Python Create Folder If Not Exists
In Python, there are multiple ways to check if a folder exists and create it if it doesn’t. This article will cover different methods using both the `os` and `pathlib` modules. We will also discuss how to handle exceptions and create nested folders. Additionally, we’ll explore how to create multiple folders at once and use a try-except block for folder creation.
Checking if the Folder Exists
To begin, let’s examine how to check if a folder exists before creating it. The `os` module and the `pathlib` module offer different approaches for this task.
– Using the `os` module to check for the folder’s existence:
The `os` module provides a function called `path.exists()` that can be used to check if a folder or file exists. Here’s an example of how to use it to check if a folder exists:
“`python
import os
folder_path = ‘/path/to/folder’
if not os.path.exists(folder_path):
print(“Folder does not exist”)
else:
print(“Folder exists”)
“`
– Using the `pathlib` module to check for the folder’s existence:
The `pathlib` module provides the `Path` class, which has a method called `exists()` that checks if a folder or file exists. Here’s an example:
“`python
from pathlib import Path
folder_path = Path(‘/path/to/folder’)
if not folder_path.exists():
print(“Folder does not exist”)
else:
print(“Folder exists”)
“`
Creating the Folder
Once we have checked if the folder exists, we can proceed to create it if it doesn’t. Again, both the `os` and `pathlib` modules offer different ways to achieve this.
– Using the `os` module to create the folder:
The `os` module provides the `mkdir()` method, which can be used to create a folder. Here’s an example:
“`python
import os
folder_path = ‘/path/to/new/folder’
os.mkdir(folder_path)
“`
– Using the `pathlib` module to create the folder:
The `Path` class from the `pathlib` module also allows us to create a folder using the `mkdir()` method. Here’s an example:
“`python
from pathlib import Path
folder_path = Path(‘/path/to/new/folder’)
folder_path.mkdir()
“`
Handling Exceptions
It’s important to handle exceptions when creating folders, as there might be cases where the creation fails due to various reasons.
– Dealing with permissions errors when creating the folder:
When creating a folder, there can be permission errors if the user does not have sufficient privileges. We can handle this by catching the `PermissionError` exception. Here’s an example:
“`python
import os
folder_path = ‘/path/to/new/folder’
try:
os.mkdir(folder_path)
print(“Folder created successfully”)
except PermissionError:
print(“Permission denied. Cannot create folder”)
“`
– Handling race conditions when multiple processes try to create the folder simultaneously:
In scenarios where multiple processes attempt to create the same folder simultaneously, a race condition can occur. To prevent this, we can use the `os.makedirs()` method, which creates the entire directory path even if intermediate directories don’t exist. This ensures that all required directories are created, even if another process creates the parent directory simultaneously. Here’s an example:
“`python
import os
folder_path = ‘/path/to/new/folder’
os.makedirs(folder_path, exist_ok=True)
“`
Checking and Creating Nested Folders
In some cases, you may need to check if a nested folder exists and create it if it doesn’t. Here’s how to accomplish this:
– Checking if a nested folder exists:
To check if a nested folder exists, you can use the same methods demonstrated earlier. Simply specify the complete path to the nested folder.
– Creating a nested folder that does not exist:
To create a nested folder, you can use the `os.makedirs()` method with the path to the nested folder. This method creates intermediate directories as needed, ensuring the entire nested path is created. Here’s an example:
“`python
import os
nested_folder_path = ‘/path/to/nested/folder’
os.makedirs(nested_folder_path, exist_ok=True)
“`
Creating Multiple Folders at Once
If you need to create multiple folders simultaneously, Python provides convenient methods for this task.
– Creating multiple folders using `os.makedirs()`:
The `os.makedirs()` method can be used to create multiple folders at once by providing a list of paths. Here’s an example:
“`python
import os
folder_paths = [‘/path/to/folder1’, ‘/path/to/folder2’, ‘/path/to/folder3’]
for folder_path in folder_paths:
os.makedirs(folder_path, exist_ok=True)
“`
– Creating multiple folders using `pathlib.Path().mkdir()`:
The `Path` class from the `pathlib` module also allows us to create multiple folders at once using the `mkdir()` method. Here’s an example:
“`python
from pathlib import Path
folder_paths = [‘/path/to/folder1’, ‘/path/to/folder2’, ‘/path/to/folder3’]
for folder_path in folder_paths:
Path(folder_path).mkdir(parents=True, exist_ok=True)
“`
Using a Try-Except Block for Folder Creation
To handle exceptions more effectively, we can use a try-except block when creating folders.
– Using a try-except block to handle folder creation exceptions:
By using a try-except block, we can catch any exceptions that occur during folder creation and handle them accordingly. Here’s an example:
“`python
import os
folder_path = ‘/path/to/new/folder’
try:
os.mkdir(folder_path)
print(“Folder created successfully”)
except Exception as e:
print(f”An error occurred: {e}”)
“`
– Differentiating between different types of exceptions for customized handling:
In some cases, we might want to handle different types of exceptions differently. By specifying multiple except blocks, we can customize the handling based on the specific exception type. Here’s an example:
“`python
import os
folder_path = ‘/path/to/new/folder’
try:
os.mkdir(folder_path)
print(“Folder created successfully”)
except PermissionError:
print(“Permission denied. Cannot create folder”)
except FileExistsError:
print(“Folder already exists”)
except Exception as e:
print(f”An error occurred: {e}”)
“`
In conclusion, Python offers various methods for checking if a folder exists and creating it if it doesn’t. The `os` and `pathlib` modules provide different approaches, and it’s important to handle exceptions appropriately. By understanding these methods, you can efficiently work with folders in your Python applications.
FAQs
1. What is the difference between `os.mkdir()` and `os.makedirs()`?
– `os.mkdir()` creates a single directory, while `os.makedirs()` creates all intermediate directories as well if they don’t exist.
2. How can I handle permission errors when creating a folder?
– You can use a try-except block and catch the `PermissionError` exception to handle permission errors.
3. What happens if I try to create a folder that already exists?
– If you use `os.mkdir()` or `pathlib.Path().mkdir()` to create a folder that already exists, it will raise a `FileExistsError`. You can catch this exception to handle such cases.
4. Can I create multiple folders at once?
– Yes, you can use a loop to create multiple folders using either `os.makedirs()` or `pathlib.Path().mkdir()`.
5. How can I create a nested folder in Python?
– You can use `os.makedirs()` or `pathlib.Path().mkdir()` and provide the complete path to the nested folder to create it.
6. What is a race condition when creating folders?
– A race condition occurs when multiple processes try to create the same folder simultaneously. This can be avoided by using `os.makedirs()` with the `exist_ok=True` parameter.
Python Os Check If Folder Exists | Python Create Folder If Not Exist | Create File If Doesn’T Exist
How To Check If A Directory Exists If Not Create It In Python?
In Python, there are several ways to check if a directory exists and create it if it doesn’t. In this article, we will explore different methods and provide examples for each approach. Whether you are working on a file management script or need to create a new directory for storing data, these techniques will prove to be invaluable.
Method 1: Using the `os` module
The `os` module in Python provides a wide range of methods for interacting with the operating system. One of its functions, `os.path.isdir()`, allows us to determine whether a given path exists and is a directory. Here’s an example:
“`python
import os
directory = ‘/path/to/directory’
if not os.path.isdir(directory):
os.makedirs(directory)
“`
In the code above, we use the `os.path.isdir()` function to check if the specified directory exists. If it doesn’t, we create the directory using `os.makedirs()`.
Method 2: Using the `Path` class from the `pathlib` module
Python’s `pathlib` module provides an object-oriented approach to handle file and directory paths. We can use the `Path` class along with its `exists()` method to check if a directory exists. If it doesn’t, we can create it using the `mkdir()` method. Here’s an example:
“`python
from pathlib import Path
directory = Path(‘/path/to/directory’)
if not directory.exists():
directory.mkdir()
“`
The code snippet above demonstrates the usage of `exists()` to check if the directory exists and `mkdir()` to create it if necessary.
Method 3: Using the `os.path.exists()` method
Although the `os.path.exists()` method can check the existence of both files and directories, it can also be used to verify directories specifically. Here’s an example:
“`python
import os
directory = ‘/path/to/directory’
if not os.path.exists(directory):
os.makedirs(directory)
“`
In this code snippet, `os.path.exists()` is used to check if the directory exists, and if it doesn’t, the directory is created with `os.makedirs()`.
Method 4: Using the `try-except` block
An alternative approach to checking the existence of a directory is by catching a `FileNotFoundError` exception. This method relies on attempting to access the directory with `os.listdir()` and handling the exception accordingly. Here’s an example:
“`python
import os
directory = ‘/path/to/directory’
try:
os.listdir(directory)
except FileNotFoundError:
os.makedirs(directory)
“`
In the code above, we try to list the contents of the directory using `os.listdir()`. If a `FileNotFoundError` occurs, indicating that the directory doesn’t exist, we create the directory with `os.makedirs()`.
FAQs
Q1. What is the difference between `os.path.isdir()` and `os.path.exists()`?
The `os.path.isdir()` function specifically checks if a given path exists and is a directory. On the other hand, `os.path.exists()` checks if a path exists regardless of whether it is a file or a directory.
Q2. What if my directory path contains variables or user input?
If your directory path contains variables or user input, you can use string concatenation or string formatting to construct the path dynamically. Just ensure that the resulting path is valid and properly escaped.
