Sequence Contains No Elements
1. Definition of an Empty Sequence
In the realm of mathematics and computer science, a sequence is a collection of elements that are arranged in a specific order. However, it is also possible for a sequence to contain no elements at all. Such a sequence is commonly referred to as an “empty sequence.”
An empty sequence is essentially a set of elements that is devoid of any contents. It is void of any data, values, or elements that can be manipulated or processed. While it may seem paradoxical, the concept of an empty sequence plays an important role in various fields of study.
2. Properties and Characteristics of an Empty Sequence
To better understand the properties and characteristics of an empty sequence, let’s take a closer look at its defining attributes:
2.1. Length: Unlike traditional sequences that have an identifiable length, an empty sequence has a length of zero. This implies that it does not contain any elements, and thus its count is zero.
2.2. Accessing Elements: In most programming languages and mathematical frameworks, accessing elements of a sequence involves referencing their indices. However, due to its lack of elements, an empty sequence does not have any indices to reference. Attempting to access elements from an empty sequence often results in errors or exceptions.
2.3. Equality: When comparing an empty sequence to another sequence, the two are considered equal if both are empty. It is important to note that an empty sequence is not the same as a null or uninitialized sequence, as those have a distinct state within programming languages.
3. Examples and Illustrations of Empty Sequences
To further illustrate the concept of an empty sequence, consider the following examples:
3.1. Empty String: In many programming languages, an empty string (“” or ”) can be considered as an empty sequence of characters. It represents a sequence without any symbols or letters.
3.2. Empty List: Similarly, an empty list ([] or List<>) can be viewed as an example of an empty sequence. The list does not contain any elements, and hence its length is zero.
3.3. Empty Set: In set theory, an empty set (∅) is an example of an empty sequence. It is a collection with no members, signifying the absence of any elements.
4. Empty Sequence in Mathematics and Computer Science
The concept of an empty sequence is widely used in mathematics and computer science. Here are some of its applications and significance:
4.1. Error Handling: When working with sequences, it is crucial to handle scenarios where the sequence may not contain any elements. By utilizing the concept of an empty sequence, programmers can safeguard against potential errors or exceptions when dealing with empty data sets.
4.2. Sequence Processing: In some algorithms or operations, an empty sequence may act as an initial state or a base case. It provides a starting point for iterative processes or calculations, allowing the algorithm to proceed with its logic even when the sequence is empty.
4.3. Querying and Filtering: Many programming languages, such as C#, provide built-in query and filtering mechanisms using language features like LINQ (Language-Integrated Query). These features allow developers to manipulate sequences efficiently, including scenarios where the sequence may be empty.
5. FAQs
5.1. What is the difference between First and FirstOrDefault in C# LINQ?
The difference lies in their behavior when the sequence contains no elements. The First method throws an exception, whereas the FirstOrDefault method returns the default value of the element type (null for reference types, 0 for numeric types, etc.) when the sequence is empty.
5.2. How do I check if a sequence contains no elements in C# LINQ?
One way to determine if a sequence contains no elements is to utilize the Any method. If the Any method returns false, it signifies that the sequence is empty.
5.3. What does the DefaultIfEmpty method in C# LINQ do?
The DefaultIfEmpty method in C# LINQ returns a new sequence that contains the original elements if the sequence is not empty. However, if the sequence is empty, it returns a new sequence with a single default value element.
6. Conclusion
Understanding the concept of an empty sequence is vital in the fields of mathematics and computer science. Despite its lack of elements, an empty sequence plays a crucial role in error handling, algorithm design, and data manipulation. By utilizing features like C# LINQ’s FirstOrDefault or DefaultIfEmpty, developers can efficiently handle scenarios where a sequence contains no elements, ensuring smoother program execution and enhanced code reliability.
Sequence Contains No Elements In C# Sql
Keywords searched by users: sequence contains no elements C# Linq FirstOrDefault, LINQ First, Defaultifempty list c#, Single LINQ, List FirstOrDefault c#, DefaultIfEmpty, First vs FirstOrDefault, System linq enumerable max ienumerable 1 source
Categories: Top 21 Sequence Contains No Elements
See more here: nhanvietluanvan.com
C# Linq Firstordefault
Introduction:
In the world of C# programming, LINQ (Language Integrated Query) is a powerful tool that enables developers to query and manipulate data in a concise and expressive manner. Among the numerous LINQ operators available, FirstOrDefault stands out as a convenient method for retrieving the first element of a sequence, or a default value if the sequence is empty. In this article, we will dive deep into the world of C# LINQ FirstOrDefault, exploring its syntax, possible variations, use cases, and frequently asked questions.
