Spread Types May Only Be Created From Object Types
In the world of programming, understanding different types is crucial for writing clean and efficient code. One important concept that developers come across frequently is spread types, which are closely linked to object types. Spread types provide a convenient way to create new objects by spreading or merging the properties of existing objects. However, it’s important to note that spread types may only be created from object types, and not from primitive types. In this article, we will explore the concept of spread types, their relation to object types, and the benefits they offer in programming.
Types in Programming:
Before diving into spread types and their relationship to object types, let’s first understand the concept of types in programming. In many programming languages, including JavaScript and TypeScript, variables can have different types that determine the kind of data they can hold or the operations that can be performed on them.
In statically typed languages like TypeScript, types are checked at compile-time, reducing the likelihood of runtime errors. On the other hand, dynamically typed languages like JavaScript perform type-checking at runtime, which allows for more flexibility but also increases the chances of unexpected behavior.
Static Typing:
Static typing refers to the practice of declaring types explicitly for variables and function parameters before compiling or executing the code. This helps catch potential errors at compile-time and enables tooling to provide better code suggestions and documentation. TypeScript is a popular statically typed superset of JavaScript that adds type annotations, interfaces, and other features to the language.
Dynamic Typing:
Dynamic typing, on the other hand, allows variables to hold different types of values at different times during program execution. In dynamically typed languages like JavaScript, the type of a variable is inferred based on the value it holds. This flexibility can make the code easier to write and read, but it also introduces the possibility of bugs caused by unexpected type conversions.
Object Types:
In programming, an object type is a composite data type that represents a collection of properties, each with its own name and value. Object types are commonly used to define complex data structures and encapsulate related data. In JavaScript, object types can be created using object literals, constructors, or classes.
Primitive Types:
While object types can be used to represent complex data structures, primitive types represent simple, single values. JavaScript has six primitive types: string, number, boolean, null, undefined, and symbol. These types are immutable and don’t have any properties or methods associated with them.
Spread Types:
Spread types allow for the creation of new objects by spreading or merging the properties of existing objects. In JavaScript, spread syntax (denoted by the three dots “…”) can be used to create a shallow copy of an object and add or override properties. This syntax simplifies object manipulation and reduces the need for repetitive code.
Spread Types and Object Types:
It’s important to note that spread types can only be created from object types, not from primitive types. Attempting to spread a primitive value will result in a runtime error. For example, spreading a string or a number will throw a TypeError. The reason for this restriction is that primitive types do not have properties that can be merged or spread.
Benefits of Using Spread Types:
Using spread types in programming offers several benefits:
1. Code Reusability: Spread types enable developers to create new objects by merging properties from existing objects. This promotes code reuse and reduces the need to define similar objects multiple times.
2. Simplicity and Expressiveness: Spread syntax provides a concise and intuitive way to manipulate objects. It allows developers to add, remove, or modify properties without modifying the original objects.
3. Avoidance of Repetitive Code: By leveraging spread types, developers can avoid writing repetitive code to manually copy properties from one object to another. This reduces the chances of introducing bugs and makes the code more maintainable.
4. Immutability: Spread types create shallow copies of objects, ensuring that the original objects remain unchanged. This helps prevent unintended modifications and facilitates the use of immutable data structures and functional programming practices.
FAQs:
Q: Can spread types be used with React Hook Form?
A: Yes, spread types can be used with React Hook Form to facilitate form handling and validation. The spread syntax can be employed to spread form values into the input components, simplifying the code required for form manipulation.
Q: How are spread types related to TypeScript?
A: TypeScript, being a statically typed superset of JavaScript, provides support for spread types through its object spread feature. This feature allows developers to easily merge properties of objects, creating new objects with combined properties.
Q: What does Ts2698 error mean?
A: The Ts2698 error is a TypeScript error that occurs when an attempt is made to spread properties from a non-object type. This error highlights the requirement for spread types to be created from object types.
Q: How can I add a new object to another object in JavaScript?
A: You can add a new object to another object in JavaScript by using the spread syntax. For example, you can create a new object by spreading the properties of the original object and adding new properties as needed.
Q: What does “Is not assignable to type ‘IntrinsicAttributes'” mean in TypeScript?
A: This error message is commonly encountered in TypeScript when there is an issue with how an object is being assigned to a component. It often occurs due to mismatched property types or incorrect usage of spread types.
Q: How can I copy an object in JavaScript using spread types?
