Ruby Write To File
Ruby provides powerful functionality for reading and writing files. In this article, we will explore how to write to a file in Ruby, including opening a file, different writing methods, appending to an existing file, creating a new file, writing variable and string data, writing data from arrays or hashes, and closing the file properly. Additionally, we will cover some frequently asked questions related to file handling in Ruby.
1. Opening a File in Ruby:
The ‘File’ class in Ruby provides various methods for file manipulation. When opening a file, we need to specify the mode as read, write, or append. The modes are represented by ‘r’, ‘w’, and ‘a’ respectively. Here is the syntax for opening a file using the ‘File’ class:
file = File.open(“filename”, “mode”)
2. Writing to a File in Ruby:
Ruby offers different methods for writing data to a file. The most commonly used methods are ‘write’, ‘puts’, and ‘print’. Each method has its own characteristics:
– ‘write’: This method writes a string to a file without a line break.
– ‘puts’: This method writes a string to a file with a line break at the end.
– ‘print’: This method writes a string to a file without a line break, similar to ‘write’.
Let’s take a look at some examples:
file = File.open(“filename.txt”, “w”)
file.write(“Hello, World!”)
file.puts(“This is a new line”)
file.print(“This is on the same line”)
file.puts(“Another line”)
This will write the following content to “filename.txt”:
Hello, World!
This is a new line
This is on the same line
Another line
3. Appending to an Existing File:
To append data to an existing file, we need to open the file in append mode. Append mode is denoted by ‘a’ instead of ‘w’ when opening the file. Here is an example:
file = File.open(“filename.txt”, “a”)
file.puts(“This line will be appended to the existing file”)
This will add the line “This line will be appended to the existing file” to the end of “filename.txt”, without overwriting the existing content.
4. Creating a New File:
To create a new file, we can use the ‘w’ mode when opening the file. If the file already exists, using ‘w’ mode will overwrite its content. To handle this scenario, we can check if the file exists before creating it. Here’s an example:
if File.exist?(“filename.txt”)
puts “File already exists!”
else
file = File.open(“filename.txt”, “w”)
file.puts(“This is a new file”)
end
This code will create a new file “filename.txt” with the line “This is a new file” if it doesn’t exist. Otherwise, it will display the message “File already exists!”.
5. Writing Variable and String Data:
To write variable data to a file, we need to convert the variable to a string using the ‘to_s’ method. We should also be careful with special characters in the data. Here’s an example:
name = “John Doe”
age = 25
file = File.open(“data.txt”, “w”)
file.puts(“Name: ” + name.to_s)
file.puts(“Age: ” + age.to_s)
This will write the following content to “data.txt”:
Name: John Doe
Age: 25
6. Writing Data from Arrays or Hashes:
In addition to writing variables, we can also write array or hash data to a file. Various methods, like ‘join’ for arrays and ‘to_json’ for hashes, can be used to format the data properly. Let’s see an example:
fruits = [“apple”, “banana”, “orange”]
prices = { apple: 1.99, banana: 0.99, orange: 1.49 }
file = File.open(“grocery.txt”, “w”)
file.puts(“Fruits: ” + fruits.join(“, “))
file.puts(“Prices: ” + prices.to_json)
This will write the following content to “grocery.txt”:
Fruits: apple, banana, orange
Prices: {“apple”: 1.99, “banana”: 0.99, “orange”: 1.49}
7. Closing the File:
It is important to close the file after writing to free up system resources. We can manually close the file using the ‘close’ method:
file = File.open(“file.txt”, “w”)
# Perform writing operations
file.close
Alternatively, we can use the ‘File.open’ block, which automatically closes the file for us:
File.open(“file.txt”, “w”) do |file|
# Perform writing operations
end
FAQs:
Q1. How can I write to a file line by line in Ruby?
A1. To write to a file line by line, you can use the ‘puts’ method with each line as an argument. Example:
file = File.open(“file.txt”, “w”)
file.puts(“Line 1”)
file.puts(“Line 2”)
file.close
Q2. How can I append to a file in Ruby?
A2. To append data to an existing file, open the file in append mode by using the ‘a’ mode when opening the file. Example:
file = File.open(“file.txt”, “a”)
file.puts(“This line will be appended”)
file.close
Q3. How can I read a file in Ruby?
A3. To read a file in Ruby, use the ‘File.open’ method with the ‘r’ mode and then use methods like ‘read’ or ‘readlines’ to retrieve the content. Example:
file = File.open(“file.txt”, “r”)
content = file.read
file.close
Q4. How can I load a JSON file in Ruby?
A4. To load a JSON file in Ruby, require the ‘json’ library and then use the ‘File.read’ method to read the JSON file. Example:
require ‘json’
data = JSON.parse(File.read(“data.json”))
Q5. How can I delete a file in Rails using Ruby?
A5. In Rails, we can use the ‘File.delete’ method to delete a file. Example:
File.delete(“file.txt”)
In conclusion, writing to a file in Ruby is a fundamental skill that every developer should possess. By understanding the different modes for opening a file, various writing methods, appending to an existing file, creating a new file, writing variable and string data, writing data from arrays or hashes, and closing the file properly, you can efficiently handle file manipulation in your Ruby projects.
Ruby Lesson 12 : Read And Write Into A File In Ruby: Part_1
Keywords searched by users: ruby write to file Ruby write to file line by line, Ruby append to file, Rails file, Read file Ruby, Ruby load json file, Rails file open mode, ruby file write append, Delete file rails
Categories: Top 41 Ruby Write To File
See more here: nhanvietluanvan.com
Ruby Write To File Line By Line
Writing to a file line by line can be essential in situations where data needs to be stored or processed incrementally. This approach allows us to append new information without overwriting the existing content, making it suitable for numerous scenarios, such as logging systems or collecting user input data.
In Ruby, there are multiple ways to achieve this functionality. One common approach involves using the `File` class and its associated methods. To demonstrate this, let’s consider a simple example where we want to write a list of names to a file called “names.txt.” We can follow these steps:
Step 1: Open the file for writing
“`ruby
file = File.open(“names.txt”, “w”)
“`
In this step, we use the `File.open` method to open the “names.txt” file in write mode (“w”). This mode allows us to overwrite any existing data in the file. If the file does not exist, Ruby will create one for us.
Step 2: Write each name on a new line
“`ruby
names = [“John”, “Jane”, “James”]
names.each do |name|
file.puts name
end
“`
In this step, we iterate over an array of names and use the `puts` method to write each name to a new line in the file. The `puts` method adds a newline character after each name, ensuring that they are written on separate lines.
Step 3: Close the file
“`ruby
file.close
“`
Finally, we close the file using the `close` method. This step is crucial as it frees up system resources and ensures that all data is written to the file.
By following these steps, we have successfully written the list of names to the “names.txt” file line by line. We can verify this by opening the file and observing its contents.
While the above method is straightforward, Ruby provides alternative means to achieve the same result. For instance, we can use the `File.write` method to simplify the process. Let’s modify our previous example to illustrate this approach:
“`ruby
names = [“John”, “Jane”, “James”]
File.write(“names.txt”, names.join(“\n”))
“`
In this case, instead of opening and closing the file explicitly, we directly call the `File.write` method, which automatically handles these operations in a single line. We pass the name of the file as the first argument and use the `join(“\n”)` method to concatenate the names together, separated by newline characters.
Now that we’ve explored the basics of writing files line by line in Ruby, let’s address some frequently asked questions about this topic:
Q: Can I append new data to an existing file without overwriting the original content?
A: Yes, Ruby provides a mode called “append” (“a”) that allows you to append data to an existing file. You can replace the “w” mode with “a” in the `File.open` method to accomplish this.
Q: How can I ensure that my data is written to the file immediately, without waiting for the file to be closed?
A: Ruby buffers file operations for efficiency, meaning that data is not always written directly to the file with each operation. To force immediate writing, you can use the `flush` method after each write operation or open the file in unbuffered mode (“w:unbuffered”).
Q: Is it possible to write data to a file in a specific encoding?
A: Yes, you can specify the encoding when opening the file by adding a string parameter to the `File.open` method. For example, to write in UTF-8 encoding, you can use `File.open(“file.txt”, “w:utf-8”)`.
Q: Are there any performance considerations when writing files line by line in Ruby?
A: Efficiently writing large amounts of data can be achieved by batching the write operations using methods like `write` instead of `puts` or using buffered I/O routines. If performance is critical, consider leveraging libraries like `FileUtils` or exploring lower-level IO operations.
In conclusion, writing to a file line by line is a common programming task that can be accomplished using a variety of methods in Ruby. Whether you choose to use the `File` class or the simplified `File.write` method, understanding the underlying concepts and functionalities will enable you to effectively handle your file writing requirements. Remember to consider the specific needs of your application and leverage the available options and techniques accordingly. Happy coding!
