Reading Csv File In C#
Understanding CSV Files: An Overview
CSV (Comma-Separated Values) is a common file format used for storing tabular data. It consists of plain text where each line represents a row, and within each line, values are separated by commas. CSV files are widely used for data exchange between different software systems because of their simplicity and easy readability.
Retrieving CSV File Data: Importing and Reading in C#
When working with CSV files in C#, you need to first import the necessary libraries. The System.IO namespace provides the StreamReader class, which allows you to read characters from a stream such as a file. Additionally, the System.Data namespace provides the DataTable class to handle tabular data.
Opening and Accessing a CSV File in C#
To open a CSV file in C#, you can use the OpenText method of the StreamReader class. This method takes the file path as a parameter and returns a StreamReader object. You can then use this object to read the contents of the file line by line.
“`csharp
using System.IO;
StreamReader sr = File.OpenText(“data.csv”);
string line;
while ((line = sr.ReadLine()) != null)
{
// Process each line
}
sr.Close();
“`
Parsing CSV Files: Extracting Data from Rows and Columns
Once you have opened the CSV file, you can start extracting data from it. You can split each line into an array of values using the Split method, which splits a string into substrings based on a specified delimiter, in this case, the comma.
“`csharp
string[] values = line.Split(‘,’);
“`
This will create an array of values, where each element represents a cell in the CSV file. You can then access individual cells by their index.
Handling Header Information in CSV Files
CSV files often contain a header row that describes the data in each column. To skip the header row when reading the file, you can use a boolean flag that gets set after reading the first line.
“`csharp
bool isFirstLine = true;
while ((line = sr.ReadLine()) != null)
{
if(isFirstLine)
{
isFirstLine = false;
continue;
}
// Process each line
}
“`
Filtering CSV Data: Selecting Specific Rows or Columns
In some cases, you may only be interested in specific rows or columns of the CSV file. You can use conditional statements to filter the data based on certain criteria.
“`csharp
while ((line = sr.ReadLine()) != null)
{
string[] values = line.Split(‘,’);
if (values[0] == “John”)
{
// Process data for John
}
}
“`
Performing Data Manipulation: Sorting, Aggregating, and Transforming CSV Data
C# provides various methods and techniques to manipulate CSV data. You can sort the data using the Array.Sort or List.Sort methods, aggregate data using LINQ queries, or transform the data using string manipulation and formatting functions.
Error Handling and Exception Handling in CSV File Reading
When reading CSV files in C#, it is essential to handle any errors or exceptions that may occur. You should wrap your file reading code within try-catch blocks to handle potential exceptions such as file not found, file format errors, or unexpected data.
Best Practices and Tips for Efficiently Reading CSV Files in C#
To efficiently read large CSV files in C#, consider the following best practices:
1. Use the CsvHelper library: CsvHelper is a popular third-party library that provides powerful functionalities for working with CSV files in C#.
2. Use parallel processing: If the data processing logic allows, you can use parallel processing techniques such as multi-threading or async/await to improve performance.
3. Use a streaming approach: Instead of loading the entire CSV file into memory, consider using a streaming approach where you read and process data line by line.
4. Dispose of resources properly: Always ensure to properly close and dispose of StreamReader objects and other resources to avoid memory leaks.
FAQs about reading CSV files in C#:
Q: How can I read a CSV file in C?
A: In C, you can read a CSV file using the standard C file I/O functions such as fopen, fread, and fclose.
Alternatively, you can use libraries like libcsv or csvparser to simplify the task.
Q: How can I read a CSV file into an array in C++?
A: In C++, you can read a CSV file into an array by creating a 2-dimensional array or a vector of vectors to store the data.
You can then use file I/O functions like ifstream and getline to read and parse the CSV file line by line.
Q: How can I read a CSV file in C#?
A: To read a CSV file in C#, you can use the StreamReader class from the System.IO namespace.
The StreamReader class provides various methods for reading data from a file, such as Read, ReadLine, and ReadToEnd.
Q: How can I read an Excel file in C#?
A: To read an Excel file in C#, you can use the ExcelDataReader library, which provides a simple and efficient way to read data from Excel files.
The library supports both .xls and .xlsx formats.
Q: How can I read a CSV file in C++?
A: In C++, you can read a CSV file using the ifstream class from the
You can use getline and stringstream to parse the data and store it in a suitable data structure.
Q: How can I read a file in C?
A: To read a file in C, you can use the fopen function to open the file, fread function to read data from the file, and fclose function to close the file.
