Python Get Number Of Days In Month
Introduction:
In Python programming, there are several ways to obtain the number of days in a particular month. This article will explore different methods, including using the datetime module and creating a dictionary to map month names and their respective lengths. Additionally, we will discuss how to handle invalid input and identify leap years. By the end, you will have a comprehensive understanding of how to obtain the number of days in any given month using Python.
Months in a Year and their Respective Lengths:
Before diving into the methods of getting the number of days in a month, let’s first list the months of a year and their respective lengths:
1. January – 31 days
2. February – 28 or 29 days (leap year: 29 days)
3. March – 31 days
4. April – 30 days
5. May – 31 days
6. June – 30 days
7. July – 31 days
8. August – 31 days
9. September – 30 days
10. October – 31 days
11. November – 30 days
12. December – 31 days
Identifying a Leap Year:
Before determining the number of days in February, we must identify if the year is a leap year. A leap year occurs every 4 years, except for years that are divisible by 100 but not divisible by 400. Here’s a Python function that can help us identify a leap year:
“`python
def is_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
return True
return False
return True
return False
“`
Calculating the Number of Days in a Month:
Now that we know how to determine if a year is a leap year, we can calculate the number of days in a month. Here’s a Python function that takes in a month and a year as input and returns the number of days in that month:
“`python
def get_days_in_month(month, year):
if month == 2:
if is_leap_year(year):
return 29
return 28
elif month in [4, 6, 9, 11]:
return 30
else:
return 31
“`
Handling Invalid Input:
When working with user input, it’s important to handle invalid input gracefully. In our case, we need to ensure that the month entered is within range (1-12). Here’s an example of how to handle invalid input using a try-except block:
“`python
try:
month = int(input(“Enter the month (1-12): “))
if month < 1 or month > 12:
raise ValueError
year = int(input(“Enter the year: “))
days_in_month = get_days_in_month(month, year)
print(f”The number of days in month {month} of year {year} is {days_in_month}.”)
except ValueError:
print(“Invalid input. Please enter a valid month and year.”)
“`
Using the datetime Module to Get the Number of Days:
Python’s datetime module provides a convenient way to determine the number of days in a month. Here’s an example:
“`python
import datetime
month = int(input(“Enter the month (1-12): “))
year = int(input(“Enter the year: “))
days_in_month = (datetime.date(year, month % 12 + 1, 1) – datetime.date(year, month, 1)).days
print(f”The number of days in month {month} of year {year} is {days_in_month}.”)
“`
Alternate Method: Creating a Dictionary to Map Month Names and Days:
Another approach to obtaining the number of days in a month is by creating a dictionary that maps month names to their respective lengths. Here’s an example:
“`python
month_lengths = {
“January”: 31,
“February”: 28,
“March”: 31,
“April”: 30,
“May”: 31,
“June”: 30,
“July”: 31,
“August”: 31,
“September”: 30,
“October”: 31,
“November”: 30,
“December”: 31
}
month = input(“Enter the month: “)
if month in month_lengths:
days_in_month = month_lengths[month]
print(f”The number of days in {month} is {days_in_month}.”)
else:
print(“Invalid month name.”)
“`
FAQs:
Q: How can I get the current month and year in Python?
A: You can use the datetime module to obtain the current month and year. Here’s an example:
“`python
import datetime
current_month = datetime.datetime.now().month
current_year = datetime.datetime.now().year
“`
Q: How can I get the last day of a month in Python?
A: Here’s an example using the calendar module:
“`python
import calendar
month = int(input(“Enter the month (1-12): “))
year = int(input(“Enter the year: “))
_, last_day = calendar.monthrange(year, month)
print(f”The last day of month {month} of year {year} is {last_day}.”)
“`
Q: How can I get the first day of a month in Python?
A: Here’s an example using the datetime module:
“`python
import datetime
month = int(input(“Enter the month (1-12): “))
year = int(input(“Enter the year: “))
first_day = datetime.date(year, month, 1).strftime(“%A”)
print(f”The first day of month {month} of year {year} is {first_day}.”)
“`
Q: How can I calculate the number of days between two dates in Python?
A: You can subtract the two dates using the datetime module. Here’s an example:
“`python
import datetime
start_date = datetime.date(2021, 1, 1)
end_date = datetime.date(2021, 12, 31)
days_between = (end_date – start_date).days
print(f”The number of days between {start_date} and {end_date} is {days_between}.”)
“`
In conclusion, Python provides various methods to obtain the number of days in a month. From using the datetime module to creating a dictionary, you can choose the approach that suits your needs. Remember to handle invalid input to ensure your program runs smoothly. Now you have the knowledge to easily calculate the number of days in any given month using Python.
Keywords: Get number of days in month Python, Get day in month python, Get all day in month Python, Write a program to display the number of days in a month given the month and the year Python, Get month from date python, Get last day of month python, Get first day of month Python, How to calculate number of days between two dates in Pythonpython get number of days in month.
Python Programs #2: Find Number Of Days Of A Specific Month Of Any Year
How To Calculate Number Of Working Days In A Month In Python?
In many situations, it is necessary to calculate the number of working days in a particular month. Whether you are dealing with scheduling, project management, or any other time-sensitive task, having a reliable method to determine the number of working days can be extremely useful. Fortunately, Python provides a variety of tools and libraries that can help us achieve this goal. In this article, we will explore different approaches to calculate the number of working days in a month using Python.
Method 1: Using the datetime and calendar modules
Python’s datetime module provides a powerful toolset for working with dates and times. By combining it with the calendar module, we can easily obtain the number of working days in a month.
First, we import the necessary modules:
“`python
import datetime
import calendar
“`
Next, we define a function that takes a year and a month as input and calculates the number of working days:
“`python
def get_working_days(year, month):
num_days = calendar.monthrange(year, month)[1]
working_days = 0
for day in range(1, num_days + 1):
date = datetime.date(year, month, day)
if date.weekday() < 5: # Monday to Friday (0 to 4)
working_days += 1
return working_days
```
To test our function, we can call it with a specific year and month:
```python
year = 2023
month = 5
print(get_working_days(year, month))
```
This will output the number of working days in May 2023.
Method 2: Using the pandas library
Another approach to calculate the number of working days in a month is by using the pandas library. Although pandas is primarily known for its data analysis capabilities, it offers convenient functions for handling dates as well.
