Python Dict Check If Key Exists
A key feature of dictionaries in Python is the ability to store and retrieve data using key-value pairs. And in many scenarios, we need to check if a specific key already exists in a dictionary before accessing or modifying its value. This article will explore various methods to check if a key exists in a Python dictionary. We will also cover additional topics such as adding values to a key, finding elements in a dictionary, sorting by key, and checking if a value exists in a dictionary.
Methods to Check if a Key Exists in a Python Dictionary:
1. Using the “in” operator:
The simplest and most common method to check if a key exists in a dictionary is by using the “in” operator. This operator returns True if the specified key is present in the dictionary, and False otherwise. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
if ‘name’ in my_dict:
print(“Key ‘name’ exists”)
else:
print(“Key ‘name’ does not exist”)
“`
2. Using the dictionary’s “get()” method:
The `get()` method provides an alternative way to retrieve the value associated with a key in a dictionary. It also allows us to specify a default value to be returned if the key is not found. By checking the return value of `get()`, we can determine if the key exists. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
if my_dict.get(‘name’) is not None:
print(“Key ‘name’ exists”)
else:
print(“Key ‘name’ does not exist”)
“`
3. Using the dictionary’s “keys()” method:
The `keys()` method returns a view object that contains all the keys present in the dictionary. We can use the `in` operator with this view object to check if a key exists. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
if ‘name’ in my_dict.keys():
print(“Key ‘name’ exists”)
else:
print(“Key ‘name’ does not exist”)
“`
4. Using the “has_key()” function (Python 2.x):
In older versions of Python (2.x), the `has_key()` function was available to check if a key exists in a dictionary. However, this function is deprecated in Python 3.x and should not be used in new code. Here’s an example of using `has_key()`:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
if my_dict.has_key(‘name’):
print(“Key ‘name’ exists”)
else:
print(“Key ‘name’ does not exist”)
“`
5. Using the “in” keyword with try-except block:
We can also use a try-except block with the `in` operator to handle the KeyError that may occur if a key does not exist in the dictionary. By catching the exception, we can determine if the key exists. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
try:
value = my_dict[‘name’]
print(“Key ‘name’ exists”)
except KeyError:
print(“Key ‘name’ does not exist”)
“`
6. Using the try-except block with KeyError:
Similar to the previous method, we can use a try-except block with the KeyError exception to check if a key exists. However, instead of accessing the dictionary directly, we catch the exception when using the `get()` method. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
try:
value = my_dict.get(‘name’)
if value is not None:
print(“Key ‘name’ exists”)
else:
print(“Key ‘name’ does not exist”)
except KeyError:
print(“Key ‘name’ does not exist”)
“`
7. Using the try-except block with “in” operator:
This method combines the try-except block with the `in` operator to handle the KeyError exception. By catching the exception, we can determine if the key exists. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
try:
if ‘name’ in my_dict:
print(“Key ‘name’ exists”)
else:
print(“Key ‘name’ does not exist”)
except KeyError:
print(“Key ‘name’ does not exist”)
“`
8. Using the “in” keyword with “not” operator:
If we want to check if a key does not exist in a dictionary, we can use the `not` operator along with the `in` keyword. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
if ‘address’ not in my_dict:
print(“Key ‘address’ does not exist”)
else:
print(“Key ‘address’ exists”)
“`
FAQs:
Q1. How to check if a key does not exist in a Python dictionary?
A: To check if a key does not exist in a Python dictionary, we can use the `not` operator along with the `in` keyword. For example: `if ‘key’ not in my_dict:`.
Q2. How to check if a key exists in an array in Python?
A: In Python, we cannot directly check if a key exists in an array because arrays are indexed by integers, not keys. However, if you are referring to a list of dictionaries, you can use any of the methods mentioned above to check if a key exists in each dictionary within the list.
Q3. How to add a value to a key in a dictionary in Python?
A: To add or modify the value associated with a key in a Python dictionary, simply assign the new value to the key. If the key already exists, the value will be updated. If the key does not exist, a new key-value pair will be added.
Q4. How to find an element in a dictionary in Python?
A: To find an element in a dictionary in Python, you can use any of the methods mentioned above to check if a specific key exists. If the key exists, you can retrieve its associated value.
Q5. How to check if a value exists in a Python dictionary?
A: To check if a value exists in a Python dictionary, you can use the `in` operator with the dictionary’s `values()` method. For example: `if value in my_dict.values():`.
Q6. How to sort a dictionary by key in Python?
A: To sort a dictionary by key in Python, you can use the `sorted()` function along with the dictionary’s `keys()` method. Here’s an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ’email’: ‘[email protected]’}
sorted_dict = {k: my_dict[k] for k in sorted(my_dict.keys())}
“`
In conclusion, checking if a key exists in a Python dictionary is a common requirement in many programming scenarios. This article covered various methods to accomplish this task, including the use of the “in” operator, the dictionary’s “get()” and “keys()” methods, try-except blocks, and more. Additionally, we discussed other related topics such as adding values to a key, finding elements, checking for the existence of a value, and sorting by key.
How To Check If A Key Exists In A Python Dictionary | Python Beginner Tutorial
How To Check If Key Exists In Dict Python?
Python is a powerful programming language that offers various data structures to store and manipulate data. One of these data structures is a dictionary, which provides a way to store data in key-value pairs. As dictionaries are widely used in Python, it becomes crucial to be able to check if a specific key exists within a dictionary. In this article, we will explore different methods to check whether a key exists in a dictionary, providing examples and explanations along the way.
Method 1: The `in` operator
The most straightforward way to check if a key exists in a dictionary is by using the `in` operator. This operator is very intuitive and easy to understand. It returns a boolean value, `True`, if the specified key is present in the given dictionary; otherwise, it returns `False`.
Here is an example to demonstrate the usage of the `in` operator:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘orange’: 2}
if ‘apple’ in my_dict:
print(“Key ‘apple’ exists in the dictionary.”)
else:
print(“Key ‘apple’ does not exist in the dictionary.”)
“`
In this example, we first create a dictionary `my_dict` with three key-value pairs. We then check if the key `’apple’` exists in the dictionary using the `in` operator. If the key exists, we print a corresponding message. Otherwise, if the key does not exist, we print a different message.
Method 2: The `get()` method
Another way to check if a key exists in a dictionary is by using the `get()` method. The `get()` method is a built-in method for dictionaries that takes the key as an argument and returns the corresponding value. However, if the key does not exist, it returns a default value specified as the second argument. By not providing a default value, it returns `None` if the key is not found.
Here is an example to illustrate the usage of the `get()` method:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘orange’: 2}
if my_dict.get(‘apple’) is not None:
print(“Key ‘apple’ exists in the dictionary.”)
else:
print(“Key ‘apple’ does not exist in the dictionary.”)
“`
In this example, we use the `get()` method to check if the key `’apple’` exists in the dictionary `my_dict`. If the key is found (i.e., not `None`), we print a corresponding message. Otherwise, if the key is not found (i.e., `None`), we print a different message.
Method 3: The `keys()` method
The `keys()` method returns an iterable view object of all the keys in a dictionary. We can use this method in combination with the `in` operator to check if a specific key exists in the dictionary.
Here is an example of utilizing the `keys()` method:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘orange’: 2}
if ‘apple’ in my_dict.keys():
print(“Key ‘apple’ exists in the dictionary.”)
else:
print(“Key ‘apple’ does not exist in the dictionary.”)
“`
In this example, we obtain all the keys of `my_dict` using the `keys()` method and used the `in` operator to check if `’apple’` is present within the keys. If the key exists, we print a corresponding message. Otherwise, if the key does not exist, we print a different message.
FAQs:
Q1: Can we check if a key exists in a dictionary using the `try-except` mechanism?
Yes, we can use a `try-except` block to check if a key exists in a dictionary. We can try to access the value associated with the key using square brackets, and if a `KeyError` exception is raised, it means the key does not exist in the dictionary.
Here is an example:
“`python
my_dict = {‘apple’: 5, ‘banana’: 3, ‘orange’: 2}
try:
value = my_dict[‘apple’]
print(“Key ‘apple’ exists in the dictionary.”)
except KeyError:
print(“Key ‘apple’ does not exist in the dictionary.”)
“`
In this example, we try to access the value of `’apple’` using the square brackets. If the key exists, we print a corresponding message. Otherwise, if a `KeyError` is raised, we print a different message.
Q2: Is there any performance difference between the different methods to check if a key exists in a dictionary?
Yes, there can be performance differences between the different methods. The `in` operator has an average runtime complexity of O(1) because it uses hashing to perform the key lookup. On the other hand, the `get()` method has a similar average runtime complexity of O(1) due to the hash table implementation.
However, using the `keys()` method to check if a key exists can be less efficient, especially for large dictionaries. The `keys()` method creates a new list of all the keys in the dictionary, which consumes additional memory and increases the runtime complexity to O(n), where n is the number of keys.
To optimize the performance, it is recommended to use the `in` operator or the `get()` method if you only need to check the existence of a key.
In conclusion, checking if a key exists in a dictionary is a crucial task when working with Python dictionaries. In this article, we explored different methods, including the `in` operator, the `get()` method, and the `keys()` method, to accomplish this task. Each method has its own advantages and considerations, so choose the one that suits your requirements and optimizes performance.
How To Check If Dict Has Key-Value?
