Remove First Character From String Python
Subheading 1: Overview of removing the first character from a string in Python
Removing the first character from a string is a common task in Python programming. Whether you need to clean up the data you’re working with or manipulate the string for further processing, there are several methods you can use to achieve this. This article will explore different approaches to remove the first character from a string in Python, including using string slicing, the replace() function, regular expressions, and more.
Subheading 2: Using string slicing to remove the first character
One way to remove the first character from a string in Python is by using string slicing. String slicing allows you to extract a portion of a string by specifying the starting and ending indices. To remove the first character, you can simply slice the string from the second character onwards.
For example, consider the following code snippet:
“`python
string = “Hello World”
new_string = string[1:]
print(new_string)
“`
Output: “ello World”
In this example, the string slicing operation `string[1:]` returns a new string that starts from the second character (index 1) and goes until the end of the string. As a result, the first character “H” is removed from the original string.
Subheading 3: Understanding the string indexing in Python
To fully grasp how string slicing works in Python, it’s important to understand string indexing. In Python, strings are zero-indexed, meaning that the first character has an index of 0, the second character has an index of 1, and so on. Therefore, when using string slicing to remove the first character, you need to specify the starting index as 1.
Subheading 4: Utilizing the slice operator to remove the first character
In addition to using string slicing, you can also utilize the slice operator to remove the first character from a string in Python. The slice operator is denoted by the colon “:” and allows you to specify the starting and ending indices for the slice.
For example, consider the following code snippet:
“`python
string = “Hello World”
new_string = string[1:]
print(new_string)
“`
Output: “ello World”
In this example, the slice operator is used to remove the first character from the string. By specifying the starting index as 1 and omitting the ending index, the slice operator includes all characters from index 1 to the end of the string.
Subheading 5: Demonstrating the usage of slice operator with examples
Let’s explore a few more examples to understand the usage of the slice operator for removing the first character from a string in Python:
Example 1:
“`python
string = “Hello”
new_string = string[1:]
print(new_string)
“`
Output: “ello”
Example 2:
“`python
string = “Python”
new_string = string[1:]
print(new_string)
“`
Output: “ython”
Example 3:
“`python
string = “World”
new_string = string[1:]
print(new_string)
“`
Output: “orld”
In these examples, the slice operator is used to remove the first character from the given strings. The resulting new strings would only include the characters starting from the second position (index 1) till the end of the original strings.
Subheading 6: Using the replace() function to remove the first character
Another approach to remove the first character from a string in Python is by using the replace() function. The replace() function allows you to replace occurrences of a specific substring within a string with another substring. In this case, you can replace the first character with an empty string, effectively removing it from the original string.
For example, consider the following code snippet:
“`python
string = “Hello”
new_string = string.replace(string[0], “”, 1)
print(new_string)
“`
Output: “ello”
In this example, the replace() function is invoked on the string with the arguments being the first character to replace, the replacement string (empty in this case), and the count of replacements to perform (1, to replace only the first occurrence). The resulting new string, obtained by removing the first character, is then printed.
Subheading 7: Exploring the advantages and limitations of using replace()
Using the replace() function to remove the first character from a string can be useful in some scenarios. However, it’s important to be aware of its limitations. The replace() function performs a sequential search for every occurrence of the substring to replace, which can be inefficient when dealing with large strings or multiple replacements. Additionally, it may not work as expected if the original string contains multiple occurrences of the character to remove.
Subheading 8: Applying regular expressions to remove the first character
Regular expressions provide a powerful way to manipulate strings in Python, including removing the first character. Regular expressions (regex) are patterns that are used to match and manipulate text strings. By defining a pattern that matches the first character, you can use regex functions to replace it with an empty string.
Here’s an example of using regular expressions to remove the first character from a string in Python:
“`python
import re
string = “Hello”
new_string = re.sub(“^.”, “”, string)
print(new_string)
“`
Output: “ello”
In this example, the re.sub() function is used to substitute the matched pattern (in this case, the first character denoted by `^`) with an empty string. The resulting new string, obtained by removing the first character, is then printed.
Subheading 9: Discussing the flexibility of regular expressions in removing characters
Using regular expressions to remove the first character from a string offers great flexibility. You can define complex patterns to match specific characters, digits, or even special characters. Regular expressions are not limited to removing only the first character, but allow you to handle more advanced transformations as well.
Subheading 10: Highlighting potential scenarios where character removal is useful
There are various scenarios where removing the first character from a string in Python can be useful. Some of these scenarios include:
1. Data cleaning: When working with datasets, it’s often necessary to remove or modify certain characters at the beginning of strings to ensure data consistency. Removing the first character using the discussed methods can help in this process.
2. Text manipulation: If you need to manipulate strings for text analysis or natural language processing tasks, removing the first character may be required to prepare the data for further analysis.
3. String formatting: In certain cases, removing the first character from a string can be used to format the string in a desired way, such as removing leading zeros in numbers.
In conclusion, removing the first character from a string in Python can be accomplished through various methods such as string slicing, the replace() function, regular expressions, and more. Each method offers its own advantages and flexibility, allowing you to choose the most suitable approach for your specific requirements.
FAQs:
Q1: How can I remove the first and last characters from a string in Python?
A1: To remove both the first and last characters from a string in Python, you can combine string slicing with the len() function. For example:
“`python
string = “Hello World”
new_string = string[1:len(string)-1]
print(new_string)
“`
Output: “ello Worl”
Q2: How do I remove the last character from a string in Python?
A2: Similar to removing the first character, you can remove the last character from a string using string slicing. By specifying the ending index as -1, you can exclude the last character from the slice. For example:
“`python
string = “Hello World”
new_string = string[:-1]
print(new_string)
“`
Output: “Hello Worl”
Q3: Can I remove a specific character from a string in Python?
A3: Yes, you can remove a specific character from a string in Python by using the replace() function. For example, to remove all occurrences of the character ‘a’ from a string, you can use the following code:
“`python
string = “banana”
new_string = string.replace(‘a’, ”)
print(new_string)
“`
Output: “bn”
Q4: How can I get the first character of a string in Python?
A4: To get the first character of a string in Python, you can use string indexing. By specifying the index 0, you can access the first character. For example:
“`python
string = “Hello”
first_character = string[0]
print(first_character)
“`
Output: “H”
Q5: How can I get a specific character from a string in Python?
A5: To get a specific character from a string in Python, you can use string indexing. By specifying the desired index, you can access the corresponding character. For example:
“`python
string = “Hello”
third_character = string[2]
print(third_character)
“`
Output: “l”
Q6: Can I remove characters from a string within a given range of indices?
A6: Yes, you can remove characters from a string within a given range of indices using string slicing. By specifying the starting and ending indices, you can extract a substring from the original string. For example:
“`python
string = “Hello World”
new_string = string[:3] + string[6:]
print(new_string)
“`
Output: “HelWorld”
Q7: How can I remove the first element from a list of strings in Python?
A7: To remove the first character from each string in a list, you can utilize list comprehension and string slicing. Here’s an example:
“`python
strings = [“Hello”, “World”]
new_strings = [string[1:] for string in strings]
print(new_strings)
“`
Output: [“ello”, “orld”]
Q8: How can I remove the first character from a string using the pop() function in Python?
A8: The pop() function is primarily used to remove elements from lists, not strings. To remove the first character from a string, string slicing or the replace() function would be more suitable options.
How To Remove The First Character From A String In Python
Keywords searched by users: remove first character from string python Remove first and last character from string Python, Pandas remove first character from string, Remove last character from string Python, Remove character in string Python, Get first character of string Python, Get character from string Python, Remove in string in Python, Pop string Python
Categories: Top 37 Remove First Character From String Python
See more here: nhanvietluanvan.com
Remove First And Last Character From String Python
In Python, manipulating strings is a common task, and sometimes you may need to remove the first and last characters from a given string. Whether you want to eliminate a specific character or extract a substring by excluding the first and last characters, Python offers several methods to accomplish this. In this article, we will discuss various techniques to remove the first and last character from a string in Python, along with some useful examples.
Method 1: Using string slicing
A straightforward approach to remove the first and last character from a string is by using string slicing. Python allows us to extract a portion of a string by specifying the start and end indices. To remove the first character, we use start index 1, and to remove the last character, we use the end index -1. Here’s an example:
“`python
string = “Hello World”
new_string = string[1:-1]
print(new_string)
“`
Output:
“`
ello Worl
“`
In this example, we removed the first character ‘H’ and the last character ‘d’, resulting in the string ‘ello Worl’.
Method 2: Using the strip() method
Python’s built-in strip() method is primarily used to remove leading and trailing whitespace from a string. However, you can also specify characters to remove from both ends of the string. By passing the characters to remove as an argument to the strip() method, we can achieve the desired result. Here’s an example:
“`python
string = “Hello World”
new_string = string.strip(“Hd”)
print(new_string)
“`
Output:
“`
ello Worl
“`
In this example, we removed the characters ‘H’ and ‘d’ from both ends of the string, resulting in the same output as before.
Method 3: Using the join() and split() methods
Another approach to remove the first and last character from a string is by using the join() and split() methods in combination. First, we split the string into a list of characters using the split() method. Then, we can exclude the first and last characters from the list and join the remaining characters back into a string using the join() method. Here’s an example:
“`python
string = “Hello World”
# Split the string into a list of characters
characters = list(string)
# Exclude the first and last character
new_characters = characters[1:-1]
# Join the characters back into a string
new_string = “”.join(new_characters)
print(new_string)
“`
Output:
“`
ello Worl
“`
In this example, we split the string ‘Hello World’ into a list of characters, excluding the first and last characters ‘H’ and ‘d’. Finally, we join the remaining characters back into a string, resulting in ‘ello Worl’.
FAQs:
Q1. Can I remove the first and last characters from a string of any length?