Syntax and Usage:
FirstOrDefault is a LINQ extension method that belongs to the Enumerable class and is widely used to return the first element of a sequence or the default value when the sequence is empty. Let’s take a look at the basic syntax:
“`
public static TSource FirstOrDefault
“`
The FirstOrDefault method has a type parameter, TSource, which represents the type of the elements in the sequence being queried. It takes in an IEnumerable
Consider the following example, where we have a list of integers, numbers, and we want to find the first number greater than 5:
“`csharp
List
int firstNumberGreaterThan5 = numbers.FirstOrDefault(x => x > 5);
Console.WriteLine(firstNumberGreaterThan5);
“`
The output will be 7, as it is the first number greater than 5 in the list.
Variations and Customizations:
FirstOrDefault can be customized further by providing a predicate function that filters the elements before selecting the first one. This allows you to find the first element that matches a specific condition. Let’s extend our previous example to find the first even number greater than 5:
“`csharp
int firstEvenNumberGreaterThan5 = numbers.FirstOrDefault(x => x > 5 && x % 2 == 0);
Console.WriteLine(firstEvenNumberGreaterThan5);
“`
In this case, the output will be 2, as it is the first even number greater than 5.
Use Cases:
1. Handling Empty Collections:
One of the primary use cases of FirstOrDefault is handling empty collections gracefully. By providing a default value, such as null or a user-defined default object, you can prevent runtime exceptions when attempting to retrieve the first element from an empty sequence.
2. Conditional Search:
FirstOrDefault allows you to efficiently search for the first element that satisfies a particular condition. This is especially useful when working with large datasets, as it provides a quick way to find the desired element without iterating over the entire sequence.
3. Handling Unordered Sequences:
When dealing with unordered sequences, the concept of the “first” element becomes arbitrary. However, FirstOrDefault ensures predictable behavior by returning the element that satisfies the specified condition and occurs earliest in the sequence.
FAQs:
Q1. What is the difference between First and FirstOrDefault in LINQ?
A1. The main difference lies in how they handle empty collections. First throws an exception if the sequence is empty, while FirstOrDefault returns the default value for the type of elements in the sequence.
Q2. How can I specify a custom default value in FirstOrDefault?
A2. FirstOrDefault has an overloaded version that allows you to set a custom default value. You can use the following syntax:
“`csharp
TSource FirstOrDefault
“`
Q3. What if there are multiple elements satisfying the condition?
A3. FirstOrDefault will still return the first element that satisfies the condition and occurs earliest in the sequence.
Q4. Can I use FirstOrDefault with other LINQ operators?
A4. Yes, you can combine FirstOrDefault with other LINQ operators like Where, Select, and OrderBy to further tailor your query.
Q5. Are there any performance considerations when using FirstOrDefault?
A5. While FirstOrDefault provides an efficient way to retrieve the first element, it still involves iterating over the sequence until a match is found. Therefore, it is recommended to use FirstOrDefault judiciously, especially with large data sets.
Conclusion:
In this article, we covered the C# LINQ FirstOrDefault operator comprehensively. We explored its syntax, variations, and common use cases where it shines. FirstOrDefault is a valuable tool for developers dealing with collections, allowing them to gracefully handle empty sequences, conditionally search for elements, and guarantee predictable behavior with unordered data. By understanding the intricacies of FirstOrDefault, C# programmers can write more concise and effective code.
Linq First
In the world of software development, efficient data manipulation is key to creating functional and dynamic applications. Traditionally, accessing and manipulating data required writing complex and lengthy code. However, LINQ (Language Integrated Query) has revolutionized the way developers handle data by providing a simplified and streamlined approach. In this article, we will explore LINQ First, a fundamental component of LINQ that allows developers to retrieve the first element of a sequence based on a specific condition. We will delve into the inner workings of LINQ First, its benefits, and how it can be effectively used in practical scenarios.
