Random Number 1 To 41
Generating Random Numbers in Python
Python provides a built-in module called “random” which offers various functions for generating random numbers. Let’s discuss some of the most commonly used techniques.
1. Importing the random module:
To use the random module in Python, you need to import it first. You can do this by adding the following line at the beginning of your code:
“`python
import random
“`
2. Using the random.randint() function:
The `random.randint()` function generates random integers between the specified start and end values, inclusive. To generate a random number between 1 and 41, you can use the following code:
“`python
random_number = random.randint(1, 41)
“`
This will generate a random integer between 1 and 41, including both endpoints.
3. Using the random.random() function:
The `random.random()` function generates random floating-point numbers between 0 and 1. You can modify the generated number to obtain the desired range. To generate a random number between 1 and 41, you can use the following code:
“`python
random_number = random.random() * 40 + 1
“`
In this case, `random.random()` generates a number between 0 and 1, which is then multiplied by 40 and incremented by 1 to obtain a number between 1 and 41.
4. Generating random numbers within a specific range:
If you need to generate random numbers within a specific range, other than 1 to 41, you can use the `random.randrange()` function. It takes three arguments: start, stop, and step. The function generates random integers starting from the “start” value and incrementing by “step” until it reaches or exceeds the “stop” value. Here’s an example code that generates random numbers between 10 and 50, incrementing by 5:
“`python
random_number = random.randrange(10, 51, 5)
“`
This will generate random numbers like 10, 15, 20, 25, …, 45, 50.
5. Generating random floating-point numbers:
If you need to generate random floating-point numbers within a specific range, you can use the `random.uniform()` function. It takes two arguments: start and end. The function generates random floating-point numbers between the start and end values. Here’s an example code that generates random numbers between 2.5 and 7.5:
“`python
random_number = random.uniform(2.5, 7.5)
“`
This will generate random numbers like 3.2, 6.1, 5.9, etc.
6. Generating random numbers from a custom sequence:
You can use the `random.choice()` function to select a random element from a given sequence. Here’s an example code that generates random numbers from a list of values:
“`python
numbers = [1, 5, 9, 13, 17, 21, 25, 29, 33, 37, 41]
random_number = random.choice(numbers)
“`
This will select a random number from the list and assign it to the `random_number` variable.
7. Generating a random number from a weighted sequence:
If you have a sequence with weighted elements, you can use `random.choices()` function to select random elements based on their weights. Here’s an example code that generates random numbers from a weighted list:
“`python
numbers = [1, 2, 3]
weights = [0.1, 0.8, 0.1]
random_number = random.choices(numbers, weights)[0]
“`
This will generate random numbers with more chances of getting 2 than 1 or 3, due to the higher weight assigned to it.
8. Generating a random number with a specific probability distribution:
If you need to generate random numbers that follow a specific probability distribution, you can use various functions from the `random` module, such as `random.normalvariate()` for generating numbers from a normal distribution or `random.expovariate()` for generating numbers from an exponential distribution. These functions require additional parameters to define the distribution, such as mean and standard deviation for the normal distribution.
9. Generating random numbers with a seed value:
If you want to generate the same sequence of random numbers each time you run your program, you can set a seed value using the `random.seed()` function. The seed value can be any hashable object. For example, to generate the same sequence of random numbers every time, you can use the following code:
“`python
random.seed(42)
“`
After setting the seed value, subsequent calls to random functions will produce the same sequence of random numbers.
10. Generating a list of unique random numbers:
If you need to generate a list of unique random numbers, you can use the `random.sample()` function. It takes two arguments: a sequence and the number of unique elements to be selected from the sequence. Here’s an example code that generates a list of 10 unique random numbers between 1 and 100:
“`python
unique_numbers = random.sample(range(1, 101), 10)
“`
This will generate a list containing 10 random numbers, each of which is unique and lies between 1 and 100.
Now that we have covered various techniques for generating random numbers in Python, let’s move on to the frequently asked questions section.
FAQs (Frequently Asked Questions)
Q1. How can I generate random numbers between 1 and 42?
To generate random numbers between 1 and 42, you can use the `random.randint()` function as shown earlier. Here’s the code:
“`python
random_number = random.randint(1, 42)
“`
Q2. How can I generate random numbers between 1 and 40?
Similar to generating numbers between 1 and 42, you can use `random.randint(1, 40)` to generate random numbers between 1 and 40.
Q3. How can I generate a random number from 1 to 41 using a random wheel?
A random wheel is not a standard functionality provided by the random module in Python. However, you can create your own random wheel using a list and the `random.choice()` function. Here’s an example code that demonstrates this:
“`python
numbers = [1, 2, 3, …, 41] # Replace … with the remaining numbers
random_number = random.choice(numbers)
“`
Make sure to replace `…` with the remaining numbers to form the complete list.