Ruby Append To File
Appending data to a file in Ruby can be done in several ways. One method is by using the `File` class, which provides various methods for working with files. One popular method is `File.open`, which allows you to open a file and perform actions on it, such as appending data. Here’s an example:
“`ruby
File.open(‘filename.txt’, ‘a’) do |file|
file.puts ‘This is a new line appended to the file.’
end
“`
In the code snippet above, we open the file named `filename.txt` in append mode by passing `’a’` as the second parameter to `File.open`. The `’a’` mode opens the file for writing at the end, and if the file doesn’t exist, it will be created. Then, we use the `file.puts` method to append the desired data to the file.
Another approach to append data to a file is by using the `IO` class methods. The `IO.write` method allows you to open a file in append mode and write to it. Here’s an example:
“`ruby
IO.write(‘filename.txt’, “This is a new line appended to the file.\n”, mode: ‘a’)
“`
In this example, the `IO.write` method is used to append data to the file named `filename.txt`. The `mode: ‘a’` option specifies the append mode. Additionally, we include a newline character at the end of the string to ensure that each appended line starts on a new line.
Besides the two methods mentioned above, Ruby also provides the `File` class method `File.write` that simplifies the process of appending data to a file. It automatically opens the file in append mode, writes the data, and then closes the file. Here’s an example:
“`ruby
File.write(‘filename.txt’, “This is a new line appended to the file.\n”, mode: ‘a’)
“`
As you can see, `File.write` streamlines the process by handling the file operations internally.
Now that we have covered the basics of appending data to a file in Ruby, let’s address some commonly asked questions regarding this topic:
### FAQs
**Q: Can I append multiple lines of data to a file in a single operation?**
A: Yes, you can append multiple lines of data by providing a string with newline characters (`\n`) for each line. For example:
“`ruby
data = “Line 1\nLine 2\nLine 3”
File.write(‘filename.txt’, data, mode: ‘a’)
“`
This will append three separate lines to the file `filename.txt`.
**Q: What happens if the file doesn’t exist when I attempt to append data?**
A: If the file specified doesn’t exist, Ruby will create a new file with the provided name and append the data to it. However, if the file is in a directory that doesn’t have proper write permissions, an error will occur.
**Q: Is there a limit to the size of the file I can append data to?**
A: The ability to append data to a file in Ruby is limited by the available storage capacity of your system. As long as you have enough free disk space, you can append data to files of any size.
**Q: Are there any potential pitfalls or considerations when appending data to a file?**
A: It’s important to handle exceptions, such as when the disk is full or the file is read-only, to avoid unexpected crashes or errors in your application. Additionally, be mindful of the file’s format and encoding to ensure that the appended data is written correctly.
**Q: Can I append data to a specific line in a file?**
A: No, appending data to a specific line in a file is not straightforward. The easiest approach is to read the content of the file, modify the desired line, and then write it back to the file. However, this is not considered a true append operation.
In conclusion, appending data to a file in Ruby is a relatively straightforward task thanks to the many built-in methods and techniques provided by the language. By using the `File` or `IO` class methods, developers can easily append data to a file without complex workarounds. Understanding these methods and their usage is crucial for effectively manipulating files in your Ruby applications. So go ahead and leverage Ruby’s file handling capabilities to create sophisticated file manipulation processes in your projects!
Rails File
Introduction:
Ruby on Rails (Rails) is a popular web development framework known for its ease of use, convention over configuration approach, and efficient file management capabilities. In this article, we will delve into the Rails file structure, explore various file types and their purposes, and highlight best practices for efficient file management. Additionally, we will address frequently asked questions (FAQs) regarding Rails file management.
Understanding the Rails File Structure:
The Rails framework follows a specific file structure that provides organization and helps developers locate and manage different components of their application. Here is a brief overview of the key directories and files in a typical Rails application:
1. app: This directory contains the core application code, including models, views, and controllers. The models directory holds the application’s data models, while the views directory contains the HTML templates rendered by controllers. The controllers directory houses the logic that connects the models and views.
2. config: This directory holds configuration files for the application. Notably, the database.yml file stores database connection details, routes.rb defines the application’s routes, and environment.rb sets up the application environment.
3. db: The db directory stores database-related files, such as schema.rb (schema definition file), migrations (versioned files for modifying the database structure), and seeds.rb (file for populating initial data).
4. public: This directory stores static files, such as images, stylesheets, and JavaScript files. These files are accessible directly by users via the application’s URL.
5. test: The test directory contains test cases and fixtures used for automated testing of the application. Rails advocates for testing to ensure application stability and quality.
6. Gemfile and Gemfile.lock: These files, located in the application’s root directory, specify the application’s dependencies (gems). They are essential for package management and ensuring consistent environments across development teams.
Types of Files in Rails:
1. Models: Models represent the application’s data structure and encapsulate logic related to data manipulation and validation. They typically reside in the app/models directory and have a corresponding database table. Rails uses an Object-Relational Mapping (ORM) called ActiveRecord to simplify database interactions.
2. Views: Views contain the presentation logic of an application and are responsible for generating the HTML sent to the user’s browser. Views are stored in the app/views directory and typically have the “.html.erb” extension, indicating a mix of HTML and embedded Ruby code.
3. Controllers: Controllers handle user requests and communicate with models and views. They gather data from models and pass it to the corresponding views, responding to user actions. Controllers are located in the app/controllers directory and are typically named after the resource they manage (e.g., UsersController).
4. Migrations: Migrations are versioned files that enable seamless modification of the database structure. They can create, modify, or delete database tables, add columns, or alter the schema. Migrations are stored in the db/migrate directory and are executed in order to reflect changes in the database.
5. Routes: Routes connect incoming HTTP requests to the appropriate controller actions. They define the endpoints, HTTP verbs, and URL patterns for handling user requests. Routes are specified in the config/routes.rb file using a concise domain-specific language.
6. Assets: Assets comprise static files, such as images, stylesheets, and JavaScript files, utilized by the application. They are stored in the app/assets directory and can be further categorized into subdirectories (e.g., app/assets/images, app/assets/stylesheets).
Rails File Management Best Practices:
1. Follow the “convention over configuration” principle: Rails encourages developers to follow naming conventions and adhere to the default file structure. This approach minimizes configuration and enables seamless collaboration within a Rails project.
2. Separate concerns: Ensure each file has a clear and specific purpose. Follow the “Single Responsibility Principle” by assigning distinct responsibilities to models, views, and controllers. Avoid putting excessive logic in views; instead, keep them focused on presentation.
3. Leverage assets pipeline: Rails provides an assets pipeline that handles the compilation, minification, and organization of assets. Utilize this pipeline to manage assets efficiently and improve application performance.
4. Apply proper database migrations: When making structural changes to your database, create and apply migrations to ensure consistency across environments and simplify collaborative development.
5. Employ version control: Use a version control system like Git to track changes in your application’s files. This allows for easy collaboration, reverting to previous versions, and managing conflicts between team members.
FAQs:
Q1: Can I change the default file structure in Rails?
A: While it’s possible to deviate from the default Rails file structure, it’s generally recommended to follow the conventions to ensure smooth collaboration and ease of use for developers new to the project.
Q2: How can I upload and process files in Rails?
A: Rails provides various file uploading libraries, such as CarrierWave and Active Storage, to handle file attachments. These libraries simplify the process of uploading, processing, and associating files with models.
Q3: How can I optimize asset loading in production?
A: To optimize asset loading, Rails offers features like asset fingerprinting, concatenation, and minification. These techniques combine and compress assets for efficient delivery, reducing network requests and improving page load times.
Q4: Can I use a different database management system with Rails?
A: Yes, Rails supports multiple database management systems (DBMS) including MySQL, PostgreSQL, SQLite, and Oracle. Simply update the database.yml configuration file to specify the desired DBMS.
Conclusion:
Efficient file management is crucial for organizing and maintaining a Rails application. By following Rails conventions, leveraging the appropriate file types, and understanding best practices, developers can achieve a well-structured and maintainable codebase. The Rails framework, with its comprehensive file structure and built-in tools, provides an excellent foundation for effective file management in Ruby on Rails projects.
