Python Print Environment Variables
Environment variables are important elements in Python programming as they store information about the environment in which the program is being executed. These variables hold values that can be accessed and utilized by the program during runtime. In this article, we will explore various ways to print environment variables in Python, along with best practices and commonly asked questions on this topic.
1. Understanding Environment Variables in Python
In Python, environment variables are stored as key-value pairs, where the key represents the variable name and the value represents the associated information. These variables can be system-level (available to all users) or user-level (specific to a particular user).
Environment variables provide a flexible way of configuring various aspects of a program, such as file paths, database connection details, API keys, and more. They can be accessed and used by the program at runtime, eliminating the need to hardcode such values within the code.
2. Accessing Environment Variables using the os Module
Python provides the “os” module, which offers a set of functions for interacting with the operating system. This module includes a dictionary-like object called “environ” that stores all the environment variables.
To access and retrieve the values of environment variables, the “environ” dictionary can be used in combination with the variable name. For example, to access the value of the “HOME” environment variable, you can use the following code snippet:
“`python
import os
home_dir = os.environ[‘HOME’]
print(home_dir)
“`
3. Printing All Environment Variables
To print all the environment variables, you can iterate over the “environ” dictionary and print each key-value pair. Here’s an example:
“`python
import os
for key, value in os.environ.items():
print(f”{key}: {value}”)
“`
This code will print all the available environment variables and their associated values.
4. Printing Specific Environment Variables
To print a specific environment variable, you can use the same method mentioned earlier, but with the specific variable name. For instance, to print the value of the “PATH” environment variable, you can use the following code:
“`python
import os
path_value = os.environ.get(‘PATH’)
if path_value:
print(f”PATH: {path_value}”)
else:
print(“PATH environment variable is not set”)
“`
This code checks if the “PATH” environment variable exists and prints its value accordingly.
5. Printing Environment Variables in a User-friendly Format
While printing environment variables using the above methods provides the necessary information, sometimes it’s beneficial to format the output for better readability. To achieve this, you can use predefined formatting techniques, such as tabular representation or grouping variables based on certain criteria.
Formatting the output is often done using string manipulation techniques, such as f-strings or other string formatting functions available in Python.
6. Understanding the Importance of Environment Variables in Python
Environment variables play a crucial role in Python programming by providing a dynamic and configurable way of storing information. This flexibility allows developers to separate configuration details from the code, making it easier to maintain and deploy applications in different environments.
By leveraging environment variables, Python programs can be made more portable, allowing them to run seamlessly across different systems without any modifications.
7. Best Practices for Handling and Printing Environment Variables
When working with environment variables, it is essential to follow some best practices for better code maintainability and security. Here are a few recommendations:
– Use descriptive names for environment variables to enhance readability.
– Always validate the existence of an environment variable before accessing its value.
– Avoid hardcoding sensitive information in the code and use environment variables instead.
– Separate configuration details from code logic by externalizing them in environment variables.
– Encrypt or secure sensitive environment variable values if needed.
These best practices ensure that environment variables are used appropriately, allowing for more secure and scalable applications.
FAQs:
Q1: How can I set environment variables in a Python script?
A1: Environment variables can be set within a Python script using the “os” module. You can use the `os.environ` dictionary to set key-value pairs for environment variables.
Q2: How do I access environment variables in Django?
A2: In Django, you can access environment variables using the `os.environ` dictionary. Make sure to import the `os` module and use the dictionary accordingly.
Q3: How can I print environment variables in a specific order?
A3: To print environment variables in a specific order, you can create a list of the desired variable names and iterate over it while accessing and printing the values.
Q4: Can I modify environment variables during runtime?
A4: Yes, you can modify environment variables during runtime, but keep in mind that changes to environment variables will only apply to the current process and its child processes.
Q5: Can I pass environment variables to a Python script from the command line?
A5: Yes, you can pass environment variables to a Python script from the command line using the `-E` option, followed by the variable assignments. For example: `python -E script.py VAR=value`.
In conclusion, understanding and utilizing environment variables in Python is essential for creating flexible and configurable programs. By accessing and printing these variables, developers can gain valuable insights into the execution environment and ensure smooth functioning of their applications.
Load Environment Variables From .Env Files In Python
How To Read Env Variables In Python?
Environment variables are an essential component of any programming environment. They store specific values or configurations that can be accessed by programs or scripts. In Python, reading environment variables is a straightforward process that allows developers to access sensitive information such as API keys, database credentials, or configuration settings without hardcoding them in the code. In this article, we will explore various methods to read environment variables in Python, enabling you to incorporate this functionality into your own projects.
Methods for Reading Environment Variables in Python
1. Using the os Module:
The os module in Python provides functions for interacting with the operating system. To read environment variables using this module, we can make use of the os.environ dictionary. The dictionary contains key-value pairs, where the keys are the environment variable names, and the values are their corresponding values. Here’s an example:
“`python
import os
my_variable = os.environ.get(‘MY_VARIABLE_NAME’)
“`
In this example, `os.environ.get(‘MY_VARIABLE_NAME’)` retrieves the value associated with the environment variable ‘MY_VARIABLE_NAME’. If the variable is not found, it returns `None`.
2. Using the dotenv Module:
The dotenv module simplifies reading environment variables by loading them from a `.env` file into the `os.environ` dictionary. To use the dotenv module, you need to install it by running `pip install python-dotenv` in your terminal. Then, create a `.env` file in the root directory of your project and define your environment variables inside it. The format should be `VARIABLE_NAME=VALUE`. For example:
“`
API_KEY=your_api_key
DATABASE_URL=your_database_url
“`
To load the variables into your script, you can use the following code:
“`python
from dotenv import load_dotenv
load_dotenv()
my_variable = os.environ.get(‘MY_VARIABLE_NAME’)
“`
By using the `load_dotenv()` function, the environment variables defined in the `.env` file will be added to the `os.environ` dictionary, making them accessible in your script.
3. Using the decouple Module:
The decouple module is another popular choice for reading settings from `.env` files. To use it, install the module by running `pip install python-decouple`. Similar to the dotenv module, create a `.env` file with your environment variables. However, the format should be `VARIABLE_NAME=VALUE`, just like before. Here’s an example:
“`
API_KEY=your_api_key
DATABASE_URL=your_database_url
“`
To read the variables into your script, the following code can be used:
“`python
from decouple import config
my_variable = config(‘MY_VARIABLE_NAME’)
“`
The `config()` function from the decouple module retrieves the value associated with the environment variable specified in the parameter.
Frequently Asked Questions (FAQs):
Q1: Can environment variables be set or modified within a Python script?
A1: No, environment variables are managed by the operating system and cannot be directly modified within a Python script. However, you can change their values before running the script from the command line or modify them in the system environment settings.
Q2: How can I set environment variables on different operating systems?
A2: The process for setting environment variables varies depending on the operating system. On Windows, you can use the `set` command in the command prompt or modify them in the System Properties dialog. On UNIX-like systems, you can use the `export` command in the terminal or modify the `.bashrc` or `.bash_profile` file.
Q3: Why should I use environment variables instead of hardcoding values in my code?
A3: Hardcoding sensitive information directly into your code poses security risks, as the code may be accessed or shared by unauthorized individuals. By using environment variables, you can store sensitive information outside of the codebase, making it easier to manage and secure sensitive data.
Q4: How can I check if an environment variable exists before trying to read it?
A4: Before accessing an environment variable, you can check if it exists in the `os.environ` dictionary. For example:
“`python
if ‘MY_VARIABLE_NAME’ in os.environ:
my_variable = os.environ.get(‘MY_VARIABLE_NAME’)
else:
print(‘Environment variable not found.’)
“`
Q5: Can I use environment variables with frameworks like Django or Flask?
A5: Yes, both Django and Flask have built-in support for reading environment variables. You can use the methods mentioned above within your Django or Flask applications to access variables defined in your `.env` file.
In conclusion, reading environment variables is a fundamental skill that Python developers should be familiar with. By leveraging the os, dotenv, or decouple modules, you can easily access environment variables within your Python scripts. This helps you maintain clean and secure code, as well as facilitates the management of sensitive information.
How To Print All Environment Variables Command?
Environment variables are crucial pieces of information that serve as placeholders to define specific properties of a computer system. These variables are dynamic and can be modified, retrieved, and used within various applications and programs. When working with an operating system, knowing how to print all environment variables using the command line can be extremely helpful in troubleshooting, system optimization, and understanding the system’s configuration. In this article, we will explore the various methods to print all environment variables command in different operating systems, providing a comprehensive guide for Windows, macOS, and Linux.
Windows:
To print all environment variables in Windows, we can utilize the “SET” command. Follow these steps:
1. Open the command prompt: Press Win + R, type “cmd,” and hit Enter.
2. Type “SET” and hit Enter: This command displays all the environment variables and their corresponding values.
3. Scroll through the list: Examine the output to view all available environment variables and their details.
macOS (Bash):
For macOS users, we can employ the “printenv” command in the terminal to print all environment variables. Simply follow these steps:
1. Open the terminal: Press Command + Spacebar, type “Terminal,” and hit Enter.
2. Type “printenv” and hit Enter: This command prints all the environment variables and their respective values on the screen.
3. Examine the output: Scroll through the list to view all the available environment variables along with their details.
Linux (Bash):
Linux users can use the “env” command to print all environment variables in the terminal. Here’s how:
1. Open the terminal: Press Ctrl + Alt + T to launch the terminal.
2. Type “env” and hit Enter: This command displays all environment variables along with their values.
3. Analyze the output: Scroll through the list to browse all the available environment variables and their specific details.
FAQs:
Q: Why would I need to print all environment variables?
A: Printing all environment variables can be useful in various scenarios such as troubleshooting, debugging applications, verifying configurations, and understanding how the system operates. It allows you to have a comprehensive view of the various components that contribute to the system’s functionality.
Q: Can I modify environment variables?
A: Yes, you can modify environment variables based on your requirements. However, caution should be exercised as modifying certain variables without proper knowledge can potentially disrupt the system’s operations.
Q: Are there any predefined environment variables?
A: Yes, operating systems come with a set of predefined environment variables that provide essential information about the system. These variables include “PATH,” “HOME,” “USER,” “TEMP,” and many more. Printing all environment variables allows you to have insights into both predefined and user-defined variables.
Q: How can I filter the printed environment variables?
A: If the printed list of environment variables is too long, you can utilize the “grep” command (available in macOS and Linux) or the “findstr” command (in Windows) to filter the variables based on specific keywords. For example, in Linux: “env | grep keyword”.