To use pandas, we first need to install the library by executing the following command:
```python
pip install pandas
```
Once installed, we can import the library and utilize its date_range function to generate a range of dates for a specific month:
```python
import pandas as pd
def get_working_days(year, month):
start_date = datetime.date(year, month, 1)
end_date = datetime.date(year, month, calendar.monthrange(year, month)[1])
all_dates = pd.date_range(start_date, end_date)
working_days = all_dates[all_dates.weekday < 5] # Filter weekdays
return len(working_days)
```
The resulting DataFrame contains only the weekdays, allowing us to easily calculate the number of working days by taking its length.
Frequently Asked Questions (FAQs)
Q1: How are weekends identified as non-working days?
A1: In both methods presented above, weekends are assumed to be Saturday and Sunday. This is the default behavior in Python's datetime module and the pandas library. If your organization follows a different working week, you can modify the code to account for the specific non-working days.
Q2: Can we consider certain holidays as non-working days?
A2: Yes, it is possible to include holidays as non-working days. However, you would need to have a list of holidays and modify the code accordingly. For example, you could create a list of holidays and check if a date falls within it, subtracting such days from the total working days.
Q3: Is it necessary to install the pandas library?
A3: No, it is not strictly necessary to use the pandas library if the datetime and calendar modules suffice for your specific requirements. However, pandas offers additional functionalities and may be more convenient for handling more complex date-related calculations.
Q4: Can these methods be used for calculating the number of working days in multiple months or over a longer time span?
A4: Yes, both methods can be easily adapted to calculate the number of working days in multiple months or over longer time spans. You could create a loop or call the function repeatedly for each month or range of dates, as required.
In conclusion, calculating the number of working days in a month using Python involves utilizing either the datetime and calendar modules or the pandas library. Both methods provide straightforward and efficient approaches to obtain the desired result. By understanding and implementing the code presented in this article, you will have a valuable tool at your disposal whenever the need arises to calculate the number of working days.
How Were The Number Of Days In Each Month Determined?
Have you ever wondered why some months have 30 days while others have 31? Perhaps you’ve questioned why February is the shortest month of the year, with only 28 days most of the time. The determination of the number of days in each month is a fascinating historical journey that involves various cultural, religious, and astronomical factors. In this article, we will explore the origins and reasoning behind the length of each month, shining a light on this intriguing aspect of our calendar system.
The earliest calendar systems were based on lunar cycles, as people observed the moon’s phases to measure time. In these lunar calendars, each month began with the appearance of a new moon and lasted until the next new moon. As a result, months varied in duration, typically ranging from 29 to 30 days. This lunar calendar approach was preferred by ancient civilizations such as the Egyptians and the Greeks.
Later on, the Romans, who heavily influenced our modern calendar, introduced the solar calendar, which was based on the Earth’s orbit around the sun. This calendar system, known as the Julian calendar, had months with lengths primarily determined by alternating between 30 and 31 days. The month of February, however, had a more complex origin.
Initially, February only had 29 days in the Roman calendar. It was the last month of the year and was considered an unfortunate time associated with religious purification rituals. Legend has it that the Roman king Numa Pompilius added an extra month to the calendar to equalize the year’s length, thus creating the 355-day Roman calendar year. However, over time, it was found that this calendar failed to align accurately with the solar year.
To resolve this discrepancy, Julius Caesar introduced calendar reforms in 45 BCE known as the Julian calendar, named after him. These reforms redistributed the days across the months, but February remained shorter to honor the original length of the month. It was assigned 28 days, while the additional day was intercalated, or added, every four years as an extra day at the end of the month, creating what we now know as a leap year.
While the Julian calendar was an improvement over previous systems, it still didn’t perfectly match the solar year. The length of the solar year is approximately 365.2425 days, and the Julian calendar’s approximation was slightly longer, causing a gradual shift in seasonal alignment. This issue was finally addressed in 1582 CE when Pope Gregory XIII introduced the Gregorian calendar as a reform of the Julian calendar.
The Gregorian calendar retained most aspects of its predecessor but introduced additional rules regarding leap years to account for the discrepancy between the solar year and the Julian calendar. The new rule stated that a century year (divisible by 100) would be a leap year only if it was divisible by 400. This rule eliminated some leap years and helped bring the average length of the year closer to 365.2425 days.
With the establishment of the Gregorian calendar, the length of each month became more standardized. January, March, May, July, August, October, and December were assigned 31 days, while April, June, September, and November were allocated 30 days. February retained its 28 days but had the addition of a leap day every four years.
Frequently Asked Questions (FAQs):
Q: Why does February have the fewest days?
A: The month of February was initially the last month of the Roman calendar and was established with 29 days. It was lengthened by Julius Caesar to align the calendar with the solar year and was designated as having 28 days, with an intercalated leap day occurring every four years.
Q: Which ancient civilization influenced our current calendar system the most?
A: The Roman civilization heavily influenced our modern calendar system. Julius Caesar’s calendar reforms formed the basis of the Julian calendar, which later underwent modifications, leading to the creation of the Gregorian calendar we presently use.
Q: Are there any exceptions or variations to the number of days in each month?
A: While the Gregorian calendar’s standardization of month lengths is widely followed globally, there are a few exceptions. For example, in non-Gregorian calendars, such as the Hebrew or Islamic calendar, the months have differing lengths based on different cultural, religious, or astronomical considerations.
Q: How often does a leap year occur?
A: A leap year occurs every four years, adding an extra day (February 29th) to the calendar year. This adjustment helps keep the solar year in sync with the calendar year by accounting for the remaining fraction of a day.
In conclusion, the number of days in each month has a rich historical background tied to various calendar systems and cultural practices. From lunar-based calendars to solar reforms, our current calendar evolved to strike a balance between celestial events and the practicality of tracking time. While months may appear arbitrary, they are the result of centuries of scientific and cultural advancement, ensuring our calendar remains synchronized with the changing seasons.
