Python Create Empty Set
An empty set is a data structure in Python that is used to store a collection of unique elements. In this article, we will discuss various methods to create an empty set in Python and explore some common use cases for empty sets. We will also provide examples demonstrating the practical usage of empty sets.
Using the set() function
One way to create an empty set in Python is by using the set() function. This function initializes an empty set.
Example:
“`python
set_var = set()
“`
Using curly braces {}
Another method to create an empty set in Python is by using curly braces. Simply enclose the curly braces within a variable assignment to initialize an empty set.
Example:
“`python
set_var = {}
“`
Using the set literal syntax
A third method to create an empty set in Python is by using set literal syntax. This involves using the set keyword followed by empty parentheses.
Example:
“`python
set_var = set()
“`
Understanding the characteristics of an empty set
An empty set in Python has several characteristics that are important to understand:
1. Mutable data type: An empty set is considered to be a mutable data type, which means that its elements can be modified or updated.
2. No duplicate elements: In an empty set, duplicate elements are not allowed. Each element in the set must be unique.
3. Output: When an empty set is printed, it will output an empty set object, represented by empty curly braces: `{}`.
Common use cases for empty sets
Empty sets are commonly used in various scenarios in Python. Some of the common use cases for empty sets are:
1. Collecting unique elements: Empty sets are useful for collecting a collection of elements where duplicates are not allowed. The unique property of sets can be leveraged to easily collect distinct elements.
2. Set operations: Empty sets can be used in set operations such as union, intersection, and difference. They can be combined with other sets to perform these operations efficiently.
3. Placeholder for future data: Empty sets can be used as placeholders for future data. They can be initialized and later be filled with elements as and when required.
Examples demonstrating the use of empty sets
Let’s now look at some examples that illustrate the practical usage of empty sets in Python.
Example: Combining sets using the union operation with an empty set.
“`python
set1 = {1, 2, 3}
empty_set = set()
combined_set = set1.union(empty_set)
print(combined_set) # Output: {1, 2, 3}
“`
Example: Finding common elements using the intersection operation with an empty set.
“`python
set1 = {1, 2, 3}
empty_set = set()
common_elements = set1.intersection(empty_set)
print(common_elements) # Output: set()
“`
Example: Obtaining the difference between two sets using the difference operation with an empty set.
“`python
set1 = {1, 2, 3}
empty_set = set()
difference = set1.difference(empty_set)
print(difference) # Output: {1, 2, 3}
“`
In the above examples, we can see how empty sets can be combined with other sets to perform set operations such as union, intersection, and difference.
In conclusion, creating an empty set in Python can be done using various methods such as the set() function, curly braces, or set literal syntax. Empty sets are versatile data structures that are commonly used for collecting unique elements, performing set operations, and serving as placeholders for future data. By understanding the characteristics and practical usage of empty sets, you can effectively utilize them in your Python programs.
FAQs
Q: How do I add elements to a set in Python?
A: Elements can be added to a set using the add() method or by using the union operation with another set.
Q: How can I check if a set is present in another set in Python?
A: You can use the issubset() method to check if a set is present in another set. This method returns True if all elements of the set are present in the other set, otherwise it returns False.
Q: How can I check if a set is empty in Python?
A: You can use the len() function to check the length of a set. If the length is zero, then the set is empty.
Q: How can I append elements to a set in Python?
A: Sets in Python are unordered collections of unique elements, so they do not support the append() method. To add elements, you can use the add() method.
Q: How do I convert a list to a set in Python?
A: You can convert a list to a set using the set() function. Simply pass the list as an argument to the set() function.
Q: How can I perform set operations on two sets in Python?
A: To perform set operations such as union, intersection, or difference on two sets in Python, you can use the built-in methods union(), intersection(), and difference() respectively.
Q: What are some other methods available for sets in Python?
A: Some other useful methods available for sets in Python include discard(), remove(), pop(), clear(), symmetric_difference(), and isdisjoint().
Q: How can I delete a set in Python?
A: You can use the del keyword to delete a set in Python.
How To Create An Empty Set In Python ? Questions #Shorts
Can You Create An Empty Set In Python?
Python is a powerful and versatile programming language that offers a wide array of data structures to effectively manipulate and store information. One of the essential data types provided by Python is a set. Sets are unordered collections of unique elements that are widely used when there is a need to store and analyze distinct items.
Python provides a straightforward method to define an empty set. By using the curly brackets { } or the set() function, you can create an empty set. However, it’s crucial to differentiate between creating an empty set and creating an empty dictionary in Python, as both structures use curly brackets. If you initialize a variable using only curly brackets, Python will assume it is an empty dictionary, not a set. To explicitly create an empty set, you must invoke the set() function without any arguments or use the set literal syntax with curly brackets.
Here are a few examples to illustrate how to create an empty set in Python:
“` python
empty_set = set()
print(empty_set) # Output: set()
“`
In this example, the set() function is used without any argument to create an empty set and assign it to the variable “empty_set.” The print statement displays the contents of the set, which is an empty set represented by “set()”.
“` python
empty_set = {}
print(type(empty_set)) # Output:
“`
In this case, using only curly brackets initializes an empty dictionary, as indicated by the output of the type() function. It clarifies the importance of employing the set() function or the explicit set literal syntax.
To verify whether a set is empty, you can use the built-in len() function. The len() function returns the number of elements in a data structure. If the length of a set is zero, it means the set is empty.
“` python
empty_set = set()
print(len(empty_set)) # Output: 0
“`
The len() function is applied to the empty set, and the output of zero confirms that the set is empty.
FAQs:
Q: Is there any difference between an empty set and a set with no elements?
A: No, there is no difference. An empty set and a set with no elements are the same conceptually. Both indicate the absence of any elements in the set.
Q: Can you add elements to an empty set?
A: Yes, you can add elements to an empty set. The primary purpose of creating an empty set is to populate it with elements later on.
Q: Can a set contain duplicate elements?
A: No, a set does not allow duplicate elements. When adding elements to a set, any duplicates are automatically eliminated, ensuring that each element remains unique.
Q: How does a set differ from other data structures?
A: A set has distinct characteristics that set it apart from other data structures in Python. First, a set can only store unique elements. Second, sets are unordered, meaning the elements are not stored in a specific sequence. Lastly, sets are mutable, allowing for the addition and removal of elements.
Q: What are some common use cases for sets in Python?
A: Sets are commonly used when there is a need to store and manipulate distinct elements. Some use cases include removing duplicates from a list, performing set operations such as union, intersection, and difference, and checking for membership or existence of elements.
In conclusion, creating an empty set in Python is a simple task. By using either the set() function or the explicit set literal syntax, you can define an empty set. Remember to distinguish between an empty set and an empty dictionary, as both use curly brackets. Sets offer a powerful and efficient way to handle distinct elements in Python and are fundamental when solving various programming problems.
How To Initialize A Set In Python?
In Python, a set is an unordered collection of unique elements enclosed within curly braces {}. Initializing a set is the process of creating a new set and adding elements into it. Sets in Python are quite similar to mathematical sets and provide several useful methods for performing operations like union, intersection, and difference on them. This article will guide you through the process of initializing a set in Python and cover the topic in depth.
Initializing an Empty Set
To initialize an empty set in Python, you can use the set() function. The set() function returns an empty set. Let’s see an example:
“`python
myset = set()
print(myset)
“`
Output:
“`
set()
“`
In this example, we created an empty set using the set() function and assigned it to the variable “myset”. The print statement then displays the contents of the set, which is an empty set.
Initializing a Set with Elements
To initialize a set with elements, you can pass an iterable (such as a list, tuple, or string) to the set() function. The set() function will extract unique elements from the iterable and create a set. Let’s see an example:
“`python
myset = set([1, 2, 3, 4, 4, 5])
print(myset)
“`
Output:
“`
{1, 2, 3, 4, 5}
“`
In this example, we passed a list `[1, 2, 3, 4, 4, 5]` to the set() function. The set() function extracted the unique elements from the list and initialized a set with those elements.
It’s important to note that sets only contain unique elements. If an iterable contains duplicate elements, the set will only store one instance of each element.
Initializing a Set using Curly Braces
Another way to initialize a set is by using the curly braces {} directly. However, this method has a limitation: if you want to create an empty set, you should use the set() function as shown earlier. Let’s see an example of initializing a set using curly braces:
“`python
myset = {1, 2, 3, 4, 5}
print(myset)
“`
Output:
“`
{1, 2, 3, 4, 5}
“`
In this example, we initialized a set with the elements 1, 2, 3, 4, and 5 using curly braces. The result is the same as when we used the set() function.
Frequently Asked Questions (FAQs):
Q1. Can a set contain duplicate elements?
No, a set in Python cannot contain duplicate elements. If you try to add duplicate elements to a set, only one instance of each element will be stored in the set.
