React Test Library Waitfor
One of the key features of React Testing Library is the waitFor function. The waitFor function enables developers to wait for certain conditions to be met before proceeding with their tests. This can be useful when testing asynchronous code, waiting for elements to appear or disappear, or waiting for specific conditions to be true.
So, what exactly is the waitFor function in React Testing Library? The waitFor function is an asynchronous function that provides a way to wait for a condition to be met before proceeding with the test. It takes in a callback function that defines the condition to wait for and returns a promise that resolves when the condition is met, or rejects if the condition is not met within a specified timeout.
The waitFor function works by repeatedly calling the provided callback function until either the condition is met or the timeout is reached. It uses a combination of polling and timing intervals to check the condition at regular intervals. This approach ensures that the test does not move on until the condition is met, even if the condition takes some time to be fulfilled.
One common use case for the waitFor function is waiting for elements to appear or disappear on the page. For example, if you have an application that dynamically renders a component based on an asynchronous API call, you can use the waitFor function to wait for the component to be rendered before asserting on its contents.
To wait for an element to appear, you can use the waitFor function in combination with query selectors provided by React Testing Library, such as `getElementsByClassName` or `getByName`. You can define the condition to wait for by checking for the presence of the desired element in the DOM. Once the element appears, the waitFor function will resolve, and you can proceed with your assertions.
Similarly, you can use the waitFor function to wait for elements to disappear from the DOM. This can be useful when testing scenarios where elements are removed from the page after a certain action or condition is met. You can define the condition to wait for by checking for the absence of the element in the DOM.
Another use case for the waitFor function is waiting for async actions to complete. When testing code that involves asynchronous operations, such as API calls or event listeners, you can use the waitFor function to wait for the async action to finish before making assertions on the resulting state or UI changes.
To wait for async actions, you can define the condition to wait for by checking for the desired state or UI change that occurs after the async action is complete. Once the condition is met, the waitFor function will resolve, and you can proceed with your assertions.
In addition to waiting for elements or async actions, the waitFor function can also be used to wait for specific conditions to be true. You can define the condition to wait for by using custom logic in the callback function. This can be useful when testing complex scenarios that require specific conditions to be met before proceeding.
When using the waitFor function, it is important to handle timeout and error scenarios appropriately. The waitFor function allows you to specify a timeout value, which determines how long the function will wait for the condition to be met before throwing an error. If the timeout is reached and the condition is not met, the waitFor function will reject with a timeout error.
To handle timeout errors, you can use the `.catch` method on the promise returned by the waitFor function to catch and handle the error. You can then perform any necessary cleanup or additional assertions based on the error.
To ensure effective usage of the waitFor function, here are some best practices to consider:
1. Use specific queries or selectors to target the elements you are waiting for. This helps narrow down the search and reduces the chance of false positives or false negatives.
2. Keep the timeout value reasonable. Setting it too high can lead to longer test execution times, while setting it too low can cause false negatives. Adjust the timeout value based on the expected delay in the condition being met.
3. Utilize custom assertions in combination with the waitFor function. This allows you to make more specific and meaningful assertions on the state or UI changes that occur after the condition is met.
Now, let’s look at some examples and test cases showcasing the usage of the waitFor function in React Testing Library.
Example 1: Waiting for an element to appear
“`javascript
test(‘renders a component after an API call’, async () => {
render(
await waitFor(() => {
const element = screen.getByText(‘Hello, World!’);
expect(element).toBeInTheDocument();
});
});
“`
In this example, we render the `App` component and use the waitFor function to wait for the element with the text content ‘Hello, World!’ to appear in the DOM. Once the element is present, the waitFor function resolves, and we assert that the element is indeed in the document.
Example 2: Waiting for an async action to complete
“`javascript
test(‘updates UI after an API call’, async () => {
render(
fireEvent.click(screen.getByText(‘Load Data’));
await waitFor(() => {
expect(screen.getByText(‘Data loaded!’)).toBeInTheDocument();
});
});
“`
In this example, we render the `App` component and simulate a click event on a button labeled ‘Load Data’. We then use the waitFor function to wait for the text content ‘Data loaded!’ to appear in the document. Once the text is present, the waitFor function resolves, and we assert that the text is indeed in the document.
Overall, the waitFor function in React Testing Library provides a powerful tool for waiting for conditions to be met in your tests. Whether you are waiting for elements to appear or disappear, waiting for async actions to complete, or waiting for specific conditions to be true, the waitFor function helps ensure that your tests reflect real-world usage scenarios. By following best practices and leveraging the capabilities of the waitFor function, you can write more robust and effective tests for your React applications.
Now, let’s address some frequently asked questions about the waitFor function:
1. What is React testing library timeout in waitFor?
The React Testing Library timeout in waitFor refers to the maximum amount of time the waitFor function will wait for a condition to be met before throwing a timeout error. It is important to set a reasonable timeout value to allow enough time for the condition to be fulfilled without unnecessarily prolonging the test execution.
2. What is React-test-renderer?
React-test-renderer is a package provided by React that allows you to render React components in a virtual DOM environment during testing. It provides a lightweight and easy-to-use API for testing React components without the need for a full browser environment.
3. What is Testing library/react?
Testing library/react is a package that provides a set of utilities and matchers specifically designed for testing React components. It is built on top of React Testing Library and includes additional features and enhancements for testing React applications.
4. What is fireEvent in react-testing-library?
fireEvent is a utility function provided by react-testing-library that allows you to simulate user events, such as click, input, or submit, on React components. It provides a simple and intuitive API for triggering events and updating the state of your components during testing.
5. What is getElementsByClassName in React Testing Library?
getElementsByClassName is a query selector provided by React Testing Library that allows you to select elements based on their CSS class name. It returns an array-like object containing all the elements that match the specified class name.
6. What is getByName in React Testing Library?
getByName is not a built-in query selector in React Testing Library. However, you can use the getByLabelText or getByRole queries to select elements based on their label text or role attribute, respectively. These selectors allow you to target elements based on their associated label or their intended role in the UI.
7. What is toHaveTextContent in react-testing-library?
toHaveTextContent is a matcher provided by react-testing-library that allows you to assert that an element has a specific text content. It is useful for making assertions on the rendered output of your React components, ensuring that the expected text is present in the DOM.
8. Why should we avoid wrapping testing-library util calls in actreact test library waitFor?
Wrapping testing-library util calls in `act` is unnecessary when using the waitFor function. The waitFor function automatically wraps the callback function in an `act` call, ensuring that any updates to the component state or UI are properly handled during testing. Therefore, there is no need to manually wrap the testing-library util calls in `act` when using the waitFor function.
In conclusion, the waitFor function in React Testing Library is a valuable tool for writing effective and reliable tests for your React applications. By understanding its concepts, usage, and best practices, you can leverage the power of the waitFor function to write robust and efficient tests that accurately reflect real-world scenarios.
React Testing Library – Events And Async
What Is The Use Of Waitfor In React Testing Library?
The waitFor function in the React Testing Library plays a crucial role in ensuring that your tests accurately reflect the behavior of an asynchronous operation. As React applications often rely on asynchronous events such as API calls, user interactions, or timers, it is essential to have a way to wait for these asynchronous operations to complete before asserting the outcomes of the test.
