Python Zip Two Lists
The zip() function in Python is a built-in function that allows you to efficiently merge two or more lists together. It takes multiple iterables as input, such as lists, tuples, or strings, and returns an iterator of tuples, where each tuple contains the corresponding elements from the input iterables.
1. Overview of the zip() function:
The zip() function takes multiple iterables as arguments and returns an iterator of tuples. It creates a new iterable object that aggregates elements from each of the input iterables and stops when the shortest input iterable is exhausted.
2. Syntax and parameters of the zip() function:
The syntax for using the zip() function is as follows:
“` python
zip(*iterables)
“`
The zip() function can take any number of iterables as arguments, separated by commas. It returns an iterator of tuples, where each tuple contains the corresponding elements from the input iterables.
II. Merging two lists using the zip() function
The zip() function is commonly used to merge two lists together. It pairs up elements from two or more lists based on their index positions and creates a new list of tuples.
1. Explanation of how zip() merges two lists:
When two lists are passed to the zip() function, it pairs up the elements at corresponding index positions and creates a new list of tuples. The first element of the first list is paired with the first element of the second list, the second element with the second element, and so on.
2. Example code snippet demonstrating list merging with zip():
Here’s an example that demonstrates how to merge two lists using the zip() function:
“` python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = list(zip(list1, list2))
print(merged_list)
“`
Output:
“`
[(1, 4), (2, 5), (3, 6)]
“`
In this example, the zip() function combines the elements from `list1` and `list2` into a new list `merged_list`. Each tuple in `merged_list` contains the corresponding elements from `list1` and `list2` at the same index position.
III. Handling lists of unequal length with zip()
When the lists passed to the zip() function have different lengths, the zip() function stops iterating as soon as the shortest iterable is exhausted. This behavior can be useful in some scenarios, but it’s important to be aware of it to avoid unexpected results.
1. Dealing with unequal length lists in zip():
When the lists have different lengths, the zip() function stops pairing up elements as soon as the shortest list is exhausted. The remaining elements from the longer list will be ignored.
2. Illustration of various scenarios and their outcomes:
Let’s consider an example to illustrate the behavior of zip() with lists of unequal lengths:
“` python
list1 = [1, 2, 3, 4]
list2 = [‘a’, ‘b’, ‘c’]
merged_list = list(zip(list1, list2))
print(merged_list)
“`
Output:
“`
[(1, ‘a’), (2, ‘b’), (3, ‘c’)]
“`
In this example, the third element from `list1` and `list2` is not included in the merged list because `list2` is shorter. The zip() function stops pairing up elements as soon as `list2` is exhausted.
IV. Unpacking the zipped list
The result obtained from the zip() function is an iterator of tuples. To access individual elements from the zipped list, we can unpack the tuples using the * operator.
1. Exploring the result obtained from zip():
When the zip() function is used, it returns an iterator of tuples. Each tuple contains the corresponding elements from the input iterables.
2. Unpacking the zipped list using the * operator:
To access individual elements from the zipped list, we can unpack the tuples using the * operator. The * operator can be used to unpack the zipped list into separate variables or to convert it back into separate lists.
V. Alternative methods to zip two lists in Python
While the zip() function is a convenient way to merge two or more lists, there are alternative methods available in Python that can achieve similar results. Some of these methods include list comprehension and using the map() function.
1. Introducing alternative approaches to merging lists:
In addition to the zip() function, there are alternative approaches to merging lists in Python. These approaches provide flexibility and can be used based on specific requirements.
2. Comparison of zip() with other methods such as list comprehension and map():
List comprehension is a concise way to merge lists in Python. It allows for more complex logic and transformations during the merging process. On the other hand, the map() function can be used to apply a specified function to each corresponding pair of elements from the input lists.
VI. Practical examples and use cases
The zip() function can be applied in various real-life examples and use cases to simplify and enhance the efficiency of code.
1. Real-life examples where zip() proves useful:
– Combining data from multiple sources: The zip() function can be used to merge data from multiple lists or dictionaries, making it easier to analyze and process the combined information.
– Iterating over pairs of elements: Using zip(), you can iterate over pairs of elements from two or more lists simultaneously, performing operations or comparisons.
– Creating dictionaries from two lists: By zipping together two lists, one containing keys and the other containing values, you can easily create a dictionary with the elements from both lists.
2. Use of zip() for tasks like combining data, iterating over pairs, etc.:
a. Zip two lists with different length Python:
When you have lists of different lengths and you want to combine them, the zip() function allows you to pair up elements based on their index positions, stopping when the shortest list is exhausted.
b. Sort 2 lists together Python:
You can use the zip() function along with the sorted() function to sort two lists together. The zip() function pairs up elements from the two lists, and the sorted() function sorts these pairs based on a specified key.
c. For 2 list Python:
Using the zip() function in a for loop with two or more lists, you can iterate over pairs of elements from the lists and perform operations or comparisons on them.
d. Zip list of list Python:
The zip() function can be used to zip together multiple lists into a single list of tuples. This is useful when you want to combine multiple lists element-wise.
e. Zip() trong Python:
The phrase “Zip() trong Python” is not clear and does not seem to provide any specific use case or example. Could you please provide more context or clarification?
f. Unzip list Python:
To unzip a list of tuples, you can use the zip() function with the * operator.
g. Python zip geeksforgeeks:
GeeksforGeeks is a popular platform for learning programming languages, and they have various articles and tutorials on Python. Searching for “Python zip geeksforgeeks” on the GeeksforGeeks website will provide articles and resources related to the zip() function in Python.
h. 2 list to dictionary Python:
By zipping together two lists, one containing keys and the other containing values, you can create a dictionary with the elements from both lists.
In conclusion, the zip() function in Python allows you to efficiently merge two or more lists together. It provides a convenient way to pair up elements based on their index positions and create a new list of tuples. The zip() function can handle lists of unequal lengths and offers flexibility through the ability to unpack the zipped list. While zip() is a powerful tool, there are alternative methods available in Python for merging lists, such as list comprehension and the map() function. The zip() function finds utility in various real-life examples and use cases, providing simplicity and efficiency to code implementation.
Python – How To Zip Two Lists
Can I Zip Two Lists In Python?
Python is a versatile and powerful programming language that provides numerous features to simplify various tasks. One such task is combining or merging lists in Python. While there are several methods to achieve this, one commonly used approach is to employ the zip() function. In this article, we will explore in-depth the functionality of zip(), discuss its implementation, examine some use cases, and address frequently asked questions.
The zip() function in Python is used to combine or merge multiple lists into a single list. It takes in two or more iterables as arguments and returns an iterator of tuples. Each tuple contains elements from the corresponding positions of the input lists.
Let’s consider an example to understand the implementation of zip():
“`
list1 = [1, 2, 3]
list2 = [‘a’, ‘b’, ‘c’]
zipped = zip(list1, list2)
“`
In this example, we have two lists, `list1` and `list2`, consisting of integer and string elements, respectively. By calling `zip(list1, list2)`, we create a zipped object that combines the elements of both lists. The resulting `zipped` object is an iterator of tuples, where each tuple contains one element from `list1` and one element from `list2`.
To access the elements of the zipped object, we can convert it into a list or iterate over it using a loop. Consider the following code snippet:
“`
zipped_list = list(zipped)
print(zipped_list)
for item in zipped_list:
print(item)
“`
The `list()` function is used to convert the zipped object into a list called `zipped_list`. When we print `zipped_list`, the output would be: `[(1, ‘a’), (2, ‘b’), (3, ‘c’)]`. This corresponds to a list of tuples containing the merged elements of `list1` and `list2` at their respective indices. In the loop, we iterate over the zipped list and print each tuple, resulting in the following output:
“`
(1, ‘a’)
(2, ‘b’)
(3, ‘c’)
“`
Now that we understand the basic implementation of zip(), let’s discuss some useful applications.
Applications of zip():
1. Parallel Iteration: The zip() function is beneficial when we need to process multiple lists in parallel. Consider the following example:
“`
names = [‘Alice’, ‘Bob’, ‘Charlie’]
ages = [25, 30, 35]
countries = [‘USA’, ‘UK’, ‘Canada’]
for name, age, country in zip(names, ages, countries):
print(f”{name} is {age} years old and lives in {country}.”)
“`
In this example, we have three lists representing names, ages, and countries. By using zip(), we iterate over these lists in parallel and print the corresponding information. The output would be:
“`
Alice is 25 years old and lives in USA.
Bob is 30 years old and lives in UK.
Charlie is 35 years old and lives in Canada.
“`
2. Merging Lists of Different Lengths: zip() is particularly useful when merging lists of different lengths.
“`
list1 = [1, 2, 3]
list2 = [‘a’, ‘b’]
zipped = zip(list1, list2)
for item in zipped:
print(item)
“`
In this case, where `list1` has three elements and `list2` has only two, zip() will stop at the end of the shorter list. Hence, the output would be:
“`
(1, ‘a’)
(2, ‘b’)
“`
3. Unzipping: Aside from merging lists, zip() also allows for unzipping, which means splitting a zipped list back into its original components. We can achieve this by using the * operator alongside the zip() function.
