Reading A Csv File In C#
CSV (Comma-Separated Values) is a widely used format for storing and exchanging tabular data. It is popular among data analysts, developers, and business professionals due to its simplicity and compatibility with various software applications. In this article, we will explore how to read a CSV file in C# programming language and process its contents.
Step 1: Adding necessary namespaces and libraries
To read a CSV file in C#, you need to include the following namespaces and libraries:
“`csharp
using System;
using System.IO;
“`
The `System` namespace provides core functionality, while the `System.IO` namespace contains classes for working with input and output operations.
Step 2: Creating a StreamReader object
Next, you need to create a `StreamReader` object. This class provides methods for reading characters from a stream, such as a file. Here’s how you can create a `StreamReader` object:
“`csharp
StreamReader reader = new StreamReader(“path/to/your/csv/file.csv”);
“`
Replace `”path/to/your/csv/file.csv”` with the actual path to your CSV file.
Step 3: Opening the CSV file
To start reading the CSV file, you need to open it. You can do this by calling the `Open()` method of the `StreamReader` object. Here’s the code for opening the CSV file:
“`csharp
reader.Open();
“`
Step 4: Reading the CSV file line by line
Once the CSV file is open, you can start reading its contents line by line. This involves iterating over each line, extracting the necessary information, and processing it according to your requirements. Here’s an example of how you can read the CSV file line by line:
“`csharp
string line;
while ((line = reader.ReadLine()) != null)
{
// Process the line here
}
“`
The `ReadLine()` method reads the next line from the CSV file and returns it as a string. The loop continues until there are no more lines to read.
Step 5: Parsing and processing the data
Inside the loop, you can parse and process the data from each line according to your needs. By default, the values in a CSV file are separated by commas. You can split the line into individual values using the `Split()` method and process them as required. Here’s an example of how you can parse and process the data:
“`csharp
string[] values = line.Split(‘,’);
foreach (string value in values)
{
// Process each value here
}
“`
In this example, we split the line using a comma as the separator and assign the resulting values to an array called `values`. Then, we iterate over each value and process it as needed.
FAQs
Q: Can I read a CSV file in C without using any libraries?
A: Yes, it is possible to read a CSV file in C without using any additional libraries. However, it can be more cumbersome and time-consuming compared to using libraries like `StreamReader`.
Q: How can I read an Excel file in C#?
A: To read an Excel file in C#, you can use libraries like `EPPlus` or `Microsoft.Office.Interop.Excel`. These libraries provide methods and classes for reading and manipulating Excel files.
Q: Is it possible to read a file line by line in C?
A: Yes, it is possible to read a file line by line in C. You can use the `fscanf()` function or the `fgets()` function to read each line from the file.
Q: Can I read a CSV file in C++?
A: Yes, you can read a CSV file in C++ as well. You can use libraries like `fstream`, `stringstream`, or external libraries like `CSVParser` to parse and process the CSV data.
In conclusion, reading a CSV file in C# is a straightforward process that involves adding the necessary namespaces, creating a `StreamReader` object, opening the CSV file, reading it line by line, and parsing and processing the data. By following these steps, you can easily import and work with CSV data in your C# applications.
Keywords: Read file CSV in C, Read excel file in c, Read file in C, C read file line by line, File in C, Read CSV file C++, Fscanf() in C, C++ read csv file into arrayreading a csv file in c#
C Programming Tutorial – How To Read Csv File
How To Read A Csv File In C?
CSV (Comma Separated Values) is a popular file format used to store tabular data. It consists of plain text data which is organized into rows and columns, with each column separated by a comma. Reading data from a CSV file is a common task in many applications, and in this article, we will explore how to read a CSV file in the C programming language.
Before diving into the implementation, let’s discuss the steps involved in reading a CSV file:
1. Open the CSV file: C provides several file operations, including fopen(), which allows you to open a file. In this case, we need to provide the file path and the mode. To read from a file, we use the “r” mode.
2. Read the file line by line: Once the file is open, we need to read its contents line by line. C provides fgets() function that reads a line from the file and stores it in a buffer.
