Python Prepend To List
Python is a popular programming language known for its simplicity and versatility. It offers a wide range of functionalities, including the ability to manipulate lists. In this article, we will explore the concept of “prepending” in Python, its significance, and various methods to prepend elements to a list.
What is Python?
Python is a high-level, interpreted programming language that emphasizes code readability and simplicity. It was created in the late 1980s by Guido van Rossum and has since gained immense popularity among developers due to its ease of use, vast standard library, and active community support. Python is widely used for web development, data analysis, artificial intelligence, and much more.
What is a list in Python?
In Python, a list is an ordered collection of items enclosed within square brackets ([]), separated by commas. It is a versatile data structure that can hold different types of elements, such as numbers, strings, objects, and even other lists. Lists in Python are mutable, meaning that their elements can be modified.
What is ‘prepend’ in Python?
The term “prepend” refers to the action of adding an element or a list of elements at the beginning of an existing list. It allows you to insert new items before the existing ones, effectively shifting the current elements to the right.
How to prepend an element to a list in Python using the ‘+’ operator?
Python provides the ‘+’ operator, which performs concatenation on lists. To prepend an element to a list, you can simply use this operator.
“`python
my_list = [2, 3, 4]
new_element = 1
my_list = [new_element] + my_list
“`
In the above example, the variable `my_list` is initially `[2, 3, 4]`. By using the ‘+’ operator, we concatenate a new list `[1]` with the existing list, resulting in `[1, 2, 3, 4]`. The new element is effectively added to the beginning of the list.
How to prepend an element to a list in Python using the list.insert() method?
Python offers the `list.insert()` method, which allows you to insert an element at any desired position within a list. In the case of prepending, you need to specify an index of 0 to insert the element at the beginning.
“`python
my_list = [2, 3, 4]
new_element = 1
my_list.insert(0, new_element)
“`
Here, the `list.insert()` method is used to insert the `new_element` at index 0 of `my_list`. As a result, the element is effectively prepended to the list.
How to prepend multiple elements to a list in Python using the ‘+’ operator?
To prepend multiple elements to a list using the ‘+’ operator, you can create a new list containing the elements you want to prepend and concatenate it with the original list.
“`python
my_list = [2, 3, 4]
new_elements = [0, 1]
my_list = new_elements + my_list
“`
In the above example, the `new_elements` list contains the elements `[0, 1]`. By using the ‘+’ operator, we concatenate this list with the `my_list`, resulting in `[0, 1, 2, 3, 4]`. The new elements are effectively added to the beginning of the list.
How to prepend multiple elements to a list in Python using the list.insert() method?
Similarly, you can use the `list.insert()` method to prepend multiple elements to a list. By specifying an index of 0, you can insert the elements at the beginning.
“`python
my_list = [2, 3, 4]
new_elements = [0, 1]
for i in range(len(new_elements)-1, -1, -1):
my_list.insert(0, new_elements[i])
“`
In this example, a loop iterates over the `new_elements` list, starting from the last index and moving towards the first index. The `list.insert()` method is used to insert the elements at index 0 of `my_list`, effectively prepending them to the list.
Prepending a list to another list in Python
In Python, you can also prepend one list to another list. This can be achieved using the ‘+’ operator or the `list.insert()` method.
Using the ‘+’ operator:
“`python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1 = list2 + list1
“`
Here, the `list2` is concatenated with `list1` using the ‘+’ operator, resulting in `[4, 5, 6, 1, 2, 3]`. The elements of `list2` are effectively prepended to `list1`.
Using the `list.insert()` method:
“`python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
for i in range(len(list2)-1, -1, -1):
list1.insert(0, list2[i])
“`
Similarly, a loop iterates over `list2`, starting from the last index and moving towards the first index. The elements are then inserted at index 0 of `list1`, effectively prepending `list2` to `list1`.
Prepending a string to a list in Python
In Python, you can also prepend a string to a list by converting the string to a list and using the methods mentioned above.
“`python
my_list = [1, 2, 3]
my_string = ‘Hello’
my_list = list(my_string) + my_list
“`
In this example, the `my_string` is converted to a list of characters using the `list()` function. Then, the ‘+’ operator is used to concatenate the string list with `my_list`, effectively prepending the string to the list.
Advantages and disadvantages of prepending elements to a list in Python
Prepending elements to a list in Python can be useful in certain scenarios, but it also has its pros and cons.
Advantages:
1. Efficient insertion: Prepending an element or a list to the beginning of a list is generally more efficient than appending at the end because it avoids the need to shift all the existing elements.
2. Maintaining order: Prepending allows you to maintain the order of elements in a list. This can be important in scenarios where the ordering of elements holds significance.
Disadvantages:
1. Complexity: If you frequently prepend elements to a large list, the complexity of the operation can increase. This is because the existing elements need to be shifted to accommodate the new ones.
2. Modification of existing data: Prepending elements to a list modifies the existing list. If the original list needs to be preserved, creating a new list might be a better approach.
In conclusion, Python provides various methods to prepend elements to a list, including the ‘+’ operator and the `list.insert()` method. These methods allow you to efficiently modify the list by adding new elements at the beginning. Understanding how to prepend elements in Python can be valuable when working with lists and manipulating their contents.
FAQs:
Q: Can I prepend elements to a list in Python without modifying the original list?
A: No, the act of prepending elements to a list modifies the original list. If you want to preserve the original list, you can create a copy and prepend elements to the new list.
Q: Is there a limit to the number of elements that can be prepended to a list?
A: There is no inherent limit to the number of elements that can be prepended to a list. However, the performance may degrade as the list grows larger due to the need to shift existing elements.
Q: Can I prepend elements to a list using a different method?
A: Yes, besides the methods mentioned in this article, you can also use list comprehension or other built-in functions to prepend elements to a list in Python.
Q: Can I prepend elements to a list that contains different types of data?
A: Yes, Python lists can hold elements of different types. You can prepend elements of any valid data type to a list.
Q: Is it possible to prepend elements to the beginning of a string in Python?
A: No, strings in Python are immutable, meaning that they cannot be modified once created. However, you can convert the string to a list, prepend the elements, and then convert it back to a string if needed.
Q: Are there any alternative data structures in Python for efficient insertion at the beginning?
A: Yes, if the requirement for efficient insertion at the beginning is crucial, you can consider using other data structures such as a deque from the collections module, which provides efficient append and prepend operations.
How Do I Prepend To A Short Python List?
Keywords searched by users: python prepend to list python prepend to string, python reverse list, python prepend string to list, list prepend c#, python list, list prepend cmake, python prepend list to another list, python list insert
Categories: Top 100 Python Prepend To List
See more here: nhanvietluanvan.com
Python Prepend To String
To prepend a string in Python, there are multiple approaches. Let’s start with the most straightforward method: using the concatenation operator. The concatenation operator (+) enables the combination of two strings into a single string. We can utilize this operator to append a new string at the beginning of an existing one.
“`python
original_string = “World”
new_string = “Hello ”
prepended_string = new_string + original_string
print(prepended_string) # Output: Hello World
“`
In the example above, “Hello ” is concatenated with “World” to create the prepended string “Hello World”. The concatenation operator provides a simple and effective way to prepend a string.
