Python If Else In One Line
The if-else statement is a fundamental construct in programming that allows for conditional execution of code. In Python, the if-else statement can be written in a concise manner using a one-liner syntax. In this article, we will explore the various aspects of the Python if-else statement and delve into using it in one line. Additionally, we will discuss best practices and provide answers to frequently asked questions regarding this topic.
What is the if-else statement?
The if-else statement is a control flow statement that executes a set of instructions based on a condition. It allows a program to make decisions and perform different actions based on whether a given condition is true or false. The if-else statement consists of the keyword “if,” followed by a condition, and is often concluded with the keyword “else” to specify the alternative action.
Basic syntax of the if-else statement:
The basic syntax of the if-else statement in Python is as follows:
“`python
if condition:
statement(s)
else:
statement(s)
“`
The condition is a Boolean expression that evaluates to either true or false. If the condition is true, the statement(s) under the “if” block will be executed. Otherwise, if the condition is false, the statement(s) under the “else” block will be executed.
Using the if-else statement for simple conditions:
The if-else statement is commonly used for simple conditions where the execution of a single statement is determined by the condition’s outcome. For example:
“`python
x = 10
if x > 5:
print(“x is greater than 5”)
else:
print(“x is less than or equal to 5”)
“`
In this case, if the value of x is greater than 5, the output will be “x is greater than 5.” Otherwise, if x is less than or equal to 5, the output will be “x is less than or equal to 5.”
Using the if-else statement for complex conditions:
The if-else statement can also handle more complex conditions by incorporating logical operators and multiple comparisons. For instance:
“`python
x = 10
y = 20
if x > 5 and y > 15:
print(“Both conditions are true”)
else:
print(“At least one condition is false”)
“`
In this example, if both x is greater than 5 and y is greater than 15, the output will be “Both conditions are true.” Otherwise, if at least one of the conditions is false, the output will be “At least one condition is false.”
Nested if-else statements:
Python allows for the nesting of if-else statements, meaning one if-else statement can be included within another. This is particularly useful when dealing with scenarios that have multiple levels of conditions. Here is an illustration:
“`python
x = 5
if x > 10:
print(“x is greater than 10”)
else:
if x > 7:
print(“x is greater than 7 but less than or equal to 10”)
else:
print(“x is less than or equal to 7”)
“`
In this nested if-else statement, the output will be “x is less than or equal to 7” if x is less than or equal to 7. If x is greater than 7 but less than or equal to 10, the output will be “x is greater than 7 but less than or equal to 10.” Lastly, if x is greater than 10, the output will be “x is greater than 10.”
Using multiple elif statements:
In addition to “if” and “else,” Python provides the “elif” keyword, which stands for “else if.” This allows for handling multiple conditions in a more concise and readable manner. Here’s an example:
“`python
x = 7
if x > 10:
print(“x is greater than 10”)
elif x > 5:
print(“x is greater than 5 but less than or equal to 10”)
else:
print(“x is less than or equal to 5”)
“`
In this case, if x is greater than 10, the output will be “x is greater than 10.” If x is greater than 5 but less than or equal to 10, the output will be “x is greater than 5 but less than or equal to 10.” Otherwise, if x is less than or equal to 5, the output will be “x is less than or equal to 5.”
Ternary operator as a shorthand for simple if-else statements:
Python offers a concise way to express simple if-else statements known as the ternary operator. It follows the format: `result_if_true if condition else result_if_false`. Consider the following example:
“`python
x = 10
output = “x is greater than 5” if x > 5 else “x is less than or equal to 5”
print(output)
“`
In this case, if x is greater than 5, the output will be “x is greater than 5.” Otherwise, if x is less than or equal to 5, the output will be “x is less than or equal to 5.” The result is assigned to the `output` variable and then printed.
Chaining multiple if-else statements for complex scenarios:
By combining multiple if-else statements and logical operators, you can handle complex scenarios with numerous conditions. Here’s an example:
“`python
x = 10
y = 20
if x > 5 and y > 15:
print(“Condition 1”)
elif x < 5 and y > 15:
print(“Condition 2”)
else:
print(“Condition 3”)
“`
In this example, if both x is greater than 5 and y is greater than 15, the output will be “Condition 1.” If x is less than 5 and y is greater than 15, the output will be “Condition 2.” Finally, if none of the previous conditions are met, the output will be “Condition 3.”
Best practices for using if-else statements in Python:
1. Keep the conditions and statements as concise and readable as possible.
2. Use meaningful variable and condition names to enhance code clarity.
3. Avoid unnecessary nesting of if-else statements to maintain code simplicity.
4. Make use of elif statements instead of multiple if statements when dealing with multiple conditions.
5. Use comments to explain complex conditions or logic for better understanding.
FAQs (Frequently Asked Questions):
Q: Can an if statement exist without an else statement in Python?
A: Yes, an if statement can exist without an else statement. In such cases, if the condition is true, the code within the if block will be executed, but if the condition is false, no alternative action will be performed.
Q: Is it possible to write an if statement in one line in Python without else?
A: Yes, it is possible to write an if statement in one line without an else statement using the ternary operator. The format would be `statement if condition else None`.
Q: How can I write a for loop in one line in Python?
A: Python provides the ability to write a for loop in one line using list comprehensions. For example: `[expression for item in iterable]`.
Q: What is the one-line Python syntax?
A: One-line Python syntax refers to writing code or statements in a single line, often to achieve conciseness and elegance. It is important to ensure that the code remains readable and does not sacrifice clarity for compactness.
Q: Is it possible to write an if-else statement in one line in JavaScript?
A: No, JavaScript does not have a specific syntax for writing if-else statements in one line. However, ternary operators can be used to achieve similar effects in a more concise manner.
Q: How can I assign a value to a variable based on an if-else condition in Python?
A: The if-else statement can be used to assign a value to a variable based on a condition. For example: `x = a if condition else b`.
Q: What is the difference between if else in Python 2 and Python 3?
A: Both Python 2 and Python 3 support the if-else statement. However, Python 3 introduced some changes and improvements in syntax and functionality. The key differences include the print statement, division operator, and handling of Unicode strings.
Q: Can I write if-else statements in one line in Java?
A: No, Java does not provide a direct syntax for writing if-else statements in a single line. If-else statements in Java must be written using the traditional block format.
In conclusion, the if-else statement is a vital tool for decision-making and branching in programming. Python’s ability to write if-else statements in a single line offers concise and elegant code solutions. By understanding the syntax, handling complex conditions, and following best practices, programmers can effectively utilize if-else statements in Python to create efficient and intuitive code structures.
One Line If Else Statement In Python
Keywords searched by users: python if else in one line Python if without else, If in one line python without else, For in one line Python, Python one line, If…else one line JavaScript, If else assignment python, If else Python 3, If else one line Java
Categories: Top 75 Python If Else In One Line
See more here: nhanvietluanvan.com
Python If Without Else
In most programming languages, the ‘else’ statement is used to define a block of code that executes when the if condition evaluates to false. However, Python offers a unique alternative to this conventional approach. By omitting the ‘else’ clause, developers can write more concise and expressive code.
The absence of ‘else’ can be particularly useful when the alternative code block is empty or irrelevant. In such cases, including an ‘else’ statement would be unnecessary and could clutter the code. By omitting ‘else’, Python allows developers to focus solely on the primary condition and its corresponding code block, resulting in cleaner and more maintainable code.
Furthermore, omitting ‘else’ can also enhance code readability, especially when handling multiple if conditions. With the ‘if without else’ approach, each if condition stands on its own, making the code easier to understand and debug. This can be particularly beneficial when dealing with complex conditions or nested if statements.
Another scenario where omitting ‘else’ is advantageous is when using guard clauses. Guard clauses are a style of coding where you return early from a function or method to handle exceptional cases or edge conditions. This approach eliminates nested if-else structures and enhances code readability. Python’s ability to exclude ‘else’ makes it a suitable language for implementing guard clauses effectively.
Although omitting the ‘else’ statement offers benefits, it is important to consider potential drawbacks. For instance, not including ‘else’ may lead to unexpected behavior if the condition is not satisfied. In such cases, the code will proceed to the next section without any indication of failure. This can be a source of bugs that are difficult to trace, especially in large codebases. Thus, careful consideration and extensive testing are recommended when using this approach.
FAQs:
Q: Can I omit ‘else’ for all if statements in Python?
A: No, it is not advisable to omit ‘else’ for all if statements. While excluding ‘else’ can make code more concise, it is essential to consider the specific situation. If the condition requires an alternative code block, it is best to include the ‘else’ statement to ensure the desired behavior.
Q: What is the alternative to ‘else’ in Python?
A: The ‘pass’ statement is often used as an alternative to ‘else’ when an empty code block is desired. Instead of defining an alternative block of code, ‘pass’ allows you to indicate that no action is necessary in that case.
Q: Are there any performance benefits of omitting ‘else’ in Python?
A: The performance impact of excluding ‘else’ is negligible. Python’s interpreter is optimized to handle both scenarios efficiently. Therefore, the choice to use or omit ‘else’ should be based on code readability and maintainability rather than performance considerations.
Q: How can I determine if excluding ‘else’ is appropriate for my code?
A: Deciding whether to omit ‘else’ depends on the specific conditions and context of your code. Consider if an alternative code block is needed and if its absence would make the code clearer. Additionally, assess if excluding ‘else’ could introduce bugs or make debugging difficult. Conduct thorough testing to ensure the desired behavior is achieved.
In conclusion, Python’s ability to write code without using the ‘else’ statement offers developers a more concise and expressive way to structure their code. This approach can enhance readability, particularly when dealing with complex conditions or implementing guard clauses. However, caution should be exercised to ensure unintended behavior or bugs are avoided. By understanding the advantages and limitations of omitting ‘else’, developers can make informed decisions about when to utilize this approach in their Python projects.