The waitFor function allows you to wait explicitly for certain conditions to be met before continuing with the test. It ensures that your test code waits until the condition is fulfilled, or until a timeout is reached. This provides a reliable way to test asynchronous operations and handle the unexpected delays that could occur during testing.
How does waitFor work?
The waitFor function takes in a callback function as its argument. This callback function should contain the assertions you want to make or the condition you want to wait for. The callback function is evaluated continuously until it either returns a truthy value or a timeout occurs.
Under the hood, the waitFor function uses a combination of polling and a timeout mechanism to continuously run the callback function. It waits for a short amount of time, usually around 100 milliseconds, and then checks if the callback function returns a truthy value. If it does, the waitFor function considers the condition fulfilled, and the test can proceed. If the callback function returns a falsy value, it will repeat the process until a timeout is reached.
To use the waitFor function, you need to import it from the React Testing Library:
“`javascript
import { waitFor } from ‘@testing-library/react’;
“`
Once imported, you can use it within your test code. Here’s an example that demonstrates the usage of waitFor:
“`javascript
import { render, screen } from ‘@testing-library/react’;
test(‘fetches and displays data asynchronously’, async () => {
render(
const dataElement = screen.getByTestId(‘data-element’);
await waitFor(() => {
expect(dataElement).toHaveTextContent(‘Data has been fetched’);
});
});
“`
In the example above, we render a component called `MyComponent`, which asynchronously fetches some data and then updates the DOM. We use the `screen.getByTestId` to get the element containing the fetched data.
We then use the `waitFor` function, passing in a callback function that asserts whether the fetched data is displayed correctly (in this case, we check if the element has the text content ‘Data has been fetched’). The `await` keyword is used to tell the test to wait until the condition is fulfilled.
Frequently Asked Questions (FAQs):
Q: What happens if the condition is not fulfilled before the timeout occurs?
A: If the condition passed to `waitFor` does not become truthy before the timeout is reached, an error will be thrown. This helps to ensure that your tests do not get stuck indefinitely, and you can identify and fix potential issues with your asynchronous code.
Q: Can I customize the timeout duration?
A: Yes, you can customize the timeout duration by passing an options object as the second argument to the `waitFor` function. For example:
“`javascript
await waitFor(() => {
// …
}, { timeout: 5000 }); // Timeout set to 5 seconds
“`
Q: How can I handle the case where an asynchronous operation fails?
A: If an asynchronous operation fails, such as an API call returning an error, the `waitFor` function will continue running until a timeout occurs. You can utilize the `.catch` method or any error handling mechanism within your callback function to handle such scenarios and throw an error accordingly.
Q: Is there any alternative to using `waitFor`?
A: The `waitFor` function is the recommended approach for handling asynchronous testing in React Testing Library. However, you can also use other methods like `act` or `wait` from the `@testing-library/react-hooks` package in certain cases. Make sure to evaluate the suitability of each approach based on your specific testing requirements.
In conclusion, the `waitFor` function is a powerful tool in the React Testing Library that allows you to handle asynchronous operations in your tests effectively. It ensures that your tests accurately reflect the behavior of your application by waiting for the completion of asynchronous events. By using `waitFor`, you can write more robust and reliable tests, resulting in a higher level of confidence in the quality of your React components.
What Is The Timeout For React Testing Library Act?
React Testing Library is a popular testing library that is widely used for testing React components and applications. It provides a set of utilities and APIs that make it easier to write tests that simulate user interactions and assert on their outcomes. One important utility in the React Testing Library is the `act` function, which allows you to wait for and assert on updates to the React component tree.
The `act` function was introduced as part of the `@testing-library/react` package in version 9.0. It is a utility function that ensures that all updates to the component tree are flushed and applied before moving onto the next step in the test. This is important because React may batch multiple updates together for performance reasons, and without using `act`, your test could assert on the wrong state of the component.
The `act` function takes a callback function as its argument, and it executes this function synchronously. It returns a Promise that resolves when all updates to the component tree have been applied. This allows you to wait for the component to settle into its final state before making any assertions.
By default, `act` has a timeout of 5000 milliseconds (5 seconds). This means that if the updates to the component tree take longer than 5 seconds to settle, the test will fail with a timeout error. However, you can override this default timeout by providing a second argument to the `act` function.
To specify a custom timeout for `act`, you can pass a number to the second argument of the function, representing the maximum number of milliseconds to wait for the updates to settle. For example:
“`jsx
import { act, render } from ‘@testing-library/react’;
test(‘example test’, async () => {
await act(async () => {
// Perform some asynchronous actions, such as fetching data
// or simulating user interactions
// Make any assertions on the component’s state
}, { timeout: 10000 }); // Set custom timeout to 10 seconds
});
“`
In the above example, the `act` function has a custom timeout of 10 seconds. This means that the test will wait for a maximum of 10 seconds for the component tree to settle.
It is important to note that increasing the timeout for `act` should be done cautiously. If your tests consistently take a long time to settle, it may indicate issues with your code or test setup. In general, it is a good practice to keep your tests fast and responsive to provide quick feedback.
FAQs:
Q: How do I know if I need to increase the timeout for `act`?
A: If your test fails with a timeout error, it means that the updates to the component tree took longer than the default timeout of 5 seconds. You can try increasing the timeout value to see if it resolves the issue. However, it is important to investigate why the updates are taking too long and if there are any optimizations that can be made.
Q: Can I have different timeouts for different `act` calls in the same test?
A: Yes, you can specify different timeouts for different `act` calls within the same test. This can be useful in cases where specific actions take longer to settle than others.
Q: Can I disable the timeout for `act` altogether?
A: No, it is not possible to disable the timeout for `act`. However, you can set a very large timeout value to effectively make it wait indefinitely. But keep in mind that this can lead to tests running for an unnecessarily long time and impair overall test performance.
Q: Is there a recommended timeout value for `act`?
A: There is no one-size-fits-all recommendation for the timeout value in `act`. It depends on the specific use case and the complexity of your component updates. However, it is generally a good practice to keep the timeout value as low as possible while still allowing the component to settle into its final state.
In conclusion, the `act` function in React Testing Library is a valuable utility for testing React components. It ensures that updates to the component tree are flushed and applied before making assertions, preventing tests from asserting on incorrect states. The default timeout for `act` is 5 seconds, but you can override this by providing a second argument to the function. However, it is important to use custom timeouts judiciously and investigate any performance issues that may be causing delayed updates.
Keywords searched by users: react test library waitfor React testing library timeout in waitFor, React-test-renderer, Testing library/react, fireEvent react-testing-library, Getelementsbyclassname React testing-library, Getbyname react testing library, toHaveTextContent react-testing-library, Avoid wrapping testing-library util calls in act
Categories: Top 98 React Test Library Waitfor
See more here: nhanvietluanvan.com
React Testing Library Timeout In Waitfor
Timeout in `waitFor`:
The `waitFor` function in React Testing Library allows developers to wait for an asynchronous action to complete before asserting its result. This can be especially useful when dealing with components that make API calls, handle animations, or depend on other external factors.
By default, `waitFor` has a timeout of 5000ms (5 seconds), which means that it will wait for 5 seconds for the asynchronous action to complete before timing out and failing the test. However, this default timeout can be modified to fit the needs of specific test scenarios.
