Random Number Between 1 And 90
Determining the Range
When it comes to generating a random number between 1 and 90, there are several methods and techniques available. The first step is to determine the range in which the number should fall. In this case, the range is clearly defined as numbers from 1 to 90.
Choosing a Suitable Method
Once the range has been determined, it is essential to choose a suitable method for generating the random number. There are various techniques available, each with its own advantages and limitations. Some of the commonly used methods include the Math.random() function, the Random.nextInt() method, and generating a random number sequence in a loop.
The Math.random() Function
One of the most commonly used methods for generating a random number is through the use of the Math.random() function. This function generates a random float between 0 and 1. To obtain a random number within a specific range, the float is then multiplied by the desired range and rounded off to an integer.
Generating a Random Float
To generate a random float using the Math.random() function, a simple formula can be used. For example, to generate a random float between 0 and 1, the formula would be:
randomFloat = Math.random();
Converting to an Integer
Once the random float is generated, it needs to be converted to an integer within the desired range. To do this, the random float is multiplied by the range and rounded off using the Math.round() function.
randomNumber = (int) (randomFloat * range) + 1;
Applying Constraints
In some cases, additional constraints may need to be applied to the randomly generated number. For example, if the number needs to be divisible by a certain value, the generated number can be multiplied by that value and rounded off before dividing it by the same value.
The Random.nextInt() Method
Another widely used method for generating random numbers is through the use of the Random.nextInt() method. This method is part of the Random class in Java and allows for more flexibility and control over the generated numbers.
Setting the Upper Bound
To generate a random number between 1 and 90 using the Random.nextInt() method, the upper bound needs to be set to 90. The syntax for generating the random number would be:
Random random = new Random();
int randomNumber = random.nextInt(90) + 1;
The Random class vs Math.random()
While both methods discussed above can generate random numbers, the Random class provides more control and flexibility. It allows for the setting of an upper bound and produces a more evenly distributed range of random numbers.
Generating a Random Number Sequence in a Loop
Sometimes, it is necessary to generate a sequence of random numbers within a loop. This can be achieved by placing the random number generation code within the loop and repeating it for the desired number of iterations.
For a more comprehensive coverage, let’s explore some frequently asked questions (FAQs) related to generating random numbers between 1 and 90:
FAQs:
Q: Can I generate a random number between 1 and 90 using an online random number generator?
A: Yes, many online random number generators allow you to specify the range. Simply input the desired range of 1 to 90, and the generator will provide you with a random number within that range.
Q: How can I generate a random number between 1 and 90 in Excel?
A: In Excel, you can use the RAND() function in combination with multiplication and rounding to generate a random number between 1 and 90. The formula would be: =ROUNDUP(RAND() * (90-1) + 1, 0).
Q: How can I generate a random number between 1 and 90 in Python?
A: In Python, you can use the random module and the randint() function to generate a random number between 1 and 90. The syntax would be: random.randint(1, 90).
Q: Is there a Star Trek random number generator that can generate a random number between 1 and 90?
A: Yes, there are several Star Trek-themed random number generators available online that can generate random numbers within a specified range. These generators often come with additional features and themes related to the Star Trek series.
Q: Can I generate a random number between 1 and 90 using a random wheel?
A: Yes, a random number wheel can be used to generate a random number within the range of 1 to 90. Simply spin the wheel and the number it lands on will be the generated random number.
In conclusion, generating a random number between 1 and 90 can be accomplished through various methods such as using the Math.random() function, the Random.nextInt() method, or generating a random number sequence in a loop. Each method has its advantages and limitations, and it is up to the user to choose the most suitable one for their specific needs. So go ahead and start generating those random numbers with confidence!
Create A List Of Random Numbers Without Repeats
What Is The Most Random Number Between 1 And 100?
Numbers hold a significant place in our lives, whether we are aware of it or not. From mathematics and science to everyday tasks like setting an alarm or counting money, numbers are integral to our daily routines. They provide structure and order to the world around us. However, amidst this sense of order, there is also a notion of randomness that emerges in the realm of numbers. One intriguing question that often arises is: What is the most random number between 1 and 100?
Exploring the concept of randomness
Before we delve into the search for the most random number between 1 and 100, we must first understand what randomness truly means. Randomness refers to a lack of pattern or predictability. A random number, therefore, is one that cannot be easily predicted or determined beforehand.
In the realm of statistics and probability, random numbers are often used to simulate real-life scenarios or create unbiased samples. These numbers are generated using various methods such as computer algorithms or physical processes. Randomness plays a crucial role in fields like cryptography, scientific research, and even gambling.
The quest for the most random number
Determining the most random number between 1 and 100 is a challenging task. It requires us to consider multiple factors such as distribution, probability, and the generation method employed. One common method to generate random numbers within a range is to use a computer algorithm. However, even these algorithms have limitations and inherent biases.
One might argue that since all the numbers between 1 and 100 appear to be equally likely, any number can be considered equally random. However, this notion quickly becomes questionable as certain patterns or biases might arise within the generated sequence. For example, a computer algorithm might favor even numbers or avoid certain combinations.
The Fibonacci sequence, where each number is the sum of the two preceding ones (1, 1, 2, 3, 5, 8, etc.), is intriguing but not truly random. It exhibits a predictable pattern and cannot be used to find the most random number between 1 and 100.
Another approach to generating random numbers is using physical processes such as atmospheric noise or radioactive decay. These methods are considered more random than computer algorithms, as they are based on unpredictable natural phenomena. However, implementing such methods for generating random numbers between 1 and 100 might be impractical or overcomplicated.
Considering frequency and probability
When analyzing randomness, it is relevant to explore the concept of probability. Probability refers to the likelihood of an event occurring. In the case of numbers between 1 and 100, each number has an equal probability of occurring if generated randomly. Thus, there might not be a single most random number, as all numbers have an equal chance of being selected.
However, if we analyze frequency, as in how often certain numbers appear in random sequences, certain patterns begin to emerge. For example, the number 37 has been highlighted as an unusually frequent occurrence in various studies on random numbers. This occurrence has been associated with human bias and perception. When asked to choose a random number between 1 and 100, individuals often end up selecting 37 due to its middle position.
Understanding the limitations
While exploring the concept of the most random number between 1 and 100, it is essential to realize the limitations of our understanding. Randomness is a complex and abstract concept that might not have a definitive answer. Our perception of randomness might be influenced by biases, patterns, and limitations of the methods used to generate or analyze random numbers.
Further research and analysis might help shed light on any potential patterns that arise from different methods of generating random numbers. However, due to the inherent complexity and limitations of the concept, defining the most random number might remain largely subjective.
FAQs:
Q: Are the numbers generated by computer algorithms truly random?
A: While computer algorithms can generate sequences that appear random, they are ultimately deterministic. They use complex mathematical formulas to produce seemingly random numbers. However, they are predictable and follow a precise pattern determined by the algorithm.
Q: Is it possible to measure randomness objectively?
A: Measuring and quantifying randomness is a challenging task. Various statistical tests have been developed to assess the randomness of a sequence of numbers. However, these tests often focus on specific types of patterns or biases, making it difficult to provide an objective measure of randomness.
Q: Are there practical applications for determining the most random number between 1 and 100?
