Python Split String In Half
Introduction to Splitting a String
In the world of programming, dealing with strings is a common task. String manipulation involves separating or modifying strings to extract valuable information or perform specific operations. Splitting a string is one such operation that allows you to divide a string into smaller sections, which can be useful for various purposes.
Why Split a String in Half?
Splitting a string in half can be particularly helpful when you want to divide a string evenly into two parts. This can be beneficial for tasks such as data analysis, text processing, and even cryptography. By splitting a string in half, you can gain insights into various patterns and structures within the data.
Implementation of Splitting a String
There are multiple ways to split a string in Python, depending on the requirements of your specific task. Let’s explore some of the most commonly used methods.
1. Using String Slicing:
Python provides an elegant and straightforward way to split a string using string slicing. By specifying the start and end indices, you can extract a substring representing a specific portion of the original string. To split a string in half, you can use the length of the string and divide it by 2 to find the midpoint. Then, you can create two new strings by slicing the original string accordingly.
2. Utilizing the split() Method:
Another option is to leverage the split() method, which divides a string into substrings based on a specified delimiter. By default, this method splits the string using whitespace as the delimiter. To split a string in half, you can utilize this method along with a custom delimiter that represents the midpoint of the string.
3. Dealing with Odd-Length Strings:
When dealing with strings of odd length, splitting them in half can present a challenge. In such cases, you can choose to include an extra character in one of the halves or divide the string with one additional character on the longer side. The approach depends on the specific requirements of your task and the desired outcome.
Examples of Splitting a String in Half
Now, let’s explore some examples to better understand how to split a string in half using Python.
1. Splitting a String with Even Length:
For strings with an even length, the splitting process is straightforward. Using either the string slicing or split() method, you can accurately divide the string into two equal halves.
2. Splitting a String with Odd Length:
When the length of a string is odd, there are different ways to split it in half. Depending on the requirements, you can choose to include an extra character in one of the halves or divide the string with one additional character on the longer side.
3. Splitting a String with Delimiters:
Sometimes, splitting a string involves dividing it into parts based on specific delimiters or markers. For example, you may split a sentence into words based on spaces or split a comma-separated list into individual elements. By customizing the delimiter used with the split() method or adapting the slicing approach, you can achieve the desired outcome.
Tips and Tricks for Splitting Strings in Python
Here are some tips and tricks to enhance your string splitting capabilities in Python:
1. Handling Whitespace and Punctuation:
When splitting strings, it’s essential to consider whitespace and punctuation. You may need to remove or handle these elements separately, depending on your task. Python provides various methods and functions that can help you achieve this, such as strip(), translate(), and regular expressions.
2. Dealing with Empty Strings:
While splitting a string, you may encounter cases where the string is empty or contains no meaningful content. In such situations, it’s important to handle these cases gracefully and consider appropriate error handling or fallback mechanisms.
3. Customizing Splitting Behavior:
Python offers flexibility in customizing the behavior of string splitting operations. You can experiment with different delimiters, change the splitting pattern, or modify the slicing indices according to your specific requirements.
4. Performance Considerations:
When working with large strings or performing intensive string splitting operations, it’s crucial to consider performance. Choosing an efficient algorithm or technique can significantly impact the execution time of your code. Additionally, using appropriate data structures and optimizing your code can help improve performance.
Conclusion
Splitting a string in half is a valuable technique in Python that enables you to process and manipulate strings more effectively. By using methods like string slicing and the split() method, you can easily divide a string into two equal parts or based on specific delimiters. The flexibility and versatility of Python’s string manipulation capabilities make it a powerful language for handling textual data.
FAQs
Q1. How do I split a string in half in JavaScript?
In JavaScript, you can split a string in half using similar techniques as in Python. You can use string slicing or the substring() method to extract the desired portions of the string based on the length.
Q2. Can I split a string in half in C++?
In C++, you can split a string in half using a variety of approaches. You can use string substr() or the substring() function along with length calculations to achieve the desired outcome.
Q3. How can I split a string into two parts in Python?
You can split a string into two parts in Python by using string slicing. Calculate the midpoint of the string length and create two new strings representing the split halves.
Q4. How do I print the second half of a string in Python?
To print the second half of a string in Python, you can use string slicing. Calculate the midpoint of the string length and print the substring from the midpoint to the end of the string.
Q5. How can I split a string in Python based on its length?
To split a string in Python based on its length, you can use string slicing or the split() method. By iterating over the string and extracting substrings of the desired length, you can achieve the desired outcome.
Q6. How do I split a string into two equal parts in Python?
To split a string into two equal parts in Python, you can use string slicing. Calculate the midpoint of the string length and create two new strings representing the split halves.
Q7. How do I split a string in Python by a specific index?
To split a string in Python by a specific index, you can use string slicing. Specify the start and end indices based on the desired split point to extract the respective portions of the string.
How To Split Strings In Python With The Split() Method
Keywords searched by users: python split string in half split string in half javascript, split string in half c++, Split string into 2 parts python, how to print the second half of a string in python, Python Split string by length, half string, string 2 python, Split string python by index
Categories: Top 39 Python Split String In Half
See more here: nhanvietluanvan.com
Split String In Half Javascript
In JavaScript, splitting a string in half can be accomplished by various methods depending on the specific requirements and the desired outcome. This article will explore the different approaches, their advantages, and limitations, providing you with a comprehensive understanding of split string in half functionality in JavaScript.
Split String in Half by Character Count
One way to split a string in half is by dividing it into two equal parts based on the character count. This technique works well when the string length is known, and the goal is to split it into two equal halves.
To achieve this, we can use the `substring()` method to extract the desired portions. Here’s an example:
“`javascript
const str = “Hello World!”;
const halfLength = Math.floor(str.length / 2);
const firstHalf = str.substring(0, halfLength);
const secondHalf = str.substring(halfLength);
console.log(firstHalf); // Output: “Hello ”
console.log(secondHalf); // Output: “World!”
“`
In this example, the `substring()` method is used to extract the substrings. The `halfLength` variable stores the character count of half the string length (as an integer value) using the `Math.floor()` function to ensure division by two results in an integer. The `substring()` method is then invoked twice: Once to retrieve the first half of the string by specifying the starting and ending index, and again to obtain the second half by specifying only the starting index.
Split String in Half by Word Count
Sometimes, splitting a string in half based on the number of words may be preferable. This approach is useful when the goal is to divide a sentence or paragraph into two equal halves without considering the individual characters.
To accomplish this, we can utilize the `split()` method to split the string into an array of words. We then divide this array in half and join the words back together to form two separate strings. Here’s an example:
“`javascript
const str = “JavaScript is a versatile programming language that runs on the web.”;
const words = str.split(” “);
const halfLength = Math.floor(words.length / 2);
const firstHalf = words.slice(0, halfLength).join(” “);
const secondHalf = words.slice(halfLength).join(” “);
console.log(firstHalf); // Output: “JavaScript is a versatile”
console.log(secondHalf); // Output: “programming language that runs on the web.”
“`
In this example, the `split()` method is used to split the original string into an array of words based on the space delimiter. The `slice()` method is then applied to create a new array containing the desired halves, which are joined back together using the `join()` method to form separate strings representing the first and second halves.
FAQs:
Q: Can I split a string in half, considering specific characters?
A: Yes, you can. By specifying the starting and ending delimiter (characters) with the `indexOf()` and `lastIndexOf()` methods, you can split the string into two portions.
Q: What happens if the string length is odd when splitting by character count?
A: In such cases, the first half will always have one less character than the second half. For example, splitting the string “Hello” would yield “Hel” and “lo”.
Q: Is it possible to split a string into more than two portions?