Q: Are there any graphical alternatives to print environment variables?
A: Yes, some graphical user interfaces (GUIs) provide simplified ways to view and manage environment variables. In Windows, you can navigate to “System Properties” > “Advanced” > “Environment Variables.” On macOS, go to “System Preferences” > “Users & Groups” > “Advanced Options” > “Environment.” Linux distributions often offer similar options within their settings or preferences menus.
In conclusion, printing all environment variables using the command line is a valuable skill that allows you to gain insights into the system’s configuration and troubleshoot potential issues efficiently. By following the instructions provided for Windows, macOS, and Linux, you can easily access and analyze the environment variables specific to your operating system. Remember to exercise caution when modifying these variables and leverage the power of this command to enhance your system administration skills.
Keywords searched by users: python print environment variables Config Python environment variables, Django environment variables, Environment variables Python, os.environ trong python, PyCharm set environment variables, Python set environment variable, PYTHONPATH environment variable, Python get environment variable windows
Categories: Top 47 Python Print Environment Variables
See more here: nhanvietluanvan.com
Config Python Environment Variables
Python environment variables are an essential aspect of programming in Python. They allow developers to set up dynamic configurations for their applications, making it easier to manage and customize software behavior. In this article, we will explore the concept of Python environment variables, how to configure them, and some commonly asked questions about their usage. By the end, you will have a solid understanding of how environment variables work in Python and how to leverage them efficiently in your projects.
What are Python Environment Variables?
Environment variables are dynamic values that can affect the behavior of programs running on a computer or within a specific execution environment. They serve as a way to store configuration settings that can be accessed by various applications or scripts. In the context of Python, environment variables are strings that are stored in a special dictionary called `os.environ`, which represents the current environment.
Environment variables in Python are particularly useful for storing sensitive information like API keys, database credentials, or any other configuration values that may change across different environments (e.g., development, staging, production). By using environment variables, you can separate the configuration from the codebase, making it easier to share and manage without revealing sensitive information.
Configuring Python Environment Variables
There are several approaches to configuring Python environment variables, depending on your specific needs and preferences. Let’s explore a few common methods to set and access environment variables in Python:
1. os Module:
The `os` module in Python provides a simple interface to access and modify environment variables. You can use `os.environ` to access and modify the values of environment variables. For example, to access the value of an environment variable named `API_KEY`, you can use `os.environ.get(‘API_KEY’)`.
To set an environment variable, use the syntax `os.environ[‘VAR_NAME’] = ‘VALUE’`. For instance, `os.environ[‘API_KEY’] = ‘myApiKey’` would set the variable `API_KEY` with the value `’myApiKey’`.
2. dotenv Library:
The `dotenv` library is a popular tool that allows you to load environment variables from a `.env` file located in your project directory. By placing your environment variables in this file, you can keep them separate from your codebase and easily manage them across various environments.
To use `dotenv`, start by installing the library with `pip install python-dotenv`. Then, create a file named `.env` in your project directory and add your environment variable assignments in `VAR_NAME=value` format, one per line. Finally, in your Python script, add `from dotenv import load_dotenv` at the top, and call `load_dotenv()` before accessing any environment variables.
Example `.env` file:
“`
API_KEY=myApiKey
DB_NAME=myDatabase
“`
3. Command Line:
Another approach is to set environment variables through command-line arguments while executing your Python script. This is useful when you want to temporarily override a specific value without modifying the codebase or creating a separate configuration file.
To set environment variables through the command line, prefix your script execution command with the required environment variable assignments. For example, `API_KEY=myApiKey python my_script.py` will set the `API_KEY` environment variable to `’myApiKey’` for the execution of `my_script.py`.
Frequently Asked Questions (FAQs):
Q1. Can I modify environment variables during runtime?
Yes, you can modify environment variables during runtime using either the `os.environ` dictionary or the `dotenv` library’s functionality. Keep in mind that changing them will affect the behavior of your program from that point onwards.
Q2. How can I handle missing environment variables?
When accessing environment variables, it is recommended to use the `os.environ.get(‘VAR_NAME’)` method over direct dictionary indexing to handle missing variables gracefully. This way, you can provide a default value if the variable is not defined.
Q3. Can I load environment variables from a file other than `.env`?
Yes, you can specify a custom filename for your environment variables file when using the `dotenv` library. Simply call `load_dotenv(‘.my_custom_env’)` instead of `load_dotenv()` to load from a file named `.my_custom_env`.
Q4. How do I remove an environment variable?
To remove an environment variable, you can use the `del os.environ[‘VAR_NAME’]` statement. This will delete the specified environment variable from the `os.environ` dictionary.
Q5. Are environment variables secure?
Environment variables are relatively secure since they are stored outside the codebase and aren’t publicly accessible. However, it is essential to handle them with care, especially sensitive information like passwords or API keys. Avoid printing or logging environment variable values.
In conclusion, configuring Python environment variables provides a flexible and secure way to manage application settings and configurations. Whether you choose to use the `os` module, the `dotenv` library, or command-line arguments, understanding how to set and access environment variables will enhance the portability and flexibility of your Python projects. Remember to handle sensitive information responsibly and leverage environment variables to streamline the configuration process for your applications.
Django Environment Variables
In the world of web development, Django has emerged as one of the most popular and powerful Python web frameworks. With its clean design, robust features, and scalability, Django has become the go-to choice for developers building web applications. One of the key aspects of Django’s flexibility lies in its ability to utilize environment variables, allowing developers to configure their applications easily. In this comprehensive guide, we will delve deep into Django environment variables, their significance, and how to utilize them effectively to enhance your Django development experience.
What are Environment Variables?
To understand Django environment variables, we must first grasp the concept of environment variables in general. Environment variables are dynamic values that can affect how processes behave within an operating system. They are system-wide accessible variables that can be utilized by various applications to customize their behavior without altering the application’s code. These variables are usually set by the operating system or by the user and provide essential information to applications, such as database connection settings, API keys, or other runtime configurations. Essentially, environment variables act as a bridge between the operating system and the applications running on it.
Why Use Environment Variables in Django?
Django, being a versatile web framework, allows developers to harness the power of environment variables for configuration. There are several compelling reasons why utilizing environment variables is advantageous in Django development:
1. Security: Embedding sensitive information, such as database credentials or API keys, directly in your codebase can put your application at risk. By using environment variables, you can keep this crucial information separate from your code, reducing the chances of accidental exposure.
2. Portability: Environment variables enable your application to be easily deployed in different environments. By using environment-specific configurations stored in variables, you can seamlessly transition your application between development, staging, and production environments without modifying the codebase.
3. Collaboration: When working in a team environment, utilizing environment variables allows each team member to customize their configuration independently without causing conflicts or requiring code changes.
4. Scalability: As your application grows in complexity, you may need to configure additional services and resources. By utilizing environment variables, you can easily expand your application’s capabilities without altering the existing code.
Setting Up Environment Variables in Django
Now that we understand the significance of environment variables, let’s explore how to set them up in Django. The Django framework provides a convenient way to access environment variable values using the python-dotenv library. Follow these steps to set up environment variables in Django:
1. Install python-dotenv: Begin by installing the `python-dotenv` package using pip, the Python package manager. Run the following command: `pip install python-dotenv`
2. Create a `.env` file: In the root directory of your Django project, create a file named `.env`. This file will store your environment variable settings.
3. Add variables to the `.env` file: In the `.env` file, add key-value pairs in the format `VARIABLE_NAME=VALUE` for each environment variable you want to define. For example, if you want to set a variable named `DATABASE_URL`, your `.env` file should contain: `DATABASE_URL=your_database_url`
4. Load environment variables in settings: Open your Django project’s `settings.py` file and add the following code at the top:
“`python
import dotenv
dotenv.load_dotenv()
“`
5. Access variables in Django: You can now access the defined environment variables using `os.getenv(‘VARIABLE_NAME’)` in your Django code files.
FAQs (Frequently Asked Questions):
Q: Can I change the values of environment variables during runtime in Django?
A: Yes, you can change the values of environment variables during runtime by modifying the `.env` file. However, note that the changes will only take effect the next time you run the application.
Q: How can I access environment variables within Django templates?
A: Django does not provide direct access to environment variables within templates. To utilize environment variables in templates, you can pass them as context variables from views.
Q: Is it necessary to add the `.env` file to the version control system?
A: No, it is generally recommended to exclude the `.env` file from version control systems like Git. Instead, each developer can create their local `.env` file with their own configuration.
Q: Can I use environment variables in Django’s settings directly without the `python-dotenv` library?
A: Yes, you can manually set environment variables in your server environment or shell and access them directly from Django’s settings using `os.environ[‘VARIABLE_NAME’]`. However, using the `python-dotenv` library provides a more convenient way to manage variables in a project-specific `.env` file.
Conclusion
In this comprehensive guide, we have explored the significance of Django environment variables and why they are essential in Django web development. By using environment variables, you can enhance security, portability, collaboration, and scalability in your Django applications. We have also provided step-by-step instructions on setting up environment variables in Django, along with a FAQ section addressing common queries. Embrace the power of Django environment variables and streamline your development workflow by separating configuration from code.
