Reading Csv File C#
CSV (Comma Separated Values) file format is widely used for data storage and interchange due to its simplicity and compatibility across different platforms and applications. In this article, we will provide a comprehensive guide on how to read CSV files in C# and explore different approaches, libraries, and techniques to handle CSV file reading efficiently.
Overview of CSV File and its Importance:
CSV is a plain-text file format that stores tabular data in a structured manner. Each line in a CSV file represents a row of data, and the values within each row are separated by commas (or any other specified delimiter). CSV files are commonly used for various purposes such as data export, data import, data backup, and data analysis.
Understanding the Structure of a CSV File:
A CSV file consists of rows and columns. Each row represents a record, and each column represents a field or attribute of that record. The values within each row are separated by commas, and the number of columns in each row should be consistent throughout the file. Additionally, CSV files may have special rules for delimiters, quoting, and escaping characters to handle the presence of commas or quotes within the data.
Choosing the Appropriate Method for Reading CSV Files in C#:
When it comes to reading CSV files in C#, there are multiple approaches available. The choice of the method depends on various factors such as the complexity of the CSV file, performance requirements, available libraries, and personal preferences. Some of the common approaches include using built-in File IO classes, utilizing third-party CSV parsing libraries, or implementing custom parsing logic.
Using the Built-in File IO Classes in C#:
C# provides built-in classes in the System.IO namespace for reading files, including CSV files. Classes like StreamReader or TextFieldParser can be used to parse CSV files and extract data. These classes offer various methods and properties to read and manipulate the CSV data. It is important to handle errors and implement best practices while using these classes, such as proper resource disposal and exception handling.
Utilizing Third-party CSV Parsing Libraries:
To simplify the process of reading CSV files in C#, several third-party libraries are available, such as CsvHelper or CsvHelper.Light. These libraries provide a higher level of abstraction and offer features like automatic mapping of CSV data to objects, advanced parsing options, and error handling mechanisms. By utilizing these libraries, developers can save time and effort in writing and maintaining custom CSV parsing code.
Handling Large CSV Files and Optimizing Performance:
Working with large CSV files can be challenging due to memory constraints and performance considerations. To handle large CSV files efficiently, buffering and streaming techniques can be employed. Instead of loading the entire file into memory, data can be read in smaller chunks. Additionally, parallel processing can be utilized to distribute the workload and improve performance.
Handling Inconsistencies and Errors in CSV File Data:
CSV files are prone to data inconsistencies and errors, such as missing or unexpected columns. To handle such issues, data validation, data cleansing, and error handling strategies can be implemented. Techniques like checking the number of columns, validating the data types, and handling missing or malformed entries can help ensure the integrity and reliability of the CSV data.
FAQs:
1. How can I read a CSV file in C# using the built-in File IO classes?
To read a CSV file using the built-in File IO classes, you can instantiate a StreamReader object and use its ReadLine method to read each line of the file. Within each line, you can split the values using the appropriate delimiter and process them accordingly.
2. What are some popular third-party CSV parsing libraries in C#?
Some popular third-party CSV parsing libraries in C# include CsvHelper, CsvHelper.Light, and FileHelpers. These libraries provide rich features, performance optimizations, and error-handling mechanisms for reading and manipulating CSV files.
3. How can I optimize the performance when reading large CSV files in C#?
To optimize performance when reading large CSV files in C#, you can use techniques like buffering, streaming, and parallel processing. By reading data in smaller chunks, processing it asynchronously, and utilizing multiple threads, you can enhance the overall performance and reduce memory consumption.
4. What should I do if my CSV file has inconsistent or missing data?
If your CSV file has inconsistent or missing data, you can implement data validation checks to ensure the integrity of the data. Techniques like checking the number of columns, validating data types, and handling missing or malformed entries can help in handling such inconsistencies and errors.
Conclusion:
Reading CSV files in C# is a fundamental task for data processing and analysis. By understanding the structure of CSV files, choosing the appropriate method for reading, utilizing libraries or built-in File IO classes, optimizing performance, and handling inconsistencies, developers can efficiently work with CSV data in their C# applications. By following best practices and leveraging available resources, they can ensure accurate and reliable data processing while maintaining code simplicity and efficiency.
C Programming Tutorial – How To Read Csv File
How To Read A Csv File In C?