Sometimes, an asynchronous action may take longer to complete due to various reasons such as slow network connections, complex computations, or external dependencies. In such cases, it’s important to increase the timeout value to ensure that the test doesn’t fail prematurely.
To adjust the timeout value in `waitFor`, developers can pass an additional configuration object as the second argument. This object can contain various options, including a `timeout` property to set a custom timeout value. For example, to set a timeout of 10 seconds, the `waitFor` function can be called as follows:
“`javascript
await waitFor(async () => {
// Asynchronous action to wait for
}, { timeout: 10000 });
“`
By increasing the timeout value, developers can allow more time for the asynchronous action to complete. This helps make the test more robust and less prone to false negatives.
FAQs:
Q: How do I handle cases where an asynchronous action never completes?
A: If an asynchronous action takes an unexpectedly long time or never completes, the `waitFor` function will eventually time out and fail the test. In such cases, it’s important to investigate the underlying cause of the delay and address it appropriately. This could involve debugging the code, optimizing network requests, or addressing any external factors that might be affecting the action’s completion.
Q: Can I explicitly fail a test when the timeout is reached?
A: Yes, you can explicitly fail a test if the timeout is reached by throwing an error inside the `waitFor` function. This can be useful when testing scenarios where the asynchronous action should never take longer than a certain threshold. For example:
“`javascript
await waitFor(async () => {
// Asynchronous action to wait for
if (/* timeout condition */) {
throw new Error(‘Timeout reached’);
}
}, { timeout: 5000 });
“`
Throwing an error will cause the test to fail immediately, providing clear feedback about the timeout condition.
Q: Are there any performance implications of using longer timeouts?
A: Longer timeouts may impact test execution time, as the `waitFor` function will wait for the specified duration before failing the test. However, it’s important to strike a balance between giving enough time for the asynchronous action to complete and ensuring that the test runs efficiently. It might be worth reconsidering the test implementation if it consistently requires excessive amounts of time to complete.
Q: Can I use `waitFor` without a timeout?
A: Yes, it’s possible to use `waitFor` without specifying a timeout. In such cases, the test will wait indefinitely for the asynchronous action to complete. However, this approach should be used with caution, as it can potentially lead to tests hanging indefinitely if the action never completes. It’s generally better to define a reasonable timeout value to avoid such scenarios.
Q: Are there any alternatives to `waitFor` for handling asynchronous actions?
A: React Testing Library provides other utilities, such as `waitForElementToBeRemoved`, which can be used to handle specific cases where the DOM should change after an asynchronous action. Additionally, the `act` function can be used to wrap code that causes side effects or triggers asynchronous actions, ensuring that they are properly handled during test execution.
In conclusion, React Testing Library’s `waitFor` function, with its customizable timeout feature, provides a robust mechanism for testing asynchronous actions in React components. By adjusting the timeout value as per specific requirements, developers can ensure that their tests accurately wait for actions to complete, leading to more reliable and comprehensive test suites.
React-Test-Renderer
The main purpose of using React-test-renderer is to test the behavior of React components in isolation. It allows developers to render components without the need for a full DOM or a browser, which makes testing faster and more reliable. With React-test-renderer, developers can render a component and then make assertions on its output, such as checking if certain elements are present, if certain props are being passed correctly, or if certain actions trigger the expected changes.
One of the key features of React-test-renderer is its ability to take snapshots of rendered components. Snapshots are a way to capture the output of a component and store it as a file. Whenever the component is rendered in the future, React-test-renderer compares the output with the stored snapshot to ensure that it has not changed unexpectedly. This is particularly useful in preventing regression bugs, where a change in one part of the code unintentionally affects another.
To use React-test-renderer, developers first need to install it as a dependency in their project. This can be done easily using a package manager like npm or yarn. Once installed, they can import React-test-renderer into their test files and start using its API.
To render a component with React-test-renderer, developers need to create a renderer instance by calling the `create` method. They can then use this instance to render a component by calling the `create` method again with the component as a parameter. The resulting object contains the rendered output, which can be accessed via the `toJSON` method.
For example, let’s say we have a simple component called `Button`:
“`jsx
import React from ‘react’;
const Button = ({ label }) => (
);
export default Button;
“`
In our test file, we can use React-test-renderer to render the `Button` component and make assertions on its output:
“`jsx
import React from ‘react’;
import renderer from ‘react-test-renderer’;
import Button from ‘./Button’;
test(‘renders correctly’, () => {
const component = renderer.create();
const tree = component.toJSON();
expect(tree).toMatchSnapshot();
});
“`
In this example, we use the `toMatchSnapshot` method to assert that the rendered output of the `Button` component matches a previously stored snapshot. If the output does not match, the test will fail, indicating that the component’s behavior has changed.
React-test-renderer provides a wide range of methods to make assertions on the rendered output of components. Developers can use methods like `toJSON`, `toTree`, and `toJSONOutput` to access the rendered output and make specific assertions, such as checking if a certain element is present or if a certain prop has a specific value.
Additionally, React-test-renderer supports simulating events on components. Developers can use the `act` method to trigger an event and then make assertions based on the resulting changes to the component. This allows for testing component behaviors that rely on user interactions, such as button clicks or form submissions.
In conclusion, React-test-renderer is a powerful tool for testing React components in isolation. Its virtual DOM implementation and snapshot functionality enable developers to write efficient and comprehensive tests. By rendering components without the need for a browser, React-test-renderer makes testing faster and more reliable. With its wide range of methods and event simulation capabilities, developers can make assertions on the output and behavior of their components with ease.
FAQs:
Q: Is React-test-renderer only for React components?
A: Yes, React-test-renderer is specifically designed for testing React components. It works with both class components and functional components.
Q: Can React-test-renderer be used with other testing frameworks?
A: Yes, React-test-renderer can be used with popular testing frameworks like Jest and Enzyme. It provides a lightweight alternative to testing components without the need for a full DOM or a browser.
Q: Can React-test-renderer test component interactions with external dependencies?
A: No, React-test-renderer is focused on testing components in isolation. If a component relies heavily on external dependencies, it might be more suitable to use a different testing approach, such as mocking those dependencies.
Q: Can React-test-renderer be used for unit testing and integration testing?
A: Yes, React-test-renderer can be used for both unit testing and integration testing. It allows developers to test individual components in isolation, as well as the interactions between multiple components.
Q: How does React-test-renderer compare to Enzyme?
A: React-test-renderer and Enzyme are both popular tools for testing React components. React-test-renderer provides a simpler and more lightweight API, while Enzyme offers a more extensive set of features and utilities for testing React components. The choice between the two depends on the specific needs and preferences of the developer.
Testing Library/React
Introduction
Testing is an integral part of software development as it enables developers to ensure the quality, reliability, and stability of their applications. When it comes to testing React applications, one of the most popular and widely used testing frameworks is Testing Library/React. This powerful tool provides a simple and intuitive API that promotes best practices for testing components, making it easier to write effective and maintainable tests. In this article, we will delve deep into the world of Testing Library/React, exploring its features, benefits, and best practices. So, let’s get started!
What is Testing Library/React?