A1. Yes, the methods described above can be used to remove the first and last character from a string of any length. However, keep in mind that if the string is empty or contains only one character, the output will be an empty string.
Q2. Are there any other ways to achieve the same result?
A2. Yes, there are multiple ways to remove the first and last character from a string in Python. You can experiment with other string manipulation methods such as list comprehensions, regular expressions, or even manually iterating over the string characters.
Q3. Do these methods modify the original string?
A3. No, these methods do not modify the original string. They return a new string with the desired modifications while leaving the original string intact. If you want to update the original string, you need to assign the modified string back to the original variable.
Q4. How can I remove specific characters from both ends of a string?
A4. You can modify the methods mentioned earlier to remove specific characters from both ends of a string. Simply replace the characters ‘H’ and ‘d’ in the examples with the ones you want to remove.
Q5. Is there a difference between using string slicing and strip() method for this task?
A5. While both string slicing and the strip() method can be used to remove the first and last character from a string, they may behave differently based on the requirement. String slicing allows you to extract a substring, while the strip() method removes specific characters from both ends of the string, while preserving the characters in between.
In conclusion, removing the first and last character from a string in Python is a common requirement, and there are several methods to achieve this. Whether you prefer using string slicing, the strip() method, or a combination of join() and split() methods, you now have multiple options to manipulate strings and extract the desired substring.
Pandas Remove First Character From String
Pandas, the popular data manipulation library in Python, offers numerous functions and methods to handle strings efficiently. When working with string data, you might come across situations where you need to remove the first character from a string. In this article, we will explore different methods provided by Pandas to accomplish this task, along with examples and explanations. So, let’s dive in!
Method 1: Using the .str.slice() Method
One way to remove the first character from a Pandas string is by utilizing the .str.slice() method. This method allows you to extract a substring from a given string, specifying the starting and ending positions. To remove the first character, we can set the starting position as 1, which excludes the first character.
Here’s an example to demonstrate the usage of .str.slice() method in removing the first character:
“`python
import pandas as pd
# Creating a sample pandas Series
series_data = pd.Series([‘apple’, ‘banana’, ‘cherry’])
# Applying the .str.slice() method
updated_series = series_data.str.slice(1)
# Displaying the updated series
print(updated_series)
“`
Output:
“`
0 pple
1 anana
2 herry
dtype: object
“`
Method 2: Using the .str[1:] Indexing Method
Another way to remove the first character from a Pandas string is by utilizing the .str[1:] indexing method. This method uses the slice notation where you can specify the range of characters you want to extract. By setting the range as [1:], we exclude the first character from the string.
Let’s see an example of how to use the .str[1:] method:
“`python
import pandas as pd
# Creating a sample pandas Series
series_data = pd.Series([‘apple’, ‘banana’, ‘cherry’])
# Applying the .str[1:] method
updated_series = series_data.str[1:]
# Displaying the updated series
print(updated_series)
“`
Output:
“`
0 pple
1 anana
2 herry
dtype: object
“`
Method 3: Using the .str.replace() Method
If you want to remove the first character from all occurrences of a character or a substring within a Pandas string, you can use the .str.replace() method. This method replaces all matched occurrences with an empty string (”).
Here’s an example to show how to remove the first character from a matching substring:
“`python
import pandas as pd
# Creating a sample pandas Series
series_data = pd.Series([‘apple’, ‘banana’, ‘cherry’])
# Applying the .str.replace() method
updated_series = series_data.str.replace(‘^a’, ”, regex=True)
# Displaying the updated series
print(updated_series)
“`
Output:
“`
0 pple
1 banana
2 cherry
dtype: object
“`
FAQs:
Q1: Can I use these methods to remove the first character from a specific index in a Pandas DataFrame column?
A1: Yes, these methods can be applied to DataFrame columns by utilizing the .str accessor. You can modify the column values by chaining the desired string manipulation method to the column using the .str accessor.
Q2: Are these methods case-sensitive?
A2: Yes, by default, these methods are case-sensitive. However, you can make them case-insensitive by setting the regex parameter to True in the .str.replace() method, and using appropriate regular expressions to capture the desired characters.
Q3: What if the string has fewer than two characters? Will these methods still work?
A3: Yes, these methods will not produce any errors if the string has fewer than two characters. They will simply return an empty string (”) for such cases.
Q4: Can I remove more than one character using these methods?
A4: Yes, you can modify the slicing ranges in methods 1 and 2 to remove more than one character from the start of the string.
In conclusion, Pandas provides multiple ways to remove the first character from a string. Whether you prefer using the .str.slice() method, the .str[1:] indexing method, or the .str.replace() method, you can achieve the desired results efficiently. By applying the appropriate method according to your specific scenario, you can effectively manipulate string data in a Pandas DataFrame or Series.
Remove Last Character From String Python
Python is a popular programming language known for its simplicity, readability, and versatility. It provides several built-in functions and methods to manipulate strings effortlessly. One common string manipulation requirement is to remove the last character from a string. In this article, we will explore various ways to accomplish this task in Python.
Method 1: Using slicing
Python allows slicing of strings using the ‘:’ operator. To remove the last character, we can slice the string excluding the last character and assign it to a new variable.
“`python
def remove_last_character(string):
new_string = string[:-1]
return new_string
“`
Here, the slice `[:-1]` denotes all the characters from the start till the second-to-last character. By omitting the second index, we instruct Python to include all the characters till the end.
Method 2: Using the `rstrip()` function
Python provides the `rstrip()` function, which removes trailing characters from the right side of a string. By passing the last character as an argument, we can remove it effectively.
“`python
def remove_last_character(string):
new_string = string.rstrip(string[-1])
return new_string
“`
The `rstrip()` function removes any occurrences of the given character from the end of the string. In this case, we use the last character of the string to ensure only the last character is removed.
Method 3: Using the `join()` function
Python’s `join()` function is a powerful tool for string manipulation. By splitting the string into individual characters and rejoining all except the last character, we can remove it easily.
“`python
def remove_last_character(string):
new_string = “”.join(string.split()[:-1])
return new_string
“`
Here, the `split()` function is used to split the string into a list of characters. We then slice the list till the second-to-last element using `[:-1]`. Finally, we use `join()` to rejoin the list of characters into a string.
Method 4: Using string concatenation
Python allows string concatenation, which is useful for removing the last character. By concatenating an empty string with all the characters except the last one, we can achieve the desired result.
“`python
def remove_last_character(string):
new_string = ”
for i in range(len(string)-1):
new_string += string[i]
return new_string
“`
This method uses a loop to iterate through each character of the string, excluding the last one. It then concatenates each character to an empty string, effectively removing the last character.
Frequently Asked Questions (FAQs):
Q1: Can I remove the last character from a string in Python without using any built-in functions or methods?
A1: Technically, it is possible to achieve this by slicing the string. However, utilizing built-in functions or methods is generally recommended for better code clarity and performance.
Q2: How can I remove the last character from a string if it contains special characters or whitespaces?
A2: The methods discussed above work regardless of whether the string contains special characters or whitespaces. The implementations are generic and will remove the last character in any case.
Q3: Is it possible to remove multiple characters from the end of a string?
A3: Yes, all the methods presented here can be modified to remove multiple characters. Simply adjust the slicing or logic to exclude the desired number of characters from the end.
Q4: Can I remove the last character from a string in-place, without creating a new string?
A4: No, strings in Python are immutable, meaning they cannot be changed directly. Therefore, a new string needs to be created when removing the last character.
In conclusion, removing the last character from a string in Python is a common task in string manipulation. By utilizing different methods like slicing, string functions, or string concatenation, we can achieve this with simplicity and efficiency. Choose the most suitable method based on your specific requirements and coding preferences.
