Python Parent Directory Import
When working on a Python project, it is common to have a directory structure with multiple subdirectories, each containing different modules or packages. In such cases, it may be necessary to import modules from the parent directory. However, Python’s import mechanism relies on the module search path, which does not include the parent directory by default. Therefore, you need to set up your project in a way that allows importing from the parent directory.
One way to achieve this is by adding the parent directory to the sys.path list at runtime. The sys module provides access to Python’s runtime environment, including variables specific to the Python interpreter. By manipulating the sys.path list, you can control the search path for module imports.
To add the parent directory to the sys.path list, you can use the sys.path.insert() method. This method takes two arguments: an index specifying the position where the directory will be inserted in the list, and the path to the directory itself. For example, if your current script is located in a subdirectory called “subdir” and you want to import a module from the parent directory, you can use the following code:
“`python
import sys
sys.path.insert(0, ‘..’)
“`
In this code, the “..” notation represents the parent directory. By inserting it at index 0, you ensure that it will be the first directory searched when importing modules. After adding the parent directory to sys.path, you can import modules from it as if they were located in the current directory or any other directory on the module search path.
Understanding Python Imports
Before diving into importing modules from the parent directory, it is important to have a clear understanding of how Python imports work in general. When you import a module in Python, the interpreter searches for it in a series of directories defined by the sys.path list. By default, the interpreter searches the current directory first, followed by the directories specified in the PYTHONPATH environment variable and the standard library directories.
When you use the import statement, Python looks for a module with the given name in each directory specified in sys.path. If it finds the module, it creates a corresponding module object and binds it to a name in the current namespace. The import statement also executes the code inside the module, which allows you to use the functions, classes, and variables defined in the module.
Absolute Imports
Python supports two types of imports: absolute and relative. Absolute imports refer to imports where the module is specified using its full path starting from the top-level package. For example, if you have a module called “module” located in a directory called “package,” you can import it using the following syntax:
“`python
import package.module
“`
This syntax is recommended for imports within a project because it provides a clear and unambiguous reference to the imported module. However, absolute imports can become cumbersome when you have a complex project structure with deeply nested subdirectories. In such cases, relative imports can offer a more concise and readable alternative.
Importing Modules from the Parent Directory
To import a module from the parent directory, you can use the absolute import syntax along with the sys.path manipulation described earlier. Assuming you have the parent directory added to sys.path, you can import a module using the following syntax:
“`python
import parent_module
“`
In this code, “parent_module” is the name of the module you want to import. As long as the module is located in the parent directory, Python will find and import it successfully. This approach is particularly useful when you have a module that is shared across multiple subdirectories or when you want to access modules located in a higher-level directory.
Using Relative Imports
Relative imports allow you to specify a module’s location relative to the current module or package. They can be a more concise alternative to absolute imports, especially when dealing with complex project structures. However, they are subject to certain limitations and require careful usage to avoid import errors.
A relative import is indicated by a leading dot (“.”) followed by the module or package name. The number of dots represents the number of parent directories to go up in the directory hierarchy. For example, if you want to import a module located in the parent directory, you can use the following syntax:
“`python
from .. import parent_module
“`
In this code, “..” represents the parent directory, and “parent_module” is the name of the module to import. The leading dots ensure that the import statement starts from the current module’s location and goes up one level in the directory hierarchy to find the parent directory.
Importing Subdirectories within the Parent Directory
In addition to importing modules from the parent directory, you may also need to import modules located within subdirectories of the parent directory. This can be achieved by combining the concepts of parent directory imports and relative imports.
Suppose you have the following directory structure:
“`
parent_directory/
subdirectory/
module.py
module.py
main_script.py
“`
To import the “module.py” file located in the “subdirectory” from the “main_script.py” file, you can use the following syntax:
“`python
from .subdirectory import module
“`
In this code, the leading dot (“.”) represents the current package or module, while “subdirectory” and “module” represent the names of the subdirectory and module, respectively. This statement tells Python to import the “module.py” file located in the “subdirectory” package within the parent directory.
Resolving Import Errors
When working with parent directory imports or relative imports, you may encounter import errors due to incorrect syntax or incompatible project structures. Here are some common import errors and their possible solutions:
1. “Attempted relative import beyond top-level package”: This error occurs when you attempt a relative import that goes beyond the top-level package. To resolve it, ensure that your directory structure and import statements are correctly organized.
2. “ImportError: No module named ‘module_name'”: This error indicates that Python could not find the specified module. Check the module’s location, spelling, and ensure that it is in a directory included in sys.path.
3. “ImportError: cannot import name ‘function_name'”: This error suggests that there is an issue with a specific function import. Check the function’s name and ensure that it is correctly defined in the module being imported.
Best Practices for Organizing and Importing Modules from the Parent Directory
When working with parent directory imports, it is important to follow some best practices to ensure a maintainable and scalable codebase. Here are a few recommendations:
1. Use absolute imports for clarity: Absolute imports provide a clear and unambiguous reference to the imported module. Although they can be verbose in complex project structures, they are generally recommended to improve code readability.
2. Limit usage of parent directory imports: While parent directory imports can be useful in certain scenarios, it is generally better to avoid excessive usage. Instead, organize your project structure in a way that promotes modularization and encapsulation.
3. Avoid circular imports: Circular imports occur when two or more modules depend on each other. They can lead to unexpected behavior and should be avoided. If you encounter a circular import issue, reconsider your module dependencies and adjust your directory structure accordingly.
4. Group related modules in subdirectories: To improve code organization, group related modules together in subdirectories within the parent directory. This helps maintain a clear and logical project structure.
FAQs
Q: How can I import a module from the parent directory in Python?
A: To import a module from the parent directory, you can add the parent directory to the sys.path list and use the import statement with the module’s name.
Q: How do I import a module from another directory in Python?
A: You can import a module from another directory by adding the directory to the sys.path list and using the import statement with the module’s name.
Q: How do I import from the parent directory in Python3?
A: In Python3, you can import from the parent directory by manipulating the sys.path list and using the import statement with the module’s name.
Q: What does “attempted relative import beyond top-level package” mean?
A: The “attempted relative import beyond top-level package” error means that you have tried to perform a relative import that goes beyond the top-level package in your directory structure.
Q: How do I import a module from a sibling directory in Python?
A: To import a module from a sibling directory, you can use relative imports by specifying the module’s location relative to the current module or package.
Q: How can I get the parent directory in Python?
A: You can get the parent directory in Python by using the “..” notation, which represents the parent directory in file paths.
Q: Can I open a file from the parent directory in Python?
A: Yes, you can open a file from the parent directory in Python by providing the correct file path relative to the current module’s location.
Q: How can I import a function from a parent folder in Python?
A: To import a function from a parent folder in Python, you can add the parent directory to sys.path and use the import statement with the function’s name.
In conclusion, importing modules from the parent directory in Python involves manipulating the sys.path list to include the parent directory and then using the import statement with the desired module’s name. Understanding the concepts of absolute and relative imports is crucial when working with complex project structures. By following best practices for organizing and importing modules, you can ensure a maintainable and scalable codebase.
