Sort Df By Date
Sorting data by date is a crucial aspect of data analysis, as it allows us to organize information in a meaningful and chronological order. Whether you are working with financial data, tracking sales, or analyzing trends over time, sorting a dataframe by date can provide valuable insights and simplify data manipulation.
When working with time series data, it is often necessary to sort the dataframe based on a specific date column. This ensures that the data is arranged in the correct temporal sequence, enabling us to perform various analyses, such as calculating trends, comparing periods, or generating visualizations.
In this article, we will explore how to sort a dataframe by date in both ascending and descending order, as well as how to sort by year, month, or day. Additionally, we will cover the handling of missing or incorrect dates, sorting by multiple columns including dates, sorting by time (date and time), and some advanced techniques for sorting dataframes by date.
Sorting a Dataframe by Date in Ascending Order
To sort a dataframe by date in ascending order, we can utilize the “sort_values” function from the Pandas library in Python. This function allows us to specify the column we want to sort by and the desired order.
Let’s say we have a dataframe named “df” with a column named “date” containing date values. We can sort this dataframe in ascending order using the following code:
“`
df_sorted = df.sort_values(by=’date’, ascending=True)
“`
The “by” parameter specifies the column we want to sort by, in this case, ‘date’. The “ascending” parameter is set to True, indicating that we want to sort in ascending order. The resulting sorted dataframe will now have the values arranged from the earliest date to the latest date.
Sorting a Dataframe by Date in Descending Order
To sort a dataframe by date in descending order, we need to set the “ascending” parameter to False in the “sort_values” function. This will arrange the dataframe in reverse chronological order, with the latest date appearing first.
Here’s an example code snippet for sorting a dataframe by date in descending order:
“`
df_sorted = df.sort_values(by=’date’, ascending=False)
“`
By setting “ascending” to False, the “sort_values” function will sort the dataframe in descending order based on the specified date column.
Sorting a Dataframe by Year, Month, or Day
In some cases, we may want to sort a dataframe based on specific components of a date, such as year, month, or day. The Pandas library provides convenient functions to extract these components from a date column and sort the dataframe accordingly.
Let’s consider a scenario where our dataframe has a ‘date’ column with dates in the format ‘YYYY-MM-DD’. To sort the dataframe by year, we can use the “sort_values” function along with the “dt.year” accessor, which extracts the year value from each date:
“`
df_sorted_by_year = df.sort_values(by=df[‘date’].dt.year)
“`
Similarly, we can sort the dataframe by month or day using the “dt.month” and “dt.day” accessors, respectively. The following examples demonstrate how to sort by month and day:
“`
df_sorted_by_month = df.sort_values(by=df[‘date’].dt.month)
df_sorted_by_day = df.sort_values(by=df[‘date’].dt.day)
“`
By utilizing these accessor functions, we can easily sort our dataframe based on specific components of a date, allowing for more precise sorting and analysis.
Handling Missing or Incorrect Dates in a Dataframe
When working with date data, it is not uncommon to encounter missing or incorrect dates in a dataframe. These inconsistencies can disrupt proper sorting and analysis. Therefore, it is crucial to handle such issues appropriately.
To handle missing dates, we can use the Pandas “dropna” function, which removes any rows containing missing values. If the missing dates are in the ‘date’ column, we can use the following code:
“`
df_cleaned = df.dropna(subset=[‘date’])
“`
The function ‘dropna’ is called on the dataframe and the ‘subset’ parameter specifies the column(s) from which the missing values should be dropped.
In the case of incorrect dates, it may be necessary to convert the date column into the appropriate date format before sorting. We can use the “to_datetime” function from Pandas to convert the column into a date format that can be sorted correctly.
“`
df[‘date’] = pd.to_datetime(df[‘date’])
df_sorted = df.sort_values(by=’date’)
“`
By converting the ‘date’ column to a datetime format, we can resolve any date inconsistencies and sort the dataframe accurately.
Sorting a Dataframe by Multiple Columns, including Date
Often, we may need to sort a dataframe by multiple columns to obtain a more nuanced order. This can be achieved by passing a list of column names to the “sort_values” function.
Assume we have a dataframe named ‘df’ containing three columns: ‘date’, ‘category’, and ‘value’. To sort the dataframe primarily by ‘date’ in ascending order and secondarily by ‘category’ in descending order, we can use the following code:
“`
df_sorted = df.sort_values(by=[‘date’, ‘category’], ascending=[True, False])
“`
The ‘by’ parameter now takes a list of column names, and the ‘ascending’ parameter also takes a list of corresponding sorting orders for each column.