Understanding LINQ First
LINQ First is an extension method that simplifies the process of extracting the first element that matches a specific condition from a sequence. A sequence can be any collection or enumerable object, such as arrays, lists, or databases. By utilizing LINQ First, developers can easily retrieve the first occurrence from a sequence without the need for complex iteration or conditional statements.
Benefits of LINQ First
1. Simplified syntax: With LINQ First, developers can avoid writing lengthy code to iterate through a sequence and find the desired element. The syntax is concise and easy to understand, reducing the chances of errors and making the code more maintainable.
2. Improved performance: LINQ First optimizes the search process by employing optimized algorithms. By utilizing these algorithms, LINQ First executes the search more efficiently, resulting in improved performance.
3. Code readability: LINQ First enhances code readability by eliminating the need for nested loops or conditional statements. This makes the code more understandable for both the original developers and any future maintainers.
4. Error handling: LINQ First automatically handles scenarios where a sequence is empty or contains no elements matching the given condition. It gracefully handles such cases by throwing an exception, allowing developers to handle these scenarios appropriately.
5. Enhanced productivity: By eliminating the need for complex iterative processes, LINQ First allows developers to write code more quickly. This streamlined approach saves time and enhances productivity.
Utilizing LINQ First in practice
Here are a few examples demonstrating how LINQ First can be effectively used in various scenarios:
1. Searching for a specific element: Suppose we have a list of customer objects and our aim is to find the first customer with a specific ID. We can achieve this using LINQ First:
“`csharp
List
Customer firstCustomer = customers.First(c => c.ID == desiredID); // Retrieves the first customer with the desired ID
“`
2. Retrieving the first element of a sorted sequence: Let’s say we have a list of numbers and we want to retrieve the first element after sorting the sequence. LINQ First can simplify this process significantly:
“`csharp
List
int firstElement = numbers.OrderBy(n => n).First(); // Retrieves the first element after sorting the sequence
“`
3. Organizing data hierarchies: Suppose we have a hierarchical data structure represented as a collection of objects. We can use LINQ First to retrieve the first child element from each parent object:
“`csharp
List
List
“`
FAQs:
Q: What happens if LINQ First is used on an empty sequence?
A: When LINQ First is utilized on an empty sequence, it throws an InvalidOperationException. This exception alerts developers to the fact that the sequence doesn’t contain any elements, allowing them to handle this scenario accordingly.
Q: Can LINQ First be used on any type of collection?
A: Yes, LINQ First can be used on any IEnumerable or IQueryable collection. This includes arrays, lists, dictionaries, and even database queries.
Q: Is it possible to retrieve the first element without specifying a condition?
A: Yes, it is possible to use LINQ First without specifying a condition. In this case, the first element of the sequence will be returned.
Q: Is LINQ First case-sensitive?
A: Yes, LINQ First is case-sensitive. When specifying a condition, make sure to consider the case sensitivity of the data being queried.
Q: What other LINQ methods can be used in conjunction with LINQ First?
A: LINQ First can be combined with other LINQ methods such as Where, Select, and OrderBy to further refine queries and manipulate data according to specific requirements.
In conclusion, LINQ First simplifies data manipulation significantly by providing a concise and efficient way to retrieve the first element of a sequence. Its benefits, including simplified syntax, improved performance, and enhanced code readability, make it a valuable tool for developers. By utilizing LINQ First in practical scenarios, developers can streamline their code, enhance productivity, and efficiently handle data manipulation tasks.
Defaultifempty List C#
In C#, working with lists is a common task. There are times when we need to manipulate the data in a list and perform various operations on it. One such operation is the need to provide a default value when a list is empty. This is where the DefaultIfEmpty method comes into play. In this article, we will explore the DefaultIfEmpty list in C#, its functionality, and how to use it effectively.
Understanding DefaultIfEmpty List
The DefaultIfEmpty method in C# is part of the LINQ (Language Integrated Query) extension methods. It is used to return a new sequence that contains the default value of the type of elements in the original sequence when the sequence is empty. In simpler terms, if a list does not contain any elements, the DefaultIfEmpty method allows us to specify a default value to be returned instead.