Another approach to prepend a string in Python is by using string formatting. Python provides the `format()` method to insert data into strings. By applying string formatting, we can prepend a string efficiently.
“`python
original_string = “World”
new_string = “Hello”
prepended_string = “{} {}”.format(new_string, original_string)
print(prepended_string) # Output: Hello World
“`
By utilizing curly brackets ({}) as placeholders in the format string and passing the desired strings as arguments, we can prepend the new string to the existing one.
Python also offers an even simpler way to prepend a string using f-strings. Introduced from Python 3.6 onwards, f-strings provide a concise and intuitive way to format strings by allowing variables to be directly embedded within string literals. Let’s see how to use f-strings to prepend a string:
“`python
original_string = “World”
new_string = “Hello”
prepended_string = f”{new_string} {original_string}”
print(prepended_string) # Output: Hello World
“`
In this example, the f-string is defined by placing an “f” before the opening quotation mark. Variables can then be enclosed in curly braces within the string literal. By incorporating the variables `new_string` and `original_string` directly into the f-string, we obtain the desired prepended string.
Besides concatenation, string formatting, and f-strings, there are a few additional methods to prepend a string in Python, such as utilizing the `join()` method or creating a custom function using slicing or list manipulation. However, the approaches mentioned earlier are the most commonly used and recommended methods for string prepending.
Now, let’s address some frequently asked questions related to string prepending in Python.
### FAQs:
**Q1: Can I only prepend one string at a time?**
A1: No, you can prepend multiple strings at once. The concatenation operator, string formatting, and f-strings enable the combination of multiple strings and variables in a single prepended string.
**Q2: Is there a limit to the length of the strings I can prepend?**
A2: No, there is no inherent limit to the length of the strings you can prepend. However, keep in mind that extremely large strings may impact memory usage and performance.
**Q3: Can I prepend numbers or other data types to strings?**
A3: Absolutely! Python allows you to prepend not only strings but also numbers, booleans, and other data types. Just ensure that the data type is converted to a string if needed.
**Q4: Are there any particular scenarios where one method is preferable over others?**
A4: The choice of method depends on the specific scenario and personal preference. However, f-strings are generally favored for their simplicity and readability.
**Q5: How can I handle special characters or escape sequences within the prepended strings?**
A5: Special characters and escape sequences can be included within the prepended string using the appropriate syntax. For example, to include a newline character, use “\n”.
In conclusion, Python provides several effective methods to prepend a string, including concatenation, string formatting, and f-strings. These methods offer flexibility and ease when it comes to manipulating strings. By understanding these techniques, you can efficiently prepend strings in your Python projects.
Python Reverse List
Introduction:
In Python, a list is an ordered collection of elements that can be modified. One common operation on lists is to reverse their order, which involves rearranging the elements in the list in the opposite direction. In this article, we will delve into various methods and techniques to reverse a list in Python, including built-in functions, slicing, and list comprehension. Additionally, we will address some frequently asked questions to provide a comprehensive understanding of reversing lists in Python.
Methods to Reverse a List in Python:
1. Using the reverse() Method:
Python lists have a built-in reverse() method that modifies the original list to reverse its elements in place. To use this method, simply apply it on the list as follows:
“`python
my_list = [1, 2, 3, 4, 5]
my_list.reverse()
print(my_list) # Output: [5, 4, 3, 2, 1]
“`
Note that reverse() does not return a new reversed list; instead, it alters the original list itself.
2. Utilizing the slicing technique:
Another approach to reverse a list is by using slicing. This technique involves specifying a step value of -1 in the slice notation, which facilitates the reversal. Consider the following code:
“`python
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
“`
Here, a new list, reversed_list, is created by slicing the original list (my_list) with a step value of -1. The resulting list contains the elements in reverse order.
3. Using the reversed() Function:
Python provides the reversed() function, which returns an iterator that yields the reversed elements of a sequence. To obtain a reversed list, this iterator can be converted into a list using the list() constructor. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
reversed_list = list(reversed(my_list))
print(reversed_list) # Output: [5, 4, 3, 2, 1]
“`
The reversed() function allows reversing not only lists but also other sequences such as tuples and strings.
4. Employing List Comprehension:
List comprehension is a concise and powerful approach to generate lists in Python. By combining list comprehension with the reversed() function, we can easily obtain a reversed list. Take a look at the following code snippet:
“`python
my_list = [1, 2, 3, 4, 5]
reversed_list = [x for x in reversed(my_list)]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
“`
In this example, the reversed() function returns an iterator, which is then used in the list comprehension to construct a new list with the elements in reverse order.
Frequently Asked Questions (FAQs):
Q1. Can I reverse a list in Python without modifying the original list?
Certainly! The methods mentioned earlier, like reverse(), alter the original list. However, if you want to reverse a list without modifying it, you can use the slicing technique. Consider the following code snippet:
“`python
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
“`
Here, the original list, my_list, remains unmodified, and the reversed list is assigned to reversed_list using slicing.
Q2. Which method is the fastest for reversing a large list in Python?
When it comes to efficiency, the reverse() method outperforms other approaches. Since it modifies the original list in place, it requires less memory and time compared to other methods. Thus, if performance is a significant concern, the reverse() method is the best option.
Q3. How does the reversed() function differ from the reverse() method?
The reverse() method directly modifies the list in place, altering its order. On the other hand, the reversed() function does not modify the original list but returns an iterator that yields the reversed elements. It allows you to obtain a reversed list by converting that iterator into a list using the list() constructor.
Q4. Is reversing a list in Python applicable to other data structures?
The techniques discussed in this article are primarily applicable to Python lists, which are mutable ordered collections. However, other data structures like arrays or collections.deque have their own methods or functions to reverse their elements.
Q5. Can I reverse a list of strings or custom objects in Python?
Absolutely! The methods described in this article work with lists containing strings, integers, floats, or even more complex objects. The same principles apply irrespective of the data type as long as the elements are comparable and mutable.
Conclusion:
Reversing a list is a common operation in Python, and understanding the various techniques available enables you to choose the most suitable approach based on your requirements. We explored methods such as the reverse() method, slicing, reversed() function, and list comprehension. Additionally, we addressed some frequently asked questions to clarify common concerns. By mastering the art of reversing lists, you can enhance your Python programming skills and effectively manipulate data in your applications.
Python Prepend String To List
Introduction:
Python provides a multitude of functionalities that make it an incredibly versatile programming language. One such useful feature is the ability to prepend a string to a list. This article will delve into how to prepend a string to a list in Python, exploring different approaches and discussing potential use cases.
Methods for Prepending a String to a List:
Method 1: Using the insert() method
The insert() method in Python allows us to insert an element at a specified position within a list. By leveraging this method, we can position a string at the beginning of a list. Here’s an example:
“`
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_string = ‘orange’
my_list.insert(0, my_string)
print(my_list)
“`
Output:
[‘orange’, ‘apple’, ‘banana’, ‘cherry’]
In the above example, we use the insert() method to insert the string `’orange’` at index 0, which places it at the beginning of the `my_list`.
