String To Dictionary Python
Defining and Creating a Dictionary in Python:
To define a dictionary in Python, enclose key-value pairs within curly braces ({}):
“`
my_dict = {“key1”: value1, “key2”: value2, “key3”: value3}
“`
Alternatively, you can also create an empty dictionary and add elements to it later:
“`
my_dict = {}
“`
Adding and Modifying Elements in a Dictionary:
You can add or modify elements in a dictionary by assigning a value to a specific key:
“`
my_dict = {“name”: “John”, “age”: 25}
my_dict[“name”] = “Alice” # Modifying value
my_dict[“city”] = “New York” # Adding a new key-value pair
“`
Accessing and Retrieving Values from a Dictionary:
To access the value associated with a specific key in a dictionary, you can use square brackets []:
“`
my_dict = {“name”: “John”, “age”: 25}
print(my_dict[“name”]) # Output: John
“`
If the key doesn’t exist in the dictionary, it will raise a `KeyError`. To avoid this, you can use the `get()` method:
“`
print(my_dict.get(“name”)) # Output: John
print(my_dict.get(“city”)) # Output: None
“`
Iterating Through a Dictionary:
You can iterate over a dictionary’s keys, values, or key-value pairs using loops like `for`:
“`
my_dict = {“name”: “John”, “age”: 25, “city”: “New York”}
# Iterating over keys
for key in my_dict:
print(key)
# Iterating over values
for value in my_dict.values():
print(value)
# Iterating over key-value pairs
for key, value in my_dict.items():
print(key, value)
“`
Removing Elements from a Dictionary:
To remove a specific element from a dictionary, you can use the `del` keyword or the `pop()` method:
“`
my_dict = {“name”: “John”, “age”: 25, “city”: “New York”}
del my_dict[“age”] # Removing by key
my_dict.pop(“city”) # Removing by key and getting the value
print(my_dict) # Output: {“name”: “John”}
“`
Dictionary Methods in Python:
Python provides several built-in dictionary methods to perform various operations. Some commonly used methods include:
– `keys()`: Returns a view object containing all the keys in the dictionary.
– `values()`: Returns a view object containing all the values in the dictionary.
– `items()`: Returns a view object containing key-value pairs as tuples.
– `clear()`: Removes all elements from the dictionary.
– `copy()`: Returns a shallow copy of the dictionary.
Dictionary Comprehension in Python:
Similar to list comprehension, Python supports dictionary comprehension, which allows you to create dictionaries in a concise and elegant way:
“`python
my_dict = {key: value for key, value in iterable if condition}
“`
Nested Dictionaries in Python:
Dictionaries in Python can also be nested, meaning you can have a dictionary within a dictionary:
“`python
my_dict = {“person1”: {“name”: “John”, “age”: 25}, “person2”: {“name”: “Alice”, “age”: 30}}
“`
Common Operations and Use Cases of Dictionaries in Python:
Dictionaries are widely used in Python due to their flexibility and efficiency in solving many programming problems. Some common use cases of dictionaries include:
– Storing and retrieving configuration settings.
– Counting occurrences of items in a dataset.
– Mapping unique identifiers to corresponding objects.
– Building data structures like graphs, trees, and hash tables.
– Creating JSON-like structures for data interchange.
FAQs:
Q: How can I convert an input string to a dictionary in Python?
A: To convert a string to a dictionary, you can make use of the `eval()` or `ast.literal_eval()` functions. Here’s an example:
“`python
my_string = ‘{“name”: “John”, “age”: 25}’
my_dict = eval(my_string)
“`
Q: How can I convert a dictionary to a string in Python?
A: You can use the `str()` or `json.dumps()` functions to convert a dictionary to a string. Here’s an example using `json.dumps()`:
“`python
import json
my_dict = {“name”: “John”, “age”: 25}
my_string = json.dumps(my_dict)
“`
Q: How can I convert a string to a list in Python?
A: To convert a string to a list, you can use the `split()` method, specifying the delimiter. Here’s an example:
“`python
my_string = “apple,banana,orange”
my_list = my_string.split(“,”)
“`
Q: How can I check if a string is present in a dictionary in Python?
A: You can use the `in` keyword to check if a string is a key in the dictionary:
“`python
my_dict = {“name”: “John”, “age”: 25}
if “name” in my_dict:
print(“String is present in the dictionary”)
“`
Q: How can I convert a string to JSON in Python?
A: Python provides the `json` module that allows you to convert a string to JSON using the `json.loads()` function:
“`python
import json
my_string = ‘{“name”: “John”, “age”: 25}’
json_data = json.loads(my_string)
“`
Q: How can I convert a Python string to a dictionary in JSON format?
A: You can use the `json.loads()` function to convert a string to a dictionary in JSON format:
“`python
import json
my_string = ‘{“name”: “John”, “age”: 25}’
my_dict = json.loads(my_string)
“`
Q: How can I stringify a Python dictionary?
A: You can use the `json.dumps()` function from the `json` module to stringify a dictionary in Python:
“`python
import json
my_dict = {“name”: “John”, “age”: 25}
my_string = json.dumps(my_dict)
“`
Q: How can I read a file into a dictionary in Python?
A: You can use the `json` module to read a JSON file into a dictionary using the `json.load()` function:
“`python
import json
with open(“data.json”) as f:
my_dict = json.load(f)
“`
In conclusion, dictionaries are an essential data structure in Python that allow you to store and retrieve data efficiently using unique keys. They have versatile use cases and can be easily manipulated using various built-in methods. Whether you need to convert strings to dictionaries or perform operations on dictionary objects, Python provides a convenient and powerful set of tools to work with dictionaries effectively.
How To Convert String Into Dictionary In Python
How To Convert A String Into Dictionary In Python?
Python is a versatile and powerful programming language widely used for its simplicity and flexibility. One of its notable features is the ability to convert different data types, including strings, into dictionaries. This can be a valuable skill when working with data manipulation and extraction. In this article, we will explore various methods to convert a string into a dictionary in Python.
Before diving into the methods, let’s understand what a dictionary is in Python. A dictionary is an unordered collection of key-value pairs, where each value is accessed using its associated key. Unlike lists or arrays, dictionaries have no concept of indexes, as the keys act as unique identifiers for their associated values.
Method 1: Using the eval() function
The eval() function in Python allows us to evaluate and execute a string as a Python expression. By utilizing this function, we can convert a string representation of a dictionary into an actual dictionary. Let’s consider an example:
“`python
string_dict = ‘{“key1”: “value1”, “key2”: “value2”, “key3”: “value3”}’
dict_obj = eval(string_dict)
print(dict_obj)
“`
In this case, the eval() function converts the string representation of a dictionary into a dictionary object. The output will be:
“`
{‘key1’: ‘value1’, ‘key2’: ‘value2’, ‘key3’: ‘value3’}
“`
Method 2: Using the json module
Python provides the json module, which allows us to work with JSON (JavaScript Object Notation) data. JSON is a lightweight data interchange format commonly used for transmitting data between a server and a web application. The json module includes functions to handle encoding and decoding of JSON objects. To convert a string into a dictionary, we can use the json.loads() method. Let’s see an example:
“`python
import json
string_dict = ‘{“key1”: “value1”, “key2”: “value2”, “key3”: “value3”}’
dict_obj = json.loads(string_dict)
print(dict_obj)
“`
The output will be identical to the previous example:
“`
{‘key1’: ‘value1’, ‘key2’: ‘value2’, ‘key3’: ‘value3’}
“`
Method 3: Using the ast.literal_eval() function
The ast.literal_eval() function is a safer alternative to eval() as it only evaluates literals, such as strings, numbers, tuples, lists, booleans, and None. It prevents the execution of arbitrary code, making it a secure option for converting a string into a dictionary. Let’s take a look at an example:
“`python
import ast
string_dict = ‘{“key1”: “value1”, “key2”: “value2”, “key3”: “value3”}’
dict_obj = ast.literal_eval(string_dict)
print(dict_obj)
“`
Again, the output will be the converted dictionary:
“`
{‘key1’: ‘value1’, ‘key2’: ‘value2’, ‘key3’: ‘value3’}
“`
FAQs:
Q: Can I convert a string with nested dictionaries into a dictionary?
A: Yes, the methods mentioned above can handle strings with nested dictionaries. However, the string should be in valid JSON format, as JSON supports nested structures.
Q: What happens if the string contains invalid syntax for a dictionary?
A: If the string does not adhere to the required dictionary syntax, such as missing key-value pairs or using incorrect quotations, the conversion will raise a syntax error.
Q: Can I convert a string into a dictionary if the keys and values are of different data types?
A: Yes, dictionaries in Python can contain keys and values of different data types. The conversion methods explained earlier will work regardless of the data type of the keys and values.
Q: Are there any other methods to convert a string into a dictionary?
A: Yes, there are other methods available, such as using regular expressions or manually splitting and processing the string. However, the methods covered in this article are considered safer and more efficient.
In conclusion, converting a string into a dictionary in Python can be accomplished through various approaches. By utilizing the eval(), json.loads(), or ast.literal_eval() methods, we can easily convert a string representation of a dictionary into a dictionary object. These conversion methods provide flexibility and security while working with strings and dictionaries in Python.
How To Convert String Key Value To Dictionary In Python?
Python offers a wide range of tools and functionalities that make it one of the most popular programming languages. In certain scenarios, you might come across situations where you need to convert a string key value into a dictionary structure. This article will guide you through the process of converting string key value to a dictionary in Python, exploring various techniques and providing comprehensive examples.
Understanding the Basics
Before we delve into the methods of converting string key values to a dictionary, let’s first understand the basic concepts involved:
– String Key Value: A string key value is a combination of characters that represents the key associated with a specific value in a dictionary.