Q4. How can I generate random numbers between 1 and 40, excluding certain numbers?
To generate random numbers between 1 and 40, excluding certain numbers, you can use the `random.choice()` function with a modified sequence. Here’s an example code that excludes numbers 5 and 10 from the random selection:
“`python
numbers = [1, 2, 3, 4, 6, 7, 8, 9, 11, …, 40] # Replace … with the remaining numbers
random_number = random.choice(numbers)
“`
Replace `…` with the remaining numbers, excluding 5 and 10.
Q5. How can I generate random numbers using a random number generator algorithm?
The built-in `random` module already uses a random number generator algorithm. You can simply import the module and use the available functions to generate random numbers.
Q6. How can I generate random numbers between 1 and 42 with equal probabilities?
By default, the `random.randint()` function generates random numbers with equal probabilities. Hence, you can use the code shown earlier (`random_number = random.randint(1, 42)`) to generate random numbers between 1 and 42 with equal probabilities.
In conclusion, Python provides several techniques for generating random numbers. Whether you need integers or floating-point numbers, within a specific range or from a custom sequence, Python’s random module has got you covered. With the knowledge gained from this article, you can now easily generate random numbers ranging from 1 to 41, using various methods discussed. So go ahead, explore the possibilities, and enhance your programs with the power of randomness.
Keywords: random number 1 to 42, Random 1-40, random number 1-40, Random wheel 1 41, number 1 to 40, random number generator, Random 1 42, 1 to 41 numbers, random number 1 to 41
The Random Number Generator Key On A Casio Scientific Calculator … Generate Random Numbers 1 To 10
Why Is 17 The Least Random Number?
When discussing numbers and their randomness, the topic of 17 often comes up. For some reason, many people consider 17 to be the least random number. But why is this so? In this article, we will explore the various reasons behind this intriguing phenomenon and provide a thorough understanding of why 17 holds this unique distinction.
Historical Significance:
Firstly, let’s delve into the historical significance of the number 17. Throughout history, numerous cultures have attributed special meaning to this number. In ancient Egypt, 17 was believed to bring good fortune and prosperity. Similarly, in ancient Greece, it was considered an auspicious number associated with success. This historical reverence for 17 has seeped into popular culture and consciousness, thus perpetuating the idea of its non-randomness.
Numerological Properties:
Another reason why 17 is often seen as the least random number is its unique numerological properties. One of the hallmark features of 17 is its indivisibility. Unlike many other numbers, 17 cannot be divided evenly by any number except for 1 and itself. This mathematical property makes 17 stand out from others, leading some to believe that it lacks the randomness found in more divisible numbers.
Additionally, 17 holds significance in number theory due to its prime nature. A prime number is one that can only be divided by 1 and itself without leaving a remainder. Since 17 is a prime number, it is mathematically distinct and appears less random compared to composite numbers, which are divisible by other factors. This innate uniqueness contributes to the perception of 17 as a less random number.
Conspiracy Theories and Cultural Influences:
It is worth mentioning that cultural influences and conspiracy theories have also played a role in labeling 17 as a particularly non-random number. Some conspiracy theorists claim that the number 17 is connected to secret societies, occult practices, and other mysterious phenomena. These speculative beliefs, though lacking any substantial evidence, have sparked public interest and curiosity, thus perpetuating the notion that 17 is far from random.
Confirmation Bias and Subjectivity:
Additionally, the perception of 17 being the least random number may also stem from confirmation bias and human subjectivity. Confirmation bias refers to the tendency to favor information that confirms pre-existing beliefs. Once the idea of 17 being non-random gained traction, individuals sought out instances where 17 appeared more frequently or held some significance, reinforcing the notion.
Subjectivity also plays a role in the perception of randomness. When considering randomness, one must define precisely what is meant by “random.” Depending on the context and interpretation, different numbers could be perceived as more or less random. Our biases and preconceptions influence our perception of randomness, and thus, 17 may be seen as the least random number due to these subjective factors.
FAQs:
Q: Is there any scientific evidence to support the claim that 17 is the least random number?
A: No, there is no scientific evidence to confirm that 17 is the least random number. The perception of 17 as the least random number is primarily subjective and influenced by personal beliefs, cultural factors, and historical significance.
Q: Are there any practical implications to the idea of 17 being the least random number?
A: No, the belief that 17 is the least random number does not have any direct practical implications or impact on everyday life. It is merely an interesting concept that has captured public fascination.
Q: Can a number be completely random?
A: The concept of a completely random number is complex. In mathematics and computer science, numbers generated by random processes are considered random to some extent. However, it is nearly impossible to generate a truly random number, as all random processes are influenced by some underlying factors or algorithms.
Q: Are there any other numbers that are considered less random than 17?
A: The concept of a “least random” number is subjective and varies from person to person. While some may consider 17 the least random number, others may argue in favor of different numbers based on cultural or personal beliefs.