If In One Line Python Without Else
If Statements in Python
In Python, the if statement is used to check if a certain condition is true or false. It follows a specific syntax:
“`python
if condition:
# code to be executed if the condition is true
else:
# code to be executed if the condition is false
“`
The `condition` inside the `if` statement can be any expression that returns a boolean value (either True or False). If the condition is true, the code within the `if` block will be executed. Otherwise, the code within the `else` block will be executed.
Using “if” Without “else”
There are instances where we only want to execute a block of code when a certain condition is met, without specifying any actions if the condition is not fulfilled. In these cases, we can omit the `else` block and use the “if” statement alone, without an accompanying “else”. Here’s an example:
“`python
x = 10
if x > 5:
print(“The value of x is greater than 5.”)
“`
In this example, the code checks if the value of `x` is greater than 5. If the condition is true, it will print the corresponding message. If the condition is false, nothing will happen, and the program will continue executing the next line of code after the “if” block.
Benefits of Using “if” Without “else”
Using “if” without an “else” can simplify our code when we don’t need to specify any actions for when the condition is false. It makes our code more concise and easier to read by removing unnecessary code blocks. Additionally, it can help avoid potential logical errors by explicitly expressing that we don’t want any actions when the condition is false.
Potential Drawbacks and Considerations
When using “if” without “else”, it’s important to consider the potential impact on code readability and maintainability. While this approach can be useful in certain scenarios, it may make the code less understandable, especially for other developers trying to comprehend its logic. Therefore, its usage should be limited to cases where the condition being tested is straightforward and the purpose of the conditional statement is clear.
FAQs
Q: Can we nest “if” statements without an “else”?
A: Yes, we can nest “if” statements without an “else” block just like we do with the regular “if” statements. For example:
“`python
x = 10
y = 5
if x > 5:
if y > 2:
print(“Both x and y meet the conditions.”)
“`
Q: Is it mandatory to have an “else” statement after an “if” statement in Python?