Syntax and Usage
The syntax for using the DefaultIfEmpty method is as follows:
var result = originalList.DefaultIfEmpty(defaultValue);
Here, originalList refers to the list on which the method is called, and defaultValue is the value that will be returned if the original list is empty.
Let’s consider a scenario to understand its usage better. Suppose we have a list of integers called numbers, and we want to perform some operation on it. However, if the list is empty, we want to return -1 as the default value. We can achieve this using the DefaultIfEmpty method as shown below:
var result = numbers.DefaultIfEmpty(-1);
In this case, the result will be a new list that contains -1 if the numbers list is empty.
Benefits of Using DefaultIfEmpty
The DefaultIfEmpty method provides several benefits when working with lists in C#:
1. Handling Empty Lists: When dealing with lists, it is crucial to handle the scenario where the list might be empty. With DefaultIfEmpty, we can provide a default value or a specific action to be performed when an empty list is encountered.
2. Smooth Operation: By explicitly providing a default value, we can avoid potential errors or exceptions that may occur due to referencing an empty list.
3. Flexibility: The DefaultIfEmpty method allows us to choose any default value of our choice. This flexibility ensures that the returned value fits our specific requirements.
FAQs
Q: Can the DefaultIfEmpty method be used with any collection type in C#?
A: Yes, the DefaultIfEmpty method can be used with any collection type, be it a list, array, or any other collection that implements IEnumerable.
Q: What happens if the original list contains elements?
A: If the original list contains one or more elements, the DefaultIfEmpty method will return the elements of the original list as is.
Q: Is it possible to chain multiple LINQ methods with DefaultIfEmpty?
A: Yes, the DefaultIfEmpty method can be combined with other LINQ methods like Where, Select, OrderBy, etc., to manipulate and perform complex operations on the list.
Q: Can I call the DefaultIfEmpty method with no arguments?
A: Yes, the DefaultIfEmpty method can be called with no arguments when we want to use the default value of the element type.
Q: Can I provide a custom default value that is not of the element type?
