String To Byte Array In C#
The String data type in C# is used to represent a sequence of characters. It is a widely used data type in C# programming and provides various methods and properties for manipulating and working with strings.
The String class in C#
The String class in C# provides various methods and properties for working with strings. It is an immutable class, meaning that once a string object is created, it cannot be modified. Instead, any operations on a string object create a new string object. Some of the commonly used methods and properties of the String class include:
– Length: Returns the number of characters in the string.
– Substring: Returns a specified portion of the string.
– Replace: Replaces all occurrences of a specified string in the current string.
– ToUpper/ToLower: Converts the string to uppercase or lowercase.
– Concat: Concatenates two or more strings.
– Trim: Removes all leading and trailing white spaces from the string.
Creating a Byte Array in C#
A byte array in C# is a fixed-size collection of bytes. Each byte in the array represents a sequence of 8 bits, with a value ranging from 0 to 255. Byte arrays are commonly used in C# for various purposes, such as storing binary data or representing images and audio files.
To create a byte array in C#, you can use the byte data type and initialize it with a fixed size. For example:
byte[] byteArray = new byte[10];
This code creates a byte array named “byteArray” with a size of 10.
Converting a String to a Byte Array
There are several methods available in C# to convert a string to a byte array. One common approach is to use the Encoding.GetBytes() method, which is provided by the System.Text namespace.
The Encoding.GetBytes() method takes a string as input and returns a byte array that represents the string in the specified character encoding. Here’s an example of how to use this method to convert a string to a byte array:
string inputString = “Hello, World!”;
byte[] byteArray = Encoding.UTF8.GetBytes(inputString);
In this example, the string “Hello, World!” is converted to a byte array using the UTF8 encoding. The resulting byte array can be used for further processing or storage.
Handling character encodings
Character encoding is the process of representing characters as bytes in computer systems. Different character encodings can be used to represent characters in different languages or scripts. In C#, the Encoding class provides various encodings, such as UTF8, UTF16, ASCII, and more.
When converting a string to a byte array, it is important to consider the appropriate character encoding to use. The choice of character encoding depends on the specific requirements of your application and the characters that need to be represented.
Error handling and limitations
When converting a string to a byte array, it is important to handle any potential exceptions that may occur. The common exception that can be thrown during this process is the `EncoderFallbackException`, which occurs when the string contains characters that cannot be represented in the given encoding.
To handle such exceptions, you can use try-catch blocks to catch and handle the exceptions. It is also recommended to use the appropriate character encoding that supports the characters in your string to avoid any potential issues during the conversion process.
Working with Unicode characters
Unicode is a character encoding standard that aims to support all characters from all writing systems. It provides a unique number for every character, regardless of the platform, program, or language.
In C#, the built-in string data type uses Unicode to represent characters. This means that when converting a string to a byte array, the resulting byte array will contain Unicode characters encoded in the specified character encoding.
Use cases and practical examples
There are several use cases where converting a string to a byte array is required. Some common scenarios include:
– Storing and transmitting binary data: When working with binary data, such as images or audio files, converting the data to a byte array allows for efficient storage and transmission.
– Encryption and hashing: Many cryptographic algorithms require input data in the form of byte arrays. Converting a string to a byte array allows for easy integration with these algorithms.
– Network communication: When sending data over a network, it is often necessary to convert strings to byte arrays for efficient transfer.
Best practices and tips
When converting a string to a byte array in C#, there are several best practices and tips to consider:
– Choose the appropriate character encoding based on the characters you need to represent.
– Handle exceptions that may occur during the conversion process.
– Be mindful of the memory usage, especially when working with large strings or frequently converting strings to byte arrays.
– Be aware of potential security vulnerabilities, such as buffer overflows or injection attacks, when dealing with user input.
In conclusion, converting a string to a byte array in C# is a common task in many applications. Understanding the methods and considerations involved in this process can help you efficiently convert strings to byte arrays and handle any potential issues that may arise.
FAQs:
Q: What is the purpose of converting a string to a byte array in C#?
A: Converting a string to a byte array is necessary in various scenarios, such as storing and transmitting binary data, encryption and hashing, and network communication.
Q: How can I convert a string to a byte array in C# using the Encoding.GetBytes() method?