“`
zipped_list = [(1, ‘a’), (2, ‘b’), (3, ‘c’)]
unzipped = list(zip(*zipped_list))
print(unzipped)
“`
In this example, the zipped list `[(1, ‘a’), (2, ‘b’), (3, ‘c’)]` is unzipped using the * operator and the zip() function. The output would be:
“`
[(1, 2, 3), (‘a’, ‘b’, ‘c’)]
“`
Frequently Asked Questions:
1. Can I zip more than two lists?
Yes, zip() can handle any number of iterables. You can provide three or more arguments to the zip() function, and it will create an iterator of tuples containing elements from all the lists.
2. Can I zip lists with different data types?
Certainly! zip() is not restricted to lists of the same data type. You can combine lists with different data types, such as integers, strings, floats, etc.
3. What happens if the input lists have different lengths?
When the input lists have different lengths, zip() will stop at the end of the shortest list. The resulting zipped object will only contain tuples up to the length of the shortest list.
4. How can I unzip a zipped list?
To unzip a zipped list, you can use the * operator alongside zip(). This will convert the zipped list into separate tuples or lists, effectively undoing the zipping process.
In conclusion, the zip() function in Python is a powerful tool for combining or merging lists. It allows parallel iteration and merging of lists with different lengths or data types. Additionally, it provides the capability to unzip a zipped list. Understanding the functionality and various applications of zip() can significantly simplify list manipulation tasks in Python.
Is Zip () In Python 2?
Python is a versatile programming language known for its simplicity and readability. It provides a wide range of built-in functions that make coding easier and more efficient. One such function is zip(), which allows you to combine multiple iterables into a single iterable object. In this article, we will explore how zip() works in Python 2 and delve into its various use cases.
The zip() function in Python 2 takes two or more iterables as arguments and returns an iterator of tuples. Each tuple contains the corresponding elements from the input iterables. In other words, the function pairs up elements with the same index from each of the iterables and creates a new iterable object.
To better understand zip(), let’s consider an example:
“`python
numbers = [1, 2, 3]
letters = [‘a’, ‘b’, ‘c’]
result = zip(numbers, letters)
print list(result)
“`
Output:
“`
[(1, ‘a’), (2, ‘b’), (3, ‘c’)]
“`
In this example, we have two iterables, `numbers` and `letters`. After applying zip(), the resulting iterable `result` contains tuples that pair up the corresponding elements of both iterables. As we can see from the output, each tuple contains one element from the `numbers` list and one element from the `letters` list.
One important thing to note is that zip() stops when the shortest input iterable is exhausted. This means that if one of the iterables has fewer elements than the others, zip() will only process up to the length of the shortest iterable. This behavior ensures that the resulting iterable does not contain any incomplete tuples.
Zip() is not limited to just two iterables; it can take any number of iterables as arguments. For example:
“`python
name = [‘John’, ‘Mary’, ‘David’]
age = [25, 30, 35]
profession = [‘Engineer’, ‘Teacher’, ‘Doctor’]
result = zip(name, age, profession)
print list(result)
“`
Output:
“`
[(‘John’, 25, ‘Engineer’), (‘Mary’, 30, ‘Teacher’), (‘David’, 35, ‘Doctor’)]
“`
In this case, zip() takes three input iterables – `name`, `age`, and `profession`. It pairs up the corresponding elements from each iterable and creates tuples with three elements each. The resulting iterable contains tuples containing the name, age, and profession of each person.
Zip() is commonly used in scenarios where you need to iterate over multiple lists simultaneously. It provides an elegant way to process and manipulate data from multiple sources in a synchronized manner.
Now let’s move on to the FAQ section to address some common queries related to zip() in Python 2.
## FAQs
**Q: Can zip() be used with different types of iterables?**
A: Yes, zip() can be used with different types of iterables, such as lists, tuples, and strings. The only requirement is that the input iterables should have the same length, or else zip() will truncate the result to the length of the shortest iterable.
**Q: Is the resulting iterable from zip() mutable?**
A: No, the resulting iterable from zip() is not mutable. It is an iterator that can only be traversed once. If you want to modify the contents or access elements multiple times, convert it into a mutable data structure like a list or tuple.
**Q: How does zip() handle iterables of different lengths?**
A: Zip() pairs up elements with the same index from each iterable until the shortest iterable is exhausted. Once the shortest iterable is exhausted, zip() stops, discarding any remaining elements from the longer iterables. This ensures that the resulting iterable contains complete tuples.
**Q: What happens when zip() is called with no arguments?**
A: Calling zip() with no arguments will result in an empty iterable. Since there are no input iterables to pair up elements from, the resulting iterable will be empty as well.
In conclusion, zip() is a versatile function in Python 2 that allows you to combine multiple iterables into a single iterable of tuples. It simplifies the process of iterating over multiple lists simultaneously and provides a concise way to process synchronized data. By understanding the behavior and use cases of zip(), you can enhance your coding capabilities and make your programs more efficient.
Keywords searched by users: python zip two lists Zip two lists with different length Python, Sort 2 lists together Python, For 2 list Python, Zip list of list Python, Zip() trong Python, Unzip list Python, Python zip geeksforgeeks, 2 list to dictionary Python
Categories: Top 93 Python Zip Two Lists
See more here: nhanvietluanvan.com
Zip Two Lists With Different Length Python
Python provides a versatile built-in function called `zip()` that allows you to zip or combine multiple lists together. However, using `zip()` can become a bit challenging when the lists have different lengths. This article will guide you through the process of zipping two lists with different lengths in Python, providing explanations and code examples for a better understanding.
## Understanding `zip()` in Python
Before diving into zipping lists with different lengths, let’s first understand the basic functioning of the `zip()` function. The `zip()` function takes two or more sequences as input and returns an iterator that generates tuples with elements from each input sequence.
For example, consider the following lists:
“`python
list1 = [1, 2, 3]
list2 = [‘a’, ‘b’, ‘c’]
list3 = [‘x’, ‘y’, ‘z’]
“`
Using `zip()` on these lists:
“`python
zipped_lists = zip(list1, list2, list3)
“`
The resulting `zipped_lists` will be an iterator of tuples:
“`python
[(1, ‘a’, ‘x’), (2, ‘b’, ‘y’), (3, ‘c’, ‘z’)]
“`
Notice that the `zip()` function only zips up to the shortest list length. This behavior poses a challenge when we want to zip two lists of different lengths. However, there are several ways to tackle this problem.
## Zip Lists with Different Lengths using `zip_longest()`
To zip two lists of unequal lengths, we can utilize the `zip_longest()` function from the `itertools` module. `zip_longest()` pads the shorter list with a specified value, commonly `None`, filling the missing elements.
To use `zip_longest()`, first, you need to import it from the `itertools` module:
“`python
from itertools import zip_longest
“`
Now, let’s look at an example:
“`python
list1 = [1, 2, 3, 4]
list2 = [‘a’, ‘b’]
zipped_lists = zip_longest(list1, list2, fillvalue=None)
“`
In this example, the resulting `zipped_lists` will be:
“`python
[(1, ‘a’), (2, ‘b’), (3, None), (4, None)]
“`
As you can see, the shorter list is padded with `None` to match the length of the longer list.
## Zip Lists with Different Lengths using List Comprehension
Another way to zip two lists with different lengths is by using list comprehension and the built-in `zip()` function. This method is particularly useful when you want to handle the uneven lengths differently, such as assigning a specific value to the missing elements.
Here’s an example to illustrate the concept:
“`python
list1 = [1, 2, 3, 4]
list2 = [‘a’, ‘b’]
zipped_lists = [(list1[i], list2[i]) if i < len(list2) else (list1[i], 'N/A') for i in range(len(list1))] ``` In this example, we use a conditional statement (`if...else`) inside the list comprehension to assign a default value of `'N/A'` for missing elements in the shorter list. The resulting `zipped_lists` would be: ```python [(1, 'a'), (2, 'b'), (3, 'N/A'), (4, 'N/A')] ``` ## FAQs **Q1: Can I zip more than two lists?** Yes, the `zip()` function allows you to zip any number of lists together. Simply pass all the lists as separate arguments to the `zip()` function. **Q2: Is there an alternative to `zip_longest()` for padding with a specific value other than `None`?** Yes, you can specify any value to use for padding by providing it as the `fillvalue` parameter in `zip_longest()`. For example, if you want to pad with zeros, you can use `fillvalue=0`. **Q3: Can I zip lists with different data types?** Absolutely! The `zip()` function can handle lists with different data types. It will combine elements from each list into tuples, regardless of the data type. **Q4: How can I unzip a zipped list?** To unzip a zipped list, you can use the `zip()` function again. By passing the zipped list to `zip()`, you will obtain the original individual lists. Here's an example: ```python zipped_lists = [(1, 'a'), (2, 'b'), (3, None), (4, None)] unzipped_lists = list(zip(*zipped_lists)) ``` In this example, `unzipped_lists` would be: ```python [(1, 2, 3, 4), ('a', 'b', None, None)] ``` Now you know how to zip two lists with different lengths in Python. Whether you prefer to utilize `zip_longest()` or list comprehension, you can effortlessly combine lists and handle mismatches between their lengths. Harnessing the power of `zip()` enhances your ability to manipulate data effectively and efficiently in Python.
Sort 2 Lists Together Python
Python, being a versatile programming language, offers a variety of ways to sort data. Sorting is an essential operation in many programming tasks, and it becomes even more interesting when two lists need to be sorted together. In this article, we will explore various methods and techniques to sort two lists together in Python. So, let’s dive right in!
