Python Print To Stderr
Overview
When working with Python, there are times when you may need to print output to the standard error (stderr) stream instead of the standard output (stdout) stream. In this article, we will explore what stderr is and why you would want to print to it. We will also discuss how to use the sys module to print to stderr, how to redirect stderr to a file or variable, and how to handle exceptions and print error messages to stderr. Additionally, we will cover best practices for using stderr and common use cases for printing to stderr. Finally, we will briefly touch on the use of stderr in popular third-party libraries and frameworks.
What is stderr?
In Python, stderr refers to the standard error stream. It is a default output stream that is used to display error messages and diagnostic information. Unlike the standard output stream (stdout), which is typically used for regular output, stderr is specifically designed for error-related messages.
Why would you want to print to stderr?
Printing to stderr instead of stdout can be useful in many situations. Here are a few common reasons why you might choose to print to stderr:
1. Distinguishing error messages: By printing error messages to stderr, you can easily differentiate them from regular output. This can make it easier for users or developers to identify and address issues.
2. Error logging: Redirecting error messages to a log file allows for easy tracking and analysis of errors. This can be especially important in production environments where monitoring and debugging are necessary.
3. Debugging and troubleshooting: When developing or debugging code, printing debugging information or error messages to stderr can provide valuable insights into the state of the program and help identify the source of issues.
Using the sys module
The sys module in Python provides access to system-specific parameters and functions. It allows us to interact with the stderr stream and print messages to it.
Importing the sys module
To use the sys module, we need to import it at the beginning of our Python script using the following statement:
“`python
import sys
“`
Accessing the stderr object
The sys module provides a variable called “stderr,” which represents the stderr stream. This stream can be directly accessed and used for writing error messages.
Printing to stderr using sys.stderr.write()
To print a message to stderr, we can use the write() method of the sys.stderr object. Here’s an example:
“`python
sys.stderr.write(“This is an error message.\n”)
“`
The message will be written to stderr, and if the program is run in a terminal, it will be displayed as an error message.
Flushing the stderr buffer
By default, stderr is a buffered stream, meaning the messages are stored in a buffer before being displayed. To ensure that the error messages are immediately printed, we can flush the stderr buffer using the flush() method:
“`python
sys.stderr.flush()
“`
Redirecting stderr
In addition to printing to stderr, Python allows us to redirect the stderr stream to other destinations, such as a file or a variable.
Changing the default output stream
By default, both stdout and stderr are displayed on the terminal. However, we can change the default output stream to redirect stderr to a different output location. Consider the following example:
“`python
import sys
# Redirect stderr to stdout
sys.stderr = sys.stdout
# Now both stdout and stderr will be displayed together
print(“This is a regular message.”)
print(“This is an error message.”, file=sys.stderr)
“`
In this example, we redirect stderr to stdout, so both the regular message and the error message will be displayed together in the terminal.
Redirecting stderr to a file
To redirect stderr to a file, we need to open a file object in write mode and assign it to sys.stderr. Here’s an example:
“`python
import sys
# Open a file in write mode
error_file = open(“error.log”, “w”)
# Redirect stderr to the file object
sys.stderr = error_file
# Now any messages written to stderr will be stored in the file
sys.stderr.write(“This is an error message.\n”)
# Close the file
error_file.close()
“`
In this example, the error message will be written to a file called “error.log” instead of being displayed on the terminal.
Redirecting stderr to a variable
In some cases, you may want to capture the error messages in a variable rather than redirecting them to a file. To do this, we can use the StringIO class from the io module. Here’s an example:
“`python
import sys
import io
# Create a StringIO object
error_buffer = io.StringIO()
# Redirect stderr to the StringIO object
sys.stderr = error_buffer
# Now any messages written to stderr will be stored in the buffer
sys.stderr.write(“This is an error message.\n”)
# Get the contents of the buffer
error_message = error_buffer.getvalue()
# Print the error message
print(error_message)
# Close the buffer
error_buffer.close()
“`
In this example, the error message will be stored in the `error_buffer` variable, and we can retrieve its contents using the `getvalue()` method.
Exception handling
When writing code, it’s common to encounter exceptions or errors. Python provides the try-except block for handling exceptions and printing error messages to stderr.
Using try-except blocks to catch exceptions
To catch and handle exceptions, we wrap the code that might raise an exception in a try block and specify how to handle the exception(s) in one or more except blocks. Here’s an example:
“`python
try:
# Code that might raise an exception
result = 10 / 0
except ZeroDivisionError:
# Handle the specific exception
sys.stderr.write(“Error: Division by zero.\n”)
“`
In this example, we try to divide the number 10 by zero, which raises a ZeroDivisionError. We catch the error and print an error message to stderr.
Printing error messages to stderr
When handling exceptions, it’s important to provide clear and informative error messages to help users or developers understand the issue. We can use the sys.stderr.write() method to print specific error messages to stderr. Here’s an example:
“`python
try:
# Code that might raise an exception
result = open(“nonexistent_file.txt”)
except FileNotFoundError:
# Handle the specific exception
sys.stderr.write(“Error: File not found.\n”)
“`
In this example, we try to open a file that doesn’t exist, which raises a FileNotFoundError. We catch the error and print an error message to stderr.