Images related to the topic python print environment variables
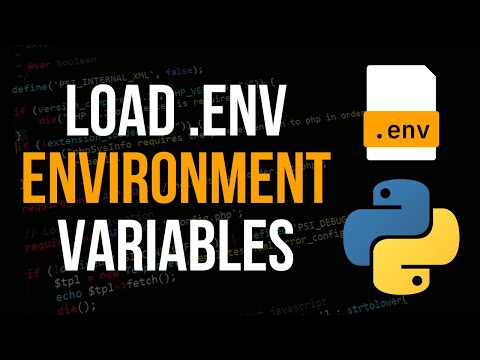
Found 34 images related to python print environment variables theme
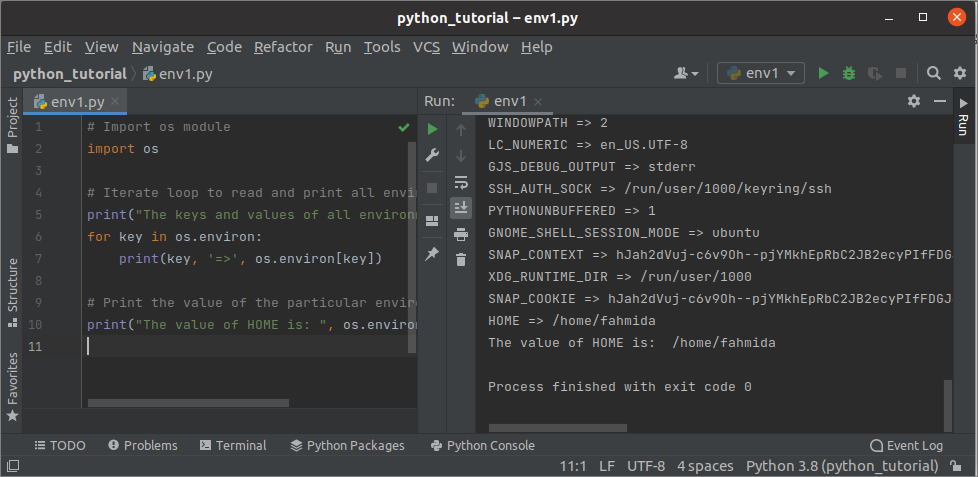
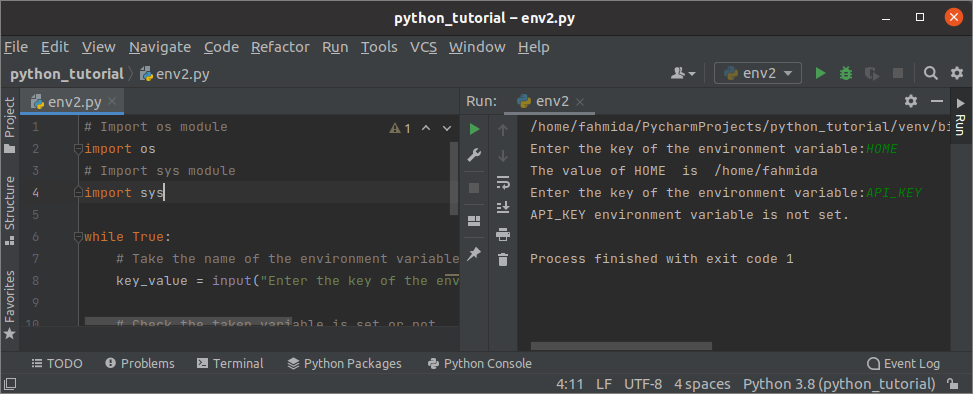

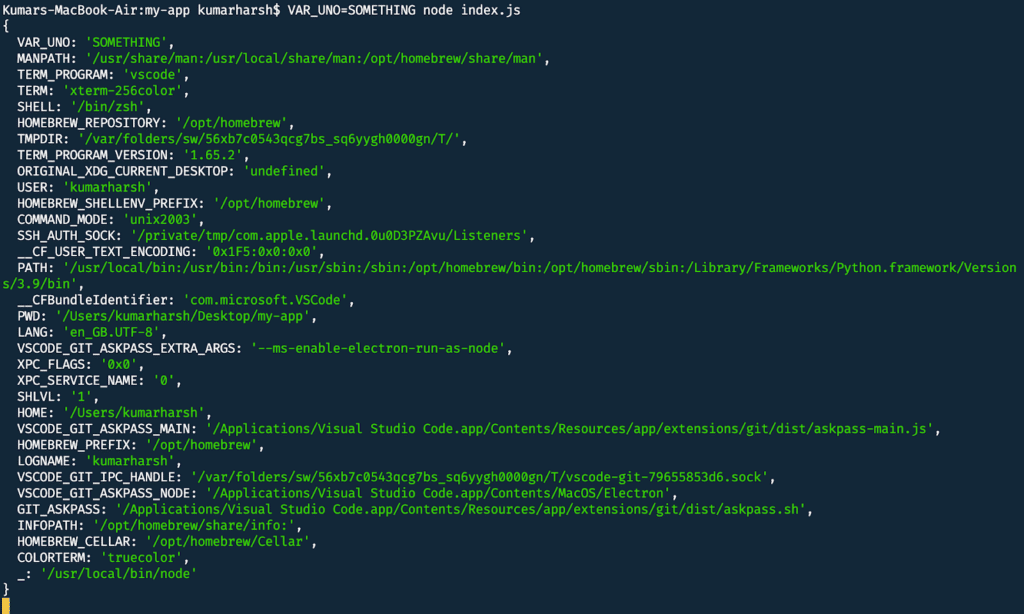
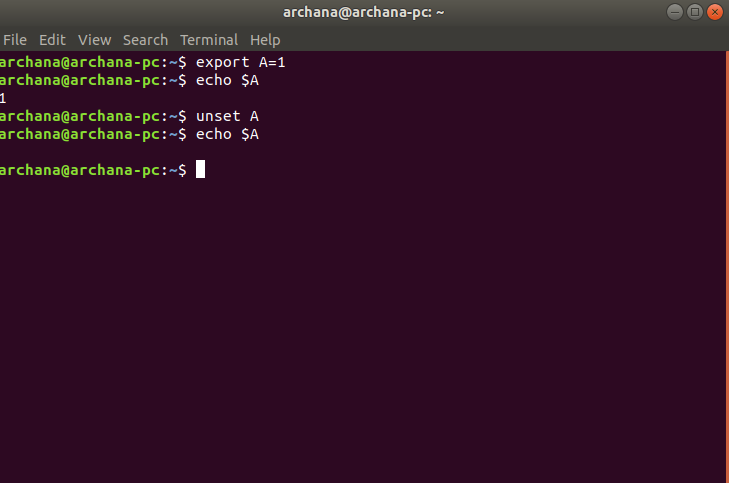
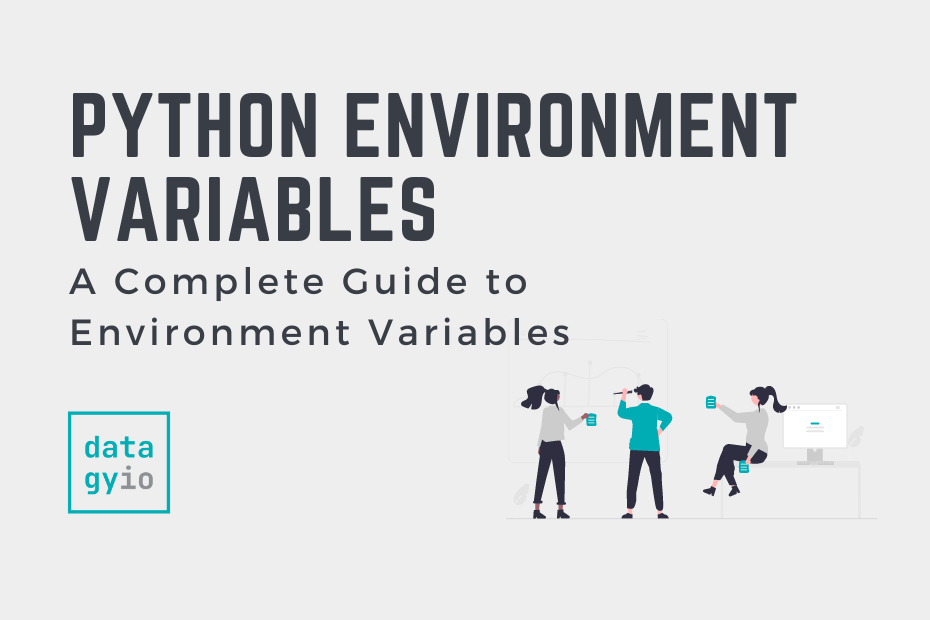
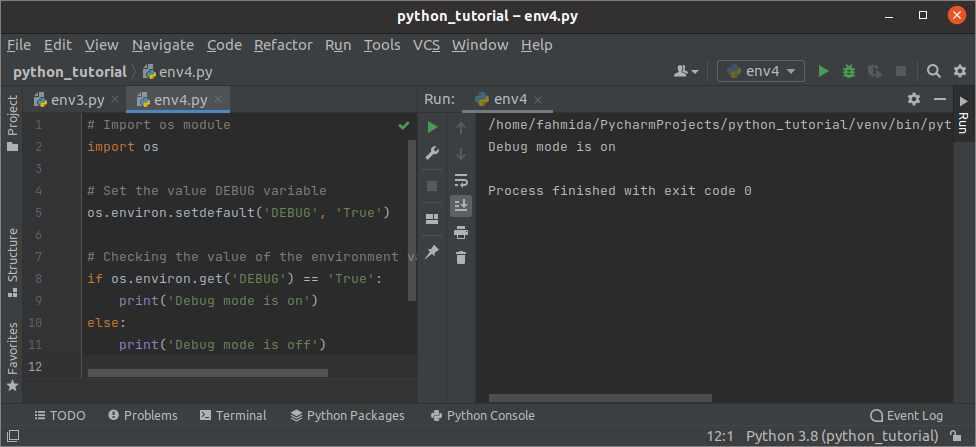
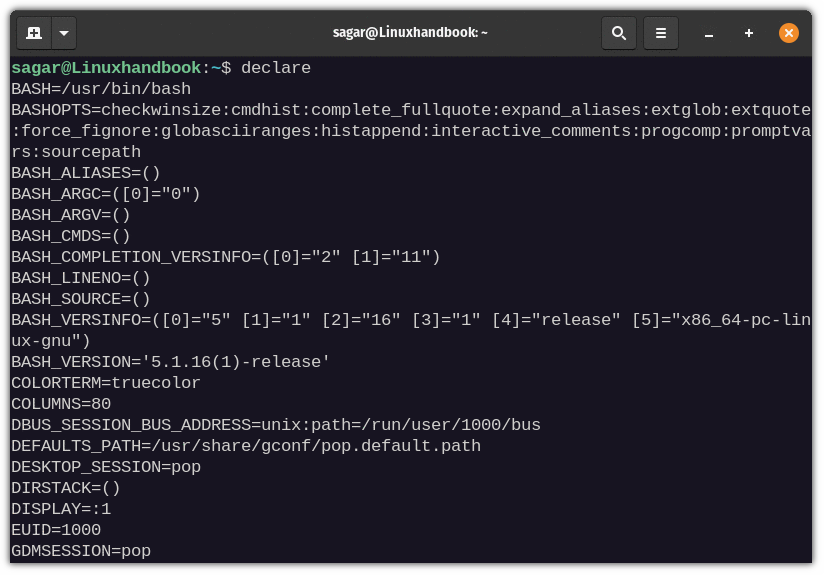

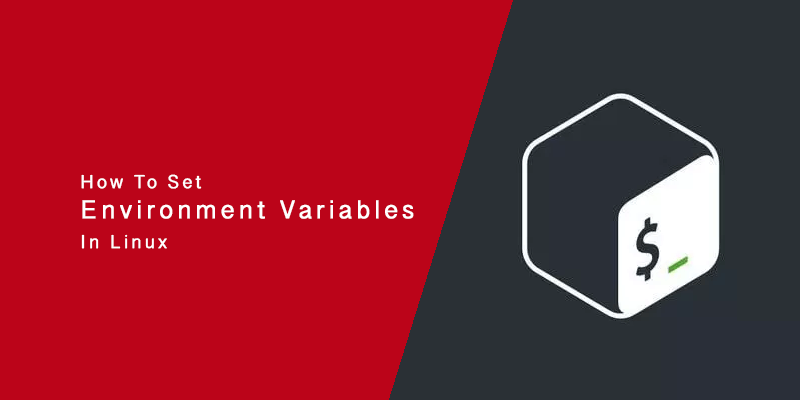
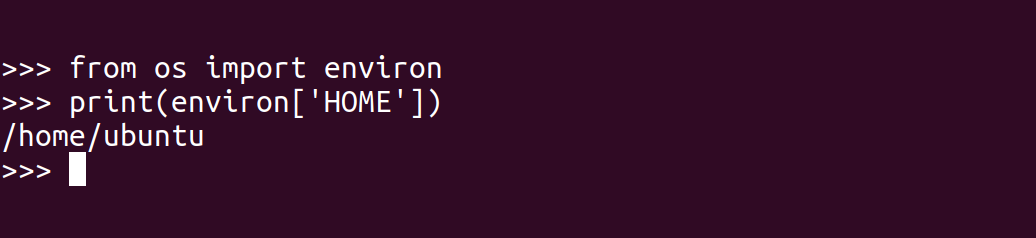