Python – Importing Your Modules (Part 2: Import From A Different Folder ~7 Mins! No Ads)
Keywords searched by users: python parent directory import Python import module from parent directory, Python import module from another directory, Python3 import from parent directory, attempted relative import beyond top-level package, Python import from sibling directory, Get parent directory Python, Python open file from parent directory, Import function from parent folder python
Categories: Top 77 Python Parent Directory Import
See more here: nhanvietluanvan.com
Python Import Module From Parent Directory
Introduction
When working on a complex Python project, organizing your code into modules and packages is essential for maintainability and reusability. In some cases, you might encounter a scenario where you need to import a module from a parent directory. While Python offers simple ways to import modules from the same directory or subdirectories, importing a module from a parent directory can be a bit more challenging. This article will explore various techniques to import modules from a parent directory in Python, discussing their advantages, disadvantages, and potential pitfalls.
Importing Modules from the Same Directory
Before diving into importing modules from a parent directory, let’s briefly understand how modules are typically imported in Python. In the most common scenario, when a module resides in the same directory as the script or module trying to import it, you can simply use the `import` statement followed by the name of the module.
For instance, consider a file structure like this:
“`
├── main.py
└── utils.py
“`
To import the `utils` module into the `main.py` module, you would use:
“`python
import utils
“`
This approach works well when the module is present in the same directory or subdirectories. However, importing modules from parent directories requires additional techniques.
Importing Modules from a Parent Directory
There are multiple ways to import a module from a parent directory in Python. Let’s explore the most commonly used techniques.
1. Adding the Parent Directory to sys.path
The first approach involves modifying the `sys.path` list to include the parent directory. This method allows Python to locate the module when executing the script.
Consider the following file structure:
“`
├── directory
│ ├── main.py
│ └── utils.py
└── samples
└── sample.py
“`
To import the `utils` module into the `sample.py` module, you can modify `sys.path` inside the `sample.py` script:
“`python
import sys
sys.path.append(“../directory”)
import utils
“`
By appending the parent directory to `sys.path`, Python can locate and import the `utils` module successfully. However, this approach has some limitations. It modifies the global state, potentially affecting other parts of the codebase. Additionally, if the file structure changes, the relative path must be updated accordingly, introducing more maintenance overhead.
2. Using the runpy Module
The runpy module provides functions for running modules separately from the main program and can be handy for importing modules from a parent directory.
Continuing with the same file structure, to import the `utils` module into the `sample.py` module, you can utilize the `run_path()` function from the runpy module:
“`python
import runpy
utils = runpy.run_path(“../directory/utils.py”)
“`
The `run_path()` function executes the Python code specified in the given file path and returns the resulting namespace. This approach allows you to import modules dynamically by executing the module file directly from a parent directory. However, similar to the previous method, it may introduce maintenance issues if the file structure changes.
FAQs
Q1. Can I import modules from multiple parent directories?
Yes, you can import modules from multiple parent directories by including all the required paths in sys.path or executing the module files using runpy.run_path().
Q2. Is it possible to import modules from a grandparent directory?
Yes, it is possible to import modules from a grandparent directory or any higher-level parent directory by appending the appropriate relative path to sys.path or using runpy.run_path().
Q3. Can I import a specific function or class from a module in a parent directory?
Yes, after successfully importing the module using the techniques mentioned, you can access specific functions or classes using dot notation. For example, if `utils.py` contains a function named `helper_func`, you can access it using `utils.helper_func()`.
Q4. Are there any best practices for importing modules from parent directories?
While there are no strict guidelines, it is generally recommended to minimize the usage of parent directory imports to reduce complexity and maintain a clear project structure. If possible, organize your codebase such that modules that need to be shared across different directories are placed in separate packages.
Conclusion
Importing modules from a parent directory in Python can be challenging due to the language’s import mechanisms. However, by utilizing techniques like modifying sys.path or using the runpy module, you can successfully import modules from parent directories. It is important to keep in mind the potential issues and consider the maintenance overhead that may arise when the file structure changes. By following best practices and organizing your codebase effectively, you can ensure a manageable and maintainable project structure.
Python Import Module From Another Directory
When working on larger, more complex projects in Python, it is common to organize your code into multiple modules or packages. Sometimes, however, you may need to import a module from another directory. In this article, we will explore different techniques to achieve this, ensuring that your code remains structured and manageable.
The import statement in Python allows you to bring functionality from other modules into your current script. By default, Python searches for modules in three places: the current directory, the built-in module directory, and the list of directories specified in the PYTHONPATH environment variable. However, if the module you want to import is located in another directory, you need to take additional steps.
1. sys.path Modification:
The sys module provides access to various variables and functions that interact with the Python interpreter. One of them is sys.path, which is a list of directories Python searches for modules. You can add the desired directory to this list before importing the module, like this:
“`python
import sys
sys.path.append(‘/path/to/directory’)
import my_module
“`
By appending the directory path to sys.path, Python will treat it as if it were located in one of the default directories. While technically this solves the problem, modifying sys.path is not always the best approach. It can make your code less portable and harder to maintain, as it may rely on specific directory structures.
2. PYTHONPATH Environment Variable:
An alternative to modifying sys.path is to use the PYTHONPATH environment variable. This variable specifies additional directories Python should look in for modules. You can set it via command line or in your script, before importing the module:
“`python
import os
os.environ[‘PYTHONPATH’] = ‘/path/to/directory’
import my_module
“`
This approach ensures that the specified directory is always searched for modules without modifying the interpreter’s configuration. However, similar to modifying sys.path, it can also introduce dependency on specific environment configurations.
3. Explicitly Specifying Module Path:
If you want a more flexible and reliable solution, you can explicitly specify the module’s path relative to your current script. This approach is recommended when you know the exact location of the module and want to maintain code portability. Consider the following directory structure:
“`
my_project/
| main.py
|__ my_module/
| __init__.py
| module.py
“`
To import module.py from main.py, you need to navigate to the parent directory and use the dot notation:
“`python
import sys
sys.path.append(‘../my_module’)
from module import some_function
“`
Using this technique, Python will look for the specified module relative to the location of main.py. It allows you to organize your code neatly and makes it easier to understand the structure of your project.
FAQs:
Q: Why is it important to organize code into modules?
A: Organizing code into modules promotes code reusability, maintainability, and readability. It allows you to break down your project into logical units, encouraging better collaboration, debugging, and testing.
Q: Can I import modules from subdirectories within the current directory?
A: Yes, you can import modules located in subdirectories within the current directory by specifying the relative path to the module when using the import statement. For example, if the module is in a subdirectory called “utilities,” you can use `from utilities import my_module`.
Q: What if I need to import multiple modules from different directories?
A: If you need to import multiple modules from different directories, you can combine the above techniques as necessary. You can modify sys.path or set the PYTHONPATH environment variable for each specific directory before importing the required modules.
Q: How can I ensure code portability when importing modules from other directories?
A: To ensure code portability, it is recommended to use the explicit path approach by specifying the module’s path relative to your current script. Avoid modifying sys.path or relying on specific environment settings.
Q: Are there any potential issues with importing modules from another directory?
A: Importing modules from another directory can introduce complexity, especially when working with larger projects. It is essential to maintain a clear project structure and use appropriate techniques to import modules to avoid confusion and potential conflicts.