Displaying detailed error information
When handling exceptions, it can be beneficial to display detailed information about the error, such as the type of exception and its traceback. We can use the traceback module to achieve this. Here’s an example:
“`python
import sys
import traceback
try:
# Code that might raise an exception
result = 10 / 0
except ZeroDivisionError:
# Handle the specific exception
exception_type, exception_value, exception_traceback = sys.exc_info()
error_message = f”Error: {exception_type.__name__}: {exception_value}\n”
error_message += “”.join(traceback.format_tb(exception_traceback))
sys.stderr.write(error_message)
“`
In this example, we catch the ZeroDivisionError and access the exception information using the sys.exc_info() function. We then format the error message with the exception type, value, and traceback and print it to stderr.
Best practices
When using stderr in your Python code, it’s important to follow best practices to ensure clear and efficient error handling and logging.
When to use stderr instead of stdout
Use stderr instead of stdout when printing error messages, warnings, or diagnostic information. By convention, stdout is typically used for regular program output, while stderr is specifically designed for error-related messages.
Providing clear and informative error messages
When printing error messages to stderr, make sure to provide clear and informative information about the issue. This helps users or developers understand what went wrong and how to address the problem effectively.
Properly handling and logging errors
When encountering errors, it’s important to handle them appropriately and log the error information for further analysis. You can redirect stderr to a log file, capture error messages in a variable, or use a logging library to handle and log errors consistently.
Common use cases
Here are some common use cases for printing to stderr:
Writing error logs: Redirecting error messages to a log file allows for easy tracking and analysis of errors. This is crucial in production environments where monitoring and debugging are essential.
Debugging and troubleshooting: When developing or debugging code, printing debugging information or error messages to stderr can provide valuable insights into the state of the program and help identify the source of issues.
Capturing and redirecting error messages: Sometimes, it may be necessary to capture error messages in a variable or redirect them to another output location for further processing or analysis.
Third-party libraries and frameworks
Many popular Python libraries and frameworks utilize stderr for error handling and logging purposes. For example:
– The logging module provides a powerful and flexible framework for logging messages, including error messages, to various output destinations, including stderr.
– In Django and Flask, stderr is often used for logging error messages, which can be configured and customized according to the application’s needs.
Conclusion
In conclusion, understanding how to print to stderr in Python is essential for effective error handling, logging, and debugging. By utilizing the sys module, redirecting stderr to different destinations, and properly handling exceptions, you can provide clear and informative error messages and effectively troubleshoot issues in your Python applications.
How To Print To Stderr In Python?
Keywords searched by users: python print to stderr Fprintf(stderr), Sys stderr write, Python print to file, Redirect output and error to file, File=sys stderr, Print stderr linux, Python try except print error, Bash redirect stderr
Categories: Top 92 Python Print To Stderr
See more here: nhanvietluanvan.com
Fprintf(Stderr)
Introduction:
When it comes to writing robust and reliable code, effective error messaging and debugging are essential. One of the most commonly used functions for this purpose in the C programming language is `fprintf(stderr)`. In this article, we will delve into the details of `fprintf(stderr)`, its benefits, and how to use it effectively for error handling and debugging.
What is `fprintf(stderr)`?
The `fprintf` function in C is used to write formatted output to a stream. The `stderr` stream, on the other hand, is one of the three standard I/O streams associated with the C runtime library. Unlike the standard output stream (`stdout`), which is typically used for general output, the `stderr` stream is designed specifically for printing error messages and diagnostics.
The `fprintf(stderr)` function combines the functionalities of `fprintf` with the error messaging capabilities of `stderr`. This combination makes it a powerful tool for developers to handle errors and print debugging information directly to the standard error stream.
Why use `fprintf(stderr)` for error messaging and debugging?
1. Separating regular output from error messages:
Using `fprintf(stderr)` allows developers to clearly differentiate normal program output from error messages. By directing error messages to the standard error stream, they can be easily distinguished and interpreted separately, which is invaluable when analyzing and fixing issues.
2. Capturing and saving error messages:
In addition to immediate visibility, error messages sent to `stderr` can also be redirected to a file for later analysis, if desired. This can be extremely helpful for troubleshooting issues that occur on remote or deployed systems where immediate debugging is not feasible.
3. Customizing error messages:
The `fprintf(stderr)` function provides developers the flexibility to format and customize error messages to their specific needs. It supports format specifiers and allows printing additional information such as variable values, line numbers, timestamps, and more. This greatly enhances the clarity and context of error messages, aiding in understanding and resolving issues efficiently.
4. Quick identification of errors:
Using `fprintf(stderr)` to print error messages at the point of error occurrence helps in quickly identifying the problematic code. By including relevant information such as file name, line number, and a descriptive error message, developers can pinpoint the exact location and nature of the error for faster resolution.
5. Debugging without an interactive debugger:
In some situations, using an interactive debugger may not be feasible or practical. By strategically placing `fprintf(stderr)` statements within the code, developers can obtain valuable debugging information without relying on a full-blown debugger. This makes the `fprintf(stderr)` function an invaluable tool for debugging applications running on embedded systems or in environments where interactive debugging is limited.
Best practices for using `fprintf(stderr)`:
To make the most out of `fprintf(stderr)`, it’s important to follow some best practices:
1. Use meaningful error messages:
When encountering errors, ensure that the error messages are descriptive and provide enough information to understand the cause of the problem. Ambiguous or vague error messages can hinder troubleshooting efforts and waste valuable time.
