React Pass Function As Prop
## What are props in React and how are they used?
In React, props are a way to pass information from a parent component to its child components. They are similar to parameters in a function, as they allow data to be passed into a component. Props are read-only, meaning that they cannot be modified by the component that receives them. This allows for a clear separation of concerns and promotes a unidirectional data flow in React applications.
To use props in React, you need to define them when rendering a child component. For example, let’s say we have a parent component called `App` and a child component called `Child`. We can pass a prop named `message` from the parent to the child like this:
“`jsx
// Parent component
function App() {
return (
);
}
// Child component
function Child(props) {
return (
);
}
“`
In the above example, the parent component `App` passes the prop `message` with the value `”Hello, world!”` to the child component `Child`. The child component then receives the props as an object with the name `props`. We can access the value of the `message` prop using `props.message` in the child component.
## How to pass a function as a prop in React
React allows us to pass not only data, but also functions as props. This opens up a wide range of possibilities for communication between components. By passing a function as a prop, we can enable child components to trigger actions or update state in the parent component.
### Explain the concept of passing functions as props in React
Passing functions as props in React involves passing a reference to a function from a parent component to a child component. The child component can then call the function when certain events occur, such as a button click or a form submission. This pattern is commonly used to enable interactivity in React applications.
### Show an example of passing a function as a prop
Let’s consider an example where we have a parent component called `App` and a child component called `Button`. We want the child component to notify the parent component when a button is clicked.
“`jsx
// Parent component
function App() {
function handleClick() {
console.log(“Button clicked!”);
}
return (
);
}
// Child component
function Button(props) {
return (
);
}
“`
In the above example, the parent component `App` defines a function `handleClick`. This function logs a message to the console when called. The parent component passes this function as a prop named `onClick` to the child component `Button`. The child component then attaches the `onClick` prop to the button’s `onClick` event, so that when the button is clicked, the function `handleClick` is called in the parent component.
### Discuss the benefits of passing functions as props
Passing functions as props in React has several benefits. Firstly, it allows for communication and data flow between components. By passing a function as a prop, we can trigger actions or update state in the parent component based on events that occur in the child component.
Secondly, passing functions as props promotes reusability and modularity of components. Since the logic for handling events or updating state resides in the parent component, child components can be reused in different parts of the application without having to duplicate code.
Lastly, passing functions as props makes testing easier. By passing functions as props, we can easily mock and test the behavior of child components. This facilitates unit testing and increases the overall testability of React applications.
## Binding the value of ‘this’ in the passed function
When passing a function as a prop in React, it is important to ensure that the value of `this` is correctly bound within the passed function. By default, when a function is defined in JavaScript, the value of `this` is determined by how the function is called. This can lead to unexpected behavior when the passed function is invoked in the child component.
### Explain the importance of binding ‘this’ in the passed function
In React, if a function that accesses `this` is passed as a prop and then called in a child component, the value of `this` will be undefined within the function. This is because the function loses its original context when it is detached from the object it belongs to.
To ensure that `this` refers to the correct object within the passed function, we need to explicitly bind the value of `this` before passing it as a prop. This ensures that the function maintains its original context.
### Show how to bind ‘this’ in the passed function using different approaches
There are several ways to bind the value of `this` in a passed function. Here are a few common approaches:
1. Using the arrow function syntax: By using the arrow function syntax, we can automatically bind the value of `this` to the component. This approach is concise and does not require explicit binding.
“`jsx
// Parent component
class App extends React.Component {
handleClick = () => {
console.log(“Button clicked!”);
}
render() {
return (
);
}
}
// Child component
function Button(props) {
return (
);
}
“`
2. Using the `bind()` method: The `bind()` method allows us to explicitly bind the value of `this` to a specific object. We can call `bind()` on the function and provide the context we want `this` to refer to.
“`jsx
// Parent component
class App extends React.Component {
constructor() {
super();
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log(“Button clicked!”);
}
render() {
return (
);
}
}
“`
## Sending arguments along with the passed function
In some cases, we may need to send additional arguments along with the passed function. For example, we might want to pass a unique identifier or the current state of a component. React allows us to pass arguments to functions as props, enabling us to customize the behavior of the function based on the specific context in which it is called.
### Discuss the need for sending arguments with the passed function
Sending arguments along with the passed function adds flexibility and customization to the behavior of the function. By supplying additional data to the function, we can modify its behavior based on the specific context in which it is called.
For example, imagine we have a list of items and want to delete a particular item when a button associated with that item is clicked. By passing the item’s unique identifier as an argument to the delete function, we can ensure that only the specific item is removed from the list.
### Show examples of sending arguments along with the passed function
Let’s consider an example where we have a parent component called `App` and a child component called `Item`. We want to pass a delete function from the parent component to the child component and send the item’s unique identifier as an argument.
“`jsx
// Parent component
function App() {
function handleDelete(id) {
console.log(`Item with id ${id} deleted!`);
}
return (
);
}
// Child component
function Item(props) {
return (
);
}
“`
In the above example, the parent component `App` defines a function `handleDelete` that takes an `id` argument. It logs a message to the console when called with the respective `id`. The parent component passes the function `handleDelete` as a prop named `onDelete` to the child component `Item`, supplying the unique identifier `1` as an argument. The child component attaches the `onDelete` prop to the button’s `onClick` event, so that when the button is clicked, the function `handleDelete` is called with the respective `id`.
## Handling the passed function in the receiving component
When a function is passed as a prop in React, the receiving component needs to properly handle and call the passed function. The function can be called directly within an event handler, or as part of the component’s lifecycle methods.
### Explain how to handle the passed function in the receiving component
To handle the passed function in the receiving component, we can simply call the function within an event handler or a component’s lifecycle method. The function can be called with or without arguments, depending on the specific use case.
In the case of an event handler, such as a button’s `onClick` event, we can directly call the passed function:
“`jsx
// Child component
function Button(props) {
function handleClick() {
props.onClick();
}
return (
);
}
“`
Alternatively, if the function needs to be called during a component’s lifecycle, we can call it within the appropriate lifecycle method. For example, the function can be called within the `componentDidMount` method:
“`jsx
// Child component
class Child extends React.Component {
componentDidMount() {
this.props.onMount();
}
render() {
return
;
}
}
“`
### Show how to call the passed function and retrieve its results
If the passed function returns a value, we can call the function and retrieve its results. The returned value can be used within the receiving component for further processing or rendering.
Let’s consider an example where we have a parent component called `App` and a child component called `Child`. We want to call a passed function and display its result in the child component.
“`jsx
// Parent component
function App() {
function getMessage() {
return “Hello, world!”;
}
return (
);
}
// Child component
function Child(props) {
const message = props.getMessage();
return (
);
}
“`
In the above example, the parent component `App` defines a function `getMessage` that returns a message. The parent component passes the function `getMessage` as a prop named `getMessage` to the child component `Child`. The child component then calls the `getMessage` prop as a function and assigns the returned value to the `message` variable. Finally, the `message` variable is rendered within a `div`.
## Updating component state using the passed function
Another common use case of passing a function as a prop in React is to update the state of a component. By passing a function that handles state updates, we can modify the component’s state in a predictable and controlled manner.
### Discuss how to update the state of a component using the passed function
To update the state of a component using a passed function, we can pass the function as a prop and call it with the new state as an argument. The function should handle the state update and perform any additional logic or side effects if necessary.
Let’s consider an example where we have a parent component called `App` and a child component called `Counter`. We want to pass a function from the parent component to the child component that updates the counter value.
“`jsx
// Parent component
function App() {
const [counter, setCounter] = React.useState(0);
function incrementCounter() {
setCounter(counter + 1);
}
return (
);
}
// Child component
function Counter(props) {
return (
);
}
“`
In the above example, the parent component `App` defines a state variable `counter` and a function `incrementCounter` that updates the `counter` state by incrementing it. The parent component passes the function `incrementCounter` as a prop named `onIncrement` to the child component `Counter`. The child component then attaches the `onIncrement` prop to the button’s `onClick` event, so that when the button is clicked, the function `incrementCounter` is called in the parent component, updating the counter value.
### Show examples of updating state using the passed function
Updating state using a passed function allows us to modify the component’s state based on events or actions in the child component. The passed function can be used to update the state directly or to trigger a more complex state update logic.