A: You can use the Encoding.GetBytes() method provided by the System.Text namespace. This method takes a string as input and returns a byte array that represents the string in the specified character encoding.
Q: What character encodings are commonly used in C#?
A: C# provides various character encodings, such as UTF8, UTF16, ASCII, and more. The choice of character encoding depends on the specific requirements of your application and the characters that need to be represented.
Q: Are there any limitations or potential issues when converting a string to a byte array in C#?
A: One common potential issue is the `EncoderFallbackException`, which occurs when the string contains characters that cannot be represented in the given encoding. It is important to handle such exceptions and use the appropriate character encoding to avoid any potential issues.
C# Programmers Faq – 008 String To Byte Array Ascii #Programming #Developer #Csharp #Dotnet #Shorts
How To Convert A String Into Byte Array?
In programming, it is often necessary to convert data from one type to another. One common conversion that developers often encounter is the process of converting a string into a byte array. In this article, we will explore the various methods and techniques used to accomplish this task.
Understanding Strings and Byte Arrays
Before delving into the conversion process, it is crucial to understand the two types of data being dealt with: strings and byte arrays.
A string is a sequence of characters, such as “Hello, World!” or “12345”. It is a common data type used to represent textual data in programming languages. Strings are often immutable, meaning they cannot be modified once created.
On the other hand, a byte array is a contiguous block of memory used to store binary data. Each element of the array represents a single byte, typically an 8-bit value. Byte arrays are mutable and can be modified.
Methods for Converting a String into a Byte Array
There are several approaches to convert a string into a byte array in various programming languages. Let’s explore some of the most commonly used methods.
Method 1: Using the getBytes() Method
The most straightforward way to convert a string into a byte array is by using the getBytes() method provided by most programming languages. This method converts a string into an array of bytes using the character encoding specified.
Here’s an example in Java:
“`java
String myString = “Hello, World!”;
byte[] byteArray = myString.getBytes();
“`
In this example, the getBytes() method converts the string “Hello, World!” into a byte array, storing it in the byteArray variable.
Method 2: Using Encoding Techniques
Another way to convert a string into a byte array is by utilizing encoding techniques. This method allows you to specify the character encoding explicitly. One commonly used encoding is UTF-8.
Here’s an example in C#:
“`csharp
string myString = “Hello, World!”;
byte[] byteArray = Encoding.UTF8.GetBytes(myString);
“`
In this C# example, the GetBytes() method from the Encoding class converts the string “Hello, World!” into a byte array using UTF-8 encoding.
Method 3: Using a Stream
Using streams can also be an effective way to convert a string into a byte array, especially when dealing with larger amounts of data. This method involves writing the string to a memory stream and then obtaining the byte array from it.
Here’s an example in Python:
“`python
import io
myString = “Hello, World!”
stream = io.BytesIO(myString.encode())
byteArray = stream.getvalue()
“`
In this Python example, the BytesIO class from the io module is used to create a memory stream. The string “Hello, World!” is encoded and written to the stream using the encode() method. Finally, the getvalue() method retrieves the byte array from the stream.
FAQs about Converting a String into a Byte Array
Q: Are all characters of a string converted to bytes?
A: Yes, when converting a string into a byte array, each character in the string is converted into its corresponding byte value.
Q: Which character encoding should I use?
A: The choice of character encoding depends on the requirements of your project. UTF-8 is widely used and supports a broad range of characters, making it a safe choice in most cases.
Q: Does converting a string to a byte array preserve special characters?
A: Yes, when using the appropriate character encoding, special characters present in the string will be preserved in the resulting byte array.
Q: Can I get the original string back from a byte array?
A: Yes, you can convert a byte array back to a string using the appropriate decoding techniques. The reverse process involves converting each byte in the array to its corresponding character.
Q: How can I handle unsupported characters during conversion?
A: When converting a string into a byte array, unsupported characters may cause exceptions or incorrect conversions. It is essential to handle such scenarios by using appropriate error handling techniques.
Conclusion
Converting a string into a byte array is a common task in programming. By understanding the different methods discussed in this article, you can now convert strings into byte arrays using the most suitable technique for your specific programming language. Remember to consider character encoding, handle exceptions, and choose the method that best fits your project’s requirements.
How To Get Byte Array From String?
