Python Dictionary Check If Key Exists
Overview of dictionaries in Python
In Python, dictionaries are a powerful data structure that allow you to store and retrieve data in key-value pairs. Dictionary objects are mutable, meaning you can modify them after they are created.
How to define a dictionary in Python
To define a dictionary in Python, you can use curly braces {} and separate the keys and values with a colon (:). Here’s an example:
“`
my_dict = {“key1”: “value1”, “key2”: “value2”, “key3”: “value3″}
“`
You can also use the `dict()` constructor to create a dictionary, like this:
“`
my_dict = dict(key1=”value1″, key2=”value2″, key3=”value3”)
“`
Accessing values in a dictionary
There are multiple ways to access values in a dictionary.
Accessing values using keys
You can access values by providing the key inside square brackets [], like this:
“`
value = my_dict[“key1”]
“`
If the key doesn’t exist in the dictionary, a KeyError will be raised. Therefore, it’s important to check if a key exists before accessing it.
Accessing values using dictionary methods
Python provides several dictionary methods that can be used to access values. The commonly used methods include `get()`, `keys()`, and `values()`.
The `get()` method allows you to retrieve the value associated with a key. If the key doesn’t exist, it returns None (or a default value specified as the second argument).
“`
value = my_dict.get(“key1”)
“`
Checking if a key exists in a dictionary
There are multiple ways to check if a key exists in a dictionary.
Using the ‘in’ operator
The `in` operator can be used to check if a key exists in a dictionary. It returns a boolean value (True or False).
“`
if “key1” in my_dict:
print(“Key exists in the dictionary”)
else:
print(“Key does not exist in the dictionary”)
“`
Using the ‘get()’ method
The `get()` method can also be used to check if a key exists in a dictionary. If the key exists, it returns the associated value. If the key does not exist, it returns None (or a default value specified as the second argument).
“`
value = my_dict.get(“key1”)
if value is not None:
print(“Key exists in the dictionary”)
else:
print(“Key does not exist in the dictionary”)
“`
Using exception handling
You can also use exception handling to check if a key exists in a dictionary. By using a try-except block, you can catch the KeyError that is raised when the key doesn’t exist in the dictionary.
“`
try:
value = my_dict[“key1”]
print(“Key exists in the dictionary”)
except KeyError:
print(“Key does not exist in the dictionary”)
“`
Examples of checking if a key exists in a dictionary
Checking for existing keys
“`
if “key1” in my_dict:
print(“Key1 exists”)
else:
print(“Key1 does not exist”)
if “key2” in my_dict:
print(“Key2 exists”)
else:
print(“Key2 does not exist”)
“`
Handling non-existent keys
“`
value = my_dict.get(“nonexistent_key”)
if value is not None:
print(“Nonexistent_key exists”)
else:
print(“Nonexistent_key does not exist”)
“`
Advanced techniques for key existence checking
Using the ‘setdefault()’ method
The `setdefault()` method can be used to check if a key exists and set a default value if it doesn’t. This method returns the value associated with the key if it exists, or sets the default value and returns it.
“`
value = my_dict.setdefault(“key1”, “default_value”)
“`
Using the ‘keys()’ method
The `keys()` method returns a view object that contains all the keys in the dictionary. You can use the `in` operator to check if a specific key exists.
“`
if “key1” in my_dict.keys():
print(“Key1 exists”)
else:
print(“Key1 does not exist”)
“`
Using the ‘in’ operator with dictionary methods
The `in` operator can also be used with other dictionary methods like `values()` and `items()` to check if a value or a key-value pair exists in the dictionary.
“`
if “value1” in my_dict.values():
print(“Value1 exists”)
else:
print(“Value1 does not exist”)
if (“key1”, “value1”) in my_dict.items():
print(“Key-value pair exists”)
else:
print(“Key-value pair does not exist”)
“`
Best practices and tips for working with dictionaries
Using descriptive keys
When defining keys for your dictionary, it’s best to use descriptive names that explain their purpose. This improves the readability and maintainability of your code.
Avoiding KeyError exceptions
To avoid KeyError exceptions when accessing dictionary values, you can use the `get()` method with a default value.
“`
value = my_dict.get(“nonexistent_key”, “default_value”)
“`
Efficiently iterating through dictionary keys
To iterate through all the keys in a dictionary, you can use a for loop. This will give you access to each key, and you can then retrieve the associated value.
“`
for key in my_dict:
value = my_dict[key]
print(key, value)
“`
Conclusion
In this article, we discussed various methods to check if a key exists in a Python dictionary. We learned about using the ‘in’ operator, the ‘get()’ method, and exception handling. We also explored advanced techniques such as using the ‘setdefault()’ and ‘keys()’ methods. Lastly, we provided some best practices and tips for working with dictionaries.
FAQs
Q: How do I check if a key does not exist in a dictionary in Python?
A: You can use the ‘in’ operator to check if a key exists in a dictionary and negate the result using the ‘not’ keyword. For example, `if “key” not in my_dict:`.
Q: How do I check if multiple keys exist in a dictionary?
A: You can use the ‘all()’ function along with a generator expression to check if multiple keys exist in a dictionary. For example, `if all(key in my_dict for key in keys_list):`.
Q: Can I use the ‘in’ operator with arrays to check if a key exists?
A: No, the ‘in’ operator is used specifically for checking if a key exists in a dictionary. To check if an element exists in a list or array, you can use the ‘in’ operator or the ‘index()’ method.
Q: How do I add a value to a key in a dictionary in Python?
A: You can assign a value to a key in a dictionary using the assignment operator `=`. For example, `my_dict[“key”] = “value”`.
Q: How do I iterate through key-value pairs in a dictionary?
A: You can use a for loop with the `items()` method to iterate through all the key-value pairs in a dictionary.
How To Check If A Key Exists In A Python Dictionary | Python Beginner Tutorial
Keywords searched by users: python dictionary check if key exists Check if key not exists in dictionary Python, Python check key in array, Check value in dictionary Python, Add value to key dictionary Python, For key-value in dict Python, Find element in dictionary Python, Python list key-value, Set value dictionary python
Categories: Top 39 Python Dictionary Check If Key Exists
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
Python is a versatile programming language utilized by developers for its simplicity and readability. One of its powerful data structures is the dictionary. A dictionary is a collection of key-value pairs, where each key is unique and associated with a specific value. In Python, dictionaries are implemented with curly brackets and colons to separate keys and values.
When working with dictionaries in Python, it is essential to check if a key exists before accessing its corresponding value. This is necessary because attempting to retrieve a non-existent key will result in a KeyError, an exception that halts program execution. To avoid this, we need to employ a method to determine if a key exists in a dictionary. However, there may be situations where we want to check if a key does not exist. In this article, we will explore various approaches to check if a key does not exist in a dictionary using Python.
1. The ‘in’ Operator
The simplest way to check if a key does not exist in a dictionary is by utilizing the ‘in’ operator. The ‘in’ operator returns a boolean value (‘True’ or ‘False’) based on whether the key is present in the dictionary or not. By negating this boolean value using the ‘not’ operator, we can effectively check if a key does not exist.
Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘gender’: ‘Male’}
if ‘address’ not in my_dict:
print(“Key does not exist in the dictionary.”)
“`
In this example, we check if the key ‘address’ does not exist in the dictionary ‘my_dict’. Since ‘address’ is not a key in the dictionary, the if condition evaluates to ‘True’, and the corresponding print statement is executed.
2. The get() Method
Another way to check if a key does not exist in a dictionary is by using the get() method. The get() method retrieves the value of a specified key from a dictionary. However, it can also return a default value if the key is not found in the dictionary. By providing a default value that signifies the key does not exist, we can indirectly check if the key is absent.
Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘gender’: ‘Male’}
address = my_dict.get(‘address’, ‘Key does not exist in the dictionary.’)
print(address)
“`
In this example, we use the get() method to retrieve the value associated with the key ‘address’ from the dictionary ‘my_dict’. Since ‘address’ is not a key in the dictionary, the method returns the default value ‘Key does not exist in the dictionary.’, which is then assigned to the variable ‘address’.
3. The KeyError Exception Handling
One method to check if a key does not exist in a dictionary is by handling the KeyError exception that occurs when attempting to access a non-existent key. By wrapping the key retrieval statement in a try-except block, we can catch the KeyError and perform specific actions if the key is not found.
Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘gender’: ‘Male’}
try:
address = my_dict[‘address’]
except KeyError:
print(“Key does not exist in the dictionary.”)