3. Tokenize the line: Since the data in a CSV file is separated by commas, we need to split each line into separate tokens. The strtok() function can be used to achieve this task. By specifying the comma as the delimiter, the function will return successive tokens.
4. Process the tokens: Once we have obtained the tokens, we can process them as required. For example, we may need to store the data in variables or perform calculations based on the data.
5. Close the file: After reading the entire file or when we have completed the necessary processing, it is good practice to close the file using the fclose() function.
Now, let’s explore the implementation of reading a CSV file in C:
“`c
#include
#include
#define MAX_LENGTH 100
#define MAX_COLUMNS 10
int main() {
FILE *file;
char line[MAX_LENGTH];
char *token;
char *tokens[MAX_COLUMNS];
int columnCount = 0;
file = fopen(“data.csv”, “r”);
if (file == NULL) {
printf(“Failed to open the file.\n”);
return 1;
}
while (fgets(line, sizeof(line), file)) {
columnCount = 0;
token = strtok(line, “,”);
while (token != NULL && columnCount < MAX_COLUMNS) {
tokens[columnCount] = token;
token = strtok(NULL, ",");
columnCount++;
}
// Process the tokens as required
for (int i = 0; i < columnCount; i++) {
printf("%s ", tokens[i]);
}
printf("\n");
}
fclose(file);
return 0;
}
```
In the above code, we open a file named "data.csv" in read mode. If the file fails to open, an error message is displayed, and the program terminates with a non-zero exit code. We then read the file line by line using fgets() and tokenize each line using strtok(). The resulting tokens are stored in the tokens array.
Next, we can process the tokens in any desired way. In the provided example, we simply print each token on a new line. However, you can modify this section to suit your specific needs, such as storing the data in variables or performing calculations.
Finally, we close the file using fclose(). This is crucial to ensure that system resources are properly released.
FAQs:
Q: Can I read a CSV file with a different delimiter?
A: Yes, the code can be modified to handle different delimiters. Simply replace the comma (",") with the desired delimiter in the strtok() function.
Q: How can I handle CSV files with varying column counts?
A: The code provided assumes a maximum of 10 columns. If the number of columns in your CSV file can vary, you can dynamically allocate memory for the tokens array based on the actual column count.
Q: Is it possible to skip the first line/header of the CSV file?
A: Yes, you can modify the code to read and ignore the first line before entering the while loop. To do this, use fgets() once before the loop.
Q: What happens if a token contains a comma within quotes?
A: The provided code does not handle this scenario as it assumes the data in the CSV file doesn't contain quoted commas. If your CSV file contains quoted fields, additional processing will be required to handle them correctly.
Q: Can this code be used to write data to a CSV file as well?
A: No, this code only demonstrates how to read data from a CSV file. To write data to a CSV file, you would need to use different functions like fprintf() or fputs().
In conclusion, reading a CSV file in C involves opening the file, reading it line by line, tokenizing each line, processing the tokens, and finally closing the file. By following the steps and using the provided code as a starting point, you'll be able to read and process CSV files in your C programs efficiently.
How To Read Csv File In Programming?
CSV stands for Comma Separated Values, which is a popular file format used to store tabular data, such as spreadsheets and databases. Being a widely used data exchange format, it is crucial for programmers to know how to read CSV files and extract the required data for their applications. In this article, we will explore various programming languages and libraries that support CSV file reading, and delve into the different methods and considerations involved. Let’s jump right in!
## Reading CSV Files Using Python
Python, a popular programming language for data analysis and scientific computing, provides a built-in CSV module that simplifies the process of reading CSV files. Here’s an example of how to read a CSV file using Python:
“`python
import csv
# Open the CSV file
with open(‘data.csv’, ‘r’) as file:
# Create a CSV reader object
reader = csv.reader(file)
# Iterate over each row in the CSV file
for row in reader:
# Access each cell in the row
for cell in row:
print(cell)
“`
In the above code snippet, we start by importing the CSV module. We then open the CSV file using the `open()` function and specify the desired file mode (in this case, ‘r’ for read mode). Next, we create a CSV reader object and iterate over each row in the file using a for loop. Finally, we print each cell value within the row.
