Python Get Current Year
Introduction:
Python is a versatile and popular programming language known for its simplicity and power. It offers a wide range of functionalities, including the ability to work with dates and time. In this article, we will explore how to get the current year using Python’s datetime module. Additionally, we will discuss other related topics such as retrieving the last year, getting the day of the year, extracting the year from a datetime object, and more.
1. Importing the datetime module:
Firstly, we need to import the datetime module, which provides the necessary functions and classes to work with dates and times in Python. To import the module, use the following line of code:
“`
import datetime
“`
Now we are ready to start working with dates and times.
2. Retrieving the current date and time:
To get the current date and time, we can use the `datetime` class along with its `now()` method. The `now()` method returns a datetime object representing the current date and time. Here’s an example:
“`
current_datetime = datetime.datetime.now()
“`
This will store the current date and time in the `current_datetime` variable.
3. Extracting the current year using datetime functions:
Once we have the current datetime object, we can extract the year using various datetime functions. One of the commonly used functions is `year`, which returns the year from a given datetime object. Here’s an example:
“`
current_year = current_datetime.year
“`
Now the variable `current_year` will contain the current year.
4. Printing the current year:
To display the current year, we can simply use the `print` function. For example:
“`
print(“The current year is:”, current_year)
“`
This will print the sentence “The current year is:” followed by the value stored in `current_year`.
5. Handling localization and timezones for the current year:
Python’s datetime module also provides functionality to handle localization and timezones. If you want to retrieve the current year according to a specific timezone, you can use the `astimezone()` method. This method converts the datetime object to the desired timezone. Here’s an example:
“`
import pytz # Import the pytz module for timezone support
current_datetime = datetime.datetime.now()
new_timezone = pytz.timezone(‘America/New_York’) # Set the desired timezone
localized_datetime = current_datetime.astimezone(new_timezone)
localized_year = localized_datetime.year
print(“The current year in New York is:”, localized_year)
“`
In this example, we imported the `pytz` module to obtain timezone support. We then created a new timezone object for ‘America/New_York’. After converting the datetime object to the new timezone using `astimezone()`, we extracted the year as before. Finally, we printed the current year in New York according to the new timezone.
6. Conclusion:
In this article, we explored how to get the current year in Python using the datetime module. We discussed the process of importing the module, retrieving the current date and time, extracting the year, and printing the result. Additionally, we covered how to handle localization and timezones to obtain the current year according to a specific timezone. By following these steps, you can easily retrieve the current year and incorporate it into your Python applications.
FAQs:
Q1. How can I get the last year using Python?
To retrieve the last year, you can subtract 1 from the current year. Here’s an example:
“`
last_year = current_year – 1
“`
This will subtract 1 from the current year and store the result in the `last_year` variable.
Q2. How can I get the day of the year using Python?
To get the day of the year, you can use the `timetuple()` method along with the `tm_yday` attribute. Here’s an example:
“`
day_of_year = current_datetime.timetuple().tm_yday
“`
This will return the day of the year as an integer.
Q3. How can I extract the year from a datetime object in Python?
To extract the year from a datetime object, you can use the `year` attribute. Here’s an example:
“`
year = datetime_obj.year
“`
Replace `datetime_obj` with your datetime object, and the variable `year` will contain the extracted year.
Q4. How can I get the week of the year in Python?
To retrieve the week of the year, you can use the `isocalendar()` method along with the `ISOWeek` attributes. Here’s an example:
“`
week_of_year = current_datetime.isocalendar()[1]
“`
This will return the week of the year as an integer.
Q5. How can I get the last day of the year using Python?
To obtain the last day of the year, you can increment the current year by 1 and use the `replace()` method to set the month and day to 1 and 2 respectively. Then, subtracting 1 day will give you the last day of the previous year. Here’s an example:
“`
last_day_of_year = datetime.datetime(current_year + 1, 1, 2).replace(day=1) – datetime.timedelta(days=1)
“`
This will store the last day of the year as a datetime object.
Q6. How can I get the datetime of one year ago using Python?
To obtain the datetime of one year ago, you can subtract 1 year from the current datetime using `timedelta`. Here’s an example:
“`
one_year_ago = current_datetime – datetime.timedelta(days=365)
“`
This will subtract 365 days from the current datetime and store the result in the `one_year_ago` variable.
In conclusion, obtaining the current year or performing related tasks such as retrieving the last year, getting the day of the year, extracting the year from a datetime object, and more can be easily achieved using Python’s datetime module. The provided examples and explanations should serve as a solid foundation for incorporating date and time functionalities into your Python projects.
Getting The Current Year – 1 Minute Python Tutorial #Shorts
Keywords searched by users: python get current year Get current year python, Get Last year python, Python get day of year, Getyear in python, Get year from datetime python, Get week of year Python, Get last day of year python, Python get datetime one year ago
Categories: Top 36 Python Get Current Year
See more here: nhanvietluanvan.com
Get Current Year Python
Introduction:
Python is a versatile programming language known for its simplicity, readability, and vast community support. One of the common requirements in various applications is retrieving the current year dynamically. Rather than hard-coding the year manually, Python developers can make use of a handy built-in function called “get current year.” In this article, we’ll explore how to use this function effectively, understand its underlying logic, and cover some frequently asked questions related to its usage.
Understanding the ‘Get Current Year’ Python Function:
The ‘get current year’ function is a built-in Python function that provides an easy and straightforward method to retrieve the current year dynamically. By utilizing this function, developers can effortlessly implement year-specific features in their applications without needing to update the year manually. This ensures that the year remains accurate regardless of when the code is executed.
To retrieve the current year using Python, one can utilize the ‘datetime’ module. This module provides classes and functions for working with dates and times, including the functionality to fetch the current year. The ‘datetime’ module needs to be imported at the beginning of the Python script, using the following code:
“`python
import datetime
“`
Once the ‘datetime’ module is imported, the ‘get current year’ function can be called using the following code:
“`python
current_year = datetime.datetime.now().year
“`
The ‘datetime.now()’ function retrieves the current date and time, while ‘.year’ extracts the year from the ‘datetime’ object. By assigning this value to the variable ‘current_year,’ the exact current year is stored for further use within the script.
Frequently Asked Questions (FAQs):
Q1: Can the ‘Get Current Year’ function be used to retrieve a specific year from the past or future?
A1: No, the ‘Get Current Year’ function is designed exclusively to fetch the current year. If you require a year different from the current one, you may need to use additional Python functionality to manipulate dates and time.
Q2: Is it necessary to import the ‘datetime’ module every time we need to fetch the current year?
A2: Yes, in order to utilize the ‘get current year’ function, the ‘datetime’ module must be imported at the beginning of each Python script where the function is used.
Q3: Can the ‘Get Current Year’ function be used with other Python libraries?
A3: Absolutely! The ‘get current year’ function is not dependent on any specific Python libraries. Therefore, it can be used alongside other libraries and frameworks to implement various functionalities.
Q4: What happens if the ‘datetime’ module is not imported?
A4: If the ‘datetime’ module is not imported, attempting to call the ‘get current year’ function will result in a ‘NameError’ as Python will not recognize the function or the related ‘datetime’ class.
Q5: Can I retrieve the current year in a specific timezone?
A5: Yes, you can retrieve the current year in a specific timezone. By using the ‘pytz’ module, which provides access to the Olson database, Python allows developers to fetch the current year in a specific timezone rather than relying on the system’s default timezone.
Conclusion:
The ‘get current year’ Python function is a simple-yet-powerful built-in function that simplifies the process of fetching the current year dynamically. By leveraging the functionality offered by the ‘datetime’ module, developers can ensure their applications always display the correct current year without the need for manual updating. Understanding how to retrieve the current year and its underlying logic enables developers to implement year-specific features efficiently.