“`
In this example, we try to retrieve the value associated with the key ‘address’ from the dictionary ‘my_dict’. If the key does not exist, a KeyError will be raised, triggering the except block and printing the corresponding message.
FAQs:
Q1. What happens if we try to access a non-existent key directly without any checks?
A1. If we try to access a non-existent key directly without any checks, Python raises a KeyError exception, halting program execution.
Q2. Which method is the most efficient for checking if a key does not exist in a dictionary?
A2. The ‘in’ operator is the most efficient method to check if a key does not exist in a dictionary, as it directly returns a boolean value without any unnecessary overhead.
Q3. Can we modify the default value returned by the get() method when a key does not exist?
A3. Yes, the get() method allows us to modify the default value returned by providing a desired value as the second argument. This value is returned if the key is not present.
Q4. How can we perform different actions based on the absence of a key in a dictionary?
A4. By combining the above methods with if-else statements or using the KeyError exception handling approach, we can perform different actions based on whether the key does or does not exist in the dictionary.
In conclusion, checking if a key does not exist in a dictionary is an essential aspect of working with dictionaries in Python. By utilizing methods such as the ‘in’ operator, the get() method, or exception handling, developers can effectively handle scenarios where a key might not be present. It is crucial to handle these cases to prevent potential errors and ensure smooth program execution.
Python Check Key In Array
Python is a versatile programming language known for its simplicity and powerful features. One common task programmers often face is checking whether a specific key exists within an array or dictionary. In this article, we will explore various techniques to accomplish this task efficiently. Additionally, we will address some frequently asked questions to clarify any doubts or misconceptions related to this topic.
Understanding Arrays and Dictionaries in Python:
Before delving into checking keys in an array, it is crucial to understand the basic concepts of arrays and dictionaries in Python.
1. Arrays:
In Python, arrays are homogenous data structures that can store elements of the same type. They are defined using the `array` module and provide a fast and efficient way to store and manipulate large sets of numerical data.
2. Dictionaries:
Dictionaries, on the other hand, are versatile data structures that store data as key-value pairs. Unlike arrays, dictionaries allow any immutable data type as keys and any data type as values. They provide an efficient way to access and modify data based on the keys, making them suitable for various applications.
Checking Keys in Arrays:
Python arrays, being homogenous, do not support direct key-value checking like dictionaries. However, we can achieve similar functionality by converting the array into a dictionary using the `enumerate()` function. Here’s an example:
“`python
my_array = [‘apple’, ‘banana’, ‘cherry’]
my_dict = {k: True for k in my_array}
if ‘banana’ in my_dict:
print(“Key exists in the array.”)
“`
In this example, we convert the `my_array` into a dictionary `my_dict` using a dictionary comprehension. The `k: True` assignment ensures that each element of `my_array` becomes a key in the dictionary. Finally, we check if the key ‘banana’ exists in the dictionary using the `in` operator.
Checking Keys in Dictionaries:
Checking whether a key exists in a Python dictionary is straightforward. We can utilize the `in` operator to achieve this. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if ‘banana’ in my_dict:
print(“Key exists in the dictionary.”)
“`
In this example, we directly check if the key ‘banana’ exists in the `my_dict` dictionary using the `in` operator. If the key is present, the message “Key exists in the dictionary” is printed.
However, it is important to note that direct key checking in dictionaries does not raise an error if the key does not exist. If you want to handle the situation differently, you can use the `get()` method or a `try-except` block.
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if my_dict.get(‘orange’):
print(“Key exists in the dictionary.”)
else:
print(“Key does not exist in the dictionary.”)
“`
In this example, we use the `get()` method to retrieve the value for the key ‘orange’. If the key does not exist, the `get()` method returns `None`, which evaluates to `False` in the `if` statement.
Frequently Asked Questions:
Q1. Can I check multiple keys in a single condition?
Yes, in both arrays and dictionaries, you can check multiple keys using logical operators such as `or` and `and`.
Example:
“`python
if ‘apple’ in my_dict or ‘banana’ in my_dict:
print(“At least one of the keys exists in the dictionary.”)
“`
Q2. Are these techniques case-sensitive?
Yes, these techniques are case-sensitive. If you have keys like ‘Apple’ and ‘apple’, they will be treated as two separate keys.
Q3. What happens if I try to check a key that doesn’t exist in an array or dictionary?
In arrays, the `in` operator will always return `False` since arrays don’t have keys. In dictionaries, the `in` operator will return `False` if the key doesn’t exist.
Q4. Which method is more efficient for checking keys: `in` operator or `get()` method?
The `in` operator is generally more efficient as it directly checks for the key’s existence. The `get()` method, on the other hand, introduces an extra method call and is useful when you also require the value associated with the key.
In conclusion, checking whether a key exists in a Python array or dictionary can be approached differently. While arrays require conversion into a dictionary for key checking, dictionaries support key checking directly. Understanding these concepts and techniques will help you efficiently handle key-based operations in your Python programs.
Check Value In Dictionary Python
A dictionary in Python is a powerful data structure that stores key-value pairs. It allows efficient retrieval of values based on their associated keys. One common task in working with dictionaries is to check whether a particular value is present in the dictionary or not. In this article, we will explore various methods to check the value in a dictionary in Python.
Method 1: Using the “in” operator
Python provides an “in” operator that can be used to check if a value exists in a dictionary. This operator returns a boolean value, True if the value exists and False otherwise. Here’s an example:
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘John’ in dictionary.values():
print(“Value exists in the dictionary”)
else:
print(“Value doesn’t exist in the dictionary”)
“`
Output:
“`
Value exists in the dictionary
“`
In the above example, we check if the value ‘John’ exists in the dictionary using the “in” operator on the `values()` method of the dictionary. Since the value exists, the condition evaluates to True and the corresponding message is printed.
Method 2: Using a loop
Another way to check the value in a dictionary is by using a loop. We can iterate over the dictionary’s values and compare each value with the desired value. If the value is found, we can set a flag variable to True and break out of the loop. Here’s an example:
“`python
dictionary = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
value_to_check = ‘John’
value_found = False
for value in dictionary.values():
if value == value_to_check:
value_found = True
break
if value_found:
print(“Value exists in the dictionary”)
else:
print(“Value doesn’t exist in the dictionary”)
“`
Output:
“`
Value exists in the dictionary
“`
In the above example, we iterate over the dictionary’s values using a for loop and compare each value with the desired value ‘John’. If the value is found, we set the flag variable `value_found` to True and break out of the loop. Finally, we check the value of `value_found` to determine if the value exists or not.
FAQs:
Q: Are multiple values allowed for a single key in a dictionary?
A: No, dictionaries store unique keys. However, multiple keys can have the same value.
Q: Can we check the presence of a key using the same methods?
A: Yes, the “in” operator and loop methods can also be used to check if a key exists in a dictionary.
Q: What happens if we try to check the presence of a value that doesn’t exist in the dictionary?
A: The “in” operator returns False and the loop method doesn’t set the flag variable, indicating that the value doesn’t exist.
Q: Is there any difference in performance between the “in” operator and the loop method?
A: The “in” operator is generally more efficient as it leverages the internal implementation of dictionary values. The loop method requires iterating over all values and can take longer for large dictionaries.
Q: Can we check the presence of a value without iterating through all the values?
A: No, both methods require checking each value individually. There is no direct method to check the presence without iterating.
In conclusion, checking the value in a dictionary is a common task in Python programming. By using the “in” operator or a loop, we can efficiently determine whether a value exists in a dictionary or not. The choice of method depends on the specific use case and performance requirements.
