Str Object Doesn’T Support Item Assignment
When working with strings in Python, you may come across the error message “str object doesn’t support item assignment”. This can be confusing for beginners and even for experienced programmers who are new to Python. In this article, we will delve into the nature of the str object, explore the concept of item assignment, understand why str objects are immutable, and discuss alternative ways to modify str objects. We will also address common scenarios where this error message may be encountered, provide troubleshooting tips, and offer insight into how to avoid this error. Let’s explore this error in detail.
Exploring the nature of the str object in Python
In Python, a string is represented as a sequence of unicode characters enclosed within single or double quotes. The str object is immutable, meaning that once a string is assigned to a variable, it cannot be changed. This immutability ensures the integrity of strings and avoids unexpected modifications.
The concept of item assignment in Python
Item assignment in Python allows you to modify specific elements within an object. For example, in a list, you can assign a new value to a specific index by using the syntax `list_name[index] = new_value`. This enables developers to update or replace elements in a mutable object.
Why str objects are immutable and do not support item assignment
Str objects are designed to be immutable for various reasons. It ensures the stability and predictability of strings, avoiding unintended changes that could impact the correctness of the program. This immutability allows strings to be used as keys in dictionaries, guarantees thread-safety, and optimizes memory usage. Therefore, attempting to modify a specific index or character in a str object results in the error message “str object doesn’t support item assignment”.
Alternative ways to modify str objects in Python
Although str objects do not support item assignment, there are alternative ways to modify or manipulate strings in Python:
1. Concatenation: Using the `+` operator, you can concatenate multiple strings together.
Example:
“`python
string1 = “Hello”
string2 = “World”
new_string = string1 + ” ” + string2 # Output: “Hello World”
“`
2. String methods: Python provides various built-in string methods that can be used to manipulate strings.
Example:
“`python
string = “Hello World”
new_string = string.replace(“Hello”, “Hi”) # Output: “Hi World”
“`
3. String slicing: Slicing allows you to obtain specific portions of a string, and you can further modify the sliced portions if needed.
Example:
“`python
string = “Hello World”
new_string = string[:5] + “Python” + string[5:] # Output: “Hello Python World”
“`
Consequences of attempting item assignment on a str object
If you attempt to assign a new value to a specific index or character in a str object, you will encounter the “str object doesn’t support item assignment” error message. This error acts as a guardrail, preventing unintended modifications to strings and maintaining the integrity of the data.
Common scenarios where the error message may be encountered
1. Trying to change a specific character in a string:
“`python
string = “Hello”
string[0] = “J” # Results in “str object doesn’t support item assignment” error
“`
2. Attempting to modify a substring within a string:
“`python
string = “Hello World”
string[6:11] = “Python” # Results in “str object doesn’t support item assignment” error
“`
3. Mistakenly assuming that Python’s string behaves like arrays in other programming languages:
“`python
string = “Hello”
string[1] = “a” # Results in “str object doesn’t support item assignment” error
“`
Tips for troubleshooting and avoiding the “str object doesn’t support item assignment” error
To troubleshoot and avoid encountering the “str object doesn’t support item assignment” error, consider the following tips:
1. Understand the difference between mutable objects (such as lists) and immutable objects (such as strings).
2. Double-check your code to ensure that you are not attempting item assignment on a str object.
3. Utilize string manipulation techniques like concatenation, string methods, and slicing to achieve the desired modifications.
4. If you need mutable objects, consider using lists or other mutable data types instead of str objects.
5. Make use of the error message “str object doesn’t support item assignment” as a learning opportunity to reinforce your understanding of immutability and its benefits.
FAQs
Q: What does the error message “str object doesn’t support item assignment” mean?
A: The error message indicates that you are trying to modify a specific index or character in a str object, which is not allowed because str objects are immutable.
Q: Are there any methods that allow modifying str objects directly?
A: No, str objects cannot be modified directly using item assignment. However, you can use string manipulation techniques like concatenation, string methods, and slicing to achieve the desired modifications.
Q: Why are str objects immutable in Python?
A: Str objects are immutable to ensure the stability and predictability of strings, prevent unintended changes that could impact the correctness of the program, enable the usage of strings as keys in dictionaries, ensure thread-safety, and optimize memory usage.
Q: Can I change a specific character or substring within a string?
A: No, you cannot directly change a specific character or substring within a str object using item assignment. Instead, you can utilize string manipulation techniques like concatenation, string methods, and slicing to achieve the desired modifications.
Q: Can I use the “str object doesn’t support item assignment” error message to detect other types of objects that are immutable?
A: Yes, the “str object doesn’t support item assignment” error message highlights the immutability of str objects. However, it does not necessarily indicate immutability for other types of objects.
In conclusion, the error message “str object doesn’t support item assignment” occurs when attempting to modify a specific index or character within a str object. Str objects in Python are immutable, meaning they cannot be changed once assigned to a variable. However, alternative methods such as concatenation, string methods, and slicing can be used to modify strings. Understanding the nature of immutability and applying appropriate string manipulation techniques will help you avoid this error and efficiently work with str objects in Python.
Python Typeerror: ‘Str’ Object Does Not Support Item Assignment
What Does Str Object Does Not Support Item Assignment Mean?
Python is a popular programming language known for its simplicity and versatility. However, like any programming language, it has its own set of rules and limitations. One common error that Python programmers encounter is the “str object does not support item assignment” message. This article aims to explain what this error means, its causes, and some possible solutions.