Q2. What happens if I try to initialize a set with a non-iterable?
If you try to initialize a set with a non-iterable object, such as an integer or a floating-point number, you will encounter a TypeError. The set() function requires an iterable object to create a set.
Q3. Can I modify the elements of a set after initialization?
No, the elements of a set are immutable. Once a set is initialized, you cannot modify its elements. However, you can add or remove elements from a set using specific methods like add() and remove().
Q4. Can a set contain elements of different data types?
Yes, a set in Python can contain elements of different data types. For example, a set can contain a combination of integers, strings, or any other data type.
Q5. How can I check the number of elements in a set?
To check the number of elements in a set, you can use the len() function. The len() function returns the number of elements in a set.
Q6. Is the order of elements maintained in a set?
No, sets in Python are unordered. This means that the elements in a set do not have a specific order. If you need to maintain the order of elements, you can use a different data structure, such as a list.
Conclusion
Initializing a set in Python is a straightforward process. You can use the set() function to create an empty set or initialize it with elements from an iterable. Additionally, curly braces {} can be used to initialize a set directly. This article covered the topic in depth and provided some frequently asked questions related to set initialization. With this knowledge, you can now initialize sets in Python and begin leveraging their powerful capabilities.
Keywords searched by users: python create empty set python set(), Add element to set Python, Check Set in set Python, Check set empty python, Python set append, List to set Python, Set1 set2 python, Python set methods
Categories: Top 87 Python Create Empty Set
See more here: nhanvietluanvan.com
Python Set()
In Python, a set is an unordered collection of unique elements. It is a fundamental built-in data type that provides a range of powerful operations. This article will delve into the details of using the set() function, its features, and the benefits it brings to Python programmers. Moreover, a Frequently Asked Questions (FAQs) section is included to address common queries and clarifications regarding this versatile data structure.
1. Initialization and Basic Operations
Creating a set in Python is incredibly simple. The set() function can be used without any arguments to create an empty set, or it can be initialized with data in a specific format. For instance, a set of integers can be created as follows:
“`python
my_set = set([1, 2, 3, 4, 5])
“`
Sets have no duplicate elements, so any duplicate values present in the input list will be automatically removed. Furthermore, elements in a set are not stored in any particular order.
Sets in Python support a range of fundamental operations, including membership testing, length determination, and element removal. These operations can be performed using built-in functions such as len(), in, and remove(). Additionally, sets can be modified using set-specific operations like union, intersection, or difference.
2. Key Features and Functions
Python sets provide several advantageous features for programmers. Some key features include:
2.1. Uniqueness of Elements: Sets ensure that each element is unique, making them ideal for deduplication operations. This simplifies code logic and improves efficiency, especially when dealing with large datasets.
2.2. Fast Membership Testing: Sets offer fast membership testing using the ‘in’ keyword. Checking whether an element exists in a set is significantly faster than searching through a list or tuple.
2.3. Set Operations: Python sets support various set operations that facilitate data manipulation. These operations include union (|), intersection (&), difference (-), and symmetric_difference (^). These functions can be used to perform set arithmetic efficiently.
2.4. Mutable and Immutable Sets: Sets in Python can be both mutable and immutable. Mutable sets can be modified by adding or removing elements, while immutable sets provide the assurance that the elements within them will not change.
3. Benefits of Using Sets
The use of sets has several advantages, making them a valuable tool for Python programmers. Some notable benefits include:
3.1. Data Deduplication: Sets automatically remove any duplicate elements, making them perfect for deduplicating data. By converting a list into a set and then back to a list, duplicates can be easily removed.
3.2. Fast Membership Testing: Sets offer faster membership testing compared to lists or tuples. When working with large datasets, this advantage can significantly improve the efficiency of the code.
3.3. Set Operations: Set operations simplify complex tasks like finding unique elements, common elements between sets, or elements that appear in one set but not the other. These operations can be implemented compactly using built-in functions, reducing the lines of code and improving code readability.
3.4. Mathematical Set Operations: Python sets naturally represent mathematical sets, providing easy and efficient solutions for problems that involve set theory. These operations are particularly valuable while solving problems in fields like data science, operations research, and discrete mathematics.
FAQs:
Q1. Can a set contain elements of different data types?
Yes, a set can contain elements of different data types. Python sets are versatile and can include objects of various types such as integers, floats, strings, tuples, or even other sets.
Q2. Can a set contain mutable objects like lists or dictionaries?
No, a set cannot contain mutable objects like lists or dictionaries. Sets require their elements to be immutable to guarantee consistency and the uniqueness property. However, sets can contain tuples, as tuples are immutable collections.
Q3. Are sets ordered in Python?
No, sets are unordered collections in Python. Elements in a set do not have a fixed position or index. If order is important, one can use the ordered variant called “OrderedSet” from external libraries such as ‘collections’.
Q4. How do sets differ from lists and tuples?
Sets are distinct from both lists and tuples in Python. Sets are unordered and provide uniqueness of elements with fast membership testing, while lists and tuples are ordered collections that allow duplicate elements. Moreover, sets have specific set operations, while lists and tuples have their respective operations like indexing and slicing.
Q5. Are sets faster than lists or dictionaries?
The speed of operations can depend on various factors, including the specific use case and the data size. Sets excel at membership testing and deduplication operations due to their unique nature. On the other hand, lists or dictionaries may be more suitable for tasks like indexing or key-value pair access. It is essential to consider the specific requirements of the project when choosing the appropriate data structure.
In conclusion, the Python set() function provides a powerful data structure for handling collections of unique elements. Sets offer unique features like fast membership testing, set operations, and data deduplication capabilities. Utilizing sets can significantly simplify coding tasks and improve the efficiency of programs. By understanding the nuances and leveraging the strengths of sets, Python programmers can enhance their coding experience and produce more elegant and efficient code.
Add Element To Set Python
Sets are an essential data type in Python that allow you to store multiple unique elements in a single variable. One of the significant advantages of sets is their ability to quickly determine if an element is part of the set, thanks to their efficient hash-based implementation. Python provides several methods to manipulate sets efficiently, including adding elements to a set. In this article, we will delve into the details of adding elements to sets in Python, discussing various approaches and highlighting use cases.
Adding Elements to a Set
Python offers a couple of methods to add elements to a set: `add()` and `update()`. Let’s explore each of these methods in detail.
1. add():
The `add()` method allows you to add a single element to a set. If the element already exists in the set, the set remains unchanged, as sets do not allow duplicate elements.
Let’s take a look at the following example, demonstrating the usage of the `add()` method:
“`
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
“`
In the above code snippet, the element 4 is added to the set `my_set` using the `add()` method. The resulting set will contain {1, 2, 3, 4}.
2. update():
The `update()` method allows you to add multiple elements to a set at once. You can pass any iterable object, such as lists, tuples, or even other sets, to this method. The elements in the iterable are, then, added to the target set.
Consider the following example:
“`
my_set = {1, 2, 3}
my_set.update([4, 5, 6])
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
“`
In the above code snippet, the `update()` method adds the elements [4, 5, 6] to the set `my_set`, resulting in {1, 2, 3, 4, 5, 6}.
Use Cases and Considerations
Adding elements to a set can be particularly useful in numerous scenarios. Some notable use cases include the following:
1. Combining Sets:
You can use the `add()` or `update()` methods to combine multiple sets into one. This operation can be easily achieved by adding the elements from each set into a new set. For instance:
“`
set1 = {1, 2, 3}
set2 = {4, 5, 6}
set3 = set1.copy()
set3.update(set2)
print(set3) # Output: {1, 2, 3, 4, 5, 6}
“`
In the above example, we create a new set `set3` and add the elements from `set1` using the `update()` method. Then, the elements from `set2` are added to `set3`, resulting in {1, 2, 3, 4, 5, 6}.
2. Filtering Duplicate Values:
Since sets do not allow duplicate values, adding elements to a set can help filter out duplicates from other data structures, such as lists. By converting a list into a set, you automatically eliminate any duplicate values. For example:
“`
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_set = set(my_list)
print(unique_set) # Output: {1, 2, 3, 4, 5}
“`
In the above code snippet, the list `my_list` contains duplicate values. By converting it into a set using the `set()` constructor, we automatically remove the duplicates, resulting in {1, 2, 3, 4, 5}.
FAQs:
Q1. Can a set contain duplicate elements in Python?
Answer: No, sets in Python do not allow duplicate elements. If you try to add an element that already exists in the set, it will not be added.
Q2. What happens if I use the `add()` method to add an element that already exists in the set?
Answer: If you use the `add()` method to add an element that already exists in the set, the set will remain unchanged. Sets automatically handle the uniqueness of elements.
Q3. Can I add multiple elements to a set using the `add()` method?