A: Certainly! By adjusting the parameters and implementing looping or recursion mechanisms, you can split a string into multiple portions.
Q: Are there any limitations or performance concerns with splitting large strings?
A: Splitting large strings can be memory-intensive since new strings are created. It is essential to consider memory usage when dealing with significant amounts of data to avoid potential performance issues.
Q: Can I split a string into halves without using the Math object?
A: Yes, alternatively, you can divide the string length by two using the truncation operator (`str.length / 2 | 0`) or bitwise right shift operator (`str.length >> 1`) instead of the `Math.floor()` function.
In conclusion, JavaScript offers various approaches to split a string in half. Depending on the specific requirements, you can choose between dividing it by character count or word count. By understanding these techniques, you can manipulate strings efficiently and achieve your desired results.
Split String In Half C++
When working with strings in C++, it is often necessary to manipulate or extract certain parts of the string. One common requirement is splitting a string in half, which divides the string into two equal parts. Splitting a string in half can be useful in various scenarios, such as dividing a line of text into two separate lines, extracting values from a particular part of the string, or performing further operations on the individual parts.
In this article, we will explore different approaches to splitting a string in half in C++. We will cover both traditional methods and some modern tricks using the built-in string manipulation functions and operations available in C++.
Traditional Method: Using Substring Function and Indexing
One straightforward way to split a string in half is through the use of the substring function and indexing. The substring function allows us to extract a portion of the original string based on a starting position and a length. By calculating the midpoint of the string, we can use it as the starting position for the substring function.
Consider the following code snippet:
“`cpp
#include
#include
int main() {
std::string input = “Hello World”;
int midpoint = input.length() / 2;
std::string firstHalf = input.substr(0, midpoint);
std::string secondHalf = input.substr(midpoint);
std::cout << "First Half: " << firstHalf << std::endl;
std::cout << "Second Half: " << secondHalf << std::endl;
