String To Byte Array C#
Working with string and byte arrays is a common task in programming, especially when dealing with data encoding and storage. In C#, there are various methods and options available to convert a string to a byte array, and vice versa. In this article, we will explore different approaches to achieve this conversion, and also discuss some common issues that may arise during the process.
Declaring a String
Before we dive into the conversion methods, let’s first understand how to declare a string in C#. In C#, string is a built-in data type that represents a sequence of characters. You can declare a string variable using the keyword “string” followed by the variable name, as shown below:
“`csharp
string myString = “Hello, World!”;
“`
The above line declares a string variable named “myString” and assigns the value “Hello, World!” to it.
Converting a String to a Byte Array in C#
Now, let’s explore some of the methods available to convert a string to a byte array in C#.
1. Using the GetBytes Method in the Encoding Class
The Encoding class in C# provides a method called GetBytes, which can be used to convert a string to a byte array. This method automatically uses the default encoding (UTF-8) to perform the conversion. Here’s an example:
“`csharp
string myString = “Hello, World!”;
byte[] byteArray = Encoding.Default.GetBytes(myString);
“`
In the above code, we convert the string “Hello, World!” to a byte array using the GetBytes method of the Encoding class. The resulting byte array will contain the UTF-8 encoded representation of the string.
2. Using the GetBytes Method in Specific Encoding Classes
In addition to the default encoding, C# provides specific encoding classes such as UTF8Encoding, UnicodeEncoding, and ASCIIEncoding. These classes can also be used to convert a string to a byte array, allowing you to have more control over the encoding. Here’s an example using the UTF8Encoding class:
“`csharp
string myString = “Hello, World!”;
byte[] byteArray = new UTF8Encoding(true).GetBytes(myString);
“`
In the above code, we create an instance of the UTF8Encoding class with the constructor parameter “true”, which indicates that a byte order mark (BOM) should be included in the byte array. This ensures that the resulting byte array is properly encoded as UTF-8.
3. Converting a String Directly to a Byte Array using ASCII Encoding
If you specifically want to convert a string to a byte array using ASCII encoding, you can use the ASCIIEncoding.GetBytes method directly. Here’s an example:
“`csharp
string myString = “Hello, World!”;
byte[] byteArray = ASCIIEncoding.ASCII.GetBytes(myString);
“`
In the above code, we convert the string “Hello, World!” to a byte array using the ASCIIEncoding.GetBytes method. The resulting byte array will contain the ASCII encoded representation of the string.
4. Converting a String to a Byte Array using Other Encoding Options
Apart from the default encoding and specific encoding classes, C# provides numerous other encoding options. Some of the commonly used ones include UTF32Encoding, BigEndianUnicodeEncoding, and UnicodeEncoding. You can utilize these encoding options accordingly to convert a string to a byte array. Here’s an example using the UTF32Encoding class:
“`csharp
string myString = “Hello, World!”;
byte[] byteArray = new UTF32Encoding(true, true).GetBytes(myString);
“`
In the above code, we create an instance of the UTF32Encoding class with two constructor parameters set to “true”. This ensures that both a byte order mark (BOM) and big-endian byte order should be included in the byte array.
Converting a Byte Array to a String in C#
Now, let’s explore some methods to convert a byte array back to a string in C#.
1. Using the GetString Method in the Encoding Class
The Encoding class in C# provides a method called GetString, which can be used to convert a byte array to a string. This method automatically uses the default encoding (UTF-8) to perform the conversion. Here’s an example:
“`csharp
byte[] byteArray = { 72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33 };
string myString = Encoding.Default.GetString(byteArray);
“`
In the above code, we convert the byte array { 72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33 } to a string using the GetString method of the Encoding class. The resulting string will be “Hello, World!”.
2. Using Specific Encoding Classes to Convert Byte Array to String
Similar to converting string to byte array, you can use specific encoding classes to convert a byte array to a string, providing more control over the encoding. Here’s an example using the UTF8Encoding class:
“`csharp
byte[] byteArray = { 72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33 };
string myString = new UTF8Encoding().GetString(byteArray);
“`
In the above code, we create an instance of the UTF8Encoding class and use its GetString method to convert the byte array to a string. The resulting string will be “Hello, World!”.