A dictionary is a powerful data structure in Python, which allows you to store and manipulate key-value pairs. Often, during the development process, you may come across a situation where you need to check whether a dictionary contains a specific key-value. In this article, we will explore several methods that will help you efficiently perform this task. So, let’s dive in and learn how to check if a dictionary has a key-value!
I. Using the ‘in’ operator:
The simplest and most commonly used method to check if a dictionary has a key-value pair is by using the ‘in’ operator. The ‘in’ operator returns True if a key is found in the dictionary, otherwise, it returns False. Consider the following code snippet that demonstrates its usage:
“`python
# Creating a dictionary
my_dict = {‘apple’: 10, ‘banana’: 5, ‘orange’: 2}
# Checking if a key-value pair exists
if ‘apple’ in my_dict:
print(“Key-value pair exists!”)
else:
print(“Key-value pair doesn’t exist!”)
“`
Output:
“`
Key-value pair exists!
“`
In the above example, we first create a dictionary named ‘my_dict’, which contains key-value pairs representing the number of fruits. Then, we use the ‘in’ operator to check if the key ‘apple’ exists in the dictionary. Since the key-value pair exists, the output is “Key-value pair exists!”
Note that this method only checks for the existence of a key and not the specific key-value pair as a whole.
II. Using the ‘get()’ method:
Another approach to determine if a dictionary contains a key-value is by using the ‘get()’ method. The ‘get()’ method takes a key as an argument and returns the corresponding value if found in the dictionary. If the key doesn’t exist, it returns None by default. Have a look at the code snippet below:
“`python
# Creating a dictionary
my_dict = {‘apple’: 10, ‘banana’: 5, ‘orange’: 2}
# Checking if a key-value pair exists
value = my_dict.get(‘apple’)
if value:
print(“Key-value pair exists!”)
else:
print(“Key-value pair doesn’t exist!”)
“`
Output:
“`
Key-value pair exists!
“`
In the above code, we use the ‘get()’ method to retrieve the value associated with the key ‘apple’. Since the key exists in the dictionary, the value is assigned to the variable ‘value’. We then check if the value is not None and print the corresponding output.
III. Using the ‘items()’ method:
The ‘items()’ method provides a way to iterate through all the key-value pairs in a dictionary. Utilizing this method, you can directly check if a specific key-value exists. Here’s an example illustrating its utilization:
“`python
# Creating a dictionary
my_dict = {‘apple’: 10, ‘banana’: 5, ‘orange’: 2}
# Checking if a key-value pair exists
if (‘apple’, 10) in my_dict.items():
print(“Key-value pair exists!”)
else:
print(“Key-value pair doesn’t exist!”)
“`
Output:
“`
Key-value pair exists!
“`
In this example, we iterate through all the key-value pairs in the dictionary using the ‘items()’ method. We then check if the specific key-value pair (‘apple’, 10) exists. Since it is present in the dictionary, the output is “Key-value pair exists!”
IV. Using Exception Handling:
An alternate method to confirm if a key-value pair exists in a dictionary is by using exception handling. When a specified key is absent in the dictionary, it raises a ‘KeyError’ exception. We can utilize this exception to determine if a key-value pair exists. Observe the code below:
“`python
# Creating a dictionary
my_dict = {‘apple’: 10, ‘banana’: 5, ‘orange’: 2}
# Checking if a key-value pair exists
try:
value = my_dict[‘apple’]
print(“Key-value pair exists!”)
except KeyError:
print(“Key-value pair doesn’t exist!”)
“`
Output:
“`
Key-value pair exists!
“`
In the above code snippet, we use the ‘try-except’ construct to catch a ‘KeyError’ if the specified key (‘apple’) is not present in the dictionary. If the exception is not raised, it means that the key-value pair exists and we print the corresponding message.
FAQs:
Q: Can I use multiple criteria to check for a key-value pair in a dictionary?
A: Yes, you can combine multiple conditions using logical operators (and, or) when using the ‘in’ operator or the ‘items()’ method.
Q: What happens if I check for a key-value pair that doesn’t exist?
A: If you check for a non-existent key-value pair, the ‘in’ operator and the ‘items()’ method will return False. The ‘get()’ method will return None, and an exception (KeyError) will be raised when using exception handling.
Q: How can I check if a dictionary has a specific value?
A: To check if a dictionary contains a specific value, you can utilize the ‘values()’ method and apply the same techniques mentioned above to search for the desired value.
In conclusion, checking if a dictionary has a key-value pair is essential to ensure the correctness and efficiency of your code. By implementing the methods described above – using the ‘in’ operator, ‘get()’ method, ‘items()’ method, or exception handling – you can easily determine if a dictionary contains a desired key-value pair. Remember to choose the method that suits your requirements and coding style.
Keywords searched by users: python dict check if key exists Check if key not exists in dictionary Python, Python check key in array, Dict in Python, Add value to key dictionary Python, Find element in dictionary Python, How to check if value exists in python, Check value in dictionary Python, Sort dictionary by key Python
Categories: Top 23 Python Dict Check If Key Exists
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
In Python, dictionaries are a powerful data structure that allows you to store key-value pairs. They provide a convenient way to access and manipulate data efficiently. Sometimes, you may need to check if a key does not exist in a dictionary before performing certain operations. In this article, we will explore various methods to accomplish this task in Python.
Method 1: Using the in keyword
The simplest and most common way to check if a key does not exist in a dictionary is by using the ‘in’ keyword. The ‘in’ keyword allows you to check for membership in a dictionary. By default, it checks for membership in the dictionary keys.
Here’s an example:
“`python
# Create a dictionary
my_dict = {‘apple’: 5, ‘banana’: 10, ‘orange’: 7}
# Check if key ‘grape’ does not exist
if ‘grape’ not in my_dict:
print(“Key ‘grape’ does not exist in the dictionary”)
# Output: Key ‘grape’ does not exist in the dictionary
“`
In the above example, we check if the key ‘grape’ does not exist in the dictionary ‘my_dict’. If the key is not present, it prints the corresponding message.
Method 2: Using the dictionary get() method
Another method to check if a key does not exist in a dictionary is by using the dictionary’s ‘get()’ method. The ‘get()’ method returns the value for a given key if it exists in the dictionary. If the key is not found, it returns a default value. By passing a default value of None, we can determine if the key does not exist in the dictionary.
Here’s an example:
“`python
# Create a dictionary
my_dict = {‘apple’: 5, ‘banana’: 10, ‘orange’: 7}
# Check if key ‘grape’ does not exist
if my_dict.get(‘grape’) is None:
print(“Key ‘grape’ does not exist in the dictionary”)
# Output: Key ‘grape’ does not exist in the dictionary
“`
In the above example, we use the ‘get()’ method to check if the key ‘grape’ does not exist in the dictionary ‘my_dict’. If the value returned by ‘get()’ is None, it means the key is not present.
Method 3: Using exception handling
An alternate approach to check if a key does not exist in a dictionary is by using exception handling. Python provides a KeyError exception that can be used for this purpose. By wrapping the key access in a try block, we can catch the exception and handle it accordingly.
Here’s an example:
“`python
# Create a dictionary
my_dict = {‘apple’: 5, ‘banana’: 10, ‘orange’: 7}
# Check if key ‘grape’ does not exist
try:
value = my_dict[‘grape’]
print(“Key ‘grape’ exists in the dictionary”)
except KeyError:
print(“Key ‘grape’ does not exist in the dictionary”)
# Output: Key ‘grape’ does not exist in the dictionary
“`
In the above example, we attempt to access the key ‘grape’ in the dictionary ‘my_dict’. If the key is not present, a KeyError exception is raised, and we handle it by printing the corresponding message.
Frequently Asked Questions (FAQs)
Q1. What happens if I try to access a key that does not exist in a dictionary without using any of the mentioned methods?
If you try to access a key that does not exist in a dictionary without using any of the mentioned methods, a KeyError exception will be raised. It is always recommended to handle this exception to avoid program crashes.
Q2. Can we use the ‘in’ keyword to check for membership in dictionary values instead of keys?
No, by default, the ‘in’ keyword checks for membership in the dictionary keys. If you want to check for membership in the values, you can use the ‘in’ keyword in combination with the ‘values()’ method.
Here’s an example:
“`python
# Create a dictionary
my_dict = {‘apple’: 5, ‘banana’: 10, ‘orange’: 7}
# Check if value 10 exists in the dictionary
if 10 in my_dict.values():
print(“Value 10 exists in the dictionary”)
# Output: Value 10 exists in the dictionary
“`
In the above example, we use the ‘in’ keyword along with the ‘values()’ method to check if the value 10 exists in the dictionary ‘my_dict’.
Q3. Is one method better than the others?
The choice of method depends on the specific requirements and constraints of your program. Both the ‘in’ keyword and ‘get()’ method are commonly used to check for key existence. The exception handling method may be preferred when you want to perform additional operations based on the presence or absence of a key.
Conclusion
Checking if a key does not exist in a dictionary is a common task in Python. In this article, we explored three methods to accomplish this task: using the ‘in’ keyword, using the ‘get()’ method, and using exception handling. Remember to choose the method that best suits your needs based on the program’s context.
Python Check Key In Array
Python is a versatile programming language that offers a wide range of data structures, including arrays. Arrays in Python are known as lists and they provide a convenient way to store and manipulate data. One common task when working with arrays is to check if a specific key or element exists within it. In this article, we will explore various methods to check if a key is present in an array and delve into their implementations.