A: You can make a shallow copy of an object in JavaScript by using the spread syntax. Simply spread the properties of the original object into a new object, as shown in the following example:
“`
const originalObject = { foo: ‘bar’ };
const copiedObject = { …originalObject };
“`
In conclusion, spread types offer a powerful way to create new objects by merging properties from existing objects. However, it’s important to remember that spread types may only be created from object types and cannot be used with primitive types. Understanding the concepts of object types and spread syntax can greatly enhance code reusability, simplify object manipulation, and contribute to the overall maintainability of a project.
Object Spread Syntax – Javascript Tutorial
Can Spread Types Only Be Created From Object Types?
In the world of programming, understanding the difference between object types and spread types is essential. While object types are a widely used concept, spread types are a relatively new addition and can lead to confusion among developers. One common question that arises is whether spread types can only be created from object types. In this article, we will delve into this topic, explaining the nature of spread types and their relationship with object types.
Understanding Object Types:
Before we delve into spread types, let’s first clarify the concept of object types. In programming, an object type is a blueprint that defines the structure and behavior of an object. It enables developers to create instances of objects based on predefined characteristics. Object types typically consist of properties, which define the object’s attributes, and methods, which define its behavior.
Object types are integral to many programming languages, including JavaScript and TypeScript. They provide a way to organize and manipulate data, making code more modular and maintainable. Instances of object types can be created using constructors or classes, allowing developers to reuse code and create multiple objects with similar characteristics.
Introducing Spread Types:
Spread types, on the other hand, are a feature introduced in TypeScript to enable the spreading of properties of one type into another. The spread operator, denoted by three dots (e.g., …), is used to expand an object’s properties within another object. This can be done during object creation or through a process called object merging.
Contrary to popular belief, spread types are not limited to object types alone. While they can be used to spread the properties of one object into another, they can also be applied to other types, including arrays, tuples, and even primitive types like strings and numbers.
Creating Spread Types from Object Types:
One of the most common use cases for spread types is to create new object types by merging existing object types. This is particularly helpful when dealing with large projects where objects may have similar properties. Rather than duplicating code, spread types allow developers to merge object types, ensuring reusability and reducing redundancy.
Let’s consider an example to illustrate this concept. Assume we have two object types: “Person” and “Employee.” Both types have common properties, such as “name” and “age,” but the “Employee” type has an additional property called “role”. With spread types, we can easily merge the properties of “Person” and “Employee” into a new object type, “PersonEmployee”:
“`
type Person = {
name: string;
age: number;
};
type Employee = {
role: string;
};
type PersonEmployee = Person & Employee;
“`
In this example, the `&` operator is used to merge the properties of “Person” and “Employee” into “PersonEmployee”. Now, “PersonEmployee” will have all the properties of “Person” and “Employee”, making it a versatile type that can be used whenever both sets of properties are required.
Frequently Asked Questions:
Q: Can spread types only be created from object types?
A: No, spread types can be created from a wide range of types, including object types, arrays, tuples, and primitive types.
Q: What are the benefits of using spread types?
A: Spread types enable developers to merge properties from multiple types, reducing duplicated code and improving code reusability. They also make code more modular and maintainable.
Q: Can spread types be used in all programming languages?
A: Spread types are a feature introduced in TypeScript, a superset of JavaScript. While other programming languages may have similar concepts, the syntax and implementation may differ.
Q: Are there any limitations to using spread types?
A: Spread types are generally straightforward to use, but they can lead to potential conflicts when merging properties with the same name. Care should be taken to avoid naming conflicts and ensure the desired behavior of the merged type.
In conclusion, spread types offer a powerful way to merge properties from multiple types, including object types. While they are not limited only to object types, they can be applied to various types, making code more reusable and maintainable. Understanding the relationship between object types and spread types is crucial for developers in order to harness the full potential of this feature in their programming projects.
What Is Spread Operator In Typescript?
TypeScript is a statically-typed superset of JavaScript that adds optional types to the language. It offers more capabilities and features to JavaScript developers, making it easier to build large-scale applications. One of the features introduced in TypeScript is the spread operator, which provides a concise syntax for working with arrays and objects.
The spread operator is represented by three consecutive dots: ….
When used with arrays, it allows you to expand an array into individual elements. This can be particularly useful when you need to combine multiple arrays or pass an array as arguments to a function. Let’s take a look at some examples to better understand how the spread operator works with arrays:
“`typescript
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const combinedArray = […array1, …array2];
console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
“`
In this example, the spread operator is used to combine two arrays, array1 and array2, into a new array called combinedArray. The resulting array contains all the elements from both arrays.