## Reading CSV Files Using Java
For Java developers, reading and parsing CSV files can be accomplished using libraries such as OpenCSV or Commons CSV. Here’s an example using OpenCSV:
“`java
import com.opencsv.CSVReader;
import java.io.FileReader;
import java.io.IOException;
public class CSVReaderExample {
public static void main(String[] args) {
try {
// Create a CSV reader object
CSVReader reader = new CSVReader(new FileReader(“data.csv”));
String[] nextLine;
// Read each line until the end of file
while ((nextLine = reader.readNext()) != null) {
// Access each cell in the line
for (String cell : nextLine) {
System.out.println(cell);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
“`
In the above Java code, we import the OpenCSV library and create a CSV reader object. We then specify the CSV file to be read and use a while loop to iterate over each line in the file. Within the loop, we access each cell in the line and print its value.
## Reading CSV Files Using R
R, a programming language commonly used for statistical computing, also provides functionalities for reading CSV files. The `read.csv()` function in R allows us to easily import data from a CSV file into a dataframe. Here’s an example:
“`R
# Read the CSV file into a dataframe
data <- read.csv("data.csv")
# Access the values in the dataframe
for (i in 1:nrow(data)) {
for (j in 1:ncol(data)) {
print(data[i, j])
}
}
```
In this R code, we use the `read.csv()` function to read the CSV file into a dataframe called `data`. We then use nested for loops to iterate over each row and column of the dataframe and access its values.
## FAQs
Q: What if my CSV file contains headers?
A: If your CSV file contains headers, you can skip the first row while iterating over the file to avoid treating the headers as data.
Q: What if my CSV file contains special characters or custom delimiters?
A: When reading a CSV file, you can specify the delimiter used in the file. By default, Python's `csv.reader` assumes a comma as the delimiter, while OpenCSV uses a comma as well. However, you can customize the delimiter in both libraries, depending on your file's format.
Q: Can I read large CSV files efficiently?
A: Yes, some CSV libraries (such as pandas in Python) provide efficient methods for reading and processing large CSV files. They often load the data in memory in chunks rather than all at once, which improves performance and reduces memory usage.
Q: Are there any other file formats commonly used for tabular data?
A: Yes, other file formats include Excel (.xls, .xlsx), JSON, and SQLite databases. However, CSV remains widely used due to its simplicity and compatibility across various platforms.
In conclusion, knowing how to read CSV files is an essential skill for programmers. With the examples and explanations provided above, you should now have a solid understanding of how to read CSV files using Python, Java, and R. Remember to consider your specific requirements, such as header presence and custom delimiters, when implementing CSV file reading in your programming projects.
Keywords searched by users: reading a csv file in c# Read file CSV in C, Read excel file in c, Read file in C, C read file line by line, File in C, Read CSV file C++, Fscanf() in C, C++ read csv file into array
Categories: Top 13 Reading A Csv File In C#
See more here: nhanvietluanvan.com
Read File Csv In C
CSV (Comma Separated Values) files are widely used in data exchange as they provide a simple and efficient way to store tabular data. Reading a CSV file in C is a fundamental skill that every programmer should possess. In this article, we will delve into the intricacies of reading CSV files in C, exploring various techniques and providing practical examples. So, let’s begin our journey into the world of reading file CSV in C.
Understanding CSV Files:
Before we get into the nitty-gritty of reading CSV files, it is essential to understand their structure. CSV files consist of rows and columns, where each row represents a record, and each column represents a field within that record. The values within the columns are separated by commas, which serve as delimiters.
Reading CSV Files in C:
To read a CSV file, we need to follow a series of steps, including opening the file, parsing the data, and storing it in appropriate variables or data structures. Let’s explore these steps in detail.