Images related to the topic ruby write to file
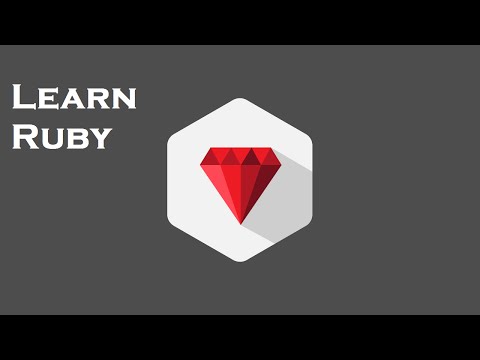
Found 34 images related to ruby write to file theme
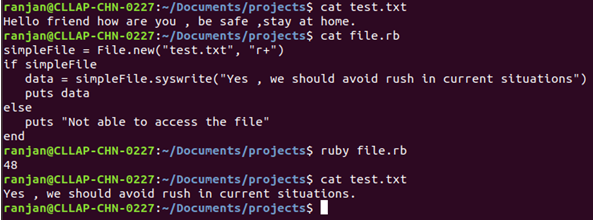
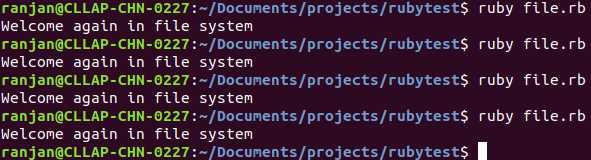
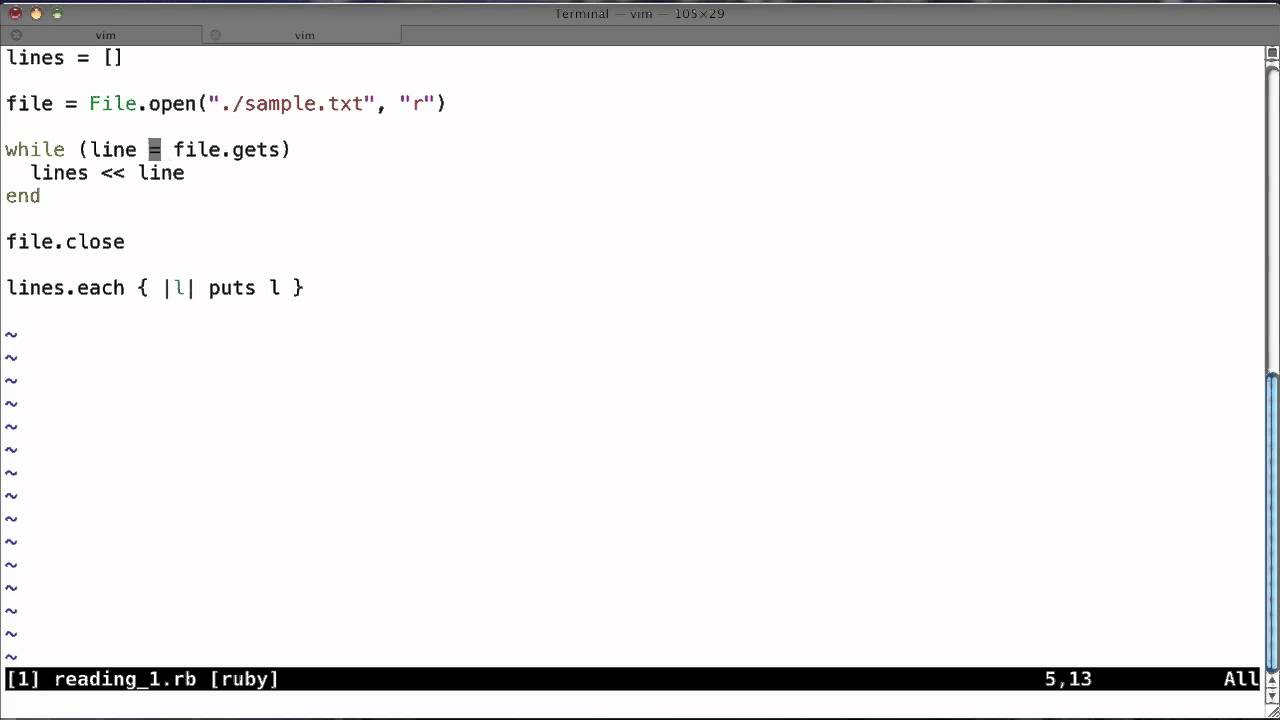
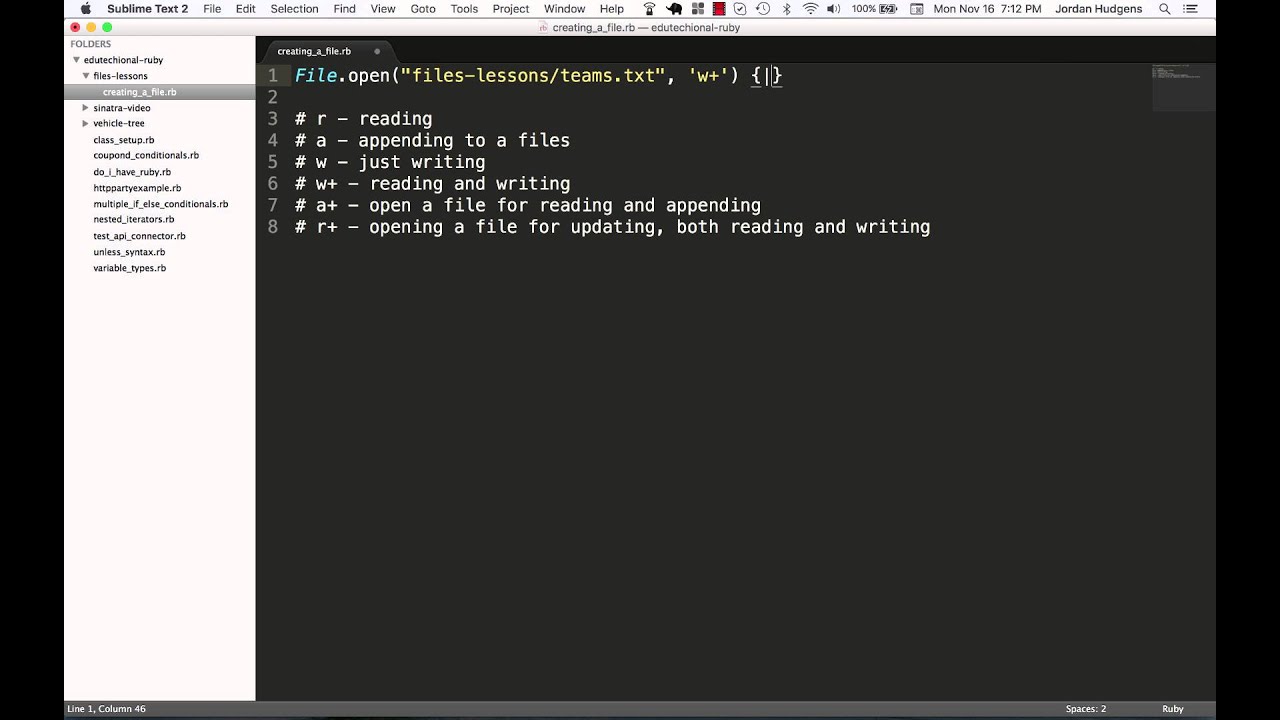