– Dictionary: In Python, a dictionary is an unordered collection of key-value pairs enclosed within curly braces {}. Each key-value pair is separated by a colon (:) and individual pairs are separated by commas (,). Dictionaries provide efficient lookup, insertion, and deletion of elements.
Conversion Methods
There are multiple ways to convert a string key value into a dictionary in Python. Let’s explore some commonly used methods.
1. Using the eval() function:
The eval() function is a built-in Python function that evaluates a string expression and returns the result. When used with the string containing a dictionary, eval() can convert it into a dictionary object.
Example:
“`
string_dict = “{ ‘fruit’:’apple’, ‘color’:’red’, ‘shape’:’round’ }”
dict_conversion = eval(string_dict)
print(dict_conversion)
“`
Output:
“`
{‘fruit’: ‘apple’, ‘color’: ‘red’, ‘shape’: ’round’}
“`
2. Using the json module:
The json module provides a powerful set of tools to work with JSON data structures. It includes the json.loads() method that can convert a JSON formatted string into a dictionary.
Example:
“`
import json
string_dict = “{ ‘fruit’:’apple’, ‘color’:’red’, ‘shape’:’round’ }”
dict_conversion = json.loads(string_dict)
print(dict_conversion)
“`
Output:
“`
{‘fruit’: ‘apple’, ‘color’: ‘red’, ‘shape’: ’round’}
“`
3. Iterating through the string and splitting:
This method involves splitting the string based on delimiters (such as comma) and iterating over the resulting list to create a dictionary.
Example:
“`
string_dict = “{ ‘fruit’:’apple’, ‘color’:’red’, ‘shape’:’round’ }”
dict_conversion = {}
string_dict = string_dict.strip(‘{}’)
for pair in string_dict.split(‘,’):
key, value = pair.strip().split(‘:’)
dict_conversion[key.strip().strip(“‘”)] = value.strip().strip(“‘”)
print(dict_conversion)
“`
Output:
“`
{‘fruit’: ‘apple’, ‘color’: ‘red’, ‘shape’: ’round’}
“`
4. Using the ast.literal_eval() function:
The ast.literal_eval() function converts a string that represents a Python literal structure into its corresponding value. It is a safer option than using eval() as it only evaluates literals and raises an exception if an expression is encountered.
Example:
“`
import ast
string_dict = “{ ‘fruit’:’apple’, ‘color’:’red’, ‘shape’:’round’ }”
dict_conversion = ast.literal_eval(string_dict)
print(dict_conversion)
“`
Output:
“`
{‘fruit’: ‘apple’, ‘color’: ‘red’, ‘shape’: ’round’}
“`
FAQs
Q1. Can I convert a string representing a nested dictionary into a dictionary?
Yes, the mentioned methods can handle nested dictionaries as well. For instance, the json.loads() method can easily convert a string containing a JSON formatted nested dictionary into a dictionary. Similarly, the other methods mentioned above can also be applied.
Q2. Is there any specific format that the input string representing the dictionary should follow?
The input string should follow a specific format that conforms to the dictionary structure in Python. It should include key-value pairs, with keys and values enclosed within quotes (either single or double) and separated by a colon. Additionally, the entire string should be wrapped in curly braces.
Q3. Which method should I prefer for string key value conversion?
The choice of method depends on the specific use case and personal preference. The eval() function is more convenient to use but can be risky if the string input is not trustworthy. The json.loads() method is a safer option, whereas, iterating through the string is suitable for scenarios where you have control over the string format. The ast.literal_eval() function is recommended when the input string represents Python literals.
Conclusion
In this article, we explored various methods to convert a string key value into a dictionary in Python. We discussed the underlying concepts and presented comprehensive examples to illustrate the conversion process. Additionally, we answered some frequently asked questions to address common concerns. Now armed with this knowledge, you can effortlessly convert string key values to dictionary objects in Python.
Keywords searched by users: string to dictionary python Convert input string to dictionary Python, Dict to string Python, Convert string to list Python, String in dictionary python, Convert string to JSON Python, Python string to dict json, Stringify python dict, Read file to dictionary Python
Categories: Top 75 String To Dictionary Python
See more here: nhanvietluanvan.com
Convert Input String To Dictionary Python
Python is a powerful and versatile programming language known for its simplicity and readability. One of the most common tasks in any programming language is converting data from one format to another. In this article, we will discuss how to convert an input string into a dictionary in Python, exploring different methods and scenarios that can arise during the conversion process.
A dictionary in Python is an unordered collection of key-value pairs. It is mutable, meaning you can modify its elements by adding, removing, or updating key-value pairs. The key-value pairs are enclosed in curly braces {} and separated by a colon. To convert an input string into a dictionary, we need to parse the string and extract the key-value pairs.
Method 1: Manual Parsing
The simplest way to convert an input string into a dictionary is by manually parsing the string. This method is useful when the input string follows a specific format. Let’s consider an example:
input_string = “name:John, age:25, city:New York”
To convert this string into a dictionary, we can split the string at each comma ‘,’ and then split each pair at the colon ‘:’. Here’s the code snippet for this method:
“`python
input_string = “name:John, age:25, city:New York”
dictionary = {}
pairs = input_string.split(‘, ‘)
for pair in pairs:
key, value = pair.split(‘:’)
dictionary[key] = value
print(dictionary)
“`
Output:
{‘name’: ‘John’, ‘age’: ’25’, ‘city’: ‘New York’}
Method 2: JSON Conversion
JSON (JavaScript Object Notation) is a lightweight data interchange format. Python provides a built-in library called `json` which enables us to convert JSON data into Python objects. By leveraging this library, we can convert an input string into a dictionary.
Here’s an example:
“`python
import json
input_string = ‘{“name”: “John”, “age”: 25, “city”: “New York”}’
dictionary = json.loads(input_string)
print(dictionary)
“`
Output:
{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
Note that in this method, the input string should be formatted as valid JSON.
Method 3: Using eval()
The `eval()` function in Python allows us to evaluate an expression. Although it can be powerful, it should be used with caution as it can execute arbitrary code. However, in certain scenarios, it can be employed to convert an input string into a dictionary.
“`python
input_string = “{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}”
dictionary = eval(input_string)
print(dictionary)
“`
Output:
{‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
FAQs
Q1. How can I convert a string with nested dictionaries into a Python dictionary?
Converting a string with nested dictionaries is a bit more complex. You can either implement a recursive function to handle the nested dictionaries, or you can use the `eval()` method discussed earlier. However, it is important to be cautious when using `eval()` due to security risks.
Q2. What if my input string has duplicate keys?
If your input string contains duplicate keys, only the last occurrence will be stored in the dictionary. This is because dictionaries in Python cannot have duplicate keys.
Q3. Can I convert a dictionary to an input string?
Yes, you can convert a dictionary into a string using the `str()` function. However, keep in mind that the resulting string won’t necessarily be in the same format as the original input string.
Q4. How can I handle errors and invalid input during the conversion process?
To handle errors and invalid input, you can use various techniques such as exception handling and input validation. For example, you can use try-except blocks to catch any potential exceptions raised during the conversion process and handle them accordingly.
Q5. Are there any limitations or restrictions when converting a string to a dictionary?
The limitations or restrictions when converting a string to a dictionary depends on the method you choose. In general, you need to ensure that the input string follows a specific format, such as being a valid JSON string or adhering to certain formatting rules if manually parsing the string.
In conclusion, converting an input string into a dictionary can be accomplished using various methods in Python. Whether you choose manual parsing, JSON conversion, or the `eval()` function, understanding the format and structure of the input string is crucial for a successful conversion. Additionally, it is important to handle exceptions and validate input to ensure smooth functioning of the conversion process.
Dict To String Python
Python is a versatile programming language that is widely used for various applications, including data manipulation and analysis. One frequent task when working with Python is converting a dictionary into a string. In this article, we will explore the different ways to achieve dict to string conversion in Python and discuss the reasons why you might need to perform this operation.
What is a Dictionary in Python?
Before diving into dict to string conversions, it is important to understand what a dictionary is in Python. A dictionary is an unordered collection of key-value pairs, where each key is unique. It is denoted by curly braces ({}) and can be modified by adding, removing, or modifying its elements. Dictionaries are commonly used to represent structured data, such as a database record or a configuration file.
Why Convert a Dictionary to a String?
There are several scenarios where you might need to convert a dictionary to a string in Python. One common use case is when you want to store the dictionary in a file or database that only accepts string inputs. Another scenario is when you need to transmit the dictionary over a network or use it as part of a web API, where string representation is preferred. Additionally, converting the dictionary to a string allows you to visualize and analyze its contents more easily.
Approaches to Dict to String Conversion
Python provides multiple approaches to convert a dictionary to a string, depending on the requirements and formatting preferences. Let’s explore the most commonly used methods:
1. Using the str() Function:
The simplest approach to converting a dictionary to a string is by using the str() function. This function takes any Python object as input and returns its string representation. When applied to a dictionary, it returns a string with the dictionary’s curly brackets and contents.
Example:
“`
my_dict = {“name”: “John”, “age”: 25}
my_str = str(my_dict)
print(my_str) # Output: {‘name’: ‘John’, ‘age’: 25}
“`
However, it is important to note that the str() function does not guarantee any specific formatting for the dictionary’s key-value pairs. It is primarily used for getting a quick string representation rather than precise control over the output.
2. Using the json Module:
If you require a more structured and standardized way to convert a dictionary to a string, the json module in Python can be used. This module provides functions to serialize Python objects, including dictionaries, into JSON format. The resulting string can be easily stored or transmitted and conveniently deserialized back into a dictionary when needed.