In the world of programming, it is often necessary to convert data from one format to another. This holds true when attempting to convert a string into a byte array. Converting a string to a byte array can be crucial in many scenarios, such as when sending data over a network or when storing data in a binary file.
In this article, we will delve into the various methods and techniques that can be utilized to convert a string into a byte array. We will cover both the manual and library-assisted approaches, ensuring that you have a comprehensive understanding of the process. So, let’s begin!
Manual Conversion Methods:
There are several manual conversion methods that can be used to convert a string to a byte array. These methods involve iterating through each character of the string and converting them to their corresponding ASCII values.
1. Using the getBytes Method:
One of the simplest ways to convert a string into a byte array is by leveraging the built-in Java method `getBytes()`. This method can be called on a string object and it provides a straightforward way to convert the string into a byte array. The `getBytes()` method takes an optional charset argument to specify the character encoding if required.
“`java
String str = “Example String”;
byte[] byteArray = str.getBytes();
“`
2. Using the toCharArray Method:
Another manual approach involves using the `toCharArray()` method to convert the string into a character array. Once the string is converted into a character array, each character can be individually cast into a byte value using type casting.
“`java
String str = “Example String”;
char[] charArray = str.toCharArray();
byte[] byteArray = new byte[charArray.length];
for(int i = 0; i < charArray.length; i++) { byteArray[i] = (byte) charArray[i]; } ``` Using Library-Assisted Conversion Methods: Various libraries provide helpful methods to simplify the process of converting a string to a byte array. These libraries often offer additional functionality, such as handling character encodings and complex character sets. 1. Using the Apache Commons Codec Library: The Apache Commons Codec library is a widely-used library that provides many encoding and decoding utilities. To convert a string into a byte array, we can utilize the `StringUtils.getBytesUtf8()` method from the library. ```java import org.apache.commons.codec.binary.StringUtils; String str = "Example String"; byte[] byteArray = StringUtils.getBytesUtf8(str); ``` 2. Using Java's StandardCharsets: From Java 7 onwards, the `StandardCharsets` class was introduced, which provides convenient constants for character encodings. The `StandardCharsets` class can be used to convert a string to a byte array using the `getBytes()` method. ```java import java.nio.charset.StandardCharsets; String str = "Example String"; byte[] byteArray = str.getBytes(StandardCharsets.UTF_8); ``` FAQs: Q1. Why do we need to convert a string into a byte array? A1. There are various scenarios where the conversion of a string into a byte array is required. For instance, when working with network communication, it is common to send data in the form of byte arrays. Additionally, when storing data in binary format, byte arrays are often utilized. Converting strings to byte arrays allows for more efficient data handling in such situations. Q2. Can we directly convert a string to a byte array without any manual or library-assisted methods? A2. Technically, a string can be directly converted into a byte array by manually assigning each element of the byte array the ASCII value of the respective character in the string. However, this approach is not recommended as it can be error-prone and inefficient. Utilizing language-specific methods or libraries streamlines the process and ensures accurate results. Q3. How does character encoding affect the conversion process? A3. Character encoding plays a significant role in string to byte array conversion, especially when dealing with non-ASCII characters. Different character encodings handle characters differently, and it is crucial to ensure that the chosen encoding matches the expected format. Using libraries or language-specific functions that handle character encoding internally, such as `getBytes()` or libraries like Apache Commons Codec, is recommended to avoid complications related to character encoding. Q4. Are there any performance considerations when converting a string to a byte array? A4. The performance impact of string to byte array conversion depends on the size of the string and the specific method used for conversion. In general, the built-in Java methods and libraries provide high-performance solutions optimized for such conversions. However, it is always advisable to benchmark and optimize performance-critical sections of code if needed. In conclusion, converting a string into a byte array is a common task in programming. It is essential to understand and implement suitable techniques to ensure accurate and efficient conversion. By utilizing language-specific methods or libraries, developers can simplify the conversion process and handle complexities related to character encoding effectively.
Keywords searched by users: string to byte array in c# String to byte array C#, Hex string to byte array in C, String to byte c, Convert string to byte C#, convert string to byte[] c#, String to byte online, Int to byte in C, Convert string to byte 32 c#
Categories: Top 34 String To Byte Array In C#
See more here: nhanvietluanvan.com
String To Byte Array C#
In development projects, it is often necessary to convert a string into a byte array. This conversion can be required for various reasons, such as encoding or encryption purposes. In the C# programming language, several methods and functions are available for converting strings to byte arrays efficiently and conveniently. This article will explore these techniques in detail and provide a comprehensive understanding of the process.