Sorting a Dataframe by Time, including Date and Time
In some cases, data may include a time component in addition to the date. Sorting a dataframe by both date and time becomes necessary to have a comprehensive chronological order.
To sort a dataframe by date and time, we can combine the date and time columns into a single column of datetime objects. We then use this new column for sorting.
Assume our dataframe ‘df’ contains two columns, ‘date’ and ‘time’. We can create a new column named ‘datetime’ by combining these two columns using the ‘pd.to_datetime’ function:
“`
df[‘datetime’] = pd.to_datetime(df[‘date’] + ‘ ‘ + df[‘time’])
df_sorted = df.sort_values(by=’datetime’)
“`
We first concatenate the ‘date’ and ‘time’ columns using the ‘+’ operator and pass the result to ‘pd.to_datetime’ to convert it into a datetime format. The resulting ‘datetime’ column can then be used for sorting the dataframe.
Advanced Techniques for Sorting Dataframes by Date
Beyond the basics covered so far, there are several advanced techniques and functionalities available for sorting dataframes by date.
Sort dataframe based on date column: This can be achieved using the ‘sort_values’ function, as shown in previous examples.
Sort date in Python: Python provides powerful libraries like Pandas and Numpy that offer various methods to sort dates in different formats.
Sort index datetime Pandas: Dataframes in Pandas can have indexed columns. To sort the dataframe based on the index datetime column, we can use the ‘sort_index’ function.
Pandas sort by timestamp: If a dataframe includes a timestamp column in addition to the date, we can sort the dataframe by timestamp using the same techniques mentioned earlier.
Pd to_datetime: The ‘pd.to_datetime’ function in Pandas converts a column or array of values to a datetime format, facilitating proper sorting of date-related data.
Sort value Pandas: In addition to the ‘sort_values’ function, Pandas also provides the ‘sort_index’ function, which sorts the dataframe based on the index values. ‘sort_index’ is particularly useful when sorting by date index.
Pandas filter date: The ‘loc’ function in Pandas allows us to filter a dataframe based on specific date ranges or conditions, enabling us to focus on specific periods of interest and sort the results accordingly.
Pandas sort by day of the week: We can sort a dataframe by the day of the week using the ‘sort_values’ function in conjunction with the ‘dt.dayofweek’ accessor.
In conclusion, sorting dataframes by date is an essential skill for any data analyst or scientist. Understanding how to sort by ascending or descending order, as well as sorting by year, month, or day, allows us to arrange data in a meaningful and chronological manner. Handling missing or incorrect dates, sorting by multiple columns (including dates), and sorting by time are additional techniques that can further enhance data analysis. With the advanced techniques and powerful functionalities offered by libraries like Pandas, Python provides an excellent environment for sorting dataframes efficiently and effectively.
Sort Pandas Dataframe By Date In Python (Example) | Order/Rearrange Rows | To_Datetime \U0026 Sort_Values
Keywords searched by users: sort df by date Sort dataframe based on date column, Sort date in Python, Sort index datetime Pandas, Pandas sort by timestamp, Pd to_datetime, Sort value Pandas, Pandas filter date, Pandas sort by day of week
Categories: Top 37 Sort Df By Date
See more here: nhanvietluanvan.com
Sort Dataframe Based On Date Column
When working with data in pandas, it is often necessary to sort the data based on a specific column. One common scenario is sorting a dataframe based on a date column. In this article, we will explore different approaches to achieve this task and provide a comprehensive guide to sorting a dataframe by date.
Sorting a dataframe based on a date column is useful in situations where you want to arrange your data chronologically. This is often the case when working with time series data or any data that has a temporal aspect to it. By sorting the dataframe by date, you can easily analyze trends, identify patterns, and make data-driven decisions based on the chronological order.
There are several ways to sort a dataframe based on a date column. Let’s take a look at some of the most commonly used methods.
Method 1: Using the sort_values() function
The sort_values() function in pandas allows us to sort a dataframe based on one or more columns. To sort a dataframe by a date column, you can simply pass the name of the desired date column as a string to the sort_values() function, like this:
“`
df.sort_values(‘date_column’)
“`
By default, this function sorts the dataframe in ascending order. If you want to sort it in descending order, you can specify the `ascending=False` parameter, like this:
“`
df.sort_values(‘date_column’, ascending=False)
“`
Method 2: Using the sort_index() function
Another way to sort a dataframe by a date column is by using the sort_index() function. This function sorts the dataframe based on the index values. To sort the dataframe by the date column, you need to set the date column as the index first, and then use the sort_index() function. Here’s an example:
“`
df.set_index(‘date_column’).sort_index()
“`
This will set the date_column as the index and sort the dataframe based on the index values.