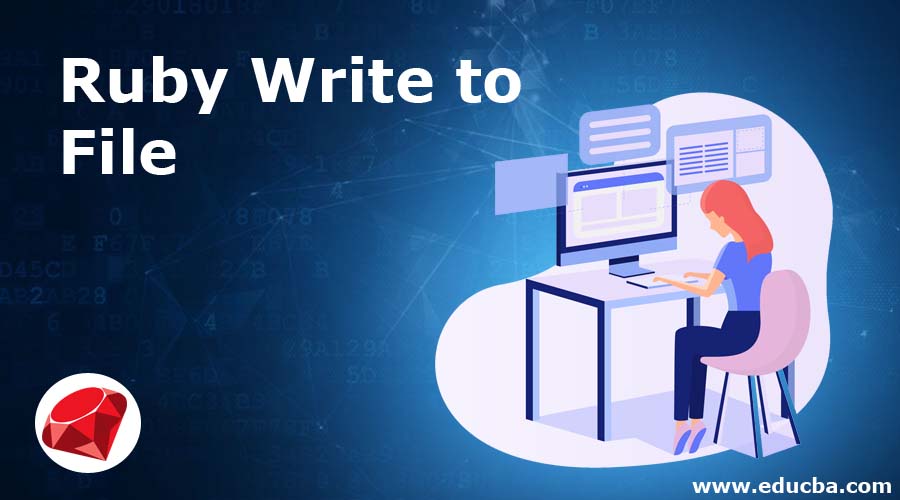

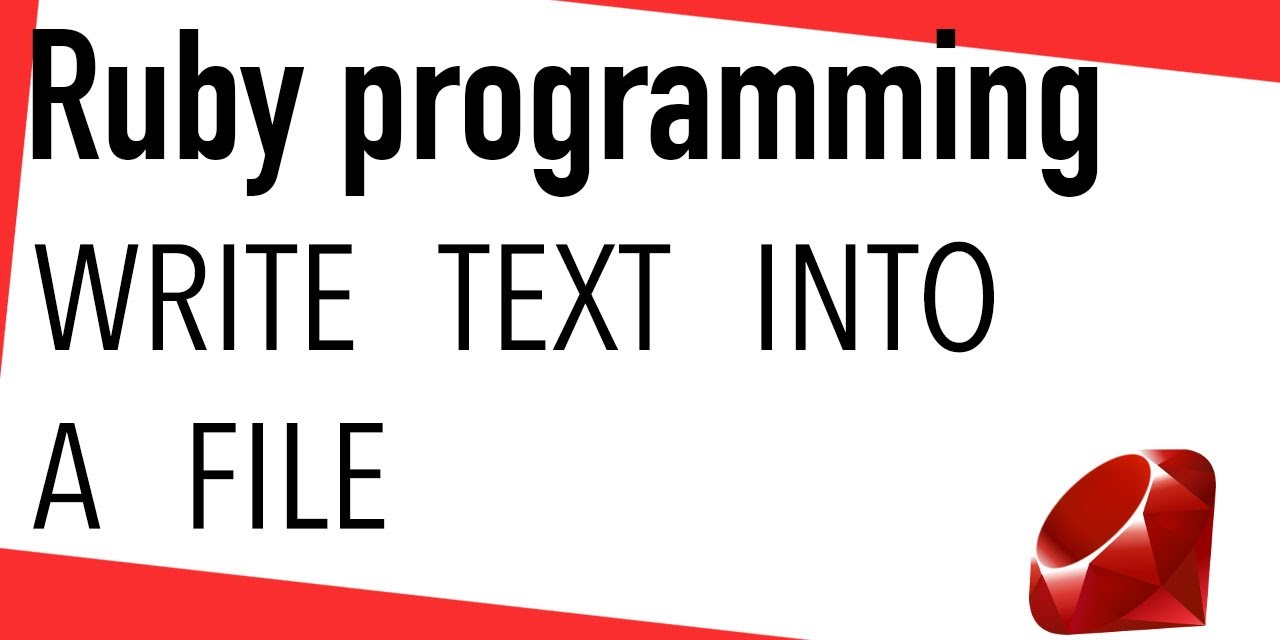
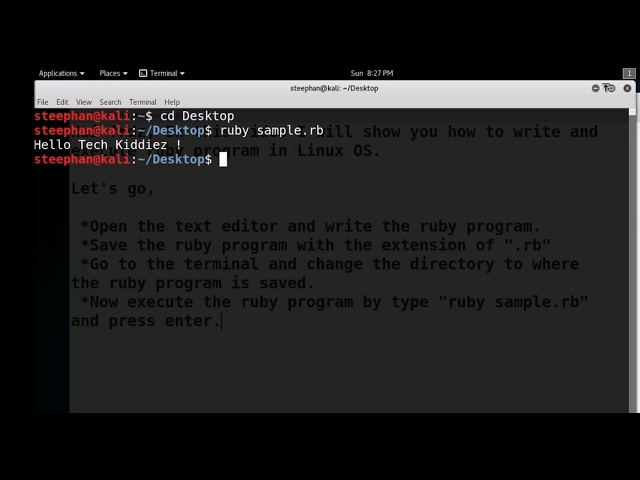
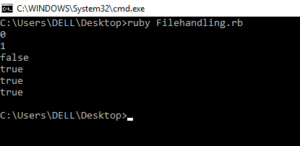
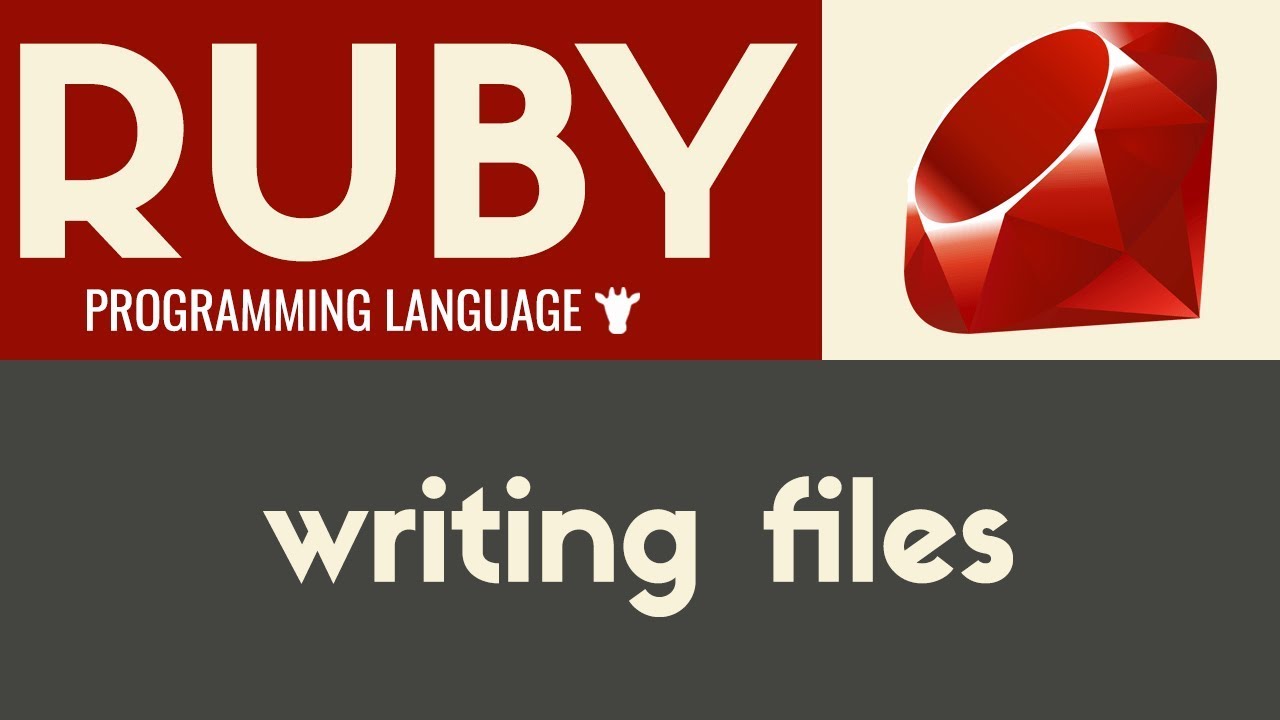
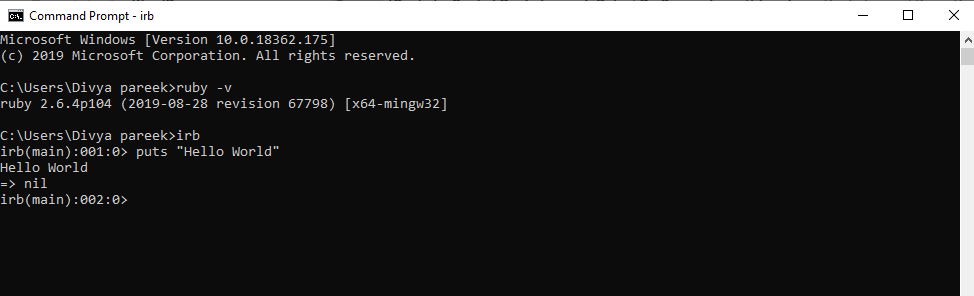
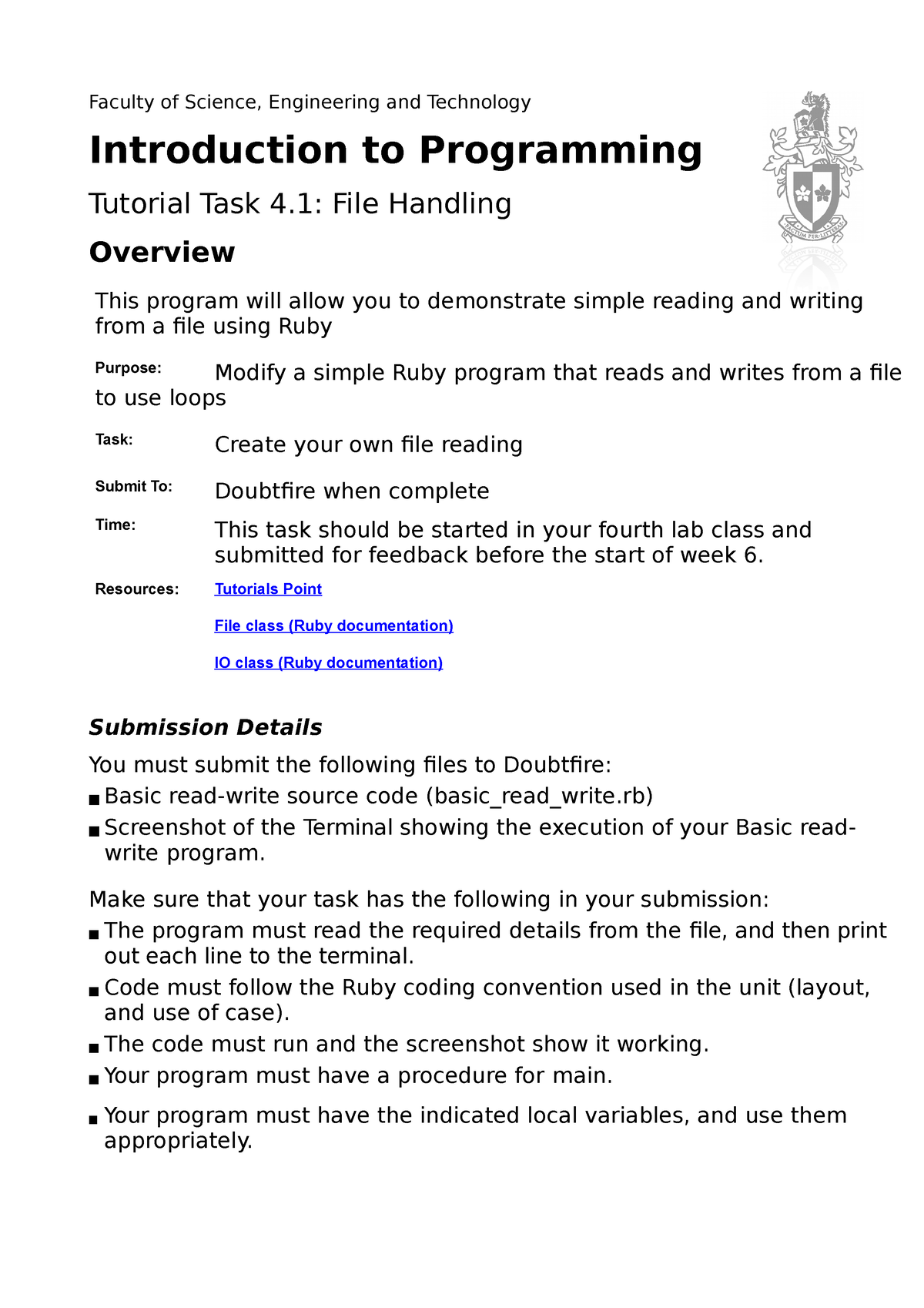
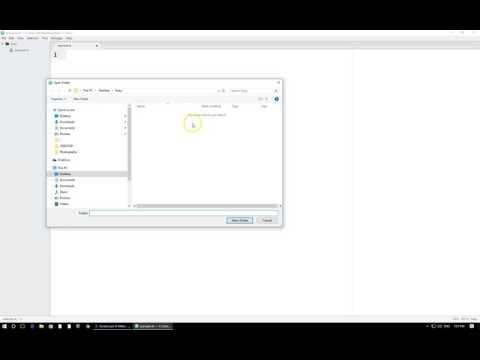