Images related to the topic python dictionary check if key exists
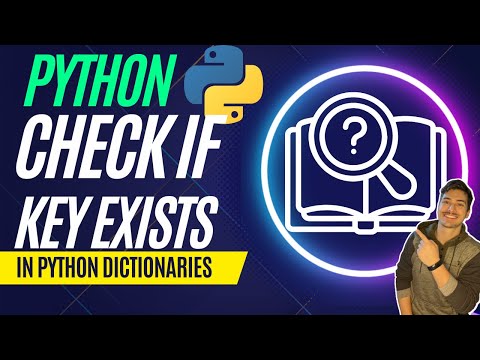
Found 16 images related to python dictionary check if key exists theme


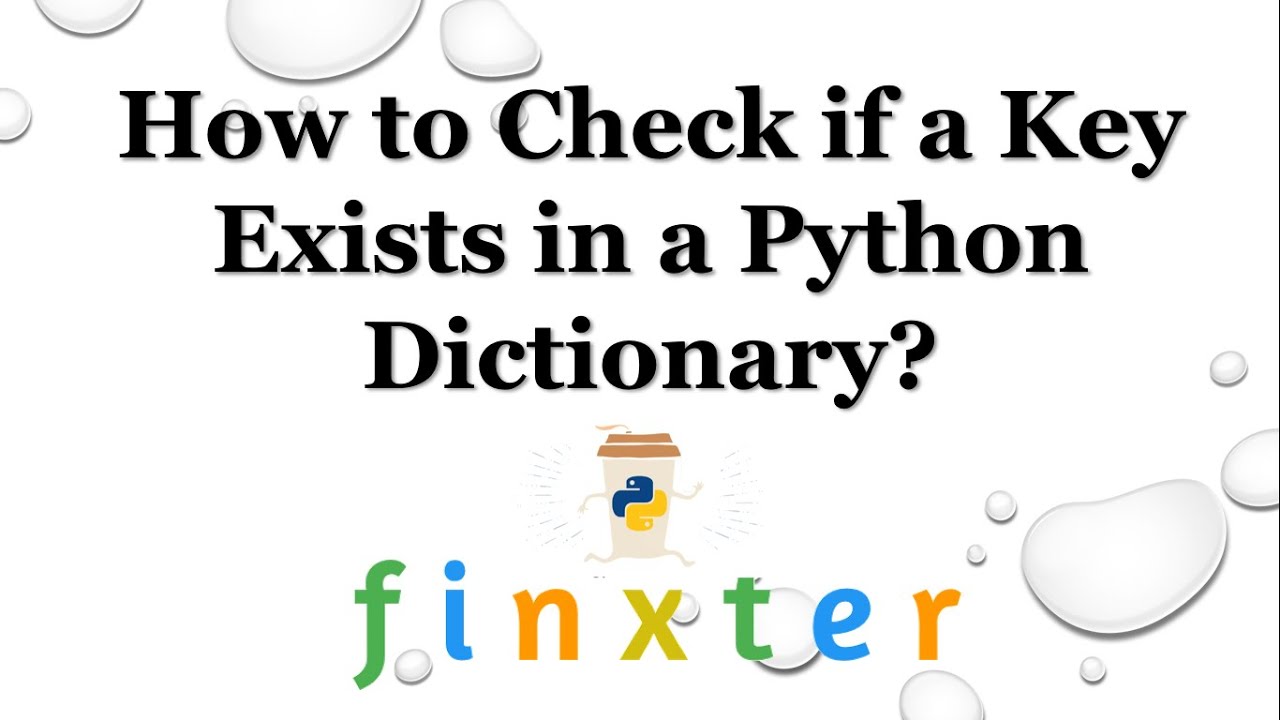
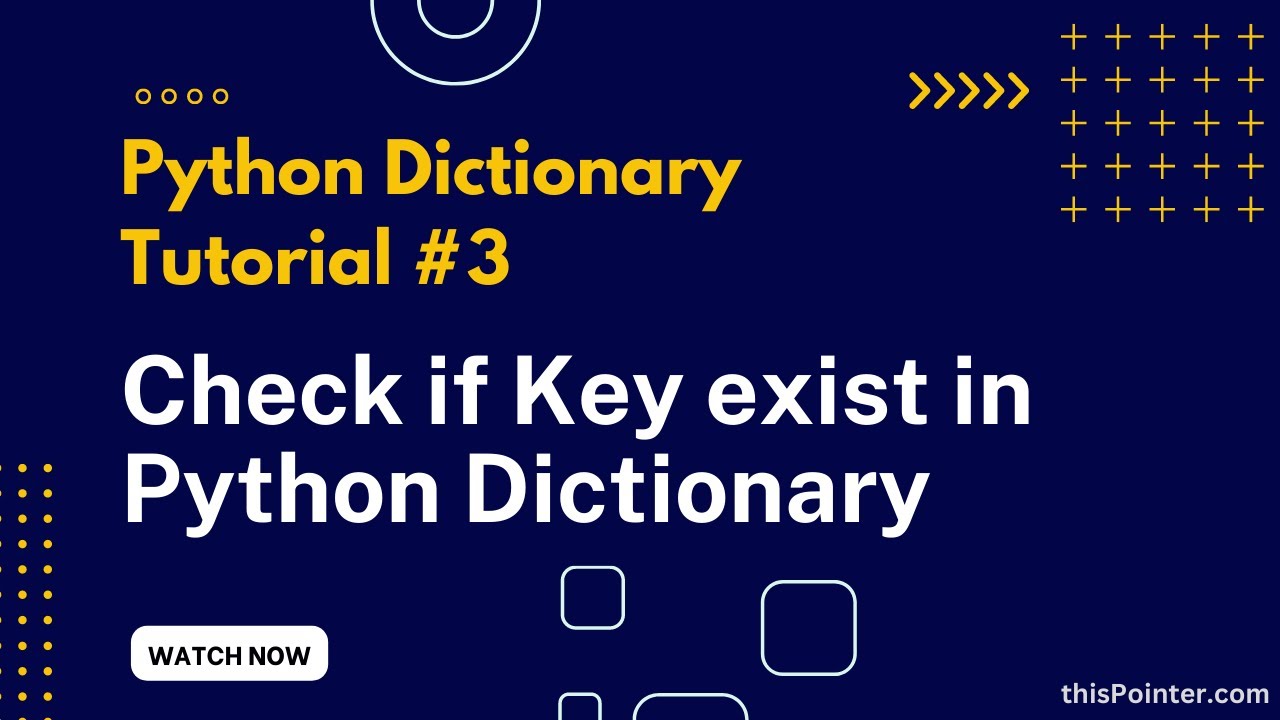

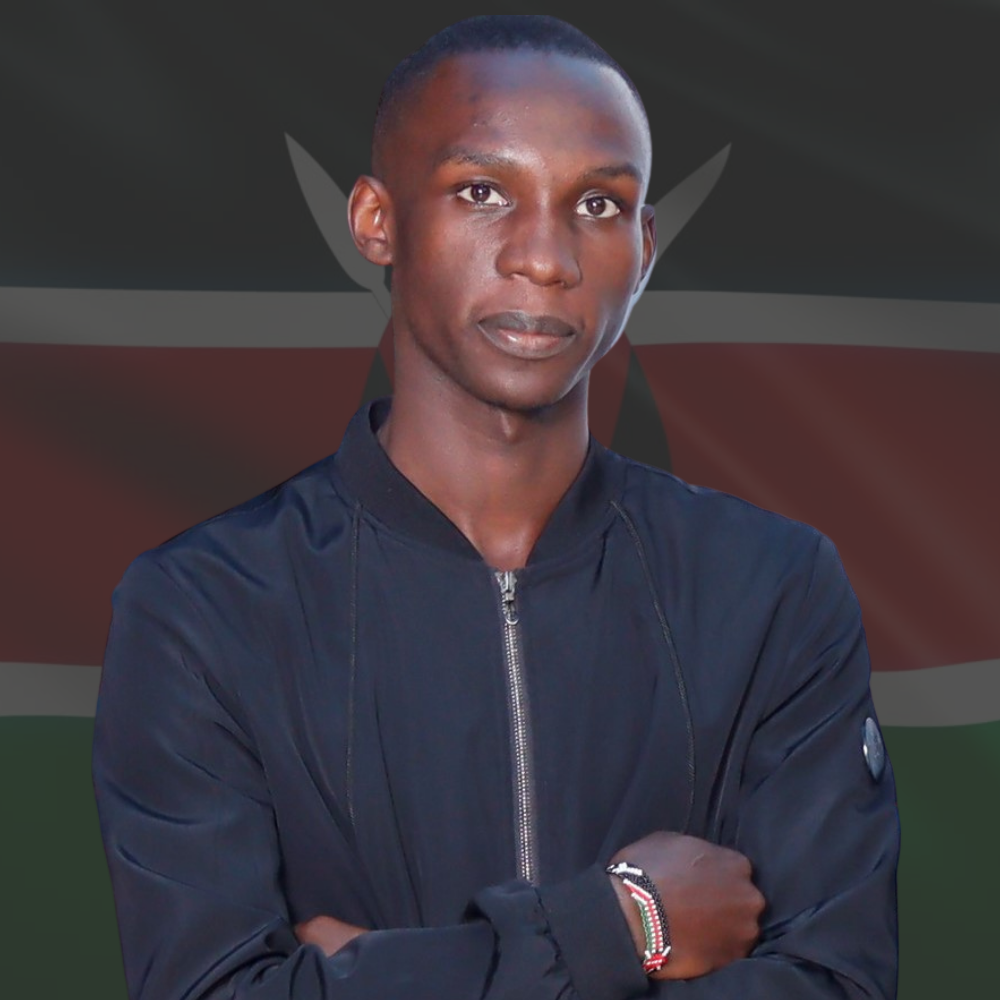
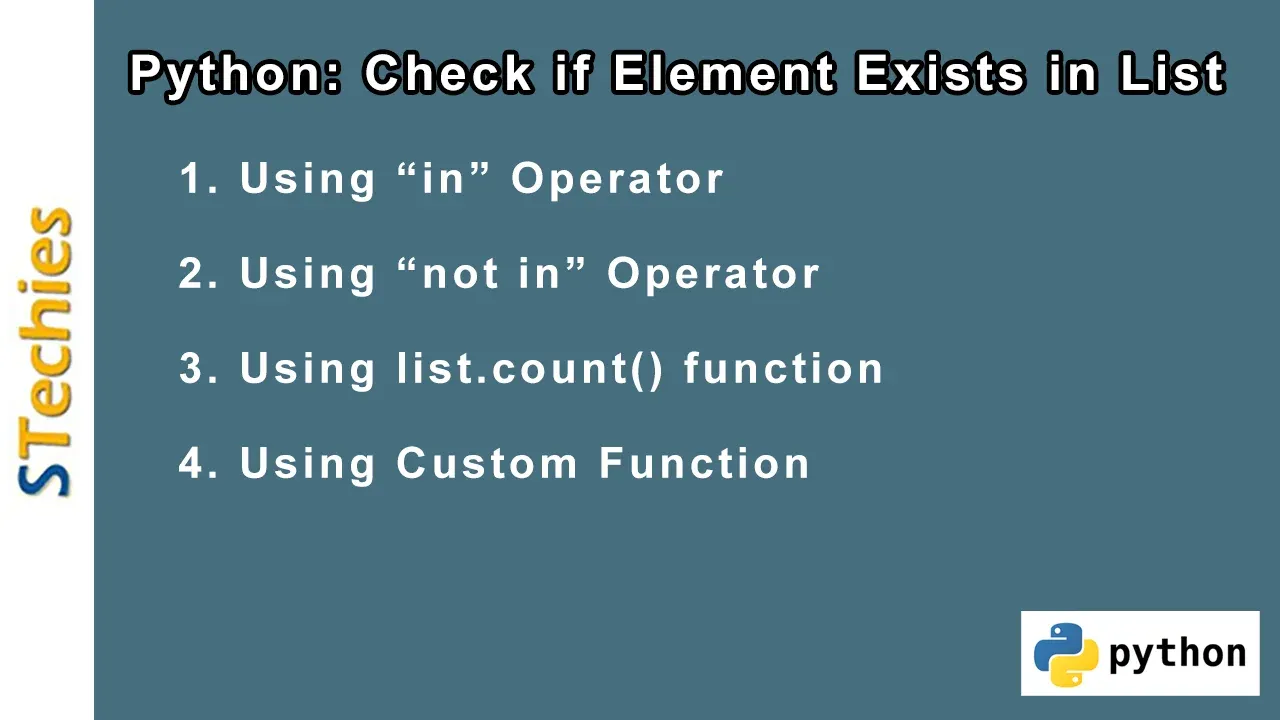