2. Add contextual information:
Along with error messages, consider adding additional contextual information that might aid in identifying the root cause of the error. This can include variable values, function names, file paths, timestamps, and any other relevant details.
3. Utilize different log levels:
Consider implementing different log levels for error messages, warnings, and informational/debugging messages. This allows filtering and prioritizing the logged information for efficient debugging and maintenance.
4. Leverage logging frameworks:
For larger projects, utilizing logging frameworks can provide additional benefits. These frameworks often offer more advanced features, such as log rotation, log file rotation, filtering, and integration with other tools.
5. Avoid excessive logging:
While logging is essential for troubleshooting, it’s important not to overuse `fprintf(stderr)`. Excessive logging can hinder performance and create unnecessary clutter in the error output, making it harder to identify important information.
FAQs:
Q1: Can I use `fprintf(stderr)` for general program output instead of `printf`?
A1: Although `fprintf(stderr)` can technically be used for general program output, it is not recommended. The standard output stream `stdout` is more appropriate for regular program output, as it allows developers to easily distinguish normal output from error messages and diagnostics.
Q2: How can I redirect `stderr` to a file?
A2: In Unix-like systems, the `stderr` stream can be easily redirected to a file using the command-line syntax `./program 2> error.log`. This redirects standard error output to the file named `error.log` in the current directory. The exact method may differ in different operating systems.
Q3: Is `fprintf(stderr)` thread-safe?
A3: The C standard does not explicitly guarantee thread-safety for `fprintf(stderr)`. However, most modern C runtime libraries provide thread-safe implementations, ensuring that multiple threads can write to `stderr` without conflicts. It’s always recommended to consult the documentation of your specific compiler or runtime library.
Q4: Are there alternatives to `fprintf(stderr)` for error messaging and debugging in C?
A4: Yes, there are alternative approaches, such as using log files, interactive debuggers, or specialized debugging libraries. However, `fprintf(stderr)` remains a simple and effective choice for error messaging and debugging due to its ease of use and portability.
Conclusion:
In the world of programming, efficient error messaging and debugging play pivotal roles in ensuring the stability and reliability of applications. The `fprintf(stderr)` function proves to be an indispensable tool, allowing developers to display error messages, print debugging information, and facilitate troubleshooting. By leveraging the power of `fprintf(stderr)`, developers can significantly improve their code’s maintainability and enhance the overall software development process.
Sys Stderr Write
In the realm of programming, the ability to accurately identify and handle error conditions is of paramount importance. One of the tools that aid in this process is the sys stderr, which allows developers to write error messages to the standard error stream. This article will explore the functionalities and applications of sys stderr, along with providing answers to commonly asked questions about its usage.
What is sys stderr?
In Python, sys.stderr is a built-in object that represents the standard error stream in the system. When we run a program, the standard error stream is utilized to display diagnostic messages, warnings, and error information regarding the program’s execution. By leveraging sys.stderr, programmers can precisely control the flow of error messages and make the debugging process more efficient.
How does sys stderr work?
By default, the standard error stream is connected to the console or terminal where the program is being executed. Whenever an error occurs, the Python interpreter writes the error message to sys.stderr, allowing it to be displayed in the console for the developer to see. However, sys.stderr is more than just a passive output stream – it can be used as a file-like object to redirect, capture, or suppress error messages.
Redirecting Error Output
One powerful feature of sys.stderr is its ability to redirect error messages to a file instead of the console. By assigning a new value to sys.stderr, developers can ensure that all error messages are written to a specific file, providing a detailed log for later analysis or troubleshooting. The following code snippet demonstrates this functionality:
“`python
import sys
with open(“error.log”, “w”) as f:
sys.stderr = f
# Now all error messages will be written to the file error.log
“`
Capturing Error Output
In certain scenarios, it may be useful to capture error messages programmatically for further processing or error handling. Python’s StringIO module allows us to create an in-memory buffer that mimics a file object. By redirecting sys.stderr to this buffer, we can capture error messages as strings. Here is an example:
“`python
import sys
from io import StringIO
error_buffer = StringIO()
sys.stderr = error_buffer
# Code that may generate errors
# Capturing the error message
error_message = error_buffer.getvalue()
# Further processing or error handling
“`
Suppressing Error Messages
In some cases, we may want to suppress error messages from being displayed entirely. This could be useful when running automated scripts where error messages may clutter the output. By redirecting sys.stderr to the null file object, all error messages will effectively be discarded. The following code demonstrates how to achieve this:
“`python
import sys
sys.stderr = open(“/dev/null”, “w”) # Discards all error messages
“`
FAQs:
Q: What is the difference between sys.stdout and sys.stderr?
A: While sys.stderr is used for error messages and diagnostic information, sys.stdout represents the standard output stream, which is typically used for displaying normal program output. Both streams can be redirected or captured in a similar fashion.
Q: Can sys.stderr be redirected to another programming language’s console?
A: No, sys.stderr is specific to Python and cannot be directly redirected to another language’s console. However, error messages can still be captured in Python and subsequently transmitted to another system or language if needed.
Q: Is it possible to restore the original sys.stderr behavior after redirection?
