:
In Terraform, null represents the absence or lack of value for a particular variable. It is commonly used to denote the absence of a default value or when a variable has not been assigned any value explicitly. Null can be encountered when working with optional or conditional attributes in resource configurations.
Checking for Null:
Terraform provides several approaches to check for null values effectively. Let’s explore them in detail:
1. Conditional Expressions:
Terraform offers conditional expressions to evaluate whether a given value is null. Commonly used syntax includes `null` or `null_resource` to check if a value is null. For example, `${var.example == null}` checks if the variable `example` is null.
2. Coalescing Operators:
Terraform also supports coalescing operators, such as the `ternary conditional operator`, to handle null values effectively. Using this operator, you can define a default value to fall back on if a variable is null. For instance, `var.example != null ? var.example : “default”` assigns the default value “default” to the variable `example` if it is null.
3. Built-in Functions:
Terraform offers built-in functions to facilitate null checks. Two commonly used functions are `coalesce` and `default`. The `coalesce` function returns the first non-null argument from a given set of arguments. On the other hand, the `default` function checks if a given value is null or unset, returning the specified default value if so. These functions are particularly useful when working with multiple variables or complex expressions.
4. Conditional Resources:
In some cases, you may want to conditionally create resources based on the presence or absence of null values. Terraform allows you to achieve this using conditional resource blocks. By wrapping a resource block within an `if` statement, you can ensure that the resource is only created if a particular condition, such as a variable being null, is met.
FAQs:
Q1. How can I check if a variable is null in Terraform?