CSV (Comma Separated Values) is a common file format used to store tabular data, where each line represents a row and each field within a row is separated by a comma. Being able to read and parse CSV files is a crucial skill for many C developers, as it allows them to easily access and manipulate data stored in this format. In this article, we will explore the process of reading a CSV file using the C programming language, providing a step-by-step guide to help you master this essential task.
Step 1: Include the Necessary Libraries
Before we can start reading a CSV file, we need to include the necessary libraries in our C program. The most important library required for CSV file operations is `
Step 2: Open the CSV File
To read a CSV file, we first need to open it. This can be done using the `fopen()` function, which takes two arguments: the name of the file to open and the mode in which it should be opened. In our case, we want to open the file in read-only mode, so we use “r” as the mode argument. Here’s an example code snippet to open a CSV file named “data.csv”:
“`c
FILE* file = fopen(“data.csv”, “r”);
if (file == NULL) {
printf(“Failed to open the CSV file.”);
return -1;
}
“`
Step 3: Read the CSV File Line by Line
Once the file is open, we can read its contents line by line. We will use the `fgets()` function to read each line from the file. This function takes three arguments: the character array where the line will be stored, the maximum number of characters to read, and the file pointer. Here’s an example code snippet to read lines from the CSV file:
“`c
char line[256];
while (fgets(line, sizeof(line), file)) {
// Process the line
}
“`
Step 4: Parse Each Line into Fields
Now that we are reading lines from the CSV file, our next step is to parse each line into separate fields. In CSV files, fields are separated by commas. To split the line into fields, we can use the `strtok()` function from the `
“`c
char* field = strtok(line, “,”);
while (field) {
// Process the field
field = strtok(NULL, “,”);
}
“`
Step 5: Store and Process the Field Data
Once we have parsed the line into individual fields, we can store and process the field data according to our requirements. For example, we can store the field data in an array or structure to access it later. Here’s an example code snippet to store and process the field data:
“`c
typedef struct {
int id;
char name[50];
float score;
} Student;
Student students[100];
int numberOfStudents = 0;
// Inside the field processing loop
students[numberOfStudents].id = atoi(field);
strcpy(students[numberOfStudents].name, field);
students[numberOfStudents].score = atof(field);
numberOfStudents++;
“`
Step 6: Close the File
Once we have finished reading and processing the CSV file, it is important to close it to release system resources. This can be done using the `fclose()` function, which takes the file pointer as an argument. Here’s an example code snippet to close the CSV file:
“`c
fclose(file);
“`
Frequently Asked Questions (FAQs):
Q1: Can I read a CSV file with a different delimiter instead of a comma?
A1: Absolutely. The `strtok()` function can be used with any delimiter character you specify. Simply replace the comma in the function call with your desired delimiter.
Q2: How do I handle CSV files with headers?
A2: If your CSV file includes a header line, you can skip it by adding an initial `fgets()` call before the loop that reads the file line by line.
Q3: How do I handle CSV fields with quotes or special characters?
A3: If your CSV file contains fields with quotes or special characters, you may need to use additional parsing techniques to handle them correctly. One approach is to read the entire line as a string and then process it according to the CSV parsing rules.
Q4: What happens if a field is empty in a CSV file?
A4: If a field is empty in a CSV file, the corresponding field will be represented as an empty string. You can check for empty fields using string comparison functions like `strcmp()`.
Conclusion:
Reading and parsing CSV files is an essential skill for C developers working with tabular data. By following the step-by-step guide provided in this article, you can easily read and process CSV files in your C programs. Remember to close the file once you have finished reading it to ensure proper resource management. With this newfound knowledge, you can confidently handle CSV files in your C programming endeavors.
How To Read Csv File In Programming?
CSV (comma-separated values) files are widely employed in programming for data storage and exchange due to their simplicity and compatibility with a variety of applications. In this article, we will delve into the details of reading CSV files in programming languages, exploring different techniques and libraries for efficient data extraction. Whether you are a seasoned programmer or a beginner, understanding how to read CSV files is essential for working with data effectively.
Table of Contents:
1. Introduction to CSV Files
2. Manual Parsing
3. Built-in Libraries and Modules
4. CSV Parsing Libraries
5. Common Challenges and Solutions
6. FAQs
1. Introduction to CSV Files:
CSV files consist of plain text data where each line represents a row, and each field within a row is separated by a delimiter, typically a comma. While commas are widely used as delimiters, other characters like tabs, semicolons, or even custom characters can be employed. CSV files are often used for storing tabular data, making it easily readable by both humans and machines.