Methods for String to Byte Array Conversion:
The .NET Framework offers several methods to convert strings to byte arrays in C#. Let’s delve into each of these methods one by one.
1. Encoding.GetBytes Method:
The most common method used for string to byte array conversion is the GetBytes method available in the Encoding class. This method is widely used as it provides support for various character encodings, including ASCII, UTF-8, and Unicode. Here’s an example of how to use this method:
“`
string inputString = “Hello World!”;
byte[] byteArray = Encoding.ASCII.GetBytes(inputString);
“`
2. BitConverter.GetBytes Method:
Another approach to convert a string to a byte array is by utilizing the BitConverter class. This method is useful when the string consists of numeric values or represents a series of bytes in a specific format. Consider the following example:
“`
string inputString = “12345”;
byte[] byteArray = BitConverter.GetBytes(int.Parse(inputString));
“`
3. Encoding.Unicode.GetBytes Method:
In situations where you need to convert a string to a byte array using the Unicode encoding scheme, the Encoding.Unicode.GetBytes method can be employed. This method ensures that the byte array represents the string using the Unicode character set.
“`
string inputString = “Hello World!”;
byte[] byteArray = Encoding.Unicode.GetBytes(inputString);
“`
4. Other Encoding Schemes:
Apart from ASCII and Unicode, C# provides support for several other character encodings, including UTF-8, UTF-32, UTF-7, and more. You can use the respective encoding’s GetBytes method to convert a string to a byte array in the desired encoding scheme.
FAQs:
Q1. Can you provide an example of converting a byte array back to a string in C#?
Certainly! Reversing the process and converting a byte array back to a string is also possible in C#. Here’s a simple example:
“`
byte[] byteArray = { 72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100, 33};
string outputString = Encoding.ASCII.GetString(byteArray);
“`
Q2. How does the UTF-8 encoding differ from ASCII?
ASCII represents characters using 7 bits, with a total of 128 possible characters. On the other hand, UTF-8 is a variable-length character encoding scheme that can represent any Unicode character. UTF-8 is backward compatible with ASCII, meaning that ASCII characters can be represented in UTF-8 using a single byte.
Q3. Are these methods efficient for large strings?
The efficiency of these methods depends on the size of the string being converted. If the string is extremely large, the conversion process may consume significant memory and processing resources. In such cases, it is advisable to convert the string in chunks instead of performing the conversion on the entire string at once.
Q4. Can byte arrays be converted to strings using different encodings?
Yes, byte arrays can be converted back to strings using the appropriate encoding scheme. For example, if a byte array represents a string encoded in UTF-8, you can use Encoding.UTF8.GetString to convert it back to a string.
In conclusion, converting a string to a byte array is a common requirement in C# development. This article explored several methods available in the .NET Framework to accomplish this task, including Encoding.GetBytes, BitConverter.GetBytes, and Encoding.Unicode.GetBytes. Additionally, we covered some frequently asked questions related to this topic. By understanding these techniques and considering the specific encoding requirements, developers can efficiently convert strings to byte arrays in their C# applications.
Hex String To Byte Array In C
Converting a hex string to a byte array in C involves transforming each hex character into its corresponding byte representation. A hex string is composed of hexadecimal digits ranging from 0 to 9 and A to F, representing values from 0 to 15. Each hex digit corresponds to 4 bits of data, and combining two hex digits creates a byte consisting of 8 bits.
To start, we need to define the size of the byte array. It will be half the length of the hex string since two hex digits represent one byte. We can use the `strlen` function from the `string.h` library to determine the length of the hex string.
“`c
#include
#include
int main() {
char hexString[] = “DEADBEEF”;
int stringLength = strlen(hexString);
int byteArraySize = stringLength / 2;
}
“`
Now that we have obtained the size of the byte array, we can proceed with the conversion. One approach is to iterate over the hex string, processing two characters at a time, and converting them into bytes using the `sscanf` function. The `sscanf` function can extract formatted data from a string and store it in variables.
