Python Write File No Such File Or Directory
1. Understanding the Error
When working with file operations in Python, you may encounter the error message “No such file or directory.” This error occurs when the specified file or directory cannot be found at the given path. It signifies that the Python script is unable to read from or write to the file or directory.
There can be various causes for this error, including typos or incorrect file paths, lack of proper permissions to access the file, or the file simply not existing. It is crucial to address this error promptly to ensure proper functioning of the Python script.
2. Checking File Paths
Verifying the accuracy of the file path is essential in resolving the “No such file or directory” error. Common mistakes in specifying file paths include:
– Misspelling or mistyping the file or directory name.
– Incorrectly formatting the file path, such as missing or using incorrect slashes.
– Using absolute or relative paths incorrectly.
Properly specifying the file path can significantly minimize the chances of encountering this error. Understanding the difference between absolute paths (complete path from the root directory) and relative paths (path relative to the current working directory) is crucial in avoiding this error.
3. File Location Verification
Verifying the existence of the specified file or directory is essential in troubleshooting the “No such file or directory” error. The `os.path` module can be used for file path operations in Python.
By using functions like `os.path.exists()` and `os.path.isdir()`, you can check if the file or directory exists and if it is a directory, respectively. Handling cases where files or directories are missing is important for proper error handling and program flow.
4. Permissions and Access Issues
In some cases, the “No such file or directory” error may occur due to file permissions or access issues. File permissions control who can access and perform operations on files.
You can check file permissions using the `os` module’s functions, such as `os.access()` and `os.stat()`. These functions allow you to determine if you have the necessary permissions to read from or write to a file, and to retrieve information about the file’s permissions.
Resolving permission-related errors may involve adjusting the permissions of the file or the directory containing it. This can be done using the `os.chmod()` function.
5. Handling File Not Found Errors
Employing exception handling using `try-except` blocks is a good practice when dealing with file operations. To specifically handle the “No such file or directory” error, you can catch the `FileNotFoundError` exception.
By wrapping the file operations within a `try` block and providing a suitable error message in the `except` block, you can display helpful information to the user when a file is not found.
6. Creating New Files Dynamically
In certain scenarios, you may need to write files dynamically when they don’t exist. To accomplish this, you can use the `open()` function with appropriate parameters.
By specifying the file mode as “w”, you can create a new file for writing. Additionally, you can ensure that the specified directory exists before attempting to create the file. This can be achieved using the `os.makedirs()` function to create any necessary directories recursively.
7. Renaming or Moving Files
When renaming or moving files, it is important to handle errors to avoid encountering the “No such file or directory” error. Before performing any operations, it is necessary to check if the file exists at the specified path.
The `shutil` module in Python provides functions like `shutil.move()` and `shutil.copy()` for efficient and error-tolerant file operations. These functions handle the renaming or moving of files seamlessly, reducing the chances of encountering errors.
8. Cross-Platform Considerations
It is essential to understand that file paths may differ across different operating systems. Properly considering these platform differences can help prevent the “No such file or directory” error.
The `os.path` module provides tools for working with file paths in a platform-independent manner. By using functions like `os.path.join()` and `os.path.sep`, you can construct file paths that are compatible across different platforms.
9. Debugging Techniques
Debugging techniques can be employed to identify the root causes of the “No such file or directory” error. Using a debugger allows you to inspect the file path and related variables during runtime, helping you pinpoint the issue.
Stepping through the code and examining variable values can help identify any typos, incorrect file paths, or logical errors that may be causing the error. Debugging is an effective way to troubleshoot and fix issues related to file operations.
10. Best Practices and Error Prevention
To avoid the “No such file or directory” error, it is important to adopt good file handling practices from the start. Some best practices include:
– Double-checking file paths for accuracy.
– Providing informative error messages to facilitate better troubleshooting.
– Regularly testing file operations to catch and address potential errors.
By following these best practices, you can minimize the chances of encountering this error in your Python code.
FAQs:
Q1. What does the error message “No such file or directory” mean?
– This error message indicates that the specified file or directory cannot be found at the given path. It signifies that the Python script is unable to read from or write to the file or directory.
Q2. What are some common causes of the “No such file or directory” error?
– Some common causes include typos or incorrect file paths, lack of proper permissions to access the file, or the file simply not existing.
Q3. How can I check if a file or directory exists in Python?
– You can use the `os.path.exists()` function to check if a file exists, and the `os.path.isdir()` function to check if a directory exists.
Q4. How can I handle the “No such file or directory” error in Python?
– You can handle this error by using exception handling with `try-except` blocks. Specifically, you can catch the `FileNotFoundError` exception and display a helpful error message to the user.
Q5. How can I create a new file dynamically in Python if it doesn’t exist?
– You can use the `open()` function with the file mode set to “w” to create a new file for writing. Additionally, you can ensure that the specified directory exists before creating the file by using the `os.makedirs()` function.
Q6. Are there any cross-platform considerations when working with file paths in Python?
– Yes, file paths may differ across different operating systems. To ensure compatibility, you can use the `os.path` module for platform-independent file path operations.
In conclusion, encountering the “No such file or directory” error in Python while performing file operations can be frustrating. However, by understanding the causes of this error and implementing appropriate solutions, you can effectively address and prevent it. Taking precautions, verifying file paths, handling permissions, and using proper debugging techniques will contribute to error-free file handling in your Python programs.
Filenotfounderror Errno 2 No Such File Or Directory Python Error Solved
How To Create A Text File In Python?
Python is an incredibly versatile programming language that allows you to perform a wide range of tasks. One of the most common tasks you may encounter is creating a text file. Whether you are storing data, writing logs, or generating reports, Python provides simple and efficient methods to create and manipulate text files. In this article, we will explore the various techniques and functions available in Python to create a text file.
1. Opening a text file:
Before we can create a text file, we need to open it first. Python provides the built-in `open()` function to perform this task. The `open()` function takes two parameters – the file name and the mode. In the case of creating a text file, we need to use the mode ‘w’ which stands for write mode.
“`python
file = open(“example.txt”, “w”)
“`
2. Writing content to the text file:
Once the file is open, we can start writing content to it. Python provides the `write()` method to write a string to a file. This method can be called multiple times to write different lines or pieces of text.
“`python
file.write(“Hello, this is my first line!”)
file.write(“\nAnd this is the second line.”)
“`
Note that we used the escape character `\n` to insert a line break between the two lines.
3. Closing the file:
After writing the content, it’s important to close the file. This ensures that all the data is saved and prevents any memory leaks. The `close()` method is used for this purpose.
“`python
file.close()
“`
4. Simplifying the process with a context manager:
While the above approach works perfectly fine, Python provides a more efficient way of handling file operations using a context manager. A context manager automatically takes care of opening and closing the file, even if an exception occurs during the operation. It ensures that system resources are properly managed.
“`python
with open(“example.txt”, “w”) as file:
file.write(“Hello, this is my first line!”)
file.write(“\nAnd this is the second line.”)
“`
The `with` statement ensures that the file is automatically closed once the indented block of code finishes executing.
FAQs:
Q: Can I create a text file in a specific directory?
A: Yes, by specifying the absolute or relative path of the file while opening it. For example, to create a file named “example.txt” in the directory “documents,” you can use `open(“documents/example.txt”, “w”)`.
Q: What if the file already exists?
A: When opening a file in write mode, if the file already exists, Python clears its contents and starts afresh. If you want to preserve the existing content, you should use the append mode by specifying ‘a’ instead of ‘w’ while opening the file.
Q: How can I check if the file creation was successful?
A: You can use a `try-except` block to catch any exceptions that may occur during file operations. If an exception is raised, it means that the file creation process has failed.
Q: How do I add new lines in the text file?
A: As shown earlier, you can use the `\n` escape character to insert line breaks in the text. Additionally, you can also use the `write()` method with the argument as a string containing multiple lines.
Q: Is it possible to create a file in a different file format, like a CSV or JSON file?
A: Yes, Python allows you to create files in various formats. Instead of using the ‘w’ mode, you can use ‘wb’ for binary files, ‘a’ for append mode, and different file extensions (.csv, .json, .xml, etc.) to specify the file format.