Keywords searched by users: python get number of days in month Get number of days in month Python, Get day in month python, Get all day in month Python, Write a program to display the number of days in a month given the month and the year Python, Get month from date python, Get last day of month python, Get first day of month Python, How to calculate number of days between two dates in Python
Categories: Top 47 Python Get Number Of Days In Month
See more here: nhanvietluanvan.com
Get Number Of Days In Month Python
Python is a versatile programming language that offers a wide range of functionalities. One useful feature is the ability to determine the number of days in a specific month. Whether you are working on a calendar application, scheduling tasks, or simply need to calculate the duration of a month, Python provides various methods to accomplish this task efficiently.
In this article, we will explore different approaches to obtain the number of days in a month using Python. We will cover both built-in functions and third-party libraries, offering different solutions suited to your specific needs. So, let’s dive in!
Method 1: Using the calendar Module
Python’s built-in calendar module provides a simple and intuitive way to retrieve the number of days in a month. We can utilize the `monthrange()` method of this module to achieve our goal.
Here’s an example:
“`python
import calendar
year = 2022
month = 12
days_in_month = calendar.monthrange(year, month)[1]
print(f”The number of days in month {month} of year {year} is {days_in_month}.”)
“`
In this code snippet, we import the `calendar` module, specify the desired year and month, and then use the `monthrange()` method to retrieve a tuple containing the first weekday of the month and the number of days in the month. We extract the number of days by accessing the second element of the tuple, denoted by `[1]`. Finally, we display the result using a formatted string.
Method 2: Using the datetime Module
Another built-in module, `datetime`, can be used to determine the number of days in a month. Although this method is slightly more comprehensive, it provides a more generalized approach.
Let’s have a look at the following code example:
“`python
import datetime
year = 2022
month = 12
first_day = datetime.date(year, month, 1)
next_month = first_day.replace(day=28) + datetime.timedelta(days=4)
last_day = next_month – datetime.timedelta(days=next_month.day)
days_in_month = last_day.day
print(f”The number of days in month {month} of year {year} is {days_in_month}.”)
“`
In this code, we use the `datetime` module to create a date object representing the first day of the desired month. Next, we replace the day with “28” and add 4 days to ensure we move into the following month.
By subtracting one day from the start of the next month, we obtain the last day of the original month. Finally, we extract the day value from the obtained date, which gives us the number of days in the month.
Method 3: Using the NumPy Library
If you are already using the NumPy library in your project, you can take advantage of its `numpy.busday_count()` function. Although primarily designed to count business days, it can be effectively used to calculate the number of days in any given month.
Here’s an example:
“`python
import numpy as np
year = 2022
month = 12
first_day = np.datetime64(f'{year}-{month:02}-01′, ‘D’)
last_day = first_day + np.timedelta64(1, ‘M’) – np.timedelta64(1, ‘D’)
days_in_month = np.busday_count(first_day, last_day)
print(f”The number of days in month {month} of year {year} is {days_in_month}.”)
“`
In this code snippet, we utilize NumPy’s date manipulation capabilities to generate the first day of the desired month. By adding one month and subtracting one day, we obtain the last day of the month.
The `numpy.busday_count()` function takes the start and end date as arguments and returns the number of valid days (not including weekends) between them. Since we want to count all days, we pass the obtained dates to this function to get the total number of days in the month.
FAQs:
Q1: Can these methods handle leap years?
Yes, all three methods presented in this article consider leap years and provide accurate results. The `calendar` and `datetime` modules have built-in mechanisms to handle leap years. The `numpy` library, on the other hand, takes leap years into account automatically when calculating the number of days.
Q2: Are these methods dependent on the system’s date settings?
No, these methods are independent of the system’s date settings. They provide consistent and reliable results, regardless of the date format or settings used by the underlying system.
Q3: Which method should I choose?
The method to choose depends on your specific requirements and the modules or libraries you are already utilizing in your project. If you only need to determine the number of days in a month and do not require any additional functionalities, the `calendar` module provides a simple and reliable solution. On the other hand, if you are already using the `datetime` module or the `numpy` library, their respective approaches are also viable options.
In conclusion, obtaining the number of days in a month using Python is a straightforward task with multiple available methods. By leveraging the built-in `calendar` and `datetime` modules or opting for the `numpy` library, developers can easily retrieve this information for various applications.
Get Day In Month Python
Python is a versatile programming language known for its simplicity and readability. One of the common tasks you may come across while working with dates or time is to determine the day in the month. Fortunately, Python offers several built-in functions and modules that can help you effortlessly accomplish this task. In this article, we will explore various methods to get the day in the month using Python.
Method 1: Using the datetime Module
The datetime module in Python provides classes for manipulating dates and times. To get the day in the month, we can make use of the datetime.date class. Here’s an example code snippet:
“`python
import datetime
current_date = datetime.date.today()
day_in_month = current_date.day
print(day_in_month)
“`
In this code, we import the datetime module and use the date.today() method to get the current date. Then, we access the day attribute of the current date object to obtain the day in the month.
Method 2: Using the calendar Module
Python’s calendar module offers a range of functionalities related to dates and calendars. To get the day in the month using this module, we can make use of the monthrange() function. Here’s an example:
“`python
import calendar
current_date = datetime.date.today()
_, day_in_month = calendar.monthrange(current_date.year, current_date.month)
print(day_in_month)
“`
In this code, we import the calendar module and call the monthrange() function, passing the current year and month as arguments. The function returns a tuple containing the weekday of the first day of the month and the number of days in the month. We then discard the first value (weekday) by using an underscore (_) and store the second value (day_in_month) in a variable.
Frequently Asked Questions (FAQs):
Q1: When should I use Method 1 over Method 2, and vice versa?
A1: Method 1 is useful when you only need the day in the month and do not require additional functionalities offered by the calendar module. However, if you wish to perform more advanced operations, such as determining the weekday of the first day or finding leap years, Method 2 with the calendar module would be more appropriate.
Q2: Can I specify a different date instead of the current one?
A2: Yes, you can replace `datetime.date.today()` with a specific date. For example, you can use `datetime.date(2022, 12, 31)` to get the day in the month for December 31, 2022.
Q3: How can I format the output to display the day name instead of the day number?
A3: You can utilize the strftime() method available in the datetime class. For example, `current_date.strftime(‘%A’)` would return the full weekday name, such as ‘Monday’, instead of just the day number.
Q4: Is there any other module that can be used to get the day in the month?
A4: Besides the datetime and calendar modules, there is also the dateutil module, which provides additional functionalities for working with dates and times. However, for obtaining the day in the month specifically, the datetime and calendar modules are generally suitable and widely used.
