Python Write Line To File
Opening/Definition of “Python Write Line to File”:
Python, an open-source, high-level programming language, offers a wide range of file handling capabilities. Among these capabilities is the ability to write lines to a file. Writing lines to a file involves opening a file in write mode, writing the desired content to the file, and then closing the file. This article will explore Python’s file handling features and provide a step-by-step guide on how to write lines to a file using Python. Additionally, it will address frequently asked questions regarding this topic.
Overview of Python’s File Handling Capabilities:
Python provides a robust and intuitive way to handle files. With its built-in functions and methods, developers can easily read, write, and manipulate files to store and retrieve data. Python supports various file formats, including text files, binary files, CSV files, JSON files, and more. Additionally, Python allows for seamless integration with external tools and libraries to facilitate complex file operations.
Understanding File Objects in Python:
In Python, file operations are performed using file objects. A file object represents a specific file and provides methods and attributes to interact with the file’s content. To perform file operations, such as reading or writing data, a file object must be created. Python offers a built-in function called `open()` to create a file object for a specified file.
Creating a File Object in Python:
To create a file object in Python, the `open()` function is used. The function takes two arguments: the file name (including the path) and the mode in which the file should be opened. The mode can be set to read, write, append, or a combination of these modes. For the purpose of writing lines to a file, we will focus on the write mode.
Opening a File in Write Mode:
To open a file in write mode, the mode argument passed to the `open()` function should be set as `”w”`. This mode will create a new file if it doesn’t exist or overwrite the existing file. If the file already contains data, opening it in write mode will erase all its contents. Here’s an example of how to open a file in write mode:
“`
file = open(“example.txt”, “w”)
“`
Writing a Single Line to a File in Python:
Once the file is opened in write mode, we can use the file object’s `write()` method to write content to the file. To write a single line to a file, we use the `write()` method followed by the desired line of text. It’s important to note that the `write()` method does not automatically add newline characters, so they need to be explicitly added if desired. Here’s an example of writing a single line to a file:
“`
file.write(“This is a single line”)
“`
Writing Multiple Lines to a File in Python:
To write multiple lines to a file, we can utilize the `write()` method in combination with newline characters. By adding a newline character (`”\n”`) at the end of each line, we can separate them. Here’s an example of writing multiple lines to a file:
“`
file.write(“Line 1\n”)
file.write(“Line 2\n”)
file.write(“Line 3\n”)
“`
Appending Lines to a File in Python:
In some cases, we may want to add new lines of text to an existing file, rather than overwriting its contents entirely. Python provides an append mode (`”a”`) that allows us to open a file for writing without deleting the existing content. Here’s an example of how to open a file in append mode:
“`
file = open(“example.txt”, “a”)
“`
Closing a File Object in Python:
After we have finished writing to a file, it is essential to close the file object. Closing a file saves any changes made to the file and releases resources associated with it. To close a file, we can use the `close()` method of the file object. Here’s an example:
“`
file.close()
“`
Python Write Line to File – FAQs:
Q: Can I use Python to replace a specific line in a text file?
A: Yes, Python provides a way to replace a specific line in a text file. You can open the file in read mode, read its contents into memory, make the desired changes, and then write back the modified content to the file.
Q: How can I write a list of lines to a file in Python?
A: To write a list of lines to a file, you can iterate over the list and use the `write()` method to write each line to the file, separating them with newline characters.
Q: Can I write an array to a file in Python?
A: Yes, you can write an array to a file in Python. Before writing, you may need to convert the array to a suitable format, such as a string or a binary representation.
Q: Are there any differences in writing to a file between Python 2 and Python 3?
A: Python 2 and Python 3 have some differences in file handling. The most notable difference is that Python 3 requires opening files in text mode explicitly using the `’t’` flag, whereas Python 2 treats files as text by default.
In conclusion, Python offers powerful file handling capabilities, including the ability to write lines to a file. By understanding file objects, opening files in write mode, and utilizing the write methods, developers can easily accomplish this task. Whether it’s writing a single line or multiple lines, Python provides an intuitive and flexible approach to manage file operations efficiently.
Writing To Files | Python | Tutorial 29
Keywords searched by users: python write line to file Python write file, Replace line text file python, Python write to file multiple lines, Write list to file Python, Python3 write to file, Python write to txt file, 3. write a list of lines to a file, Write array to file Python
Categories: Top 39 Python Write Line To File
See more here: nhanvietluanvan.com
Python Write File
Python, with its simple yet powerful syntax, has become one of the most popular programming languages for a variety of applications. In this article, we will explore the concept of writing files in Python and delve into the intricacies of this essential functionality. Whether you’re a beginner seeking to learn the basics or an experienced programmer aiming to enhance your skills, this comprehensive guide will answer all your questions about file writing in Python.
Understanding File Writing in Python:
File writing is a fundamental aspect of programming, allowing us to store data persistently for future use. In Python, writing to a file involves a straightforward process. Here’s a step-by-step breakdown:
1. Opening a File:
Before writing to a file, it must be opened in the appropriate mode. Python provides several modes, such as read (‘r’), write (‘w’), append (‘a’), and more. The ‘w’ mode is commonly used for writing new files or overwriting existing ones. To open a file in write mode, we use the built-in `open()` function, specifying the file name and the desired mode.
2. Writing to the File:
Once the file is opened in write mode, we can start writing data to it. Python offers various ways to write content, depending on the nature of the data. We can use the `write()` method to write a string, the `writelines()` method to write a list of strings, or even the `print()` function with the `file` parameter to send output directly to the file.
3. Closing the File:
To ensure data integrity and avoid resource leaks, it is crucial to close the file after writing. Python provides the `close()` method to release the file handle. Although Python automatically closes the file when the program terminates, explicitly closing it is a best practice that should be followed consistently.
Writing to a File: Examples and Best Practices:
Let’s explore some practical examples to demonstrate file writing in Python:
Example 1: Writing a String to a File
“`python
file = open(‘example.txt’, ‘w’)
file.write(‘Hello, World!’)
file.close()
“`
In this example, we open a file named `’example.txt’` in write mode, write the string `’Hello, World!’`, and then close the file. If the specified file doesn’t exist, Python will create it. If it already exists, the previous content will be overwritten.
Example 2: Writing a List of Strings to a File
“`python
lines = [‘Line 1\n’, ‘Line 2\n’, ‘Line 3\n’]
file = open(‘example.txt’, ‘w’)
file.writelines(lines)
file.close()
“`
In this case, we define a list of strings called `lines`, which contains three lines of text. We open the file in write mode, use the `writelines()` method to write each line in the list to the file, and finally close it.
FAQs about Python File Writing:
1. How can I write numeric data to a file?
To write numeric data to a file, you need to convert it into a string representation using functions like `str()` or by employing formatted string literals (`f-strings`).
2. Can I write data to a specific location in the file?
No, file writing in Python is a sequential operation. You cannot directly write data to a specific location in a file. However, you can read the content, modify it, and then write the modified data back if needed.
3. How can I append content to an existing file without overwriting it?
To append content to an existing file without overwriting it, open the file in append mode (‘a’) instead of write mode (‘w’). This mode ensures that new content will be added at the end of the existing file.
4. Is it necessary to include the close() method after writing to a file?
Although Python automatically closes the file when the program ends, it is good practice to explicitly close the file using the `close()` method after writing. This helps prevent resource leaks and ensures data integrity.
5. How can I handle errors while writing to a file?
Writing to a file can lead to various errors, such as insufficient disk space or file permissions. To handle such errors, use exception handling techniques, such as `try-except` blocks, around the file writing code and take appropriate actions in case of exceptions.
Conclusion:
In this article, we’ve explored the Python file writing process in depth. We learned how to open a file in write mode, write content to it, and close the file properly. We also discussed practical examples and highlighted best practices. By now, you should have a solid understanding of Python file writing and be ready to harness this essential functionality for your own projects. Happy coding!
Replace Line Text File Python
Python, being a versatile programming language, provides numerous functions to manipulate files. One common task is replacing specific lines of a text file with new text. In this article, we will explore various techniques to achieve this goal using Python. Whether you are a beginner or an experienced developer, this guide will equip you with the knowledge required to efficiently replace lines in text files.