Methods to Check a Key in Python Array:
1. Using the ‘in’ keyword:
The most straightforward way to check if a key exists in a Python array is to use the ‘in’ keyword. It is a powerful operator that checks if a given value is present in a sequence. To implement this method, simply use the ‘in’ keyword followed by the array name and the desired key. For example:
“`python
array = [“apple”, “banana”, “orange”]
if “apple” in array:
print(“Key exists!”)
“`
This code snippet will print “Key exists!” since “apple” is present in the array. However, if the key is not found, nothing will be printed.
2. Using a for loop:
Another approach to check if a key exists in a Python array is to iterate through the array using a for loop and compare each element with the desired key. This method allows you to perform additional tasks when a match is found. Here’s an example:
“`python
array = [“apple”, “banana”, “orange”]
key = “banana”
for element in array:
if element == key:
print(“Key exists!”)
break
“`
In this case, the code will print “Key exists!” if “banana” is present in the array. The ‘break’ statement is used to exit the loop once a match is found, preventing unnecessary iterations.
3. Using the index() method:
Python arrays, also known as lists, provide an inbuilt method called index() that returns the index of the first occurrence of an element. By checking if the index is -1, we can determine if the key is present in the array. Consider the following example:
“`python
array = [“apple”, “banana”, “orange”]
key = “orange”
if array.index(key) != -1:
print(“Key exists!”)
“`
This code snippet will output “Key exists!” if “orange” is present in the array. However, it should be noted that using the index() method raises a ValueError if the key is not found. Thus, it is crucial to handle this exception or use the ‘in’ keyword approach to avoid potential errors.
FAQs:
Q1. Can an array contain duplicate keys in Python?
