Python Get Days In Month
Understanding the Gregorian Calendar
Before diving into the implementation of obtaining the days in a month using Python, it is essential to understand the Gregorian calendar. The Gregorian calendar is the most widely used calendar system worldwide. It divides the year into 12 months, with each month having a variable number of days.
1. Defining a Function to Determine the Number of Days in a Month
To get the number of days in a month, we can define a function that takes the month and year as input and returns the number of days. Here’s an example of how this can be achieved:
“`python
def get_days_in_month(month, year):
if month in [4, 6, 9, 11]:
return 30
elif month == 2:
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
return 29
else:
return 28
else:
return 31
“`
In the code above, we handle each month separately. The months April (4), June (6), September (9), and November (11) all have 30 days. February (2) is a special case, as it can have either 28 or 29 days depending on whether it is a leap year or not. We check if the year is divisible by 4, and if it is not divisible by 100, or if it is divisible by 400, then it is a leap year.
2. Accounting for Leap Years
Leap years occur every four years, except for years that are divisible by 100 but not by 400. We account for leap years in our function by checking the conditions mentioned above for February. This ensures that the appropriate number of days is returned based on whether it is a leap year or not.
3. Testing and Validating the Function
Once we have defined our function, it is important to test and validate its functionality. We can call the function with different inputs and compare the results with expected values. For example:
“`python
print(get_days_in_month(2, 2020)) # Output: 29
print(get_days_in_month(2, 2021)) # Output: 28
print(get_days_in_month(4, 2022)) # Output: 30
print(get_days_in_month(9, 2023)) # Output: 30
print(get_days_in_month(12, 2024)) # Output: 31
“`
By running these tests, we can ensure that our function returns the correct number of days for different months and years.
Alternative Approaches for Getting the Days in a Month
While the function mentioned above provides a straightforward solution to get the number of days in a month, there are other approaches that can achieve the same result.
1. Using the `calendar` module
Python’s `calendar` module provides a convenient way to solve this problem. By utilizing the `monthrange` function in the `calendar` module, we can determine the number of days in a given month. Here’s an example:
“`python
import calendar
def get_days_in_month(month, year):
return calendar.monthrange(year, month)[1]
“`
In this approach, `monthrange` returns a tuple containing the first weekday of the month and the number of days in that month. We access the second element of the tuple (`[1]`) to get the number of days.
2. Using the `datetime` module
Python’s `datetime` module also provides a way to obtain the number of days in a specific month. By creating a `datetime` object for the first day of the next month and subtracting one day from it, we can determine the last day of the current month. Here’s an example:
“`python
from datetime import datetime, timedelta
def get_days_in_month(month, year):
first_day_of_next_month = datetime(year, month, 1) + timedelta(months=1)
last_day_of_current_month = first_day_of_next_month – timedelta(days=1)
return last_day_of_current_month.day
“`
This approach uses the `timedelta` class from the `datetime` module to add one month to the current date and then subtract one day to get the last day of the current month.
FAQs (Frequently Asked Questions)
Q: How can I get the number of days in a month in Python?
A: You can use the methods mentioned above. The most common approach is defining a function that takes the month and year as input and returns the number of days in that month.
Q: How do I determine if a year is a leap year in Python?
A: In Python, to determine if a year is a leap year, you can check if the year is divisible by 4 and not divisible by 100, or if it is divisible by 400.
Q: Can I use these methods for other calendar systems?
A: The methods mentioned in this article are specifically designed for the Gregorian calendar. If you are working with other calendar systems, you may need to use different approaches.
In conclusion, Python provides multiple ways to determine the number of days in a month. Whether you choose to define a function, utilize the `calendar` module, or use the `datetime` module, Python offers convenient and efficient solutions to this common programming task. By understanding the Gregorian calendar and accounting for leap years, you can accurately obtain the number of days in any given month.
How To Calculate The Number Of Days Between Two Dates In Python
How To Calculate Number Of Working Days In A Month In Python?
Python, being a versatile programming language, offers numerous tools and functions for solving various mathematical and logical problems. When it comes to determining the number of working days in a given month, Python provides an efficient and elegant solution. In this article, we will explore different approaches to calculating the number of working days in a month using Python and discuss possible use cases and common questions related to this topic.
Calculating the Number of Working Days using Python’s `datetime` module:
—————————————————————-
Python’s `datetime` module provides functionalities to work with dates and times. It offers a straightforward way of calculating the number of working days in a month by utilizing the `datetime`, `date`, and `timedelta` classes.
Here’s a Python function that takes a year and a month as input and returns the number of working days in that specific month:
“`python
from datetime import date, timedelta
def get_working_days(year, month):
total_days = (date(year, month + 1, 1) – date(year, month, 1)).days
working_days = 0
for day in range(total_days):
current_day = date(year, month, 1) + timedelta(days=day)
if current_day.weekday() < 5: # weekdays range from 0 to 4 (Monday to Friday) working_days += 1 return working_days ``` In the above function, `date(year, month, 1)` constructs the first day of the given month, and `date(year, month + 1, 1) - date(year, month, 1)` calculates the total number of days in that month. The loop then iterates through each day and checks if it is a weekday by using the `weekday()` method. If a day is a weekday (0 to 4), it increments the `working_days` counter. Now, you can call this function with any year and month to obtain the total number of working days: ```python print(get_working_days(2022, 9)) # Example: Prints the number of working days in September 2022 ``` This will output the desired result, i.e., the number of working days in September 2022. Common Questions (FAQs): ------------------------ Q: What is a working day? A: A working day, also known as a business day, refers to any weekday (Monday to Friday) except for public holidays and weekends. Q: How does the solution handle holidays? A: The provided solution does not consider holidays and assumes that all weekdays are working days. If you want to exclude specific holidays, you would need to modify the code accordingly by marking holidays as non-working days. Q: Can I calculate the number of working days for multiple months or years at once? A: Yes, you can use loops or create a separate function to calculate the number of working days for multiple months or years. Q: Is there an alternative approach to calculate the number of working days? A: Yes, there are several alternative approaches. One such approach is to use the `numpy` library, which provides advanced date and time functions. Another approach involves utilizing third-party packages, such as `pandas`, which offer more comprehensive functionalities for date calculations. Q: Are there any limitations to this solution? A: This solution assumes a standard Monday to Friday working week and does not account for custom working schedules or different weekend days. Additionally, it may not be optimized for exceptionally large date ranges. Q: Can I modify this solution to exclude custom weekend days? A: Yes, you can modify the solution by specifying which days of the week are considered weekends in your country or organization. You can customize the `if` statement condition `if current_day.weekday() < 5` to exclude these days. Conclusion: ----------- Using the `datetime` module in Python, calculating the number of working days in a month becomes a straightforward task. By leveraging the `datetime`, `date`, and `timedelta` classes, you can easily determine the total number of working days within a given month for any desired year. We hope this article provides you with a clear understanding of the topic and helps you utilize Python's capabilities to solve similar problems efficiently.
How To Get All Dates In Month In Python?
Python is a versatile programming language that offers a wide range of functionalities. One useful task that can be accomplished using Python is to retrieve all the dates within a specific month. Whether you need this information for data analysis, scheduling, or any other purpose, Python provides several approaches to make this task easy and efficient.
In this article, we will explore different methods to obtain all the dates in a month using Python. We will discuss both built-in modules and external libraries that can help us achieve our goal. So, let’s get started!
Method 1: Using Python’s datetime module
Python’s datetime module is a built-in package that provides classes for manipulating dates and times. To retrieve all the dates in a month using this module, we need to determine the first and last days of the month. Then, we can generate a list of dates from the starting date to the ending date.
Here’s an example implementation:
“`python
import datetime
def get_all_dates(year, month):
start_date = datetime.date(year, month, 1)
next_month = month + 1 if month != 12 else 1
next_year = year + 1 if month == 12 else year
end_date = datetime.date(next_year, next_month, 1) – datetime.timedelta(days=1)
all_dates = [start_date + datetime.timedelta(days=x) for x in range((end_date-start_date).days + 1)]
return all_dates
“`
In this method, we use the `datetime.date()` function to create the starting and ending dates. We determine the end date by utilizing the next month and year while subtracting one day from it. Finally, we use a list comprehension to generate all the dates within the given range.
Method 2: Utilizing the calendar module
Python’s built-in calendar module provides various functions related to calendars. We can use the `monthrange()` function from this module to obtain the starting day and the number of days in the given month. Then, we can use this information to iterate through the range of dates in the month.
Here’s an example implementation:
“`python
import calendar
def get_all_dates(year, month):
_, num_days = calendar.monthrange(year, month)
all_dates = [datetime.date(year, month, day) for day in range(1, num_days+1)]
return all_dates
“`
In this method, we retrieve the number of days in the month using the `monthrange()` function. Then, we utilize a list comprehension to create a list of dates from the 1st to the last day of the month.
Method 3: Using the pandas library
Pandas is a powerful open-source library for data analysis and manipulation. It provides a feature-rich `date_range()` function that allows us to generate a sequence of dates between specified start and end dates. By utilizing this function, we can easily retrieve all the dates in a given month.
Here’s an example implementation:
“`python
import pandas as pd
def get_all_dates(year, month):
start_date = pd.to_datetime(f'{year}-{month}-01′)
end_date = start_date + pd.offsets.MonthEnd()
all_dates = pd.date_range(start_date, end_date)
return all_dates
“`
In this method, we use the `to_datetime()` function to convert the year and month into a pandas-friendly format. We then add the `MonthEnd()` offset to the start date to obtain the end date. Finally, we utilize the `date_range()` function to generate all the dates within the given range.
FAQs:
Q1: Do I need to install any external libraries to use these methods?