A: Yes, to restore the original sys.stderr behavior, assign sys.__stderr__ to sys.stderr, as shown in the example below:
“`python
import sys
# Save the original sys.stderr
original_stderr = sys.stderr
# Redirection code here…
# Restore sys.stderr to its original state
sys.stderr = sys.__stderr__
“`
In conclusion, sys.stderr is a valuable tool for developers, enabling them to manage error messages effectively. By understanding how to redirect, capture, or suppress error output, programmers can streamline their debugging processes and improve the overall reliability of their programs.
Images related to the topic python print to stderr
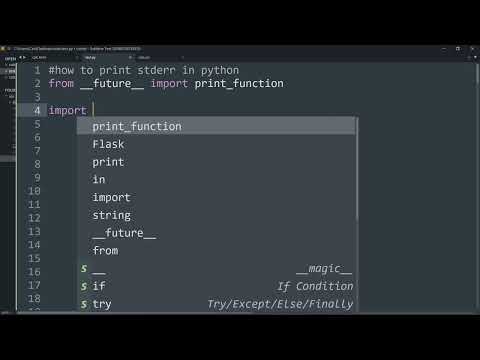
Found 5 images related to python print to stderr theme
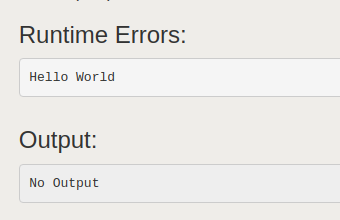


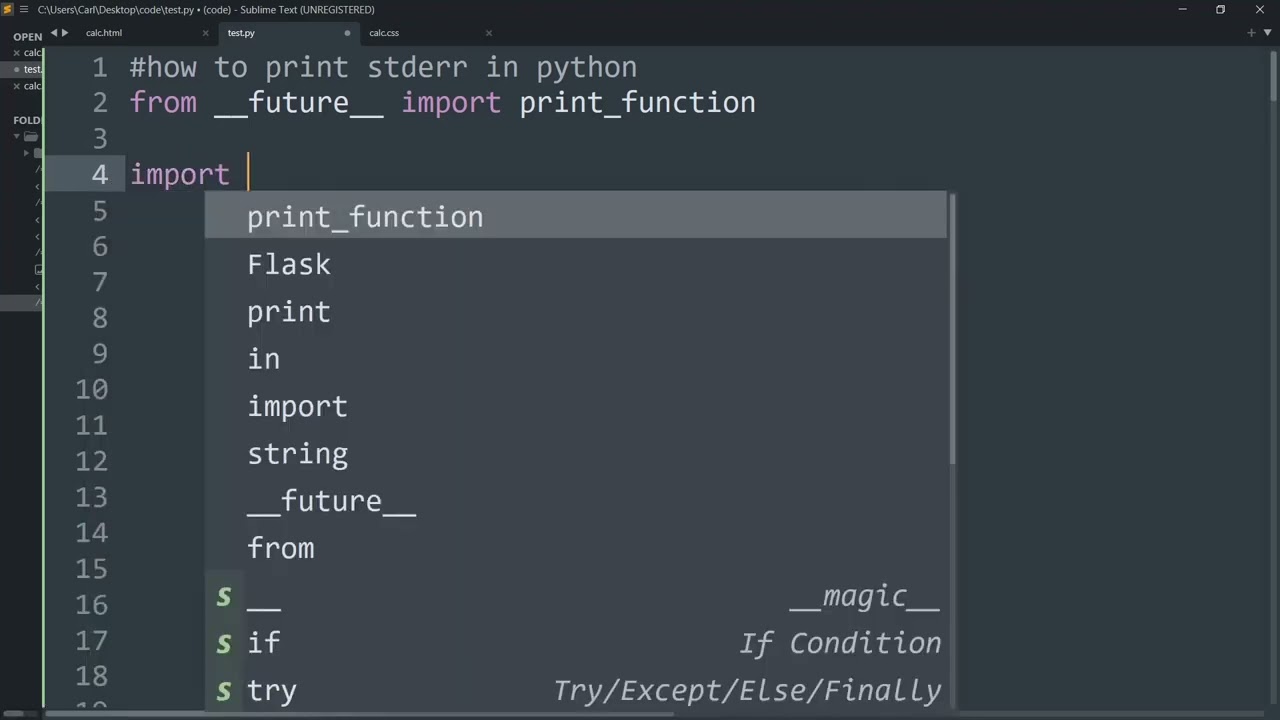
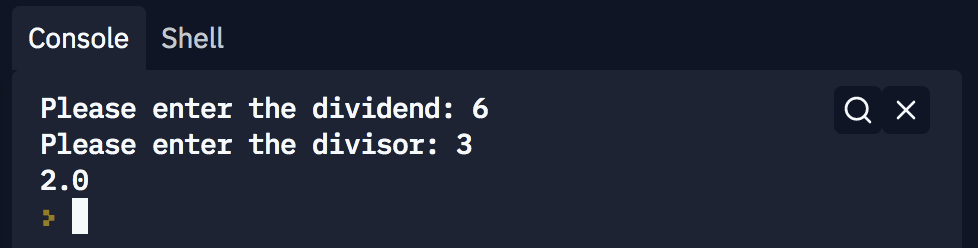

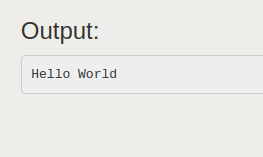
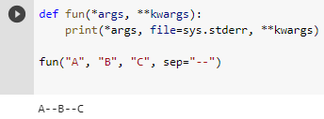


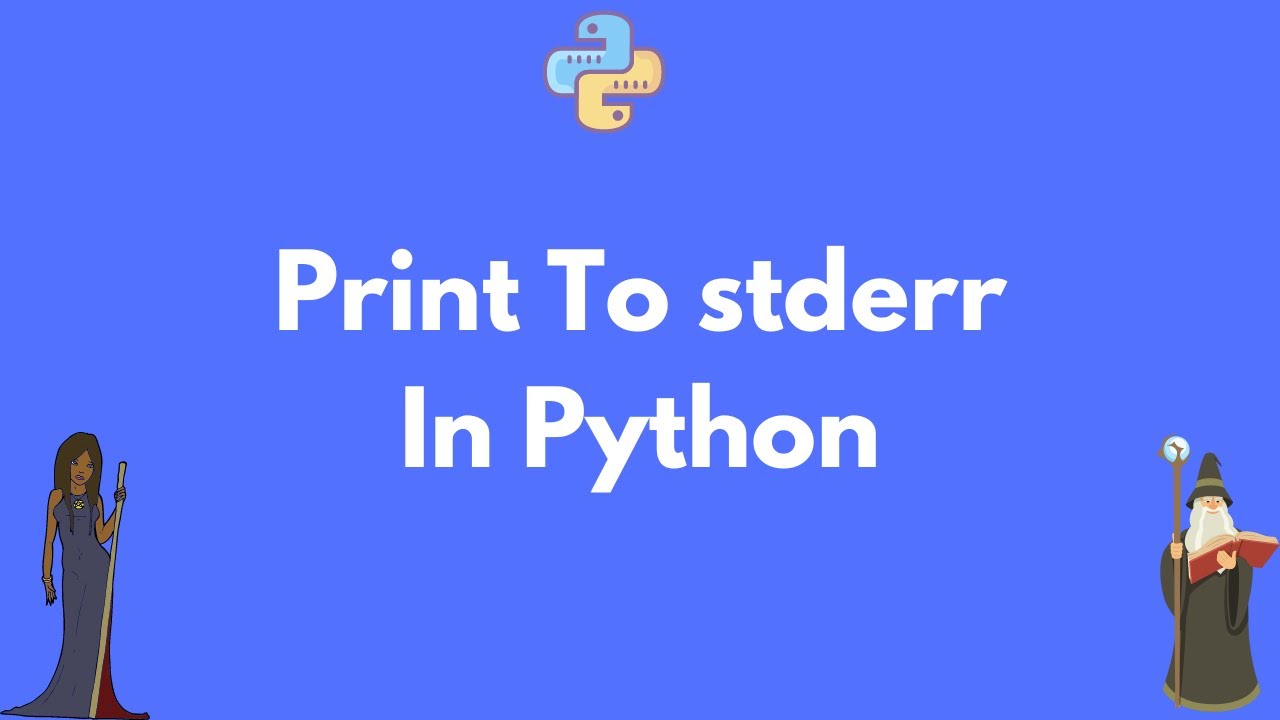