A1. Yes, an array in Python can contain duplicate keys. The methods mentioned above will still work correctly and return true if any instance of the key is present in the array.
Q2. What happens if the key is not found in the array using the index() method?
A2. When the index() method fails to find the key in the array, it raises a ValueError. It is recommended to handle this exception using a try-except block or use the ‘in’ keyword approach to avoid the error.
Q3. Are there any performance differences between the methods mentioned?
A3. While the performance differences between these methods are negligible for smaller arrays, the ‘in’ keyword approach tends to be more efficient. It takes advantage of algorithms that have been optimized for checking membership in sequences.
Q4. Are there any alternative data structures that provide more efficient key existence checks?
A4. Yes, Python offers alternative data structures like sets and dictionaries that provide more efficient key existence checks. Sets use hashing to store unique elements, allowing for faster lookup times. Dictionaries, on the other hand, provide direct key-value mappings, making key existence checks extremely efficient.
Conclusion:
Checking if a key exists in a Python array is a common task during programming. In this comprehensive guide, we explored various methods, including using the ‘in’ keyword, for loops, and the index() method. Each method has its advantages, and the choice depends on the specific requirements of your program. For more efficient key existence checks, consider utilizing alternative data structures like sets or dictionaries.
Images related to the topic python dict check if key exists
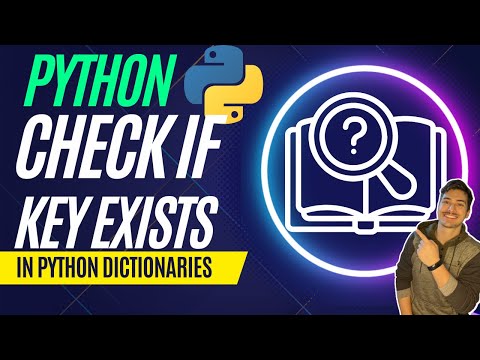
Found 11 images related to python dict check if key exists theme
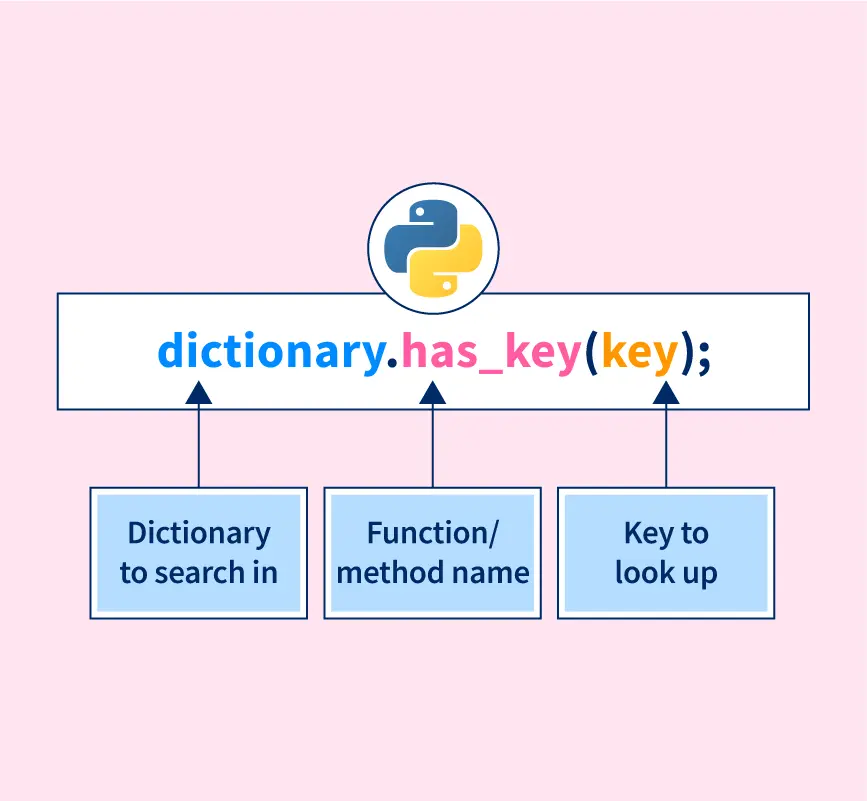
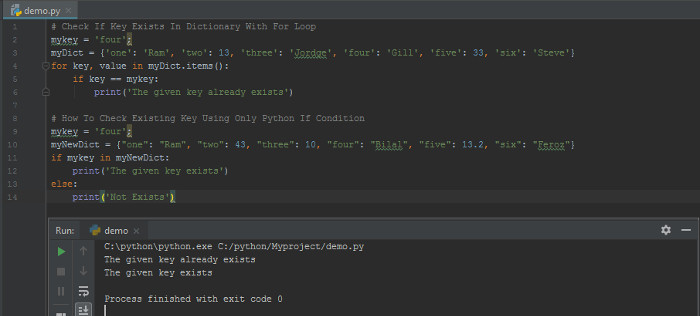
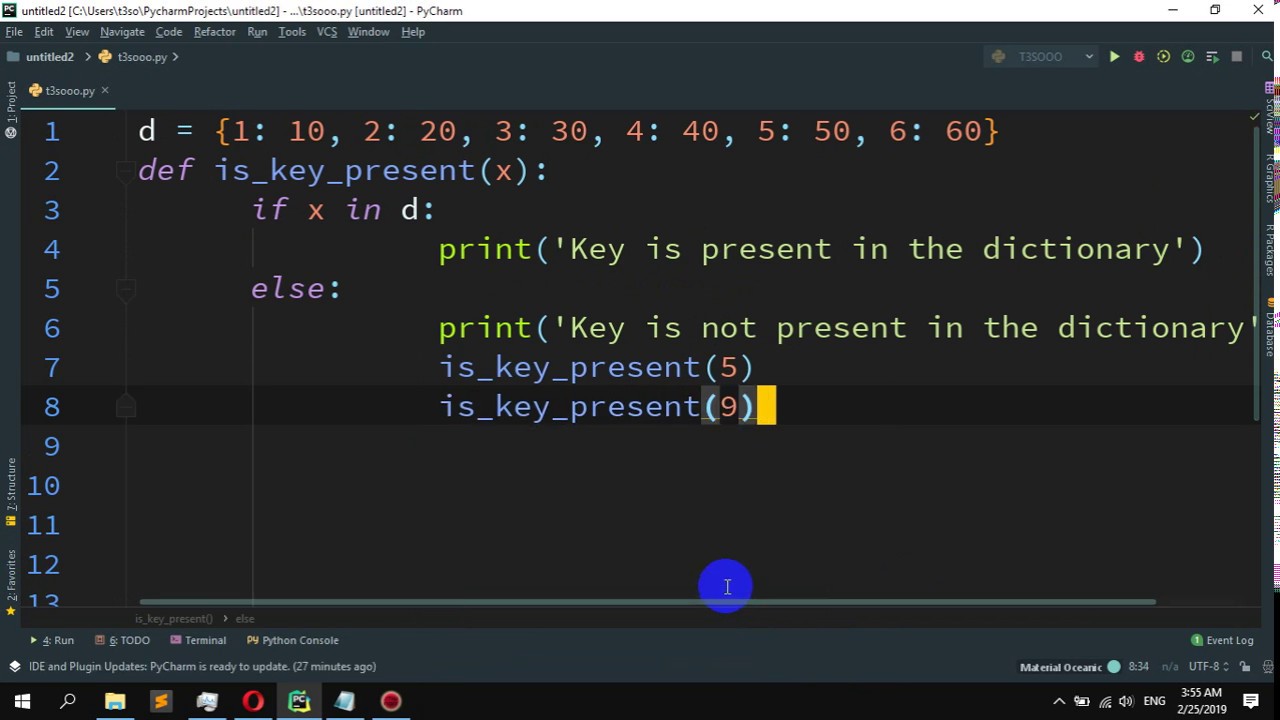
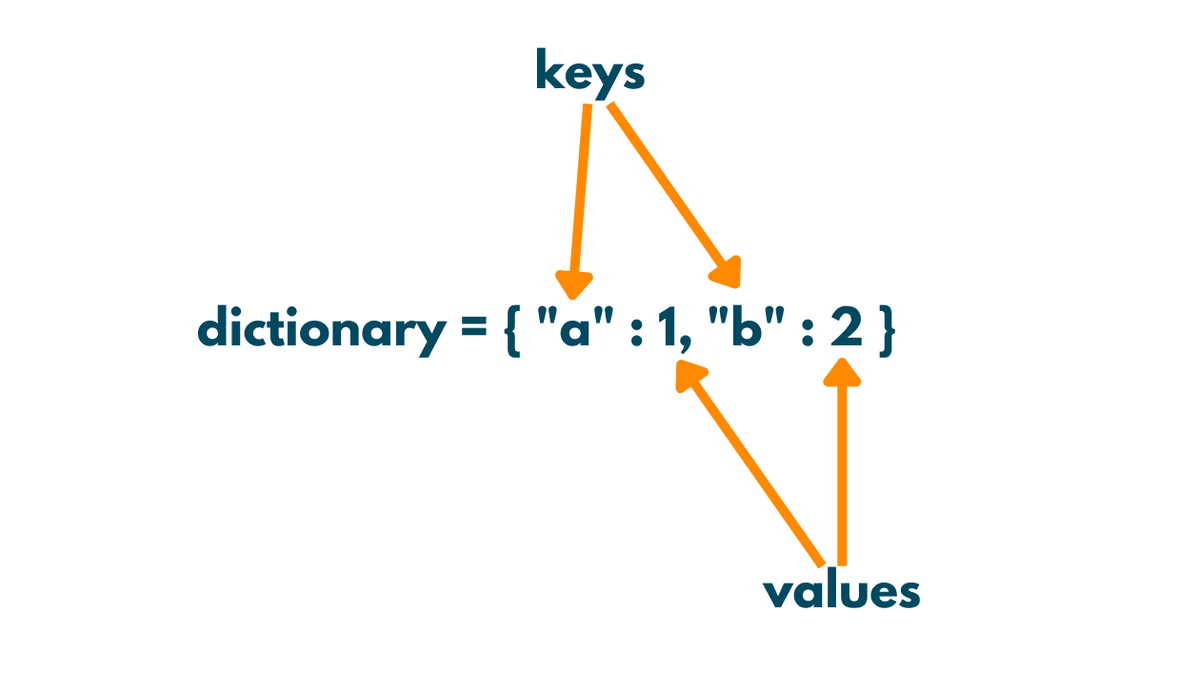

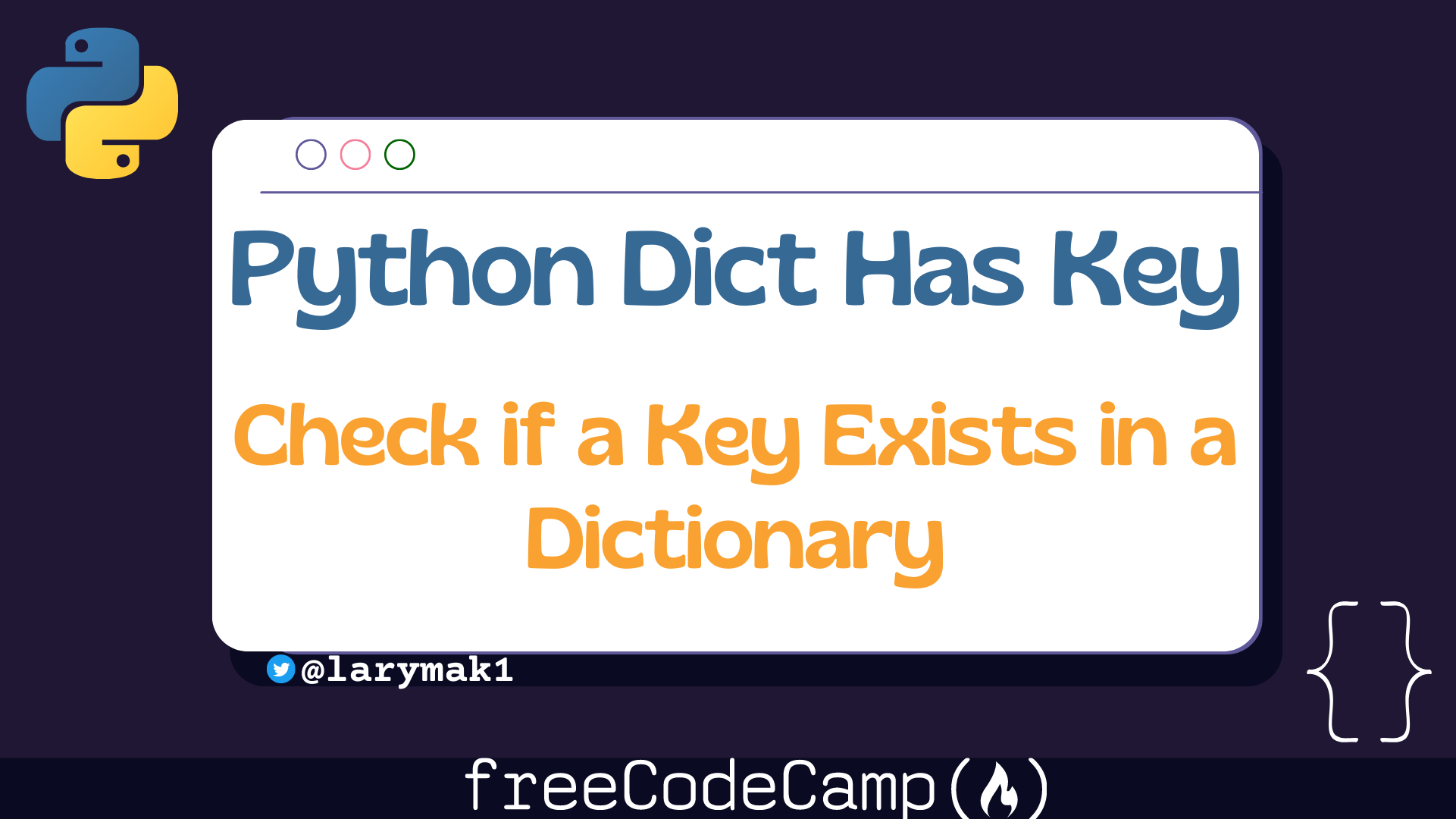
