R List Of Data Frames
Overview of Data Frames
In the field of data analysis and programming, data frames are widely used data structures that organize and store tabular data in R and Python. A data frame is a list of data frames, where each data frame represents a dataset with rows and columns. This allows for efficient analysis and manipulation of multiple datasets simultaneously, making data frames a valuable tool for data scientists and analysts.
Defining the Concept of Data Frames
A data frame is a two-dimensional data structure that is similar to a table or spreadsheet. It organizes data in rows and columns, where each row represents an observation or case, and each column represents a variable or attribute. Data frames can store different types of data, such as numeric, character, and factor variables.
Characteristics and Structure of Data Frames
Data frames have several key characteristics that distinguish them from other data structures. Firstly, data frames are rectangular, meaning that all columns have the same length. Secondly, variables in a data frame can have different data types. Lastly, data frames allow for both row and column names, enabling easy and intuitive access to specific data points within the structure.
Importing Data into Data Frames
One of the most common tasks when working with data frames is importing data from external sources. Both R and Python provide numerous functions and packages to import data from various file formats, such as CSV, Excel, and SQL databases. By utilizing these functions, data can be seamlessly imported into data frames and be ready for analysis.
Exploring the Content of Data Frames
Once the data is imported into data frames, it’s vital to explore and understand its content. Several functions in R and Python allow for basic exploratory analysis of data frames. These functions include summarizing numeric variables, counting categories in factor variables, and plotting distributions or relationships between variables.
Modifying and Manipulating Data Frames
Data frames provide numerous capabilities for modifying and manipulating data. Users can add, remove, or reorder columns, change values within specific cells, or create new variables based on existing ones. These modifications can be achieved using built-in functions or by applying specific operations and conditions to subsets of the data frame.
Filtering and Sorting Data Frames
Filtering and sorting data frames are essential tasks when working with large datasets. Filtering allows users to subset the data based on specific criteria, such as selecting rows with certain values or within a particular range. Sorting orders the rows of a data frame based on one or more variables, enabling easier interpretation and analysis.
Performing Statistical Analysis on Data Frames
With data frames, performing statistical analysis becomes straightforward. R and Python provide a wide range of packages and functions for statistical analysis, including descriptive statistics, hypothesis testing, regression, and more advanced techniques. By simply applying these functions to the relevant columns or subsets of the data frame, users can obtain valuable insights and draw meaningful conclusions.
Exporting Data Frames
After analyzing and visualizing data frames, it’s common to wish to share or save the results. R and Python offer various methods for exporting data frames, such as saving to CSV, Excel, or SQL databases. These functions allow users to preserve the structure and content of the data frame, enabling seamless integration into other applications or platforms.
Now, let’s address some FAQs related to working with a list of data frames.
FAQs:
Q: How can I create a list of data frames in R using a loop?
A: To create a list of data frames using a loop in R, you can first create an empty list, then iteratively create and append data frames to the list within a loop. Below is an example:
“`R
data_list <- list() # Creating an empty list
for (i in 1:5) {
df <- data.frame(x = rnorm(10), y = rnorm(10)) # Creating a data frame
data_list[[i]] <- df # Appending the data frame to the list
}
```
Q: How can I merge a list of data frames in R?
A: To merge a list of data frames in R, you can use the `Reduce()` function along with the `merge()` function. The `Reduce()` function applies a binary function to pairs of elements in the list, progressively combining them. Here's an example:
```R
merged_df <- Reduce(function(df1, df2) merge(df1, df2, by = "common_column"), data_list)
```
Q: How can I add a data frame to a list in R?
A: To add a data frame to an existing list in R, you can use the concatenation operator `c()` or the `append()` function. Here are two examples:
```R
# Using the concatenation operator
new_df <- data.frame(a = 1:5, b = letters[1:5])
updated_list <- c(data_list, new_df)
# Using the append() function
updated_list <- append(data_list, list(new_df))
```
Q: How can I access data frames within a list in R?
A: To access specific data frames within a list in R, you can use double square brackets `[[ ]]` or the `$` operator. Here are two examples:
```R
# Using double square brackets
df1 <- data_list[[1]] # Accessing the first data frame
# Using the $ operator
df2 <- data_list$df_name # Accessing a data frame by its name
```
Q: What is the difference between a list and a data frame in R?
A: A list is a general-purpose data structure in R that can hold various types of objects, including other lists and data frames. On the other hand, a data frame is a specific type of list that organizes and stores tabular data. Data frames are rectangular in shape and require all columns to have the same length, whereas lists can have different lengths for their elements.
Q: How can I combine multiple data frames vertically in R?
A: To combine multiple data frames vertically, you can use the `rbind()` function in R. This function stacks data frames on top of each other, assuming they have the same column structure. Here's an example:
```R
combined_df <- do.call(rbind, data_list)
```
In conclusion, understanding and mastering data frames is crucial for efficient data analysis in both R and Python. By effectively importing, exploring, modifying, and manipulating data frames, analysts can unleash the full potential of their datasets and derive valuable insights. Whether it's creating a list of data frames in a loop or performing statistical analysis, data frames offer a versatile and powerful toolset for handling complex data structures.
Data Frames And Lists In R
Can I Make A List Of Data Frames In R?
In R, a data frame is a popular data structure widely used for storing and manipulating data. It is a tabular structure that allows you to organize data into columns and rows, similar to a spreadsheet or a database table. One common question that arises when working with data frames in R is whether it is possible to create a list of data frames. In this article, we will explore the concept of a list of data frames in R and discuss its importance and potential applications.
Why do I need a list of data frames?
While a single data frame can be useful for organizing and analyzing a specific set of data, there are situations where having multiple data frames can be beneficial. For example, you may have different datasets from various sources that you want to store separately. Instead of cluttering your workspace with multiple individual data frames, a list of data frames allows you to group them together in a structured and organized manner. This can improve efficiency, simplify data management, and enhance code reusability.
Creating a list of data frames
Creating a list of data frames in R is straightforward. You can use the `list()` function to create an empty list and then populate it with data frames. Here’s an example:
“`R
# Create an empty list
my_list <- list()
# Create and add data frames to the list
df1 <- data.frame(x = 1:5, y = 6:10)
df2 <- data.frame(a = c("A", "B", "C"), b = c(11, 12, 13))
my_list <- list(df1, df2)
```
In this example, we first create an empty list named `my_list` using the `list()` function. We then create two data frames (`df1` and `df2`) and add them to the list using the assignment operator (`<-`). Now, `my_list` contains two data frames, `df1` and `df2`.
Accessing and manipulating data frames in a list
Once you have created a list of data frames, you can access and manipulate individual data frames using indexing. To access a specific data frame, you can use double square brackets (`[[]]`) or the dollar sign (`$`) operator. Here's how you can access the first data frame (`df1`) from the example above:
```R
# Accessing the first data frame in the list
df1 <- my_list[[1]]
```
Similarly, you can access specific columns or perform operations on individual data frames within the list. For example, if you want to calculate the mean of the 'x' column in `df1`, you can do the following:
```R
# Calculate the mean of the 'x' column in df1
mean_x <- mean(my_list[[1]]$x)
```
By using indexing, you can easily perform operations on data frames within a list separately or collectively, depending on your requirements.