Q: How can I add additional data to an existing text file?
A: While opening the file, you need to use the append mode, ‘a’, instead of the write mode. This ensures that the new data is appended to the end of the file without overwriting the existing content.
In conclusion, creating a text file in Python is a straightforward process. With the `open()` function and the `write()` method, you can easily create and write content to a text file. Remember to close the file after you finish working with it, or utilize the context manager for efficient resource management. Python’s flexibility even allows you to create files in different formats or append new data to existing files. Happy file creation in Python!
Why Python Cannot Find A File In Directory?
Python, being a popular and versatile programming language, is widely used for various purposes, including file handling and data processing. However, a common issue faced by many Python developers is when Python cannot find a file in a directory. This can be frustrating, especially for beginners who are still learning the nuances of the language. In this article, we will delve into the reasons why Python may fail to locate a file in a directory, possible solutions, and provide some frequently asked questions for further clarity.
Possible Reasons for Python’s Inability to Find a File:
1. Incorrect File Path:
One of the most common reasons why Python cannot locate a file is an incorrect file path. It is crucial to provide the correct path to the file, including any necessary directories or subdirectories. Be aware of any differences in the path based on the operating system you are using, as Windows and Unix-like systems handle paths differently.
2. Working Directory Mismatch:
Python uses the current working directory as a reference when searching for files. If you are executing your script from a different directory than where the file is located, Python might not be able to find it. Ensure that you are in the correct directory or provide an absolute path to the file to avoid any confusion.
3. File Not Found in Directory:
Another reason Python cannot find a file is that the file simply does not exist in the specified directory. Double-check the spelling and case of the filename, as they are case-sensitive in most operating systems. Additionally, verify that the file has not been moved, deleted, or renamed unintentionally.
4. Permissions and Access Rights:
If the file you are trying to access has restrictive permissions or access rights, Python may not have the necessary privileges to locate or open the file. Ensure that you have the appropriate permissions to access the file, especially if you are working with sensitive files or directories.
How to Resolve File Not Found Issues:
1. Verify the File Path:
Carefully review the file path you are providing in your code to ensure its accuracy. Consider using built-in Python functions like `os.path` module to manipulate and construct file paths correctly. Use the `os.path.exists()` function to check if a file actually exists in the specified path.
2. Set the Working Directory:
Explicitly set the working directory in your Python script using the `os.chdir()` function. This can help avoid any confusion about the current directory, especially if you are executing your script from different locations. Before executing file-related operations, use `os.getcwd()` to confirm that you are in the correct directory.
3. Use Absolute Path:
Instead of relying on relative paths, consider using absolute paths to locate your file. By providing the full path from the root directory, you eliminate any uncertainty caused by varying working directories. Absolute paths remain constant regardless of where your script is executed from.
4. Debug Your Code:
If you are still unable to locate the file, debug your code by adding print statements or using debugging tools like `pdb`. This helps in identifying any logical errors or issues in your code that may prevent Python from finding the file.
FAQs:
Q1. Can Python search for files in subdirectories?
Yes, Python can search for files in subdirectories. You can use the `os.walk()` function to traverse through a directory and its subdirectories, enabling you to locate files even in nested folders.
Q2. What should I do if the file name contains special characters or spaces?
If a file name contains special characters or spaces, you need to ensure that you are properly escaping or handling those characters. Use escape characters such as a backslash (“\”) or put the file name in quotes to avoid any parsing issues.
Q3. How can I handle file path differences across operating systems?
To handle file path differences across operating systems, Python provides the `os.path` module. It includes functions like `os.path.join()` and `os.path.sep` that assist in constructing platform-independent file paths. By leveraging these functions, you can write code that runs smoothly on various operating systems.
Q4. Why does Python provide relative paths instead of absolute paths by default?
Python provides relative paths by default to enhance code portability. Relative paths make it easier to move code between systems without affecting its functionality or requiring changes in the file paths. However, when working with specific files, using absolute paths is generally recommended for consistency and to avoid any ambiguity.
In conclusion, Python’s inability to locate a file in a directory can be traced back to various causes, including incorrect file paths, working directory mismatches, or simply not finding the file in the directory. By ensuring accurate file paths, setting the correct working directory, and verifying proper permissions, you can overcome these challenges and successfully locate files using Python. Happy coding!
Keywords searched by users: python write file no such file or directory Python create file if not exists, FileNotFoundError, Python open file create folder if not exist, Python save file to directory, Python open filenotfounderror, Python directory path, Create file Python, Check path is directory Python
Categories: Top 54 Python Write File No Such File Or Directory
See more here: nhanvietluanvan.com
Python Create File If Not Exists
Creating a file if not exists is a common requirement when working with file-based operations. Python provides several built-in methods and libraries to facilitate this task. Here, we will discuss some of the most commonly used techniques.
The first approach involves using the built-in `open()` function, which is used to open a file in Python. By specifying the ‘x’ mode as the second argument, we can instruct Python to create a file if it does not already exist. Let’s take a look at an example:
“`python
def create_file(filename):
try:
file = open(filename, ‘x’)
file.close()
print(f”File ‘{filename}’ created successfully!”)
except FileExistsError:
print(f”File ‘{filename}’ already exists!”)
create_file(“example.txt”)
“`
In the above code snippet, we define a function `create_file()` which takes a filename as a parameter. Inside the function, we open the file using the `open()` function with the ‘x’ mode. If the file does not exist, it will be created and then closed using the `close()` method. If the file already exists, a `FileExistsError` exception will be raised and we can handle it accordingly.
Another way to create a file if not exists is by using the `Path` class from the `pathlib` module, which provides an object-oriented approach to working with files and directories. The `Path` class has a convenient method called `touch()` that accomplishes our task effortlessly. Here’s an example:
“`python
from pathlib import Path
def create_file(filename):
file = Path(filename)
if not file.is_file():
file.touch()
print(f”File ‘{filename}’ created successfully!”)
else:
print(f”File ‘{filename}’ already exists!”)
create_file(“example.txt”)
“`
In the above code, we use the `Path` class to create a Path object representing our file. Then, we check if the file already exists using the `is_file()` method. If the file does not exist, we call the `touch()` method to create it. If the file exists, we handle it accordingly.
Furthermore, the `os` module in Python provides various functions for working with operating system-dependent operations, including file creation. We can utilize the `os.path.exists()` function to check if a file exists or not, and combine it with the `open()` function to create a file. Consider the following example:
“`python
import os
def create_file(filename):
if not os.path.exists(filename):
file = open(filename, ‘w’)
file.close()
print(f”File ‘{filename}’ created successfully!”)
else:
print(f”File ‘{filename}’ already exists!”)
create_file(“example.txt”)
“`
In this code snippet, we use the `os.path.exists()` function to check if the file exists or not. If the file does not exist, we proceed to create it using the `open()` function with the ‘w’ mode, which creates a new file for writing. Finally, we close the file and print an appropriate message.
Now, let’s address some frequently asked questions about creating files if they do not exist in Python.
## FAQs
#### Q1. Can I specify a different location for the file to be created?
Absolutely! By providing a different path along with the filename, you can choose the location where you want to create the file. For example:
“`python
create_file(“/path/to/folder/example.txt”)
“`
In the above code, the file “example.txt” will be created in the “folder” directory located at “/path/to/”.
#### Q2. What happens if there are permission issues while creating the file?
If the user does not have sufficient permissions to create a file in the desired location, a `PermissionError` exception will be raised. You can handle this exception in an appropriate way, such as notifying the user or trying to create the file in a different location.
#### Q3. How can I create multiple directories along with the file?
To create multiple directories along with the file, you can use the `os.makedirs()` function. This function creates all the intermediate directories in the specified path if they do not already exist. Here’s an example:
“`python
import os
def create_file(filename):
os.makedirs(os.path.dirname(filename), exist_ok=True)
if not os.path.exists(filename):
with open(filename, ‘w’) as file:
print(f”File ‘{filename}’ created successfully!”)
else:
print(f”File ‘{filename}’ already exists!”)
create_file(“path/to/folder/example.txt”)
“`
In this code, we use the `os.makedirs()` function to create the necessary directories. The `os.path.dirname()` function extracts the directory path from the full file path.