Q: How can I read an integer from a file in C?
A: To read an integer from a file in C, you can use the fscanf function with the appropriate format specifier “%d”.
The fscanf function reads formatted input from a file and stores it into the specified variable.
Q: How can I split a string into words by space in C?
A: To split a string into words by space in C, you can use the strtok function from the
The strtok function splits a string into tokens based on a specified delimiter, in this case, a space character.
C Programming Tutorial – How To Read Csv File
Keywords searched by users: reading csv file in c# Read file CSV in C, C++ read csv file into array, Read CSV file C#, Read excel file in c, Read CSV file C++, Read file in C, Read integer from file in C, Write a program in C to split string by space into words
Categories: Top 74 Reading Csv File In C#
See more here: nhanvietluanvan.com
Read File Csv In C
CSV (Comma Separated Values) is a popular file format used to store tabular data. It is commonly employed for data interchange between different applications. Most spreadsheet applications can read and write CSV files, making it a widely supported format.
In this article, we will discuss how to read a CSV file in the C programming language. We will cover the essential steps and provide a complete example to demonstrate the process. Additionally, we will address some frequently asked questions related to reading CSV files in C.
Getting started
Before we dive into reading a CSV file, we need to make sure we have a valid CSV file to work with. A CSV file typically consists of plain text data organized in rows and columns. Each line represents a row, and the values within the row are separated by commas. Here’s an example of a simple CSV file:
“`
Name, Age, City
John, 25, New York
Alice, 30, London
Bob, 27, Paris
“`
To read this CSV file in C, we first need to open the file using the `fopen()` function. This function takes two parameters: the name of the file and the mode in which it will be accessed. In this case, we will use the “r” mode to open the file for reading.
“`c
FILE* file = fopen(“data.csv”, “r”);
“`
After opening the file, we can start reading its contents. The `fscanf()` function can be used to read data from the file. This function reads formatted data from a stream, which in our case is the CSV file. We need to specify a format string that describes the expected structure of our CSV file.
“`c
char name[100];
int age;
char city[100];
while (fscanf(file, “%[^,], %d, %[^\n]”, name, &age, city) == 3) {
// Process data…
}
“`
In the above example, we provide a format string that matches the structure of our CSV file. The `%[^,]` specifier reads a string until it encounters a comma, `%d` reads an integer, and `%[^\n]` reads a string until a newline character is encountered. The `^` character inside the square brackets means “any character except.”
Reading the CSV file line by line
To read the entire CSV file line by line, we can use a loop that continues until the `fscanf()` function returns a value other than 3. This indicates that there is no more data to read or that an error has occurred while reading.
“`c
while (fscanf(file, “%[^,], %d, %[^\n]”, name, &age, city) == 3) {
// Process data…
}
“`
Within the loop, we have access to the values read from each line of the CSV file. We can perform any required operations, such as printing the data or storing it in a data structure for further processing.
Handling errors
While reading a CSV file, it is essential to handle potential errors. The `fscanf()` function returns the number of items successfully assigned as specified by the format string. By checking this return value, we can ensure that the data was read correctly.
“`c
while (1) {
int result = fscanf(file, “%[^,], %d, %[^\n]”, name, &age, city);
if (result == EOF) {
// Reached end of file
break;
} else if (result != 3) {
// Error occurred while reading
printf(“Error reading CSV file.\n”);
break;
}
// Process data…
}
“`
In the above example, we check for two possible outcomes. If the result is `EOF`, we have reached the end of the file, and we can break out of the loop. If the result is not 3, an error occurred while reading the CSV file, and we can display an error message and break the loop.
Frequently Asked Questions
Q: Can I use a different delimiter instead of a comma in a CSV file?
A: Yes, CSV files can use different delimiters, such as semicolons or tabs. To read a CSV file with a different delimiter, you need to modify the format string in your code accordingly.
Q: How can I handle CSV files with varying numbers of columns?
A: In C, when reading a CSV file with varying numbers of columns, you need to dynamically allocate memory to store the read data. One common approach is to use a linked list or a similar data structure that can grow dynamically as new data is read.
Q: How can I handle CSV files with quoted values?
A: If your CSV file contains values enclosed in quotes (e.g., “John Doe”,”25″,”New York”), you can modify your format string to take into account the quotes while reading the data. For example: `%\”[^\”]\”, %d, \”%[^\”]\”`.
Q: Is there a library available to read CSV files in C?