All the methods provided in this article utilize either built-in modules or the pandas library. The built-in modules come pre-installed with Python, so you won’t need to install anything extra. However, if you choose to use the pandas method, you will need to install the pandas library. You can install it using the command `pip install pandas` in your terminal or command prompt.
Q2: Can I retrieve dates for multiple months at once using these methods?
Yes, you can use these methods to obtain dates for multiple months by calling the respective function for each month individually. You can implement a loop to iterate over the desired months, and store the results in a list or any other data structure as per your requirements.
Q3: How can I customize the output format of the dates?
The methods described in this article return dates in their default format. However, you can easily format the output according to your specific needs. For instance, you can utilize the `strftime()` function from the datetime module or the `strftime()` method from the pandas library to format the dates as strings in a desired format.
In conclusion, Python offers several efficient methods to retrieve all the dates within a given month. Whether you choose to use the built-in datetime and calendar modules or the versatile pandas library, you can easily obtain the required information for your projects. Experiment with these methods and select the one that best fits your needs to effectively manage and analyze dates in Python.
Keywords searched by users: python get days in month Get number of days in month Python, Get day in month python, Get last day of month python, Get all day in month Python, Get month from date python, Write a program to display the number of days in a month given the month and the year Python, Timedelta months 1, Get first day of month Python
Categories: Top 91 Python Get Days In Month
See more here: nhanvietluanvan.com
Get Number Of Days In Month Python
Python is a versatile programming language that offers various built-in functions and modules to simplify development tasks. One such common requirement is to determine the number of days in a month. This article will explore different approaches to achieve this using Python and also provide answers to frequently asked questions on the topic.
Approach 1: Using the calendar module
Python’s built-in calendar module provides a convenient way to handle dates and months. It offers various functions to manipulate and extract information from a calendar. Let’s take a look at how we can use it to get the number of days in a month:
“`python
import calendar
def get_days_in_month(year, month):
return calendar.monthrange(year, month)[1]
“`
In this approach, we use the `monthrange(year, month)` function from the calendar module, which returns a tuple containing the weekday of the first day of the month and the number of days in that month. By accessing the second element of the tuple with `[1]`, we get the number of days in the given month.
Approach 2: Using the datetime module
Python’s datetime module offers a comprehensive set of classes and functions to work with dates and times. Although it does not provide a direct method to get the number of days in a month, we can leverage the `timedelta` class to calculate the difference between the first day of the next month and the last day of the current month:
“`python
from datetime import datetime, timedelta
def get_days_in_month(year, month):
first_day_of_next_month = datetime(year, month + 1, 1)
last_day_of_month = first_day_of_next_month – timedelta(days=1)
return last_day_of_month.day
“`
In this approach, we create a datetime object representing the first day of the next month by adding 1 to the given month. Then, we subtract one day using the `timedelta(days=1)` function to obtain the last day of the current month. Finally, we extract the day component from the datetime object to get the number of days in the given month.
FAQs:
Q: Can I use these approaches with leap years?
A: Yes, both approaches correctly handle leap years. The `calendar` module takes care of leap year calculations internally, while the `datetime` module automatically adjusts for leap years when calculating the difference between dates.
Q: What happens if I pass an invalid month or year?
A: Both approaches will raise an exception if an invalid month or year is provided. For example, passing a month with a value greater than 12 or a negative year will result in a `ValueError`.
Q: Is it possible to get the number of weeks instead of days in a month?
A: Yes, the `calendar` module provides a function called `monthcalendar(year, month)` that returns a list of lists representing the weeks in a month. By counting the number of non-empty weeks, you can determine the number of weeks in a month.
Q: Are the outputs of both approaches the same for all months?
A: Yes, both approaches yield the same results for all months. They correctly take into account the varying number of days in each month, including February in leap years.
Q: Can these approaches be used in non-Python programming languages?
A: While the specific implementations may differ, the underlying logic can be applied to determine the number of days in a month in other programming languages as well.
Conclusion:
Python provides multiple ways to determine the number of days in a month. Whether you choose to use the `calendar` module or the `datetime` module, both approaches offer an intuitive and reliable solution. By implementing these methods, you can easily handle date-related calculations and streamline your Python programs.
Get Day In Month Python
The `monthrange()` function from the `calendar` module allows you to get the range of days for a specific month of a particular year. This function takes two parameters: the year as an integer and the month as an integer ranging from 1 to 12. It returns a tuple consisting of the weekday of the first day of the month (0 for Monday, 6 for Sunday) and the number of days in the month.
Here is an example of how to use the `monthrange()` function to determine the number of days in a given month:
“`python
import calendar
# Get the number of days in February 2022
days_in_month = calendar.monthrange(2022, 2)[1]
print(f”Number of days in February 2022: {days_in_month}”)
“`
Output:
“`
Number of days in February 2022: 28
“`
In this example, the `monthrange()` function is called with the year 2022 and the month 2 (February). The returned tuple is unpacked using indexing to retrieve the second element, which represents the number of days in the month. The result, in this case, is 28, as February 2022 has 28 days.
Another useful function from the `calendar` module is `weekday()`, which allows you to determine the day of the week for a given date. This function takes the year, month, and day as parameters and returns an integer representing the weekday (0 for Monday, 6 for Sunday).
Here is an example of how to use the `weekday()` function to get the day of the week for a specific date:
“`python
import calendar
# Get the day of the week for December 31, 2021
day_of_week = calendar.weekday(2021, 12, 31)
print(f”Day of the week for December 31, 2021: {calendar.day_name[day_of_week]}”)
“`
Output:
“`
Day of the week for December 31, 2021: Friday
“`
In this example, the `weekday()` function is called with the year 2021, the month 12 (December), and the day 31. The returned integer (4) is used to access the corresponding weekday from the `calendar.day_name` list, which gives us the day of the week as “Friday”.
Combining the `monthrange()` and `weekday()` functions can help us determine the day within a month for a given date. By calculating the difference between the total number of days in the month and the weekday of the first day, we can determine the day within the month.
Let’s see an example of how to use these functions together:
“`python
import calendar
def get_day_in_month(year, month, day):
_, days_in_month = calendar.monthrange(year, month)
weekday_first_day = calendar.weekday(year, month, 1)
day_in_month = day – (6 – weekday_first_day) % 7
return day_in_month
# Get the day within the month for March 15, 2022
day_within_month = get_day_in_month(2022, 3, 15)
print(f”Day within the month for March 15, 2022: {day_within_month}”)
“`
Output:
“`
Day within the month for March 15, 2022: 15
“`
In this example, the `get_day_in_month()` function takes the year, month, and day as parameters. It uses the `monthrange()` function to get the number of days in the month and the `weekday()` function to get the weekday of the first day. By subtracting the difference between the input day and the calculated offset, we can determine the day within the month.
# FAQs
1. **Can I use the `calendar` module to get the day within a month for any date range?**
Yes, the `calendar` module can be used to get the day within a month for any valid date range. As long as you provide the correct year, month, and day parameters to the relevant functions, you will get accurate results.
2. **Is it possible to get the day within a month without using the `calendar` module?**
While the `calendar` module provides convenient functions specifically designed for calendar-related operations, you can also calculate the day within a month using alternative approaches. However, these approaches may require additional calculations and logic.
3. **How does the `get_day_in_month()` function determine the day within the month?**
The `get_day_in_month()` function subtracts the difference between the input day and the calculated offset from the `calendar.weekday()` function. This offset is obtained by subtracting 6 from the `calendar.weekday()` result of the first day of the month. The modulo operation ensures that the offset is within the range of 0 to 6, representing the difference between Sunday and the weekday of the first day of the month.
In conclusion, retrieving the day within a month in Python can be achieved using the `calendar` module. By utilizing functions like `monthrange()` and `weekday()`, we can determine the number of days in a month and calculate the day within the month for a given date. The example code provided demonstrates the usage of these functions and their combined application. Understanding these methods can be helpful when working with dates and calendars in Python.