Frequently used in web development, financial apps, and customized calendars, the ‘get current year’ function is a convenient tool for Python programmers to enhance the user experience by presenting dynamically accurate date-related information.
FAQs section:
Q1: Can the ‘Get Current Year’ function be used to retrieve a specific year from the past or future?
A2: Is it necessary to import the ‘datetime’ module every time we need to fetch the current year?
A3: Can the ‘Get Current Year’ function be used with other Python libraries?
A4: What happens if the ‘datetime’ module is not imported?
A5: Can I retrieve the current year in a specific timezone?
Get Last Year Python
Get Last year python is a built-in function in the Python programming language that provides the capability to fetch the previous year. It simplifies the process of calculating the last year by automatically handling the complexities of accounting for the different lengths of months and leap years. This function is especially helpful when working with date and time-based applications, such as data analysis, financial projections, or event scheduling.
To implement the Get Last year python function, you can utilize the datetime library, which is part of the Python standard library. This library provides classes and functions for manipulating dates and times. One of the classes provided by this library is the datetime.datetime class, which allows detailed handling of dates and times.
To use the Get Last year python function, you need to import the datetime module into your Python script. This can be done with the following line of code at the beginning of your script:
“`python
import datetime
“`
Once the datetime module is imported, you can utilize the datetime.datetime class to fetch the current date, and then subtract one year to obtain the previous year. The code snippet below demonstrates how to achieve this:
“`python
import datetime
current_year = datetime.datetime.now().year
previous_year = current_year – 1
print(“Current Year:”, current_year)
print(“Previous Year:”, previous_year)
“`
In the above code, the `datetime.datetime.now().year` statement fetches the current year. By subtracting one from the current year, the previous year is calculated and stored in the variable `previous_year`. Finally, the results are printed to the console.
Using this simple method, you can easily retrieve the previous year in Python without worrying about the intricacies of leap years or varying month lengths. This feature saves programmers valuable time and simplifies their code, resulting in more efficient and readable programs.
FAQs:
Q1: Can I use Get Last year python for any date in the past, not just the current year?
A1: Yes, you can use the Get Last year python function with any given date. Simply replace `datetime.datetime.now().year` with the desired date in the `datetime.datetime` format. For example: `datetime.datetime(2023, 5, 10).year`.
Q2: Does Get Last year python handle leap years correctly?
A2: Yes, Get Last year python accounts for leap years automatically. The datetime module in Python handles leap years correctly and adjusts the calculations accordingly. Therefore, there is no need to manually consider leap years when using this function.
Q3: Is it possible to calculate the previous year using other Python libraries?
A3: Absolutely! While the datetime module is the most straightforward way to get the previous year in Python, other libraries, such as NumPy and Pandas, also provide similar capabilities. These libraries offer additional functionalities and more advanced date and time manipulation options, which may be beneficial for specific use cases or applications.