Images related to the topic remove first character from string python
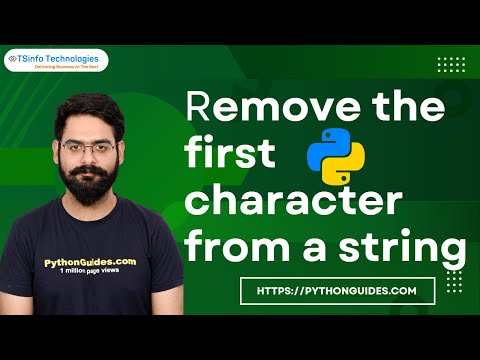
Found 48 images related to remove first character from string python theme
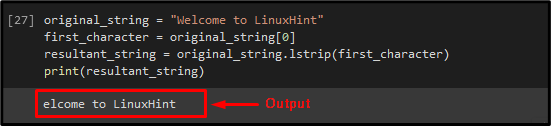
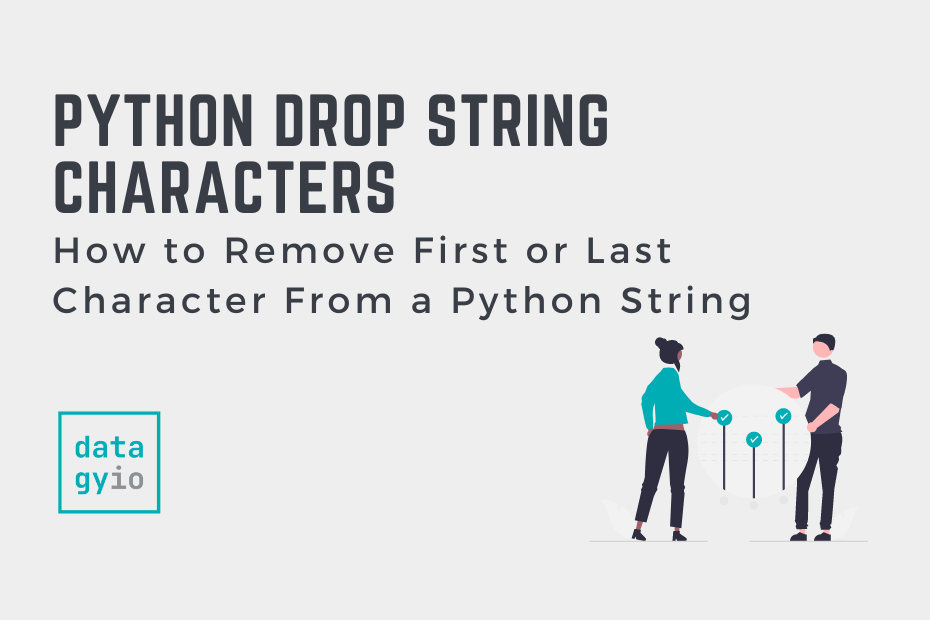

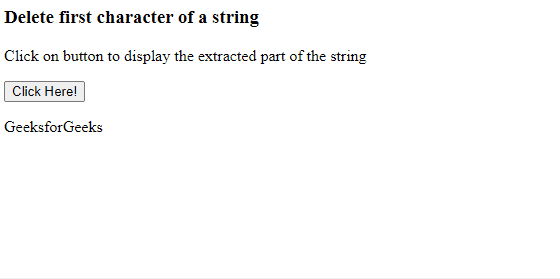
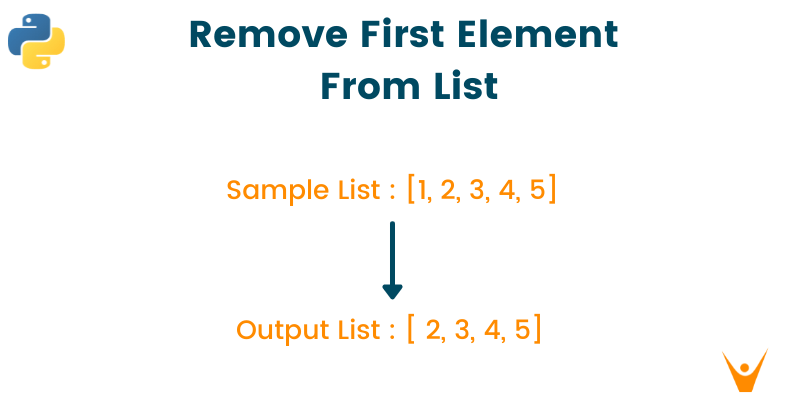
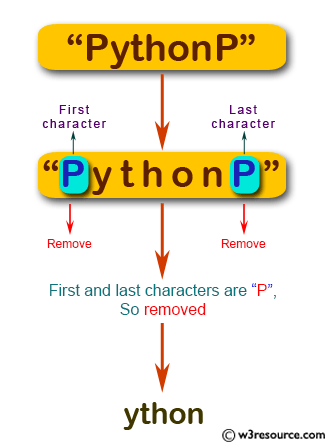
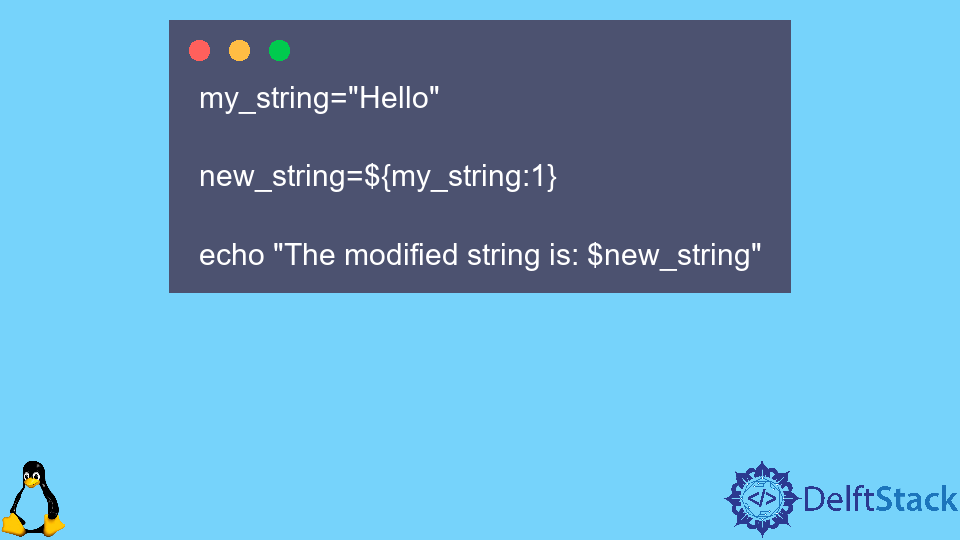

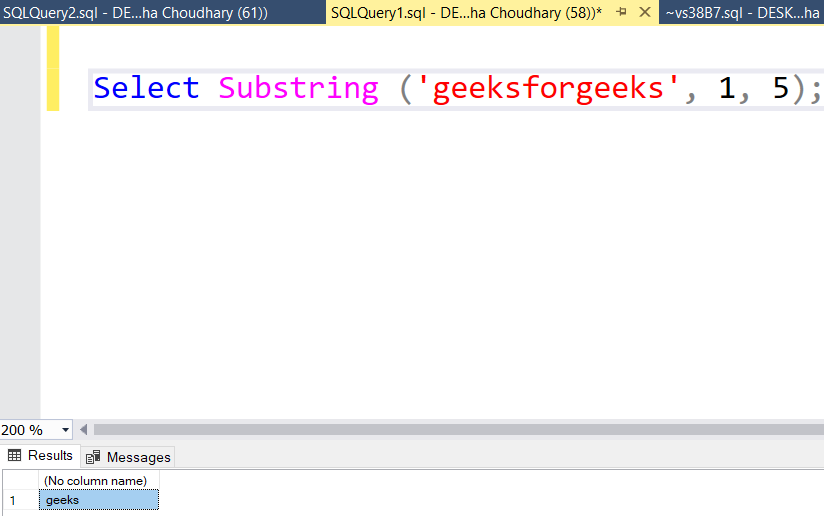