return 0;
}
```
In this example, we start by defining the input string, "Hello World." We then calculate the midpoint by dividing the length of the input string by 2. Next, we extract the first half of the string by calling the substr function with a starting position of 0 and a length equal to the calculated midpoint. Similarly, the second half is extracted by using substr with a starting position equal to the midpoint and no specified length. Finally, we print the first and second halves to the console.
Modern Method: Using String View
C++17 introduced a new feature called string view, which provides a non-owning, constant view of a sequence of characters. This feature allows for efficient manipulation and processing of strings without the need for unnecessary memory allocations or copying. We can employ string view to split a string in half as well.
The code snippet below demonstrates splitting a string using string view:
```cpp
#include
#include
int main() {
std::string_view input = “Hello World”;
std::string_view firstHalf = input.substr(0, input.length() / 2);
std::string_view secondHalf = input.substr(input.length() / 2);
std::cout << "First Half: " << firstHalf << std::endl; std::cout << "Second Half: " << secondHalf << std::endl; return 0; } ``` In this example, we make use of the string_view class instead of the traditional string class. The string_view allows us to take "views" or references to sections of the original string without the need for copying or allocation. The substr function in the string_view class operates similarly to the string class, providing the ability to extract portions of the string. The resultant firstHalf and secondHalf string_views will reflect the desired splits accordingly. FAQs Q: Can I split a string into more than two parts? A: Yes, you can split a string into multiple parts by applying the same logic repeatedly. Instead of extracting just two halves, you can recursively split the string until you achieve the desired number of parts. Q: What happens if the input string has an odd length? A: In both the traditional and modern approaches mentioned above, the resulting first half will always contain the extra character (if the input string has an odd length). For instance, if the input string is "Hello", the first half will be "Hel" and the second half will be "lo". Q: Can I split a string based on a specific delimiter instead of splitting it in half? A: Yes, instead of