Method 3: Using the sort() function
The sort() function is a method available on the dataframe object itself. It allows you to sort the dataframe based on one or more columns. To use the sort() function to sort a dataframe by a date column, you can pass the name of the desired date column to the function, like this:
“`
df.sort_values(by=’date_column’)
“`
By default, this function sorts the dataframe in ascending order. If you want to sort it in descending order, you can specify the `ascending=False` parameter, like this:
“`
df.sort_values(by=’date_column’, ascending=False)
“`
These are some of the most common methods to sort a dataframe based on a date column. Depending on your specific requirements, you can choose the method that suits your needs the best.
FAQs (Frequently Asked Questions)
Q1. Can I sort a dataframe based on multiple columns?
Yes, all the methods mentioned above support sorting a dataframe based on multiple columns. You can pass a list of column names to the sort_values() function, sort_index() function, or use the sort() function with multiple columns.
Q2. How can I sort a dataframe in place?
By default, these sorting functions return a new sorted dataframe without modifying the original dataframe. However, if you want to sort the dataframe in place, you can use the `inplace=True` parameter, like this: `df.sort_values(‘date_column’, inplace=True)`.
Q3. What if my date column is not in the correct format?
If your date column is not in the correct format, such as being stored as a string, you need to convert it to a datetime format before sorting. You can use the `pd.to_datetime()` function to convert the date column to the desired format.
Q4. How do I sort a dataframe based on a date column in a specific format?
If your date column is in a specific format that is not recognized by pandas, you can specify the format using the `format` parameter of the `pd.to_datetime()` function. For example, if your date column is in the format ‘yyyy-mm-dd’, you can convert it like this: `pd.to_datetime(df[‘date_column’], format=’%Y-%m-%d’)`.
Q5. Can I specify the sort order for individual columns?
Yes, you can specify the sort order for individual columns by using the `ascending` parameter. This parameter accepts a boolean value or a list of boolean values. By default, it is set to `True`, which sorts the column in ascending order. If you want to sort a specific column in descending order, you can set `ascending=False` for that column.
In conclusion, sorting a dataframe based on a date column is a common task when working with temporal data. We have discussed different methods to achieve this, such as using the sort_values() function, sort_index() function, and sort() function. Moreover, we have also answered some frequently asked questions related to sorting dataframes by a date column. By utilizing these techniques, you can efficiently organize and analyze your data in a chronological manner, enabling you to derive meaningful insights.
Sort Date In Python
Before we dive into the details, it’s important to note that dates in Python are typically represented as objects from the `datetime` module. These objects contain attributes like year, month, day, hour, minute, second, and others, allowing for precise manipulation and comparisons of dates.
1. Sorting Dates Using Built-in Functions:
Python’s built-in `sorted()` function can be used to sort a list of date strings. However, before sorting, it’s important to convert the date strings into comparable objects. This can be achieved by utilizing the `strptime()` function from the `datetime` module, which converts date strings to `datetime` objects.
“`python
from datetime import datetime
dates = [‘2022-01-01’, ‘2021-06-15’, ‘2023-03-10’]
sorted_dates = sorted(dates, key=lambda x: datetime.strptime(x, “%Y-%m-%d”))
print(sorted_dates)
“`
In this example, the `key` parameter is set to a lambda function that converts the date strings to `datetime` objects using the `strptime()` function. The resulting sorted list will be `[‘2021-06-15’, ‘2022-01-01’, ‘2023-03-10’]`, ensuring the dates are sorted in ascending order.
2. Sorting Dates Using the `dateutil` Library:
The `dateutil` library is a powerful alternative to Python’s built-in `datetime` module, providing additional functionality for working with dates. Sorting dates using `dateutil` can be done by employing the `parser.parse()` function from the library.
“`python
from dateutil import parser
dates = [‘2022-01-01’, ‘2021-06-15’, ‘2023-03-10’]
parsed_dates = [parser.parse(date) for date in dates]
sorted_dates = sorted(parsed_dates)
print(sorted_dates)
“`
In this example, the `parser.parse()` function converts each date string into a `datetime` object, which can then be sorted using the `sorted()` function. The resulting sorted list will be `[datetime.datetime(2021, 6, 15, 0, 0), datetime.datetime(2022, 1, 1, 0, 0), datetime.datetime(2023, 3, 10, 0, 0)]`.