3. Converting a Byte Array to String using Other Encoding Options
Just like converting a string to a byte array, you can utilize other encoding options to convert a byte array to a string in C#. Here’s an example using the UTF32Encoding class:
“`csharp
byte[] byteArray = { 0, 0, 0, 72, 0, 0, 0, 101, 0, 0, 0, 108, 0, 0, 0, 108, 0, 0, 0, 111, 0, 0, 0, 44, 0, 0, 0, 32, 0, 0, 0, 87, 0, 0, 0, 111, 0, 0, 0, 114, 0, 0, 0, 108, 0, 0, 0, 100, 0, 0, 0, 33, 0, 0, 0 };
string myString = new UTF32Encoding(true, true).GetString(byteArray);
“`
In the above code, we create an instance of the UTF32Encoding class with two constructor parameters set to “true”. This ensures that both a byte order mark (BOM) and big-endian byte order are correctly interpreted while converting the byte array to a string. The resulting string will be “Hello, World!”.
Handling Encoding Issues when Converting to Byte Array and String
During the conversion process, it is important to consider the original encoding of the string and handle any encoding issues that may arise. Here are some key points to keep in mind:
1. Considering the Original Encoding of the String
When converting a string to a byte array, it is essential to be aware of the original encoding of the string. If you are unsure about the encoding, using the default encoding or specifying a specific encoding option can help in achieving accurate results.
2. Handling Characters that are not Supported by the Selected Encoding
Some characters may not be supported by certain encodings. As a result, during the conversion process, these unsupported characters may be replaced with question marks or other placeholders. To avoid such issues, ensure that the selected encoding supports the full range of characters used in the string.
3. Dealing with Different Endianness when Converting to Byte Array and Back to String
Endianness refers to the order in which bytes are stored in the memory. Some encoding options, such as UTF-16, can have different endianness, which affects how byte arrays are interpreted. When converting a string to a byte array, and vice versa, it is crucial to use the correct endianness to ensure accurate conversions.
Working with Multi-Byte Characters
Multi-byte characters are characters that are represented by more than one byte in an encoding. They are commonly used in languages with complex character sets, such as Chinese, Japanese, and Korean. When converting strings containing multi-byte characters to byte arrays and vice versa, some additional considerations need to be made.
1. Understanding the Concept of Multi-Byte Characters
In encodings such as UTF-8 or UTF-16, multi-byte characters are represented by a sequence of bytes. The number of bytes used to represent a character depends on the character itself.
2. Handling Multi-Byte Characters when Converting String to Byte Array and Vice Versa
When converting a string that contains multi-byte characters to a byte array, make sure that the encoding used properly represents these characters as a sequence of bytes. Similarly, when converting a byte array back to a string, ensure that the correct encoding is used to interpret the multi-byte characters correctly.
3. Managing Multi-Byte Character Encoding Issues
As mentioned earlier, not all encoding options support the entire range of characters. When working with multi-byte characters, it becomes even more crucial to select an encoding that supports the specific character set used in the string. Failure to do so may result in data loss or incorrect interpretation of the characters.
Applying Byte Order Mark (BOM)
The byte order mark (BOM) is a special marker at the beginning of a file or a byte array that indicates the encoding used. Its purpose is to identify the byte order (endianness) and the encoding of the data. Here’s how you can apply BOM to a byte array or a string when necessary:
1. Understanding the Purpose of Byte Order Mark (BOM)
The BOM is particularly important in encodings such as UTF-16 and UTF-32, which can have different endianness. It helps specify whether the encoding uses little-endian or big-endian byte order.
2. Adding BOM to Byte Array or String when Necessary
To add a BOM to a byte array, you need to prepend the appropriate byte sequence at the beginning. For example, the UTF-8 BOM is represented by the bytes { 0xEF, 0xBB, 0xBF }. To add the UTF-8 BOM to a byte array, you can use the Concat method of the Enumerable class, as shown below:
“`csharp
byte[] byteArray = Encoding.UTF8.GetBytes(“Hello, World!”);
byte[] byteArrayWithBOM = new byte[] { 0xEF, 0xBB, 0xBF }.Concat(byteArray).ToArray();
“`
In the above code, we first convert the string “Hello, World!” to a byte array using UTF-8 encoding. Then, we create a new byte array by concatenating the UTF-8 BOM byte sequence and the original byte array.