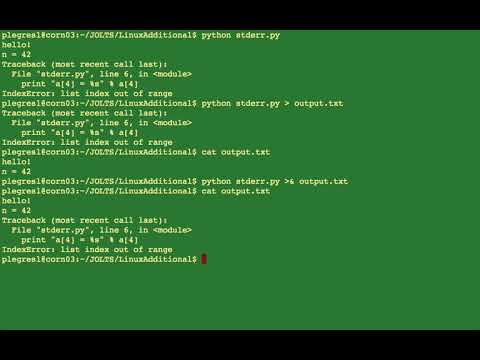

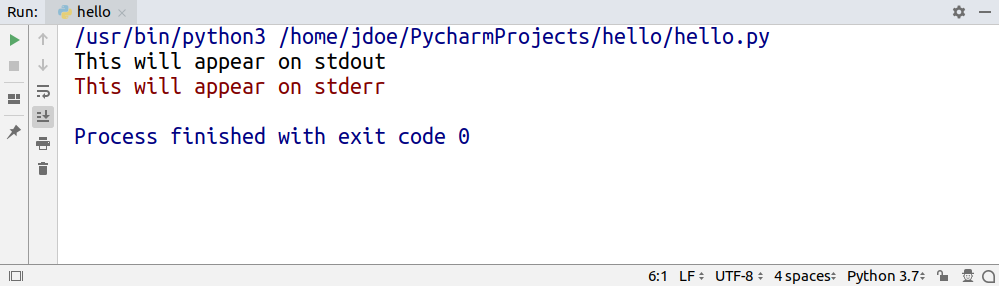

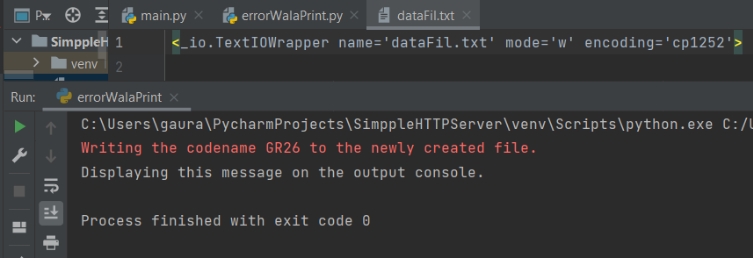

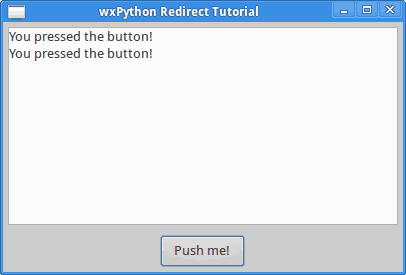
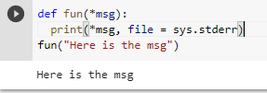
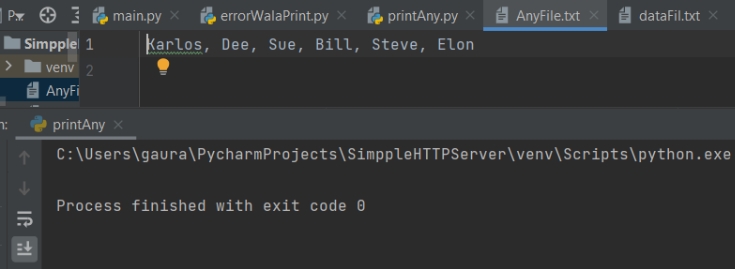


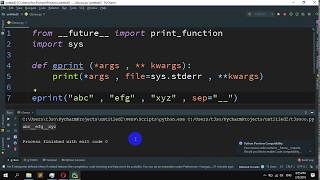
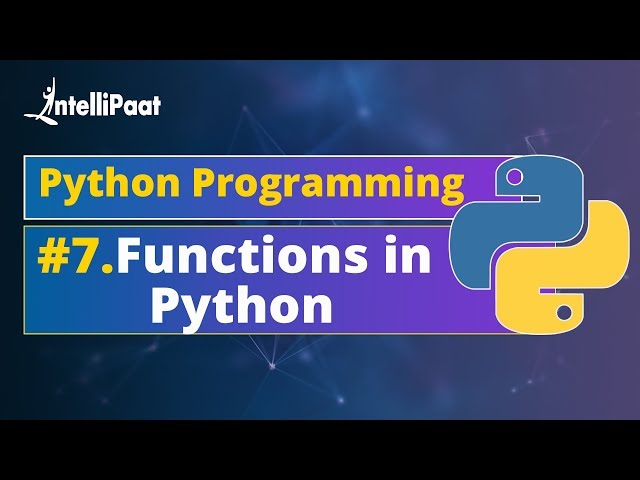
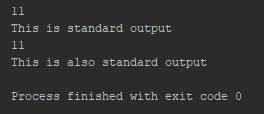
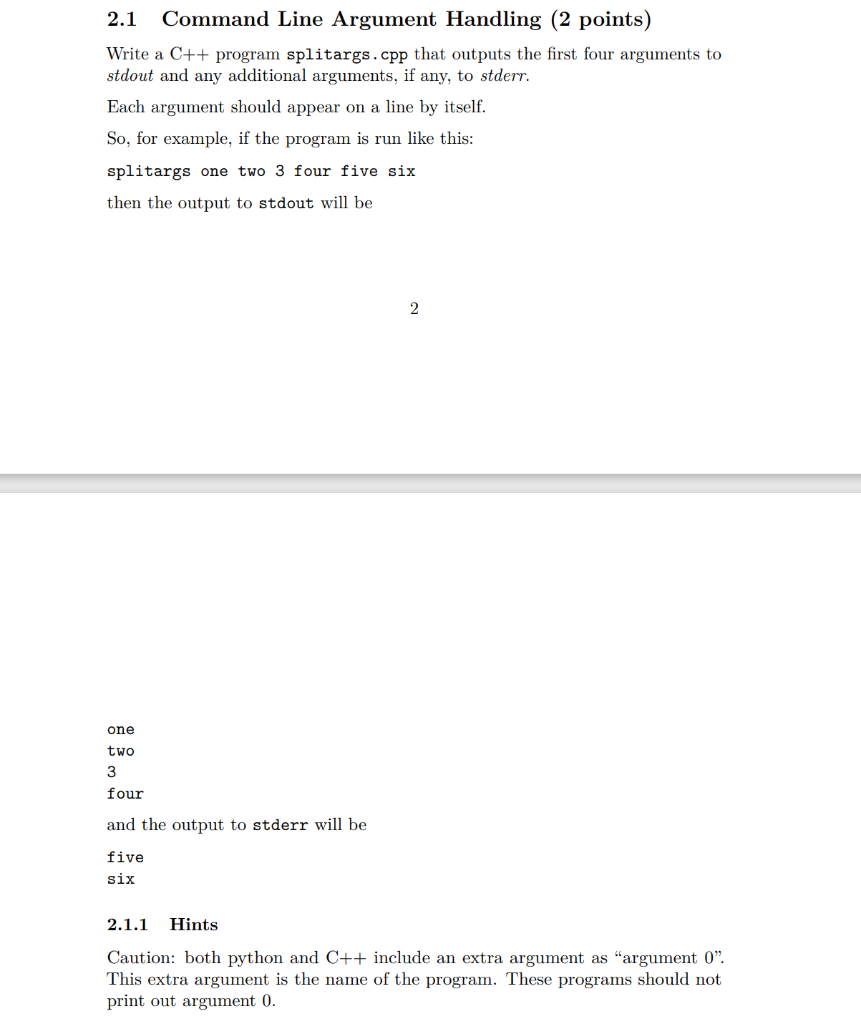
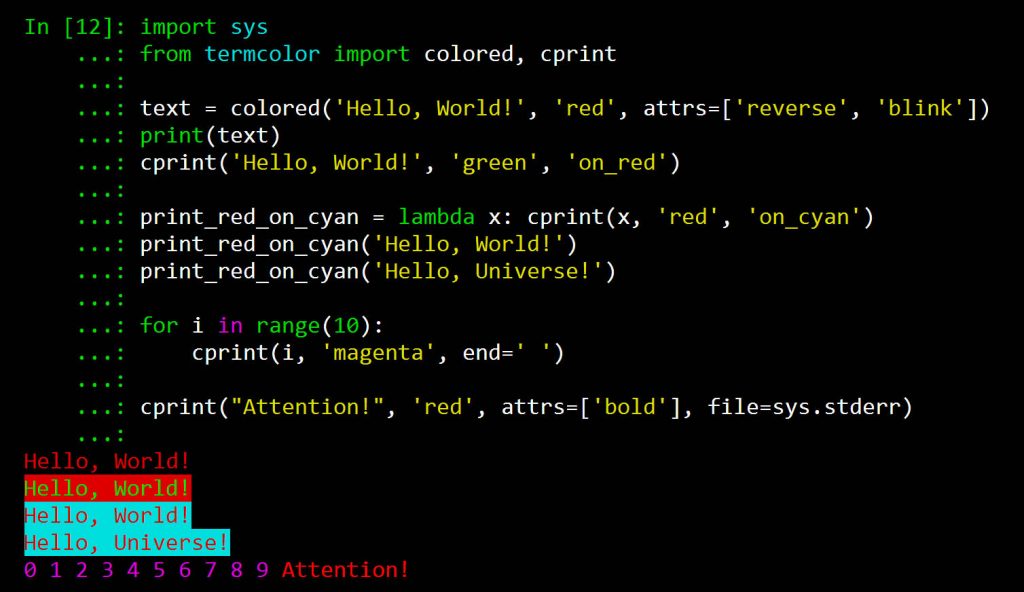
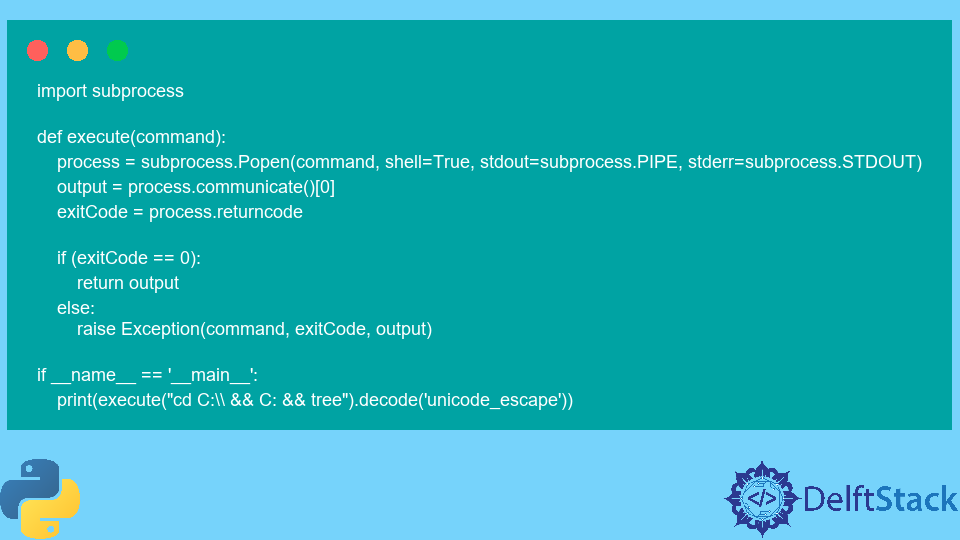

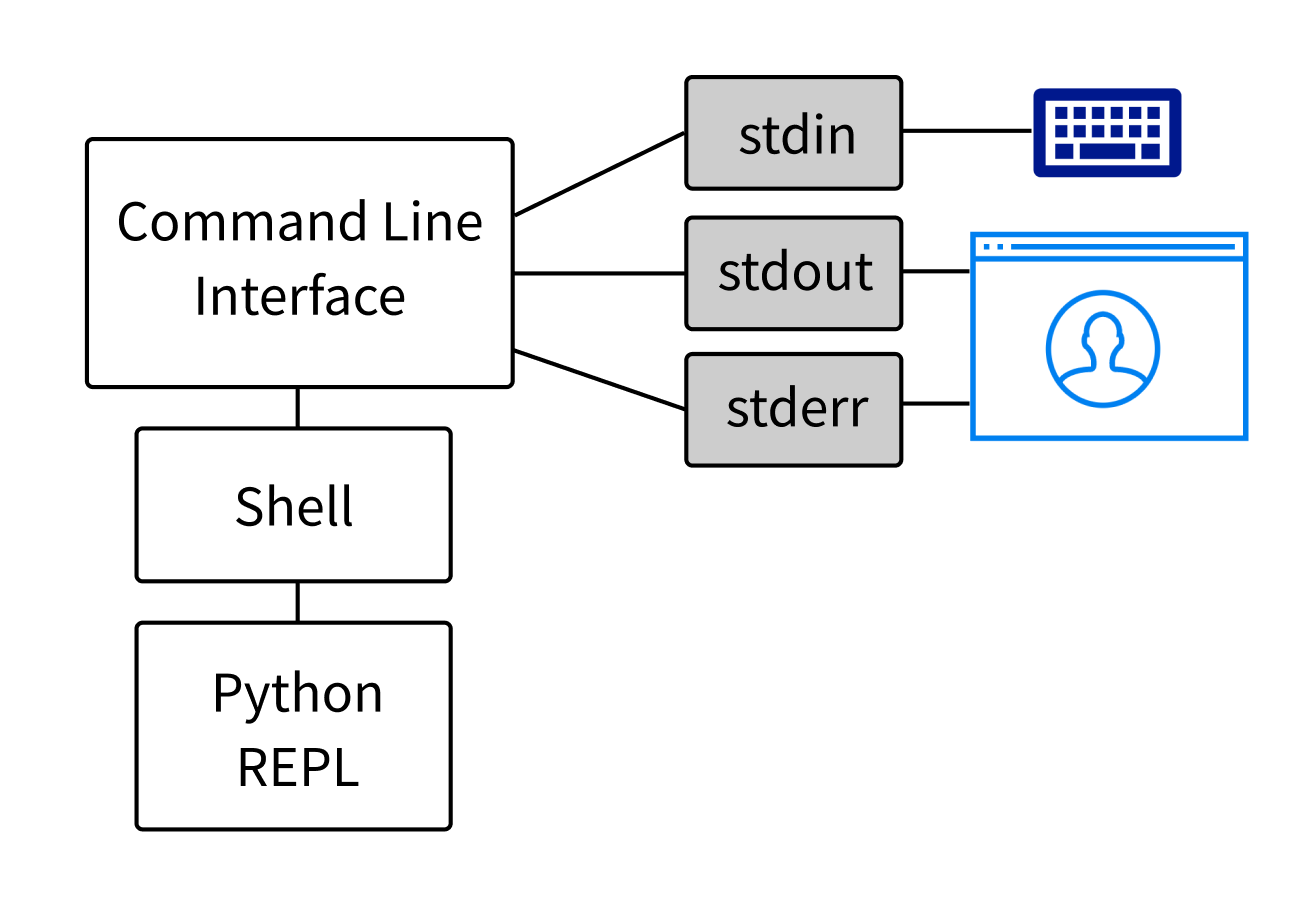