Table of Contents:
1. Introduction: Understanding the Problem
2. Working with Text Files in Python
3. Replacing Lines in a Text File
3.1. Method 1: Reading and Writing to a New File
3.2. Method 2: Modifying the Original File
4. Frequently Asked Questions (FAQs)
5. Conclusion
1. Introduction: Understanding the Problem
The task of replacing lines in a text file involves identifying a specific line(s) within the file and replacing it with new content. This could be useful in situations where you need to update a configuration file or modify specific lines in a log file.
2. Working with Text Files in Python
Before diving into the specific techniques, let us briefly discuss how to open, read, and write to a text file using Python.
To open a text file, we use the `open()` function, which accepts the file name and mode as parameters. The mode can be “r” for reading, “w” for writing, or “a” for appending to an existing file.
“`
file = open(“example.txt”, “r”)
“`
To read the contents of a text file, we can use various methods like `read()`, `readline()`, or `readlines()`. For instance, `readlines()` reads the entire file and returns a list of strings, where each string represents a line in the file.
“`
content = file.readlines()
“`
To write to a file, we need to open it in write mode (“w”) and use the `write()` method to write content. It is important to note that opening a file in “w” mode will overwrite its existing contents. To append content to an existing file, we use the append mode (“a”).
“`
file = open(“example.txt”, “w”)
file.write(“Hello, World!”)
“`
Once we are familiar with reading and writing to a text file, we can proceed to replace lines in a file.
3. Replacing Lines in a Text File
We will explore two methods to replace lines in a text file:
1. Reading and writing to a new file
2. Modifying the original file directly
3.1 Method 1: Reading and Writing to a New File
In this method, we read the entire content of the original file, modify the desired line(s), and write the updated content to a new file. This approach ensures that the original file remains intact, while allowing us to make changes.
The steps to follow are:
Step 1: Open the original file in read mode.
Step 2: Read the file content and identify the line(s) to be replaced.
Step 3: Modify the desired line(s) using string manipulations or regex.
Step 4: Open a new file in write mode.
Step 5: Write the modified content to the new file.
Step 6: Close both files and optionally delete or rename them.
The advantage of this approach is that it preserves the original file and prevents accidental data loss. However, it requires additional operations and storage space to create a new file.
3.2 Method 2: Modifying the Original File
In this method, we directly modify the original file to replace the desired lines. This approach reduces the complexity of creating a new file but comes with a higher risk of losing data if not implemented correctly.
The steps to follow are:
Step 1: Open the original file in read mode.
Step 2: Read the file content and identify the line(s) to be replaced.
Step 3: Modify the desired line(s) using string manipulations or regex.
Step 4: Open the file again in write mode to overwrite the original content.
Step 5: Write the modified content to the file.
Step 6: Close the file.
The advantage of this approach is its simplicity and avoiding the need for creating a new file. However, it is crucial to take appropriate precautions, such as creating a backup of the original file, to safeguard against data loss.
4. Frequently Asked Questions (FAQs)
Q1: Can I replace multiple lines simultaneously?
A1: Yes, you can replace multiple lines simultaneously using either of the methods described. By iterating through the lines, identifying the desired lines, and modifying them, you can replace multiple lines in a single operation.
Q2: How can I replace lines based on specific criteria, such as a condition or pattern?
A2: Python provides comprehensive support for regular expressions using the ‘re’ module. By leveraging regex, you can identify lines based on specific patterns and replace them accordingly.
Q3: What precautions should I take when modifying the original file directly?
A3: It is strongly recommended to create a backup of the original file before modifying it directly. This precaution ensures that you have a copy of the original data in case of accidental data loss.
Q4: Are there any libraries that simplify the process of replacing lines in a text file?
A4: Yes, Python provides several external libraries like ‘fileinput’ and ‘sed’ that simplify the process of replacing lines in a text file. These libraries handle operations such as reading, writing, and replacing lines, thus simplifying the implementation.
5. Conclusion
Manipulating text files is a common task in many programming projects. With the techniques discussed in this article, you are now equipped to efficiently replace specific lines in a text file using Python. Whether you choose to create a new file or modify the original file directly, understanding the risks and taking appropriate precautions is vital. By leveraging Python’s file handling capabilities and string manipulation techniques, you can easily achieve your goal of replacing lines in a text file.
Python Write To File Multiple Lines
To begin, let’s review the basic concept of writing to a file in Python. Writing to a file allows us to store data for future use or share it with others. Python offers a straightforward way to achieve this using the built-in `open()` function, which opens a file and returns a file object that can be used to read, write, or manipulate the file as needed.
When it comes to writing multiple lines to a file, there are several approaches we can take, each with its own advantages and use cases. Let’s delve into three commonly used methods:
Method 1: Using the `writelines()` method with a list
—————————————————–
The `writelines()` method is a convenient way to write multiple lines to a file. It takes a sequence of strings as input and writes them to the file without adding any additional characters between each line. Here’s an example that demonstrates how to use this method:
“`python
lines = [“First line”, “Second line”, “Third line”]
with open(“output.txt”, “w”) as file:
file.writelines(lines)
“`
In this example, we create a list called `lines` containing three strings. We then open the file `output.txt` in write mode (“w”) using a `with` statement, ensuring that the file is properly closed after writing. Finally, we call `writelines()` on the file object, passing in the `lines` list as an argument. This method writes each line in the list to the file, resulting in a file with three lines.
Method 2: Using the `print()` function with a file argument
———————————————————-
Another approach to write multiple lines to a file is by utilizing the `print()` function’s file argument. By specifying the `file` parameter, we can redirect the output to a file instead of the standard output (i.e., the console). Here’s an example:
“`python
with open(“output.txt”, “w”) as file:
print(“First line”, file=file)
print(“Second line”, file=file)
print(“Third line”, file=file)
“`
In this method, we open the file `”output.txt”` in write mode using a `with` statement. Then, we use the `print()` function with the `file` argument to write each line of text to the file directly. This approach offers more flexibility as we can format the lines of text using the full capabilities of the `print()` function, including string formatting, separators, and end characters.
Method 3: Using a loop to write multiple lines
———————————————
If you have a large number of lines or want to dynamically generate the lines based on a specific pattern or input, you can use a loop to write the lines one by one. Here’s an example that shows how to accomplish this:
“`python
lines = [“First line”, “Second line”, “Third line”]
with open(“output.txt”, “w”) as file:
for line in lines:
file.write(line + “\n”)
“`
In this example, we iterate over each line in the `lines` list and use the `write()` method to write each line to the file. We append the newline character `”\n”` at the end of each line to ensure that each line is written on a separate line in the file.
FAQs:
——
Q1: Can I append lines to an existing file instead of creating a new one?
A1: Yes, you can open a file in append mode (“a”) instead of write mode (“w”) to append lines to an existing file. Simply change the mode parameter in the `open()` function accordingly.
Q2: How can I write data other than strings to a file?
A2: The methods mentioned above work with strings. To write data of a different type, like numbers or objects, you need to convert them to strings first using appropriate functions like `str()` or formatting techniques like f-strings or the `format()` method.
Q3: How can I handle errors while writing to a file?
A3: When working with file operations, it’s important to handle possible errors. Wrapping your code in a `try-except` block allows you to catch and handle exceptions gracefully, ensuring that your program doesn’t crash unexpectedly.
In conclusion, Python provides multiple options for writing multiple lines to a file, depending on the specific requirements of your project. Whether you prefer using the `writelines()` method, the `print()` function, or a loop, understanding these techniques will empower you to effectively write and manage data in files using Python.