A: You can use conditional expressions, coalescing operators, or built-in functions like `coalesce` or `default` to check if a variable is null.
Q2. Can I set a default value if a variable is null in Terraform?
A: Yes, you can use coalescing operators like the ternary conditional operator to assign a default value if a variable is null.
Q3. What if I need to check multiple variables for null values?
A: Terraform’s built-in functions such as `coalesce` and `default` are helpful in handling multiple variables and complex expressions.
Q4. How can I conditionally create resources in Terraform based on null values?
A: Terraform provides conditional resource blocks where you can wrap a resource block within an `if` statement to create resources only when a specific condition, such as a variable being null, is met.
Q5. Are there any potential issues in handling null values in Terraform?
A: While handling null values in Terraform, it is crucial to ensure consistent use of null checks and appropriate fallback values. Failing to do so might result in resource misconfigurations or unexpected behavior.
Conclusion:
Checking null values in Terraform configurations is an essential skill for developers and infrastructure teams working with the tool. By utilizing conditional expressions, coalescing operators, built-in functions, and conditional resource blocks, users can effectively handle null values and ensure smooth provisioning of infrastructure resources. By following the guidelines outlined in this article, developers can overcome the challenges associated with null values while utilizing Terraform for infrastructure automation.
Images related to the topic terraform if not null
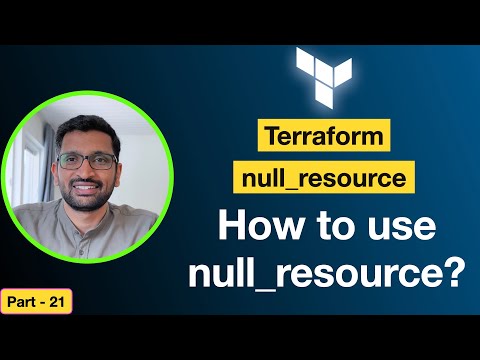
Found 38 images related to terraform if not null theme
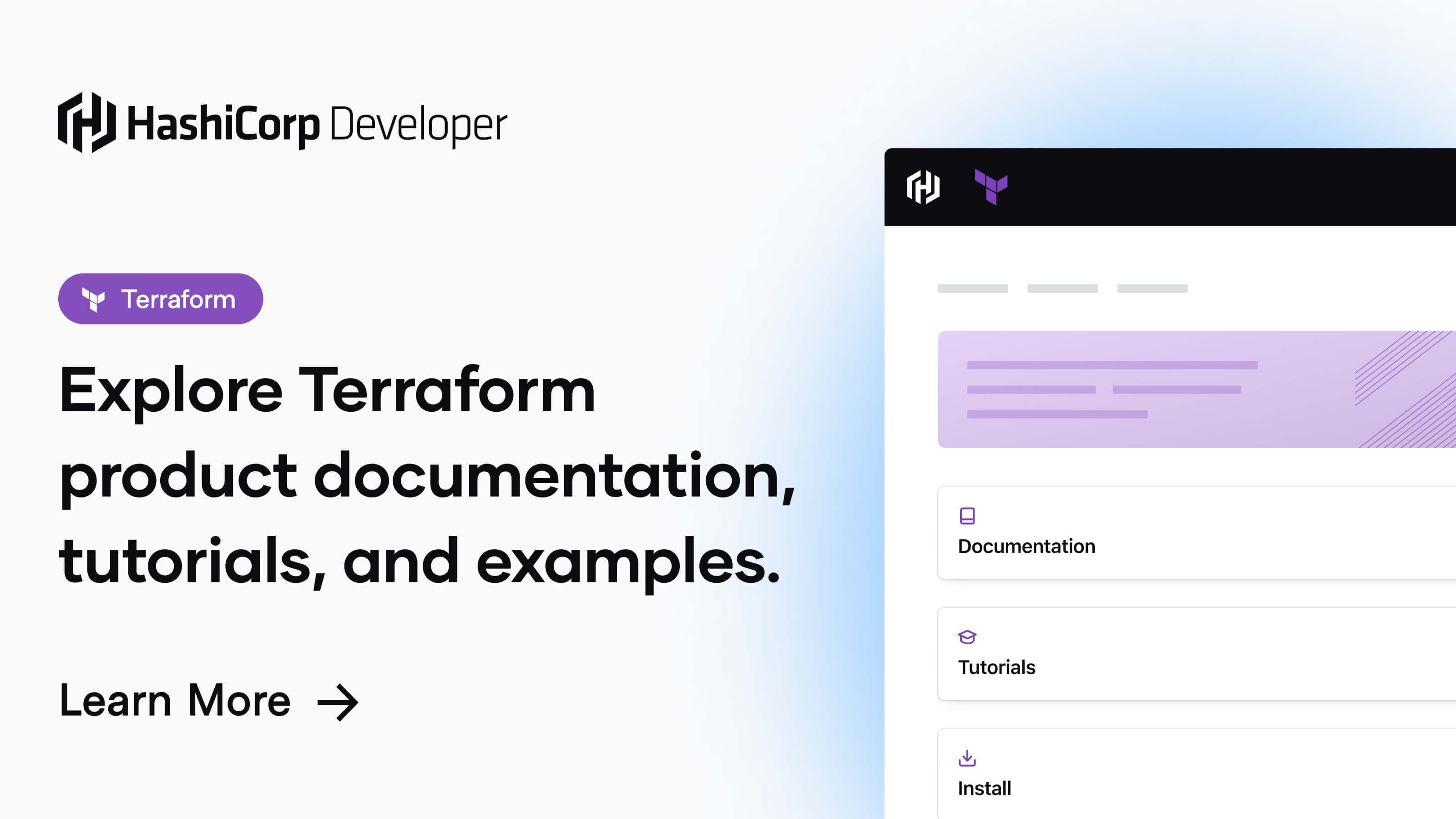
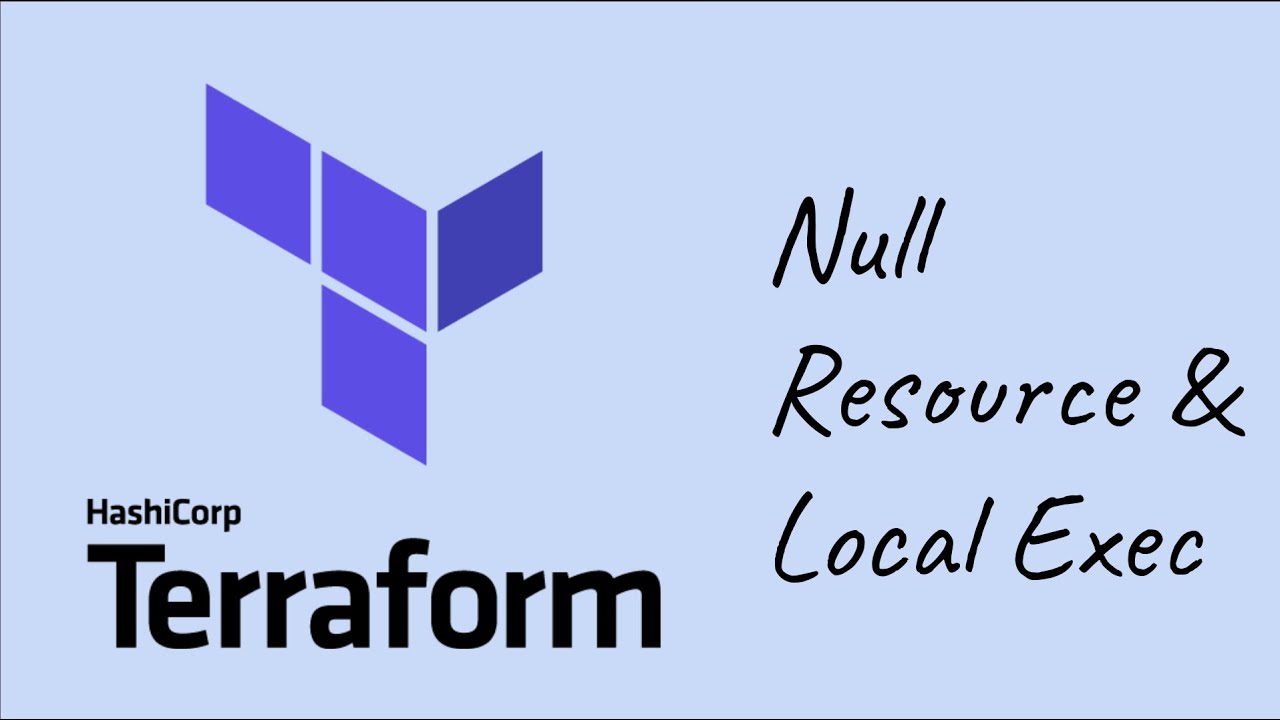




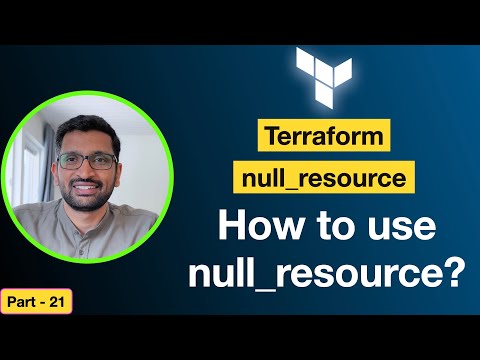
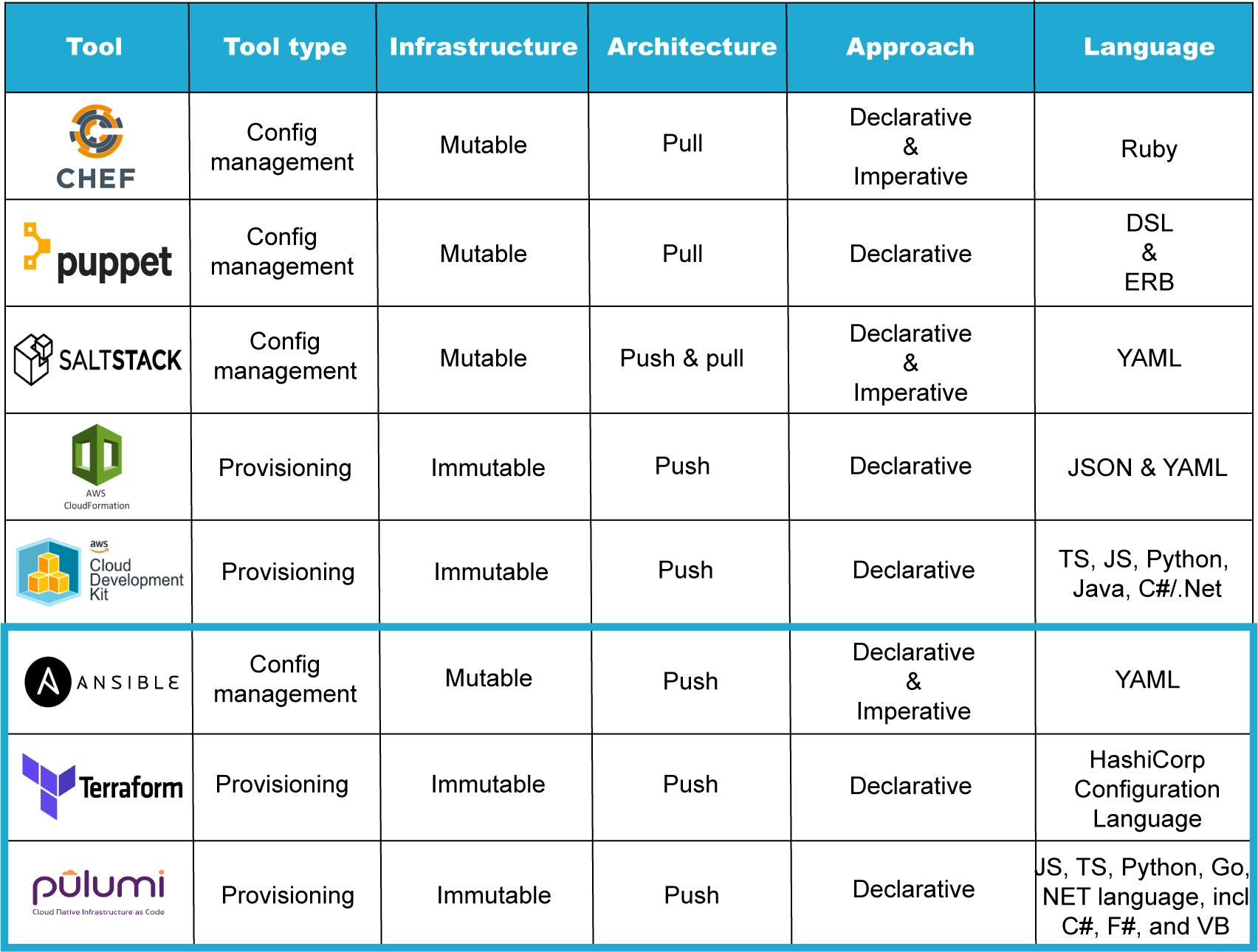
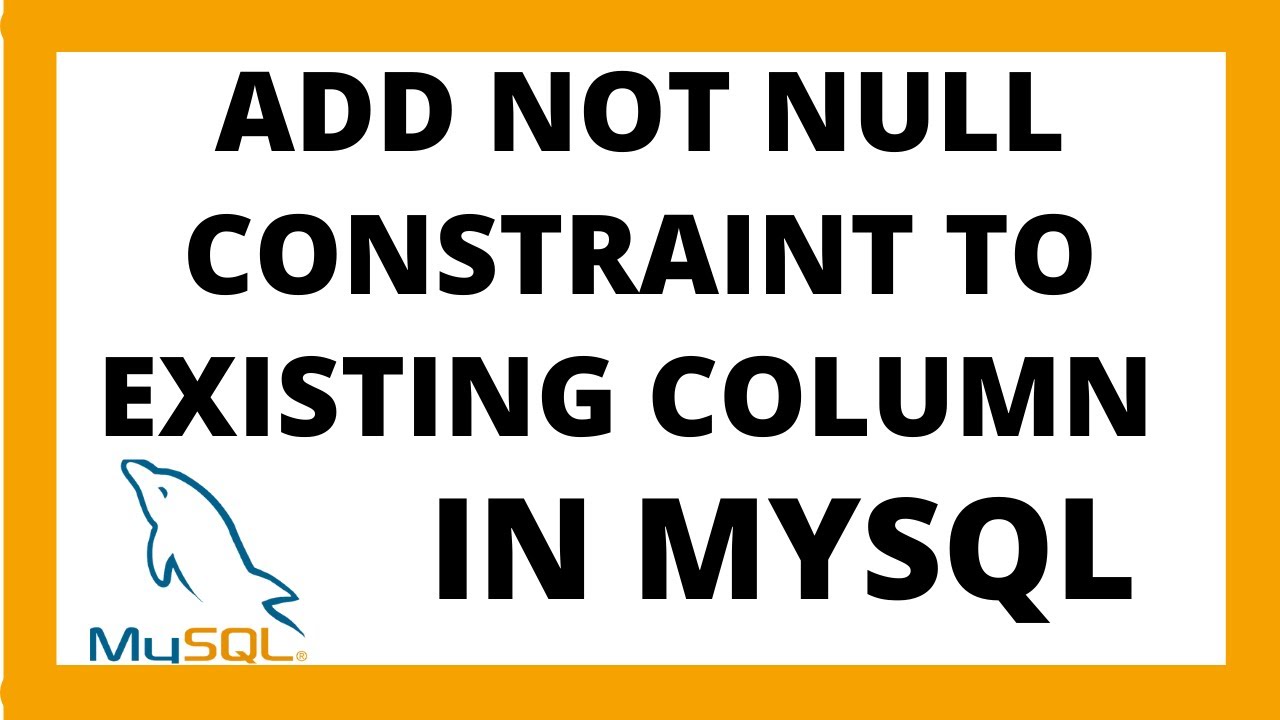





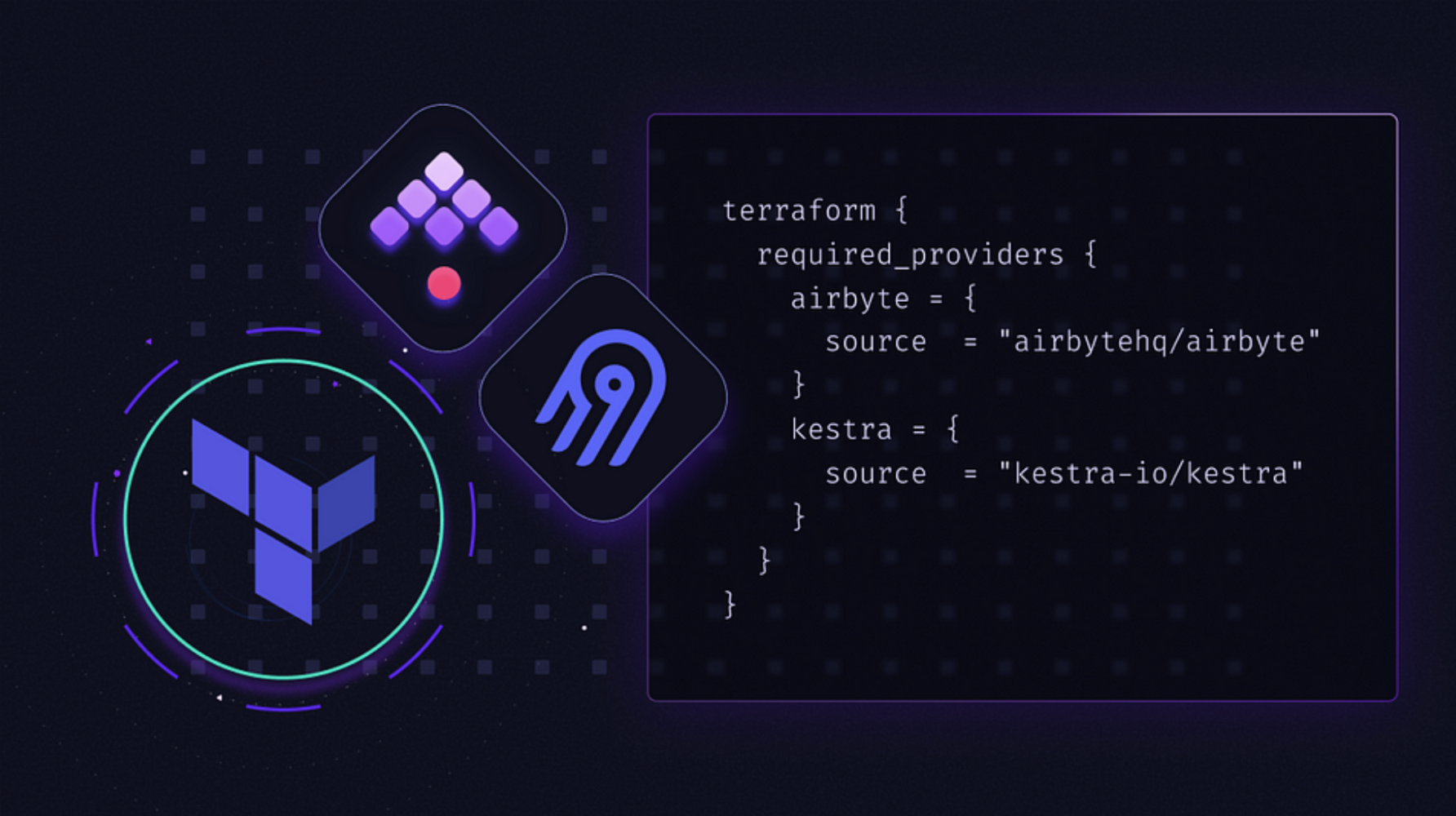

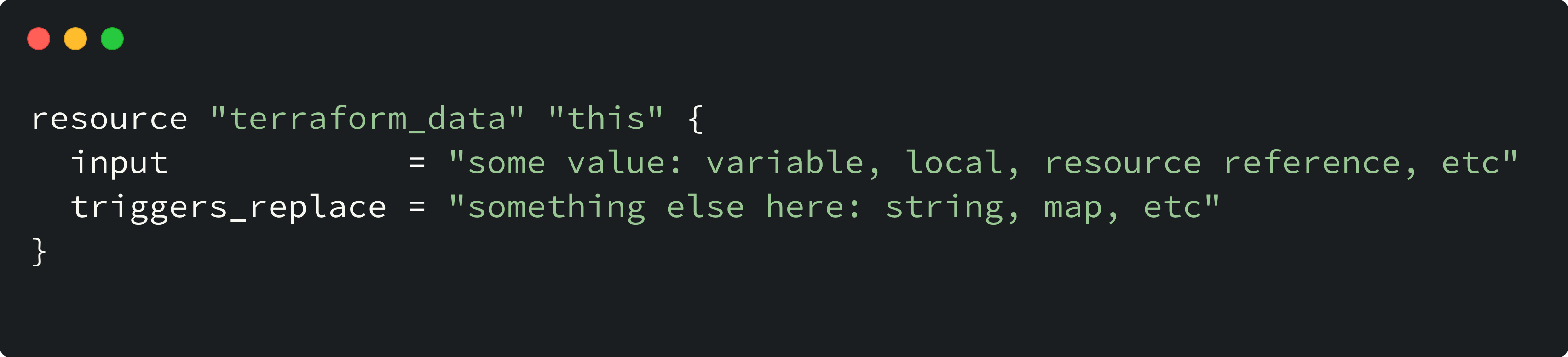

