Python If Variable Exists
Checking Variable Presence using `try-except` Block
One way to check if a variable exists in Python is by using a `try-except` block. This method involves trying to access the variable and handling the `NameError` exception if the variable is undefined. Here’s an example:
“`python
try:
variable
except NameError:
print(“Variable does not exist.”)
“`
Using `try-except` to Handle an Undefined Variable
By using a specific `try-except` block, you can handle only the `NameError` exception that occurs when an undefined variable is accessed. This approach allows you to execute specific code when the variable does not exist. Here’s an example:
“`python
try:
variable
except NameError:
print(“Variable does not exist.”)
else:
print(“Variable exists.”)
“`
Using `locals()` Function to Verify Variable Existence
The `locals()` function returns a dictionary containing the current local symbol table. By checking if the variable exists in the dictionary returned by `locals()`, you can verify its existence. Here’s an example:
“`python
if ‘variable’ in locals():
print(“Variable exists.”)
else:
print(“Variable does not exist.”)
“`
Using `globals()` Function to Check if a Variable is Defined
Similar to the `locals()` function, the `globals()` function returns a dictionary containing the current global symbol table. You can use it to check if a variable is defined by checking its presence in the dictionary returned by `globals()`. Here’s an example:
“`python
if ‘variable’ in globals():
print(“Variable exists.”)
else:
print(“Variable does not exist.”)
“`
Using the `dir()` Function to Validate the Existence of a Variable
The `dir()` function returns a list of names in the current local or global scope. By checking if the variable is present in the list returned by `dir()`, you can validate its existence. Here’s an example:
“`python
if ‘variable’ in dir():
print(“Variable exists.”)
else:
print(“Variable does not exist.”)
“`
Evaluating Variable Presence with the `hasattr()` Function
The `hasattr()` function takes an object and a string as arguments and returns `True` if the object has an attribute with the given name. You can use this function to check if a variable exists by passing the object in which the variable should be present. Here’s an example:
“`python
if hasattr(obj, ‘variable’):
print(“Variable exists.”)
else:
print(“Variable does not exist.”)
“`
Using `get()` Method to Check Variable Existence in Dictionaries
In Python, dictionaries have a `get()` method that allows you to retrieve the value of a particular key. By using the `get()` method, you can check if a variable exists in a dictionary. If the key is present, it returns the corresponding value; otherwise, it returns a default value, which can be set to `None` if you only want to check the existence of the key. Here’s an example:
“`python
my_dict = {“variable”: 42}
if my_dict.get(“variable”) is not None:
print(“Variable exists.”)
else:
print(“Variable does not exist.”)
“`
Verifying Variable Existence in Lists using the `in` Operator
Lists in Python support the `in` operator, which allows you to check if an element is present in the list. By using this operator, you can verify the existence of a variable in a list. Here’s an example:
“`python
my_list = [“variable”, 42]
if “variable” in my_list:
print(“Variable exists.”)
else:
print(“Variable does not exist.”)
“`
Differentiating between an Undefined and None Variable
It is important to note that an undefined variable is different from a variable whose value is `None`. An undefined variable has not been assigned any value, while a variable with a `None` value has been explicitly assigned that value. You can use the `is` operator to differentiate between the two. Here’s an example:
“`python
if ‘variable’ in locals() and locals()[‘variable’] is not None:
print(“Variable exists and is not None.”)
else:
print(“Variable does not exist or is None.”)
“`
FAQs:
Q: How can I check if an environment variable exists in Python?
A: You can use the `os.environ` dictionary to access environment variables. To check if a specific environment variable exists, you can use the `get()` method. Here’s an example:
“`python
import os
if os.environ.get(“ENV_VARIABLE”):
print(“Environment variable exists.”)
else:
print(“Environment variable does not exist.”)
“`
Q: How can I check if a variable is empty in Python?
A: To check if a variable is empty, you can use the `not` operator along with the variable. Here’s an example:
“`python
variable = “”
if not variable:
print(“Variable is empty.”)
else:
print(“Variable is not empty.”)
“`
Q: How can I check if a variable does not exist in Python?
A: You can use the `try-except` block or any other method discussed above to check if a variable does not exist. If an exception is raised or the variable is not found, it means the variable does not exist.
Q: How can I check if a variable is unbound in Python?
A: In Python, a variable is unbound if it has been defined but not assigned a value. To check if a variable is unbound, you can use the `locals()` or `globals()` function and verify if the variable is present but has a value of `None`.
Q: How can I check if a key exists in an array in Python?
A: Python does not have a native array data structure. If you meant checking if a key exists in a list, you can use the `in` operator as shown in the examples above. However, if you meant checking if a key exists in a dictionary, you can use the `in` operator with the dictionary or the `get()` method.
Q: How can I write an if-else statement in one line in Python?
A: Python allows you to write an if-else statement in one line using the ternary operator. Here’s an example:
“`python
variable = 42
print(“Variable exists.”) if ‘variable’ in locals() else print(“Variable does not exist.”)
“`
Q: Is there an equivalent of isset() in Python to check if a variable exists?
A: The `isset()` function in PHP determines if a variable is declared and is different from `None`. In Python, you can use the methods discussed above to check if a variable exists and differentiate between an undefined variable and a variable with a `None` value.