3. Sorting Dates Using the `pandas` Library:
For more complex projects involving large datasets, the `pandas` library can be a valuable tool for manipulating and sorting dates. `pandas` provides the `to_datetime()` function, which conveniently converts a sequence of date-like objects (strings or numeric values) to `datetime64[ns]` objects. These objects can then be easily sorted using the `sort_values()` function.
“`python
import pandas as pd
dates = [‘2022-01-01’, ‘2021-06-15’, ‘2023-03-10’]
df = pd.DataFrame({‘dates’: dates})
df[‘dates’] = pd.to_datetime(df[‘dates’])
sorted_df = df.sort_values(by=’dates’)
print(sorted_df[‘dates’].tolist())
“`
In this example, the `to_datetime()` function converts the date strings in the DataFrame column into `datetime64[ns]` objects. The resulting sorted list will be `[Timestamp(‘2021-06-15 00:00:00’), Timestamp(‘2022-01-01 00:00:00’), Timestamp(‘2023-03-10 00:00:00′)]`.
FAQs:
Q1: Can these methods handle different date formats?
A1: Yes, all the methods mentioned above can handle different date formats. However, it is important to ensure that the format specified in the conversion functions (`strptime()`, `parser.parse()`, `to_datetime()`) matches the format of the dates being converted.
Q2: Can these methods handle time zones?
A2: Yes, the `datetime` module handles time zones by providing the `datetime` object with the `tzinfo` attribute. Similarly, the `pandas` library can handle time zones through its `to_datetime()` function, which has a `utc` parameter to convert the dates to UTC.