In conclusion, Python offers various methods to easily obtain the day in the month. Method 1 using the datetime module is straightforward and sufficient for basic requirements. However, if you need more advanced functionalities or operations related to calendars, Method 2 with the calendar module can be utilized. By leveraging these built-in modules, you can efficiently handle date-related tasks in your Python projects.
Get All Day In Month Python
Introduction:
When working with dates and time in Python, it is often necessary to retrieve all the days in a specific month. This could be useful in various applications, such as generating monthly reports, analyzing historical data, or performing date calculations. In this article, we will explore different approaches to obtain all the days in a month using Python. We will discuss both built-in modules and external libraries, providing step-by-step instructions along with code examples. Additionally, we will address common questions in the FAQs section, offering solutions to potential challenges that may arise during the process.
Built-in Modules:
Python offers several built-in modules that provide functionalities for working with dates and times. The most commonly used modules for obtaining all days in a month are `datetime` and `calendar`. Let’s explore these modules one by one.
1. The `datetime` Module:
The `datetime` module is part of the Python standard library and provides classes for manipulating dates and times. To retrieve all days in a specific month, we can utilize the `datetime` module in combination with a loop. Here’s an example:
“`python
import datetime
def get_all_days_in_month(year, month):
days_in_month = []
for day in range(1, 32):
try:
date_obj = datetime.datetime(year, month, day)
days_in_month.append(date_obj)
except ValueError:
break
return days_in_month
“`
In this example, the `get_all_days_in_month` function takes `year` and `month` as input parameters. It initializes an empty list `days_in_month` to store the date objects. Using a loop, it iterates over the possible days in the month (ranging from 1 to 31). Inside the loop, it attempts to create a `datetime` object for each day. If a `ValueError` occurs, it means that the day is beyond the valid range for that month, and the loop breaks. Finally, the function returns the list of all date objects.
2. The `calendar` Module:
The `calendar` module provides various classes and functions for working with calendars. To retrieve all days in a specific month using this module, we can utilize the `monthcalendar` function. Here’s an example:
“`python
import calendar
def get_all_days_in_month(year, month):
days_in_month = []
for week in calendar.monthcalendar(year, month):
for day in week:
if day != 0:
days_in_month.append(day)
return days_in_month
“`
In this example, the `get_all_days_in_month` function takes `year` and `month` as input parameters. It initializes an empty list `days_in_month` to store the day numbers. The `monthcalendar` function is invoked, which provides a matrix representation of the month’s calendar. We iterate over each week in the matrix and then iterate over each day in that week. If the day is nonzero, indicating a valid day in the month, it is appended to the list. Finally, the function returns the list of all days.
External Libraries:
Although the built-in modules in Python are powerful, external libraries offer additional flexibility and advanced functionalities, making them suitable for more complex scenarios. Two widely used libraries for date and time manipulation are `dateutil` and `Pandas`. Let’s explore their usage for retrieving all days in a month.
1. The `dateutil` Library:
`dateutil` is a third-party library that provides various extensions to the standard Python `datetime` module. One of its features is the `rrule` class, which stands for “recurrence rule.” Using the `rrule` class, we can easily generate a list of all the days in a month without worrying about the varying length of each month. Here’s an example:
“`python
from dateutil.rrule import rrule, DAILY
from datetime import datetime
def get_all_days_in_month(year, month):
start_date = datetime(year, month, 1)
end_date = datetime(year, month + 1, 1) – datetime.timedelta(days=1)
days_in_month = list(rrule(DAILY, dtstart=start_date, until=end_date))
return days_in_month
“`
In this example, we import the `rrule` class from the `dateutil` library and the `datetime` module. The `get_all_days_in_month` function takes `year` and ` month` as input parameters. It creates a `start_date` object with the first day of the specified month and a `end_date` object with the last day of the month. By subtracting one day from the beginning of the next month, we ensure that only the days within the desired month are included. Finally, the `rrule` function is used to generate a list of all the days, which is returned as the output.
2. The `Pandas` Library:
`Pandas` is a powerful library widely used for data manipulation and analysis. It provides a rich set of tools for handling time series data. To obtain all the days in a month using `Pandas`, we can create a `DatetimeIndex` object and extract the days from it. Here’s an example:
“`python
import pandas as pd
def get_all_days_in_month(year, month):
days_in_month = pd.date_range(start=f”{year}-{month}-1″, periods=31, freq=’D’).day
return days_in_month
“`
In this example, we import the `pandas` library as `pd`. The `get_all_days_in_month` function takes `year` and ` month` as input parameters. Using the `pd.date_range` function, we create a range of dates starting from the first day of the specified month (`YYYY-MM-1`) to a period of 31 days with a daily frequency (`freq=’D’`). Finally, we extract the day component from each date and return the list of days.
FAQs:
Q1. What happens if I provide an invalid month or year as input to the mentioned functions?
All the functions mentioned in this article have basic error handling mechanisms to handle invalid input. For example, if you provide a month greater than 12 or a negative year, an appropriate ValueError or IndexError will be raised. It’s always recommended to perform input validation before using any of these functions.
Q2. How can I get the days in a month sorted in descending order?
To retrieve the days in a month in descending order, you can simply reverse the resulting list of days. For example, if you are using the `calendar` module, you can modify the return statement as follows: `return sorted(days_in_month, reverse=True)`.
Q3. Can I retrieve the days in a month in a format other than a list?
Absolutely! The examples presented here return the days as a list, but you can easily modify the functions to return them in a different format. For instance, you can return a comma-separated string of days, a set, or any other custom data structure.
Q4. How can I exclude weekends or specific weekdays from the list of days in a month?