Images related to the topic python write line to file
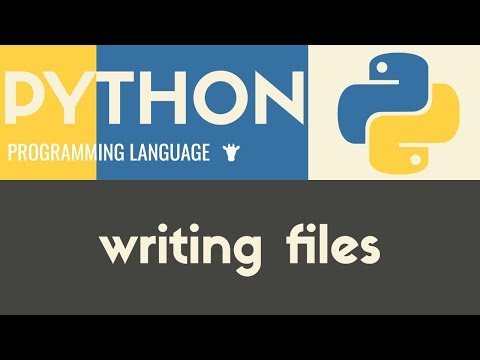
Found 8 images related to python write line to file theme
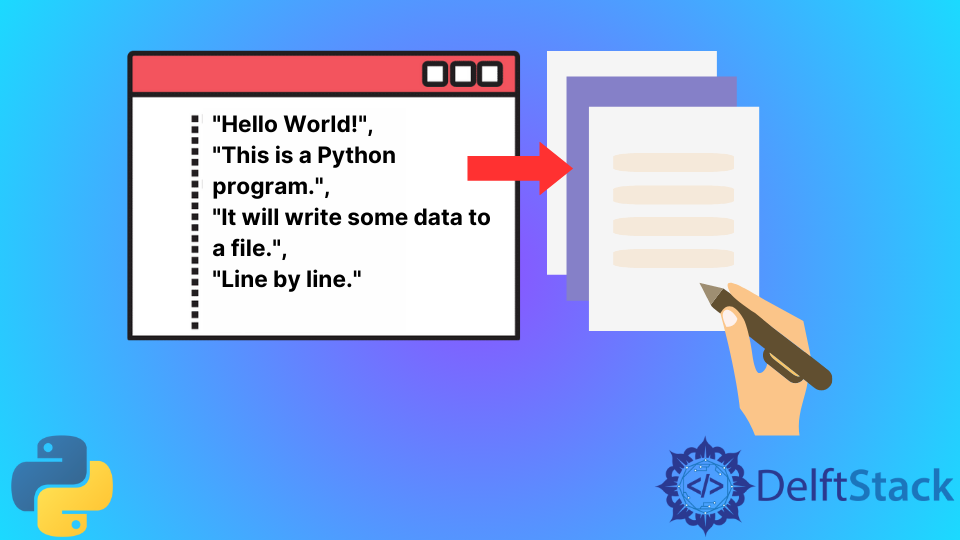
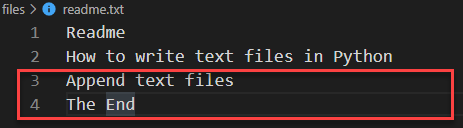
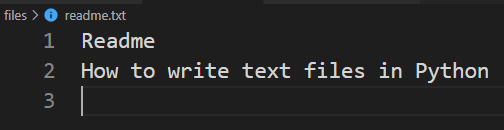
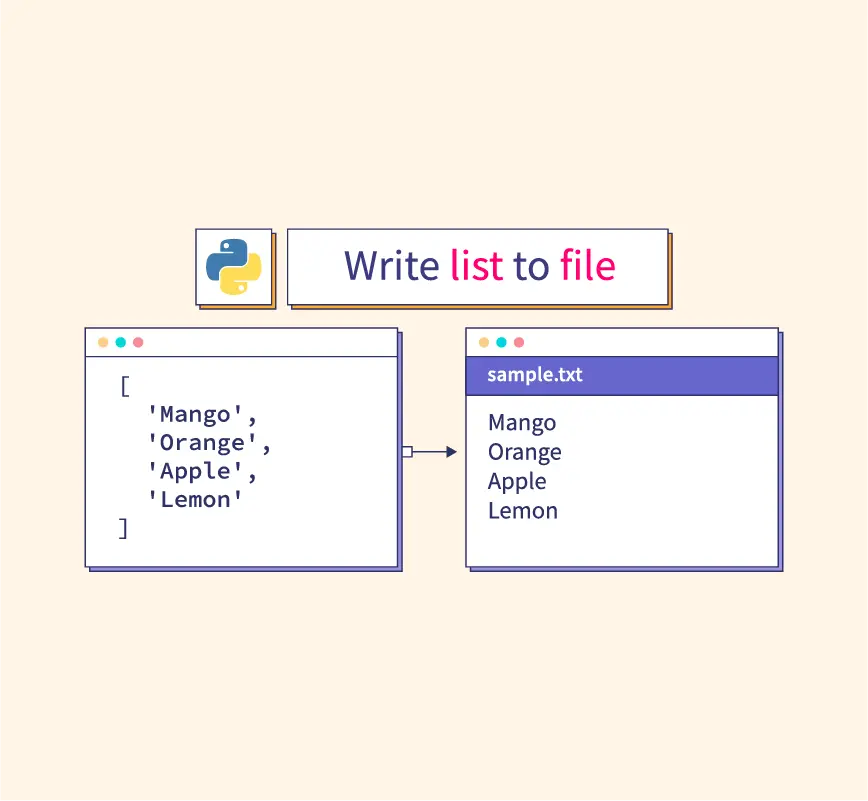
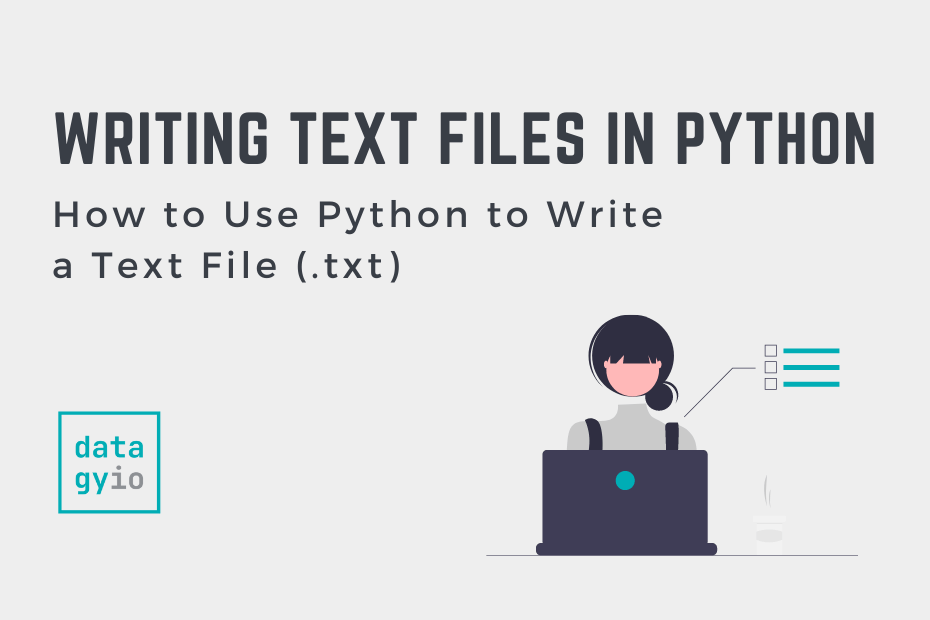

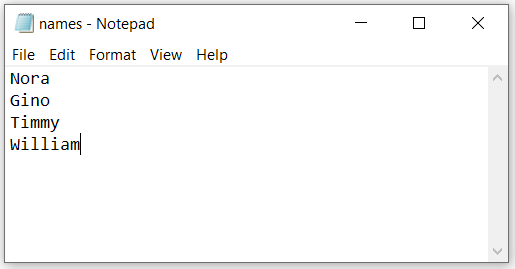