Understanding the Problem:
When we talk about sorting two lists together, it generally means that both lists have some kind of association between their elements. For example, if we have two lists, one containing the names of students and the other containing their corresponding exam scores, we might want to sort them together based on the scores. This way, the names and scores stay aligned after the sorting process.
Method 1: Zip and Sort
The zip function in Python combines elements from two or more lists into tuples. By using the zip function and applying a sorting mechanism, we can sort two lists together easily. Let’s take a look at an example:
“`python
names = [‘Alice’, ‘Bob’, ‘Charlie’]
scores = [85, 72, 90]
sorted_data = sorted(zip(names, scores), key=lambda x: x[1])
sorted_names, sorted_scores = zip(*sorted_data)
print(sorted_names) # Output: (‘Bob’, ‘Alice’, ‘Charlie’)
print(sorted_scores) # Output: (72, 85, 90)
“`
Here, we first combine the lists using the zip function and then sort them using the sorted function. In this case, we sort the elements based on the second item in each tuple, which represents the scores. Finally, we unzip the sorted tuples to obtain the sorted names and scores separately.
Method 2: Sort Indices
Another method to sort two lists together is by sorting the indices of one of the lists based on the corresponding values in the other list. Here’s an example:
“`python
names = [‘Alice’, ‘Bob’, ‘Charlie’]
scores = [85, 72, 90]
sorted_indices = sorted(range(len(scores)), key=lambda k: scores[k])
sorted_names = [names[i] for i in sorted_indices]
sorted_scores = [scores[i] for i in sorted_indices]
print(sorted_names) # Output: [‘Bob’, ‘Alice’, ‘Charlie’]
print(sorted_scores) # Output: [72, 85, 90]
“`
In this approach, we first sort the indices of the scores list. The key parameter of the sorted function determines the sorting order based on the corresponding score values. Finally, we use the sorted indices to re-order both lists accordingly.
Method 3: Numpy Arrays