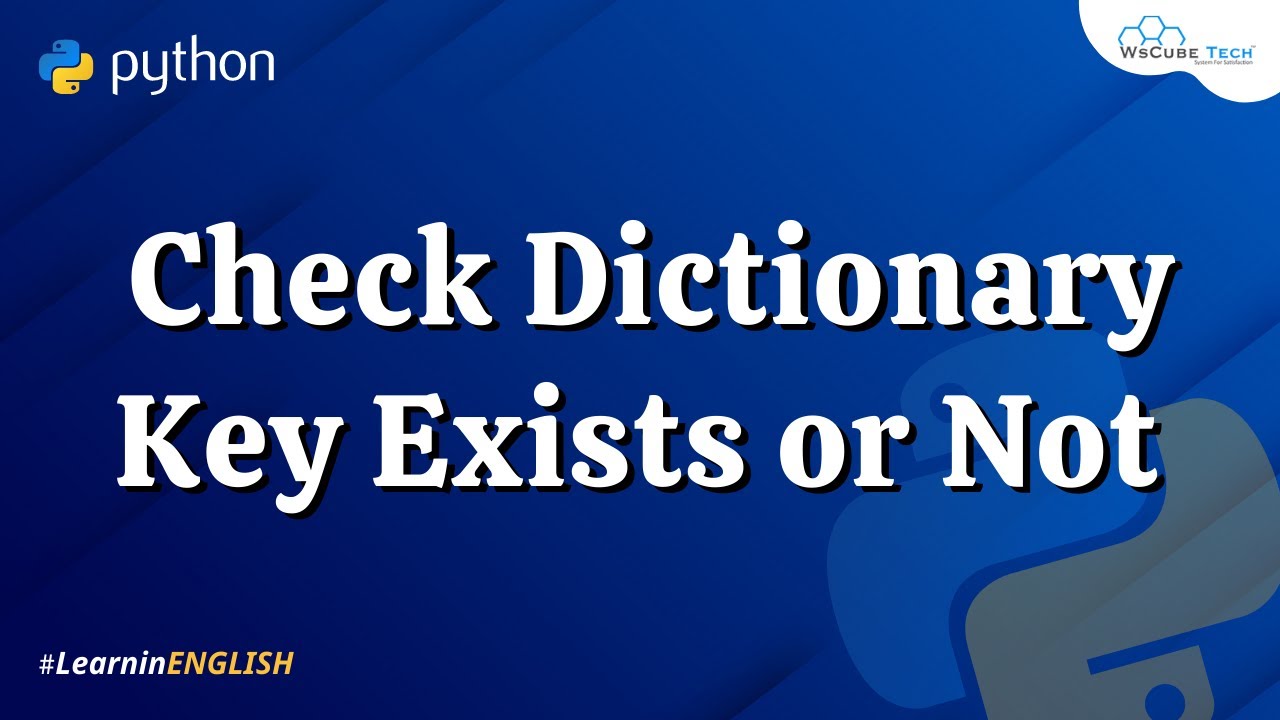
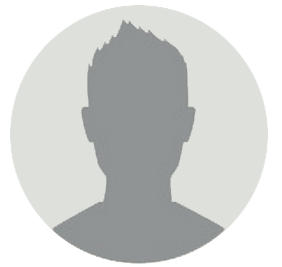
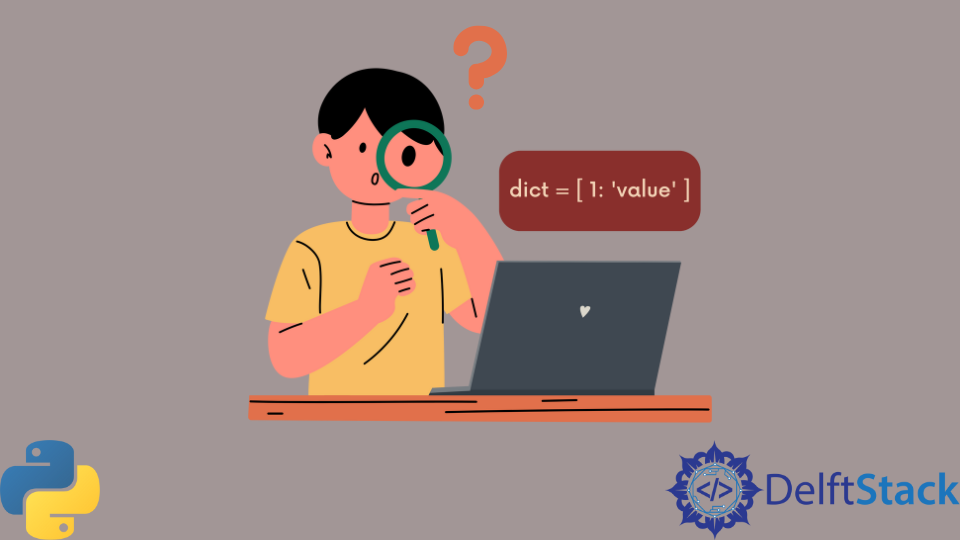
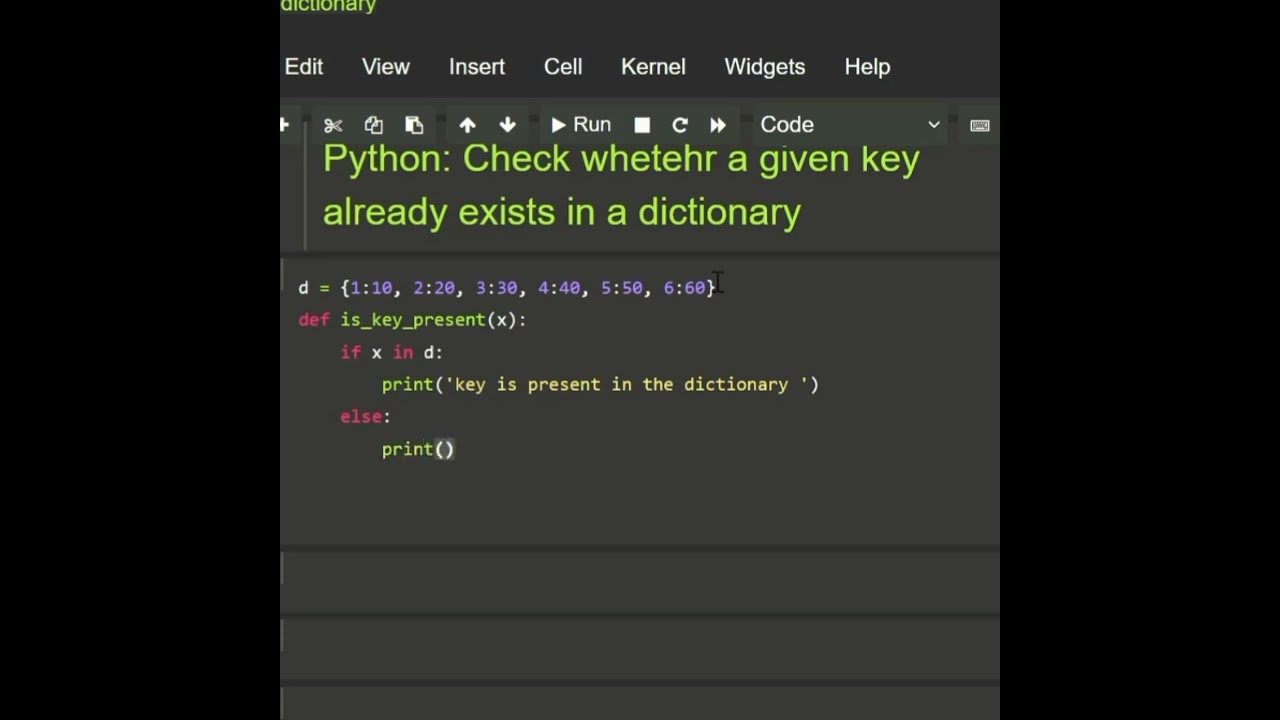

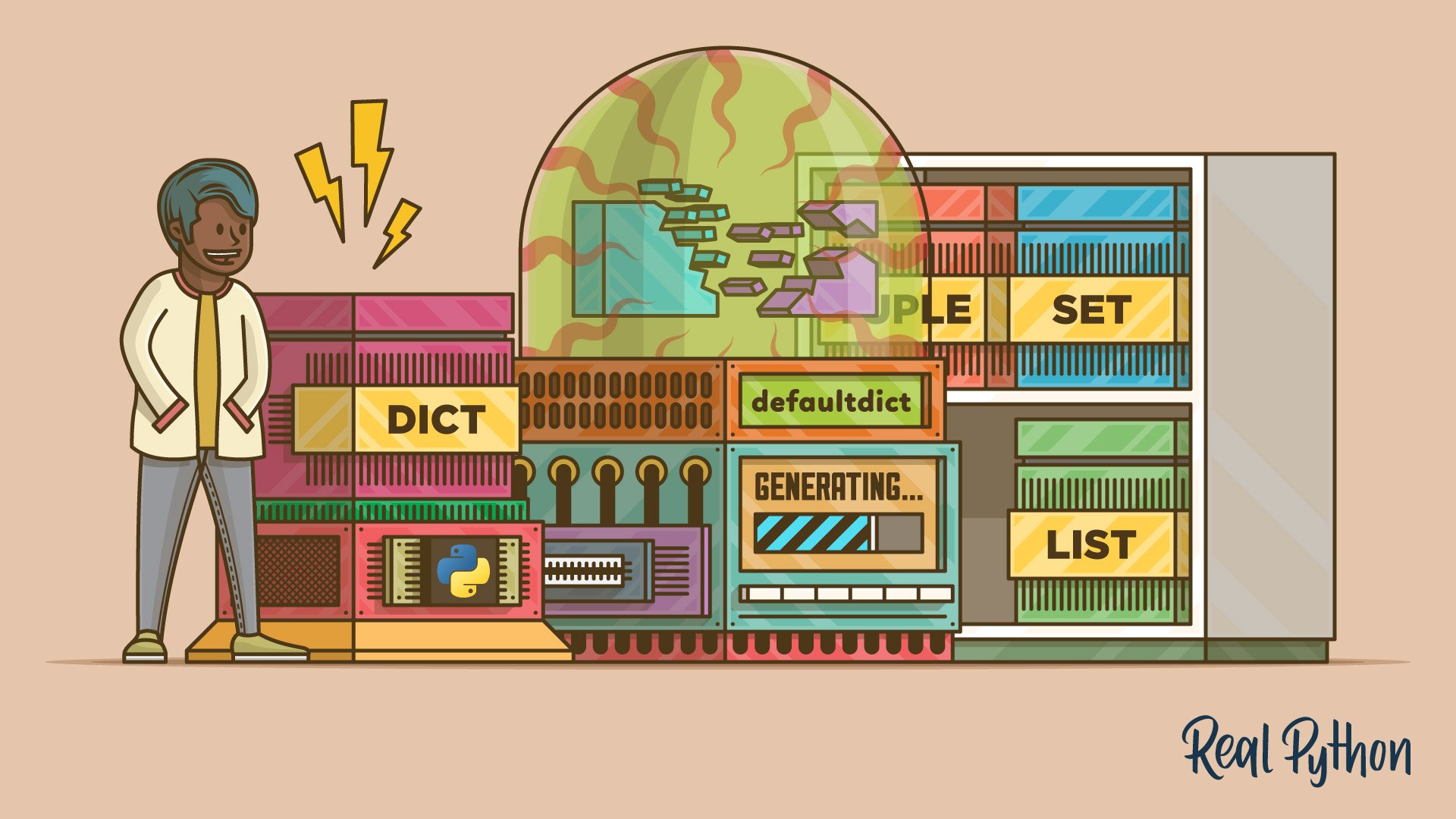

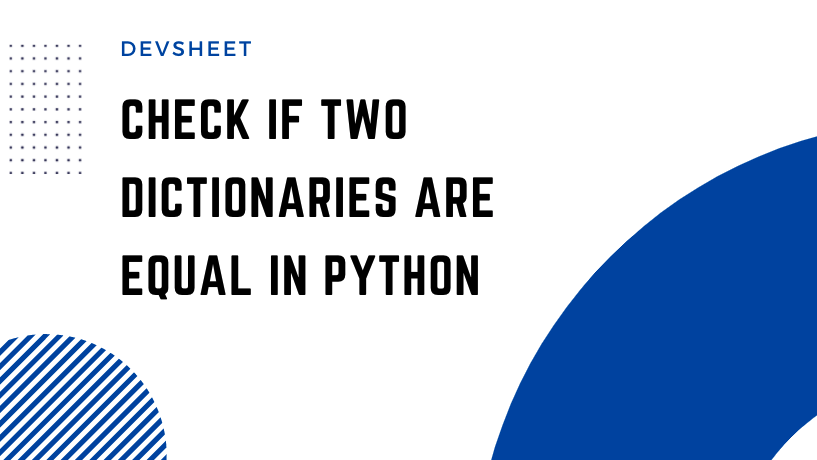
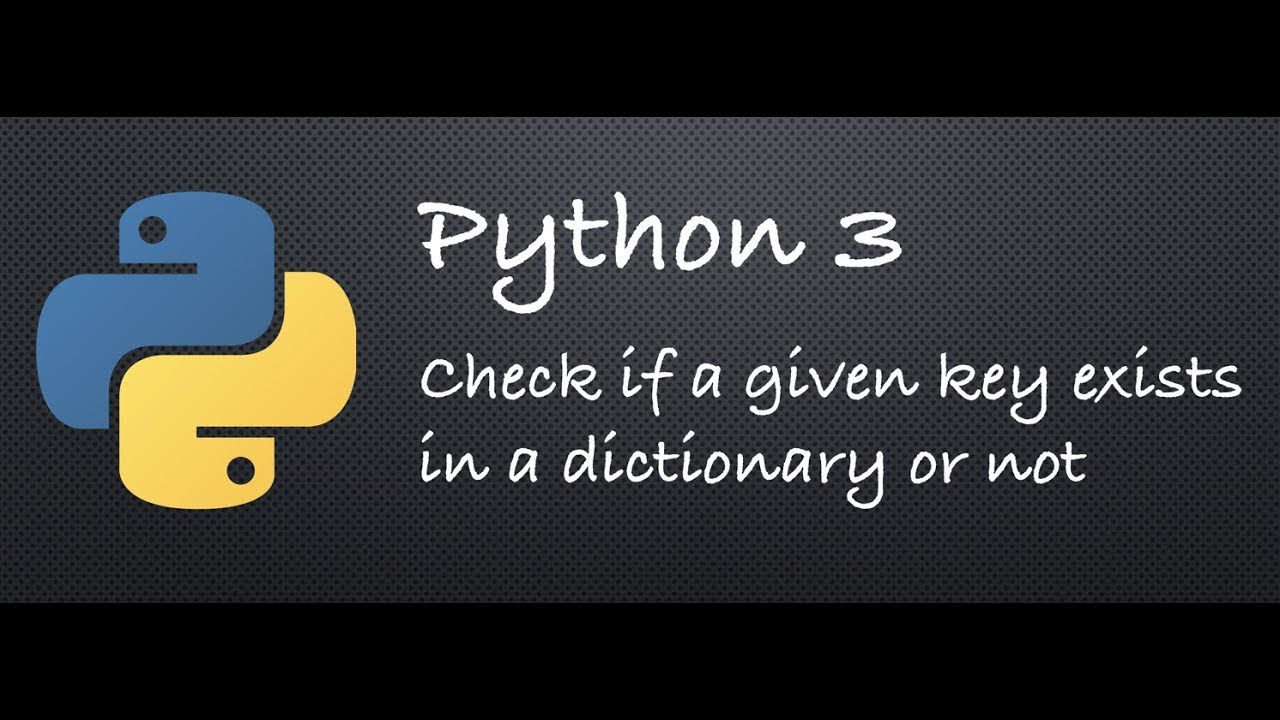
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example3.jpg)