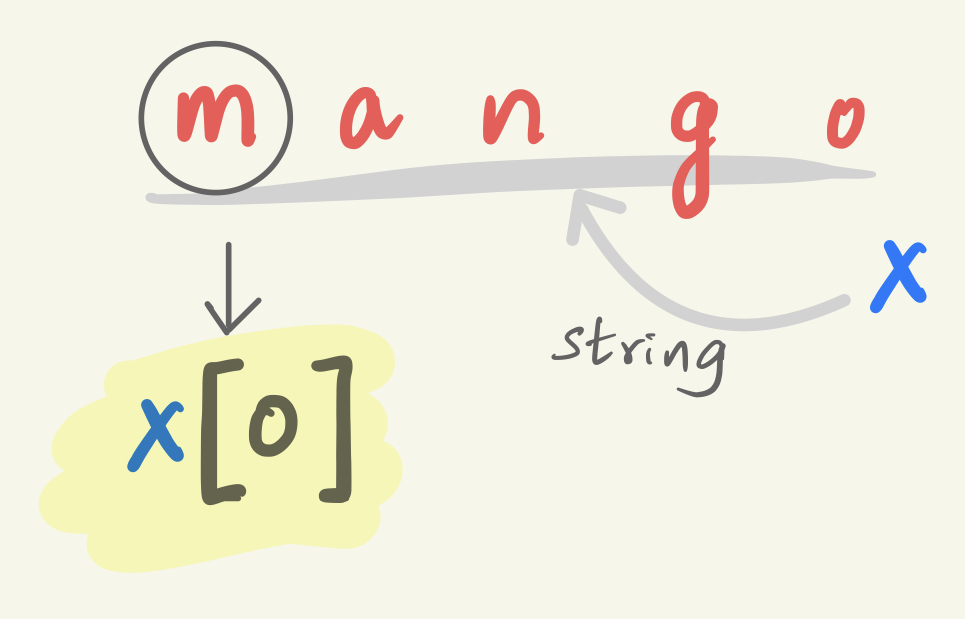
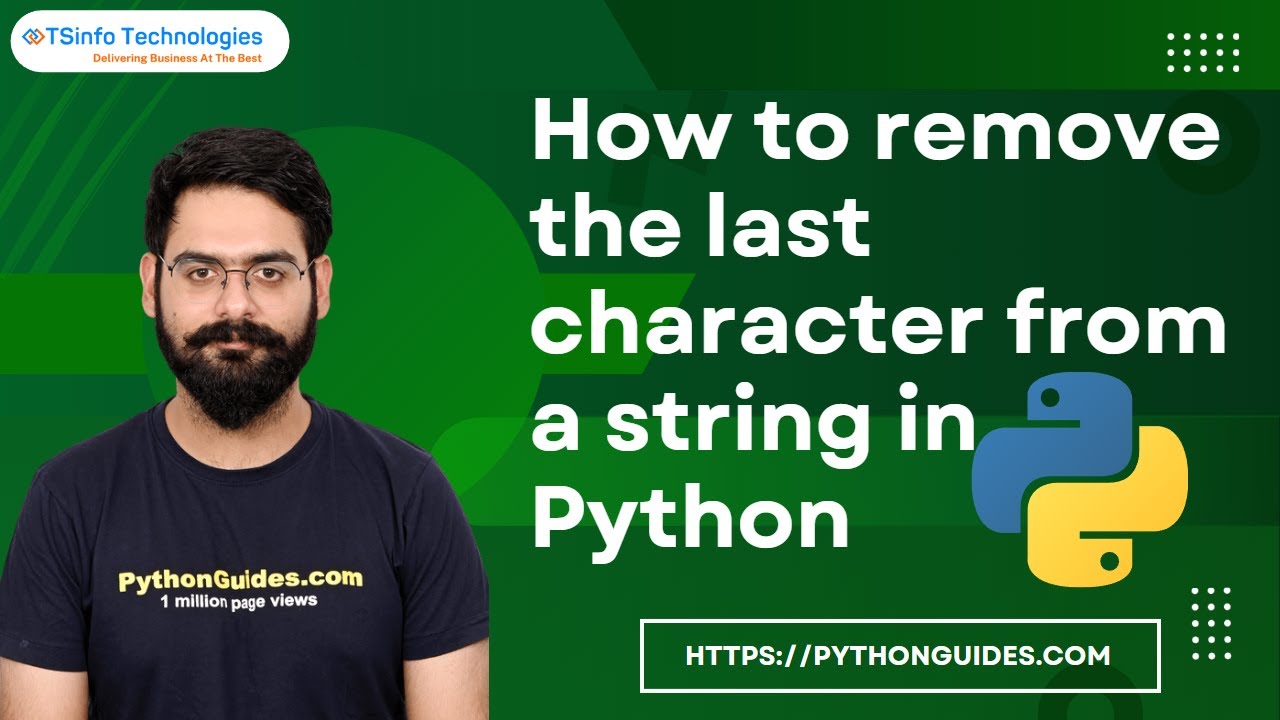
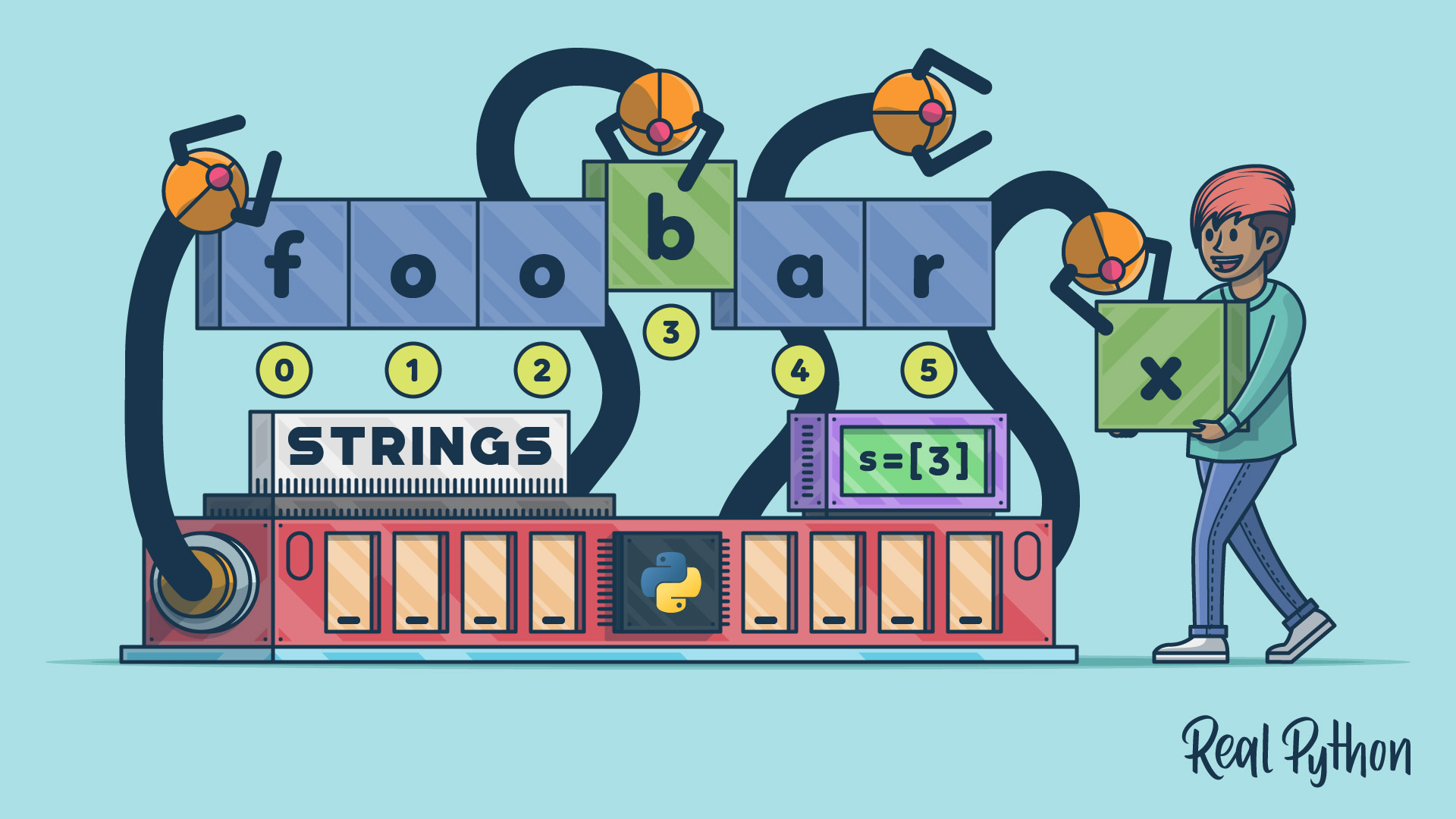
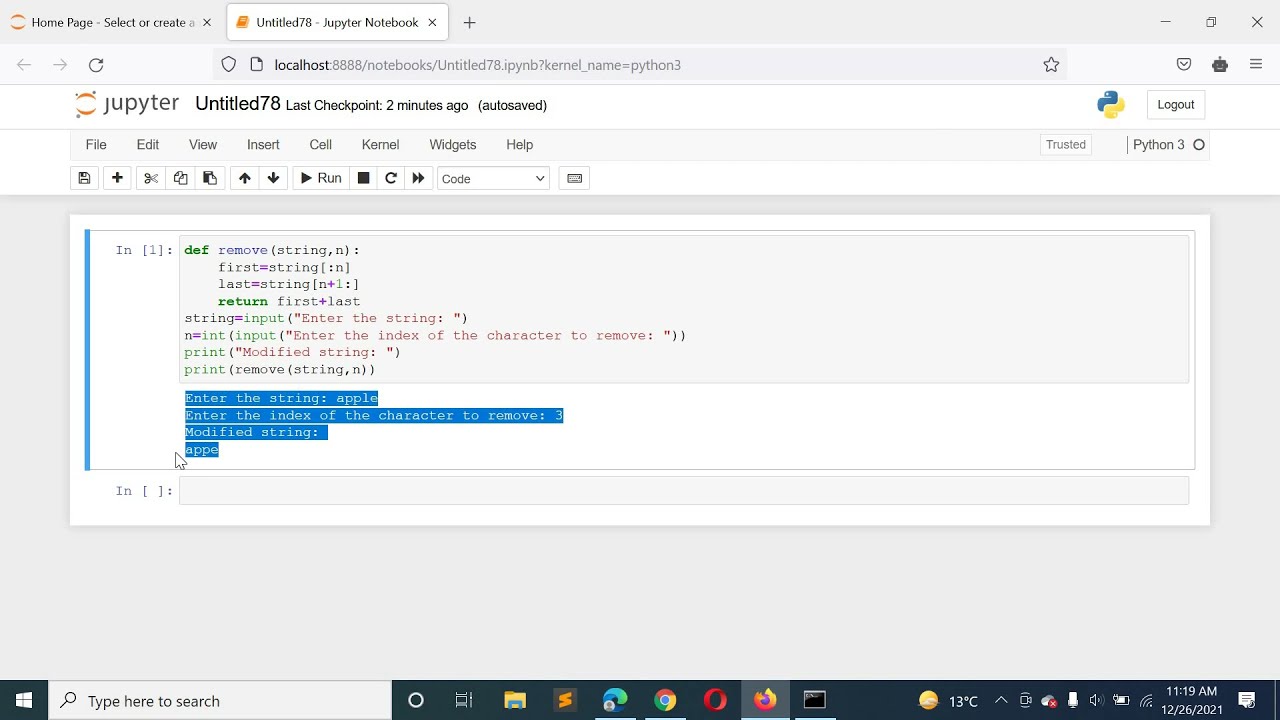
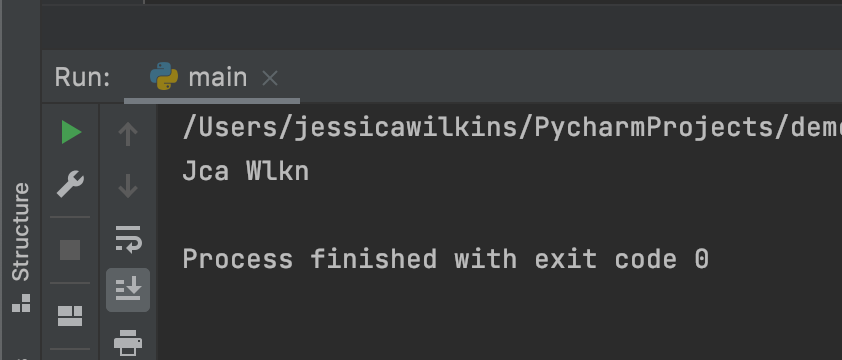
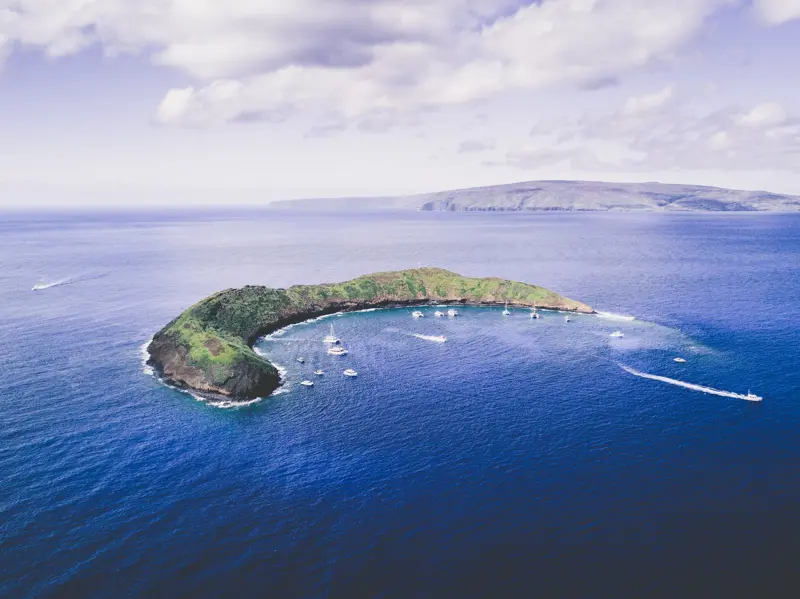
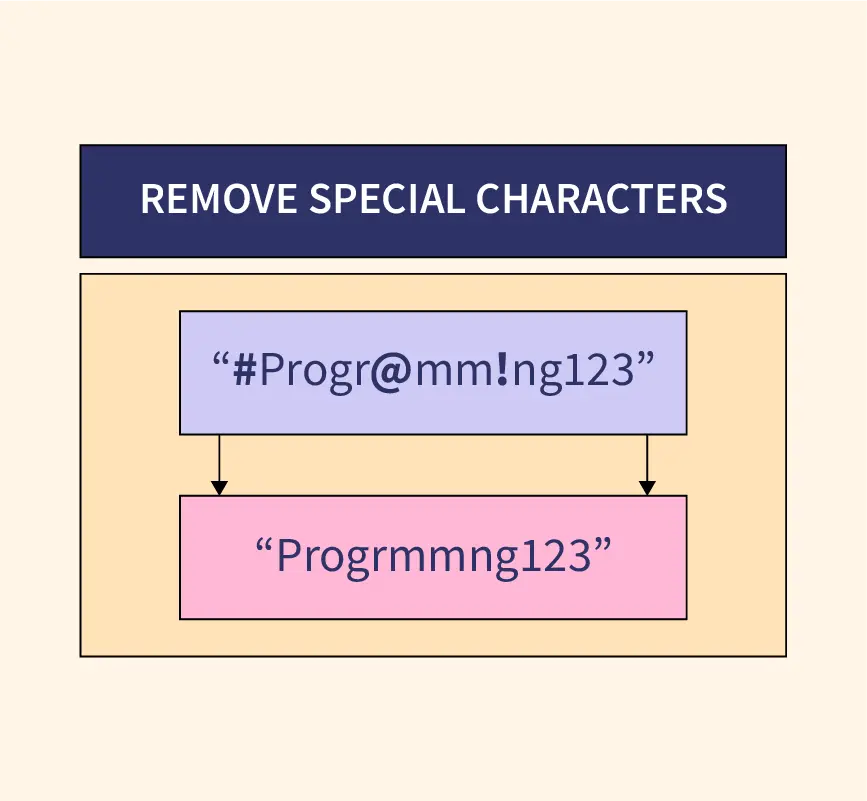
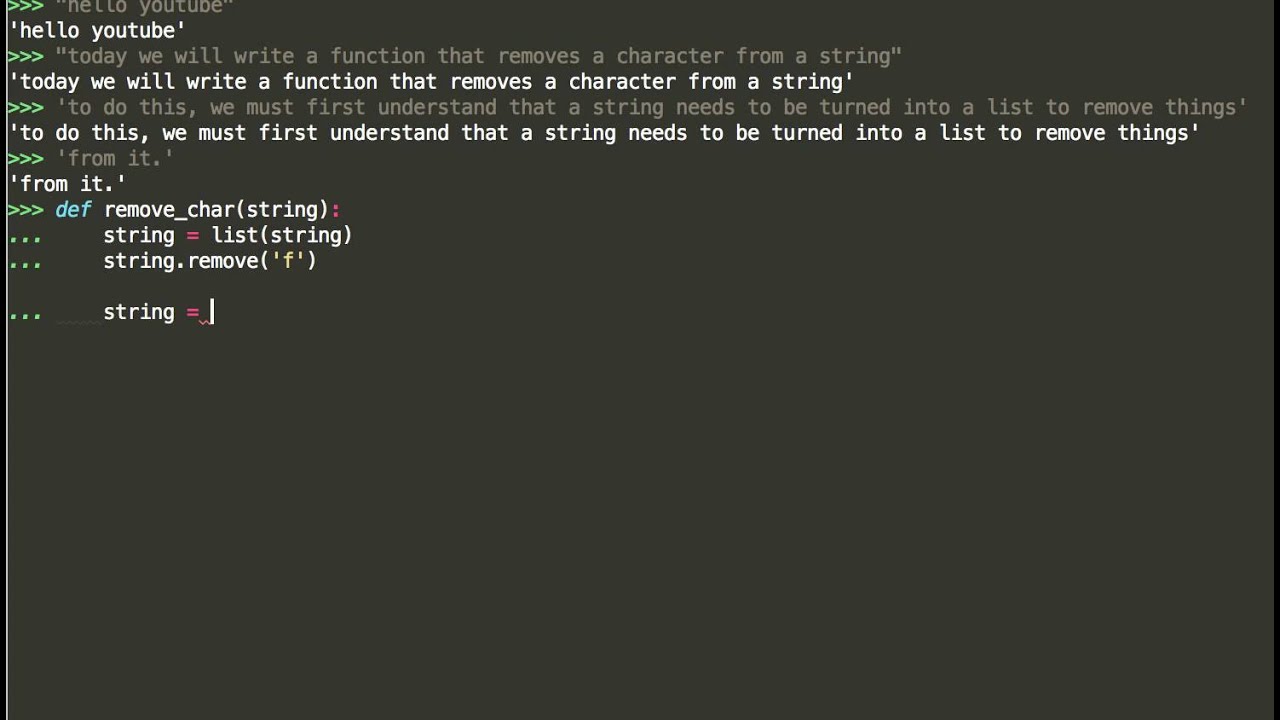
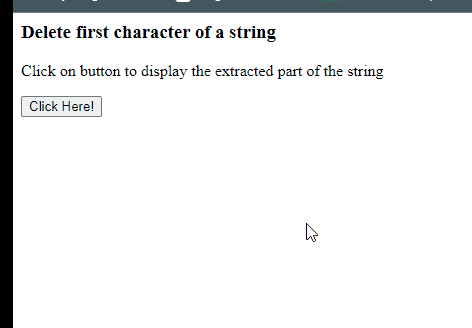
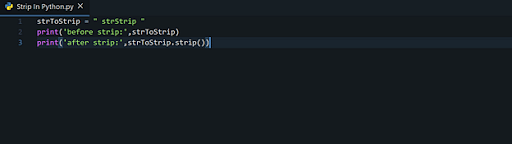
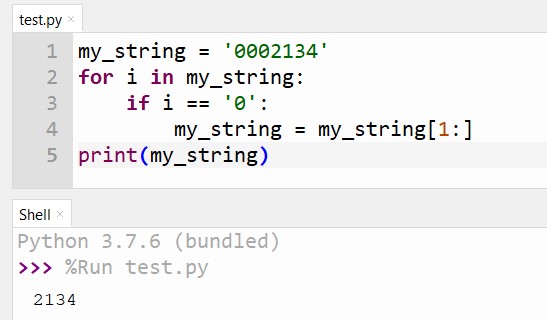

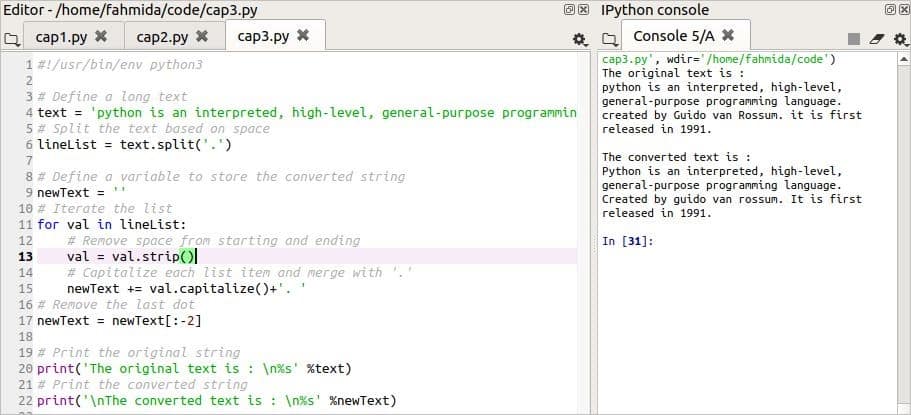