If you are working with numerical data and have the numpy library installed, using numpy arrays can be an efficient way to sort two lists together. Numpy arrays allow element-wise operations, making it easy to sort them based on a specific column. Let’s see an example:
“`python
import numpy as np
names = np.array([‘Alice’, ‘Bob’, ‘Charlie’])
scores = np.array([85, 72, 90])
sorted_indices = np.argsort(scores)
sorted_names = names[sorted_indices]
sorted_scores = scores[sorted_indices]
print(sorted_names) # Output: [‘Bob’ ‘Alice’ ‘Charlie’]
print(sorted_scores) # Output: [72 85 90]
“`
Here, we use the numpy.argsort function to obtain the indices that would sort the scores array. We then use these indices to re-order the names and scores arrays accordingly. Using numpy arrays can provide a significant performance boost when dealing with large datasets.
Frequently Asked Questions (FAQs):
Q1: Can I sort more than two lists together using the methods mentioned above?
A1: Yes, the methods described above can be extended to sort more than two lists together. Simply combine the additional lists using the zip function or apply the sorting mechanism to their indices accordingly.
Q2: What if the lists have different lengths?
A2: If the two lists have different lengths, they can still be sorted together using appropriate techniques. For example, you can use the zip function on only the common elements or sort the indices based on one of the lists and handle the mismatched elements separately.
Q3: Can I sort lists in descending order using the methods mentioned?
A3: Absolutely! By modifying the sorting mechanism or specifying the reverse parameter in the sorted function, you can sort the lists in descending order. For example, `sorted(zip(names, scores), key=lambda x: x[1], reverse=True)` would sort the lists in descending order of scores.
Conclusion:
Sorting two lists together in Python is a common requirement in many programming scenarios. In this article, we explored three methods to achieve this: using the zip function, sorting indices, and utilizing numpy arrays. By understanding these methods, you can efficiently sort multiple lists and keep their elements aligned. If you encounter any challenges, the provided FAQs section should help clarify any doubts. Happy sorting!