Let’s consider an example where we have a parent component called `App` and a child component called `Toggle`. We want to toggle the visibility of a message when a button is clicked in the child component.
“`jsx
// Parent component
function App() {
const [showMessage, setShowMessage] = React.useState(false);
function toggleMessage() {
setShowMessage(!showMessage);
}
return (
{showMessage &&
);
}
// Child component
function Toggle(props) {
return (
);
}
// Child component
function Message() {
return (
);
}
“`
In the above example, the parent component `App` defines a state variable `showMessage` that determines the visibility of a message. It also defines a function `toggleMessage` that toggles the value of `showMessage`. The parent component passes the function `toggleMessage` as a prop named `onToggle` to the child component `Toggle`. The child component then attaches the `onToggle` prop to the button’s `onClick` event, so that when the button is clicked, the function `toggleMessage` is called in the parent component, updating the `showMessage` state. The message component is then conditionally rendered based on the value of `showMessage`.
### Cover any potential pitfalls or considerations when updating state this way
When updating state using a passed function, there are a few potential pitfalls and considerations to keep in mind:
1. Be mindful of the order of operations: When updating state using a passed function, it’s important to consider the timing and order of operations. React batches state updates for performance reasons, so calling the passed function multiple times within a single event handler may not result in the expected state updates. To ensure that state updates are applied correctly and consistently, consider using functional updates or `useEffect` hooks.
2. Avoid unnecessary renders: When passing a function that updates state as a prop, the component may re-render more frequently than necessary. To avoid unnecessary renders, consider using memoization techniques such as memo or useCallback to memoize the passed function.
3. Keep the state update logic in the parent component: When passing a function to update state, it is generally recommended to keep the state update logic in the parent component. This allows for better separation of concerns and prevents child components from having to manage state directly.
## Advanced techniques and best practices for passing functions as props
Passing functions as props in React opens up a wide range of possibilities for communication and interaction between components. Here are some advanced techniques and best practices to consider when working with props:
1. Pass functions within objects or arrays: Instead of passing a single function as a prop, you can pass an object or an array containing multiple functions. This allows for more flexibility and promotes code organization.
“`jsx
// Parent component
function App() {
function handleIncrement() {
console.log(“Incrementing counter!”);
}
function handleDecrement() {
console.log(“Decrementing counter!”);
}
const buttonProps = {
onIncrement: handleIncrement,
onDecrement: handleDecrement,
};
return (
);
}
// Child component
function Counter(props) {
return (
);
}
“`
2. Use TypeScript to enforce type safety: If you’re using TypeScript, you can leverage its type system to enforce type safety when passing functions as props. By defining the expected prop types and function signatures, you can catch potential errors at compile time.
“`jsx
// Parent component (TypeScript)
type ButtonProps = {
onClick: () => void;
};
function App() {
function handleClick() {
console.log(“Button clicked!”);
}
const buttonProps: ButtonProps = {
onClick: handleClick,
};
return (
);
}
// Child component (TypeScript)
function Button(props: ButtonProps) {
return (
);
}
“`
3. Avoid creating unnecessary anonymous functions: When passing functions as props, it’s important to avoid creating unnecessary anonymous functions. Anonymous functions can cause unnecessary re-renders and degrade performance
Full React Tutorial #13 – Functions As Props
Can We Pass A Function As A Prop In React?
React is a popular JavaScript library used for building user interfaces. One of the key features of React is its ability to handle complex state management and allow for the passing of data between components. This is achieved using props, short for properties.
In React, props are used to pass data from a parent component to child components. This allows for the reusability of code and enables components to communicate with each other. Props can consist of any data type, including strings, numbers, arrays, objects, and even functions.
Passing a function as a prop in React is a powerful capability that allows for the implementation of various functionality. By passing a function as a prop, we can enable child components to interact with the parent component and trigger actions based on user input or other events.
To pass a function as a prop, we need to define the function in the parent component and then pass it as a prop to the child component. The child component can then call the function and execute the desired functionality. Let’s look at an example to better understand how this works:
“`
// ParentComponent.js
import React from ‘react’;
import ChildComponent from ‘./ChildComponent’;
class ParentComponent extends React.Component {
handleClick() {
console.log(‘Button clicked!’);
}
render() {
return (
);
}
}
export default ParentComponent;
“`
“`
// ChildComponent.js
import React from ‘react’;
const ChildComponent = (props) => {
return (
);
}
export default ChildComponent;
“`
In this example, the ParentComponent defines a handleClick function that simply logs a message to the console. The ChildComponent is rendered inside the ParentComponent and receives the handleClick function as a prop, named handleClick.
The ChildComponent then uses this prop as the event handler for the button’s onClick event. When the button is clicked, the handleClick function is called, and the message ‘Button clicked!’ is logged to the console.
Passing functions as props in React becomes particularly useful when dealing with form submission, toggling component visibility, or updating state based on user actions. By passing a function as a prop, we can encapsulate the required logic within the parent component and easily reuse it in multiple child components.
Frequently Asked Questions (FAQs):
Q: Can we pass arguments to a function prop in React?
A: Yes, we can pass arguments to a function prop in React. To do so, we can either bind the argument to the function when passing it as a prop or use an arrow function in the child component to pass the arguments. Here’s an example:
“`
// ParentComponent.js
import React from ‘react’;
import ChildComponent from ‘./ChildComponent’;
class ParentComponent extends React.Component {
handleClick(name) {
console.log(`Hello ${name}!`);
}
render() {
return (
);
}
}
export default ParentComponent;
“`
“`
// ChildComponent.js
import React from ‘react’;
const ChildComponent = (props) => {
return (
);
}
export default ChildComponent;
“`
In this example, we pass the name ‘John’ to the handleClick function as an argument when the button is clicked. The parent component then logs the message ‘Hello John!’ to the console.
Q: Can we pass functions as props to nested child components?
A: Yes, we can pass functions as props to nested child components in React. The process remains the same as when passing functions as props to immediate child components. Simply define the function in the parent component, pass it as a prop to the immediate child component, and then pass the function prop down to the nested child components, if required.
Q: Can we pass callbacks as props in React?
A: Yes, callbacks can be passed as props in React. A callback function is simply a function that is passed as an argument to another function and is later invoked within that function. By passing a callback function as a prop, we can achieve dynamic behavior and execute different logic based on user actions or other events.
In conclusion, passing a function as a prop in React is an essential feature that enhances the flexibility and reusability of components. It allows for seamless communication between parent and child components, enabling the creation of interactive and dynamic user interfaces. By understanding how to pass functions as props, developers can leverage React’s capabilities to build powerful and robust applications.
How To Pass A Function As A Prop From Child To Parent In React?
React is a popular JavaScript library for building user interfaces, and it utilizes a component-based architecture. In React, components can pass data between each other by using props. While passing data from a parent component to a child component is straightforward, passing a function as a prop from a child to a parent might seem tricky at first. However, with a clear understanding of React’s unidirectional data flow, it becomes easier to pass a function as a prop from a child component to its parent.
In React, data typically flows from parent components to children through props. This is because child components are usually more reusable and isolated, while parent components control the state and actions for the entire application. However, there are scenarios where it is useful for a child component to be able to communicate with its parent, and passing a function as a prop is the way to achieve this.
Here is a step-by-step guide on how to pass a function as a prop from a child component to its parent:
Step 1: Define the Function in the Parent Component
First, you need to create a function in the parent component that will be called from the child component. This function will typically update the state or perform any desired action in the parent component. For example, let’s create a function called `handleClick` that updates the parent component’s state when a button is clicked:
“`javascript
class ParentComponent extends React.Component {
state = {
counter: 0,
};
handleClick = () => {
this.setState((prevState) => ({
counter: prevState.counter + 1,
}));
};
render() {
return (
Counter: {this.state.counter}
);
}
}
“`
Step 2: Pass the Function as a Prop in the Child Component
Now that we have the function `handleClick` defined in the parent component, we can pass it as a prop to the child component. In the child component, we can access and invoke this function whenever needed. For example, let’s create a button in the child component that calls the `handleClick` function when clicked:
“`javascript
class ChildComponent extends React.Component {
render() {
return (
);
}
}
“`
Step 3: Utilize the Function in the Child Component
In the child component, you can now use the passed function `handleClick` and invoke it when necessary. In this case, clicking the button triggers the function defined in the parent component, updating the counter state.