A: No, the default value provided must be of the same type as the elements in the original list.
Conclusion
In this article, we explored the DefaultIfEmpty list in C# and its usage. We learned how to use the DefaultIfEmpty method to return a specific default value when a list is empty, and how it can handle different scenarios. By using the DefaultIfEmpty method appropriately, we can ensure smooth and error-free operations while working with lists in C#.
Images related to the topic sequence contains no elements
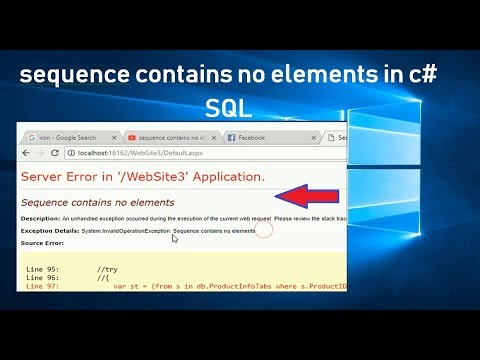
Found 20 images related to sequence contains no elements theme

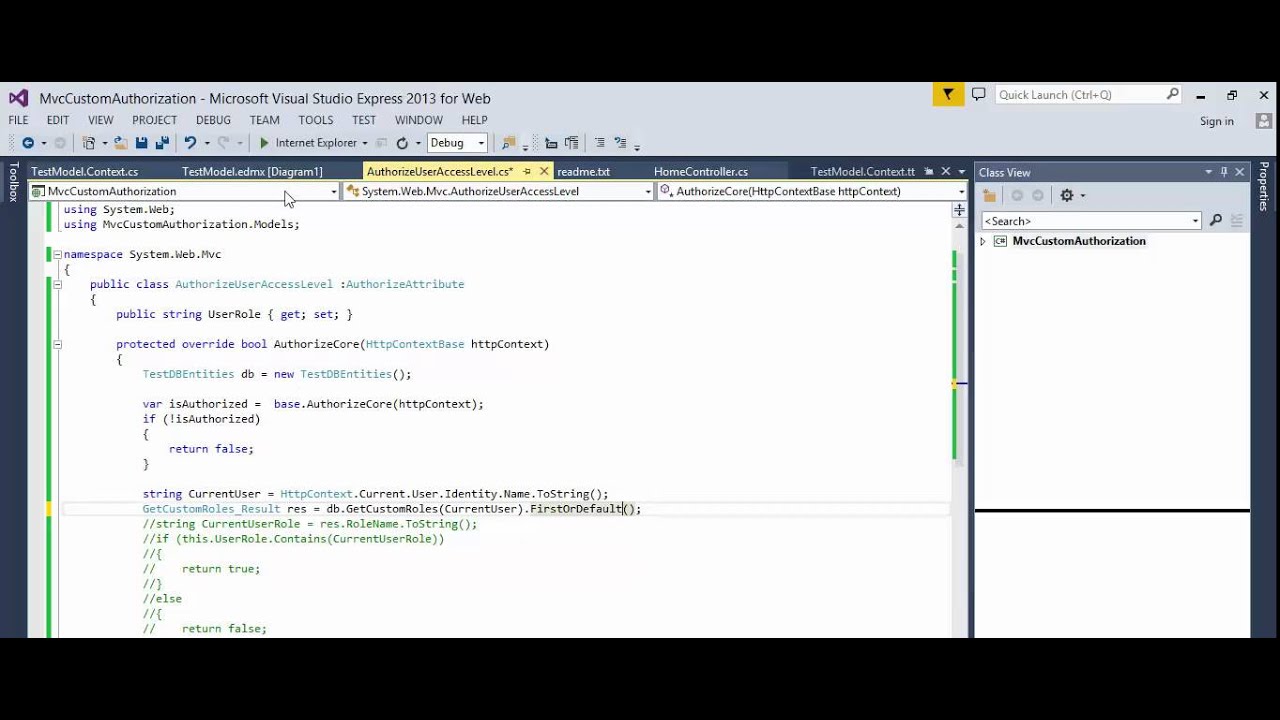


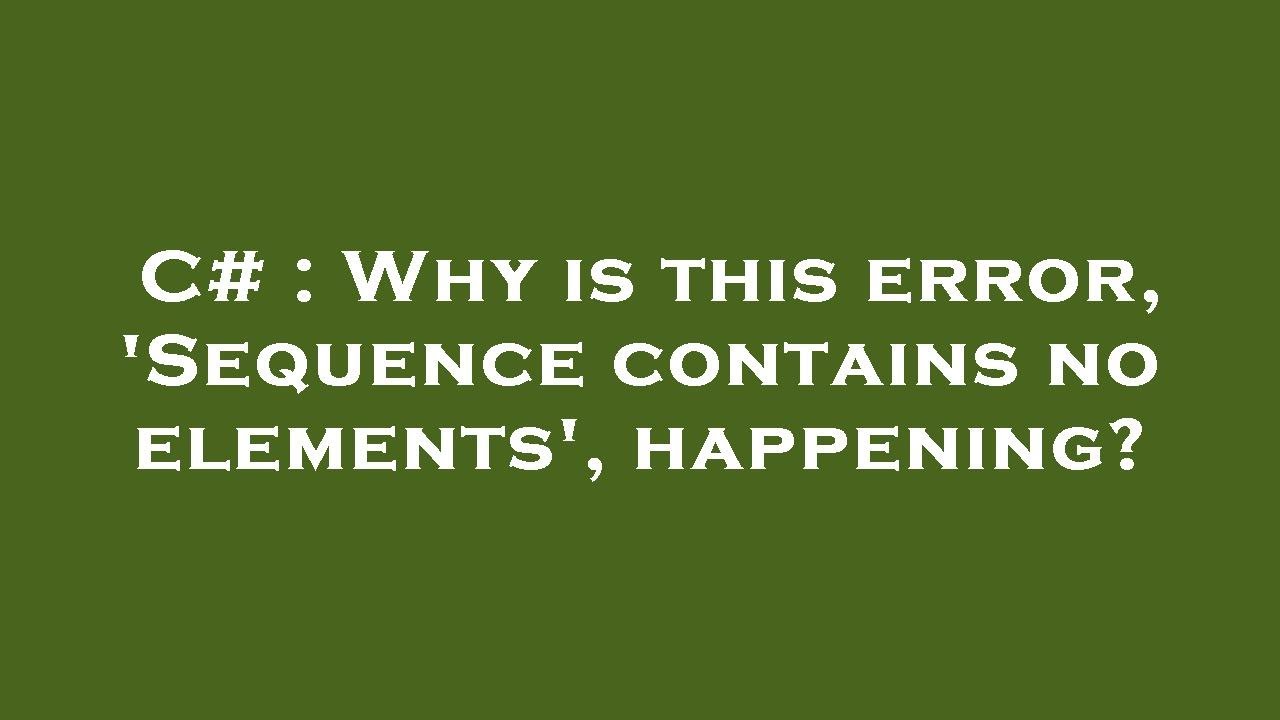
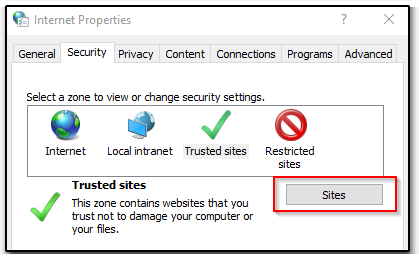