Frequently Asked Questions (FAQs)
Q1. Are there any limitations on the number of data frames I can include in a list?
A1. No, there are no inherent limitations on the number of data frames you can include in a list. You can add as many data frames as you need, based on your data processing requirements.
Q2. Can I add different types of objects to a list of data frames?
A2. Yes, you can add different types of objects to a list, including vectors, matrices, and even other lists. However, it is generally recommended to keep objects within a list of the same data structure for clarity and consistency.
Q3. How can I iterate over a list of data frames?
A3. You can use a loop or apply functions like `lapply()` or `sapply()` to iterate over the data frames within a list, and perform operations on each data frame individually.
Q4. Can I modify a data frame within a list without affecting the original data frame?
A4. Yes, you can modify a data frame within a list without affecting the original data frame. R creates a copy of the data frame within the list, allowing you to make changes specific to that list.
Conclusion
In conclusion, creating a list of data frames in R provides a convenient way to organize and manipulate multiple datasets. With the ability to access and modify individual data frames within the list, you can easily perform operations collectively or separately, enhancing code reusability and data management. So, the answer to the question "Can I make a list of data frames in R?" is a resounding yes, and it offers a powerful tool for data analysis and manipulation in R.
Can A Data Frame Be A List?
When working with data in R, two common data structures that you may encounter are data frames and lists. Both structures are used to organize and store data, but they have distinct characteristics and uses. While data frames are typically used for storing structured tabular data, lists provide a more flexible structure that can contain different types of objects, including other lists and data frames. But can a data frame be a list? Let’s explore this question in depth.
Understanding Data Frames
A data frame is a two-dimensional structure in R that stores data in a tabular format, similar to a spreadsheet or a SQL table. It consists of rows and columns, where each column represents a variable or attribute, and each row represents an observation or case. This structure is particularly useful for handling structured data, where different variables are associated with specific observations.
Data frames are extensively used in data analysis, statistics, and machine learning tasks. They are the default structure for reading and manipulating data in R because they offer a convenient way to organize, manipulate, and analyze data effectively. Moreover, data frames have built-in functions to handle missing values, merge data sets, and perform various computations.
Understanding Lists
In contrast to data frames, lists are diverse and flexible structures that can contain any combination of objects in R. These objects can be other lists, vectors, matrices, data frames, or even functions. Unlike data frames, lists are not restricted to a two-dimensional tabular structure and can be of any length or complexity.
Lists are powerful data structures that allow you to store and organize arbitrary collections of data. They offer a high level of flexibility because they can store objects of different types and sizes within a single list. This makes lists an ideal choice when dealing with complex and heterogeneous data that cannot be easily represented in a tabular format.
Can a Data Frame Be a List?
In R, a data frame cannot be a list, but a list can contain a data frame. This is because a data frame is a specific type of object with its unique structure and attributes, while a list is a more general and flexible structure that can contain any type of object, including data frames.
To illustrate further, let’s consider an example:
“`
# Creating a data frame
df <- data.frame(ID = 1:5, Name = c("John", "Emily", "David", "Emma", "Michael"), Age = c(32, 25, 41, 28, 36))
# Creating a list that contains the data frame
my_list <- list(my_df = df)
# Accessing the data frame within the list
my_list$my_df
```
In the example above, we first create a data frame called `df`, containing information about individuals. Then, we create a list called `my_list` and assign the `df` data frame to it. Finally, we access the data frame within the list using the `$` operator and the name `my_df`. This demonstrates that while a data frame cannot be a list, it can be an element within a list.
Why Use Lists Containing Data Frames?
Lists that contain data frames can be advantageous in certain situations. Here are a few reasons why you might want to use lists that contain data frames:
1. Organization: Lists can help you organize and manage multiple data frames. For instance, if you have different datasets related to a specific project, you can store them in a list, making it easier to access, manipulate, and analyze them.
2. Flexibility: Data frames within a list can have different structures and sizes. This flexibility allows you to handle diverse data sets within a single list efficiently.
3. Handling Nested Data: Lists can store not only data frames but also other lists. This enables you to handle nested or hierarchical data structures, where each element can contain further sub-elements, including data frames.
4. Grouping and Conditioning: When conducting analyses, you may need to perform operations on specific subsets of data frames. Using lists, you can group related data frames together, making conditional operations more straightforward and efficient.
FAQs:
1. Can a data frame contain a list?
No, a data frame cannot contain a list within a single cell. However, individual cells in a data frame can hold complex objects, including lists, within their own structure.
2. Can a list contain both data frames and lists?
Yes, a list can contain both data frames and other lists within its structure, allowing you to handle complex and heterogeneous data.
3. Are lists more efficient than data frames?
Lists and data frames have different purposes and characteristics. While lists provide more flexibility and versatility, data frames are specifically designed for structured data and offer built-in functions to handle common data operations efficiently.
In conclusion, a data frame cannot be a list, but a list can contain a data frame. Data frames and lists are both valuable data structures in R, each with its own characteristics and use cases. Understanding the differences between these structures allows you to choose the most appropriate data structure for your specific needs, whether it involves working with tabular data or organizing diverse and complex collections of objects.
Keywords searched by users: r list of data frames r create list of data frames in loop, merge list of data frames in r, list of dataframes python, add dataframe to list r, r list dataframes in environment, rbind list of dataframes r, difference between list and dataframe in r, list of dataframe python
Categories: Top 76 R List Of Data Frames
See more here: nhanvietluanvan.com
R Create List Of Data Frames In Loop
Loops are a crucial component of programming, allowing us to automate tedious tasks. In R, loops can be used to create a list of data frames, which can prove to be a handy technique for organizing and manipulating data efficiently. This article will guide you through the process of creating a list of data frames using loops in R.
Understanding Data Frames in R
Before we delve into creating a list of data frames using loops, let’s briefly understand what data frames are in R. A data frame is a two-dimensional tabular structure that can store data of different types (numeric, character, logical, etc.) in columns. Each column represents a variable, and each row represents an observation or case.
Data frames provide an efficient way to organize and work with structured data. They are widely used in data analysis, statistics, and machine learning projects. Now, let’s see how we can create a list of data frames using loops in R.
Creating a List of Data Frames
To begin, we need to have an idea of the number of data frames we want to create and the structure of each data frame. For the sake of demonstration, let’s assume we want to create three data frames, each consisting of three variables: “ID,” “Name,” and “Age.” We will use a loop to automate the creation process.