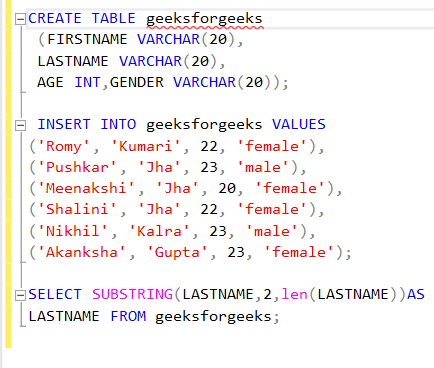
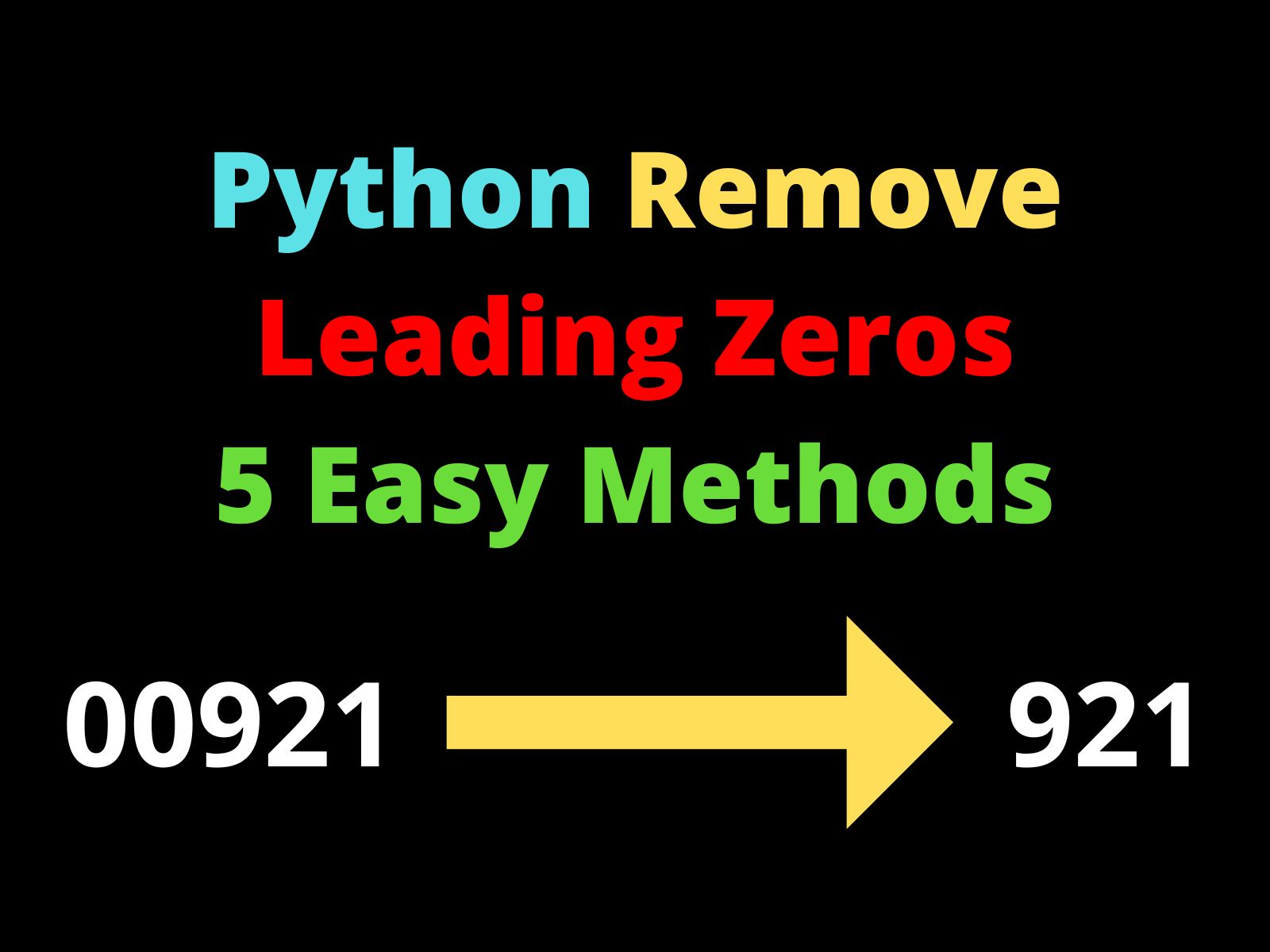
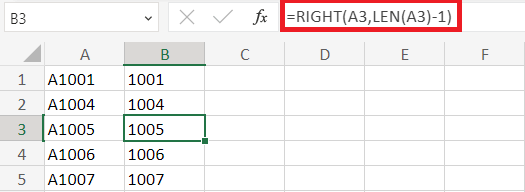
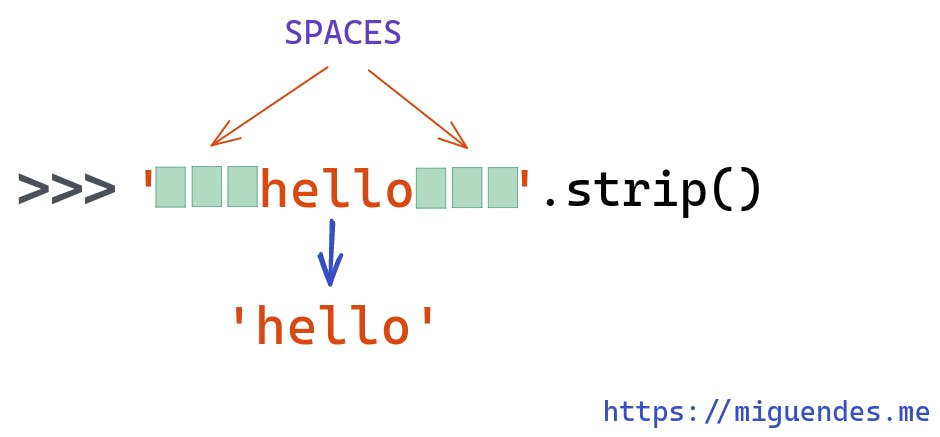
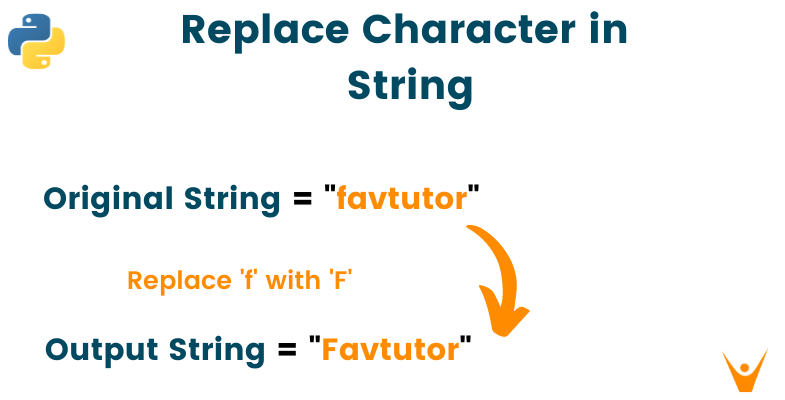
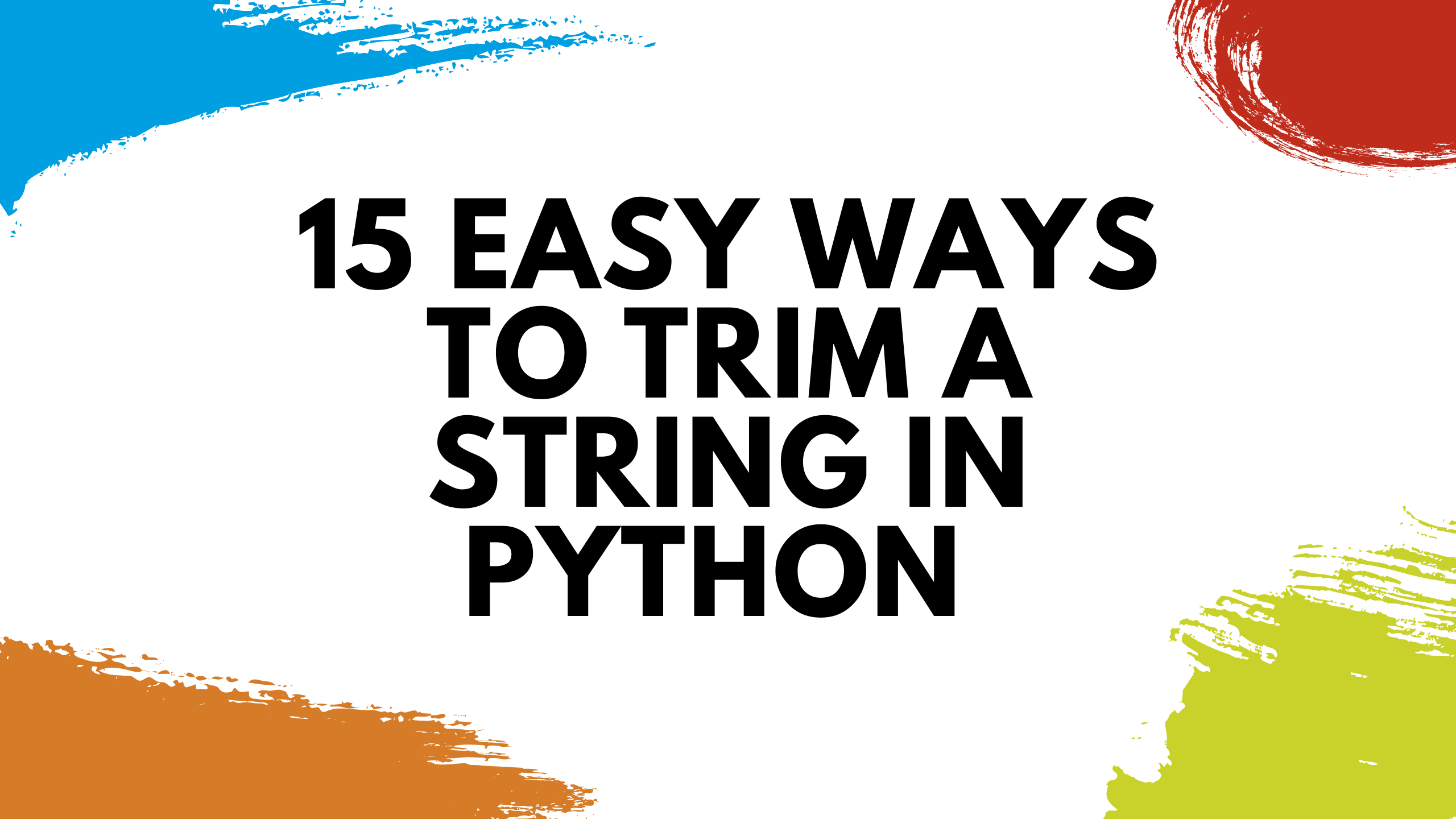

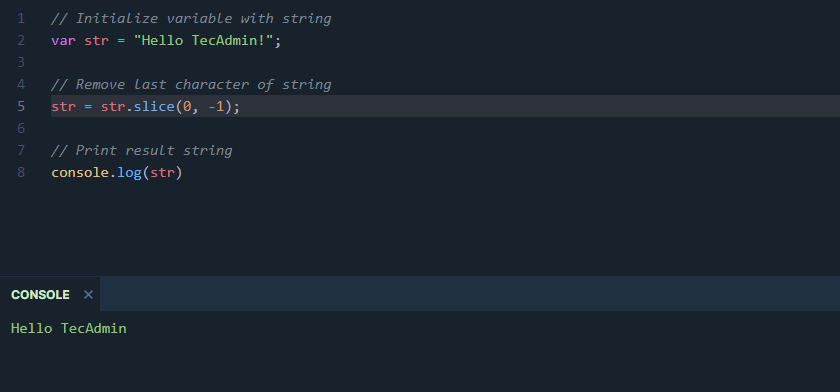
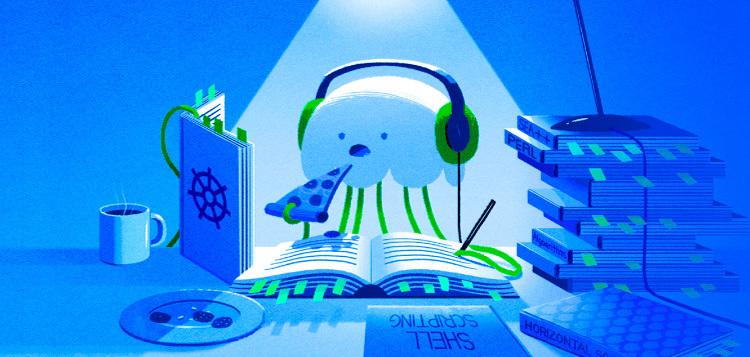
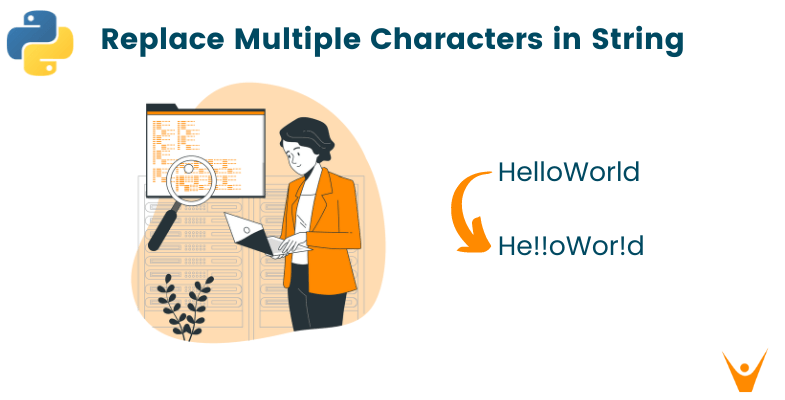
![How to remove characters from string in Power Automate? [with examples] - SPGuides How To Remove Characters From String In Power