A: While the search for the most random number may not have immediate practical implications, understanding randomness is crucial in various fields. Random numbers are used in cryptography to secure data, in simulations to model real-world scenarios, and in statistical analysis to create unbiased samples.
Q: Should we consider biases or patterns when searching for the most random number?
A: Biases and patterns are important factors to consider when assessing randomness. Understanding the limitations of the method used to generate random numbers and analyzing frequency distributions can help identify potential biases. However, it is important to remember that true randomness should lack any predictable patterns or biases.
How To Generate Random Numbers Between 1 And 100 In R?
In statistical analysis and simulation studies, generating random numbers is a fundamental task. In the R programming language, there are several ways to generate random numbers. In this article, we will explore various methods that allow us to generate random numbers between 1 and 100 in R.
1. Using the `sample()` Function:
The `sample()` function in R provides a simple way to generate random numbers. With this function, we can generate a random sample of integers within a specified range. By default, it performs sampling without replacement, meaning each generated number will be unique.
To generate random numbers between 1 and 100, we can use the following code:
“`R
random_numbers <- sample(1:100, size = n, replace = FALSE)
```
In the above code, `n` represents the number of random numbers you wish to generate. By changing `n` to a different value, you can generate different sets of random numbers.
2. Using the `runif()` Function:
The `runif()` function generates random numbers from a uniform distribution. This means that the generated numbers are equally likely to occur within a specified range. To generate random numbers between 1 and 100 using `runif()`, we can utilize the following code:
```R
random_numbers <- ceiling(runif(n, 1, 100))
```
Similar to the previous method, `n` represents the number of random numbers we want to generate. By modifying the parameters `1` and `100`, you can change the range of random numbers.
3. Using the `sample.int()` Function:
Another approach to generate random numbers between 1 and 100 is by using the `sample.int()` function. This function returns a random permutation of integers in a specified range. It is generally faster than the `sample()` function when generating a large number of random numbers.
To generate random numbers between 1 and 100 using `sample.int()`, implement the following code:
```R
random_numbers <- sample.int(100, n, replace = FALSE)
```
In this code, `100` represents the upper bound of the range, and `n` is the number of random numbers to generate.
4. Using the `set.seed()` Function:
In statistical analysis, it is often desirable to generate the same sequence of random numbers repeatedly. The `set.seed()` function allows us to set a specific seed value, which generates a reproducible sequence of random numbers. This is particularly useful when we need to share our code with others or replicate our analysis.
Consider the following example:
```R
set.seed(123)
random_numbers <- sample(1:100, n, replace = FALSE)
```
The `set.seed(123)` statement ensures that every time this code is executed, the same sequence of random numbers will be generated. Changing the seed value will result in a different sequence.
5. Generating Random Numbers in Specific Proportions:
In some cases, we may need to generate random numbers with specific proportions. For instance, we might want to create a random sample where each number occurs with equal probability. To achieve this in R, we can use a combination of the `sample()` function and the `rep()` function.
To generate random numbers with equal probabilities between 1 and 100, we can use the following code:
```R
random_numbers <- sample(rep(1:100, each = n/100))
```
In this code, `n` represents the total number of random numbers to generate.
FAQs:
Q1. Can we generate decimal random numbers between 1 and 100?
Yes, we can generate decimal random numbers using the above methods. Instead of specifying a range of integers, you need to change the range to include decimal numbers. For example, `runif(n, 1, 100)` will generate decimal random numbers between 1 and 100.
Q2. Can we generate random numbers between other ranges?
Yes, you can easily modify the code provided to generate random numbers within any desired range. Simply change the lower and upper bounds within the respective functions. For instance, `sample(10:50, n, replace = FALSE)` will generate random numbers between 10 and 50.
Q3. How can we generate random numbers with a specific distribution?
R provides various functions to generate random numbers with specific distributions. For example, the `rnorm()` function generates random numbers from a normal distribution, while the `rexp()` function generates random numbers from an exponential distribution. By understanding the characteristics of different distributions, you can choose the appropriate function for your requirements.
Q4. Can we generate random numbers following a non-uniform distribution?
Yes, R offers a wide range of functions that enable the generation of random numbers following specific non-uniform distributions. Some examples include the `rgamma()` function for the gamma distribution, the `rpois()` function for the Poisson distribution, and the `rbinom()` function for the binomial distribution. By specifying the appropriate parameters, you can generate random numbers that adhere to your desired distribution.
Q5. How can I generate a set of random numbers without replacement?
By default, most of the methods mentioned above generate random numbers without replacement. However, if you want to generate random numbers with replacement, you can modify the `replace` parameter to be `TRUE`.
Generating random numbers in R is a valuable skill when working on statistical analysis, simulation studies, or general programming tasks. By employing the methods discussed in this article, you can easily generate random numbers between 1 and 100, modify the range as needed, and even achieve specific distributions as required. Remember to use the `set.seed()` function when reproducibility is important for sharing or replicating your code.
Keywords searched by users: random number between 1 and 90 1 to 90, Random number, Random wheel 1 90, random number wheel 1-1000, random 1-100, Star Trek random number generator, random số 1-99, Random số 1 999
Categories: Top 47 Random Number Between 1 And 90
See more here: nhanvietluanvan.com
1 To 90
Numbers are an integral part of our lives, and they play a significant role in everyday situations. From counting objects to understanding mathematics, numbers are essential for communication and problem-solving. In this article, we will delve deep into the numbers 1 to 90, providing you with an in-depth understanding of each number and its significance. So, let’s embark on this numerical journey together!
The Numbers 1 to 90:
1. Number 1: The number one symbolizes uniqueness, independence, and new beginnings. It is considered the building block of all other numbers, representing determination and individuality.
2. Number 2: Manifesting duality, balance, and cooperation, the number two signifies harmonious relationships and partnerships. It is believed to have a calming influence when encountered.
3. Number 3: This number represents creativity, self-expression, and enthusiasm. Often associated with social interactions and communication, it fosters a positive and optimistic outlook on life.
4. Number 4: Known for stability, practicality, and hard work, the number four provides a solid foundation for achievement and success. It embodies discipline and responsibility.
5. Number 5: The number five is associated with freedom, adventure, and versatility. It encourages individuals to embrace change and seek excitement and new opportunities.
6. Number 6: Symbolizing love, nurturing, and responsibility, the number six represents harmony and balance within relationships, family, and community.
7. Number 7: Revered for its spiritual significance, the number seven represents wisdom, introspection, and inner growth. It is often regarded as a number of divine perfection.
8. Number 8: Known for its association with abundance, strength, and material success, the number eight is highly regarded in business and financial matters.
9. Number 9: Often considered a symbol of completion and humanitarianism, the number nine embodies empathy, intuition, and enlightenment. It encourages individuals to help others and make a positive impact in the world.
10. Number 10: Comprising the numbers one and zero, the number ten represents new beginnings, potential, and opportunities. It signifies the start of a new cycle and fresh endeavors.
11. Number 11: Often referred to as a master number, the number eleven represents intuition, spiritual awareness, and higher consciousness. It is believed to possess immense energy and a connection to the spiritual realm.
12. Number 12: Known for its representation of time and space, the number twelve holds significance in calendars, divisions, and measurements. It symbolizes completeness and perfection.
13. Number 13: Often considered an unlucky number in many cultures, the number thirteen has an intriguing history. While it carries negative connotations for some, others associate it with transformation and growth.