Images related to the topic python get days in month
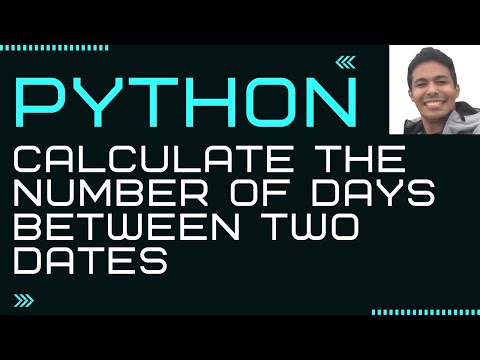
Found 34 images related to python get days in month theme
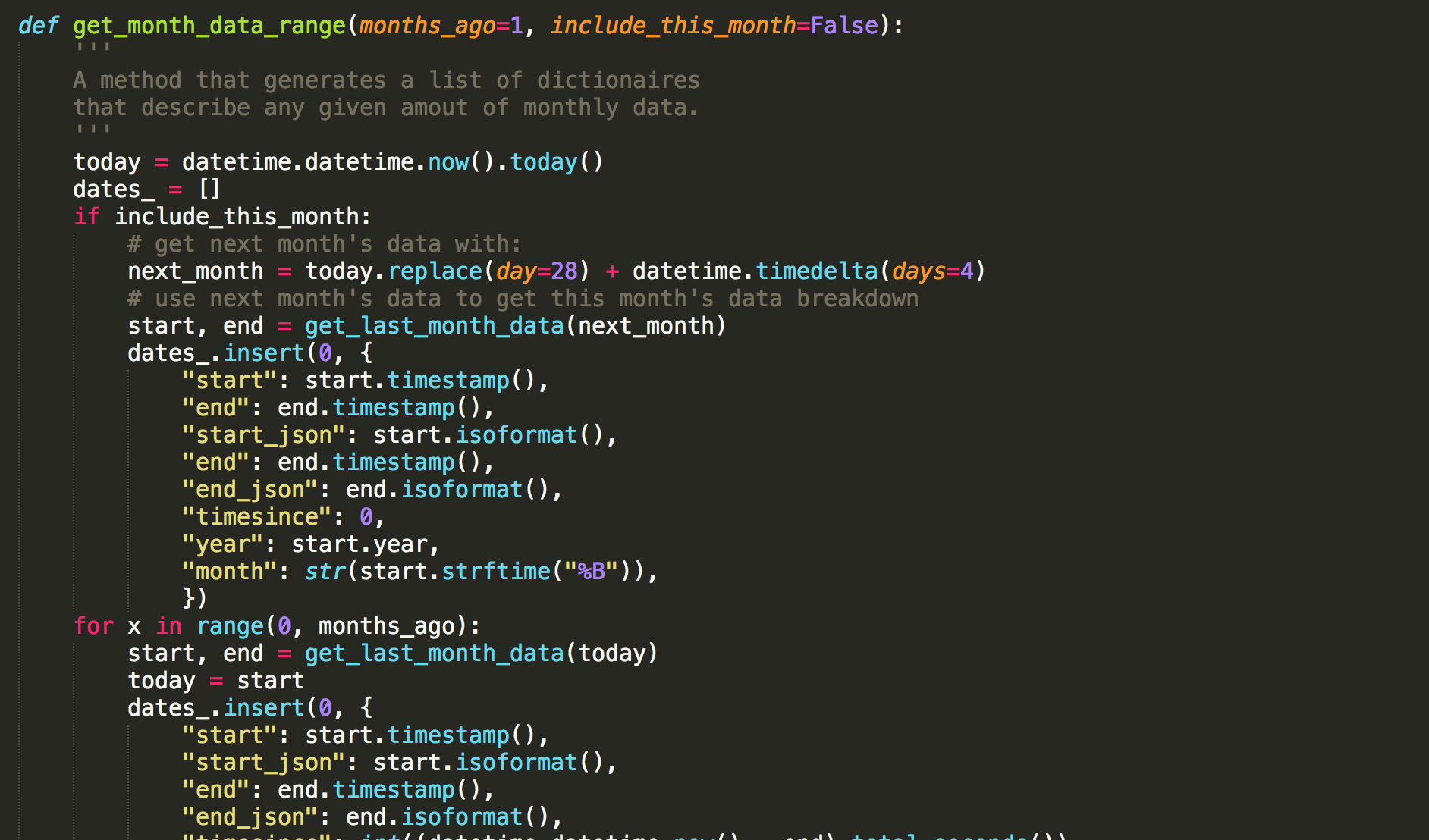
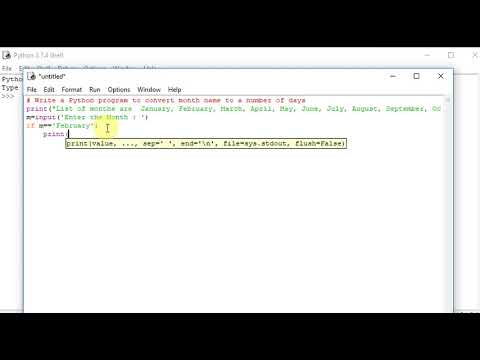
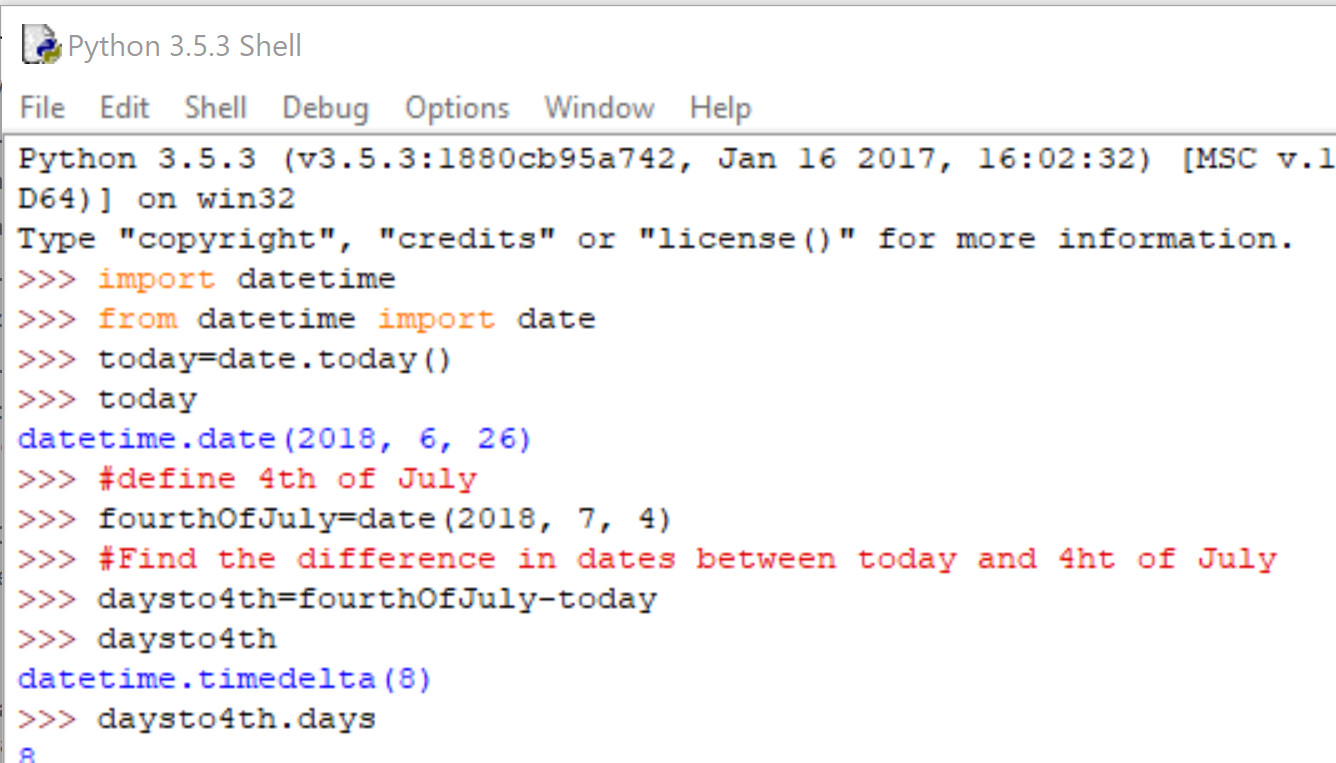
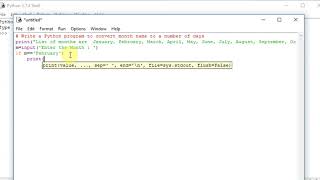
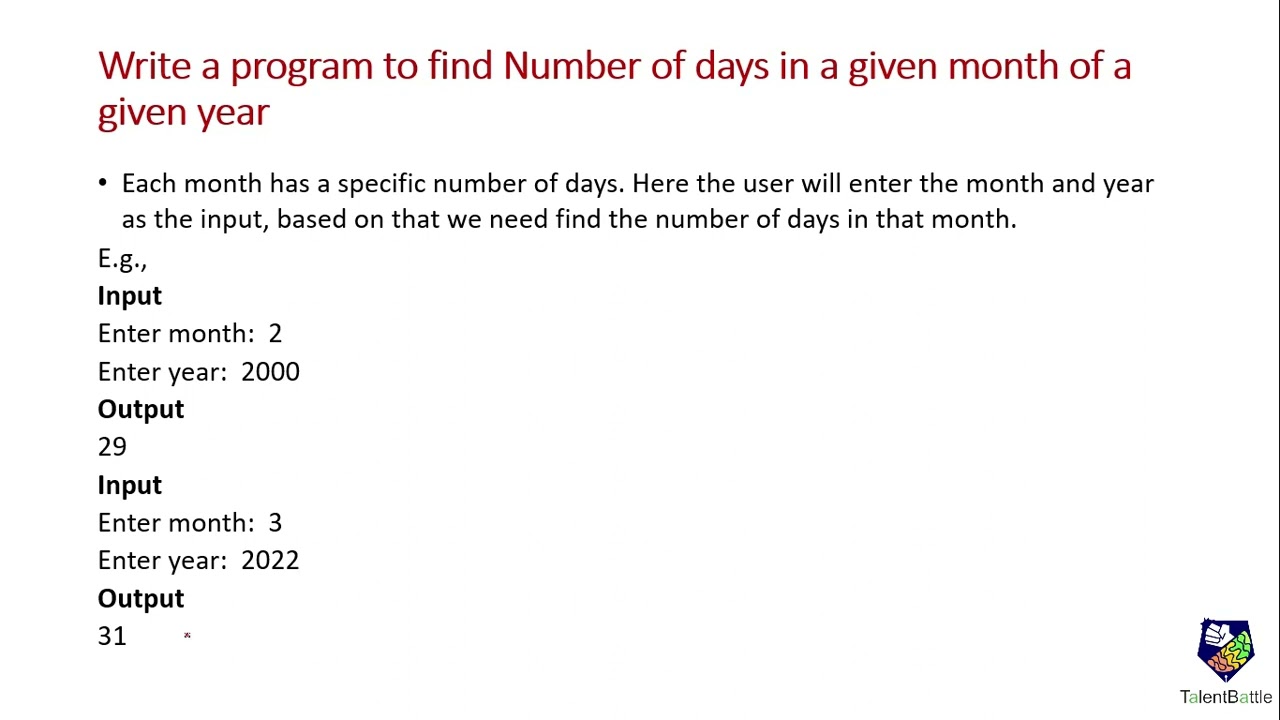


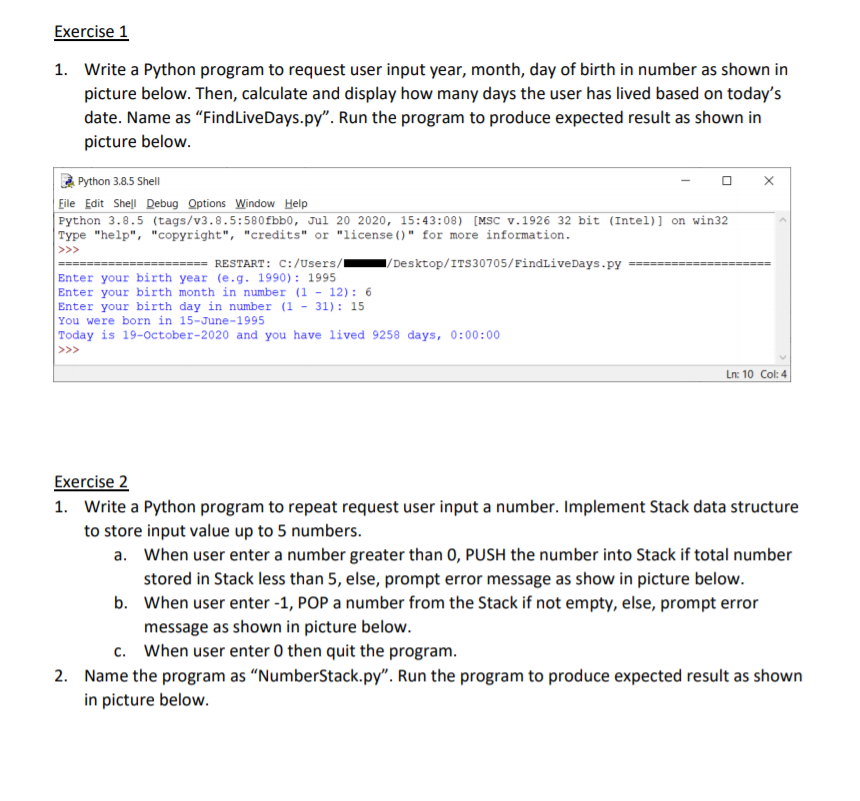

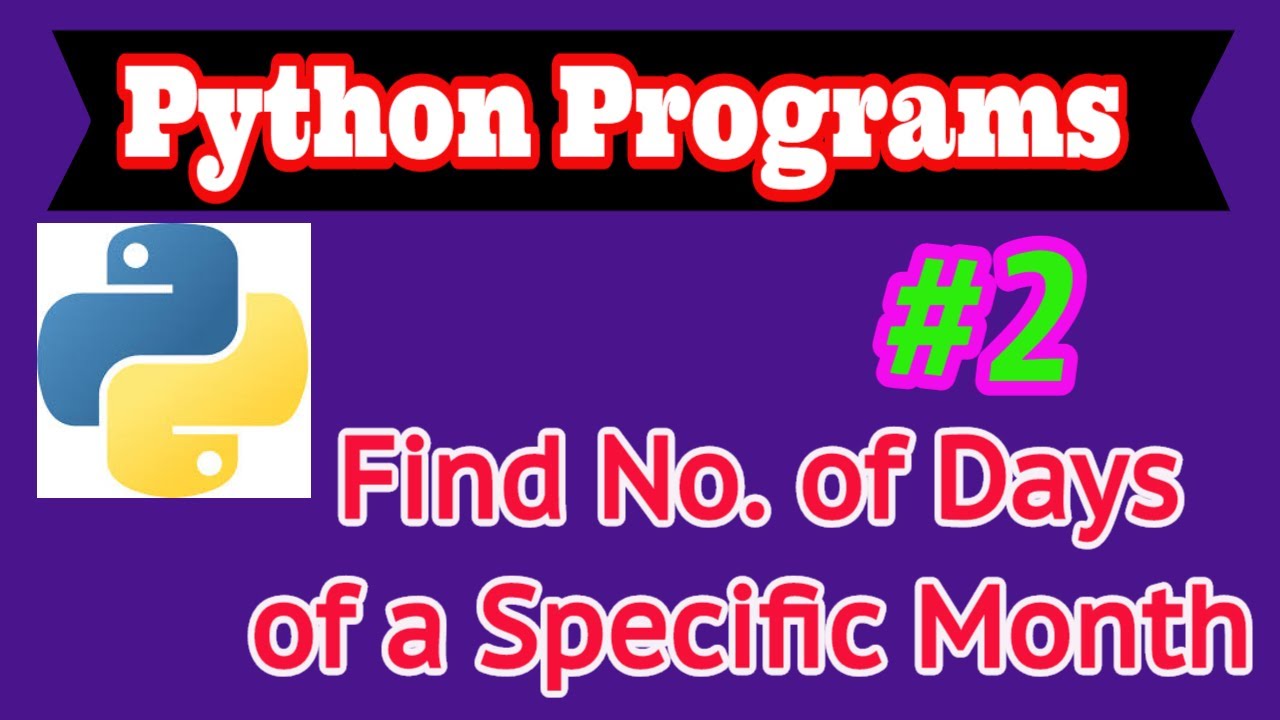