Understanding the Error Message
When you see the error message “str object does not support item assignment,” it means that you are trying to change or modify the value of an individual character in a string using the assignment operator (=). The error typically occurs when you try to assign a new value to a specific index of a string, like this:
“`python
message = “Hello, World!”
message[0] = “h”
“`
In the above example, the code tries to change the first character of the string message from “H” to “h.” However, this operation is not allowed for string objects in Python, resulting in the aforementioned error message.
Possible Causes
There are a few common scenarios that can lead to this error:
1. Attempting to modify a string directly: Python strings are immutable, meaning their values cannot be changed after they are created. This limitation is in place for efficiency reasons, as immutable objects are easier to work with, especially in multi-threaded environments.
2. Using an incorrect data type: Another possible cause is trying to assign values to individual characters of a variable that is not a string. For example, if you mistakenly assigned an integer or a list to a variable initially declared as a string, you may encounter this error when you attempt to modify it.
Solutions
While you cannot directly modify individual characters in a string, there are alternative approaches to achieve the desired results. Here are some possible solutions to resolve the “str object does not support item assignment” error:
1. Use concatenation: Instead of modifying a single character, you can concatenate the desired string with the remaining portion of the original string. For example:
“`python
message = “Hello, World!”
message = “h” + message[1:]
“`
In this case, we create a new string by concatenating the desired character “h” with the subset of the original string starting from index 1.
2. String transformation: If you need to change multiple characters within a string, you can use string transformation methods such as `replace`. For instance:
“`python
message = “Hello, World!”
message = message.replace(“H”, “h”)
“`
By utilizing the `replace` method, we replace all occurrences of the character “H” with “h” in the string.
3. Convert the string to a mutable object: If you truly need to modify individual characters of a string, you can convert it into a mutable object such as a list. After making the necessary modifications, you can convert it back to a string, like this:
“`python
message = “Hello, World!”
message_list = list(message)
message_list[0] = “h”
message = “”.join(message_list)
“`
In this example, we convert the string to a list using the `list()` function. After making the desired modifications, we convert the list back to a string using the `””.join()` method.
FAQs
Q: Can we modify a string in Python?
A: No, Python strings are immutable, meaning they cannot be changed after they are created. However, there are alternative approaches to achieve similar results.
Q: Why are strings immutable in Python?
A: Strings are immutable in Python to ensure efficiency, as immutable objects are easier to work with, especially in situations where multiple threads are involved.
Q: Can I change individual characters in a string using a loop?
A: Since strings are immutable, you cannot change individual characters in a string using a loop. You would need to follow one of the alternative approaches mentioned earlier.
Q: Are all data types immutable in Python?
A: No, not all data types in Python are immutable. Immutable types include strings, tuples, and numbers, whereas lists and dictionaries are mutable.
Q: Are there any performance implications when using alternative approaches to modifying strings?
A: It’s important to note that alternative approaches like concatenation and string transformation may have performance implications, especially when dealing with large strings. Therefore, it is recommended to assess the specific requirements of your program and choose the approach that best suits your needs.
In conclusion, encountering the “str object does not support item assignment” error message while trying to modify individual characters in a Python string is a common issue. However, understanding the error, its causes, and the available solutions can help programmers overcome this limitation and achieve the desired results in their Python programs.
Why Is My Str Object Not Callable?
If you have ever encountered the error message “type object ‘str’ is not callable” while working with Python, you are not alone. This error can be frustrating and might seem cryptic at first. In this article, we will delve into the reasons behind this error and explore potential solutions. By the end, you will have a clear understanding of why this error occurs and how to troubleshoot it effectively.
Understanding the Basics
Before we delve into the specific causes of the “str object not callable” error, it’s essential to have a solid grasp of the basics. In Python, `str` is a built-in class representing strings. It provides various methods and attributes that allow you to manipulate and work with string objects.
When you call a method on a string object, such as `str.upper()`, you are essentially invoking that method and executing its functionality. At times, however, you might mistakenly treat the string object itself as a function and attempt to call it, which results in the ‘str object not callable’ error.
Possible Causes
1. Attempting to call a string object
The most common cause of this error is treating a string object as a function and attempting to invoke it. For example, if you have a variable `my_string` that contains a string, calling it like a function such as `my_string()` will result in the ‘str object not callable’ error.
2. Overwriting the string object
If you inadvertently overwrite the `str` class or any of its methods, you can encounter the ‘str object not callable’ error. This can happen if, for instance, you assign a different value to the `str` variable within your code.
3. Mismatched parentheses or brackets
Another possible cause of this error is a mismatch in the use of parentheses or brackets. If you mistakenly add parentheses or brackets after a string object, Python interprets it as a function call rather than accessing the string’s attributes and methods.
Troubleshooting the Error
Now that we have covered the potential causes of the ‘str object not callable’ error, let’s explore some troubleshooting steps to resolve it.
1. Verify your code for calling string objects
Carefully review your code and ensure that you are not trying to call your string objects as functions. Remember, string objects are not callable, and attempting to do so will raise this error. If you intended to manipulate the string itself, use the appropriate methods and attributes without parentheses or brackets.
2. Check for variable overwrites
Inspect your code to see if you have mistakenly overwritten the `str` class or any of its built-in methods. If you inadvertently reassigned a different value (e.g., `str = 42`) to the `str` variable, it will mask the original string class, causing the ‘str object not callable’ error. To fix this, change the variable name to something different.
3. Verify your parentheses and brackets
Make sure that the use of parentheses and brackets in your code is appropriate. Incorrectly placed or mismatched parentheses or brackets can lead to this error. Double-check the syntax to ensure that you are not accidentally treating string objects as functions.