Answer: No, the `add()` method is specifically designed to add a single element to a set. If you want to add multiple elements, you should utilize the `update()` method.
Q4. Is there any difference between the `update()` method and the `add()` method?
Answer: Yes, the primary difference is that the `update()` method allows you to add multiple elements to a set at once, whereas the `add()` method only adds a single element. Additionally, the `update()` method accepts an iterable object, such as a list or a set.
Q5. Can I add elements to a set using the `+` operator like in lists?
Answer: No, sets do not support the `+` operator for concatenation. You should use the `update()` method to add multiple elements to a set.
In conclusion, adding elements to a set in Python is both simple and efficient. Python provides the `add()` and `update()` methods to accomplish this task. Whether you need to add a single element or multiple elements, sets offer an excellent choice due to their unique feature of maintaining only distinct elements. By understanding the concepts explained in this article, you can now confidently manipulate sets in Python, adding elements as needed to enhance your code’s capabilities.
Images related to the topic python create empty set
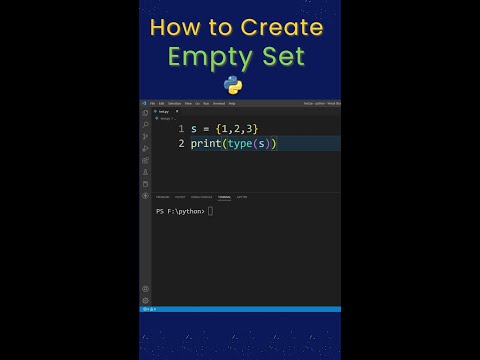
Found 44 images related to python create empty set theme




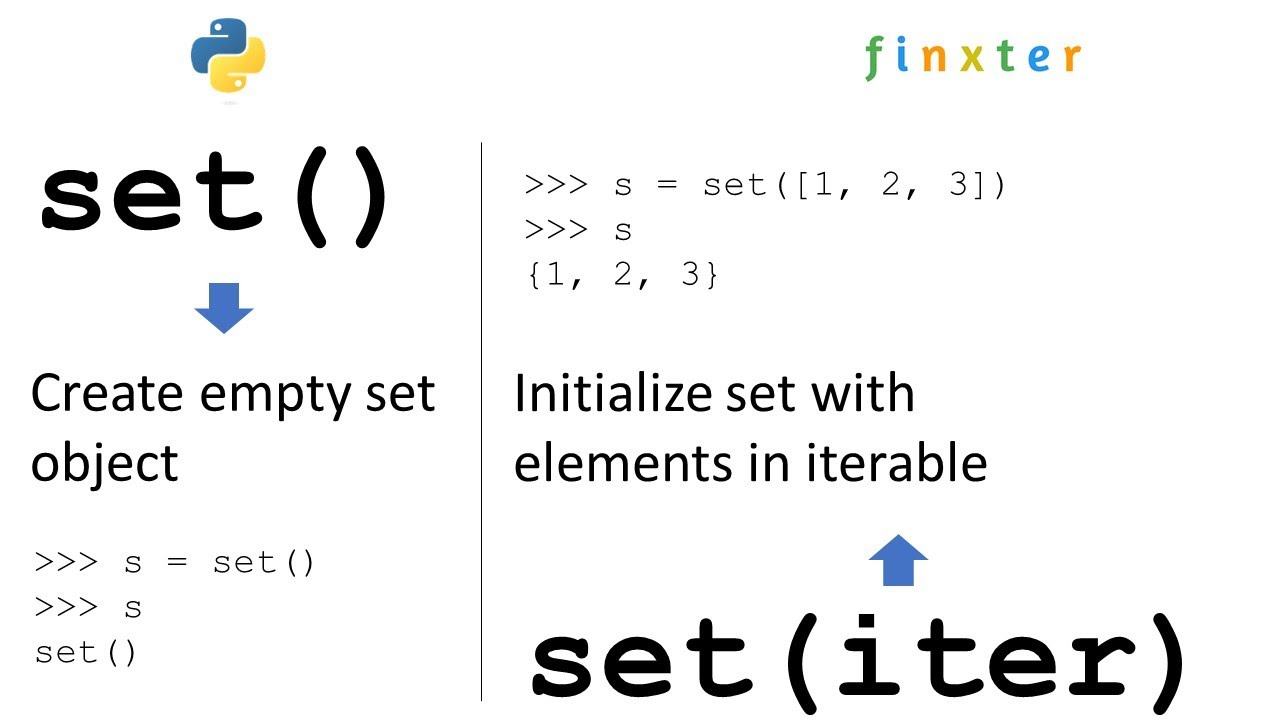
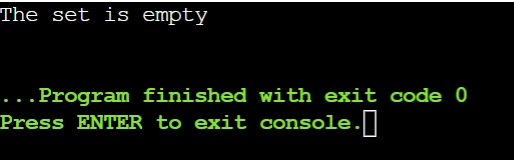
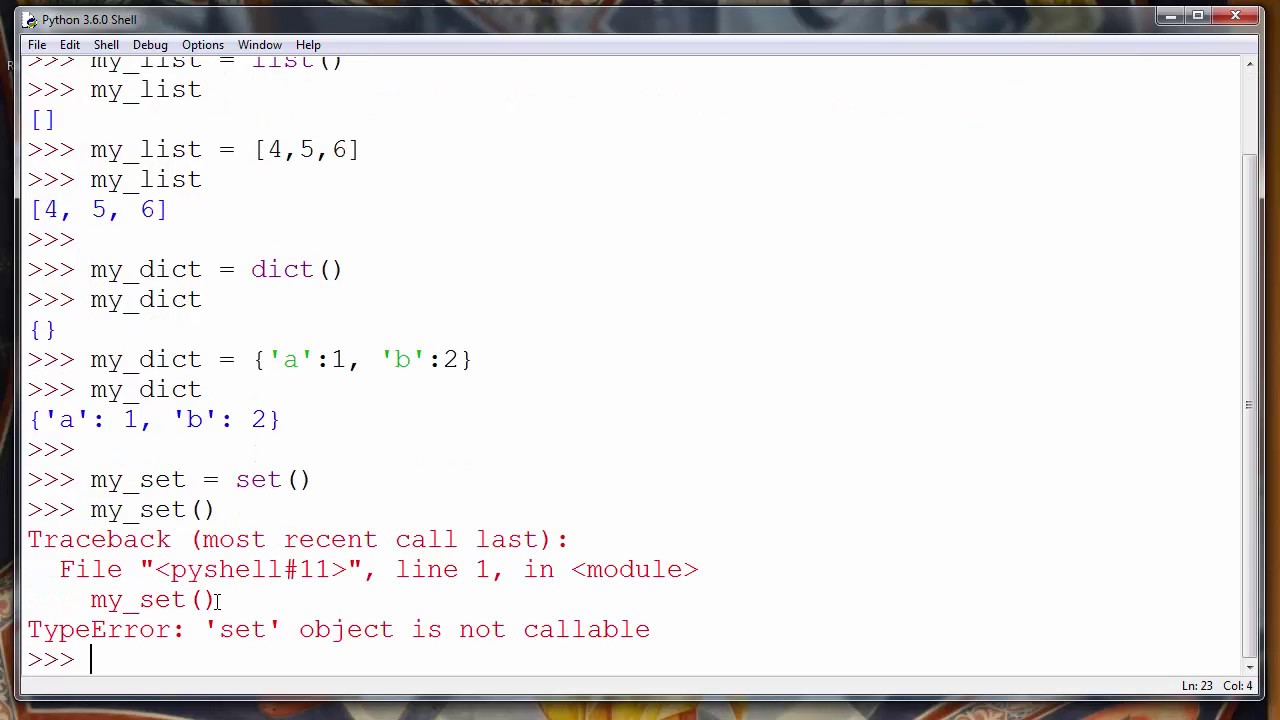

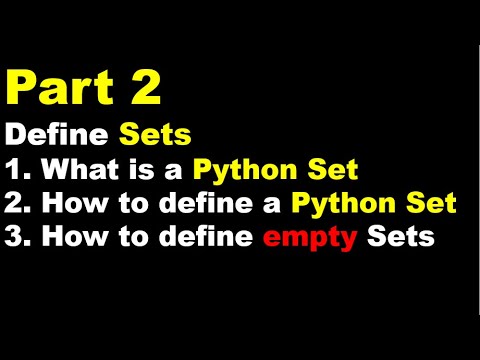
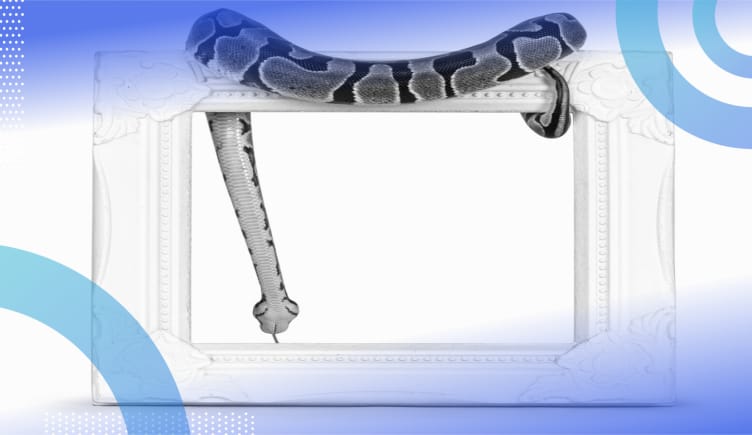

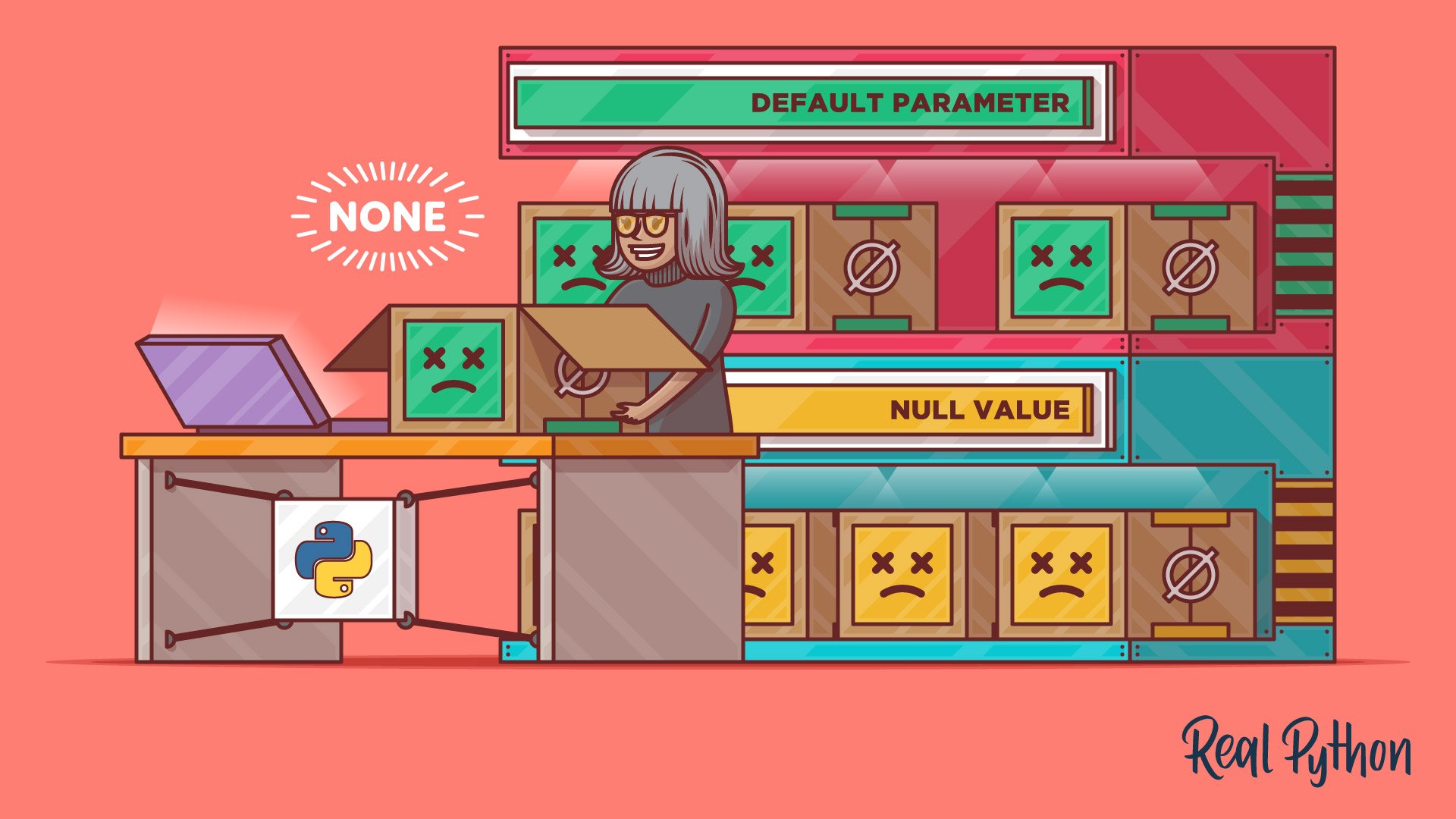