A: No, an “else” statement is not mandatory after an “if” statement in Python. Depending on the specific requirements of your program, you may choose to omit the “else” block if it’s not required.
Q: What happens if the condition in an “if” statement without “else” is false?
A: If the condition in an “if” statement without an “else” block is false, nothing will happen, and the program will continue executing the next line of code after the “if” block.
In conclusion, the “if” statement in Python can be used without an accompanying “else” block when we only need to execute a block of code if a specific condition is met. This approach allows for cleaner and more concise code in scenarios where actions for false conditions are not necessary. However, it’s important to use this approach judiciously to maintain code readability and understandability.
Images related to the topic python if else in one line
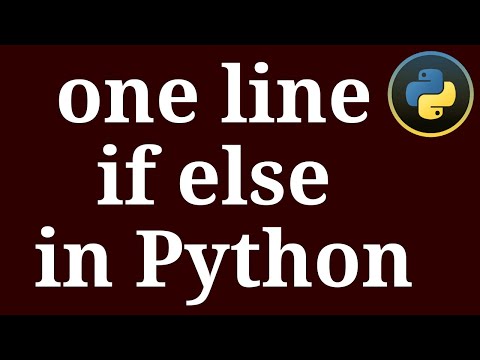
Found 13 images related to python if else in one line theme
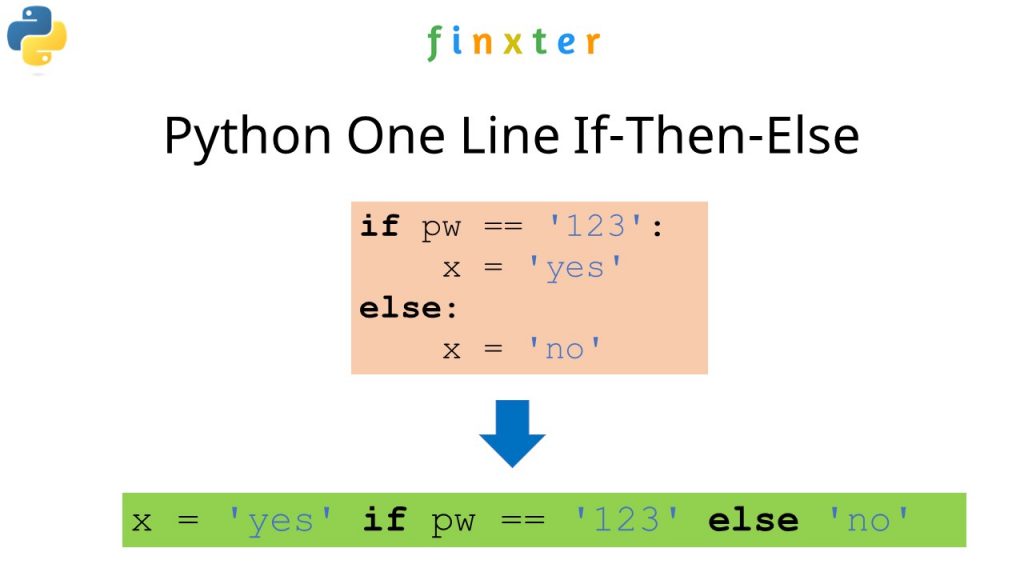
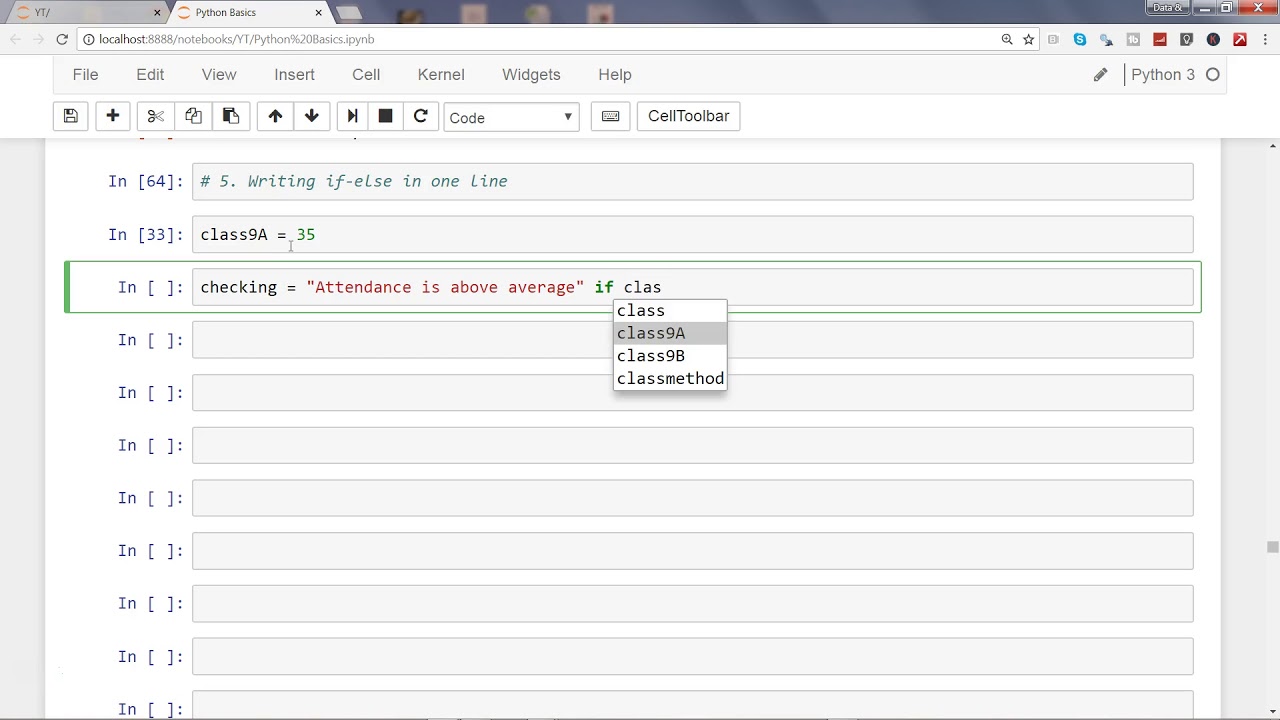
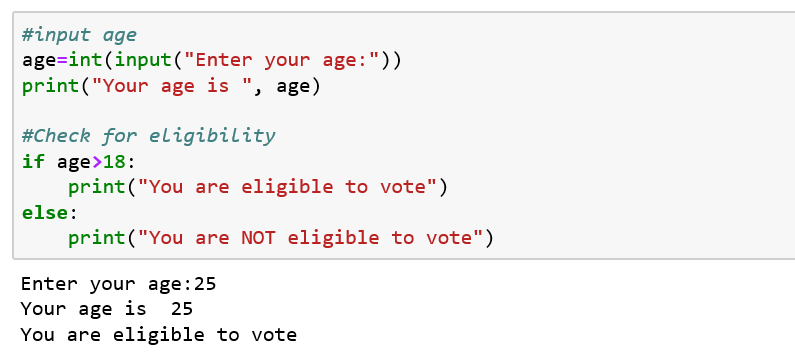
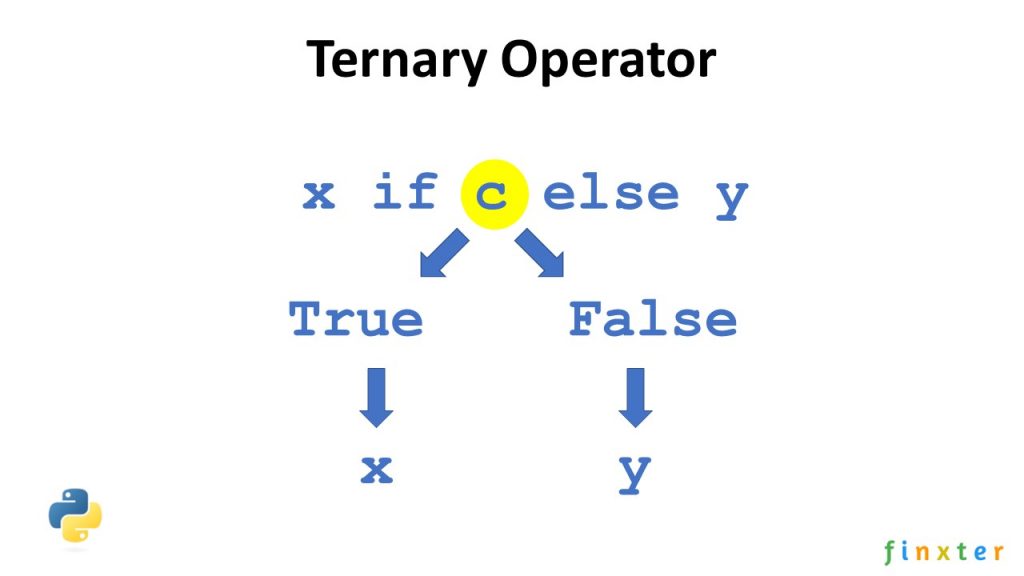
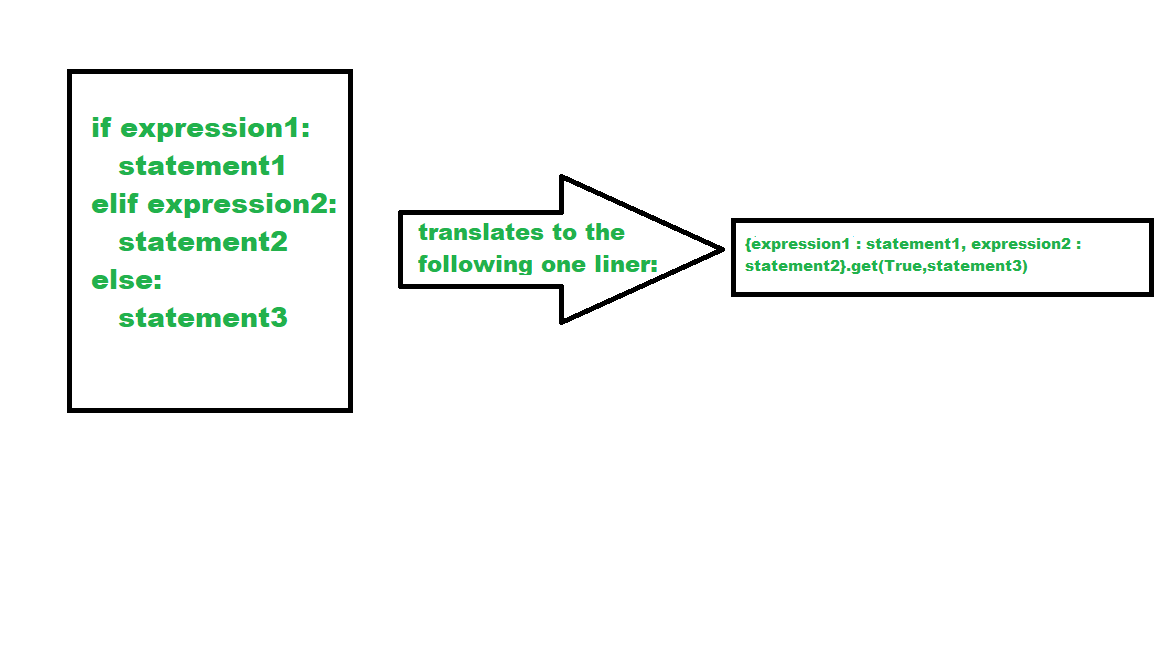
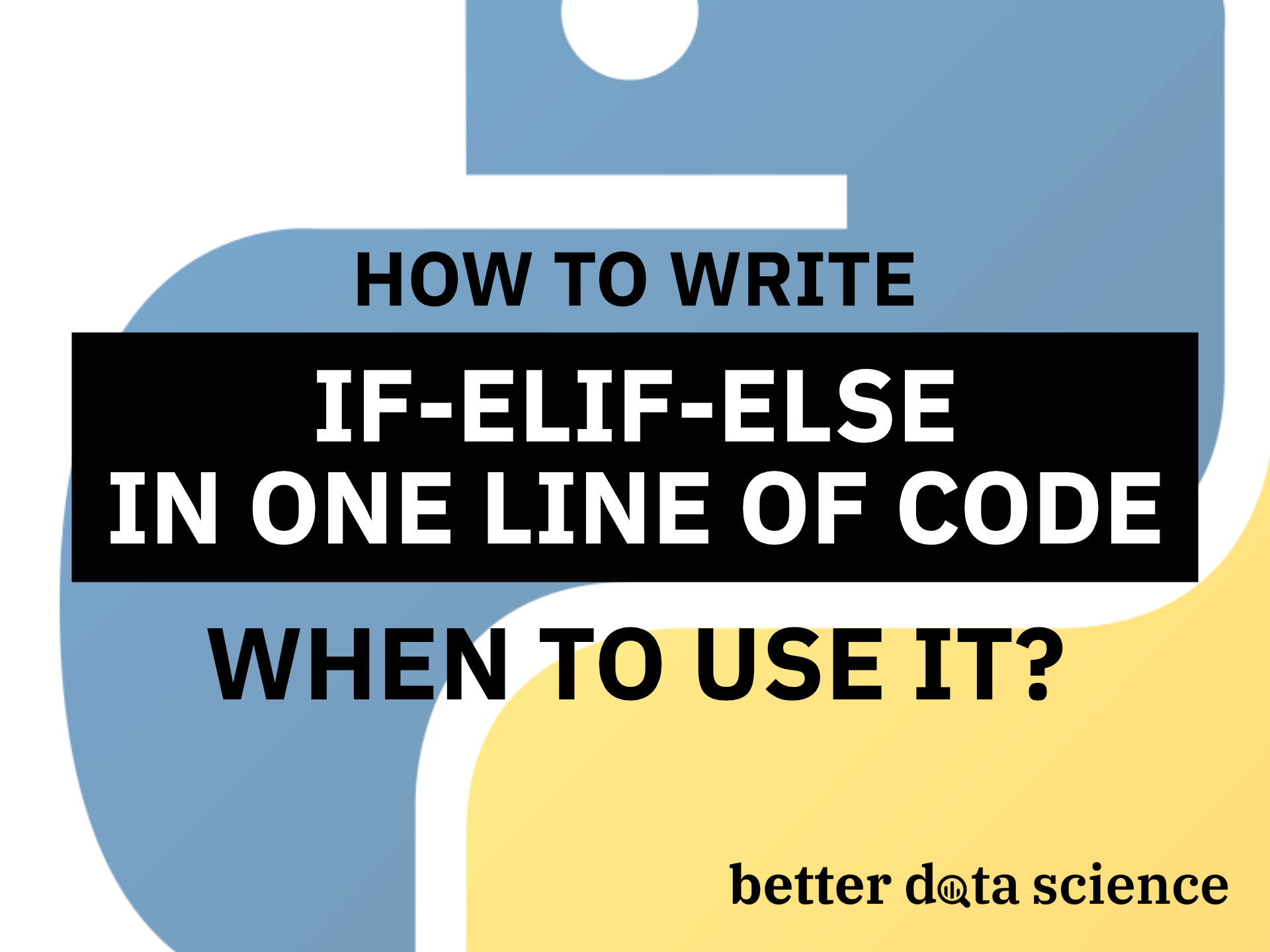
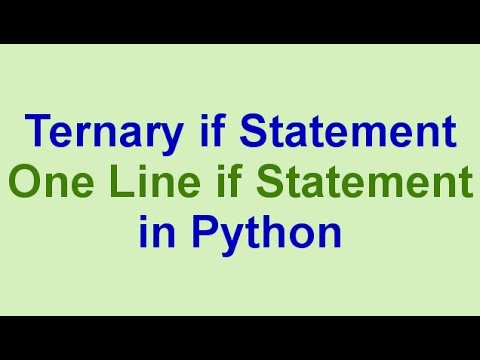
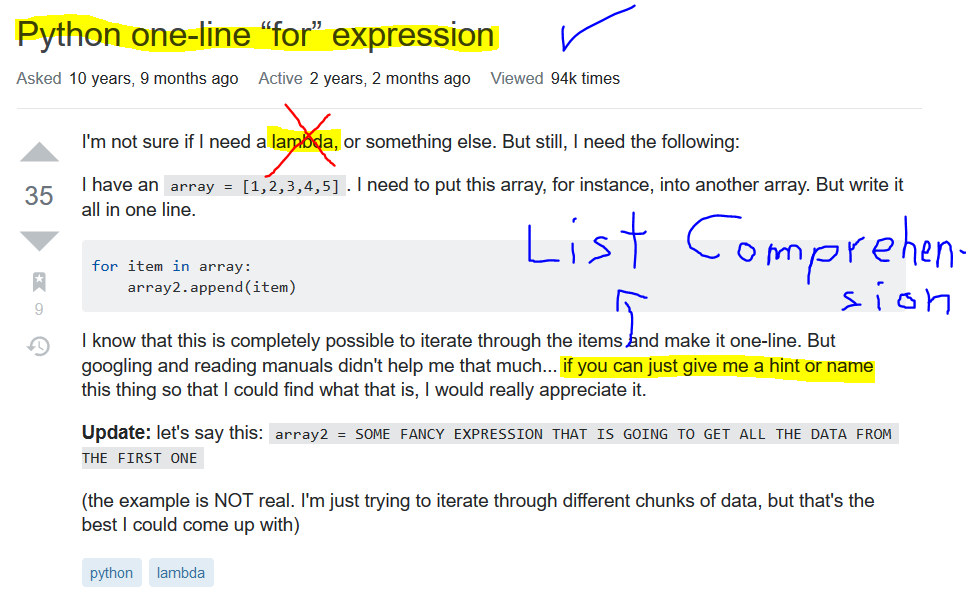
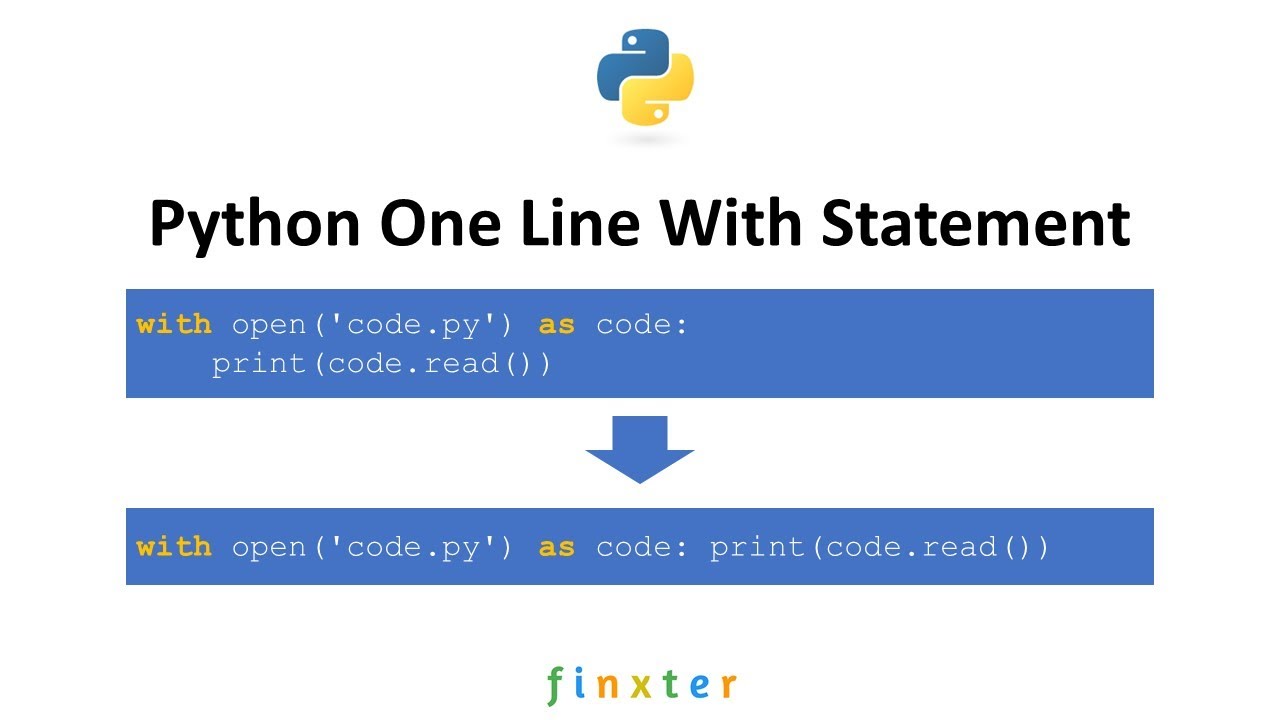



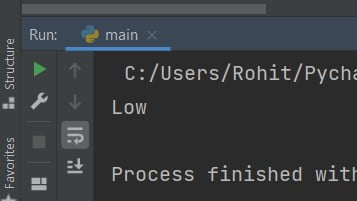
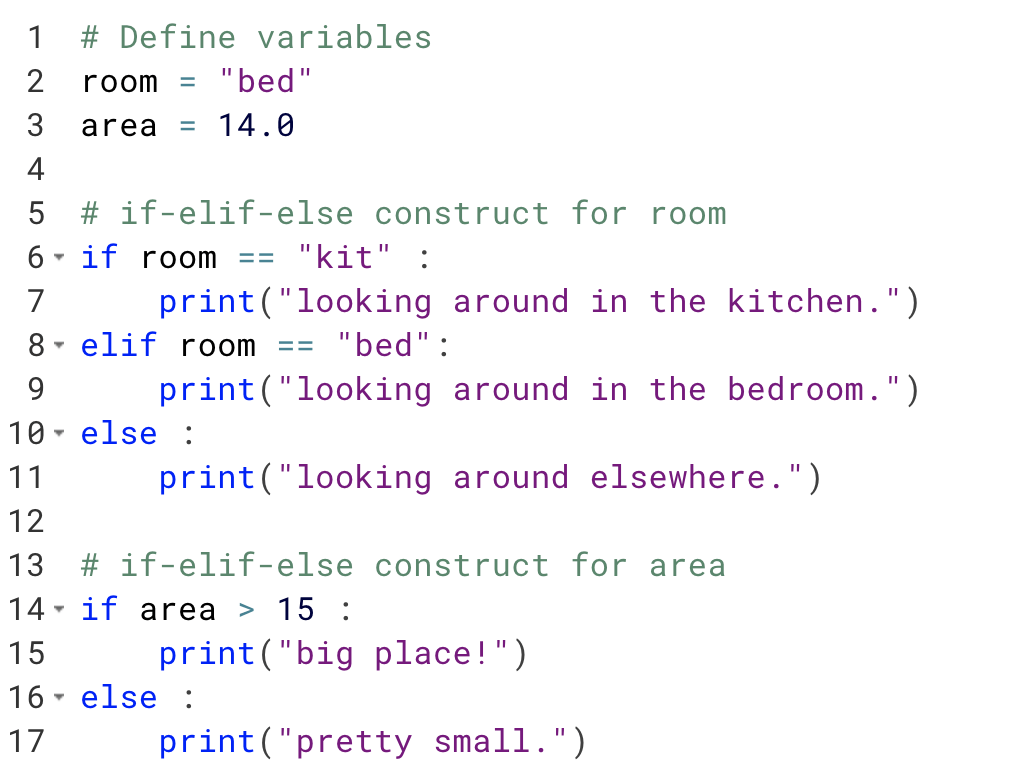
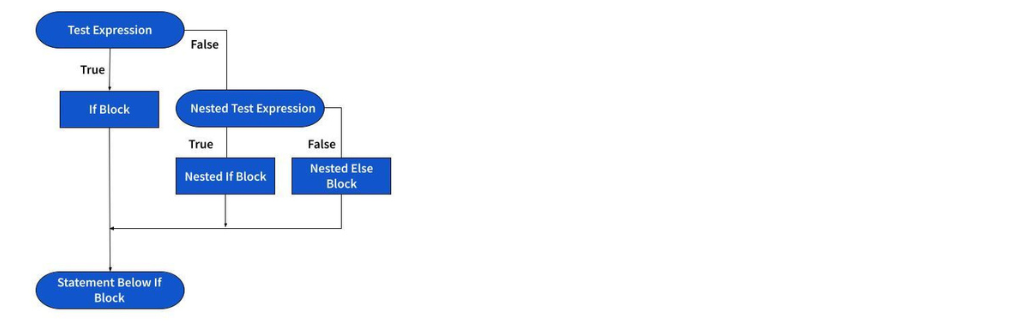

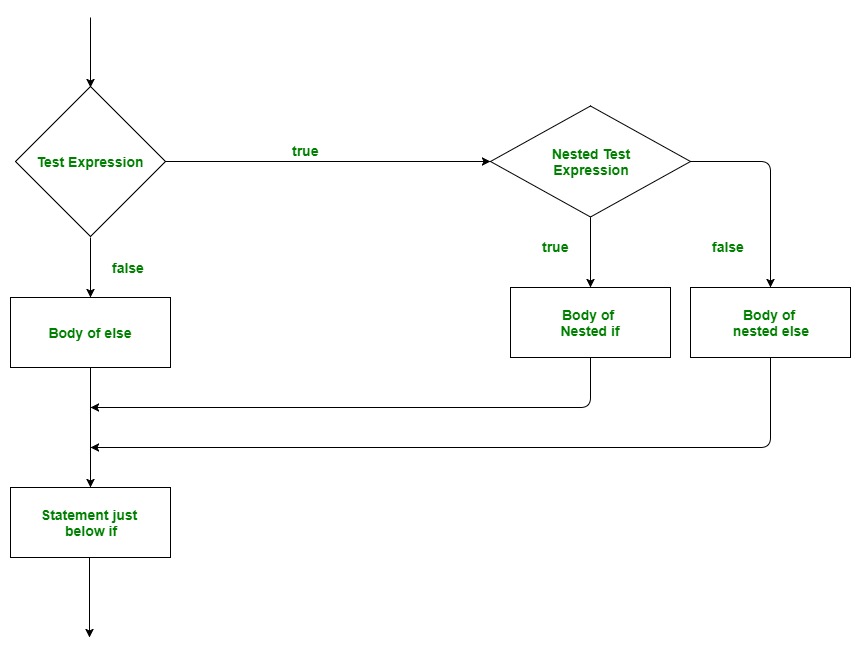

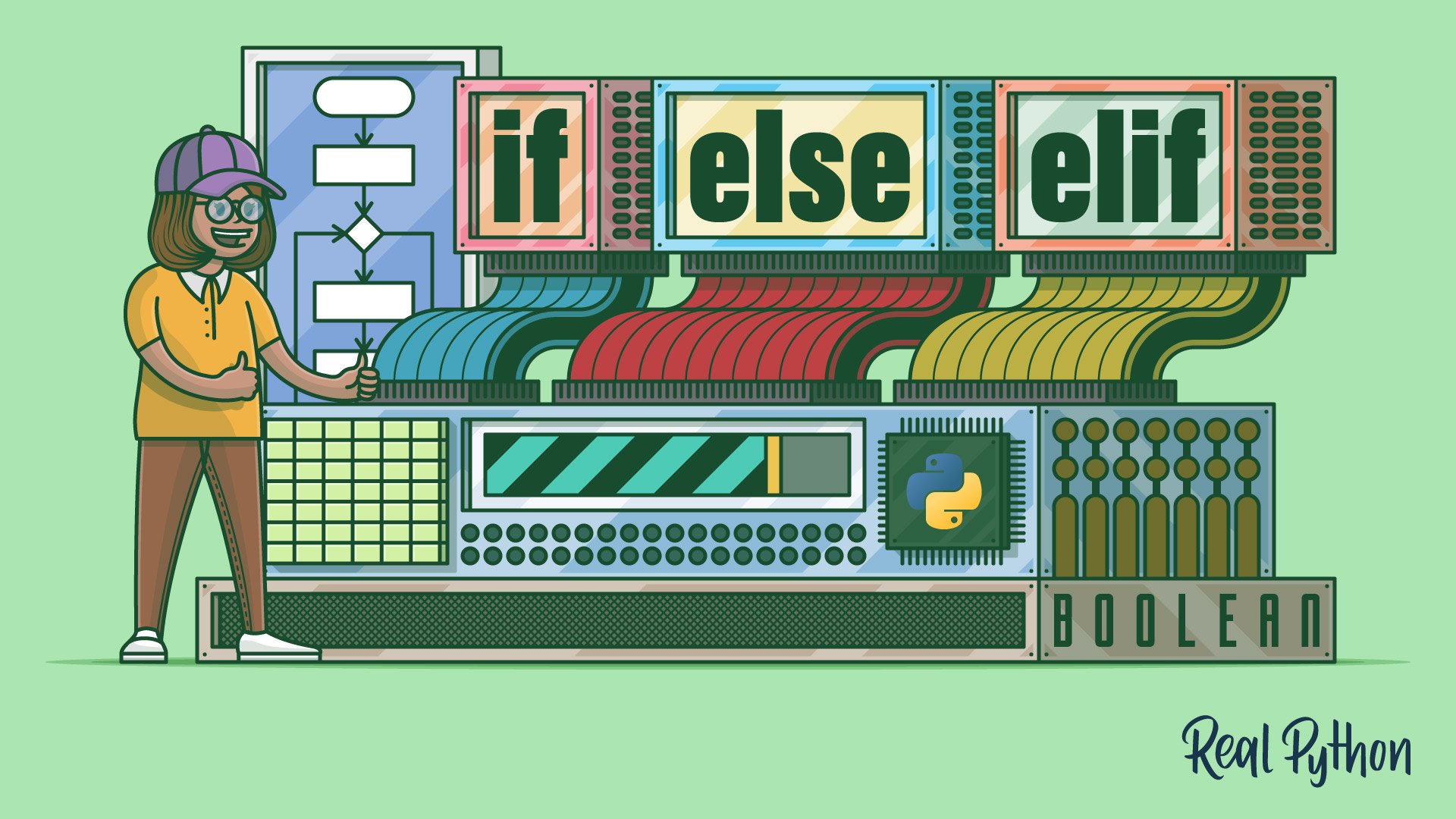
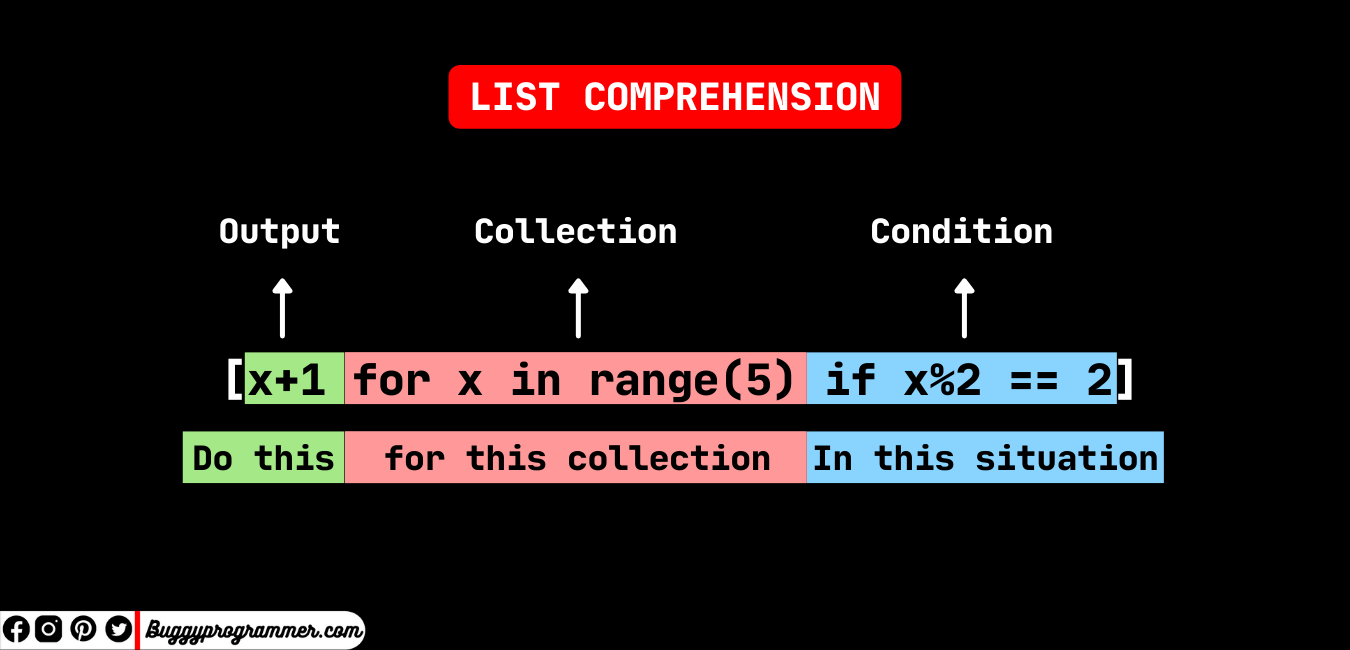
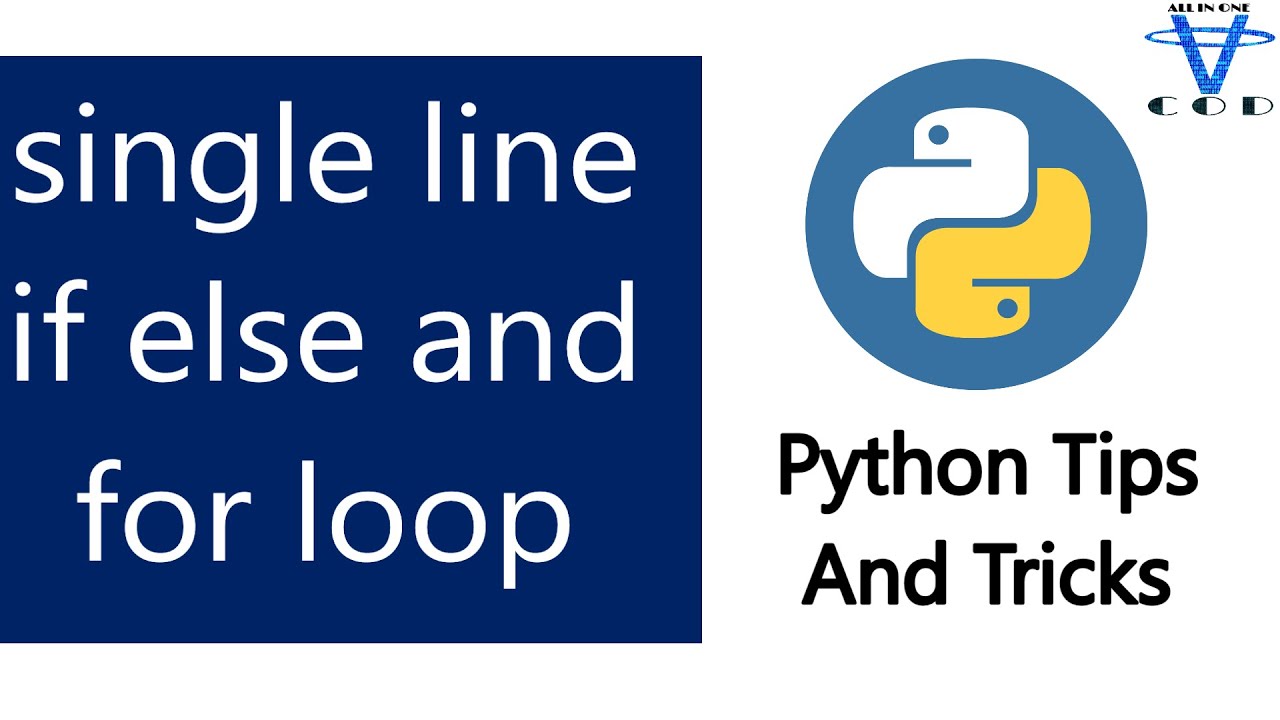
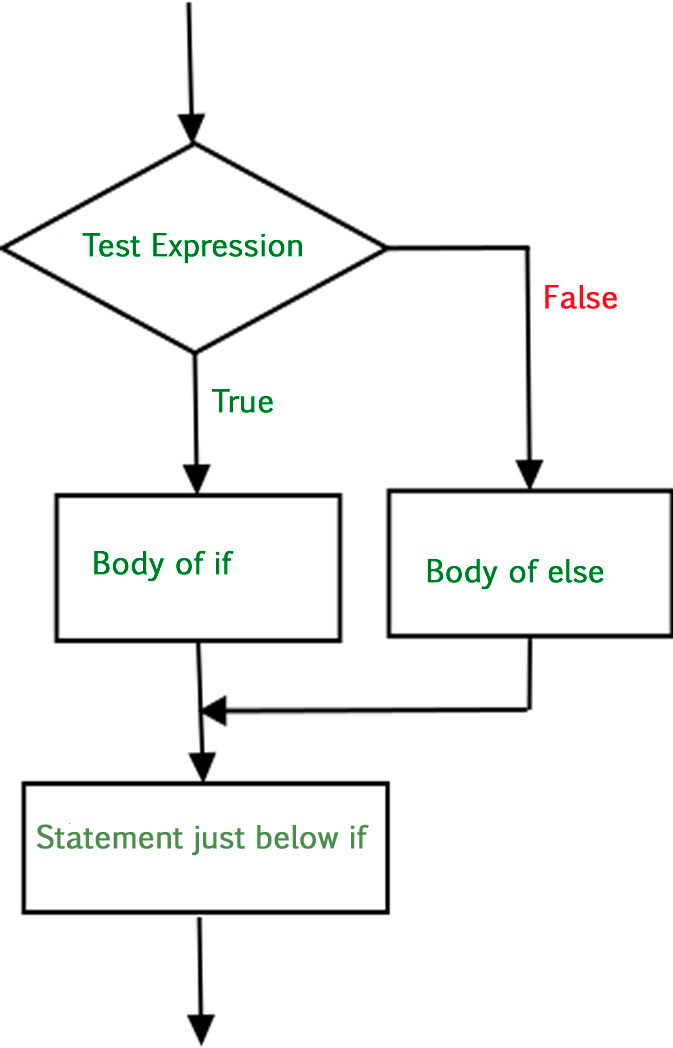