Q3. Can I use these methods to check for the existence of multiple directories simultaneously?
Yes, you can utilize these methods to check the existence of multiple directories by iterating over a list of directories and applying the appropriate check or creation logic to each.
Q4. What if I want to create multiple nested directories at once?
All of the methods discussed in this article support the creation of nested directories. You can simply provide a path that includes multiple levels of directories, and the methods will create the entire hierarchy if it doesn’t exist.
Q5. What other operations can I perform on directories using these methods?
In addition to checking and creating directories, you can also perform operations such as renaming directories, deleting directories, moving directories, or obtaining directory statistics using the methods provided by the `os` module and `pathlib` module.
In conclusion, we have explored various methods to check if a directory exists and create it if it doesn’t in Python. By utilizing the `os` module, the `pathlib` module, or utilizing exception handling, you can easily handle directory creation in your Python scripts. These techniques provide flexibility and robustness to handle different scenarios when working with directories.
How To Check If A Folder Exists In Python?
When working with file operations in Python, it is often necessary to check whether a folder exists before performing any operations on it. Whether you are creating a new folder, reading files from a specific directory, or writing files to an existing folder, it is crucial to ensure that the folder exists beforehand. In this article, we will explore various methods to determine if a folder exists in Python and discuss when and how to utilize each approach effectively.
## Checking for a Folder’s Existence: The Basics
Python provides several ways to check if a folder exists, each with its own advantages and use cases. Let’s take a look at the most commonly used methods:
### 1. os.path.exists()
One of the simplest ways to check if a folder exists in Python is by using the `os.path.exists()` function. This method takes a path as input, whether it is an absolute or relative path, and returns `True` if the path exists, and `False` otherwise. However, it is worth mentioning that this method also returns `True` if a file with the specified name exists.
“`python
import os
folder_path = ‘/path/to/folder’
if os.path.exists(folder_path):
print(“Folder exists”)
else:
print(“Folder does not exist”)
“`
### 2. os.path.isdir()
Another useful method to determine if a folder exists is `os.path.isdir()`. This function returns `True` if the specified path exists and corresponds to a directory (i.e., folder), and `False` otherwise. Unlike `os.path.exists()`, this method specifically checks if the given path corresponds to a directory rather than a file.
“`python
import os
folder_path = ‘/path/to/folder’
if os.path.isdir(folder_path):
print(“Folder exists”)
else:
print(“Folder does not exist”)
“`
### 3. glob.glob()
The `glob` module, widely used for file searching operations, can also be leveraged to check folder existence. By using the `glob.glob()` function, we can search for the directory name and retrieve the matching paths. If there are any matches, it indicates that the folder exists. However, keep in mind that this method returns a list of matching paths rather than a single boolean value.
“`python
import glob
folder_path = ‘/path/to/folder’
matches = glob.glob(folder_path)
if matches:
print(“Folder exists”)
else:
print(“Folder does not exist”)
“`
### 4. pathlib.Path()
Introduced in Python 3.4, the `pathlib` module provides an object-oriented approach to handle file system paths. We can utilize the `Path()` function from this module to check if a folder exists. By calling the `Path().exists()` method, we can directly determine if the specified path exists.
“`python
import pathlib
folder_path = ‘/path/to/folder’
if pathlib.Path(folder_path).exists():
print(“Folder exists”)
else:
print(“Folder does not exist”)
“`
## FAQs
### Q1: Can these methods be used to check for the existence of a file?
Yes, some of the methods mentioned above, such as `os.path.exists()` and `glob.glob()`, can also be used to check if a file exists. However, it is important to remember that these methods do not specifically distinguish between files and directories. To check solely for the existence of a file, you can use `os.path.isfile()`.
### Q2: What is the difference between `os.path.exists()` and `os.path.isdir()`?
While `os.path.exists()` checks if a given path exists, regardless of whether it corresponds to a file or a folder, `os.path.isdir()` specifically verifies the existence of a directory/folder. The latter function returns `True` only if the path exists and is a directory.
### Q3: Which method should I use to check for a folder’s existence?
The choice of method depends on your specific requirements. If you need a simple boolean result regarding the existence of a folder, `os.path.exists()` or `os.path.isdir()` are suitable options. On the other hand, if you also require the matching paths or a more object-oriented approach, `glob.glob()` or `pathlib.Path()` respectively can be used.
### Q4: What is a relative path and how does it differ from an absolute path?
A relative path is a path specified relative to the current working directory. It does not include the entire directory hierarchy starting from the root directory. An absolute path, on the other hand, specifies the complete directory hierarchy starting from the root directory. Operating systems like Linux and macOS use forward slashes (/) to represent the directory hierarchy, whereas Windows uses backslashes (\).
## Conclusion
Ensuring the existence of a folder before performing file operations is essential for a smooth and error-free program execution. In this article, we covered various methods to check if a folder exists in Python, including `os.path.exists()`, `os.path.isdir()`, `glob.glob()`, and `pathlib.Path()`. Each method has its own strengths, and the choice depends on the desired output and specific requirements of your project. By utilizing these techniques effectively, you can handle folders and files with confidence in your Python programs.
Keywords searched by users: python create folder if not exists Os mkdir if not exists, Python create file if not exists, Path mkdir if not exists, Create folder Python, Python check directory exists if not create, Python copy file auto create directory if not exist, Os mkdir exist ok, Check folder exist Python
Categories: Top 37 Python Create Folder If Not Exists
See more here: nhanvietluanvan.com
Os Mkdir If Not Exists
Introduction:
In the realm of file and directory manipulation using Python, the “os.mkdir if not exists” command holds significant significance. This command aids in creating a directory with a check to ensure that it doesn’t already exist, preventing errors and eliminating redundancy. In this article, we will delve into the details of this command, exploring its functionalities, implementations, and use cases.
Understanding “os.mkdir if not exists” Command:
“os.mkdir if not exists” is a Python function that allows developers to conveniently create a new directory on their system. The command checks if the directory already exists before trying to create it, ensuring that unnecessary errors are avoided. This feature eliminates the need for additional error handling mechanisms while adding simplicity to the code.
Implementation:
To use the “os.mkdir if not exists” command, first, we need to import the ‘os’ module in Python. This module provides a way to interact with different operating systems and perform necessary operations. Next, import the ‘errno’ module to handle errors efficiently. The code snippet below demonstrates the implementation:
“`python
import os
import errno
directory = “new_directory”
try:
os.mkdir(directory)
except OSError as e:
if e.errno != errno.EEXIST:
raise
“`
In this example, we attempt to create a new directory named “new_directory” using the os.mkdir() function. However, if the directory already exists, the OSError exception is raised. By checking the error number (errno) received, we can ensure that the command only raises the exception if the directory creation fails for reasons other than it already existing.
Use Cases:
The “os.mkdir if not exists” command finds its applications in various scenarios. Here are a few examples:
1. Automated Data Storage:
When developing programs that handle data storage, it is often necessary to create new directories. The “os.mkdir if not exists” command enables the creation of directories with dynamically generated names, ensuring no duplication of directories occurs.
2. Logging and Debugging:
Many projects rely on logging and debugging techniques for error tracking and output monitoring. By incorporating the “os.mkdir if not exists” command, developers can systematically organize log and debug directories, simplifying the management of generated logs and debug files.
3. Data Processing and Analysis:
While working on data-driven projects, creating directories to store datasets, intermediate outputs, or analysis results becomes crucial. This command assists in automatically creating the necessary directories, eliminating the risk of overwriting existing files or encountering unnecessary errors.
4. Command-Line Tools:
Developers often create command-line tools to streamline repetitive tasks. Using “os.mkdir if not exists” in such tools can ensure that required directories are automatically created, reducing the need for manual intervention.
FAQs:
Q1. Can the “os.mkdir if not exists” command create multiple directories at once?
A1. No, the command is designed to create a single directory at a time. To create multiple directories, you can iterate through a list using a loop like a for-loop or a list comprehension.
Q2. How can I specify the path for the directory I want to create?
A2. To specify the directory path, concatenate the desired path with the directory name, ensuring proper separators. For example, `folder_path = ‘/path/to/folder/’ + directory_name`.
Q3. Can I use relative paths when creating directories with “os.mkdir if not exists”?
A3. Yes, you can use relative paths while creating directories. The current working directory (CWD) will be the starting point based on which the path will be resolved.
Q4. How can I handle the situation when the directory cannot be created due to permission issues?
A4. If you encounter a permission denied error while trying to create a directory, you can handle it by catching the OSError exception and then taking appropriate actions based on your requirements. For example, presenting a user-friendly error message or changing the target directory.
Q5. Is there a way to disable the exception and silently continue if the directory already exists?
A5. While it is advisable to handle exceptions to ensure the execution of reliable code, you can use the os.path.exists() function before attempting to create the directory to bypass the exception-raising mechanism.
Conclusion:
The “os.mkdir if not exists” command in Python simplifies the process of creating directories by eliminating errors related to duplicate directories. By incorporating this command into your code, you can ensure that your programs create new directories only when necessary and handle pre-existing ones smoothly. Understanding the implementation and use cases of this command will allow you to create cleaner and more efficient Python code.
Python Create File If Not Exists
Python is a versatile programming language that provides numerous functionalities for file handling. In this article, we will focus on creating a file in Python, specifically addressing the scenario of creating a file only if it does not already exist. We will explore different approaches, explain their implementation, and provide useful code snippets. Additionally, we will answer some frequently asked questions related to this topic.