How To Check If A Variable Exists In Python
Keywords searched by users: python if variable exists Python check environment variable exists, If variable is empty Python, If not exist python, Python check if variable unbound, Exist python variable, Python check key in array, Python if-else one line, Isset python
Categories: Top 33 Python If Variable Exists
See more here: nhanvietluanvan.com
Python Check Environment Variable Exists
Python is a versatile programming language that allows developers to create applications and scripts for various purposes. One important aspect of developing in Python is being able to interact with the environment in which the code is running. This includes checking whether certain environment variables exist. In this article, we will explore how to check if an environment variable exists in Python, covering various methods and scenarios.
Python provides a straightforward way to access environment variables through the `os` module. The `os.environ` dictionary holds all the environment variables as key-value pairs, where the keys represent the names of the variables. To check if a specific environment variable exists, we can simply use the `in` operator to check if the variable name is present in the dictionary:
“`python
import os
if ‘MY_VARIABLE’ in os.environ:
print(‘MY_VARIABLE exists!’)
else:
print(‘MY_VARIABLE does not exist.’)
“`
This basic approach works well, but there are a few things to consider. First, keep in mind that environment variable names are case-sensitive. Therefore, `’my_variable’` and `’MY_VARIABLE’` are considered as two separate variables. Make sure to use the correct case when checking for the existence of an environment variable.
Furthermore, it is always a good idea to handle cases where the environment variable does not exist. In the example above, we simply printed a message stating whether the variable exists or not. However, you can customize this behavior based on your requirements. For instance, you may choose to display an error message, log the event, or even terminate the script if a specific variable is not present.
Another approach to check if an environment variable exists is by using the `get()` method provided by the `os.environ` dictionary. This method returns the value of an environment variable if it exists, or a specified default value if it does not. By setting a default value, you can avoid potential errors when attempting to access a non-existent environment variable directly. Here’s an example:
“`python
import os
my_variable = os.environ.get(‘MY_VARIABLE’, ‘default_value’)
if my_variable != ‘default_value’:
print(‘MY_VARIABLE exists with the value:’, my_variable)
else:
print(‘MY_VARIABLE does not exist or has the default value.’)
“`
In this case, if the environment variable `’MY_VARIABLE’` exists, its value will be assigned to `my_variable`. Otherwise, `my_variable` will be set to the specified default value, `’default_value’`. You can adjust the default value as per your needs.
Now, let’s cover some frequently asked questions related to checking environment variable existence in Python:
Q: Can I modify or create new environment variables using Python?
A: Yes, you can modify the value of existing environment variables or create new ones using the `os.environ` dictionary. For example, `os.environ[‘MY_VARIABLE’] = ‘new_value’` would change the value of `’MY_VARIABLE’` to `’new_value’`. However, note that these changes are only valid for the current Python process.
Q: How can I check if multiple environment variables exist at once?
A: To check multiple environment variables simultaneously, you can utilize a loop or list comprehension. Iterate over the desired variable names and check each one individually using the `in` operator or `get()` method. This approach allows you to handle multiple variables efficiently in a single script.
Q: Are environment variables limited to strings only?
A: Environment variable values are typically represented as strings. However, you can convert them to other data types as needed. For example, if the value represents an integer, you can use `int(os.environ[‘MY_VARIABLE’])` to convert it to an integer.
Q: Can I remove an environment variable using Python?
A: Yes, you can remove an environment variable by using the `del` keyword together with `os.environ` and the variable name. For example, `del os.environ[‘MY_VARIABLE’]` would remove the environment variable named `’MY_VARIABLE’`.
In conclusion, checking if an environment variable exists in Python is a fundamental task when developing scripts and applications. Using the `os.environ` dictionary, we can easily verify the existence of a variable and perform actions accordingly. Whether you prefer the `in` operator or the `get()` method, Python provides multiple options to handle this requirement. Remember to consider case sensitivity, provide default values when necessary, and customize your error handling to accommodate different scenarios.
If Variable Is Empty Python
In the realm of programming, it is common to come across situations where we need to check if a variable is empty before performing certain operations. This is especially crucial when dealing with user inputs or data from external sources. In Python, there are multiple methods to determine if a variable is empty in order to avoid potential errors or bugs. In this article, we will explore these methods in-depth and address frequently asked questions related to this topic.
Checking for an Empty Variable:
Before we delve into the various methods, it is essential to understand what we mean by an “empty” variable in Python. An empty variable is one that has not been assigned a value or contains the default value of None. It is crucial to differentiate between an empty variable and a variable that contains an empty value, such as an empty string, list, or dictionary.
Method 1: Using the “if” statement with a length check:
One straightforward way to check if a variable is empty is by using the “if” statement in combination with a length check. For example, if we have a variable named “my_variable” and want to check if it is empty, we can use the following code snippet:
if len(my_variable) == 0:
print(“Variable is empty”)
This method works well for variables that can have a length, such as strings, lists, tuples, and dictionaries. However, it is important to note that it can raise a TypeError if applied to an inappropriate type, like an integer or a boolean.
Method 2: Using the “not” operator:
Another approach is to use the “not” operator to check if a variable is empty. By using this method, we can directly check if the variable evaluates to False or None. Let’s consider an example where we have a variable called “my_variable” that we want to check:
if not my_variable:
print(“Variable is empty”)
This method is more concise and works well for variables that do not have a length, such as integers, booleans, or NoneType objects. It is often considered a more Pythonic way of checking for an empty variable.