“`c
#include
#include
int main() {
char hexString[] = “DEADBEEF”;
int stringLength = strlen(hexString);
int byteArraySize = stringLength / 2;
unsigned char byteArray[byteArraySize];
for (int i = 0; i < stringLength; i += 2) {
sscanf(&hexString[i], "%2hhx", &byteArray[i / 2]);
}
// The byte array is ready to be used
}
```
In this example, we declare an unsigned char byte array of appropriate size to store the converted values. The loop iterates over the hex string, incrementing `i` by 2 on each iteration to process two characters at a time. The `sscanf` function is responsible for converting the two hex characters into a byte, using the `%2hhx` format specifier to indicate the desired conversion.
After the loop finishes, the byte array contains the converted values, ready to be utilized in further operations.
Another method to convert a hex string to a byte array is by using bitwise operations. In this approach, each hex digit is transformed into its numeric representation using arithmetic operations. By shifting the bits appropriately, the hex digits can be combined to form bytes.
```c
#include
#include
int hexCharToDecimal(char c) {
if (c >= ‘0’ && c <= '9') {
return c - '0';
}
else if (c >= ‘A’ && c <= 'F') {
return c - 'A' + 10;
}
else if (c >= ‘a’ && c <= 'f') {
return c - 'a' + 10;
}
return -1; // Invalid hex digit
}
int main() {
char hexString[] = "DEADBEEF";
int stringLength = strlen(hexString);
int byteArraySize = stringLength / 2;
unsigned char byteArray[byteArraySize];
for (int i = 0; i < stringLength; i += 2) {
int highNibble = hexCharToDecimal(hexString[i]);
int lowNibble = hexCharToDecimal(hexString[i + 1]);
if (highNibble == -1 || lowNibble == -1) {
printf("Invalid hex digit encountered!");
return -1;
}
unsigned char byte = (highNibble << 4) | lowNibble;
byteArray[i / 2] = byte;
}
// The byte array is ready to be used
}
```
In this example, we introduce a helper function, `hexCharToDecimal`, which takes a hex character as input and returns its decimal value. This function handles both uppercase and lowercase hex digits.
Similar to the previous approach, we declare the byte array, iterate over the hex string, and process two characters at a time. Instead of `sscanf`, we manually convert the hex characters to decimal values using `hexCharToDecimal`. Then, the high nibble (first hex digit) is shifted left by 4 bits (`<< 4`) and combined with the low nibble (second hex digit) through bitwise OR (`|`).
Now, let's address some frequently asked questions related to converting a hex string to a byte array in C:
**Q: Can I convert a hex string with an odd number of characters to a byte array?**
A: No, since two hex digits are required to form a byte, a hex string must have an even number of characters. If you have an odd-length hex string, you must pad it with a leading zero.
**Q: What is the maximum length of a hex string that can be converted to a byte array?**
A: The maximum length of the hex string depends on the available memory in the system. However, keep in mind that the resultant byte array will be half the size of the hex string since two hex digits create one byte.
**Q: How can I validate the input hex string to ensure it contains only valid characters?**
A: To validate the input hex string, you can check each character individually using the `hexCharToDecimal` helper function mentioned earlier. If any character returns a value of -1, it means an invalid hex digit was encountered.
**Q: Are there any libraries in C that simplify the process of converting hex strings to byte arrays?**
A: There are no built-in libraries in C that provide direct functions for hex string to byte array conversion. However, you can utilize third-party libraries or implement your own helper functions, as demonstrated earlier.
In conclusion, converting a hex string to a byte array in C involves transforming each hex digit into its corresponding byte representation using different techniques. By implementing the methods discussed in this article, you can efficiently convert hex strings to byte arrays for further processing or storage in your C programs.
String To Byte C
Introduction:
In C programming, handling string manipulation and byte conversion is a fundamental task for developers. Understanding the process of converting strings to bytes or vice versa is essential when working with networks, file I/O operations, and cryptography. This article aims to provide a thorough understanding of string to byte conversion in the C programming language, explaining relevant concepts and offering practical examples.
1. String to Byte Conversion:
1.1 ASCII Encoding:
In C, characters are internally represented as ASCII values. To convert a string to a byte array, ASCII encoding is the simplest approach. ASCII encoding assigns a fixed numerical value to each character using the ASCII table, ranging from 0 to 255.