14. Number 14: Representing harmony and balance, the number fourteen embodies a sense of stability and self-control. It encourages individuals to find equilibrium in various aspects of their lives.
15. Number 15: This number symbolizes charisma, enthusiasm, and adventure. It signifies the pursuit of freedom, individuality, and the exploration of new experiences.
16. Number 16: The number sixteen is associated with intuition, determination, and analytical thinking. It encourages individuals to trust their instincts and make thoughtful decisions.
17. Number 17: Known for its influence on creativity and self-expression, the number seventeen encourages individuals to embrace their unique talents and abilities.
18. Number 18: Symbolizing abundance and financial success, the number eighteen is often associated with career achievements and material wealth.
19. Number 19: The number nineteen signifies transformation, self-discovery, and spiritual growth. It urges individuals to embrace change and seek personal fulfillment.
20. Number 20: Representing cooperation and partnerships, the number twenty symbolizes harmony and balance within relationships and groups. It encourages individuals to collaborate and find common ground.
21. Number 21: Often attributed to luck and new opportunities, the number twenty-one represents innovation and continuous growth. It signifies the development of new ideas and the pursuit of success.
22. Number 22: Similar to the number eleven, the number twenty-two is considered a master number associated with immense power and potential. It symbolizes manifestation and the ability to turn dreams into reality.
23. Number 23: Often associated with creativity and adventure, the number twenty-three embodies a free-spirited and adaptable nature. It represents the pursuit of personal passions and the exploration of new horizons.
24. Number 24: Symbolizing harmony and stability, the number twenty-four embodies the nurturing aspects of family and community. It encourages individuals to prioritize their loved ones and create a conducive environment.
25. Number 25: This number signifies curiosity, adaptability, and versatility. It encourages individuals to embrace change and seek new experiences and knowledge.
26. Number 26: Known for its association with diplomacy and negotiation, the number twenty-six represents fairness and justice. It encourages individuals to find resolutions and compromises.
27. Number 27: Often considered a spiritual number, the number twenty-seven denotes intuition and self-belief. It encourages individuals to trust their inner wisdom and connect with their spirituality.
28. Number 28: Symbolizing abundance and financial stability, the number twenty-eight is often associated with career success and wealth accumulation.
29. Number 29: This number signifies spiritual advancement and intuitive abilities. It encourages individuals to explore their spiritual path and seek higher knowledge.
30. Number 30: Known for its representation of communication and self-expression, the number thirty symbolizes creativity and social interactions. It encourages individuals to express themselves freely.
31. Number 31: Often attributed to creativity, inspiration, and independence, the number thirty-one embodies fearlessness to pursue individual passions and take risks.
32. Number 32: Symbolizing compassion and harmony, the number thirty-two encourages individuals to seek balance in their relationships and prioritize the well-being of others.
33. Number 33: As a master number, the number thirty-three represents inspiration, compassion, and selfless service. It embodies the highest form of love and encourages individuals to make a positive impact on humanity.
34. Number 34: Known for its association with creativity and self-expression, the number thirty-four urges individuals to embrace their unique talents and creative potential.
35. Number 35: Symbolizing adventure and adaptability, the number thirty-five encourages individuals to step out of their comfort zones and explore new opportunities.
36. Number 36: Often attributed to domestic affairs and nurturing, the number thirty-six represents family unity and emotional connections. It encourages individuals to foster harmonious relationships within their homes.
37. Number 37: This number signifies intuition, introspection, and spiritual growth. It urges individuals to seek inner wisdom and trust their intuition.
38. Number 38: Known for its association with business and financial success, the number thirty-eight signifies abundant opportunities for growth and wealth accumulation.
39. Number 39: Often considered a number of transformation and completion, the number thirty-nine symbolizes the end of a significant phase in life and the beginning of a new one.
40. Number 40: Representing stability and determination, the number forty embodies perseverance and self-discipline. It encourages individuals to stay focused on their goals.
41. Number 41: Known for its representation of creativity and inspiration, the number forty-one urges individuals to embrace their artistic abilities and pursue their passions with enthusiasm.
42. Number 42: Symbolizing cooperation and partnerships, the number forty-two encourages individuals to work collaboratively to achieve common goals and find solutions.
43. Number 43: Often attributed to personal growth and self-improvement, the number forty-three represents the urge to expand one’s horizons and seek knowledge.
44. Number 44: As a master number, the number forty-four signifies practicality, hard work, and success. It encourages individuals to channel their efforts into tangible outcomes.
45. Number 45: This number signifies versatility, adaptability, and freedom. It encourages individuals to embrace change and seek new opportunities.
46. Number 46: Known for its representation of responsibility and discipline, the number forty-six encourages individuals to approach their tasks and obligations with determination and focus.
47. Number 47: Often associated with spirituality and intuition, the number forty-seven represents inner wisdom and a deep connection to the spiritual realm.
48. Number 48: Symbolizing practicality and organization, the number forty-eight encourages individuals to create order and structure in their lives.
49. Number 49: Often considered a number of transformation and change, the number forty-nine urges individuals to seek personal growth and embrace new beginnings.
50. Number 50: This number signifies individuality, freedom, and adventure. It encourages individuals to express themselves authentically and explore new experiences.
51. Number 51: Known for its representation of motivation and ambition, the number fifty-one symbolizes the pursuit of personal goals and success.
52. Number 52: Symbolizing adaptability and versatility, the number fifty-two encourages individuals to embrace change and seek new opportunities.
53. Number 53: This number signifies creativity, self-expression, and enthusiasm. It urges individuals to embrace their unique talents and pursue their passions.
54. Number 54: Known for its association with love and nurturing, the number fifty-four represents harmonious relationships and family unity.
55. Number 55: Often attributed to transformation and change, the number fifty-five signifies personal growth and the courage to make necessary life changes.
56. Number 56: Symbolizing practicality and financial stability, the number fifty-six encourages individuals to prioritize their material well-being.
57. Number 57: This number signifies intuition, introspection, and spiritual growth. It urges individuals to seek inner wisdom and trust their inner guidance.
58. Number 58: Known for its association with business and success, the number fifty-eight signifies abundant opportunities in career and financial matters.
59. Number 59: Often considered a number of completion and transformation, the number fifty-nine represents the end of a significant cycle and the beginning of a new one.
60. Number 60: Symbolizing balance and harmony, the number sixty encourages individuals to seek equilibrium in all aspects of their lives.
61. Number 61: This number signifies creativity, inspiration, and new beginnings. It encourages individuals to pursue their passions with enthusiasm.
62. Number 62: Known for its representation of cooperation and partnerships, the number sixty-two signifies collaboration and collective achievements.
63. Number 63: Symbolizing growth and expansion, the number sixty-three signifies personal development and the pursuit of knowledge.
64. Number 64: Often attributed to practicality and organization, the number sixty-four encourages individuals to create structure and order in their lives.
65. Number 65: This number signifies versatility, adaptability, and freedom. It encourages individuals to embrace change and seek new experiences.
66. Number 66: Known for its association with responsibility and nurturing, the number sixty-six represents family unity and emotional connections.
67. Number 67: Often considered a spiritual number, the number sixty-seven signifies the exploration of inner wisdom and a connection to the spiritual realm.