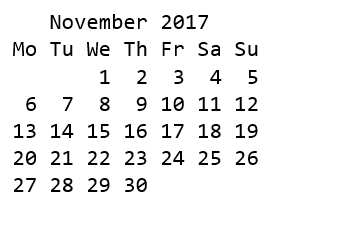
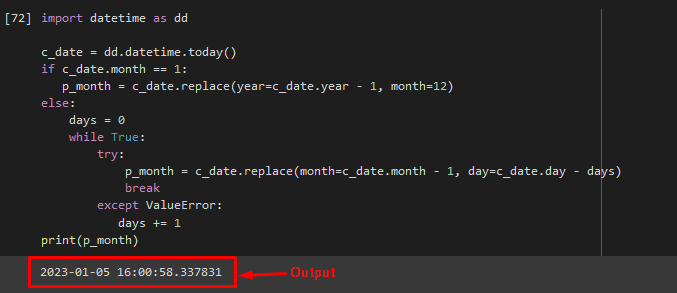
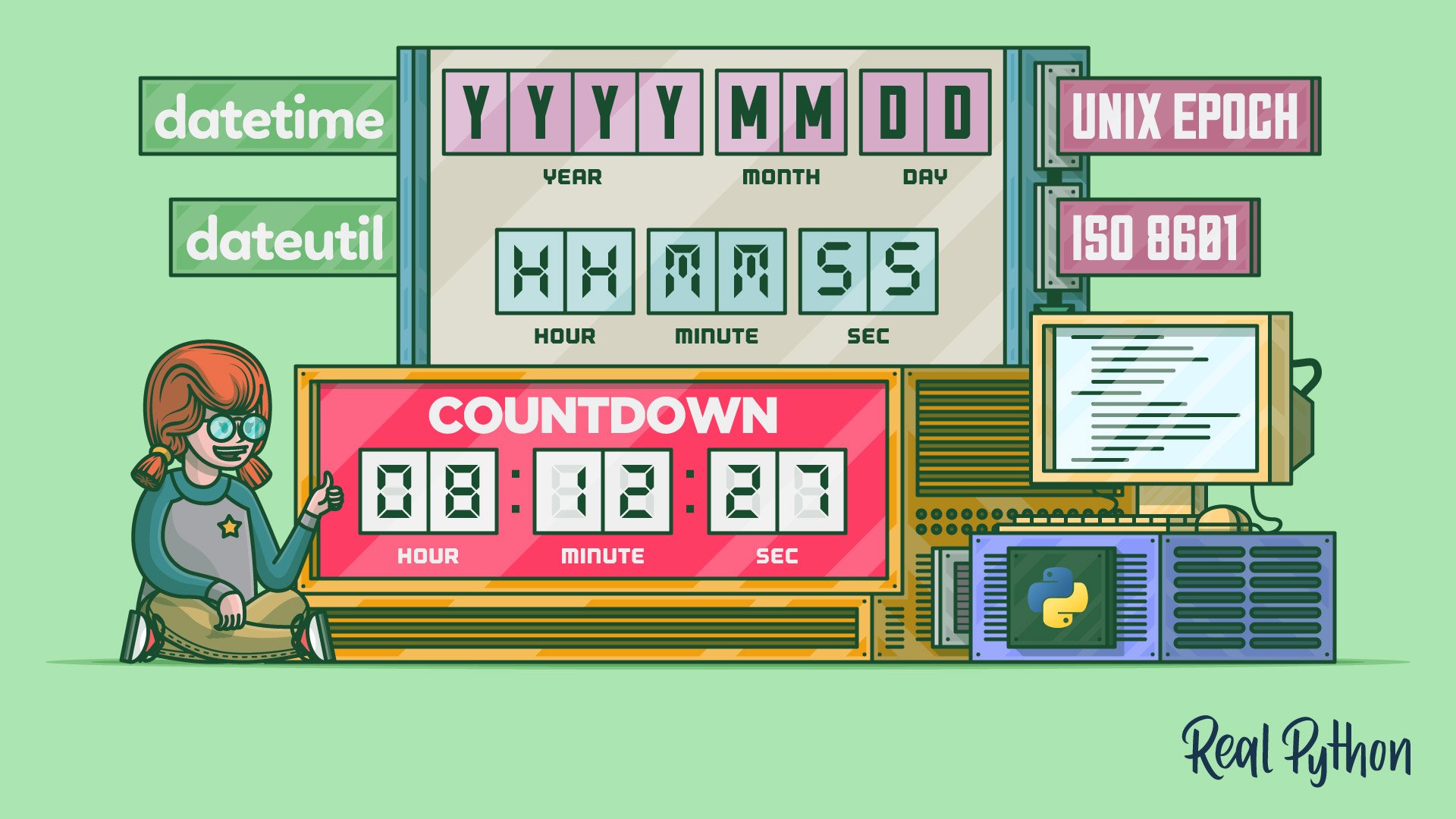
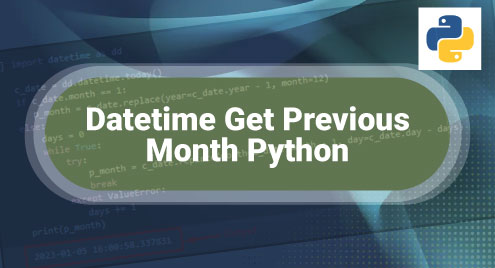
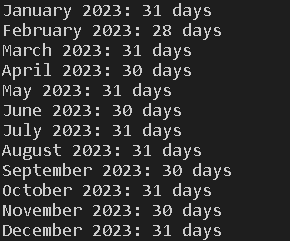

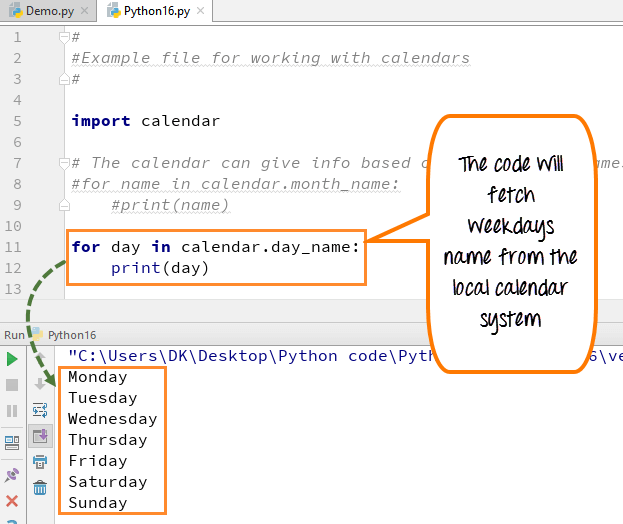
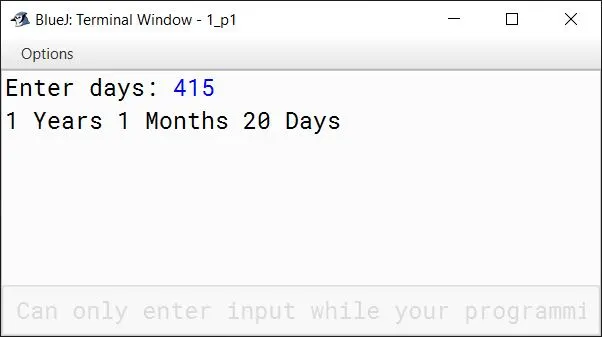
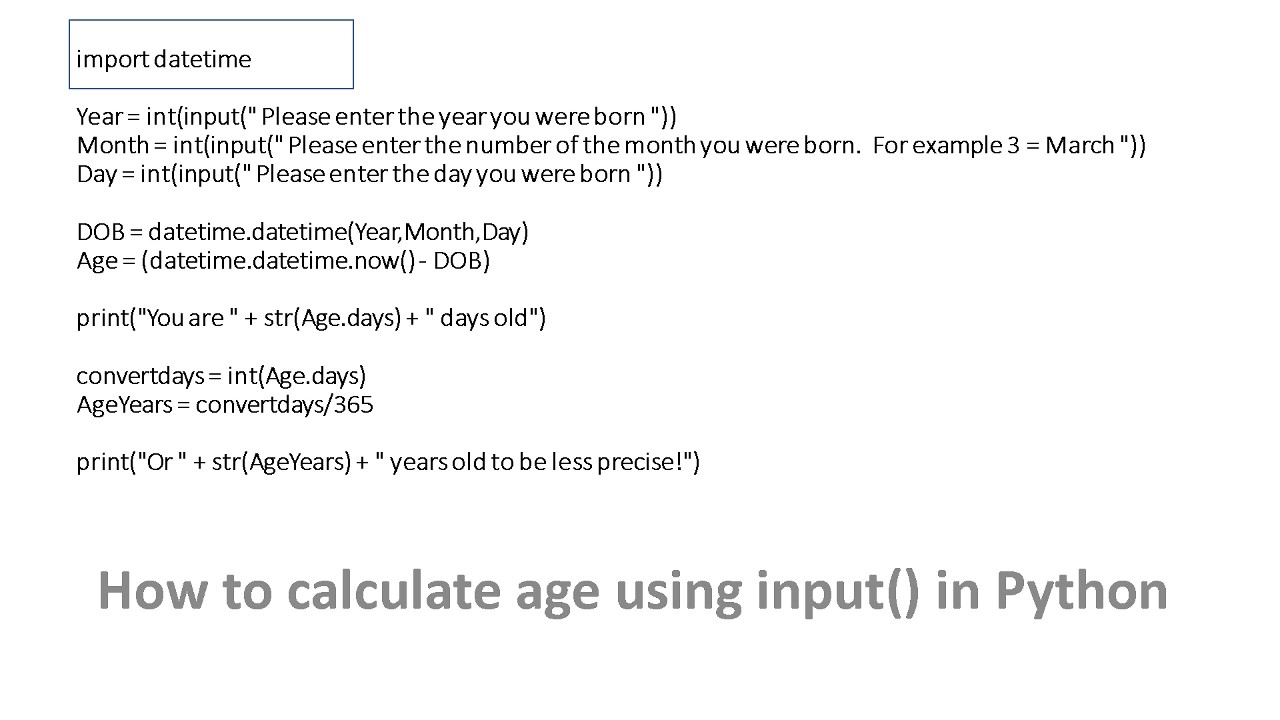
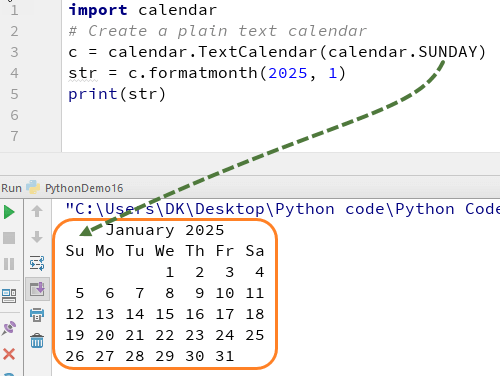
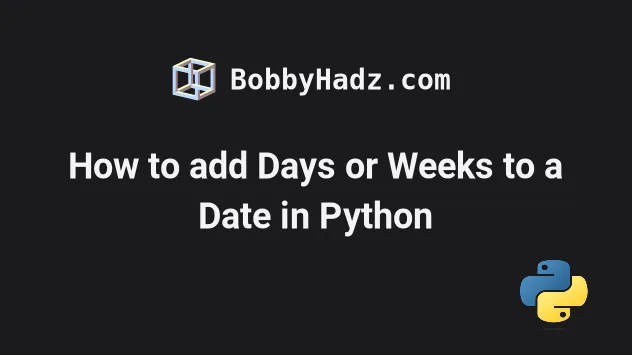