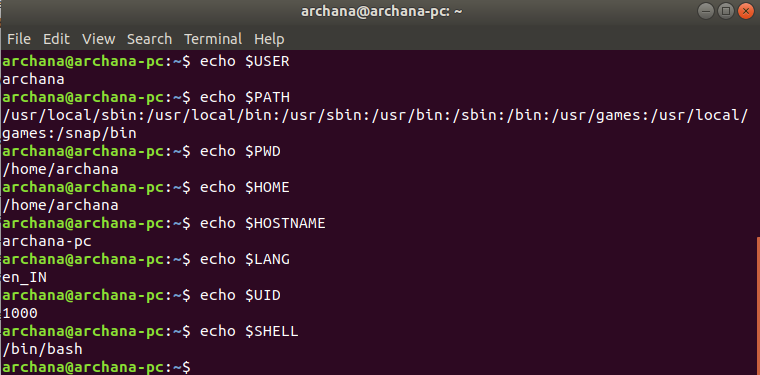

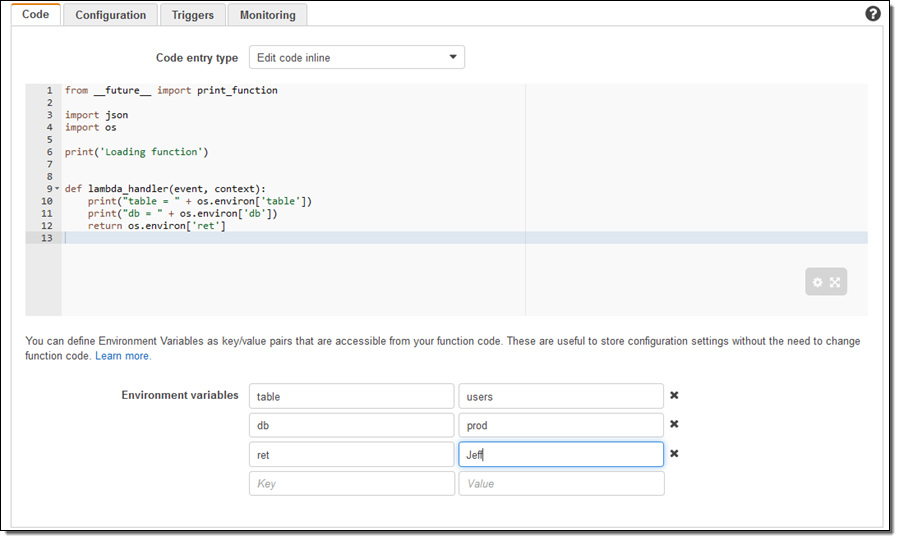

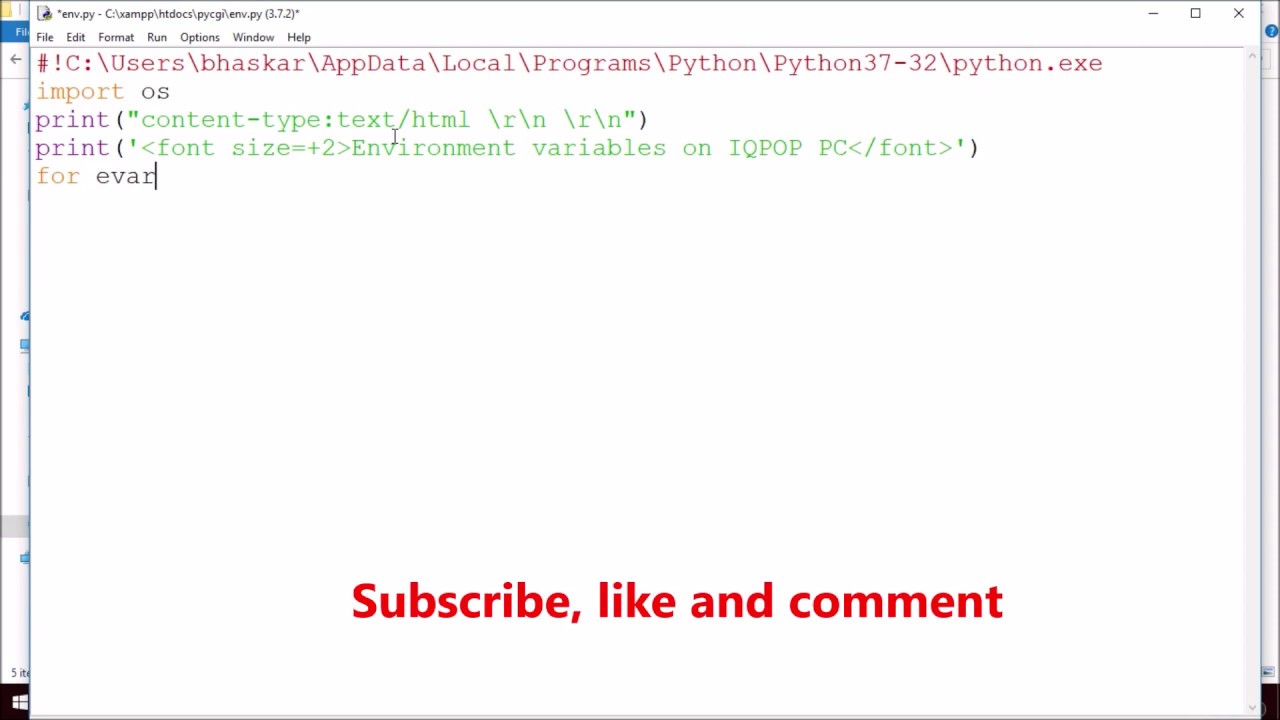

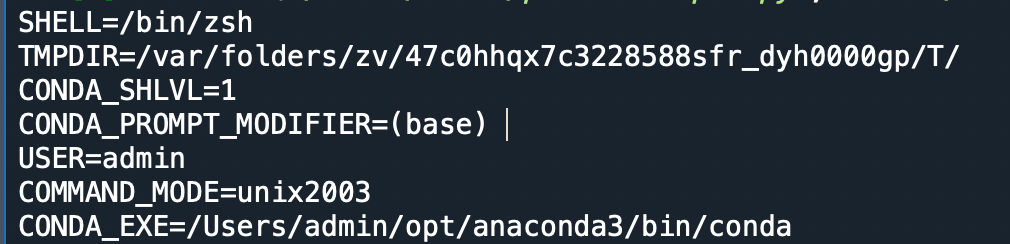
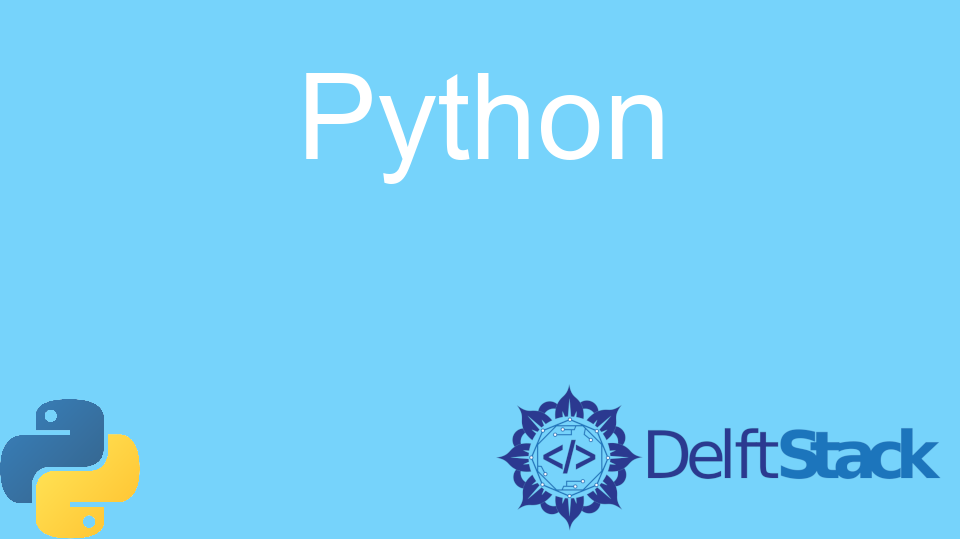
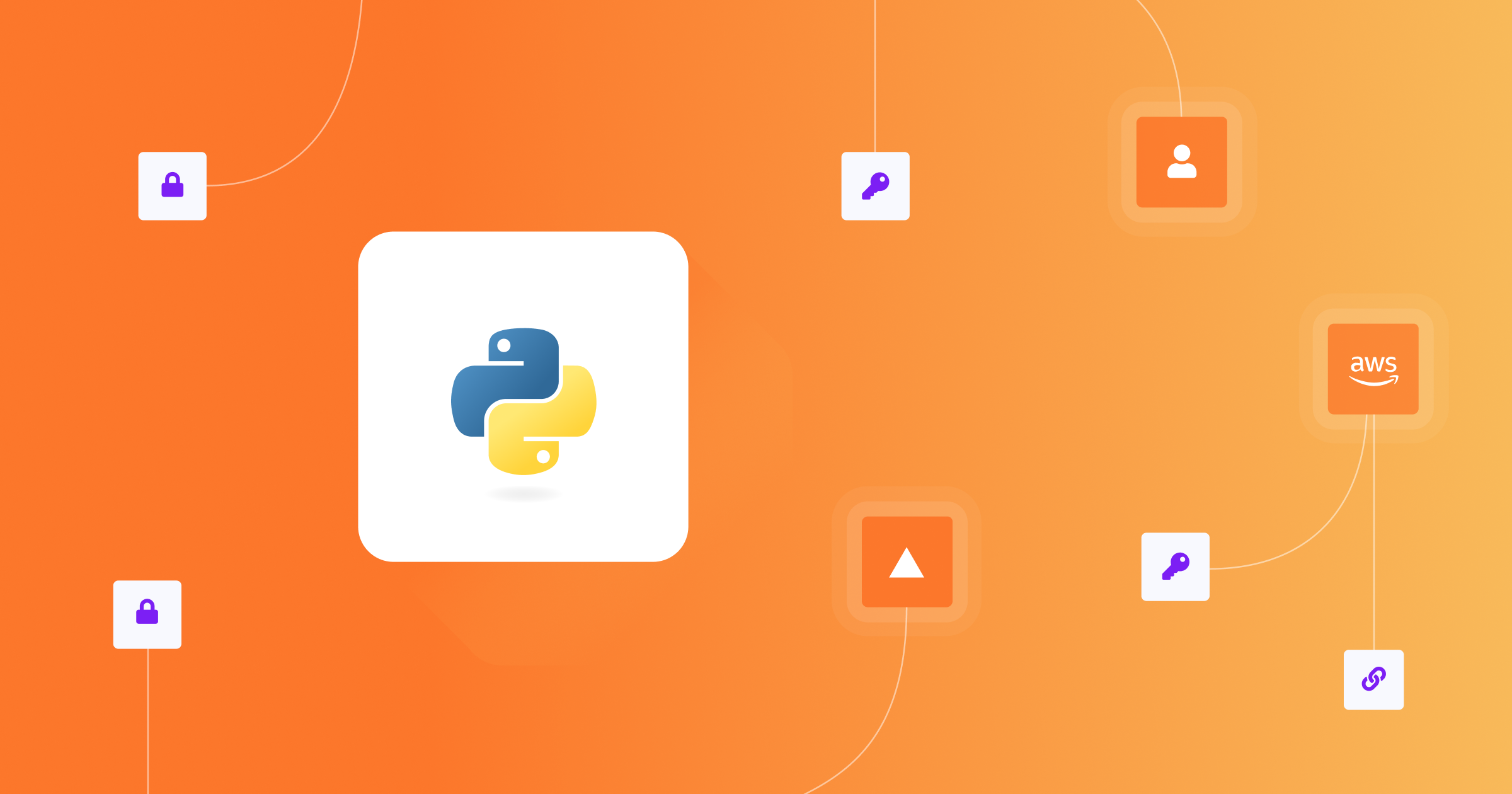
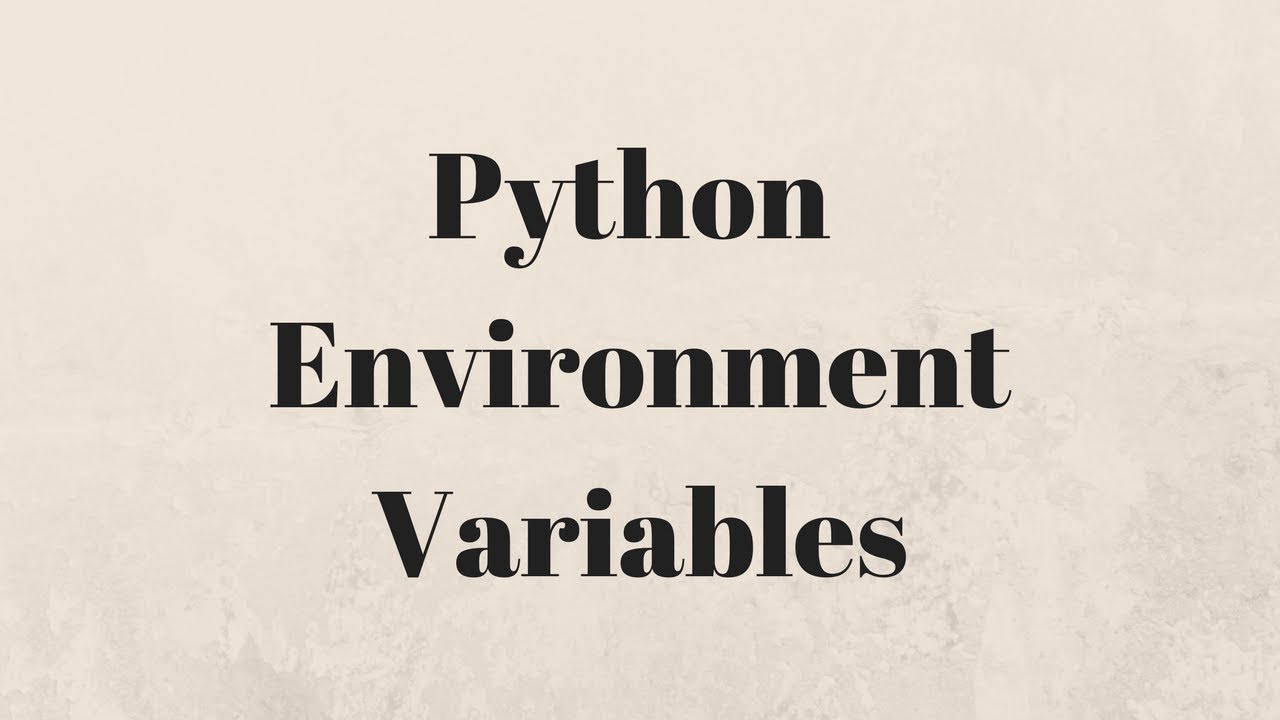
![Global Variable in Python With Examples [Updated] | Simplilearn Global Variable In Python With Examples [Updated] | Simplilearn](https://www.simplilearn.com/ice9/free_resources_article_thumb/GlobalVariableinPython_5.png)

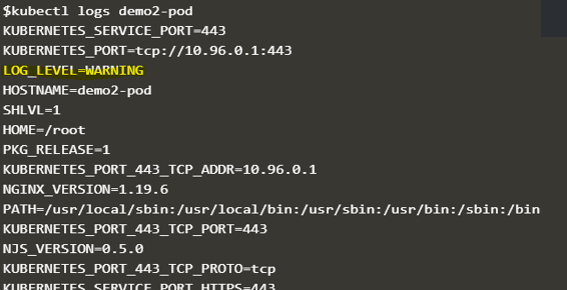