68. Number 68: Symbolizing practicality and financial success, the number sixty-eight encourages individuals to prioritize their material well-being.
69. Number 69: This number represents harmony and balance within relationships. It signifies an emphasis on mutual understanding and compromise.
70. Number 70: Known for its representation of spiritual growth and enlightenment, the number seventy symbolizes the exploration of higher consciousness.
71. Number 71: Symbolizing creativity and self-expression, the number seventy-one encourages individuals to embrace their unique abilities and express themselves authentically.
72. Number 72: Often attributed to cooperation and partnerships, the number seventy-two signifies collaboration and collective success.
73. Number 73: This number signifies introspection, intuition, and spiritual growth. It encourages individuals to seek inner wisdom and trust their inner guidance.
74. Number 74: Known for its association with business success and abundance, the number seventy-four signifies opportunities for growth and financial stability.
75. Number 75: Symbolizing adaptability and versatility, the number seventy-five encourages individuals to embrace change and seek new opportunities.
76. Number 76: Often considered a number of stability and practicality, the number seventy-six represents the value of organization and discipline.
77. Number 77: This number signifies spiritual awakening and inner growth. It encourages individuals to delve deep into their spiritual journey.
78. Number 78: Known for its representation of wealth and financial success, the number seventy-eight encourages individuals to prioritize their material well-being.
79. Number 79: Symbolizing transformation and change, the number seventy-nine signifies personal growth and new beginnings.
80. Number 80: Often attributed to balance and harmony, the number eighty represents equilibrium and a well-rounded approach to life.
81. Number 81: This number signifies creativity, inspiration, and personal growth. It encourages individuals to embrace their unique abilities.
82. Number 82: Known for its association with collaboration and cooperation, the number eighty-two signifies collective achievements and successful partnerships.
83. Number 83: Symbolizing introspection and spiritual growth, the number eighty-three encourages individuals to seek inner wisdom and connect with their spirituality.
84. Number 84: Often considered a number of practicality and organization, the number eighty-four represents the value of structure and order.
85. Number 85: This number signifies versatility, adaptability, and the pursuit of freedom. It encourages individuals to navigate through change and seek new experiences.
86. Number 86: Known for its representation of responsibility and discipline, the number eighty-six emphasizes the value of hard work and perseverance.
87. Number 87: Symbolizing spiritual awakening and enlightenment, the number eighty-seven encourages individuals to explore their spiritual path and seek higher wisdom.
88. Number 88: Often attributed to financial success and abundance, the number eighty-eight signifies immense wealth and opportunities for prosperity.
89. Number 89: This number signifies personal transformation, achievement, and progress. It encourages individuals to embrace change and seek personal growth.
90. Number 90: Known for its representation of completion and fulfillment, the number ninety symbolizes the end of a significant phase in life and the realization of one’s goals.
FAQs:
Q: Are there any superstitions or cultural beliefs associated with the numbers 1 to 90?
A: Yes, different cultures have varying beliefs and interpretations of numbers. For example, in Western cultures, the number 13 is often considered unlucky, while in China, the number 8 is considered particularly auspicious.
Q: Are there any significant historical or mathematical facts related to the numbers 1 to 90?
A: Several significant historical and mathematical facts are associated with these numbers. For instance, the number 1 is called “unity” and is the basis of all mathematics. The number 90 represents a right angle in geometry.
Q: How can we apply the knowledge of numbers 1 to 90 in our daily lives?
A: Understanding the significance of numbers can help us make informed decisions, improve our relationships, and cultivate a positive mindset. It can also be useful in various fields such as finance, business, and even personal development.
Q: Are there any special events or celebrations related to these numbers?
A: While there may not be specific celebrations tied to these numbers, they hold significance in various cultural and religious contexts. For example, the number 40 is often associated with a period of trial or preparation in many religious traditions.
Q: Can numerology be applied to the numbers 1 to 90?
A: Yes, numerology can be applied to any number, including 1 to 90. Numerology explores the influence of numbers on individuals’ personalities, life paths, and relationships.
Q: Are there any popular expressions or idioms related to these numbers?
A: Yes, numbers are often used in expressions and idioms. For example, “two’s company, three’s a crowd,” “lucky number seven,” or “ten out of ten.”
Q: Is there any cultural significance associated with these numbers around the world?
A: Yes, different cultures attach diverse meanings and symbolism to numbers. For instance, the number four is considered unlucky in some East Asian cultures due to its pronunciation similarity to “death.”
In conclusion, the numbers 1 to 90 hold immense significance and symbolism in our lives. Each number encompasses unique traits, contributions, and lessons. Understanding these numbers can empower individuals to make informed decisions, deepen their relationships, and find meaning in their daily experiences. So, let’s embrace the world of numbers and unravel the secrets they hold!
Random Number
In our everyday lives, we are surrounded by numbers. From the time we wake up till we close our eyes at night, numbers govern our routines and decisions. From the clock on our walls to the distance we travel, numbers are a constant presence. But have you ever wondered about random numbers? What makes them so intriguing and mysterious? In this article, we aim to explore the concept of random numbers, shedding light on their importance, applications, and the science behind generating them.
What are Random Numbers?
Random numbers, as the name suggests, are numbers that are chosen without any discernible pattern or predictability. They are the epitome of unpredictability, appearing scattered and chaotic. The absence of a pattern makes them valuable in numerous fields, especially in computer science, cryptography, simulations, and statistical analysis. Generating truly random numbers, however, turns out to be quite a challenge.
True Randomness vs. Pseudorandomness
There are two types of random numbers: true random numbers and pseudorandom numbers. True randomness refers to numbers that are entirely unpredictable and are a result of natural phenomena. These include atmospheric noise, radioactive decay, and other random processes in the universe. Pseudorandom numbers, on the other hand, are generated through algorithms that use a starting value called a seed. While pseudorandom numbers may appear random, they are ultimately predictable as they are based on an algorithm. For most purposes, pseudorandom numbers suffice, as generating true random numbers is more complex and resource-intensive.
Applications in Computer Science and Cryptography
Random numbers play a vital role in computer science and cryptography. In computer programming, they are used for simulation, game design, randomness-based algorithms, and statistical analysis. Random numbers ensure that a program behaves differently each time it runs, adding an element of unpredictability and realism.
Cryptography heavily relies on random numbers to generate keys for encryption and secure data transmission. A strong cryptographic system relies on truly random numbers to protect sensitive information from potential adversaries. Weak or predictable random numbers can compromise the entire security foundation of a cryptographic system.
The Science Behind Generating Random Numbers
Generating random numbers, particularly true random numbers, is no easy task. In the quest for randomness, scientists and programmers employ various techniques. Here are a few methods commonly used:
1. Physical Phenomena: Researchers leverage natural phenomena, such as atmospheric noise, radio signals, and radioactive decay, to generate random numbers. These processes are deemed truly random, as they are influenced by unpredictable factors.
2. Software Algorithms: As mentioned earlier, pseudorandom numbers are generated using algorithms. These algorithms utilize a seed value to produce a sequence of seemingly random numbers. However, due to the deterministic nature of algorithms, the generated numbers will eventually repeat the same pattern unless the seed is changed.
3. Hardware Devices: Specialized hardware devices, known as random number generators (RNGs), are designed to generate random numbers. RNGs use physical processes like timing variations in electronic components or unpredictable quantum phenomena to achieve true randomness. These devices are often used when higher security is required, but they can be expensive and less accessible.