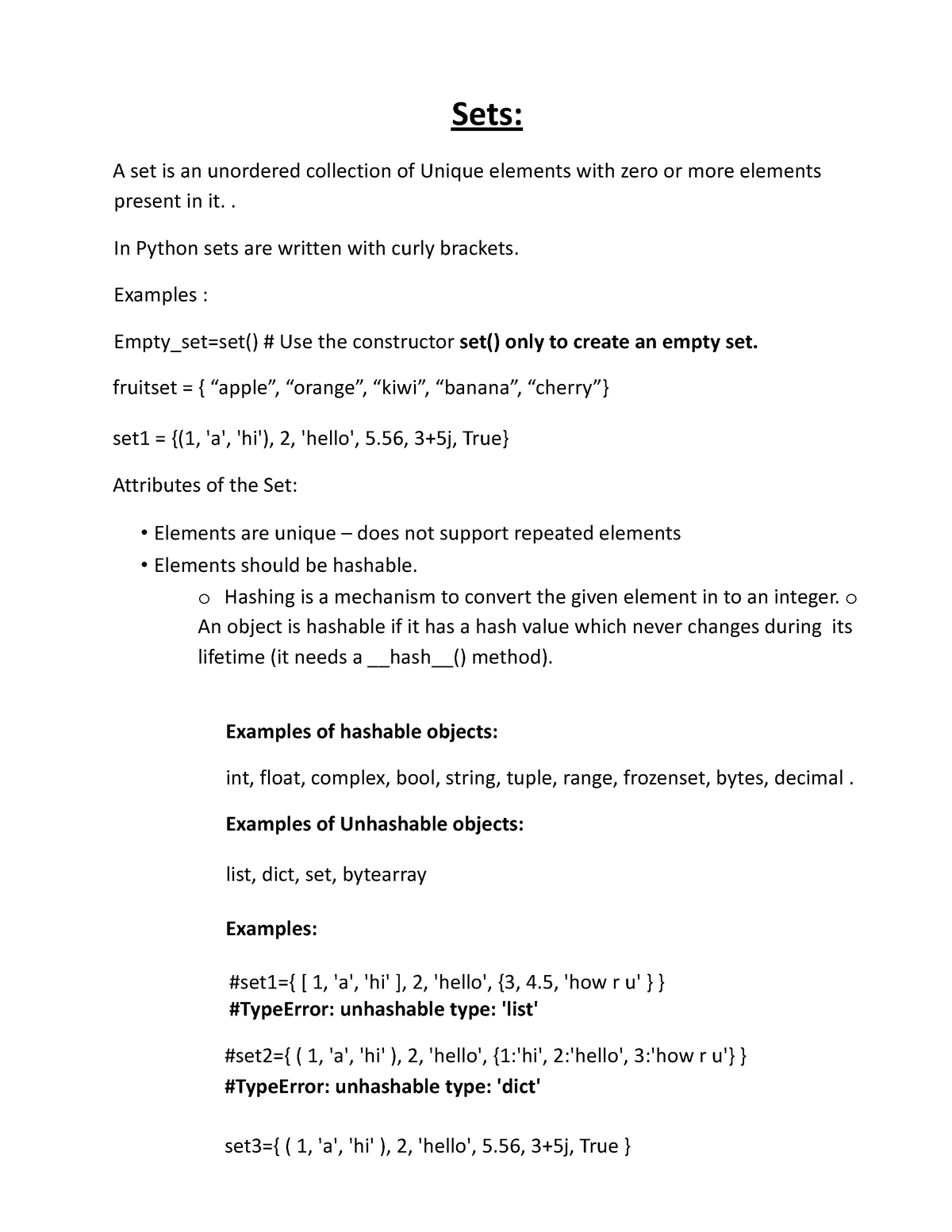
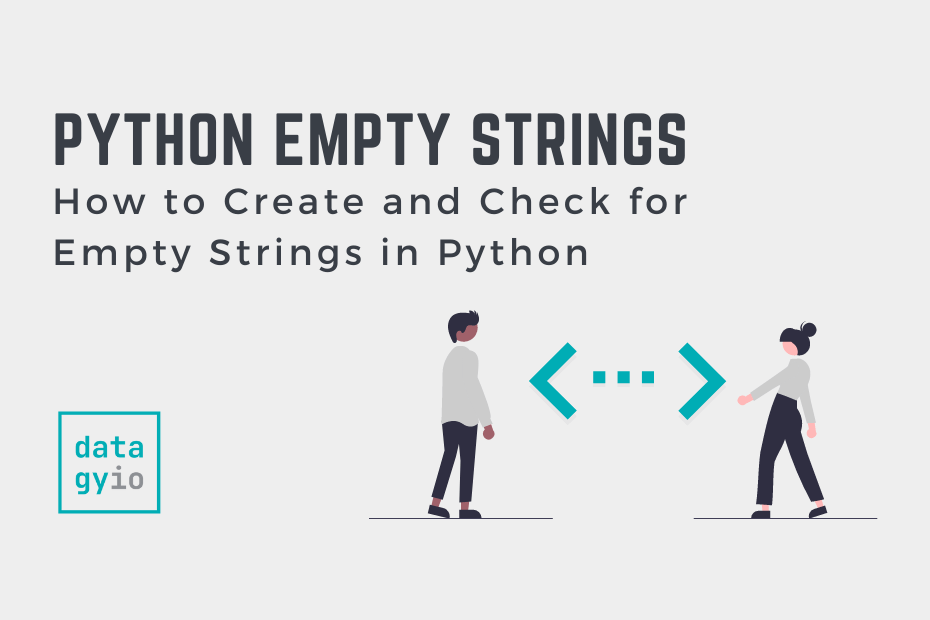
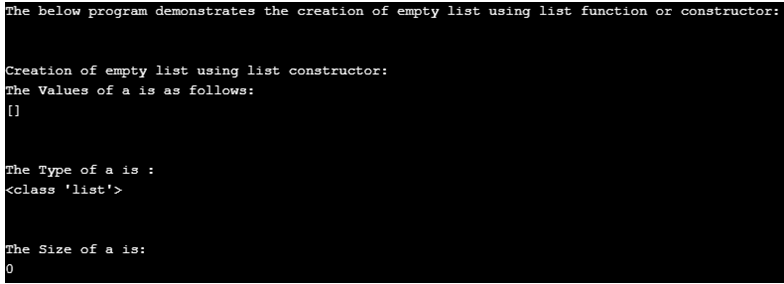


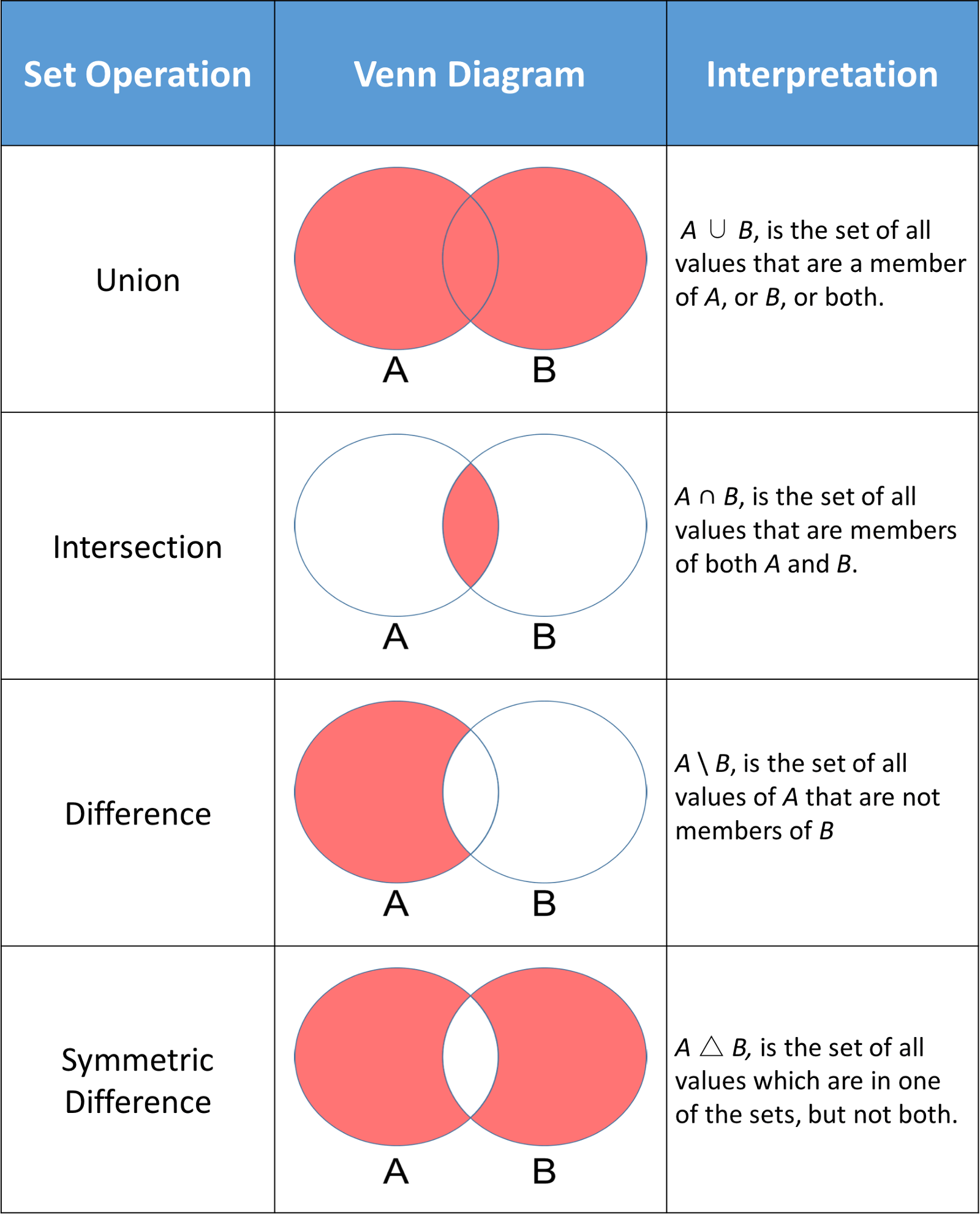
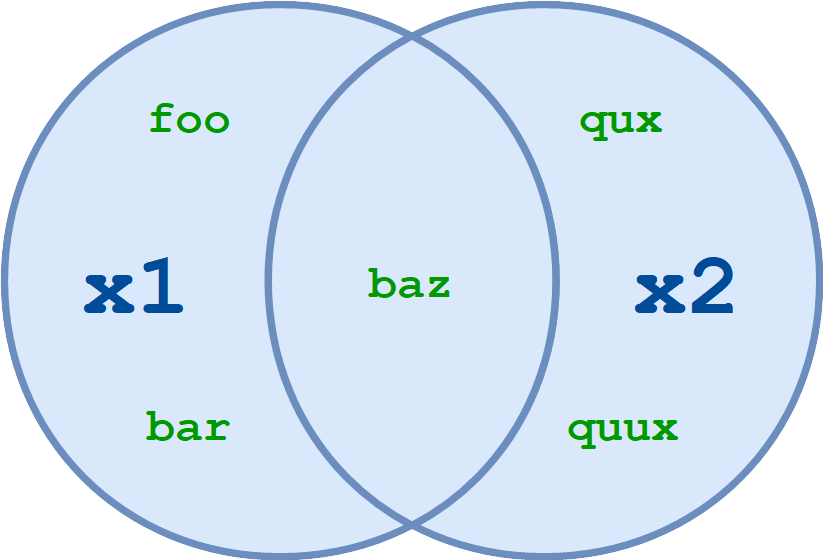
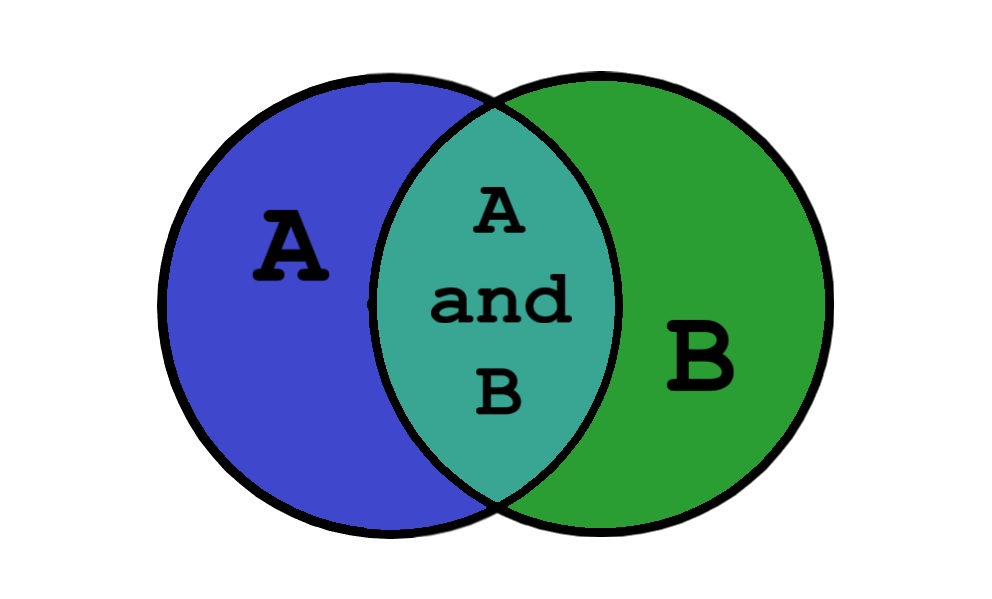
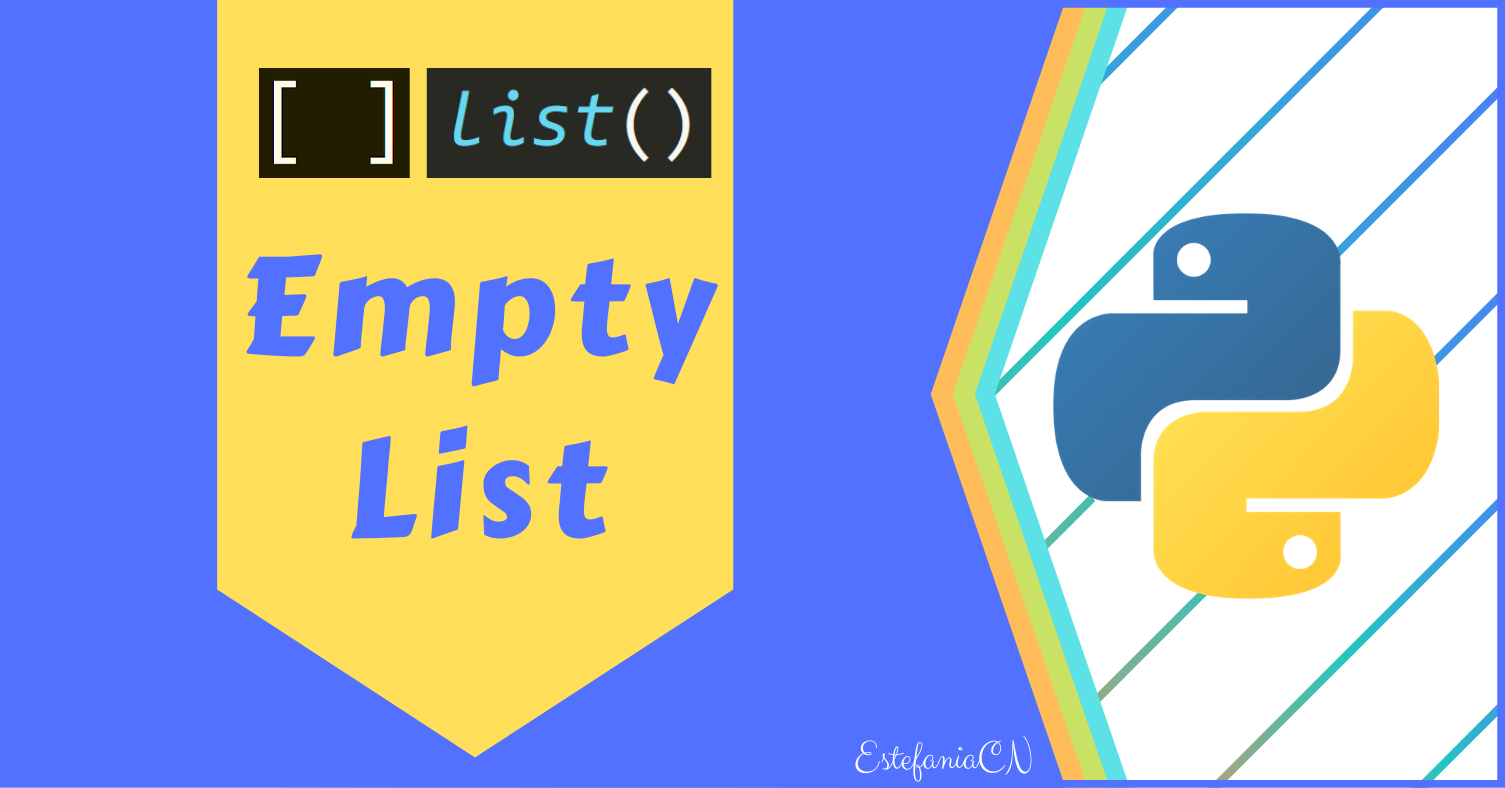
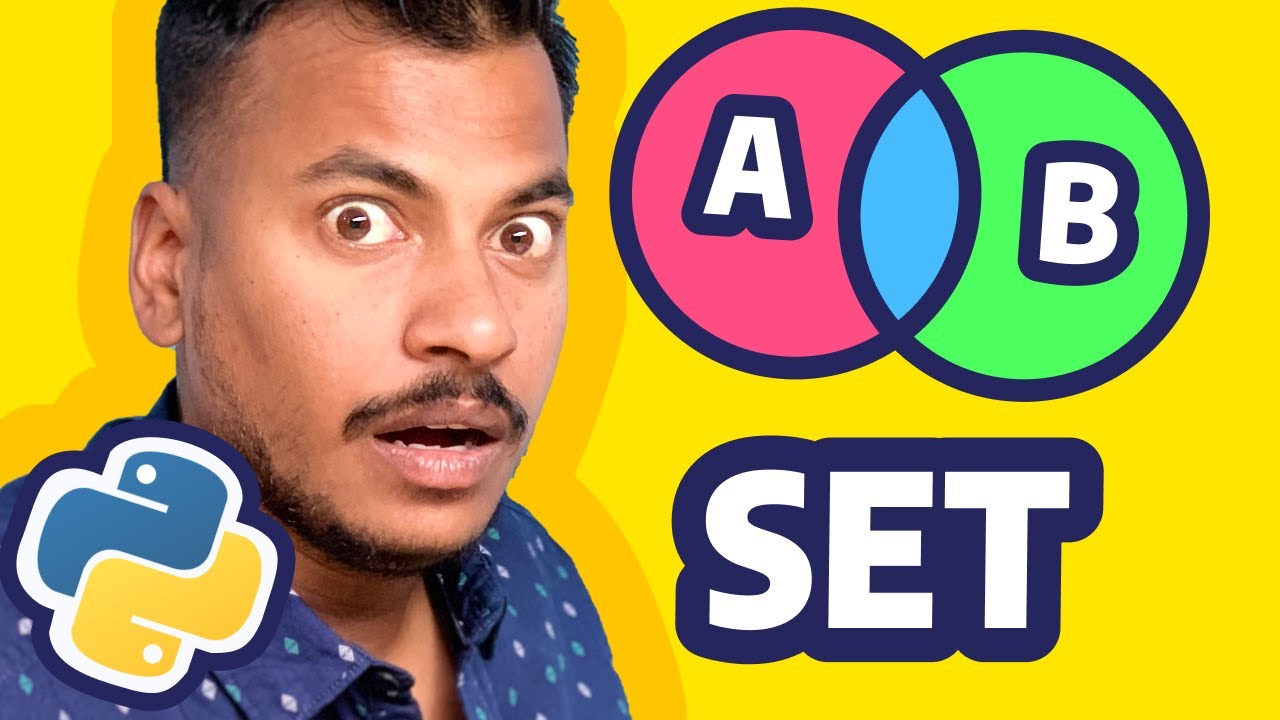

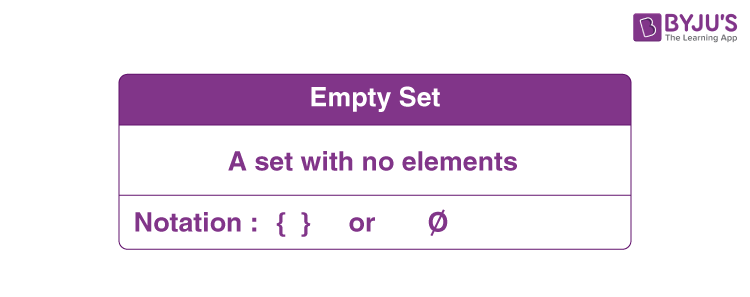
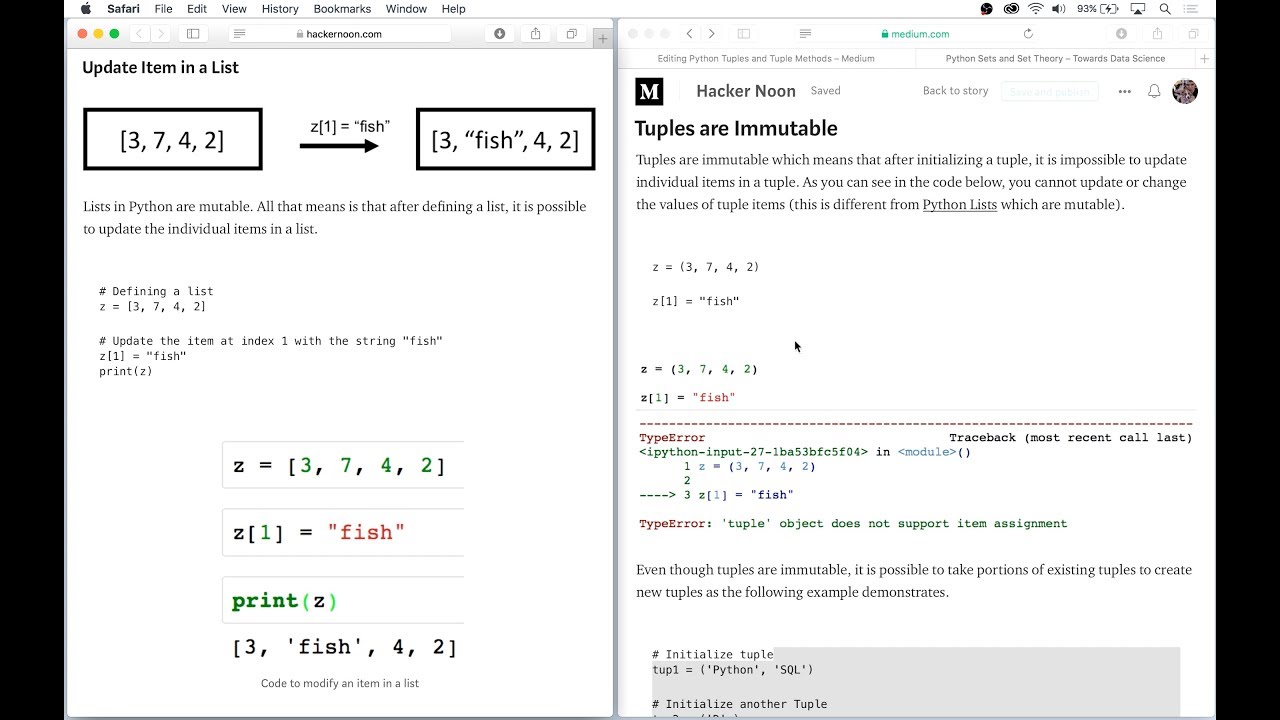
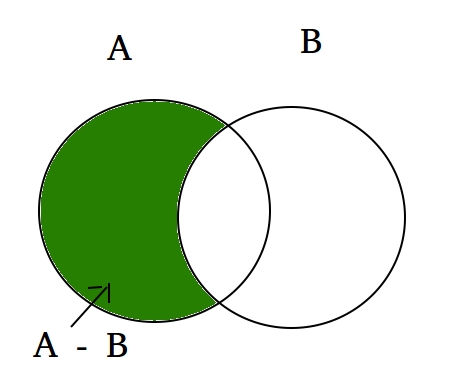
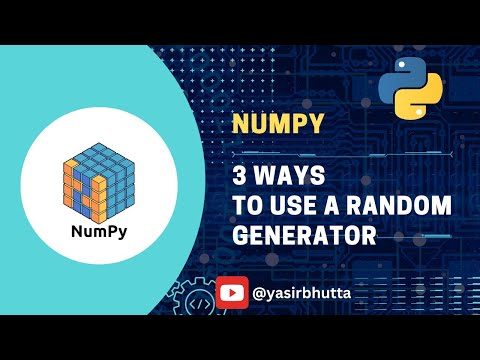
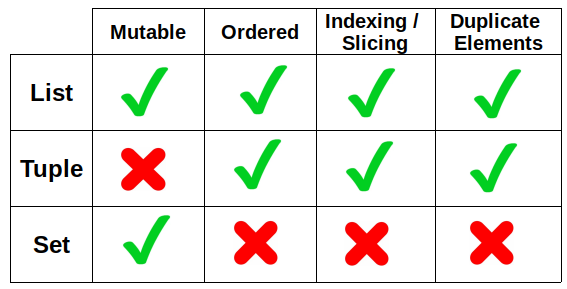