To add a BOM to a string, you can use the GetString method of the Encoding class and pass the appropriate byte sequence as a parameter. Here’s an example:
“`csharp
byte[] byteArray = Encoding.UTF8.GetBytes(“Hello, World!”);
string myStringWithBOM = Encoding.UTF8.GetString(new byte[] { 0xEF, 0xBB, 0xBF }) + Encoding.UTF8.GetString(byteArray);
“`
In the above code, we first convert the byte array to a string using UTF-8 encoding. Then, we concatenate the UTF-8 BOM string with the original string.
3. Handling BOM when Converting between Byte Array and String
When converting between byte arrays and strings, it is important to handle the presence of a byte order mark (BOM) correctly. If the byte array contains a BOM, make sure that the appropriate encoding class is used to interpret the byte sequence correctly. Similarly, when converting a string to a byte array, ensure that the existing BOM (if any) is properly handled.
Using Other Libraries and Frameworks for String to Byte Array Conversions
Apart from the built-in methods and classes provided by C#, there are various external libraries and frameworks available that can be used for string to byte array conversions. These libraries often offer additional features and performance improvements. Here are a few examples:
– Newtonsoft.Json: This popular JSON library provides methods to convert strings to byte arrays and vice versa, along with extensive support for JSON serialization and deserialization.
– System.Net.WebUtility: This class in the System.Net namespace offers methods to encode strings as URL-encoded byte arrays, which can be useful for web-related tasks.
– Google.Protobuf: This library provides powerful tools for working with Protocol Buffers, including methods to convert strings to byte arrays using the Protocol Buffers encoding format.
Advantages and Disadvantages of Using External Libraries
Using external libraries for string to byte array conversions offers several advantages, such as:
– Additional features and customization options: External libraries often provide more advanced options and features that may not be available in the built-in methods and classes.
– Performance improvements: Some libraries are specifically optimized for efficient string to byte array conversions, offering better performance compared to the built-in options.
– Simplified code: External libraries can simplify complex encoding tasks and provide cleaner code.
However, there are also some disadvantages to consider when using external libraries:
– Increased dependency: Depending on external libraries means your code will rely on additional dependencies, which may add complexity and maintenance overhead.
– Compatibility issues: External libraries may not be compatible with all versions of C# or other frameworks you are using in your project. Care should be taken to ensure compatibility across versions.
– Learning curve: New libraries often require some learning and understanding before they can be effectively used, which may require additional time and effort.
Demonstrating Efficient String to Byte Array Conversions
To demonstrate efficient string to byte array conversions, let’s use the built-in methods and classes in C#.
First, let’s convert a string to a byte array using UTF-8 encoding:
“`csharp
string myString = “Hello, World!”;
byte[] byteArray = Encoding.UTF8.GetBytes(myString);
“`
In the above code, we use the GetBytes method of the UTF8Encoding class to convert the string “Hello, World!” to a byte array. This approach is efficient and ensures accurate encoding for most scenarios.
Next, let’s convert the byte array back to a string using the UTF8Encoding class:
“`csharp
string convertedString = Encoding.UTF8.GetString(byteArray);
“`
In the above code, we use the GetString method of the UTF8Encoding class to convert the byte array back to a string. This approach maintains the original encoding and accurately represents the string.
Using built-in methods and classes offers a balance between efficiency, accuracy, and ease of use, making it a suitable choice for most string to byte array conversions.
FAQs
Q1: What is the difference between encoding and encoding options in C#?
A1: In C#, encoding refers to the process of converting characters to bytes and vice versa, using a specific character encoding such as UTF-8 or ASCII. Encoding options, on the other hand, refer to the various choices and parameters available for handling character encoding, such as byte order (endianness), presence of byte order marks (BOM), etc.
Q2: Can I convert a hex string to a byte array in C#?
A2: Yes, you can convert a hex string to a byte array in C#. One way to achieve this is by using the Convert class and the ToByte method with the base parameter set to 16. Here’s an example:
“`csharp
string hexString = “48656C6C6F2C20576F726C6421”;
byte[] byteArray = Enumerable.Range(0, hexString.Length)
.Where(x => x % 2 == 0)
.Select(x => Convert.ToByte(hexString.Substring(x, 2), 16))
.ToArray();
“`
In the above code, we use LINQ to iterate through the hex string, convert each pair of characters to a byte, and store them in a byte array.