In conclusion, the perception of 17 as the least random number stems from a mixture of historical significance, numerological properties, cultural influences, and human subjectivity. While it lacks scientific justification, the idea of 17 being less random than other numbers has become deeply ingrained in popular culture and serves as a fascinating concept for many.
Is 37 The Most Random Number?
When it comes to numbers, some seem to have a special allure. Among those frequently observed are numbers like pi (3.14159…) and e (2.71828…), celebrated for their mathematical significance. However, there is a number that often stands out from the crowd as being particularly unique: 37. This number has managed to captivate the minds of mathematicians, scientists, and even conspiracy theorists with its alleged randomness. In this article, we will delve into the fascinating world of numbers and explore whether 37 truly deserves the title of the most random number.
To understand why 37 has gained such notoriety, we should begin by examining its properties. One intriguing aspect of 37 is that it is a prime number. Prime numbers have continuously fascinated mathematicians throughout history due to their peculiar nature. Being prime means that 37 is only divisible by 1 and itself, and it has no other divisors. Such uniqueness contributes to the perception of 37 as an enigmatic and inexplicable number.
Furthermore, when we consider the occurrences of 37 in various contexts, it becomes even more intriguing. In scientific fields, such as physics and astronomy, 37 often emerges as a significant figure. Take, for instance, the number of times the Moon rotates around its axis while completing one full orbit around the Earth, known as lunar sidereal rotation. Astonishingly, this figure is not a whole number; rather, it is 37. The implications of such coincidences spark curiosity and stir the imagination.
The number 37 also appears in various cultural and religious references, further contributing to its air of mystique. In the realm of literature, many authors have chosen 37 to convey a sense of unpredictability or randomness. Douglas Adams, author of “The Hitchhiker’s Guide to the Galaxy,” famously claimed that 37 is the funniest number. Such references have helped develop a cult following around this seemingly average number.
Moreover, some conspiracy theories link 37 to signs of the divine or hidden meanings. The belief that 37 holds a sacred significance stems from its appearance in religious texts and historical events. For instance, the Islamic faith holds that there are 37 prophets mentioned in the Quran. History also intertwines with the number 37 through the notorious 37th parallel, which has been associated with strange occurrences, such as the mysterious disappearance of numerous ships and airplanes. While these connections may appear far-fetched, they contribute to the aura of randomness surrounding 37.
However, when we examine the concept of randomness itself, it becomes apparent that designating a single number as the epitome of randomness is subjective. Randomness, in its truest sense, refers to a lack of predictability or pattern. Thus, to claim that 37 is the most random number would require supporting evidence that it surpasses all other numbers in its resistance to pattern detection or predictability.
Addressing this claim, statisticians argue that no particular number can lay claim to the title of the most random. Instead, randomness occurs across the entire numerical spectrum. Statistically, each number has an equal chance of appearing in any given sequence of numbers. By this logic, the concept of a “most random number” becomes paradoxical, as it contradicts the very definition of randomness itself.
Frequently Asked Questions:
Q: Why is 37 considered a prime number?
A: A prime number is a number that is only divisible by 1 and itself. Since 37 has no other divisors, it fits this definition perfectly.
Q: Are there any mathematical properties unique to the number 37?
A: While 37 is a prime number, it does not possess any mathematical properties that differentiate it significantly from other prime numbers. However, its uniqueness lies in the widespread cultural and scientific references that involve the number.
Q: Are there other numbers that have gained similar attention?
A: Yes, several other numbers have captivated the imagination of researchers and the general public. Examples include pi (3.14159…) and e (2.71828…), which are celebrated for their importance in mathematics.
Q: Is there scientific evidence supporting the notion that 37 is the most random number?
A: No, there is no scientific evidence supporting this claim. The concept of randomness across numbers is statistically equal, and hence, no single number can be deemed more random than others.
Q: Could the fascination with 37 be purely coincidental?
A: It is possible that the fascination with 37 is a coincidence, fuelled by cultural references and individual interpretations. Many arbitrary connections could be made with any number if one looks hard enough.
In conclusion, while 37 has undoubtedly captured the attention of many due to its unique prime nature and numerous cultural references, the belief that it is the most random number lacks objective scientific evidence. Randomness is inherently distributed across all numbers, and attributing greater randomness to one specific number contradicts the very concept of randomness itself. Nevertheless, the fascination with 37 will likely continue, as humans have an innate inclination to seek patterns and meanings even where none may exist.
Keywords searched by users: random number 1 to 41 random number 1 to 42, Random 1-40, random number 1-40, Random wheel 1 41, number 1 to 40, random number generator, Random 1 42, 1 to 41 numbers
Categories: Top 40 Random Number 1 To 41
See more here: nhanvietluanvan.com
Random Number 1 To 42
Numbers are an intrinsic part of our lives, surrounding us at every turn. We use numbers to quantify, measure, calculate, and organize our world. But have you ever pondered over the significance of a random number? Today, we delve into the curious realm of random numbers, specifically exploring the range from 1 to 42.