We start by initializing an empty list to store the data frames: `df_list <- list()`. Then, we define a loop that iterates over the desired number of data frames. Inside the loop, we create each data frame, populate it with data, and add it to the list. Here's a code snippet that accomplishes this task: ```R # Initialize an empty list df_list <- list() # Number of data frames to create num_data_frames <- 3 # Loop through the desired number of data frames for (i in 1:num_data_frames) { # Create a data frame df <- data.frame(ID = c(1, 2, 3), Name = c("John", "Jane", "Bob"), Age = c(25, 30, 35)) # Add the data frame to the list df_list[[i]] <- df } ``` In this example, we create a data frame called `df` by providing the values for the "ID," "Name," and "Age" variables. Then, we append the `df` to the `df_list` using double brackets `[[i]]` to access the `i`-th element of the list. Once the loop completes, you will have successfully created a list of data frames in R. FAQs about Creating a List of Data Frames in R Q: Can I create data frames with different structures? A: Yes, you can create data frames with different structures. Simply modify the code inside the loop to specify the desired structure for each data frame. Q: How can I access individual data frames within the list? A: You can access individual data frames within the list using double brackets `[[i]]`. For example, to access the first data frame, you can use `df_list[[1]]`. Q: Can I modify the data frames within the list? A: Yes, you can modify the data frames within the list by directly referencing them using double brackets. For instance, if you want to modify the "Age" variable in the second data frame, you can use `df_list[[2]]$Age <- c(28, 33, 26)`. Q: How can I add additional variables to each data frame? A: To add additional variables to each data frame, you can extend the code inside the loop. For example, to add a new variable called "Salary" to each data frame, you can use `df$Salary <- c(5000, 6000, 7000)`. Q: Can I combine data frames from the list into a single data frame? A: Yes, you can combine the data frames from the list into a single data frame using `do.call()` and `rbind()` functions. For instance, you can use `df_combined <- do.call(rbind, df_list)` to combine all data frames vertically. Conclusion Creating a list of data frames in R using loops can significantly enhance data manipulation and organization. By automating the creation process, we can efficiently handle large amounts of structured data. This article has provided a comprehensive guide on how to create a list of data frames using loops in R. Remember, practice makes perfect, so keep experimenting with different structures and explore the possibilities that data frame lists offer in your data analysis projects.
Merge List Of Data Frames In R
When working with data frames in R, it is common to encounter situations where you need to combine or merge multiple data frames together. R provides several ways to merge data frames, and one useful technique is to merge a list of data frames. This approach is particularly handy when you have a large number of data frames to merge, as it allows you to streamline your code and avoid repetitive merge operations.
In this article, we will explore how to merge a list of data frames in R. We will cover different merging methods, their syntax, and provide examples to illustrate their usage. Additionally, we will address some frequently asked questions (FAQs) about merging data frames in R.
Merging Data Frames in R
To begin, let’s consider a scenario where we have a list of data frames that we want to merge into a single data frame. Each data frame in the list shares a common key variable that we want to use for the merge operation. We can achieve this using the “merge()” function in R.
Syntax: merge(x, y, by, …)
The “merge()” function takes two main arguments, “x” and “y”, representing the data frames to be merged. The “by” argument specifies the common key variable(s) in both data frames used for merging. Additional arguments can be passed using “…”, allowing customization of the merge operation.
Let’s look at an example to understand this concept better:
“`R
# Create example data frames
df1 <- data.frame(ID = 1:3, Name = c("John", "Emma", "Michael"))
df2 <- data.frame(ID = 2:4, Occupation = c("Engineer", "Teacher", "Doctor"))
# Merge data frames
merged_df <- merge(df1, df2, by = "ID")
# Print merged data frame
print(merged_df)
```
Output:
```
ID Name Occupation
1 2 Emma Engineer
2 3 Michael Teacher
```
In this example, we have two data frames, "df1" and "df2". The common key variable is "ID", which contains values that exist in both data frames. The merge operation combines the two data frames based on this common key, resulting in a new data frame called "merged_df". The output displays the merged result, which includes the columns from both data frames.
Merging List of Data Frames
To merge a list of data frames, we can utilize the "Reduce()" function in R. The "Reduce()" function is a powerful tool that enables us to apply a binary function iteratively to a list of values.
Syntax: Reduce(f, x)
The "f" argument represents the function to apply to the data frames, while the "x" argument denotes the list of data frames.
```R
# Create a list of example data frames
df_list <- list(df1, df2)
# Merge list of data frames
merged_list_df <- Reduce(function(x, y) merge(x, y, by = "ID"), df_list)
# Print merged list data frame
print(merged_list_df)
```
Output:
```
ID Name Occupation
1 2 Emma Engineer
2 3 Michael Teacher
```
In this example, we first create a list of data frames, "df_list", containing "df1" and "df2". The "Reduce()" function applies the merge operation iteratively to each data frame in the list, based on the common key variable "ID". The final result, "merged_list_df", is a merged data frame that combines the information from all data frames in the list.
FAQs – Frequently Asked Questions
Q1. Can I merge data frames with different column names?
Yes, you can merge data frames with different column names. In such cases, you need to specify the corresponding columns in the "by" argument of the merge function. For example:
```R
# Create example data frames with different column names
df1 <- data.frame(ID = 1:3, FullName = c("John", "Emma", "Michael"))
df2 <- data.frame(CustomerID = 2:4, Occupation = c("Engineer", "Teacher", "Doctor"))
# Merge data frames with different column names
merged_df <- merge(df1, df2, by.x = "ID", by.y = "CustomerID")
# Print merged data frame
print(merged_df)
```
Q2. How can I merge data frames based on multiple key variables?
To merge data frames based on multiple key variables, you can pass a vector of column names to the "by" argument. For example:
```R
# Create example data frames with multiple key variables
df1 <- data.frame(ID = 1:3, Name = c("John", "Emma", "Michael"), Country = c("USA", "UK", "Canada"))
df2 <- data.frame(ID = 2:4, Occupation = c("Engineer", "Teacher", "Doctor"), Country = c("USA", "UK", "Canada"))
# Merge data frames using multiple key variables
merged_df <- merge(df1, df2, by = c("ID", "Country"))
# Print merged data frame
print(merged_df)
```
These are just a few examples of how to merge data frames in R. By understanding these concepts and variations, you will be better equipped to handle merging scenarios in your data analysis projects.
List Of Dataframes Python
## Understanding Dataframes
A dataframe is similar to a table in a spreadsheet or a SQL database. It consists of rows and columns, with each column representing a variable, and each row representing an observation. Dataframes can hold different data types, such as integers, floats, strings, or even complex objects.
## Creating Dataframes
Creating a dataframe in Python is straightforward. The pandas library provides a DataFrame class that allows us to create a dataframe using various data inputs, such as lists, dictionaries, or NumPy arrays. To demonstrate this, consider the following example:
“`python
import pandas as pd
data = {‘Name’: [‘John’, ‘Amy’, ‘Bill’, ‘Emma’],
‘Age’: [25, 31, 42, 29],
‘City’: [‘London’, ‘New York’, ‘Paris’, ‘Sydney’]}
df = pd.DataFrame(data)
“`
In this example, we define a dictionary called `data`, where each key represents a column name and each value represents the data for that column. We then pass this dictionary to the `DataFrame()` function from the pandas library, creating a dataframe named `df`. This dataframe will have three columns: ‘Name’, ‘Age’, and ‘City’.
## Accessing Data in Dataframes
Once we have created a dataframe, we may need to access specific data within it. Pandas provides several methods to accomplish this. We can access an entire column by using its name as an index, like `df[‘Name’]`. This will return a pandas Series object, which is similar to a one-dimensional array. To access specific rows or cells in the dataframe, we can utilize index-based selection or boolean indexing.