4. Noise-Based Techniques: Another method uses the statistical properties of noise, such as white noise. By measuring and analyzing noise, random numbers can be generated. This technique is widely used in analog-to-digital converters and some software implementations.
FAQs about Random Numbers
Q: Are random numbers truly random?
A: True randomness, as observed in nature, is difficult to achieve. Most random numbers we encounter in computer applications are pseudorandom, generated through algorithms. However, they appear random for most practical purposes.
Q: Can random numbers be predicted or hacked?
A: Pseudorandom numbers generated through algorithms can be predicted if the seed value is known. While it may be time-consuming and computationally expensive, given enough resources, it is possible to predict the sequence of pseudorandom numbers. True random numbers, generated through physical phenomena, are considered unpredictable.
Q: Why are random numbers used in statistical analysis?
A: Random numbers are used in statistical analysis to introduce variability and simulate real-life scenarios. They are vital in ensuring unbiased and representative sampling in research studies.
Q: What is the significance of random numbers in simulations?
A: Simulations aim to replicate real-life scenarios and predict their outcomes. Random numbers introduce randomness and uncertainties into these simulations, making them more realistic and useful for decision-making.
Q: How are random numbers important in gambling and gaming?
A: Random numbers are crucial in the world of gambling and gaming. They ensure fair play and unbiased outcomes, preventing any predictability or manipulation.
In conclusion, random numbers hold great importance in various domains, ranging from cryptography to statistical analysis. While true randomness may be elusive, pseudorandom numbers generated through algorithms serve most practical purposes. The ability to introduce