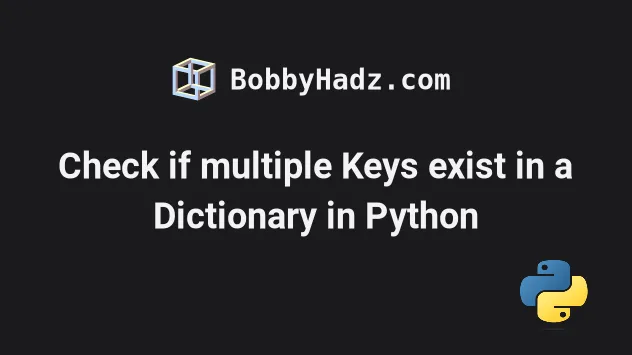
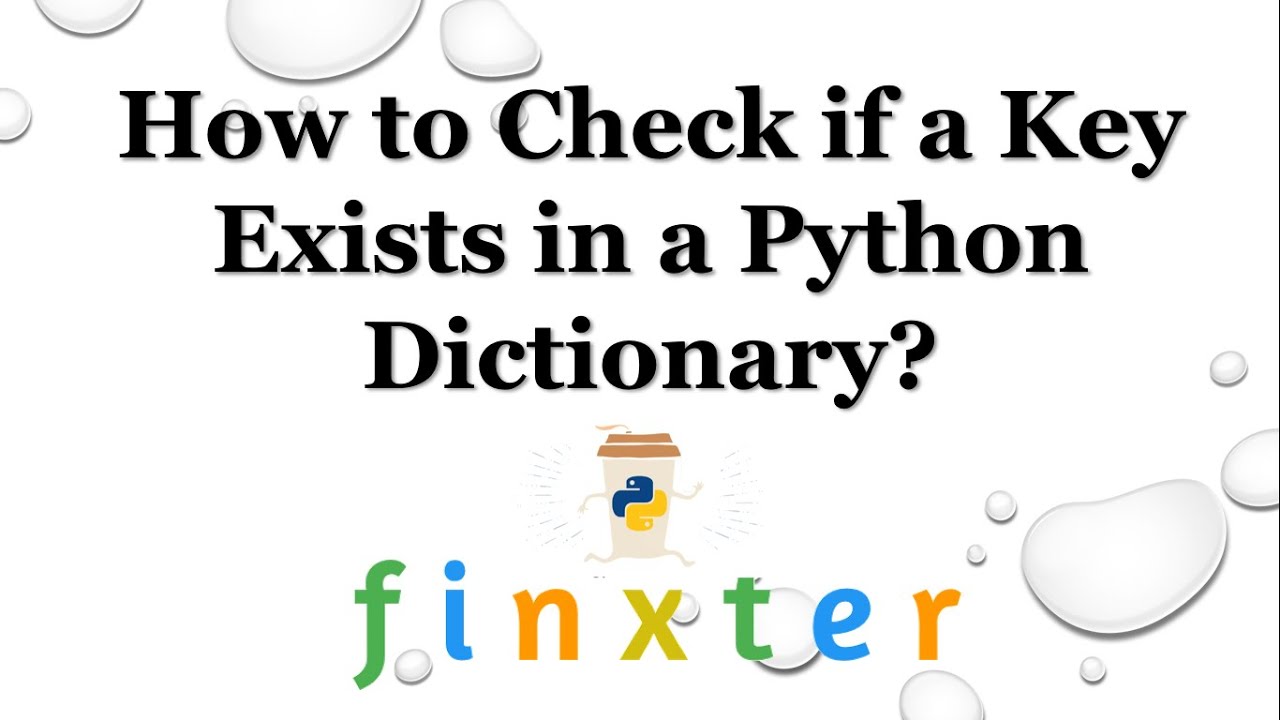

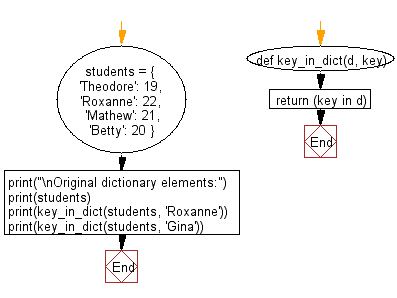

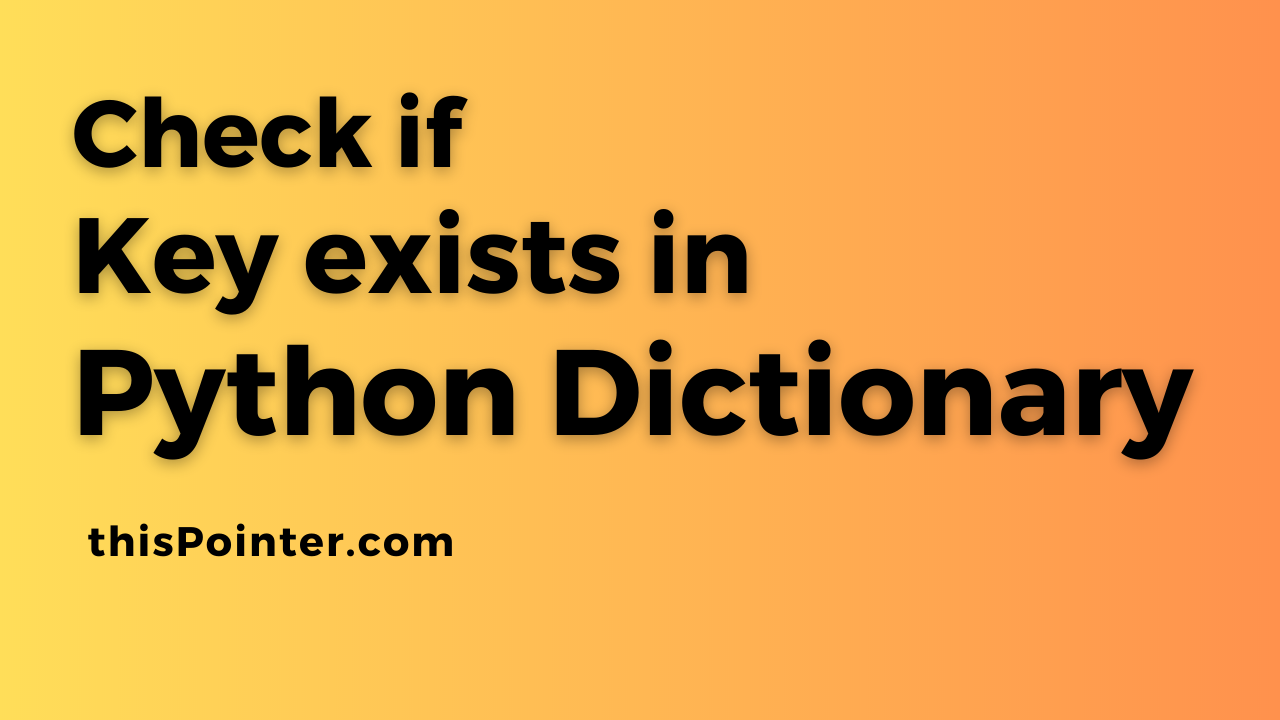
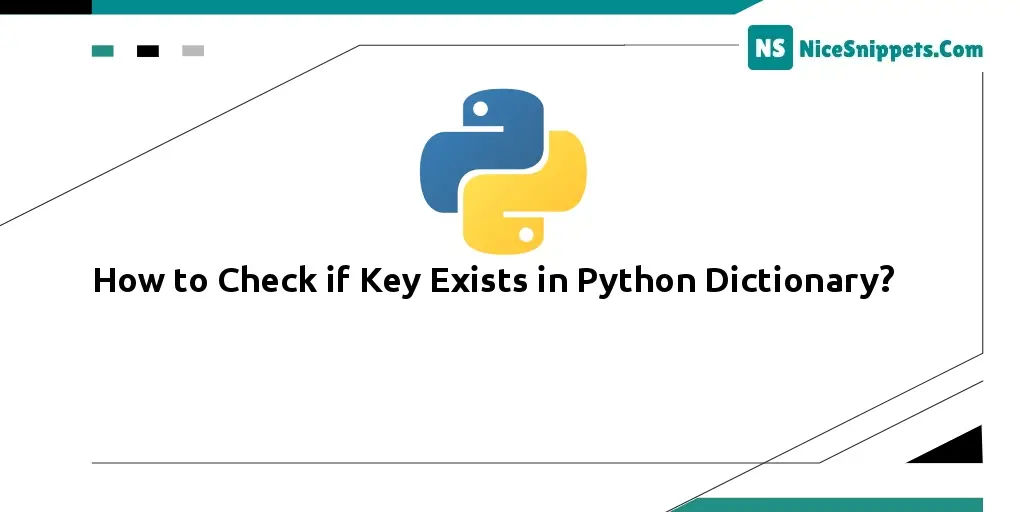


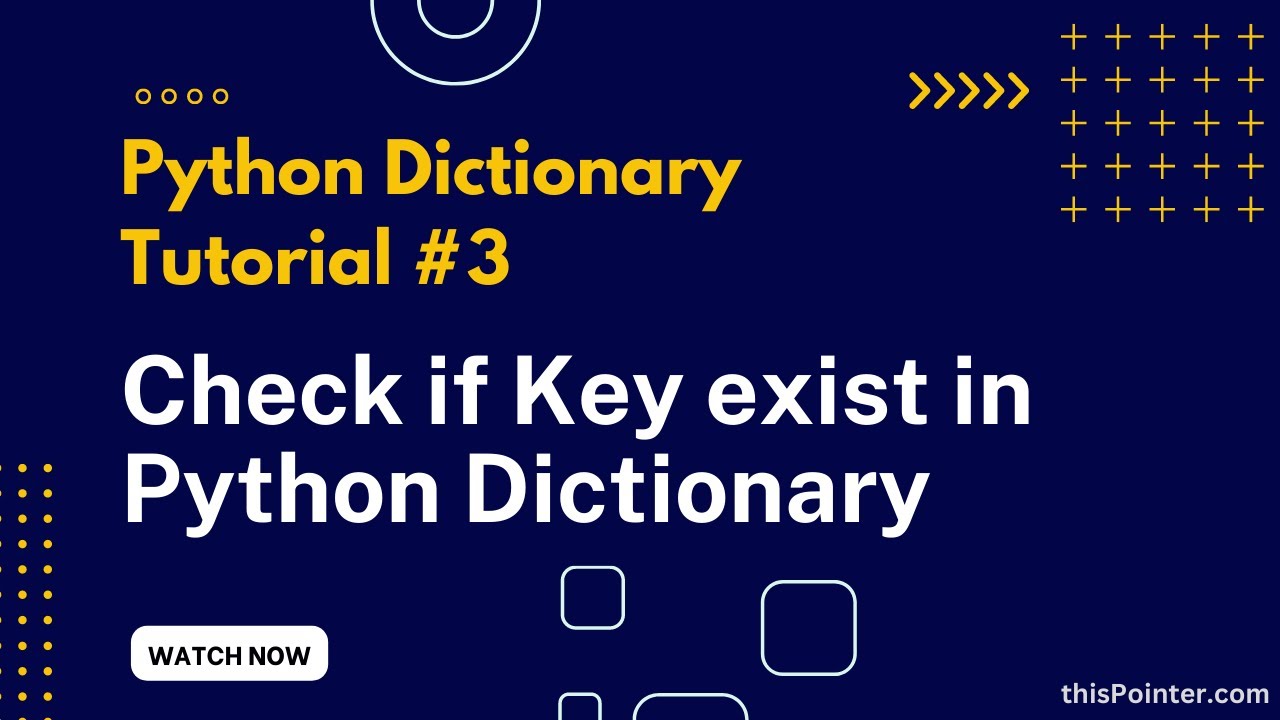

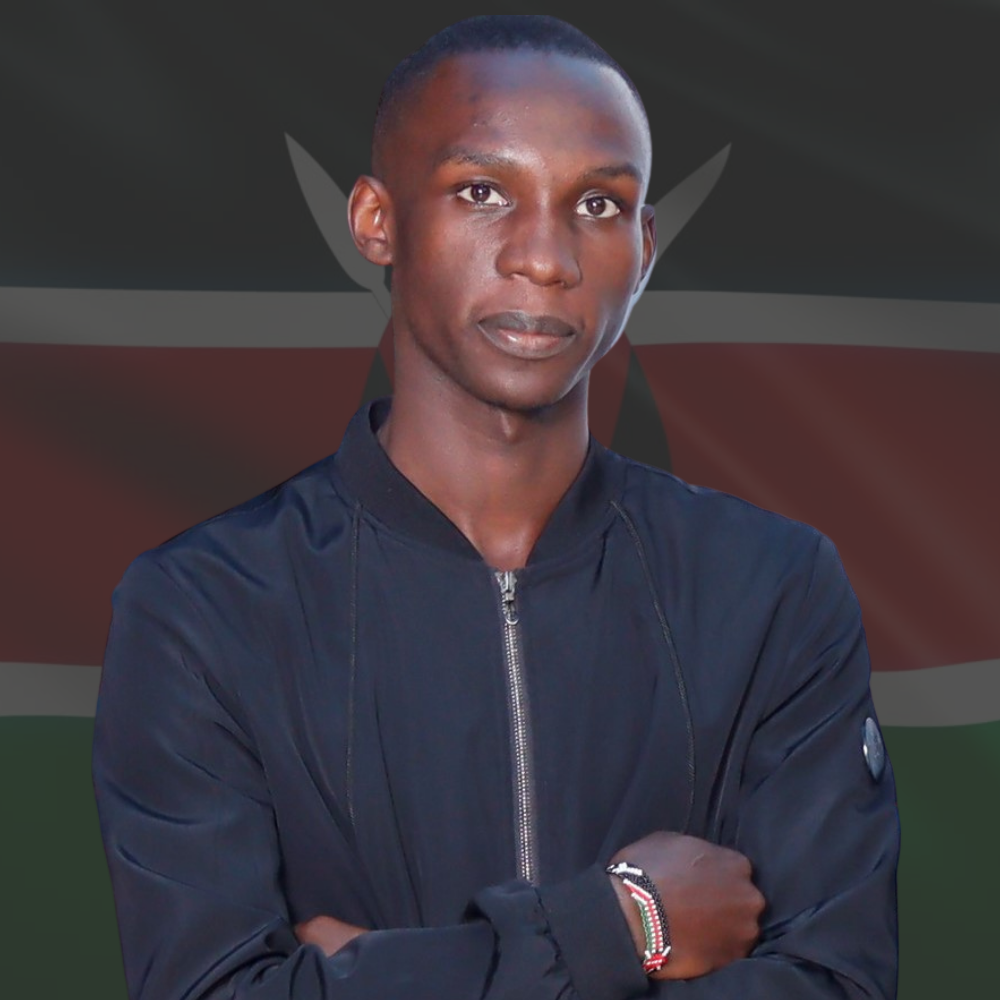
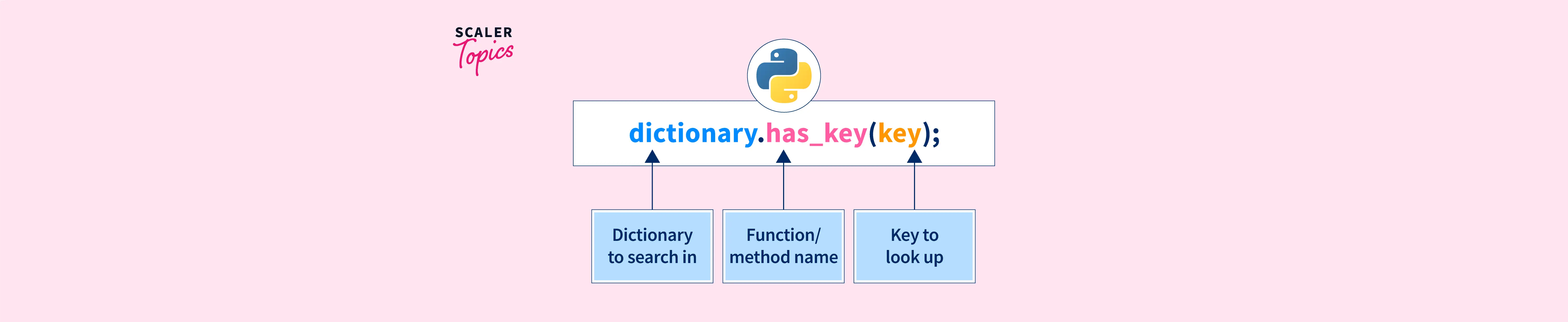
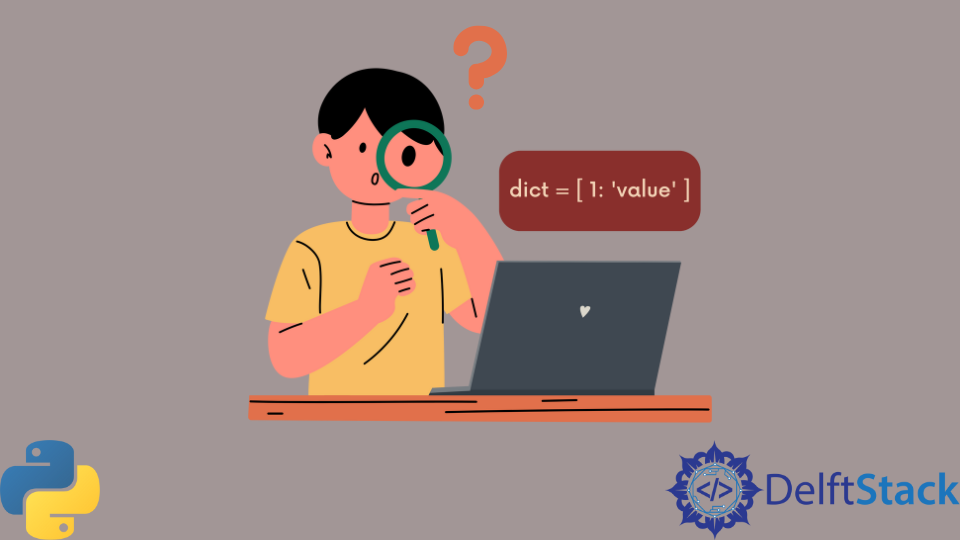
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example1.jpg)
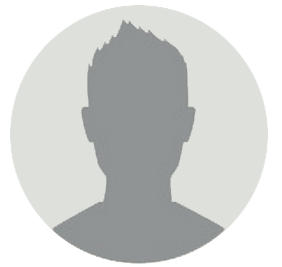


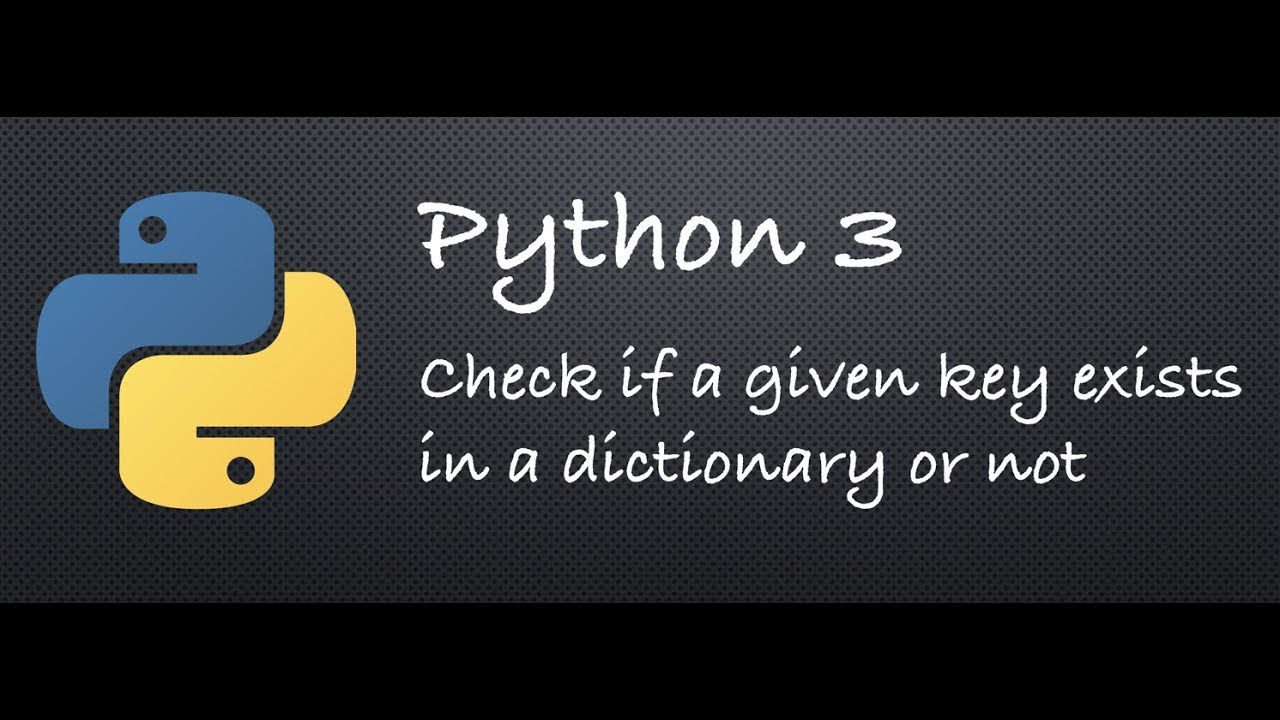
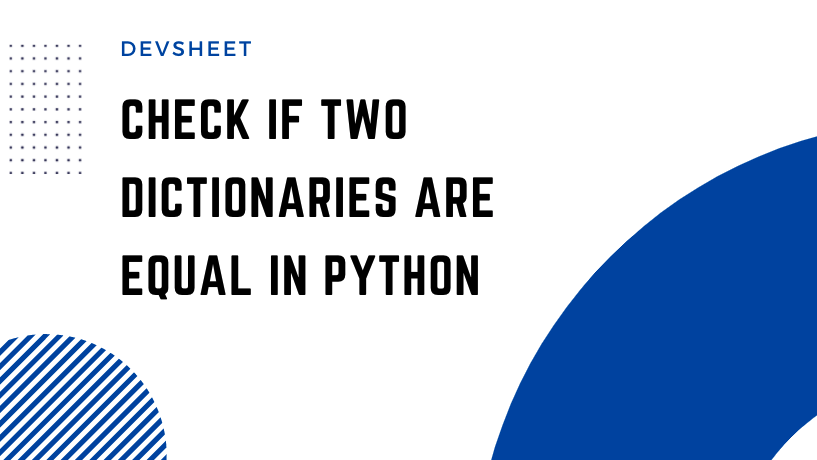
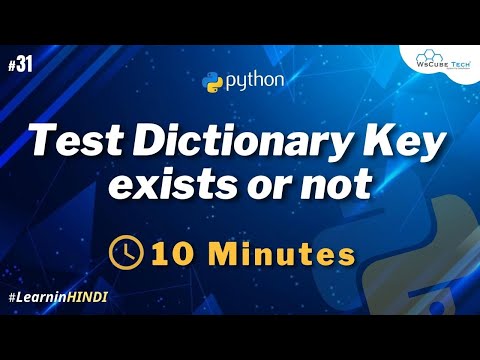
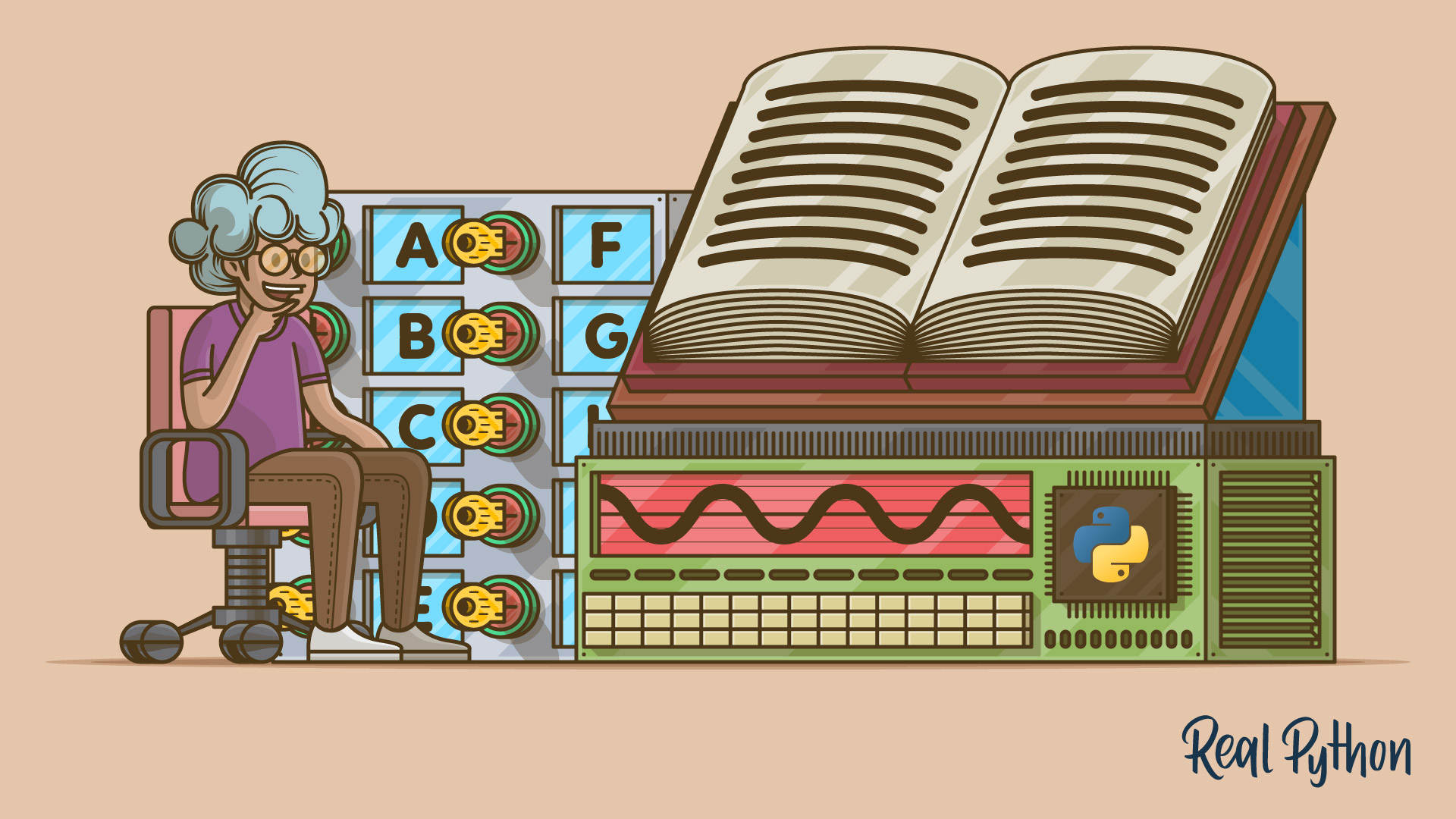
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example3.jpg)
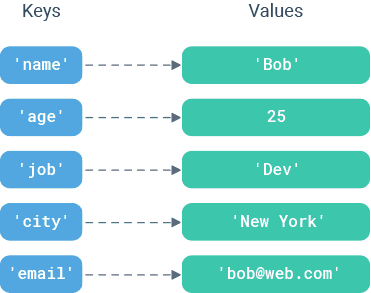
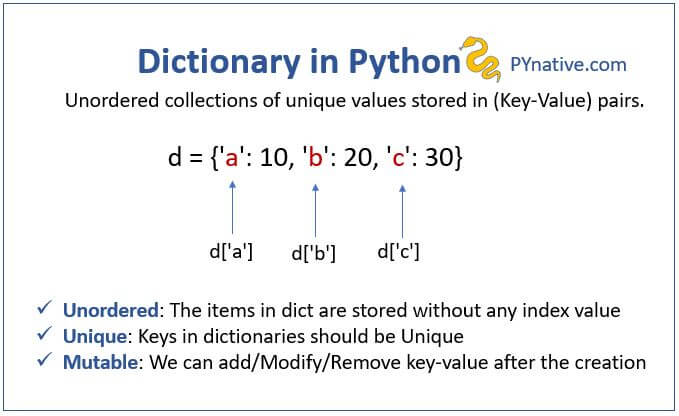
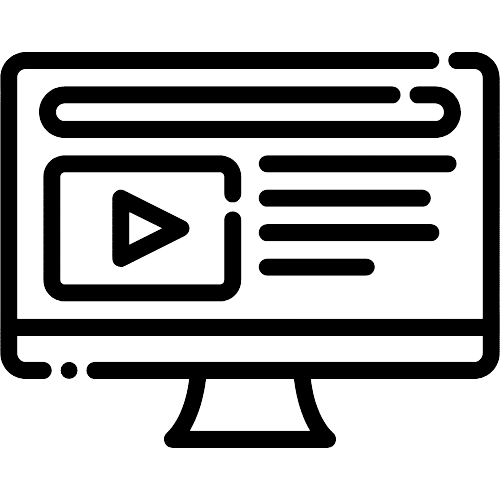
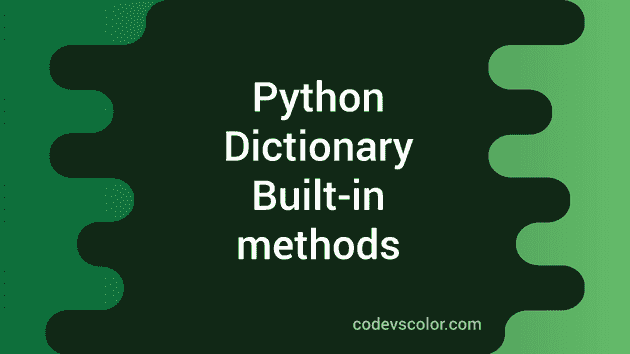
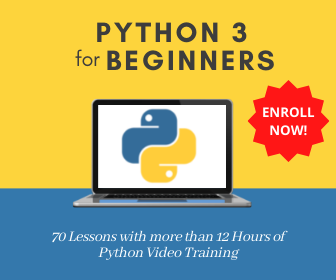
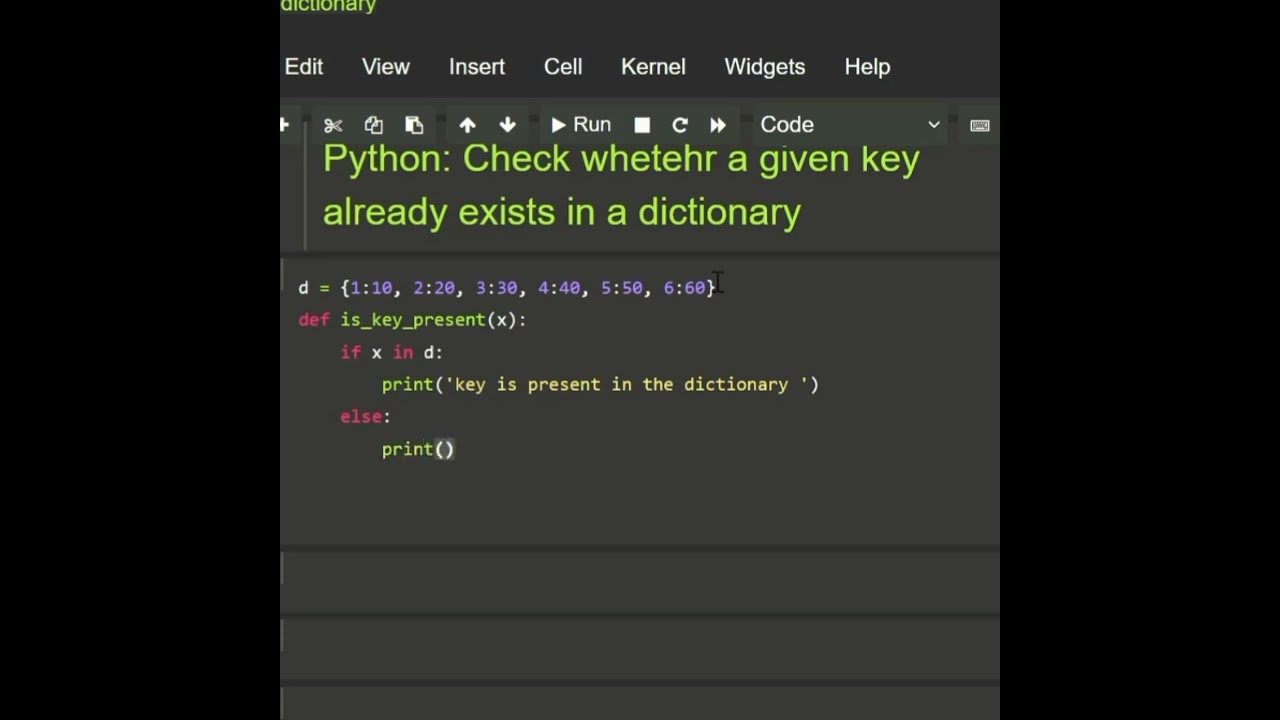