Method 3: Using the “is” operator with None:
If we specifically want to check if a variable is equal to None, we can use the “is” operator. This operator checks if two variables refer to the same object. Here is an example of how we can use it:
if my_variable is None:
print(“Variable is empty”)
Using the “is” operator with None is particularly handy when we want to ensure that a variable is not just an empty string or list but explicitly None.
Frequently Asked Questions (FAQs):
Q1. What happens if we check if an uninitialized variable is empty?
If we attempt to check the emptiness of an uninitialized variable, Python will throw an error indicating that the variable is not defined. It is good practice to initialize all variables properly before using them to avoid such errors.
Q2. Can we use the “if” statement with length checks for variables that do not support the len() function?
No, the “if” statement with length checks is only applicable to objects that support the len() function. Using it with types that do not support len() will raise a TypeError.
Q3. Is it possible for a variable to be empty but not equal to None?
Yes, in Python, a variable can be empty but not explicitly equal to None. For instance, an empty string or an empty list is considered empty but different from None.
Q4. What should I do if my variable can be either empty or None?
If your variable can be either empty or None, it is recommended to check for None first using the “is” operator, and then check for emptiness using the appropriate method based on the variable type. This ensures accurate handling of diverse scenarios.
Q5. Can we combine multiple methods to check if a variable is empty?
Yes, it is possible to combine different methods depending on the complexity of the scenario. However, it is important to ensure that the chosen methods are compatible with the variable’s data type.