Q3: Can I convert a byte array to a string in C++?
A3: Yes, you can convert a byte array to a string in C++ using various methods depending on the specific requirements and libraries used. One popular approach is to iterate through the byte array and convert each byte to a character, and then concatenate the characters to form a string object.
Q4: Is there an online tool to convert a string to a byte array?
A4: Yes, there are several online tools available that allow you to convert a string to a byte array. These tools often provide a user-friendly interface where you can input the string and select the desired encoding option. Some examples of online string to byte array converters include base64encode.net, string-functions.com, and onlinehextools.com.
Q5: How can I convert a byte array to a string in C#?
A5: To convert a byte array to a string in C#, you can use the GetString method of the Encoding class along with the appropriate encoding option. For example, to convert a byte array that represents a UTF-8 encoded string, you can use the following code:
“`csharp
byte[] byteArray = {
C# Programmers Faq – 008 String To Byte Array Ascii #Programming #Developer #Csharp #Dotnet #Shorts
How To Convert String To Byte Array In C?
In C programming, converting a string to a byte array is a common task that arises when dealing with various data transmission protocols or cryptographic operations. A byte array is a collection of bytes, which are fundamental units of digital information in computing. Fortunately, C provides several methods and techniques to convert strings to byte arrays efficiently. In this article, we will explore different approaches to achieve this objective and discuss their implications.
Method 1: Using Standard Library Functions
The C standard library offers functions that are widely used and familiar to many developers. One such function is `strcpy()` that copies a string to a character array. By providing an additional argument as the destination byte array, the string can effectively be converted to a byte array. However, it is critical to ensure that the byte array has enough space to accommodate the converted string.
Here is an example demonstrating the usage of `strcpy()`:
“`c
#include
#include
int main() {
char string[] = “Hello, World!”;
unsigned char byteArray[15];
strcpy(byteArray, string);
for (int i = 0; i < strlen(string); i++) {
printf("%02x ", byteArray[i]);
}
return 0;
}
```
This code snippet converts the string "Hello, World!" to a byte array and then prints the hexadecimal representation of each byte. Note that we have used an `unsigned char` array for the byte array, as it ensures that the values are treated as positive integers.
Method 2: Manually Converting Characters to Bytes
Another approach to convert a string to a byte array involves explicitly converting each character to its corresponding byte. This method offers more control and flexibility when dealing with specific encoding schemes or when byte manipulation is necessary.
```c
#include
int main() {
char string[] = “Hello, World!”;
int length = sizeof(string) – 1; // excluding null terminator
unsigned char byteArray[length];
for (int i = 0; i < length; i++) {
byteArray[i] = (unsigned char) string[i];
}
for (int i = 0; i < length; i++) {
printf("%02x ", byteArray[i]);
}
return 0;
}
```
Here, we iterate through each character of the string and cast it to an unsigned char, effectively extracting the byte value. The resulting byte array is then printed in hexadecimal format.
Method 3: Using Memory Copy Functions
Memory copy functions are efficient alternatives when dealing with large strings or complex data structures. The `memcpy()` function, for instance, provides a convenient mechanism to copy raw memory blocks between destinations. This makes it ideal for converting strings to byte arrays without relying on string manipulation techniques.