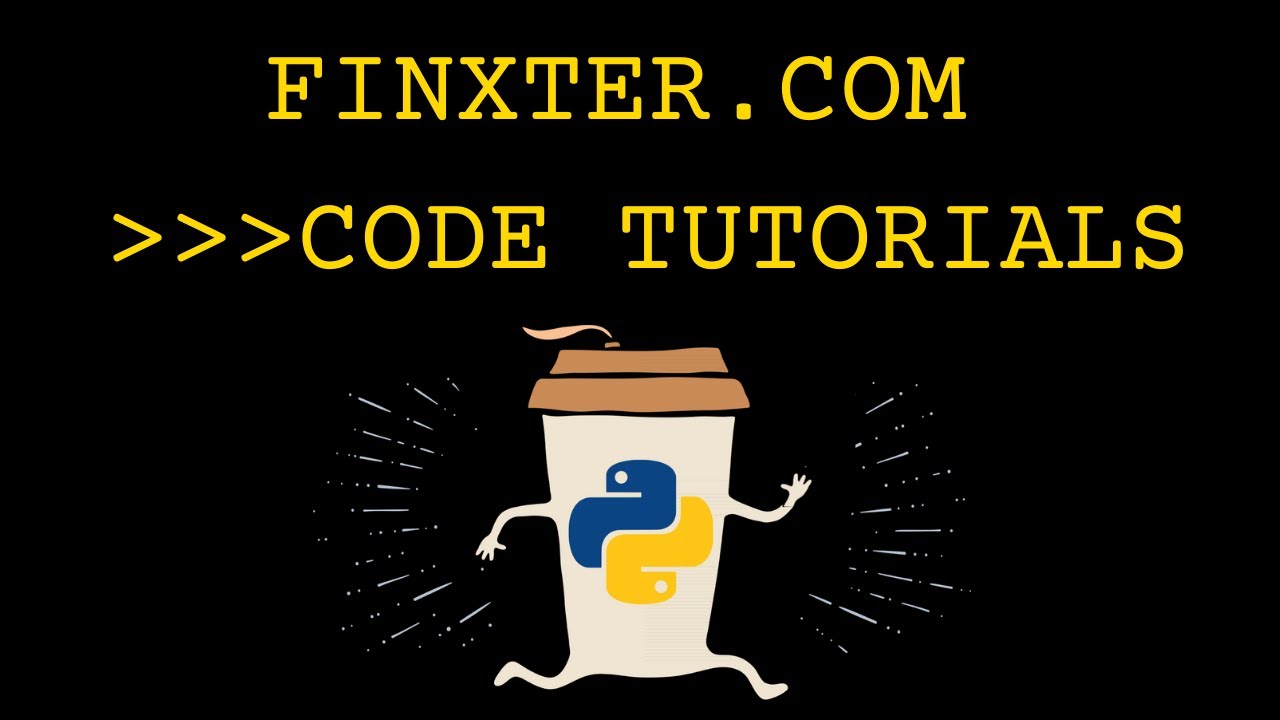
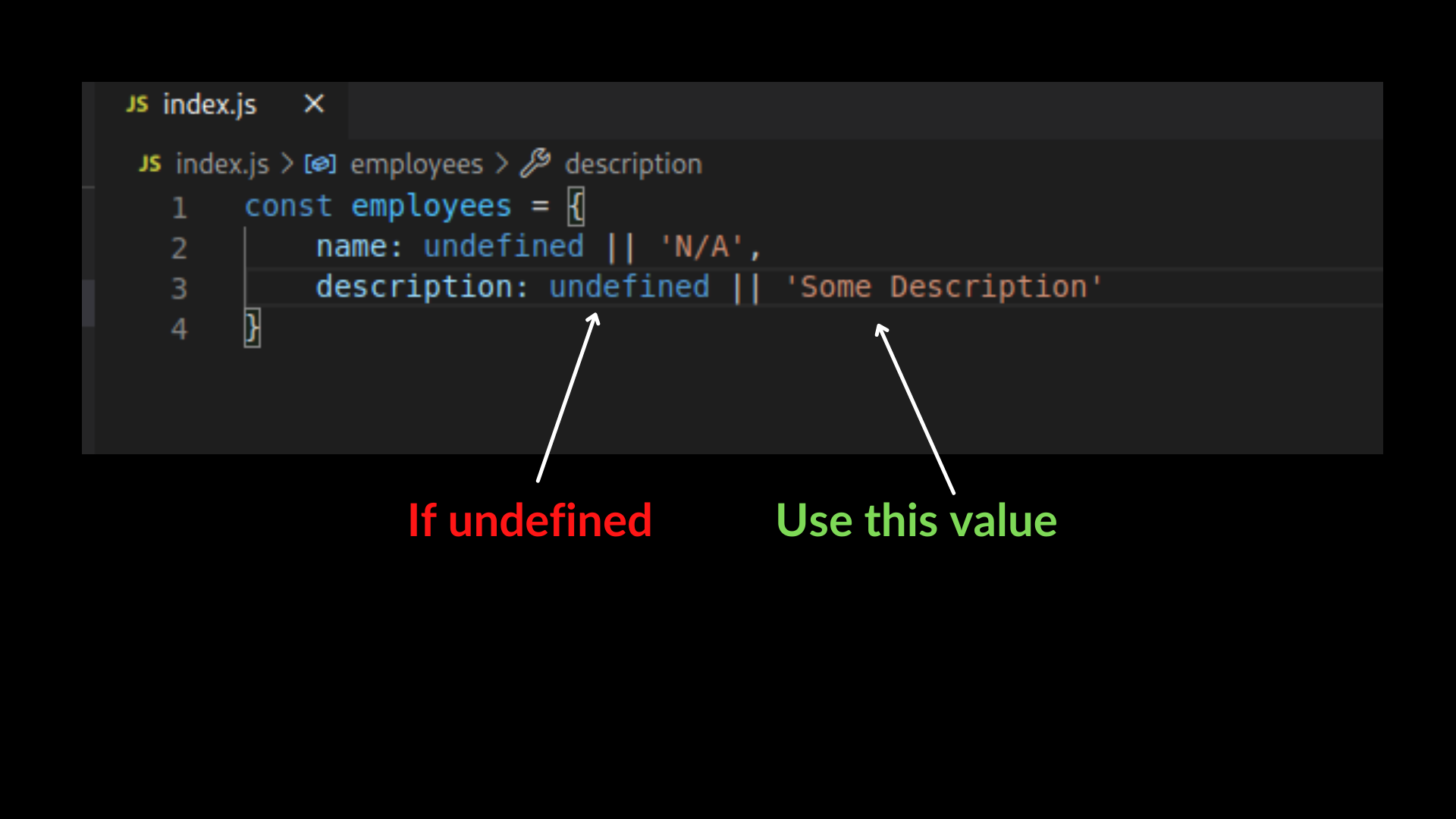
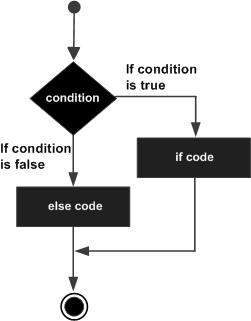
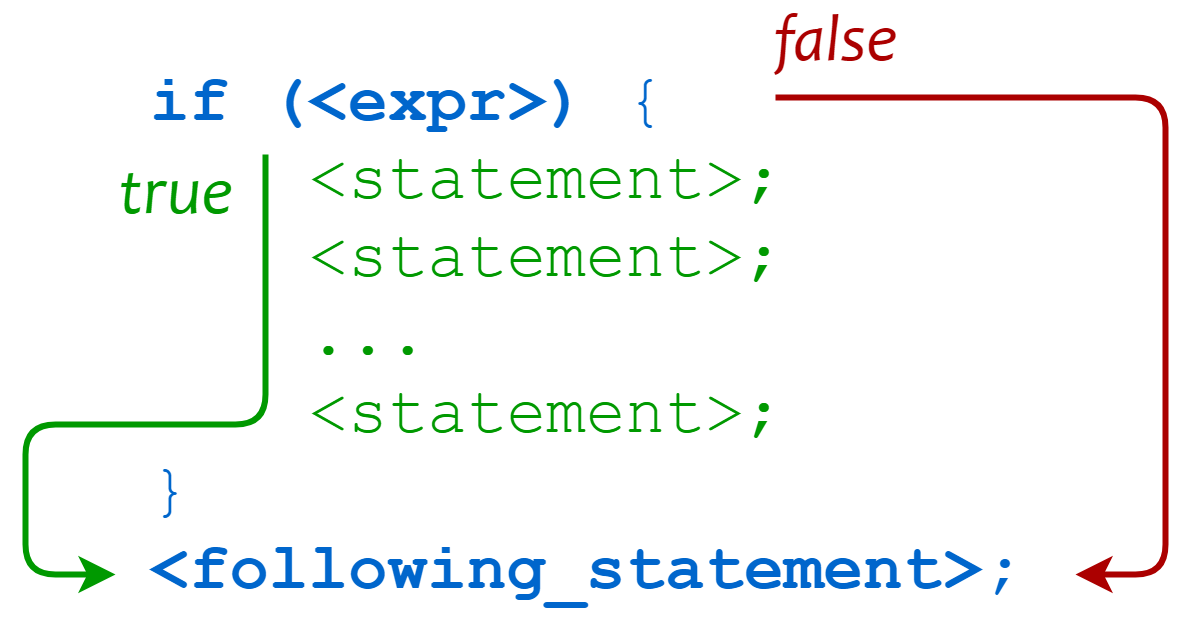



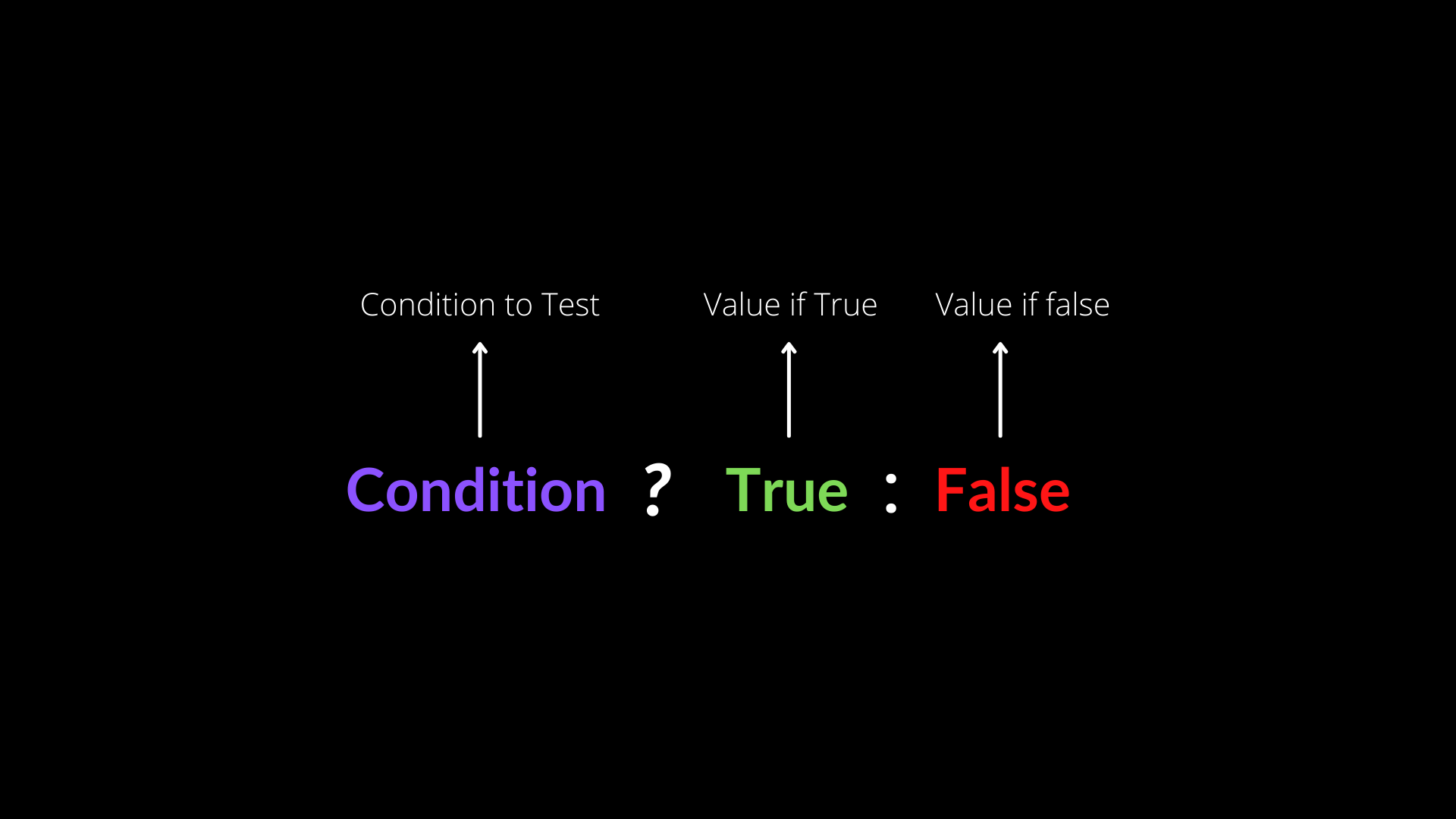
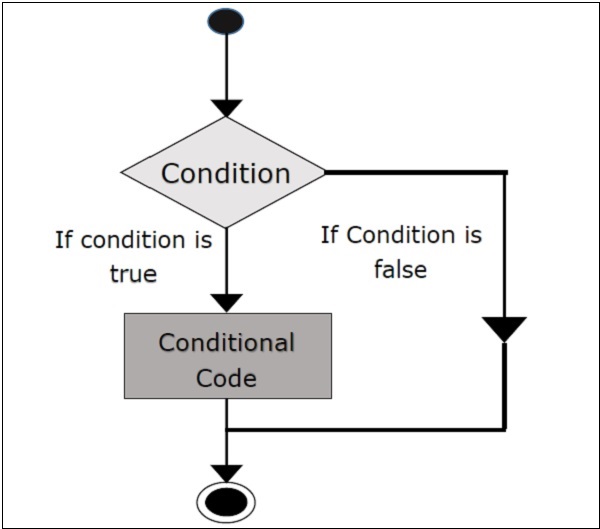

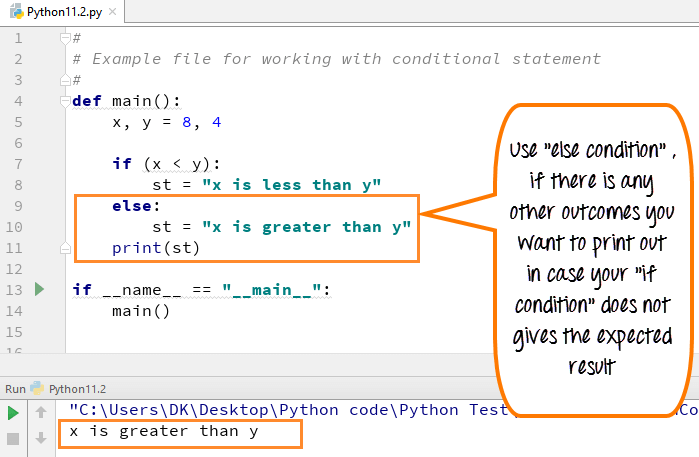
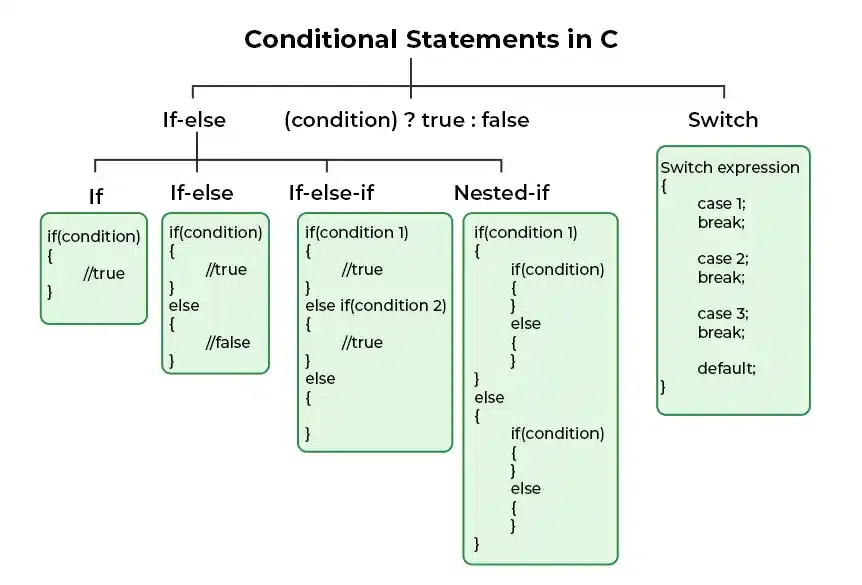
![Python One Line For Loop [A Simple Tutorial] – Be on the Right Side of Change Python One Line For Loop [A Simple Tutorial] – Be On The Right Side Of Change](https://blog.finxter.com/wp-content/uploads/2020/05/listcomp-1024x576.jpg)
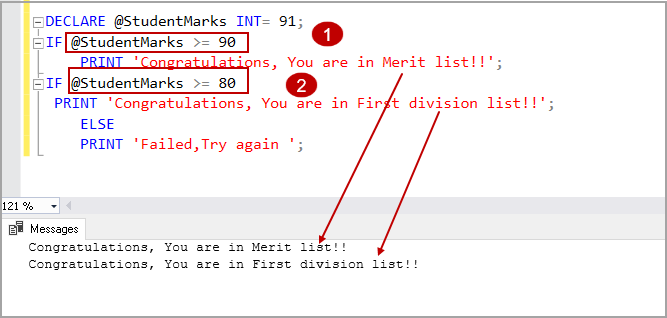

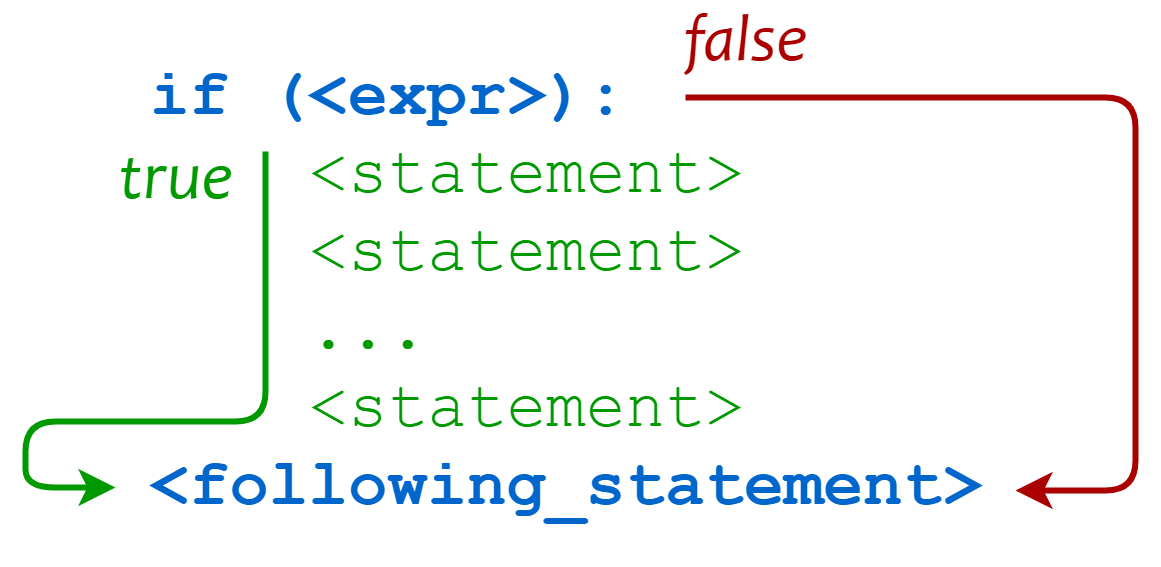



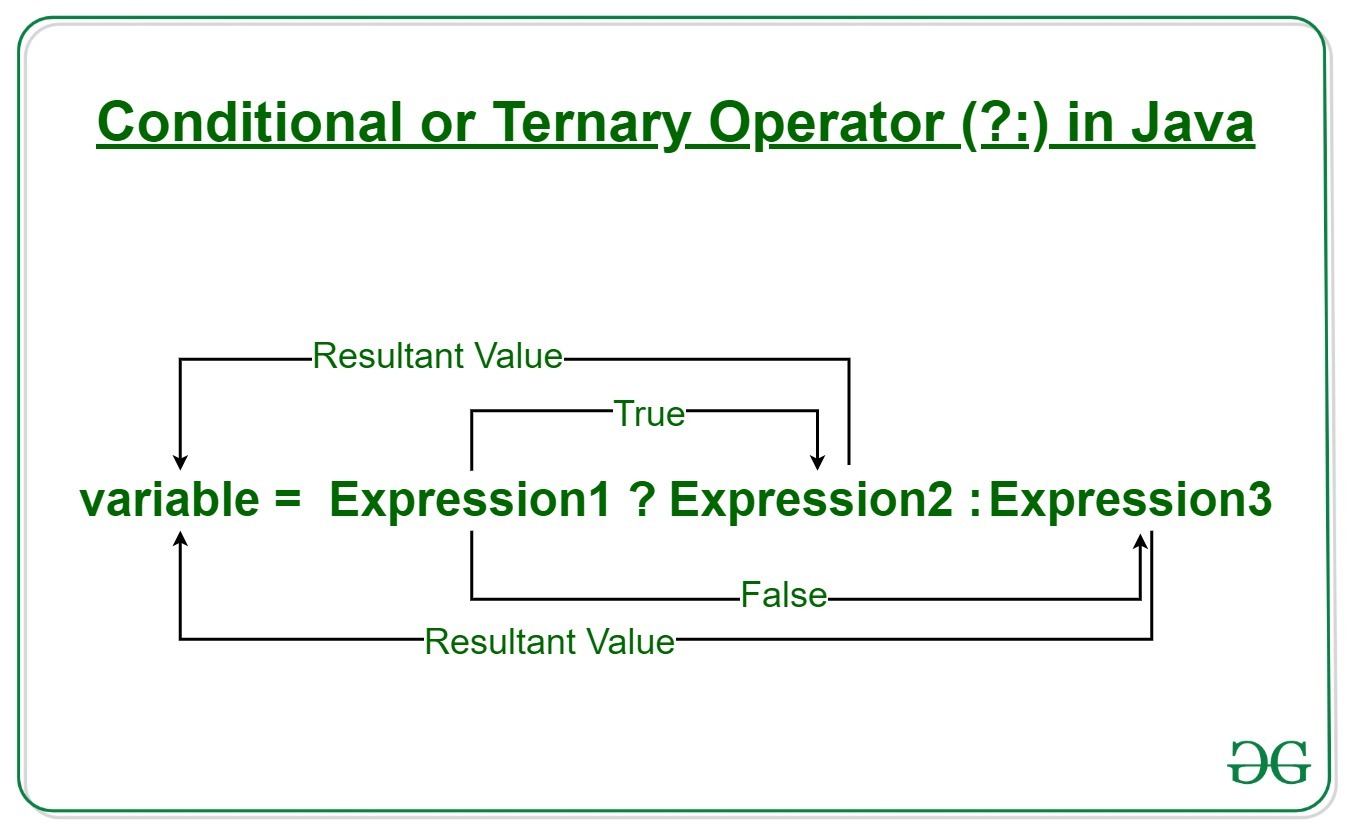
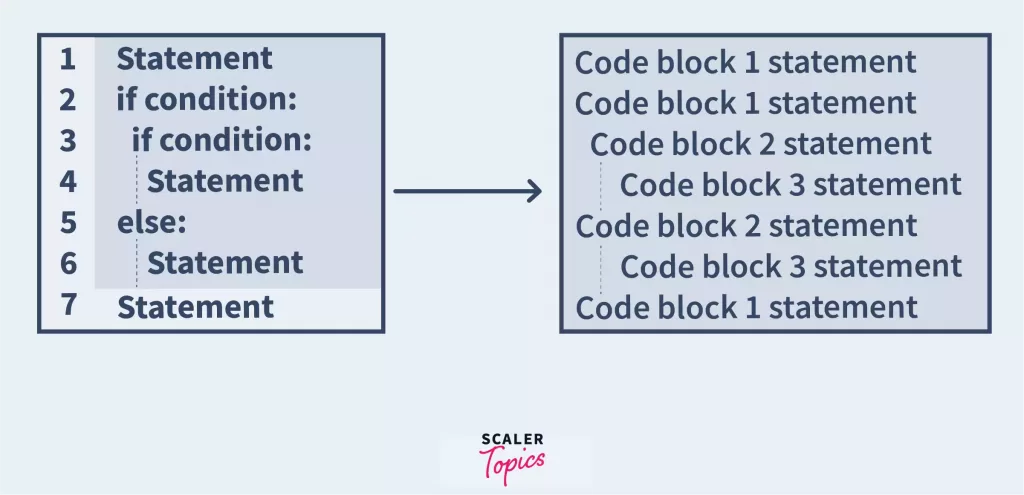

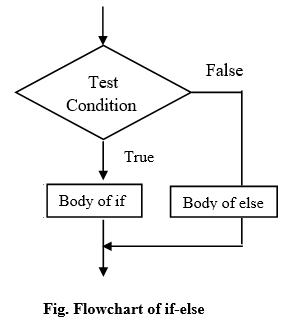

![Understanding Python If-Else Statement [Updated] | Simplilearn Understanding Python If-Else Statement [Updated] | Simplilearn](https://www.simplilearn.com/ice9/free_resources_article_thumb/Python_If_Else_Statement.jpg)
Article link: python if else in one line.
Learn more about the topic python if else in one line.
- Python If-Else on One Line – codingem.com
- Putting a simple if-then-else statement on one line [duplicate]
- How to Write the Python if Statement in one Line
- Python If-Else Statement in One Line – Ternary Operator …
- If-Then-Else in One Line Python – Finxter
- Python: if-else in one line – ( A Ternary operator ) – thisPointer
- Python Inline If-else – Linux Hint
- if-elif-else statement on one line in Python – bobbyhadz
- How to use python if else in one line with examples
- Inline If in Python: The Ternary Operator in Python – Datagy
See more: nhanvietluanvan.com/luat-hoc