In conclusion, importing modules from another directory in Python is a common task faced by developers working on complex projects. By utilizing techniques such as modifying sys.path, setting the PYTHONPATH environment variable, or explicitly specifying module paths, you can successfully import modules while maintaining code structure and portability. Remember to choose the method that best suits your project’s requirements and follow best practices to ensure robust and maintainable code.
Python3 Import From Parent Directory
When working on a Python project, you might have a directory structure that includes subdirectories containing different modules or packages. In certain scenarios, you may need to import a module or package from the parent directory, which is outside the current working directory. Let’s dive into some practical methods to achieve this.
Method 1: Modifying sys.path
One way to import from the parent directory is by modifying the `sys.path` list. The `sys.path` list contains the directories Python searches for modules when importing. By adding the parent directory to this list, we can import modules from it. Here is an example:
“`python
import sys
sys.path.append(“..”) # Add the parent directory to sys.path
from parent_module import some_function # Importing from parent directory
“`
In this method, we added the parent directory (represented by “..”) to the `sys.path` list using the `append()` method. Now, we can import modules from the parent directory like any other module.
Method 2: Using the __init__.py file
Another way to import from the parent directory is by adding an empty `__init__.py` file in the parent directory. This file is used to mark a directory as a Python package. Once the `__init__.py` file is present, you can import modules or packages from the parent directory using relative imports. Here is an example:
“`
project_directory/
__init__.py
parent_module/
__init__.py
some_module.py
child_module/
__init__.py
another_module.py
“`
In this example, we added an empty `__init__.py` file in both the parent directory and the subdirectories to mark them as Python packages. Now, we can import modules from the parent directory using relative imports:
“`python
from ..parent_module import some_module # Importing from parent directory
from ..parent_module.some_module import some_function # Importing a specific function
“`
Method 3: Using the imp module (deprecated in Python 3.4+)