In conclusion, the Get Last year python function simplifies the process of fetching the previous year in Python. By utilizing the datetime module from the Python standard library, you can easily retrieve the previous year without the need to worry about varying month lengths or leap years. This feature saves time and enhances the readability and efficiency of your code.
Images related to the topic python get current year
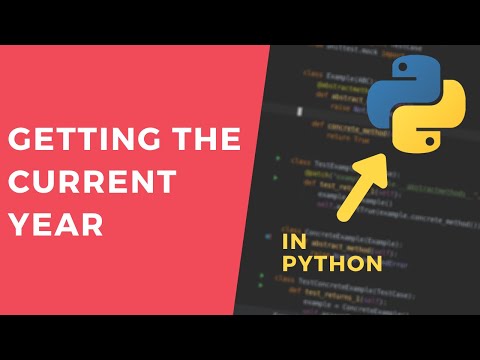
Found 30 images related to python get current year theme


![Flow of Control] Write a program to find whether a person is a senior Flow Of Control] Write A Program To Find Whether A Person Is A Senior](https://d1avenlh0i1xmr.cloudfront.net/858bb1fd-f173-48ae-8e96-f8fe42dd5f1a/long-question-1---teachoo.jpg)
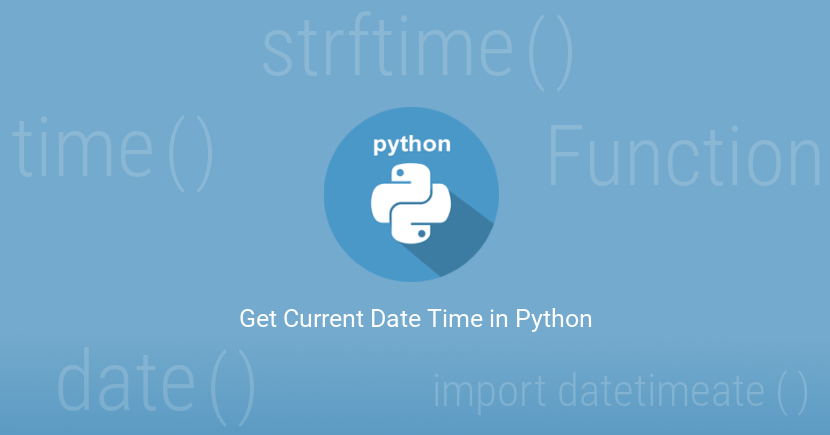

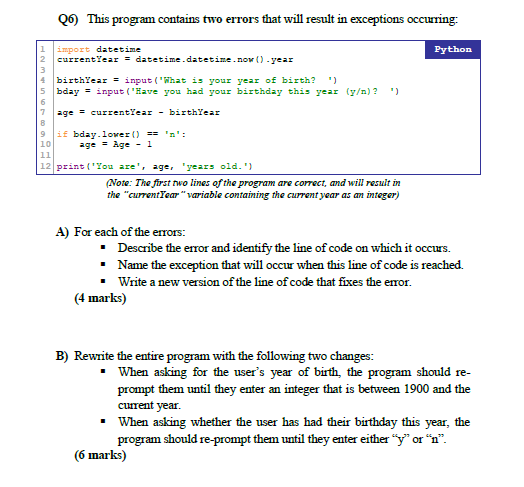
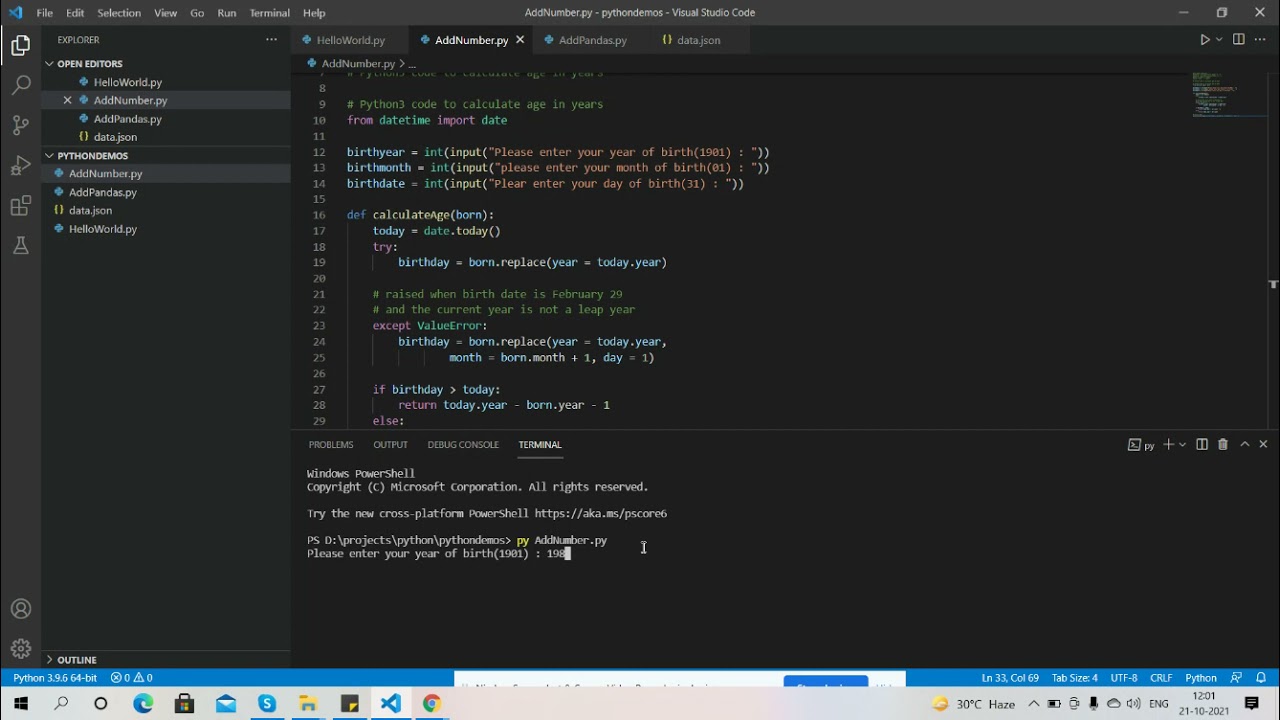