Q3: Are there any performance considerations when sorting large datasets?
A3: When working with large datasets, it is recommended to use the `pandas` library due to its efficient handling of data. However, the choice of method also depends on the specific requirements of the project and the available system resources.
Q4: Is it possible to sort dates in descending order?
A4: Yes, by default, all the methods mentioned above sort dates in ascending order. To sort dates in descending order, you can utilize the `reverse` parameter for the `sorted()` function or the `ascending` parameter for `pandas`’ `sort_values()` function.
In conclusion, sorting dates in Python can be accomplished utilizing different methods and libraries, such as the built-in functions, the `dateutil` library, or the `pandas` library. Each method offers unique capabilities and considerations, allowing developers to efficiently sort date data based on their specific requirements. By understanding these approaches, developers can easily manage and organize date-related information in their Python projects.
Images related to the topic sort df by date
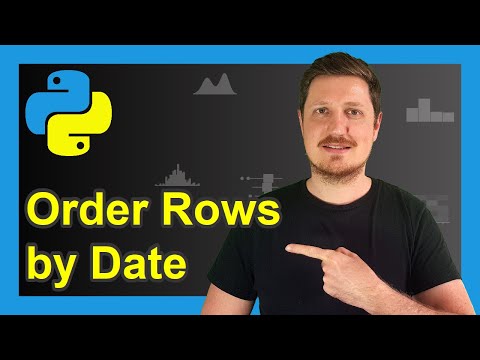
Found 26 images related to sort df by date theme
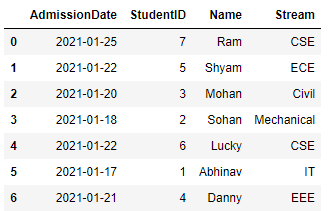
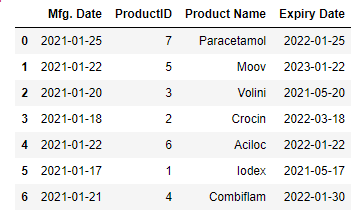
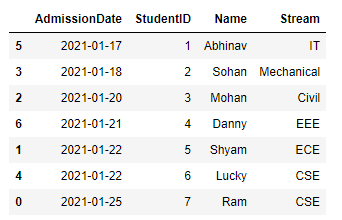
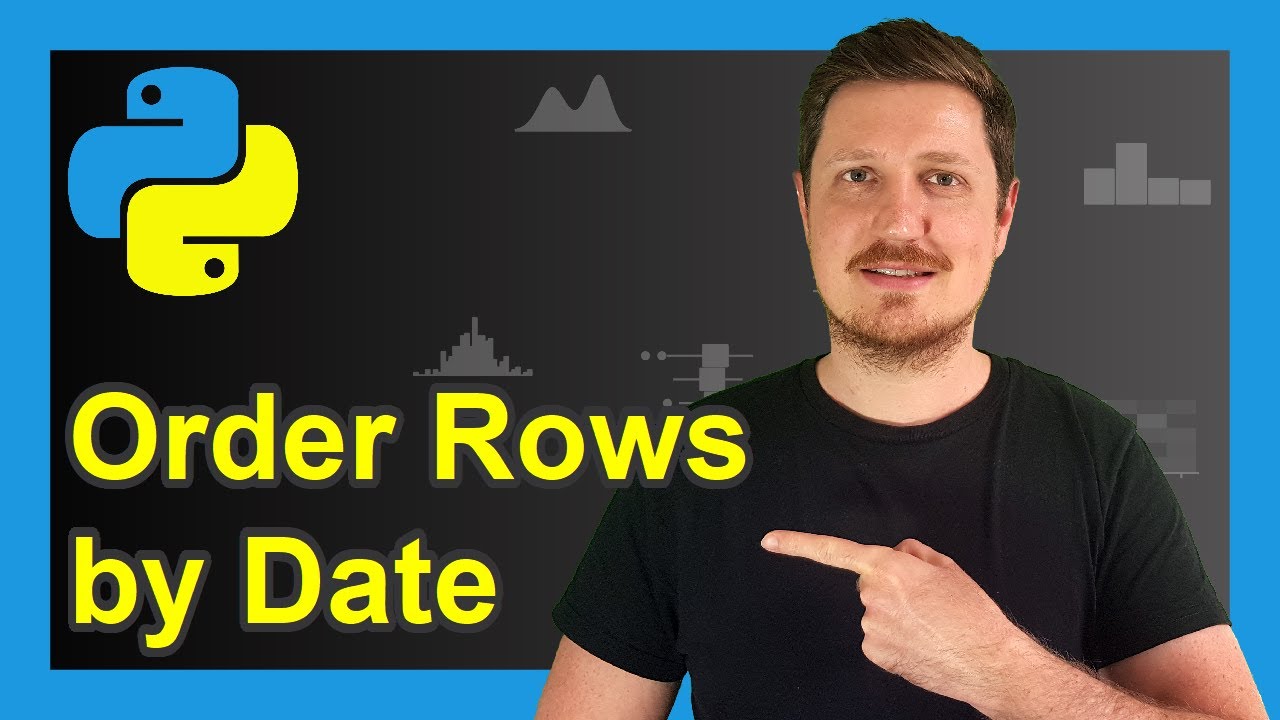

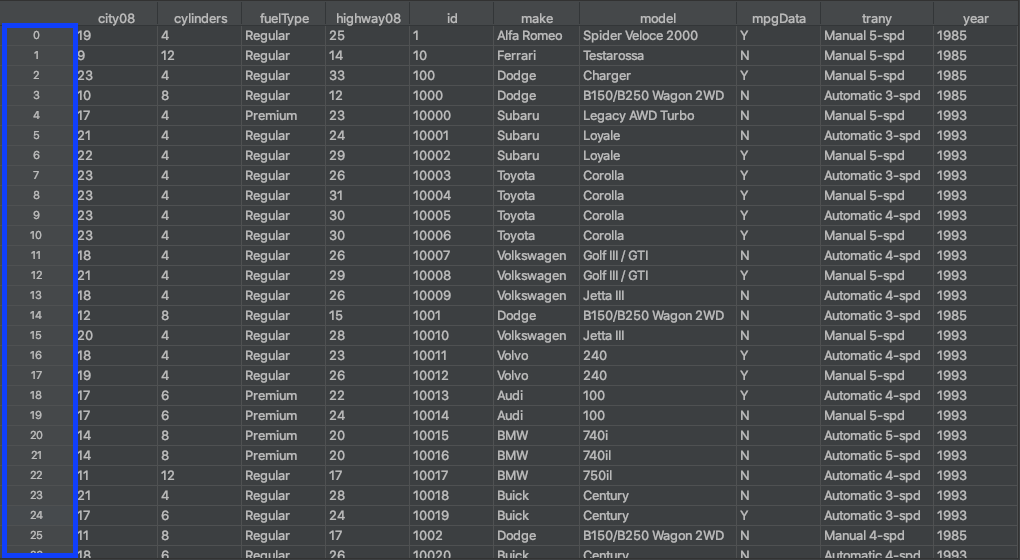
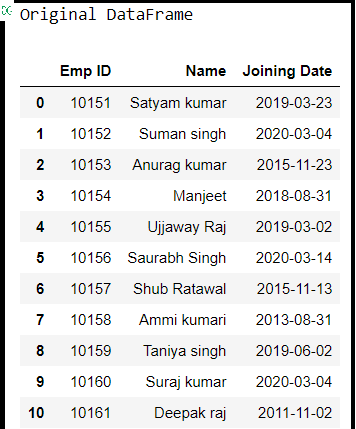
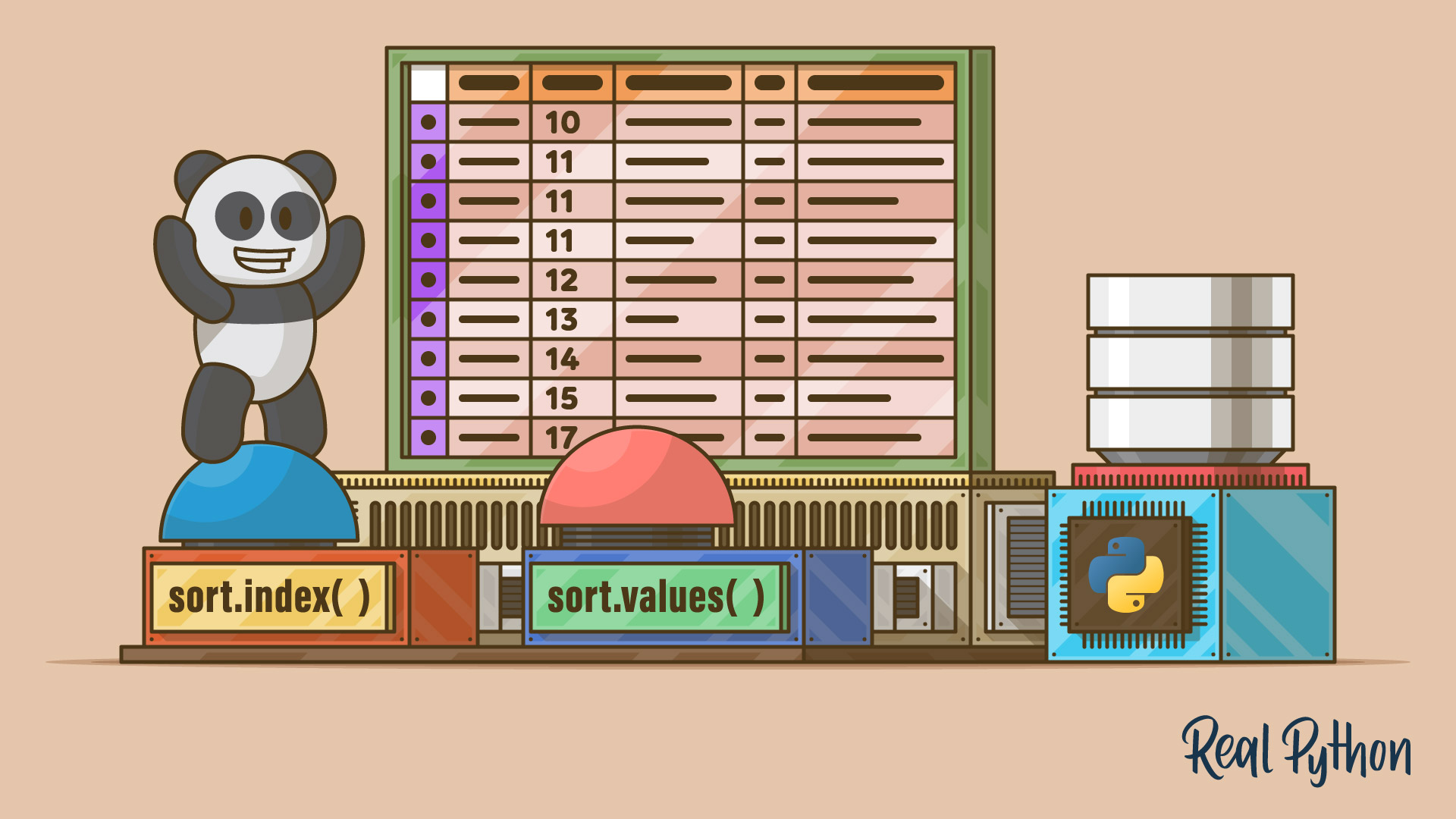
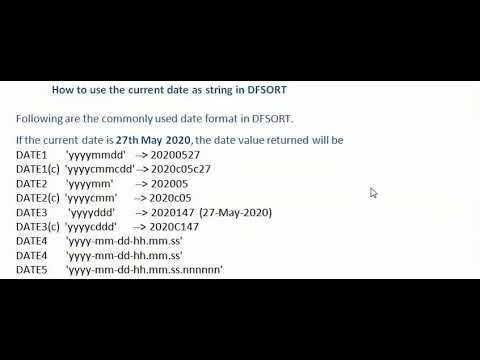
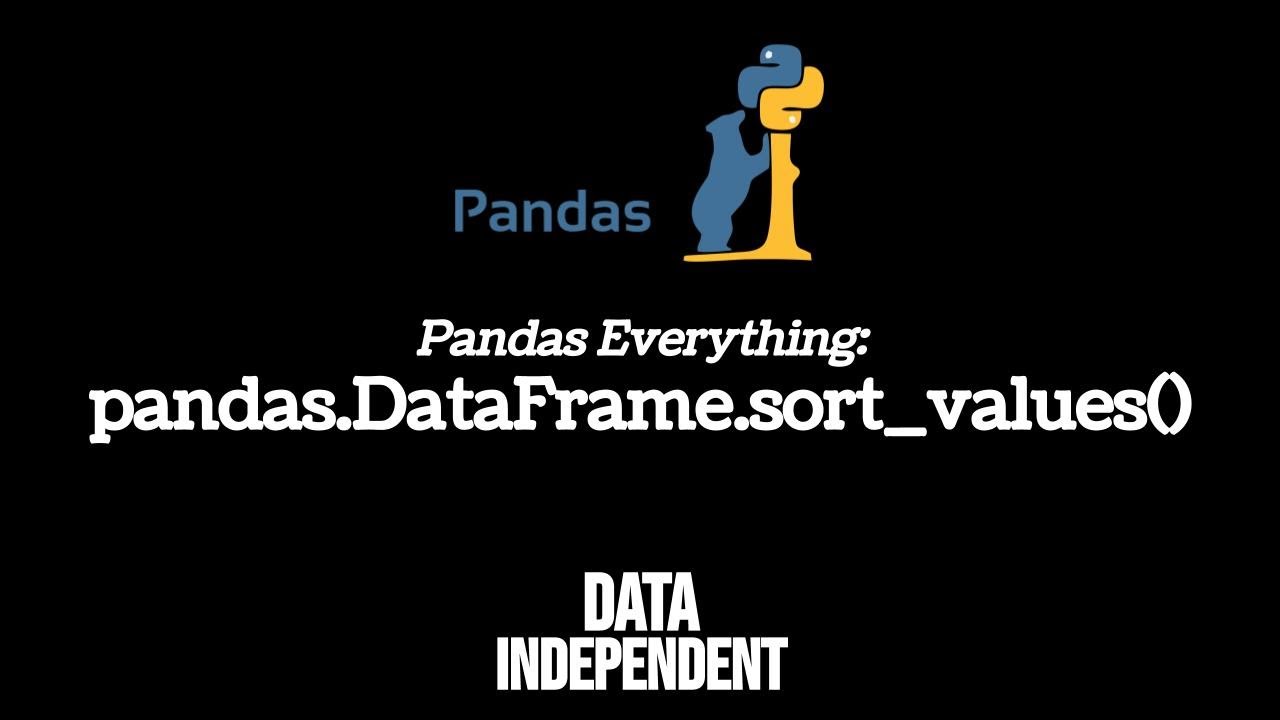