A: Yes, there are several libraries available, such as libcsv and libcsv2, that provide more advanced features for reading and manipulating CSV files in C. These libraries can offer additional functionality beyond the basic reading described in this article.
Conclusion
Reading CSV files in C is a straightforward process once you understand the basics. By utilizing the `fscanf()` function and specifying the format string corresponding to your CSV file structure, you can extract the data and use it according to your program’s requirements. Remember to handle potential errors while reading the file and consider using libraries if you require more advanced functionalities when working with CSV files in C.
C++ Read Csv File Into Array
Reading a CSV file and storing its data in an array involves several essential steps. Let’s outline each of these steps to gain a clear understanding:
1. Opening the CSV file: To start, we need to open the CSV file using the appropriate file handling mechanisms in C++. The iostream library provides us with the necessary tools to handle file input. We create an ifstream object and use its open() function to open the CSV file.
2. Parsing the CSV file: Once the file is successfully opened, we need to read its contents line by line and separate them into individual records. We can utilize the getline() function from the std::getline() standard library to achieve this. By specifying the delimiter (typically a comma in a CSV file) as the second argument, getline() will read until it encounters the delimiter and store the extracted record in a string variable.
3. Storing the CSV data: As we parse each line of the CSV file, we need to store the extracted records into an array for further processing. To achieve this, we create an array or a vector and append each record to it. This step allows us to retrieve and manipulate the data efficiently.
Now that we have covered the basic steps involved, let’s take a closer look at a code snippet that demonstrates the process:
“`cpp
#include
#include
#include
#include
#include
int main() {
std::ifstream file(“data.csv”);
std::vector
std::string line;
while (std::getline(file, line)) {
std::stringstream lineStream(line);
std::string cell;
std::vector
while (std::getline(lineStream, cell, ‘,’)) {
row.push_back(cell);
}
data.push_back(row);
}
// Array ‘data’ now contains the CSV data
// Further processing can be performed on the data array
return 0;
}
“`
In the above code, we open a CSV file named “data.csv” using an ifstream object named ‘file’. We also declare a vector of vectors of strings, ‘data’, which will store the extracted CSV records. By using nested std::getline() calls, we are able to split each line into individual fields using the comma (‘,’) delimiter and append them to the respective rows and the data vector.
At this point, you should now have a clear understanding of how to read a CSV file into an array using C++. However, it’s worthwhile to address a few frequently asked questions about this topic:
Q1. Can I read CSV files with different delimiters?
Yes, the code snippet provided above assumes the use of the comma (‘,’) delimiter. However, you can modify it to handle CSV files with different delimiters. Simply replace the ‘,’ character with the desired delimiter in the inner std::getline() call.
Q2. How can I handle CSV files with different data types?
The code presented above stores all values as strings. To handle CSV files with different data types, you can convert the strings to the desired data type after reading them into the array. You can use functions like std::stoi(), std::stof(), or std::stod() to convert strings to integer, float, or double respectively.
Q3. How can I handle CSV files with headers?
If your CSV file contains headers, you can choose to either skip reading the first line and treat it as a header row or store it separately. This can be achieved by adding a condition to check for the first line and handling it accordingly.
By following the steps and understanding the code provided in this article, you can successfully read a CSV file into an array using C++. Remember to adjust the code as necessary depending on the specifics of your CSV file, such as different delimiters or data types.
Images related to the topic reading csv file in c#
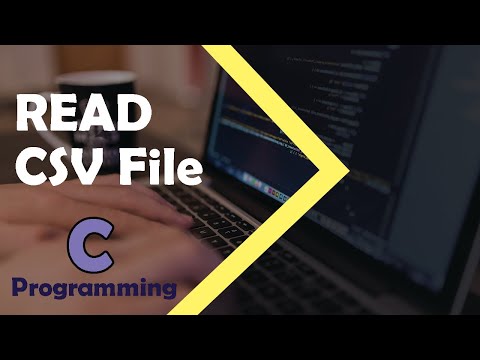
Article link: reading csv file in c#.
Learn more about the topic reading csv file in c#.
- Reading CSV files in C ::
- parsing – Read .csv file in C – Stack Overflow
- Relational Database from CSV Files in C – GeeksforGeeks
- Reading and displaying each line from a CSV file in … – Plus2net
- Read data from a .csv file in c – C Board
- How to read a CSV file and store the values into an array in C
See more: https://nhanvietluanvan.com/luat-hoc