FAQs
Q: Why do I receive the ‘str object not callable’ error even when I am not trying to call the string?
A: Even if you are not explicitly calling the string object in your code, ensure that you have not accidentally overwritten the `str` class or any of its methods. An erroneous reassignment of the `str` variable can lead to this perplexing error.
Q: How can I fix the ‘str object not callable’ error caused by mismatched parentheses or brackets?
A: Double-check your code and ensure that you are using parentheses and brackets correctly. Verify that they are appropriately placed and closed. Correcting any mismatch should resolve the error.
Q: Can I use parentheses or brackets when calling string methods?
A: No. String methods require only the dot notation to access and execute them. Parentheses or brackets should not be used when calling these methods. Treating string methods as functions can raise the ‘str object not callable’ error.
In conclusion, the ‘str object not callable’ error occurs when attempting to call a string object directly as a function or due to other related issues. By following the troubleshooting steps provided and keeping in mind the distinction between string objects and functions, you can effectively resolve this error and ensure smooth execution of your Python code.
Keywords searched by users: str object doesn’t support item assignment Str object does not support item assignment dictionary, Object does not support item assignment, TypeError: ‘int’ object does not support item assignment, Change item in List Python, Item assignment in string python, How to change a character in string python, Left Python, Python Get substring from index to end
Categories: Top 20 Str Object Doesn’T Support Item Assignment
See more here: nhanvietluanvan.com
Str Object Does Not Support Item Assignment Dictionary
Python’s str object is known for its versatility and functionality. However, there are certain limitations to its usage, one of which is that it does not support item assignment using a dictionary. In this article, we will explore the reasons behind this limitation and discuss possible workarounds.
The Reasoning Behind the Limitation
To understand why the str object does not support item assignment with a dictionary, we need to delve into its internal implementation. The str object in Python is an immutable sequence type, which means its contents cannot be changed once it is created. This immutability allows for various optimizations, such as string interning, which significantly improves performance.
When we try to assign values to specific positions using a dictionary, we essentially want to modify the existing string object. However, since str objects are immutable, any assignment operation would require creating a new string object with the desired changes. This contradicts the fundamental property of immutability, leading to the limitation.
Workaround: String Concatenation
While we cannot directly modify a string object using a dictionary, we can use string concatenation to achieve a similar effect. By breaking down the original string and inserting the desired values at the desired positions, we can create a new string object with the desired changes.
Consider the following example:
“`
my_str = “Hello, {name}! Today is {day}”
name = “John”
day = “Monday”
result = my_str.format(name=name, day=day)
print(result)
“`
In this example, we are using the `format()` method, which allows us to insert values at the desired positions using named placeholders. By passing a dictionary with the desired key-value pairs to the `format()` method, we effectively achieve item assignment without directly modifying the original string object.
FAQs
Q1: Can I modify a string in-place without creating a new object?
No, you cannot modify a string object in-place due to its immutability. Any operation that seems to modify the string actually creates a new string object with the desired changes.
Q2: Are there any performance implications to this limitation?
The limitation itself does not impact performance significantly. In fact, the immutability of strings allows for various optimizations. However, when working with large strings and frequent modifications, the overhead of constantly creating new string objects through concatenation can have performance implications. In such cases, using mutable data structures, such as lists, may be more efficient.
Q3: Are there any alternative solutions to overcome this limitation?
Yes, there are alternative approaches to achieve similar results. One such approach is to use string interpolation, which is available in Python 3.6 and later versions. Instead of using the `format()` method, you can directly embed expressions within f-strings, allowing you to dynamically insert values into the string. Another option is to use a mutable container, such as a list, instead of a string, and then join the list elements to form a final string.
Q4: How can I check if a string contains a particular substring?
To check if a string contains a specific substring, you can use the `in` keyword. For example:
“`
my_str = “Hello, world!”
if “world” in my_str:
print(“Substring found!”)
“`
Q5: Can I convert a string to a dictionary?
Yes, you can convert a string to a dictionary using various methods such as splitting the string and mapping the resulting list elements to keys and values. Here’s an example:
“`
my_str = “name=John, age=25, city=New York”
my_dict = dict(item.split(“=”) for item in my_str.split(“, “))
print(my_dict)
“`
In this example, we first split the string on commas to obtain a list of key-value pairs. Then, we split each pair on the equals sign (=) to obtain separate keys and values. Finally, we use a dictionary comprehension to create a dictionary from these key-value pairs.
Conclusion
The limitation of the str object not supporting item assignment with a dictionary is rooted in its immutability. While it may seem restrictive at first, Python offers alternate techniques such as string concatenation and interpolation to achieve similar results. Understanding these limitations and exploring alternative solutions empowers developers to efficiently work with strings in Python.
Object Does Not Support Item Assignment
Introduction:
Python is a versatile programming language known for its simplicity and readability. However, developers often encounter various challenges, such as the infamous error message “Object does not support item assignment.” In this article, we will explore the reasons behind this error, the scenarios where it commonly occurs, and practical strategies to resolve it. So, let’s dive in and demystify this common issue!
Understanding the “Object does not support item assignment” Error:
The error message “Object does not support item assignment” typically appears when attempting to modify a non-mutable object, such as strings or tuples, using item assignment syntax in Python. This means that you cannot change individual elements or characters within these objects directly.
Python provides mutable and non-mutable data types. Non-mutable objects, also called immutable objects, cannot be altered once created, while mutable objects can be modified. The error occurs when attempting to assign a new value to an element of such a non-mutable object.