Creating a file if it does not exist is a common requirement in many programming scenarios. Python offers multiple approaches to accomplish this task. Whether it is using the built-in `open()` function, the `pathlib` module, or the `os` module, developers have several options to handle file creation dynamically. By understanding these techniques and incorporating them into their code, developers can efficiently create files as needed.
Remember, it is always important to handle exceptions and potential errors that may arise during file creation, such as permission issues or invalid file paths. By incorporating error handling mechanisms, developers can ensure their application behaves robustly and gracefully handles any unforeseen issues.
Filenotfounderror
When writing code in Python, it is not uncommon to encounter errors. One such error that developers often come across is FileNotFoundError. This exception is raised when a file or directory is requested for an operation, but it is not found in the given location. In this article, we will take an in-depth look at the FileNotFoundError in Python, exploring its causes, how to handle it, and answer some frequently asked questions about this exception.
#### Understanding FileNotFoundError
FileNotFoundError is a built-in exception in Python, and it is a subclass of the IOError exception. It is raised when an operation, such as opening, reading, writing, or deleting a file or directory, is performed on a file or directory that does not exist. The error message typically provides information about the file or directory that could not be found, helping developers identify and resolve the issue.
#### Causes of FileNotFoundError
There are several reasons why FileNotFoundError may occur in Python code. Some common causes include:
1. Incorrect file path: If the file or directory path provided in the code is incorrect, the Python interpreter will raise a FileNotFoundError. Double-checking the path and ensuring it is accurate can help resolve the error.
2. File or directory not created: Another common cause is when the file or directory is expected to exist but has not been created yet. If the code attempts to access a file that has not been created, the FileNotFoundError will be raised.
3. File or directory deleted: If the file or directory was present before but has been deleted, attempting to perform any operation on it will result in a FileNotFoundError.
#### Handling FileNotFoundError
When encountering a FileNotFoundError, it is important to handle the exception gracefully in order to provide a better user experience and avoid application crashes. Here are some approaches to consider:
1. Error messages: Displaying a meaningful error message to the user is crucial. This can be done by using print statements or logging libraries to log the error information. Including details about the file or directory that was not found can help in troubleshooting the issue.
2. Input validation: To prevent FileNotFoundError, input validation should be performed before performing any file or directory operations. Verifying that the file or directory exists before proceeding with the operation can help avoid raising the exception.
3. Exception handling: Implementing try-except blocks is a recommended way to handle exceptions in Python. By surrounding the code that may raise FileNotFoundError with a try block and catching the exception in an except block, you can control the flow of the program and handle the error gracefully.
#### Frequently Asked Questions
Q: Can I check if a file exists without triggering FileNotFoundError?
A: Yes, you can check if a file exists by using the os.path module. The os.path.exists() function returns True if the file exists, allowing you to avoid FileNotFoundError.
Q: Are there any alternative exceptions to FileNotFoundError?
A: Yes, depending on the specific operation being performed, other exceptions may be raised. Some alternatives include IsADirectoryError, PermissionError, and NotADirectoryError.
Q: How can I create a file if it does not exist?
A: You can create a file using the open() function in write mode. If the file does not exist, it will be created. Here’s an example:
“`python
file_path = “example.txt”
try:
file = open(file_path, “w”) # Open file in write mode
except FileNotFoundError:
print(“File not found”)
else:
file.close()
print(“File created successfully”)
“`
Q: What should I do if I accidentally delete a file my program relies on?
A: If you accidentally delete a file that your program relies on, you can recover it from a backup if available. Otherwise, you may need to rewrite the file or, if possible, try to recover it using data recovery software.
Q: Does FileNotFoundError only apply to files or can it be raised for directories as well?
A: FileNotFoundError can be raised for both files and directories. If the specified path does not point to an existing directory, the exception will be raised.
In conclusion, understanding the FileNotFoundError exception in Python is essential for writing robust and error-free code. By handling this exception effectively and employing best practices in exception handling, developers can ensure smooth execution of their code and provide better error messages to end-users. Additionally, knowing how to check file existence and handle related exceptions can help prevent and manage file-related errors efficiently.
Python Open File Create Folder If Not Exist
To open a file in Python, you can use the built-in `open()` function. This function requires at least one argument, which is the file name or the path to the file you want to open. Here is the basic syntax of the `open()` function:
“`python
file = open(file_name, mode)
“`
The `file_name` parameter represents the name of the file, while the `mode` parameter specifies the mode in which the file should be opened. The `mode` parameter is optional, and if not specified, the file is opened in the default read-only mode.
File modes allow you to specify how the file should be opened. There are several modes available, including:
– `’r’`: Read mode, which allows you to read the contents of the file.
– `’w’`: Write mode, which allows you to write data to the file. If the file already exists, it will be overwritten.
– `’a’`: Append mode, which allows you to append data to the end of an existing file. If the file does not exist, it will be created.
– `’x’`: Exclusive creation mode, which creates a new file and raises an error if the file already exists.
Now that we know how to open a file in Python, let’s move on to creating folders if they do not exist. To create a folder, we can use the `os` module, which provides a way to interact with the operating system. The `os.path.exists()` function can be used to check if a folder already exists.
Here is how you can create a folder using Python:
“`python
import os
folder_name = ‘/path/to/folder’
if not os.path.exists(folder_name):
os.makedirs(folder_name)
print(“Folder created successfully!”)
else:
print(“Folder already exists.”)
“`
In the above code, we first define the `folder_name` variable to hold the path of the folder we want to create. We then use the `os.path.exists()` function to check if the folder already exists. If it does not exist, we create it using the `os.makedirs()` function. Finally, we print a success message if the folder is created, or an “already exists” message if the folder is already there.
Now, let’s address some frequently asked questions about opening files and creating folders in Python:
**Q: Can I open multiple files at once?**
A: Yes, you can open multiple files at once by calling the `open()` function multiple times with different file names.
**Q: What if I want to specify the encoding of the file when opening it?**
A: You can include an optional `encoding` parameter when calling the `open()` function. For example, `open(file_name, mode, encoding=’utf-8′)`.
**Q: How can I check if a file is open or closed?**
A: By calling the `file.closed` attribute, you can determine whether a file is open or closed. If the attribute returns `False`, the file is open; if it returns `True`, the file is closed.
**Q: Can I create nested folders using `os.makedirs()`?**
A: Yes, `os.makedirs()` allows you to create nested folders. You can provide a path like `’parent_folder/sub_folder’`, and Python will create both the parent and sub-folder if they do not exist.
**Q: How can I delete a file or folder in Python?**
A: To delete a file, you can use the `os.remove()` function, while to delete a folder, you can use the `os.rmdir()` function. Please note that the folder must be empty to be deleted.
In conclusion, Python offers straightforward methods for opening files and creating folders. By using the `open()` function, you can access and manipulate file contents with ease. Additionally, the `os` module provides tools for checking the existence of folders and creating new ones if necessary. By mastering these techniques, you will be equipped to efficiently handle file and folder operations in your Python programs.