2. Manual Parsing:
For simple CSV files, manual parsing can be performed without relying on external libraries. The process involves opening the file, reading line by line, and splitting each line to extract the fields. Let’s consider a basic example using Python:
“`python
import csv
with open(‘data.csv’, ‘r’) as file:
for line in file:
fields = line.strip().split(‘,’) # Split line by comma
# Process the extracted fields as required
“`
However, manual parsing may be tedious for complex CSV files, as it does not handle special cases like quoted fields or escaped delimiters. These scenarios call for the use of libraries and modules specifically designed for CSV parsing.
3. Built-in Libraries and Modules:
Many programming languages provide built-in libraries or modules for CSV parsing, simplifying the extraction process. For instance, Python’s `csv` module offers easy-to-use functions for parsing CSV files. The example below demonstrates the usage of the `csv` module:
“`python
import csv
with open(‘data.csv’, ‘r’) as file:
csv_reader = csv.reader(file)
for row in csv_reader:
# Access fields in the row as a list
# Process the extracted fields as required
“`
The built-in libraries typically handle edge cases like quoted fields or escaped delimiters, ensuring accurate parsing of complex CSV structures. Other languages, such as Java, also provide similar libraries that simplify CSV parsing operations.
4. CSV Parsing Libraries:
Apart from built-in functions, several programming languages offer powerful third-party libraries specifically designed for CSV parsing. These libraries often provide additional features and functionalities, facilitating more robust data extraction. Some popular CSV parsing libraries include:
4.1. Python:
– `pandas`: A powerful library for data manipulation, it can read CSV files and provide advanced features like filtering, sorting, and statistics.
– `numpy`: Often used in combination with `pandas`, it provides efficient data storage and manipulation capabilities.
4.2. Java:
– `OpenCSV`: A feature-rich library providing flexible CSV parsing and writing capabilities.
– `Apache Commons CSV`: A trusted library offering easy-to-use CSV parsing functionalities with comprehensive error handling.
4.3. R:
– `readr`: An efficient library designed for reading CSV files swiftly.
– `data.table`: Offers high-performance data manipulation functions and can read large CSV files efficiently.
5. Common Challenges and Solutions:
When working with CSV files, it is crucial to be aware of common challenges one may face during parsing. Here are a few challenges and their corresponding solutions:
5.1. Handling Quotes and Escaped Delimiters:
Quoted fields and escaped delimiters within fields can complicate the process of parsing CSV files. Specialized CSV libraries typically handle these cases effortlessly, ensuring accurate field extraction.
5.2. Handling Large CSV Files:
For large CSV files, reading the entire file into memory may not be feasible due to memory constraints. In such cases, streaming-based parsing techniques can be employed, where only a portion of the file is read at a time. This allows processing of large files without overwhelming system resources.
5.3. Character Encoding Issues:
CSV files may contain non-ASCII characters, which could result in encoding-related complications. It is essential to specify the appropriate character encoding while reading the CSV file to ensure data integrity.
6. FAQs:
Q1. Can CSV files contain multiple sheets like Excel files?
A1. No, CSV files do not support multiple sheets. Each CSV file represents a single sheet or table.
Q2. Are CSV files suitable for storing complex data structures like hierarchical data?
A2. CSV files are primarily suited for tabular data storage and are not designed for hierarchical structures. Other formats like JSON or XML are better suited for such scenarios.
Q3. Can CSV files contain data types other than plain text?
A3. By design, CSV files store data as plain text. When specific data types are required, appropriate conversions must be performed during the parsing process.
In conclusion, reading CSV files is a fundamental skill for efficient data handling in programming. Understanding the intricacies of CSV parsing and utilizing appropriate libraries can save substantial time and effort, especially when dealing with complex and large datasets. By following the techniques outlined in this article, you can confidently read and extract data from CSV files, empowering you to analyze and process data effectively.
Keywords searched by users: reading csv file c# Read file CSV in C, Read CSV file C++, C++ read csv file into array, Read CSV file C#, Read excel file in c, Read integer from file in C, C read file line by line, Read file in C
Categories: Top 99 Reading Csv File C#
See more here: nhanvietluanvan.com
Read File Csv In C
CSV (Comma Separated Values) files are widely used for data storage and exchange due to their simplicity and compatibility across different applications. Reading CSV files in C is a common task for developers working on data analysis, data manipulation, and various other programming tasks. In this article, we will explore the process of reading CSV files in C, providing a comprehensive guide for beginners as well as a few advanced concepts.