Creating a file in Python is a straightforward process. However, it becomes slightly more complicated when we want to avoid overwriting an existing file by accident. Fortunately, Python provides us with various methods to handle this situation.
One of the simplest ways to create a file if it does not exist is using the `open` function with the “x” mode. Let’s take a look at an example:
“`python
filename = “example_file.txt”
try:
file = open(filename, “x”)
except FileExistsError:
print(f”File {filename} already exists”)
else:
print(f”{filename} created successfully”)
file.close()
“`
In this code snippet, we define a `filename` variable to hold the desired name of our file (e.g., “example_file.txt”). We then use the `open` function with the “x” mode, which is exclusively used for creating new files but raises a `FileExistsError` if the file already exists. This error can be caught using a `try-except` block, allowing us to handle it gracefully. Within the `except` block, we inform the user that the file already exists. If the `try` block executes successfully, we close the file and print a success message.
Another approach to create a file only if it does not exist involves using the `os` module. The `os.path.exists` function checks whether a file or directory exists at a given path. By combining it with the `open` function, we can create a new file conditionally. Here’s an example:
“`python
import os
filename = “example_file.txt”
if not os.path.exists(filename):
file = open(filename, “w”)
file.close()
print(f”{filename} created successfully”)
else:
print(f”File {filename} already exists”)
“`
In this code snippet, we import the `os` module and define the `filename` variable. By using `os.path.exists` with the desired filename, we determine if the file already exists. If it doesn’t, we proceed to create a new file using the `open` function with the “w” mode (write mode). Finally, we close the file and provide feedback to the user.
While the above methods are effective, they require multiple lines of code. For a more concise solution, we can take advantage of the `pathlib` module, introduced in Python 3.4. The `Path` class from the `pathlib` module provides a simpler and more expressive way to manipulate file system paths. Here’s an example:
“`python
from pathlib import Path
filename = “example_file.txt”
file = Path(filename)
if not file.is_file():
file.touch()
print(f”{filename} created successfully”)
else:
print(f”File {filename} already exists”)
“`
In this code snippet, we import the `Path` class from the `pathlib` module. We then create a `Path` object with the desired filename. By using the `is_file` method, we determine if the file exists. If it doesn’t, we call the `touch` method to create the file. Lastly, we provide appropriate feedback to the user.
Frequently Asked Questions:
Q: Are there any risks associated with creating files using these methods?
A: When using the “x” mode or checking for file existence, keep in mind that race conditions could occur if multiple processes or threads simultaneously try to create the same file. To mitigate this risk, you can use file-locking mechanisms or consider alternative solutions.
Q: How can I create a file with a specific path?
A: To create a file with a specific path, you can provide the complete path (including directories) when defining the `filename` variable. The methods mentioned above will create the file at the specified location if it does not already exist.
Q: Can I create files with different extensions?
A: Yes, you can create files with any extension. The examples provided in this article use the “.txt” extension for simplicity, but you can create files with any desired extension by specifying it in the `filename` variable.
Q: Is it possible to create multiple files simultaneously?
A: Yes, you can create multiple files simultaneously by applying the aforementioned methods in a loop, providing different filenames at each iteration.
In conclusion, creating a file if it does not exist in Python can be easily achieved using various methods. Whether you opt for the “x” mode, the `os` module, or the `pathlib` module, Python provides flexible options to fulfill your requirements. Remember to consider possible race conditions and explore additional functionalities to enhance your file handling capabilities.
Images related to the topic python create folder if not exists
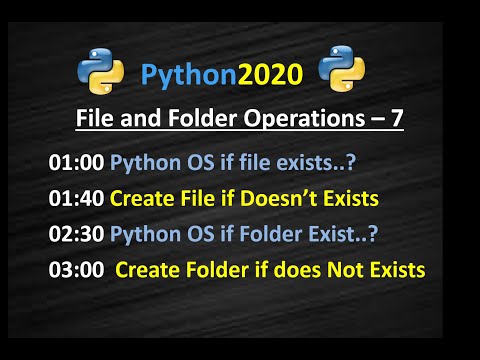
Found 48 images related to python create folder if not exists theme
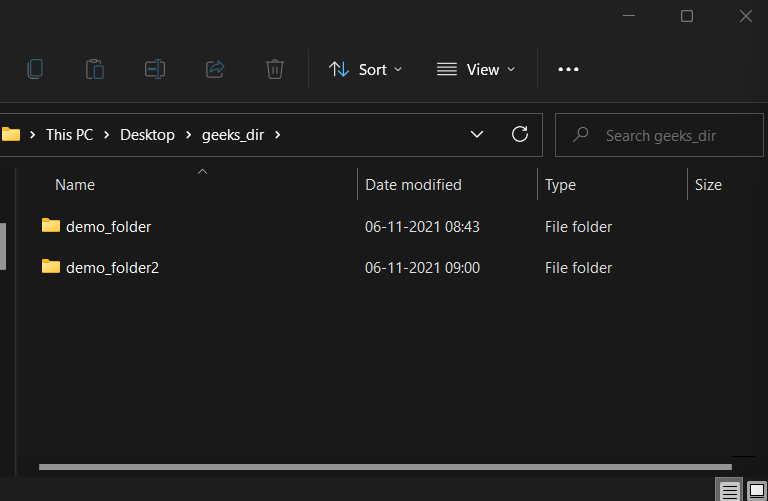
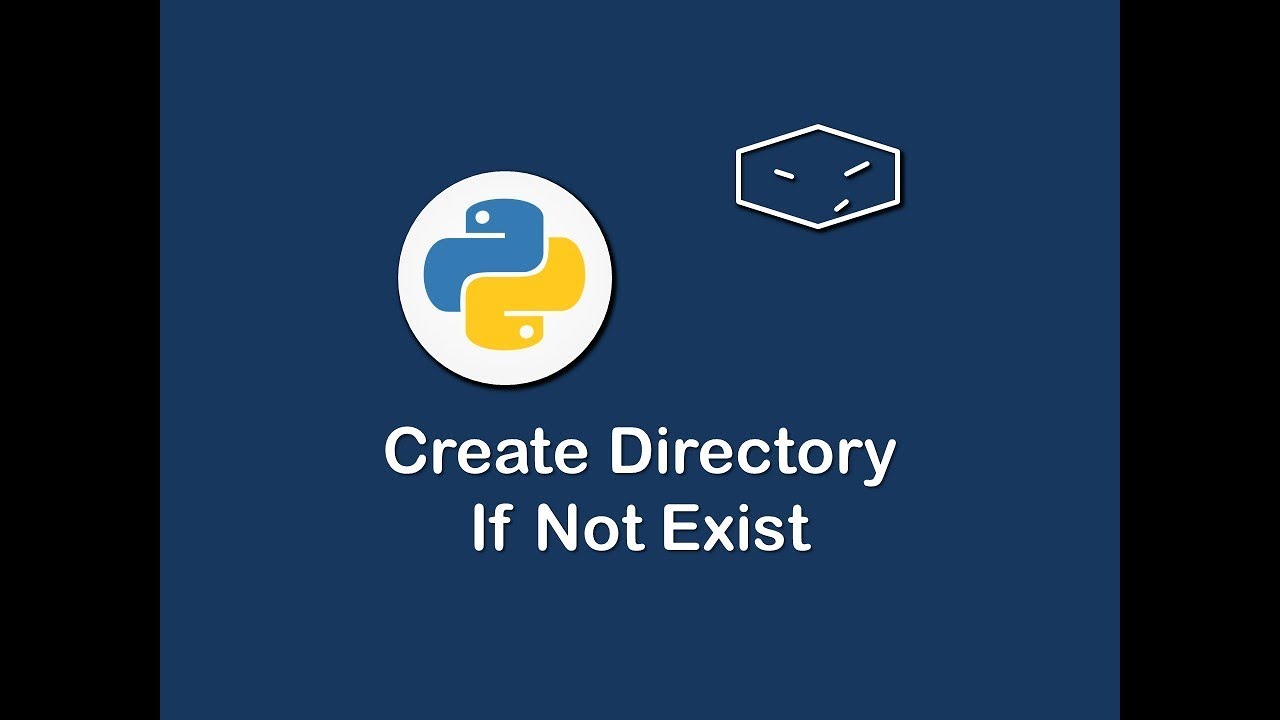
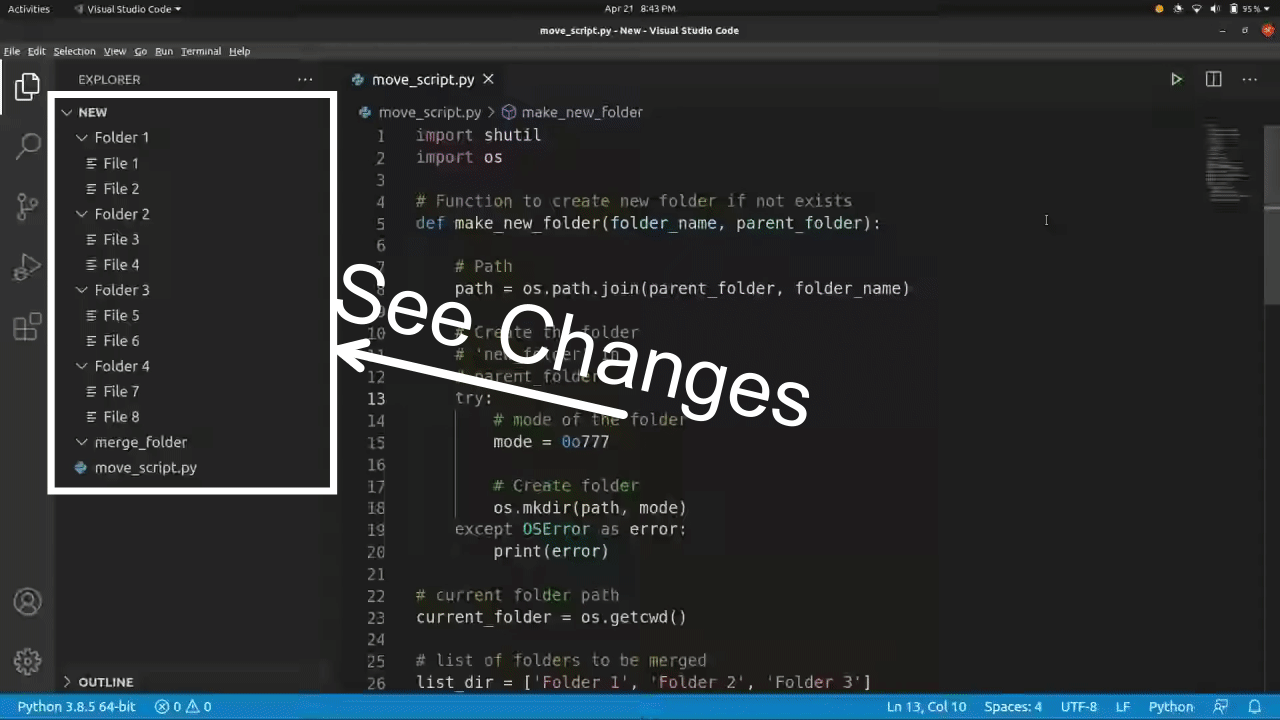
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/output-2.png)
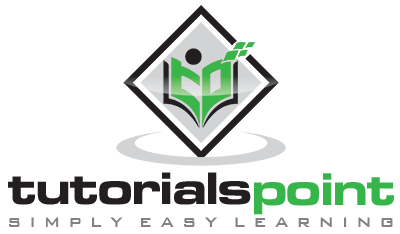

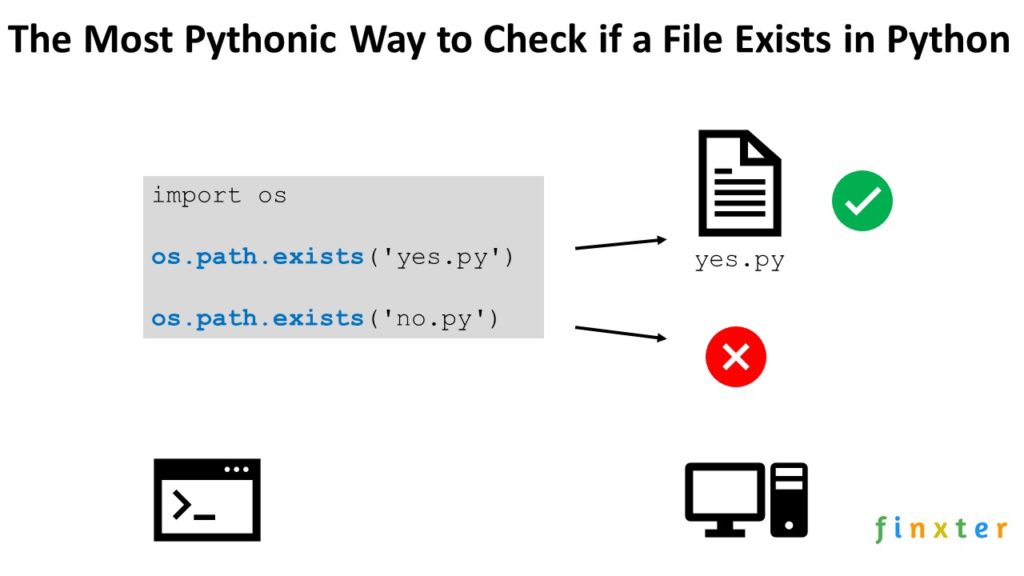

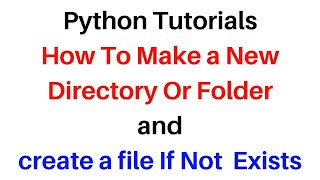
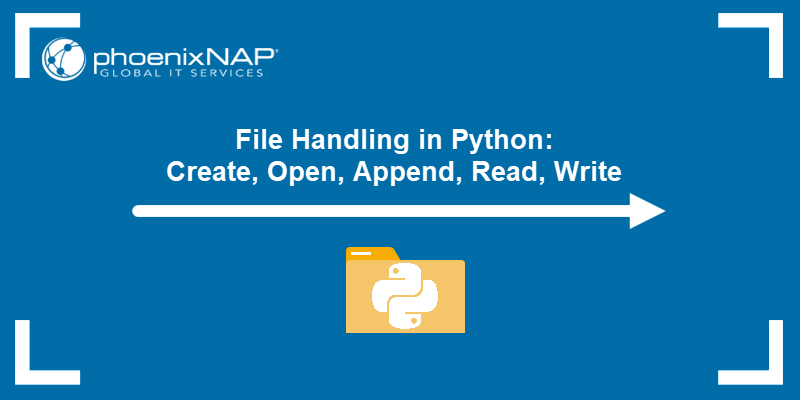
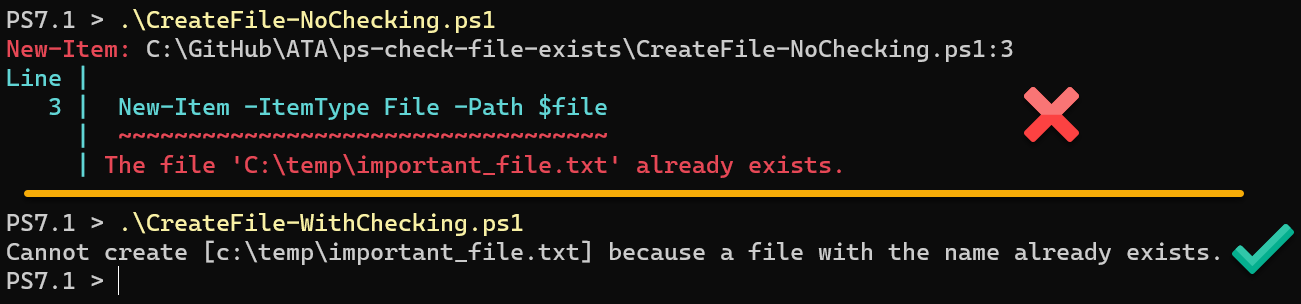