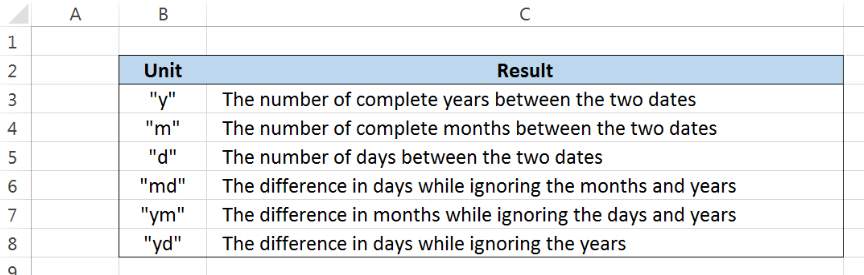
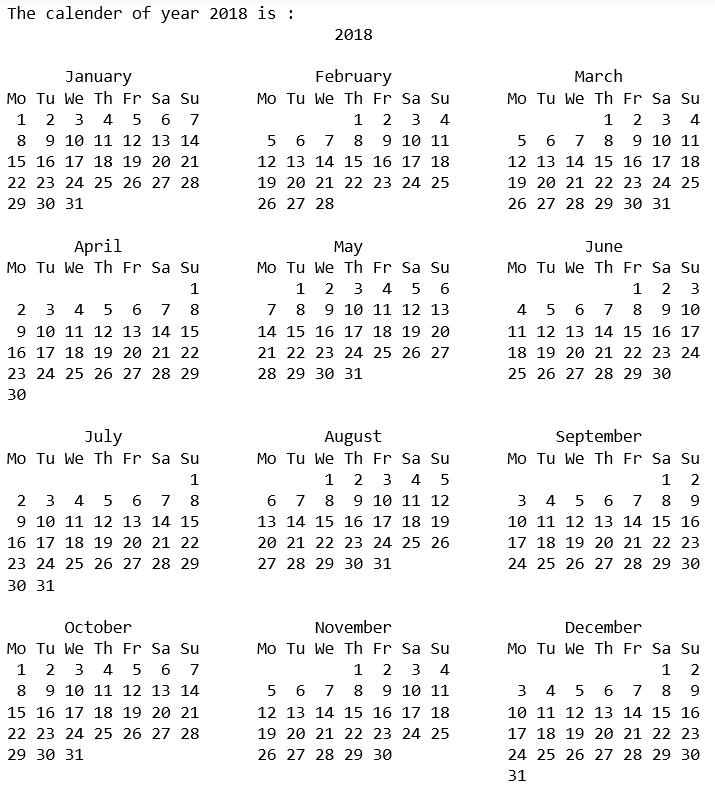
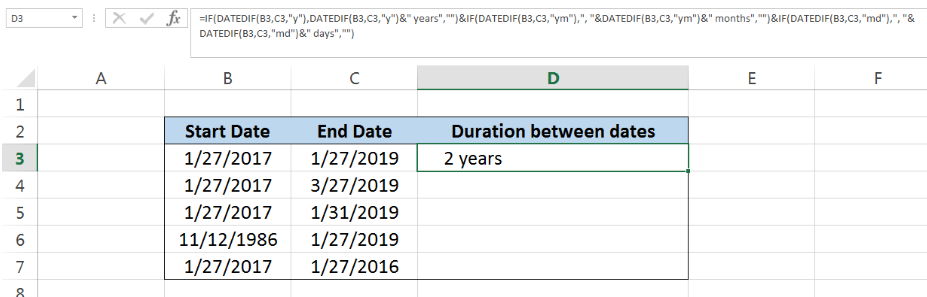


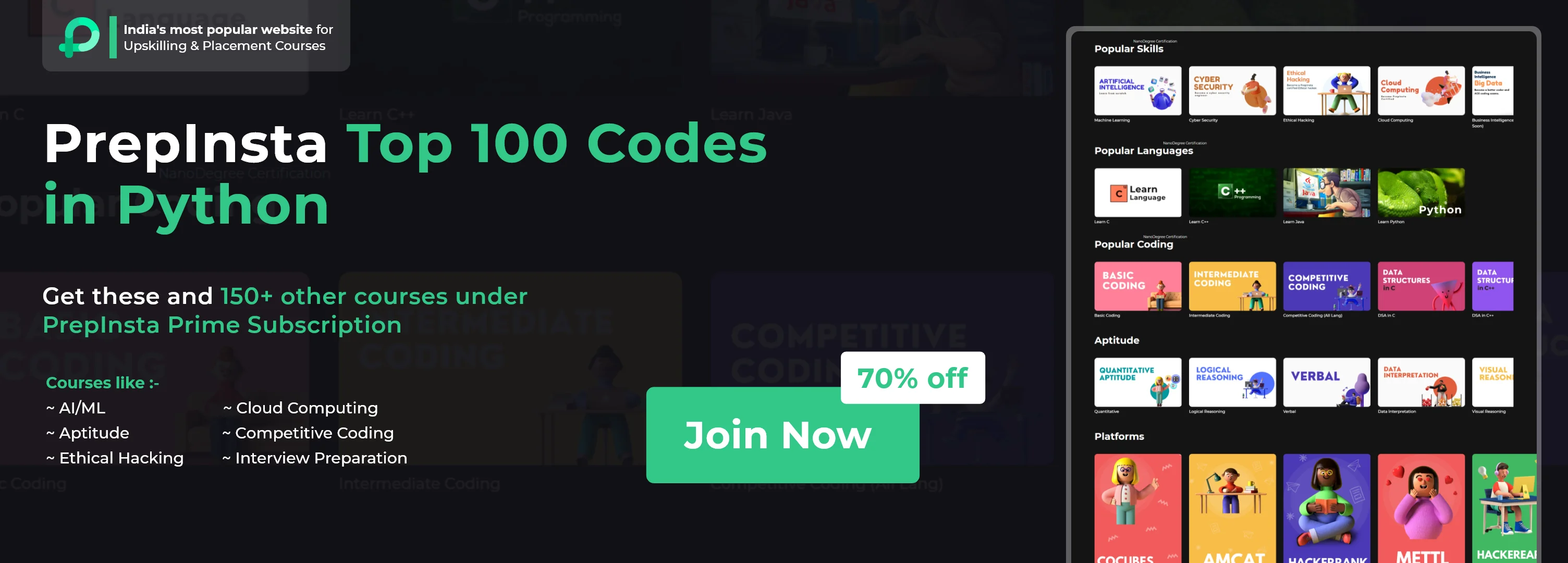

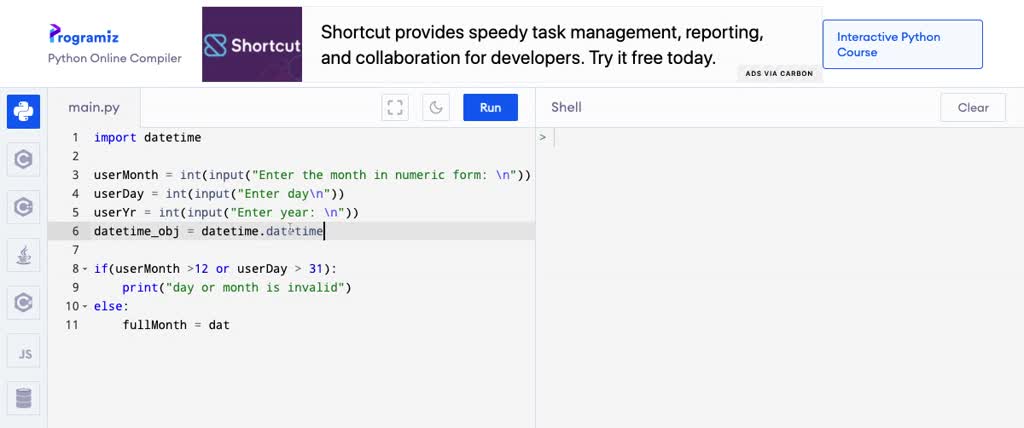

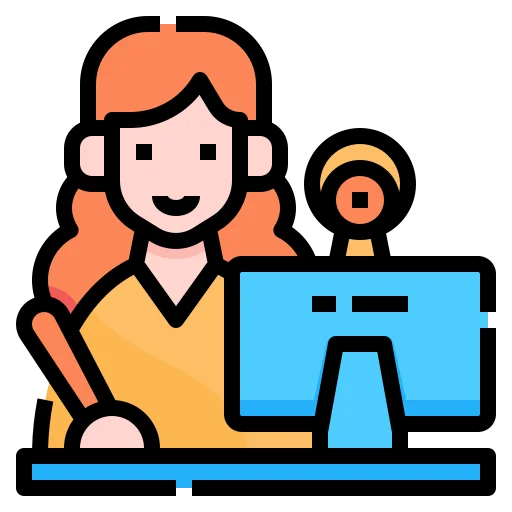
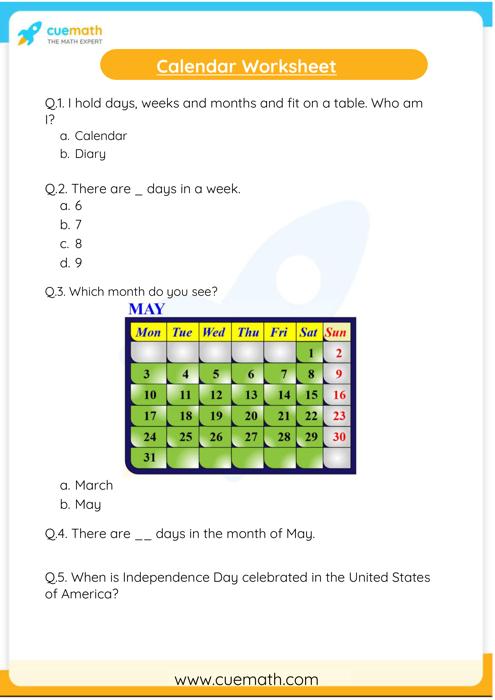

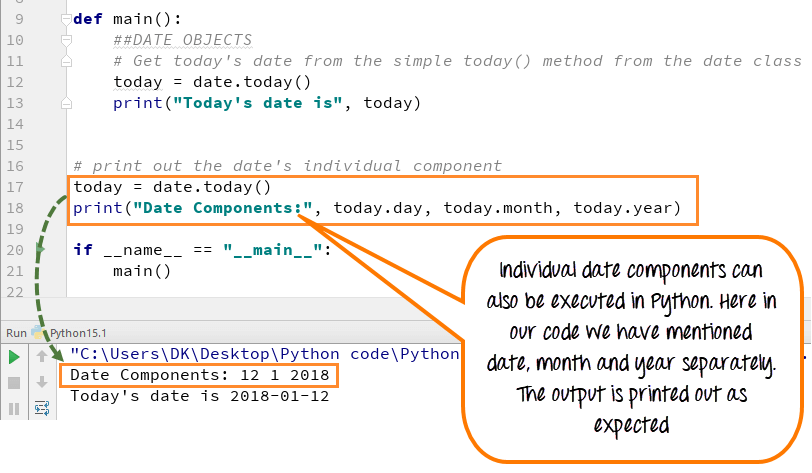
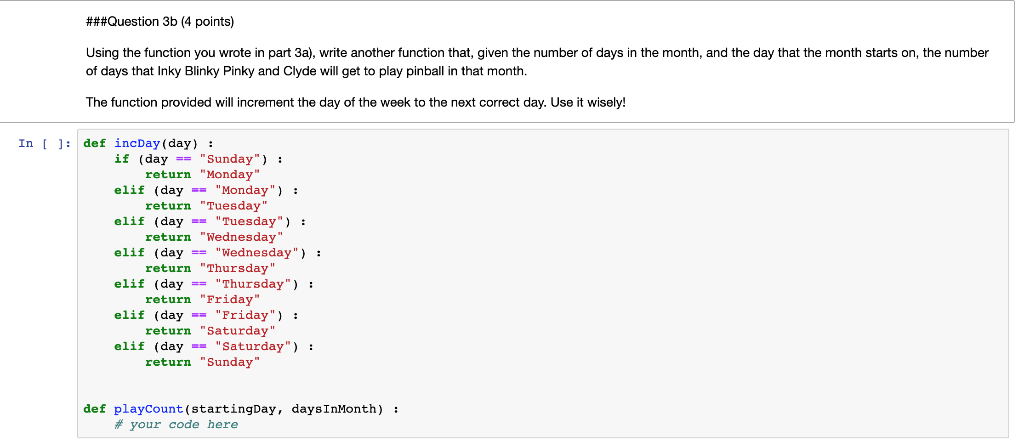
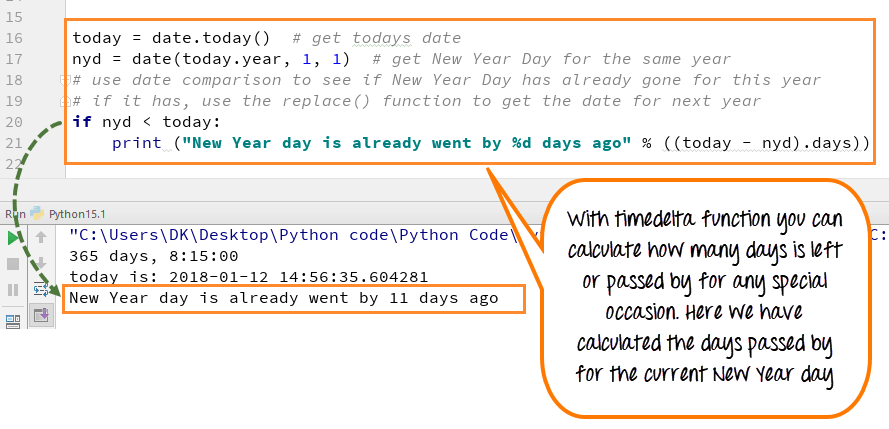