It’s important to note that passing a function as a prop from child to parent does not break the unidirectional data flow in React. Instead, it allows child components to trigger actions defined in the parent component, maintaining the separation of concerns and reusability of components.
FAQs:
Q: Can I pass arguments along with the function from the child to the parent?
A: Yes, you can pass arguments along with the function from the child to the parent. This can be achieved by passing the arguments as additional props from the child component to the parent component. The parent component can then access these arguments and use them when calling the function.
Q: Is it possible to pass a function as a prop through multiple layers of child components?
A: Yes, it is possible to pass a function as a prop through multiple layers of child components. Each intermediate child component will receive the function as a prop and can further pass it down to its own child components. This way, the function can travel through the component hierarchy until it reaches the desired parent component.
Q: Can I pass a function as a prop to a sibling component?
A: No, in React, data flow occurs from parent components to child components. Siblings do not have direct access to each other’s props. If you need to share state or functions between sibling components, it is recommended to lift the state up to their closest common ancestor component, and then pass the necessary props down to each sibling component.
Q: Is it possible to pass a function as a prop without using a class component?
A: Yes, it is possible to pass a function as a prop without using a class component. React also supports functional components, which are simpler and more lightweight than class components. You can define a function component and pass a function as a prop in the same way as described earlier.
In conclusion, passing a function as a prop from a child component to its parent is a useful feature in React that enables communication between components. By following the steps outlined in this article, you can successfully pass a function as a prop and maintain the unidirectional data flow in your React application.
Keywords searched by users: react pass function as prop Pass function as props React functional component, Pass function as props React TypeScript, Props function React TypeScript, Pass function to component React, Pass function to child component React, This props is not a function, React pass props to child function component, How to pass state as props in React
Categories: Top 64 React Pass Function As Prop
See more here: nhanvietluanvan.com
Pass Function As Props React Functional Component
In React, passing functions as props is a powerful feature that allows you to pass a function from a parent component to a child component. This functionality comes in handy when you want to modify the state of a parent component from a child component or when you want to trigger an action in a parent component based on an event in the child component. In this article, we will explore how to pass functions as props in React functional components.
What are Functional Components in React?
Functional components are a simpler way of writing components in React. They are also known as “stateless” components because they don’t have their own state. Functional components are declared as JavaScript functions and take in props as their arguments. They return JSX, which describes the structure of the component.
Passing Functions as Props
To pass a function as props from a parent component to a child component, you simply need to define the function in the parent component and then pass it as a prop to the child component. Here’s an example:
“`
// ParentComponent.js
import React from “react”;
import ChildComponent from “./ChildComponent”;
function ParentComponent() {
const handleClick = () => {
console.log(“Button clicked!”);
};
return (
);
}
export default ParentComponent;
// ChildComponent.js
import React from “react”;
function ChildComponent(props) {
return (
);
}
export default ChildComponent;
“`
In the above example, we define a function called `handleClick` in the `ParentComponent`. We then pass this function as a prop called `handleClick` to the `ChildComponent`. In the `ChildComponent`, we use this prop in the `onClick` event of a button. So when the button is clicked, the `handleClick` function is called and the message “Button clicked!” is logged to the console.
Modifying Parent Component State
One common use case of passing functions as props is to modify the state of a parent component from a child component. This is useful when you want to update the state of the parent component based on user interactions in the child component. Here’s an example:
“`
// ParentComponent.js
import React, { useState } from “react”;
import ChildComponent from “./ChildComponent”;
function ParentComponent() {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
Count: {count}
);
}
export default ParentComponent;
// ChildComponent.js
import React from “react”;
function ChildComponent(props) {
return (
);
}
export default ChildComponent;
“`
In this example, we have a `ParentComponent` with a state variable called `count` and a function called `incrementCount` to update that state. We pass the `incrementCount` function as a prop to the `ChildComponent` and use it in the `onClick` event of a button. So when the button is clicked, the `incrementCount` function is called, updating the `count` state in the `ParentComponent`.
FAQs
Q: Can I pass multiple functions as props?
A: Yes, you can pass multiple functions as props by defining them in the parent component and passing them to the child component separately.
Q: How can I pass data back from the child component to the parent component?
A: You can achieve this by passing a function from the parent component to the child component as a prop, and then calling that function in the child component with the necessary data as an argument.
Q: Can I pass functions as props to multiple levels of nested components?
A: Yes, you can pass functions as props to multiple levels of nested components. Just make sure to pass it down through each level of components until it reaches the desired child component.
Q: Can I pass functions as props from a child component to a parent component?
A: No, functions can only be passed from a parent component to a child component in React. If you need to pass data from a child component to a parent component, you can achieve this by calling a function in the parent component that was passed as a prop to the child component.
Q: Can I pass functions as props to class components?
A: Yes, you can pass functions as props to class components as well. The process is similar, where you define the function in the parent component and pass it as a prop to the child component.
In conclusion, passing functions as props in React functional components is a powerful feature that allows you to modify the state of a parent component from a child component and trigger actions in the parent component based on events in the child component. By understanding how to pass functions as props, you can create more interactive and dynamic React applications.
Pass Function As Props React Typescript
Passing functions as props enables efficient communication between different components in a React application. By doing so, we can handle events, update states, and trigger actions in the parent component from the child component. This article will explore the concept of passing functions as props in React TypeScript, its benefits, and how to effectively use it in your projects.
#### How to Pass a Function as a Prop
When passing a function as a prop, we need to follow a simple procedure. Firstly, we define the desired function in the parent component. This function can serve any purpose, such as handling an event or updating the state. Here’s an example of a parent component passing a function as a prop:
“`typescript
import React from ‘react’;
interface Props {
onClick: () => void;
}
const ParentComponent: React.FC
const handleClick = () => {
// Handle the click event
onClick();
}
return (
);
}
export default ParentComponent;
“`
In the code snippet above, we define a functional component called `ParentComponent`. It accepts a prop called `onClick`, which is a function of type `() => void`. In this example, we have a button element that triggers the `onClick` function when clicked. Inside the `handleClick` function, we invoke the `onClick` prop.
Now, let’s take a look at how to utilize this prop in a child component:
“`typescript
import React from ‘react’;
interface Props {
onClick: () => void;
}
const ChildComponent: React.FC
return (
Child Component
);
}
export default ChildComponent;
“`
In the child component, we receive the `onClick` prop and assign it to the button’s `onClick` attribute. When the button is clicked in the child component, it will execute the function passed from the parent component.
Now, let’s see how we can use these components in a parent-child relationship:
“`typescript
import React from ‘react’;
import ParentComponent from ‘./ParentComponent’;
import ChildComponent from ‘./ChildComponent’;
const App: React.FC = () => {
const handleClick = () => {
console.log(‘Button clicked’);
// Perform some action
}
return (
);
}
export default App;
“`
In the `App` component, we instantiate the `ParentComponent` and `ChildComponent`. We pass the `handleClick` function as the `onClick` prop to both components. Now, whenever the button is clicked in either the parent or child component, the `handleClick` function will be executed.
#### Benefits of Passing Functions as Props
The approach of passing functions as props brings several advantages to your React TypeScript applications:
1. **Increased Reusability**: By passing functions as props, we can create more reusable components. The same function can be utilized by different components, reducing code duplication and making the development process more efficient.
2. **Parent-Child Communication**: Passing functions as props facilitates communication between parent and child components. The child component can invoke the passed function in the parent component, allowing for seamless data flow and event handling.
3. **Event Handling**: Passing functions as props is an effective method for handling events in React. We can pass event handlers from the parent component to its children, enabling them to perform actions specific to the event.
4. **State Management**: With function props, we can update the state in the parent component from the child component. This enables child components to modify the parent’s state and triggers re-rendering for data updates across the application.
#### FAQs
**Q: Can I pass multiple functions as props to a single component?**
A: Yes, you can pass multiple functions as props to a single component. Simply define the required function props in the component’s interface and utilize them as needed.
**Q: How do I pass arguments along with the function prop?**
A: To pass arguments along with a function prop, you can use an arrow function in the parent component. For example:
“`typescript
“`
**Q: Can I pass a function as a prop to multiple nested levels of child components?**
A: Yes, you can pass a function as a prop to multiple levels of child components. The function can be passed from the parent to any child component in the hierarchy, and each child component can invoke it as needed.