![Global Variable in Python With Examples [Updated] | Simplilearn Global Variable In Python With Examples [Updated] | Simplilearn](https://www.simplilearn.com/ice9/free_resources_article_thumb/GlobalVariableinPython_1.png)
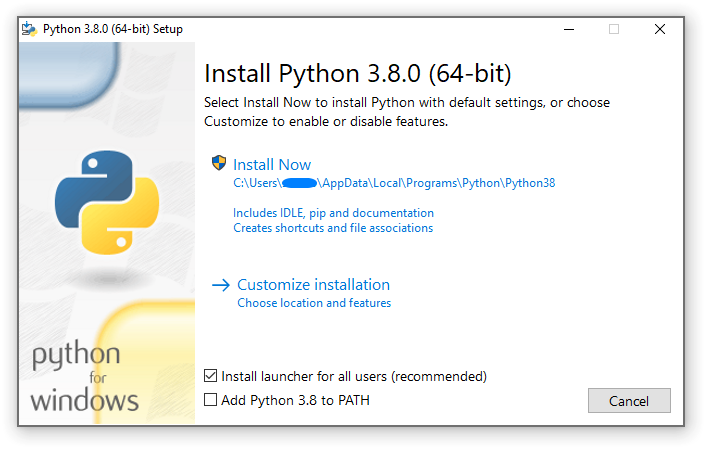
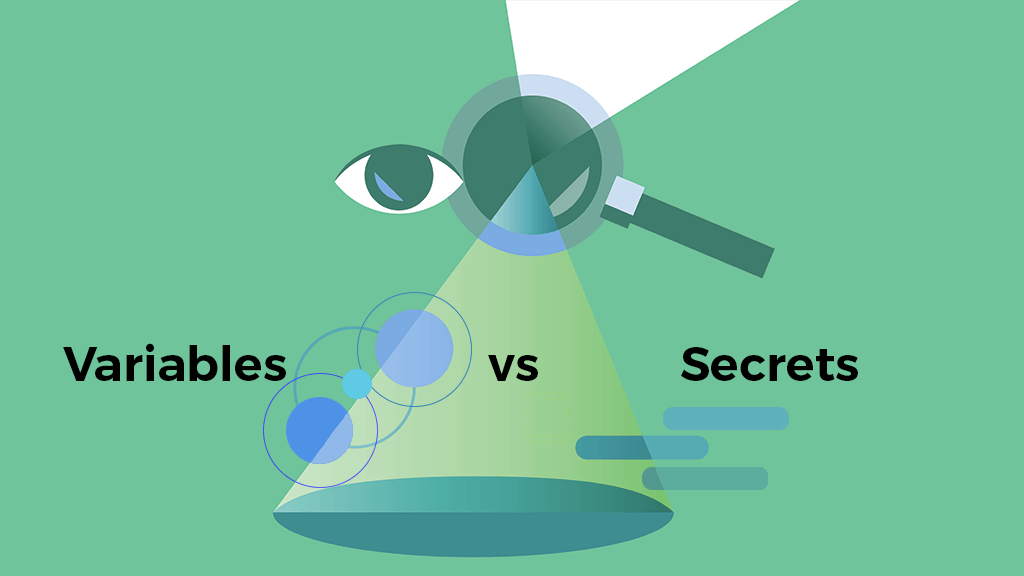
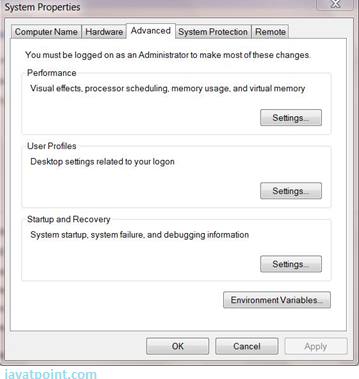
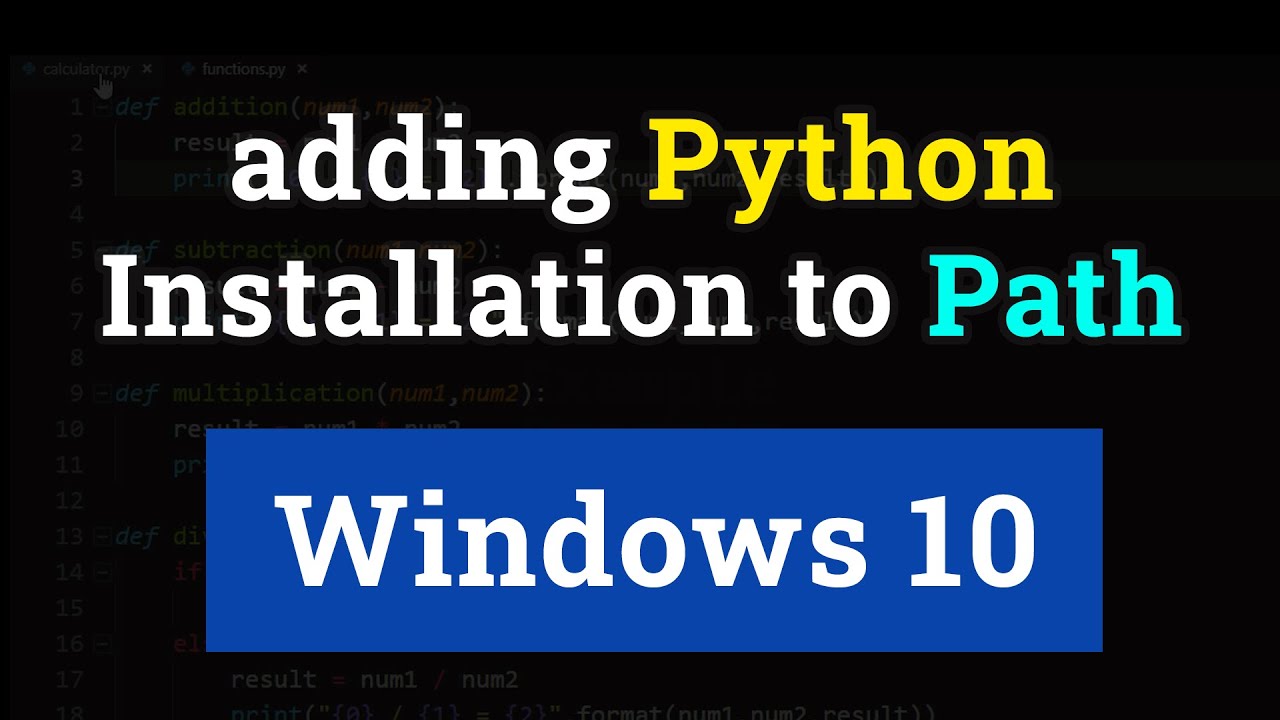
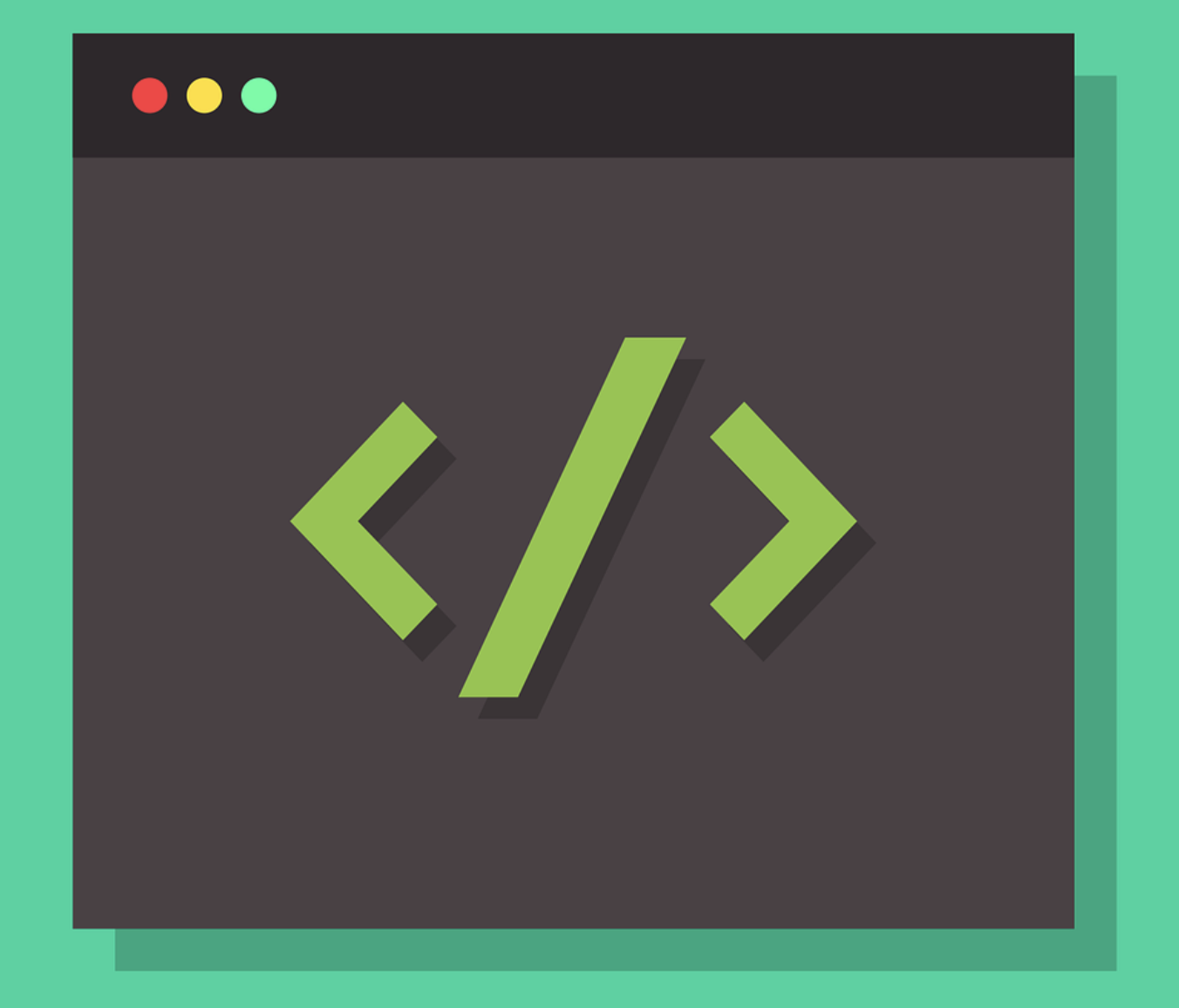

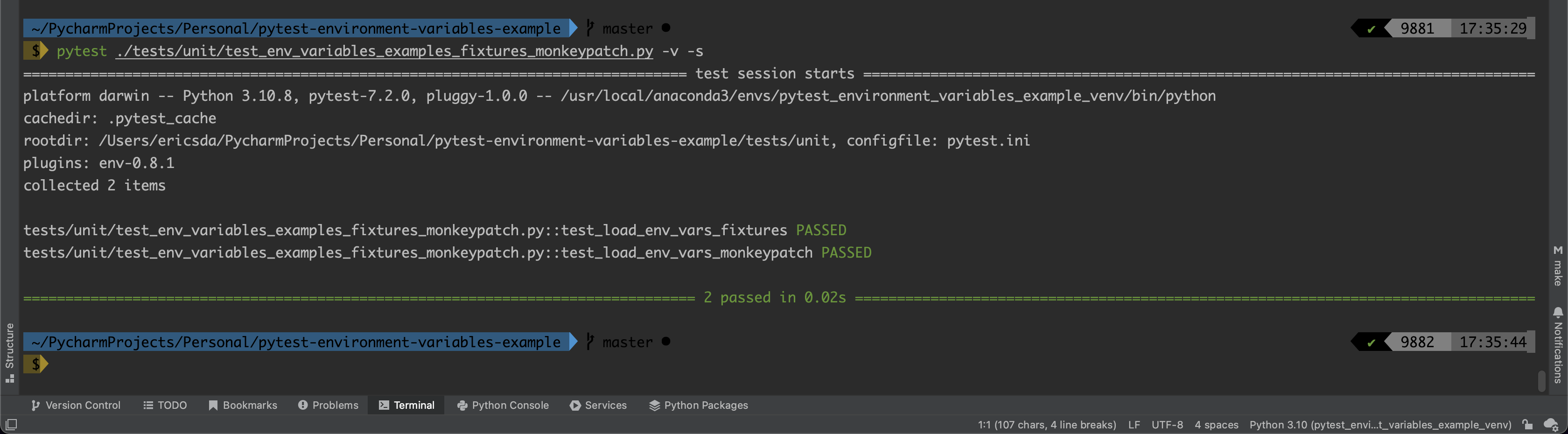
![Global Variable in Python With Examples [Updated] | Simplilearn Global Variable In