Method 2: Using concatenation
Another way to prepend a string to a list is by using string concatenation. We can achieve this by creating a new list with the desired string as the first element and concatenating it with the original list. Here’s an example:
“`
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_string = ‘orange’
new_list = [my_string] + my_list
print(new_list)
“`
Output:
[‘orange’, ‘apple’, ‘banana’, ‘cherry’]
In the above example, we combine the string `’orange’` with `my_list` using the `+` operator, creating a new list `new_list` with `’orange’` as the first element.
Method 3: Using the extend() method
The extend() method can also be utilized to prepend a string to a list. This method allows us to append elements from an iterable (in this case, a list) to the end of another list. By using this method, we can extend the original list using a new list consisting of our desired string. Here’s an example:
“`
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_string = ‘orange’
my_list.extend([my_string])
print(my_list)
“`
Output:
[‘orange’, ‘apple’, ‘banana’, ‘cherry’]
In this example, we utilize the extend() method to append `[my_string]` to `my_list`, resulting in the string `’orange’` being prepended to the original list.
FAQs:
Q1: Can I prepend multiple strings to a list at once?
A1: Yes, you can prepend multiple strings to a list at once by simply extending the list with a new list containing all the desired strings. Here’s an example:
“`
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_strings = [‘orange’, ‘mango’, ‘grape’]