Testing Library/React is a lightweight and beginner-friendly testing library for React applications. It is built on top of the React Testing Library, which is a set of utilities that encourage developers to write tests that resemble how a real user would interact with their application. The library follows a guiding principle: “The more your tests resemble the way your software is used, the more confidence they can give you.” It focuses on testing React components in a way that is close to how they will be used by actual users.
Why use Testing Library/React?
Testing Library/React offers several benefits that make it an excellent choice for testing React applications:
1. Accessibility and User Experience-Centric Testing: Testing Library/React encourages writing tests that simulate how a user interacts with the application, rather than relying on implementation details. This approach ensures that tests focus on the end-user experience, making them more reliable and valuable.
2. Simplicity and Developer Experience: Testing Library/React provides an intuitive and easy-to-learn API, making it accessible even to developers with minimal testing experience. The library is designed to be beginner-friendly, reducing the learning curve for writing tests.
3. Maintaining Test Readability and Scalability: The library promotes writing tests that are easy to read, understand, and maintain. With its declarative API and focus on user-centric testing, the tests remain readable and resilient to changes in the application’s codebase.
4. Compatibility and Community Support: Testing Library/React is actively maintained and has a large and supportive community. It works seamlessly with popular testing frameworks such as Jest, React Testing Library, and Cypress, ensuring compatibility and accessibility.
Getting Started with Testing Library/React
To start using Testing Library/React for testing your React applications, follow these steps:
1. Set up a new or existing React project with a suitable testing framework like Jest or React Testing Library.
2. Install the required dependencies by running the command `npm install –save-dev @testing-library/react`.
3. Write your tests using the Testing Library/React API.
The Testing Library/React API
The Testing Library/React API provides a set of utility functions and methods for interacting with React components during testing. Some commonly used functions include:
1. render(): Renders a React component within a testing environment, allowing you to interact with and test its output.
2. screen.getByText(): Retrieves a DOM element that contains specific text content.
3. screen.getByRole(): Retrieves a DOM element based on its defined accessibility role (e.g., button, link, input).
4. fireEvent.click(): Simulates a click event on a DOM element.
5. waitFor(): Waits until a specific condition is met before proceeding with the test.