![File Handling in Python [Complete Series] – PYnative File Handling In Python [Complete Series] – Pynative](https://pynative.com/wp-content/uploads/2021/07/file_handling_in_python.png)
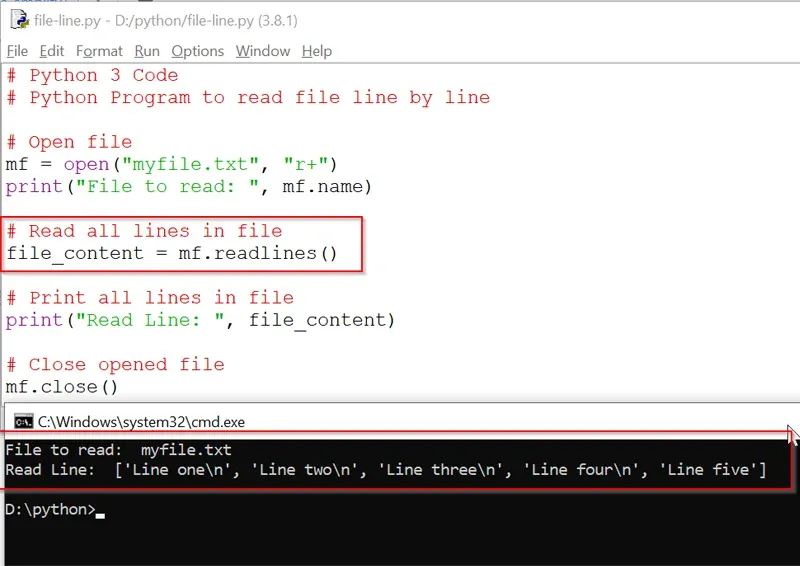
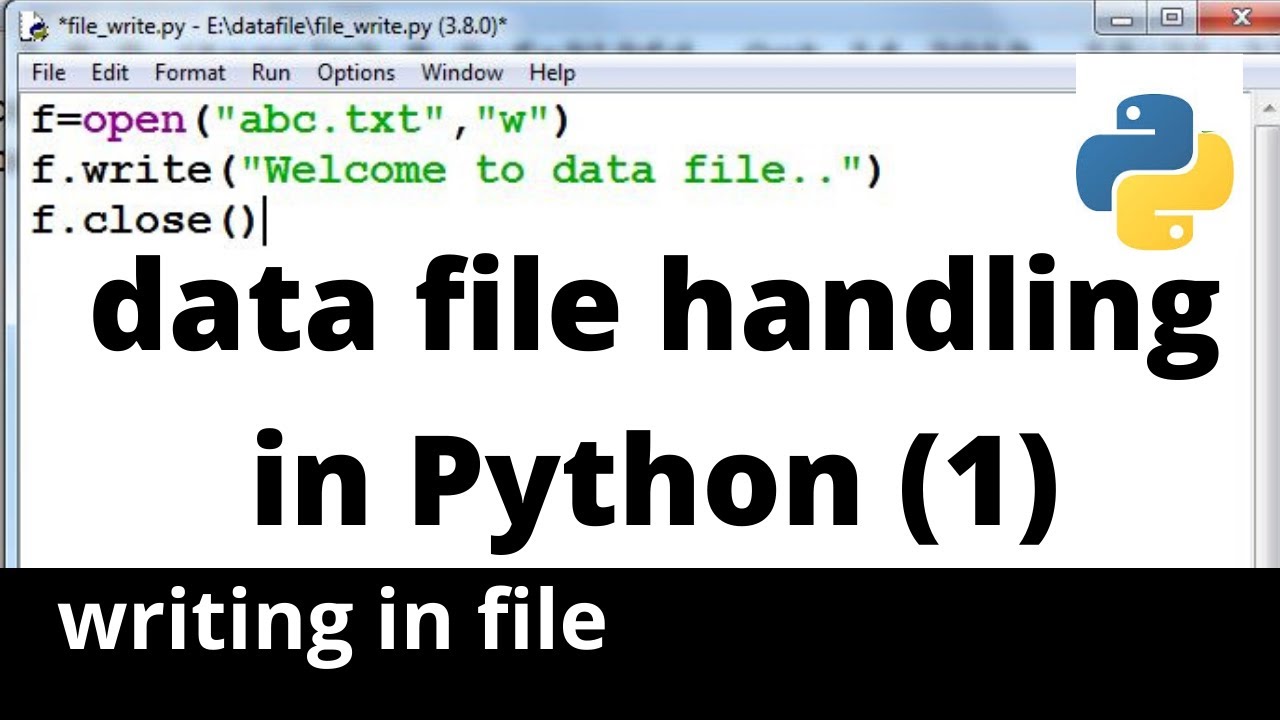
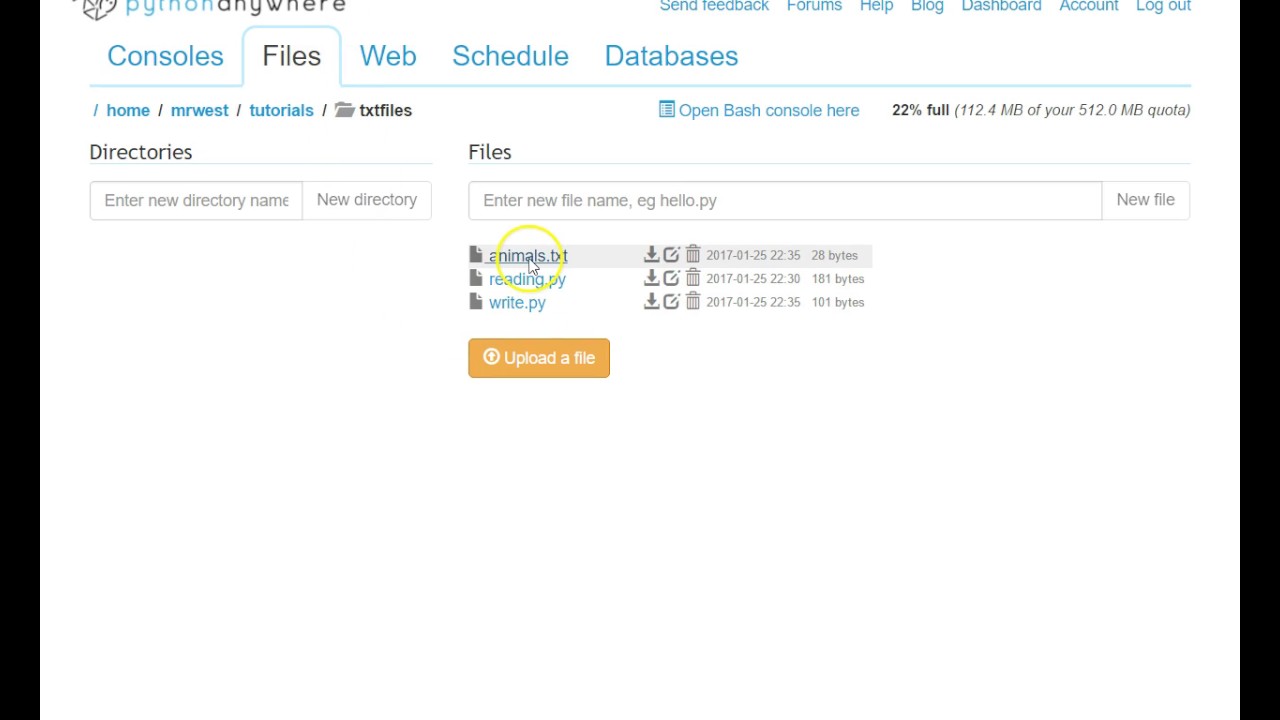
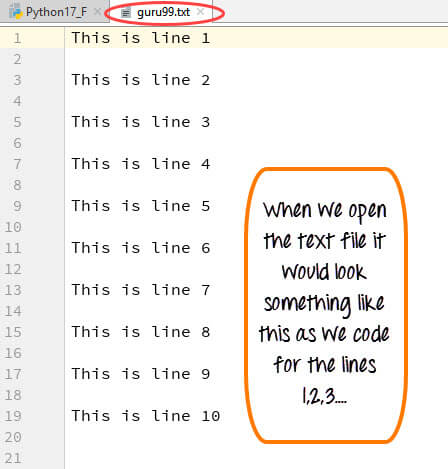
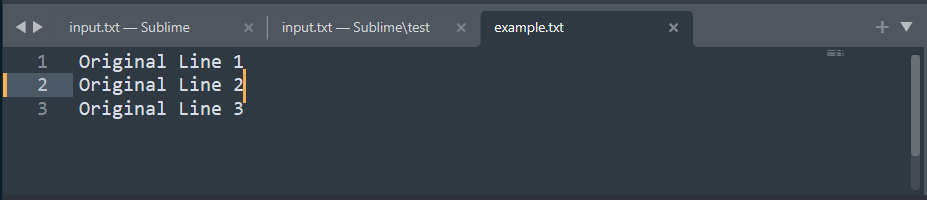
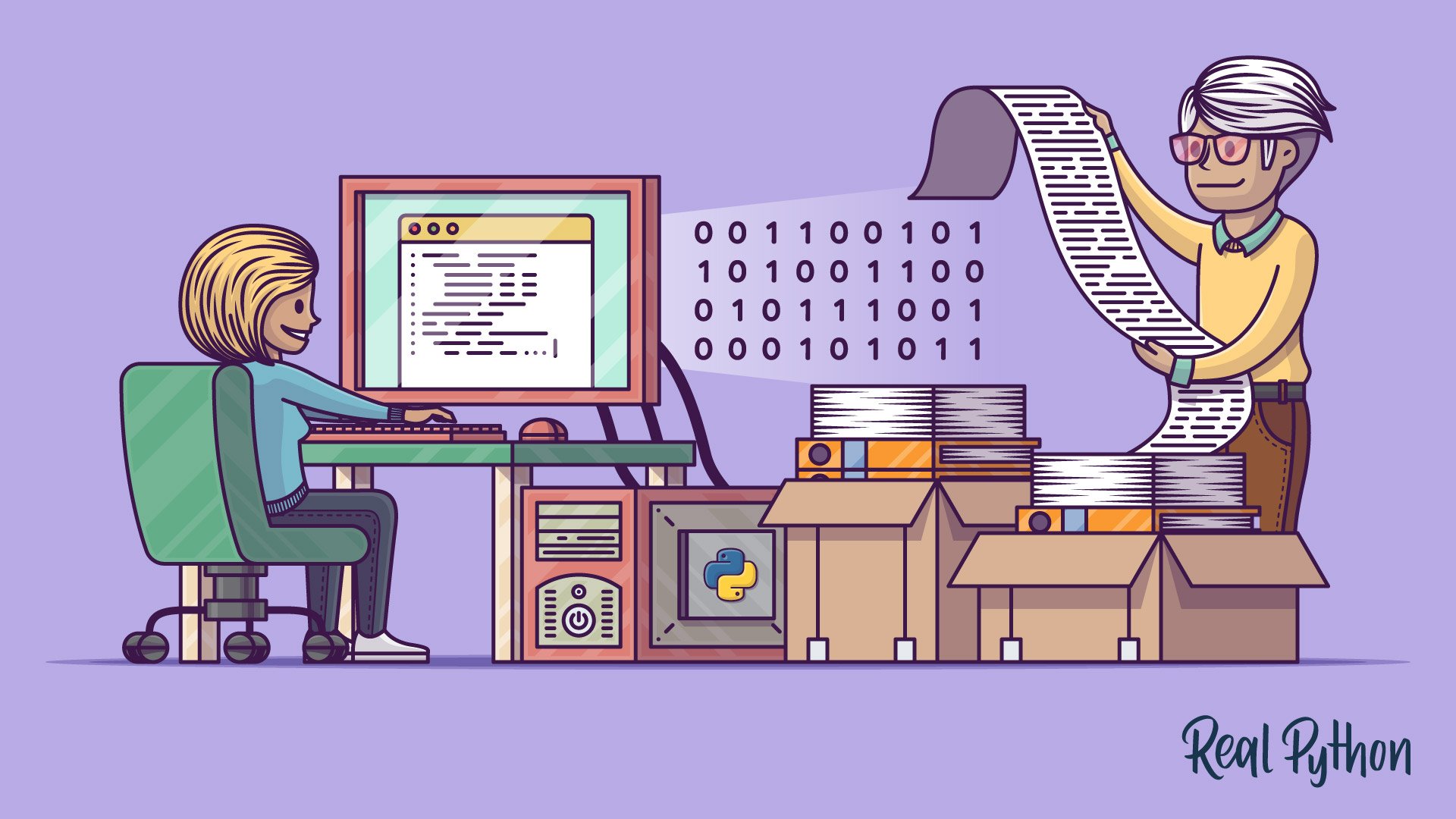