my_list = my_strings + my_list
print(my_list)
“`
Output:
[‘orange’, ‘mango’, ‘grape’, ‘apple’, ‘banana’, ‘cherry’]
By using the concatenation operator `+` to combine the `my_strings` list with `my_list`, all the specified strings are successfully prepended to the list.
Q2: How can I prepend a string to a list without modifying the original list?
A2: If you want to leave the original list unchanged, you can create a new list by concatenating the desired string with the original list. Here’s an example:
“`
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_string = ‘orange’
new_list = [my_string] + my_list
print(my_list) # Output: [‘apple’, ‘banana’, ‘cherry’]
print(new_list) # Output: [‘orange’, ‘apple’, ‘banana’, ‘cherry’]
“`
In this example, the original list `my_list` remains unaltered, while the string `’orange’` is prepended to the newly created list `new_list`.
Conclusion:
Prepending a string to a list in Python can be achieved using a variety of methods, such as insert(), concatenation, or extend(). These methods provide flexibility depending on your specific needs and allow you to easily manipulate and modify lists. By understanding these techniques, you can efficiently manipulate your lists in Python to suit your desired outcomes.
Images related to the topic python prepend to list
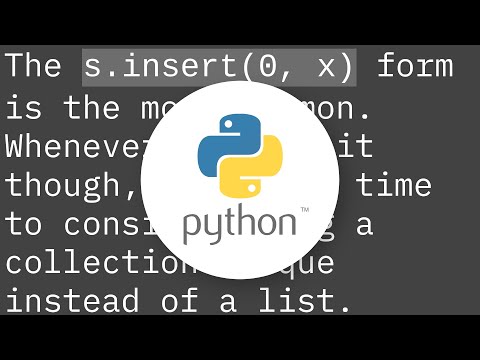
Found 5 images related to python prepend to list theme



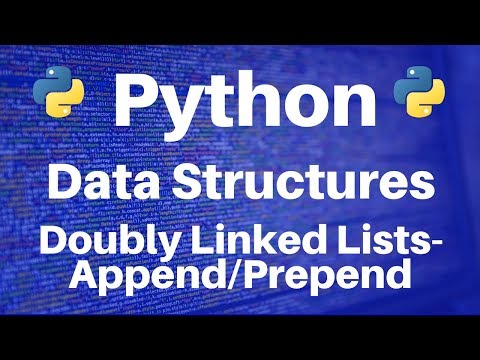

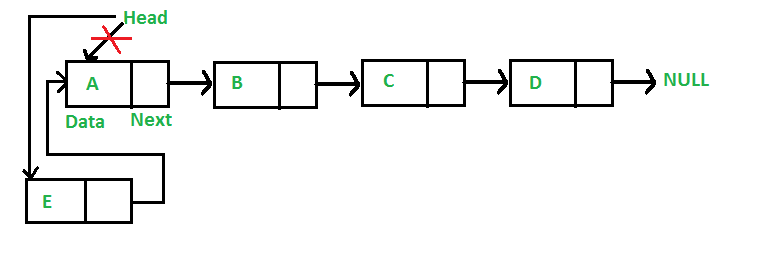