Python With Examples [Updated] | Simplilearn](https://www.simplilearn.com/ice9/free_resources_article_thumb/GlobalVariableinPython_11.png)
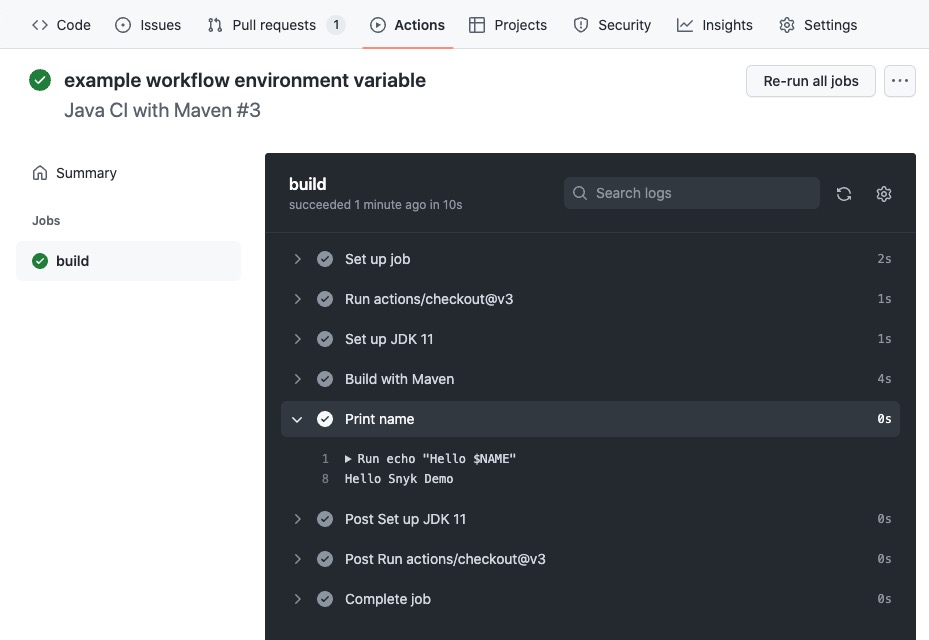

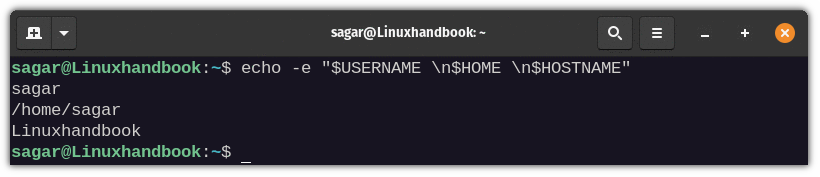
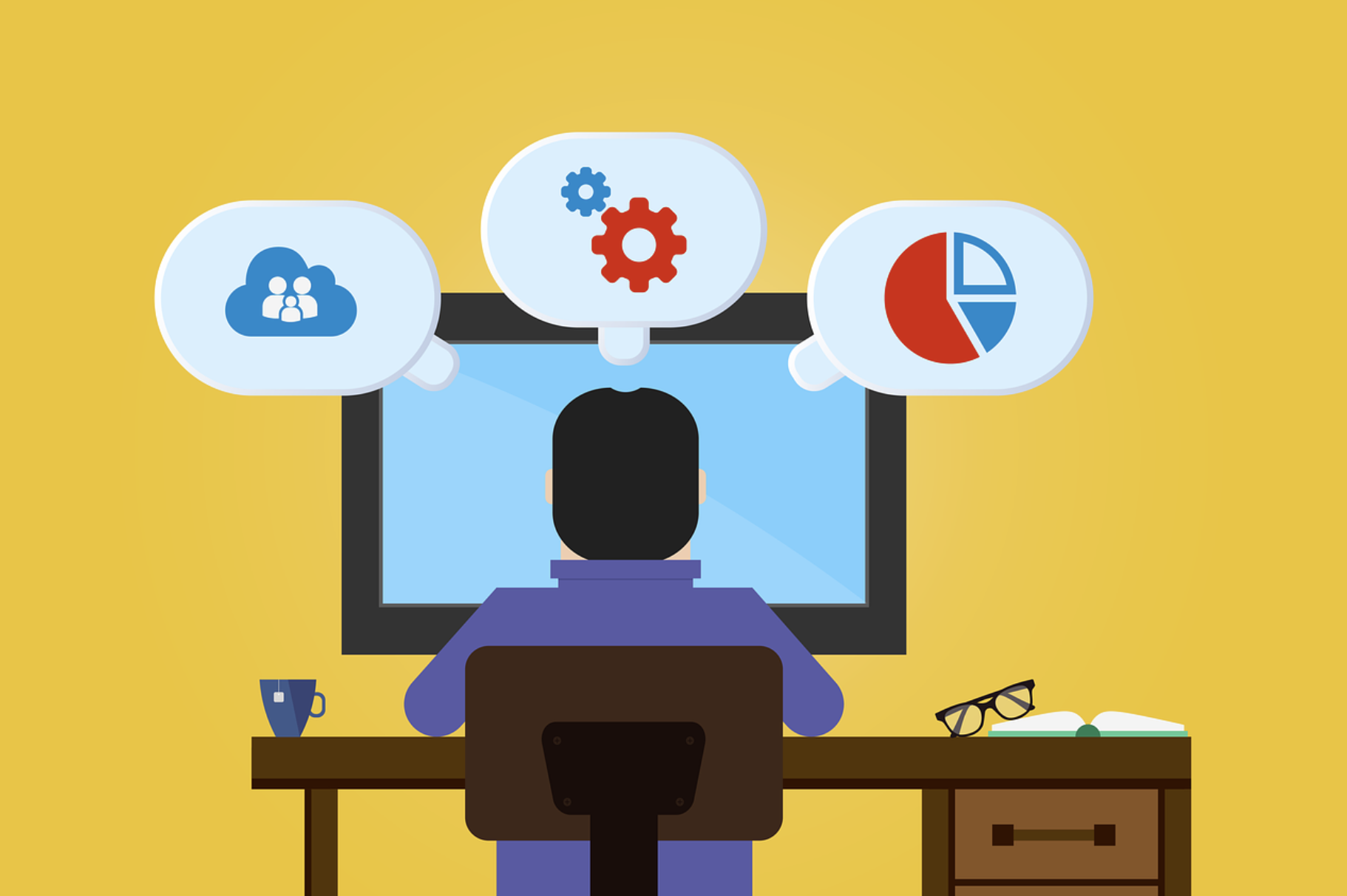
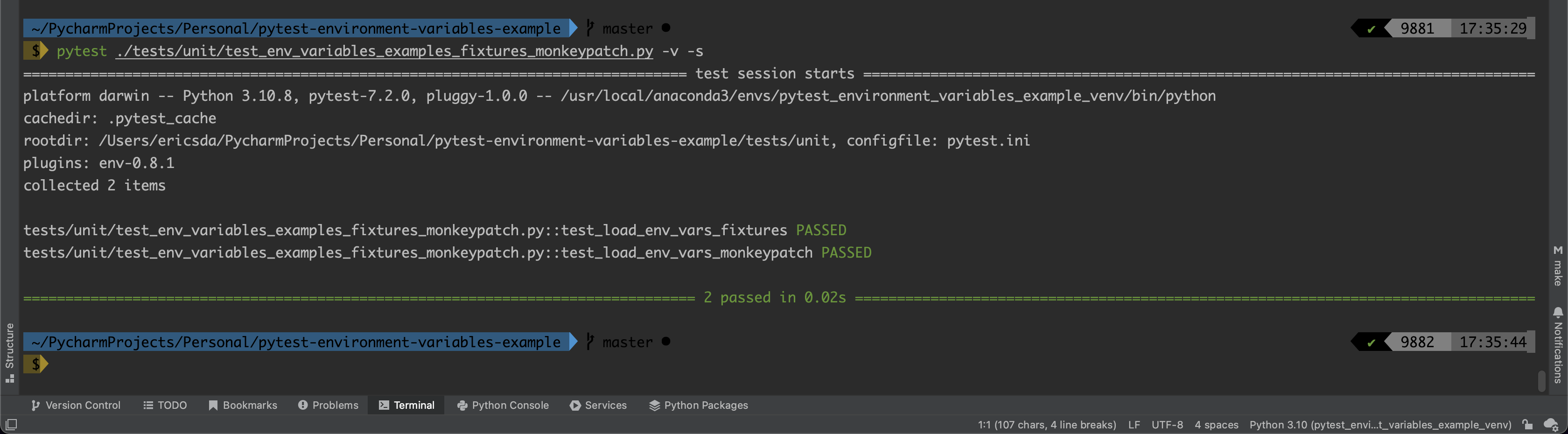
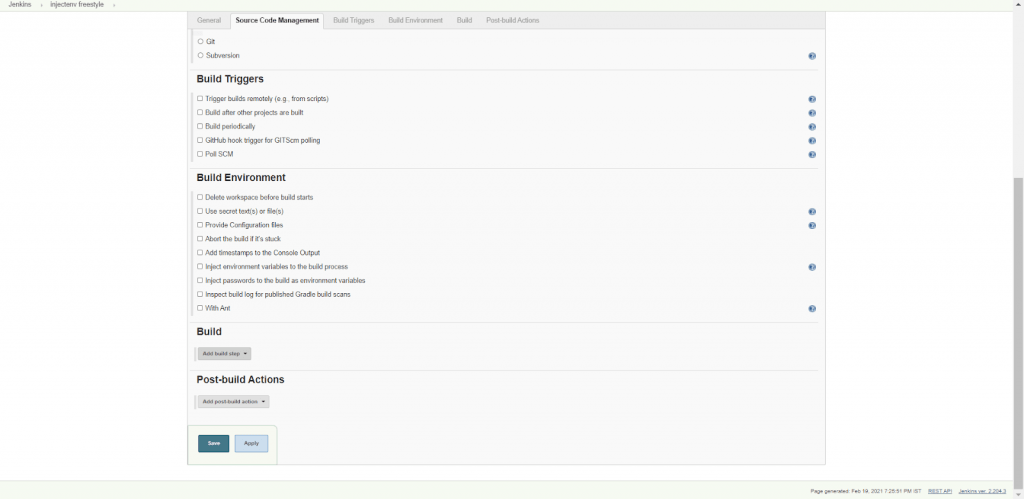


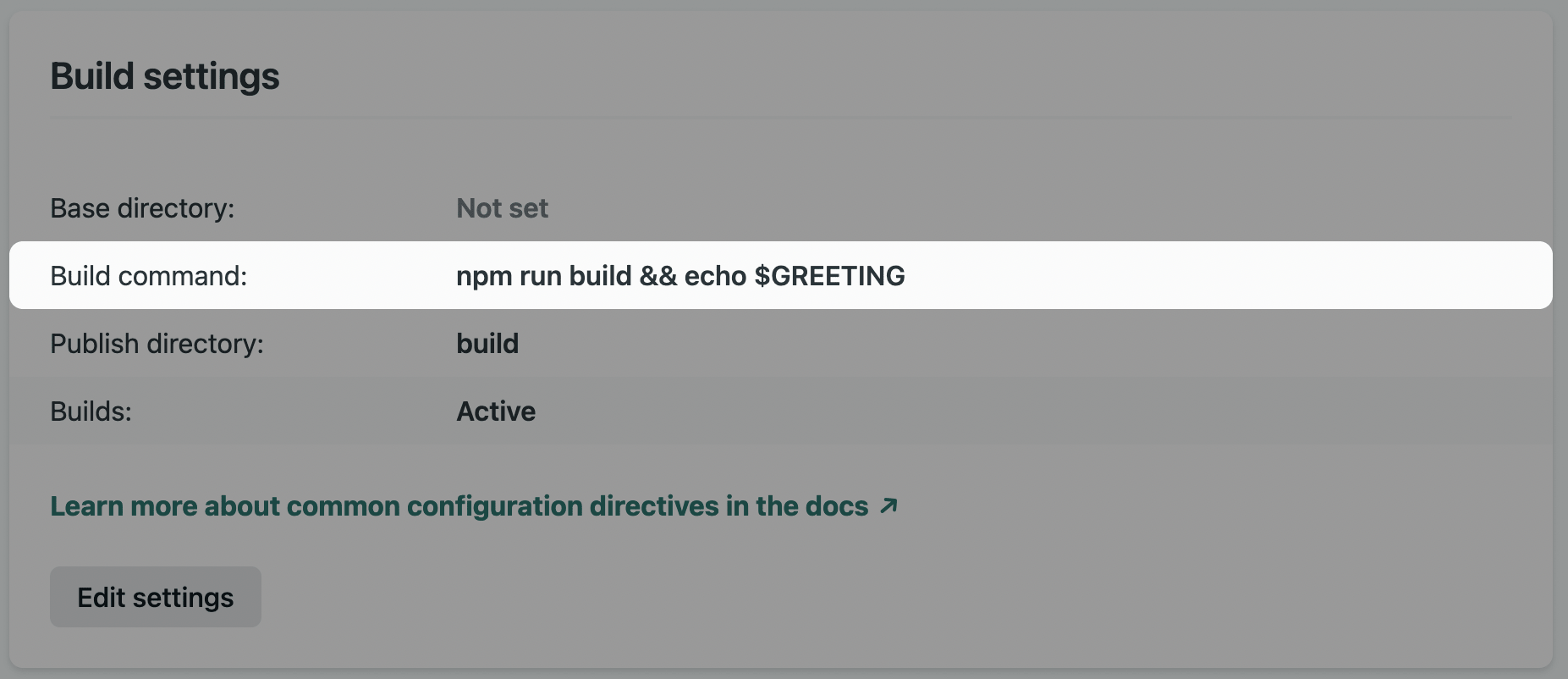
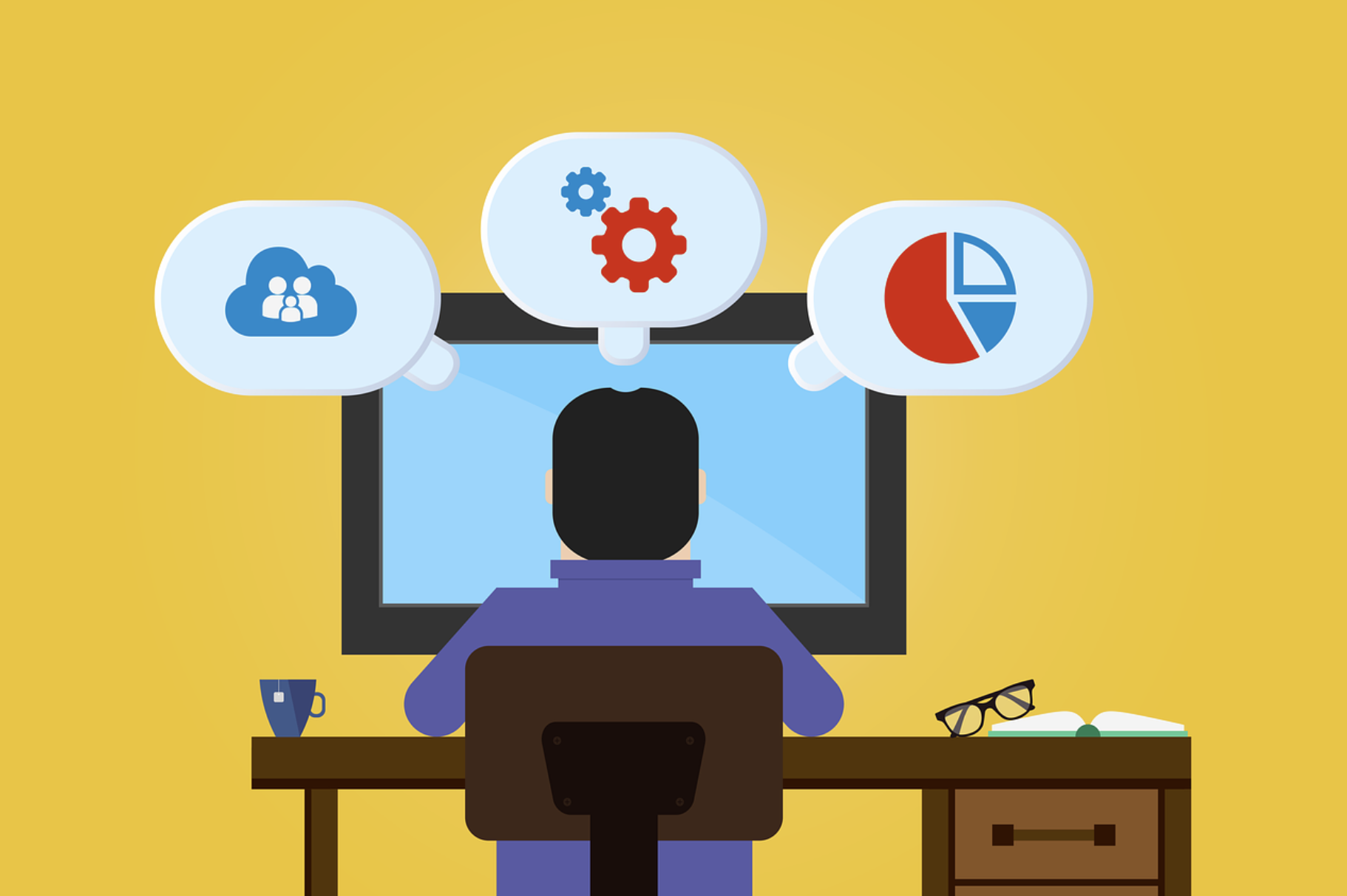
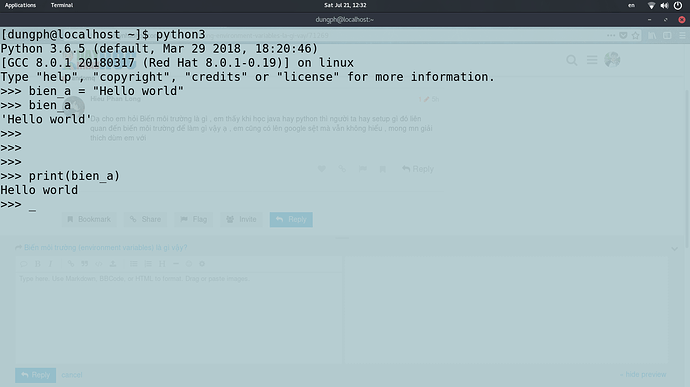
![Global Variable in Python With Examples [Updated] | Simplilearn Global Variable In