Common Scenarios Among Non-Mutable Objects:
1. Strings:
Strings are a sequence of characters enclosed within quotation marks. For example:
name = “John”
Attempting to modify a single character within a string using item assignment will raise the “Object does not support item assignment” error.
2. Tuples:
Tuples are an immutable collection of objects separated by commas and enclosed within parentheses. For example:
numbers = (1, 2, 3, 4)
Trying to modify or replace a specific element within a tuple will also trigger this error.
Reasons Behind the Error:
The error message “Object does not support item assignment” is raised due to the nature of non-mutable objects. They are designed to be unchangeable and immutable. Python enforces this immutability to ensure predictable and efficient code execution.
Non-Mutable Object Alternatives:
To overcome the “Object does not support item assignment” error, you can use mutable objects like lists or dictionaries instead of strings or tuples.
1. Lists:
Lists are mutable objects that can be modified by adding, removing, or changing elements. For instance:
numbers = [1, 2, 3, 4]
To update a specific element within a list, you can use item assignment syntax like: numbers[0] = 5
2. Dictionaries:
Dictionaries are another mutable data type, storing key-value pairs. They allow you to modify or update specific values associated with keys. For example:
person = {“name”: “John”, “age”: 25}
To update the value corresponding to the “age” key, you can use item assignment syntax: person[“age”] = 26
Strategies to Resolve the Error:
1. Convert Non-Mutable Objects:
One way to tackle the error is by converting non-mutable objects into mutable representations. For instance, you can convert a string into a list and then modify it accordingly. After completing the necessary changes, convert it back into a string if required.
2. Reconstruct New Objects:
Instead of modifying non-mutable objects directly, create new objects using concatenation, slicing, or other suitable methods. This approach doesn’t modify the original object but generates a new one according to your requirements.
Frequently Asked Questions (FAQs):
Q1. Why does Python have non-mutable objects like strings and tuples?