Step 1: Opening the CSV File:
To read a CSV file, we must first open the file using the `fopen()` function. This function takes two arguments: the name of the file to be opened and the mode in which it should be opened. In our case, we will use the mode “r” (read mode). Here’s an example of opening a CSV file named “data.csv”:
“`c
FILE* file = fopen(“data.csv”, “r”);
“`
Step 2: Reading the Contents of the CSV File:
Once the file is open, we need to read its contents line by line using the `fgets()` function. This function reads a line from the file and stores it in a character array. Here’s an example illustrating how to read the contents of a CSV file line by line:
“`c
char line[100]; // Assume maximum line length of 100 characters
while (fgets(line, sizeof(line), file) != NULL) {
// Process the line here
}
“`
Step 3: Parsing and Storing Data:
Once we have read a line from the CSV file, our next step is to parse the line and extract the values. Since the values in a CSV file are separated by commas, we can use the `strtok()` function to tokenize the line based on the comma delimiter. Here’s an example demonstrating how to parse a line of CSV data:
“`c
char* token = strtok(line, “,”); // Tokenizing with comma as delimiter
while (token != NULL) {
// Process the token here
token = strtok(NULL, “,”);
}
“`
Step 4: Handling Parsed Data:
After parsing a line and extracting the values, we can either store them in variables or utilize data structures like arrays and structs to store the data for further processing. The choice of data structure depends on the complexity and requirements of your application.
Frequently Asked Questions (FAQs):
Q1: Are there any libraries available in C for reading CSV files?
A1: While C does not have built-in libraries specifically dedicated to CSV file parsing, many open-source libraries, such as libcsv and libcsv-datatype, provide robust solutions for reading and manipulating CSV files in C.
Q2: How can I handle CSV files with varying column counts?
A2: CSV files with varying column counts can pose a challenge. One approach is to read the CSV file line by line and dynamically allocate memory to store each row’s values. Another option is to use a flexible data structure like linked lists to accommodate varying column counts.
Q3: How can I handle different data types in CSV files?
A3: CSV files usually store values as strings. To handle different data types, you need to convert the string values to the desired data types explicitly. Functions like `atoi()`, `atof()`, and `sscanf()` can be used for converting string values to integers, floating-point numbers, and other data types in C.
Q4: How can I handle CSV files with quoted values containing commas?
A4: When dealing with CSV files that contain quoted values containing commas, you need to implement a more sophisticated parsing algorithm. One approach is to tokenize the line using a CSV parsing library that supports quoted values, such as libcsv.
Q5: What precautions should I take while reading large CSV files?
A5: Reading large CSV files can consume a significant amount of memory. To mitigate this, consider processing the file line by line instead of loading the entire file into memory. Additionally, optimize your code for efficiency and consider using appropriate data structures and algorithms to handle large datasets efficiently.
In conclusion, reading CSV files in C is an essential skill for working with tabular data. By following the steps outlined in this article, you can effectively read CSV files, parse their contents, and handle various scenarios. Remember to utilize libraries like libcsv to simplify the parsing process and optimize your code for efficiency. Happy coding!
Read Excel File In C
Microsoft Excel is an immensely popular and widely used spreadsheet program that allows users to organize, analyze, and present data. While Excel offers a range of functionalities, it also provides support for exporting and importing data to and from external sources. In this article, we will explore how to read an Excel file in the C programming language. We will cover various methods, libraries, and approaches for achieving this goal, enabling developers to efficiently work with Excel data in their C applications.
Methods for Reading Excel Files in C
1. Using the OpenXML SDK:
The OpenXML SDK is a powerful library that provides functionality for creating, modifying, and parsing Excel documents. It enables developers to read, write, and manipulate Office Open XML files, including Excel spreadsheets. To read an Excel file using OpenXML SDK in C, you need to include the OpenXML headers and utilize the provided APIs to access and retrieve data from the spreadsheet.
2. Utilizing the Apache POI library:
Apache POI (Poor Obfuscation Implementation) is a popular Java library that allows developers to work with various Microsoft Office file formats, including Excel. It also provides support for the C programming language through wrapper libraries such as “poi-c” or “poi-lite.” These libraries can be used to read Excel files in C by loading the workbook and accessing the desired cells, rows, or columns.
3. Employing the LibXL library:
LibXL is a feature-rich library designed specifically for reading and writing Excel files from C and C++. It supports both the older .xls format and the newer .xlsx format. By including the LibXL headers and utilizing the provided functions, you can easily read and manipulate Excel files in your C applications. This library offers various methods for accessing and retrieving data, including fetching cell values and iterating through rows and columns.