Best Practices for Testing with Testing Library/React
To write effective tests using Testing Library/React, consider the following best practices:
1. Focus on User Stories: Write tests that simulate how a user would interact with your application. This helps ensure that your tests cover the most critical user scenarios.
2. Avoid Reliance on Implementation Details: Write tests that are resilient to changes in the application’s codebase. Instead of asserting on specific implementation details, focus on the rendered output and user interactions.
3. Use Jest Matchers: Jest provides a set of powerful matchers that can simplify your assertions. Utilize them to write clear and concise test cases.
4. Avoid Excessive Mocking: Only mock or stub external dependencies when necessary. Over-mocking can lead to brittle tests that are tightly coupled to the implementation details.
5. Leverage Async Testing: Use async/await or Promises to handle asynchronous operations during testing. The Testing Library/React API provides built-in methods like waitFor() to wait for specific conditions.
FAQs
Q1: Can I use Testing Library/React with other testing frameworks like Cypress?
A1: Yes, Testing Library/React is compatible with popular testing frameworks like Jest, React Testing Library, and Cypress.
Q2: Is Testing Library/React suitable for testing complex React applications?
A2: Yes, Testing Library/React is well-suited for testing both small and complex React applications. Its user-centric approach allows for effective testing of various components and scenarios.
Q3: Are there any additional tools or libraries I need to install to use Testing Library/React?
A3: Testing Library/React can be used with Jest or React Testing Library. Install these libraries based on your testing framework requirements.
Q4: Can Testing Library/React be used for testing React Native applications?
A4: While Testing Library/React is primarily focused on React web applications, it can be used to test certain aspects of React Native applications. However, for comprehensive testing of React Native applications, consider using tools like React Native Testing Library.
Conclusion
Testing Library/React provides a powerful and user-centric approach to testing React applications. By focusing on the end-user experience, the library promotes readability, maintainability, and reliability in tests. It is a beginner-friendly choice that offers compatibility with popular testing frameworks and has a supportive community. By following best practices and leveraging the Testing Library/React API, developers can write effective tests that ensure the quality and stability of their React applications. So, start exploring Testing Library/React and elevate the testing capabilities of your React projects.
Images related to the topic react test library waitfor
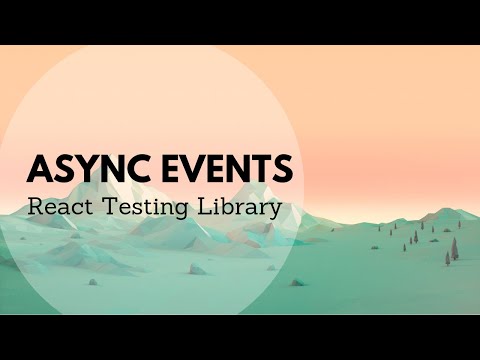