“`typescript
const array = [1, 2, 3];
const clonedArray = […array];
console.log(clonedArray); // Output: [1, 2, 3]
“`
In this example, the spread operator is used to create a clonedArray, which is an exact copy of the original array.
The spread operator can also be used with objects. When used with objects, it allows you to create new objects by copying the properties from an existing object into a new one. Here’s an example:
“`typescript
const obj1 = { name: “John”, age: 30 };
const obj2 = { …obj1, city: “New York” };
console.log(obj2); // Output: { name: “John”, age: 30, city: “New York” }
“`
In this example, the spread operator copies the properties from obj1 into obj2 and adds an additional property, city. As a result, obj2 is a new object that contains all the properties from obj1 plus the new property.
The spread operator can also be used to clone objects:
“`typescript
const obj = { name: “John”, age: 30 };
const clonedObj = { …obj };
console.log(clonedObj); // Output: { name: “John”, age: 30 }
“`
In this example, the spread operator is used to create a clonedObj, which is a shallow copy of the original object.
FAQs:
Q: What is the difference between spreading an array and using the concat method?
A: The spread operator provides a more concise and readable syntax compared to the concat method. Additionally, spreading an array allows you to combine multiple arrays or clone an array, while the concat method only concatenates two arrays.
Q: Can the spread operator be used with TypeScript interfaces?
A: Yes, the spread operator can be used with TypeScript interfaces to create new objects or merge existing ones. However, it’s important to note that the spread operator only performs a shallow copy, meaning nested objects or arrays will still be referenced.
Q: Are there any limitations or performance concerns when using the spread operator?
A: While the spread operator is a powerful feature, it’s important to keep in mind that it creates new arrays or objects, which can impact performance if used excessively or with large data sets. Additionally, as mentioned earlier, the spread operator only performs a shallow copy, so it may not be suitable for deep cloning complex objects.
In conclusion, the spread operator in TypeScript provides a concise and powerful syntax for working with arrays and objects. It allows you to easily combine arrays, clone them, or create new objects by merging existing ones. Understanding and effectively using the spread operator can greatly enhance your TypeScript development experience.
Keywords searched by users: spread types may only be created from object types Spread types may only be created from object types react hook form, TypeScript spread types, Typescript object spread, Ts2698, Add new object to object javascript, Nối 2 object javascript, Is not assignable to type ‘IntrinsicAttributes, Copy object JavaScript
Categories: Top 82 Spread Types May Only Be Created From Object Types
See more here: nhanvietluanvan.com
Spread Types May Only Be Created From Object Types React Hook Form
Spread types allow us to create form inputs by spreading an object’s properties into separate fields. This is particularly useful when dealing with forms that have numerous inputs or when working with nested data structures. By utilizing spread types, we can avoid cumbersome and error-prone manual creation of inputs, and instead let React Hook Form handle the heavy lifting.
To create form inputs using spread types, we start by defining an object that represents our form data structure. Each property of the object corresponds to a form input, and its value can specify various properties such as the input type, validation rules, and default values. We then pass this object to the `useForm` hook provided by React Hook Form.
Here’s an example to better illustrate the concept:
“`jsx
import { useForm } from ‘react-hook-form’;
const MyForm = () => {
const { register, handleSubmit } = useForm();
const onSubmit = (data) => {
// Handle form submission
};
return (
);
};
“`
In the code snippet above, we define a simple form with three inputs: `firstName`, `lastName`, and `email`. By spreading the `register` function returned by the `useForm` hook into each input’s props, we create the necessary bindings between the inputs and React Hook Form.
Now, let’s explore some frequently asked questions about spread types in React Hook Form:
**Q: Can I nest objects using spread types?**
A: Absolutely! React Hook Form supports nested data structures, allowing you to easily create inputs for complex forms. To nest objects, simply define the nested properties within your form data object.
**Q: How can I set default values for my inputs?**
A: You can set default values by specifying them in the form data object. For example, `{ firstName: ‘John’, lastName: ‘Doe’ }`. React Hook Form will automatically populate the inputs with the provided default values.
**Q: Can I apply validation rules to my inputs?**
A: Yes, you can apply validation rules by passing an object as the second argument to the `register` function. The object can include various validation rules supported by React Hook Form, such as `required`, `minLength`, `pattern`, etc.