## Manipulating Data in Dataframes
Dataframes offer a variety of powerful methods for manipulating data. We can perform tasks such as filtering rows based on specific conditions, sorting data, adding or removing columns, and aggregating data. These operations make data exploration and analysis much more efficient.
## Merging Dataframes
Often, we need to combine multiple dataframes to perform more complex analysis. Pandas provides a merge function that allows us to join two or more dataframes based on a common column. This is particularly useful when working with relational data or datasets that share common keys.
## Common Operations on Dataframes
Let’s cover some frequently asked questions about dataframes in Python:
### Q: How can I filter rows based on a condition?
A: You can use boolean indexing to filter rows. For example, to filter the dataframe `df` to only include rows where the age is greater than 30, you can use the following code:
“`python
filtered_df = df[df[‘Age’] > 30]
“`
### Q: How can I sort a dataframe?
A: To sort a dataframe based on a specific column, you can use the `sort_values()` method. For instance, to sort the dataframe `df` by age in ascending order, you can use the following code:
“`python
sorted_df = df.sort_values(‘Age’)
“`
### Q: Can I rename columns in a dataframe?
A: Yes, you can rename columns using the `rename()` method. Here’s an example:
“`python
df.rename(columns={‘Name’: ‘Full Name’}, inplace=True)
“`