**Q: Is it possible to pass functions as props in class components?**
A: Yes, functions can be passed as props in both functional and class components in React. The syntax for passing functions as props remains the same, regardless of the component type.
In conclusion, passing functions as props in React TypeScript is a powerful approach for achieving efficient communication, event handling, and state management between components. By utilizing this technique, you can enhance reusability, modularity, and development productivity in your React applications.
Images related to the topic react pass function as prop
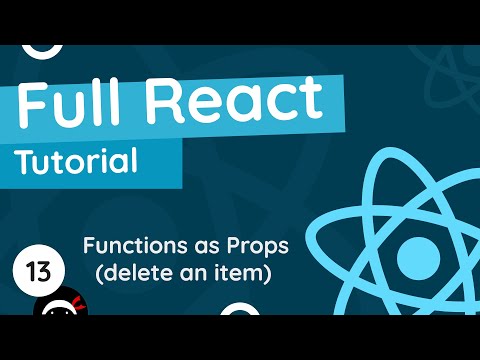
Found 17 images related to react pass function as prop theme




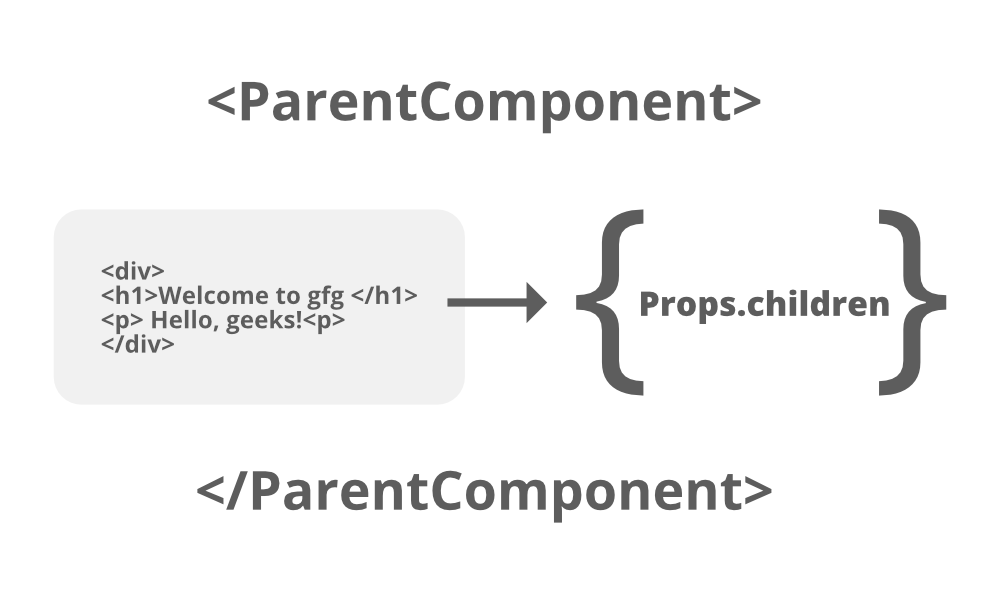
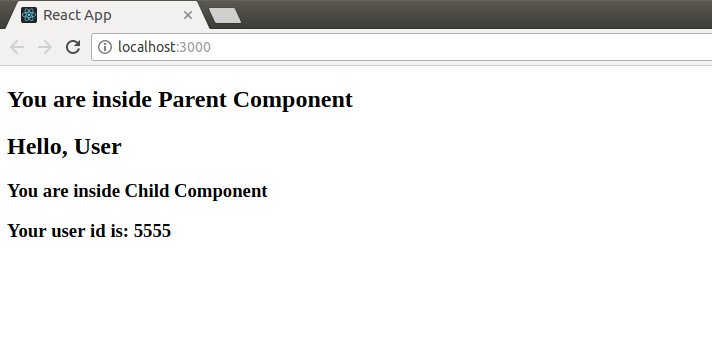
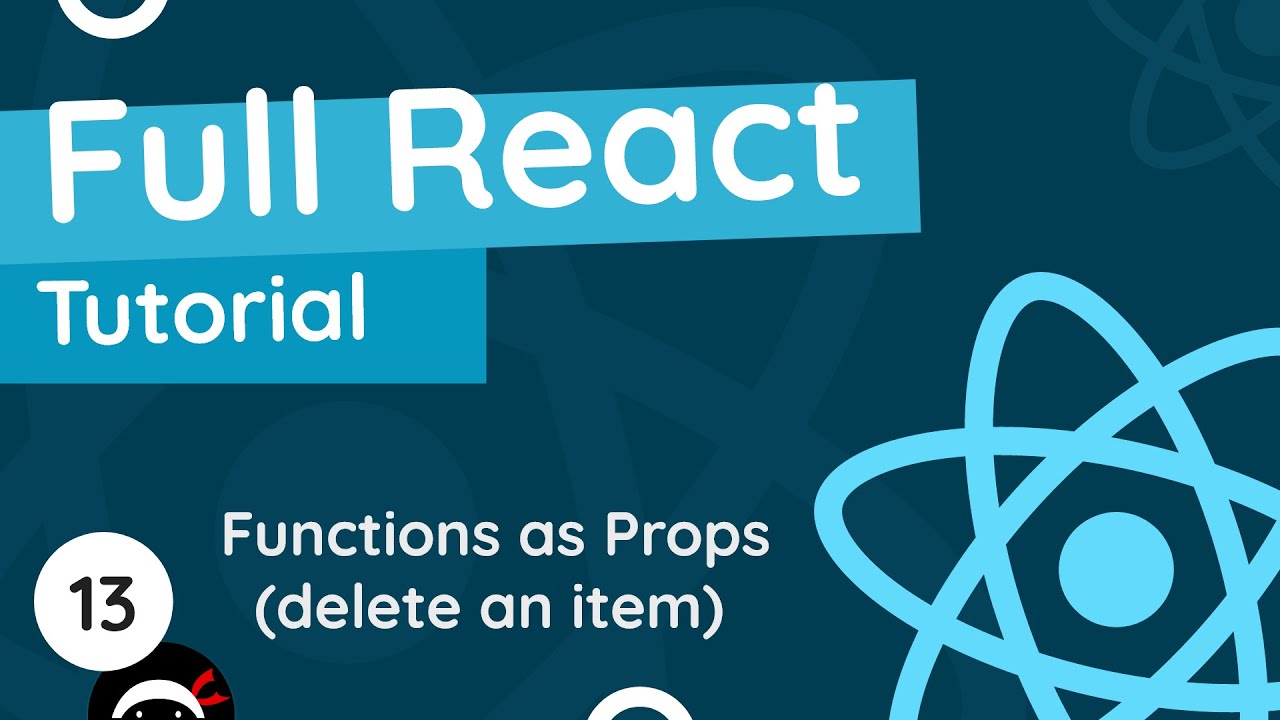
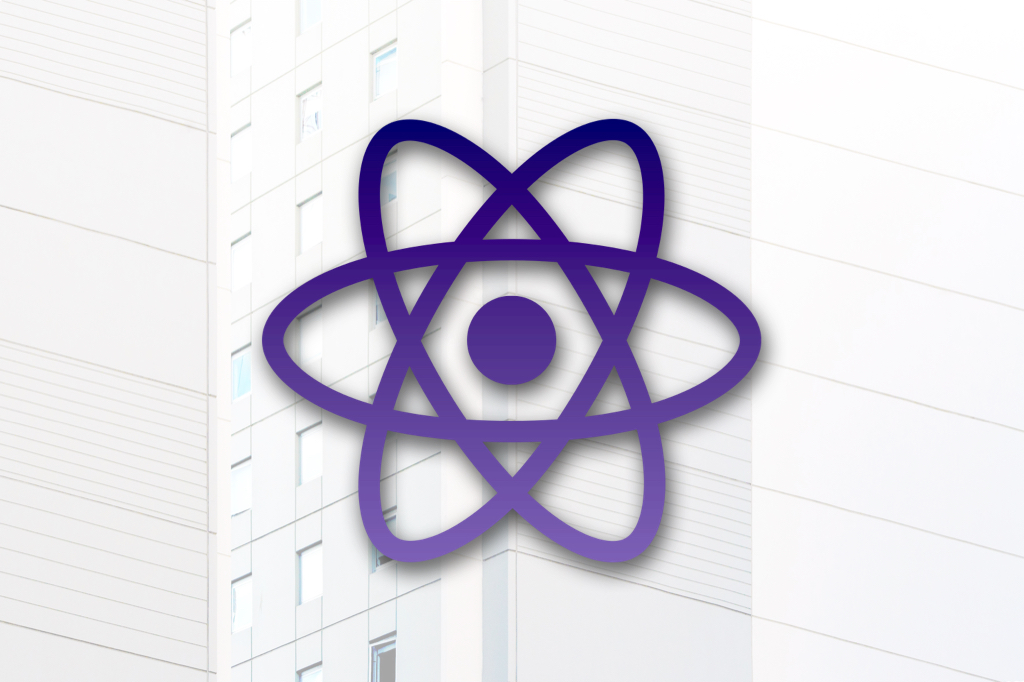
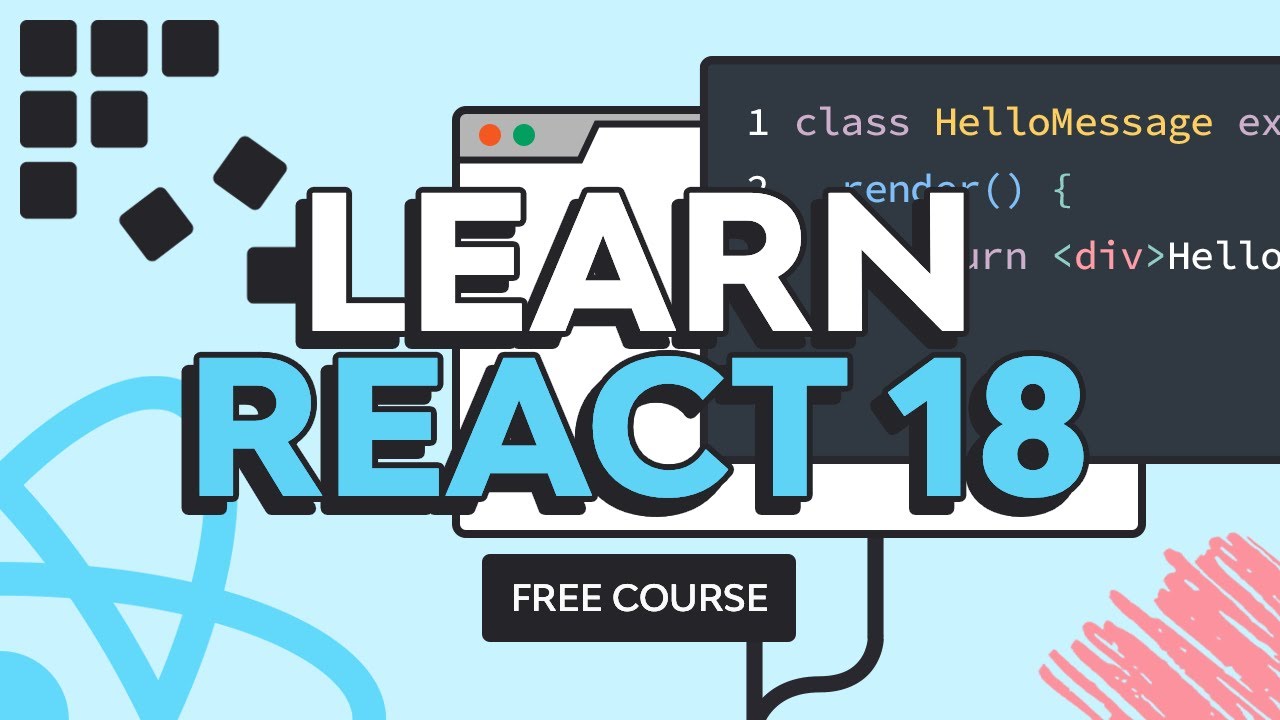