**Q: How do I access the form data after submission?**
A: When the form is submitted, the `onSubmit` function (defined in the `handleSubmit` prop) will be called with the form data as the argument. You can then handle the submission using the received data.
**Q: Are there any limitations to using spread types?**
A: Spread types offer great flexibility and convenience, but they may not be suitable for every use case. They are best suited for forms with a predefined structure. If your form has dynamically changing fields or requirements, you may need to use alternative approaches.
In conclusion, spread types are a fantastic feature in React Hook Form that simplify the creation of form inputs from object types. By spreading an object’s properties into separate fields, we can avoid repetitive and error-prone manual input creation. Spread types excel in handling complex forms with nested data structures, providing a convenient and efficient solution for form handling in React applications.
Typescript Spread Types
TypeScript, a superset of JavaScript, has gained significant popularity among developers due to its ability to provide static typing in JavaScript applications. One of TypeScript’s remarkable features is spread types, which allow developers to spread the properties of an object or an array into another object or array. This article aims to delve into TypeScript spread types, exploring their syntax, use cases, caveats, and answering frequently asked questions to provide a comprehensive understanding of this powerful feature.
Understanding Spread Types in TypeScript
Spread types in TypeScript provide a concise and efficient way to manipulate objects and arrays by spreading their properties onto another object or array. By utilizing the ellipsis (…) syntax, TypeScript enables developers to easily combine, clone, or modify objects and arrays, promoting code reuse and enhancing code readability.
Syntax and Usage
In TypeScript, spread types can be used in two contexts: object literals and array literals.
1. Object Literals:
By pre-fixing an object literal with three dots, TypeScript spreads the properties of the object onto a new object:
const originalObject = { x: 1, y: 2 };
const newObject = { …originalObject, z: 3 };
In the above example, the properties of originalObject (x: 1, y: 2) are spread onto the newObject, resulting in { x: 1, y: 2, z: 3 }.
One can also use spread types to clone an object:
const clonedObject = { …originalObject };
The clonedObject will have the same properties and values as the originalObject. Any modifications made to the clonedObject will not affect the originalObject, ensuring data integrity.
2. Array Literals:
Spread types also extend their functionality to arrays. By spreading an array onto a new array, one can combine or clone arrays easily:
const originalArray = [1, 2, 3];
const newArray = […originalArray, 4, 5, 6];
In this case, the elements of originalArray (1, 2, 3) are spread onto the newArray, resulting in [1, 2, 3, 4, 5, 6]. Similarly, one can clone an array:
const clonedArray = […originalArray];
The clonedArray will contain the same elements as the originalArray, and any modifications made to the clonedArray will not affect the originalArray.
Use Cases of Spread Types
Spread types offer a wide range of applications, aiding in various development scenarios. Here are some common use cases:
1. Object and Array Manipulation:
Spread types provide an effective means of merging or modifying objects and arrays. For instance, when working with Redux (a popular state management library), spread types facilitate the creation of new state objects by merging existing state properties with modified ones.
2. Function Arguments:
Spread types greatly simplify function calls by enabling the passing of multiple arguments as an array or spreading the properties of an object as individual arguments.
function multiply(a, b, c) {
return a * b * c;
}
const numbers = [2, 3, 4];
console.log(multiply(…numbers)); // Output: 24
In the above example, spread types are used to pass the array elements as individual arguments to the multiply() function.
3. JSX and React Components:
When working with JSX (a syntax extension for JavaScript used by React), spread types provide an elegant solution for dynamically assigning props to React components.
const props = { id: 1, name: “John Doe”, age: 25 };
return
By spreading the props onto the Component, the component receives the id, name, and age as separate props, enabling flexible component rendering.
Caveats and Best Practices
While spread types offer significant benefits, it is essential to be aware of their limitations and follow best practices for their efficient usage.
1. Shallow Copy:
The spread operator performs a shallow copy, meaning that only the top-level properties of objects or elements of arrays are copied. If the original object or array contains nested objects or arrays, they will still be referenced in the new object or array. To ensure a deep copy, developers should consider libraries like Lodash or implement custom deep cloning methods.
2. Ordering of Properties:
When spreading properties onto a new object, the ordering of properties might not be guaranteed, as JavaScript objects do not have a specific property order. Hence, reliance on the order of properties should be avoided. If order matters, consider using arrays instead.
FAQs about Spread Types in TypeScript
To further enhance understanding, let’s address some frequently asked questions about TypeScript spread types:
1. Can I spread multiple objects into one?
Yes, TypeScript allows multiple objects to be spread into a single object. For example:
const obj1 = { x: 1 };
const obj2 = { y: 2 };
const obj3 = { z: 3 };
const combinedObject = { …obj1, …obj2, …obj3 };
The combinedObject will contain properties from all three objects.