Understanding CSV File Format
Before diving into the code, it’s crucial to have a good understanding of the CSV file format. A CSV file consists of plain text data where each line represents a record, and individual values within a record are separated by commas. The first line often serves as a header, containing column names. Let’s consider an example CSV file named “employees.csv”:
“`csv
name,age,designation
John Doe,32,Manager
Jane Smith,28,Engineer
“`
In this example, the CSV file has three columns: name, age, and designation. Each subsequent line represents a record with corresponding values.
Reading CSV Files in C
To read a CSV file in C, we need to utilize a file handling mechanism provided by the standard C library. Here’s a step-by-step guide on how to read a CSV file in C:
1. Open the file: Start by opening the CSV file using the `fopen()` function. Ensure that the file exists and can be accessed in the desired mode (e.g., read-only).
2. Read line by line: While there are still lines left to read, use the `fgets()` function to read each line from the file and store it in a string variable.
3. Parse each line: To extract individual values from the read line, we can use the `strtok()` function along with the delimiter character. In the case of CSV files, the delimiter would be a comma.
4. Store the values: Once we have obtained the individual values, we can store them in appropriate variables or data structures, such as arrays or structs.
5. Process the data: Now that the data is stored, you can perform various operations, apply conditions, or utilize the data as required by your program.
6. Close the file: Once all the required data has been processed, don’t forget to close the file using the `fclose()` function.
FAQs:
Q: How do I check if a CSV file exists before reading it?
A: Before opening the file, you can use the `access()` function to check if the file exists and can be accessed. This function returns 0 if the file exists; otherwise, it returns -1.
Q: How do I handle errors while reading CSV files?
A: During file operations, errors can occur due to various reasons, such as the file not existing, insufficient permissions, or incorrect file format. To handle such errors, you can check the return values of functions like `fopen()` and `fgets()`. Additionally, you can use the `errno` variable to get detailed error information.
Q: How can I handle CSV files with different delimiters?
A: While the CSV file format primarily uses commas as delimiters, it’s possible to encounter files with different delimiters, such as tabs or semicolons. To handle such cases, you can modify the delimiter passed to the `strtok()` function accordingly.
Q: What if my CSV file contains values with commas?
A: If a CSV file contains values with commas (e.g., “Last Name, First Name”), it is common to enclose such values within quotation marks (“”). While parsing the values, you should accommodate this by ignoring commas between quotation marks or using specialized CSV parsing libraries.
Q: Are there any libraries available for reading CSV files in C?
A: Yes, there are several open-source libraries available, such as libcsv and csvkit, which provide higher-level functionality for reading CSV files in C. Utilizing these libraries can simplify the process and make it more robust.
In Conclusion
Reading CSV files in C requires understanding the file format and utilizing file handling mechanisms provided by the standard C library. By following the steps outlined in this article, you can effectively read CSV files, extract data, and perform various operations using the read data. Additionally, we discussed some frequently asked questions to address common concerns and provide further clarity on the topic. With this knowledge, you can now confidently work with CSV files in your C programs.
Read Csv File C++
CSV (Comma-Separated Values) files are a popular and widely-used format for storing and exchanging tabular data. Reading CSV files in C++ can be an essential skill for developers who need to extract information from raw data files. In this article, we will explore various methods to read CSV files using the C++ programming language and provide a thorough understanding of this process.
Table of Contents:
1. What is a CSV file?
2. Why read CSV files in C++?
3. Options to Read CSV Files in C++
a. Standard Input/Output Library
b. Third-Party Libraries
4. Reading CSV Files Using Standard Input/Output Library
a. File Stream Configuration
b. Parsing CSV Data
c. Handling Different Delimiters or Enclosing Characters
5. Reading CSV Files Using Third-Party Libraries
a. Boost C++ Libraries
b. OpenCSV C++ Library
6. Frequently Asked Questions (FAQs)
7. Conclusion
1. What is a CSV file?
A CSV file is a plain text file that typically represents tabular data, where each line of the file corresponds to a row, and the values within each line are separated by commas. CSV files allow exporting or importing large amounts of data from one program to another, making them convenient for data storage, manipulation, and transfer.