splitting a string in half, you can split it based on a specific delimiter using the string manipulation functions available in C++, such as the find and substr functions. These functions can help locate the position of the delimiter and then extract the desired portions. Q: Are there any performance differences between the traditional method and the modern method? A: In terms of functionality, both methods achieve the same outcome. However, the modern method using string view offers potential performance benefits due to reduced memory overhead and improved efficiency. The traditional method may involve additional memory allocations and copying operations, which can impact performance, especially when dealing with large strings. In conclusion, splitting a string in half is a common task in C++ programming. We explored two methods to accomplish this: the traditional method using substring function and indexing, and the modern approach using the string view feature. By understanding these techniques, you can efficiently split strings and perform further operations on the individual parts as needed.
Images related to the topic python split string in half
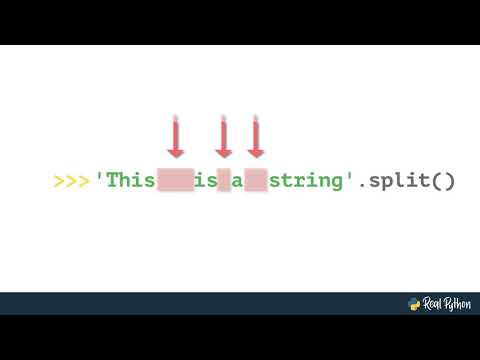