The `imp` module provides low-level import functionality in Python. Although it has been deprecated in Python 3.4 and later versions, it can still be used to import from the parent directory. Here is an example:
“`python
import imp
parent_module = imp.load_source(“parent_module”, “../parent_module.py”)
from parent_module import some_function # Importing from parent directory
“`
In this method, we use the `load_source()` function from the `imp` module to load the parent_module from the parent directory. Once loaded, we can import modules or functions from the parent module as usual.
Frequently Asked Questions (FAQs):
Q1. Can I import any module or package from the parent directory?
A1. Yes, you can import any module or package from the parent directory if it is properly structured and marked as a package (using the `__init__.py` file).
Q2. Are there any limitations or caveats when importing from the parent directory?
A2. While importing from the parent directory can be useful in certain scenarios, it is generally recommended to organize your project structure in a way that avoids excessive reliance on such imports. Relying too heavily on imports from the parent directory can make your code less maintainable and harder to understand for others.
Q3. How can I import from the parent directory in an IDE or code editor?
A3. The methods described above should work in most IDEs or code editors. However, some IDEs may provide additional features or configurations to simplify importing from the parent directory. Consult your IDE’s documentation or preferences to explore any available options.
Q4. What should I do if I encounter import errors while importing from the parent directory?
A4. Import errors could arise due to issues like incorrect directory paths or missing `__init__.py` files. Double-check that your directory structure is correct and mark the relevant directories as packages using the `__init__.py` file. If the issue persists, it could be related to your specific Python environment or project setup. Consider seeking help from the Python community or your peers.
In conclusion, importing modules or packages from the parent directory in Python 3 can be achieved using various methods. Understanding these methods gives you more flexibility and control over your project’s file structure. However, it is important to use such imports judiciously and maintain a well-organized project structure to ensure code readability and maintainability.