1.2 Conversion Steps:
To convert a string to bytes in C, one can iterate through each character in the string, assign the corresponding ASCII code to each byte in the byte array, and terminate the array with a null byte. The null byte ensures that the end of the string is marked.
2. Implementing String to Byte Conversion in C:
For a better understanding, let’s consider an example that converts a string to bytes in C:
“`c
#include
int main() {
char str[] = “hello”;
unsigned char bytes[sizeof(str)];
for (int i = 0; i < sizeof(str); ++i) { bytes[i] = str[i]; printf("%d ", bytes[i]); } return 0; } ``` Explanation: 1. We define a string `str` and an array `bytes` of unsigned characters to store the individual bytes. 2. A for loop is used to iterate through each character in the string. 3. Each character `str[i]` is then assigned to `bytes[i]`, converting the character to its corresponding ASCII value. 4. The final step involves printing the ASCII values of each byte. 3. Frequently Asked Questions (FAQs): Q1. Can we convert bytes back to a string in C? A1. Yes, bytes can be converted back to a string in C using the ASCII value of each byte. You can loop through the bytes, assigning each byte to a corresponding character using the `char` data type. Once all the bytes are combined, you will have a string. Q2. How can strings with non-ASCII characters be converted to bytes? A2. Converting strings with non-ASCII characters (e.g., Unicode characters) to bytes requires a different approach. The standard ASCII encoding does not support non-ASCII characters. Instead, you can use Unicode encodings like UTF-8 or UTF-16, which offer broader character support. Libraries, such as iconv or ICU, provide functionalities for converting between various string encodings. Q3. Are there any limitations to using ASCII encoding for string to byte conversion? A3. While ASCII encoding is suitable for strings comprising only ASCII characters, it fails to represent characters from non-ASCII character sets. Additionally, it cannot handle multi-byte encodings or characters requiring more than one byte to store their representation. Q4. What are some other encoding schemes available for string to byte conversion? A4. Besides ASCII encoding, other popular encoding schemes include UTF-8, UTF-16, and ISO-8859-1 (Latin-1), among others. These encodings provide extensive support for different languages, including characters from various character sets. Q5. How can I verify the accuracy of my string to byte conversion? A5. To verify the accuracy of your string to byte conversion, you can compare the byte array's content against the original string. Loop through each byte and compare them against the corresponding characters in the original string. Ensure that the ASCII values match, including any special characters or whitespace within the string. Conclusion: Understanding string to byte conversion in C is essential for various programming tasks. Although the ASCII encoding approach provided a simple example, developers should consider alternative encodings for broader character support. By grasping the concepts covered in this article, C programmers can confidently manipulate strings and bytes, facilitating numerous applications across networking, file I/O, and cryptography.
Images related to the topic string to byte array in c#
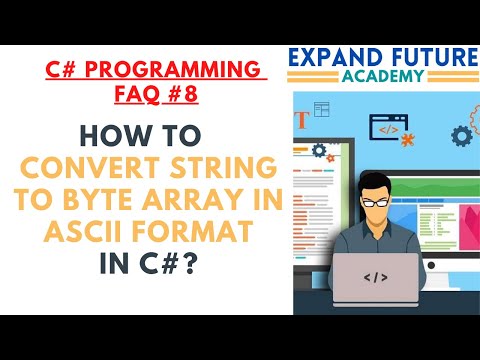
Article link: string to byte array in c#.
Learn more about the topic string to byte array in c#.
- Convert ASCII string (char[]) to BYTE array in C
- How to Convert String To Byte Array in C# – C# Corner
- Hexadecimal string to byte array in C – Stack Overflow
- String to byte array, byte array to String in Java | DigitalOcean
- How to convert a string to a byte array in C# – Dofactory
- Convert a String to Integer Array in C/C++ – GeeksforGeeks
- Converting a String to its Equivalent Byte Array in C# – GeeksforGeeks
- How to convert a string to a byte array in C# – Dofactory
- How to convert a string into bytes in C – Quora
- HELP PLEASE – How to convert string to byte array – C / C++
- Converting String To Byte Array In C# – A Comprehensive Guide
- Convert string to byte array in C++ – thisPointer
- Convert char * String to System::Byte Array – Microsoft Learn
- C# String to Byte Array – Linux Hint
See more: https://nhanvietluanvan.com/luat-hoc/