Images related to the topic python zip two lists
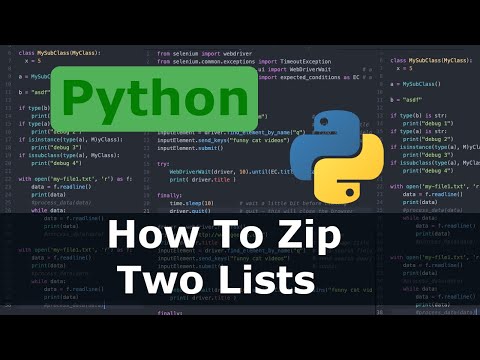
Found 42 images related to python zip two lists theme
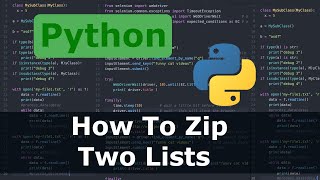
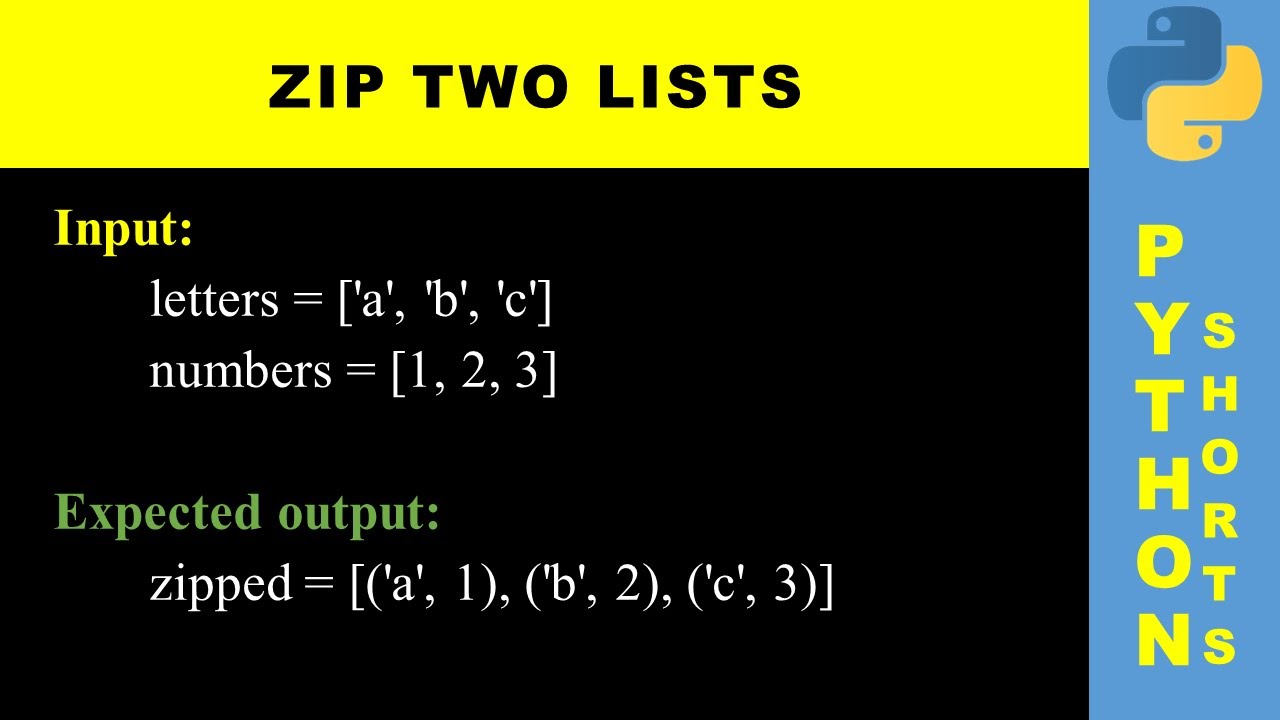


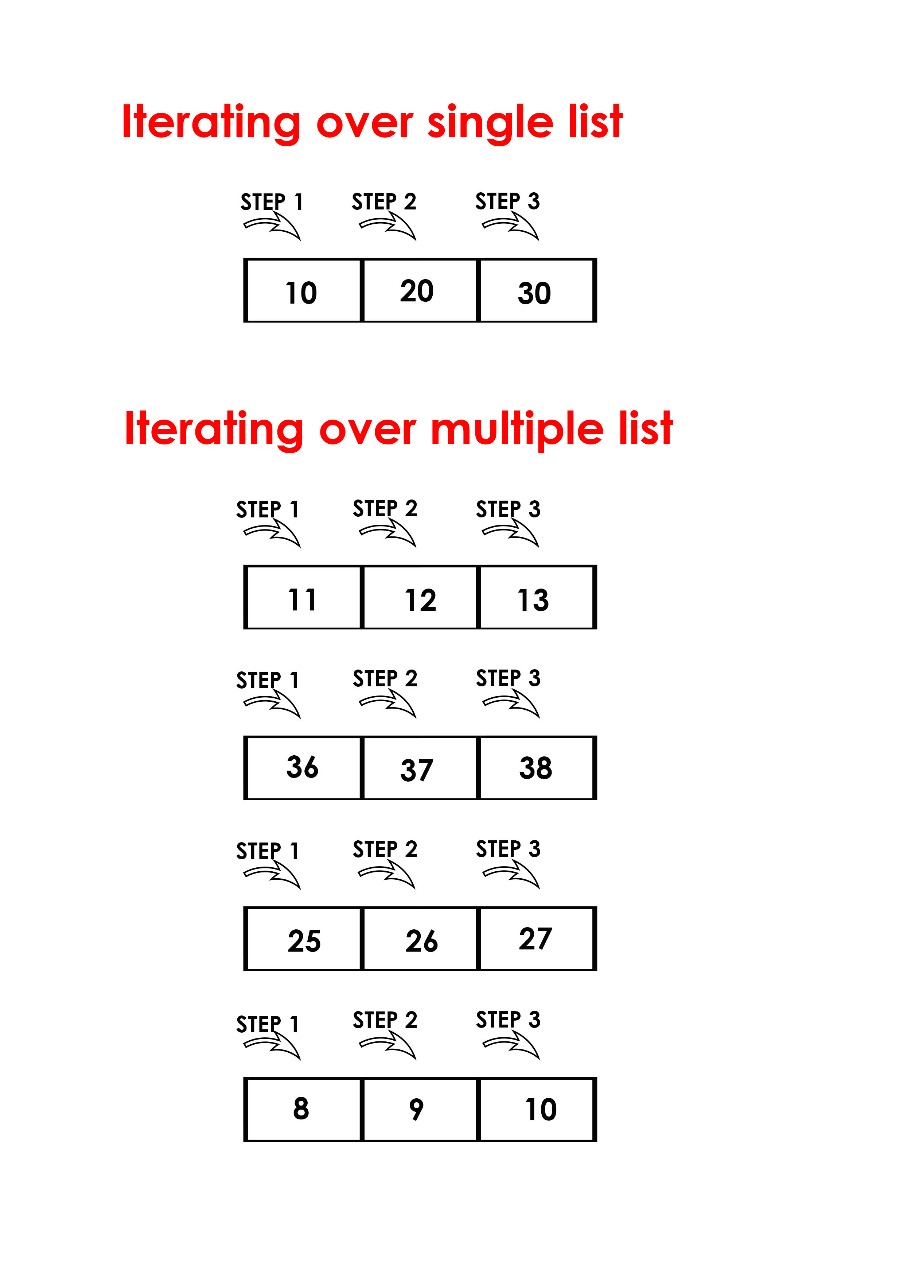
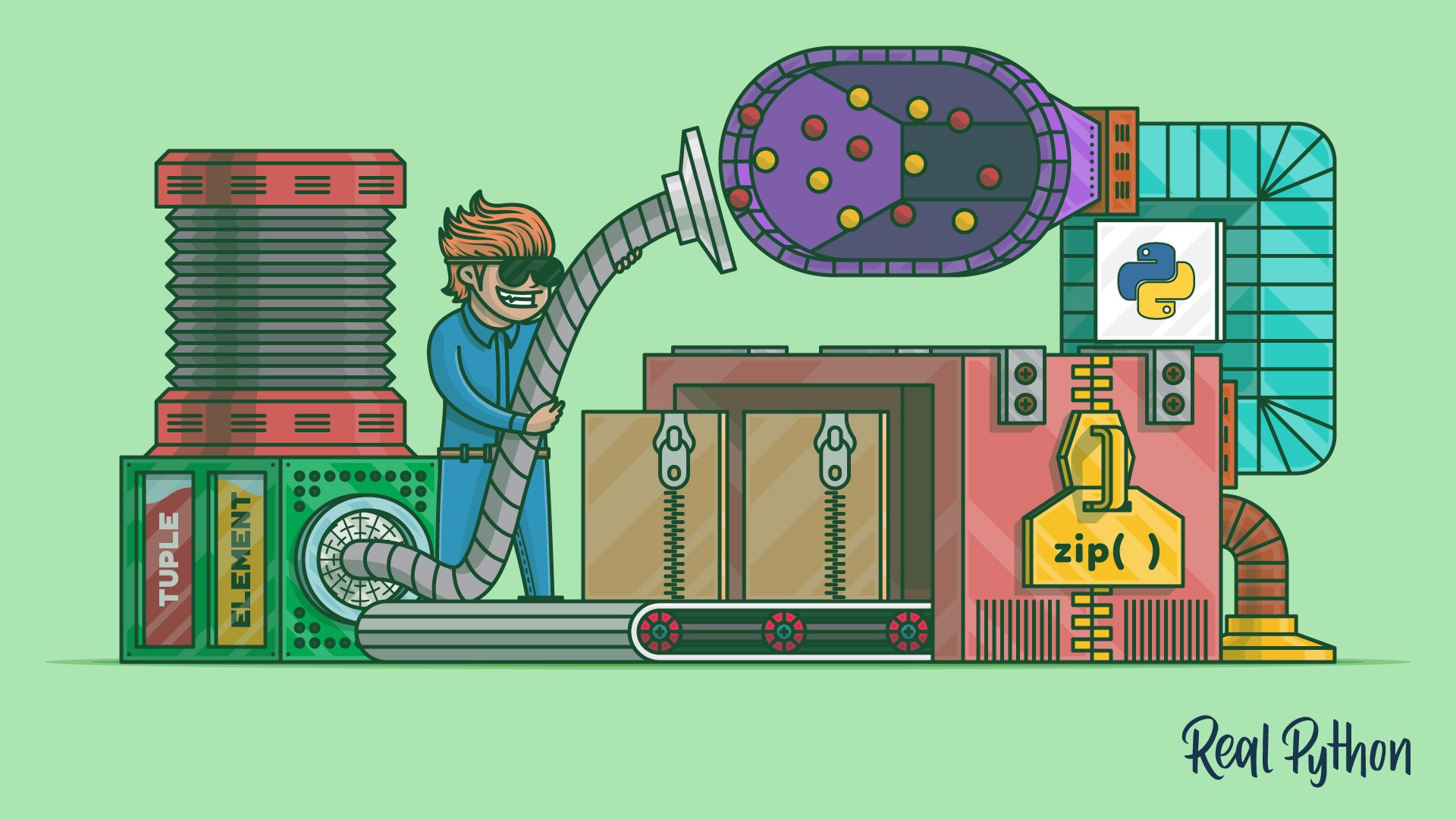




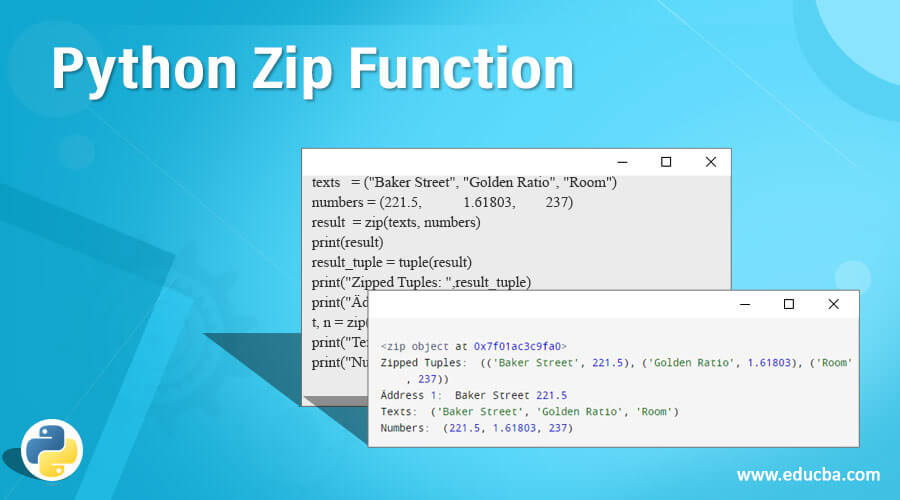
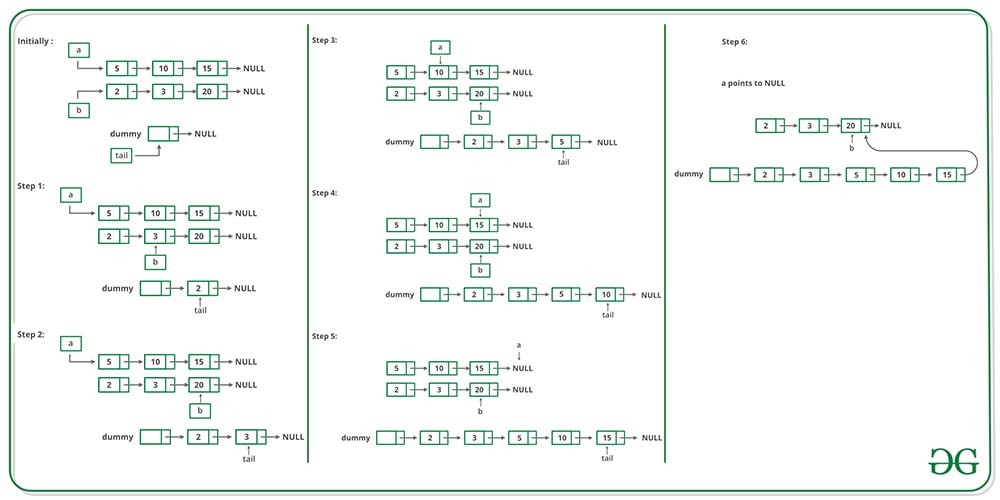

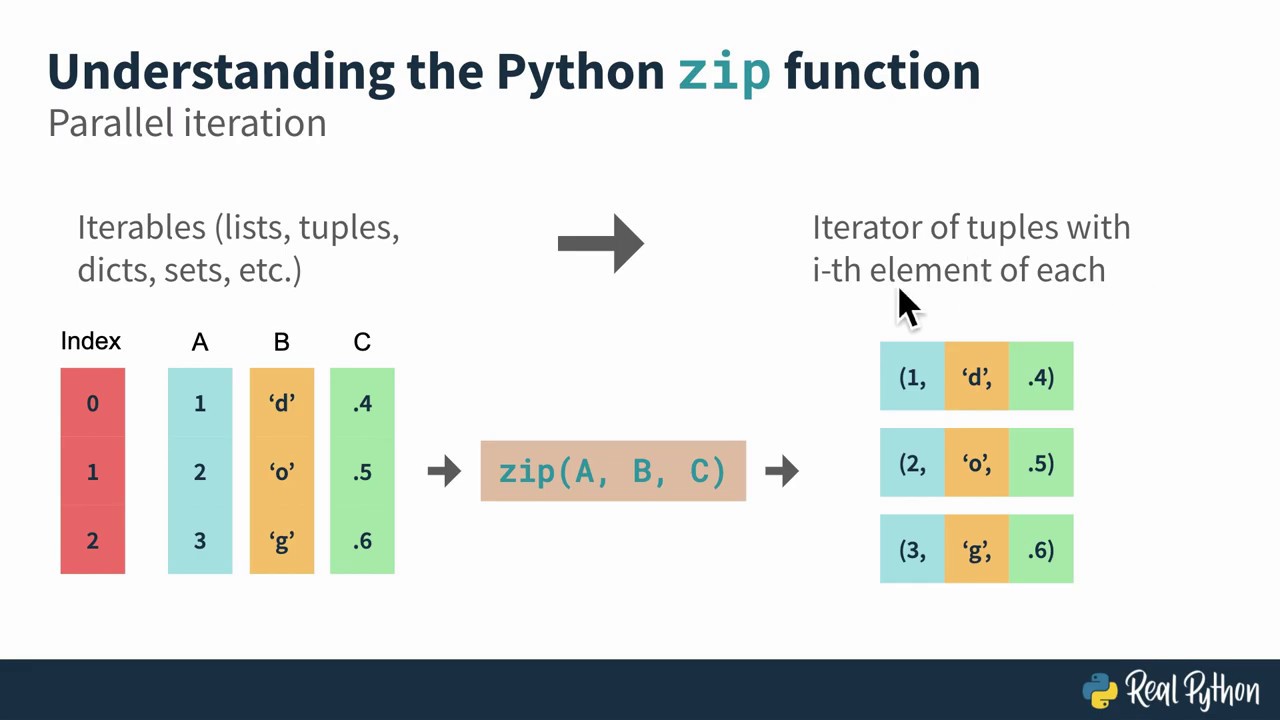
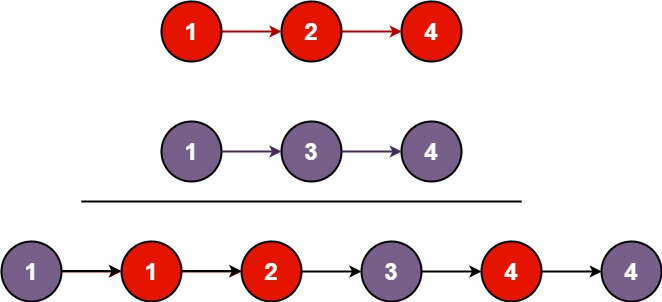

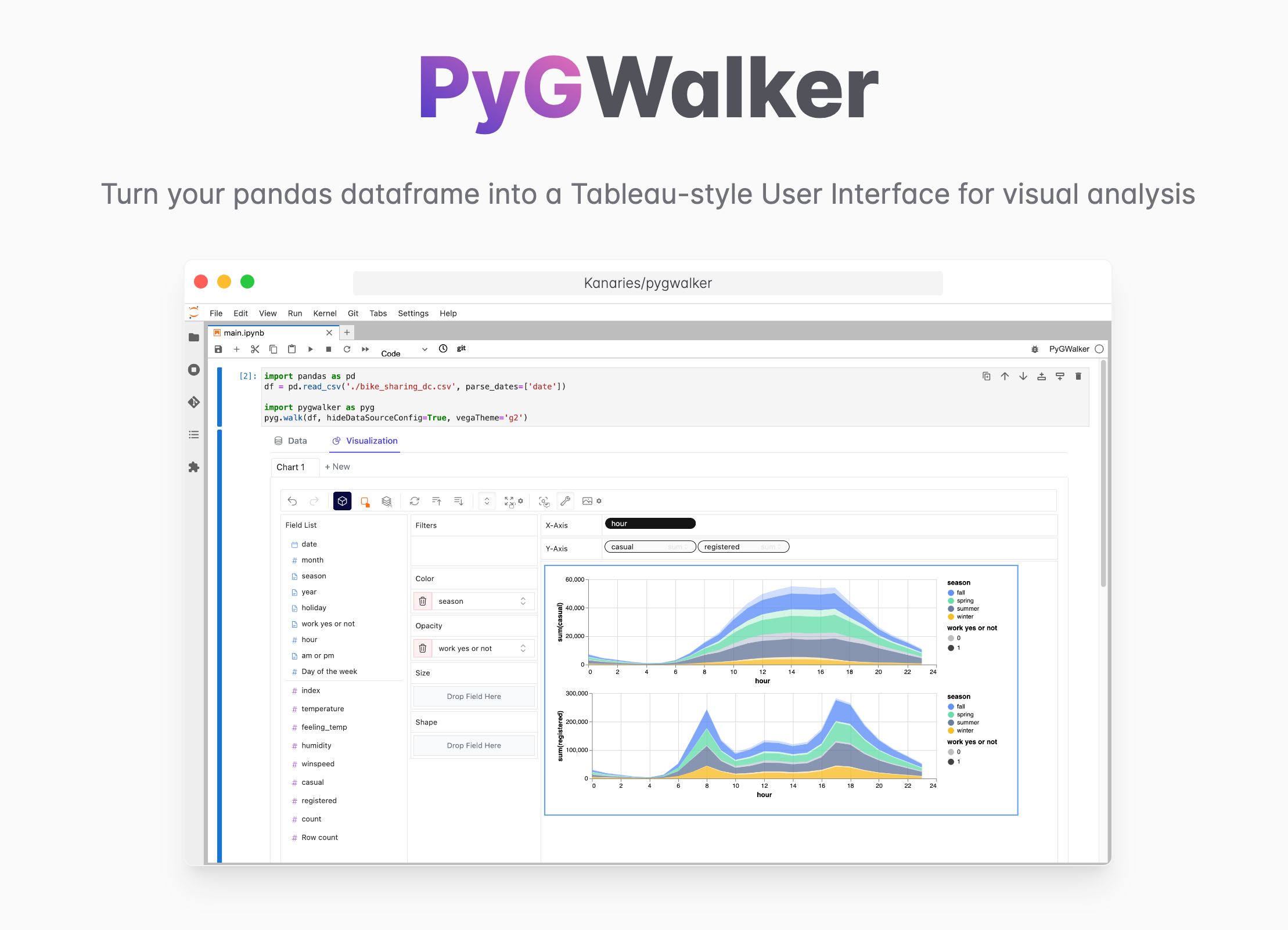
![23] How to Sum Elements of Two Lists in Python | Python for Beginners - YouTube 23] How To Sum Elements Of Two Lists In Python | Python For Beginners - Youtube](https://i.ytimg.com/vi/s7pbHysT0j4/maxresdefault.jpg)

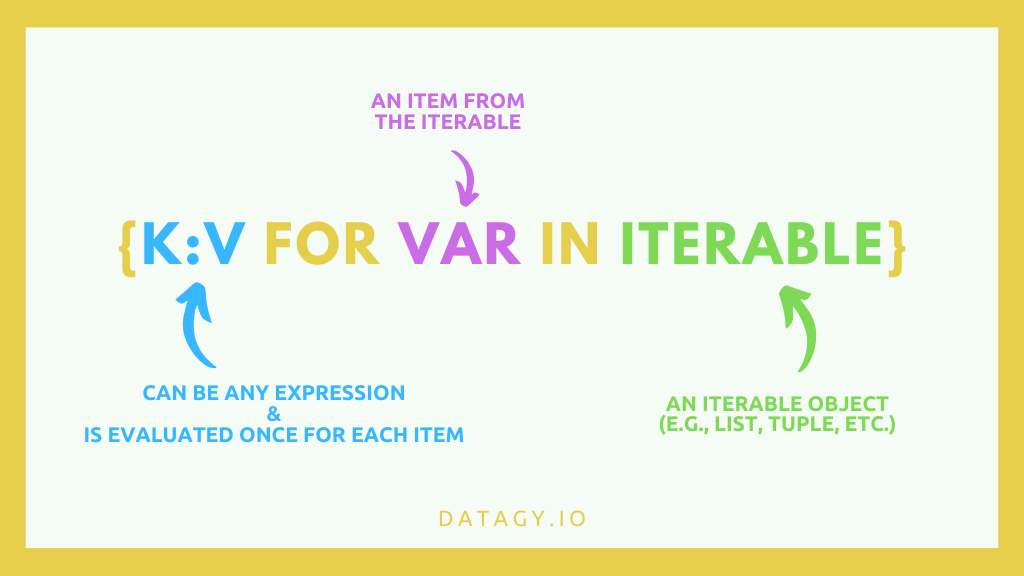
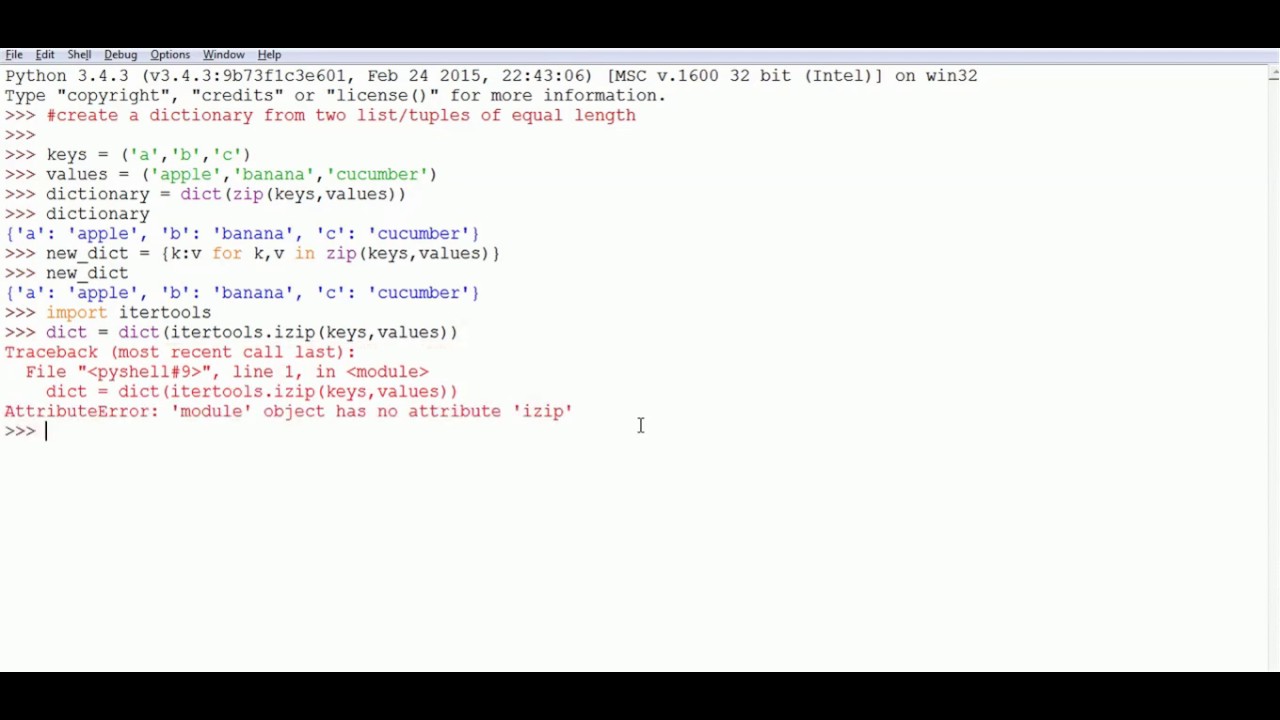


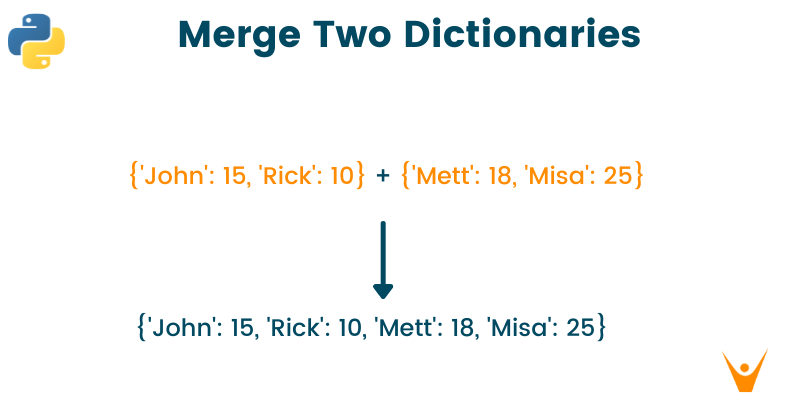
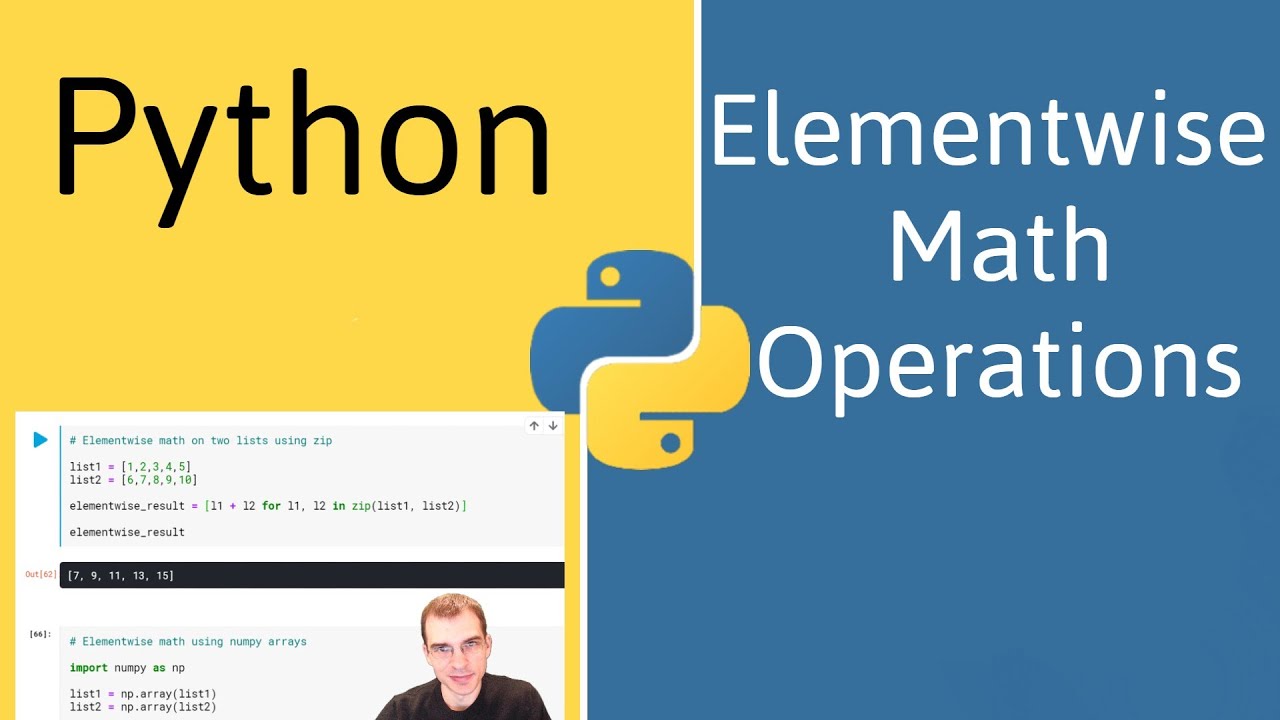
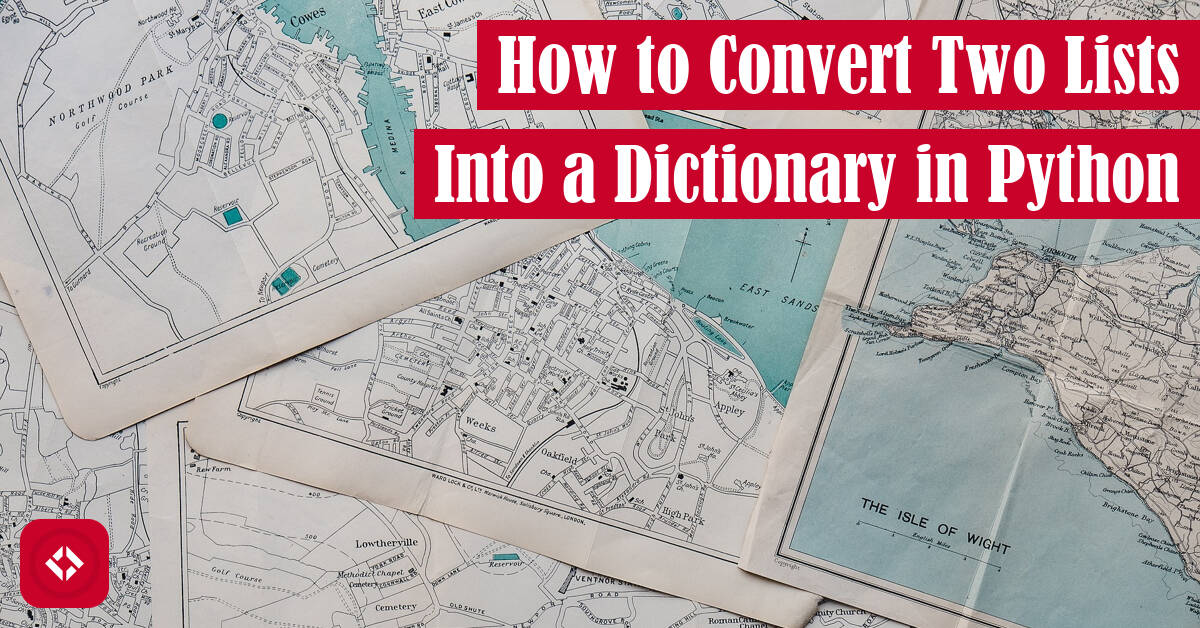
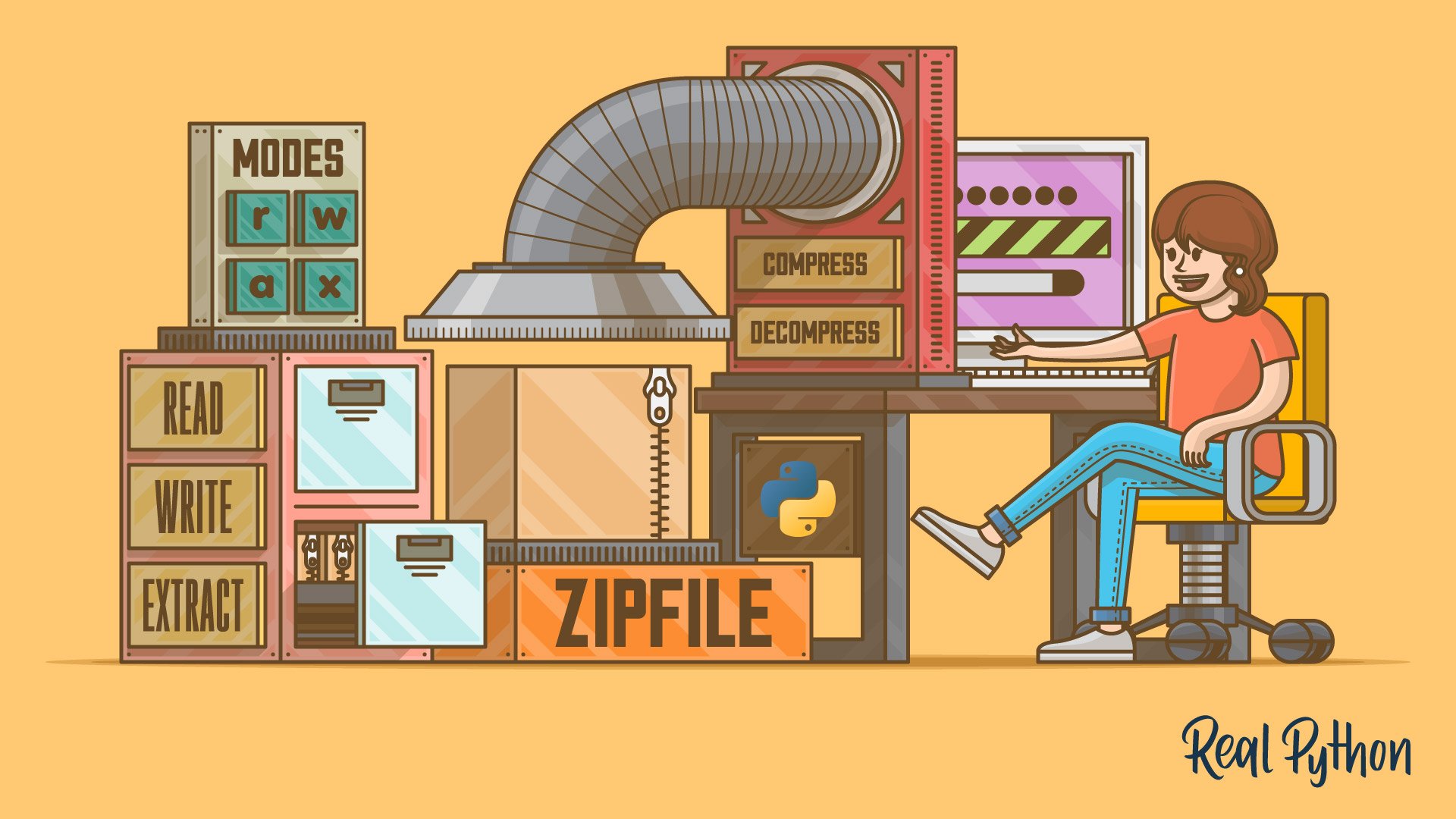

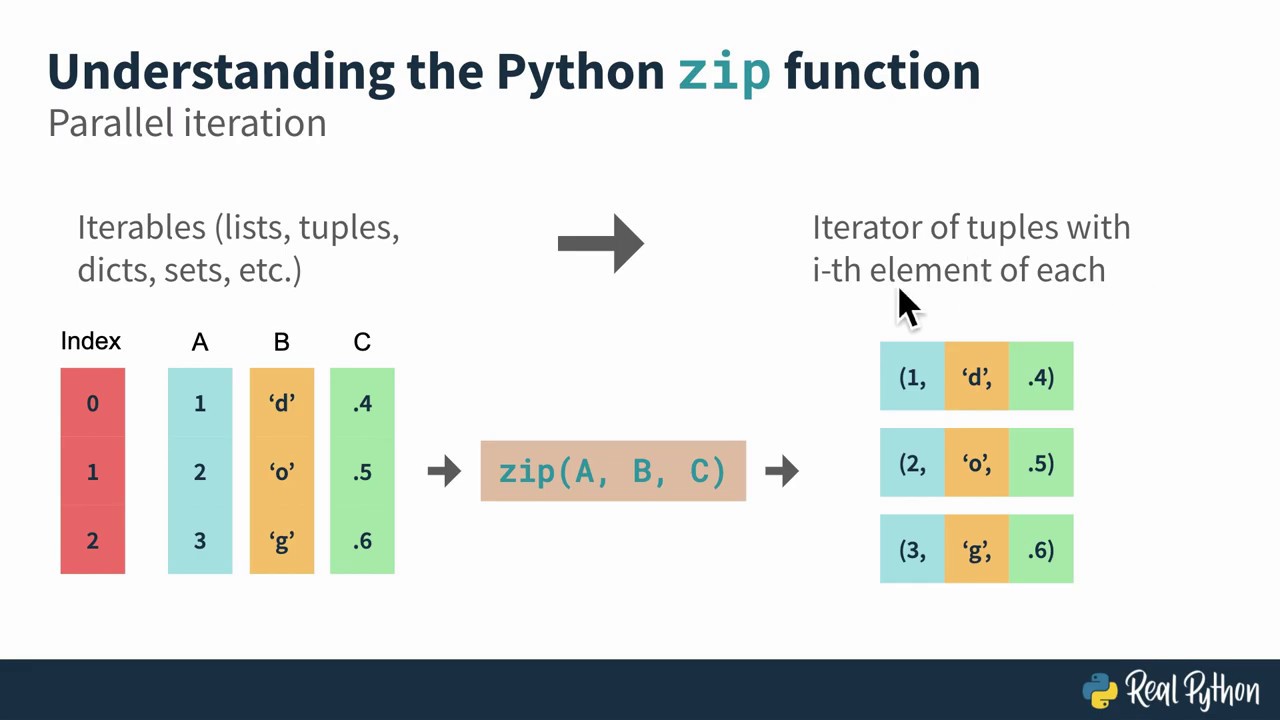