unpredictability and chaos not only brings realism to computer simulations and games but also safeguards sensitive information. Randomness, it seems, is the key to unlocking countless possibilities in the world of numbers.
Images related to the topic random number between 1 and 90
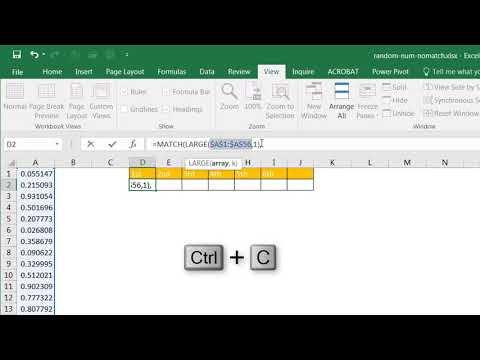
Found 24 images related to random number between 1 and 90 theme

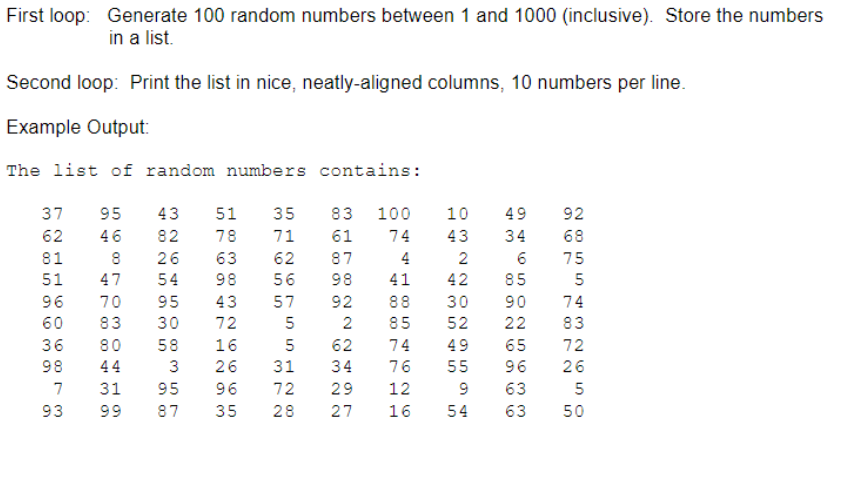
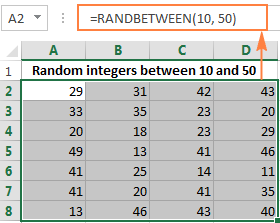
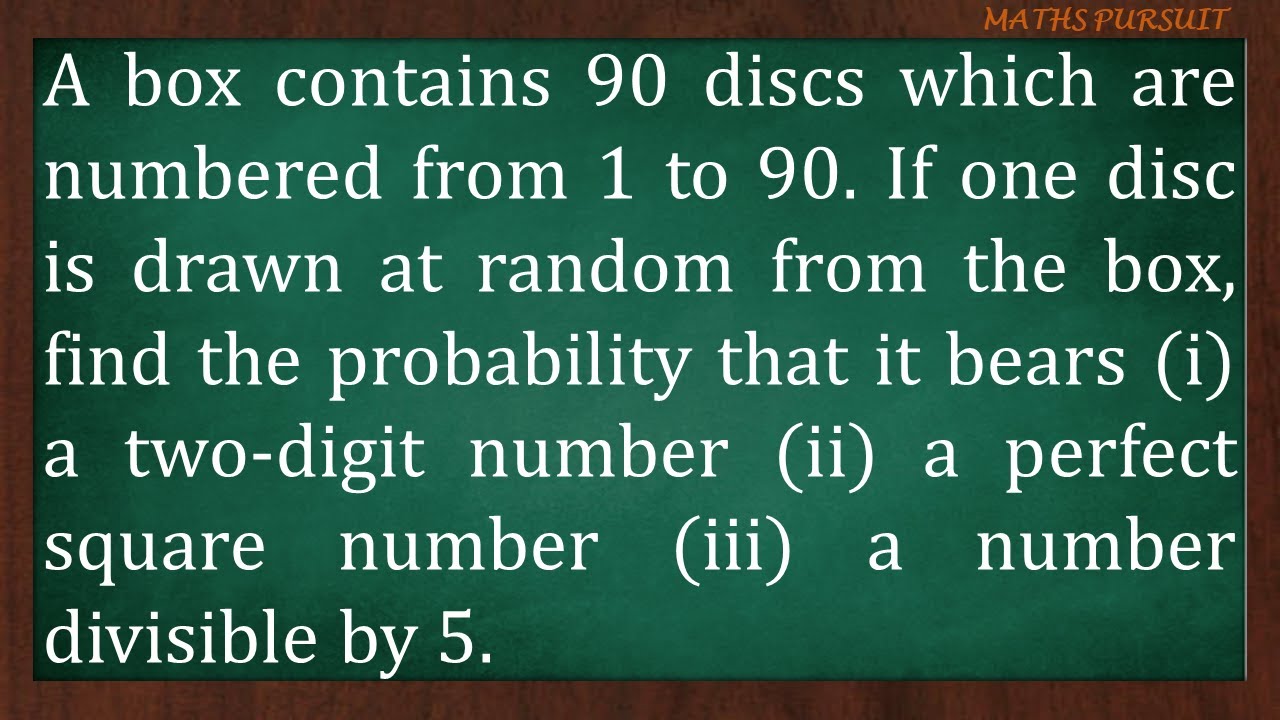

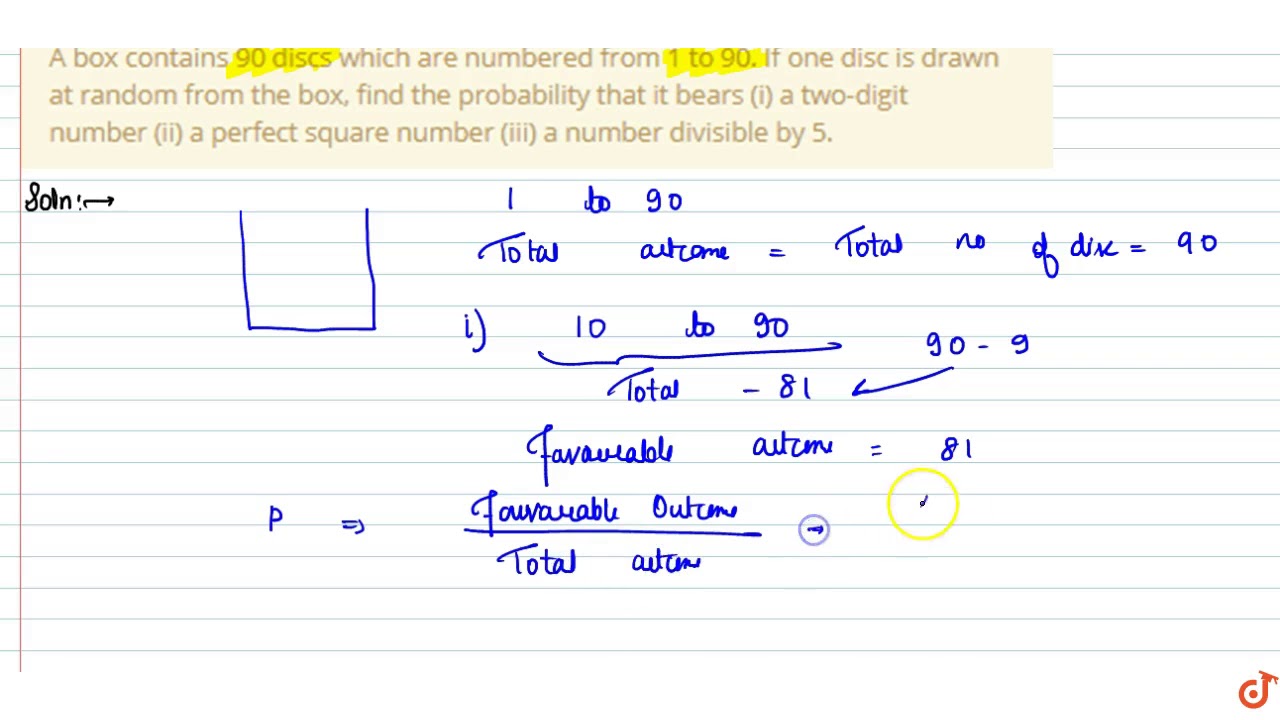
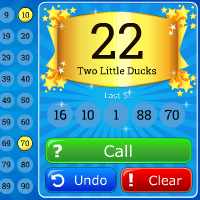


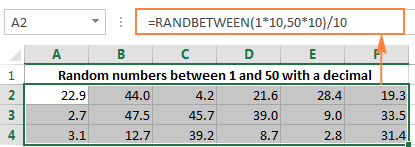
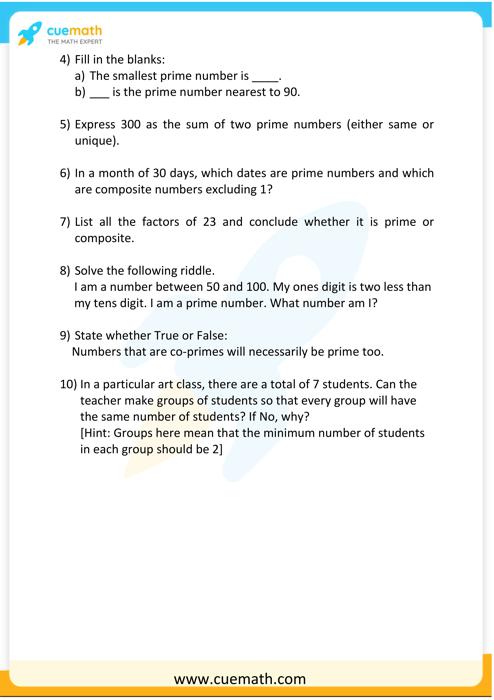

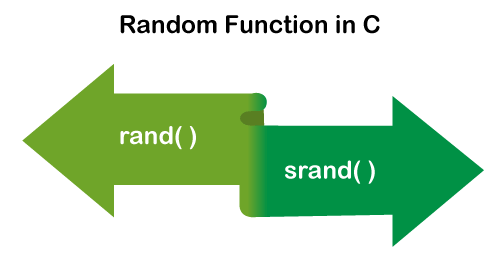
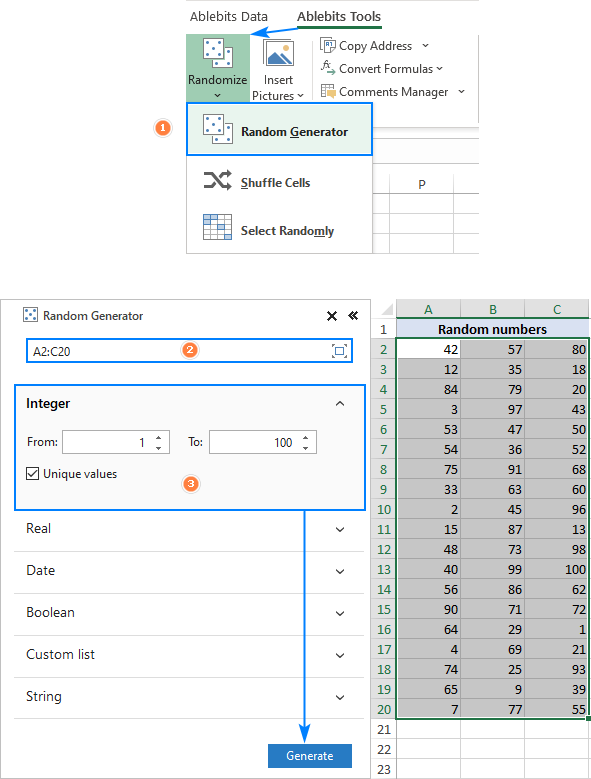
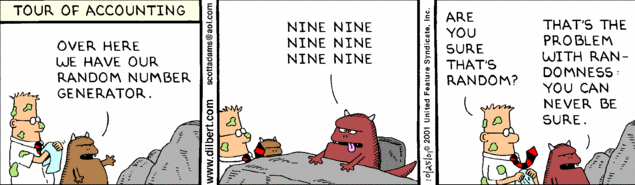

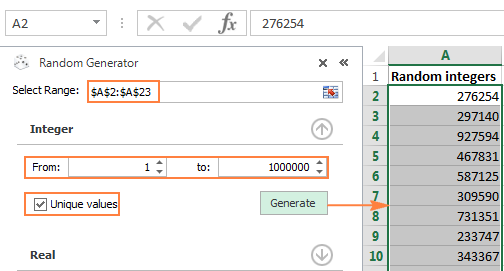
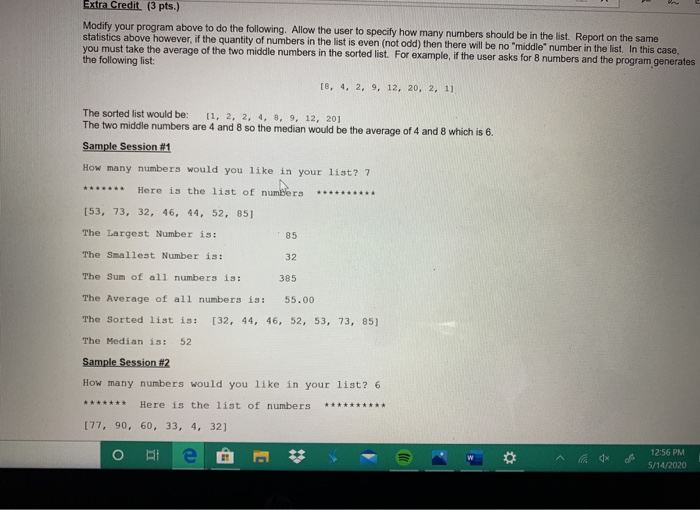
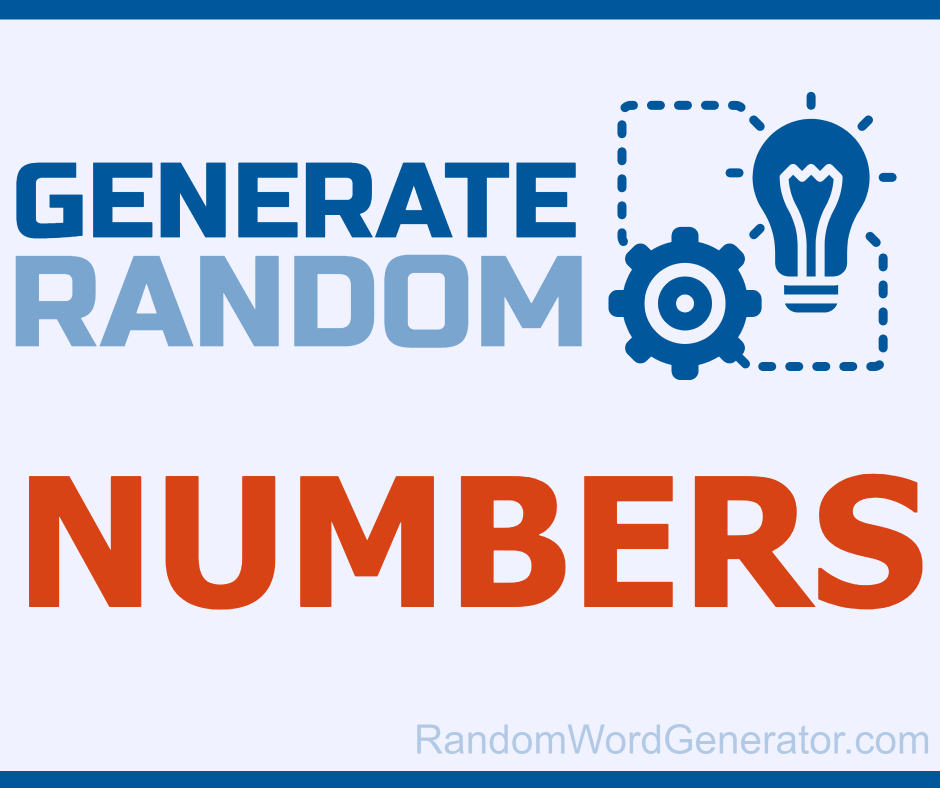
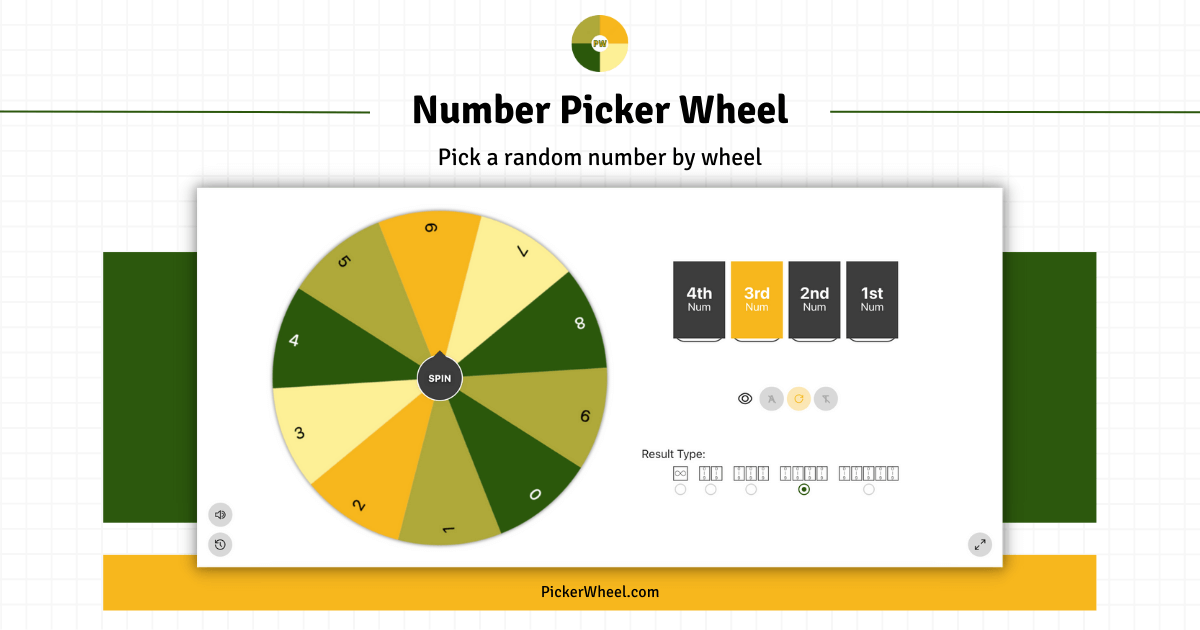
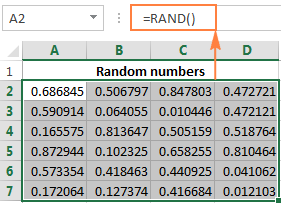