Random numbers are those that lack any discernible pattern, making them unpredictable and a true delight for mathematicians, statisticians, and researchers alike. From generating lottery numbers to computer simulations, random numbers play an essential role in a wide array of fields. Even everyday decisions like choosing a lucky number or rolling a dice depend on randomness. So, let’s explore the intriguing world of random numbers from 1 to 42 and discover more about their applications and significance.
Applications of Random Numbers
Random numbers have myriad applications across multiple domains. Let’s explore some of the most intriguing uses:
1. Cryptography: The world of computer security relies heavily on random numbers. From generating encryption keys for secure communication to ensuring secure internet transactions, random numbers are crucial in safeguarding sensitive information.
2. Simulations: Random numbers are widely used in computer simulations to replicate real-world scenarios. Fields such as physics, biology, and economics incorporate randomness to model complex systems and predict their behavior.
3. Gaming: Whether it’s rolling a dice or determining card shuffling patterns, random numbers are an integral part of gaming. They ensure fairness and unpredictability, making games more exciting and enjoyable.
4. Lottery and gambling: Randomness is the heart of lotteries and other gambling activities. The winning numbers in lotteries are determined using random number generators, ensuring fairness and transparency.
5. Statistical sampling: Random numbers are used for statistical sampling to obtain representative samples from large populations. This technique helps researchers draw reliable conclusions based on limited data.
The Significance of the Range from 1 to 42
Among the vast array of random numbers available, the range from 1 to 42 holds particular importance. This range is significant due to its presence in various contexts, both mundane and extraordinary.
In popular culture, the number 42 gained prominence through Douglas Adams’ iconic science fiction novel, “The Hitchhiker’s Guide to the Galaxy.” According to the book, the “Answer to the Ultimate Question of Life, the Universe, and Everything” is simply the number 42. This has since become a tongue-in-cheek response to life’s deepest questions, introducing 42 in popular consciousness.
Furthermore, 42 is a highly composite number, meaning it has a large number of divisors. Specifically, this range from 1 to 42 has ten divisors: 1, 2, 3, 6, 7, 14, 21, 42, making it mathematically intriguing.
FAQs about Random Numbers from 1 to 42
1. Are the numbers from 1 to 42 truly random?
Yes, random numbers generated within this range are as random as any other truly random numbers. They lack any discernible pattern and fulfill the criteria for randomness.
2. How can I generate random numbers between 1 and 42?
Several methods can be employed to generate random numbers in this range. One common technique is to use a random number generator, either from programming libraries or online tools specialized for random number generation.
3. Is the number 42 considered lucky or significant in different cultures?
While 42 holds significance in popular culture due to “The Hitchhiker’s Guide to the Galaxy,” it does not have universal cultural significance as a lucky or sacred number.
4. Can random numbers be predicted or influenced?
True random numbers cannot be predicted or influenced. They are inherently unpredictable and independent of any external factors.
5. Can random numbers have repeating patterns?
Random numbers, by definition, lack repeating patterns. However, due to the limitations of the algorithms or techniques used to generate them, pseudo-random numbers might exhibit patterns or repetitions over long sequences.
6. Are random numbers used in scientific research?
Absolutely! Random numbers play an integral role in scientific research. They are utilized in experiments, simulations, statistical analyses, and various other research endeavors.
In conclusion, random numbers from 1 to 42 hold a fascinating place in our world. They find their applications in cryptography, simulations, gaming, and statistical sampling. Moreover, the number 42 itself has gained cultural significance, both through popular fiction and its mathematical properties. So, the next time you come across these seemingly arbitrary numbers, take a moment to appreciate the hidden beauty of randomness lurking within them.
Random 1-40
Introduction
The concept of randomness has always intrigued the human mind. People have used various methods to generate random numbers for centuries, ranging from tossing dice to spinning roulette wheels. In the digital age, however, randomness has taken on new dimensions. The term “Random 1-40” refers to the generation of random numbers within the range of one to forty. This article aims to explore the various methods and applications of Random 1-40, shedding light on its significance in diverse fields.
Methods of Generating Random Numbers
1. Manual Generation
The simplest method of generating Random 1-40 numbers is through manual selection. In this approach, individuals physically choose a number between one and forty using methods like drawing from a hat or using playing cards. While simple, this method is prone to human bias and cannot ensure true randomness.
2. Mechanical Randomizers
Mechanical randomizers are devices designed to generate random numbers. These devices often employ intricate mechanisms, such as rotating drums, spinning wheels, or tumbling spheres containing numbered balls. The mechanical randomizer shuffles and picks a ball or spins to randomly select a number between one and forty. These mechanisms eliminate human bias, offering a higher degree of randomness.