Example:
“`
import json
my_dict = {“name”: “John”, “age”: 25}
my_str = json.dumps(my_dict)
print(my_str) # Output: {“name”: “John”, “age”: 25}
“`
3. Using String Concatenation:
Another approach to convert a dictionary to a string is by concatenating its key-value pairs using string manipulation techniques. This method allows you to format the output precisely according to your requirements.
Example:
“`
my_dict = {“name”: “John”, “age”: 25}
my_str = “”
for key, value in my_dict.items():
my_str += f”{key}: {value}\n”
print(my_str)
# Output:
# name: John
# age: 25
“`
By utilizing string concatenation, you can customize the output formatting by adding delimiters, line breaks, or other symbols as desired.
FAQs
Q1. Can dictionaries with nested dictionaries be converted to strings as well?
Yes, dictionaries with nested dictionaries can be converted to strings using the same methods mentioned above. The json module provides the most convenient way to serialize and deserialize such complex data structures.
Q2. Are there any limitations when using the json module for dict to string conversions?
The json module is based on the JSON data format, which supports a limited set of data types. When using the json module, make sure your dictionary does not contain unsupported types like complex numbers or custom objects. In such cases, you will need to convert the dictionary to a custom string representation using other methods.
Q3. How can I restore a string to a dictionary?