4. Parsing CSV files exported from Excel:
Excel files can also be exported as CSV (Comma-Separated Values) files, which contain tabular data separated by commas. Reading CSV files in C is comparatively simpler than reading Excel files directly. By using functions like `fopen()`, `fscanf()`, and `fclose()`, you can open the CSV file, extract data from each line, and store it in the desired data structure.
FAQs
Q: Can I read an Excel file in C without using any external libraries?
A: While it is technically possible to parse an Excel file manually in C, it is a complex task and requires an in-depth understanding of the Excel file format. Therefore, it is recommended to use external libraries that provide dedicated functionality for reading Excel files, such as the OpenXML SDK, Apache POI, or LibXL.
Q: Which library is the best for reading Excel files in C?
A: The best library for reading Excel files in C depends on your specific requirements. If you prefer an extensive set of features and support for both .xls and .xlsx formats, LibXL is a great choice. If you are already familiar with the Apache POI library or prefer Java-based libraries, you can use the “poi-c” or “poi-lite” wrappers. Lastly, the OpenXML SDK is ideal if you want to leverage the native Microsoft solution.
Q: Can I only read Excel data or also modify it using these libraries?
A: All the mentioned libraries, OpenXML SDK, Apache POI (poi-c), Apache POI (poi-lite), and LibXL, allow you to both read and modify Excel data. You can retrieve the necessary data fields, perform calculations, update values, and save the modified file back to disk.
Q: Is it possible to read and manipulate large Excel files efficiently in C?
A: Yes, it is possible to efficiently read and manipulate large Excel files in C by utilizing techniques such as batch processing, data paging, and parallel processing. By implementing these techniques, you can minimize memory usage, optimize processing speed, and efficiently handle large amounts of data.
Q: Can I use these libraries to read Excel files on operating systems other than Windows?
A: Yes, both the Apache POI libraries (poi-c and poi-lite) and the LibXL library are cross-platform and can be used to read Excel files on various operating systems, including Windows, Linux, and macOS. However, the OpenXML SDK is primarily built for use with Windows, which means it might have limited cross-platform support.
In conclusion, reading Excel files in C can be accomplished using various methods and libraries. Depending on your requirements, you can choose between the OpenXML SDK, Apache POI (poi-c or poi-lite), or LibXL. These libraries provide the necessary functionality to read and manipulate Excel data effectively, allowing developers to incorporate Excel files seamlessly into their C applications.
Read File In C
File input and output operations are an essential aspect of any programming language, as they allow developers to interact with external data files. In the C programming language, reading files is achieved through a set of functions specifically designed for this purpose. In this article, we will delve into the intricacies of reading files in C, covering various techniques and functions. Whether you are a novice programmer or a seasoned software engineer, this comprehensive guide will equip you with the knowledge needed to effectively read files in C.
### Understanding File Handling in C
Before we dive into specific techniques for reading files in C, it is crucial to understand the fundamental concepts associated with file handling in this programming language.
In C, files are handled using the `FILE` data type, which is defined in the `stdio.h` header file. The `FILE` data type represents both input and output streams, and it contains the necessary information to perform file operations. To use the `FILE` data type, we must declare a pointer of type `FILE`, which will be used to associate the file, read its contents, and perform other operations.
### Opening a File for Reading
To read a file in C, we first need to open it. The `fopen()` function is used for this purpose, and it takes two arguments: the name of the file to be opened and the mode in which the file will be accessed. The mode can be specified as `r` for read access.
“`
#include
int main() {
FILE *filePointer;
filePointer = fopen(“example.txt”, “r”);
if (filePointer == NULL) {
printf(“Unable to open file.\n”);
return 1;
}
// File opened successfully, further operations can be performed
fclose(filePointer);
return 0;
}
“`
The code snippet above demonstrates how to open a file named “example.txt” in read mode. If the file exists and can be opened successfully, `fopen()` returns a valid file pointer, which is then assigned to `filePointer`. However, if the file cannot be opened for any reason (e.g., file doesn’t exist, insufficient permissions), `fopen()` returns `NULL`, indicating an error. It is good practice to validate if the file was opened successfully to avoid any runtime errors.