In conclusion, knowing how to determine if a variable is empty in Python is crucial to avoid errors and ensure smooth execution of programs. By utilizing the discussed methods and understanding their specific use cases, developers can handle empty variables effectively and write more robust code.
Images related to the topic python if variable exists
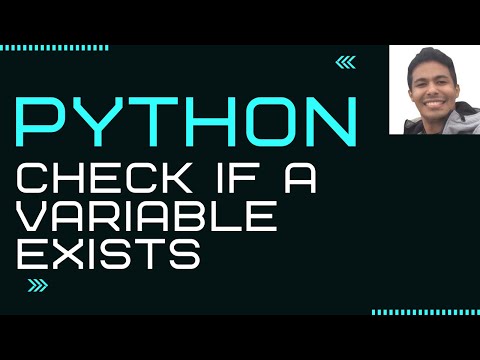
Found 9 images related to python if variable exists theme


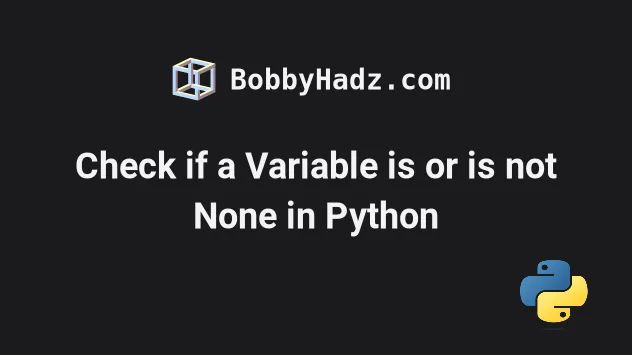
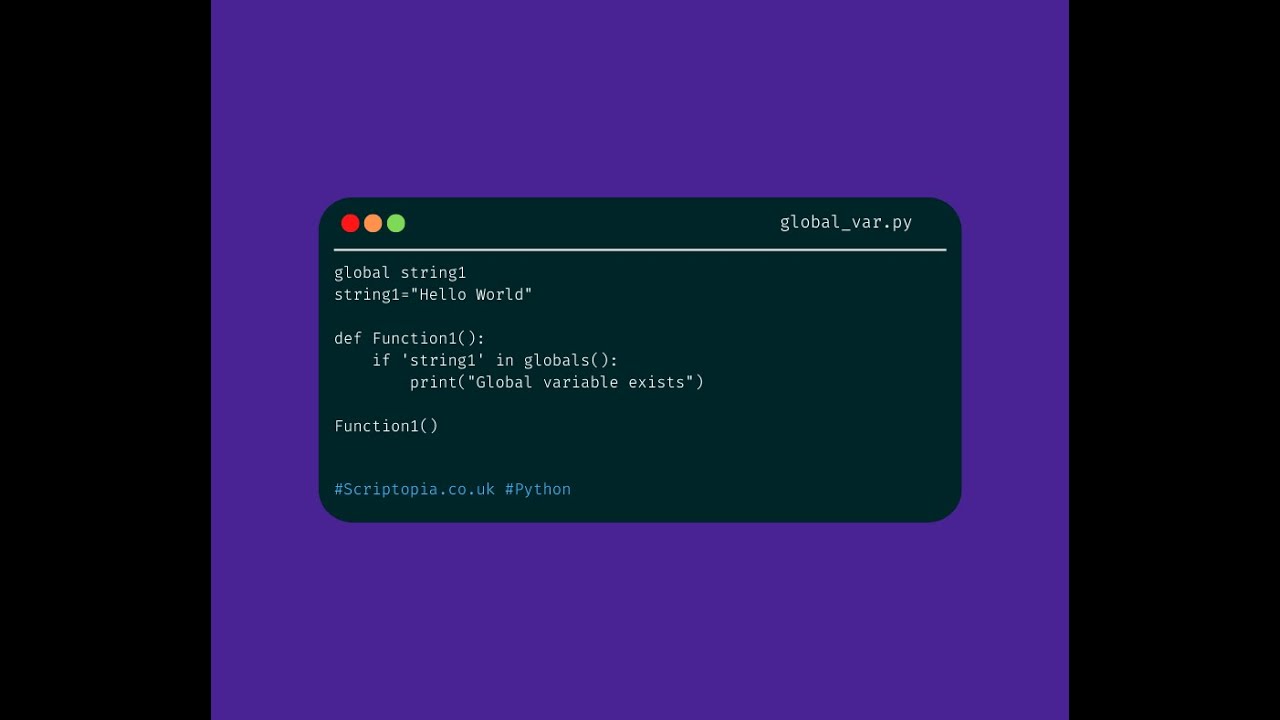

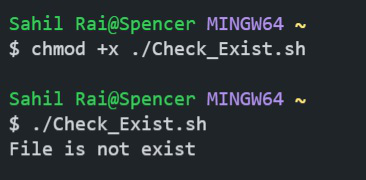




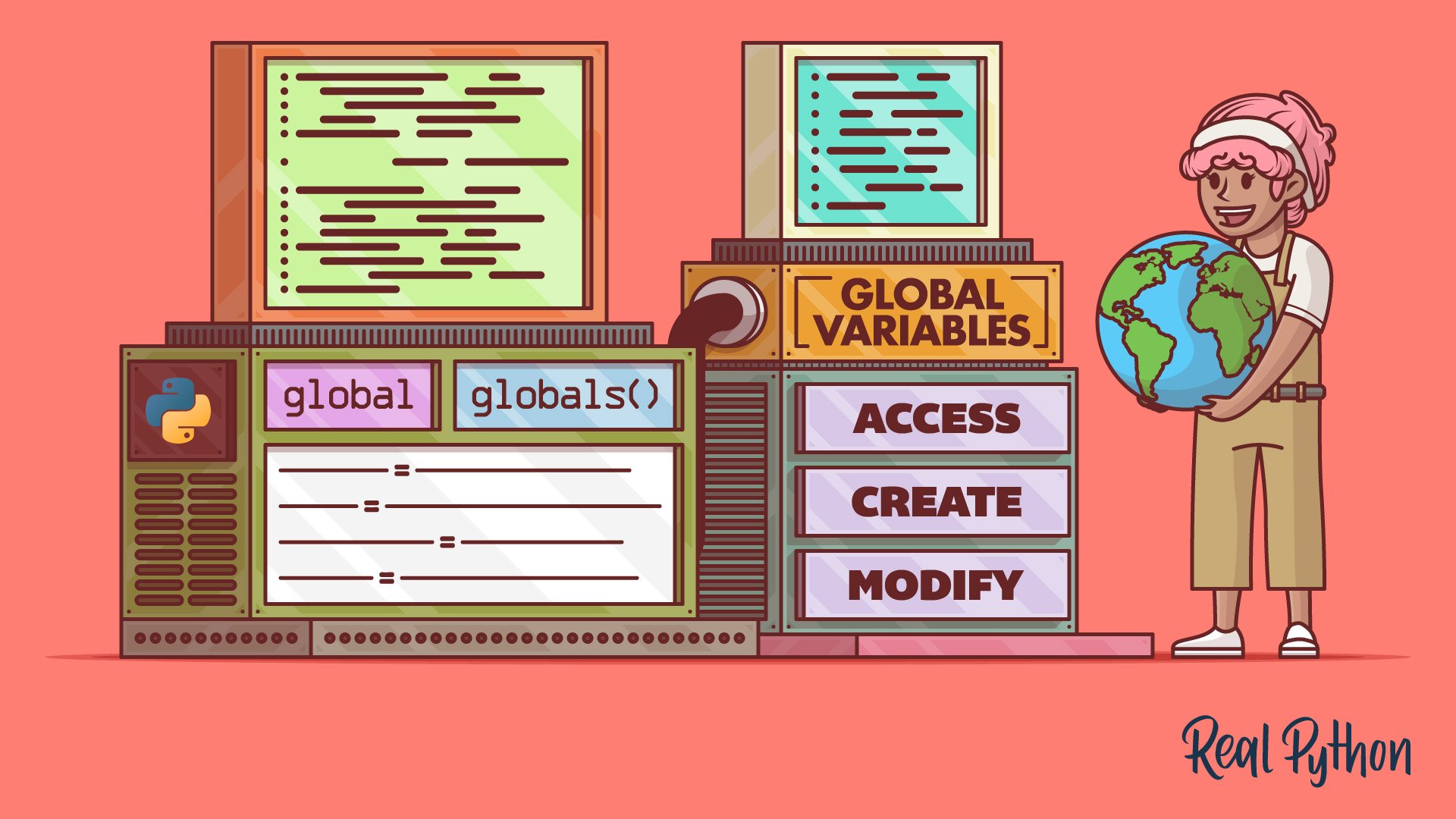