3. Computational Algorithms
In the digital era, computational algorithms have taken center stage in generating random numbers. Pseudo-random number generator (PRNG) algorithms use mathematical formulas and seed values to generate sequences of numbers that appear random. To generate Random 1-40 numbers, these algorithms limit the range between one and forty. However, it must be noted that PRNGs produce deterministic numbers and are not truly random. True random number generators (TRNGs) exploit non-deterministic physical processes, like atmospheric noise or radioactive decay, to generate numbers that are closer to true randomness.
Applications of Random 1-40
1. Games and Lotteries
Random 1-40 number generation finds extensive use in various games and lotteries. In board games, it is utilized to determine moves or outcomes, ensuring fairness and unpredictability. When it comes to lotteries, the selection of winning numbers is predominantly based on random number generation. Thus, Random 1-40 plays a crucial role in ensuring equal opportunities for participants.
2. Raffles and Contests
Organizers of raffles and contests employ Random 1-40 to impartially determine winners. Generating random numbers within the range of one to forty ensures that each participant has an equal chance of winning, enhancing the credibility and transparency of the event. Whether it is a small local raffle or a large-scale national competition, Random 1-40 number generation eliminates biases and instills fairness.
3. Statistical Sampling
In statistical research, random number generation is often used for sampling purposes. By generating Random 1-40 numbers, researchers can select a random subset of a given population, which represents the entire population more accurately. This technique is widely used in social sciences, market research, and opinion polls, facilitating the generalization of results from a smaller sample to a larger population.
4. Educational Purposes
Random 1-40 generation is also employed in educational settings. Teachers can use random numbers to assign seating arrangements, present random examples for problem-solving exercises, or randomly choose students for classroom activities. This method avoids any favoritism or predictability, ensuring an unbiased approach within the classroom.
FAQs
Q1. How can I ensure the randomness of manually generated random numbers?
To ensure the randomness of manually generated random numbers, it is essential to use an unbiased selection method. Avoid any personal biases when selecting the numbers, ensuring that each number has an equal chance of being chosen. You can incorporate external factors, like flipping a coin or rolling a die, to enhance the randomness.
Q2. Can pseudo-random number generators (PRNGs) be used for applications requiring true randomness?
While PRNGs produce numbers that appear random, they are not considered truly random. For applications requiring strong randomness, such as cryptography, it is advisable to use true random number generators (TRNGs). TRNGs leverage physical processes to generate more genuinely random numbers.
Q3. Are there any reliable online platforms for generating Random 1-40 numbers?
Yes, several online platforms offer reliable tools for generating random numbers within a specified range, including one to forty. Some popular platforms include random.org, randomnumbergenerator.com, and randomlists.com. Ensure you use reputable sources and verify the legitimacy of these platforms before relying on them.
Conclusion
Random 1-40 number generation plays a significant role in various domains, ranging from games and lotteries to statistical sampling and education. By adopting different methods, such as manual generation, mechanical randomizers, or computational algorithms, individuals and organizations can ensure fairness, eliminate biases, and generate numbers that enhance the unpredictability and validity of their respective applications.
Random Number 1-40
In the world of mathematics and statistics, random numbers play a crucial role in a variety of applications. From generating secure encryption keys to simulating complex systems, random numbers have become indispensable to many fields. In this article, we will explore the use and significance of random numbers between 1 and 40, discussing their applications, methods of generation, and potential limitations.
Applications of Random Numbers 1-40:
1. Lottery and Gambling: Random numbers are commonly used in lotteries and gambling games to ensure fairness and unpredictability. By generating a random number between 1 and 40, individuals have an equal chance of winning, providing an unbiased mechanism for determining results.
2. Sampling and Surveys: Random numbers help in selecting unbiased samples for surveys and research studies. For instance, if a population is defined by sequential numbers between 1 and 40, using random numbers to select individuals ensures each member has an equal probability of being included in the sample, reducing bias and increasing the generalizability of the findings.
3. Randomized Control Trials: In scientific experiments, random numbers play a pivotal role in determining the allocation of participants into different treatment groups. This randomization process helps to eliminate potential biases and ensures that each group has a fair chance of receiving a specific treatment.
4. Game Design: Random numbers are widely used in computer games to create unpredictable outcomes. Developers can use these numbers to determine variables such as enemy behavior, loot drops, or level design, providing players with a more engaging and dynamic gaming experience.
Methods of Generating Random Numbers 1-40:
1. Mathematical Algorithms: Pseudorandom number generators (PRNGs) are algorithms that use mathematical formulas to generate a sequence of numbers that appears random. These algorithms can be used to generate random numbers between 1 and 40 by taking the modulus of a larger range and then adding 1 to the result.