2. Why read CSV files in C++?
In many real-world scenarios, developers often encounter the need to read CSV files in their applications. By reading CSV files, developers can extract data for further processing, perform analysis, generate reports, and integrate with databases or other systems. Hence, having the ability to read and process CSV files efficiently is invaluable.
3. Options to Read CSV Files in C++
There are various ways to read CSV files in C++. One can either use the Standard Input/Output Library, which comes with C++, or choose from multiple third-party libraries available. Let’s explore both options.
a. Standard Input/Output Library:
The Standard Input/Output Library provides functionalities to work with files, including reading and writing data. While not specifically designed for CSV files, it offers sufficient utilities to handle CSV data.
b. Third-Party Libraries:
Various third-party libraries have been developed to simplify CSV file operations. These libraries often provide robust features, error handling mechanisms, and support for different CSV variations.
4. Reading CSV Files Using Standard Input/Output Library
a. File Stream Configuration:
To read a CSV file using the standard library, one needs to configure a file stream object and open the file in read mode. This can be achieved using the `ifstream` class, which provides input operations on files.
b. Parsing CSV Data:
Once the file is opened, developers can read the CSV data line by line using the `getline()` function and parse each line further to extract individual values using a delimiter (typically a comma). Various techniques, such as splitting strings or using regular expressions, can be employed to extract the values.
c. Handling Different Delimiters or Enclosing Characters:
CSV files may have different delimiters or enclosing characters, such as tabs, semicolons, quotes, or double quotes. To handle such variations, one needs to customize the parsing logic accordingly, ensuring that values are correctly extracted.
5. Reading CSV Files Using Third-Party Libraries
a. Boost C++ Libraries:
The Boost C++ Libraries offer several components for handling CSV data, including the Tokenizer and Spirit libraries. These libraries provide advanced parsing and tokenizing capabilities, making it easier to work with CSV files. However, integrating Boost libraries into your project might require additional setup and configuration.
b. OpenCSV C++ Library:
OpenCSV C++ is another popular third-party library that simplifies CSV file handling. It provides a high-level API and supports various CSV configurations, such as different separators, line endings, enclosures, and more. OpenCSV C++ makes reading and writing CSV files effortless and offers comprehensive error handling mechanisms.
6. Frequently Asked Questions (FAQs)
Q1. Can I read large CSV files using the Standard Input/Output Library?
A1. Yes, the Standard Input/Output Library can handle large CSV files efficiently by reading and processing data line by line, minimizing memory usage.
Q2. Which is the best library to read CSV files in C++?
A2. The choice of the library depends on the specific project requirements. While the Standard Input/Output Library suffices for simple CSV files, libraries like Boost or OpenCSV C++ provide advanced features and broader support for different CSV configurations.
Q3. Do third-party CSV libraries offer error handling capabilities?
A3. Yes, third-party libraries like OpenCSV C++ often have robust error handling mechanisms in place. They provide informative error messages and exceptions to handle scenarios such as missing columns, invalid data, or file corruption.
7. Conclusion
Reading CSV files in C++ is an essential skill for extracting and processing data efficiently. This article explored different methods to read CSV files, including using the Standard Input/Output Library and third-party libraries like Boost C++ and OpenCSV C++. By choosing the appropriate approach, developers can perform complex CSV file operations with ease, ensuring accurate data extraction and manipulation.
C++ Read Csv File Into Array
## Reading CSV Files into Arrays
There are multiple ways to read CSV files into arrays in C++. We will discuss two popular methods: using the `fstream` library and using third-party libraries such as `Boost` and `Pandas`.
### Using the `fstream` Library
The `fstream` library provides classes for file input/output operations in C++. To read a CSV file using `fstream`, you can follow these steps:
1. Include the necessary header files:
“`cpp
#include
#include
#include
#include
“`
2. Declare a `std::ifstream` object to represent the input file stream:
“`cpp
std::ifstream file(“data.csv”);
“`
3. Check if the file is open:
“`cpp
if (file.is_open()) {
// File is open, proceed with reading
} else {
std::cout << "Failed to open file." << std::endl;
return -1;
}
```
4. Declare a container, such as a `std::vector`, to hold the data from the CSV file:
```cpp
std::vector
“`
5. Read the file line by line and split each line into tokens using a delimiter (usually a comma for CSV files):
“`cpp
std::string line;
while (std::getline(file, line)) {
std::vector
std::istringstream tokenStream(line);
std::string token;
while (std::getline(tokenStream, token, ‘,’)) {
// Add token to the vector of tokens
tokens.push_back(token);
}
// Add the vector of tokens to the data container
data.push_back(tokens);
}
“`
### Using Third-Party Libraries
Another approach to read CSV files into arrays is by using third-party libraries, such as `Boost` or `Pandas`.