Images related to the topic python write file no such file or directory
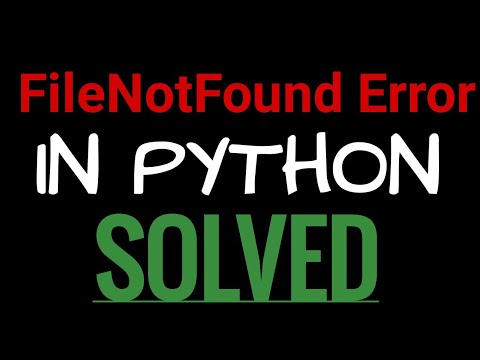
Found 33 images related to python write file no such file or directory theme
![FileNotFoundError: [Errno 2] No such file or directory: 'FSnm.png' - 🚀 Deployment - Streamlit Filenotfounderror: [Errno 2] No Such File Or Directory: 'Fsnm.Png' - 🚀 Deployment - Streamlit](https://global.discourse-cdn.com/business7/uploads/streamlit/optimized/2X/7/7a62f10889b442beaf7a1b533406adf4e2b4f636_2_1024x506.png)

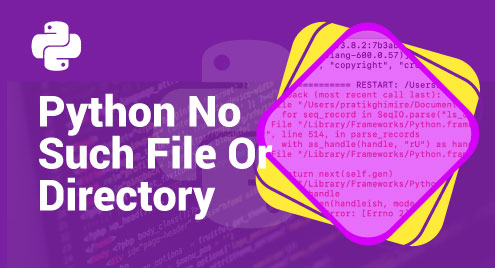
![python - FileNotFoundError: [Errno 2] No such file or directory: 'pi_digits.txt' - Stack Overflow Python - Filenotfounderror: [Errno 2] No Such File Or Directory: 'Pi_Digits.Txt' - Stack Overflow](https://i.stack.imgur.com/jUpeq.jpg)
![can't open file 'manage.py': [Errno 2] No such file or directory| SOLVED - YouTube Can'T Open File 'Manage.Py': [Errno 2] No Such File Or Directory| Solved - Youtube](https://i.ytimg.com/vi/BN20NkY-Ss0/sddefault.jpg)
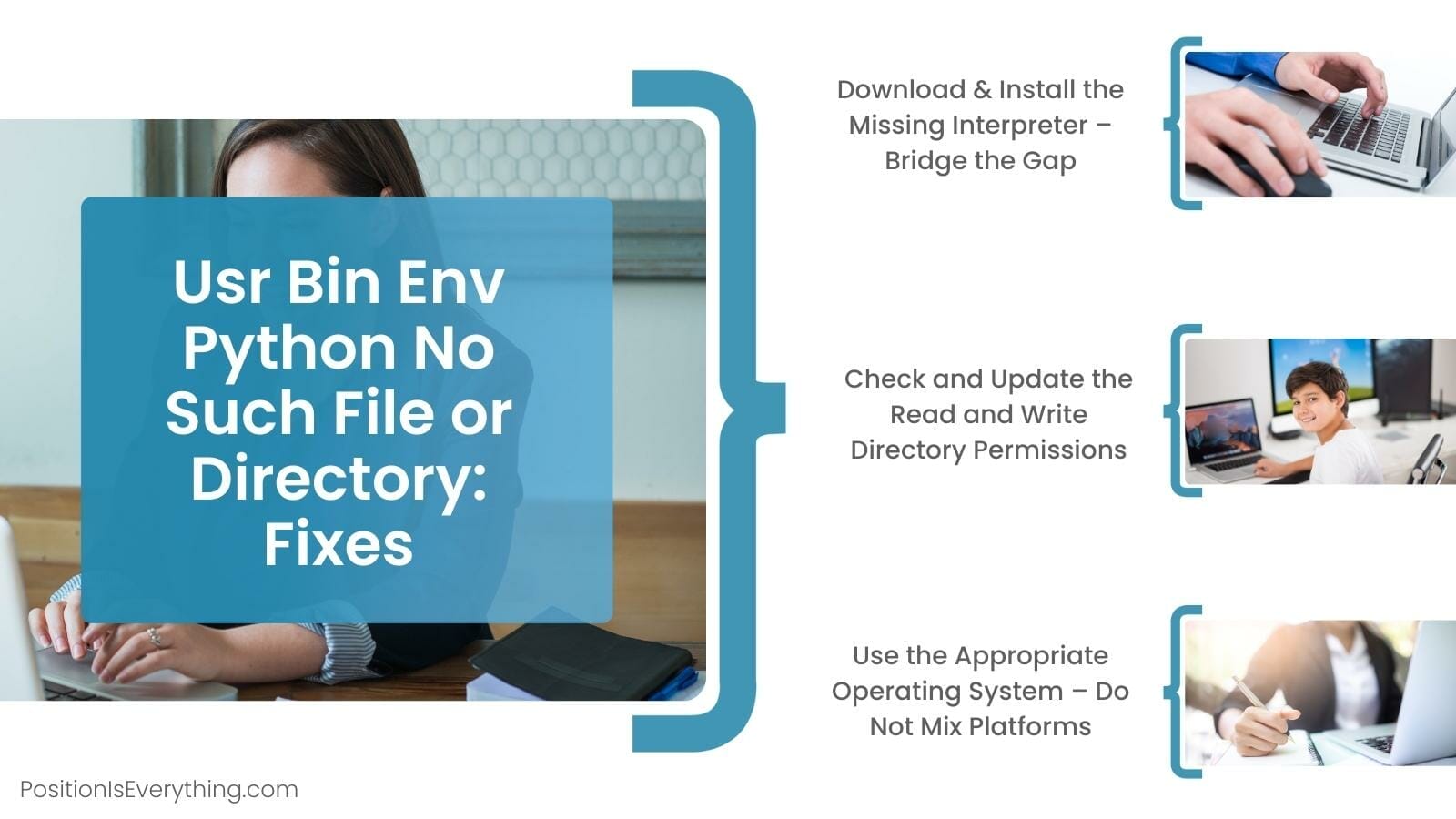

![FileNotFoundError: [Errno 2] No such file or directory: 'FSnm.png' - 🚀 Deployment - Streamlit Filenotfounderror: [Errno 2] No Such File Or Directory: 'Fsnm.Png' - 🚀 Deployment - Streamlit](https://global.discourse-cdn.com/business7/uploads/streamlit/original/2X/7/7a62f10889b442beaf7a1b533406adf4e2b4f636.png)