2. Physical Randomness: Some applications require true randomness, rather than pseudorandomness. Physical sources of randomness, such as atmospheric noise or radioactive decay, can be used to generate truly random numbers. However, this process can be more challenging than using algorithms, as it often requires specialized hardware.
3. Programming Libraries and APIs: Many programming languages offer libraries or APIs that allow developers to easily generate random numbers. These libraries often include functions for generating random numbers within a given range, making it straightforward to obtain random numbers between 1 and 40.
Limitations and Considerations:
1. Bias: When generating random numbers using algorithms, it is important to ensure that the algorithms used are unbiased. Some algorithms might introduce patterns or biases, leading to non-random results. Therefore, before using any algorithm for critical applications, thorough testing and verification should be performed.
2. Seed Values: PRNGs require a seed value to initiate the random number generation process. Using the same seed will produce the same sequence of random numbers. Therefore, it is important to select a unique and sufficiently random seed, especially in scenarios where security is paramount.
3. True Randomness: While mathematical algorithms can provide pseudorandomness, they may not be suitable for applications requiring true randomness. In such cases, specialized hardware or physical sources of randomness should be considered.
4. Randomness Quality: The randomness quality of the generated numbers is essential when used in cryptographic systems. Using compromised or low-quality random numbers can lead to vulnerabilities in encryption and security protocols. In critical systems, it is crucial to use certified random number generators or sources.
FAQs:
Q: Why are random numbers important?
A: Random numbers are important because they provide a way to introduce unpredictability and fairness into various processes, such as gambling, scientific experimentation, and cryptography.
Q: Can random numbers between 1 and 40 be used for choosing lottery numbers?
A: Yes, random numbers between 1 and 40 can be used for choosing lottery numbers, as they provide an unbiased and impartial selection method.
Q: Are there any limitations to using random numbers generated by algorithms?
A: Yes, algorithms might introduce patterns or biases, leading to non-random results. To mitigate this, careful consideration should be given to the selection of algorithm, and thorough testing and verification should be performed.
Q: Is it possible to generate truly random numbers between 1 and 40?
A: Yes, it is possible to generate truly random numbers, but it often requires specialized hardware or physical sources of randomness. Algorithms can provide pseudorandomness but might not be suitable for all applications requiring true randomness.
Q: Are random numbers used outside of mathematics and statistics?
A: Yes, random numbers are widely used in various fields such as computer science, gaming, cryptography, and simulations, to name a few. They contribute to diverse applications involving uncertainty and unpredictability.