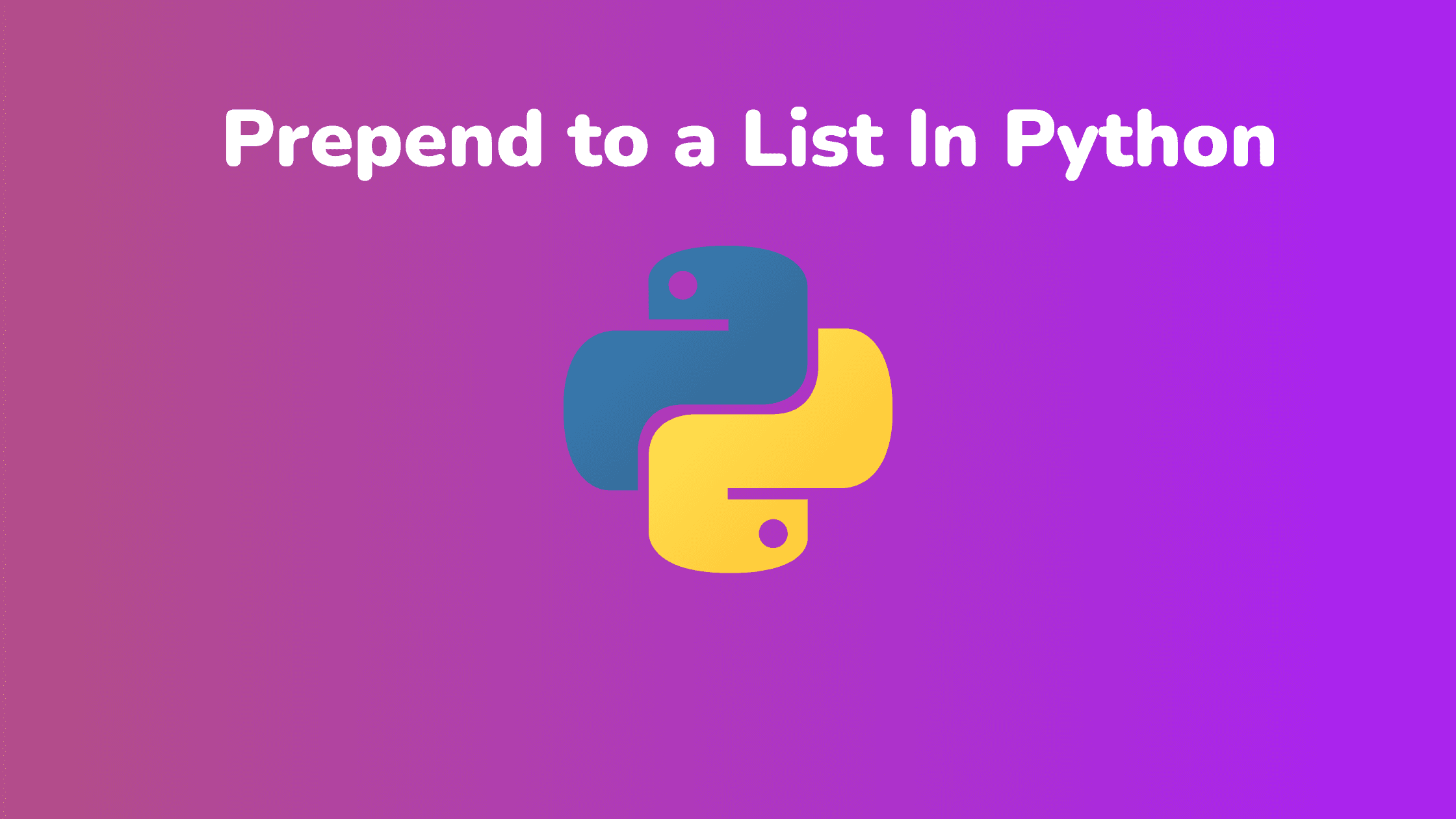
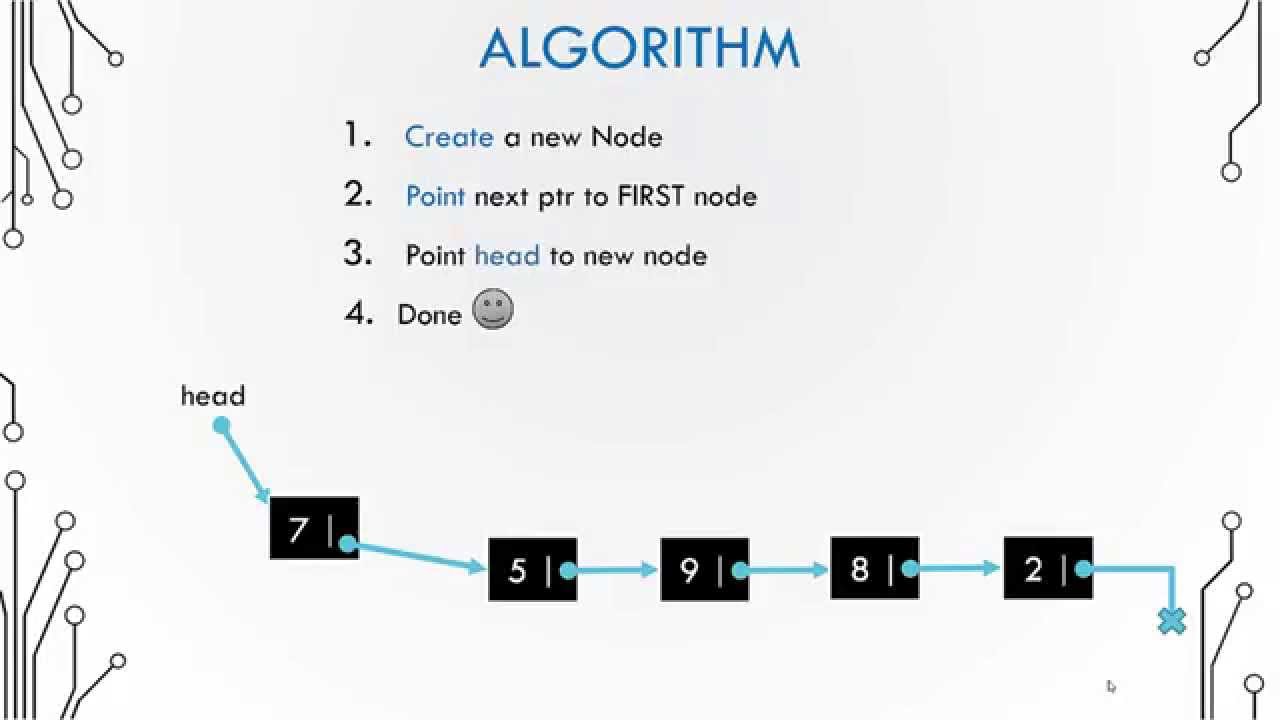
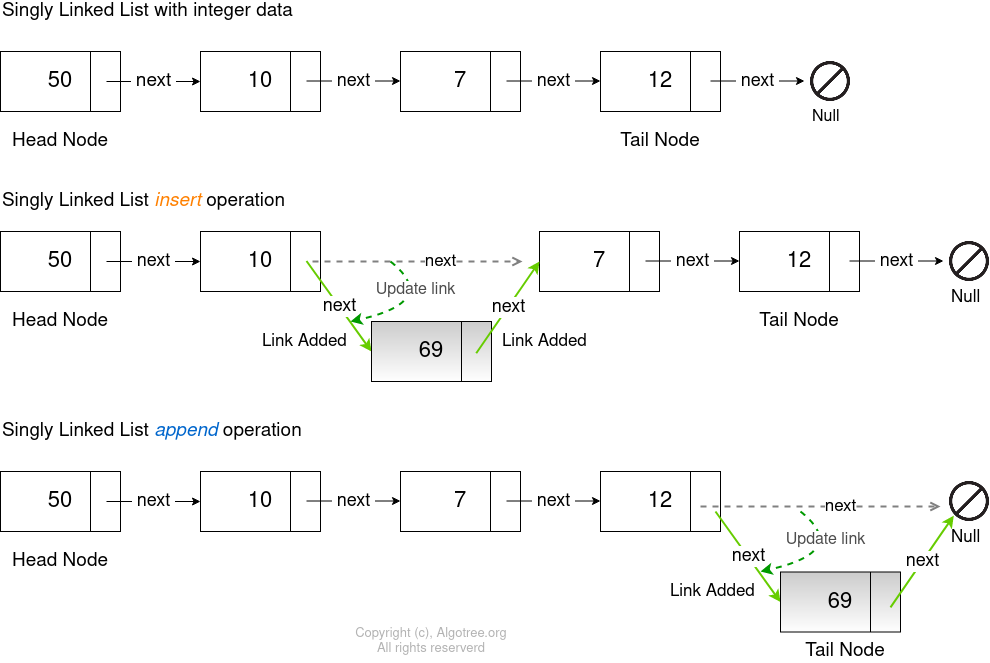

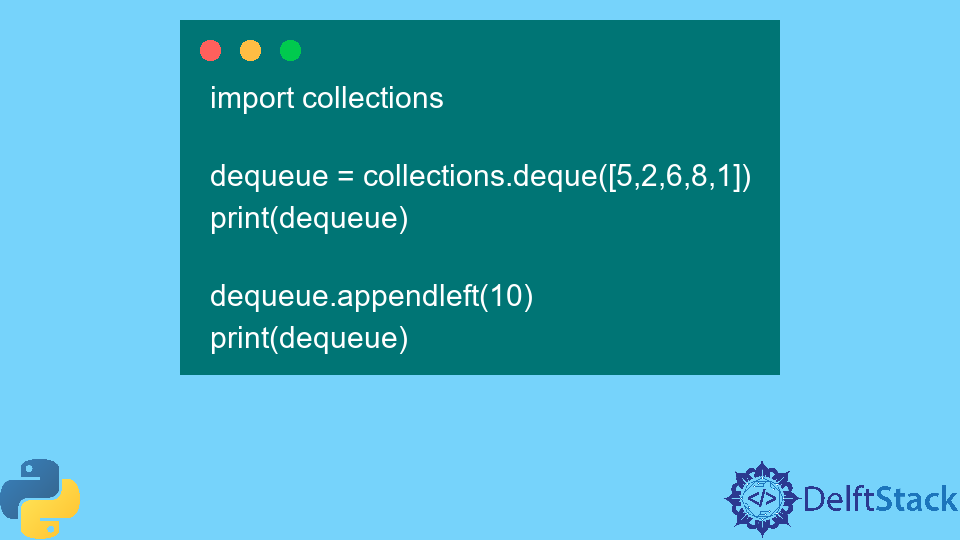

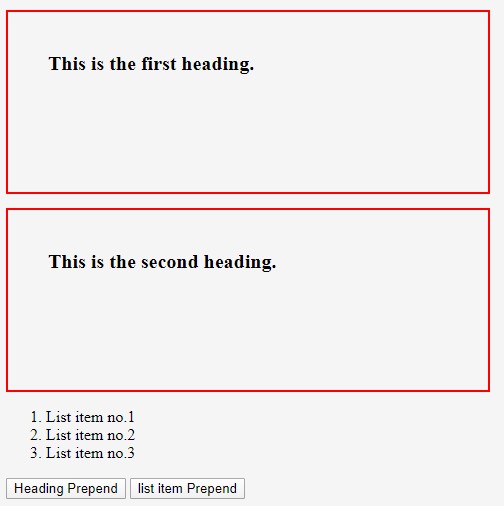
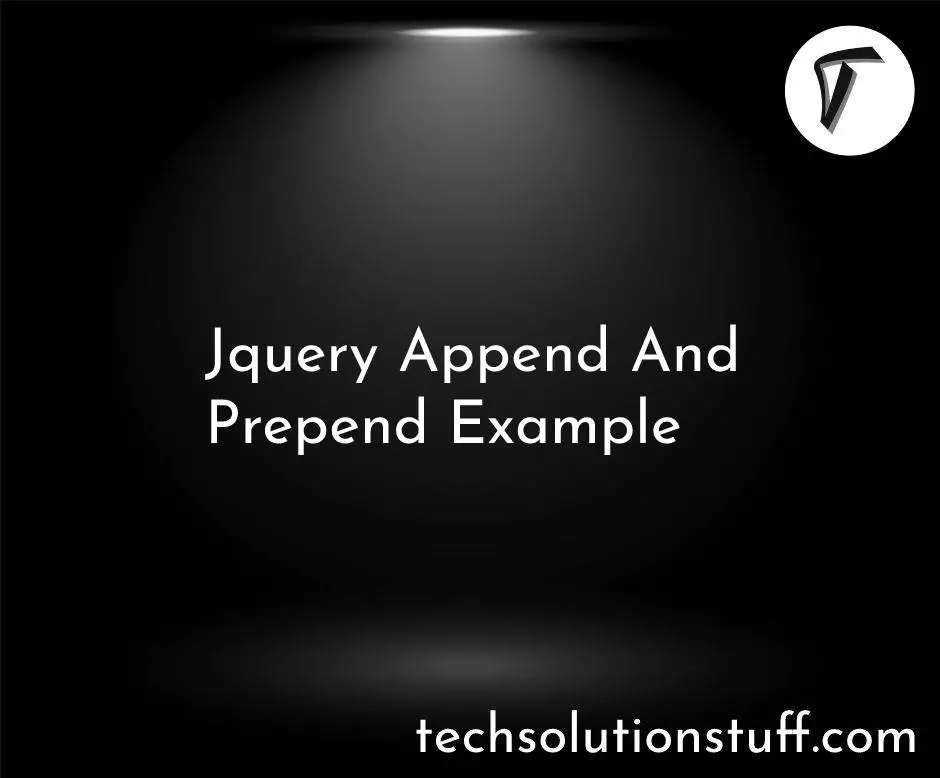
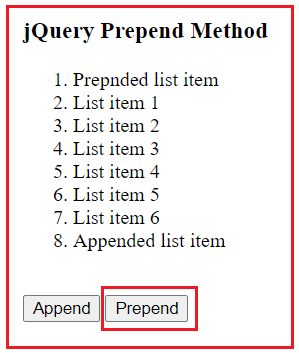
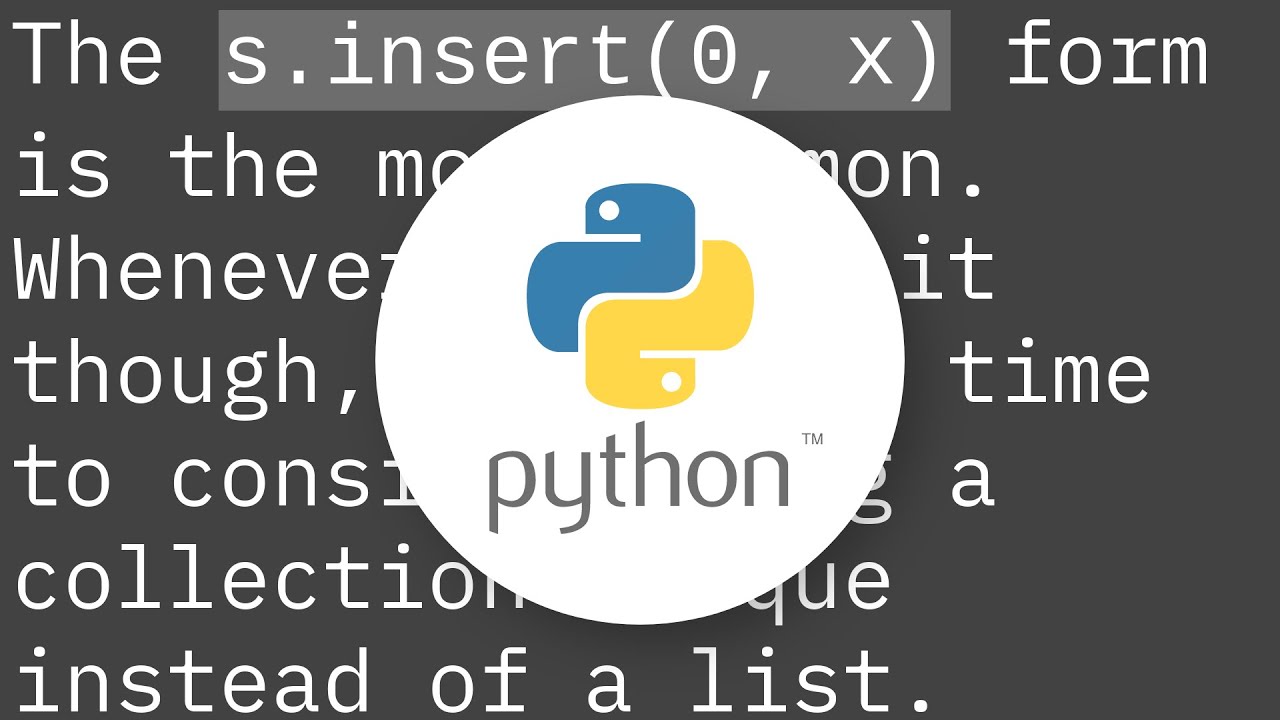



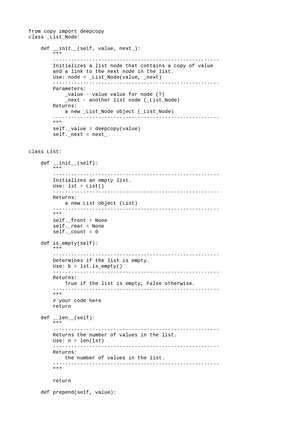

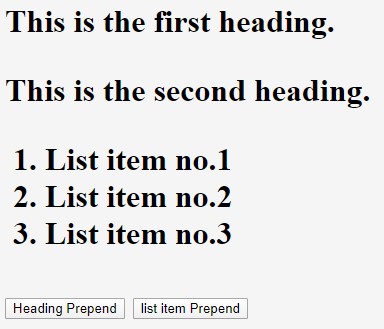
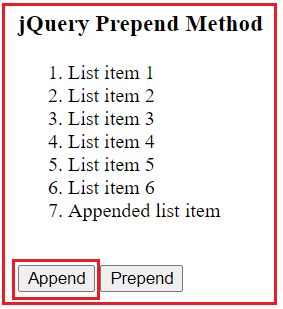
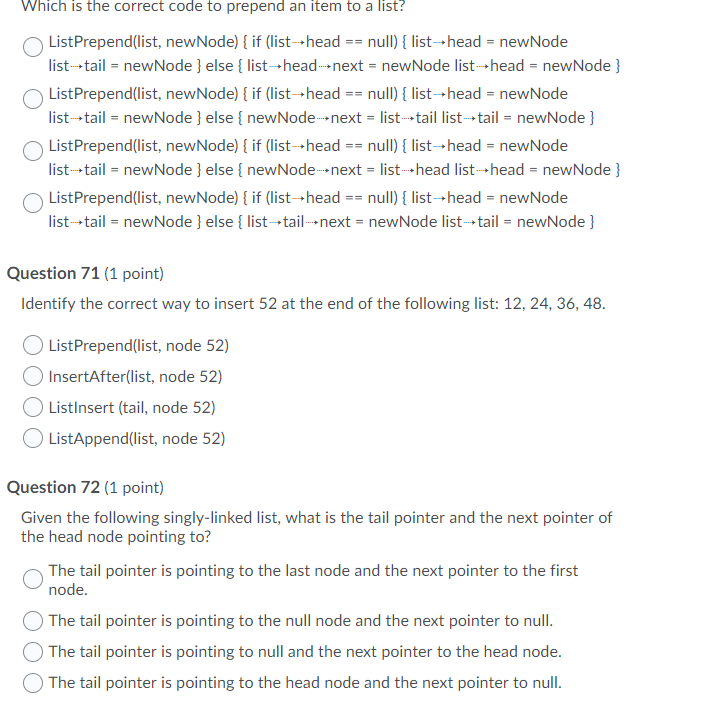
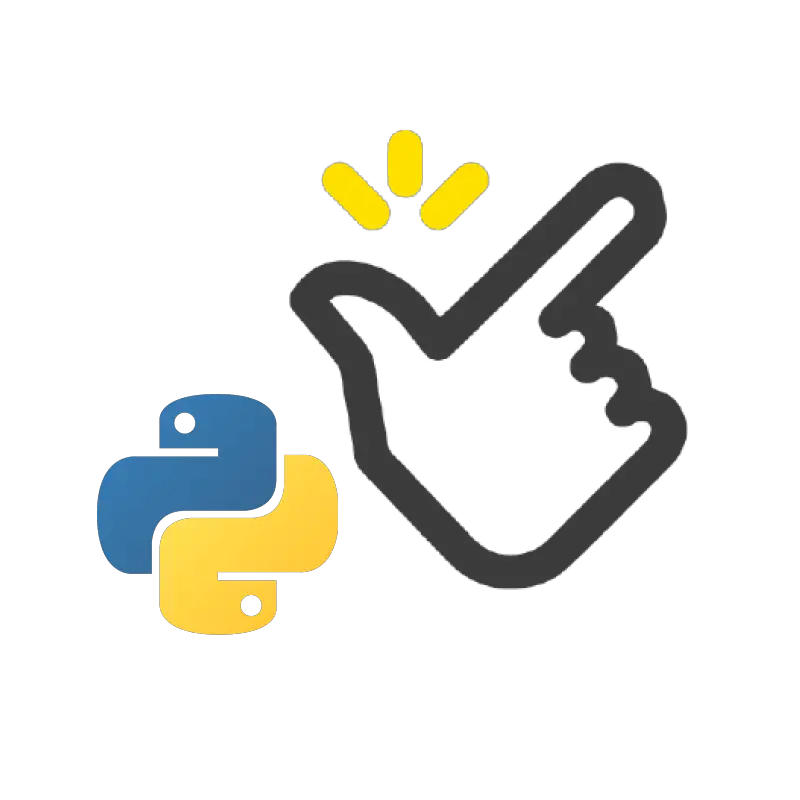


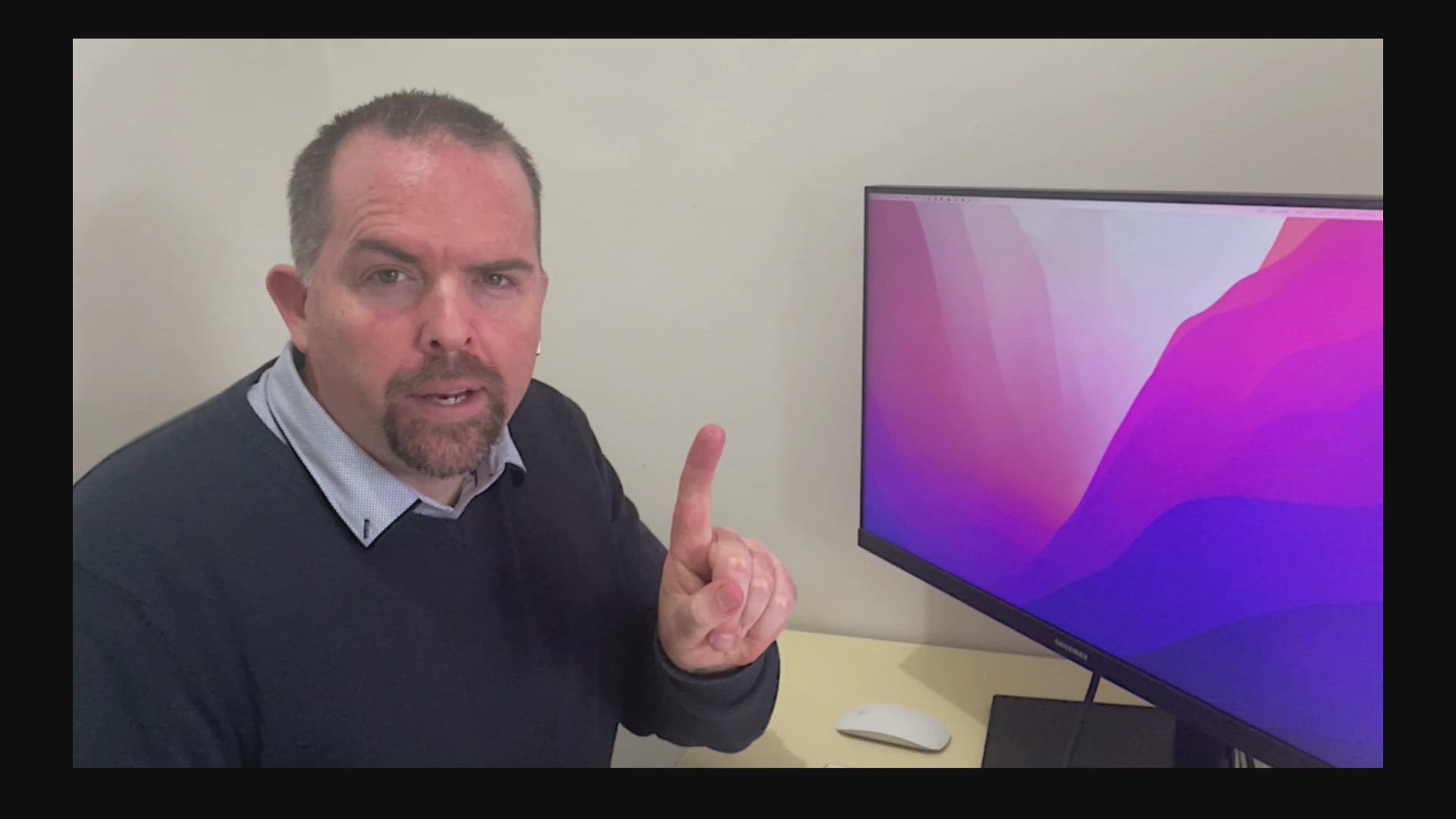
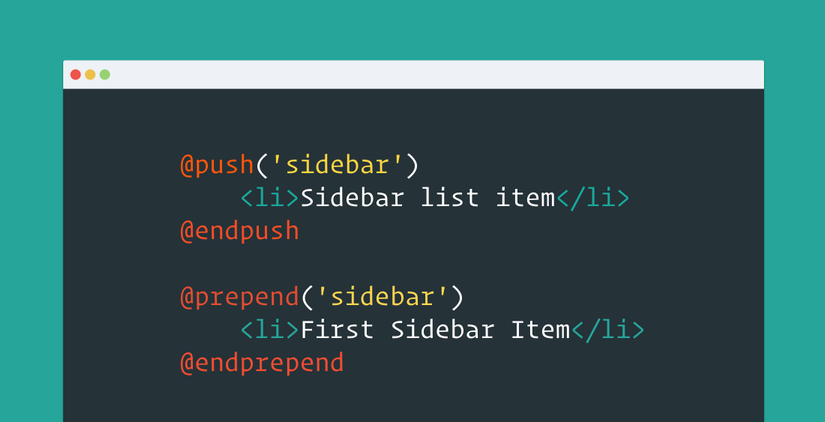

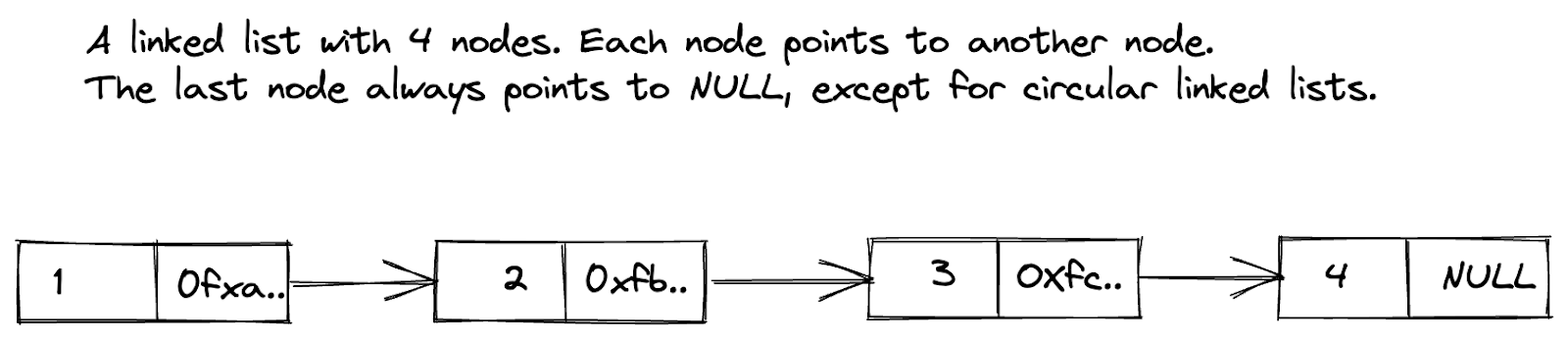

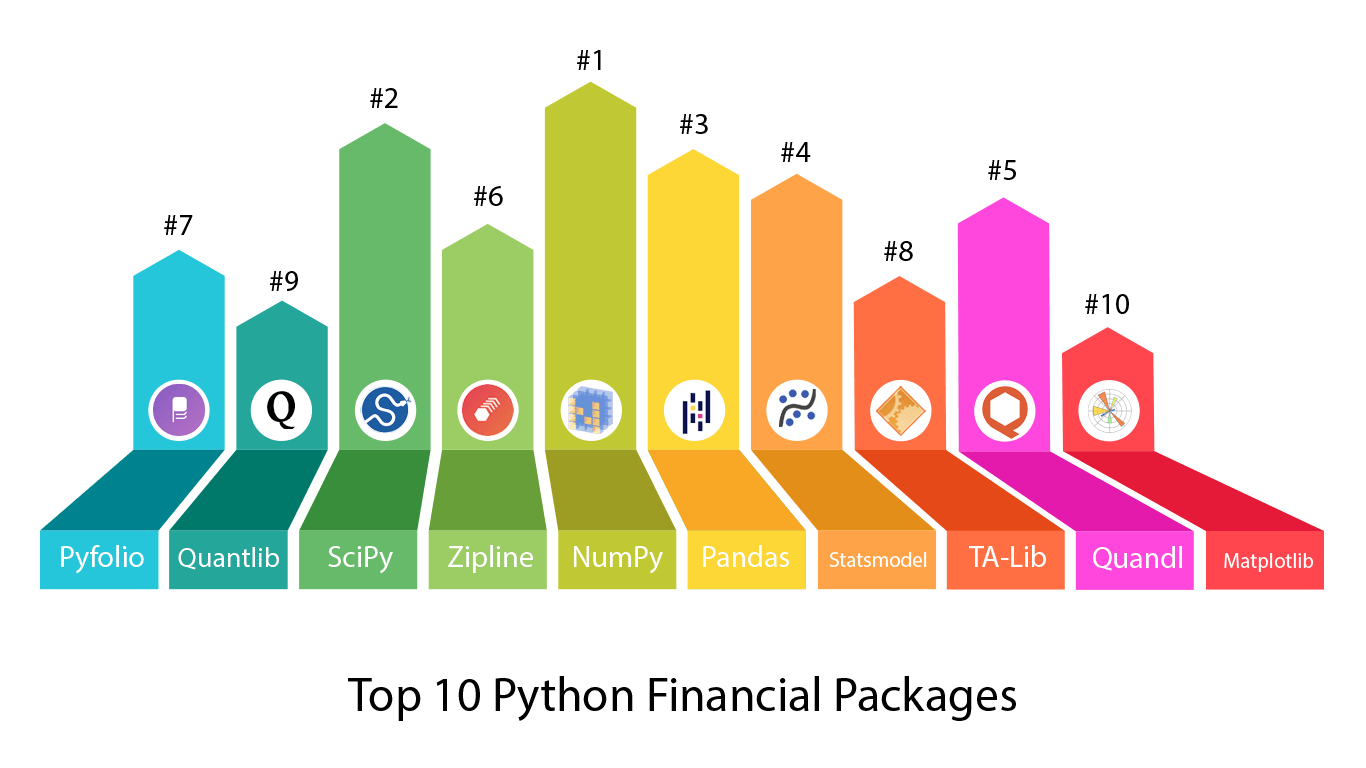
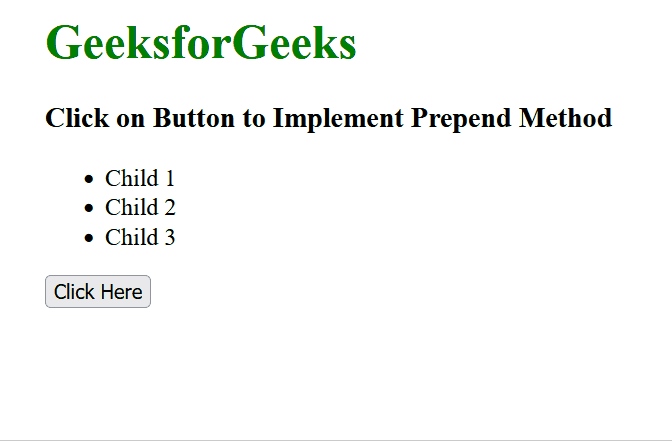
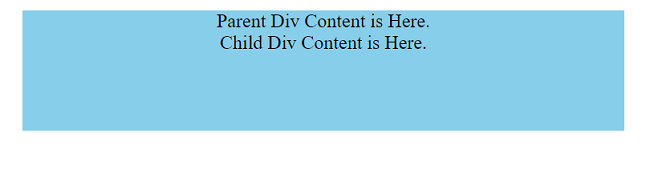