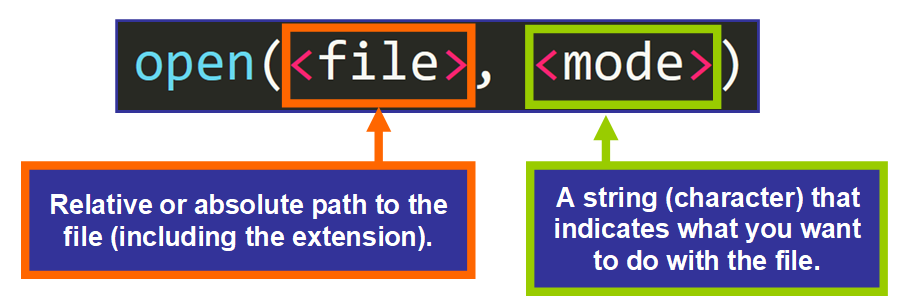
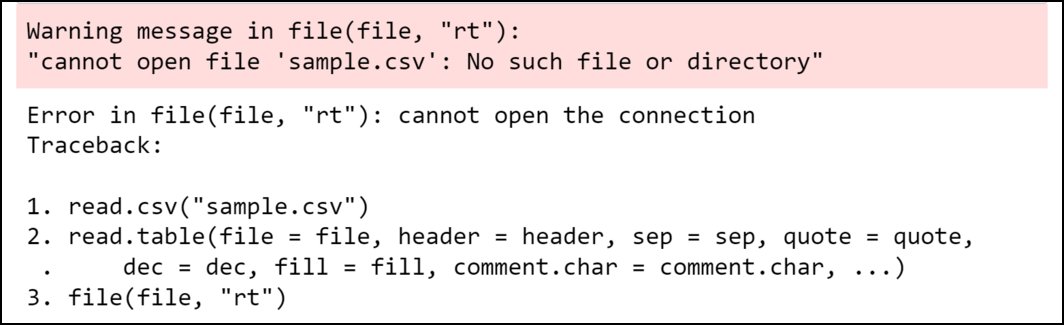
![Errno 2] No such file or directory - Notebook - Jupyter Community Forum Pyqt - Python.H: No Such File Or Directory #Include <Python.H> – Ask Ubuntu” style=”width:100%” title=”pyqt – Python.h: No such file or directory #include <Python.h> – Ask Ubuntu”><figcaption>Pyqt – Python.H: No Such File Or Directory #Include <Python.H> – Ask Ubuntu</figcaption></figure>
<figure><img decoding=](https://i.stack.imgur.com/l39J2.png)
![FileNotFoundError: [Errno 2] No such file or directory – Its Linux FOSS Filenotfounderror: [Errno 2] No Such File Or Directory – Its Linux Foss](https://itslinuxfoss.com/wp-content/uploads/2022/11/Errno-2-No-such-file-or-directory-4.png)
![파이썬(Python) FileNotFoundError: [Errno 2] No such file or directory: 에러코드 해결 : 네이버 블로그 파이썬(Python) Filenotfounderror: [Errno 2] No Such File Or Directory: 에러코드 해결 : 네이버 블로그](https://mblogthumb-phinf.pstatic.net/MjAxOTA3MThfMjk2/MDAxNTYzNDYwMTAxNDkw.80pdiiPqXISePxK_TMqY1vCtlLL8g63rjFF0IrgvhVgg.khvF6-yDMXuHJ7IBGU9PxIkPS3SW07R5OoUQwuL2I34g.PNG.kimcd1107/123.png?type=w800)

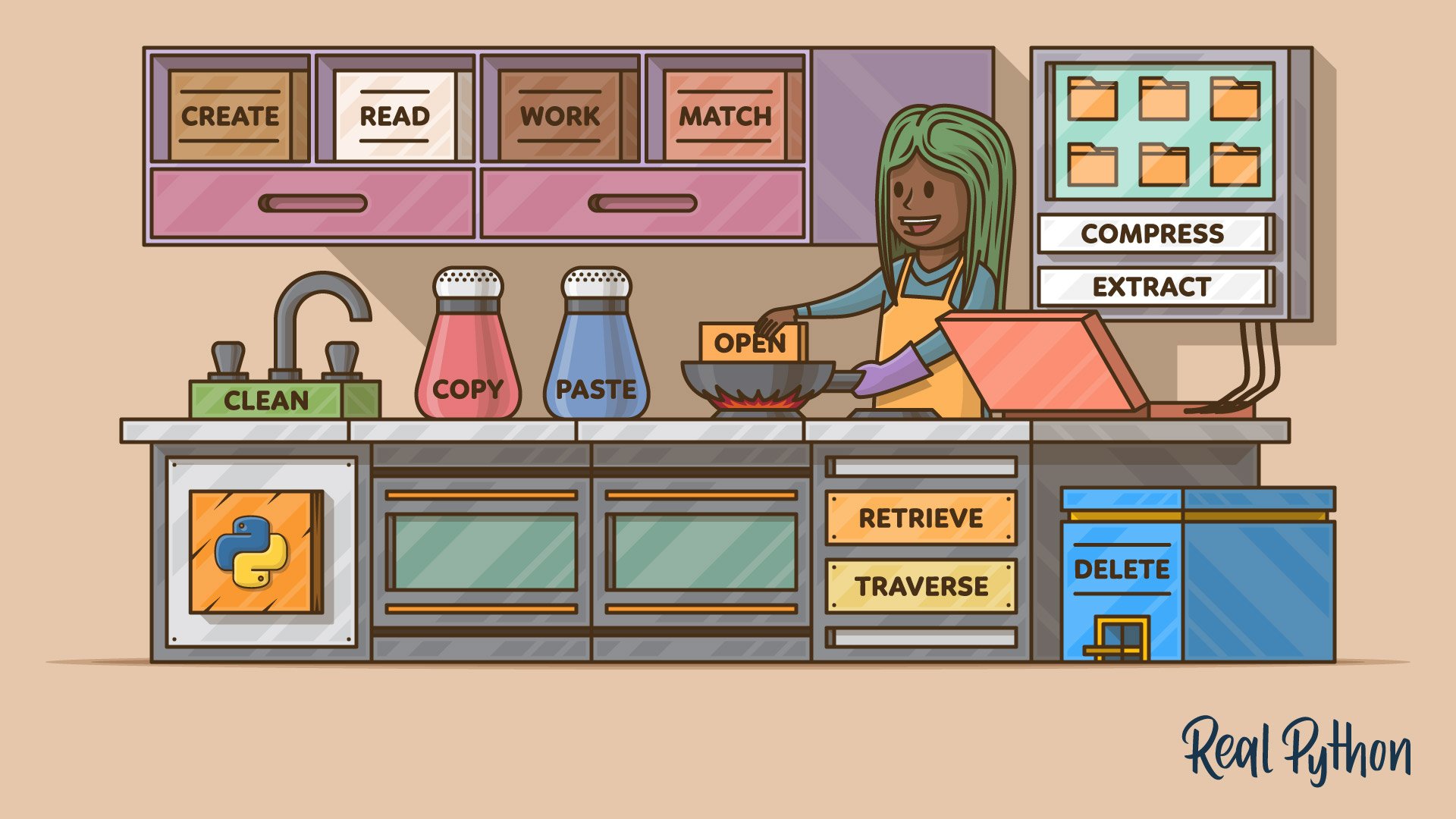