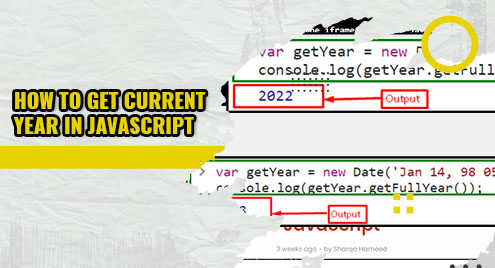

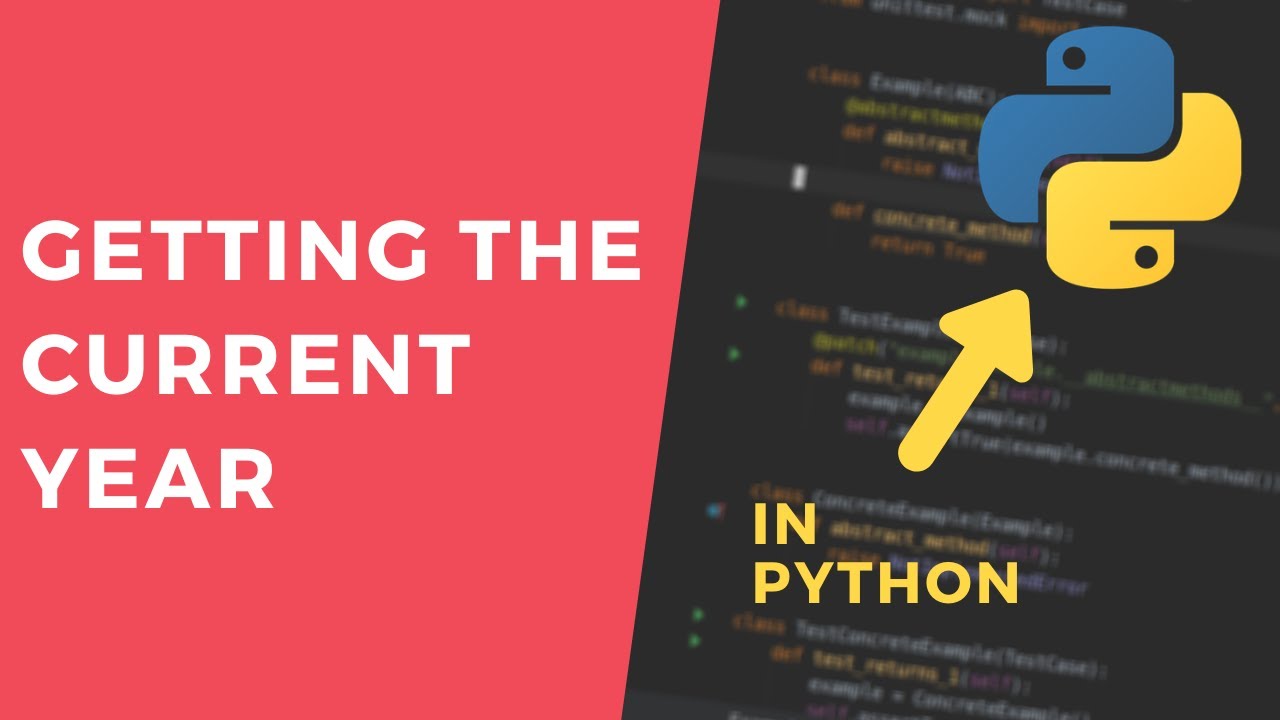
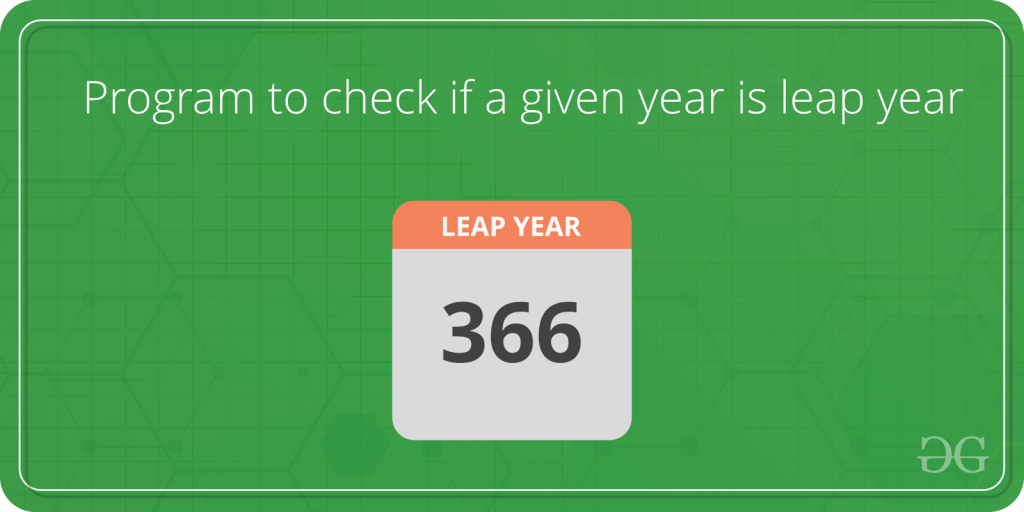
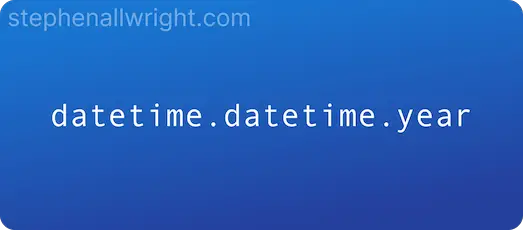
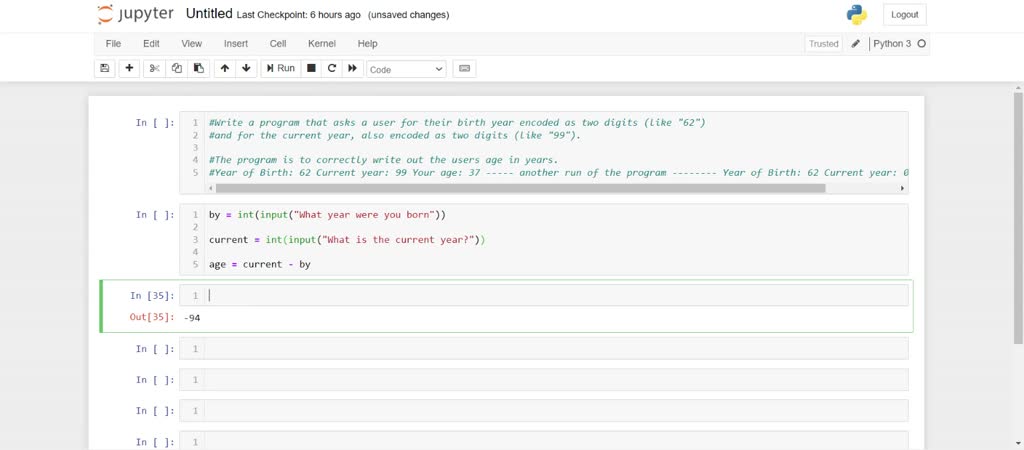
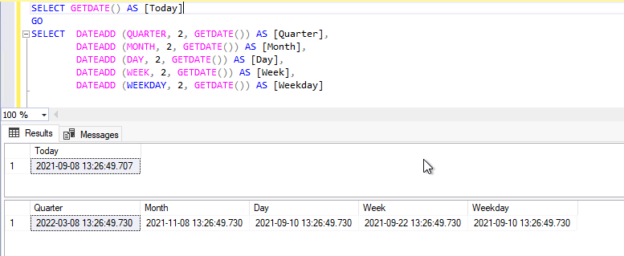
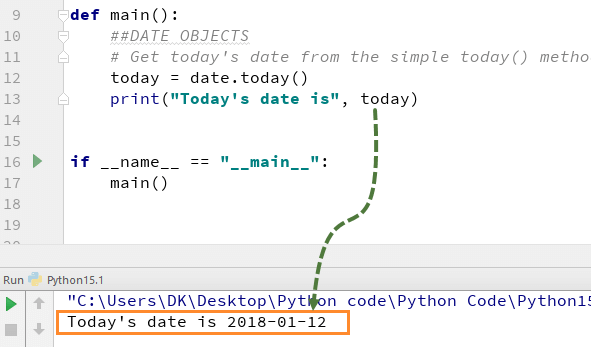
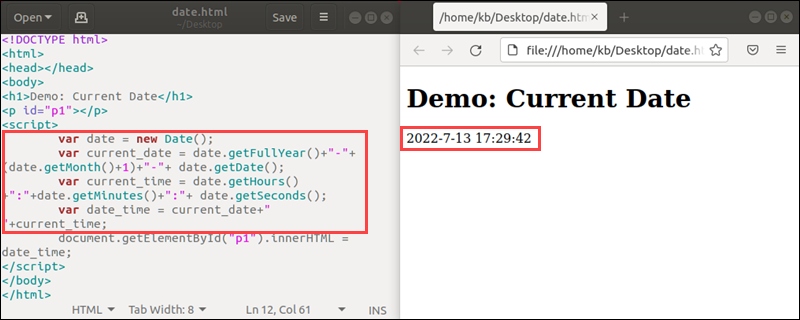
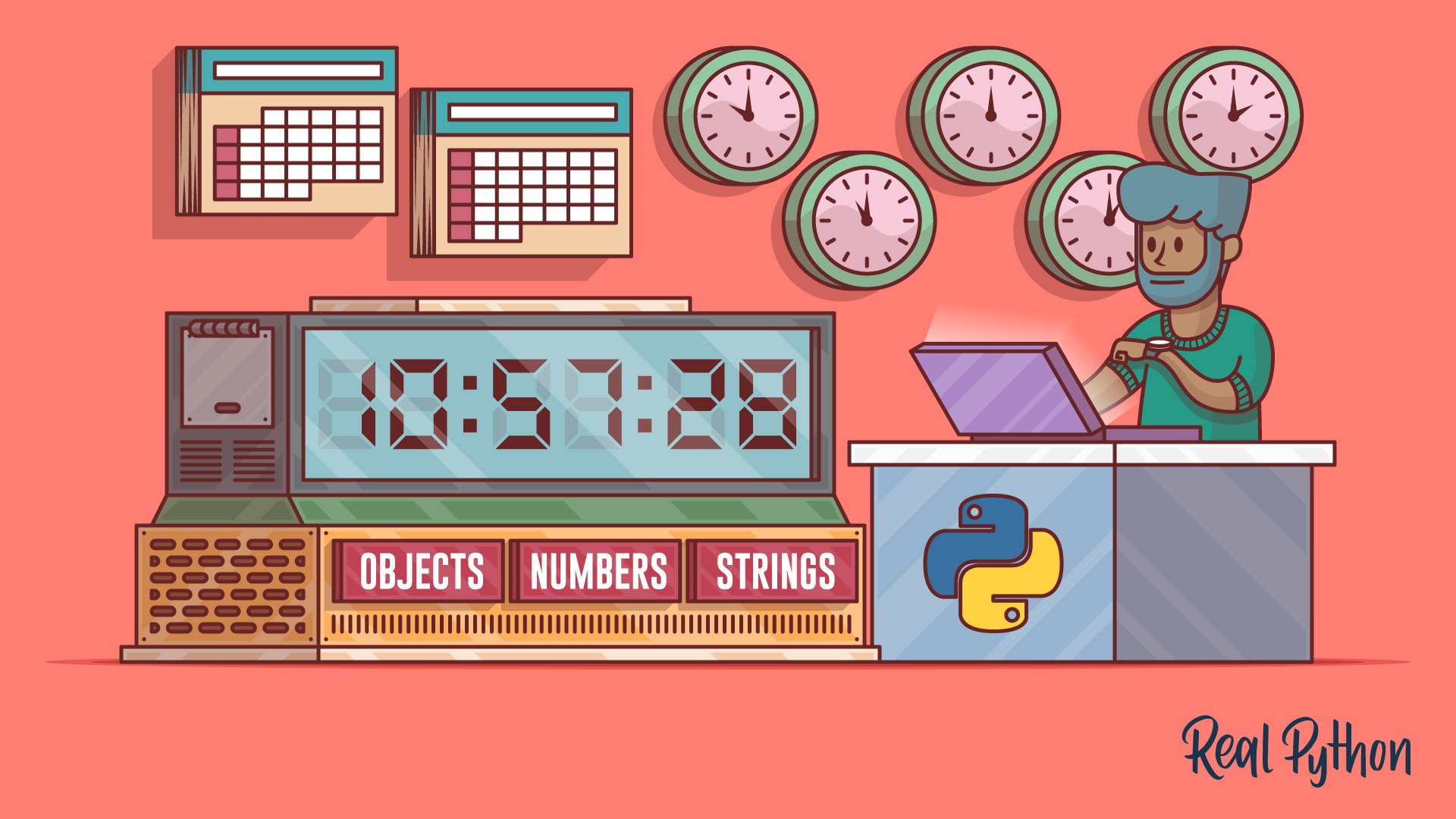
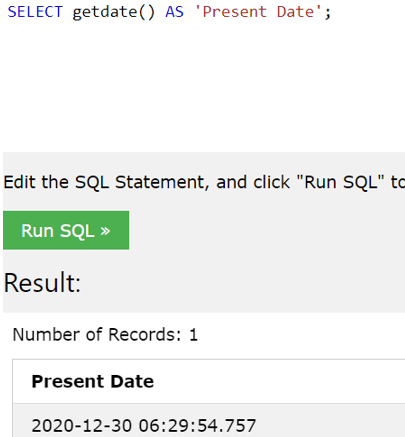
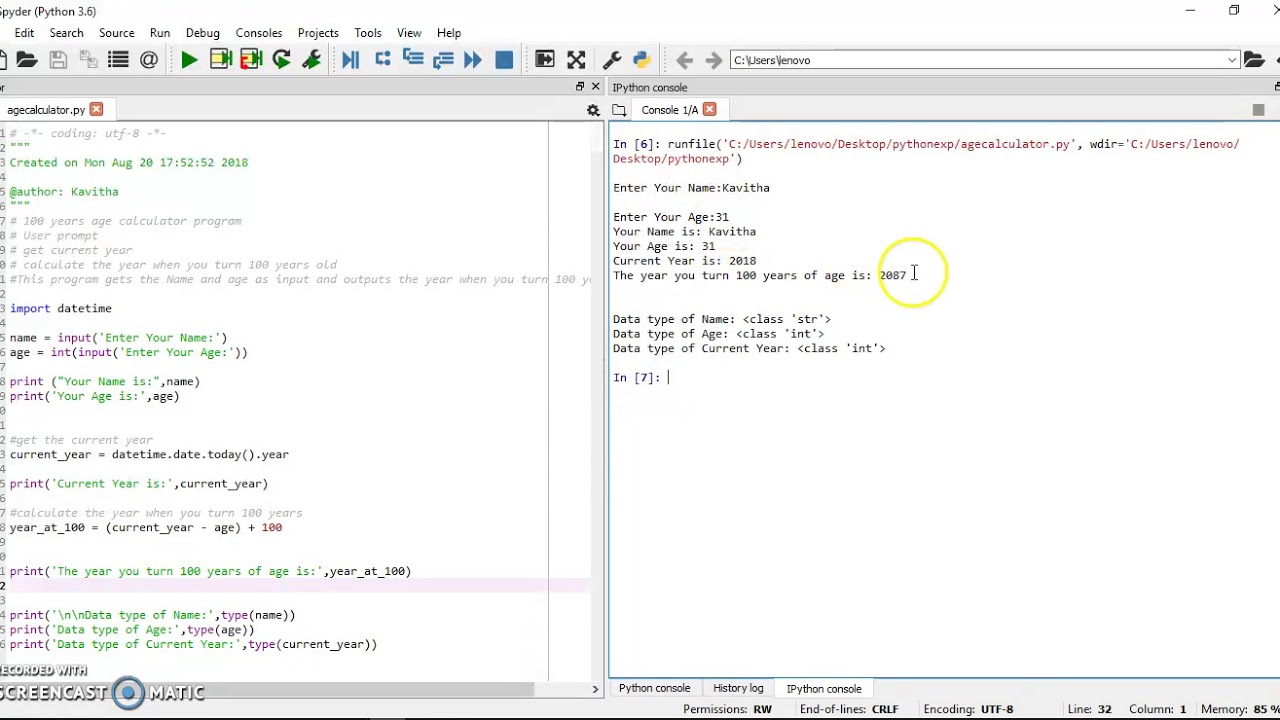
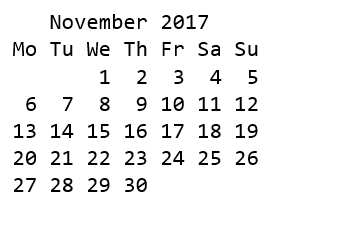


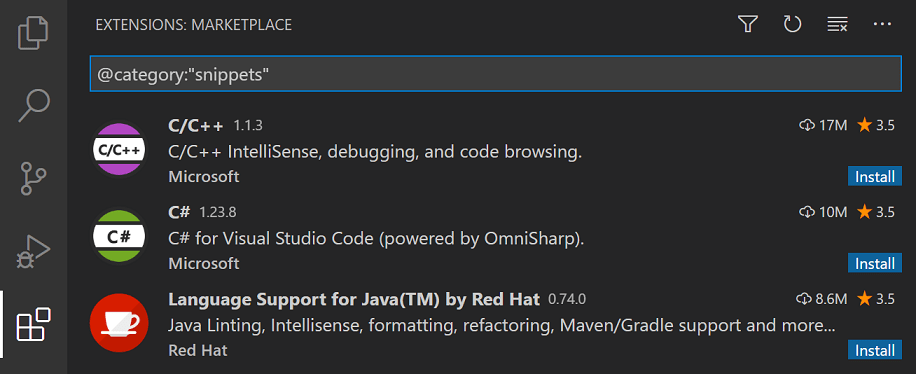

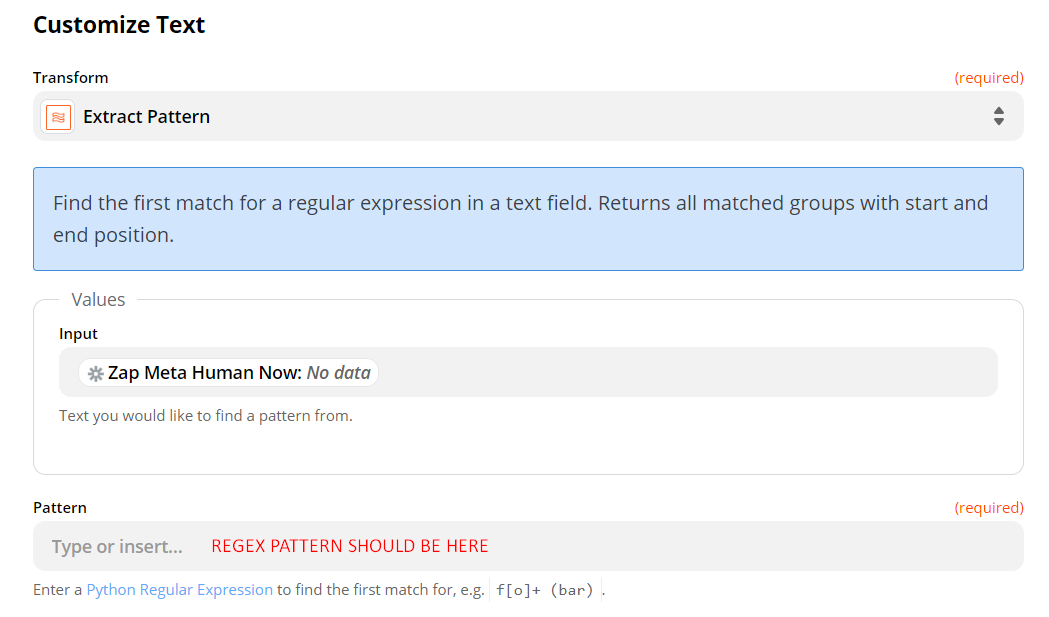

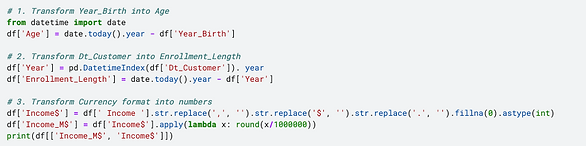
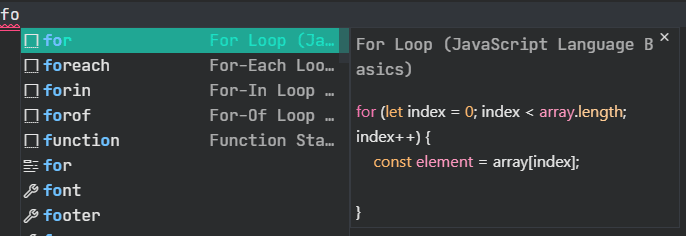
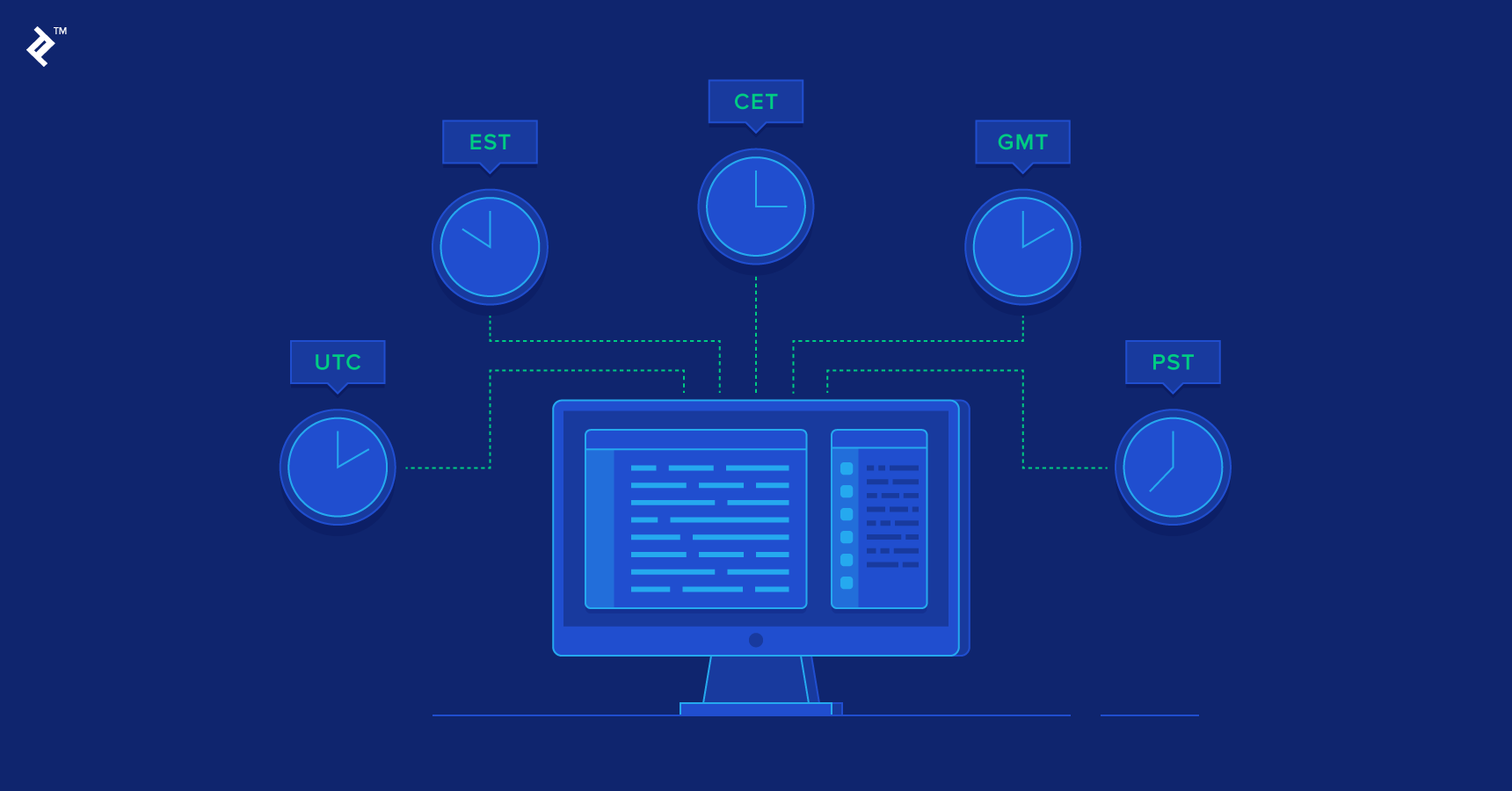
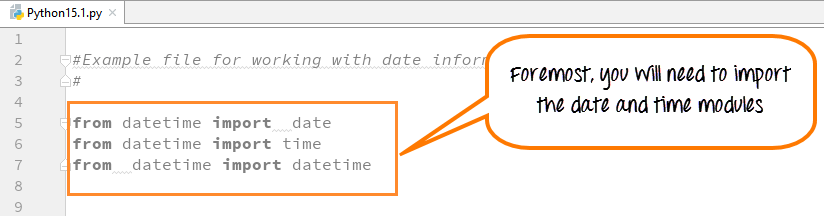
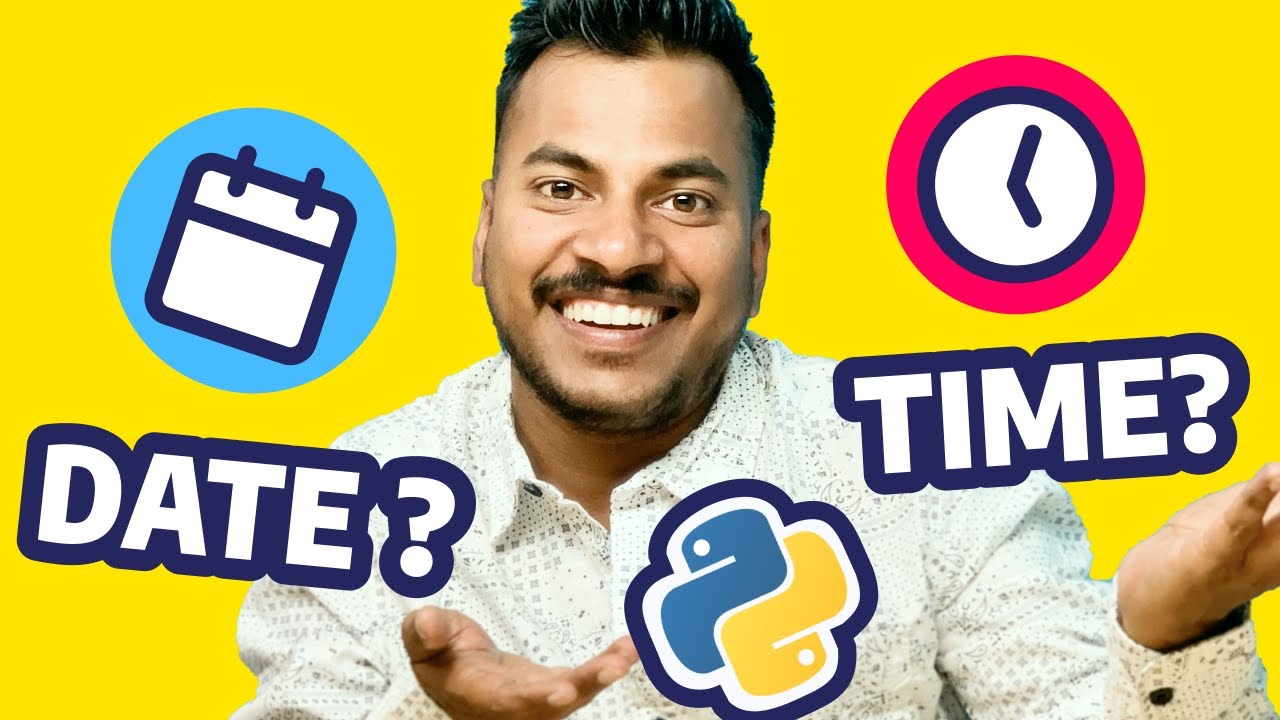
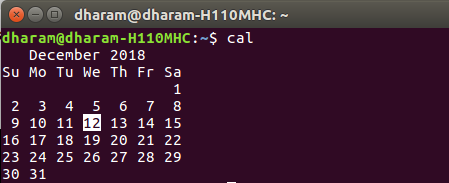

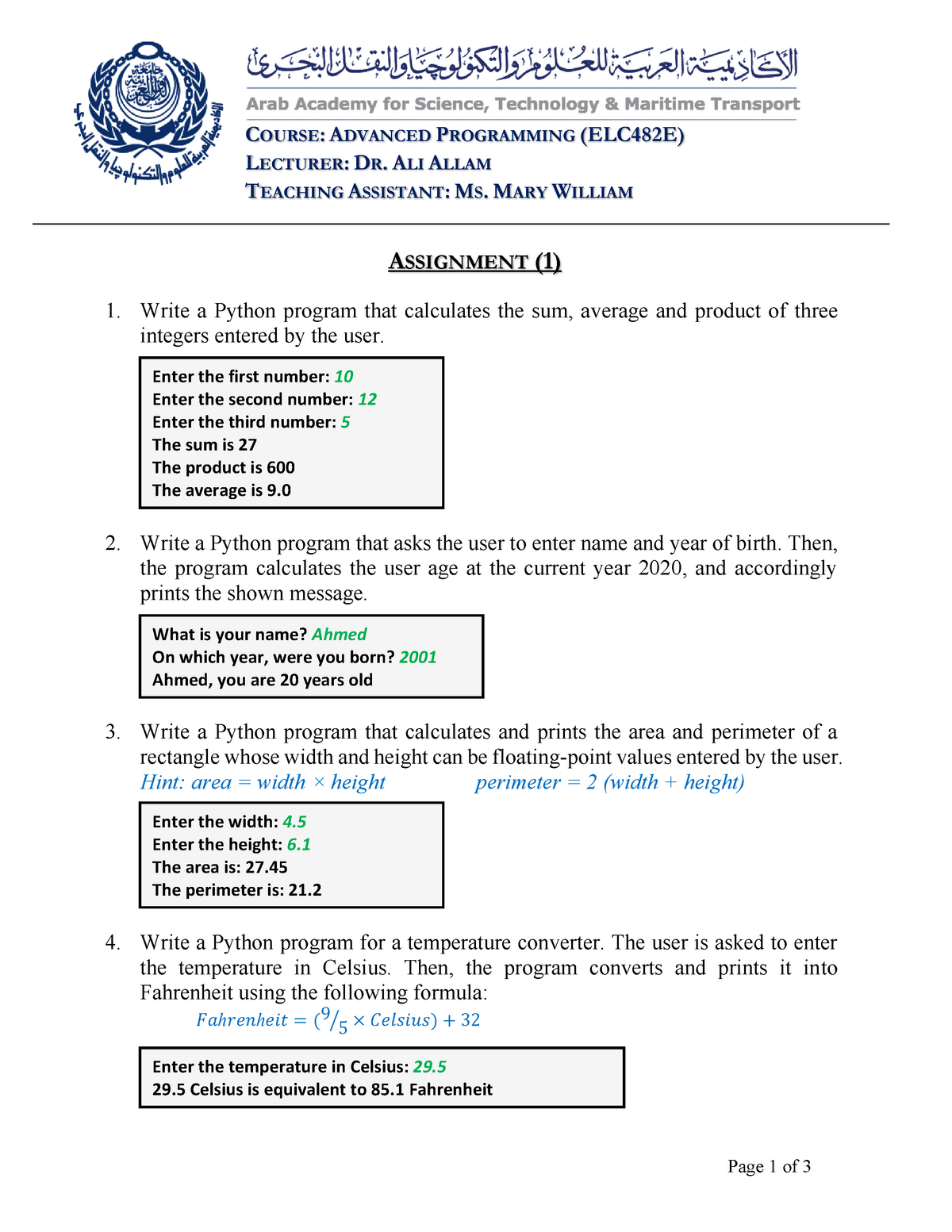
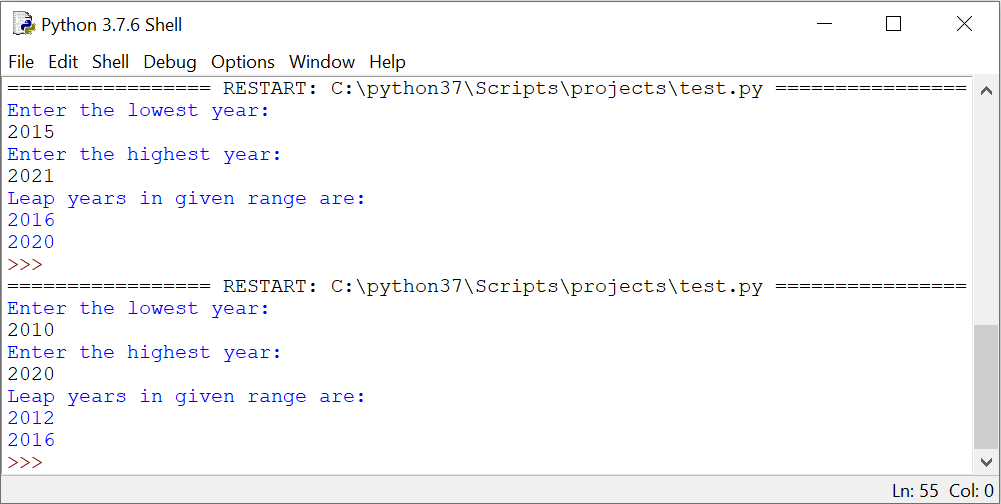