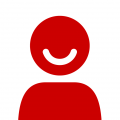




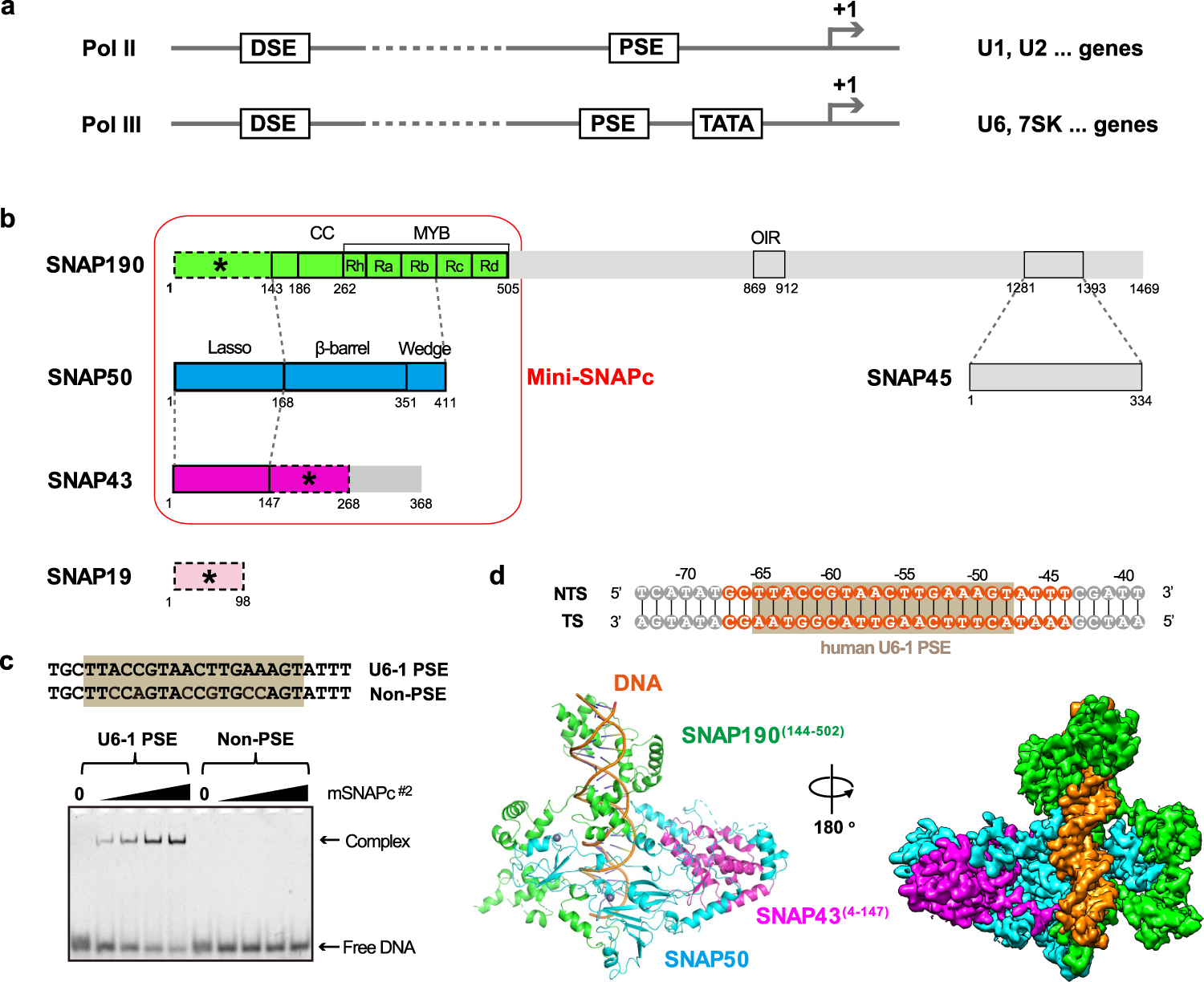
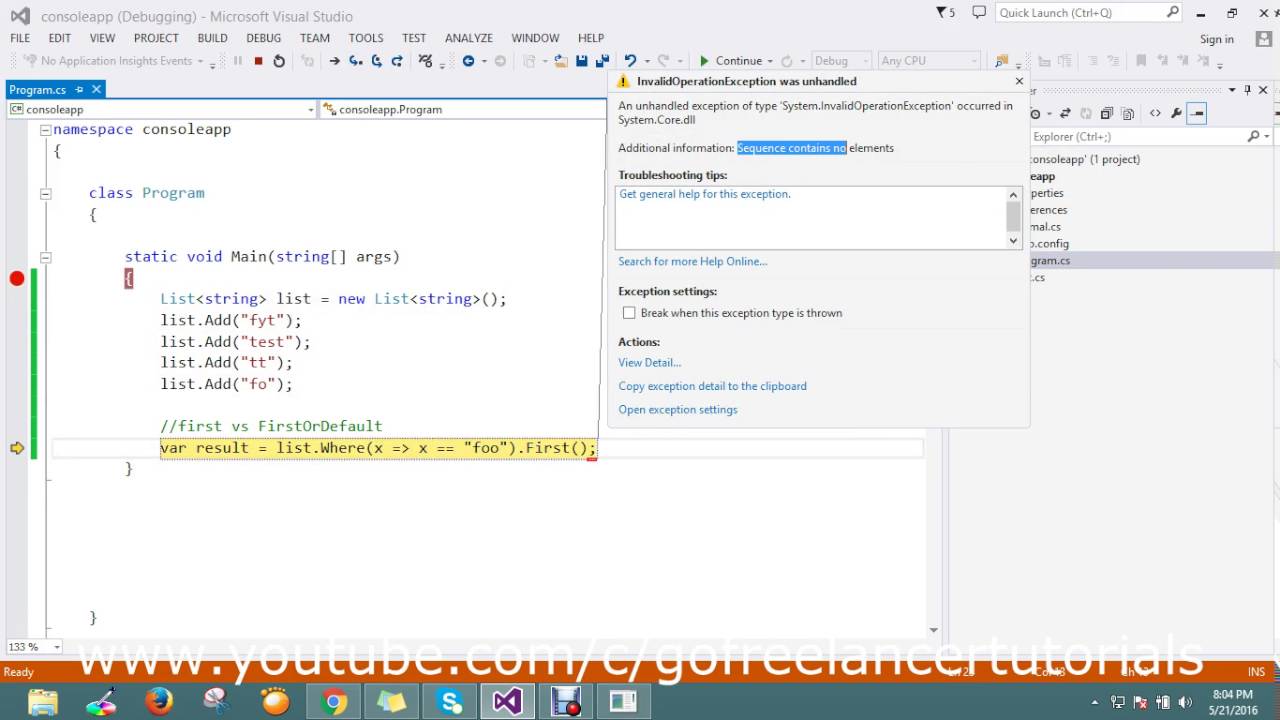

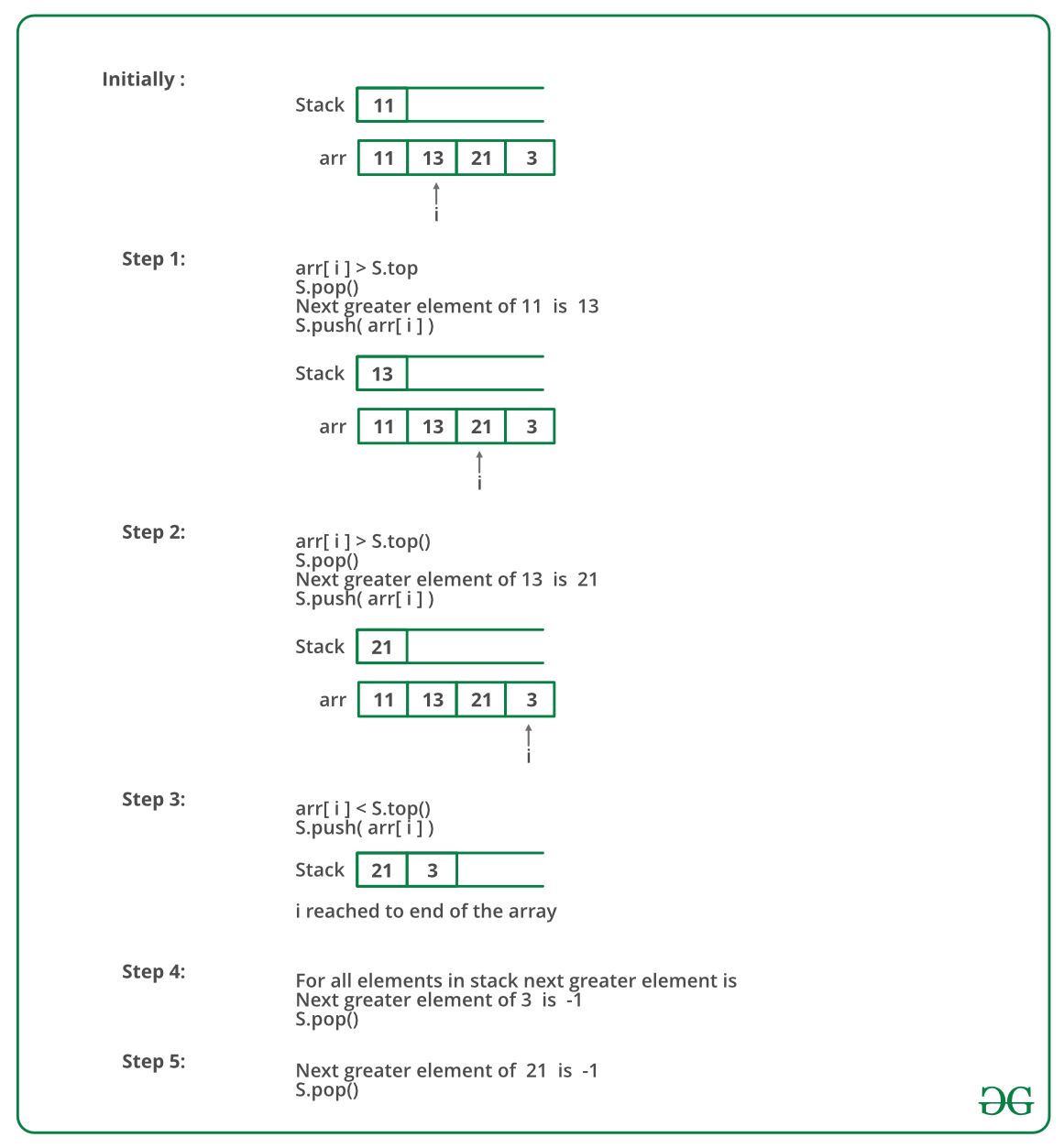
Article link: sequence contains no elements.
Learn more about the topic sequence contains no elements.
- Sequence contains no elements? – Stack Overflow
- Sequence Contains No Elements: How To Solve the C# Error
- Lỗi Select: Sequence Contains No Elements ASP.NET MVC …
- “Sequence contains no elements” error when trying to … – IBM
- “System.InvalidOperationException: Sequence contains no …
- [Solved] Sequence contains no elements(system …
- Getting error “Sequence contains no elements”
- Sequence contains no elements 7.0.0-preview.5.22302.2
See more: https://nhanvietluanvan.com/luat-hoc/