A1. Non-mutable objects like strings and tuples are designed for specific use cases where the immutability guarantees data integrity and increased performance.
Q2. Can I modify the contents of a non-mutable object indirectly?
A2. Yes, you can achieve a modified version of a non-mutable object by using string manipulation functions, list comprehension, tuple packing/unpacking, or other relevant techniques.
Q3. Are there other errors similar to “Object does not support item assignment”?
A3. Yes, in Python, you may encounter similar errors like “TypeError: ‘str’ object does not support item deletion” or “TypeError: ‘tuple’ object does not support item deletion” when attempting to delete elements.
Q4. Is it possible to modify immutable objects in other programming languages?
A4. It depends on the programming language. Some languages offer similar data types as Python, where objects are immutable, while others may allow modifications to immutable objects.
Conclusion:
The error message “Object does not support item assignment” arises when attempting to modify non-mutable objects directly. Understanding the concept of mutable and non-mutable objects, along with the alternative solutions, can help the Python developers to overcome this error effectively. By utilizing the strategies mentioned in this article, you will be able to work around this limitation and write more efficient code in Python.
Images related to the topic str object doesn’t support item assignment
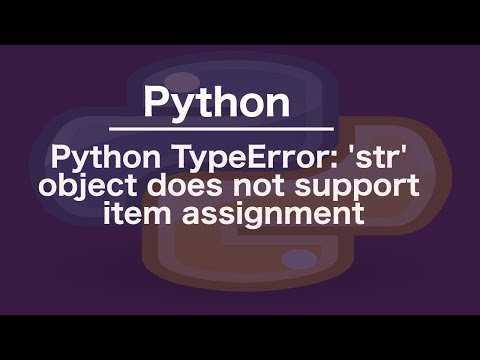
Found 10 images related to str object doesn’t support item assignment theme
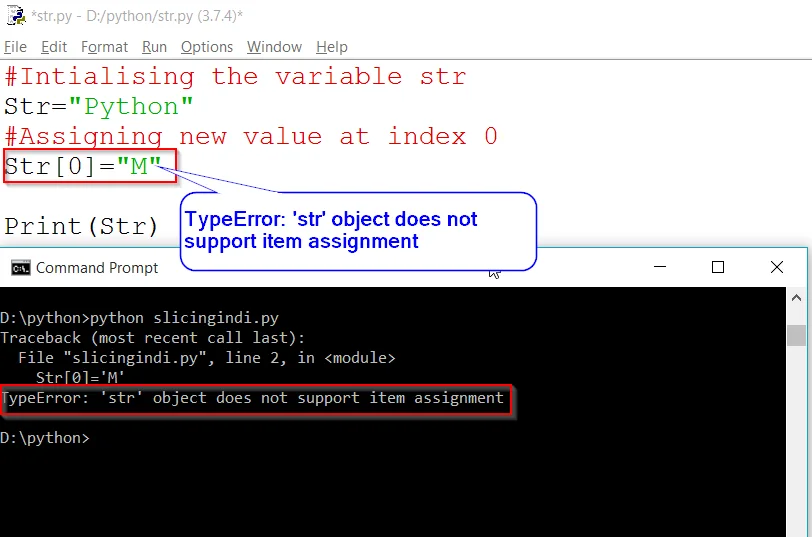
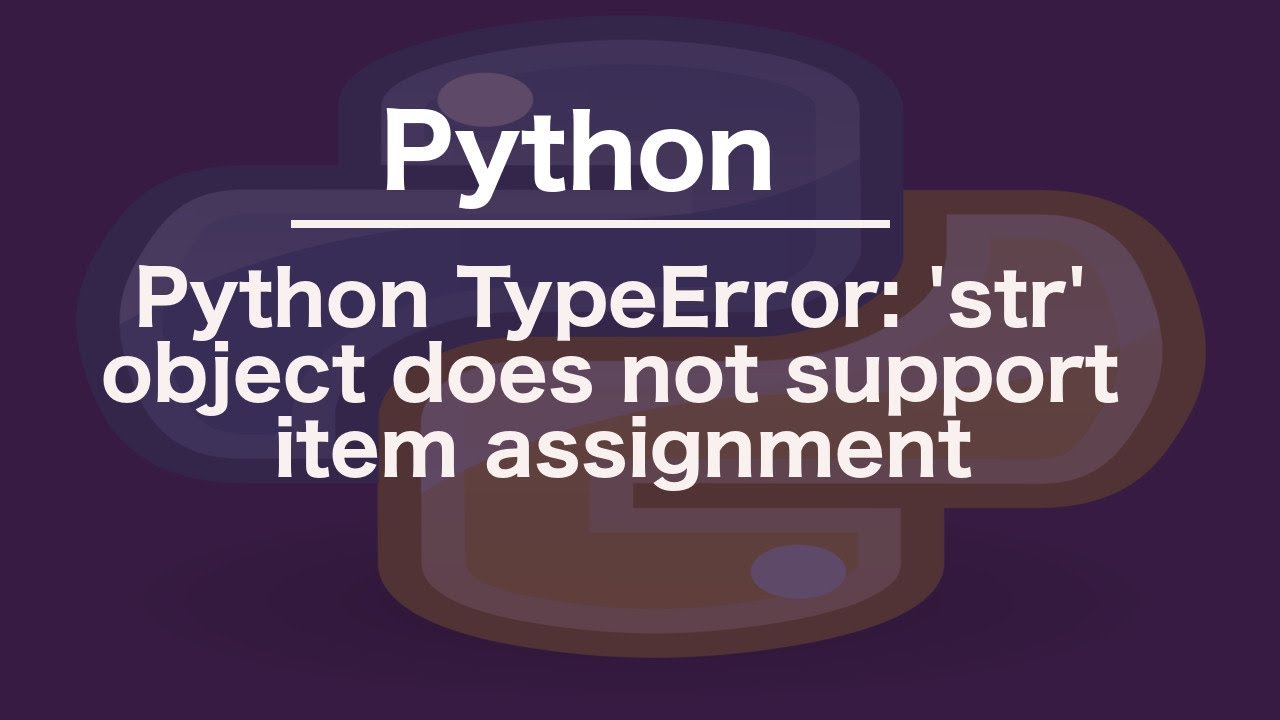
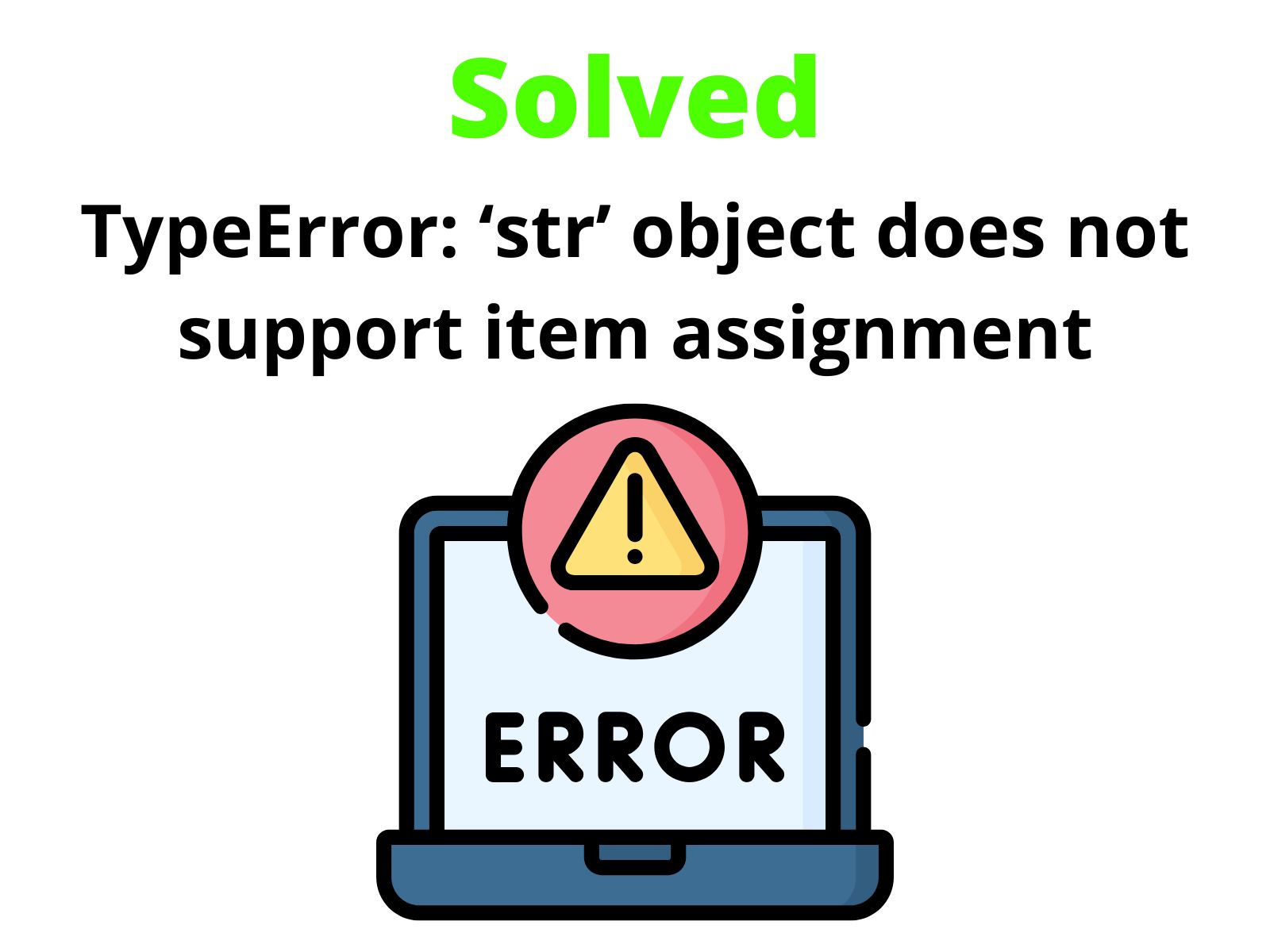
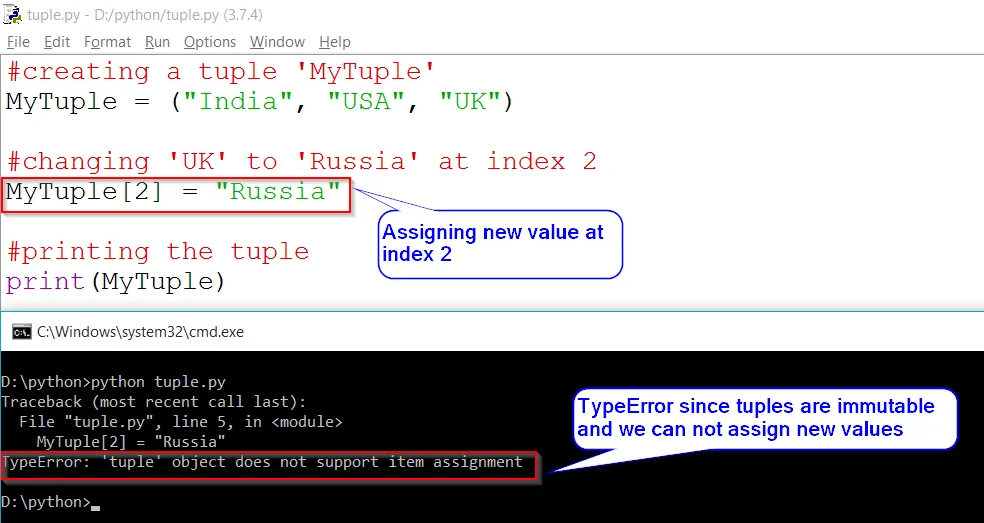
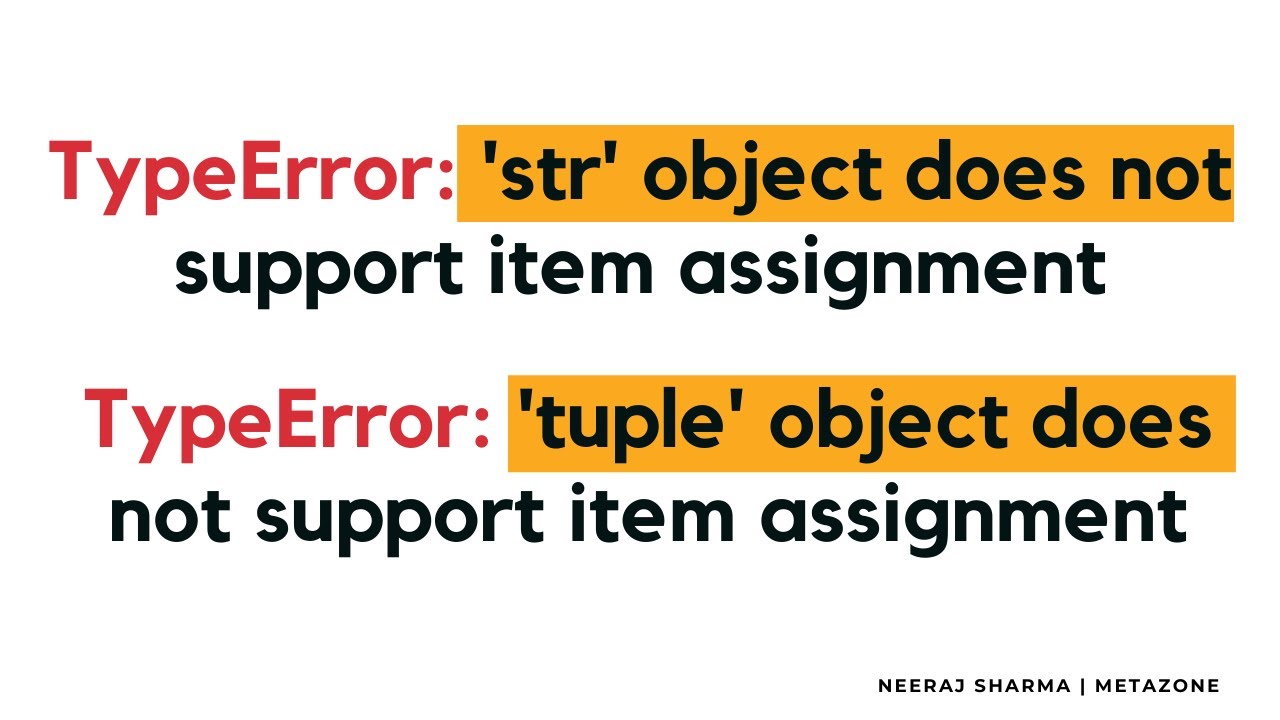
![Solved] TypeError: 'str' Object Does Not Support Item Assignment Solved] Typeerror: 'Str' Object Does Not Support Item Assignment](https://www.pythonpool.com/wp-content/uploads/2022/04/TypeErrorstr-Object-Does-Not-Support-Item-Assignment.webp)


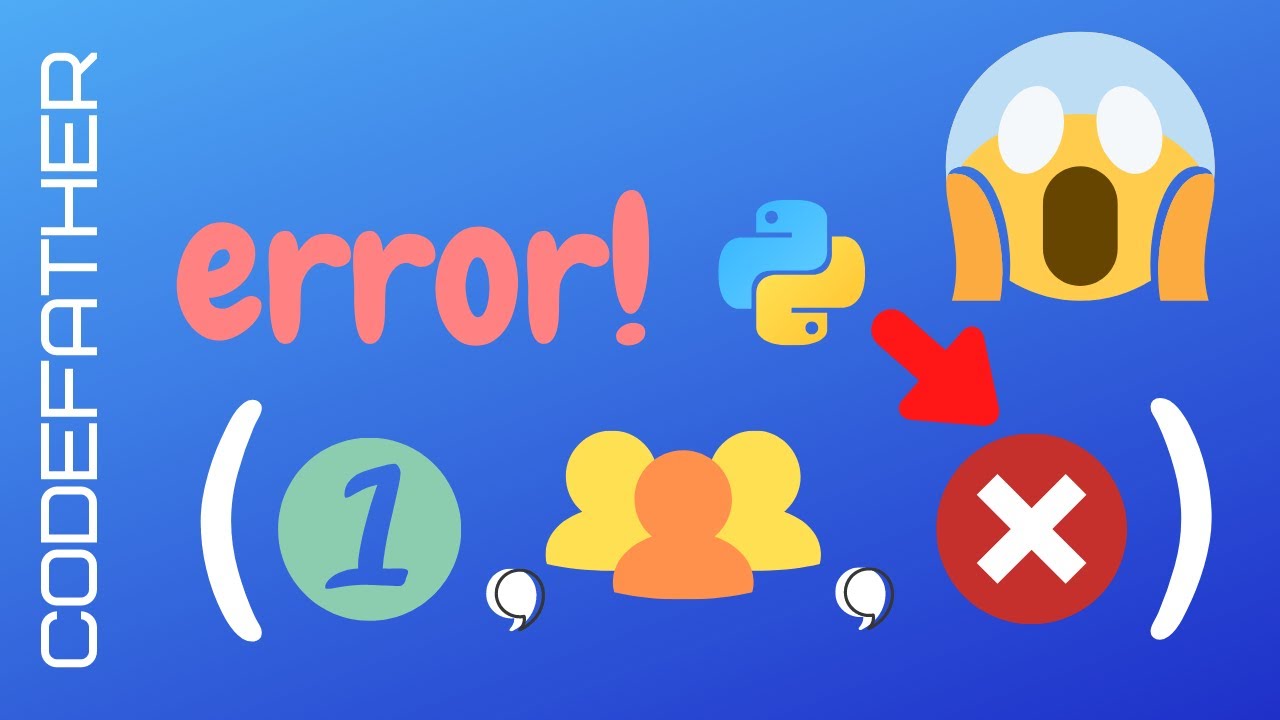
![Typeerror: int object does not support item assignment [SOLVED] Typeerror: Int Object Does Not Support Item Assignment [Solved]](https://itsourcecode.com/wp-content/uploads/2023/04/typeerror-int-object-does-not-support-item-assignment.png)