Found 15 images related to react test library waitfor theme
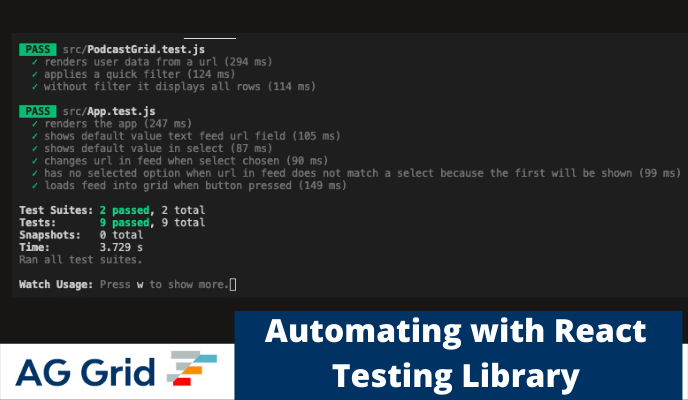
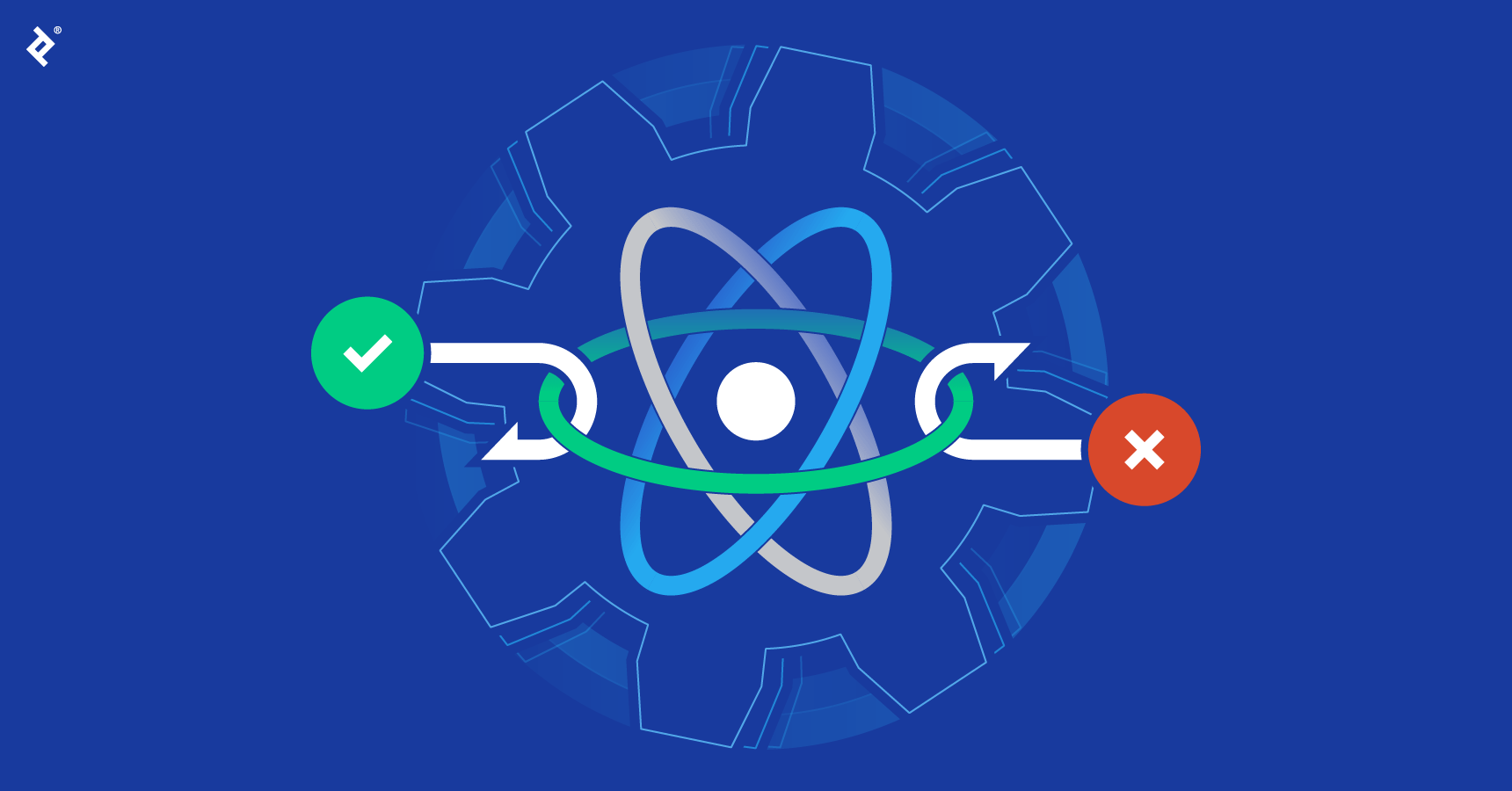
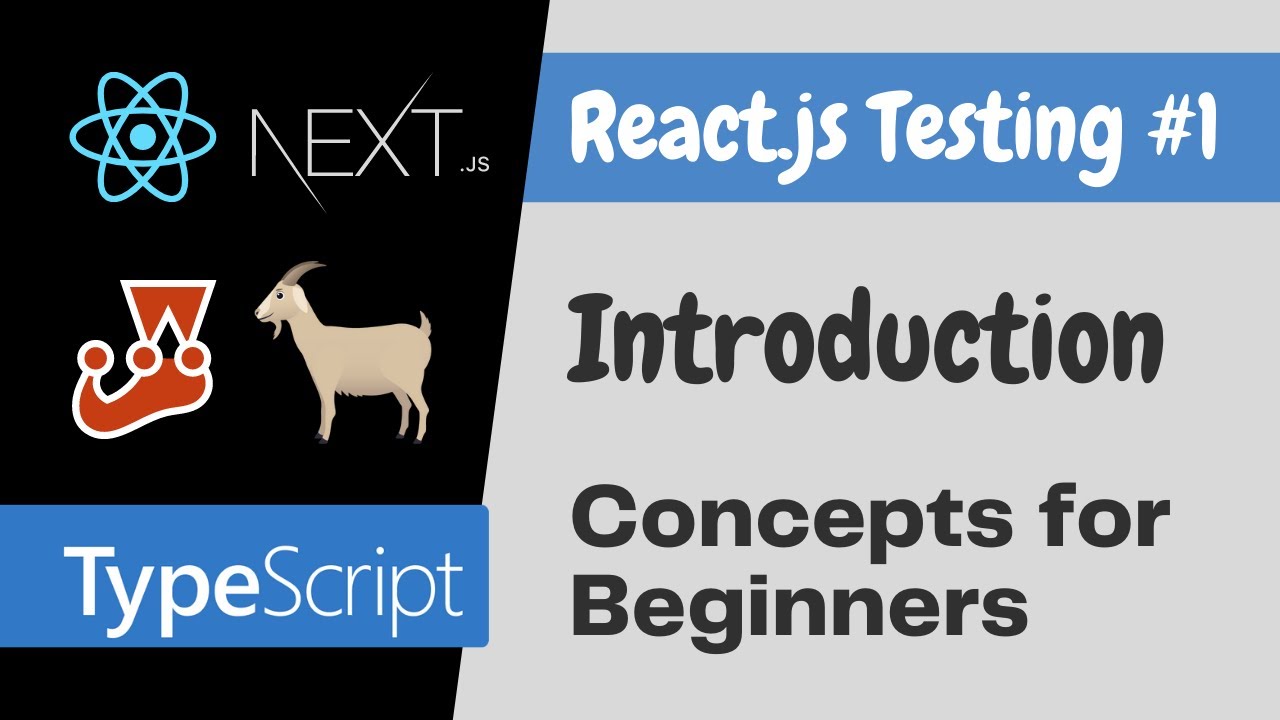


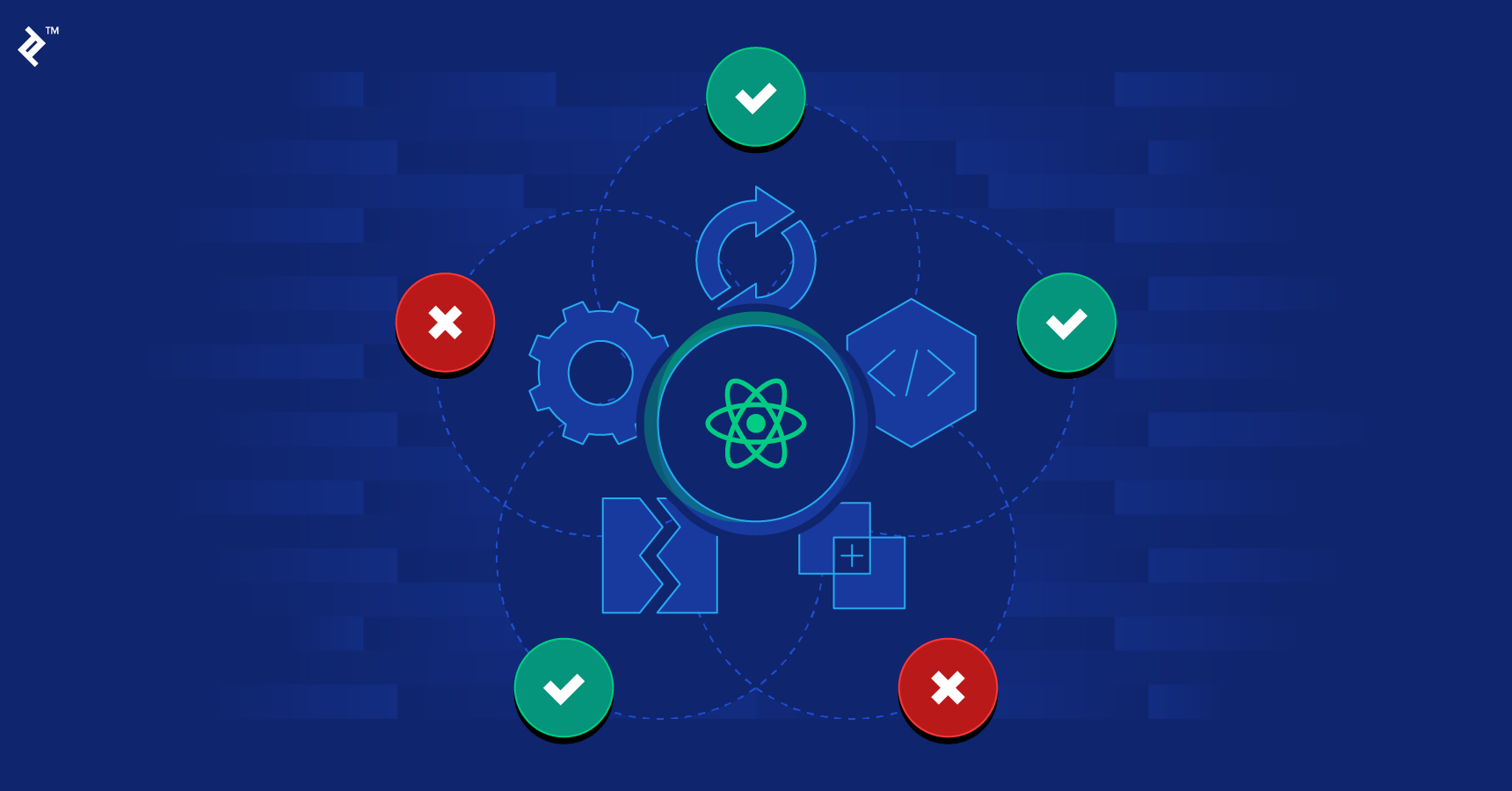
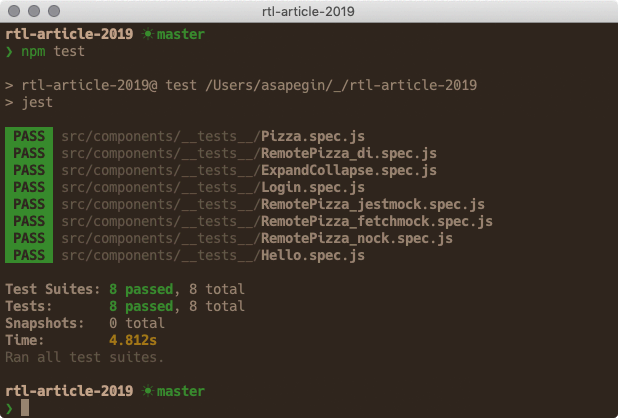
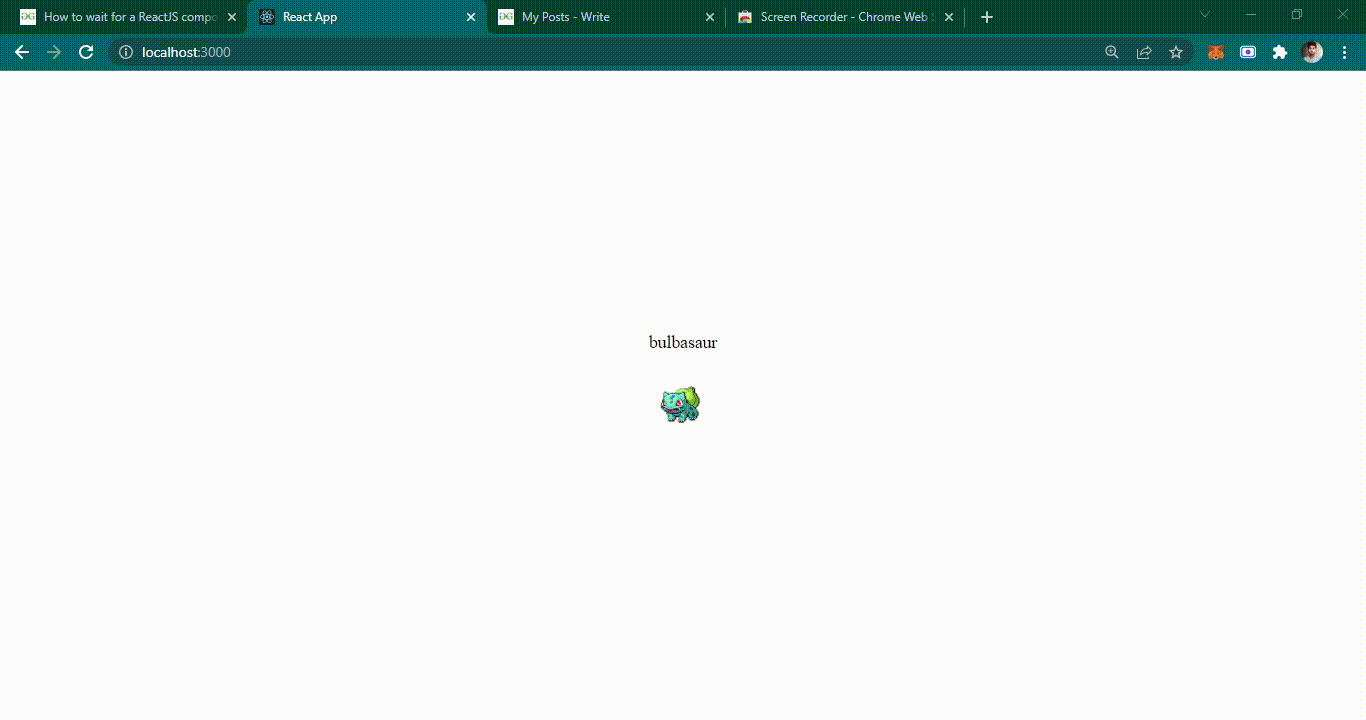
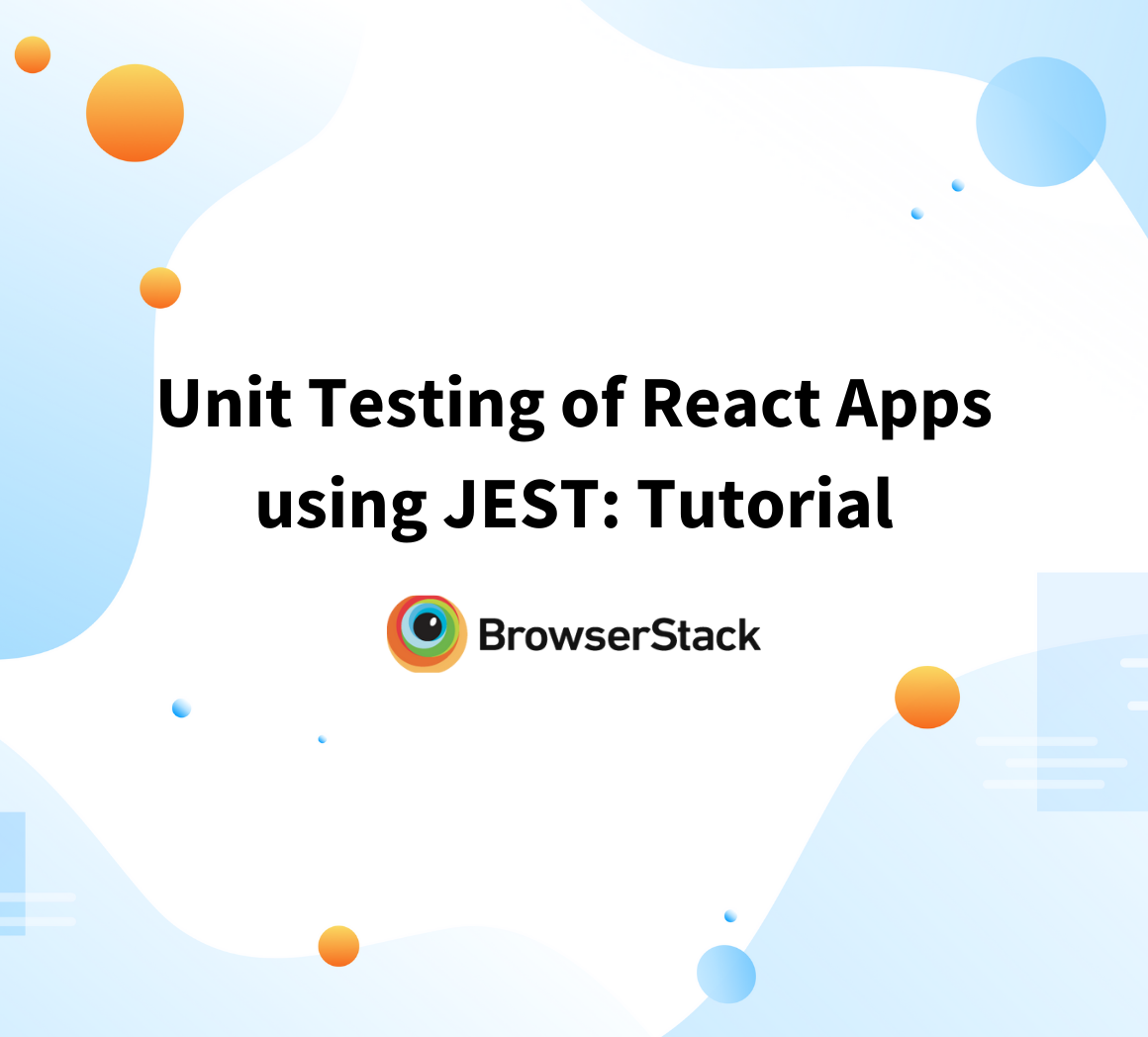
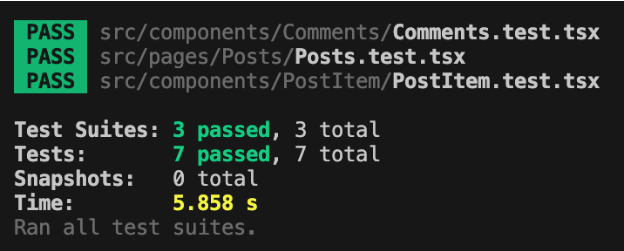

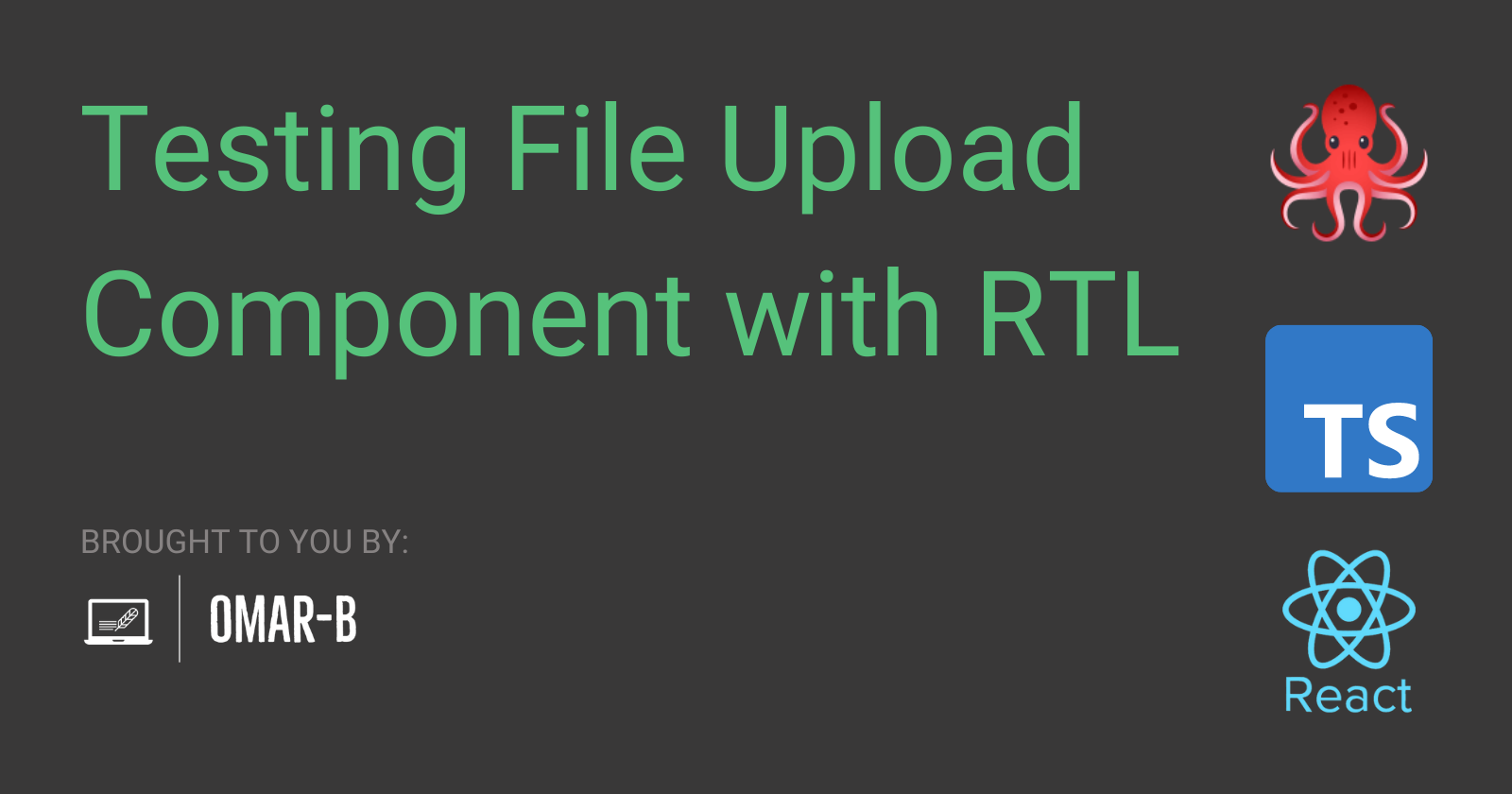
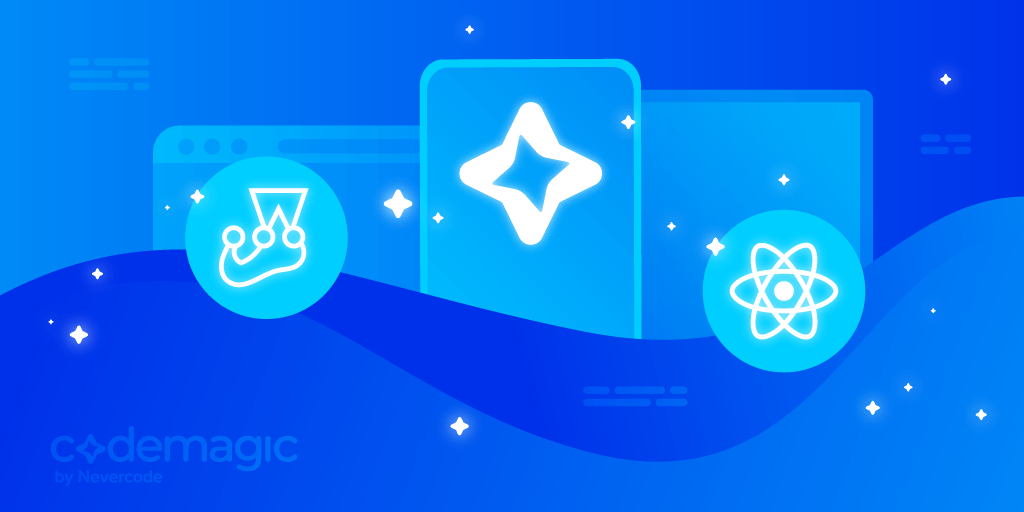


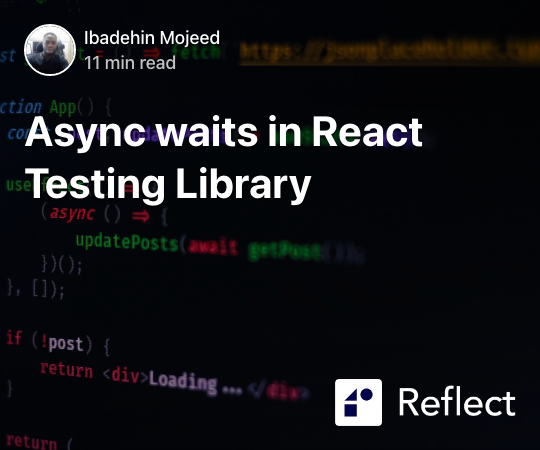
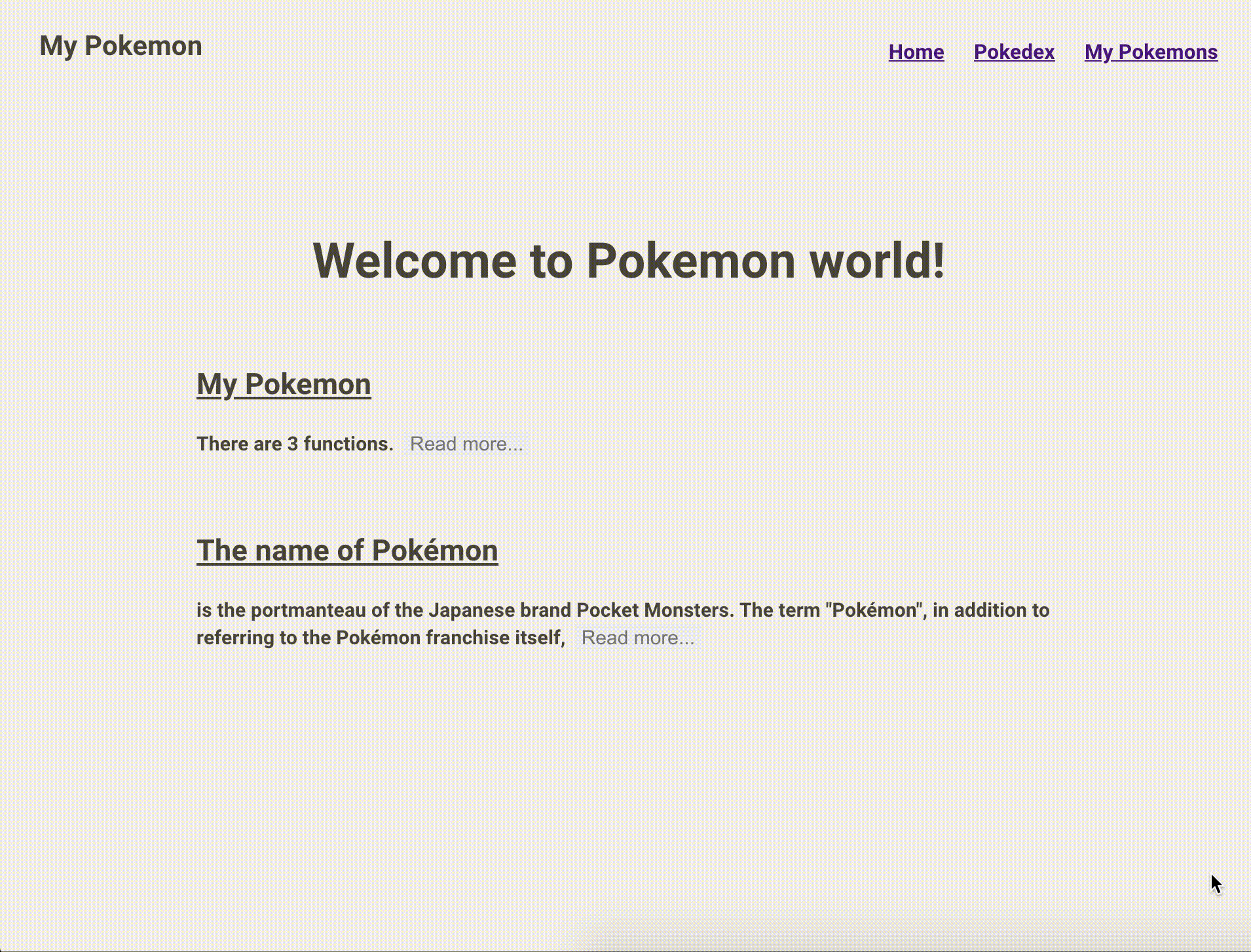
Article link: react test library waitfor.
Learn more about the topic react test library waitfor.
- Async Methods – Testing Library
- React testing library how to use waitFor – Stack Overflow
- React Testing Library waitFor: Start Using It in 6 Steps – Testim
- “act” and “waitFor” react-testing-library | by Mohammad Abbas
- “act” and “waitFor” react-testing-library | by Mohammad Abbas
- Async Methods – Testing Library
- Top Testing Libraries for React in 2023 | BrowserStack
- waitfor – Microsoft Learn
- How to Use React Testing Library to Wait for Async Elements
- react-native-testing-library/src/__tests__/waitFor.test.tsx at main
- Testing | TanStack Query Docs
- @testing-library/react waitFor TypeScript Examples
- Async waits in React Testing Library – Reflect.run
- waitfor react testing library timeout – CIO
See more: https://nhanvietluanvan.com/luat-hoc