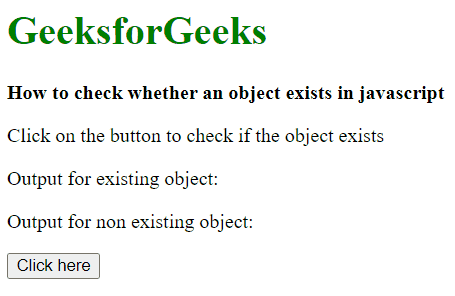
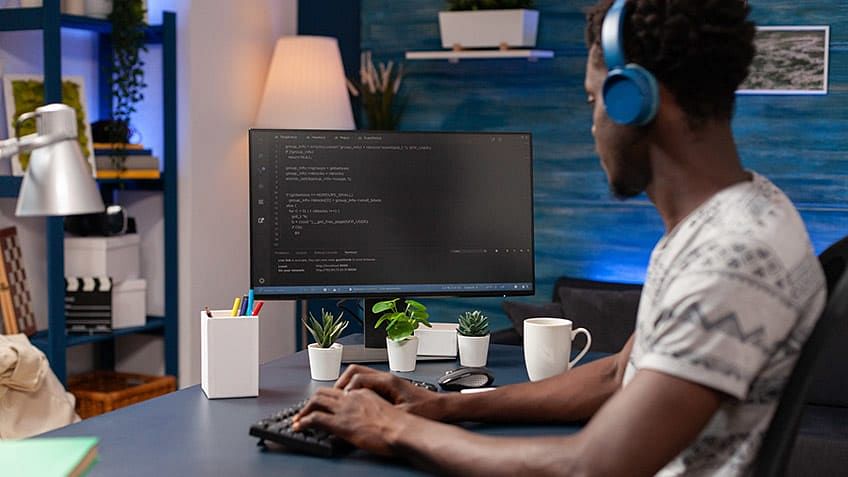
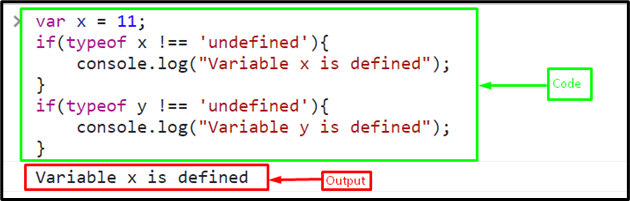
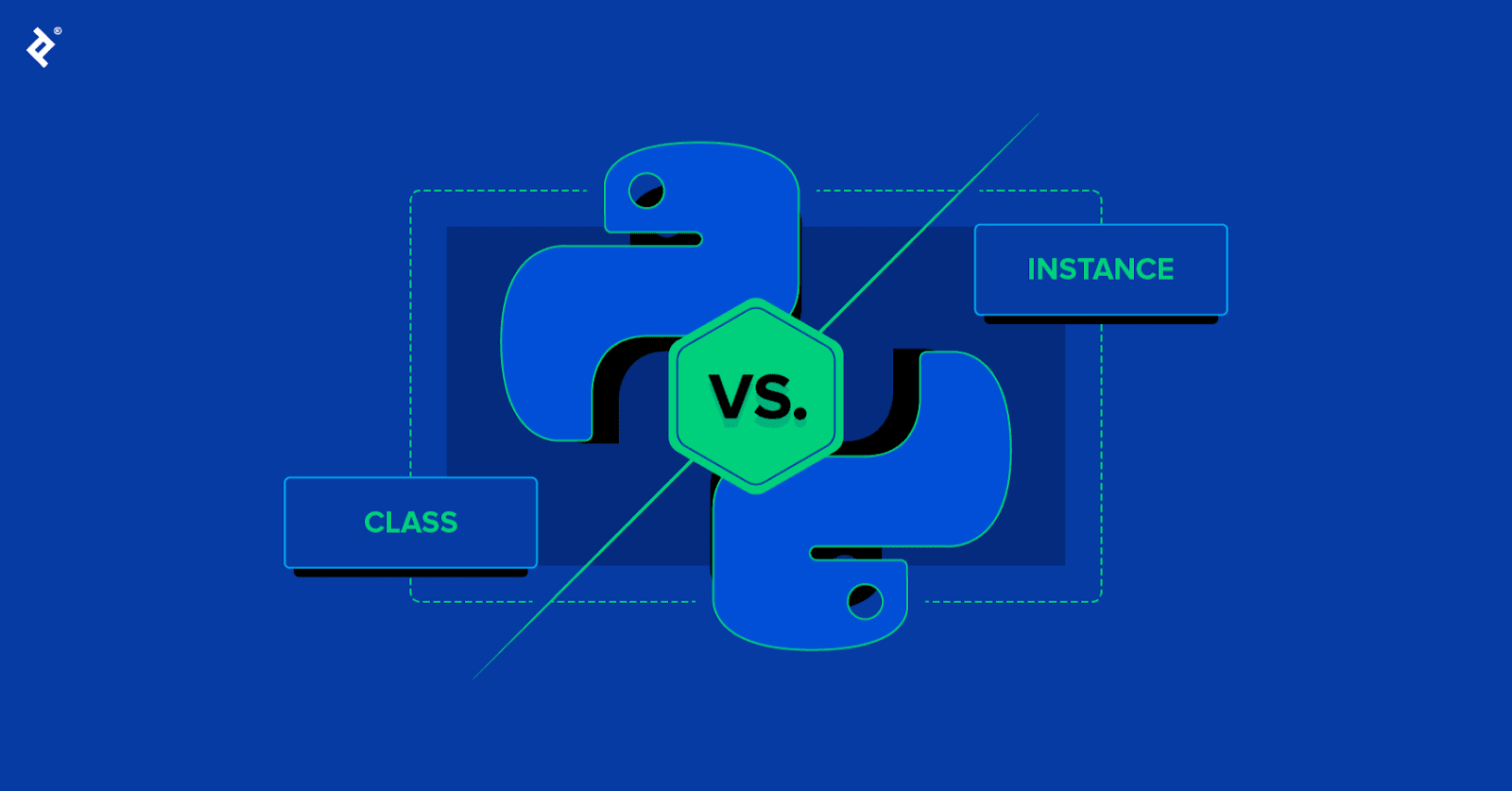
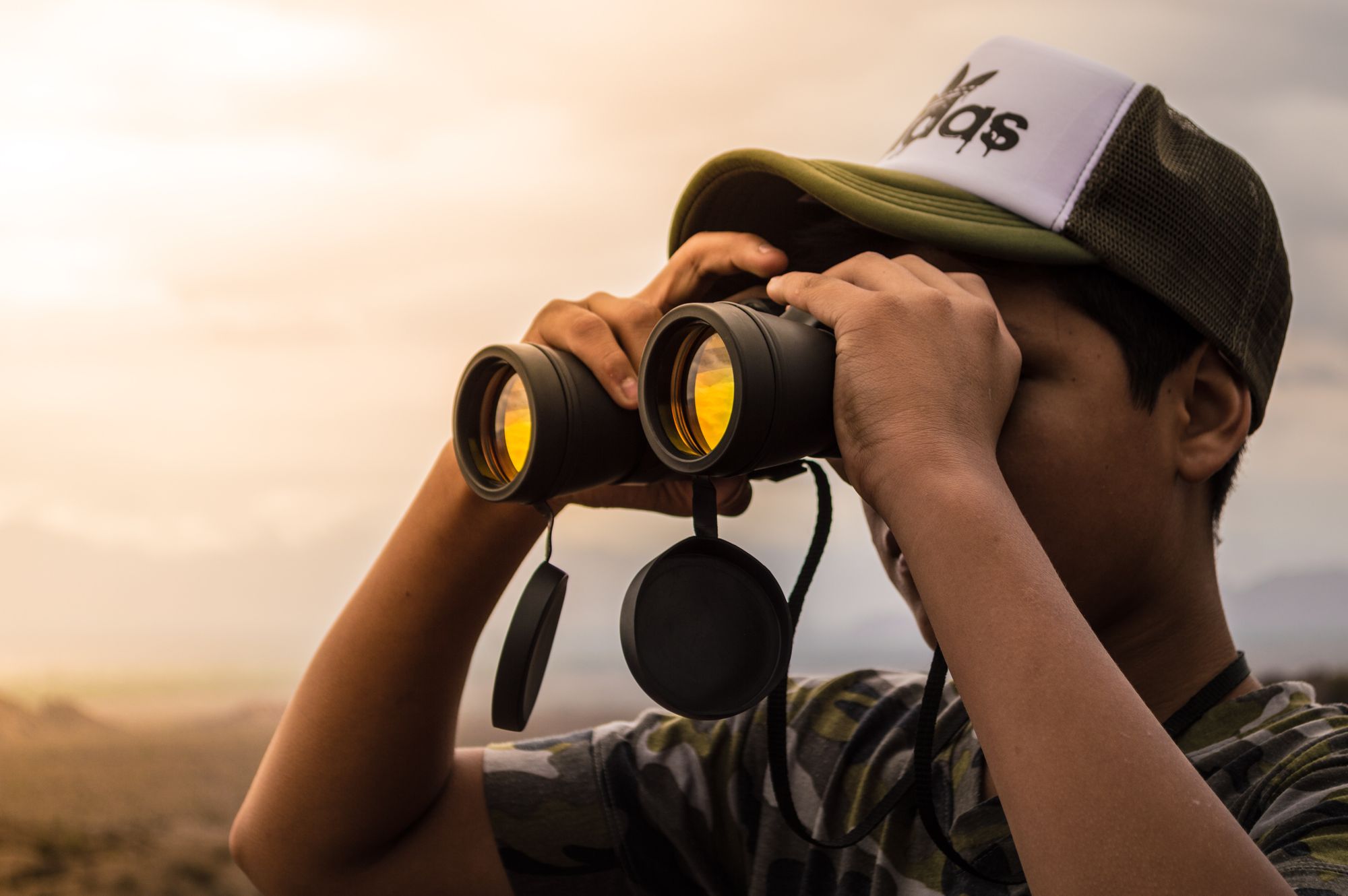
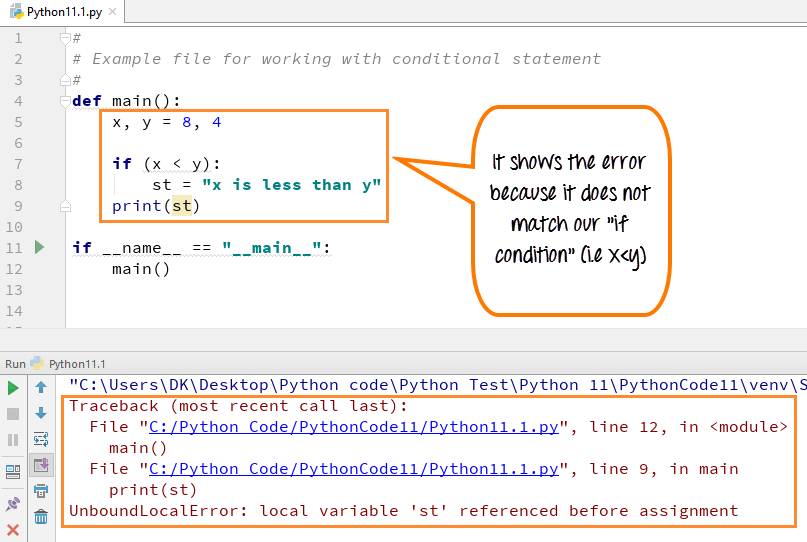

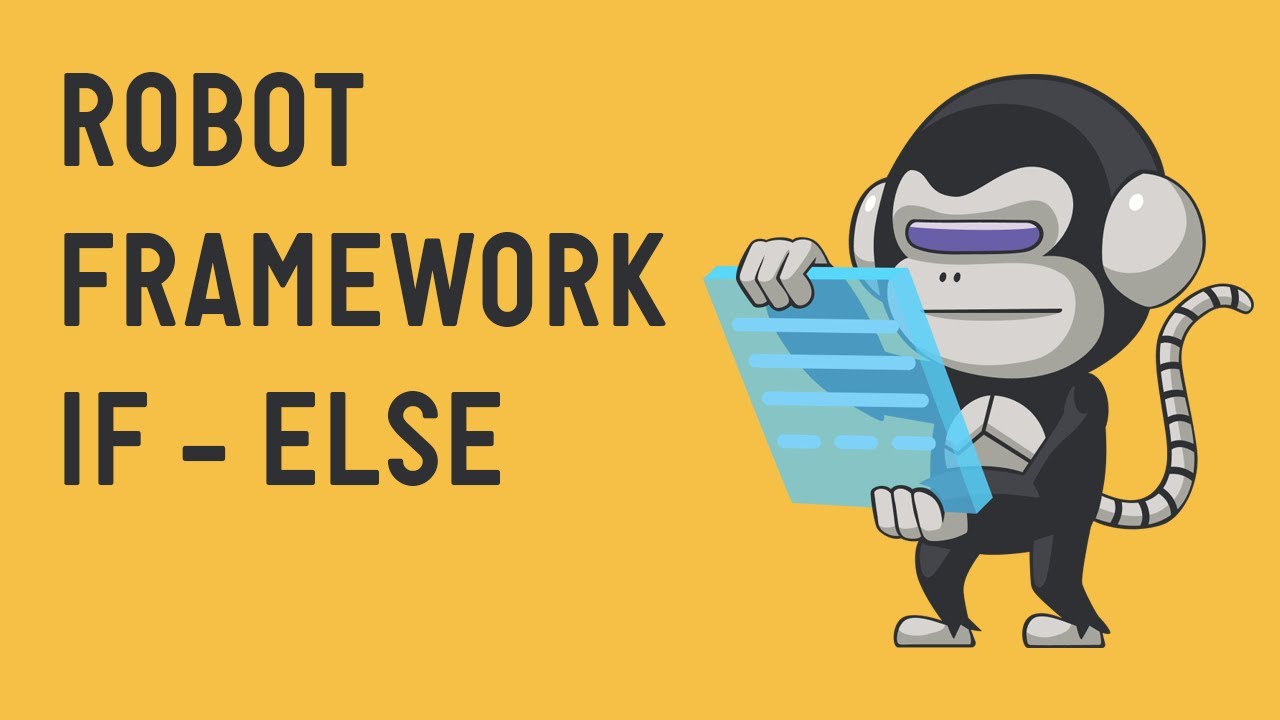
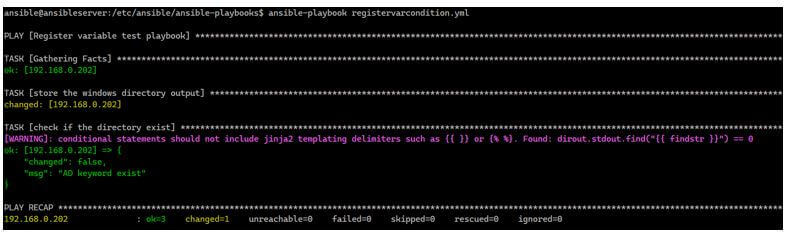
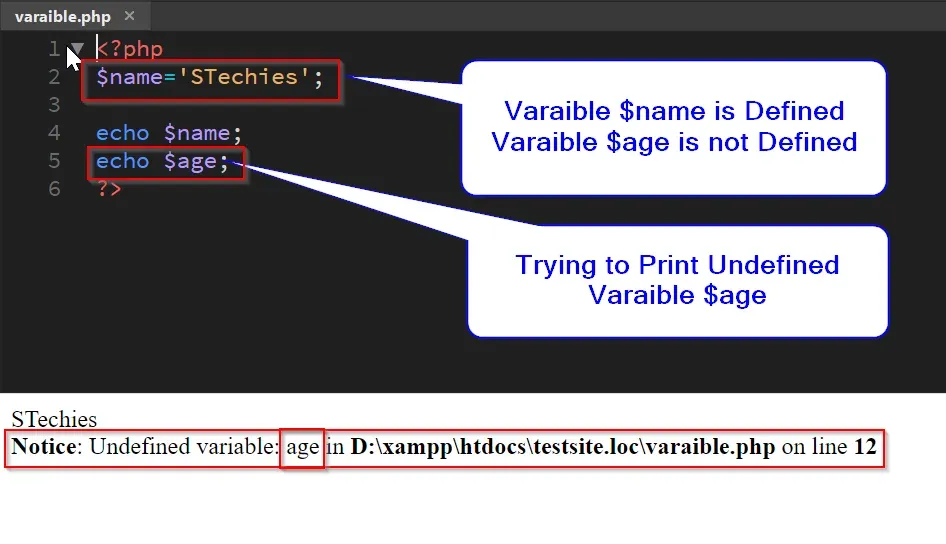

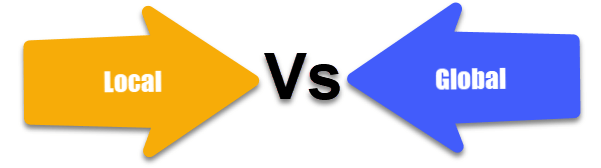

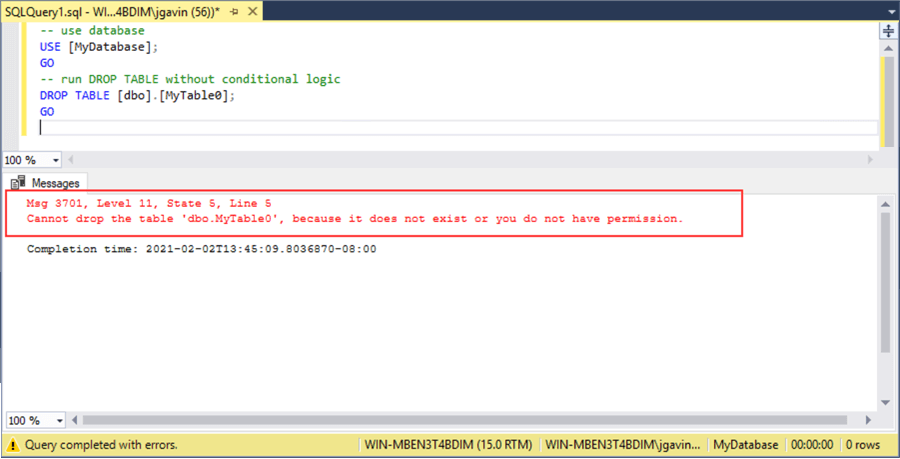
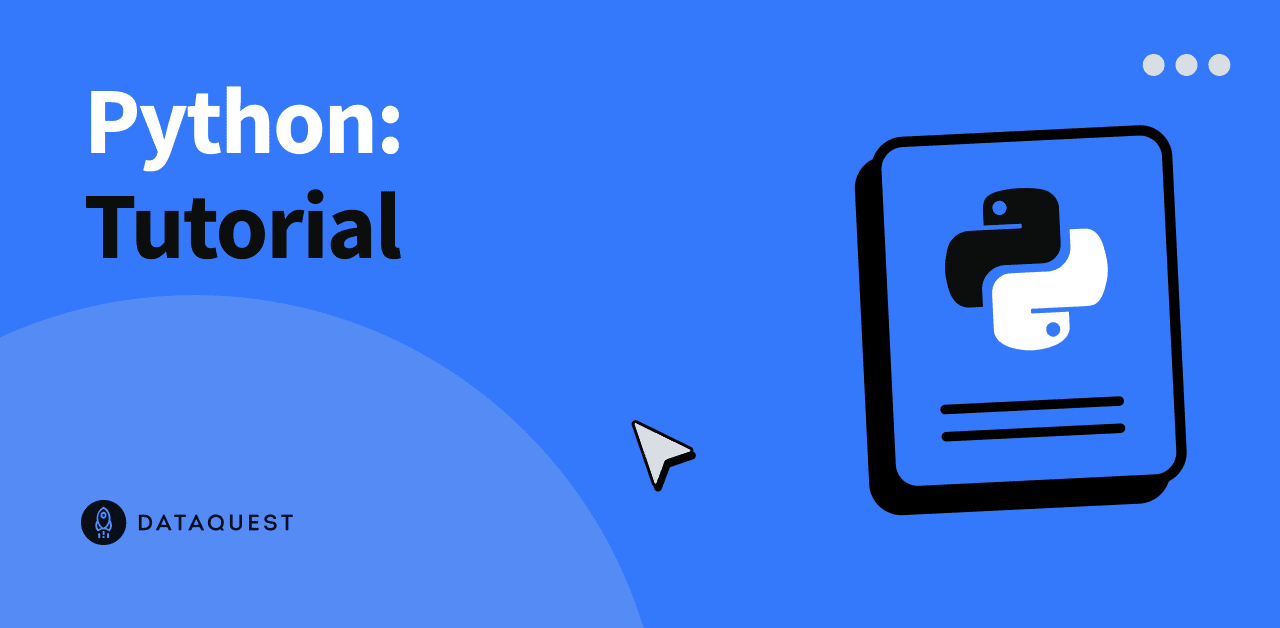
Article link: python if variable exists.
Learn more about the topic python if variable exists.
- How do I check if a variable exists? – Stack Overflow
- How to check if a Python variable exists? – GeeksforGeeks
- How to Check if a Variable Exists in Python – Studytonight
- Testing if a Variable Is Defined – Python Cookbook [Book]
- How do I check if a Python variable exists – Tutorialspoint
- 3 Easy Ways to Check If Variable Exists in Python – AppDividend
- How to check if a variable exists in Python – Adam Smith
- How do I check if a variable exists in Python? – Gitnux Blog
- How to check whether a variable exists in Python – Reactgo
- How to check if a variable exists in Python – Renan Moura
See more: nhanvietluanvan.com/luat-hoc