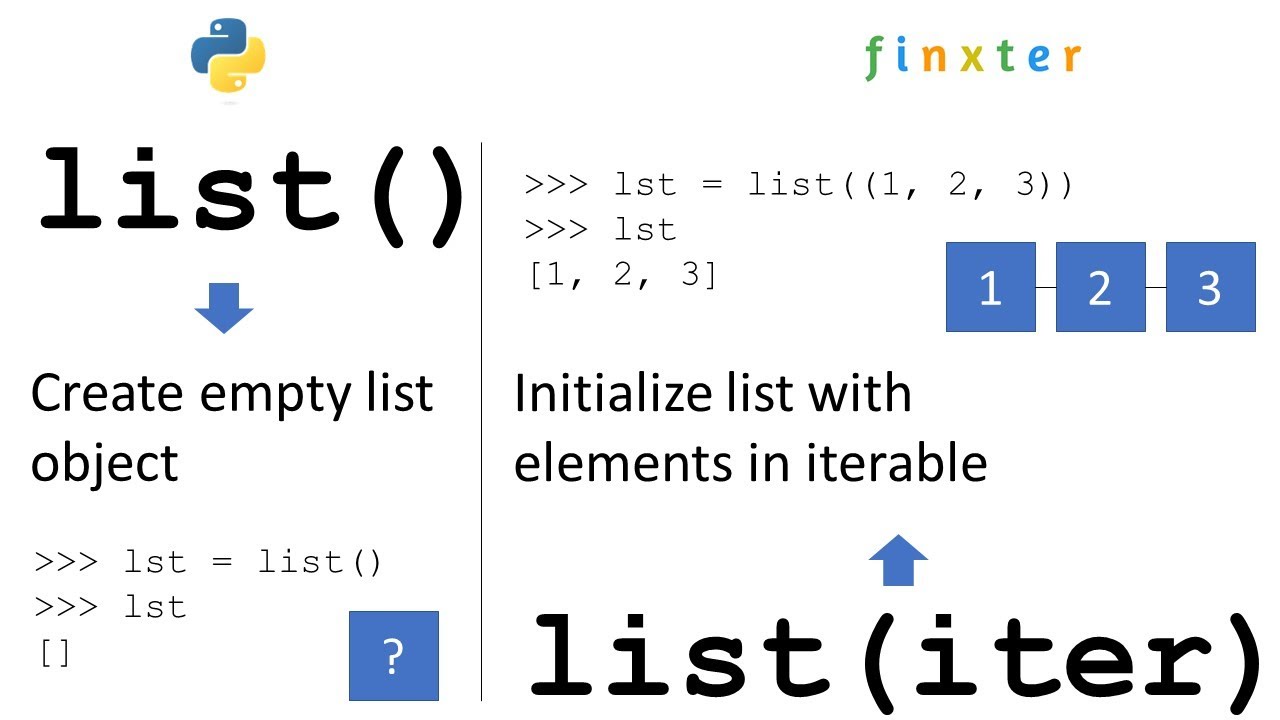

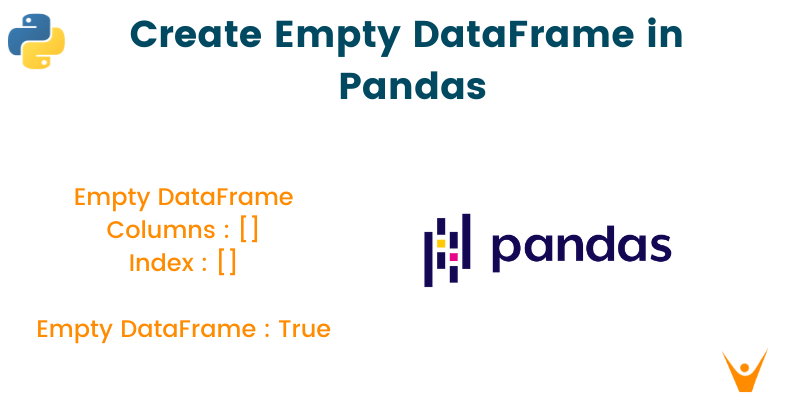
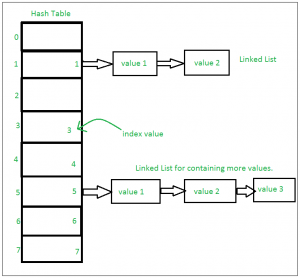
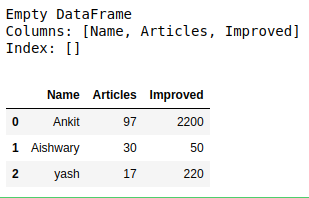


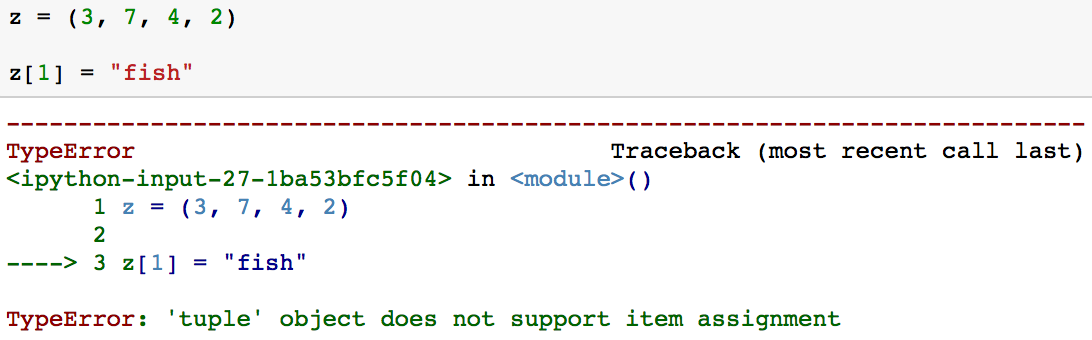

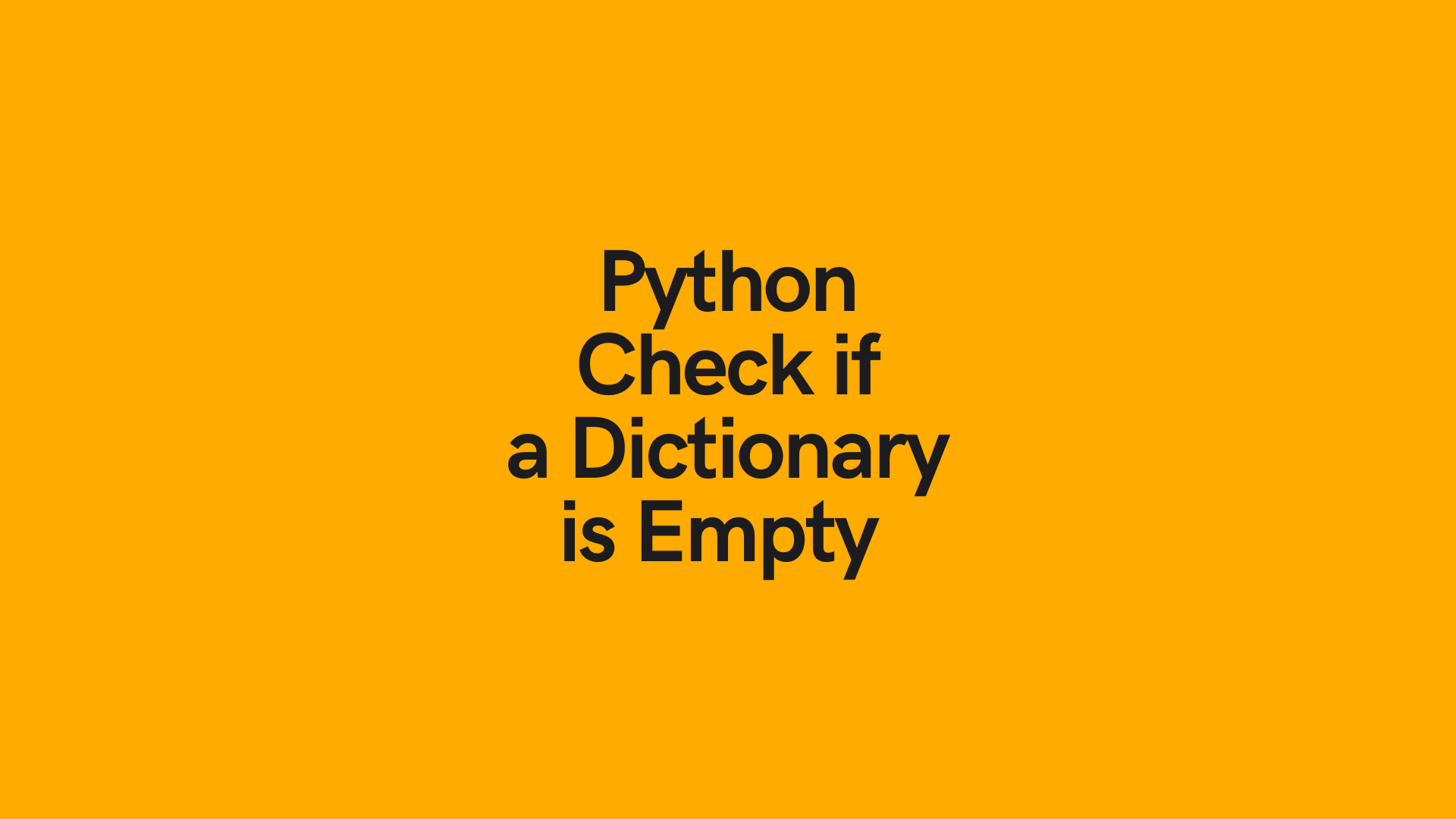


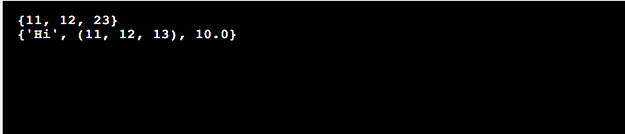
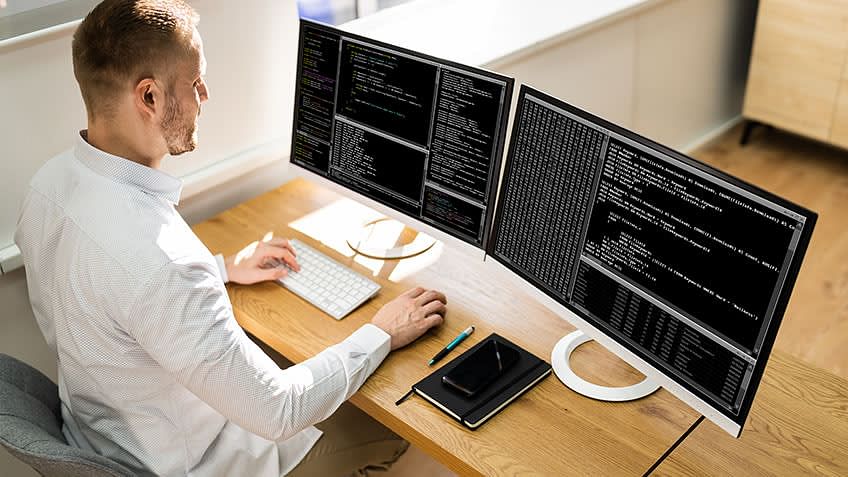
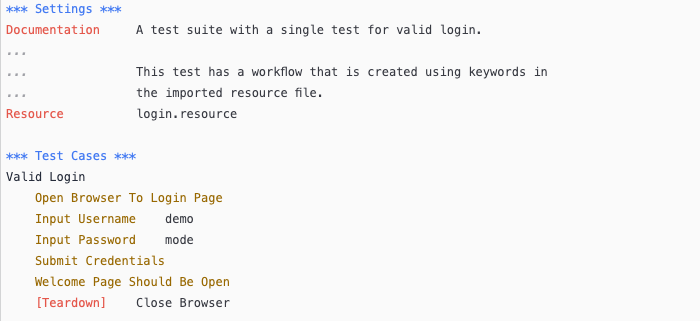


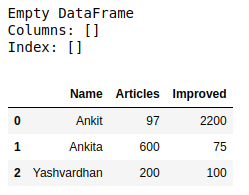
Article link: python create empty set.
Learn more about the topic python create empty set.
- Python: Create a new empty set – w3resource
- How To Create Empty Set In Python
- Python Set (With Examples) – Programiz
- Python Set (With Examples) – Programiz
- Python Set – DigitalOcean
- Question Bank * MCQ Questions
- What is a null set in mathematics? – TechTarget
- Empty Set in Python – Coding Ninjas
- Empty set literal? – python – Stack Overflow
- How to Create an Empty Set in Python – AppDividend
- Create Empty Set in Python
- How do I create an empty set in Python? – Gitnux Blog
- Python create empty set – Java2Blog
See more: nhanvietluanvan.com/luat-hoc