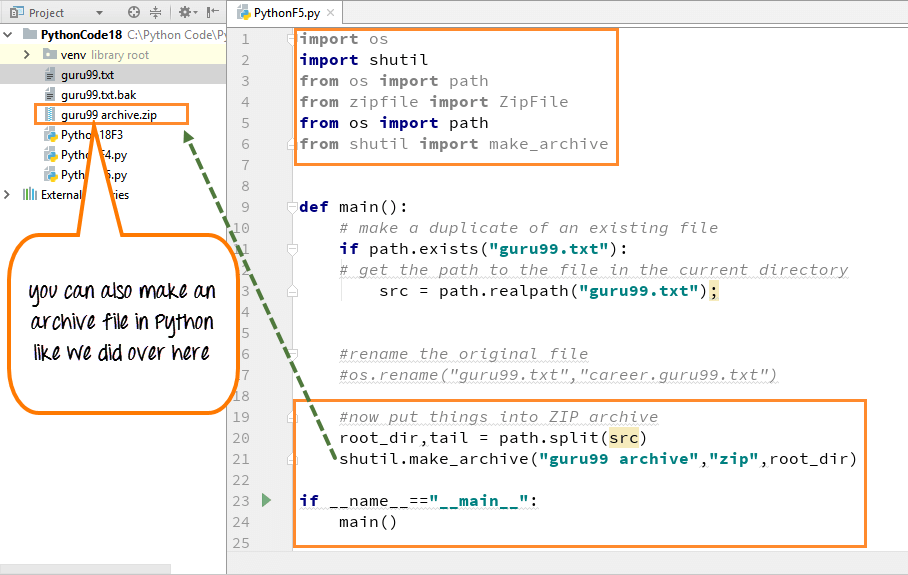


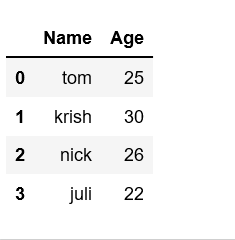
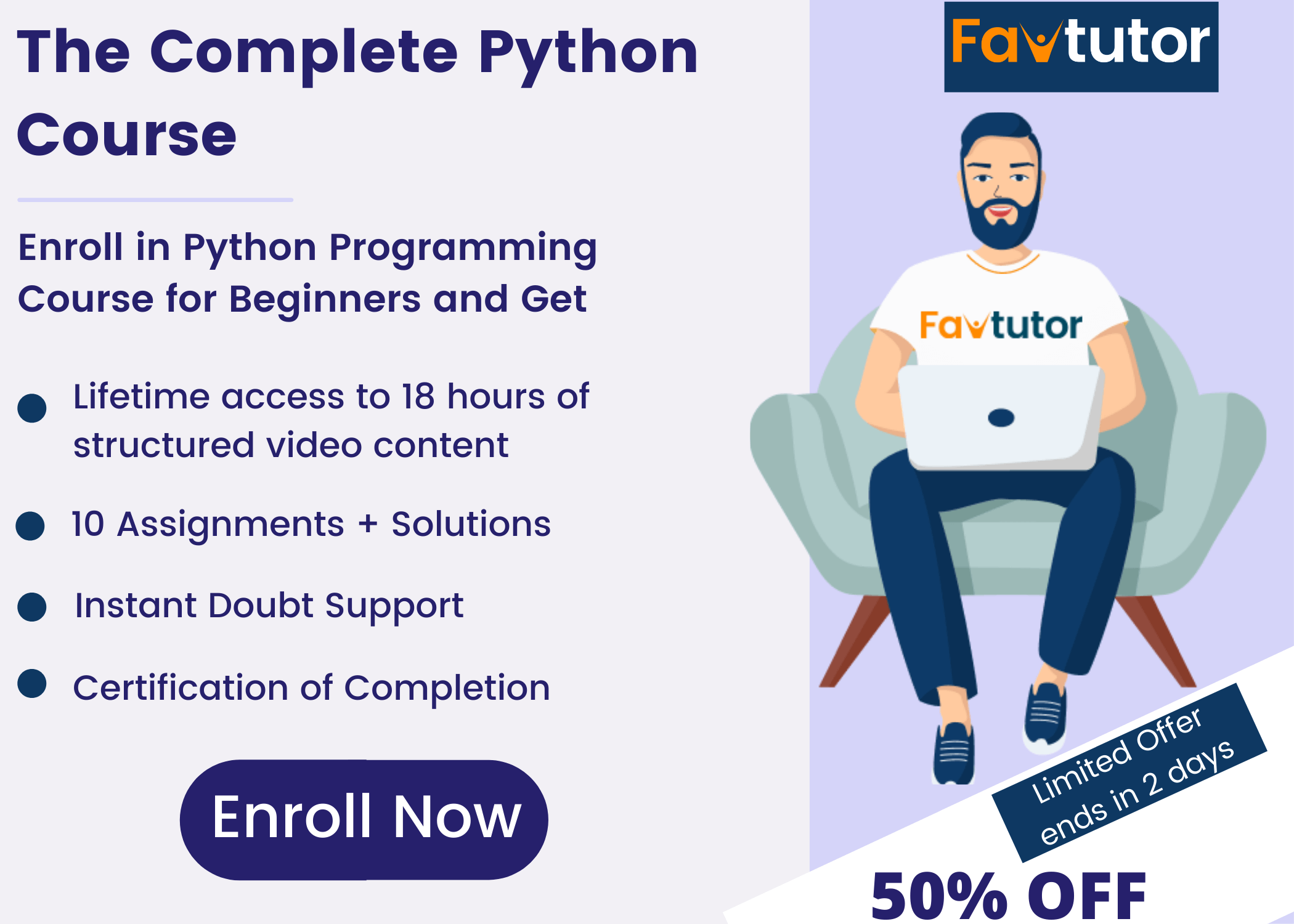

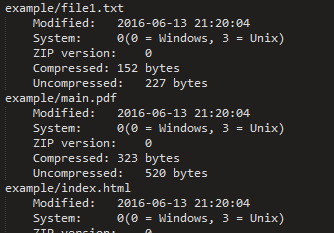
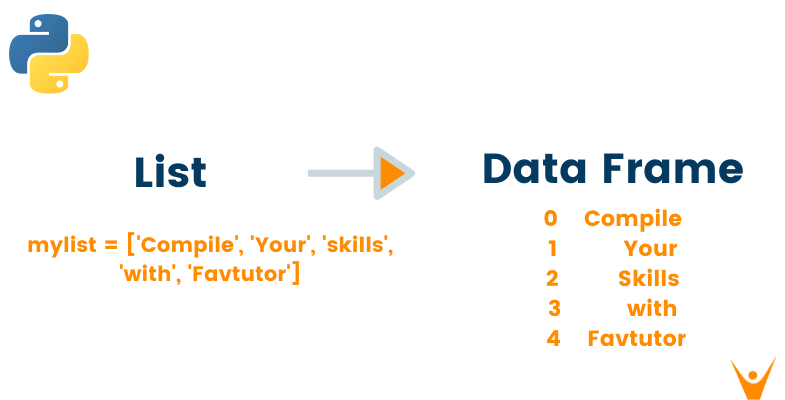



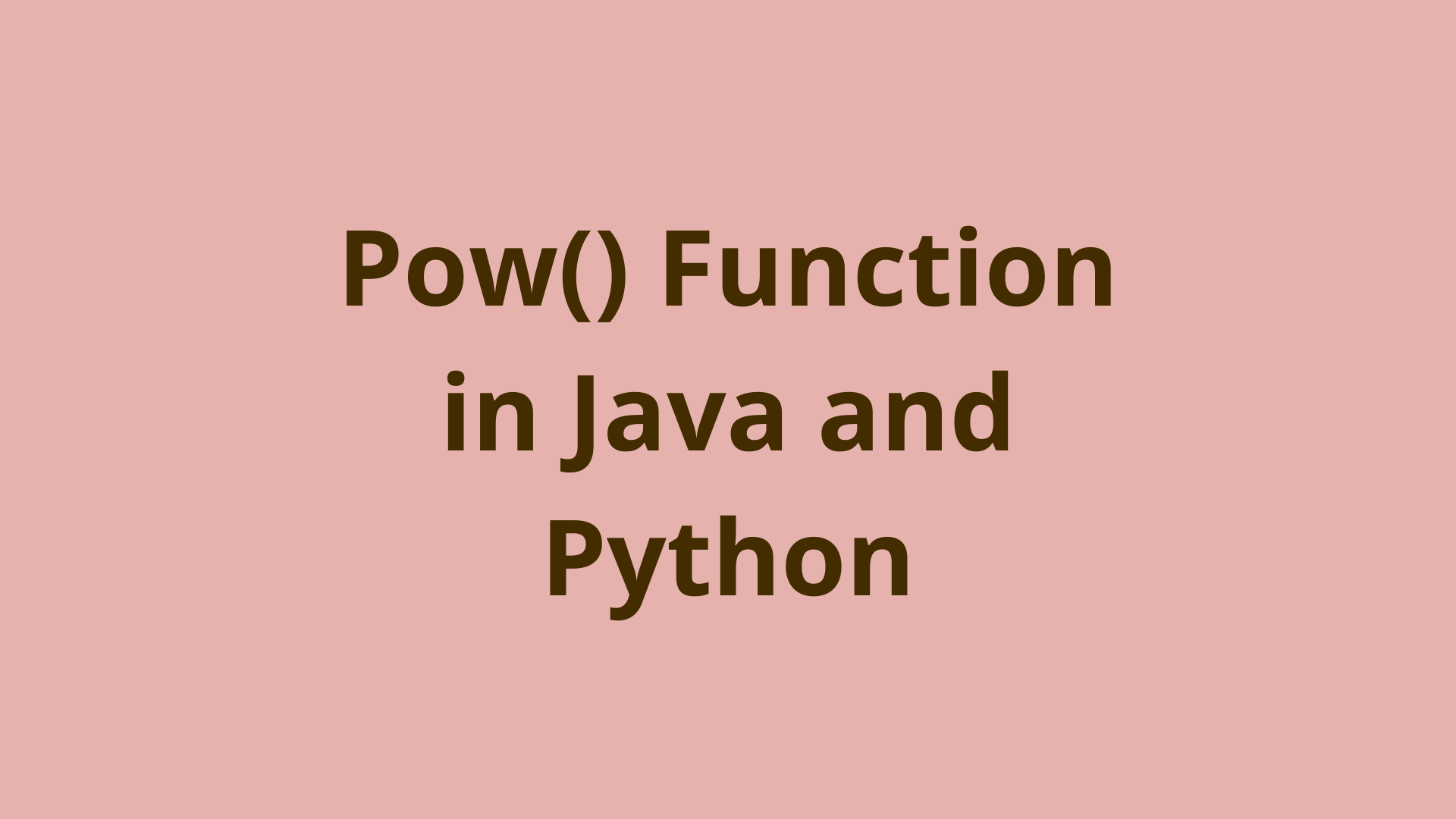
Article link: python zip two lists.
Learn more about the topic python zip two lists.
- Zip Two Lists in Python Using 3 Methods – FavTutor
- Python zip() Two Lists with Examples
- How to zip two lists in Python – Adam Smith
- How to Zip two lists of lists in Python? – GeeksforGeeks
- Zip Two or More Lists in Python – Datagy
- How to combine the elements of two lists using zip function in …
- Zip Two Lists in Python Using 3 Methods – FavTutor
- Python zip() function – ThePythonGuru.com
- Merge Two Lists in Python – Scaler Topics
- Using the Python zip() Function for Parallel Iteration
- zip() in Python: Get elements from multiple lists – nkmk note
- Zip Lists in Python – Delft Stack
- How to Zip Two Lists in Python with Ease – Kanaries Docs
See more: https://nhanvietluanvan.com/luat-hoc