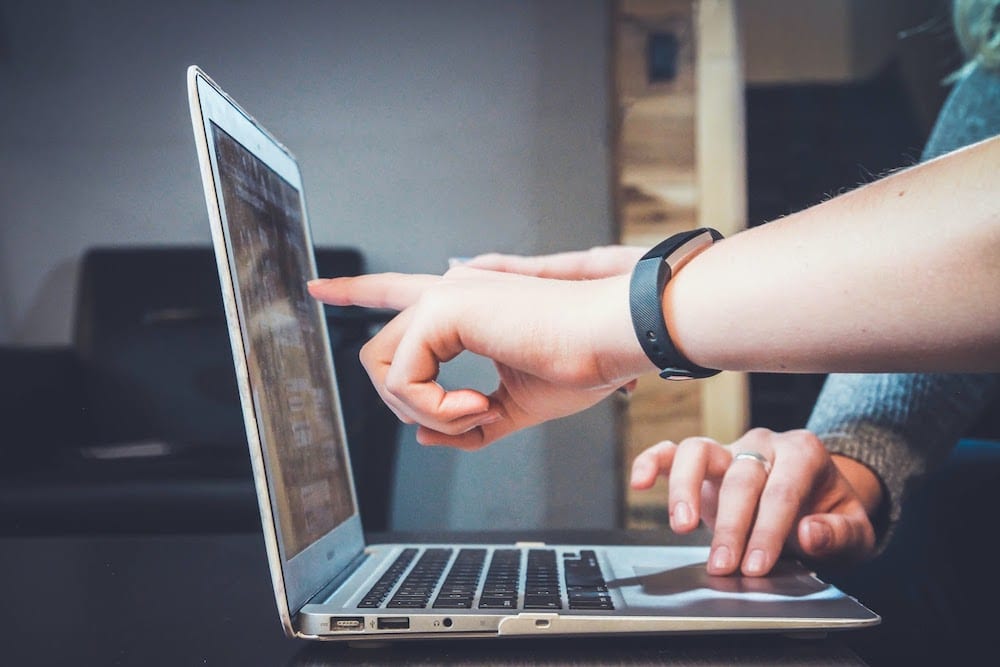
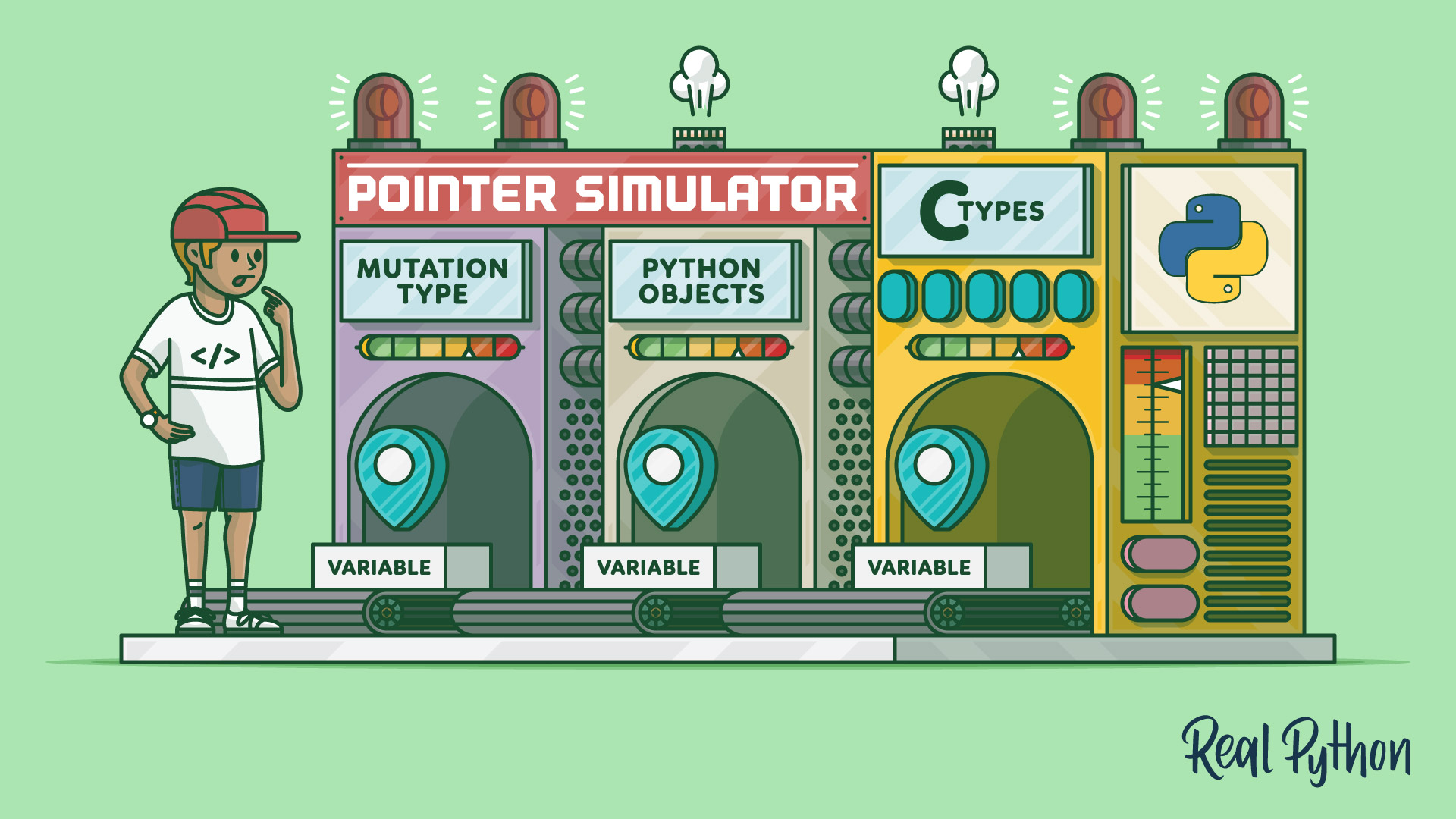


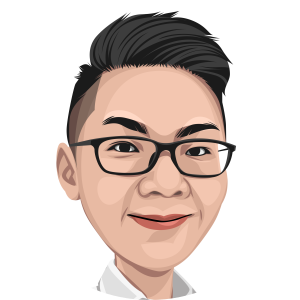
Article link: str object doesn’t support item assignment.
Learn more about the topic str object doesn’t support item assignment.
- ‘str’ object does not support item assignment – Stack Overflow
- TypeError: ‘str’ object does not support item assignment
- Python ‘str’ object does not support item assignment solution
- TypeError ‘str’ object does not support item assignment
- TypeError: ‘str’ object does not support item assignment
- TypeError ‘str’ object does not support item assignment
- Typeerror: str object is not callable – How to Fix in Python – freeCodeCamp
- TypeError: ‘str’ object cannot be interpreted as an integer [duplicate]
- TypeError ‘str’ Object Does Not Support Item Assignment – Sentry
- Fix STR Object Does Not Support Item Assignment Error in …
- Fix Python TypeError: ‘str’ object does not support item …
- getting TypeError: ‘str’ object does not support item assignment
See more: https://nhanvietluanvan.com/luat-hoc/