`Boost` is a set of high-quality libraries that extend the functionality of C++. To read a CSV file using `Boost`, you need to follow these steps:
1. Install `Boost` if it’s not already installed.
2. Include the necessary `Boost` header file:
“`cpp
#include
“`
3. Declare a container, such as a `std::vector`, to hold the data from the CSV file:
“`cpp
std::vector
“`
4. Read the file line by line and split each line into tokens using `boost::algorithm::split` with the delimiter as a comma:
“`cpp
std::string line;
while (std::getline(file, line)) {
std::vector
boost::split(tokens, line, boost::is_any_of(“,”));
// Add the vector of tokens to the data container
data.push_back(tokens);
}
“`
`Pandas` is a powerful data manipulation library for Python. To read a CSV file using `Pandas`, you can follow these steps:
1. Install `Pandas` if it’s not already installed.
2. Import the `Pandas` library:
“`cpp
#include
“`
3. Declare a container, such as a `std::vector
“`cpp
std::vector
“`
4. Execute Python code to read the CSV file using `Pandas` and convert the data into a C++ vector:
“`cpp
py::scoped_interpreter guard{};
py::module pandas = py::module::import(“pandas”);
py::object dataframe = pandas.attr(“read_csv”)(“data.csv”);
data = py::list(dataframe);
“`
### FAQs
**Q: Can I handle CSV files with different delimiters using these methods?**
A: Yes, you can change the delimiter used to split the lines in the code. For example, if your CSV file uses tabs as delimiters, you can replace the comma delimiter in the code with a tab character.
**Q: Can I read only specific columns from a CSV file using these methods?**
A: Yes, you can modify the code to read only the desired columns by extracting or skipping certain tokens within the line splitting loop. For example, if you want to read only the first and third columns, you can add a condition to store tokens at specific indices.
**Q: What should I do if my CSV file contains quoted values or special characters?**
A: If your CSV file contains quoted values or special characters, additional handling may be required. For instance, you might need to implement logic to handle escaped quotes or use specialized CSV parsing libraries that handle complex cases.
**Q: Can I handle large CSV files using these methods?**
A: When dealing with large CSV files, memory efficiency becomes crucial. In such cases, it is recommended to process the file line by line instead of storing the entire contents in memory. You can perform operations on each line as required and discard the processed data to free up memory.
Reading CSV files into arrays is an essential skill when working with data in C++. By following the approaches mentioned above, you can efficiently import the data from CSV files for further processing, analysis, or manipulation.
Images related to the topic reading csv file c#
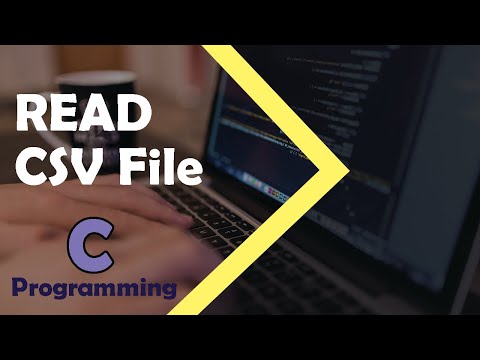
Article link: reading csv file c#.
Learn more about the topic reading csv file c#.
- parsing – Read .csv file in C – Stack Overflow
- Reading CSV files in C ::
- Relational Database from CSV Files in C – GeeksforGeeks
- How To Read A CSV File In Python – Earthly Blog
- How to write to a CSV file in C – DEV Community
- C# Read CSV File Tutorial (Without Using Interop) | IronXL – Iron Software
- Relational Database from CSV Files in C – GeeksforGeeks
- Reading and displaying each line from a CSV file in … – Plus2net
- Read data from a .csv file in c – C Board
- How to Read a CSV File in C – Techwalla
See more: https://nhanvietluanvan.com/luat-hoc