Consider the following example that utilizes `memcpy()`:
```c
#include
#include
int main() {
char string[] = “Hello, World!”;
int length = strlen(string);
unsigned char byteArray[length];
memcpy(byteArray, string, length * sizeof(unsigned char));
for (int i = 0; i < length; i++) { printf("%02x ", byteArray[i]); } return 0; } ``` Like the previous methods, this code snippet converts the string "Hello, World!" to a byte array. However, instead of using manual character conversions, `memcpy()` is utilized to directly copy the string's memory block into the byte array. Frequently Asked Questions (FAQs): Q: How do I determine the size of a string in C? A: The `strlen()` function is commonly used to determine the length of a string. It returns the number of characters in the string, excluding the null terminator. Q: What is the difference between a string and a byte array? A: In C, a string is typically represented as an array of characters and is terminated by a null character ('\0'). On the other hand, a byte array is a collection of bytes, which can hold any binary data including characters, integers, or other data types. Q: Why is the unsigned char used in converting a string to a byte array? A: The unsigned char data type represents positive integers and ranges from 0 to 255. When converting a string to a byte array, it ensures that each character is treated as a positive byte value. Q: Are there any potential risks or drawbacks when converting strings to byte arrays? A: It is crucial to ensure that the byte array has sufficient space to hold the converted string. Failure to allocate adequate memory may lead to buffer overflows or loss of data. Additionally, when dealing with non-ASCII characters or specific encodings, other considerations may arise. In conclusion, converting a string to a byte array in C can be accomplished through various methods, such as using standard library functions, manually converting characters to bytes, or employing memory copy functions. Each approach offers its own advantages depending on the specific requirements of the application. By understanding these techniques, developers can effectively perform string-to-byte array conversions while ensuring data integrity and security.
How To Convert A String Into Byte Array?
Converting a string into a byte array is a common task in programming, especially when working with network protocols, encryption algorithms, or file operations. A byte array represents a sequence of bytes that can be easily manipulated and stored efficiently. In this article, we will discuss various methods to convert a string into a byte array in several programming languages. So, let’s dive into the details and explore this topic in depth.
1. Java:
Java provides built-in methods to convert strings into byte arrays, making the conversion process straightforward and efficient. The getBytes() method in Java’s String class can be used to convert a string into a byte array. Here’s an example of how to use this method:
“`java
String inputString = “Hello, World!”;
byte[] byteArray = inputString.getBytes();
“`
2. C#:
In C#, converting a string into a byte array can be achieved using the Encoding class, which provides various encoding formats such as UTF8, ASCII, and Unicode. The GetBytes() method of the Encoding class converts the input string into a byte array. Here’s an example:
“`csharp
string inputString = “Hello, World!”;
byte[] byteArray = Encoding.UTF8.GetBytes(inputString);
“`
3. Python:
Python allows us to convert a string into a byte array by encoding the string using the encode() method. By default, it uses the UTF-8 encoding scheme. Here’s an example:
“`python
inputString = “Hello, World!”
byteArray = inputString.encode()
“`
4. JavaScript:
In JavaScript, we can convert a string into a byte array using the TextEncoder() interface, which provides an efficient way to encode strings into byte arrays. Here’s how it can be done:
“`javascript
let inputString = “Hello, World!”;
let encoder = new TextEncoder();
let byteArray = encoder.encode(inputString);
“`
FAQs:
Q1. Why would I need to convert a string into a byte array?
A1. Converting a string into a byte array is often required when dealing with binary data, encryption algorithms, or network protocols. It allows for efficient manipulation and transmission of data.
Q2. Can I specify a specific encoding format when converting a string into a byte array?
A2. Yes, some programming languages offer options to specify the encoding format during the conversion process. For example, in Java, you can pass the desired character set when using the getBytes() method.
Q3. Are there any performance considerations when converting a string into a byte array?
A3. The performance of the conversion depends on the programming language and the size of the string. Generally, the encoding process takes extra time, so it’s essential to consider the efficiency of the chosen method if working with large strings or in performance-critical scenarios.
Q4. How can I convert a byte array back into a string?
A4. Reversing the conversion process is also possible. Most programming languages provide methods or functions to decode a byte array back into a string. For example, in Java, you can use the String class’s constructor that takes a byte array as a parameter.
In conclusion, converting a string into a byte array is a fundamental operation in programming languages, and there are various methods to accomplish this task. Java, C#, Python, and JavaScript offer built-in functionalities that simplify the conversion process. Understanding the conversion process and available options in your preferred programming language empowers you to efficiently handle binary data in your applications.
Keywords searched by users: string to byte array c# String to byte array C#, String to byte c, Hex string to byte array in C, Convert string to byte C#, Convert byte to string C++, String to byte online, Byte to string C#, Char to byte C++
Categories: Top 76 String To Byte Array C#
See more here: nhanvietluanvan.com
String To Byte Array C#
### Converting a String to a Byte Array in C#
There are primarily two methods for converting a string to a byte array: using the ASCII encoding and using the UTF-8 encoding.