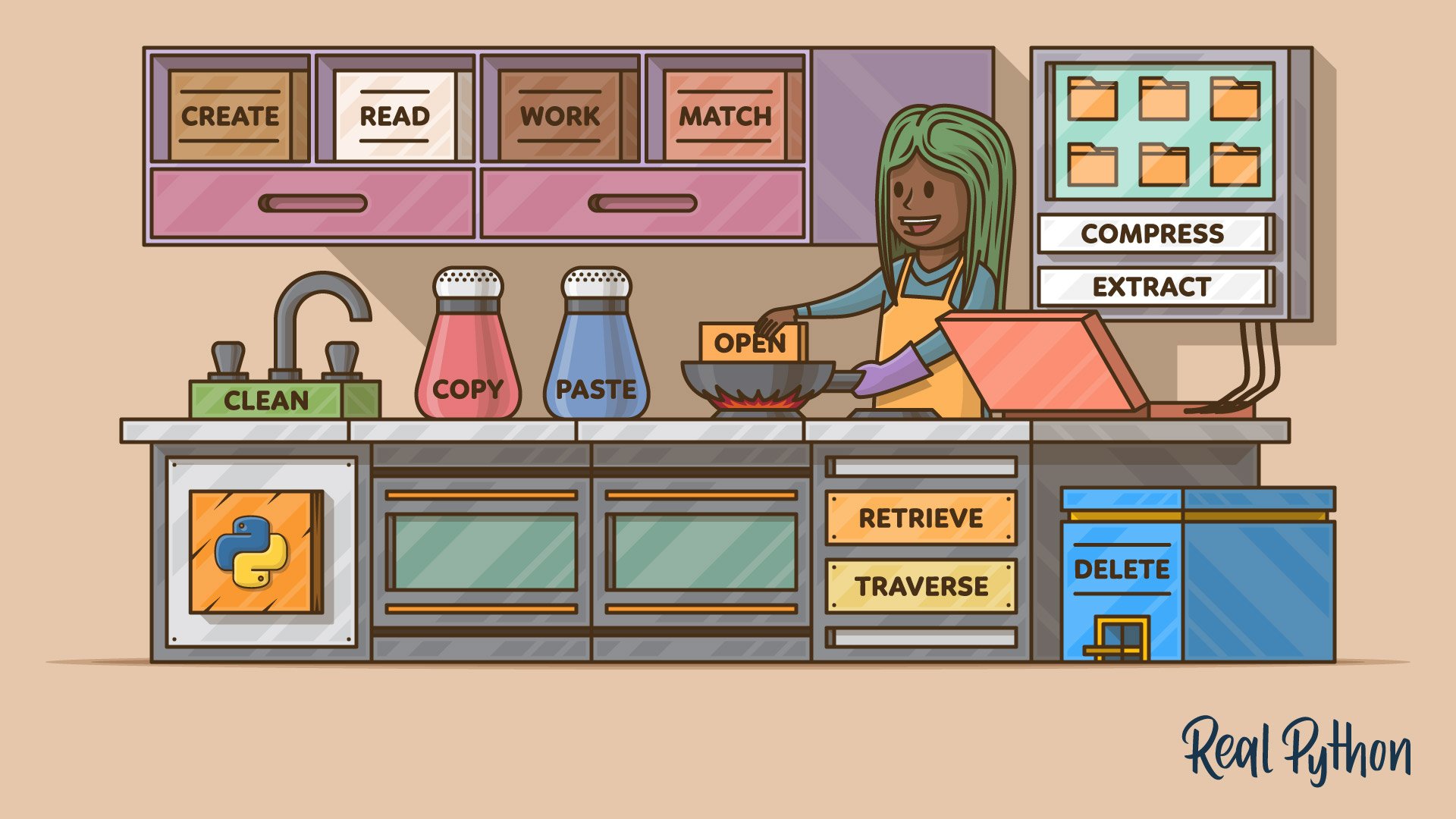
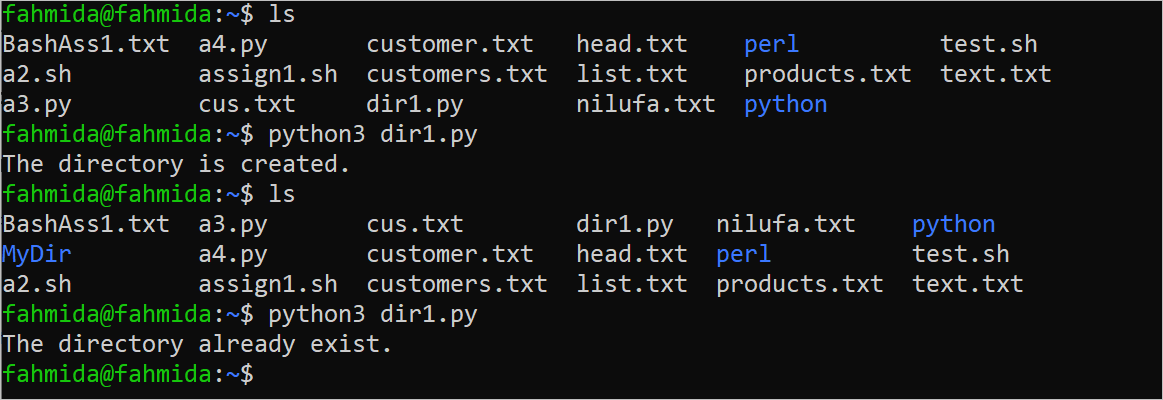
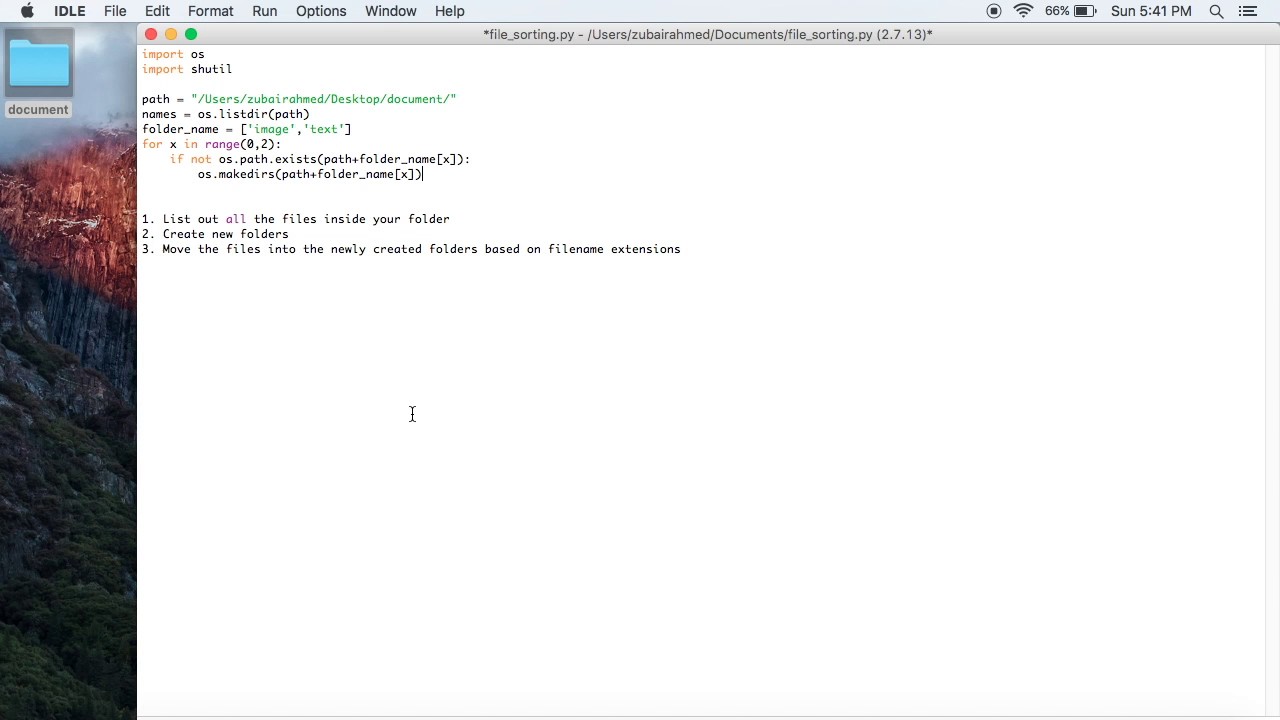

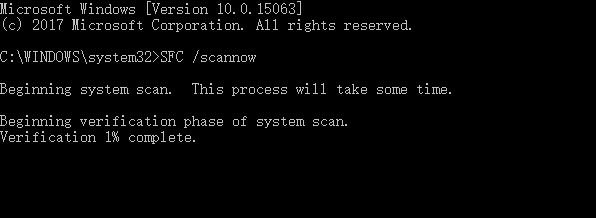

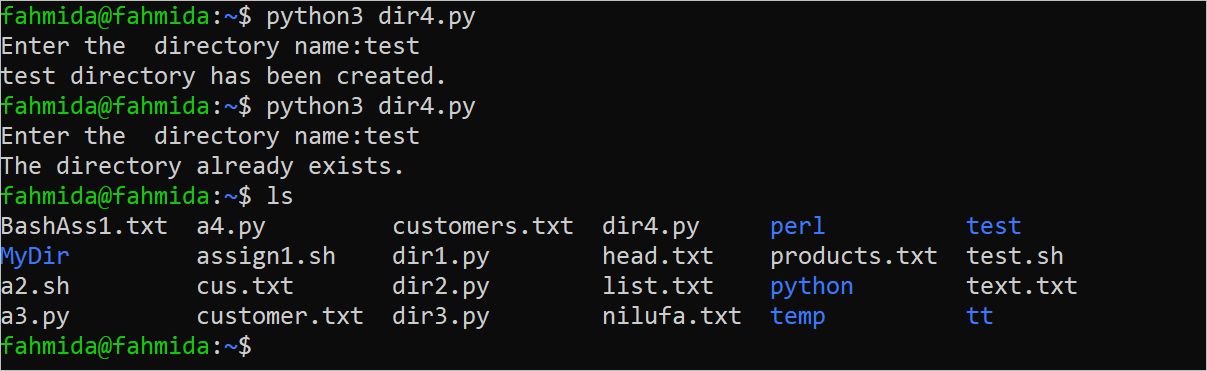
![Create File in Python [4 Ways] – PYnative Create File In Python [4 Ways] – Pynative](https://pynative.com/wp-content/uploads/2021/06/created-files.png)
![Solved: Node.js create directory if doesn't exist [Examples] | GoLinuxCloud Solved: Node.Js Create Directory If Doesn'T Exist [Examples] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/all-fs-methods-1.png)

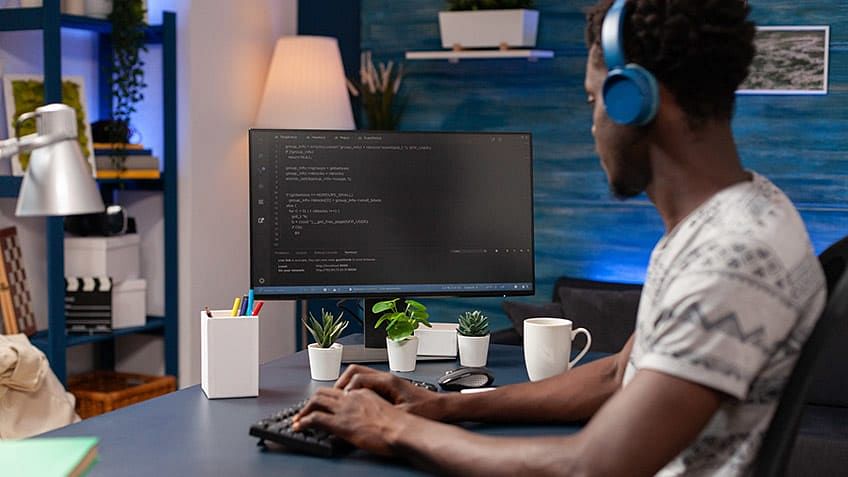
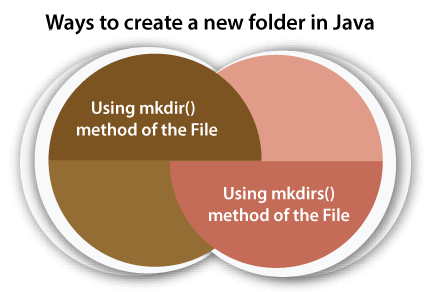
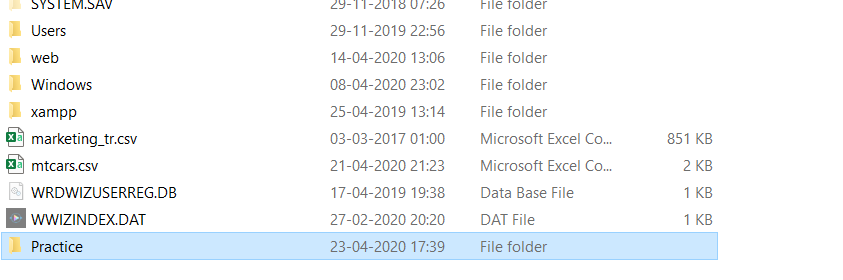
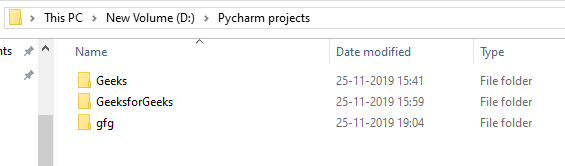
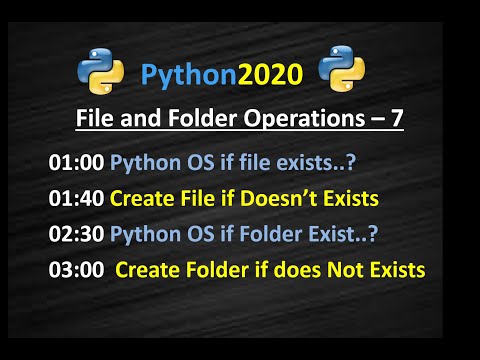
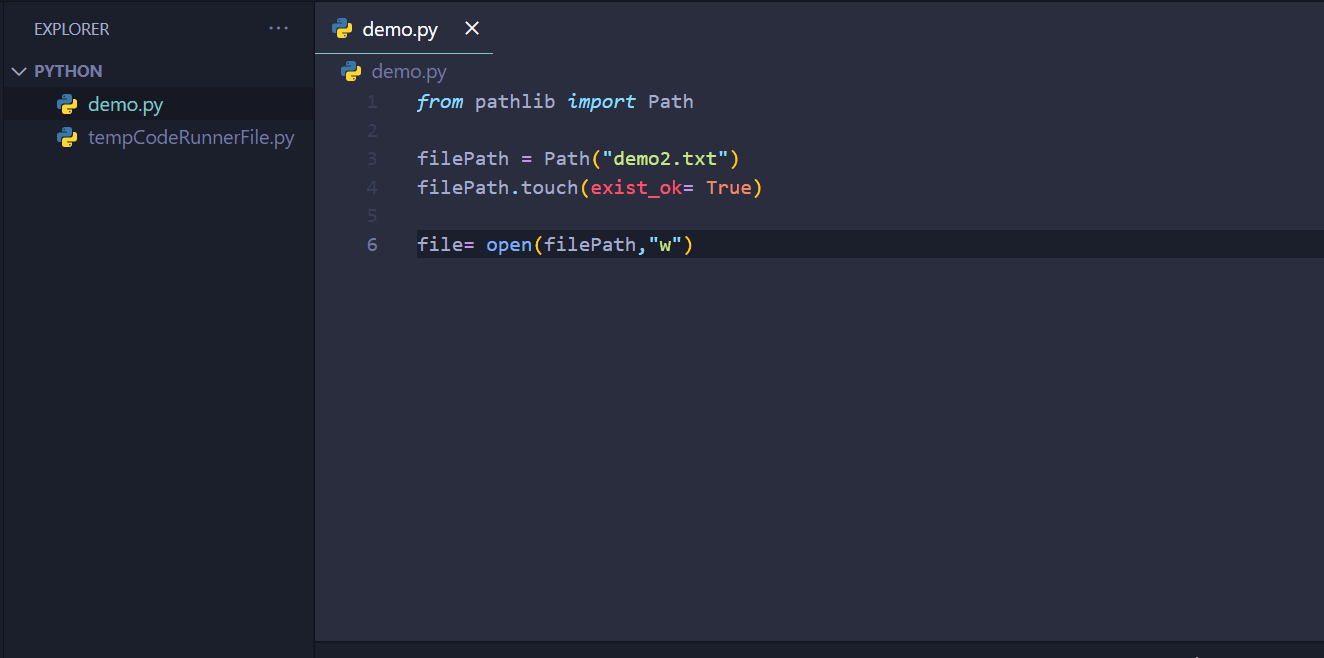
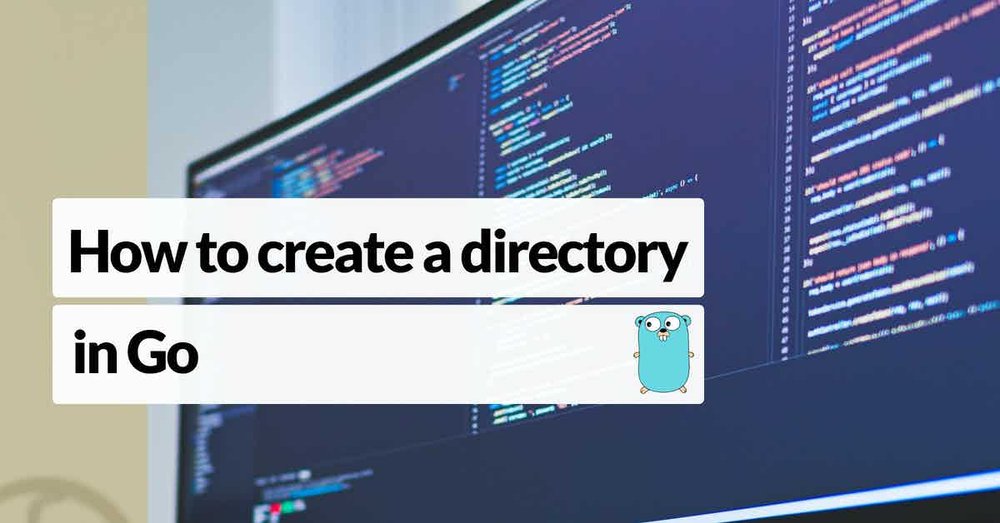
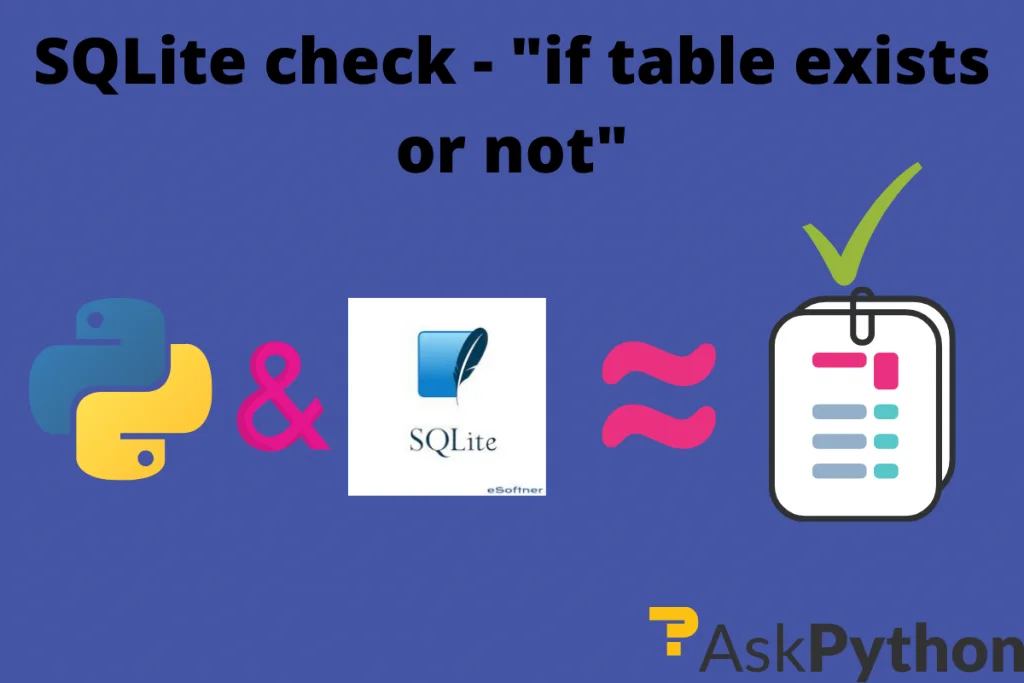

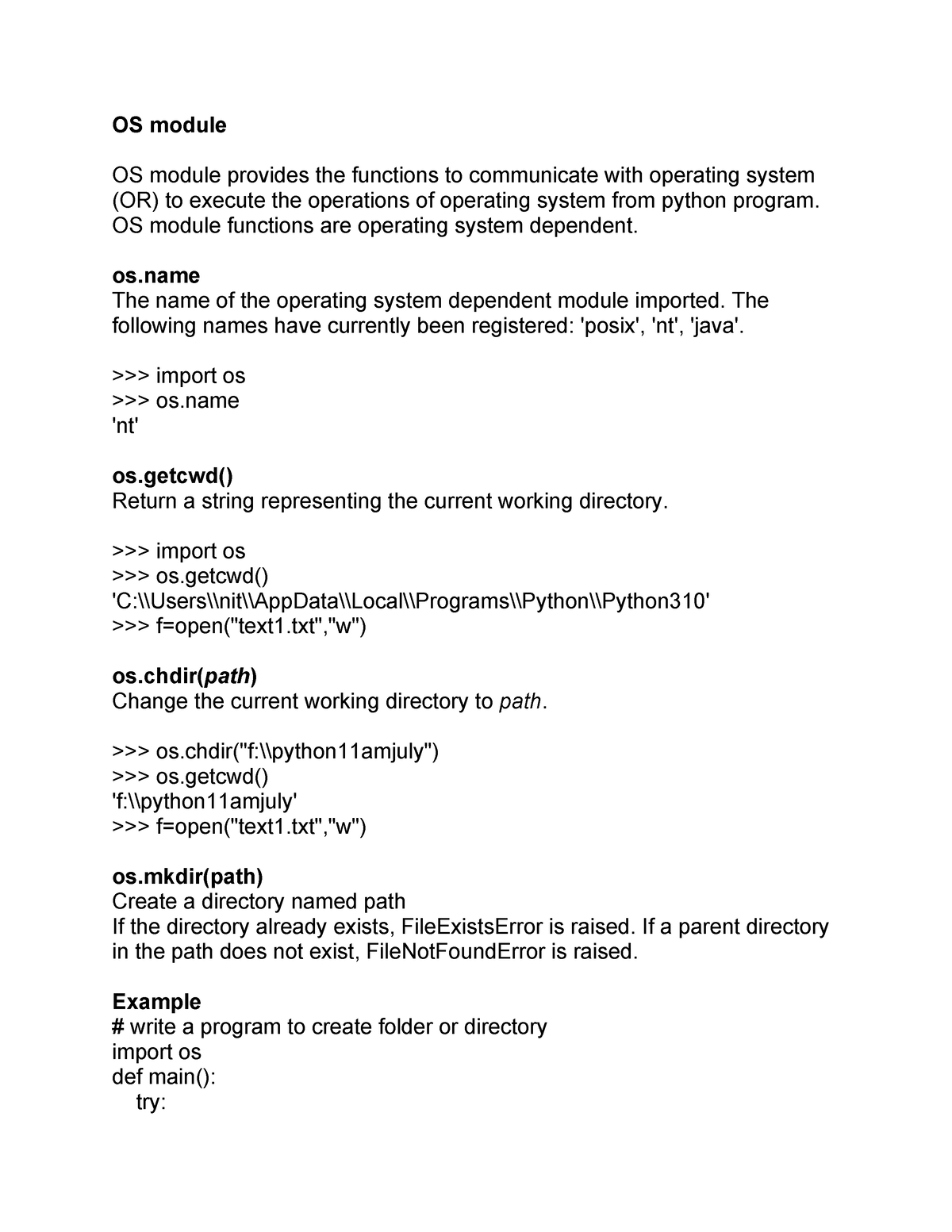
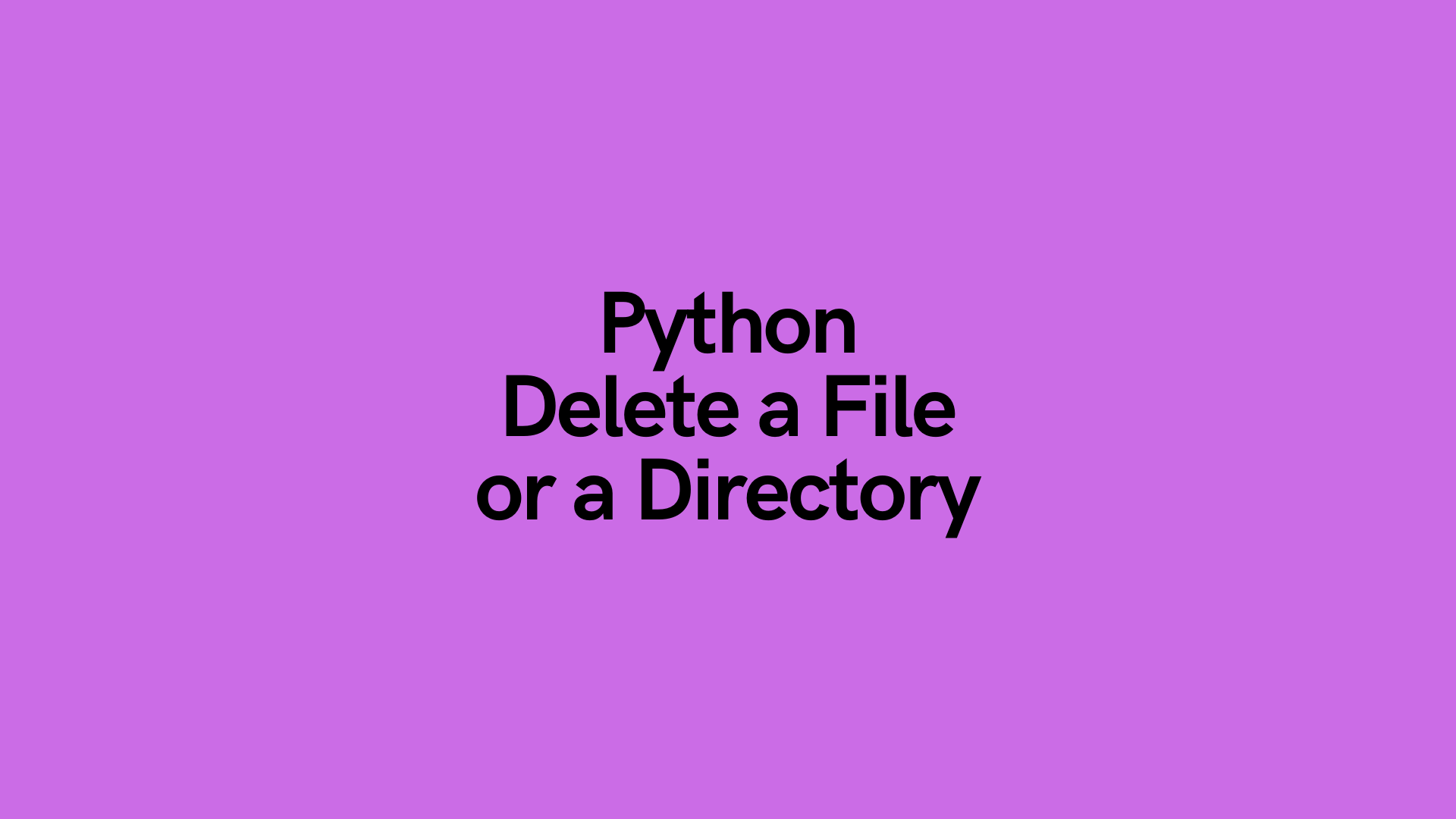
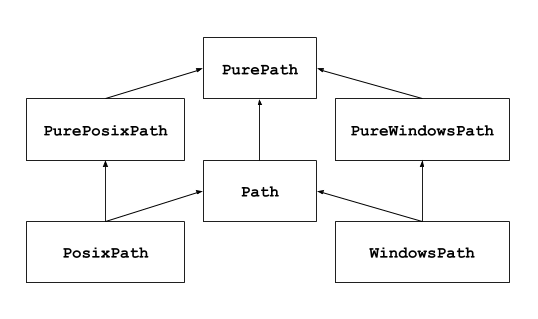
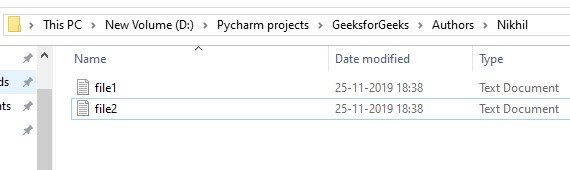
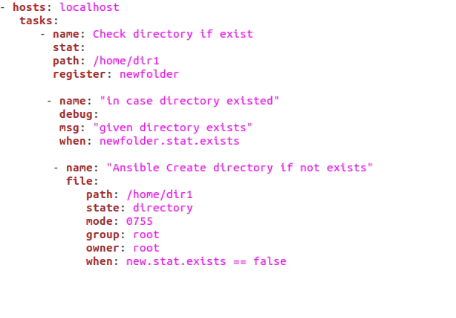

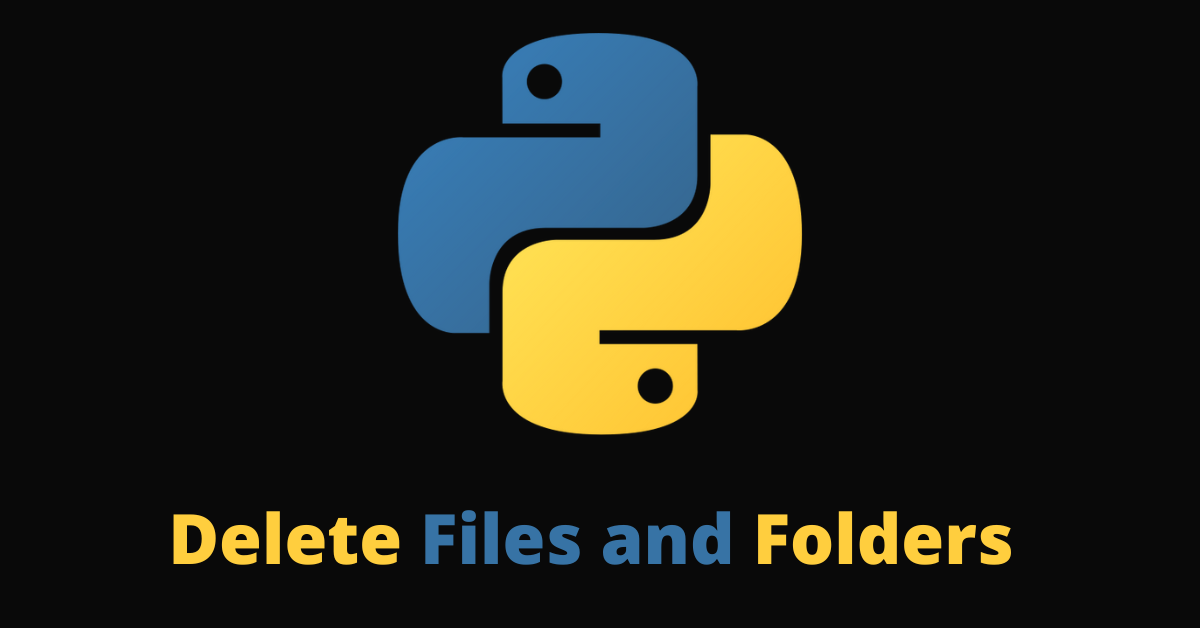