To exclude weekends or specific weekdays, you can add an additional check inside the loop or use list comprehension to filter out the undesired days. For example, to exclude weekends, you can modify the `get_all_days_in_month` function using the `datetime` module as follows:
“`python
import datetime
def get_all_days_in_month(year, month):
days_in_month = []
for day in range(1, 32):
try:
date_obj = datetime.datetime(year, month, day)
if date_obj.weekday() < 5: # Excluding days with weekday greater than or equal to 5 (Saturday and Sunday)
days_in_month.append(date_obj)
except ValueError:
break
return days_in_month
```
Conclusion:
Retrieving all the days in a specific month is a common requirement in various Python applications. In this comprehensive guide, we explored different approaches using both built-in modules (`datetime` and `calendar`) and external libraries (`dateutil` and `Pandas`). We provided step-by-step instructions and code examples for each approach, enabling users to choose the one that best suits their needs. Additionally, we addressed common questions in the FAQs section, offering solutions to potential challenges that may arise during the process. With the knowledge gained from this article, readers will be able to confidently retrieve all days in a month using Python and expand their capabilities in-date manipulation and analysis.
Images related to the topic python get number of days in month
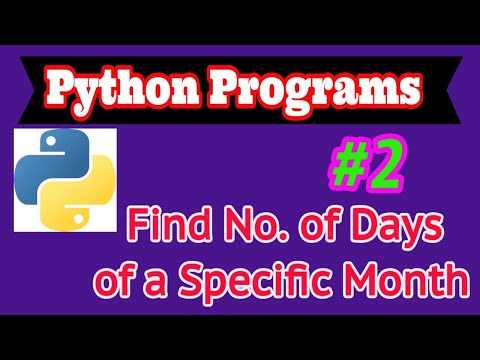
Found 6 images related to python get number of days in month theme
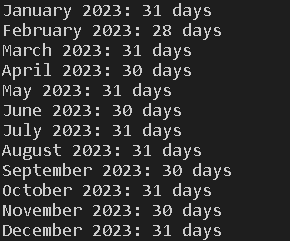
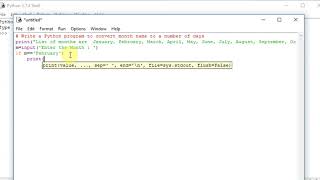

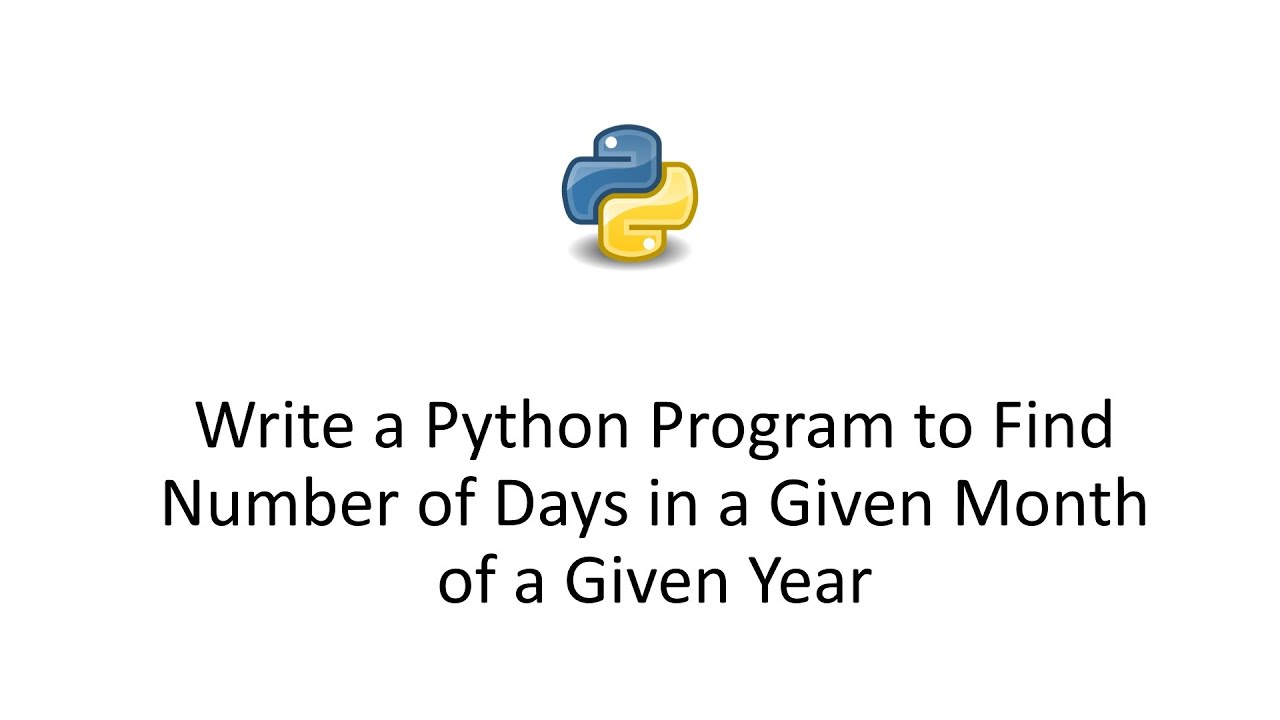
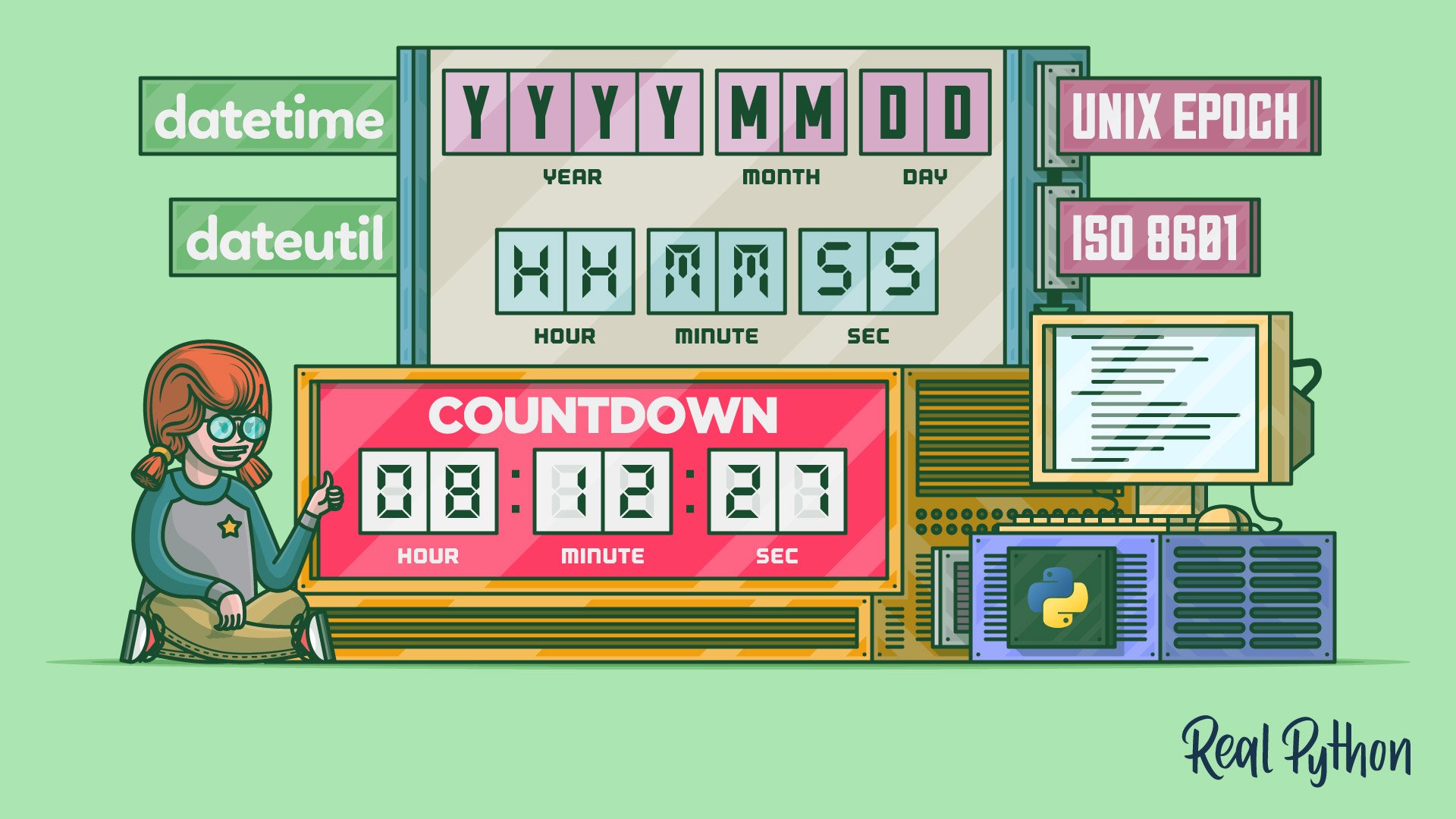