Images related to the topic python parent directory import
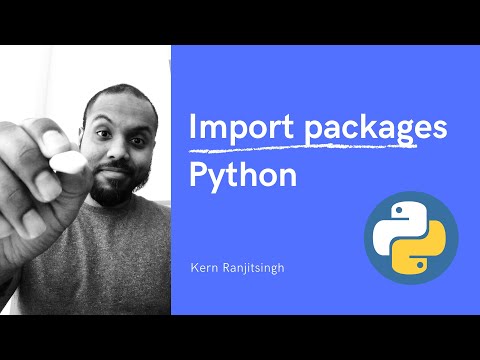
Found 14 images related to python parent directory import theme
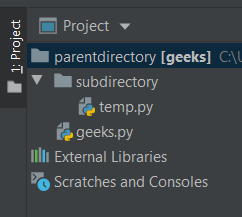
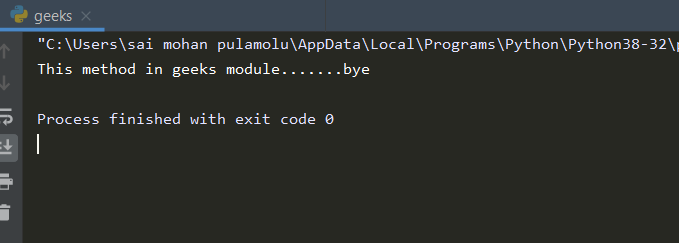
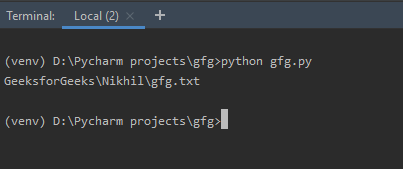
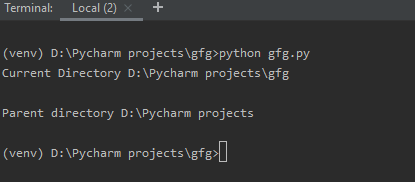
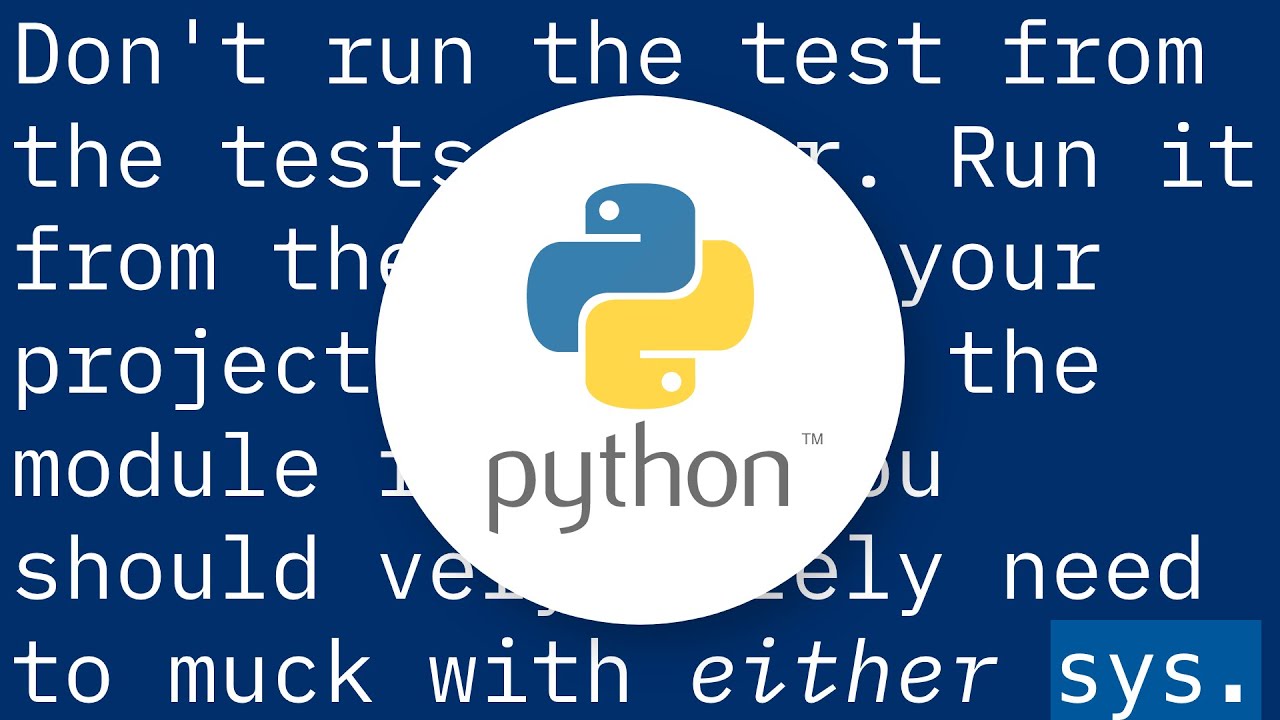
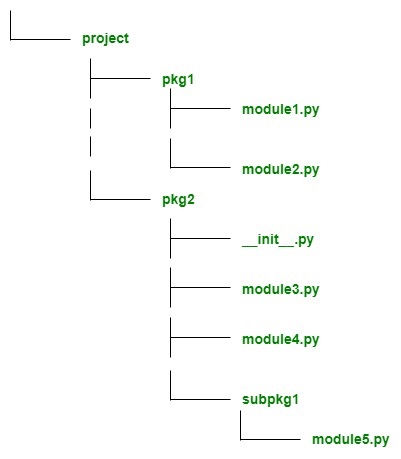
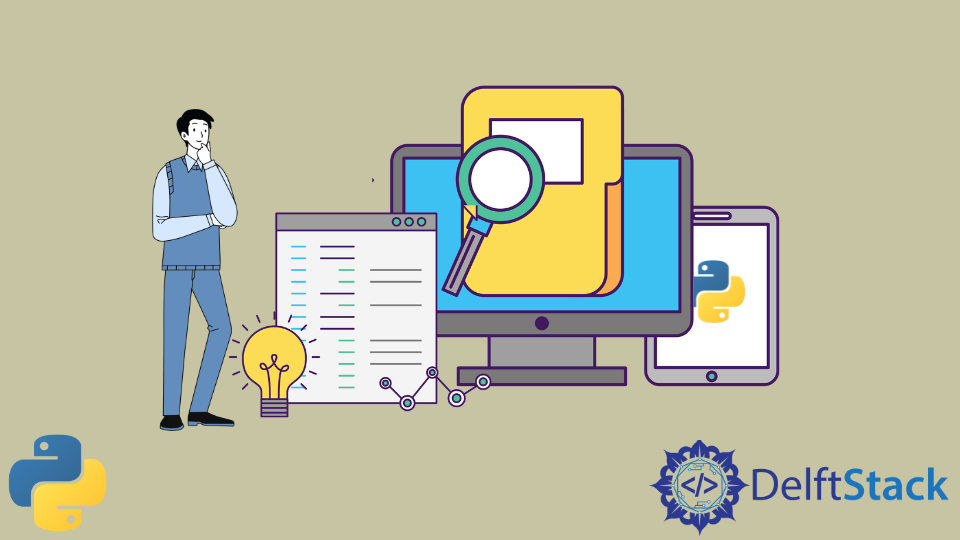



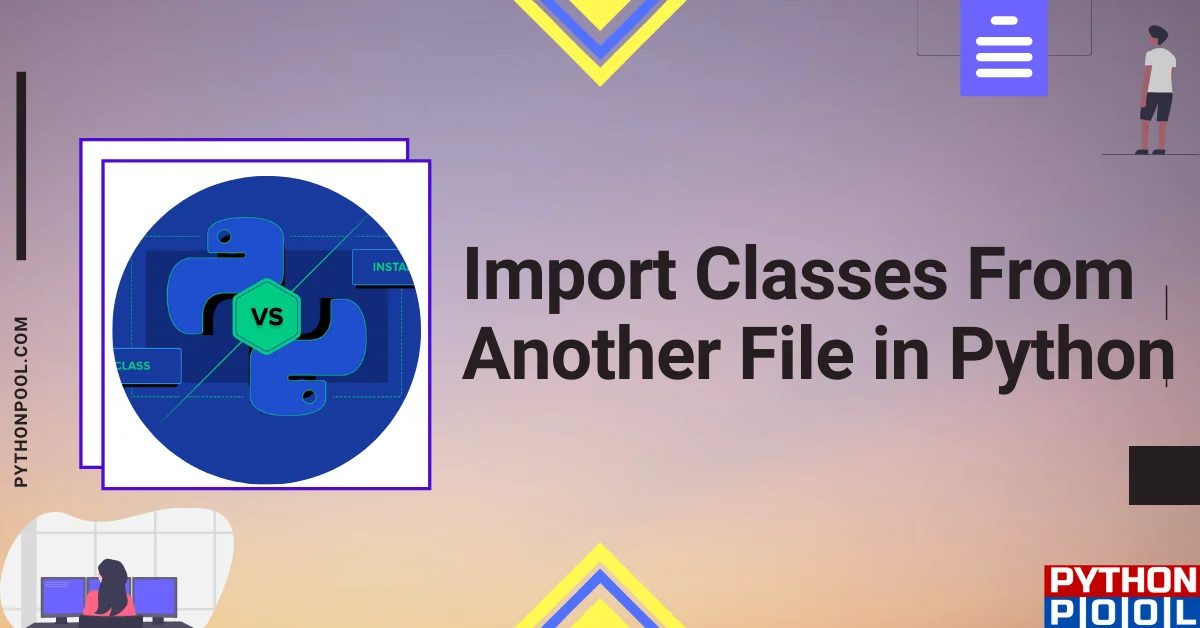
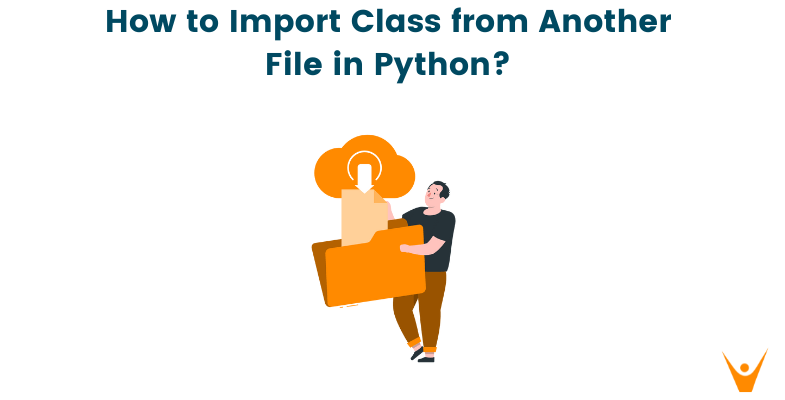
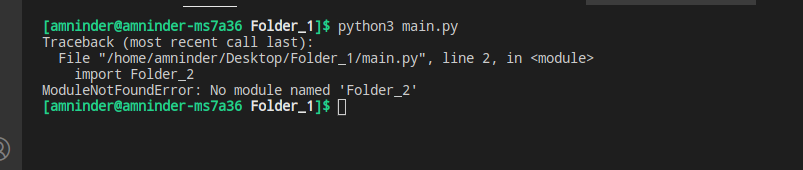
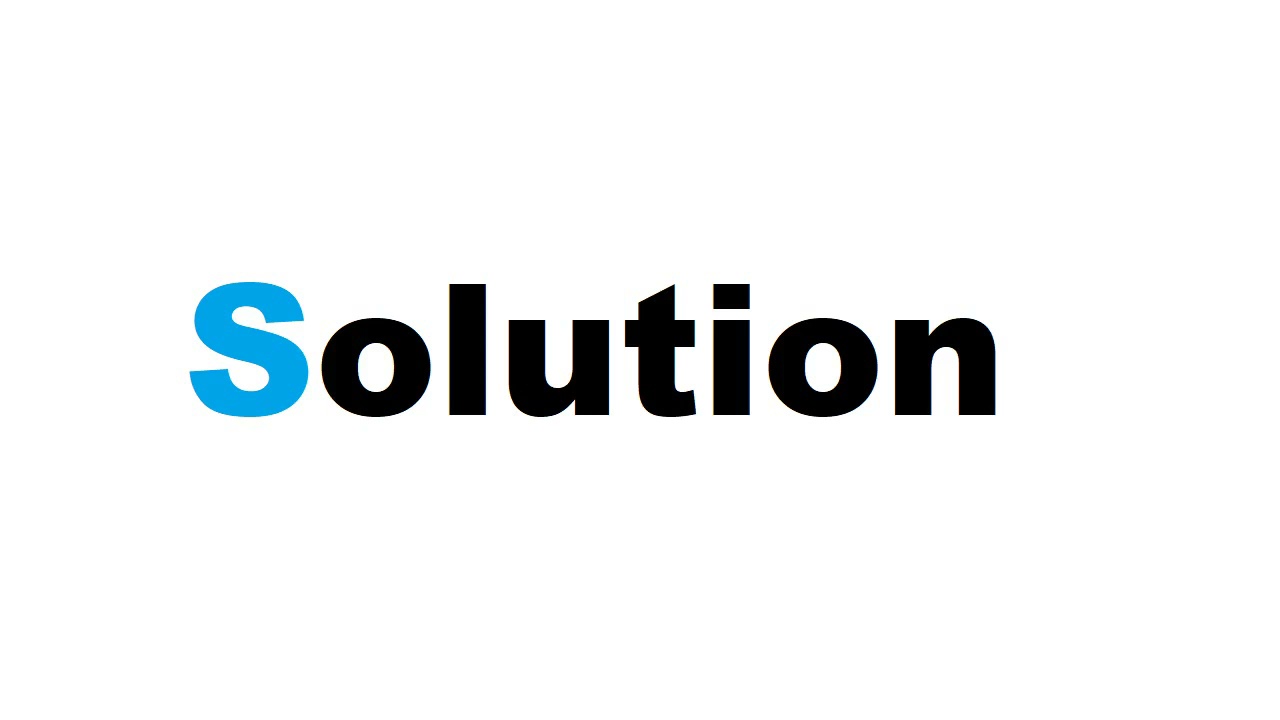

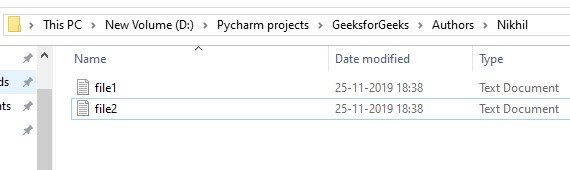
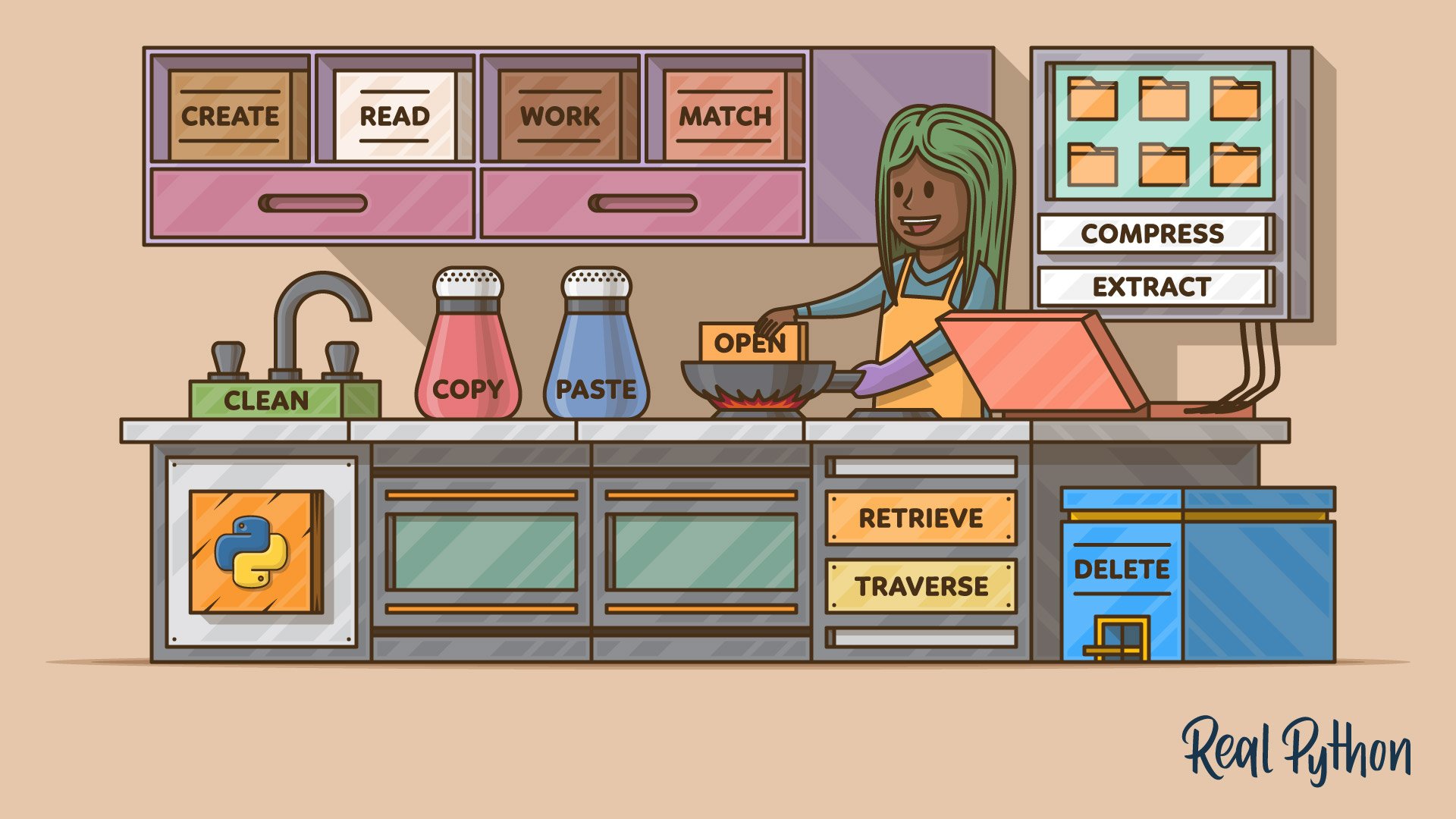

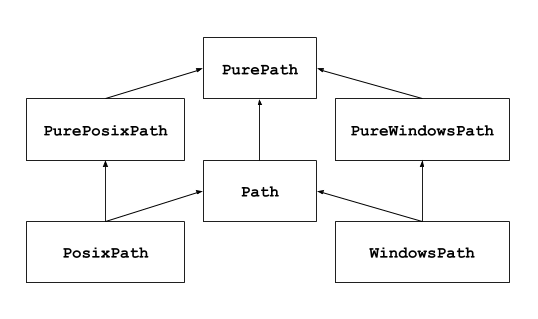
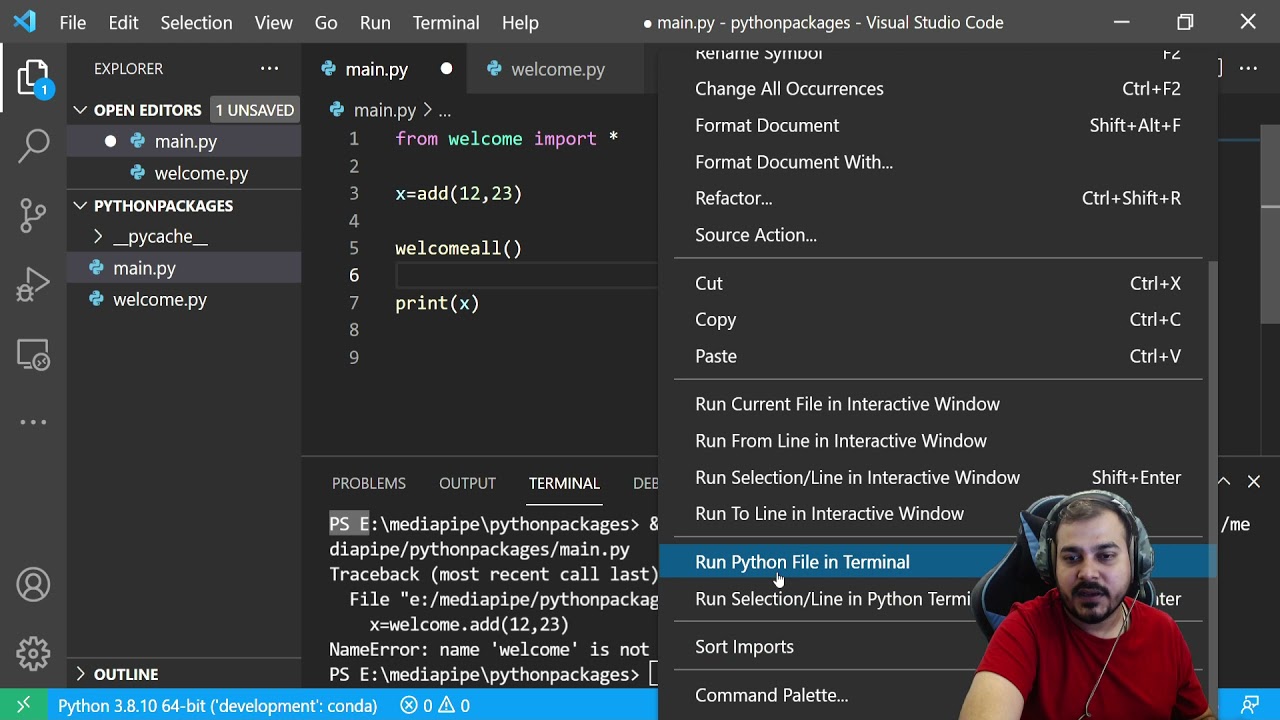
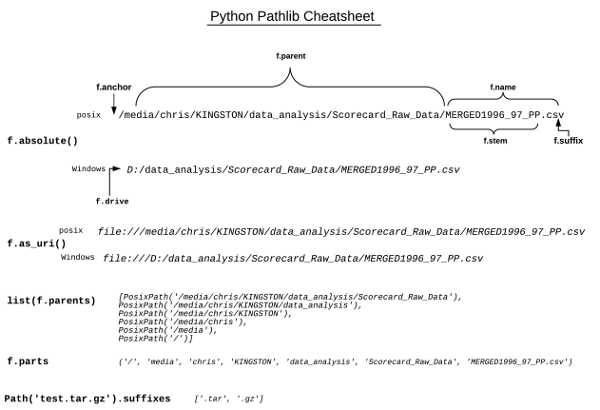
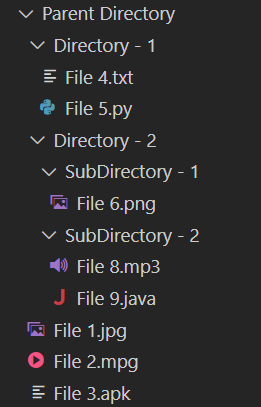



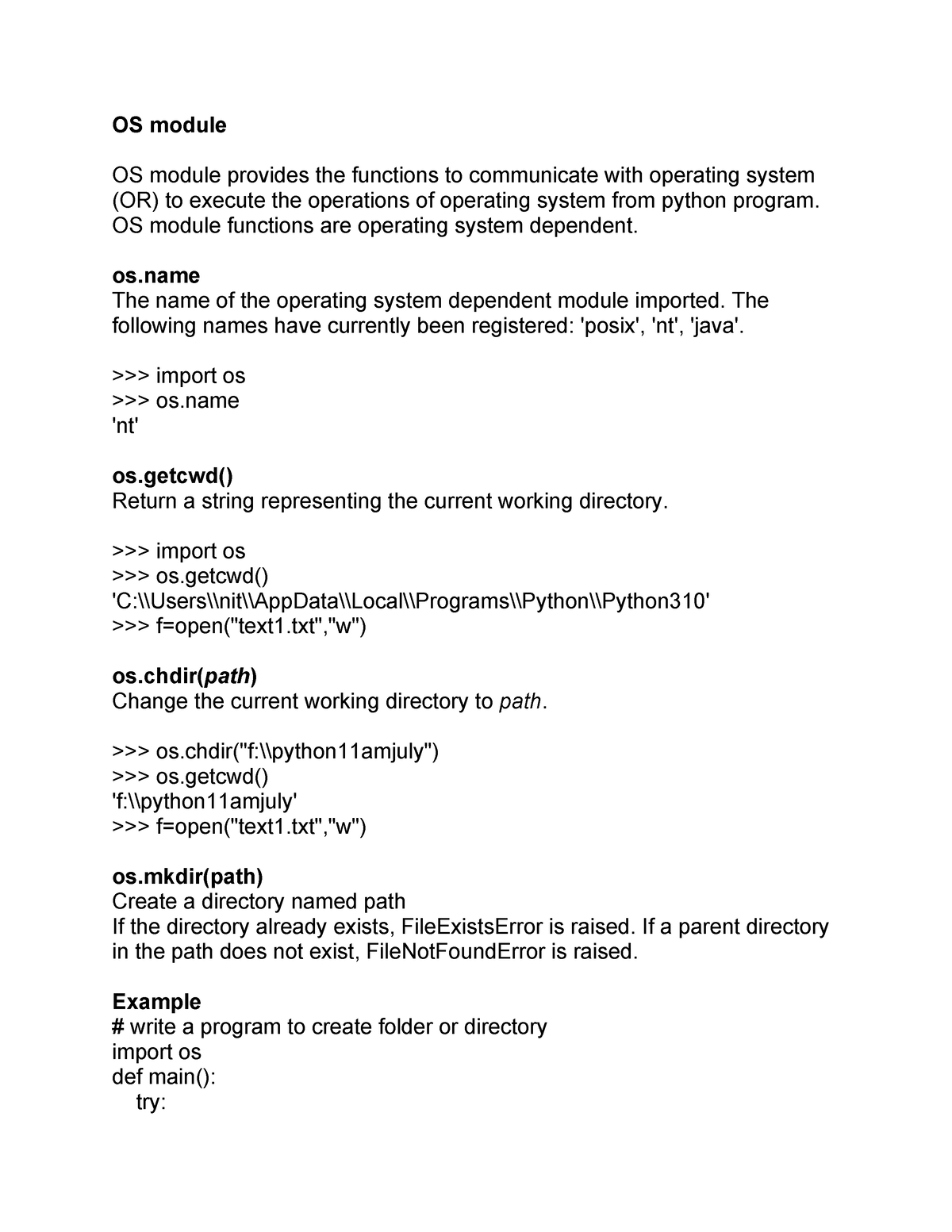
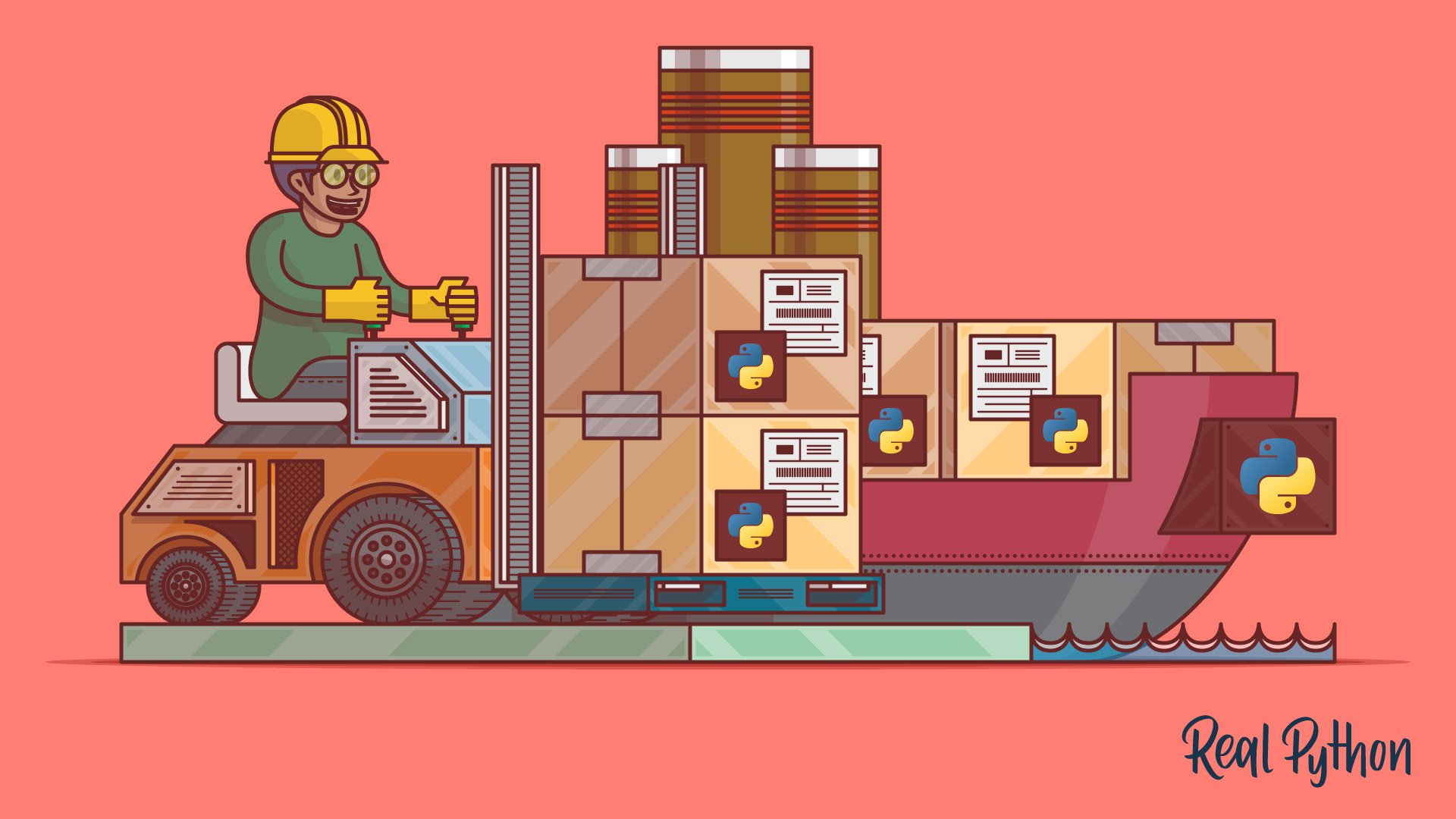


![FileNotFoundError: [Errno 2] No such file or directory: 'FSnm.png' - 🚀 Deployment - Streamlit Filenotfounderror: [Errno 2] No Such File Or Directory: 'Fsnm.Png' - 🚀 Deployment - Streamlit](https://global.discourse-cdn.com/business7/uploads/streamlit/optimized/2X/7/7a62f10889b442beaf7a1b533406adf4e2b4f636_2_1024x506.png)