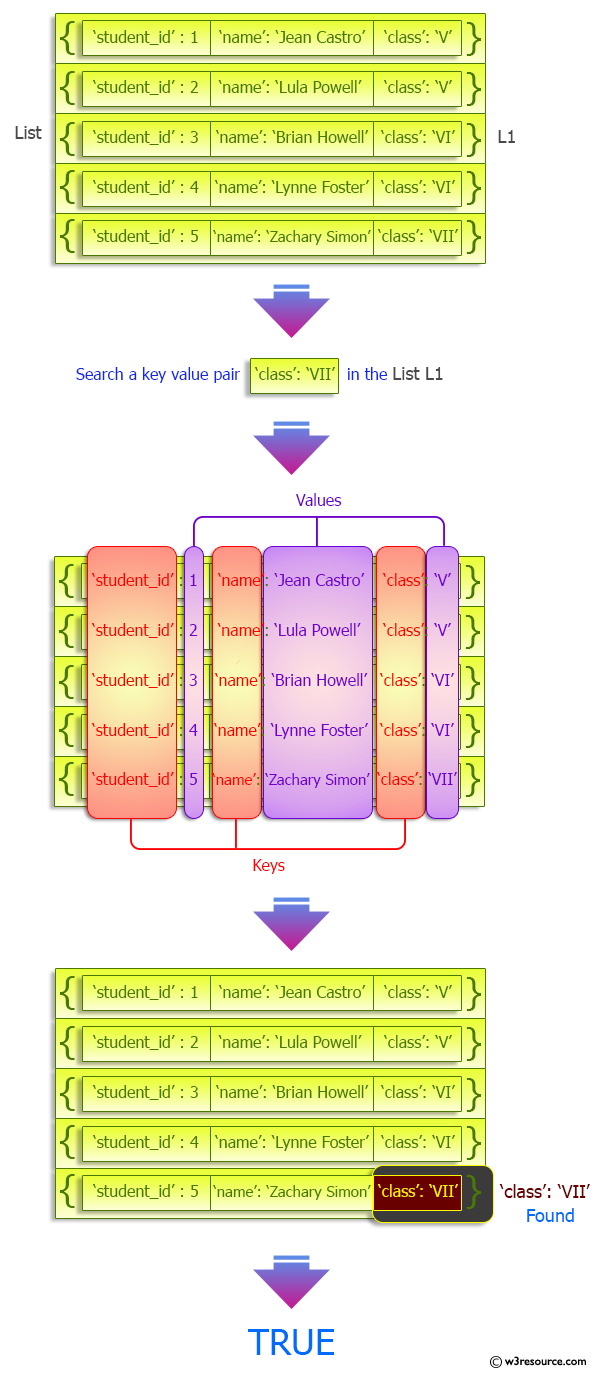
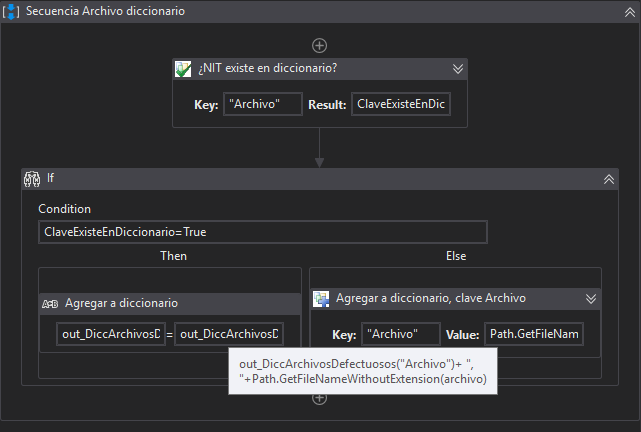

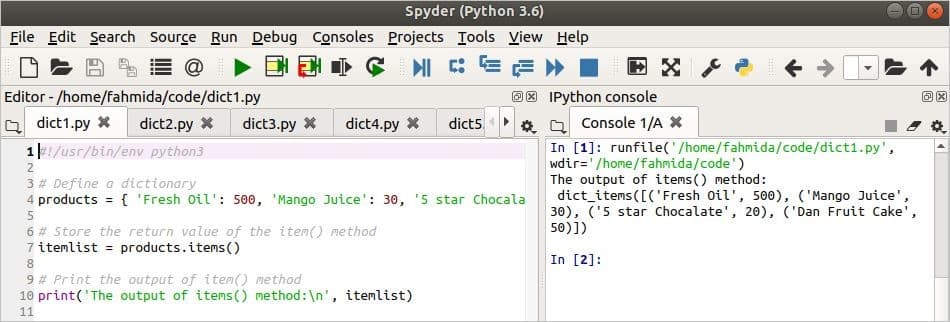
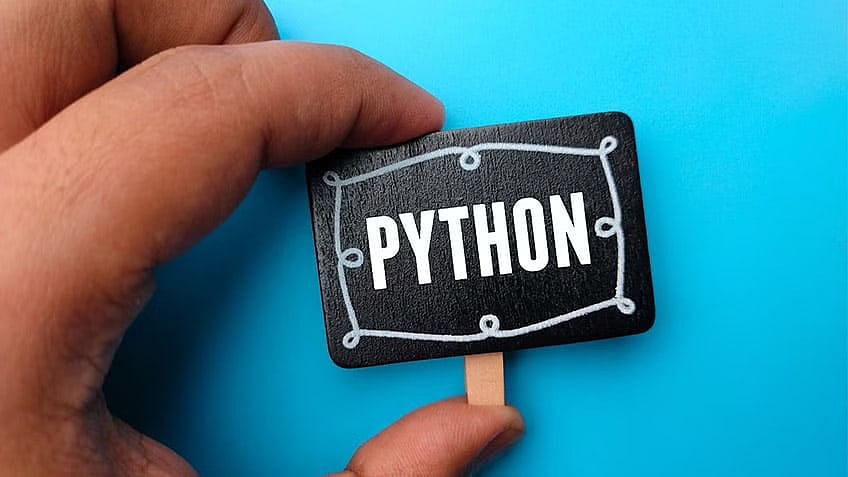
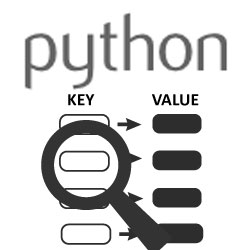
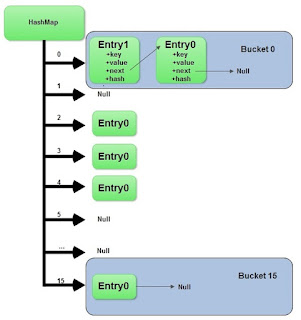
Article link: python dict check if key exists.
Learn more about the topic python dict check if key exists.
- How to check if key exists in a python dictionary? – Flexiple
- Check whether given Key already exists in a Python Dictionary
- Check if a given key already exists in a dictionary
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if key/value exists in dictionary in Python – nkmk note
- Check if Key Exists in List of Dictionaries in Python (3 Examples)
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- How To Check If Key Exists In Dictionary In Python?
- How to Check if a Key Exists in a Dictionary in Python
- Check if Key exists in Dictionary (or Value) with Python code
See more: nhanvietluanvan.com/luat-hoc