![Writeline() add empty line at end of file [Python] - Stack Overflow Writeline() Add Empty Line At End Of File [Python] - Stack Overflow](https://i.stack.imgur.com/ETAvB.png)
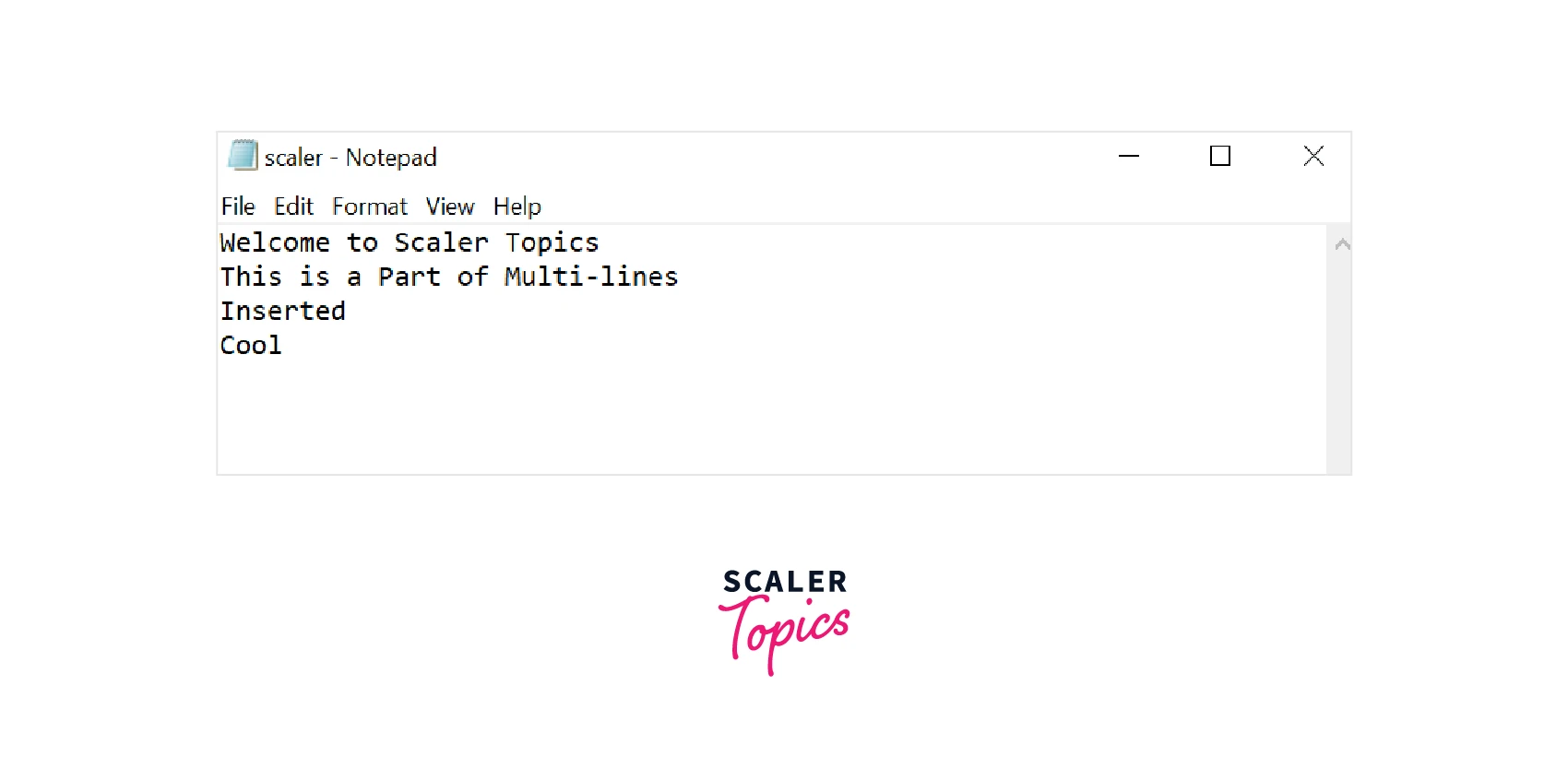
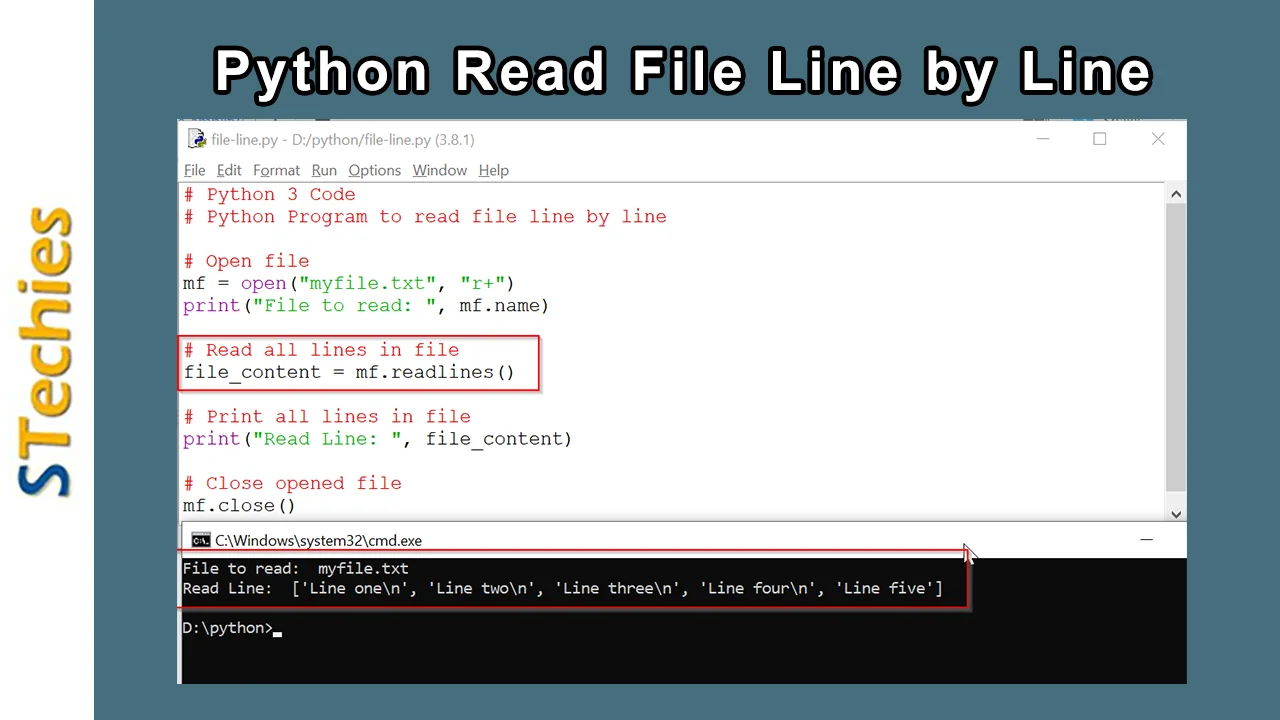
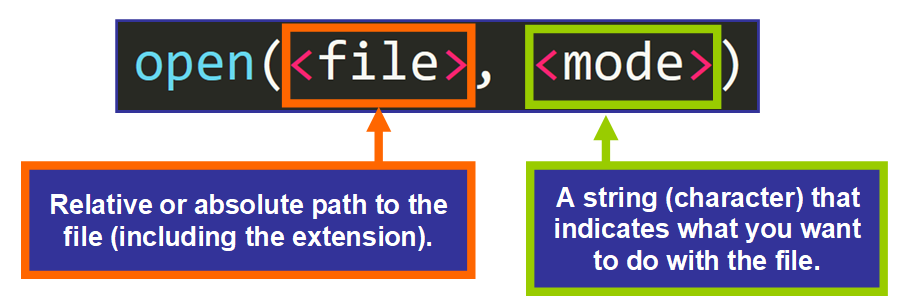
![Python Writing List to a File [5 Ways] – PYnative Python Writing List To A File [5 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/12/file_after_writing_python_list_into_it.png)