Found 39 images related to python split string in half theme
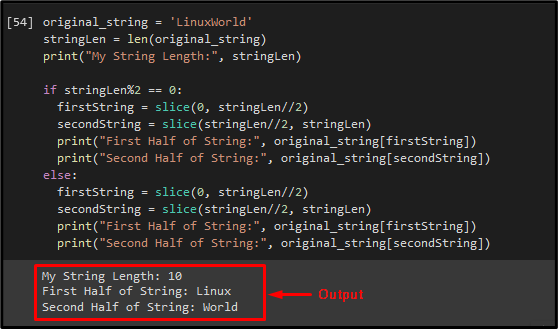


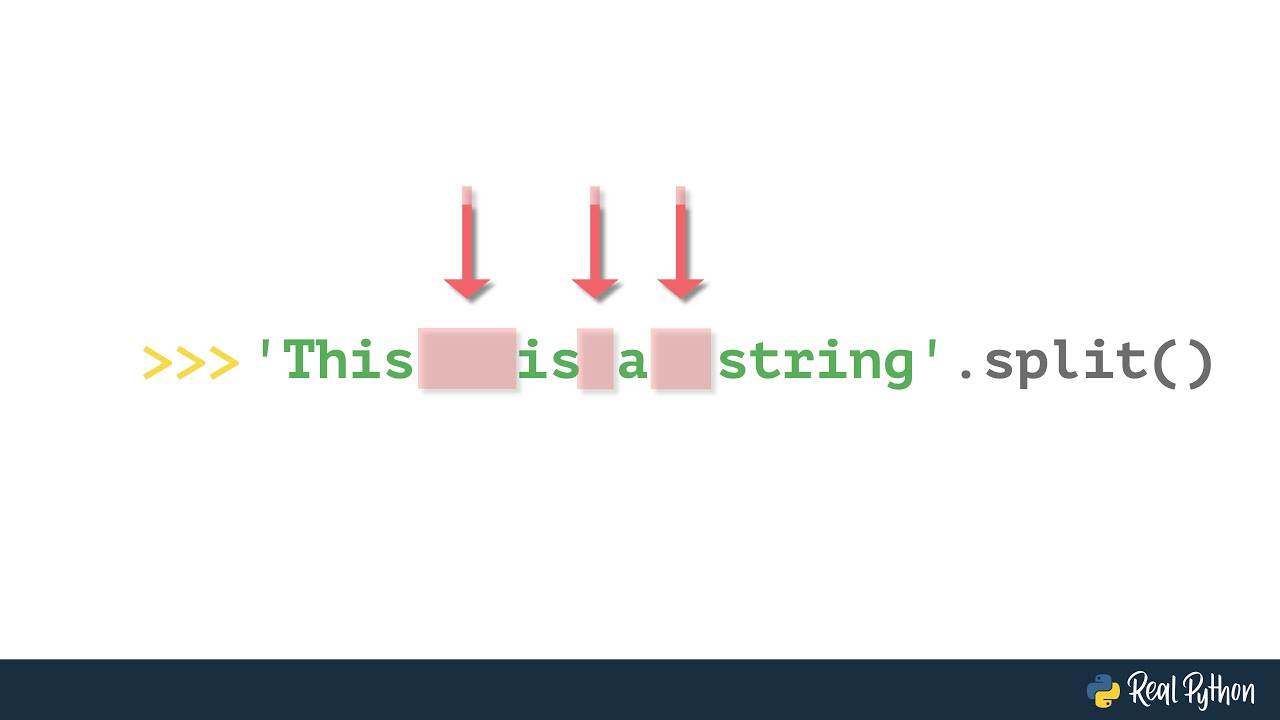

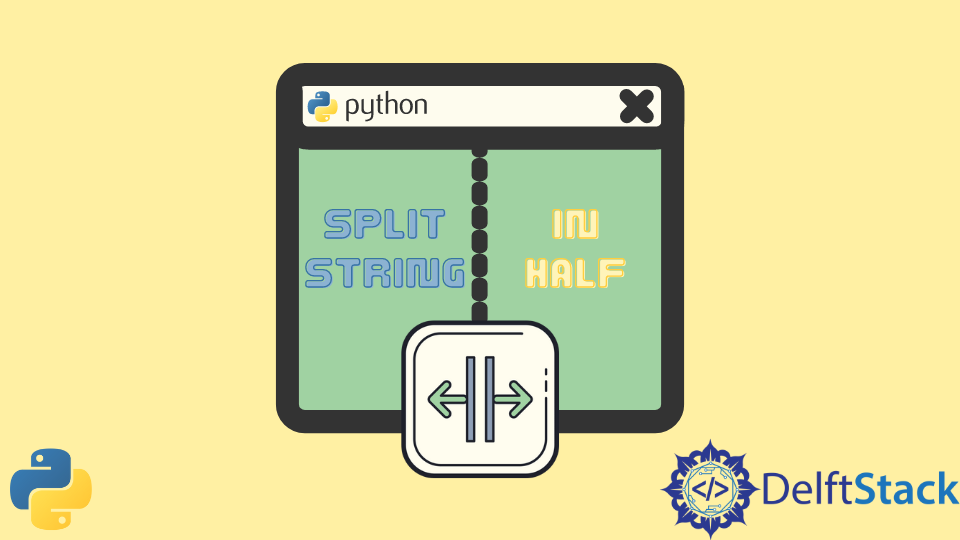
![How To Split A String By Index In Python [5 Methods] - Python Guides How To Split A String By Index In Python [5 Methods] - Python Guides](https://i0.wp.com/pythonguides.com/wp-content/uploads/2023/04/How-to-split-a-string-by-index-in-python.jpg)

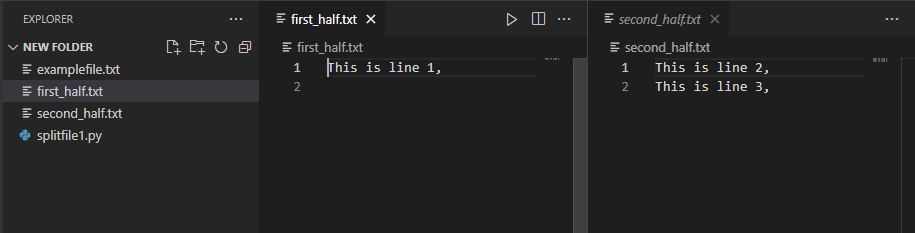
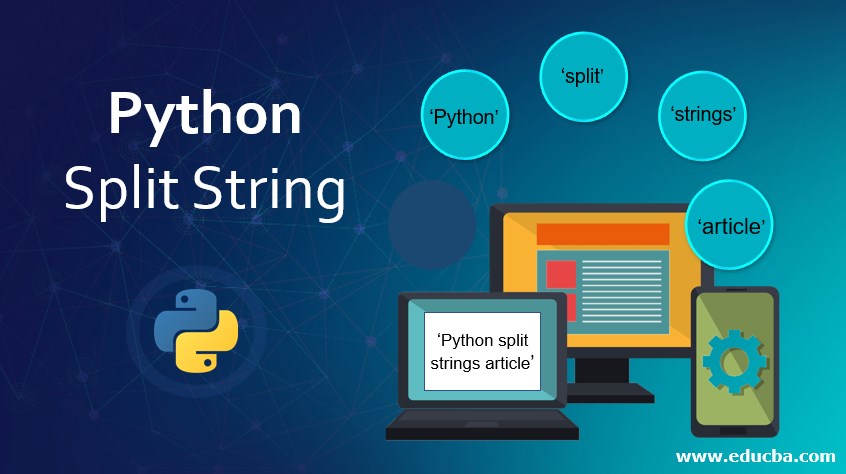
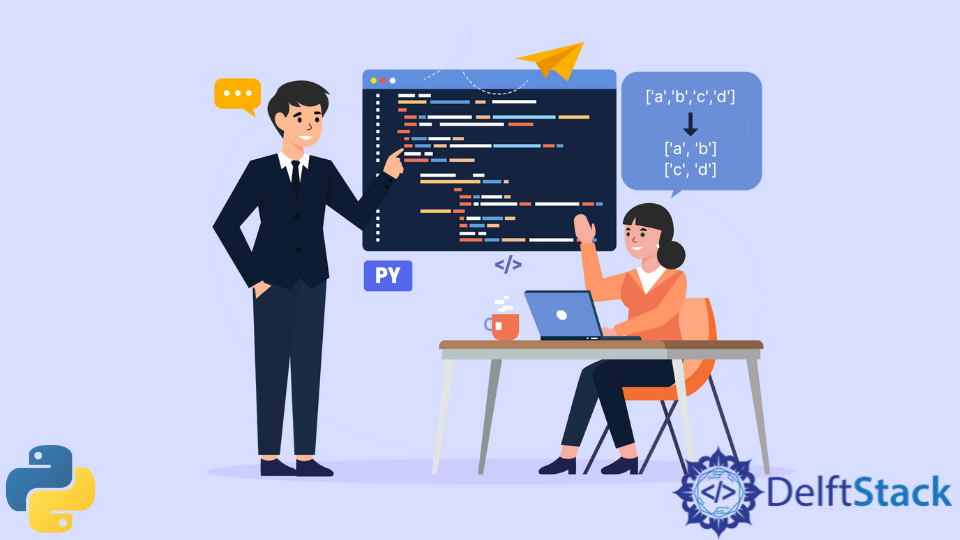
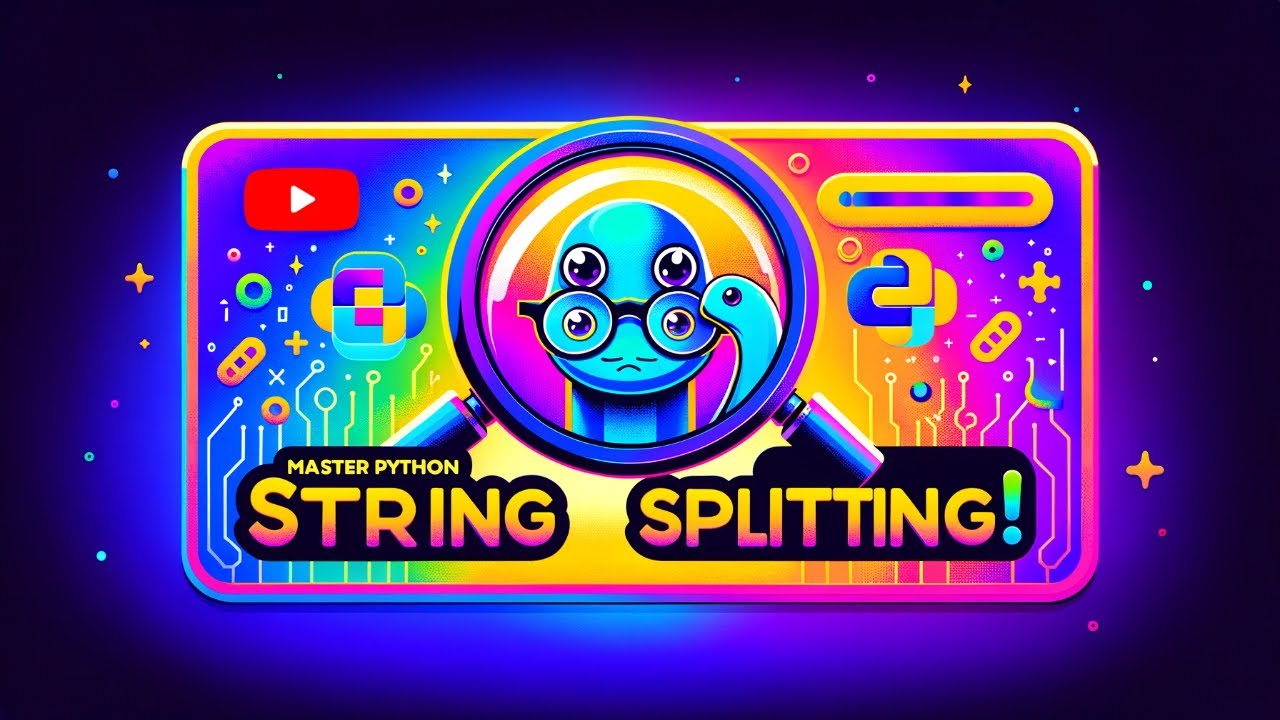
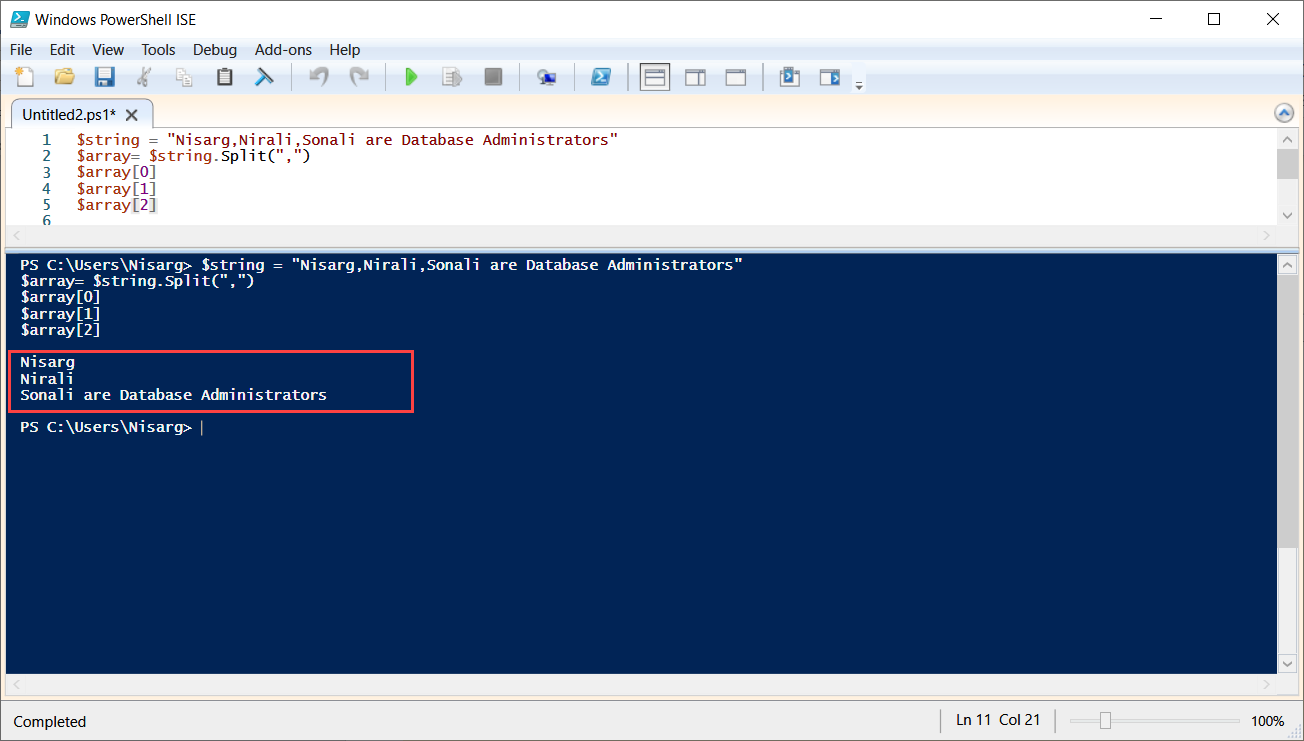
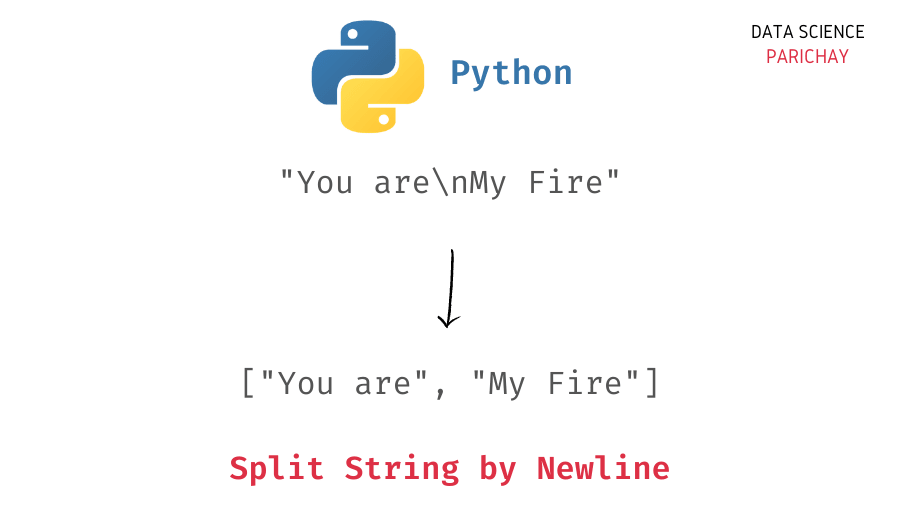
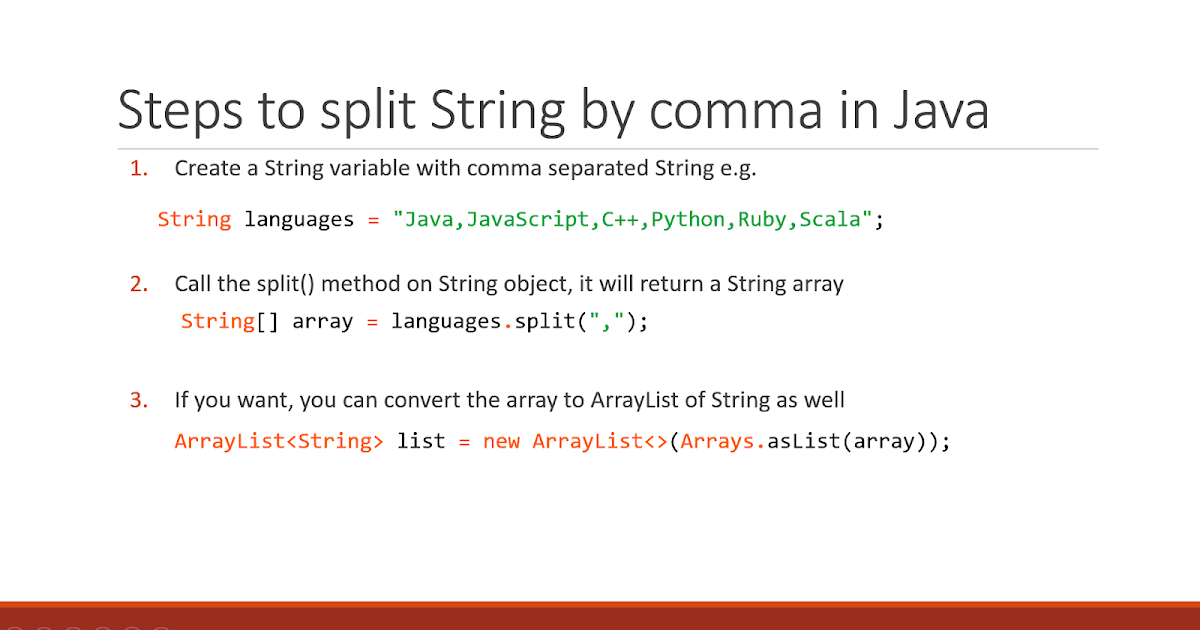

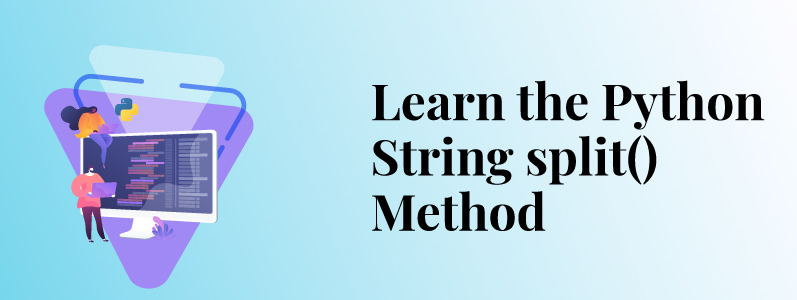
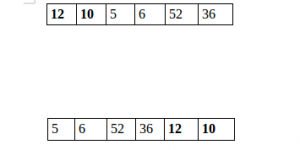
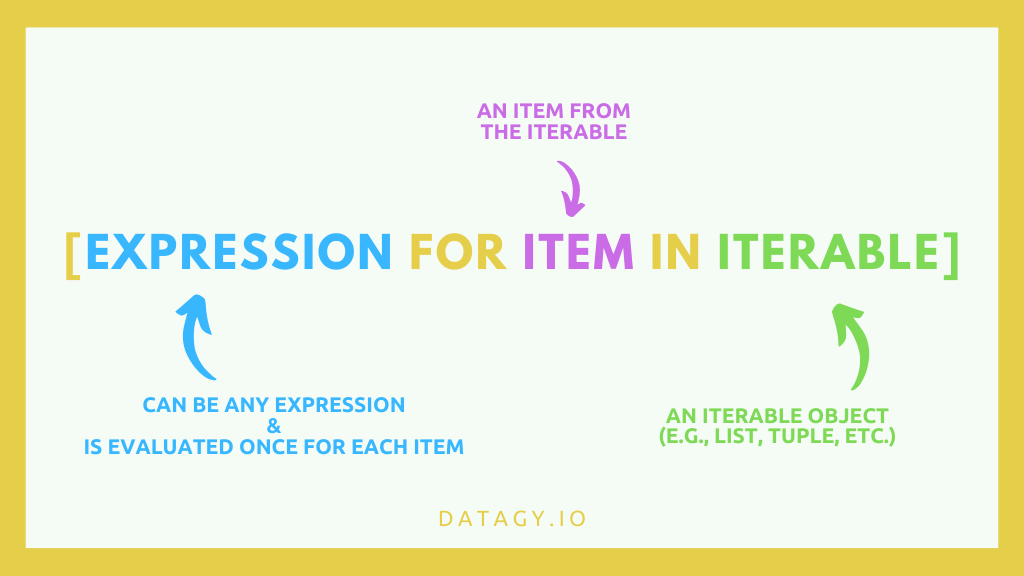
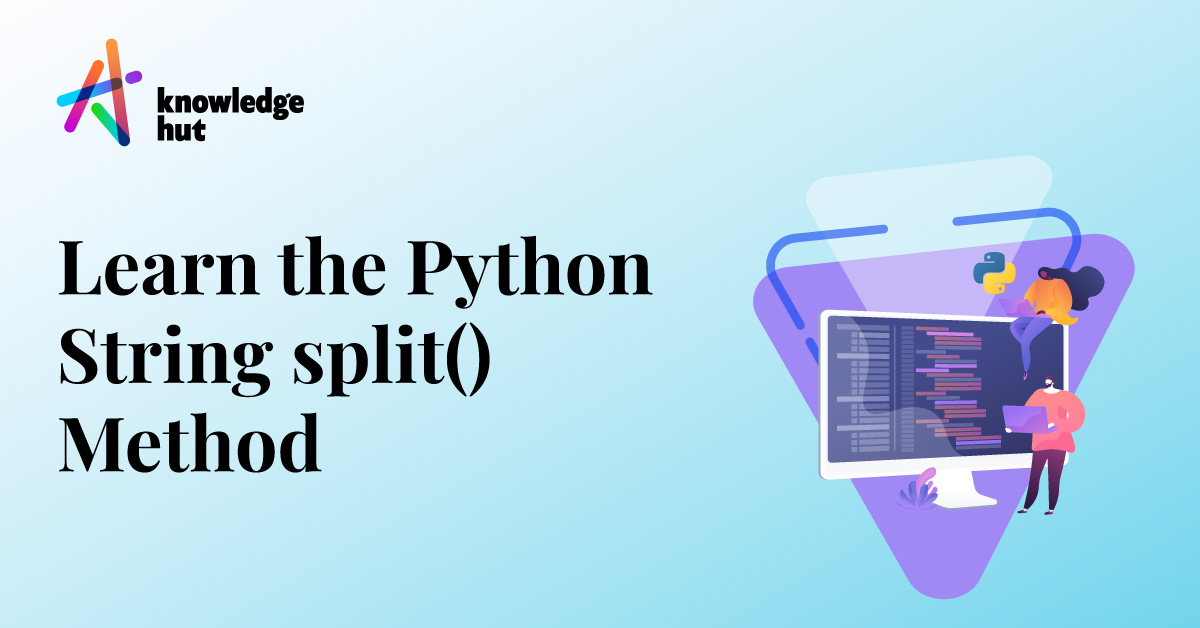
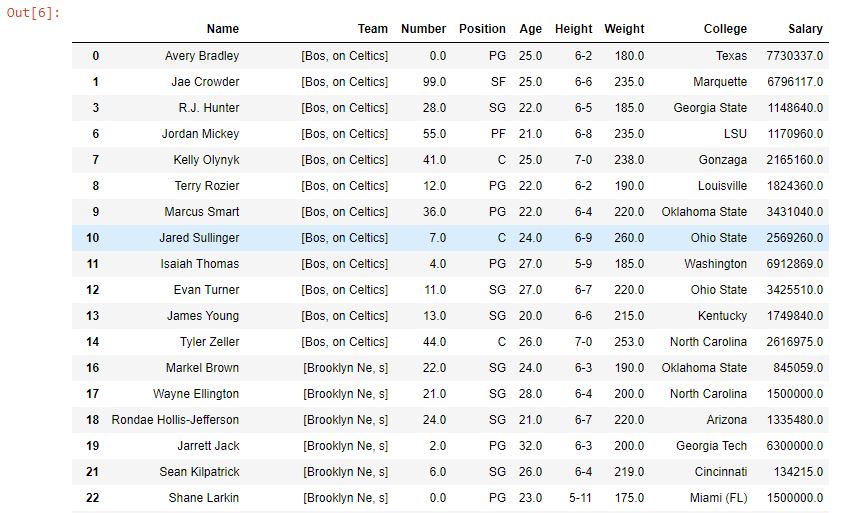
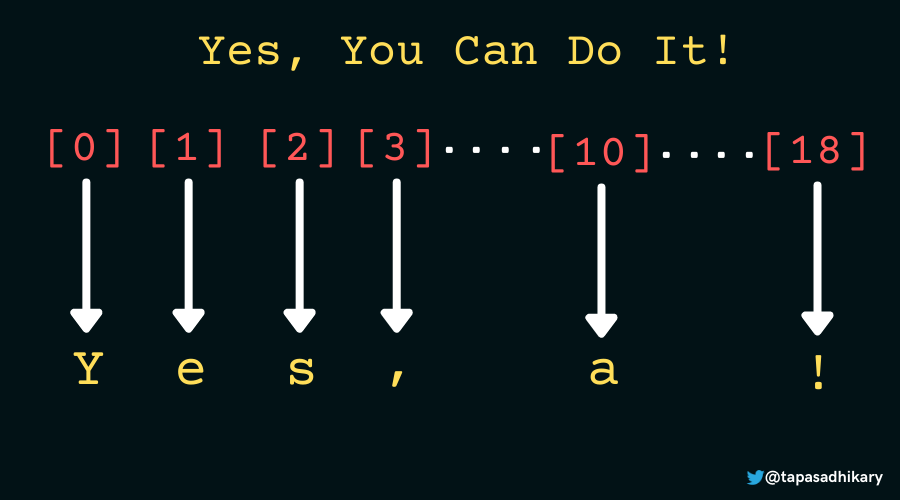
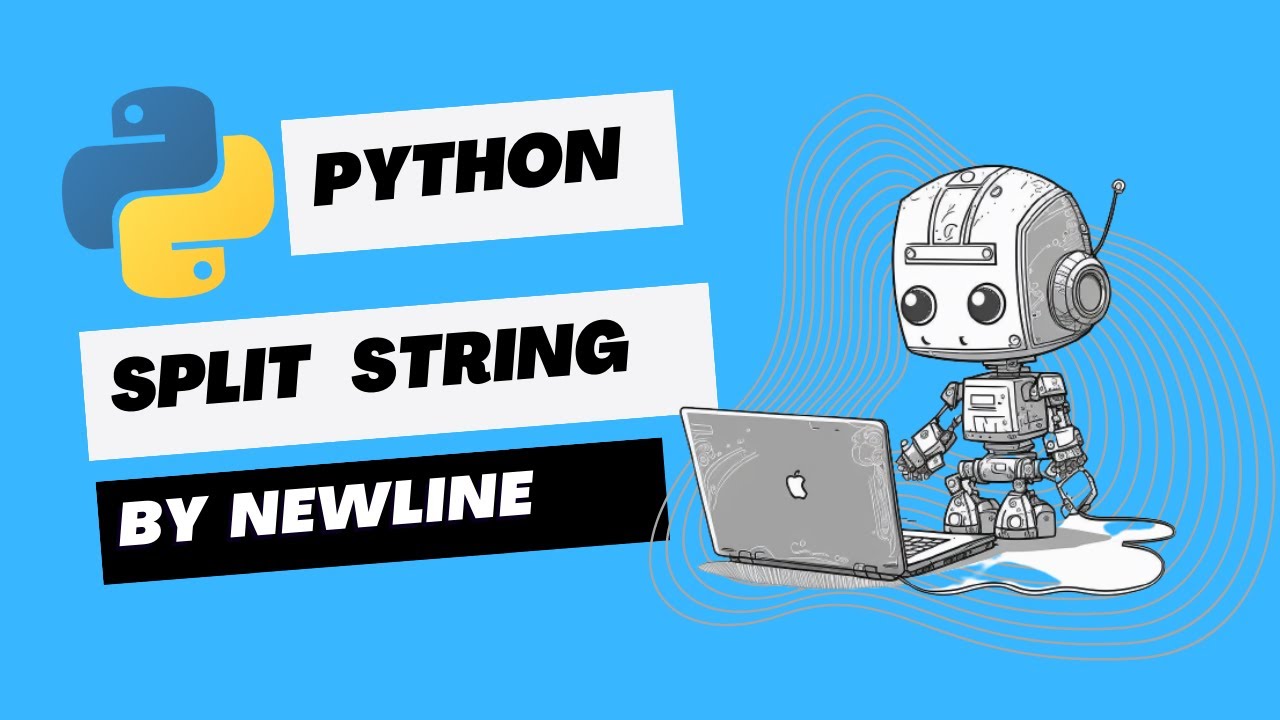
![How to Split Strings in Pandas: The Beginner's Guide [+ Examples] How To Split Strings In Pandas: The Beginner'S Guide [+ Examples]](https://blog.hubspot.com/hs-fs/hubfs/Google%20Drive%20Integration/Pandas%20Split%20String%20(V4)-3.png?width=531&height=215&name=Pandas%20Split%20String%20(V4)-3.png)