![File Handling in Python [Complete Series] – PYnative File Handling In Python [Complete Series] – Pynative](https://pynative.com/wp-content/uploads/2021/07/file_handling_in_python.png)
![FileNotFoundError: [Errno 2] No such file or directory – Its Linux FOSS Filenotfounderror: [Errno 2] No Such File Or Directory – Its Linux Foss](https://itslinuxfoss.com/wp-content/uploads/2022/11/Errno-2-No-such-file-or-directory-6.png)

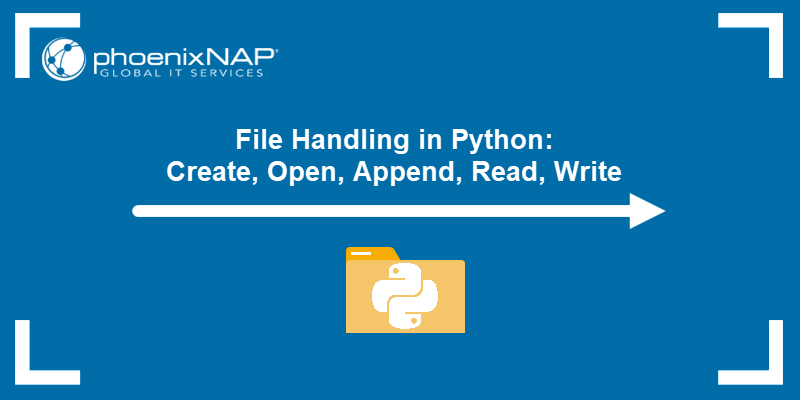
![json - Why FileNotFoundError: [Errno 2] No such file or directory if I do have it in Python? - Stack Overflow Json - Why Filenotfounderror: [Errno 2] No Such File Or Directory If I Do Have It In Python? - Stack Overflow](https://i.stack.imgur.com/UzZVK.png)

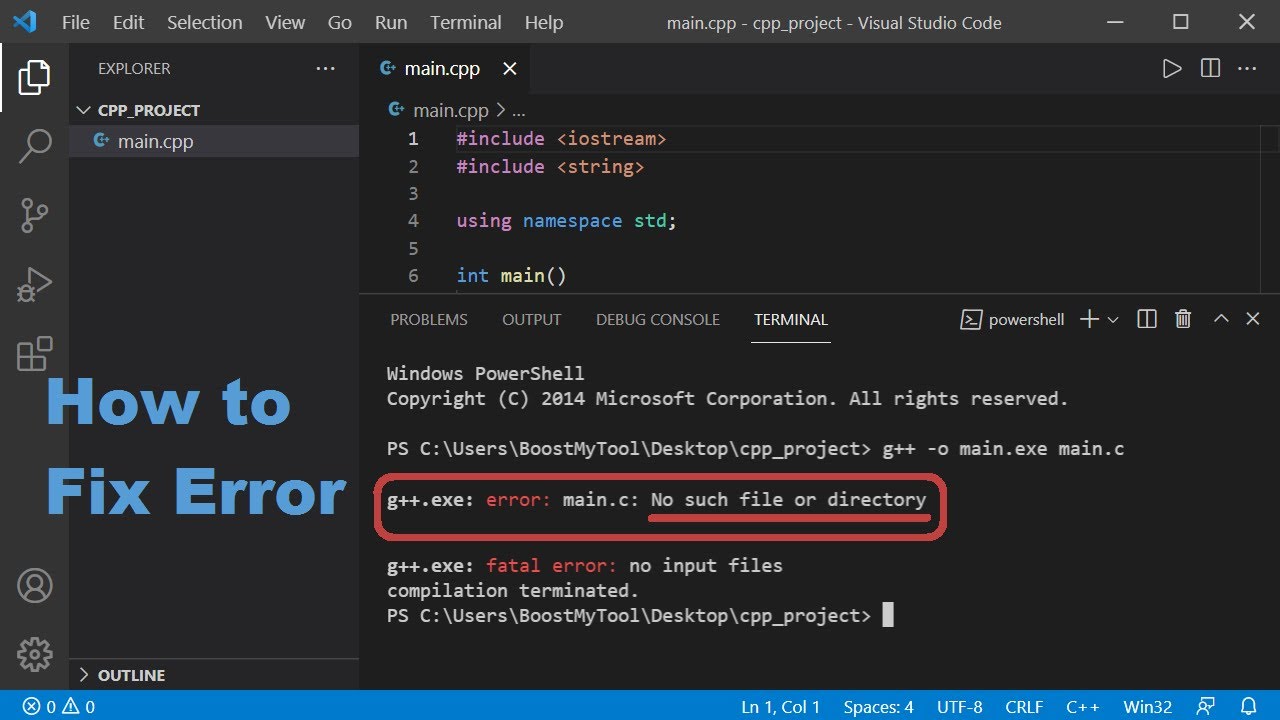
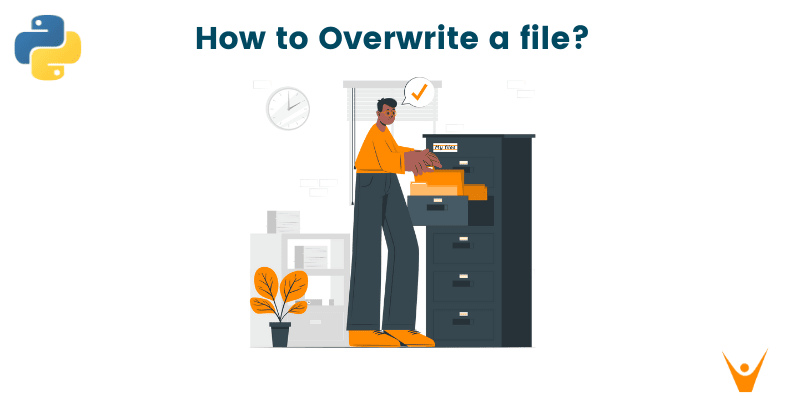
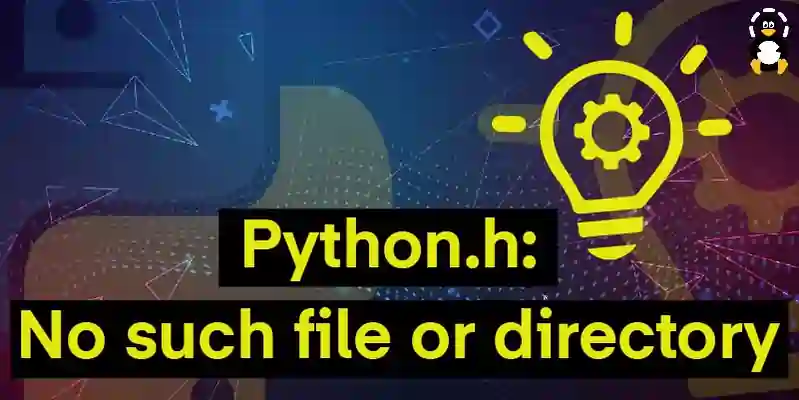

![Giúp sửa lỗi can't open file: [Errno 2] No such file or directory - programming - Dạy Nhau Học Giúp Sửa Lỗi Can'T Open File: [Errno 2] No Such File Or Directory - Programming - Dạy Nhau Học](https://daynhauhoc.s3.dualstack.ap-southeast-1.amazonaws.com/optimized/2X/c/c84da462ef79c3ec415fe44b96dfabb8f648728e_2_689x359.png)
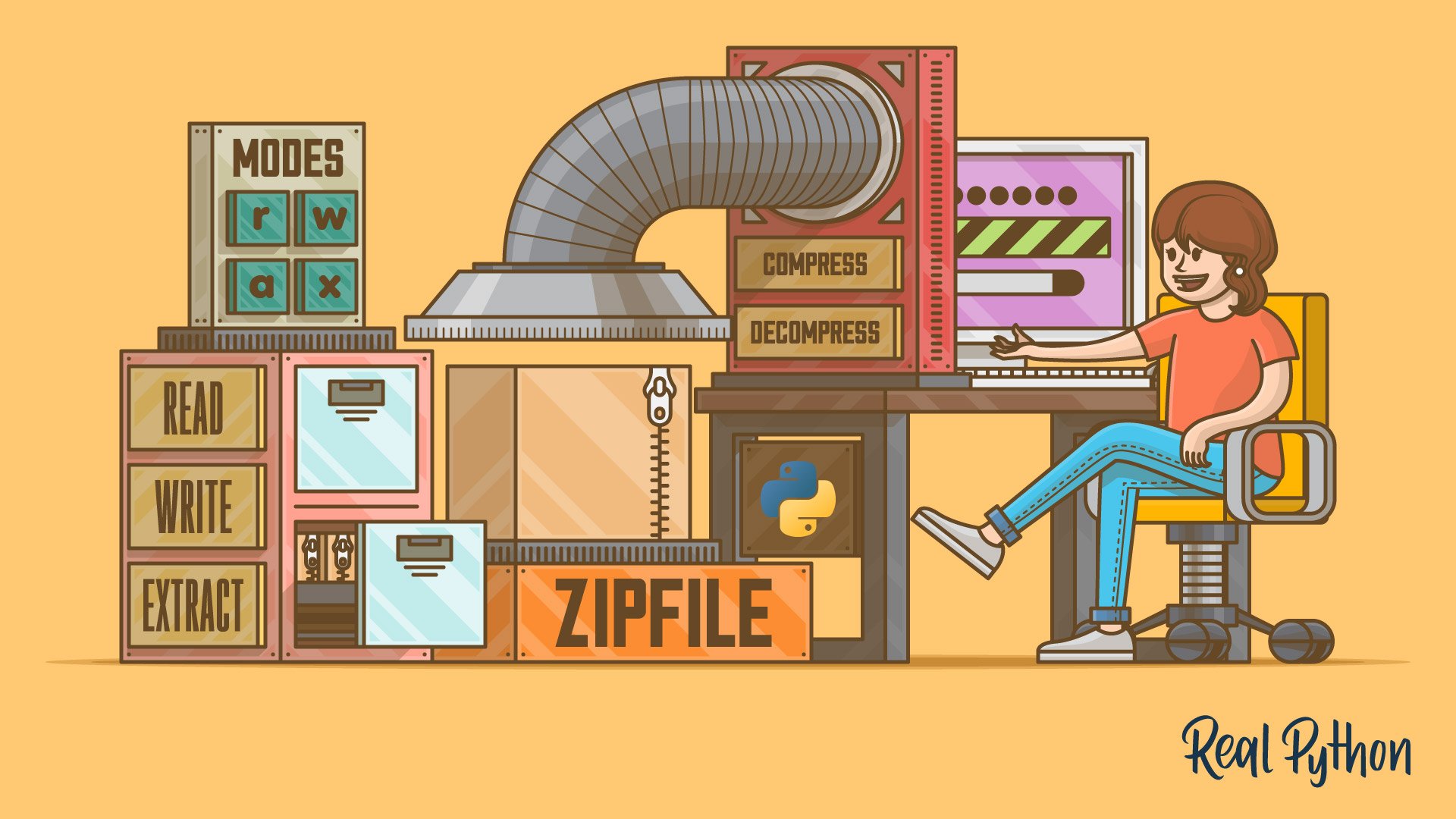
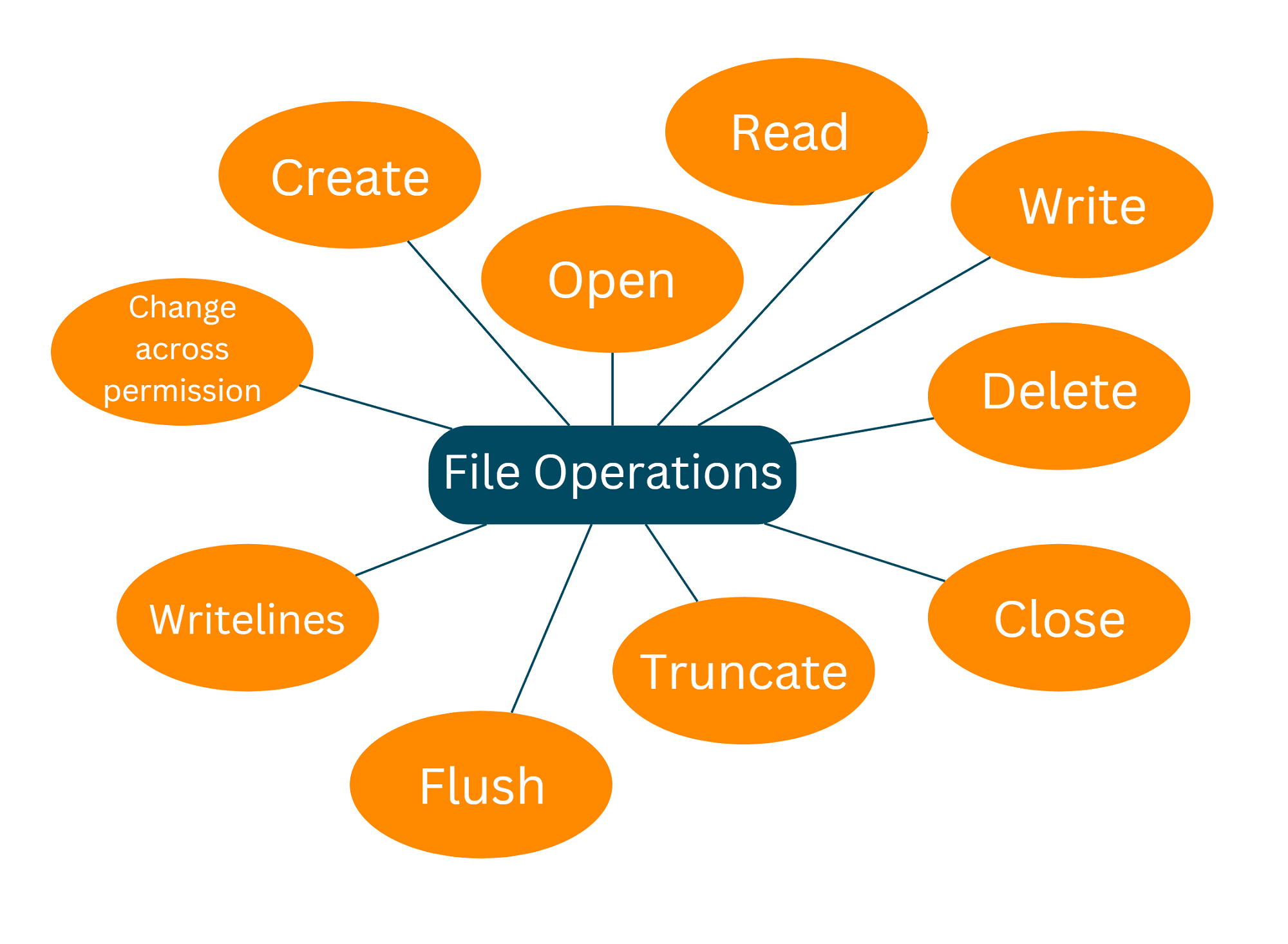
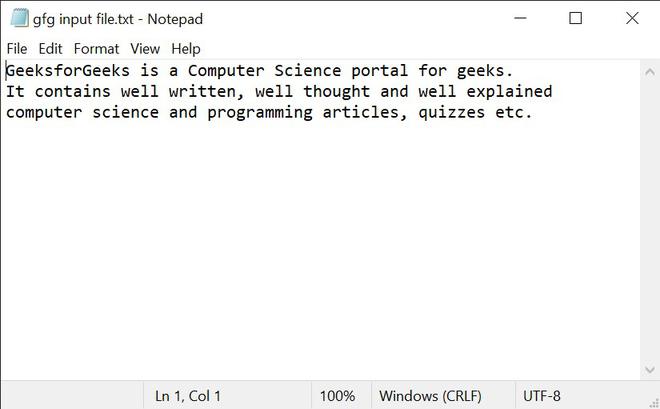