Automate? [With Examples] - Spguides](https://i0.wp.com/www.spguides.com/wp-content/uploads/2022/09/Power-Automate-remove-the-first-character-from-string-1024x592.png)

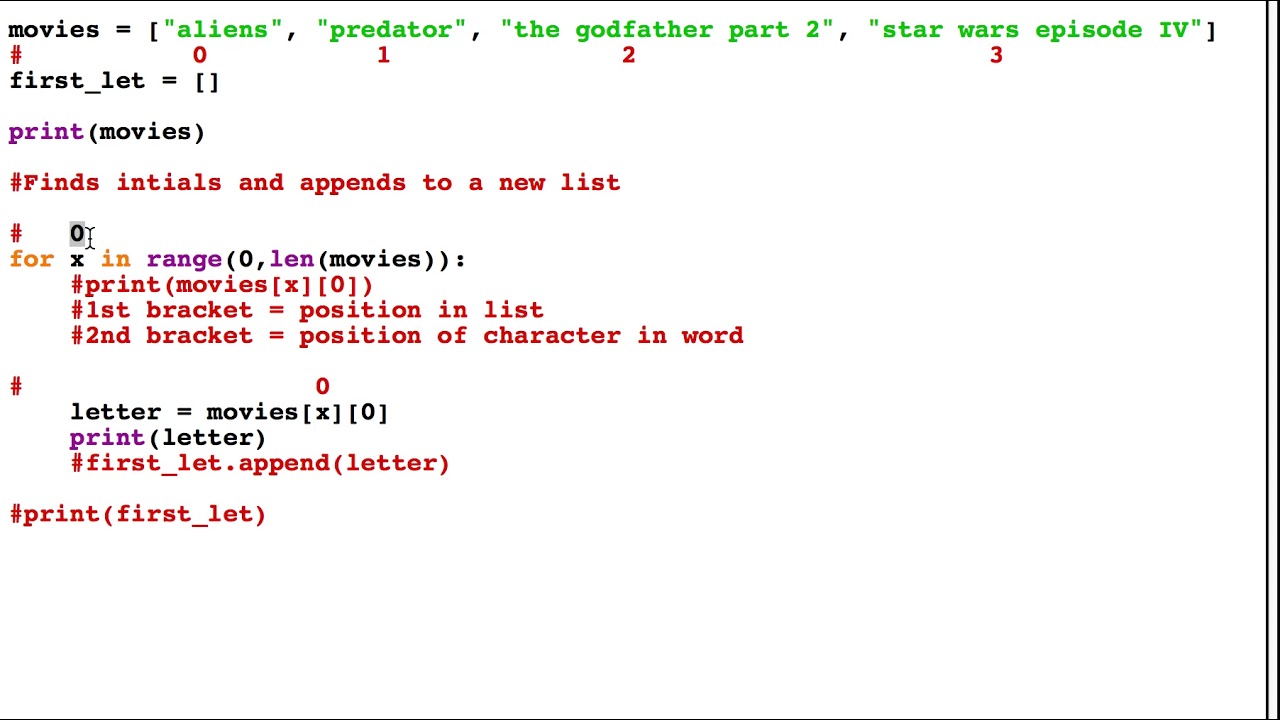


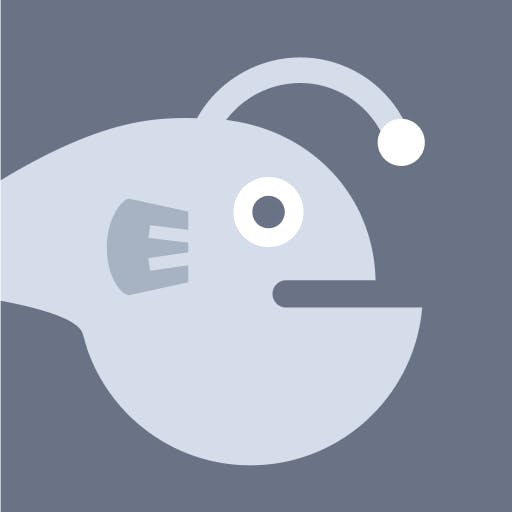

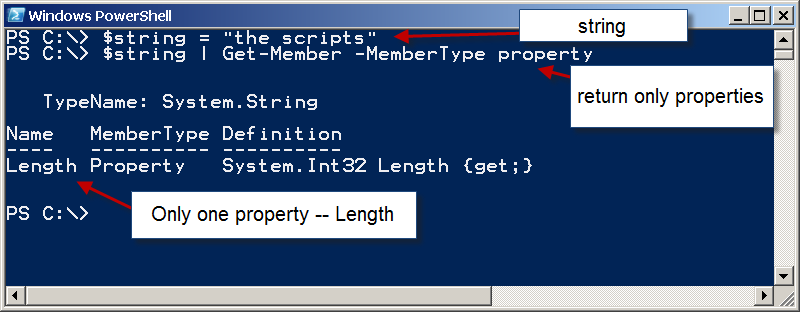
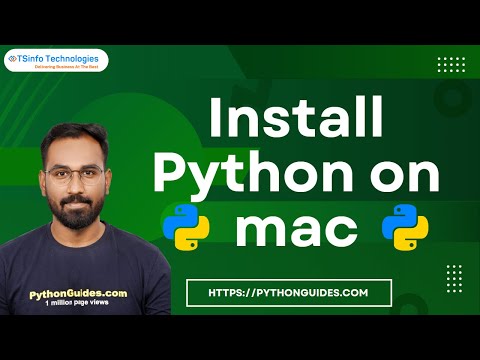
![How to remove characters from string in Power Automate? [with examples] - SPGuides How To Remove Characters From String In Power Automate? [With