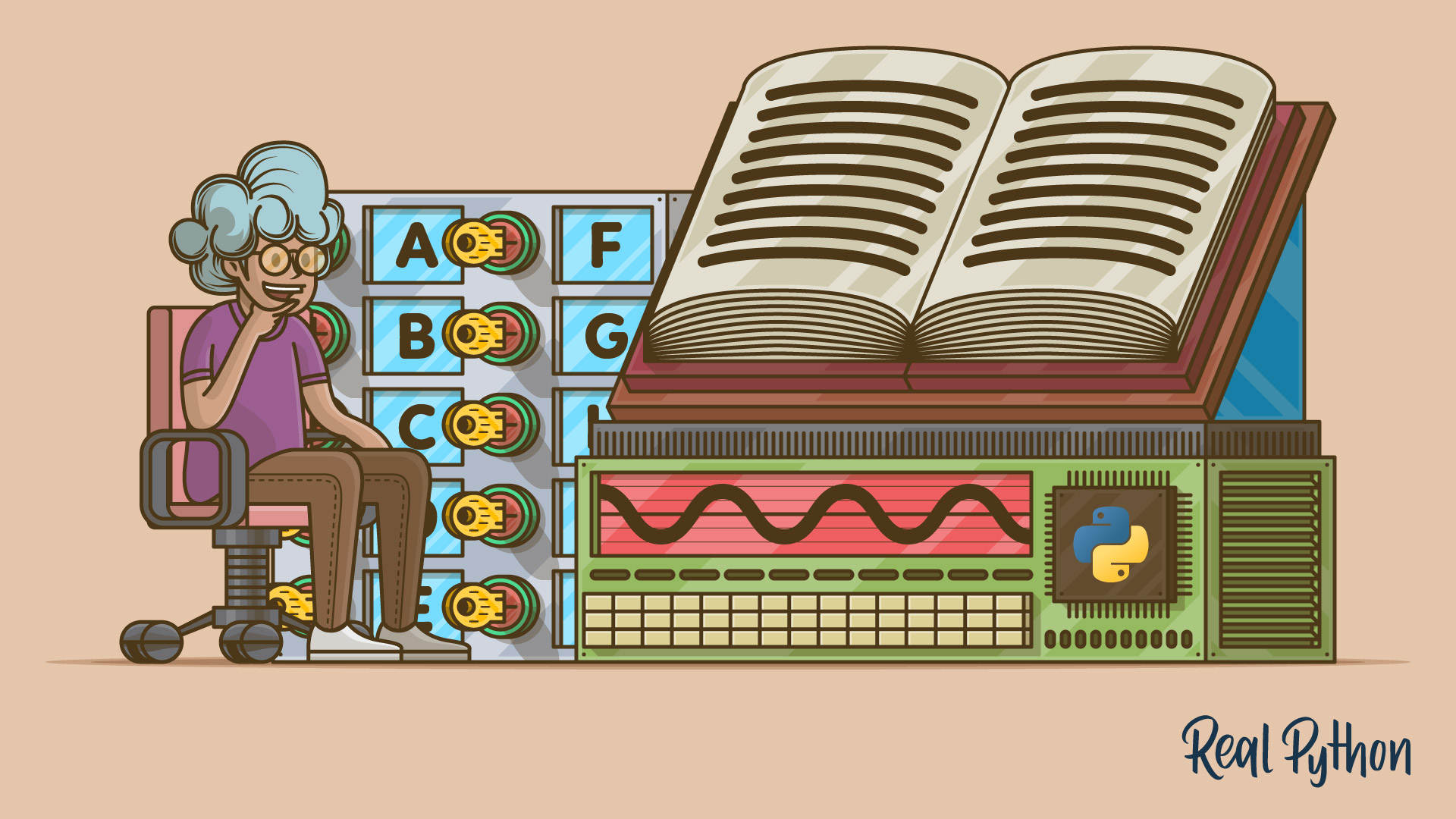
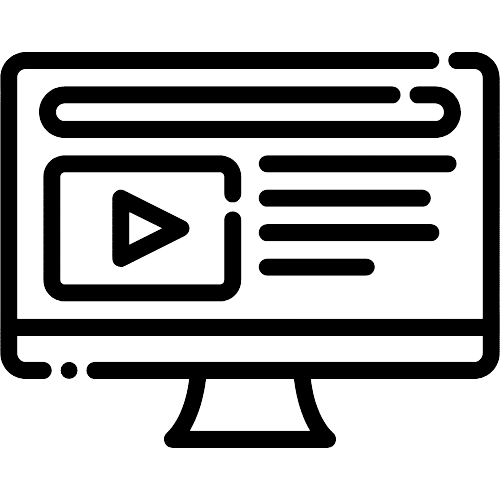
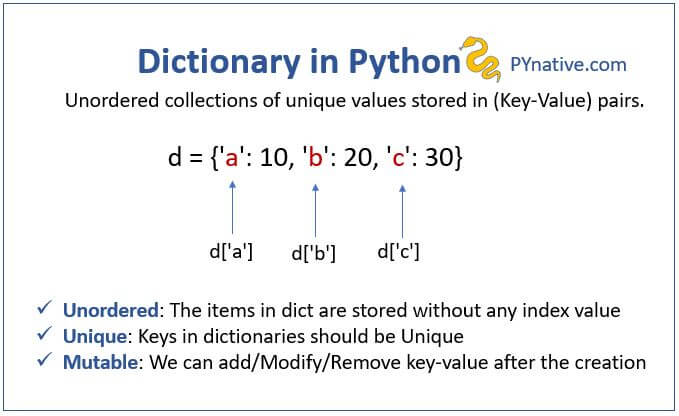
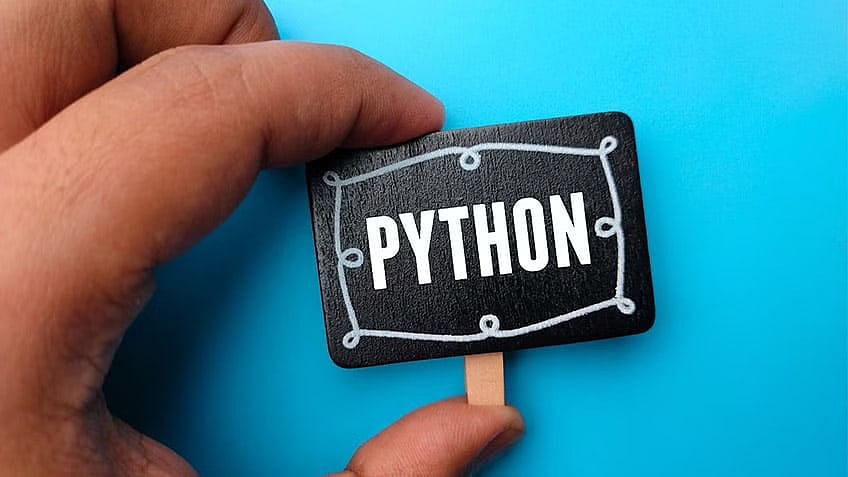


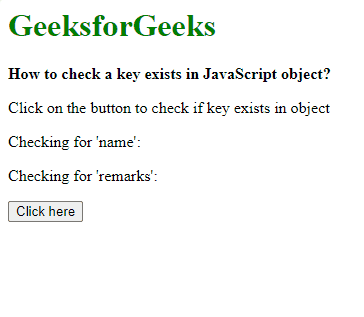
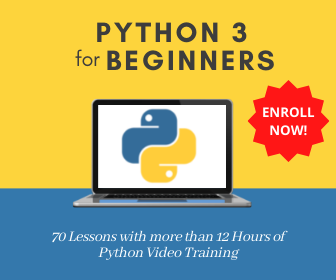
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example2.jpg)
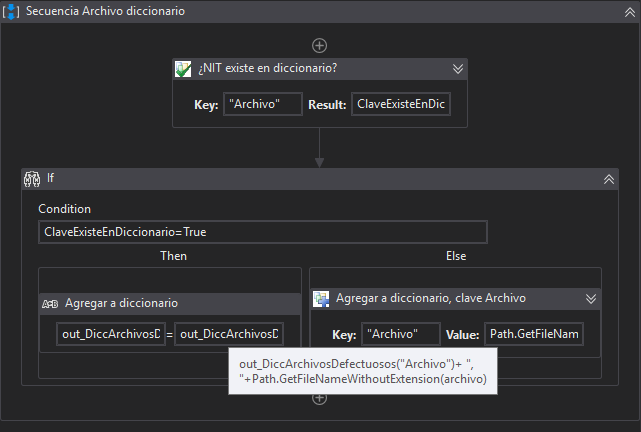