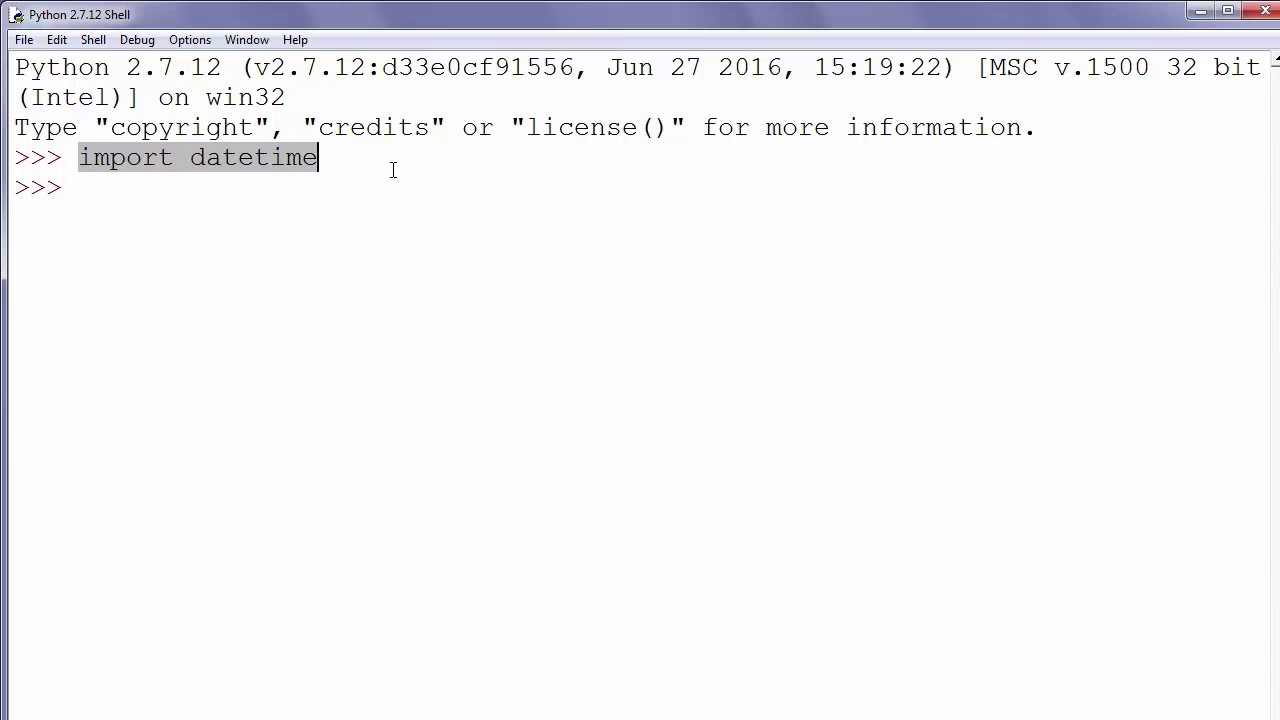
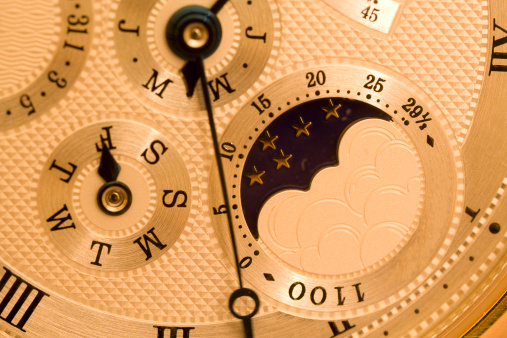
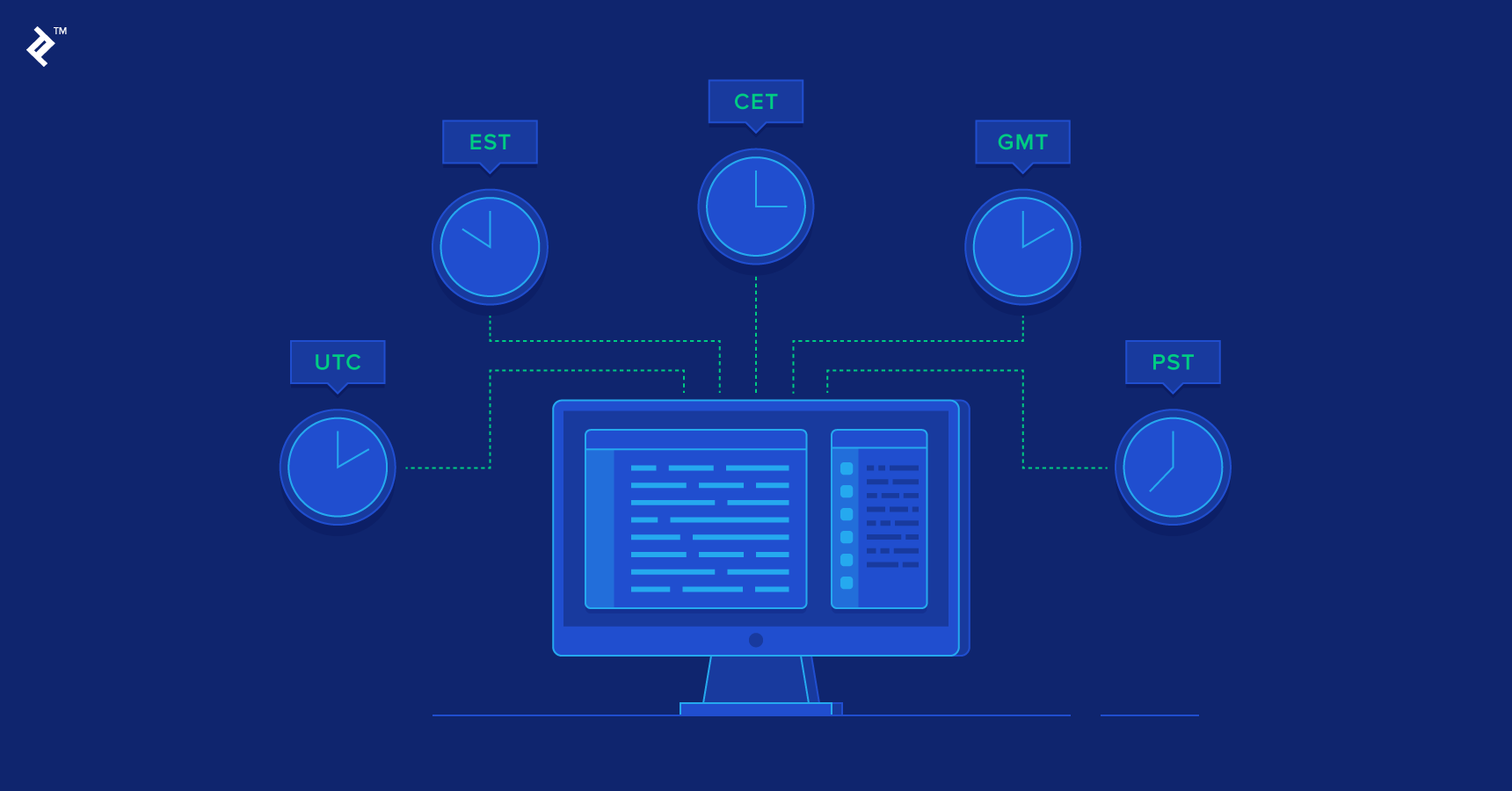
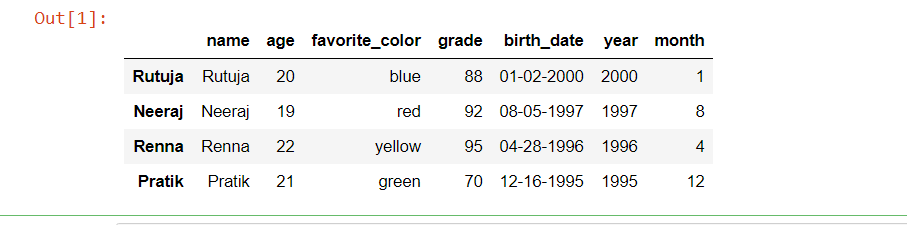
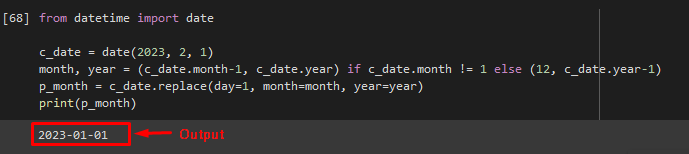
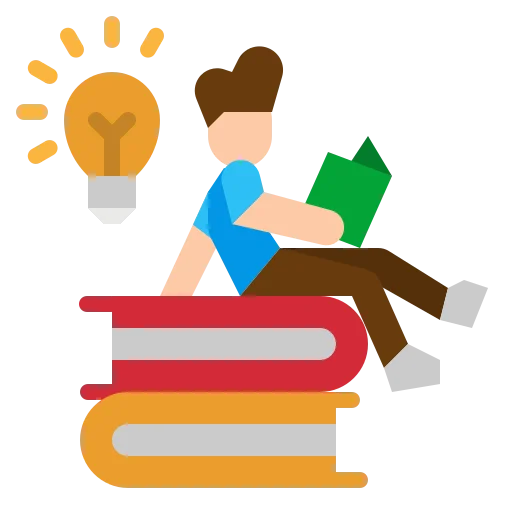
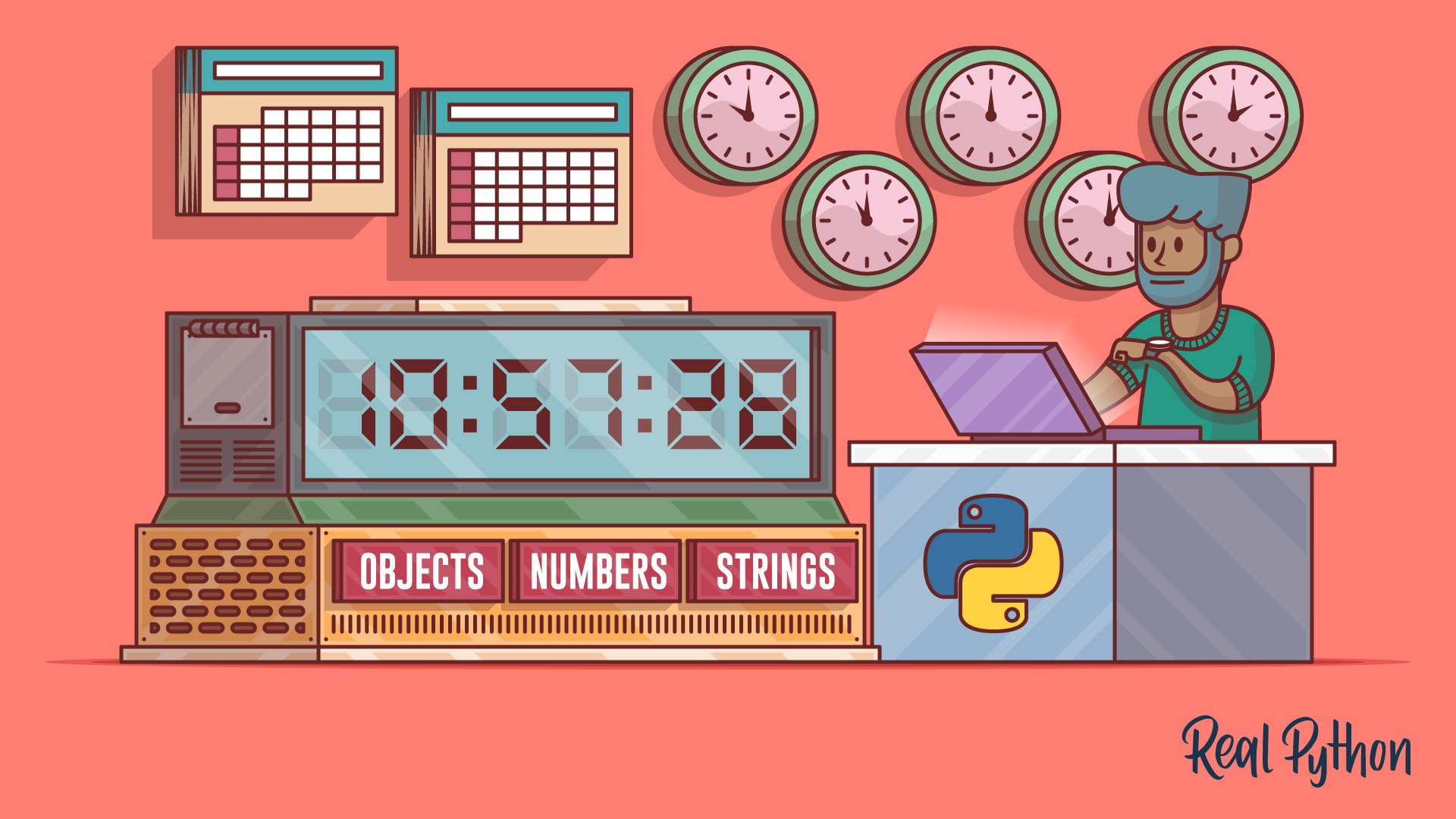
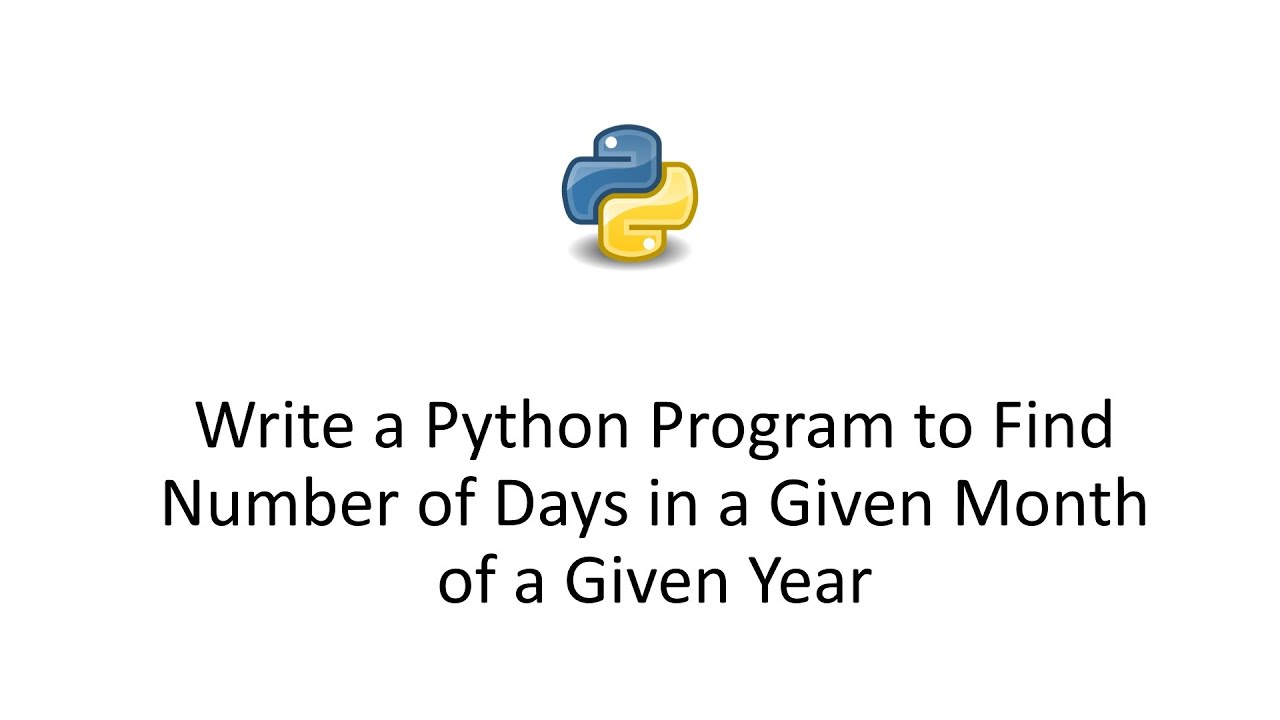
Article link: python get days in month.
Learn more about the topic python get days in month.
- Find the number of days and weeks of a month in Python
- How do we determine the number of days for a given month in …
- How to get number of days in month in Python – TechOverflow
- Determine the Number of Days in a Month with Python
- Python Get Business Days [4 Ways] – PYnative
- Display all the dates for a particular month using NumPy – GeeksforGeeks
- Pandas Get Day, Month and Year from DateTime – Spark By {Examples}
- 12 Months of the Year – Time and Date
- Number of days in a month in Python – Tutorialspoint
- How to return the number of days in a month in pandas
- pandas.Period.days_in_month — pandas 2.0.3 documentation
- Number of days in a given month of a year in python – PrepInsta
- Python: Get the number of days of a given month and year
- Python Get Last Day of Month [4 Ways] – PYnative
See more: nhanvietluanvan.com/luat-hoc