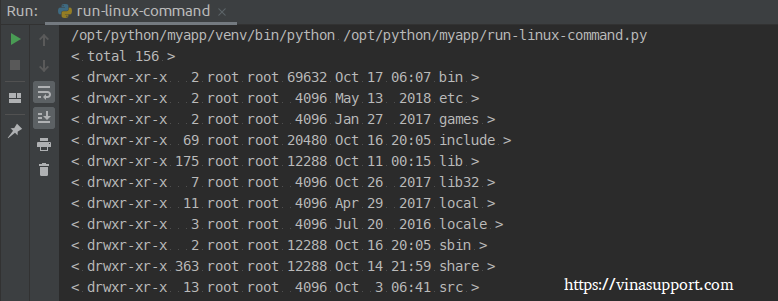




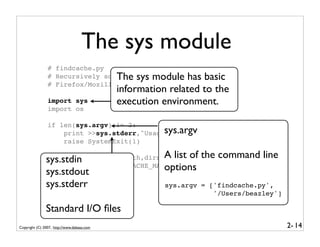


![An Introduction to Subprocess in Python With Examples [Updated] An Introduction To Subprocess In Python With Examples [Updated]](https://www.simplilearn.com/ice9/free_resources_article_thumb/SubprocessInPython_3.png)



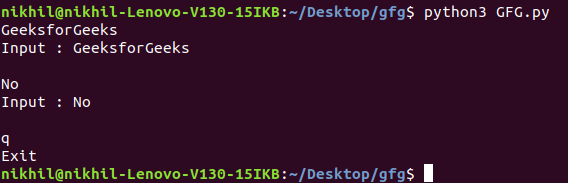

Article link: python print to stderr.
Learn more about the topic python print to stderr.
- How to print to stderr in Python – blogboard.io
- How do I print to stderr in Python? – Stack Overflow
- Here is how to print to stderr in Python in Python – PythonHow
- How to print to stderr and stdout in Python? – GeeksforGeeks
- How to Print to stderr in Python? – Spark By {Examples}
- How to Print to the Standard Error Stream in Python
- Print to stderr in Python | Delft Stack
- Recommended Ways to Print To Stderr in Python
- Python: Print to stderr – w3resource
- Python Print to Stderr – AppDividend
See more: nhanvietluanvan.com/luat-hoc