To restore a string back to a dictionary, you can use the json module’s loads() function if the string is in valid JSON format. Alternatively, you can use custom parsing techniques to split and extract the key-value pairs from the string and reconstruct the dictionary accordingly.
In conclusion, converting a dictionary to a string in Python is a common operation with various use cases. Whether you need a quick string representation or a structured format, Python provides several methods, such as str(), json module, and string concatenation. Choose the method that best suits your requirements and leverage the power of Python to perform dict to string conversions effortlessly.
Convert String To List Python
Python is a versatile programming language that offers a wide range of functionalities and built-in methods. One common task in Python programming is converting a string into a list. While it may seem like a simple operation, there are several different approaches and considerations to keep in mind. In this article, we will explore various methods to convert a string to a list in Python and cover some frequently asked questions on the topic.
Before diving into the different conversion techniques, let’s first clarify what we mean by “converting a string to a list” in Python. In Python, a string is a sequence of characters, while a list is a collection of values enclosed in square brackets and separated by commas. Converting a string to a list means transforming the individual characters of the string into elements of a list.
Method 1: Using the list() function
The most straightforward way to convert a string to a list in Python is by using the built-in list() function. This function takes an iterable as an argument and returns a list containing the elements of the iterable. When we pass a string as the argument to the list() function, it treats each character as a separate element and creates a list with those elements.
Example:
“`python
string = “Hello, World!”
list_string = list(string)
print(list_string)
“`
Output:
“`python
[‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘,’, ‘ ‘, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’, ‘!’]
“`
Method 2: Using list comprehension
List comprehension is another powerful technique in Python that allows us to create a new list based on an existing iterable. We can leverage list comprehension to convert a string to a list by iterating over each character of the string and appending it to the new list.
Example:
“`python
string = “Hello, World!”
list_string = [char for char in string]
print(list_string)
“`
Output:
“`python
[‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘,’, ‘ ‘, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’, ‘!’]
“`
Method 3: Splitting the string using the split() method
If the string contains words separated by a specific delimiter (e.g., whitespace, comma, etc.), we can use the split() method to split the string into a list of substrings. By default, the split() method splits the string using whitespace as the delimiter, but we can specify a different delimiter by passing it as an argument.