Python With Examples [Updated] | Simplilearn](https://www.simplilearn.com/ice9/free_resources_article_thumb/GlobalVariableinPython_1.png)
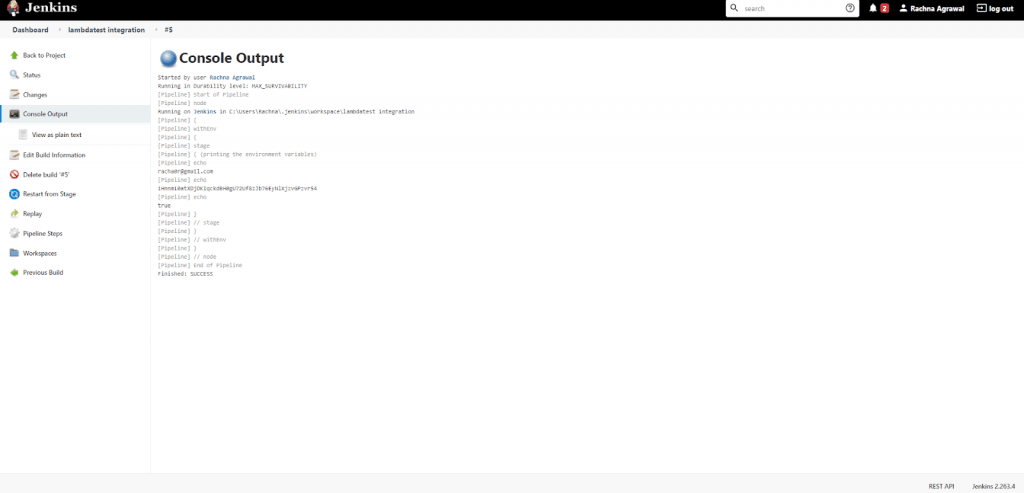
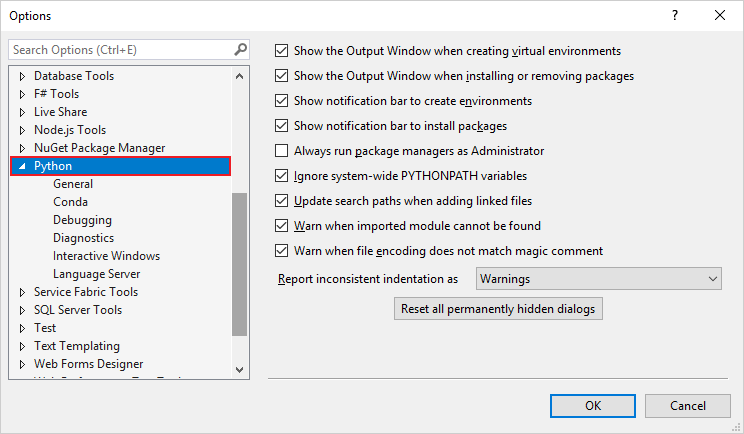
Article link: python print environment variables.
Learn more about the topic python print environment variables.
- Environment Variables in Python – Read, Print, Set – AskPython
- How can I access environment variables in Python?
- How to list all the environment variables in Python – Educative.io
- Python Read Environment Variable – Scaler Topics
- Environment Variables for Java Applications – PATH, CLASSPATH …
- Read Environment Variable in Python (Windows) – YouTube
- Linux List All Environment Variables Command – nixCraft
- Python Access Environment variable values
- Python: Environment variables – w3resource
- Using Environment Variables in Python – Datagy
- Environment Variables in Python – Read, Print, Set
- Python Read Environment Variable – Scaler Topics
- Access environment variable values in Python – Tutorialspoint
See more: https://nhanvietluanvan.com/luat-hoc