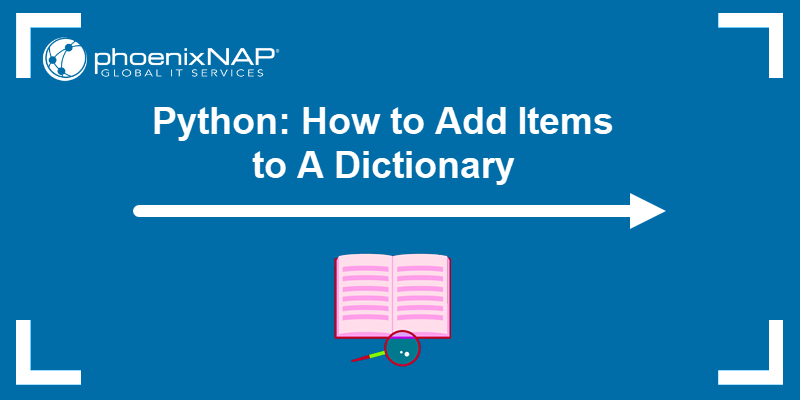


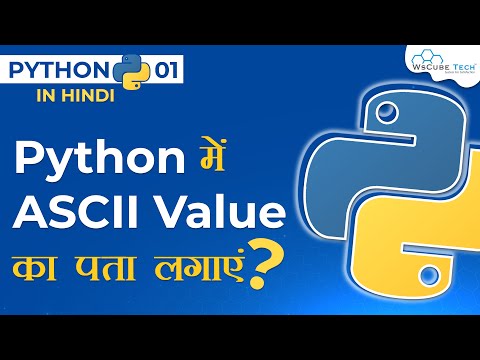


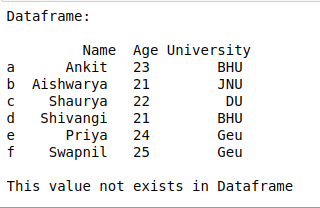
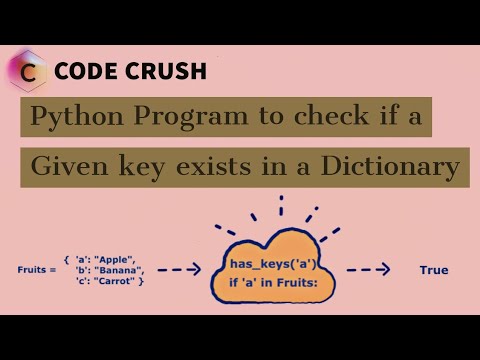
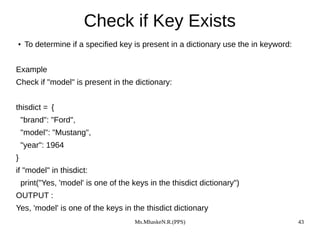

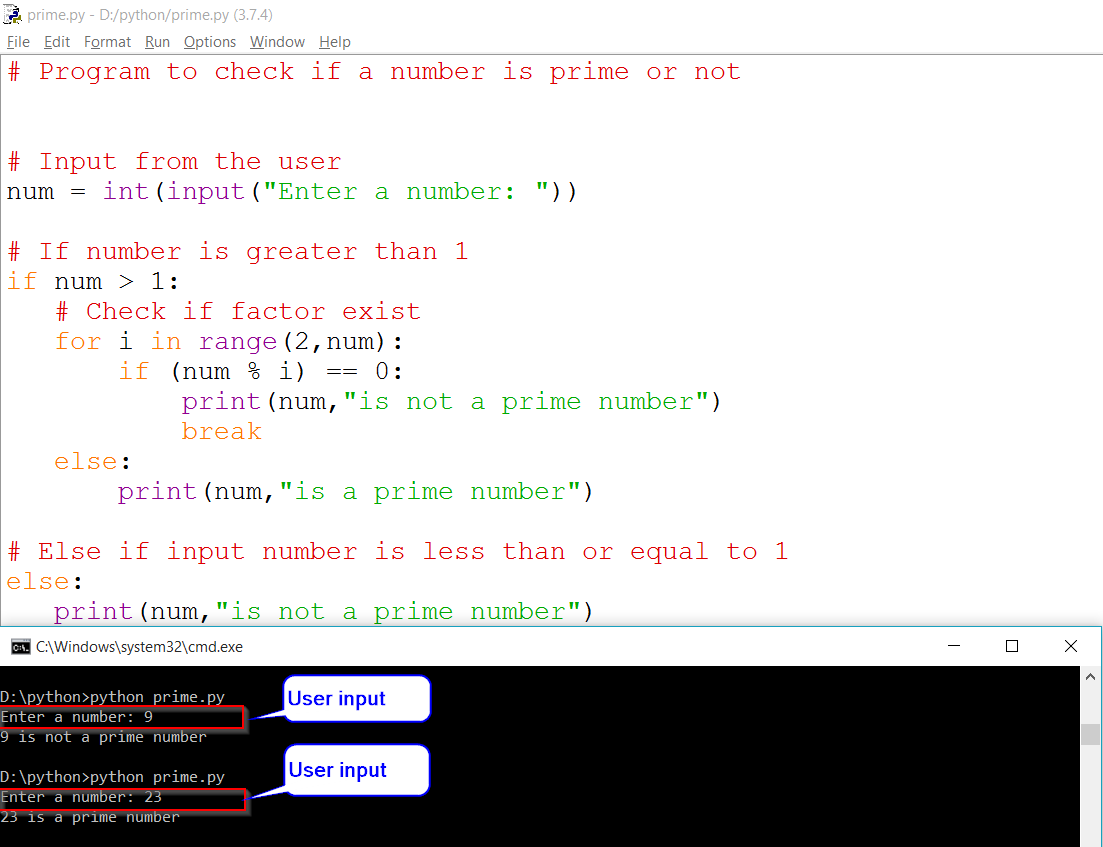
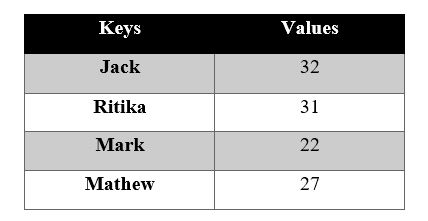

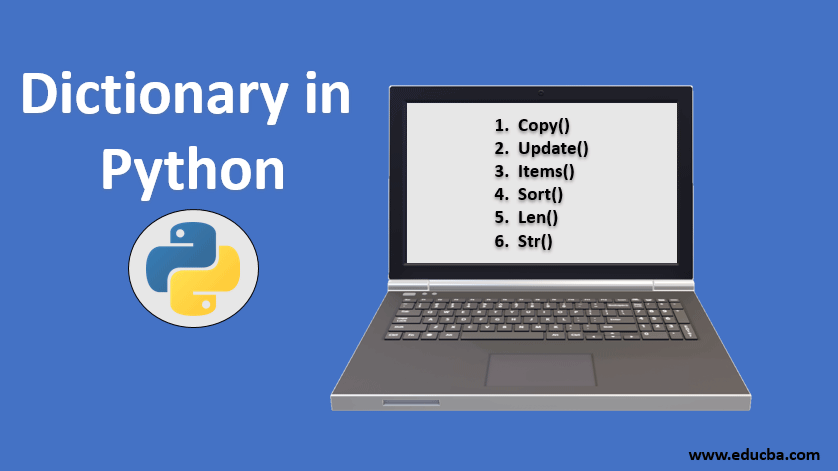
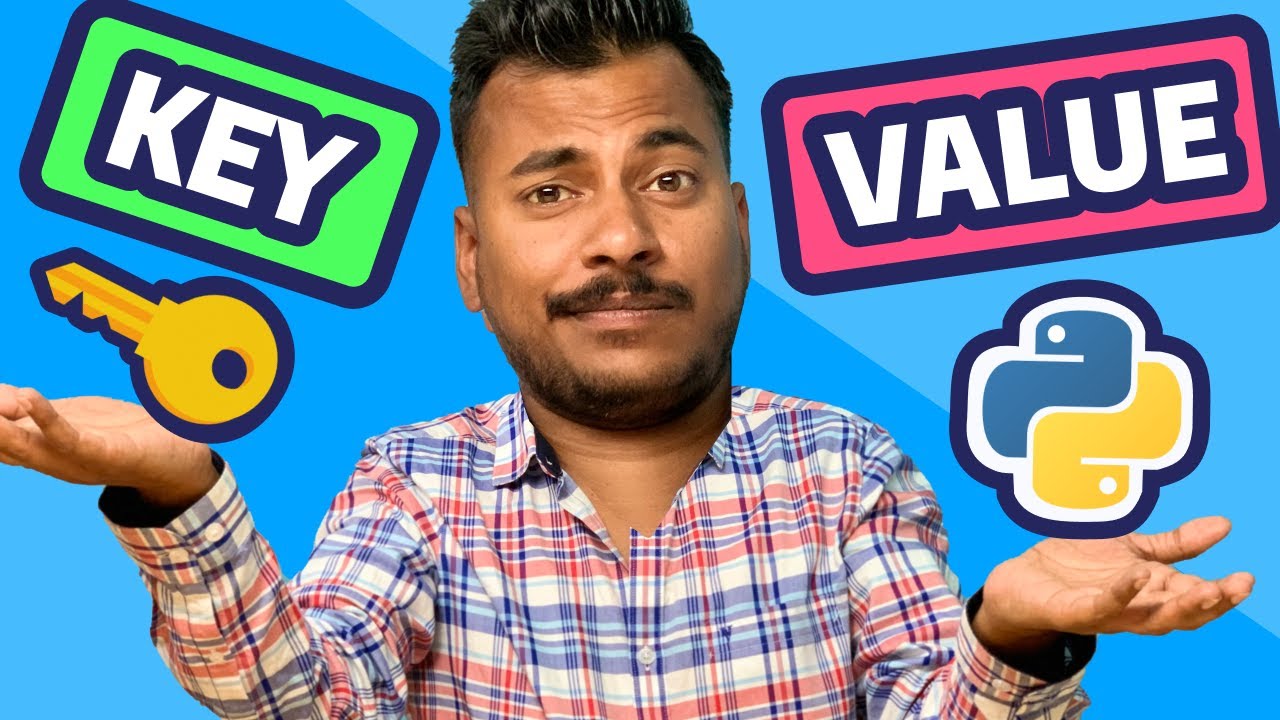