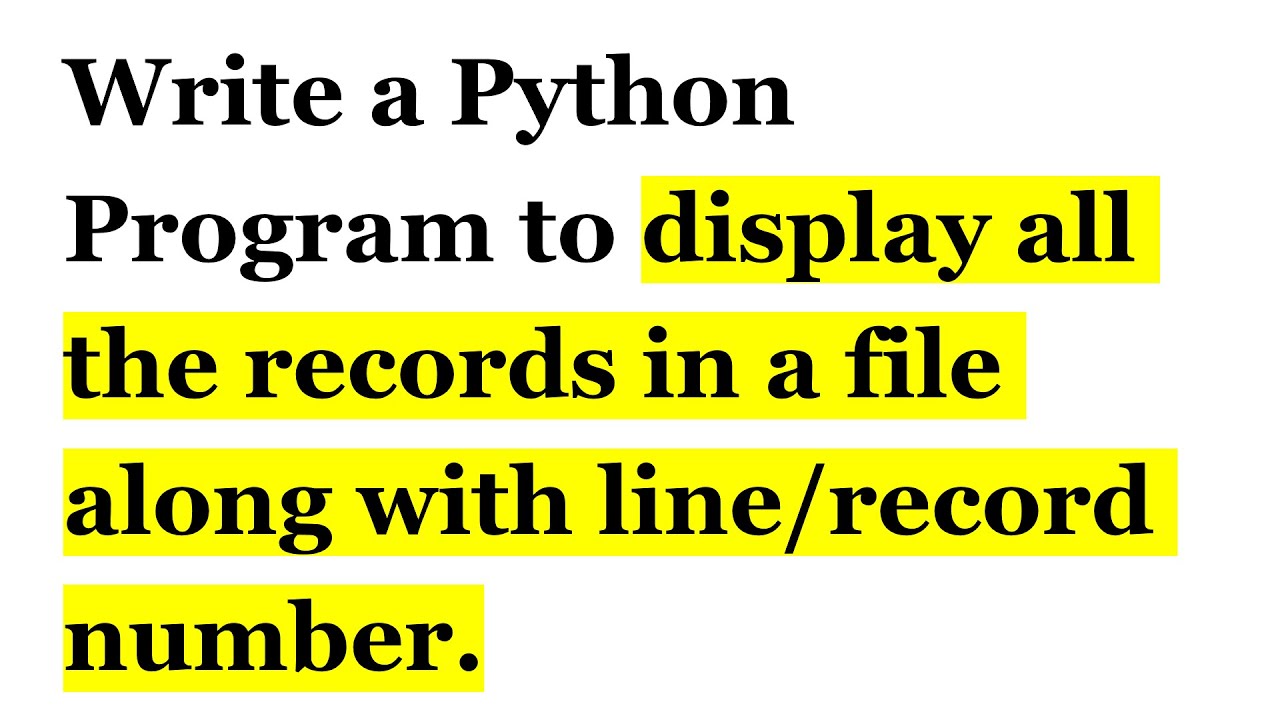
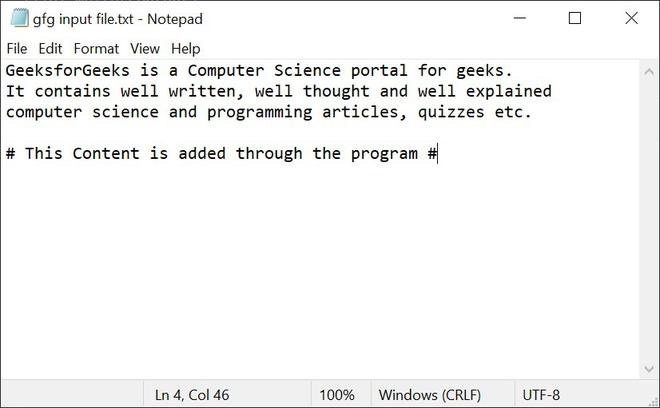

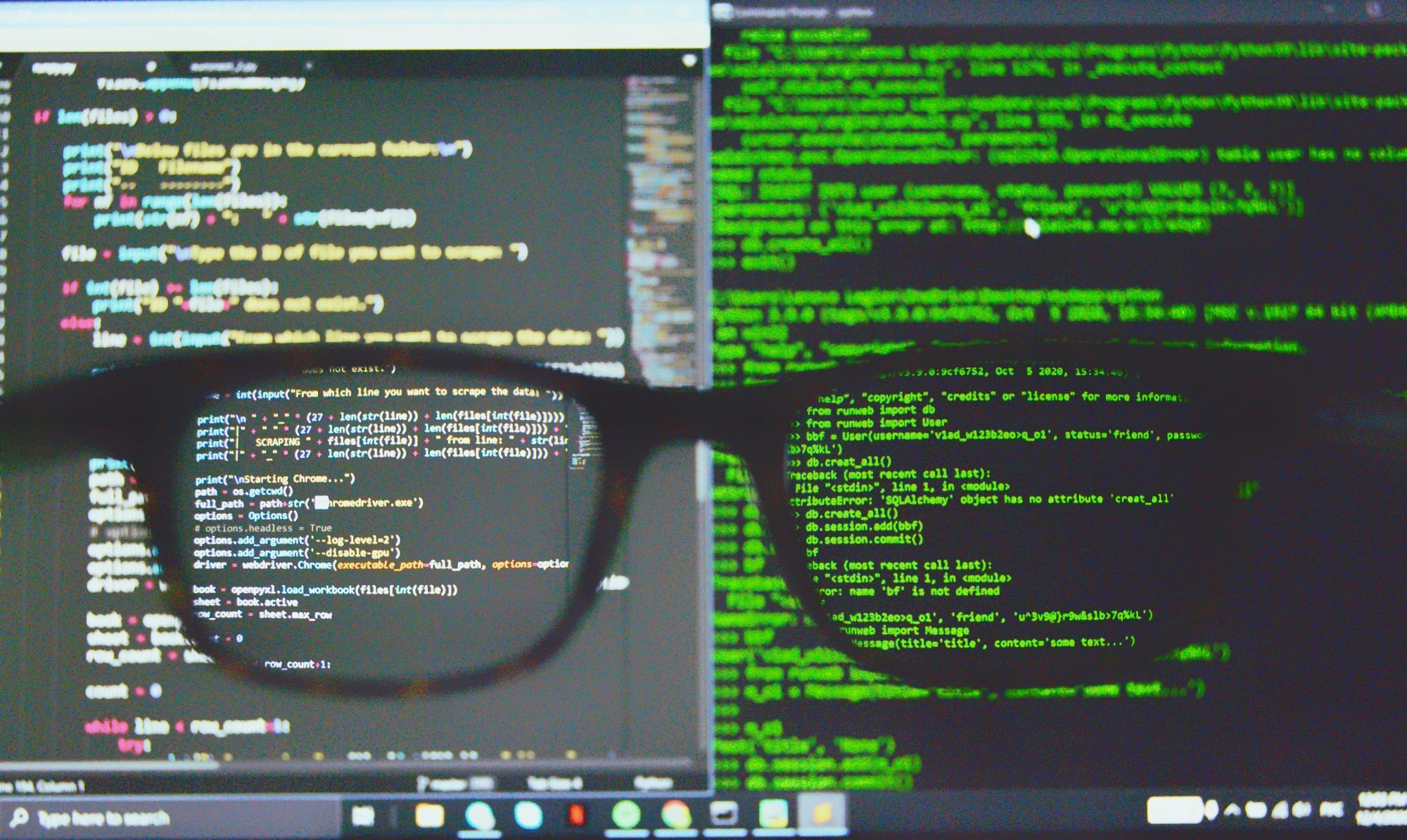
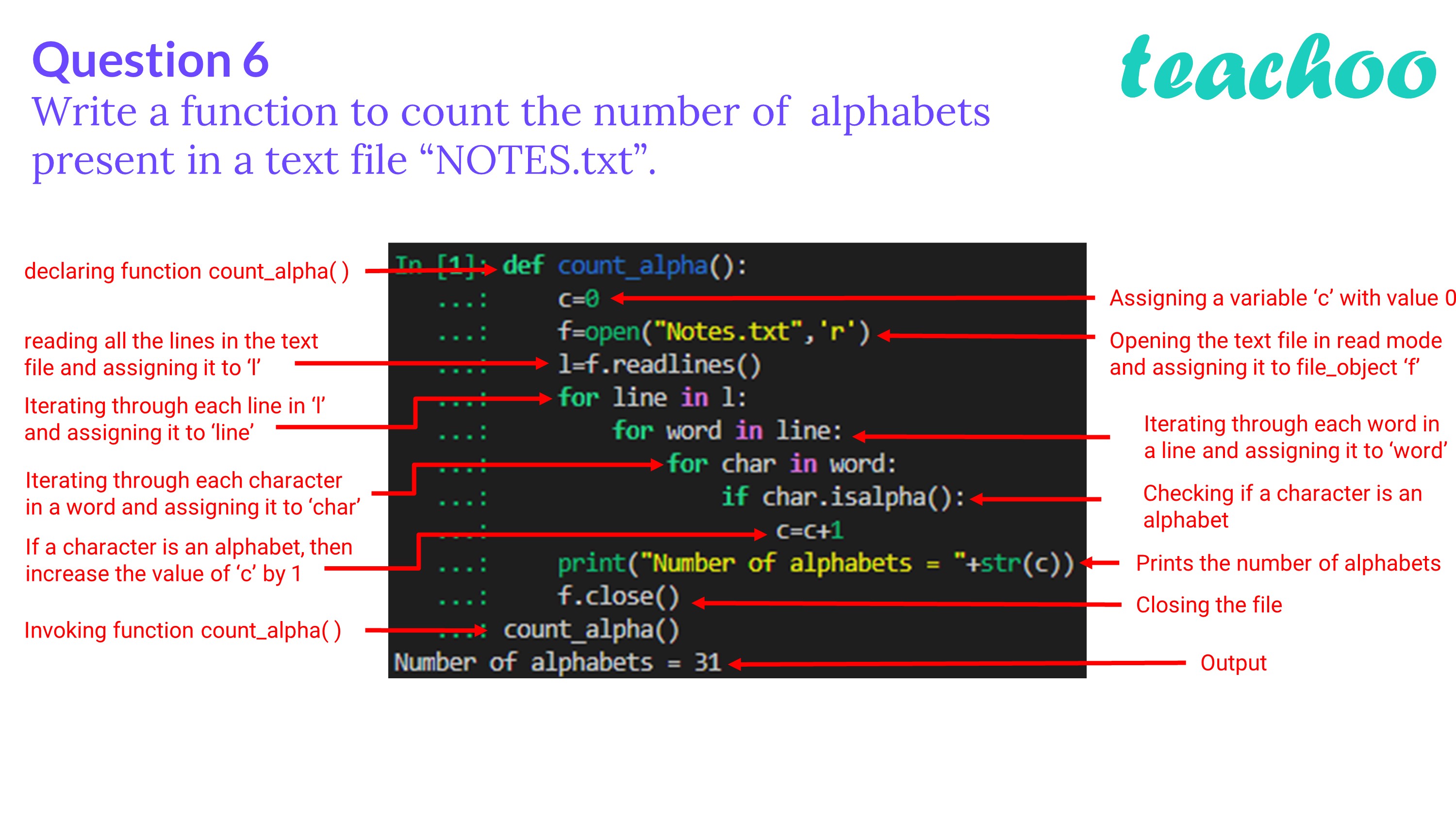
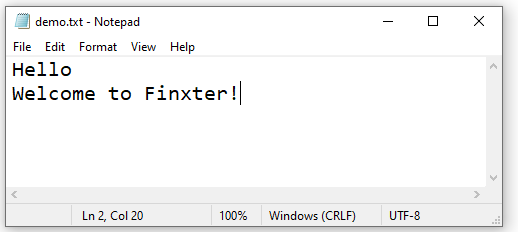



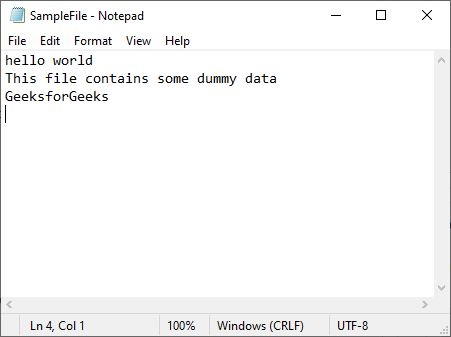
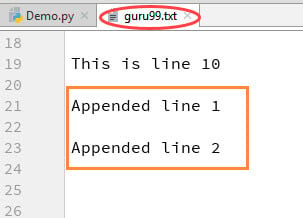

![Python Writing List to a File [5 Ways] – PYnative Python Writing List To A File [5 