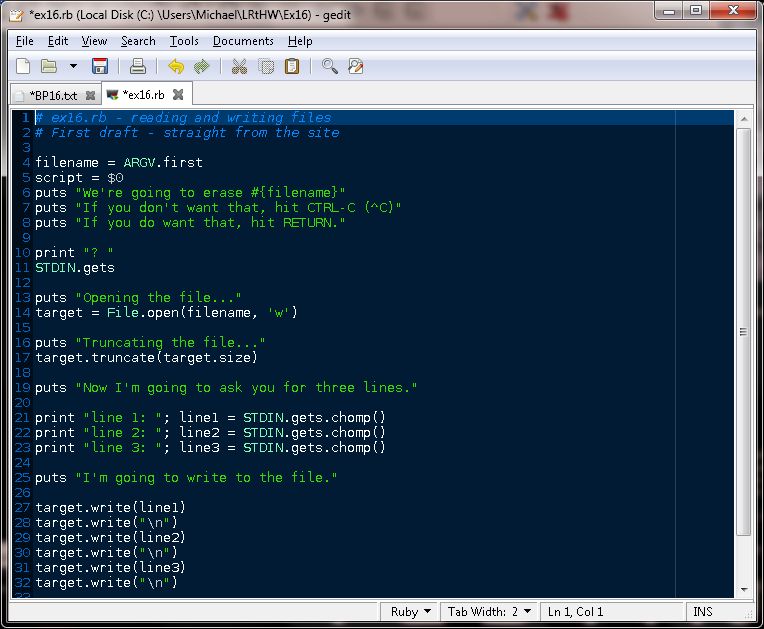
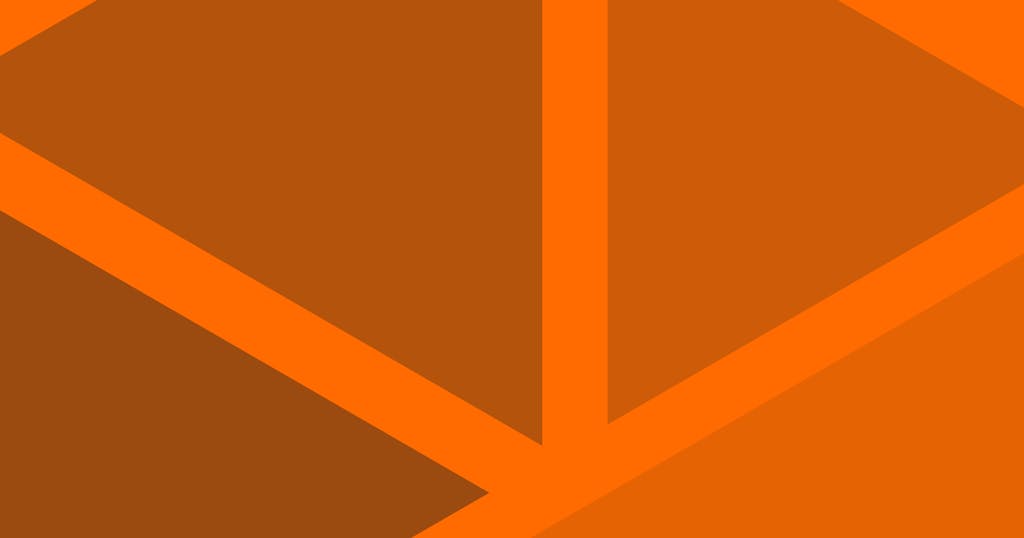


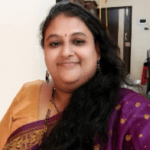
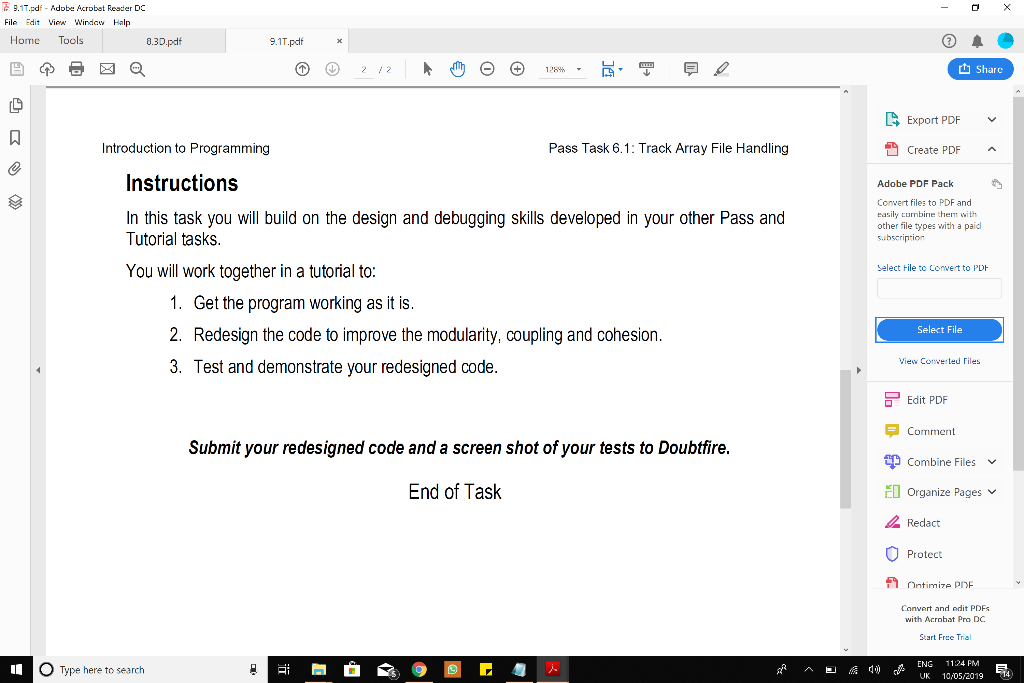


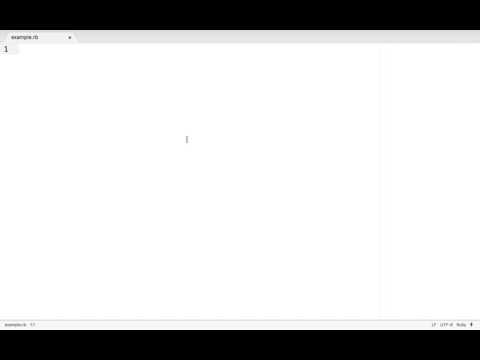


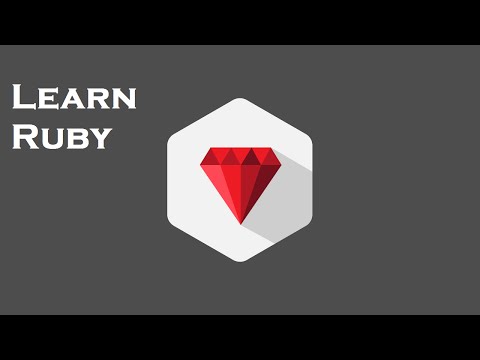


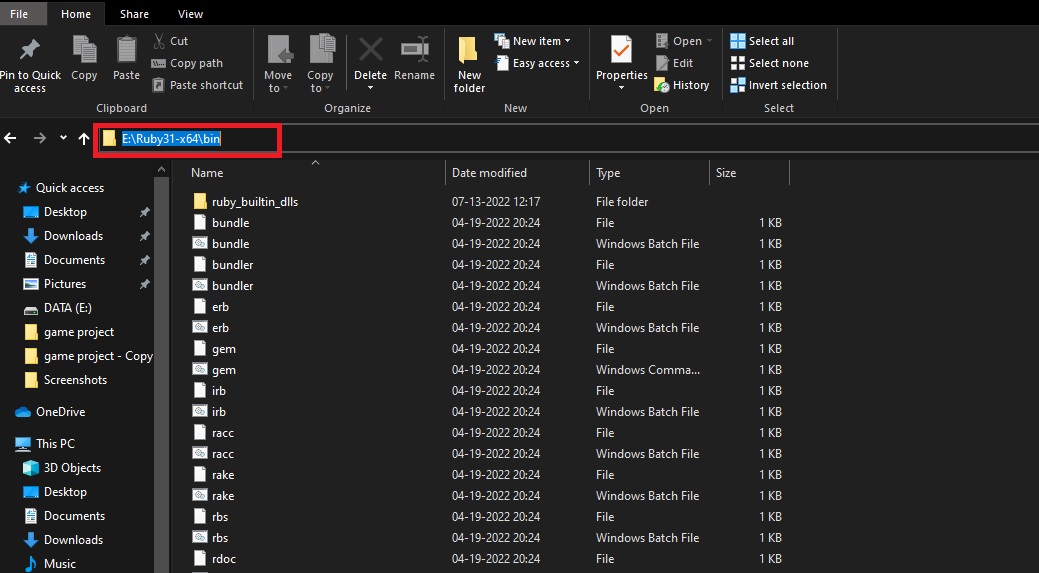
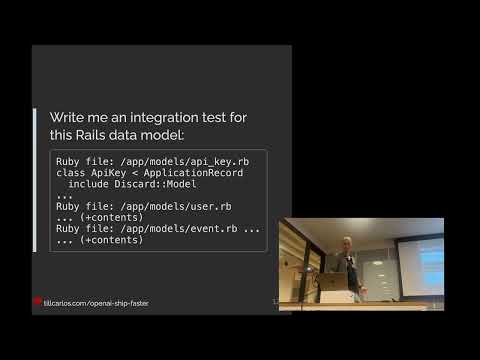