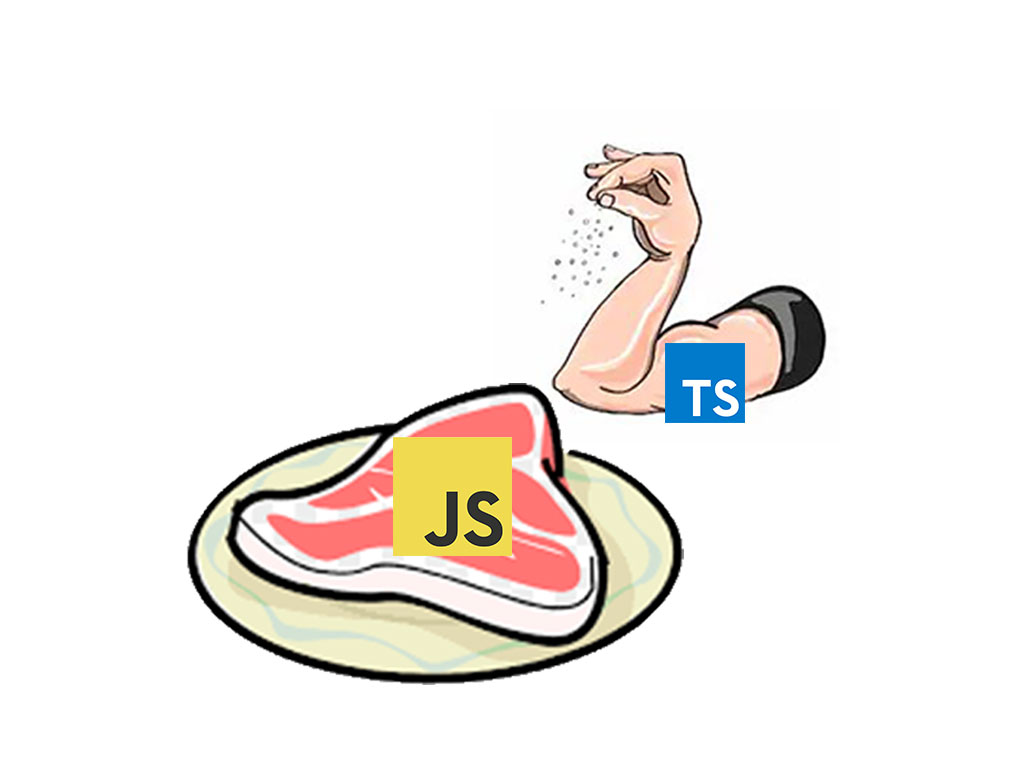
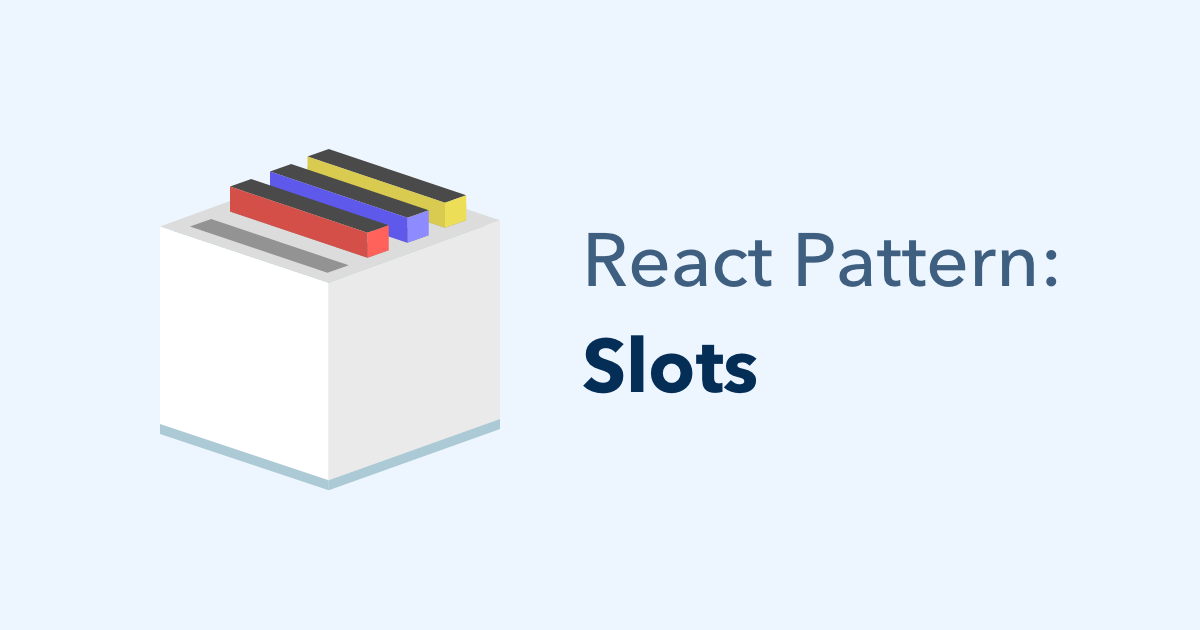



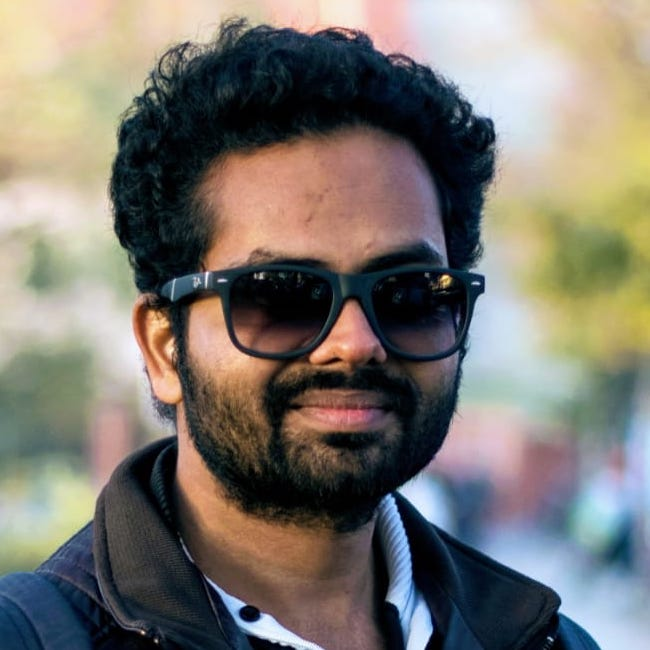
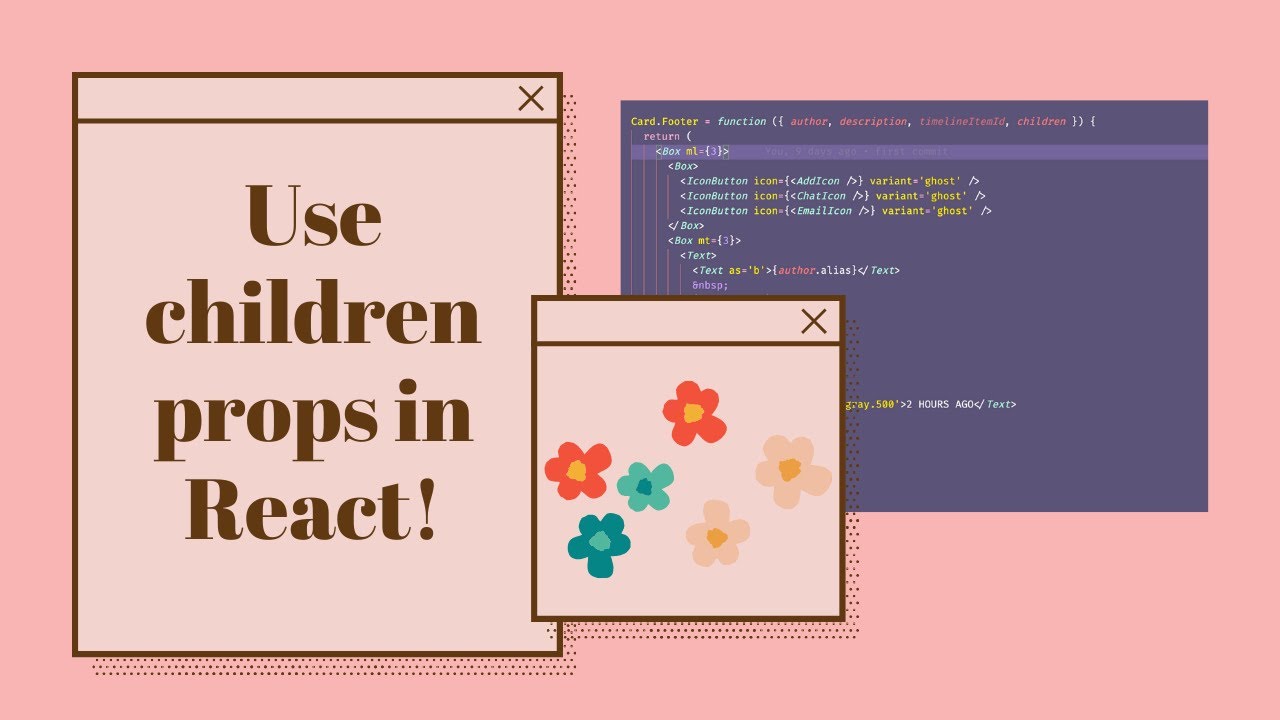

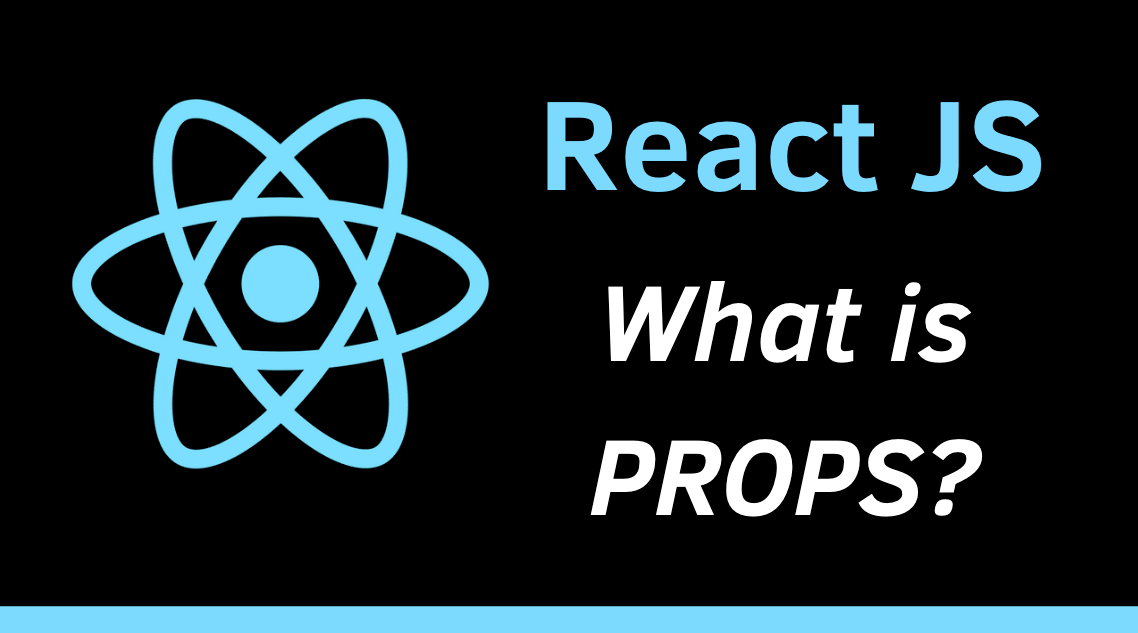



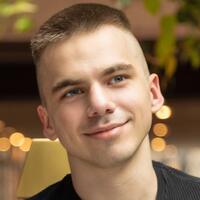
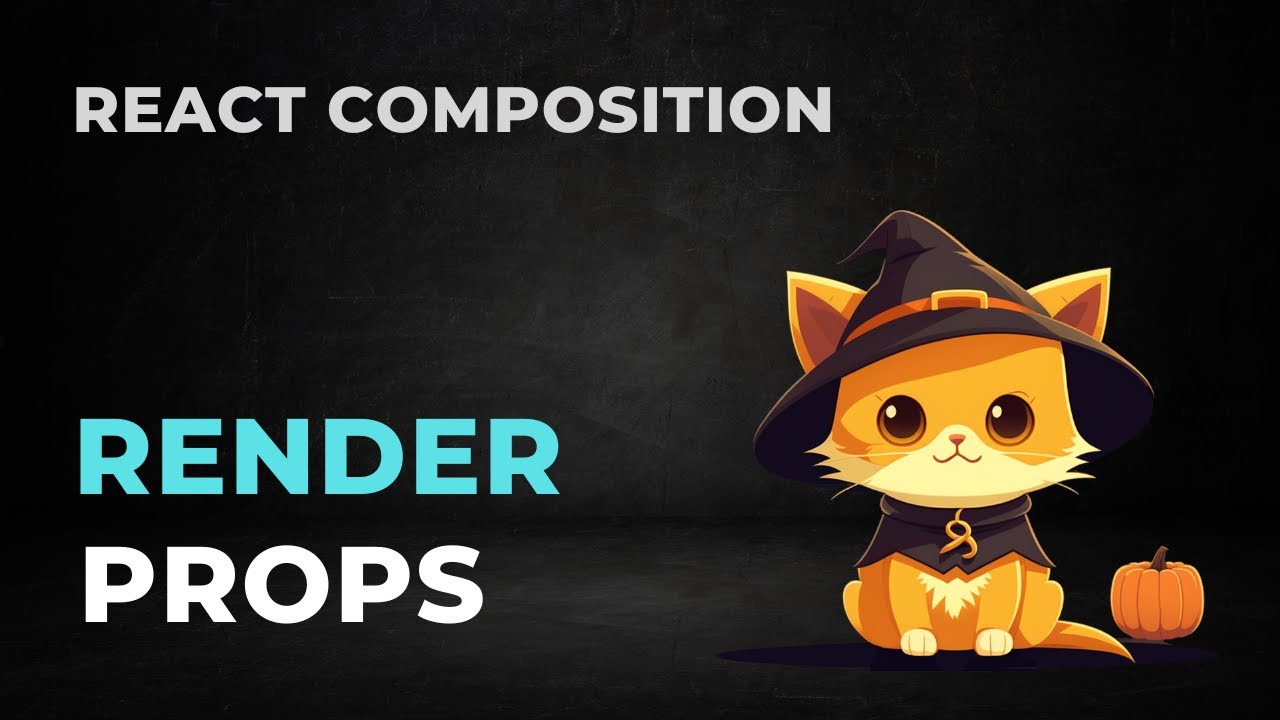
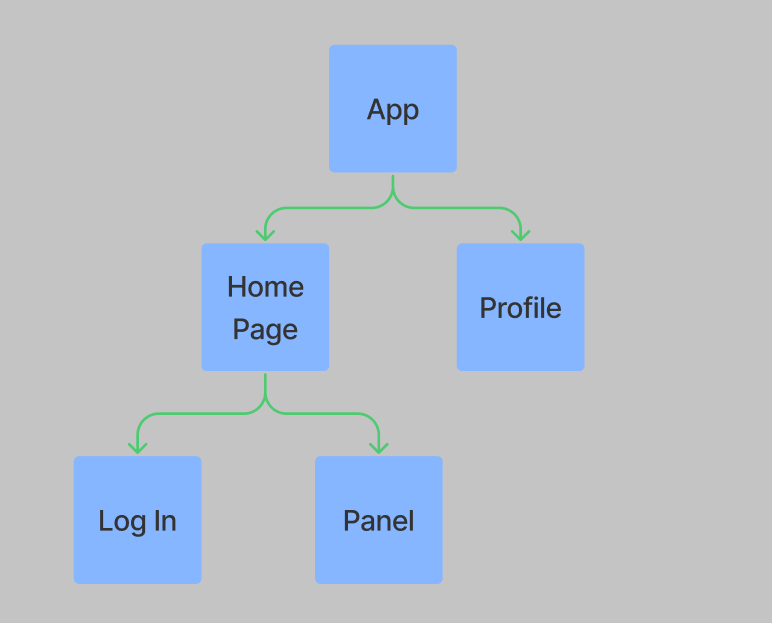


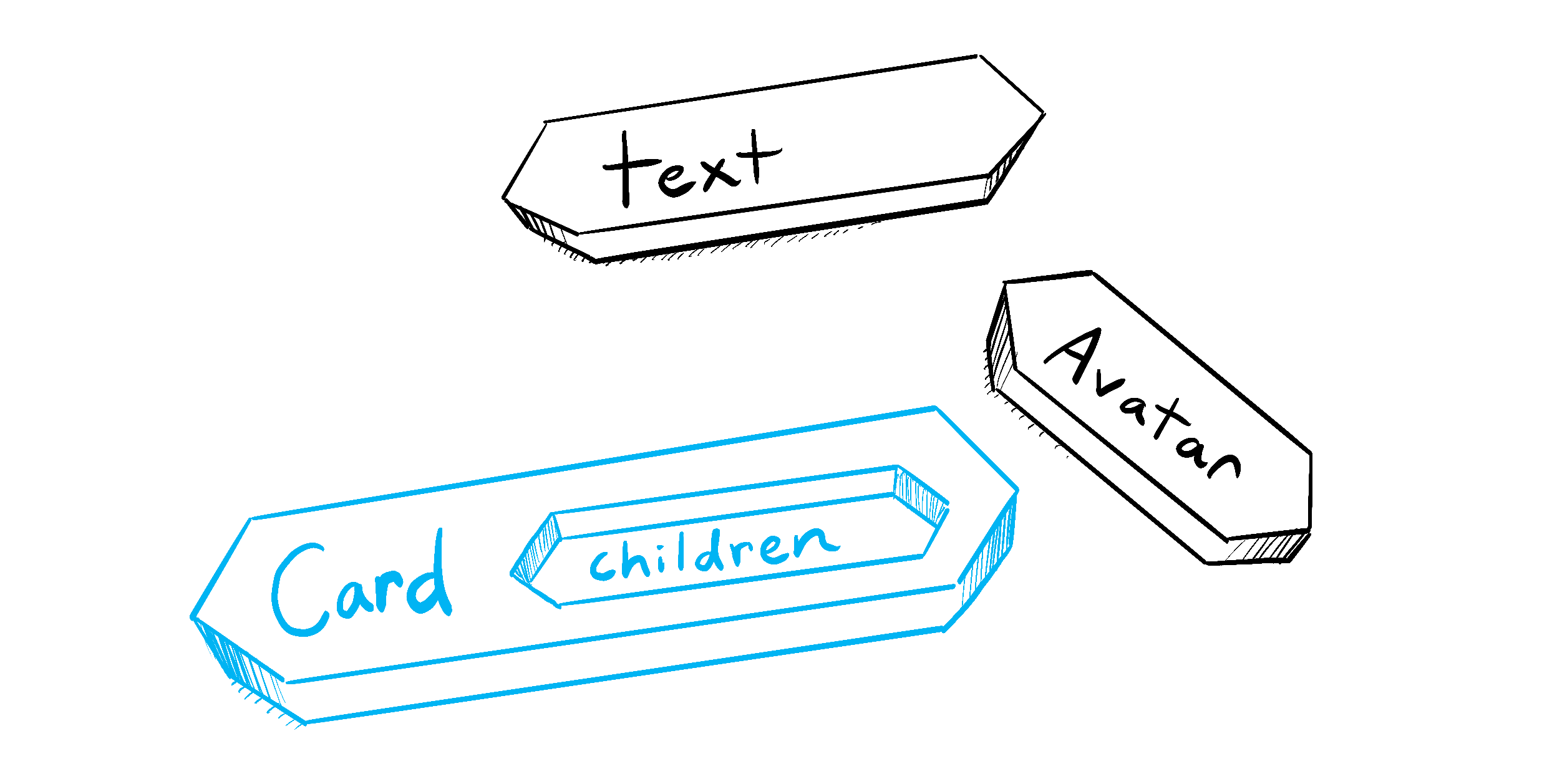

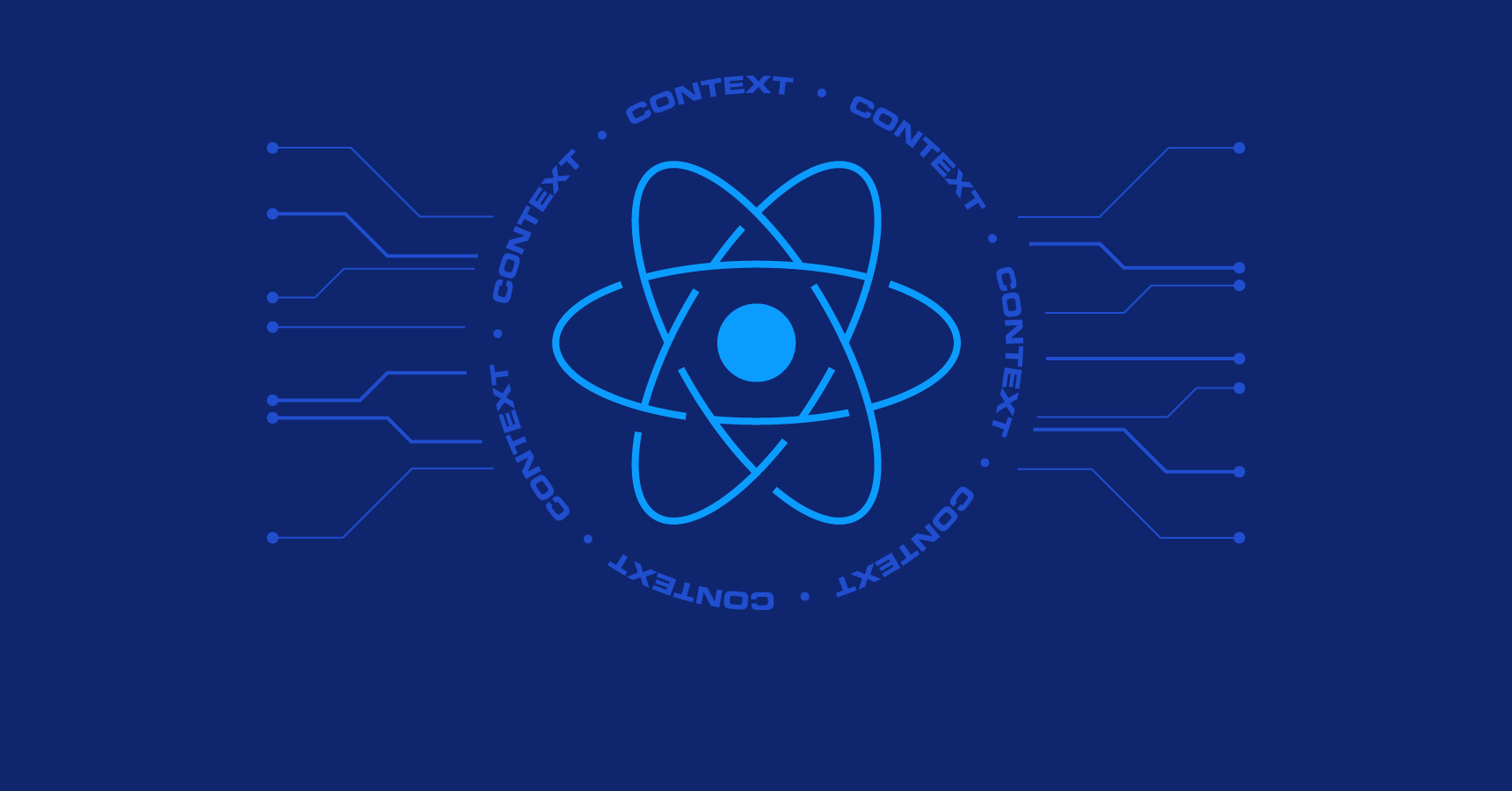