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/12/file_after_writing_python_list_into_it.png)
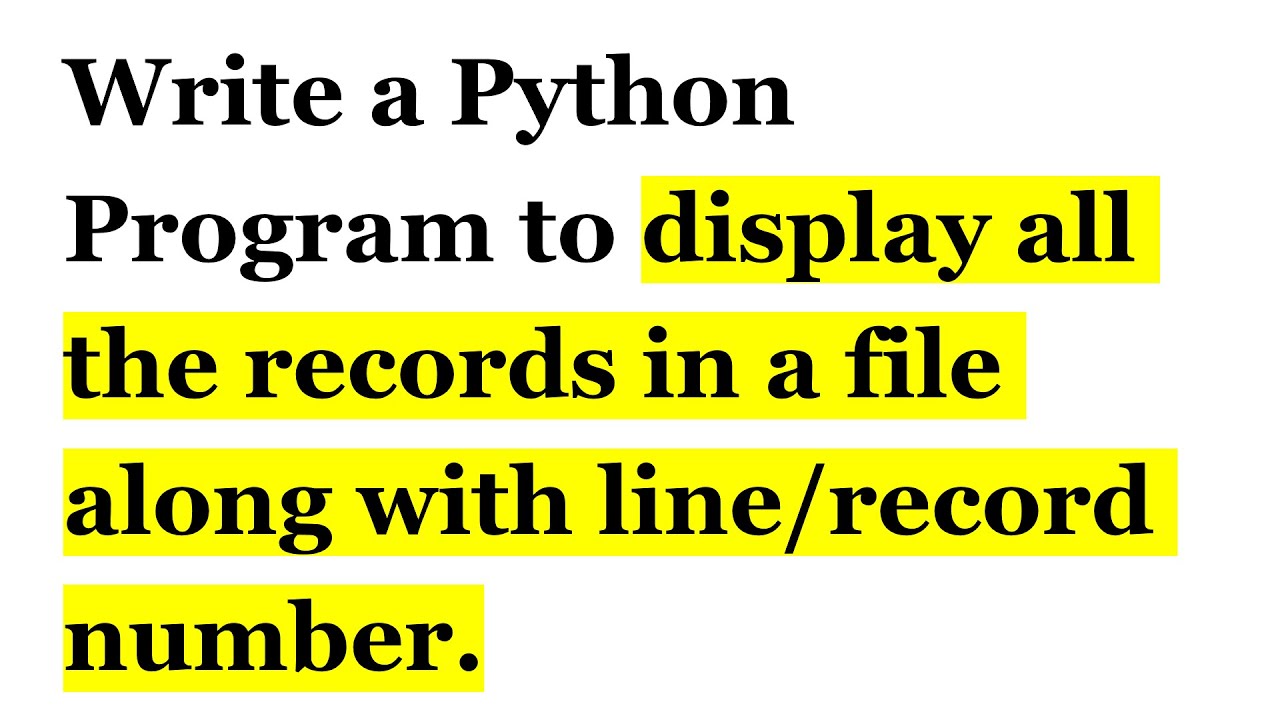

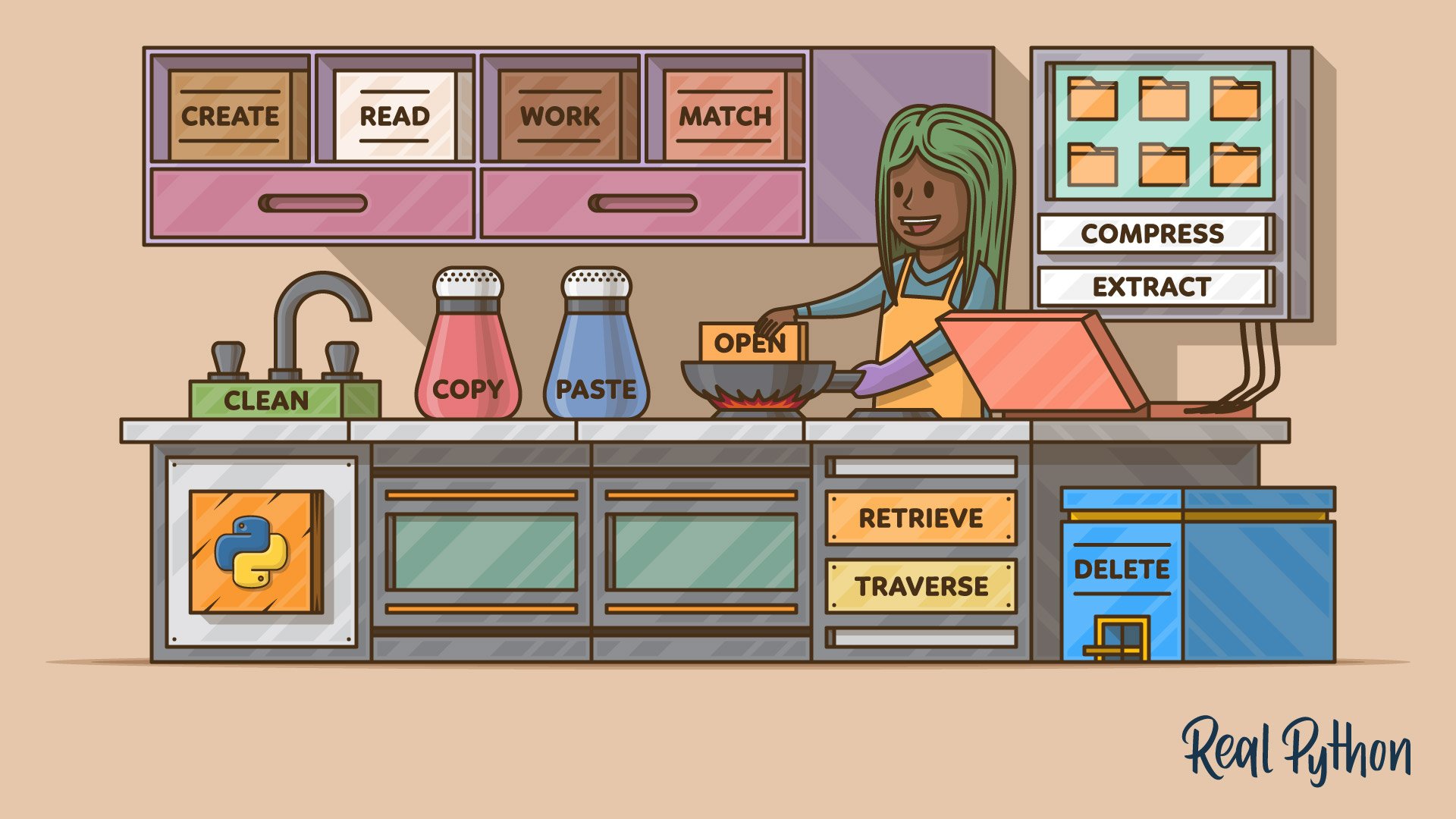


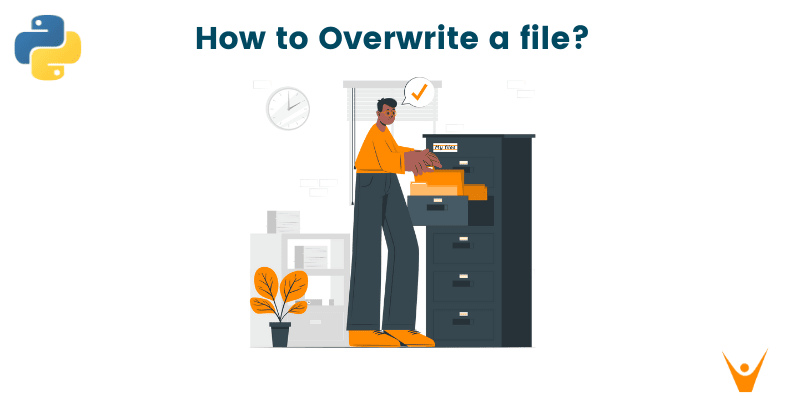
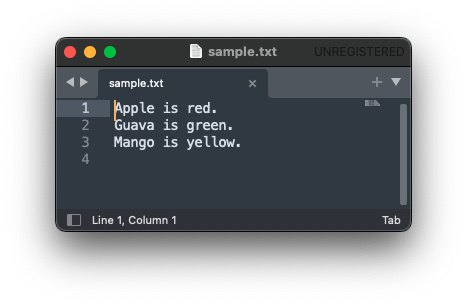
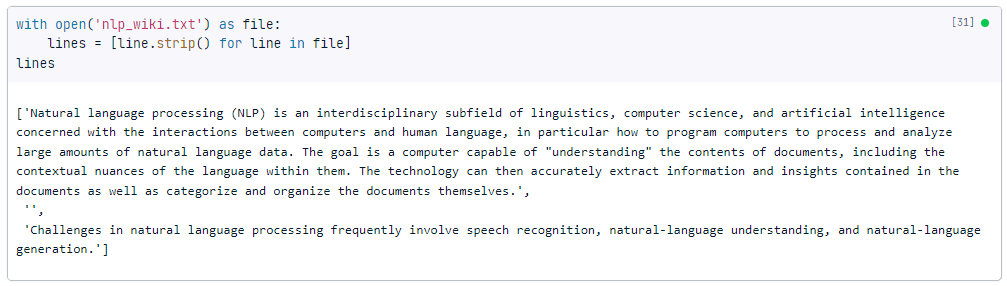
Article link: python write line to file.
Learn more about the topic python write line to file.
- Correct way to write line to file? – python – Stack Overflow
- How to Write to Text File in Python
- How to Write Line to File in Python – AppDividend
- Python File writelines() Method – W3Schools
- Correct Way to Write line To File in Python – Finxter
- How to write a string to a file on a new line every time in Python
- How to Write a File in Python – Scaler Topics
- Write a String to a File on a New Line every time in Python
See more: https://nhanvietluanvan.com/luat-hoc