Example 1 – Using whitespace as the delimiter:
“`python
string = “Hello World!”
list_string = string.split()
print(list_string)
“`
Output:
“`python
[‘Hello’, ‘World!’]
“`
Example 2 – Using comma as the delimiter:
“`python
string = “apple,banana,orange”
list_string = string.split(“,”)
print(list_string)
“`
Output:
“`python
[‘apple’, ‘banana’, ‘orange’]
“`
Method 4: Using the map() function
The map() function allows us to apply a function to each element of an iterable. By passing the list() function as the first argument to map() and the string as the second argument, we can convert the string to a list using the map() function.
Example:
“`python
string = “Hello, World!”
list_string = list(map(str, string))
print(list_string)
“`
Output:
“`python
[‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘,’, ‘ ‘, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’, ‘!’]
“`
Now let’s address some frequently asked questions related to converting strings to lists in Python:
FAQs:
Q1: Can I convert a string to a list of integers instead of characters?
A1: Yes, you can. If your string consists of only numeric characters, you can convert it to a list of integers using list comprehension along with the int() function.
Example:
“`python
string = “12345”
list_int = [int(char) for char in string]
print(list_int)
“`
Output:
“`python
[1, 2, 3, 4, 5]
“`
Q2: How can I convert a string with multiple words to a list of words?
A2: You can use the split() method with whitespace as the delimiter to split the string into a list of words.