### Using ASCII Encoding
The ASCII (American Standard Code for Information Interchange) encoding represents characters using 7 bits and supports only a limited character set of 128 characters. By default, .NET uses the UTF-16 encoding for string representation, but we can explicitly convert it to ASCII if we know that the string contains only ASCII characters. Here is an example of how to convert a string to a byte array using ASCII encoding:
“`csharp
string sampleString = “Hello, World!”;
byte[] byteArray = Encoding.ASCII.GetBytes(sampleString);
“`
### Using UTF-8 Encoding
UTF-8 (Unicode Transformation Format 8-bit) is a widely used encoding that can represent almost all characters in the Unicode standard. It is backward compatible with ASCII and requires a varying number of bytes to represent different characters. To convert a string to a byte array using UTF-8 encoding, the following code snippet can serve as an example:
“`csharp
string sampleString = “Hello, World!”;
byte[] byteArray = Encoding.UTF8.GetBytes(sampleString);
“`
### Conclusion
In this article, we explored the different methods of converting a string to a byte array in C#. We covered the usage of ASCII encoding and UTF-8 encoding, highlighting their differences and use cases. It is important to choose the appropriate encoding method based on the requirements of your project to ensure accurate conversion and compatibility with the target system.
## FAQs (Frequently Asked Questions)
**Q: Can I use other encodings to convert a string to a byte array in C#?**
A: Yes, C# provides support for various other encodings like UTF-16, UTF-32, Unicode, etc. You can use them by simply replacing the Encoding type in the conversion code snippet with the desired encoding.
**Q: What happens if the string contains characters that are not compatible with the chosen encoding?**
A: When converting a string to a byte array, some characters may not be compatible with the chosen encoding. In such cases, the encoding method will replace the incompatible characters with substitution characters (usually ‘?’) in the resulting byte array.
**Q: How can I convert a byte array back to a string in C#?**
A: To convert a byte array back to a string, you can use the appropriate decoding method provided by the Encoding class. For example, if you have a byte array and want to convert it to a string using UTF-8 encoding, you can use the following code:
“`csharp
byte[] byteArray = GetBytesFromSomewhere();
string convertedString = Encoding.UTF8.GetString(byteArray);
“`
**Q: Is there any performance difference between ASCII and UTF-8 encoding?**
A: Yes, ASCII encoding is generally faster than UTF-8 encoding because it operates on a smaller character set and requires less computation. However, the performance impact may vary depending on the size and complexity of the input string.
**Q: Can I convert a byte array to a string without specifying the encoding?**
A: No, when converting a byte array to a string, you need to specify the appropriate encoding. The encoding ensures that the byte array is correctly interpreted as a string, as different encoding schemes can represent the same byte sequences differently.
### Summary
Converting a string to a byte array in C# is a fundamental operation frequently used in many programming scenarios. By understanding the available encoding options and selecting the appropriate one based on the requirements of your project, you can ensure accurate and compatible conversion. Remember to consider factors such as the character set, encoding efficiency, and potential loss of information due to incompatible characters.
String To Byte C
Before we dive into the conversion process, let’s first understand the concepts of strings and bytes. In programming, a string is a sequence of characters, such as “Hello, World!”. On the other hand, a byte is the smallest addressable unit of memory, capable of storing a single character or numeric value within a range of 0 to 255. Bytes are essential when working with binary data or performing low-level operations on a computer.
Now, let’s discuss the process of converting a string to bytes in C. The C programming language does not have a built-in data type specifically for strings. Instead, it represents strings as arrays of characters. To convert a string to bytes in C, you need to convert each character of the string to its corresponding byte value.
One common method of converting a string to bytes in C is to use a loop to iterate through each character of the string, accessing its ASCII value. The ASCII (American Standard Code for Information Interchange) table assigns a unique numeric value to each character. By using this table, you can convert characters to their respective byte values.
Here’s an example of how you can implement the conversion using a loop:
“`c
#include
#include
void stringToByteArray(const char* str, unsigned char* bytes) {
for(int i = 0; i < strlen(str); i++) {
bytes[i] = (unsigned char)str[i];
}
}
```
In the above example, we define a function called `stringToByteArray` that takes two parameters: `str` (the string to be converted) and `bytes` (the array to store the resulting bytes). The loop iterates through each character of the string, casting it to an `unsigned char` before storing it in the array.