2. Can I mix object and array spreading?
Yes, spread types can be mixed. For instance:
const obj = { x: 1 };
const arr = [2, 3, 4];
const mixedSpread = { …obj, …arr };
The mixedSpread will merge the properties of obj and the elements of arr into a single object.
3. Can I use spread types with TypeScript generics?
Yes, spread types can be used with generics. TypeScript generics enable the reusability of code components, and spread types offer flexibility in manipulating and combining generic types.
Wrapping Up
TypeScript spread types provide a powerful mechanism for manipulating objects and arrays, facilitating code reuse and enhancing code clarity. By spreading properties onto new objects or arrays, developers can easily combine, clone, or modify data structures. Understanding the syntax, best practices, and use cases of spread types empowers developers to leverage this feature effectively, unlocking its full potential in TypeScript applications.
Images related to the topic spread types may only be created from object types
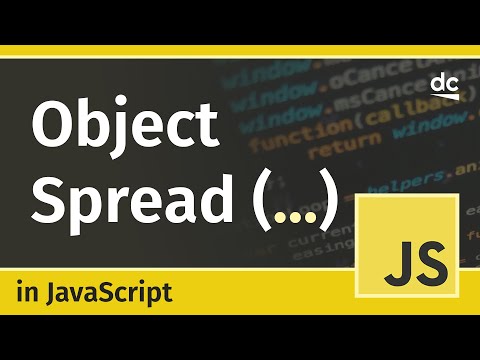