Example:
“`python
string = “Hello, how are you?”
list_words = string.split()
print(list_words)
“`
Output:
“`python
[‘Hello,’, ‘how’, ‘are’, ‘you?’]
“`
Q3: Can I convert a string to a nested list?
A3: Yes, you can. By applying one of the conversion methods mentioned above to each element of the string, you can create a nested list where each interior list represents a character or word.
Example:
“`python
string = “Hello, World!”
nested_list = [[char] for char in string]
print(nested_list)
“`
Output:
“`python
[[‘H’], [‘e’], [‘l’], [‘l’], [‘o’], [‘,’], [‘ ‘], [‘W’], [‘o’], [‘r’], [‘l’], [‘d’], [‘!’]]
“`
In conclusion, converting a string to a list in Python can be achieved using various methods such as the list() function, list comprehension, split() method, or map() function. The choice of method depends on the desired output format and specific requirements of your program. By understanding these different techniques, you can efficiently manipulate strings as lists in your Python projects.
Images related to the topic string to dictionary python
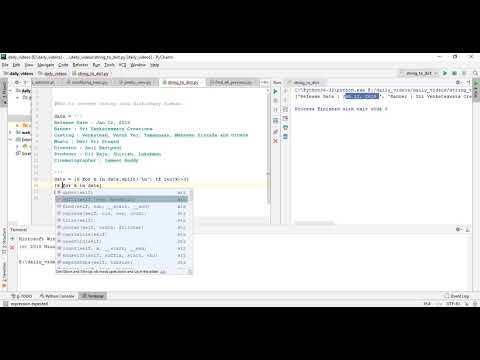
Found 7 images related to string to dictionary python theme
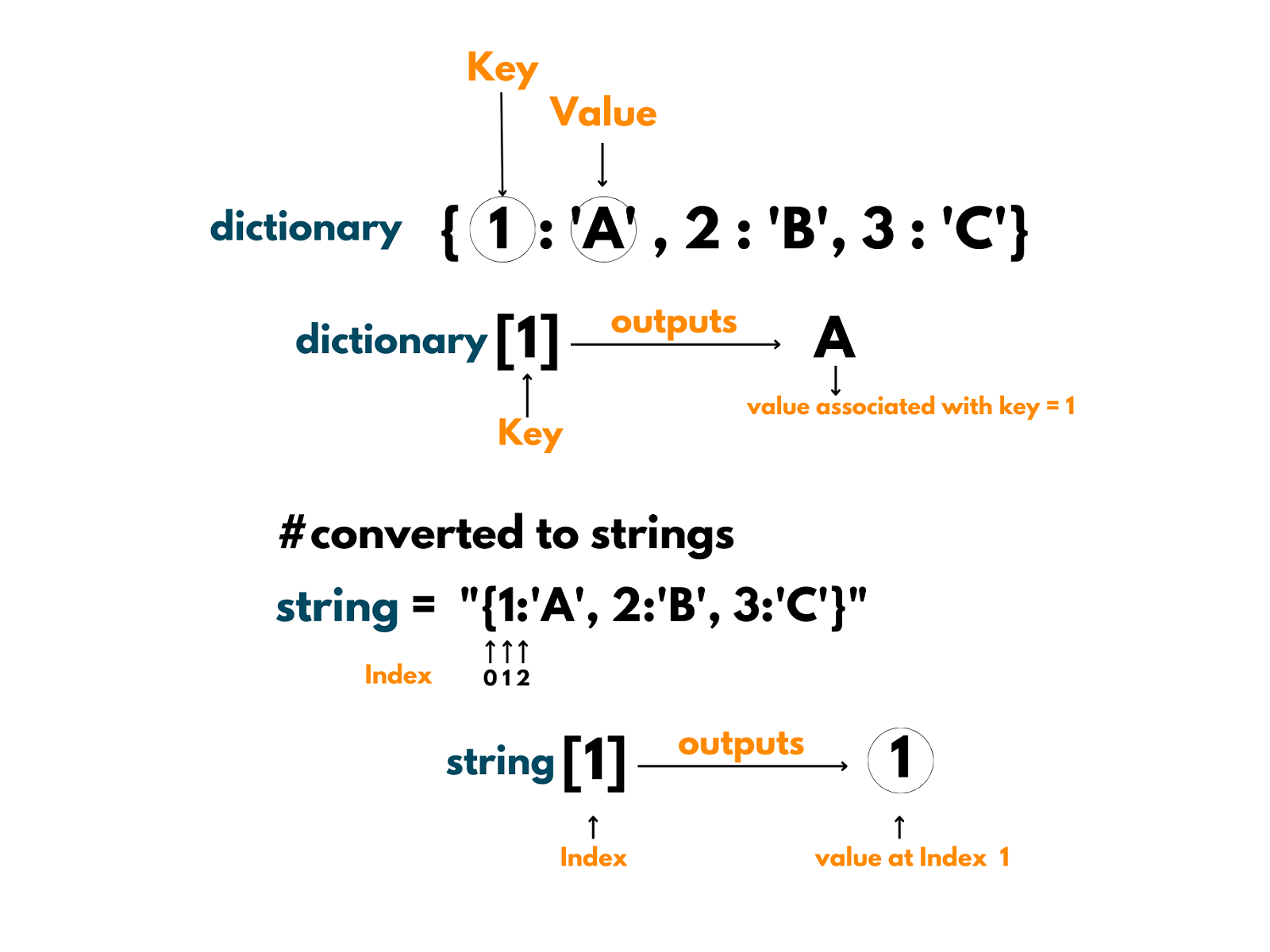
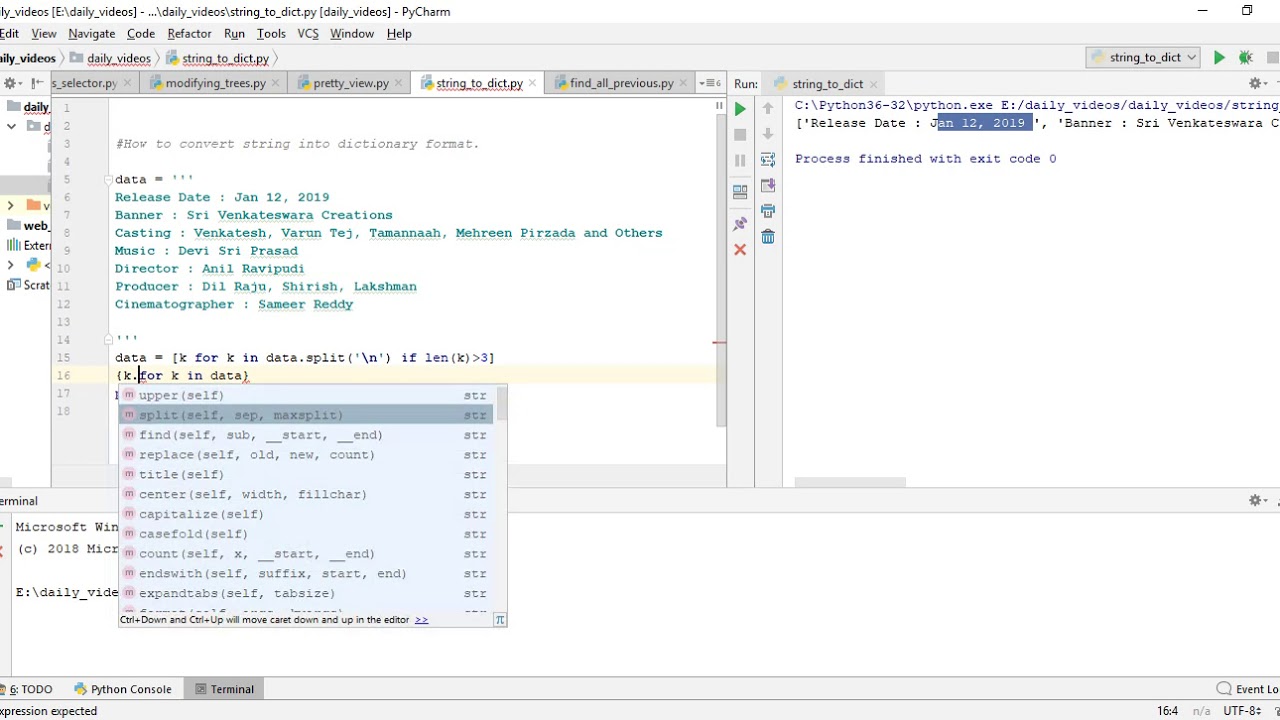
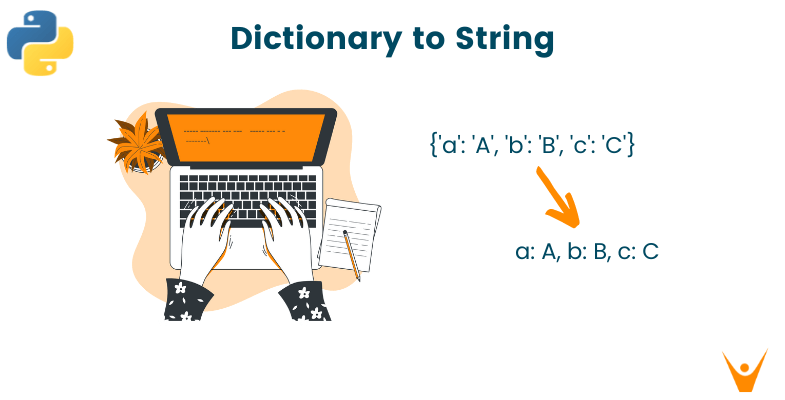
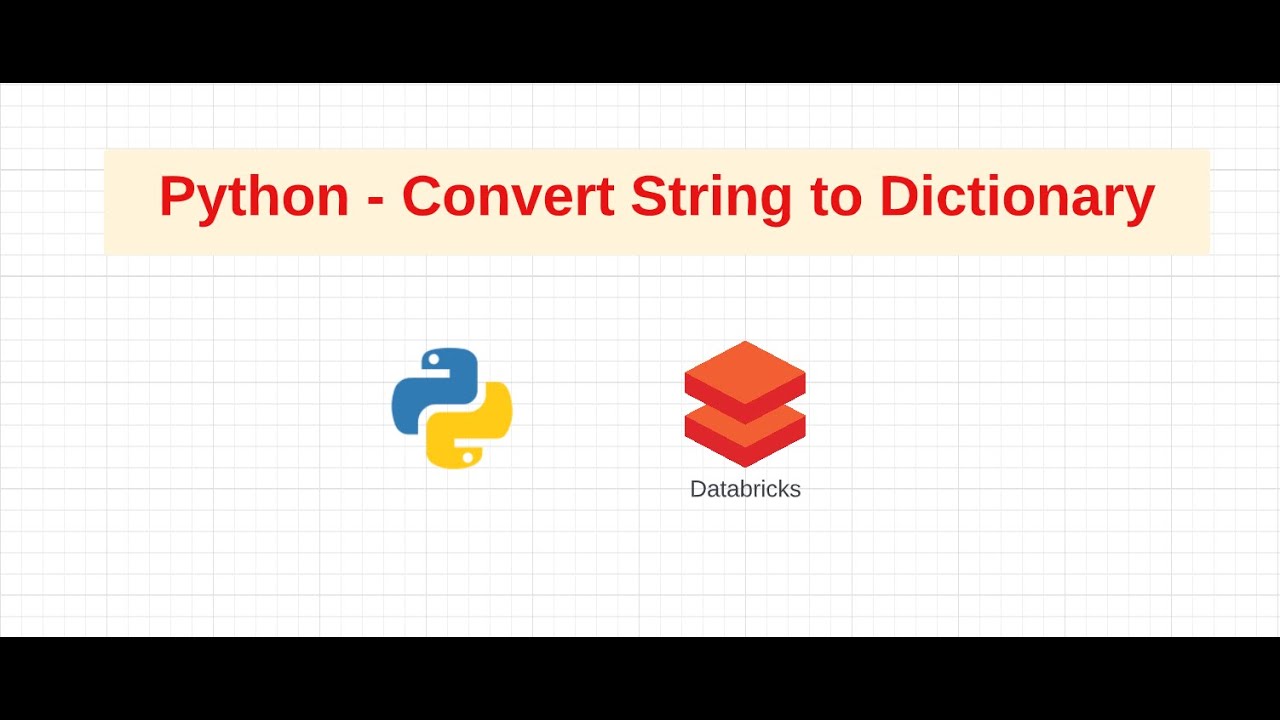
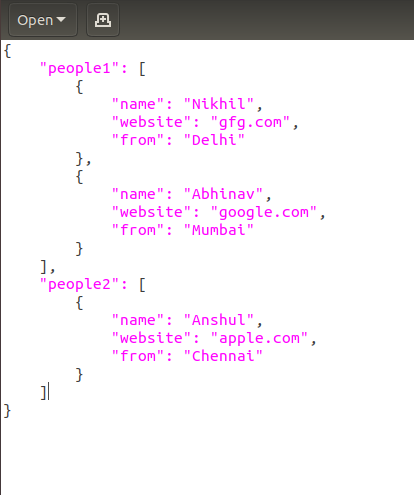

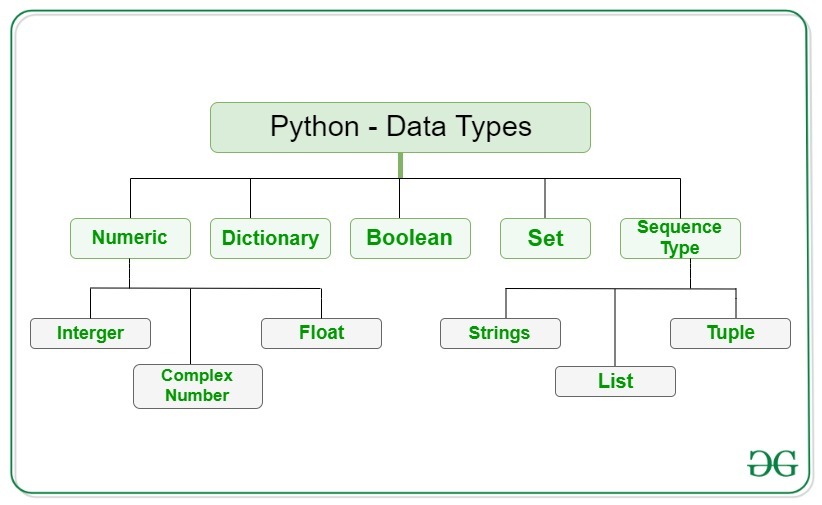
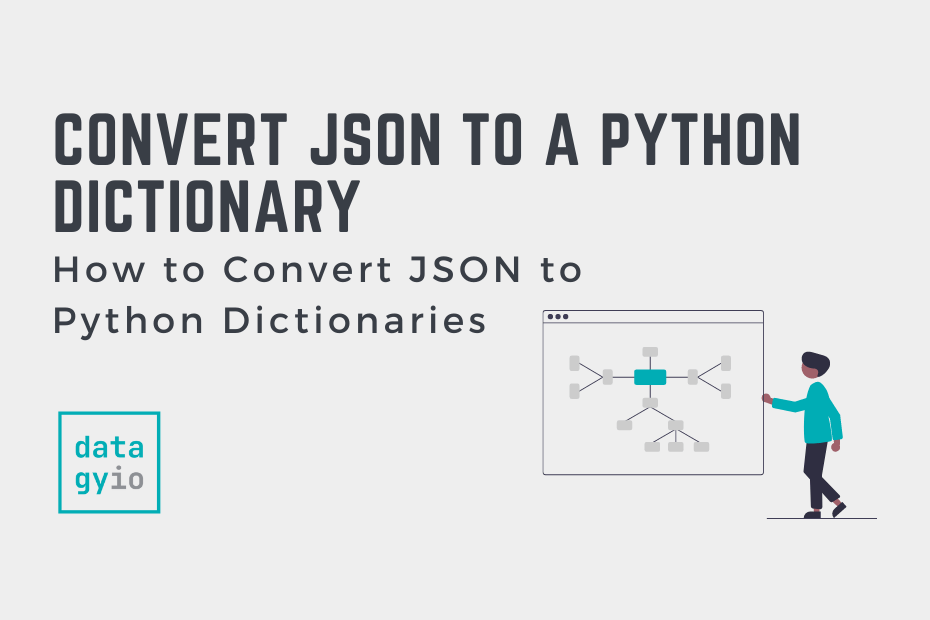
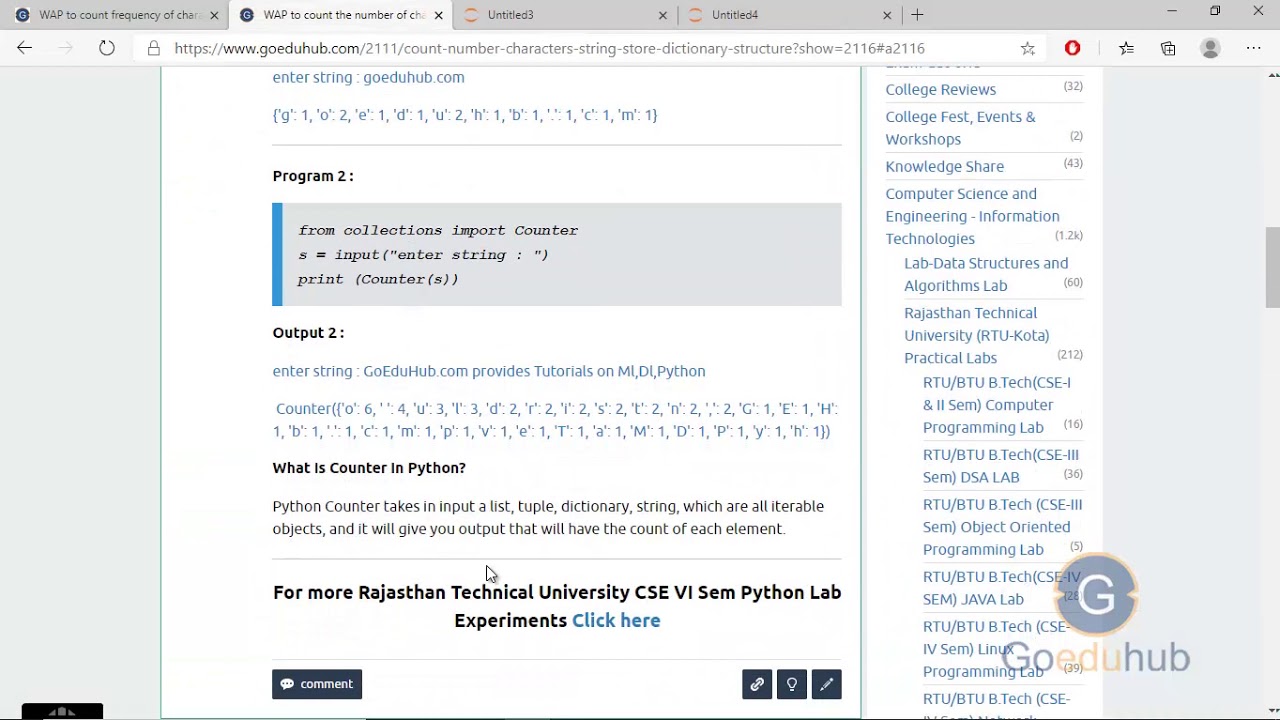

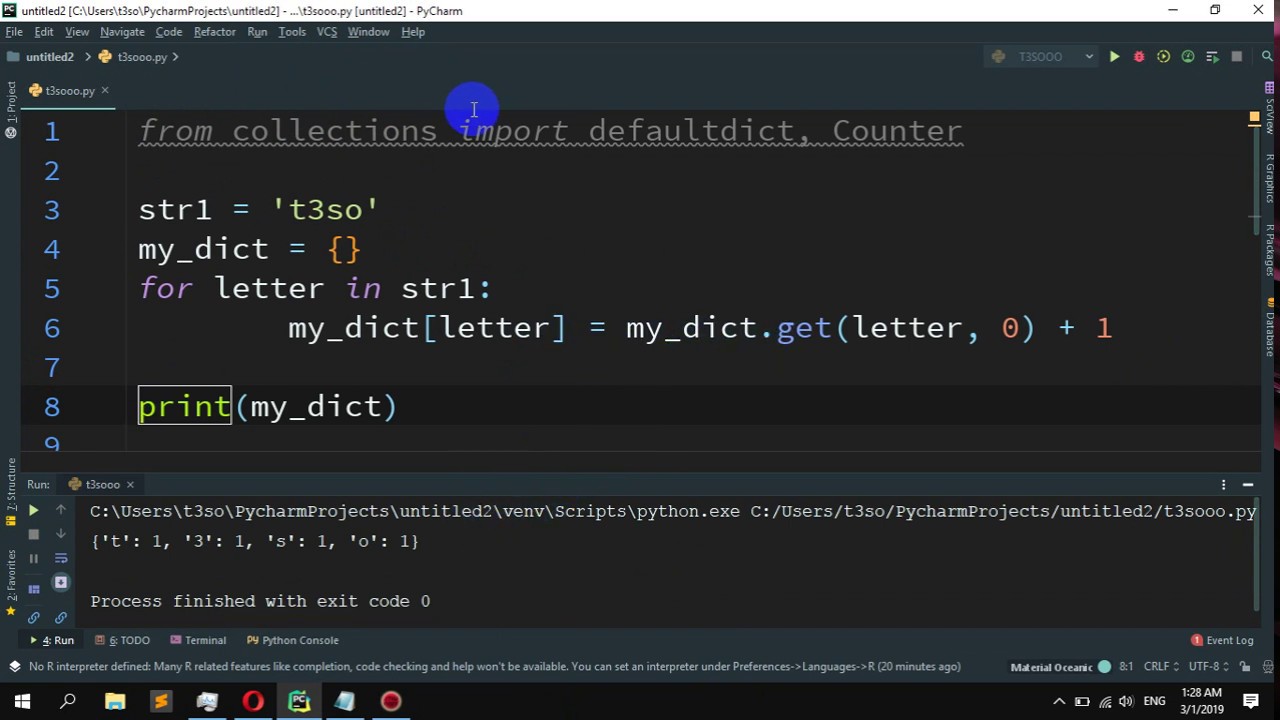