It's important to note that in C, strings are null-terminated, meaning they end with a null character '\0'. When converting a string to bytes, you may want to consider excluding the null character from the conversion process, as it is not considered a printable character and is often unnecessary for further processing.
Now, let's address some potential challenges you may encounter when converting a string to bytes in C. One important consideration is the character encoding of the string. C defaults to using ASCII encoding, but if your string contains characters from other character sets like Unicode, you need to handle the conversion accordingly.
To handle non-ASCII characters, you can make use of libraries like iconv or ICU, which provide functions for converting between different character encodings. These libraries ensure proper handling of multibyte characters, allowing you to accurately convert strings to bytes regardless of their character set.
Another aspect to consider is the memory allocation for the byte array. In the previous example, we assumed that the byte array is already allocated with sufficient memory. If the size of the byte array is not known in advance, you may need to dynamically allocate memory using functions like `malloc` or `realloc`. Be cautious about the memory management in such cases to avoid memory leaks or buffer overflows.
In terms of best practices, it's recommended to encapsulate the string-to-byte conversion logic within a function, as shown in the example above. This promotes code reusability, reduces redundancy, and improves code readability. Additionally, consider adding error handling mechanisms to handle cases where the string is empty or null, preventing potential crashes or unpredictable behavior.
Now, let's address some frequently asked questions (FAQs) related to string-to-byte conversion in C:
## FAQs
1. **Q: Can I use libraries, such as `
A: No, there are no built-in functions in C standard libraries specifically for string-to-byte conversion. You need to implement your own logic or use external libraries for handling character encodings.
2. **Q: What is the difference between converting a string to bytes and converting a string to a byte array?**
A: Converting a string to bytes means obtaining the byte representation of each character in the string without any specific container. Converting a string to a byte array implies storing the byte representation of each character in a dedicated array data structure.
3. **Q: How can I determine the size of the resulting byte array in advance?**
A: The size of the resulting byte array will be equal to the size of the string in bytes, excluding the null character at the end. You can use the `strlen` function from `
4. **Q: Can I convert a string to bytes in C++ using the same logic?**
A: Yes, you can apply similar logic to convert a string to bytes in C++ as both languages share many similarities. However, in C++, you have additional options, such as using the `std::string` class and its member functions for more convenient conversions.
In conclusion, converting a string to bytes in C involves iterating through each character of the string, accessing its ASCII value, and storing it in an array. By understanding the basics of character encodings, memory handling, and error prevention, you can effectively convert strings to bytes in C. Remember to consider the specific requirements of your project and leverage external libraries when necessary for handling non-ASCII characters or complex character encodings.
Images related to the topic string to byte array c#
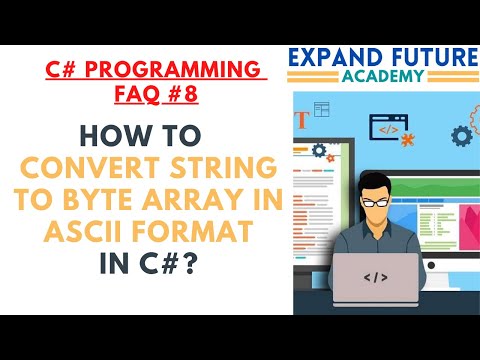
Article link: string to byte array c#.
Learn more about the topic string to byte array c#.
- Convert ASCII string (char[]) to BYTE array in C
- Hexadecimal string to byte array in C – Stack Overflow
- How to Convert String To Byte Array in C# – C# Corner
- How to convert a string to a byte array in C# – Dofactory
- HELP PLEASE – How to convert string to byte array – C / C++
- Convert string to byte array in C++ – Techie Delight
- String to byte array, byte array to String in Java | DigitalOcean
- How to convert a string to a byte array in C# – Dofactory
- How to convert a string to hexadecimal in C – TAE
- How to convert a string into bytes in C – Quora
- Convert String to Byte Array and Reverse in Java | Baeldung
- Convert string to byte array in C++ – thisPointer
- Convert string to byte array in C++ – Techie Delight
- Converting strings to bytes and vice versa – CryptoSys
See more: nhanvietluanvan.com/luat-hoc