### Q: How can I export a dataframe to a CSV file?
A: To export a dataframe to a CSV file, you can use the `to_csv()` method. For example, to export the dataframe `df` to a file named ‘data.csv’, use the following code:
“`python
df.to_csv(‘data.csv’, index=False)
“`
These are just a few examples of the many operations you can perform on dataframes in Python. With pandas, you have a powerful toolkit for working with structured data efficiently and effectively.
In conclusion, dataframes are an essential component of working with structured data in Python. They provide a convenient way to organize, manipulate, and analyze data, making it easier to extract insights and make informed decisions. Whether you are a data scientist, analyst, or simply working with datasets, having a strong understanding of dataframes in Python will greatly enhance your data manipulation capabilities.
Images related to the topic r list of data frames
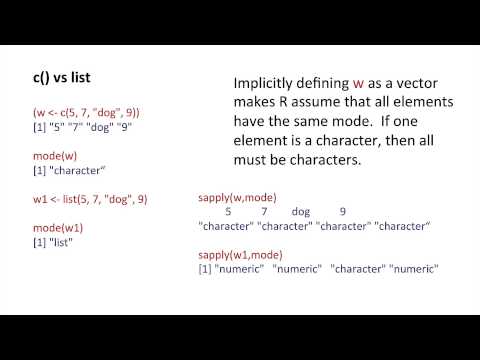
Found 33 images related to r list of data frames theme
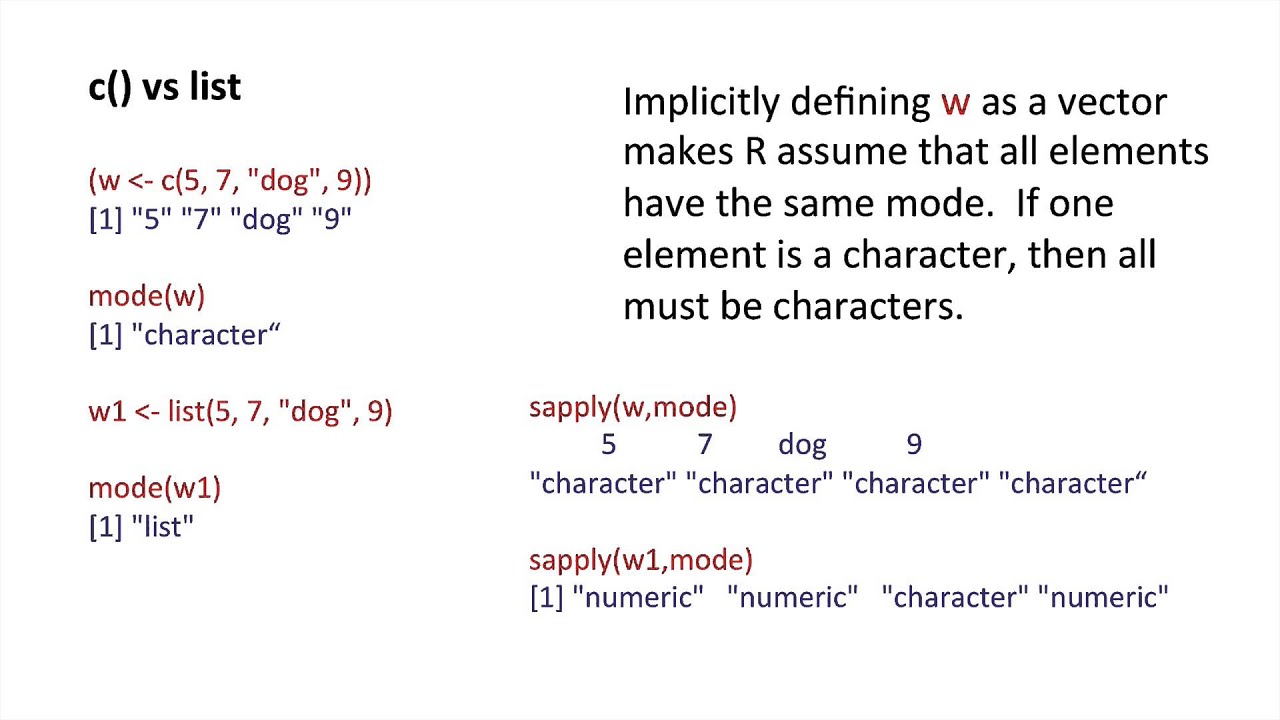
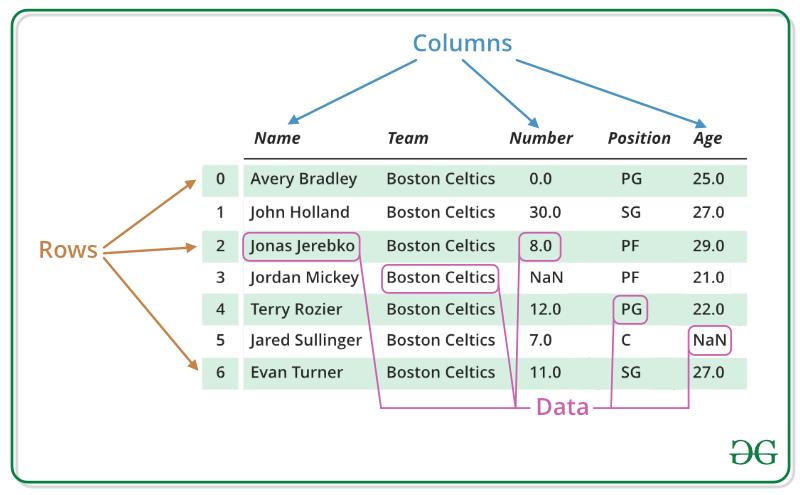
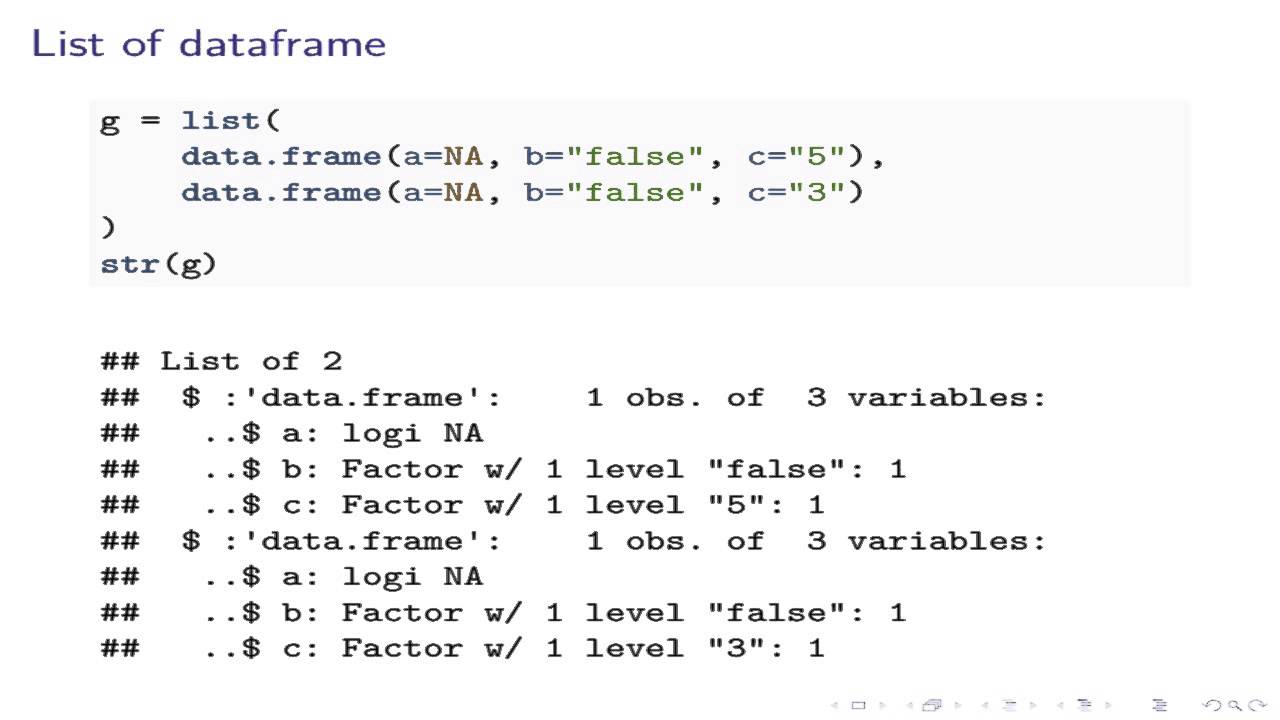

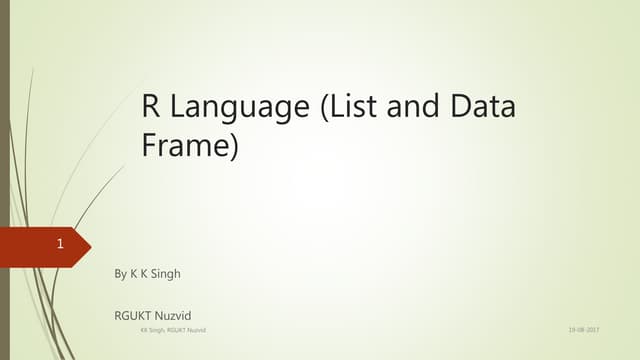

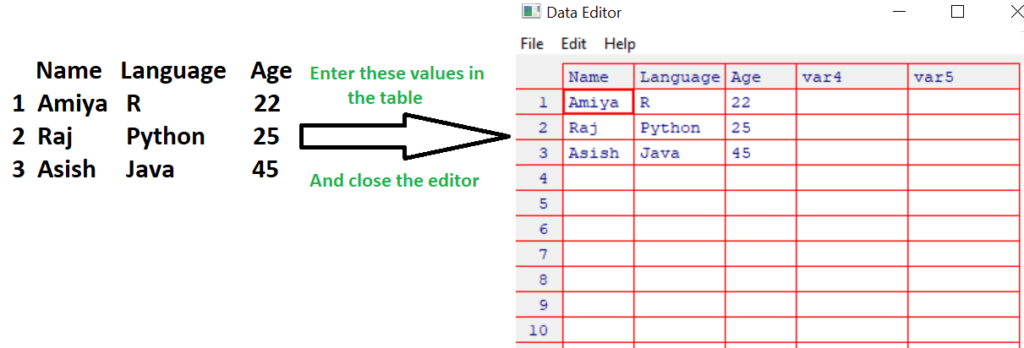
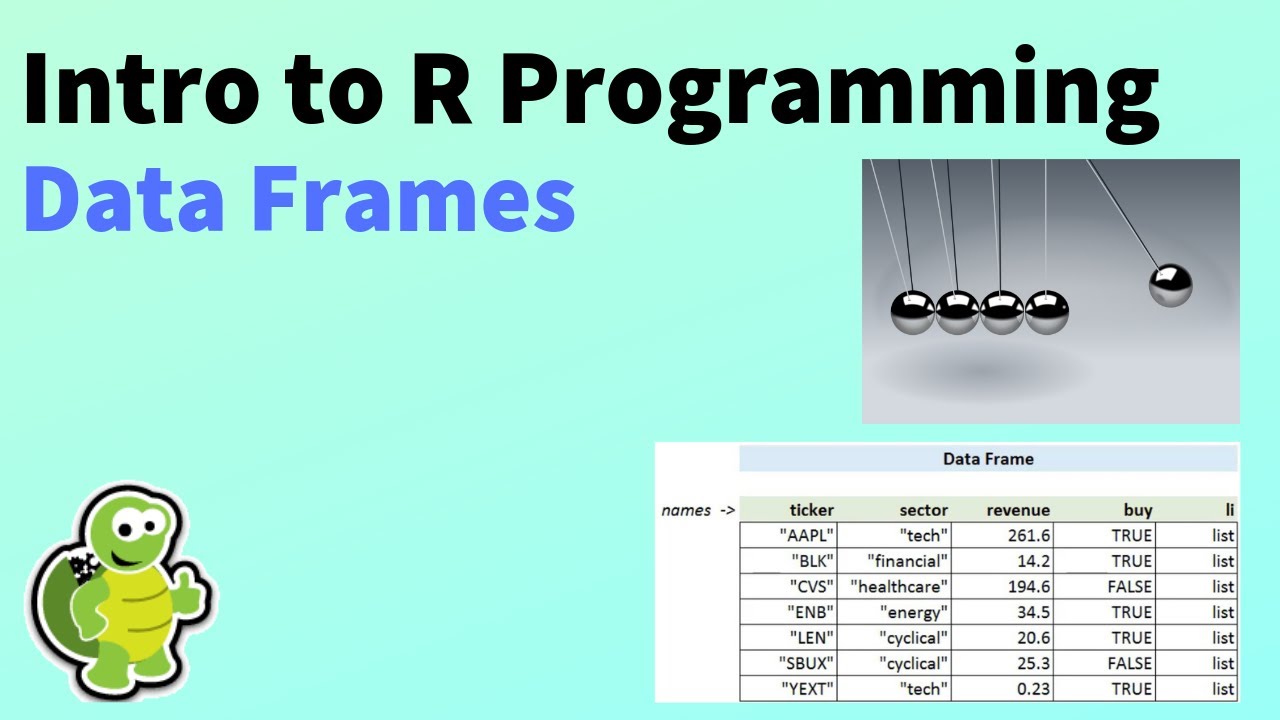
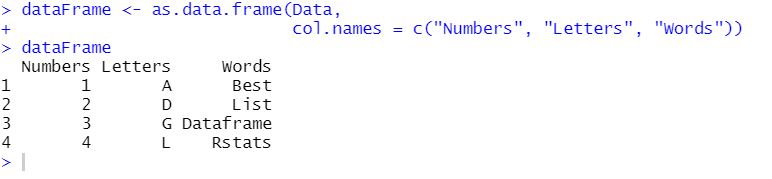

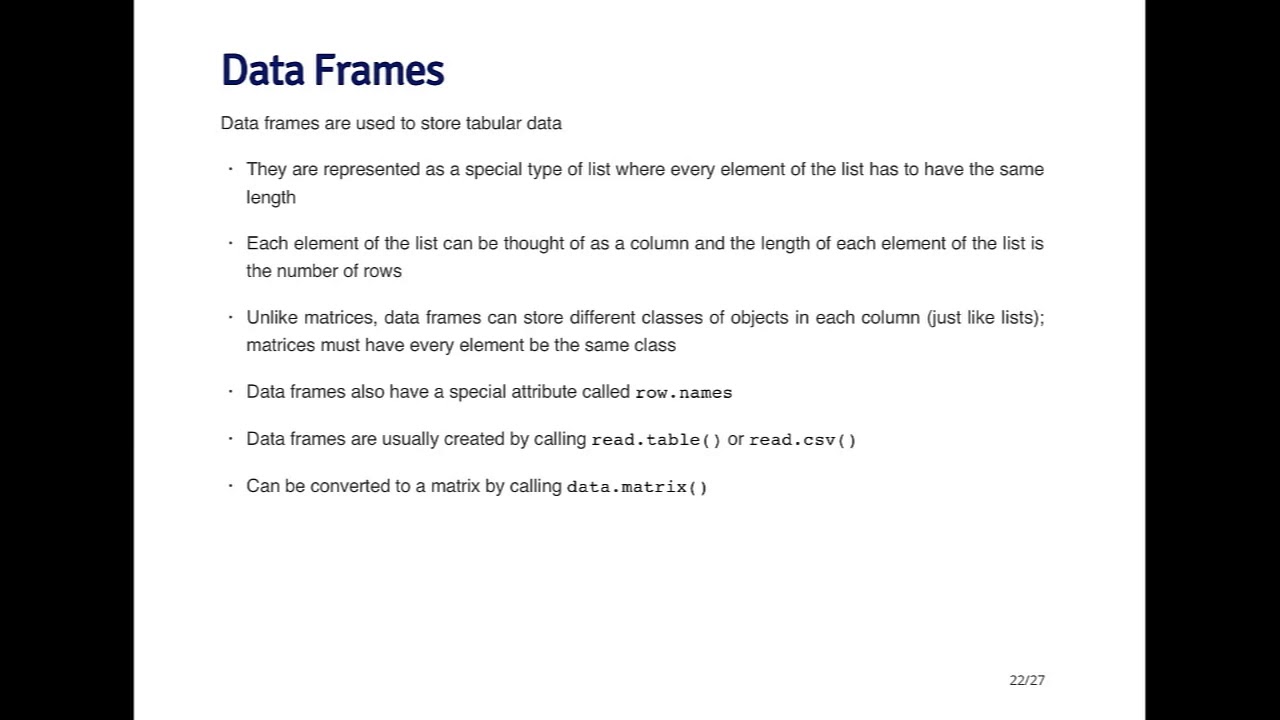
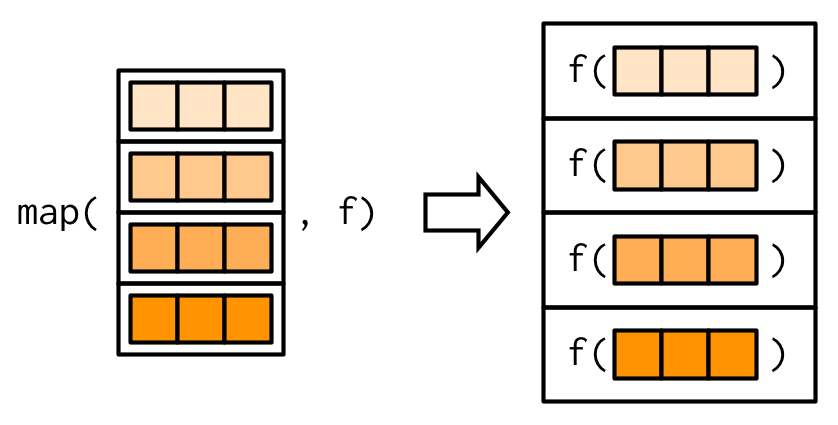
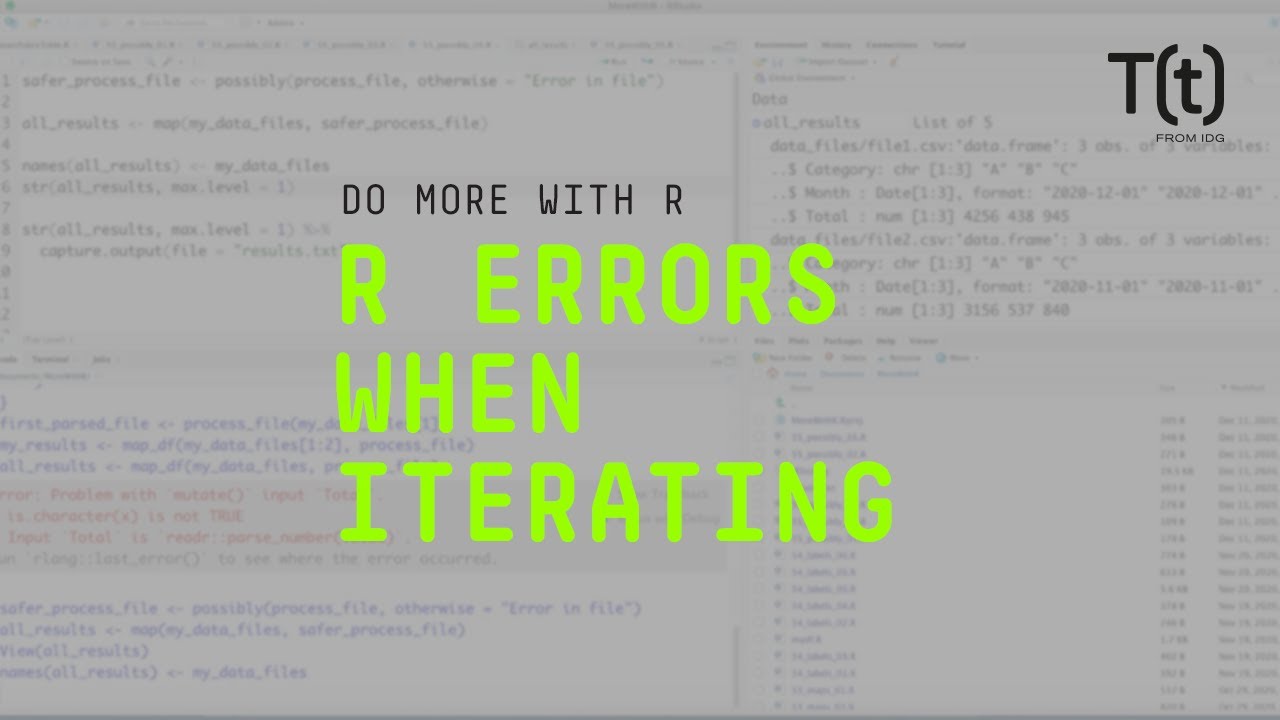
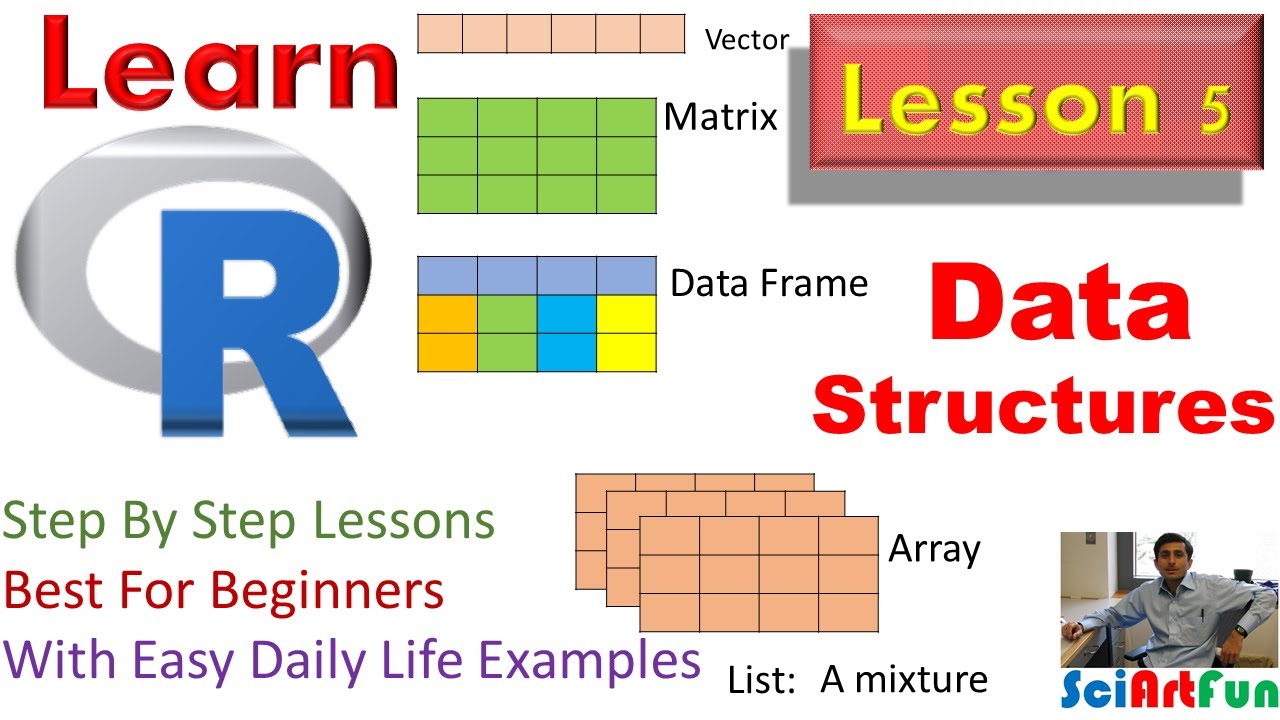
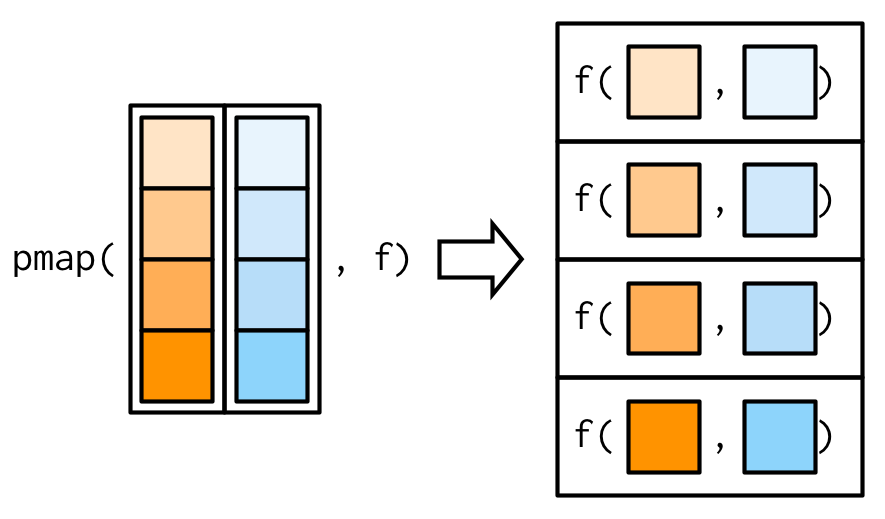
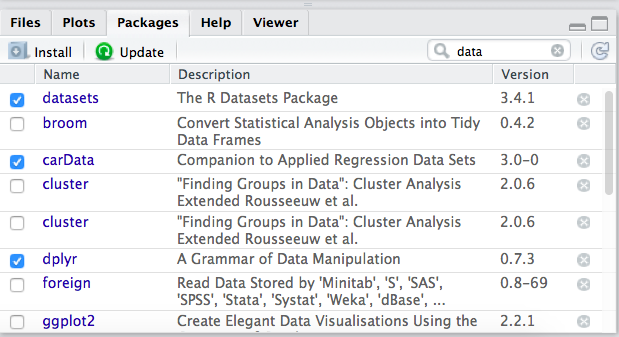
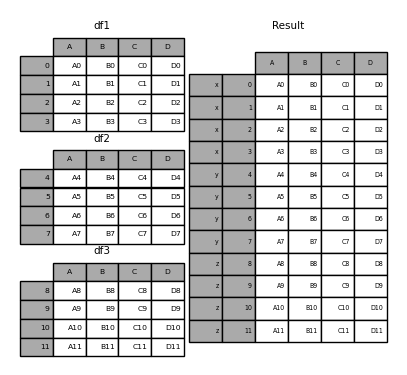
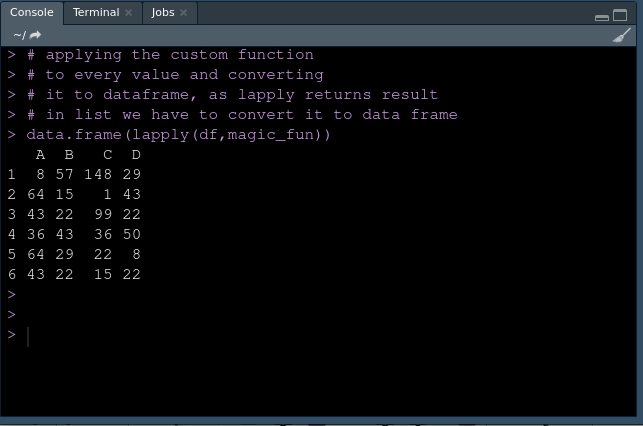