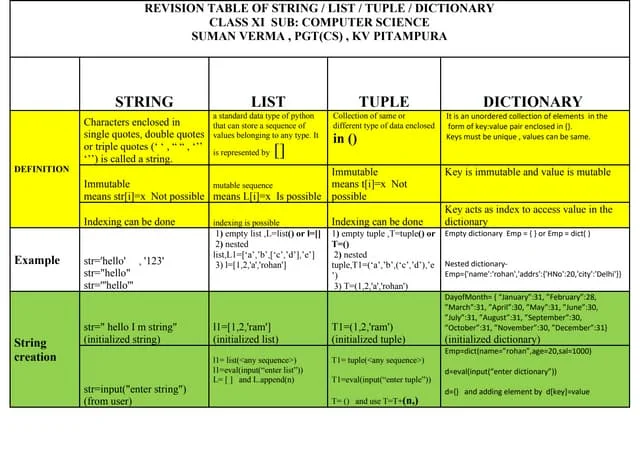
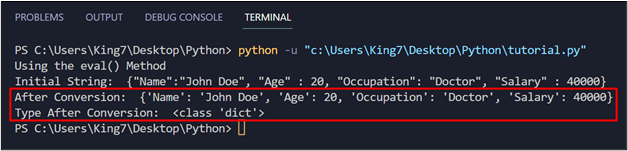
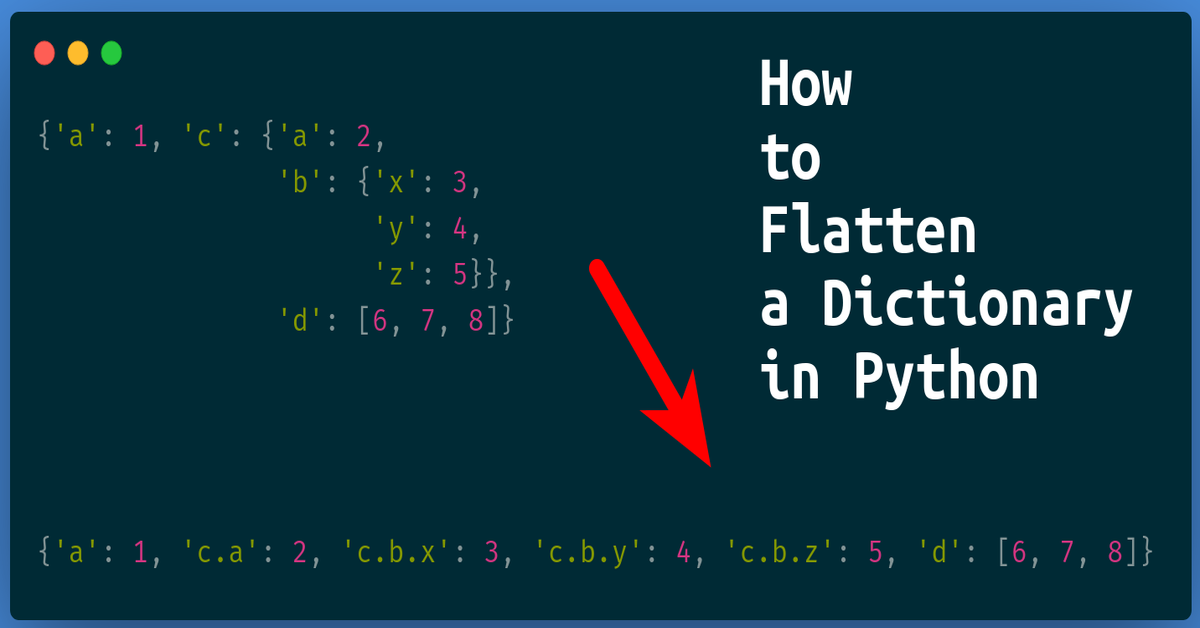
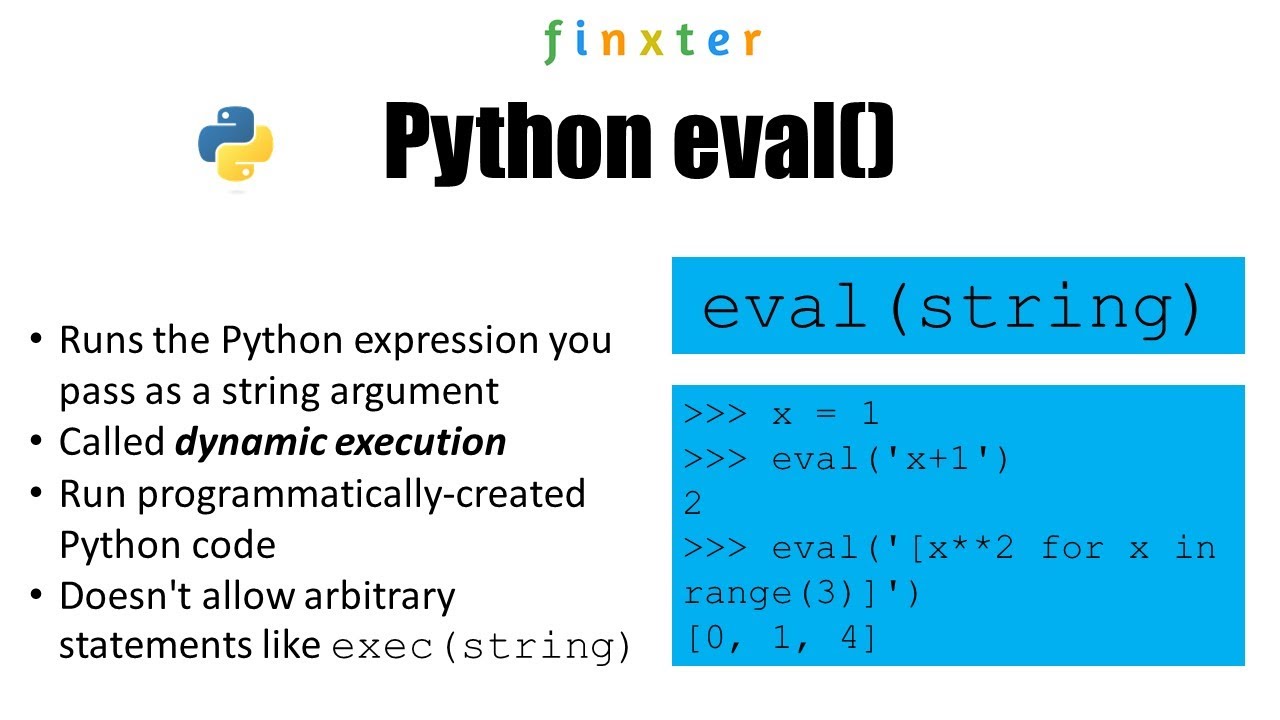

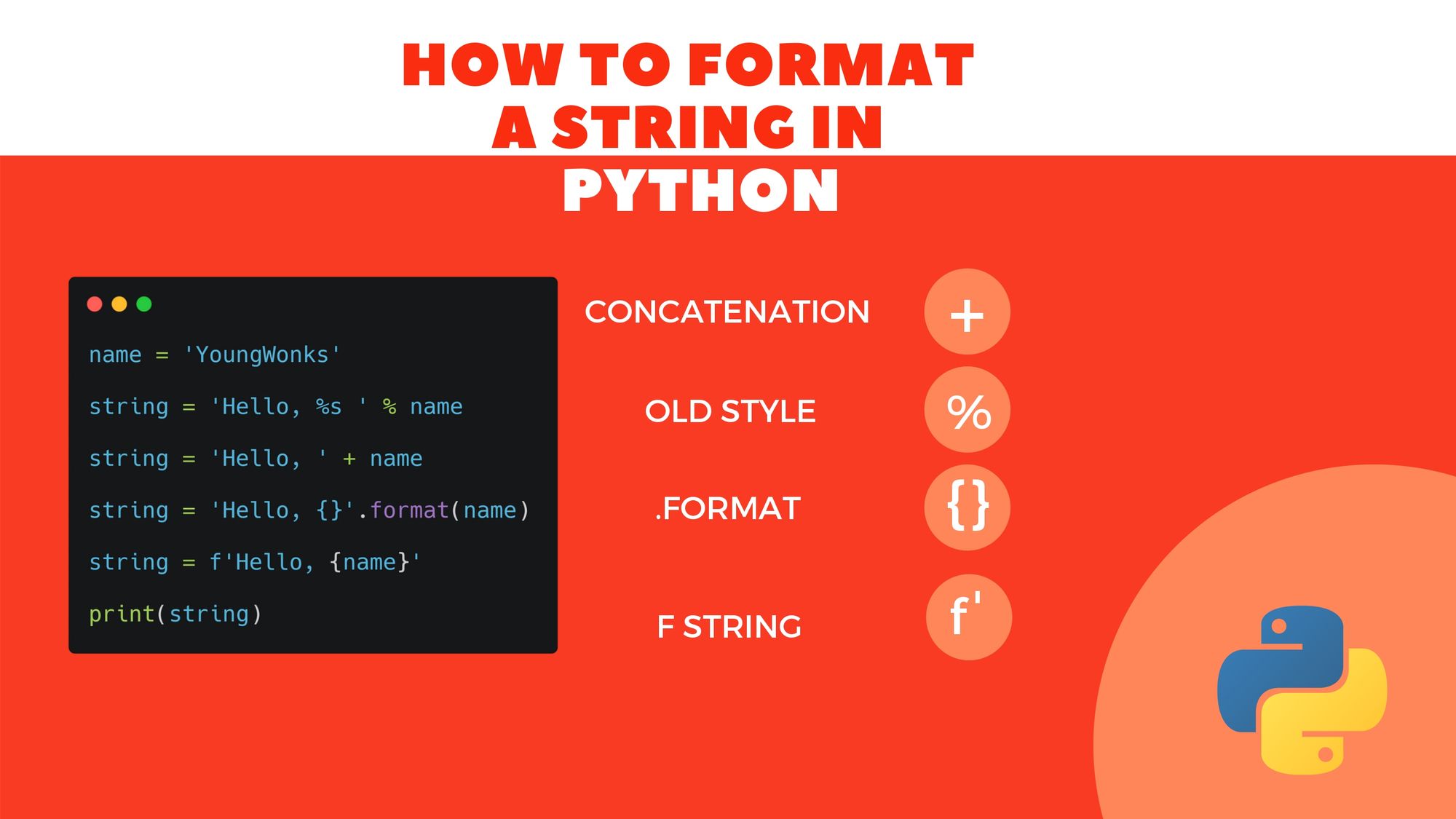
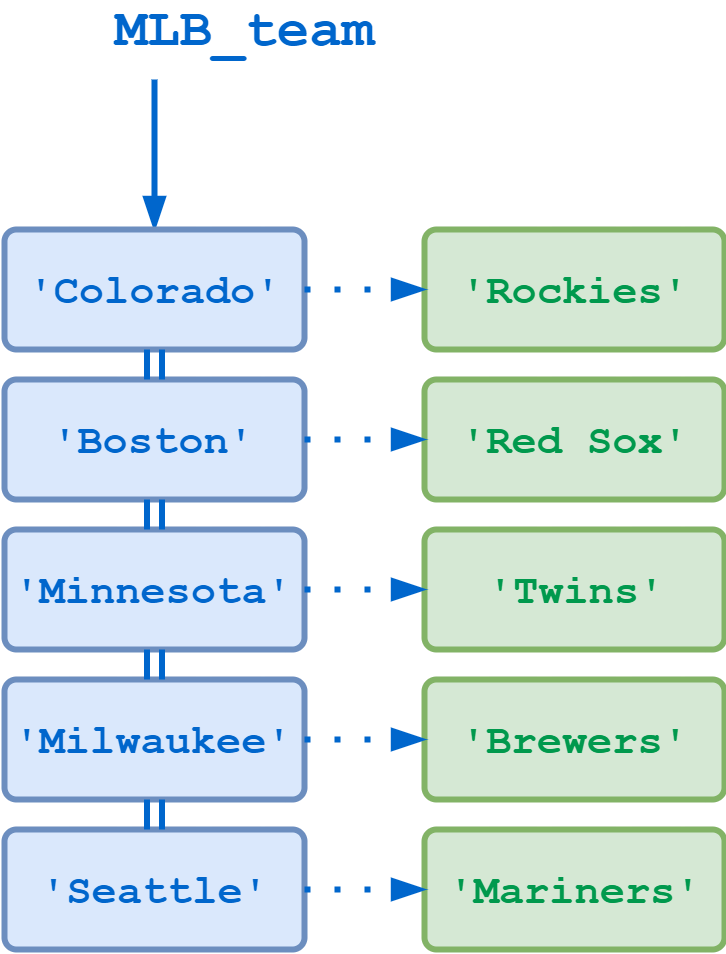


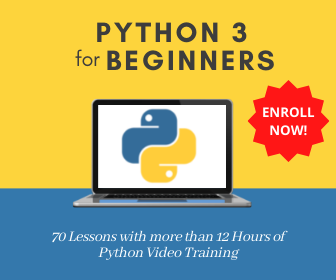

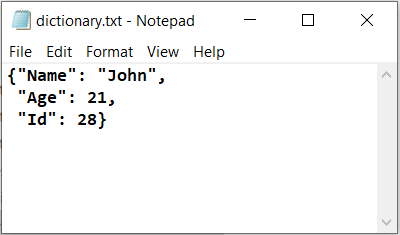
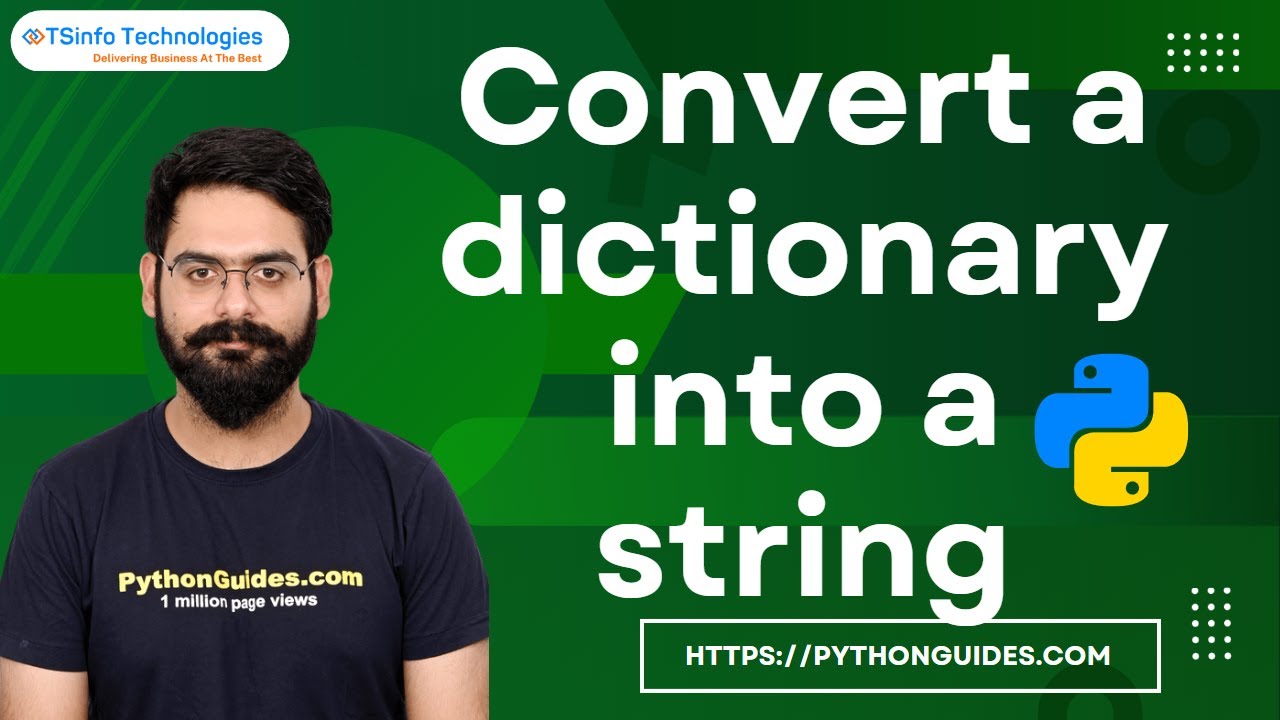
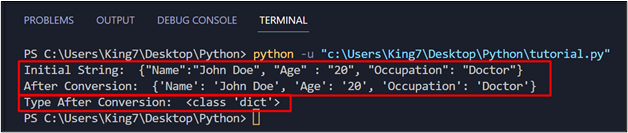
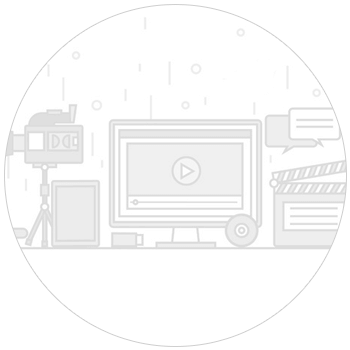

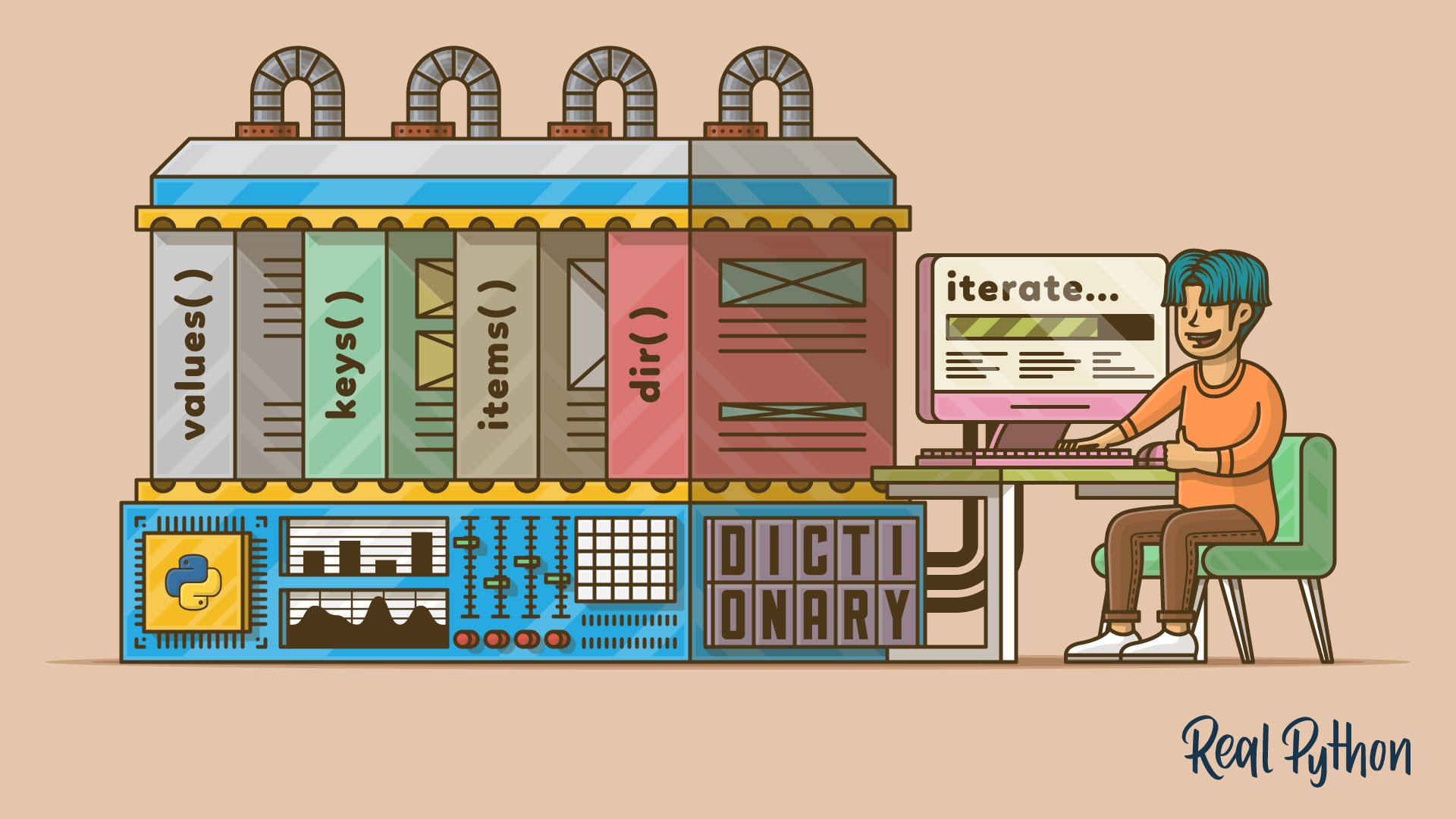
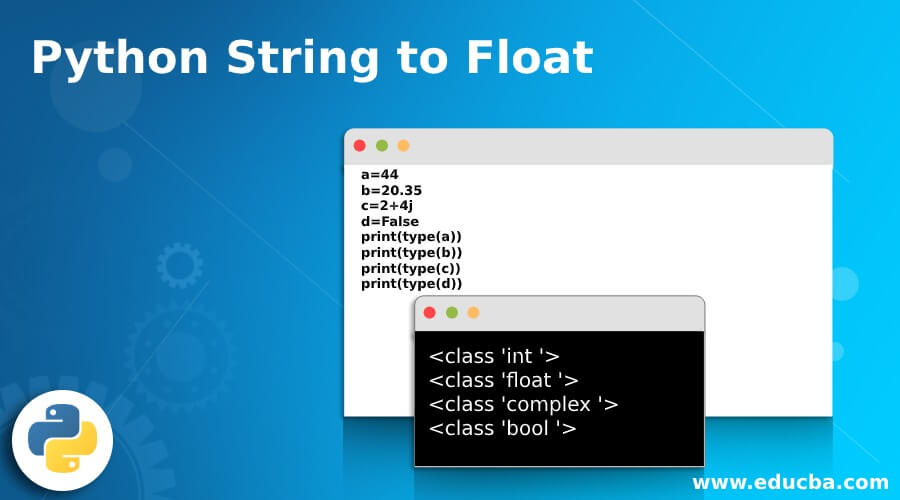
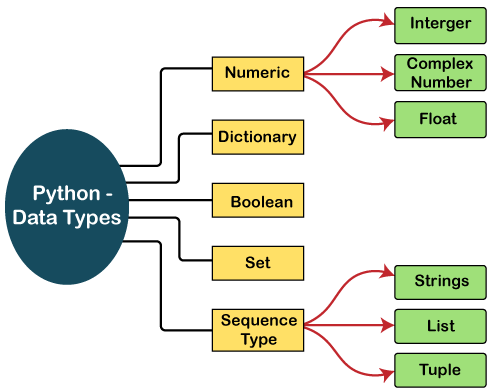
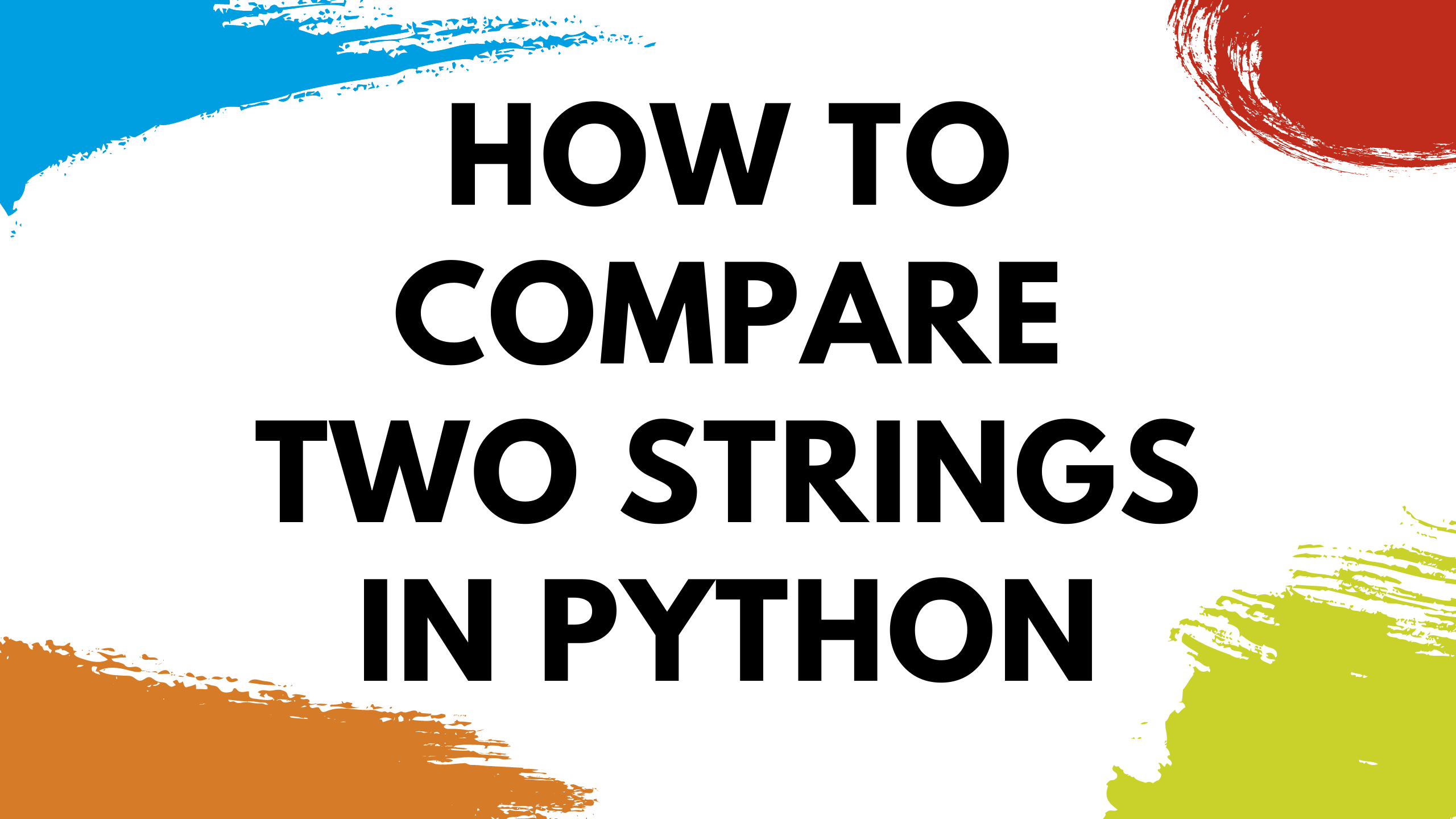
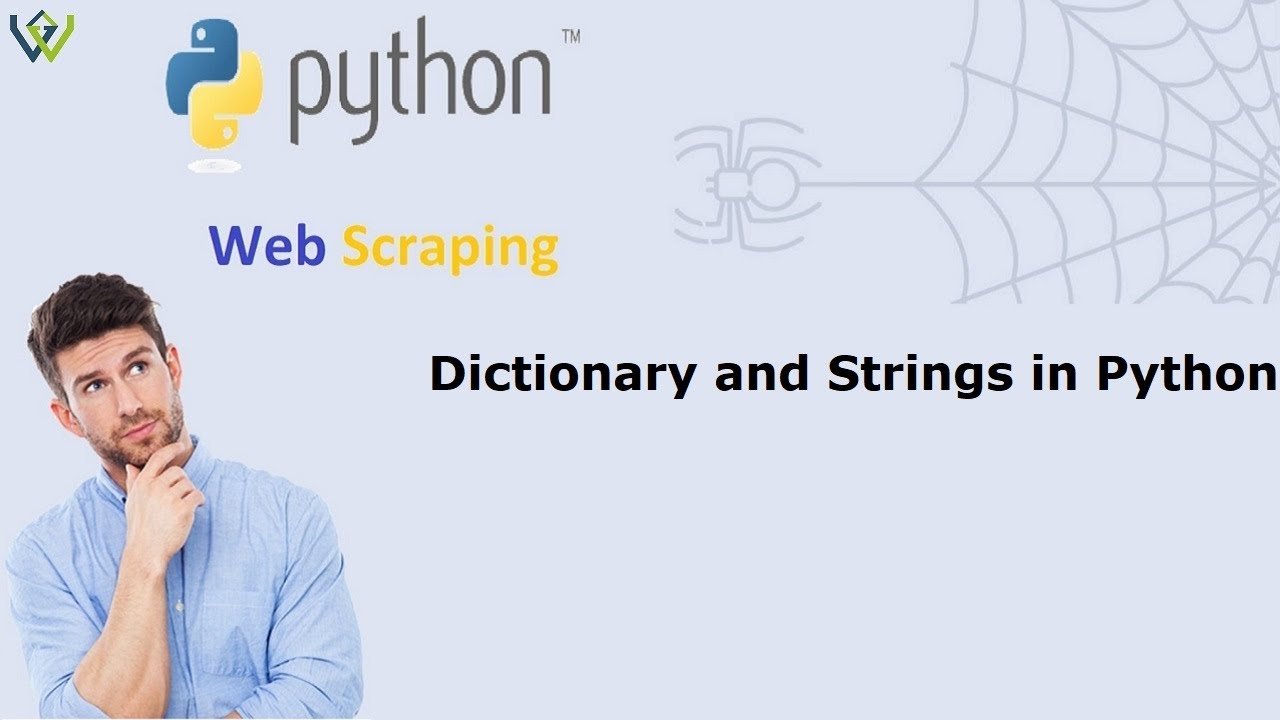
Article link: string to dictionary python.
Learn more about the topic string to dictionary python.
- Convert String Dictionary to Dictionary Python – GeeksforGeeks
- Convert a String representation of a Dictionary to a dictionary
- Convert String Dictionary to Dictionary Python – GeeksforGeeks
- Python – Convert key-value String to dictionary – GeeksforGeeks
- Convert a List to Dictionary Python – GeeksforGeeks
- Python JSON – How to Convert a String to JSON – freeCodeCamp
- Convert string to dictionary in Python – Javatpoint
- How to Convert String to Dictionary in Python – AppDividend
- Convert String to Dictionary in Python – Scaler Topics
- How to Convert String to Dictionary in Python – Data to Fish
- Python String to a Dict – Linux Hint
- How can I convert a string to a dictionary in Python?
See more: https://nhanvietluanvan.com/luat-hoc/