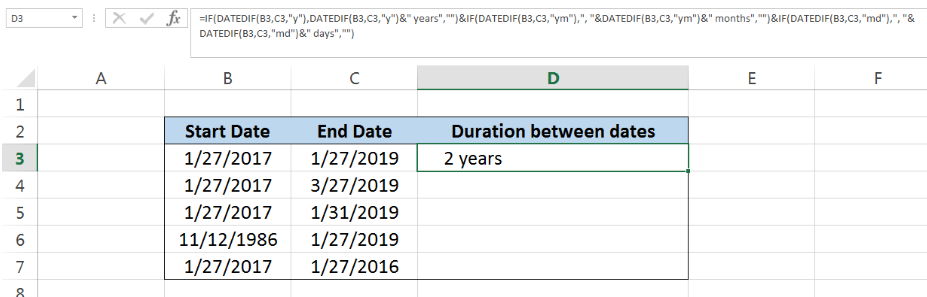
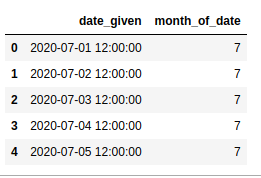

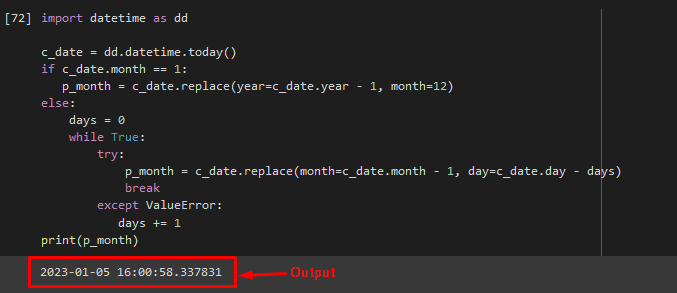
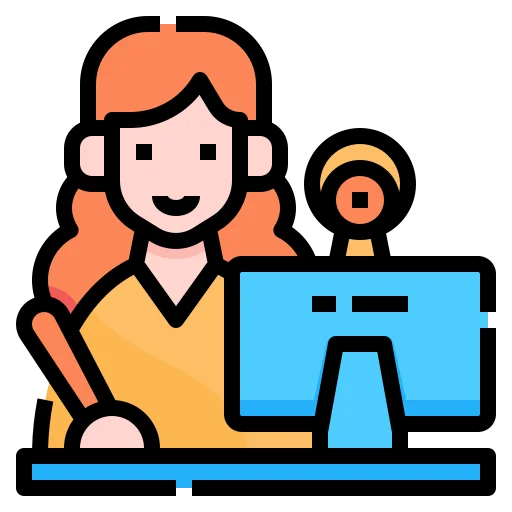
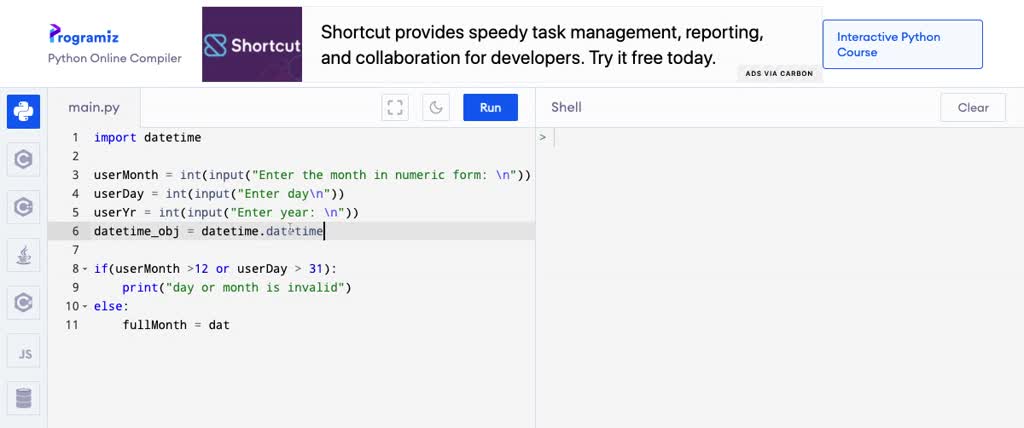

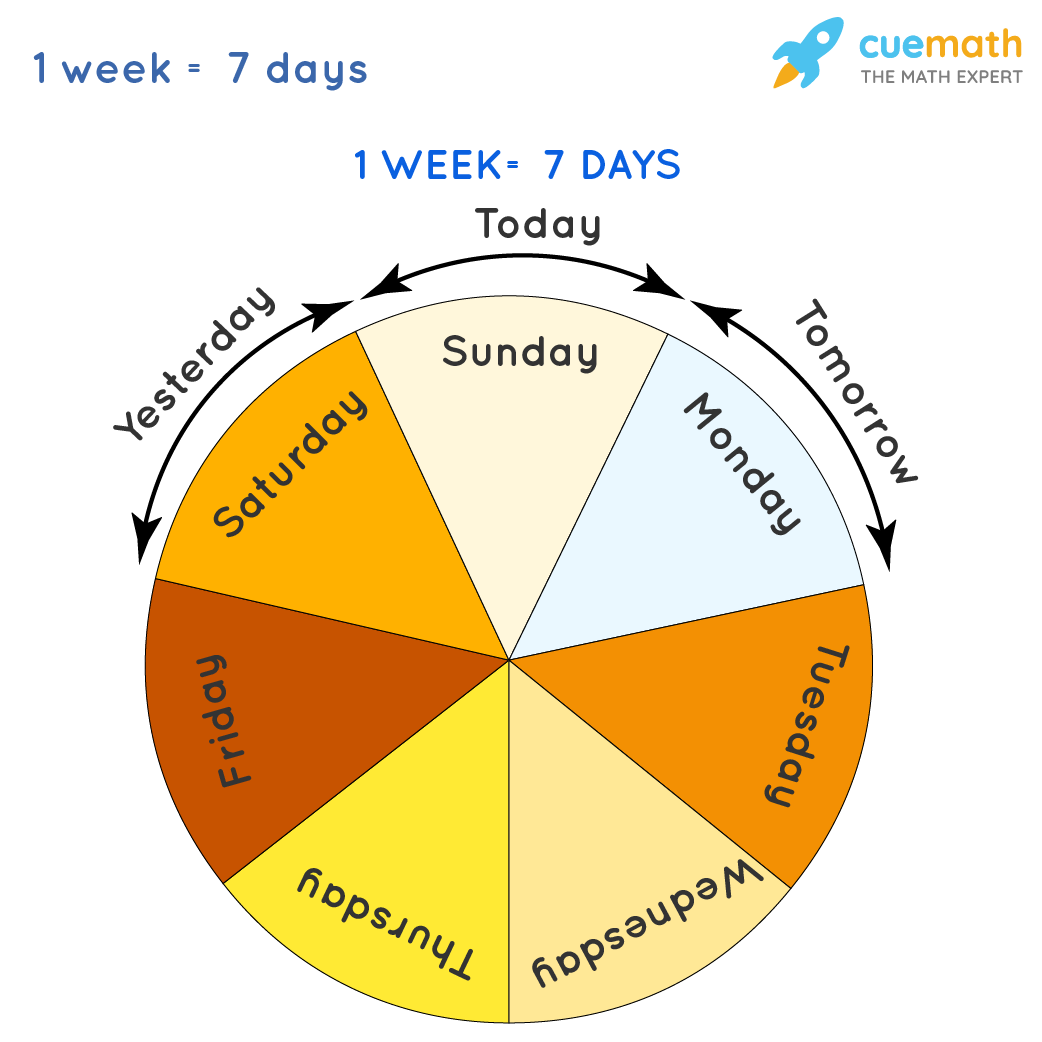
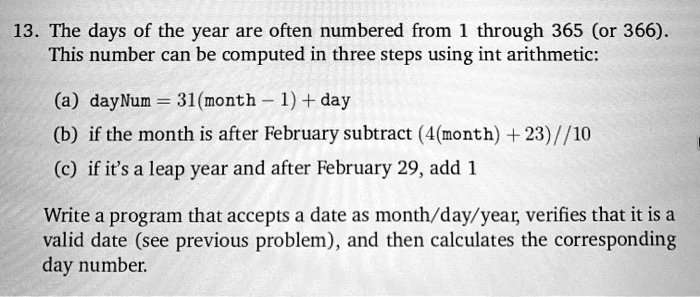
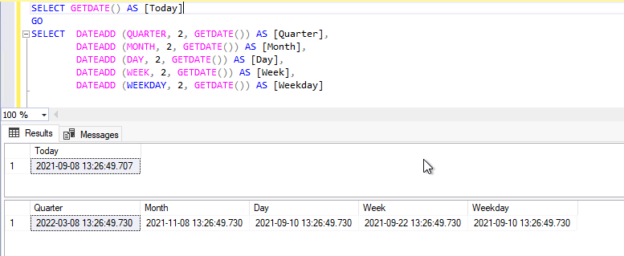
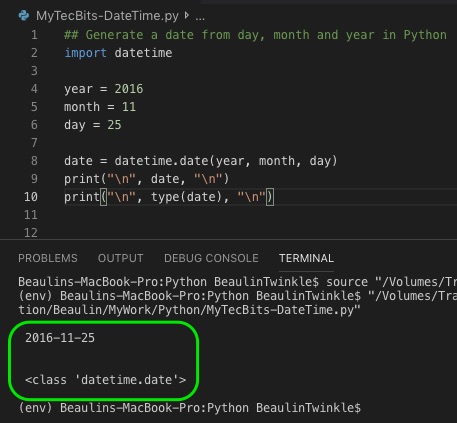


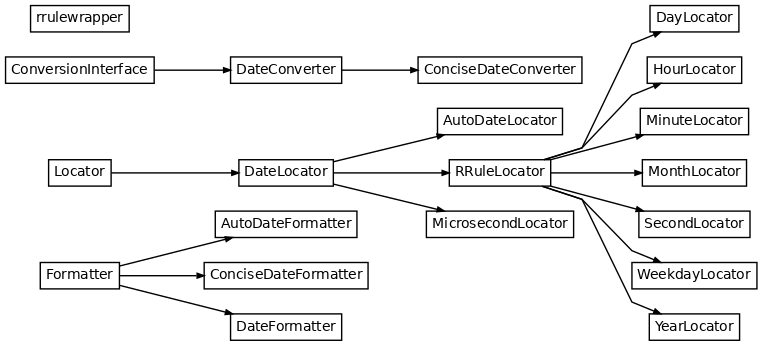

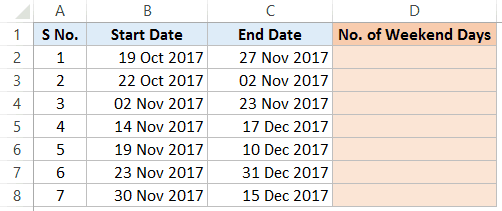
![Python Get Last Day of Month [4 Ways] – PYnative Python Get Last Day Of Month [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2019/01/vishalHule.jpg)
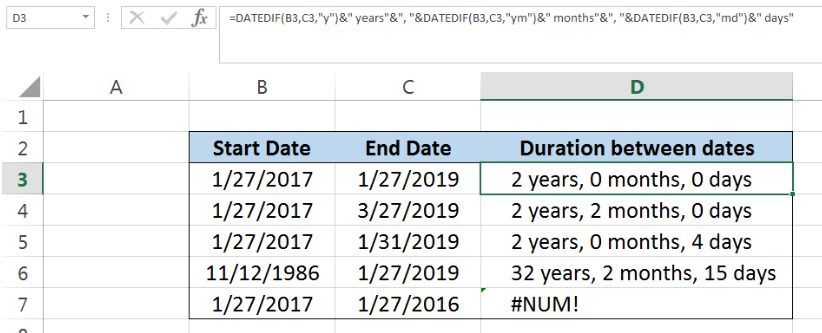

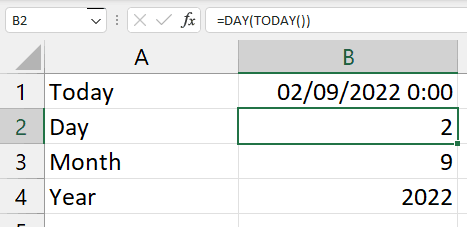
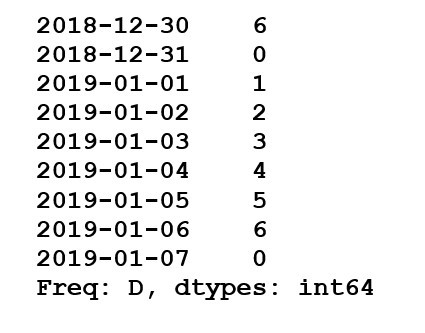
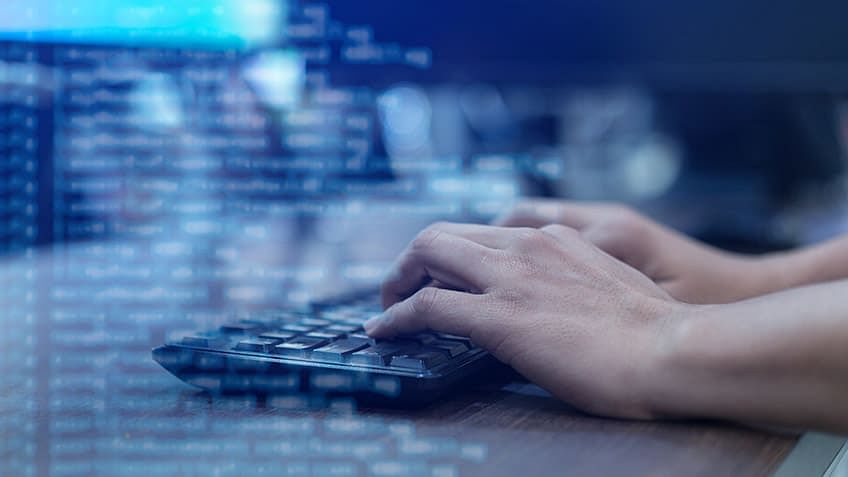
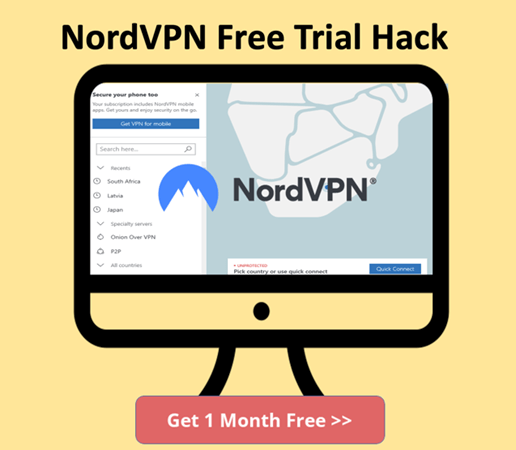
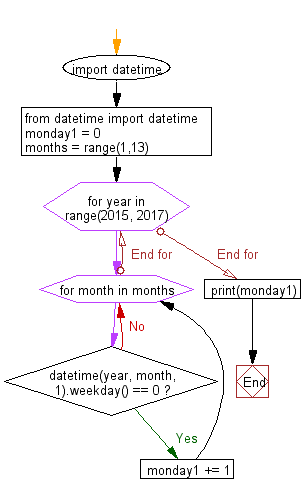
Article link: python get number of days in month.
Learn more about the topic python get number of days in month.
- Find the number of days and weeks of a month in Python
- How do we determine the number of days for a given month in …
- How to get number of days in month in Python – TechOverflow
- Determine the Number of Days in a Month with Python
- Python Get Business Days [4 Ways] – PYnative
- Month – Wikipedia
- 12 Months of the Year – Time and Date
- Display all the dates for a particular month using NumPy – GeeksforGeeks
- Number of days in a given month of a year in python – PrepInsta
- How to return the number of days in a month in pandas
- How do I get the number of days in a month in Python?
- Python: Get the number of days of a given month and year
See more: nhanvietluanvan.com/luat-hoc