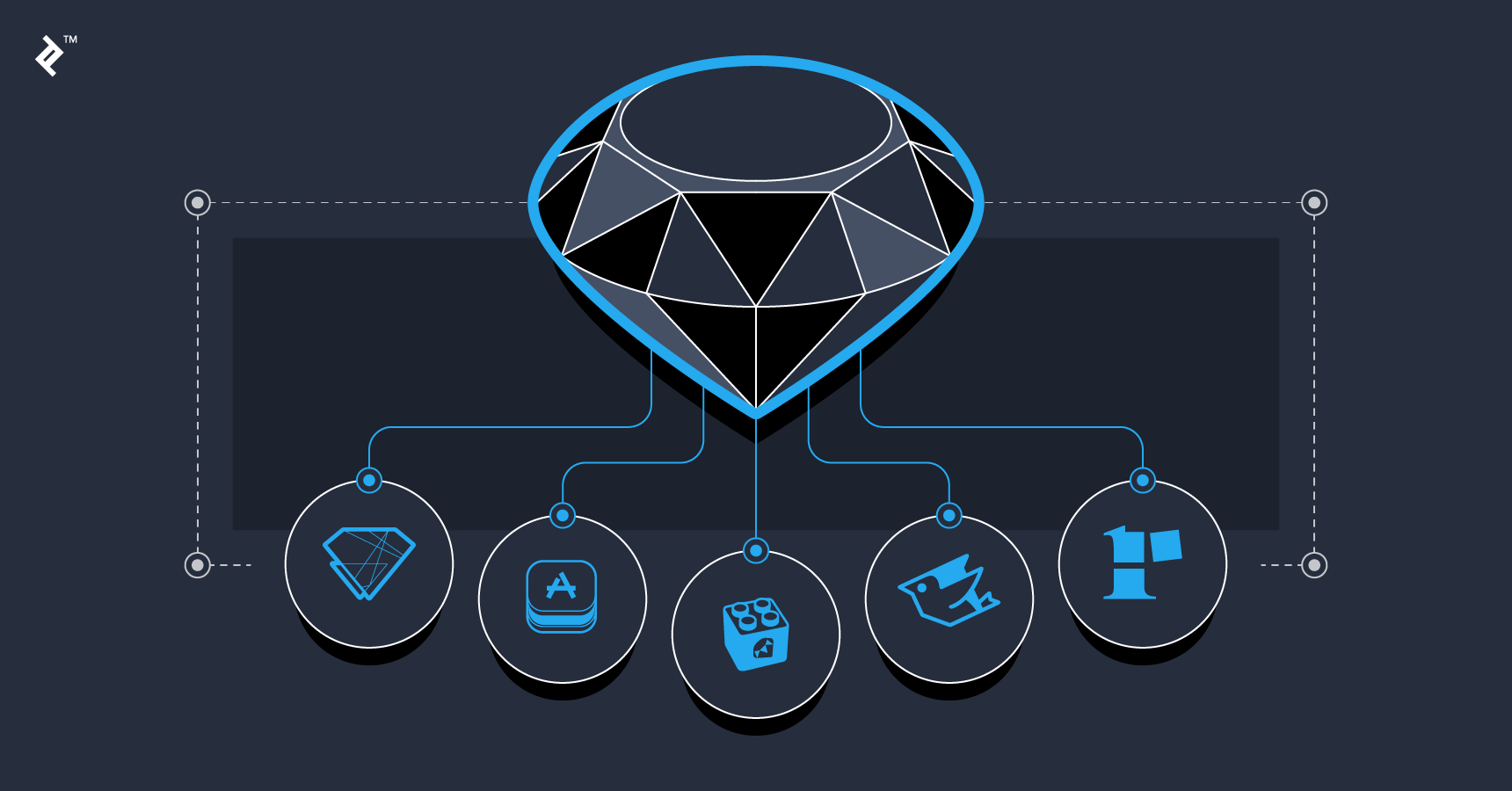
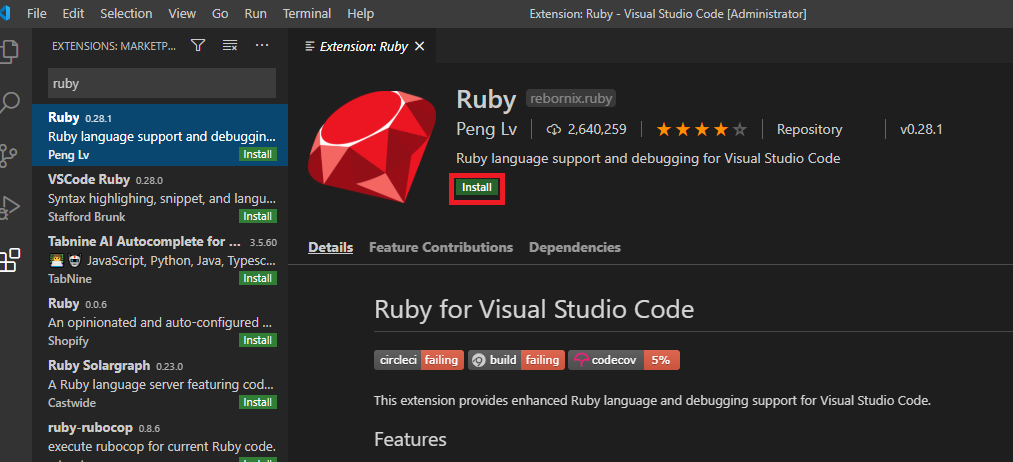
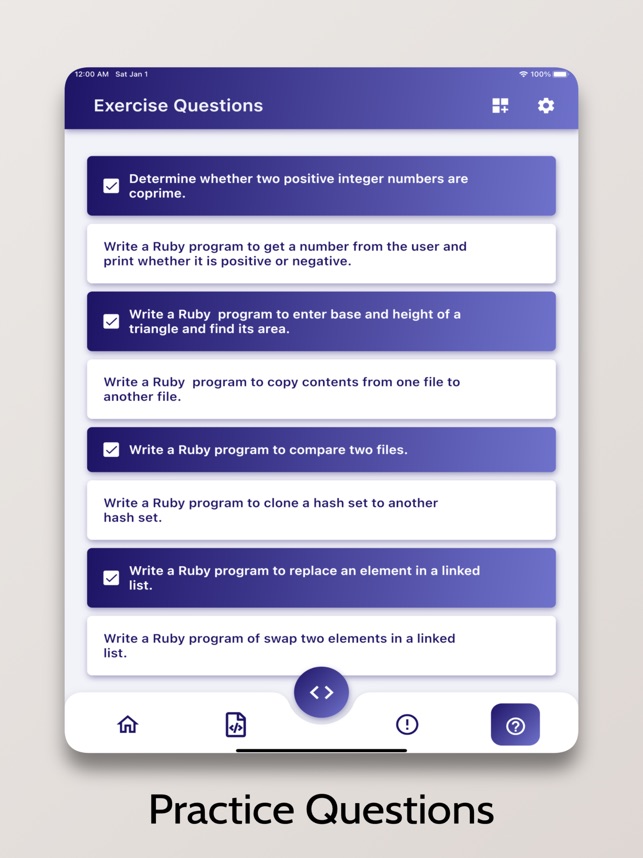
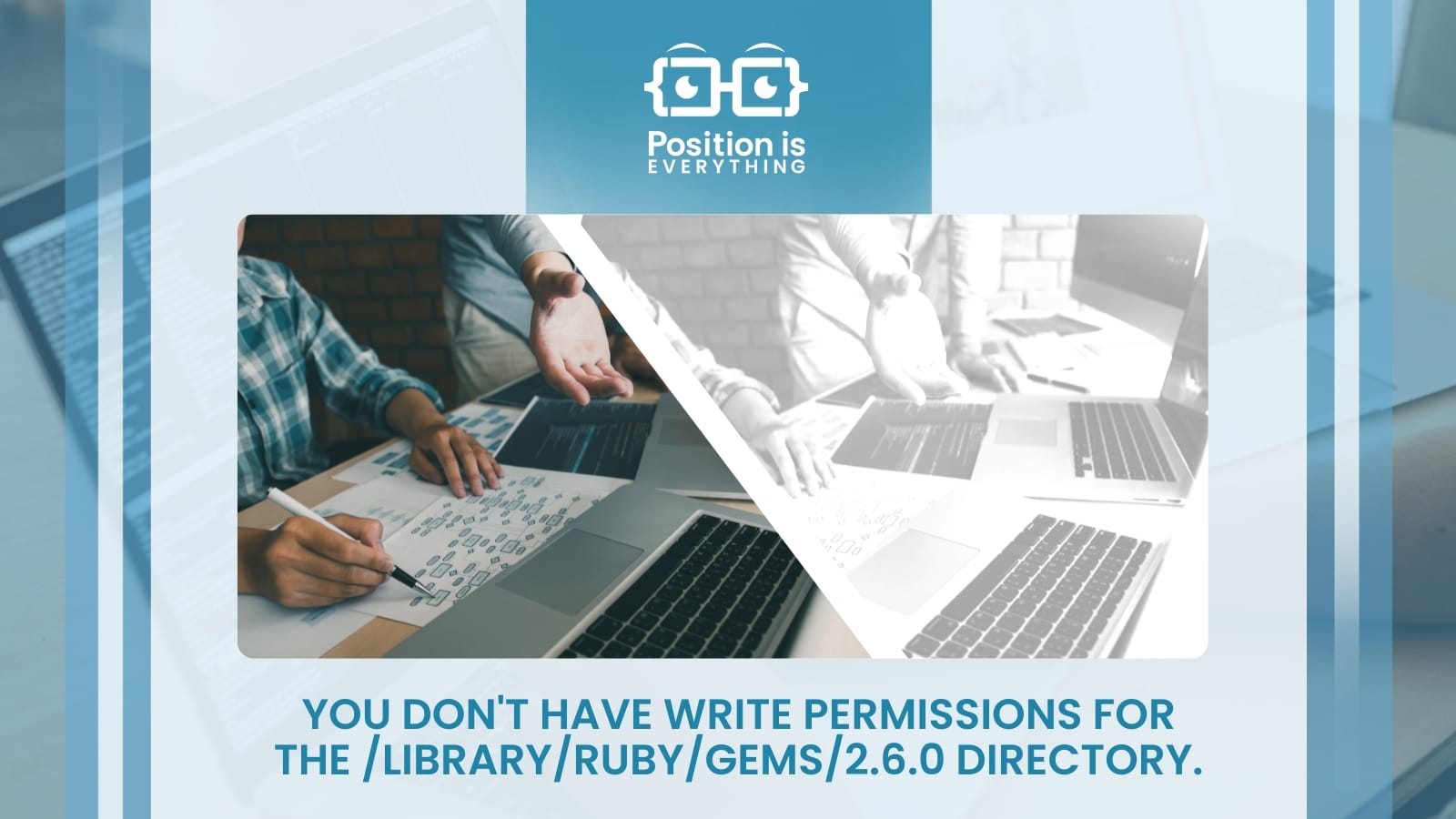

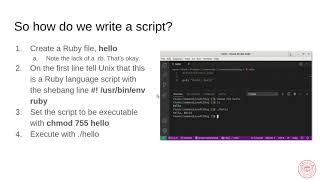
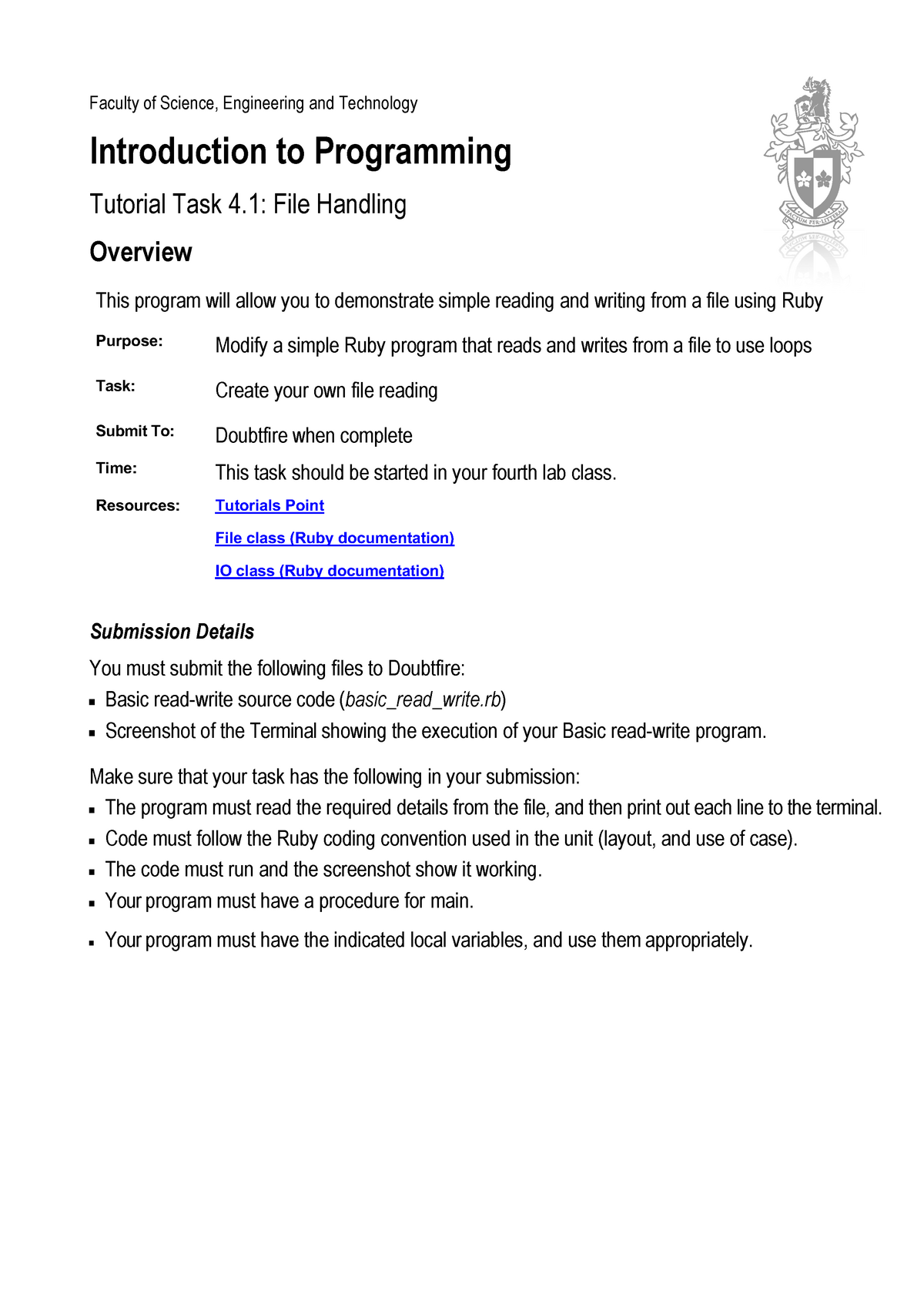
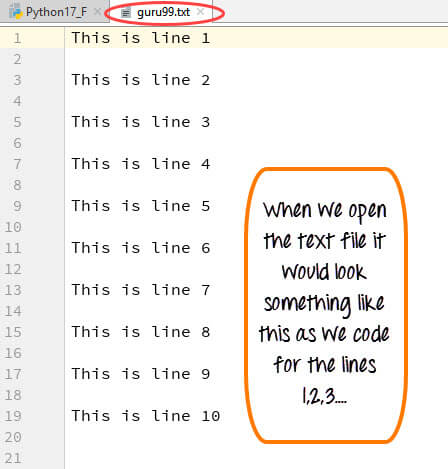

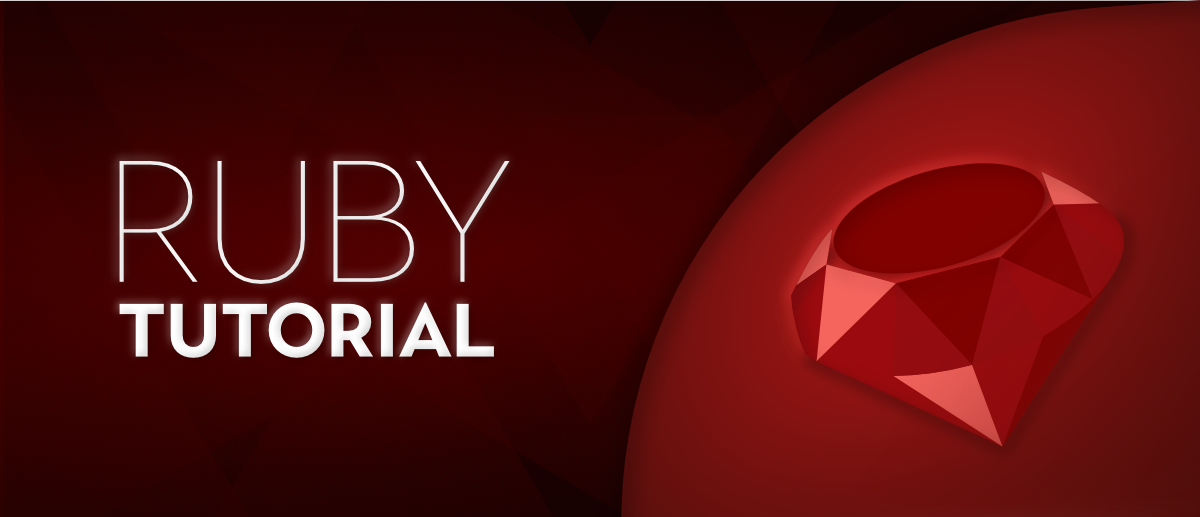