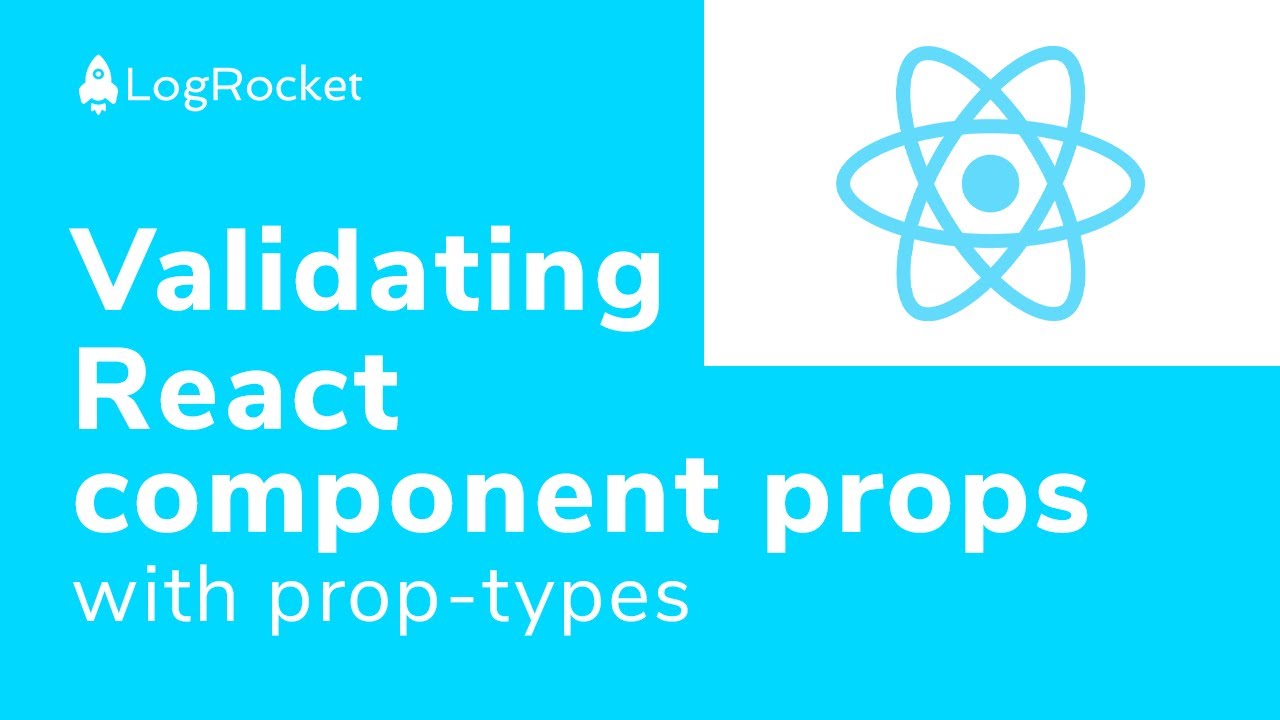
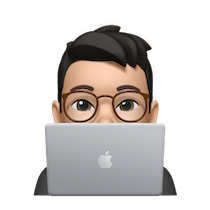
.webp)

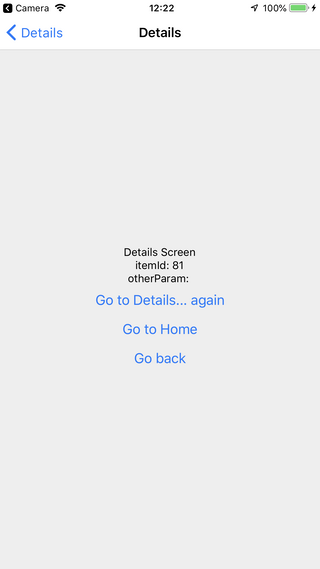
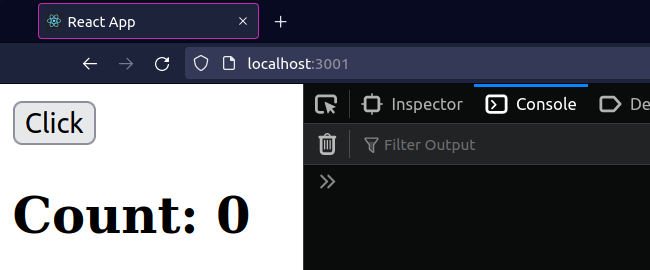
Article link: react pass function as prop.
Learn more about the topic react pass function as prop.
- How to pass a Function as a Prop in React – bobbyhadz
- Passing Functions to Components – React
- How To Pass A Function As A Prop In React?
- reactjs – How to pass function as props from functional parent …
- How to Use and Pass Functions as Props— React – Medium
- How to pass a Function as a Prop in React – bobbyhadz
- Passing data in React between Parent and Child in Functional Components
- Passing a hook as a prop – Stack Overflow
- How To Pass a Function as a Prop in React? – Upmostly
- How to Pass Function as Props in React Functional Component
- Passing Down Functions As Props in React – DEV Community
See more: https://nhanvietluanvan.com/luat-hoc