Found 10 images related to spread types may only be created from object types theme



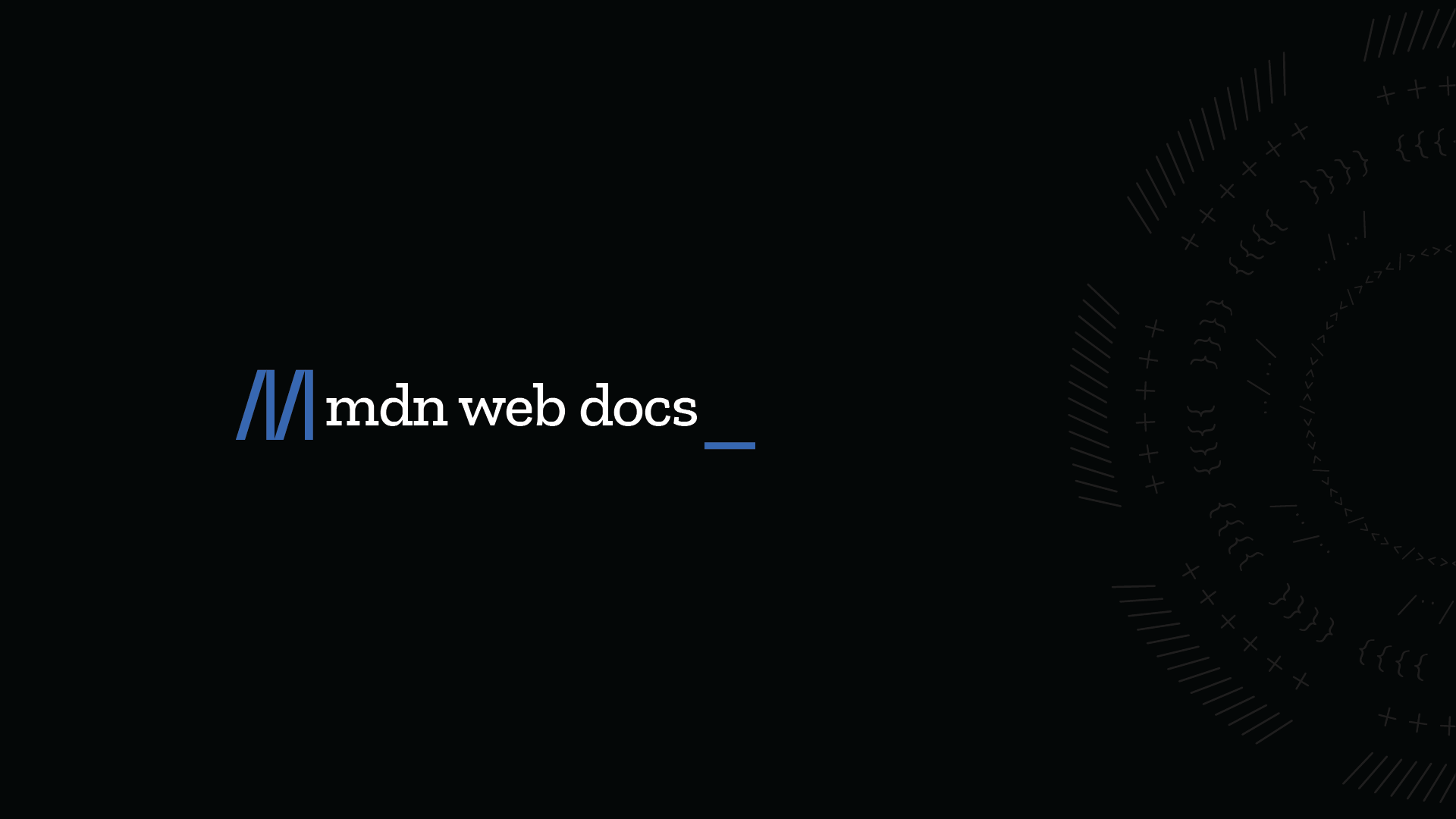

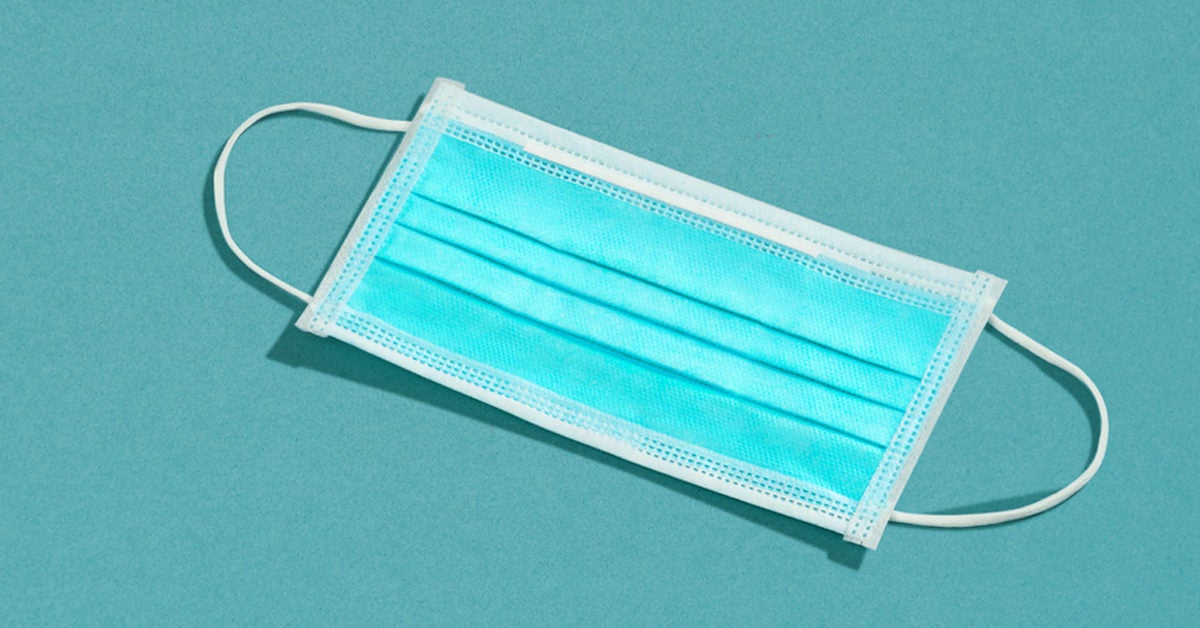
Article link: spread types may only be created from object types.
Learn more about the topic spread types may only be created from object types.
- Spread types may only be created from object types in TS
- Typescript: Spread types may only be created from object types
- [typescript] Spread types may only be created from object types.
- Spread Operator – Beginners Guide to TypeScript | newline – Fullstack.io
- Typing objects • Tackling TypeScript – Exploring JS
- TypeScript object Type
- Overlooked “Spread types may only be created from object …
- [typescript] Spread types may only be created from object types.
- Spread types may only be created from object type-Reactjs
- Documentation – Object Types – TypeScript
- Typescript: Spread types may only be created from object types
- Objects & Records – Type-Level TypeScript
- Không có tiêu đề
See more: nhanvietluanvan.com/luat-hoc