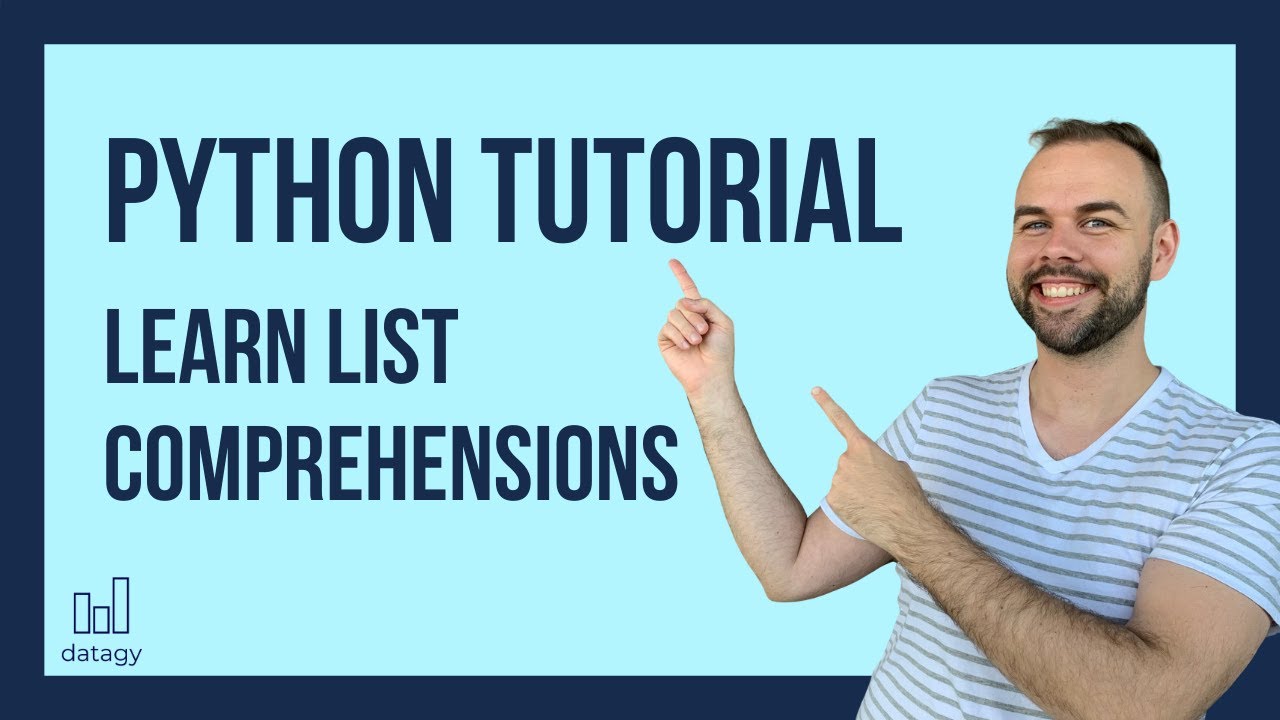
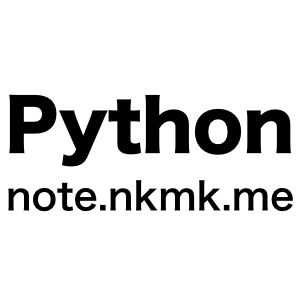
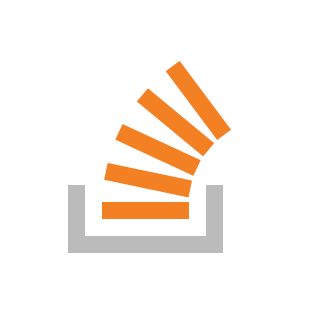

Article link: python dictionary check if key exists.
Learn more about the topic python dictionary check if key exists.
- Check whether given Key already exists in a Python Dictionary
- How to check if key exists in a python dictionary? – Flexiple
- Check if a given key already exists in a dictionary
- Check if Key Exists in Dictionary Python – Scaler Topics
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- Check if Key exists in Dictionary (or Value) with Python code
- How To Check If Key Exists In Dictionary In Python?
See more: nhanvietluanvan.com/luat-hoc