![FileNotFoundError: [Errno 2] No such file or directory – Its Linux FOSS Filenotfounderror: [Errno 2] No Such File Or Directory – Its Linux Foss](https://itslinuxfoss.com/wp-content/uploads/2022/11/Errno-2-No-such-file-or-directory-2.png)
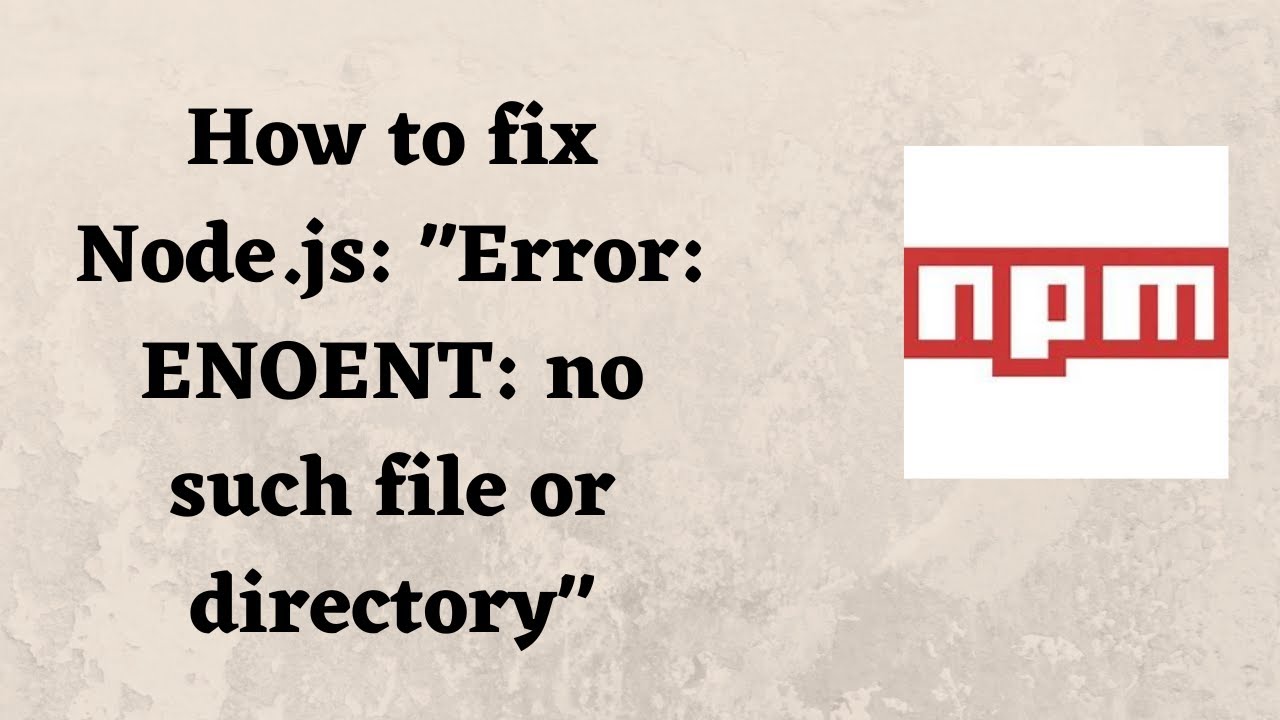
![Pythonお悩み解決】FileNotFoundError: [Errno 2] No such file or directory: を解消する方法を教えてください - Python学習チャンネル by PyQ Pythonお悩み解決】Filenotfounderror: [Errno 2] No Such File Or Directory: を解消する方法を教えてください - Python学習チャンネル By Pyq](https://cdn-ak.f.st-hatena.com/images/fotolife/A/Arty_Mireiyu/20221206/20221206123624.png)

![FileNotFoundError: [Errno 2] No such file or directory - ☁️ Streamlit Community Cloud - Streamlit Filenotfounderror: [Errno 2] No Such File Or Directory - ☁️ Streamlit Community Cloud - Streamlit](https://global.discourse-cdn.com/business7/uploads/streamlit/original/3X/6/d/6d6dd71c717a6b987a720b453a697a0437b4be5b.png)
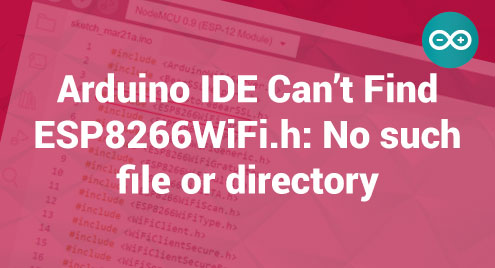

![FileNotFoundError: [Errno 2] No such file or directory – Its Linux FOSS Filenotfounderror: [Errno 2] No Such File Or Directory – Its Linux Foss](https://itslinuxfoss.com/wp-content/uploads/2022/11/Errno-2-No-such-file-or-directory-2.png)
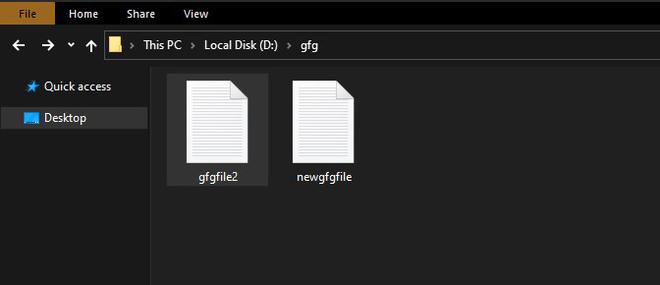
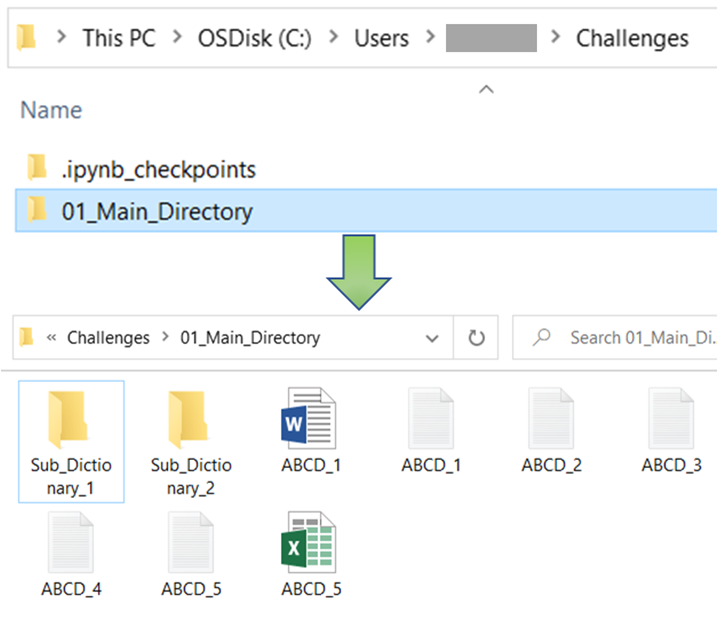