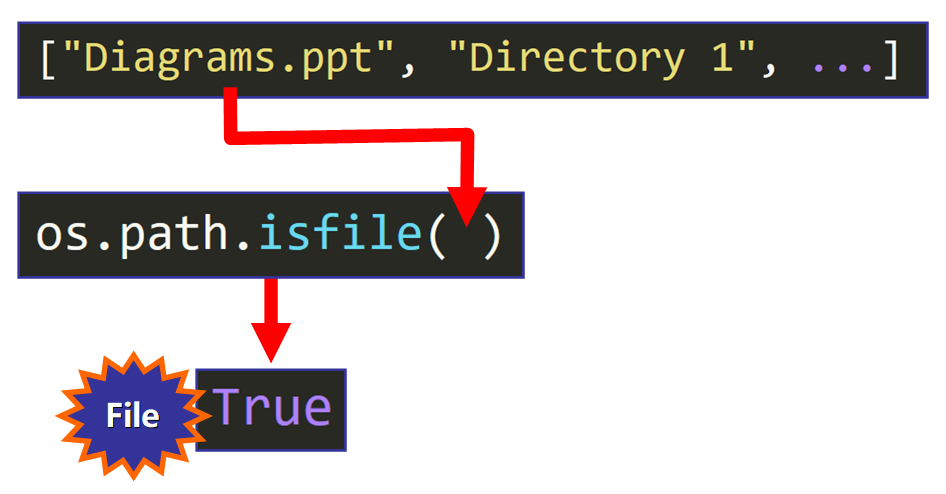


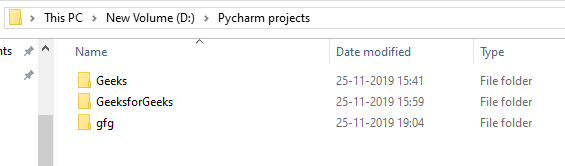

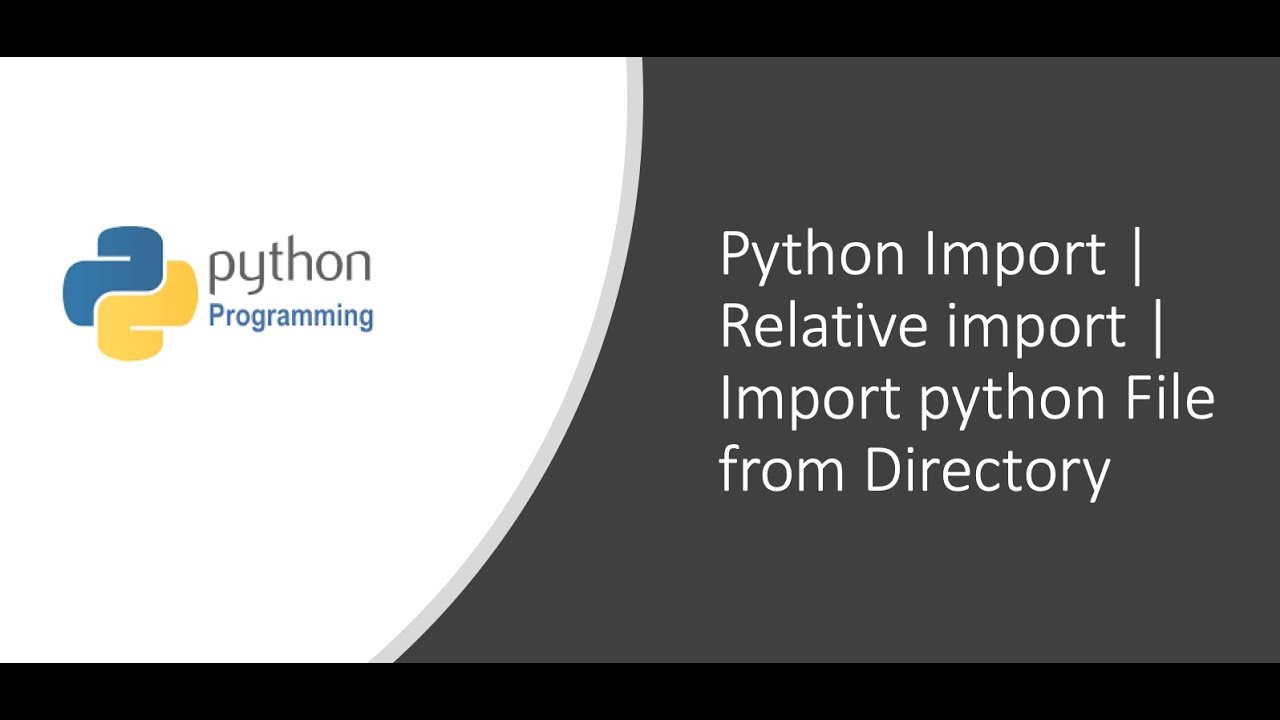
![FileNotFoundError: [Errno 2] No such file or directory: 'FSnm.png' - 🚀 Deployment - Streamlit Filenotfounderror: [Errno 2] No Such File Or Directory: 'Fsnm.Png' - 🚀 Deployment - Streamlit](https://global.discourse-cdn.com/business7/uploads/streamlit/original/2X/7/7a62f10889b442beaf7a1b533406adf4e2b4f636.png)
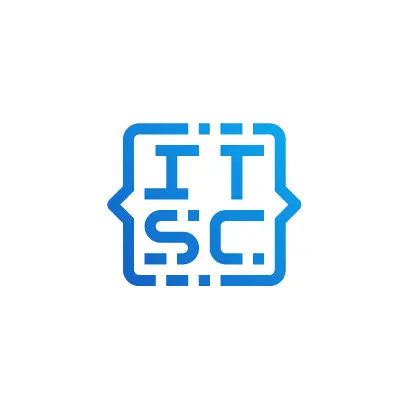
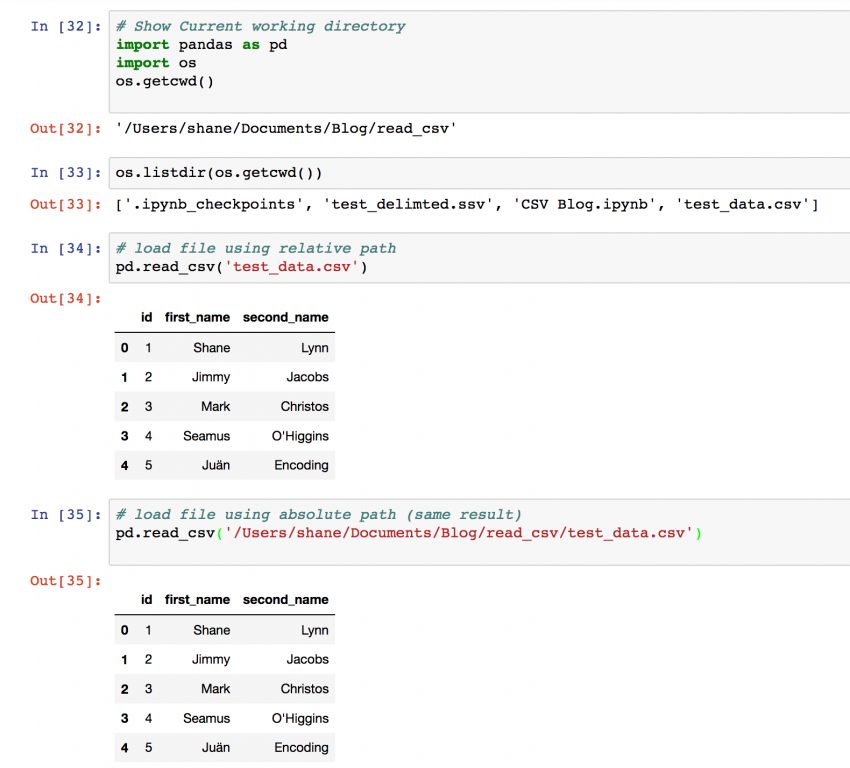
Article link: python parent directory import.
Learn more about the topic python parent directory import.
- Python – Import from parent directory – GeeksforGeeks
- Importing modules from parent folder – python – Stack Overflow
- How to Import File from Parent Directory in Python? (with code)
- How can I import a module from a parent directory in Python?
- Python Import From a Parent Directory: A Quick Guide
- Importing modules from parent folder – W3docs
- How To Import Module From Parent Directory In Python In 2022?
- Python Import Module from Parent Directory in 3 Easy Steps
- Python Import from Parent Directory in Simple Way
- Adding Parent Directory To Python Path – Krystian Safjan’s Blog