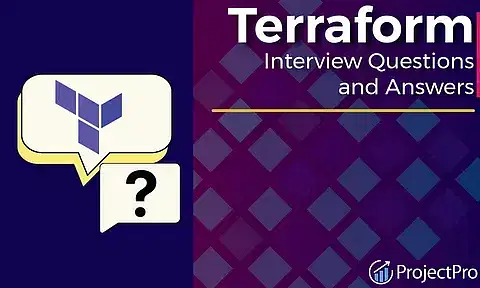
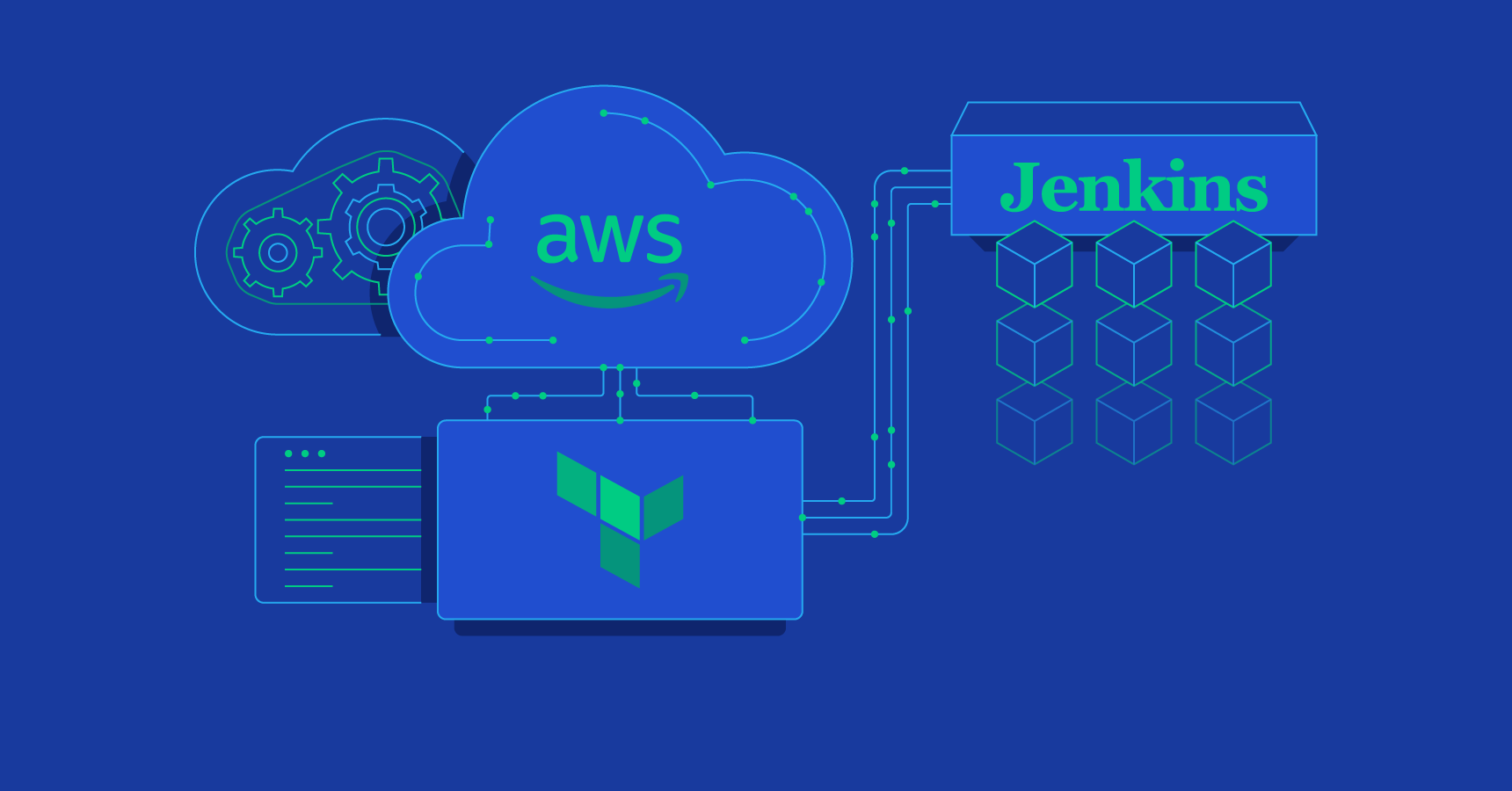
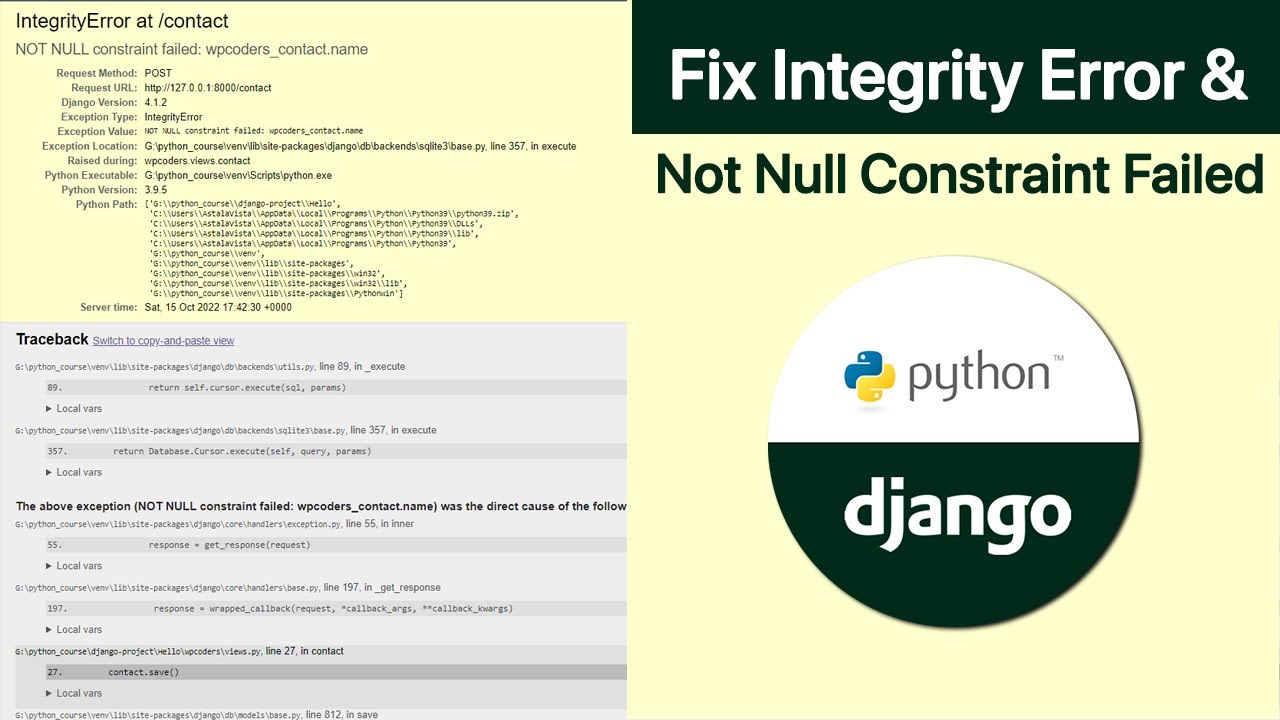


![Terraform] How to reference instance argument value created with for_each meta-argument in another instance in the same map - Developers - Cloudflare Community Terraform] How To Reference Instance Argument Value Created With For_Each Meta-Argument In Another Instance In The Same Map - Developers - Cloudflare Community](https://global.discourse-cdn.com/cloudflare/optimized/3X/8/9/894fc7acccefa37f5ad556ed7598e249080a338a_2_1024x290.png)

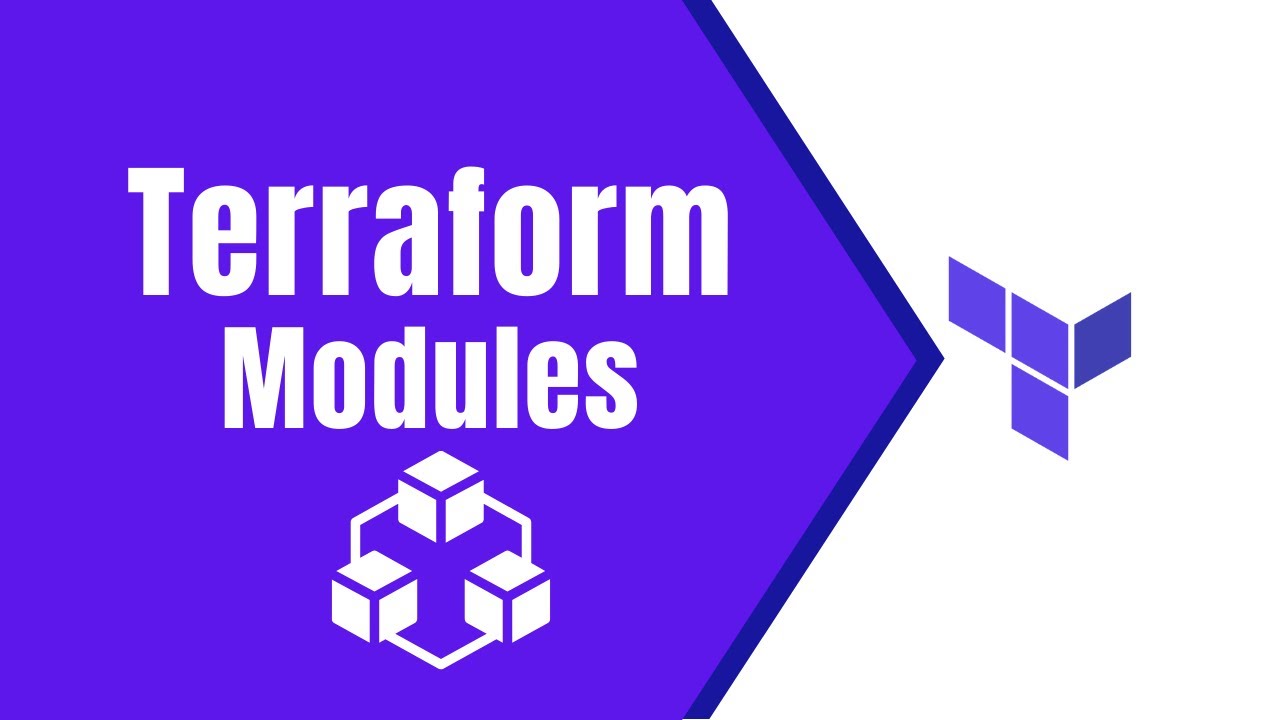
Article link: terraform if not null.
Learn more about the topic terraform if not null.
- Terraform conditionals – if variable does not exist
- Best way for “if null then default else value” – Terraform
- Terraform Optional Variables and Attributes — Using Null and …
- Variables: NULL, empty string and default values – Terraform
- Types and Values – Configuration Language | Terraform
- Understanding Null Versus the Empty String – Oracle Help Center
- Post Terraform v1.0 features I love – Brendan Thompson
- In terraform how to handle null value with default value? – Jhooq
- Validation on optional variables failing on null. : r/Terraform
- Hello Terraform Data; Goodbye Null Resource | devDosvid blog
See more: nhanvietluanvan.com/luat-hoc