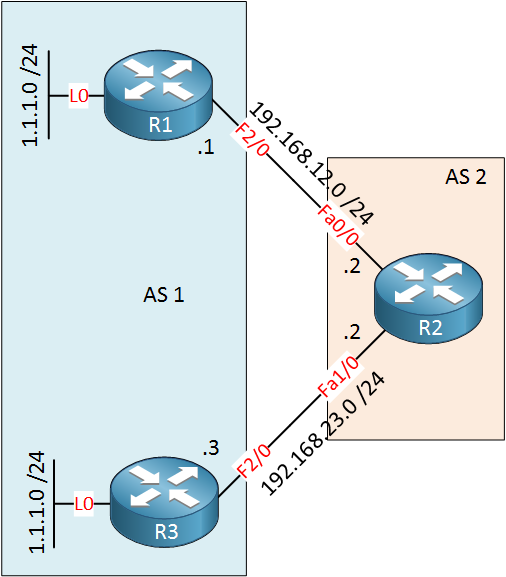

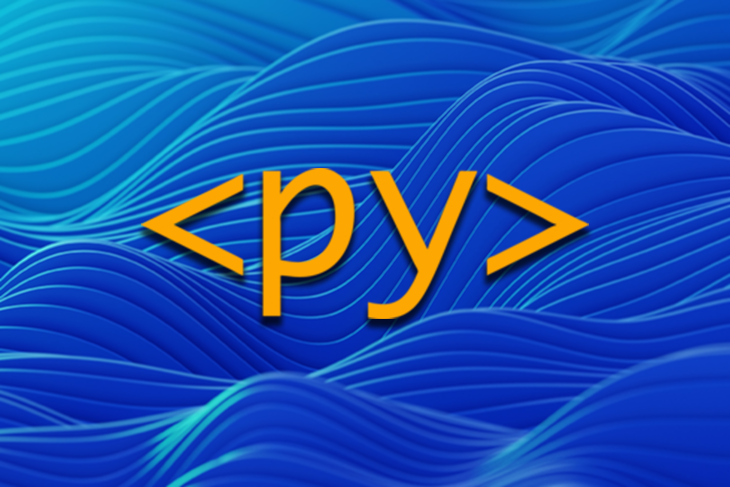
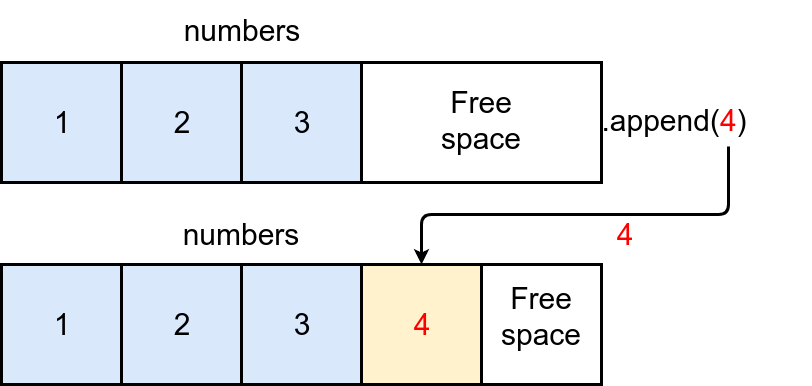
Article link: python prepend to list.
Learn more about the topic python prepend to list.
- Prepend a List in Python: 4 Best Methods (with code) – FavTutor
- How to Prepend to List in Python – AppDividend
- Python Prepend List – Linux Hint
- How do I prepend to a short python list? – Stack Overflow
- Prepend to a Python a List (Append at beginning) – Datagy
- How to prepend to a list in Python – Adam Smith
- Prepend to a List in Python | Delft Stack
- Python Prepend to List : 6 Different Easier Methods
- How do I prepend an item to a list in Python? – Gitnux Blog
- 5 Ways To Prepend Lists In Python
See more: nhanvietluanvan.com/luat-hoc