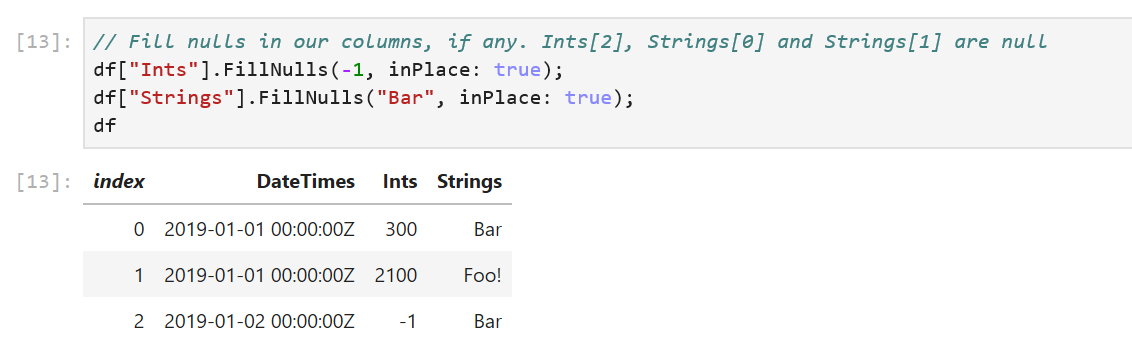
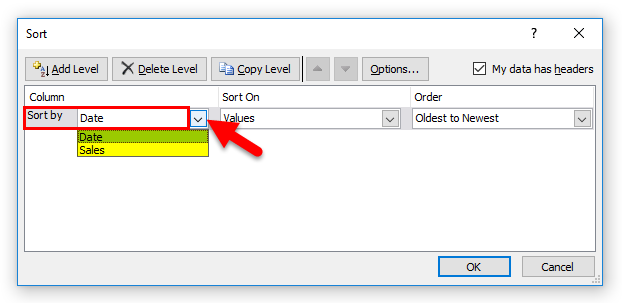


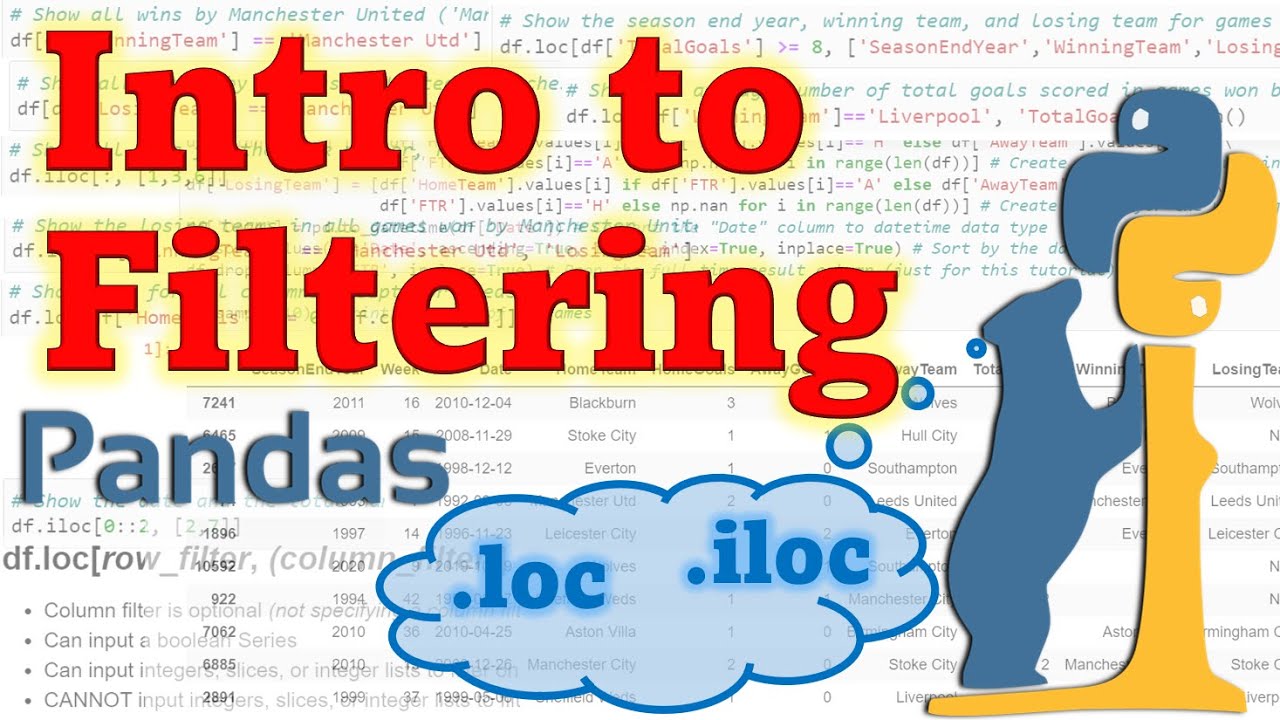
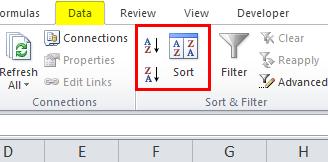


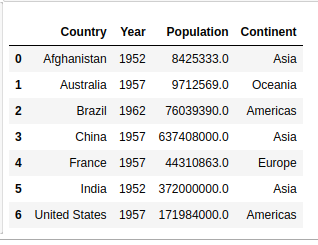
Article link: sort df by date.
Learn more about the topic sort df by date.
- How to Sort a Pandas DataFrame by Date? – GeeksforGeeks
- Sort Pandas DataFrame by Date (Datetime)
- How to Sort a Pandas DataFrame by Date – Stack Abuse
- Pandas Sort By Date – Linux Hint
- Convert Column to Date Format (Pandas Dataframe)
- Sort pandas DataFrame by Date in Python (Example)
- How to sort a Pandas dataframe by date, month and year?
- Sorting DataFrame by dates in Pandas – SkyTowner
- How to Sort a Pandas DataFrame by Date (With Examples)
See more: https://nhanvietluanvan.com/luat-hoc/