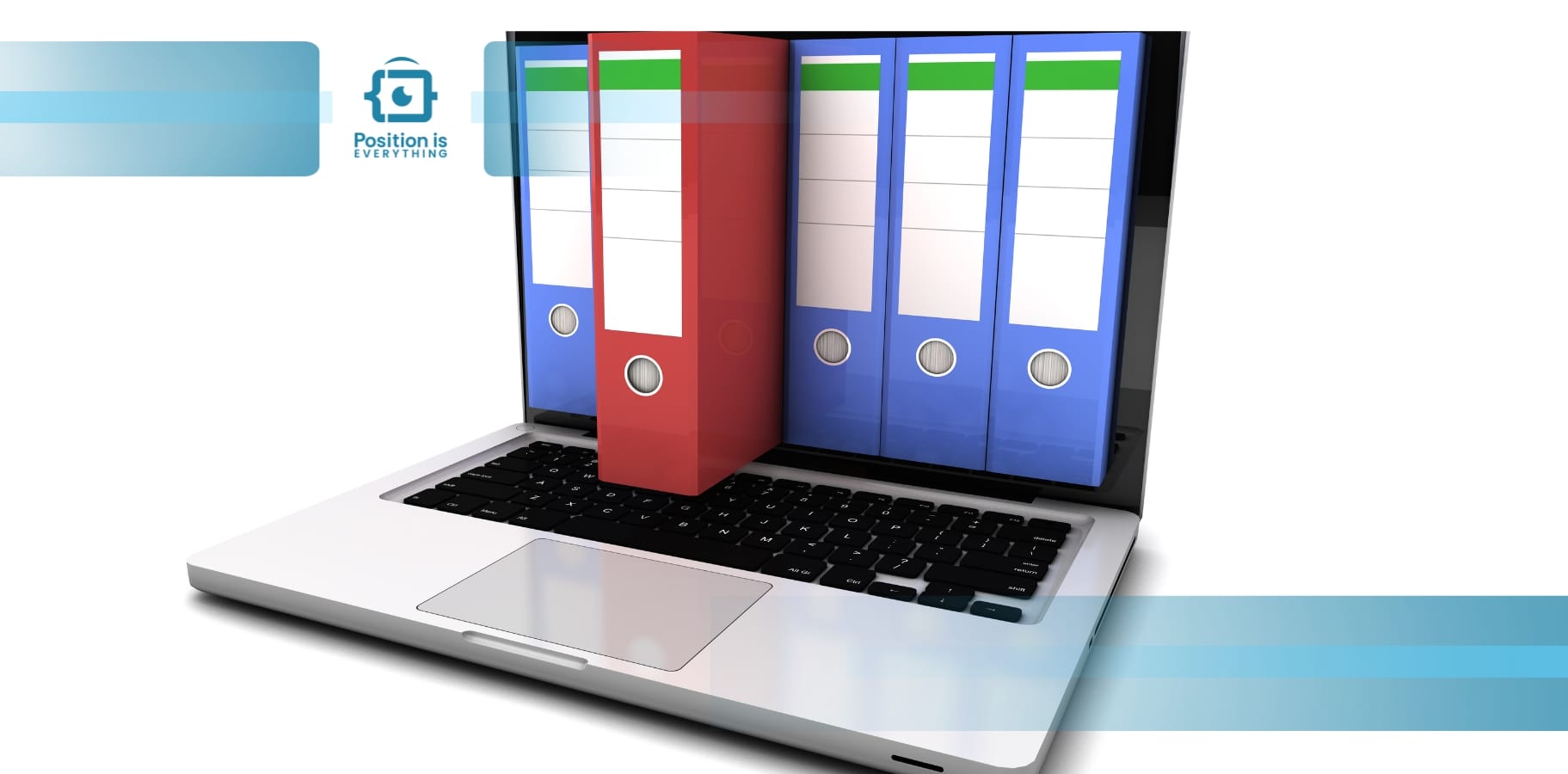

Article link: ruby write to file.
Learn more about the topic ruby write to file.
- How to write to file in Ruby? – Stack Overflow
- How To Read & Write Files in Ruby (With Examples)
- Write to File in Ruby Using Various Methods – eduCBA
- Write to a File in Ruby – Delft Stack
- A Ruby write to file example | alvinalexander.com
- How to Read and Writes Files in Ruby – Tutorialspoint
- Ruby Language Tutorial => Writing a string to a file
- How To Read & Write Files in Ruby | by Javier Goncalves
- Write to File in Ruby – SyntaxDB – Ruby Syntax Reference
See more: https://nhanvietluanvan.com/luat-hoc/