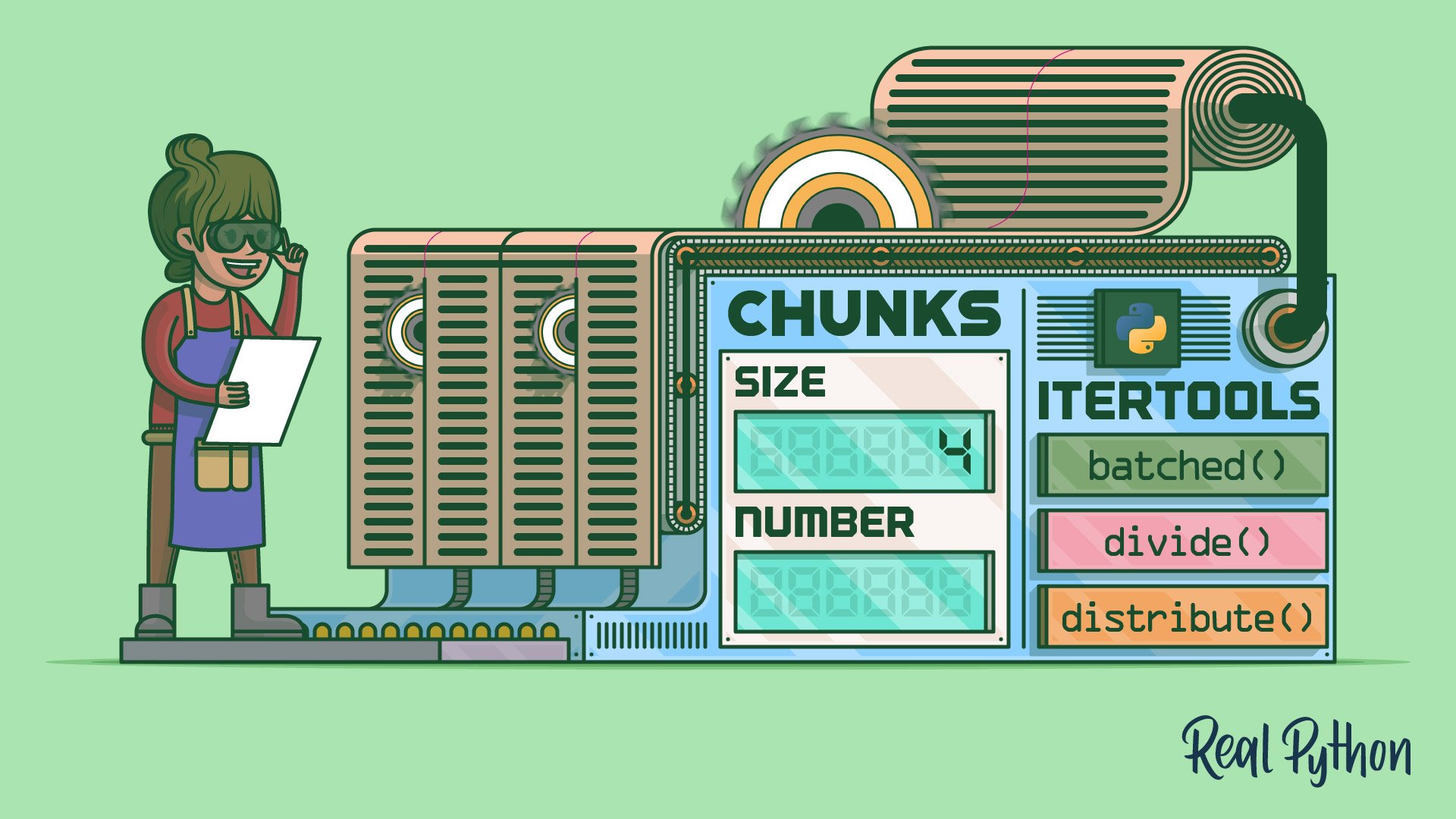
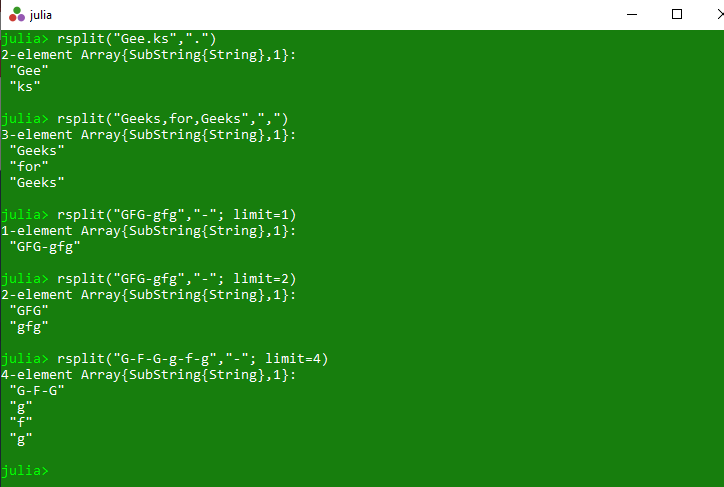
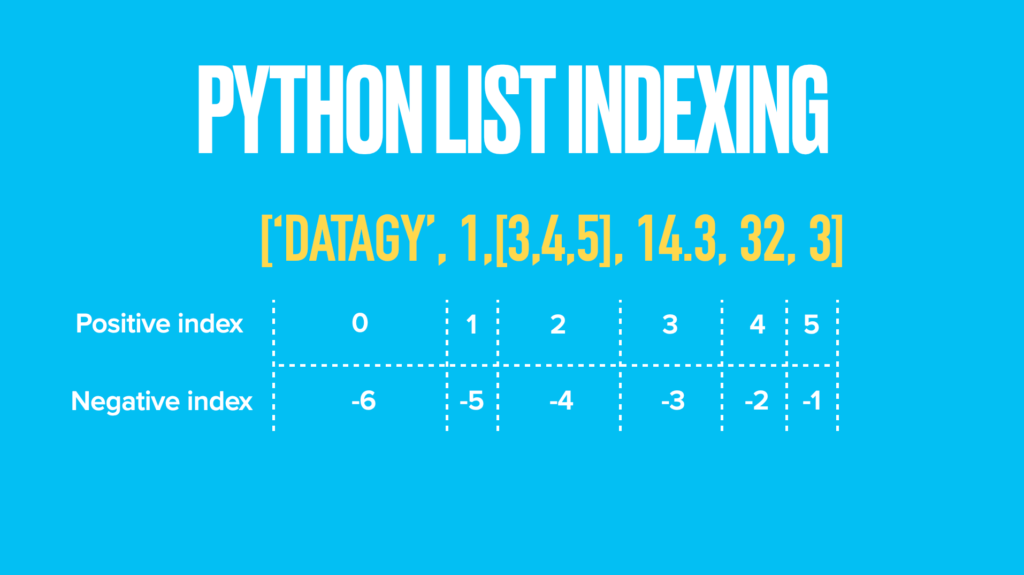

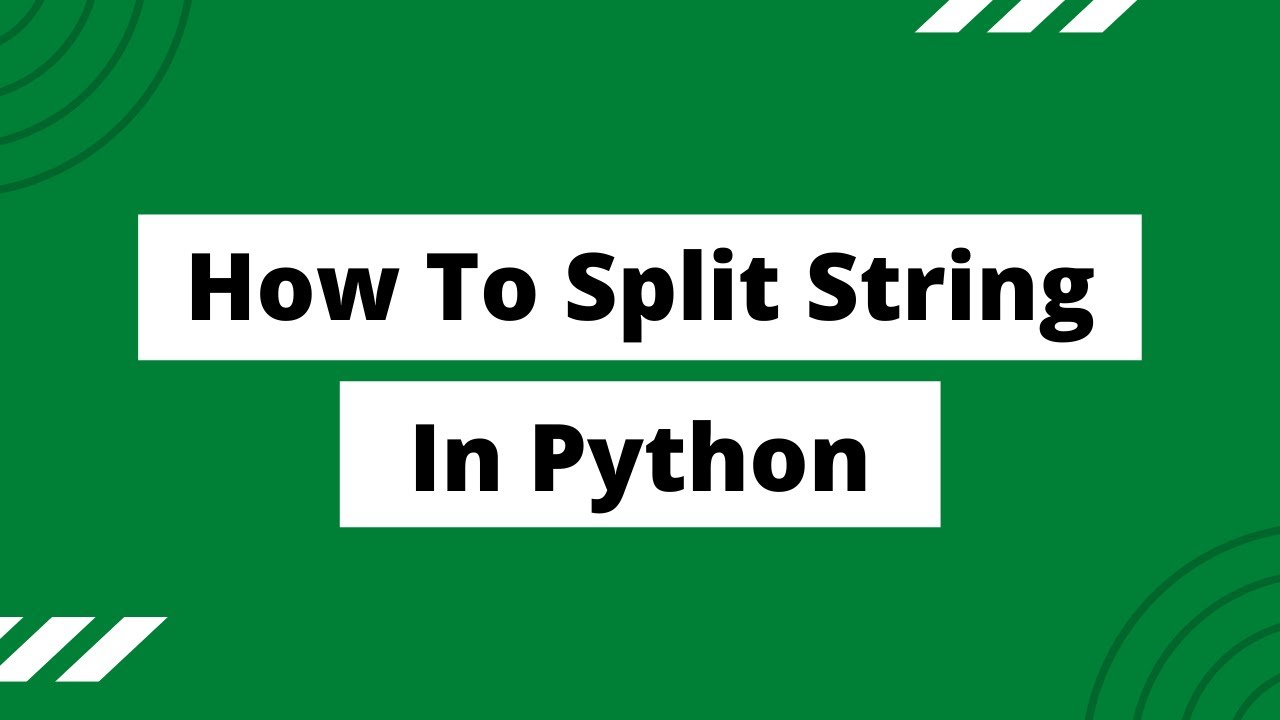
![How to Split Strings in Pandas: The Beginner's Guide [+ Examples] How To Split Strings In Pandas: The Beginner'S Guide [+ Examples]](https://blog.hubspot.com/hubfs/pandas-split-string-598px.png)
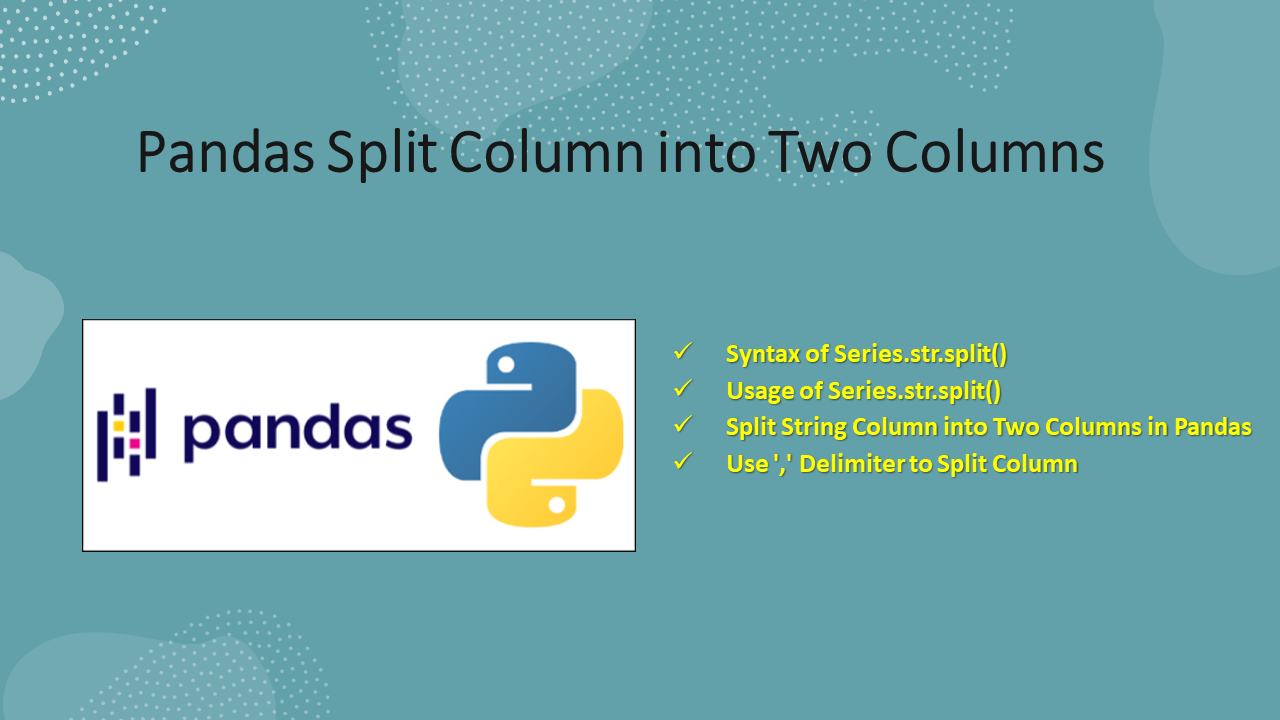
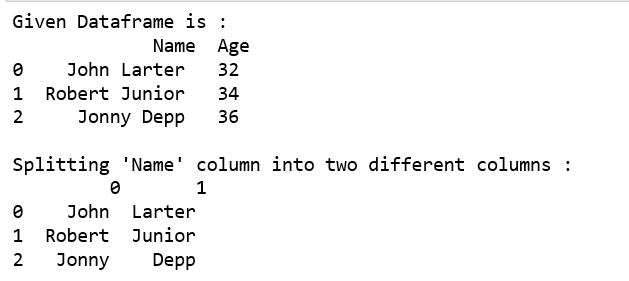
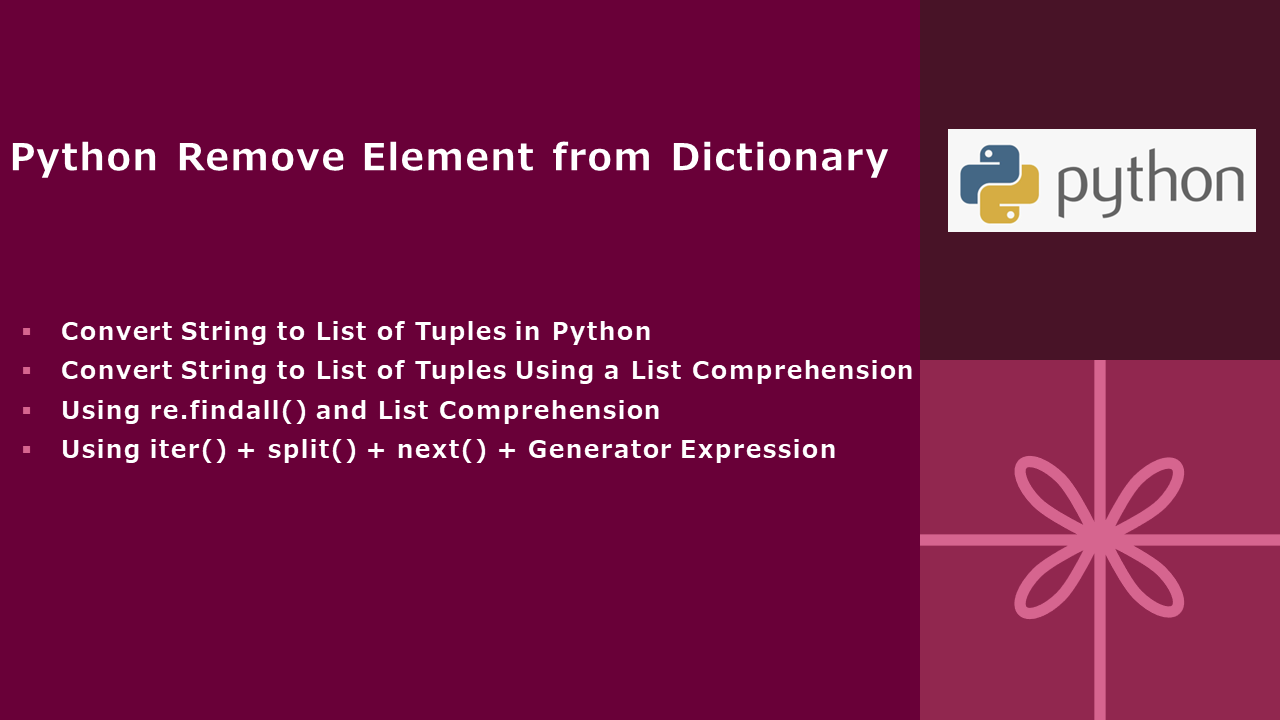
Article link: python split string in half.
Learn more about the topic python split string in half.
- Python Split a String in Half – Linux Hint
- Split a string into 2 in Python – Stack Overflow
- Python | Split String in Half – Be on the Right Side of Change
- 3 Ways to Python Split a String in Half
- Split String in Half in Python | Delft Stack
- Python | Split given string into equal halves – GeeksforGeeks
- How to split a string into two halves in Python?
- A Guide on Split () Function in Python – KnowledgeHut
- Python Split String – How to Split a String into a List or Array in …
- Python String split() Method – W3Schools
See more: https://nhanvietluanvan.com/luat-hoc