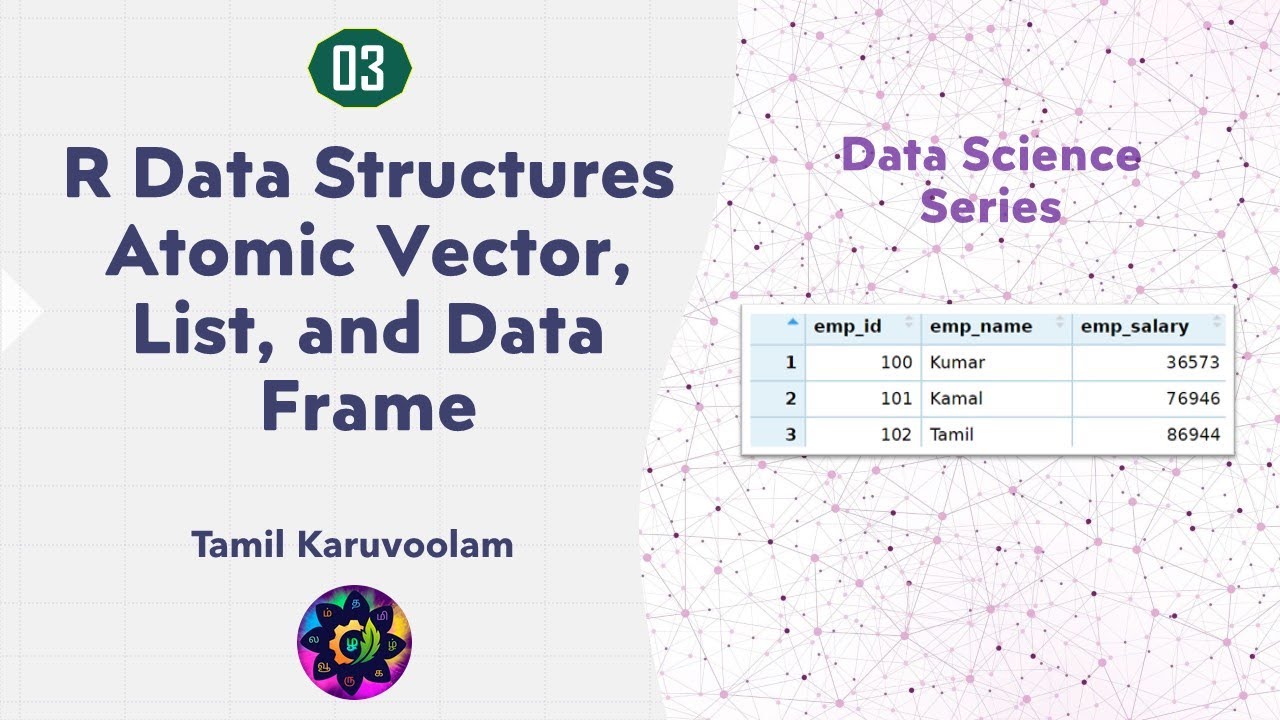
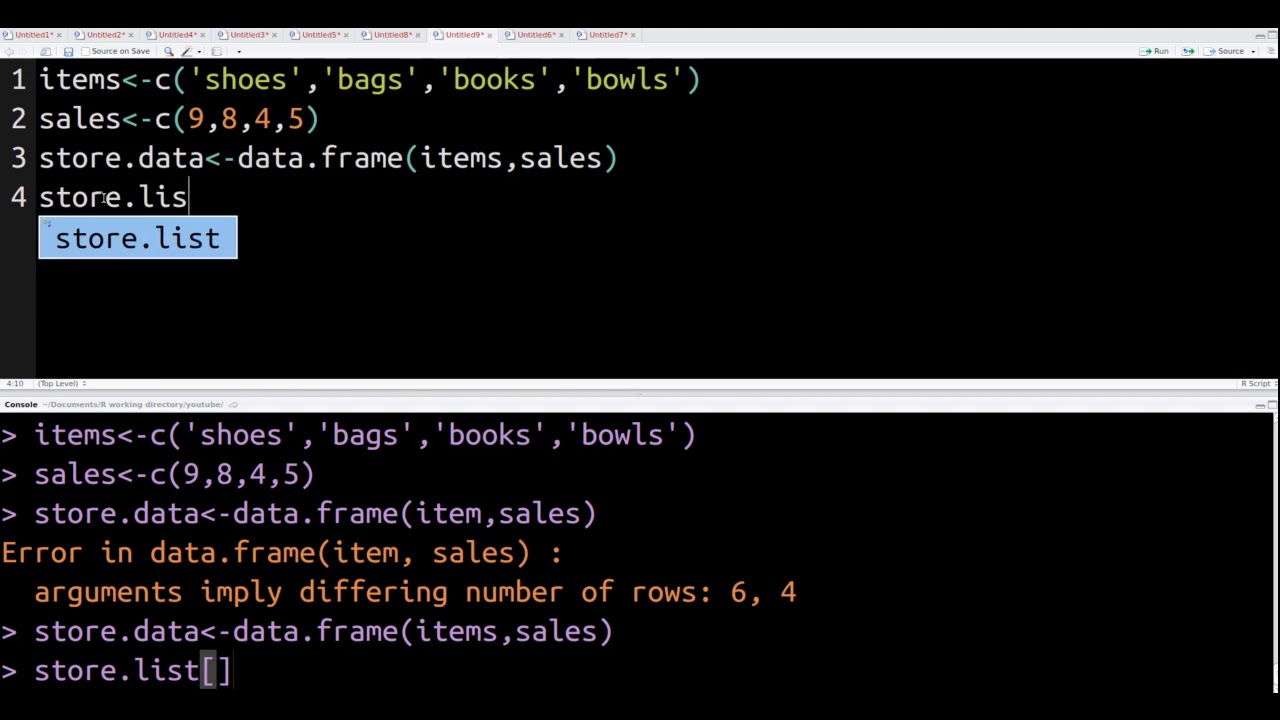

Article link: r list of data frames.
Learn more about the topic r list of data frames.
- List of Dataframes in R – GeeksforGeeks
- How do I make a list of data frames? – Stack Overflow
- Create List of Data Frames in R (Example) – Statistics Globe
- List of Dataframes in R – GeeksforGeeks
- How to Create and Manipulate Lists and Data frames in R
- How to Create and Manipulate Lists and Data frames in R
- R Program to Make a List of Dataframes – Programiz
- Chapter 3 Working with lists and data frames – Bookdown
- R Manuals :: An Introduction to R – 6 Lists and data frames
- Convert List to DataFrame in R – Spark By {Examples}
See more: https://nhanvietluanvan.com/luat-hoc