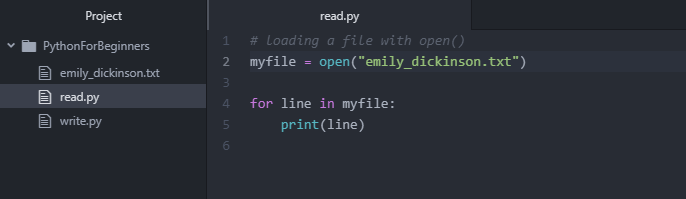

![Create File in Python [4 Ways] – PYnative Create File In Python [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/created-files.png)
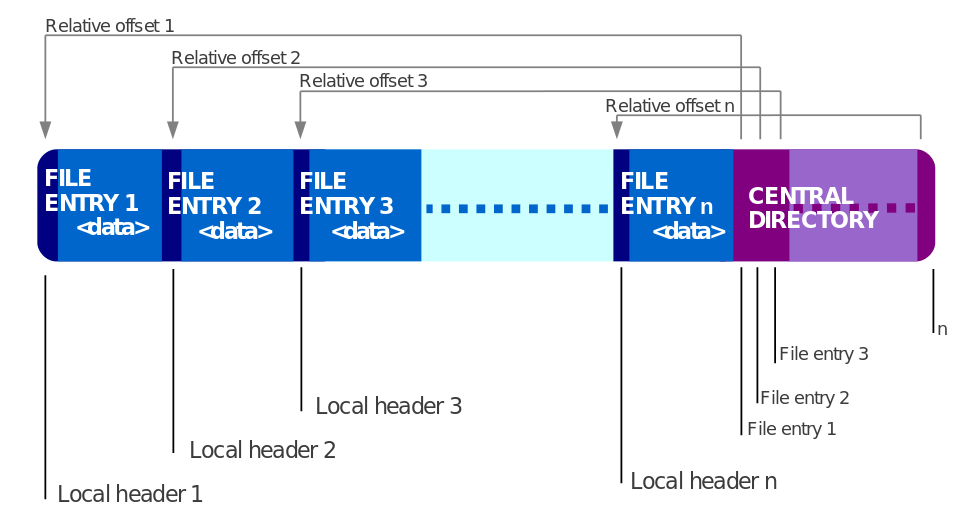


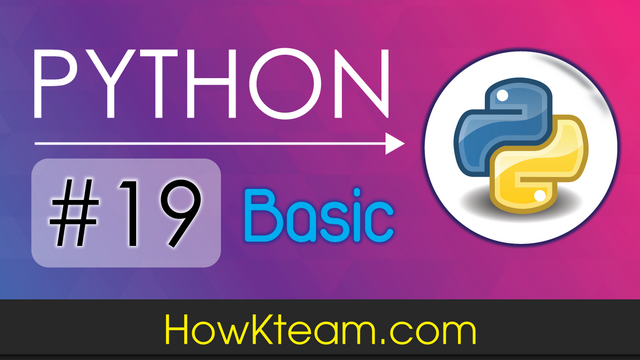
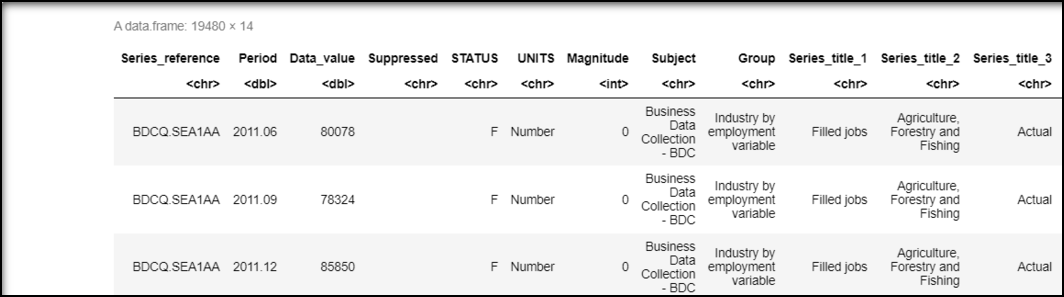
Article link: python write file no such file or directory.
Learn more about the topic python write file no such file or directory.
- open file in “w” mode: IOError: [Errno 2] No such file or directory
- How do I resolve the ‘no such file or directory’ error in Python?
- FileNotFoundError: [Errno 2] No such file or directory [Fix]
- Python FileNotFoundError: [Errno 2] No such file or directory …
- Python No Such File Or Directory – Linux Hint
- FileNotFoundError: [Errno 2] No such file or directory
- Python FileNotFoundError: [Errno 2] No such file or directory
- How to Create (Write) Text File in Python – Guru99
- Python FileNotFoundError: [Errno 2] No such file or directory …
- File Handling in Python: Create, Open, Append, Read, Write
- How to Fix FileNotFoundError: No Such File or Directory Error …
- [Errno 2] No such file or directory: ‘data/images/00018.png’
- How to Fix Python `No such file or directory` Compiler Errors …
See more: https://nhanvietluanvan.com/luat-hoc