### Reading Characters from a File
Once a file is successfully opened, we can proceed with reading its contents. The `fgetc()` function is used to read a single character from the file. This function takes the file pointer as its argument and returns the character read as an `int` value. If the end of the file is reached or an error occurs, `EOF` (end-of-file) is returned.
“`
#include
int main() {
FILE *filePointer;
int character;
filePointer = fopen(“example.txt”, “r”);
if (filePointer == NULL) {
printf(“Unable to open file.\n”);
return 1;
}
while ((character = fgetc(filePointer)) != EOF) {
printf(“%c”, character);
}
fclose(filePointer);
return 0;
}
“`
In the above code snippet, we read characters from the file using a `while` loop. The loop continues until `EOF` is encountered, implying the end of the file. Each character is then printed using `printf()`. It is important to note that `fgetc()` reads characters one by one, which means it may not be suitable for reading large files efficiently as it could have a noticeable impact on performance.
### Reading Lines from a File
While reading a file character by character works well for shorter files, reading line by line is often more practical. The `fgets()` function offers such functionality by allowing us to read a line from a file. It takes three arguments: the character array (or string) where the line will be stored, the maximum number of characters to be read, and the file pointer.
“`
#include
int main() {
FILE *filePointer;
char line[100];
filePointer = fopen(“example.txt”, “r”);
if (filePointer == NULL) {
printf(“Unable to open file.\n”);
return 1;
}
while (fgets(line, sizeof(line), filePointer) != NULL) {
printf(“%s”, line);
}
fclose(filePointer);
return 0;
}
“`
By passing a character array (`line`) to `fgets()`, we can read one line at a time from the file. The maximum number of characters to be read is set to 100 in this case.
### Frequently Asked Questions (FAQs)
#### Q1. How can I check if a file exists before reading it?
A1. To check if a file exists before reading, you can use the `access()` function from the `unistd.h` header file. This function returns `0` if the file exists and you have sufficient permissions to access it.
#### Q2. Can I read binary files using these functions?
A2. Yes, you can read binary files using the same functions mentioned in this article. However, you should be cautious while accessing binary files, as using text-based functions like `printf()` to display their contents may lead to unexpected results.
#### Q3. How do I read only specific parts of a file?
A3. To read only specific parts of a file, you can use functions like `fseek()` and `ftell()` to set the file position indicator at the desired location. Once set, you can use functions like `fgetc()` or `fgets()` to read the required portion.
#### Q4. Is it possible to read multiple files simultaneously?
A4. Yes, you can read multiple files simultaneously by opening separate file pointers for each file and performing file-specific operations using these pointers.
In conclusion, reading files in C is a crucial skill every programmer should possess. This article introduced the fundamental techniques and functions for reading files, highlighting important concepts along the way. By understanding file handling, opening files, and reading characters or lines, you can effectively interact with external data files in your C programs.
Images related to the topic reading a csv file in c#
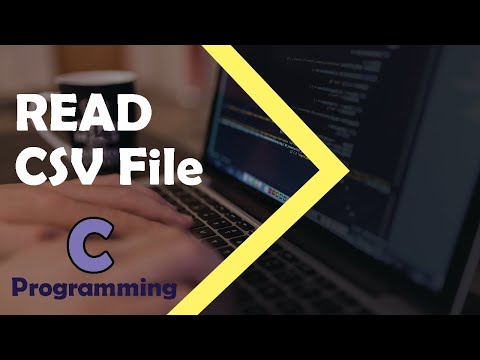
Article link: reading a csv file in c#.
Learn more about the topic reading a csv file in c#.
- parsing – Read .csv file in C – Stack Overflow
- Reading CSV files in C ::
- Relational Database from CSV Files in C – GeeksforGeeks
- Relational Database from CSV Files in C – GeeksforGeeks
- How To Read A CSV File In Python – Earthly Blog
- How to write to a CSV file in C – DEV Community
- How to Read a CSV File in C – Techwalla
- Reading and displaying each line from a CSV file in … – Plus2net
- How to read a CSV file and store the values into an array in C
See more: https://nhanvietluanvan.com/luat-hoc