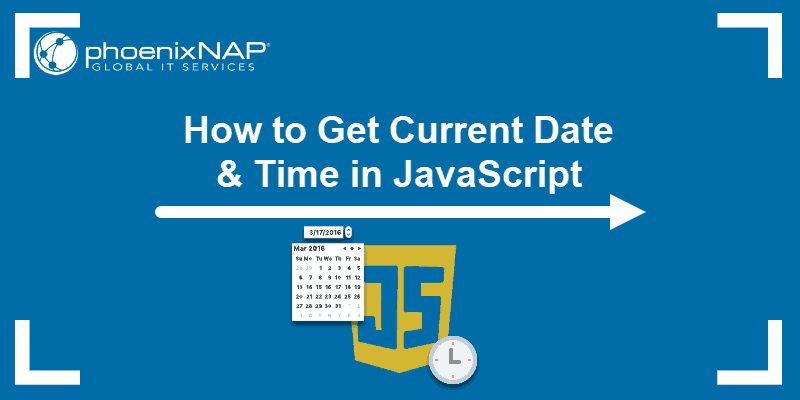

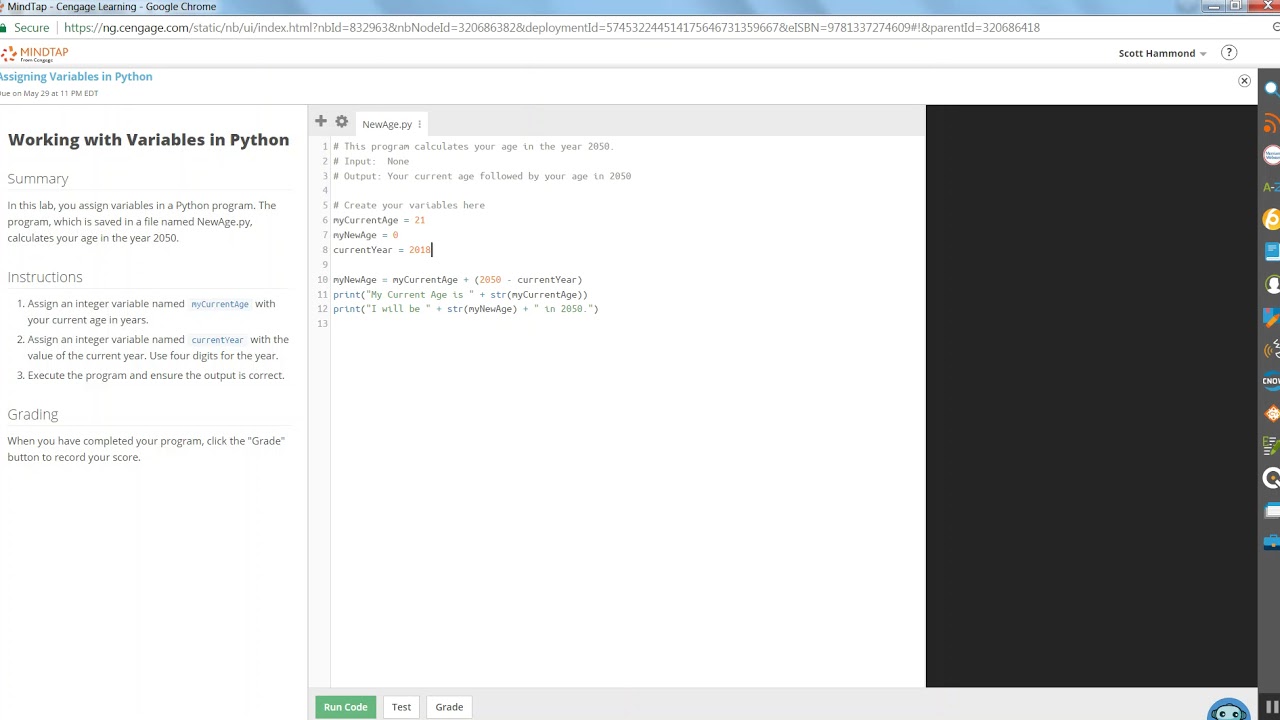
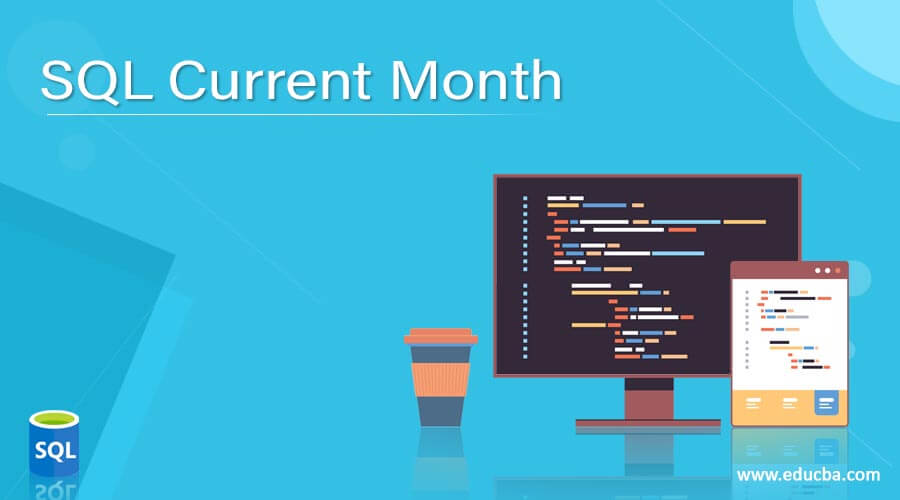
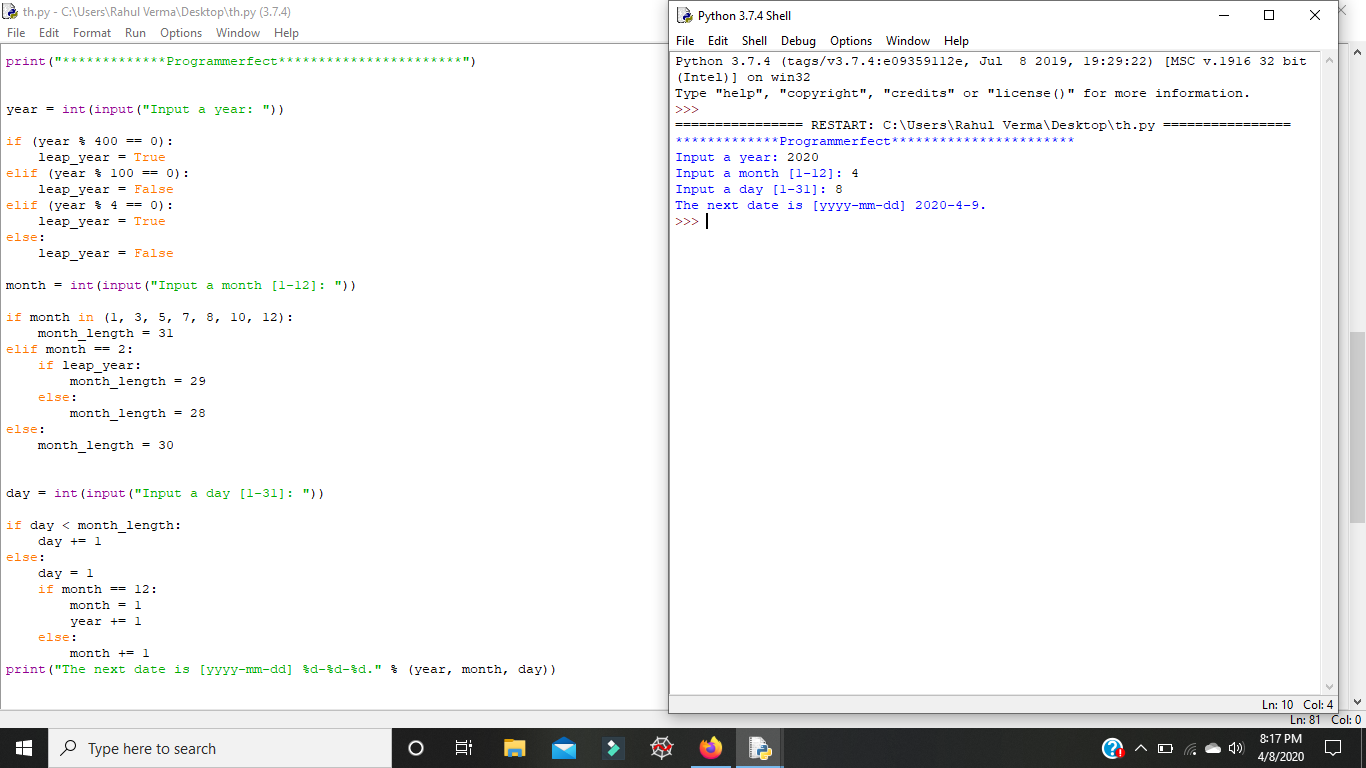
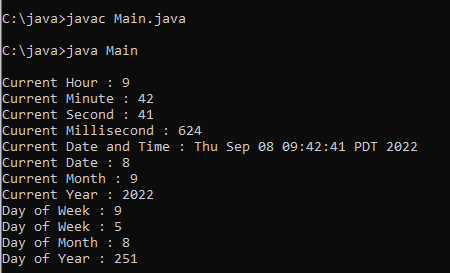


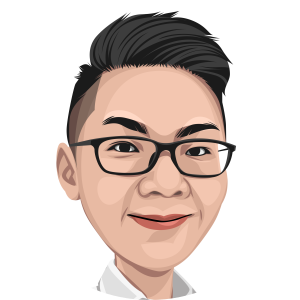



Article link: python get current year.
Learn more about the topic python get current year.
- Datetime current year and month in Python – Stack Overflow
- Get the Current Year in Python (With Examples) – FavTutor
- How to get a current year in Python – Reactgo
- Obtaining the Current Year and Month in Python – AskPython
- How to Get Current Year in Python 3 – e Learning
- How to get the current year in Python – sebhastian
- Python program to print current year, month and day
- How to Get Current Year in Python? – ItSolutionStuff.com
- Python Get Current Year Example – NiceSnippets
- Get the Current Year in Python | Delft Stack
See more: nhanvietluanvan.com/luat-hoc