In conclusion, random numbers between 1 and 40 find utility in a wide range of applications. Whether it is for selecting lottery numbers or conducting scientific experiments, the use of random numbers ensures fairness, unpredictability, and reduced bias. From mathematical algorithms to physical sources of randomness, generating random numbers requires careful consideration, considering the limitations and requirements of the specific application.
Images related to the topic random number 1 to 41
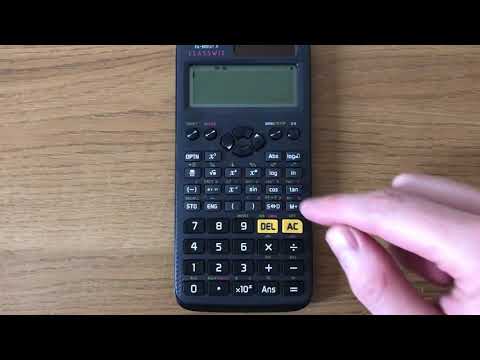
Found 19 images related to random number 1 to 41 theme
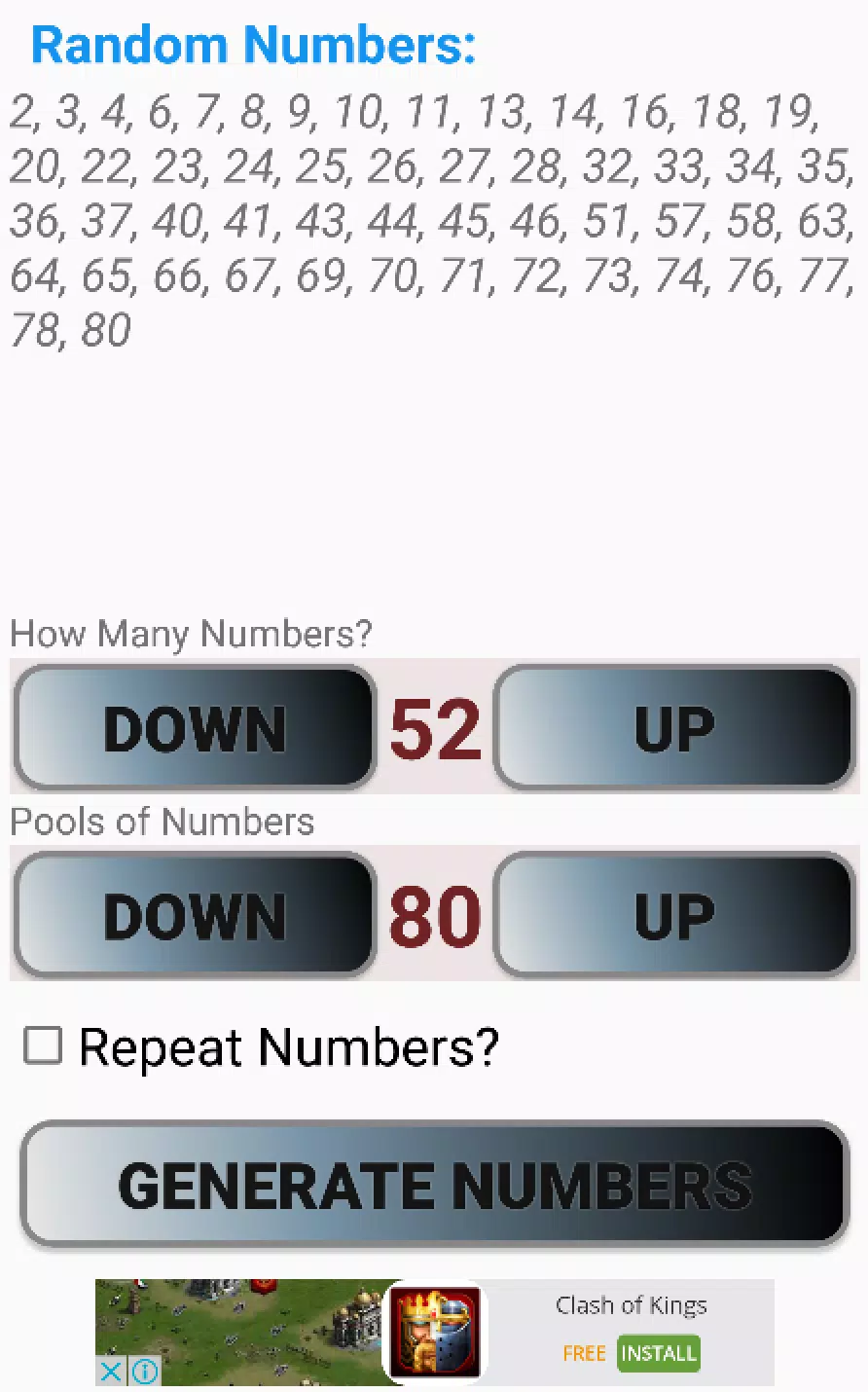
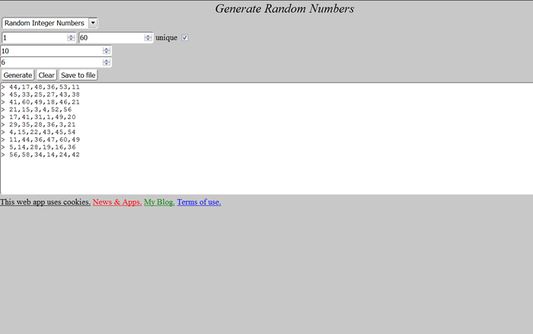
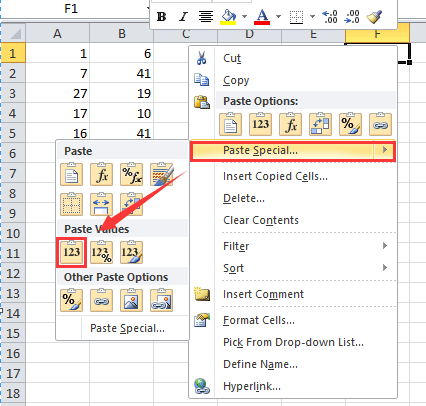
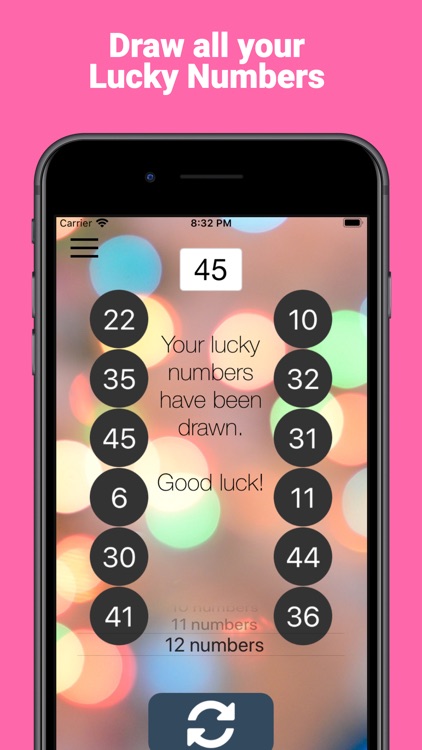

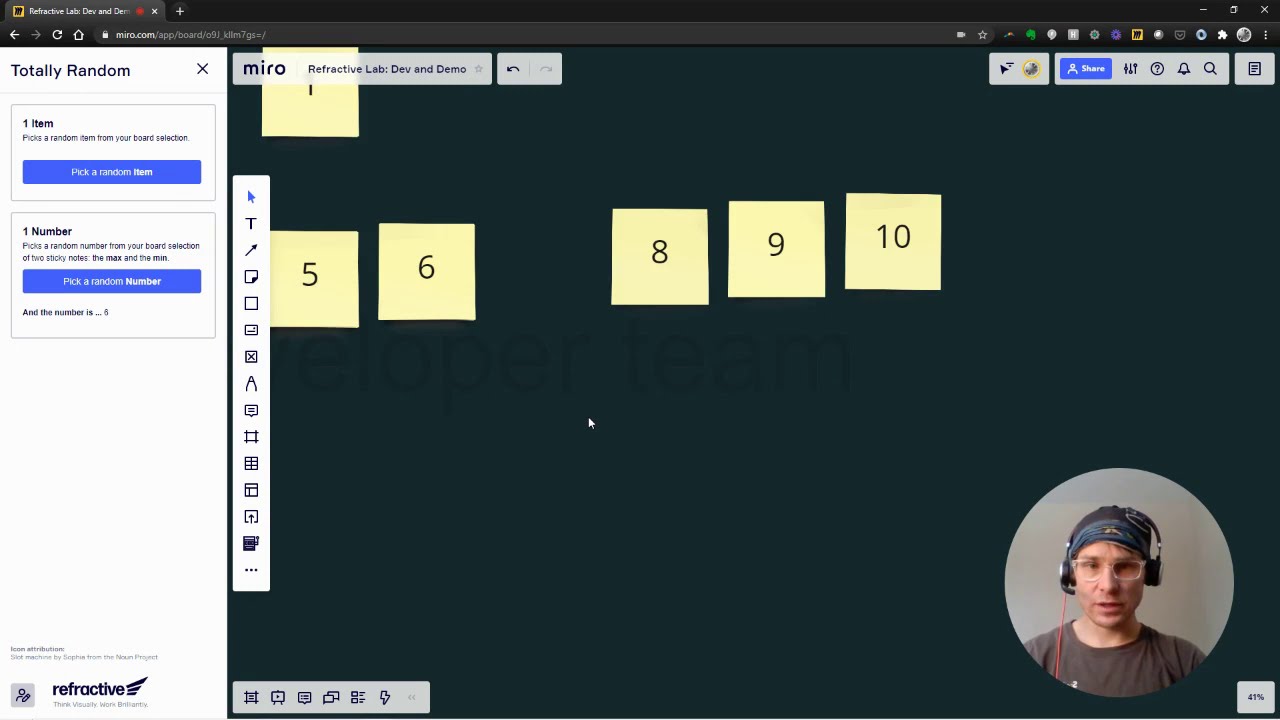

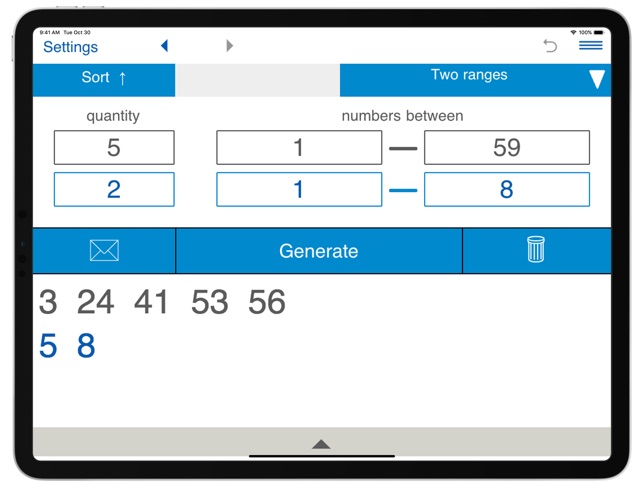

Article link: random number 1 to 41.
Learn more about the topic random number 1 to 41.
- 17 (number) – Wikipedia
- random numbers – Catb.org
- Why is 37 the most common choice when people are asked to choose a …
- Random function in C – Coding Ninjas
- Number Wheel 1-41 – Random wheel – Wordwall
- Random Number Generator: 1-41 – calcpark.com
- Random Number Generator 1-41 – Online Calculator
- 1 Random Numbers between 41 – 50
- Quay số ngẫu nhiên online • Random Number Generator 2023
- Methods of Random Number Generation – JSTOR
- Random Number Between 1 and 41 – Research Maniacs
See more: blog https://nhanvietluanvan.com/luat-hoc