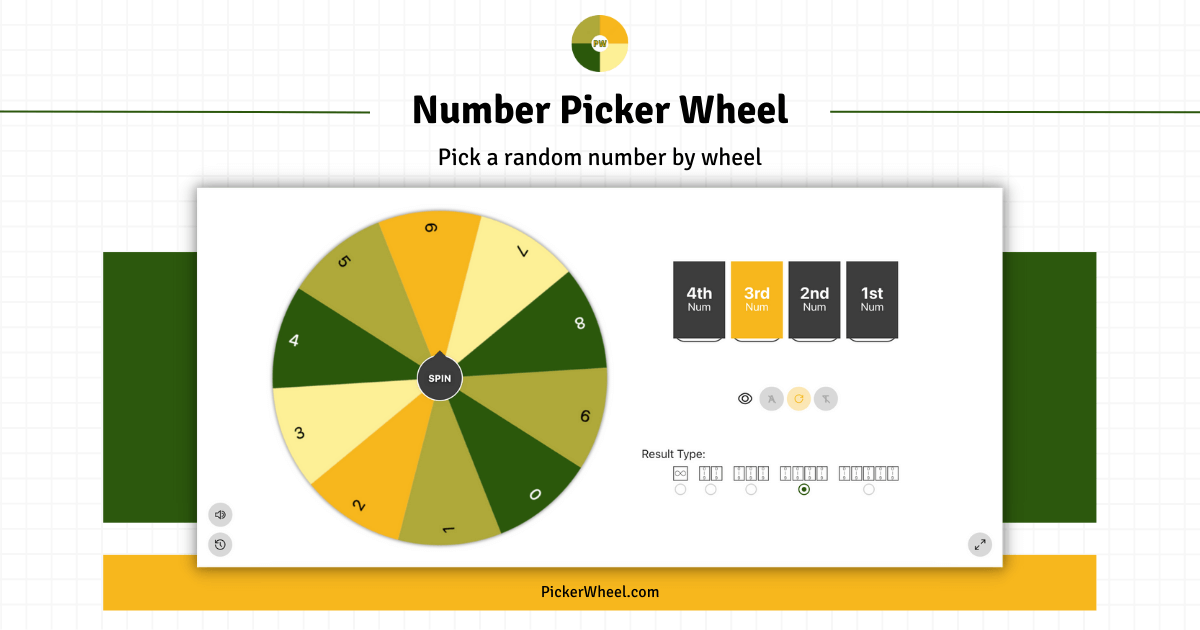
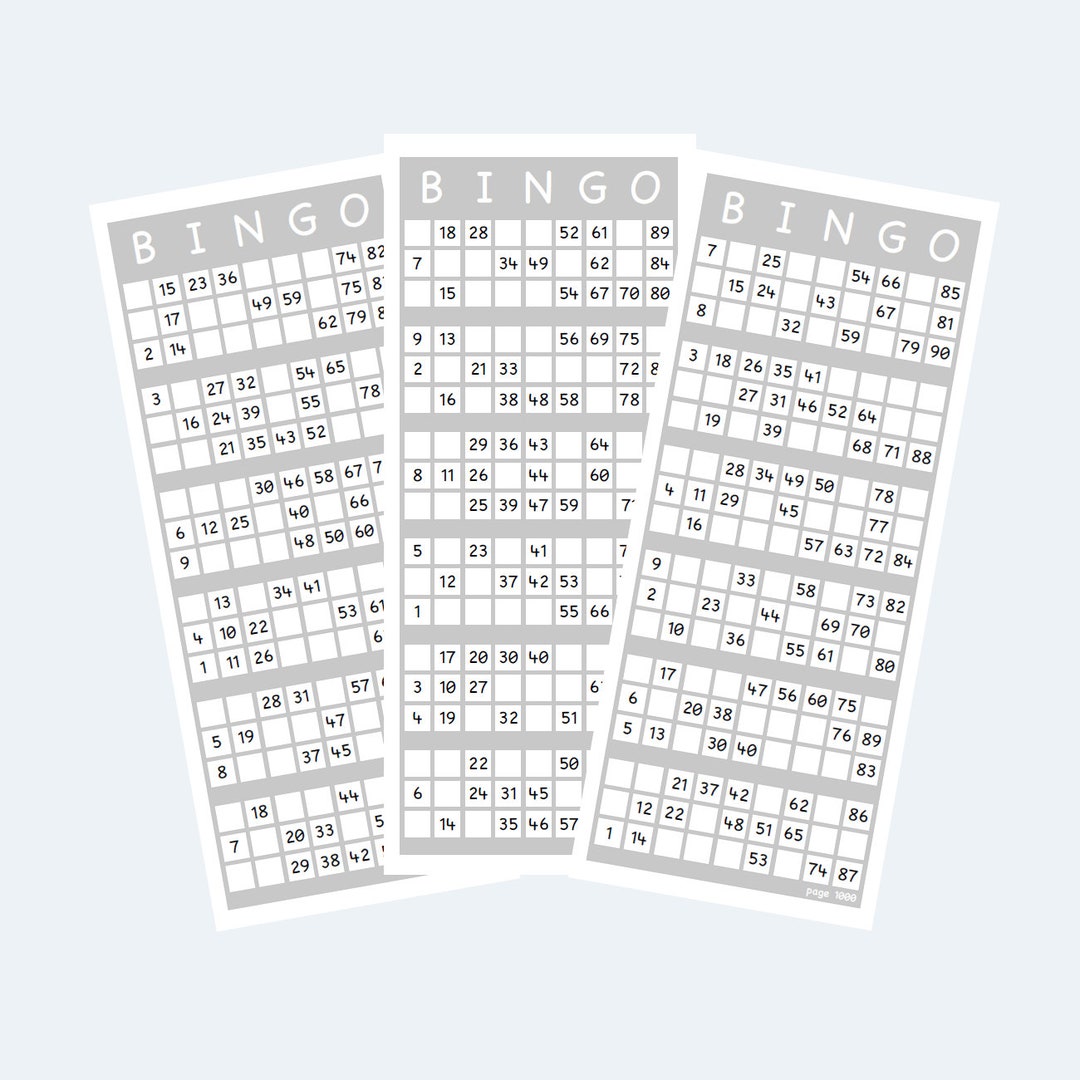

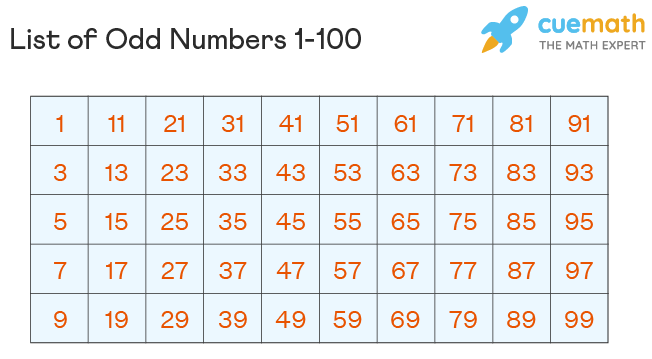
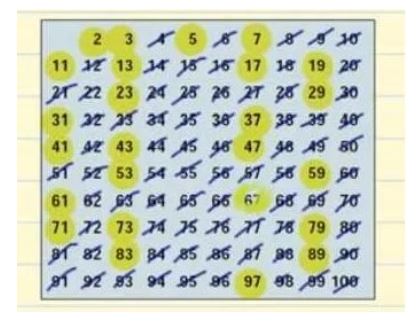
Article link: random number between 1 and 90.
Learn more about the topic random number between 1 and 90.
- Random Number between 1 and 90 – Number Generator
- Random Number Generator: 1-90 – calcpark.com
- Random Number Generator 1-90 – Online Calculator
- random numbers – Catb.org
- Random Number Generator in R | 4 Main Functions of … – eduCBA
- Random function in C – Coding Ninjas
- Random Function in C – Javatpoint
- Generate 6 random numbers from 1 to 90 – AVKG
- 1 Random Numbers between 1 – 90
- Random number generator, rng, roll number picker – MainFacts
- 1-90 Random Number Generator
- Your 3 90 Lottery Numbers – Lucky Number Generator
- Quay số ngẫu nhiên online • Random Number Generator 2023
- Number Picker Wheel – Pick Random Number by Spinning
See more: nhanvietluanvan.com/luat-hoc