Examples] - Spguides](https://i0.wp.com/www.spguides.com/wp-content/uploads/2022/09/Power-Automate-remove-the-first-character-from-string-result-1024x303.png)
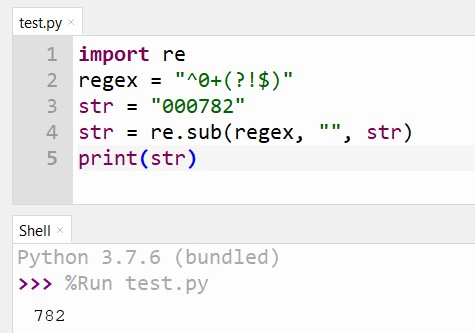
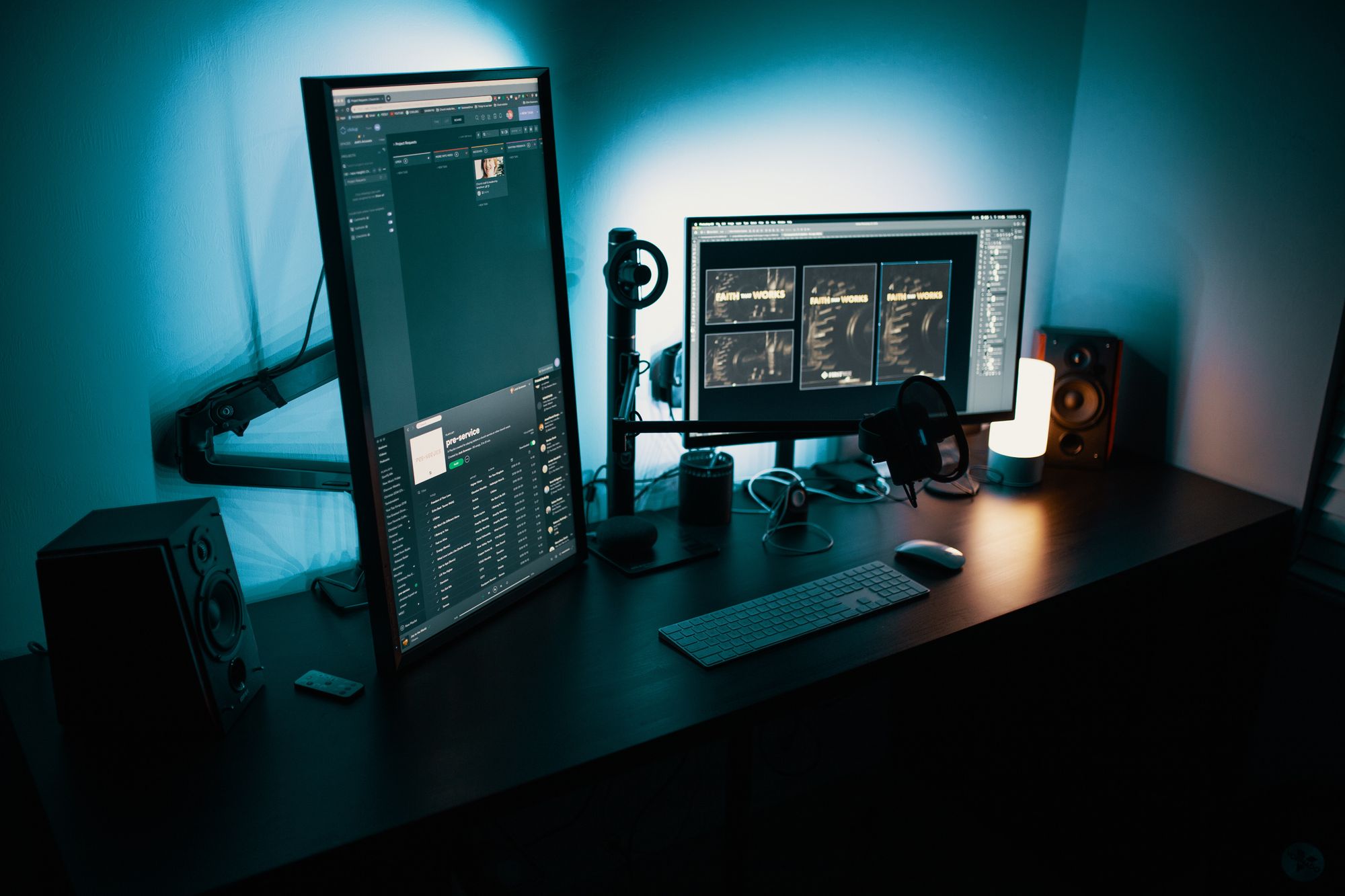

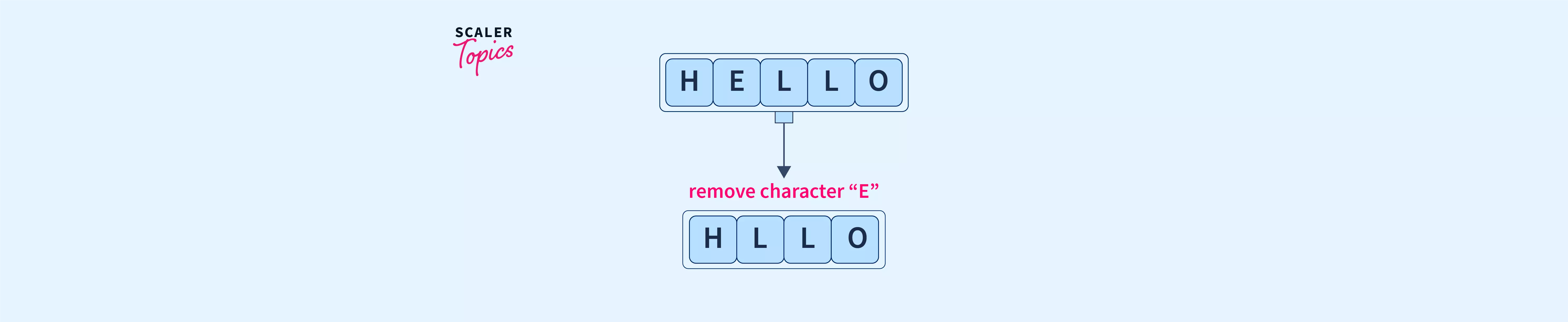
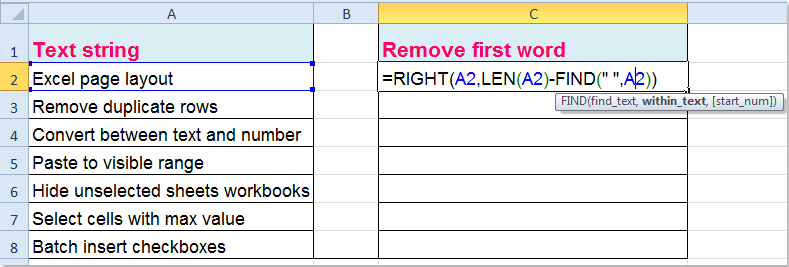
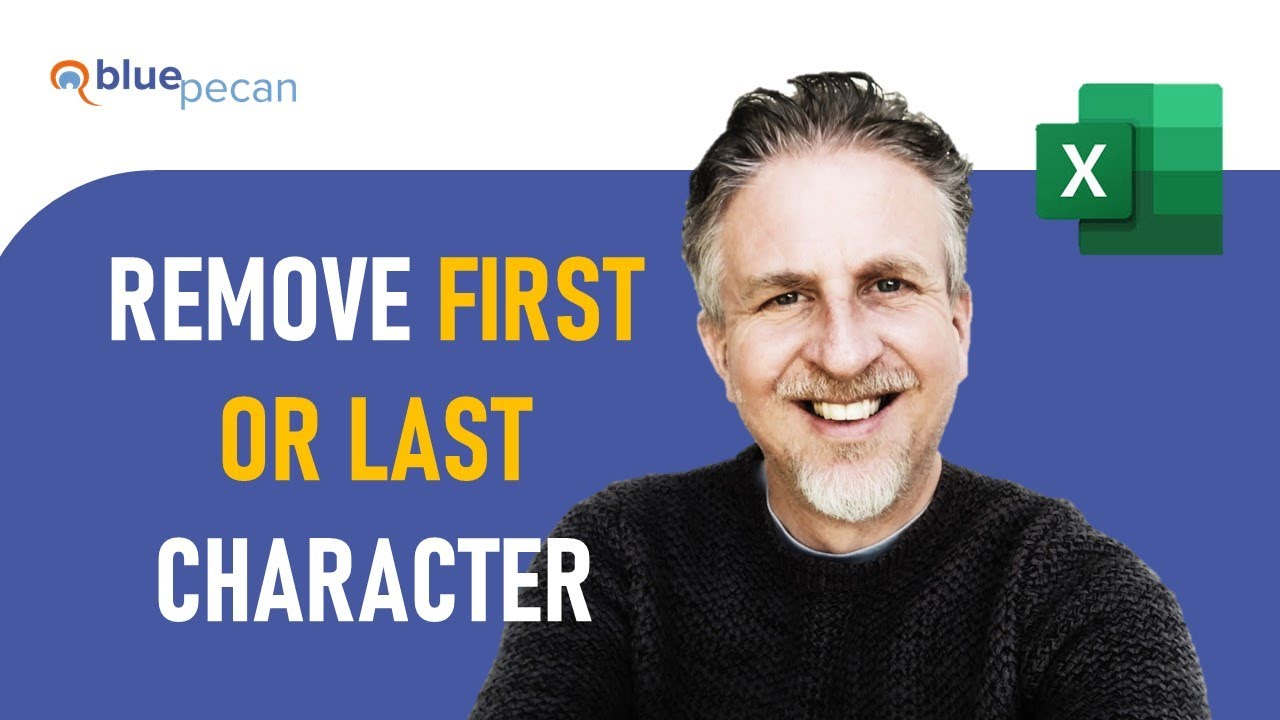
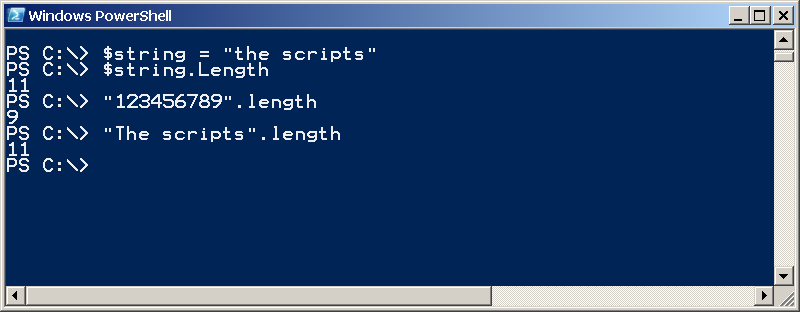
Article link: remove first character from string python.
Learn more about the topic remove first character from string python.
- Remove the first character of a string – python – Stack Overflow
- How To Remove First Character From A String In Python
- Remove First Character From String in Python – Linux Hint
- Remove first character from a Python string – Techie Delight
- Remove the First Character From the String in Python
- How to Remove the First and Last Character from a String in …
- Python – Delete First Character from String
See more: https://nhanvietluanvan.com/luat-hoc