![FileNotFoundError: [Errno 2] No such file or directory – Its Linux FOSS Filenotfounderror: [Errno 2] No Such File Or Directory – Its Linux Foss](https://itslinuxfoss.com/wp-content/uploads/2022/11/Errno-2-No-such-file-or-directory-1.png)
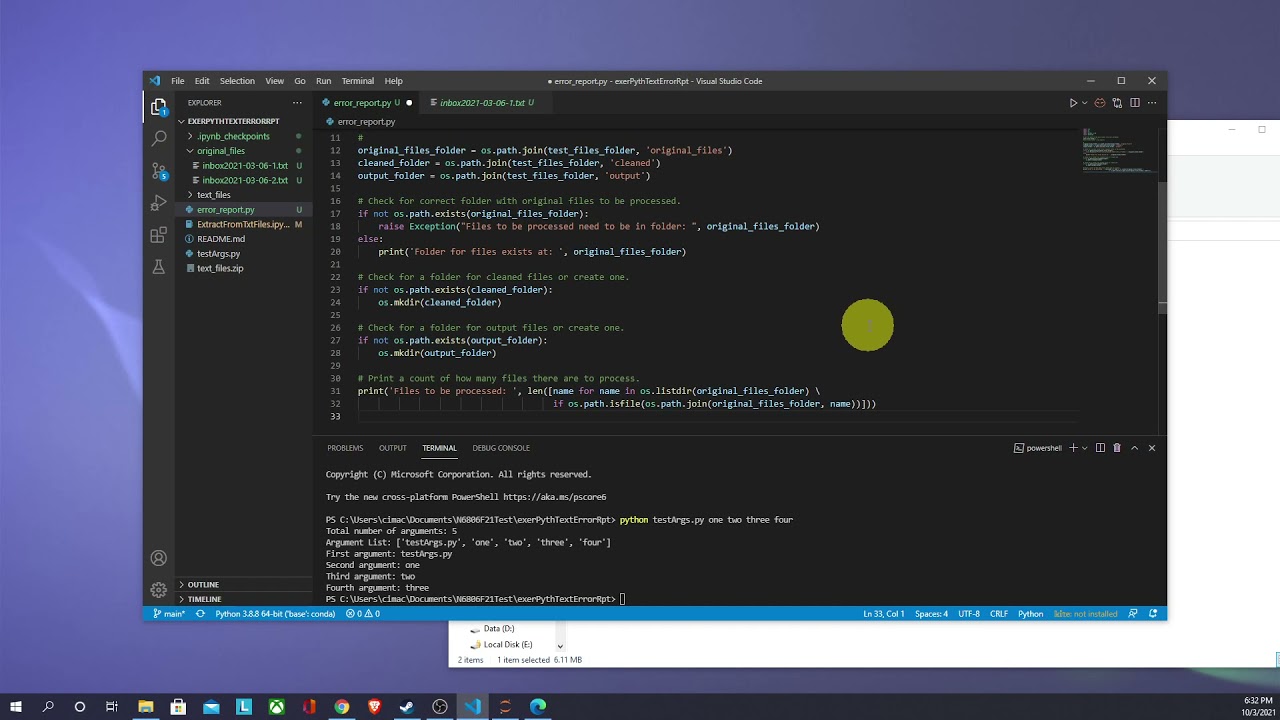


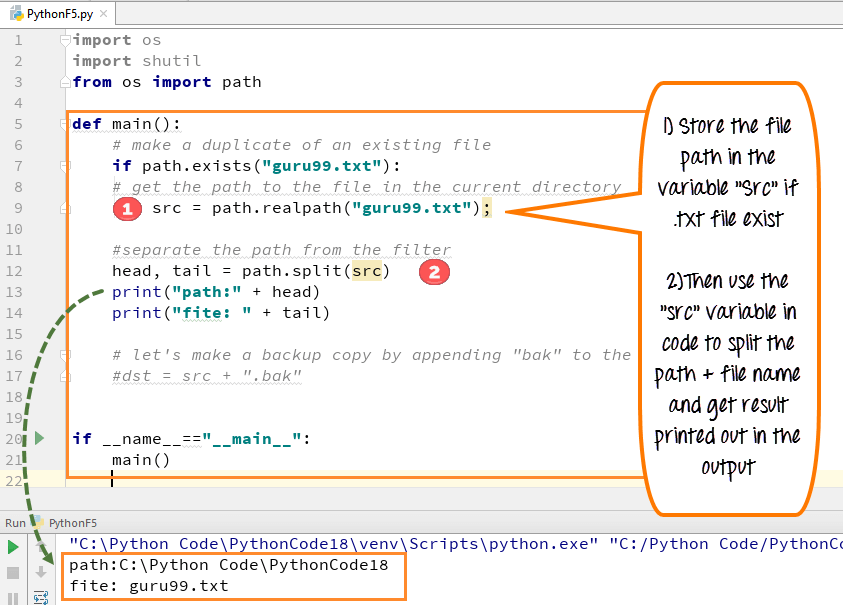
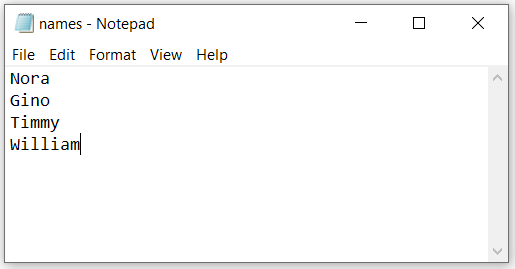

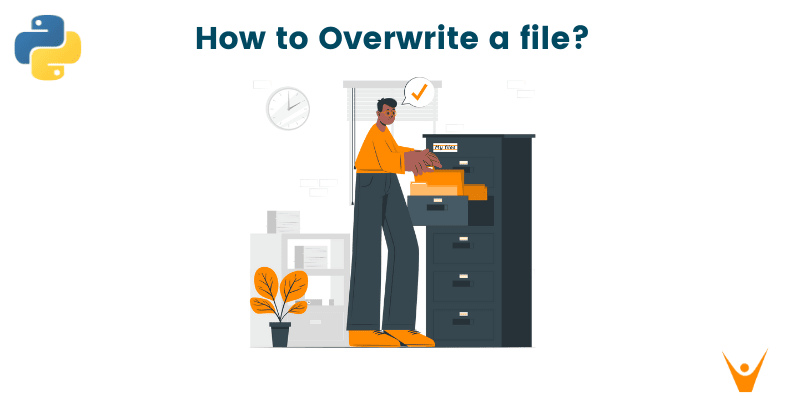
Article link: python create folder if not exists.
Learn more about the topic python create folder if not exists.
- How can I create a directory if it does not exist using Python
- How to Create Directory If Not Exists in Python – AppDividend
- How do I create a directory, and any missing parent directories?
- How can I create a directory in Python using ‘mkdir if not exists’?
- How to Create Directory If it Does Not Exist using Python?
- Python: Check if a File or Directory Exists – GeeksforGeeks
- Python Check if File Exists: How to Check if a Directory Exists?
- Python: Create a Directory if it Doesn’t Exist – Datagy
- Create directory if it does not exist in Python
- Python Create Directory If Not Exists Top 17 Latest Posts
- Creating a Directory in Python – How to Create a Folder
- Create Directory if not Exists in Python – Programming Basic
See more: nhanvietluanvan.com/luat-hoc